# Export
GET /api/v1/organization/audit-logs
Get all audit logs for an organization
# Attach
POST /api/v1/auth/aws-auth/identities/{identityId}
Attach AWS Auth configuration onto identity
# Login
POST /api/v1/auth/aws-auth/login
Login with AWS Auth
# Retrieve
GET /api/v1/auth/aws-auth/identities/{identityId}
Retrieve AWS Auth configuration on identity
# Revoke
DELETE /api/v1/auth/aws-auth/identities/{identityId}
Delete AWS Auth configuration on identity
# Update
PATCH /api/v1/auth/aws-auth/identities/{identityId}
Update AWS Auth configuration on identity
# Attach
POST /api/v1/auth/azure-auth/identities/{identityId}
Attach Azure Auth configuration onto identity
# Login
POST /api/v1/auth/azure-auth/login
Login with Azure Auth
# Retrieve
GET /api/v1/auth/azure-auth/identities/{identityId}
Retrieve Azure Auth configuration on identity
# Revoke
DELETE /api/v1/auth/azure-auth/identities/{identityId}
Delete Azure Auth configuration on identity
# Update
PATCH /api/v1/auth/azure-auth/identities/{identityId}
Update Azure Auth configuration on identity
# Retrieve certificate / chain
GET /api/v1/pki/ca/{caId}/certificate
Get current CA cert and cert chain of a CA
# Create
POST /api/v1/pki/ca
Create CA
# List CRLs
GET /api/v1/pki/ca/{caId}/crls
Get list of CRLs of the CA
# Get CSR
GET /api/v1/pki/ca/{caId}/csr
Get CA CSR
# Delete
DELETE /api/v1/pki/ca/{caId}
Delete CA
# Import certificate
POST /api/v1/pki/ca/{caId}/import-certificate
Import certificate and chain to CA
# Issue certificate
POST /api/v1/pki/ca/{caId}/issue-certificate
Issue certificate from CA
# List
GET /api/v2/workspace/{slug}/cas
# List CA certificates
GET /api/v1/pki/ca/{caId}/ca-certificates
Get list of past and current CA certificates for a CA
# Retrieve
GET /api/v1/pki/ca/{caId}
Get CA
# Renew
POST /api/v1/pki/ca/{caId}/renew
Perform CA certificate renewal
# Sign certificate
POST /api/v1/pki/ca/{caId}/sign-certificate
Sign certificate from CA
# Sign intermediate certificate
POST /api/v1/pki/ca/{caId}/sign-intermediate
Create intermediate CA certificate from parent CA
# Update
PATCH /api/v1/pki/ca/{caId}
Update CA
# Create
POST /api/v1/pki/certificate-templates
# Delete
DELETE /api/v1/pki/certificate-templates/{certificateTemplateId}
# Get by ID
GET /api/v1/pki/certificate-templates/{certificateTemplateId}
# Update
PATCH /api/v1/pki/certificate-templates/{certificateTemplateId}
# Get Certificate Body / Chain
GET /api/v1/pki/certificates/{serialNumber}/certificate
Get certificate body of certificate
# Delete
DELETE /api/v1/pki/certificates/{serialNumber}
Delete certificate
# Issue Certificate
POST /api/v1/pki/certificates/issue-certificate
Issue certificate
# List
GET /api/v2/workspace/{slug}/certificates
# Retrieve
GET /api/v1/pki/certificates/{serialNumber}
Get certificate
# Revoke
POST /api/v1/pki/certificates/{serialNumber}/revoke
Revoke
# Sign Certificate
POST /api/v1/pki/certificates/sign-certificate
Sign certificate
# Create
POST /api/v1/dynamic-secrets
# Create Lease
POST /api/v1/dynamic-secrets/leases
# Delete
DELETE /api/v1/dynamic-secrets/{name}
# Delete Lease
DELETE /api/v1/dynamic-secrets/leases/{leaseId}
# Get
GET /api/v1/dynamic-secrets/{name}
# Get Lease
GET /api/v1/dynamic-secrets/leases/{leaseId}
# List
GET /api/v1/dynamic-secrets
# List Leases
GET /api/v1/dynamic-secrets/{name}/leases
# Renew Lease
POST /api/v1/dynamic-secrets/leases/{leaseId}/renew
# Update
PATCH /api/v1/dynamic-secrets/{name}
# Create
POST /api/v1/workspace/{workspaceId}/environments
Create environment
# Delete
DELETE /api/v1/workspace/{workspaceId}/environments/{id}
Delete environment
# Update
PATCH /api/v1/workspace/{workspaceId}/environments/{id}
Update environment
# Create
POST /api/v1/folders
Create folders
# Delete
DELETE /api/v1/folders/{folderIdOrName}
Delete a folder
# Get by ID
GET /api/v1/folders/{id}
Get folder by id
# List
GET /api/v1/folders
Get folders
# Update
PATCH /api/v1/folders/{folderId}
Update folder
# Attach
POST /api/v1/auth/gcp-auth/identities/{identityId}
Attach GCP Auth configuration onto identity
# Login
POST /api/v1/auth/gcp-auth/login
Login with GCP Auth
# Retrieve
GET /api/v1/auth/gcp-auth/identities/{identityId}
Retrieve GCP Auth configuration on identity
# Revoke
DELETE /api/v1/auth/gcp-auth/identities/{identityId}
Delete GCP Auth configuration on identity
# Update
PATCH /api/v1/auth/gcp-auth/identities/{identityId}
Update GCP Auth configuration on identity
# Add Group User
POST /api/v1/groups/{id}/users/{username}
# Create
POST /api/v1/groups
# Delete
DELETE /api/v1/groups/{id}
# Get Groups in Organization
GET /api/v1/groups
# Get By ID
GET /api/v1/groups/{id}
# List Group Users
GET /api/v1/groups/{id}/users
# Remove Group User
DELETE /api/v1/groups/{id}/users/{username}
# Update
PATCH /api/v1/groups/{id}
# Create
POST /api/v1/identities
Create identity
# Delete
DELETE /api/v1/identities/{identityId}
Delete identity
# Get By ID
GET /api/v1/identities/{identityId}
Get an identity by id
# List
GET /api/v1/identities
List identities
# Update
PATCH /api/v1/identities/{identityId}
Update identity
# Create Permanent
POST /api/v1/additional-privilege/identity/permanent
Create a permanent or a non expiry specific privilege for identity.
# Create Temporary
POST /api/v1/additional-privilege/identity/temporary
Create a temporary or a expiring specific privilege for identity.
# Delete
DELETE /api/v1/additional-privilege/identity
Delete a specific privilege of an identity.
# Find By Privilege Slug
GET /api/v1/additional-privilege/identity/{privilegeSlug}
Retrieve details of a specific privilege by privilege slug.
# List
GET /api/v1/additional-privilege/identity
List of a specific privilege of an identity in a project.
# Update
PATCH /api/v1/additional-privilege/identity
Update a specific privilege of an identity.
# Create
POST /api/v1/integration
Create an integration to sync secrets.
## Integration Parameters
The integration creation endpoint is generic and can be used for all native integrations.
For specific integration parameters for a given service, please review the respective documentation below.
The ID of the integration auth object for authentication with AWS.
Refer [Create Integration Auth](./create-auth) for more info
Whether the integration should be active or inactive
The secret name used when saving secret in AWS SSM. Used for naming and can be arbitrary.
The AWS region of the SSM. Example: `us-east-1`
The Infisical environment slug from where secrets will be synced from. Example: `dev`
The Infisical folder path from where secrets will be synced from. Example: `/some/path`. The root of the environment is `/`.
Coming Soon
Coming Soon
# Create Auth
POST /api/v1/integration-auth/access-token
Create the integration authentication object required for syncing secrets.
## Integration Authentication Parameters
The integration authentication endpoint is generic and can be used for all native integrations.
For specific integration parameters for a given service, please review the respective documentation below.
This value must be **aws-secret-manager**.
Infisical project id for the integration.
The AWS IAM User Access ID.
The AWS IAM User Access Secret Key.
Coming Soon
Coming Soon
# Delete
DELETE /api/v1/integration/{integrationId}
Remove an integration using the integration object ID
# Delete Auth
DELETE /api/v1/integration-auth
Remove all integration's auth object from the project.
# Delete Auth By ID
DELETE /api/v1/integration-auth/{integrationAuthId}
Remove an integration auth object by object id.
# Get Auth By ID
GET /api/v1/integration-auth/{integrationAuthId}
Get details of an integration authorization by auth object id.
# List Auth
GET /api/v1/workspace/{workspaceId}/authorizations
List integration auth objects for a workspace.
# List Project Integrations
GET /api/v1/workspace/{workspaceId}/integrations
List integrations for a project.
# Update
PATCH /api/v1/integration/{integrationId}
Update an integration by integration id
# Create Key
POST /api/v1/kms/keys
Create KMS key
# Decrypt Data
POST /api/v1/kms/keys/{keyId}/decrypt
Decrypt data with KMS key
# Delete Key
DELETE /api/v1/kms/keys/{keyId}
Delete KMS key
# Encrypt Data
POST /api/v1/kms/keys/{keyId}/encrypt
Encrypt data with KMS key
# List Keys
Get /api/v1/kms/keys
List KMS keys
# Update Key
PATCH /api/v1/kms/keys/{keyId}
Update KMS key
# Attach
POST /api/v1/auth/kubernetes-auth/identities/{identityId}
Attach Kubernetes Auth configuration onto identity
# Login
POST /api/v1/auth/kubernetes-auth/login
Login with Kubernetes Auth
# Retrieve
GET /api/v1/auth/kubernetes-auth/identities/{identityId}
Retrieve Kubernetes Auth configuration on identity
# Revoke
DELETE /api/v1/auth/kubernetes-auth/identities/{identityId}
Delete Kubernetes Auth configuration on identity
# Update
PATCH /api/v1/auth/kubernetes-auth/identities/{identityId}
Update Kubernetes Auth configuration on identity
# Attach
POST /api/v1/auth/oidc-auth/identities/{identityId}
Attach OIDC Auth configuration onto identity
# Login
POST /api/v1/auth/oidc-auth/login
Login with OIDC Auth
# Retrieve
GET /api/v1/auth/oidc-auth/identities/{identityId}
Retrieve OIDC Auth configuration on identity
# Revoke
DELETE /api/v1/auth/oidc-auth/identities/{identityId}
Delete OIDC Auth configuration on identity
# Update
PATCH /api/v1/auth/oidc-auth/identities/{identityId}
Update OIDC Auth configuration on identity
# Delete User Membership
DELETE /api/v2/organizations/{organizationId}/memberships/{membershipId}
Delete organization user memberships
# List Identity Memberships
GET /api/v2/organizations/{orgId}/identity-memberships
Return organization identity memberships
# Get User Memberships
GET /api/v2/organizations/{organizationId}/memberships
Return organization user memberships
# Update User Membership
PATCH /api/v2/organizations/{organizationId}/memberships/{membershipId}
Update organization user memberships
# Get Projects
GET /api/v2/organizations/{organizationId}/workspaces
Return projects in organization that user is apart of
# Create
POST /api/v1/pki/alerts
Create PKI alert
# Delete
DELETE /api/v1/pki/alerts/{alertId}
Delete PKI alert
# Retrieve
GET /api/v1/pki/alerts/{alertId}
Get PKI alert
# Update
PATCH /api/v1/pki/alerts/{alertId}
Update PKI alert
# Add Collection Item
POST /api/v1/pki/collections/{collectionId}/items
Add item to PKI collection
# Create
POST /api/v1/pki/collections
Create PKI collection
# Delete
DELETE /api/v1/pki/collections/{collectionId}
Delete PKI collection
# Delete Collection Item
DELETE /api/v1/pki/collections/{collectionId}/items/{collectionItemId}
Remove item from PKI collection
# Retrieve
GET /api/v1/pki/collections/{collectionId}/items
Get items in PKI collection
# Retrieve
GET /api/v1/pki/collections/{collectionId}
Get PKI collection
# Update
PATCH /api/v1/pki/collections/{collectionId}
Update PKI collection
# Create Project Membership
POST /api/v2/workspace/{projectId}/groups/{groupId}
Add group to project
# Delete Project Membership
DELETE /api/v2/workspace/{projectId}/groups/{groupId}
Remove group from project
# Get Project Membership
GET /api/v2/workspace/{projectId}/groups/{groupId}
Return project group
# List Project Memberships
GET /api/v2/workspace/{projectId}/groups
Return list of groups in project
# Update Project Membership
PATCH /api/v2/workspace/{projectId}/groups/{groupId}
Update group in project
# Create Identity Membership
POST /api/v2/workspace/{projectId}/identity-memberships/{identityId}
Create project identity membership
# Delete Identity Membership
DELETE /api/v2/workspace/{projectId}/identity-memberships/{identityId}
Delete project identity memberships
# Get Identity by ID
GET /api/v2/workspace/{projectId}/identity-memberships/{identityId}
Return project identity membership
# List Identity Memberships
GET /api/v2/workspace/{projectId}/identity-memberships
Return project identity memberships
# Update Identity Membership
PATCH /api/v2/workspace/{projectId}/identity-memberships/{identityId}
Update project identity memberships
# Create
POST /api/v1/workspace/{projectSlug}/roles
Create a project role
You can read more about the permissions field in the [permissions documentation](/internals/permissions).
# Delete
DELETE /api/v1/workspace/{projectSlug}/roles/{roleId}
Delete a project role
# Get By Slug
GET /api/v1/workspace/{projectSlug}/roles/slug/{slug}
# List
GET /api/v1/workspace/{projectSlug}/roles
List project role
# Update
PATCH /api/v1/workspace/{projectSlug}/roles/{roleId}
Update a project role
# Create
POST /api/v1/project-templates
Create a project template.
You can read more about the role's permissions field in the [permissions documentation](/internals/permissions).
# Delete
DELETE /api/v1/project-templates/{templateId}
Delete a project template.
# Get By ID
GET /api/v1/project-templates/{templateId}
Get a project template by ID.
# List
GET /api/v1/project-templates
List project templates for the current organization.
# Update
PATCH /api/v1/project-templates/{templateId}
Update a project template.
You can read more about the role's permissions field in the [permissions documentation](/internals/permissions).
# Get By Username
POST /api/v1/workspace/{workspaceId}/memberships/details
Return project user memberships
# Invite Member
POST /api/v2/workspace/{projectId}/memberships
Invite members to project
# Get User Memberships
GET /api/v1/workspace/{workspaceId}/memberships
Return project user memberships
# Remove Member
DELETE /api/v2/workspace/{projectId}/memberships
Remove members from project
# Update User Membership
PATCH /api/v1/workspace/{workspaceId}/memberships/{membershipId}
Update project user membership
# Create
POST /api/v1/secret-imports
Create secret imports
# Delete
DELETE /api/v1/secret-imports/{secretImportId}
Delete secret imports
# List
GET /api/v1/secret-imports
Get secret imports
# Update
PATCH /api/v1/secret-imports/{secretImportId}
Update secret imports
# Create
POST /api/v1/workspace/{projectId}/tags
# Delete
DELETE /api/v1/workspace/{projectId}/tags/{tagId}
# Get By ID
GET /api/v1/workspace/{projectId}/tags/{tagId}
# Get By Slug
GET /api/v1/workspace/{projectId}/tags/slug/{tagSlug}
# List
GET /api/v1/workspace/{projectId}/tags
# Update
PATCH /api/v1/workspace/{projectId}/tags/{tagId}
# Attach tags
POST /api/v3/secrets/tags/{secretName}
Attach tags to a secret
# Create
POST /api/v3/secrets/raw/{secretName}
Create secret
# Bulk Create
POST /api/v3/secrets/batch/raw
Create many secrets
# Delete
DELETE /api/v3/secrets/raw/{secretName}
Delete secret
# Bulk Delete
DELETE /api/v3/secrets/batch/raw
Delete many secrets
# Detach tags
DELETE /api/v3/secrets/tags/{secretName}
Detach tags from a secret
# List
GET /api/v3/secrets/raw
List secrets
# Retrieve
GET /api/v3/secrets/raw/{secretName}
Get a secret by name
# Update
PATCH /api/v3/secrets/raw/{secretName}
Update secret
# Bulk Update
PATCH /api/v3/secrets/batch/raw
Update many secrets
# Get
GET /api/v2/service-token
Return Infisical Token data
This endpoint is deprecated and will be removed in the future.
We recommend switching to using [Machine Identities](/documentation/platform/identities/machine-identities).
# Attach
POST /api/v1/auth/token-auth/identities/{identityId}
Attach Token Auth configuration onto identity
# Create Token
POST /api/v1/auth/token-auth/identities/{identityId}/tokens
Create token for identity with Token Auth
# Get Tokens
GET /api/v1/auth/token-auth/identities/{identityId}/tokens
Get tokens for identity with Token Auth
# Retrieve
GET /api/v1/auth/token-auth/identities/{identityId}
Retrieve Token Auth configuration on identity
# Revoke
DELETE /api/v1/auth/token-auth/identities/{identityId}
Delete Token Auth configuration on identity
# Revoke Token
POST /api/v1/auth/token-auth/tokens/{tokenId}/revoke
Revoke token for identity with Token Auth
# Update
PATCH /api/v1/auth/token-auth/identities/{identityId}
Update Token Auth configuration on identity
# Update Token
PATCH /api/v1/auth/token-auth/tokens/{tokenId}
Update token for identity with Token Auth
# Attach
POST /api/v1/auth/universal-auth/identities/{identityId}
Attach Universal Auth configuration onto identity
# Create Client Secret
POST /api/v1/auth/universal-auth/identities/{identityId}/client-secrets
Create Universal Auth Client Secret for identity
# Get Client Secret By ID
GET /api/v1/auth/universal-auth/identities/{identityId}/client-secrets/{clientSecretId}
Get Universal Auth Client Secret for identity
# List Client Secrets
GET /api/v1/auth/universal-auth/identities/{identityId}/client-secrets
List Universal Auth Client Secrets for identity
# Login
POST /api/v1/auth/universal-auth/login
Login with Universal Auth
# Renew Access Token
POST /api/v1/auth/token/renew
Renew access token
# Retrieve
GET /api/v1/auth/universal-auth/identities/{identityId}
Retrieve Universal Auth configuration on identity
# Revoke
DELETE /api/v1/auth/universal-auth/identities/{identityId}
Delete Universal Auth configuration on identity
# Revoke Access Token
POST /api/v1/auth/token/revoke
Revoke access token
# Revoke Client Secret
POST /api/v1/auth/universal-auth/identities/{identityId}/client-secrets/{clientSecretId}/revoke
Revoke Universal Auth Client Secrets for identity
# Update
PATCH /api/v1/auth/universal-auth/identities/{identityId}
Update Universal Auth configuration on identity
# Create Project
POST /api/v2/workspace
Create a new project
# Delete Project
DELETE /api/v1/workspace/{workspaceId}
Delete project
This operation is irreversible. All data associated with the project will be deleted. Please use with caution.
# Get Project
GET /api/v1/workspace/{workspaceId}
Get project
# Roll Back to Snapshot
POST /api/v1/secret-snapshot/{secretSnapshotId}/rollback
Roll back project secrets to those captured in a secret snapshot version.
# Get Snapshots
GET /api/v1/workspace/{workspaceId}/secret-snapshots
Return project secret snapshots ids
# Update Project
PATCH /api/v1/workspace/{workspaceId}
Update project
# Authentication
Learn how to authenticate with the Infisical Public API.
You can authenticate with the Infisical API using [Identities](/documentation/platform/identities/machine-identities) paired with authentication modes such as [Universal Auth](/documentation/platform/identities/universal-auth).
To interact with the Infisical API, you will need to obtain an access token. Follow the step by [step guide](/documentation/platform/identities/universal-auth) to get an access token via Universal Auth.
**FAQ**
The Service Token and API Key authentication modes are being deprecated out in favor of [Identities](/documentation/platform/identity).
We expect to make a deprecation notice in the coming months alongside a larger deprecation initiative planned for Q1/Q2 2024.
With identities, we're improving significantly over the shortcomings of Service Tokens and API Keys. Amongst many differences, identities provide broader access over the Infisical API, utilizes the same role-based
permission system used by users, and comes with ample more configurable security measures.
There are a few reasons for why this might happen:
* You have insufficient organization permissions to create, read, update, delete identities.
* The identity you are trying to read, update, or delete is more privileged than yourself.
* The role you are trying to create an identity for or update an identity to is more privileged than yours.
There are a few reasons for why this might happen:
* The client secret or access token has expired.
* The identity is insufficently permissioned to interact with the resources you wish to access.
* You are attempting to access a `/raw` secrets endpoint that requires your project to disable E2EE.
* The client secret/access token is being used from an untrusted IP.
# Configure native integrations via API
How to use Infisical API to sync secrets to external secret managers
The Infisical API allows you to create programmatic integrations that connect with third-party secret managers to synchronize secrets from Infisical.
This guide will primarily demonstrate the process using AWS Secret Store Manager (AWS SSM), but the steps are generally applicable to other secret management integrations.
For details on setting up AWS SSM synchronization and understanding its prerequisites, refer to the [AWS SSM integration setup documentation](../../../integrations/cloud/aws-secret-manager).
Authentication is required for all integrations. Use the [Integration Auth API](../../endpoints/integrations/create-auth) with the following parameters to authenticate.
Set this parameter to **aws-secret-manager**.
The Infisical project ID for the integration.
The AWS IAM User Access ID.
The AWS IAM User Access Secret Key.
```bash Request
curl --request POST \
--url https://app.infisical.com/api/v1/integration-auth/access-token \
--header 'Authorization: ' \
--header 'Content-Type: application/json' \
--data '{
"workspaceId": "",
"integration": "aws-secret-manager",
"accessId": "",
"accessToken": ""
}'
```
Once authentication between AWS SSM and Infisical is established, you can configure the synchronization behavior.
This involves specifying the source (environment and secret path in Infisical) and the destination in SSM to which the secrets will be synchronized.
Use the [integration API](../../endpoints/integrations/create) with the following parameters to configure the sync source and destination.
The ID of the integration authentication object used with AWS, obtained from the previous API response.
Indicates whether the integration should be active or inactive.
The secret name for saving in AWS SSM, which can be arbitrarily chosen.
The AWS region where the SSM is located, e.g., `us-east-1`.
The Infisical environment slug from which secrets will be synchronized, e.g., `dev`.
The Infisical folder path from which secrets will be synchronized, e.g., `/some/path`. The root path is `/`.
```bash Request
curl --request POST \
--url https://app.infisical.com/api/v1/integration \
--header 'Authorization: ' \
--header 'Content-Type: application/json' \
--data '{
"integrationAuthId": "",
"sourceEnvironment": "",
"secretPath": "",
"app": "",
"region": ""
}'
```
Congratulations! You have successfully set up an integration to synchronize secrets from Infisical with AWS SSM.
For more information, [view the integration API reference](../../endpoints/integrations).
# API Reference
Infisical's Public (REST) API provides users an alternative way to programmatically access and manage
secrets via HTTPS requests. This can be useful for automating tasks, such as
rotating credentials, or for integrating secret management into a larger system.
With the Public API, you can create, read, update, and delete secrets, as well as manage access control, query audit logs, and more.
# Changelog
The changelog below reflects new product developments and updates on a monthly basis.
## October 2024
* Significantly improved performance of audit log operations in UI.
* Released [Databricks integration](https://infisical.com/docs/integrations/cloud/databricks).
* Added ability to enforce 2FA organization-wide.
* Added multiple resource to the [Infisical Terraform Provider](https://registry.terraform.io/providers/Infisical/infisical/latest/docs), including AWS and GCP integrations.
* Released [Infisical KMS](https://infisical.com/docs/documentation/platform/kms/overview).
* Added support for [LDAP dynamic secrets](https://infisical.com/docs/documentation/platform/ldap/overview).
* Enabled changing auth methods for machine identities in the UI.
* Launched [Infisical EU Cloud](https://eu.infisical.com).
## September 2024
* Improved paginations for identities and secrets.
* Significant improvements to the [Infisical Terraform Provider](https://registry.terraform.io/providers/Infisical/infisical/latest/docs).
* Created [Slack Integration](https://infisical.com/docs/documentation/platform/workflow-integrations/slack-integration#slack-integration) for Access Requests and Approval Workflows.
* Added Dynamic Secrets for [Elaticsearch](https://infisical.com/docs/documentation/platform/dynamic-secrets/elastic-search) and [MongoDB](https://infisical.com/docs/documentation/platform/dynamic-secrets/mongo-db).
* More authentication methods are now supported by Infisical SDKs and Agent.
* Integrations now have dedicated audit logs and an overview screen.
* Added support for secret referencing in the Terraform Provider.
* Released support for [older versions of .NET](https://www.nuget.org/packages/Infisical.Sdk#supportedframeworks-body-tab) via SDK.
* Released Infisical PKI Issuer which works alongside `cert-manager` to manage certificates in Kubernetes.
## August 2024
* Added [Azure DevOps integration](https://infisical.com/docs/integrations/cloud/azure-devops).
* Released ability to hot-reload variables in CLI ([--watch flag](https://infisical.com/docs/cli/commands/run#infisical-run:watch)).
* Added Dynamic Secrets for [Redis](https://infisical.com/docs/documentation/platform/dynamic-secrets/redis).
* Added [Alerting](https://infisical.com/docs/documentation/platform/pki/alerting) for Certificate Management.
* You can now specify roles and project memberships when adding new users.
* Approval workflows now have email notifications.
* Access requests are now integrated with User Groups.
* Released ability to use IAM Roles for AWS Integrations.
## July 2024
* Released the official [Ruby SDK](https://infisical.com/docs/sdks/languages/ruby).
* Increased the speed and efficiency of secret operations.
* Released AWS KMS wrapping (bring your own key).
* Users can now log in to CLI via SSO in non-browser environments.
* Released [Slack Webhooks](https://infisical.com/docs/documentation/platform/webhooks).
* Added [Dynamic Secrets with MS SQL](https://infisical.com/docs/documentation/platform/dynamic-secrets/mssql).
* Redesigned and simplified the Machine Identities page.
* Added the ability to move secrets/folders to another location.
* Added [OIDC](https://infisical.com/docs/documentation/platform/identities/oidc-auth/general) support to CLI, Go SDK, and more.
* Released [Linux installer for Infisical](https://infisical.com/docs/self-hosting/deployment-options/native/standalone-binary).
## June 2024
* Released [Infisical PKI](https://infisical.com/docs/documentation/platform/pki/overview).
* Released the official [Go SDK](https://infisical.com/docs/sdks/languages/go).
* Released [OIDC Authentication method](https://infisical.com/docs/documentation/platform/identities/oidc-auth/general).
* Allowed users to configure log retention periods on self-hosted instances.
* Added [tags](https://registry.terraform.io/providers/Infisical/infisical/latest/docs/resources/secret_tag) to terraform provider.
* Released [public secret sharing](https://share.infisical.com).
* Built a [native integration with Rundeck](https://infisical.com/docs/integrations/cicd/rundeck).
* Added list view for projects in the dashboard.
* Fixed offline coding mode in CLI.
* Users are now able to leave a particular project themselves.
## May 2024
* Released [AWS](https://infisical.com/docs/documentation/platform/identities/aws-auth), [GCP](https://infisical.com/docs/documentation/platform/identities/gcp-auth), [Azure](https://infisical.com/docs/documentation/platform/identities/azure-auth), and [Kubernetes](https://infisical.com/docs/documentation/platform/identities/kubernetes-auth) Native Auth Methods.
* Added [Secret Sharing](https://infisical.com/docs/documentation/platform/secret-sharing) functionality for sharing sensitive data through encrypted links – within and outside of an organization.
* Updated [Secret Referencing](https://infisical.com/docs/documentation/platform/secret-reference) to be supported in all Infisical clients. Infisical UI is now able to provide automatic reference suggestions when typing.
* Released new [Infisical Jenkins Plugin](https://infisical.com/docs/integrations/cicd/jenkins).
* Added statuses and manual sync option to integrations in the Dashboard UI.
* Released universal [Audit Log Streaming](https://infisical.com/docs/documentation/platform/audit-log-streams).
* Added [Dynamic Secret template for AWS IAM](https://infisical.com/docs/documentation/platform/dynamic-secrets/aws-iam).
* Added support for syncing tags and custom KMS keys to [AWS Secrets Manager](https://infisical.com/docs/integrations/cloud/aws-secret-manager) and [Parameter Store](https://infisical.com/docs/integrations/cloud/aws-parameter-store) Integrations.
* Officially released Infisical on [AWS Marketplace](https://infisical.com/blog/infisical-launches-on-aws-marketplace).
## April 2024
* Added [Access Requests](https://infisical.com/docs/documentation/platform/access-controls/access-requests) as part of self-serve secrets management workflows.
* Added [Temporary Access Provisioning](https://infisical.com/docs/documentation/platform/access-controls/temporary-access) for roles and additional privileges.
## March 2024
* Released support for [Dynamic Secrets](https://infisical.com/docs/documentation/platform/dynamic-secrets/overview).
* Released the concept of [Additional Privileges](https://infisical.com/docs/documentation/platform/access-controls/additional-privileges) on top of user/machine roles.
## Feb 2024
* Added org-scoped authentication enforcement for SAML
* Added support for [SCIM](https://infisical.com/docs/documentation/platform/scim/overview) along with instructions for setting it up with [Okta](https://infisical.com/docs/documentation/platform/scim/okta), [Azure](https://infisical.com/docs/documentation/platform/scim/azure), and [JumpCloud](https://infisical.com/docs/documentation/platform/scim/jumpcloud).
* Pushed out project update for non-E2EE w/ new endpoints like for project creation and member invitation.
* Added API Integration testing for new backend.
* Added capability to create projects in Terraform.
* Added slug-based capabilities to both organizations and projects to gradually make the API more developer-friendly moving forward.
* Fixed + improved various analytics/telemetry-related items.
* Fixed various issues associated with the Python SDK: build during installation on Mac OS, Rust dependency.
* Updated self-hosting documentation to reflect [new backend](https://infisical.com/docs/self-hosting/overview).
* Released [Postgres-based Infisical helm chart](https://cloudsmith.io/~infisical/repos/helm-charts/packages/detail/helm/infisical-standalone/).
* Added checks to ensure that breaking API changes don't get released.
* Automated API reference documentation to be inline with latest releases of Infisical.
## Jan 2024
* Completed Postgres migration initiative with restructed Fastify-based backend.
* Reduced size of Infisical Node.js SDK by ≈90%.
* Added secret fallback support to all SDK's.
* Added Machine Identity support to [Terraform Provider](https://github.com/Infisical/terraform-provider-infisical).
* Released [.NET SDK](https://infisical.com/docs/sdks/languages/csharp).
* Added symmetric encryption support to all SDK's.
* Fixed secret reminders bug, where reminders were not being updated correctly.
## Dec 2023
* Released [(machine) identities](https://infisical.com/docs/documentation/platform/identities/overview) and [universal auth](https://infisical.com/docs/documentation/platform/identities/universal-auth) features.
* Created new cross-language SDKs for [Python](https://infisical.com/docs/sdks/languages/python), [Node](https://infisical.com/docs/sdks/languages/node), and [Java](https://infisical.com/docs/sdks/languages/java).
* Released first version of the [Infisical Agent](https://infisical.com/docs/infisical-agent/overview)
* Added ability to [manage folders via CLI](https://infisical.com/docs/cli/commands/secrets).
## Nov 2023
* Replaced internal [Winston](https://github.com/winstonjs/winston) with [Pino](https://github.com/pinojs/pino) logging library with external logging to AWS CloudWatch
* Added admin panel to self-hosting experience.
* Released [secret rotation](https://infisical.com/docs/documentation/platform/secret-rotation/overview) feature with preliminary support for rotating [SendGrid](https://infisical.com/docs/documentation/platform/secret-rotation/sendgrid), [PostgreSQL/CockroachDB](https://infisical.com/docs/documentation/platform/secret-rotation/postgres), and [MySQL/MariaDB](https://infisical.com/docs/documentation/platform/secret-rotation/mysql) credentials.
* Released secret reminders feature.
## Oct 2023
* Added support for [GitLab SSO](https://infisical.com/docs/documentation/platform/sso/gitlab).
* Became SOC 2 (Type II) certified.
* Reduced required JWT configuration from 5-6 secrets to 1 secret for self-hosting Infisical.
* Compacted Infisical into 1 Docker image.
* Added native [Hasura Cloud integration](https://infisical.com/docs/integrations/cloud/hasura-cloud).
* Updated resource deletion logic for user, organization, and project deletion.
## Sep 2023
* Released [secret approvals](https://infisical.com/docs/documentation/platform/pr-workflows) feature.
* Released an update to access controls; every user role now clearly defines and enforces a certain set of conditions across Infisical.
* Updated UI/UX for integrations.
* Added a native integration with [Qovery](https://infisical.com/docs/integrations/cloud/qovery).
* Added service token generation capability for the CLI.
## Aug 2023
* Release Audit Logs V2.
* Add support for [GitHub SSO](https://infisical.com/docs/documentation/platform/sso/github).
* Enable users to opt in for multiple authentication methods.
* Improved password requirements including check against [Have I Been Pwnd Password API](https://haveibeenpwned.com/Passwords).
* Added native [GCP Secret Manager integration](https://infisical.com/docs/integrations/cloud/gcp-secret-manager)
## July 2023
* Released [secret referencing and importing](https://infisical.com/docs/documentation/platform/secret-reference) across folders and environments.
* Redesigned the project/organization experience.
* Updated the secrets overview page; users are now able to edit secrets directly from it.
* Added native [Laravel Forge integration](https://infisical.com/docs/integrations/cloud/laravel-forge).
* Added native [Codefresh integration](https://infisical.com/docs/integrations/cicd/codefresh).
* Added native [Bitbucket integration](https://infisical.com/docs/integrations/cicd/bitbucket).
* Added native [DigitalOcean App Platform integration](https://infisical.com/docs/integrations/cloud/digital-ocean-app-platform).
* Added native [Cloud66 integration](https://infisical.com/docs/integrations/cloud/cloud-66).
* Added native [Terraform Cloud integration](https://infisical.com/docs/integrations/cloud/terraform-cloud).
* Added native [Northflank integration](https://infisical.com/docs/integrations/cloud/northflank).
* Added native [Windmill integration](https://infisical.com/docs/integrations/cloud/windmill).
* Added support for [Google SSO](https://infisical.com/docs/documentation/platform/sso/google)
* Added support for [Okta](https://infisical.com/docs/documentation/platform/sso/okta), [Azure AD](https://infisical.com/docs/documentation/platform/sso/azure), and [JumpCloud](https://infisical.com/docs/documentation/platform/sso/jumpcloud) [SAML](https://infisical.com/docs/documentation/platform/saml) authentication.
* Released [folders / path-based secret storage](https://infisical.com/docs/documentation/platform/folder).
* Released [webhooks](https://infisical.com/docs/documentation/platform/webhooks).
## June 2023
* Released the [Terraform Provider](https://infisical.com/docs/integrations/frameworks/terraform#5-run-terraform).
* Updated the usage and billing page. Added the free trial for the professional tier.
* Added native integrations with [Checkly](https://infisical.com/docs/integrations/cloud/checkly), [Hashicorp Vault](https://infisical.com/docs/integrations/cloud/hashicorp-vault), and [Cloudflare Pages](https://infisical.com/docs/integrations/cloud/cloudflare-pages).
* Completed a penetration test with a `very good` result.
* Added support for multi-line secrets.
## May 2023
* Released secret scanning capability for the CLI.
* Released customer / license service to manage customer billing information, cloud plans, and self-hosted enterprise licenses; all instances of Infisicals now fetch/relay information from this service.
* Completed penetration test.
* Released new landing page.
* Started SOC 2 (Type II) compliance certification preparation.
* Released new deployment options for Fly.io, Digital Ocean and Render.
## April 2023
* Upgraded secret-handling to include blind-indexing (can be thought of as a fingerprint).
* Added Node SDK support for working with individual secrets.
* Released preliminary Python SDK.
* Released service accounts, a client type capable of accessing multiple projects.
* Added native Supabase integration.
* Added native Railway integration.
* Improved dashboard speed / performance.
* Released the Secrets Overview page for users to view and identify missing environment secrets within one dashboard.
* Updated documentation to include quickstarts and guides; also updated `README.md`.
## March 2023
* Added support for global configs to the Kubernetes operator.
* Added support for self-hosted deployments to operate without any attached email service / SMTP configuration.
* Added native Azure Key Vault integration.
* Released one-click AWS EC2 deployment method.
* Released preliminary Node SDK.
## Feb 2023
* Upgraded private key encryption/decryption mechanism to use Argon2id and 256-bit protected keys.
* Added preliminary email-based 2FA capability.
* Added suspicious login alerting if user logs in via new device or IP address.
* Added documentation for PM2 integration.
* Added secret backups support for the CLI; it now fetches and caches secrets locally to be used in the event of future failed fetch.
* Added support for comparing secret values across environments on each secret.
* Added native AWS Parameter Store integration.
* Added native AWS Secrets Manager integration.
* Added native GitLab integration.
* Added native CircleCI integration.
* Added native Travis CI integration.
* Added secret tagging capability for enhanced organizational structure/grouping.
* Released new dashboard design allowing more actions to be performed within the dashboard itself.
* Added capability to generate `.env.example` file.
## Jan 2023
* Added preliminary audit logging capability covering CRUD secret operations.
* Added secret overriding capability for team members to have their own branch of a secret.
* Added secret versioning capability.
* Added secret snapshot and point-in-time recovery capabilities to track and roll back the full state of a project.
* Added native Vercel integration.
* Added native Netlify integration.
* Added native GitHub Actions integration.
* Added custom environment names.
* Added auto-redeployment capability to the Kubernetes operator.
* (Service Token 2.0) Shortened the length of service tokens.
* Added a public-facing API.
* Added preliminary access control capability for users to be provisioned read/write access to environments.
* Performed various web UI optimizations.
## Nov 2022
* Infisical is open sourced.
* Added Infisical CLI support for Docker and Docker Compose.
* Rewrote the Infisical CLI in Golang to be platform-agnostic.
* Rewrote the documentation.
## Oct 2022
* Added support for organizations; projects now belong to organizations.
* Improved speed / performance of dashboard by 25x.
* Added capability to change account password in settings.
* Added persistence for logging into the organization and project that users left from in their previous session.
* Added password recovery emergency kit with automatic download enforcement upon account creation.
* Added capability to copy-to-clipboard capabilities.
* Released first native integration between Infisical and Heroku; environment variables can now be sent and kept in sync with Heroku.
## Sep 2022
* Added capability to change user roles in projects.
* Added capability to delete projects.
* Added Stripe.
* Added default environments (development, staging, production) for new users with example key-pairs.
* Added loading indicators.
* Moved from push/pull mode of secret operation to automatically pulling and injecting secrets into processes upon startup.
* Added drag-and-drop capability for adding new .env files.
* Improved security measures against common attacks (e.g. XSS, clickjacking, etc.).
* Added support for personal secrets (later modified to be secret overrides in Jan 2023).
* Improved account password validation and enforce minimum requirements.
* Added sorting capability to sort keys by name alphabetically in dashboard.
* Added downloading secrets back as `.env` file capability.
## Aug 2022
* Released first version of the Infisical platform with push/pull capability and end-to-end encryption.
* Improved security handling of authentication tokens by storing refresh tokens in HttpOnly cookies.
* Added hiding key values on client-side.
* Added search bar to dashboard to query for keys on client-side.
* Added capability to rename a project.
* Added user roles for projects.
* Added incident contacts.
# infisical export
Export Infisical secrets from CLI into different file formats
```bash
infisical export [options]
```
## Description
Export environment variables from the platform into a file format.
## Subcommands & flags
Use this command to export environment variables from the platform into a raw file formats
```bash
$ infisical export
# Export variables to a .env file
infisical export > .env
# Export variables to a .env file (with export keyword)
infisical export --format=dotenv-export > .env
# Export variables to a CSV file
infisical export --format=csv > secrets.csv
# Export variables to a JSON file
infisical export --format=json > secrets.json
# Export variables to a YAML file
infisical export --format=yaml > secrets.yaml
# Render secrets using a custom template file
infisical export --template=
```
### Environment variables
Used to fetch secrets via a [machine identities](/documentation/platform/identities/machine-identities) apposed to logged in credentials. Simply, export this variable in the terminal before running this command.
```bash
# Example
export INFISICAL_TOKEN=$(infisical login --method=universal-auth --client-id= --client-secret= --silent --plain) # --plain flag will output only the token, so it can be fed to an environment variable. --silent will disable any update messages.
```
Alternatively, you may use service tokens.
```bash
# Example
export INFISICAL_TOKEN=
```
Used to disable the check for new CLI versions. This can improve the time it takes to run this command. Recommended for production environments.
To use, simply export this variable in the terminal before running this command.
```bash
# Example
export INFISICAL_DISABLE_UPDATE_CHECK=true
```
### flags
The `--template` flag specifies the path to the template file used for rendering secrets. When using templates, you can omit the other format flags.
```text my-template-file
{{$secrets := secret "" "" ""}}
{{$length := len $secrets}}
{{- "{"}}
{{- with $secrets }}
{{- range $index, $secret := . }}
"{{ $secret.Key }}": "{{ $secret.Value }}"{{if lt $index (minus $length 1)}},{{end}}
{{- end }}
{{- end }}
{{ "}" -}}
```
```bash
# Example
infisical export --template="/path/to/template/file"
```
Used to set the environment that secrets are pulled from.
```bash
# Example
infisical export --env=prod
```
Note: this flag only accepts environment slug names not the fully qualified name. To view the slug name of an environment, visit the project settings page.
default value: `dev`
By default the project id is retrieved from the `.infisical.json` located at the root of your local project.
This flag allows you to override this behavior by explicitly defining the project to fetch your secrets from.
```bash
# Example
infisical export --projectId=XXXXXXXXXXXXXX
```
Parse shell parameter expansions in your secrets (e.g., `${DOMAIN}`)
Default value: `true`
By default imported secrets are available, you can disable it by setting this option to false.
Default value: `true`
Format of the output file. Accepted values: `dotenv`, `dotenv-export`, `csv`, `json` and `yaml`
Default value: `dotenv`
Prioritizes personal secrets with the same name over shared secrets
Default value: `true`
The `--path` flag indicates which project folder secrets will be injected from.
```bash
# Example
infisical export --path="/path/to/folder" --env=dev
```
When working with tags, you can use this flag to filter and retrieve only secrets that are associated with a specific tag(s).
```bash
# Example
infisical run --tags=tag1,tag2,tag3 -- npm run dev
```
Note: you must reference the tag by its slug name not its fully qualified name. Go to project settings to view all tag slugs.
By default, all secrets are fetched
# infisical init
Switch between Infisical projects within CLI
```bash
infisical init
```
## Description
Link a local project to your Infisical project. Once connected, you can then access the secrets locally from the connected Infisical project.
This command creates a `infisical.json` file containing your Project ID.
# infisical login
Login into Infisical from the CLI
```bash
infisical login
```
### Description
The CLI uses authentication to verify your identity. When you enter the correct email and password for your account, a token is generated and saved in your system Keyring to allow you to make future interactions with the CLI.
To change where the login credentials are stored, visit the [vaults command](./vault).
If you have added multiple users, you can switch between the users by using the [user command](./user).
When you authenticate with **any other method than `user`**, an access token will be printed to the console upon successful login. This token can be used to authenticate with the Infisical API and the CLI by passing it in the `--token` flag when applicable.
Use flag `--plain` along with `--silent` to print only the token in plain text when using a machine identity auth method.
### Authentication Methods
The Infisical CLI supports multiple authentication methods. Below are the available authentication methods, with their respective flags.
The Universal Auth method is a simple and secure way to authenticate with Infisical. It requires a client ID and a client secret to authenticate with Infisical.
Your machine identity client ID.
Your machine identity client secret.
To create a universal auth machine identity, follow the step by step guide outlined [here](/documentation/platform/identities/universal-auth).
Run the `login` command with the following flags to obtain an access token:
```bash
infisical login --method=universal-auth --client-id= --client-secret=
```
The Native Kubernetes method is used to authenticate with Infisical when running in a Kubernetes environment. It requires a service account token to authenticate with Infisical.
Your machine identity ID.
Path to the Kubernetes service account token to use. Default: `/var/run/secrets/kubernetes.io/serviceaccount/token`.
To create a Kubernetes machine identity, follow the step by step guide outlined [here](/documentation/platform/identities/kubernetes-auth).
Run the `login` command with the following flags to obtain an access token:
```bash
# --service-account-token-path is optional, and will default to '/var/run/secrets/kubernetes.io/serviceaccount/token' if not provided.
infisical login --method=kubernetes --machine-identity-id= --service-account-token-path=
```
The Native Azure method is used to authenticate with Infisical when running in an Azure environment.
Your machine identity ID.
To create an Azure machine identity, follow the step by step guide outlined [here](/documentation/platform/identities/azure-auth).
Run the `login` command with the following flags to obtain an access token:
```bash
infisical login --method=azure --machine-identity-id=
```
The Native GCP ID Token method is used to authenticate with Infisical when running in a GCP environment.
Your machine identity ID.
To create a GCP machine identity, follow the step by step guide outlined [here](/documentation/platform/identities/gcp-auth).
Run the `login` command with the following flags to obtain an access token:
```bash
infisical login --method=gcp-id-token --machine-identity-id=
```
The GCP IAM method is used to authenticate with Infisical with a GCP service account key.
Your machine identity ID.
Path to your GCP service account key file *(Must be in JSON format!)*
To create a GCP machine identity, follow the step by step guide outlined [here](/documentation/platform/identities/gcp-auth).
Run the `login` command with the following flags to obtain an access token:
```bash
infisical login --method=gcp-iam --machine-identity-id= --service-account-key-file-path=
```
The AWS IAM method is used to authenticate with Infisical with an AWS IAM role while running in an AWS environment like EC2, Lambda, etc.
Your machine identity ID.
To create an AWS machine identity, follow the step by step guide outlined [here](/documentation/platform/identities/aws-auth).
Run the `login` command with the following flags to obtain an access token:
```bash
infisical login --method=aws-iam --machine-identity-id=
```
The OIDC Auth method is used to authenticate with Infisical via identity tokens with OIDC.
Your machine identity ID.
The OIDC JWT from the identity provider.
To create an OIDC machine identity, follow the step by step guide outlined [here](/documentation/platform/identities/oidc-auth/general).
Run the `login` command with the following flags to obtain an access token:
```bash
infisical login --method=oidc-auth --machine-identity-id= --oidc-jwt=
```
### Flags
The login command supports a number of flags that you can use for different authentication methods. Below is a list of all the flags that can be used with the login command.
```bash
infisical login --method= # Optional, will default to 'user'.
```
#### Valid values for the `method` flag are:
* `user`: Login using email and password. (default)
* `universal-auth`: Login using a universal auth client ID and client secret.
* `kubernetes`: Login using a Kubernetes native auth.
* `azure`: Login using an Azure native auth.
* `gcp-id-token`: Login using a GCP ID token native auth.
* `gcp-iam`: Login using a GCP IAM.
* `aws-iam`: Login using an AWS IAM native auth.
* `oidc-auth`: Login using oidc auth.
```bash
infisical login --client-id= # Optional, required if --method=universal-auth.
```
#### Description
The client ID of the universal auth machine identity. This is required if the `--method` flag is set to `universal-auth`.
The `client-id` flag can be substituted with the `INFISICAL_UNIVERSAL_AUTH_CLIENT_ID` environment variable.
```bash
infisical login --client-secret= # Optional, required if --method=universal-auth.
```
#### Description
The client secret of the universal auth machine identity. This is required if the `--method` flag is set to `universal-auth`.
The `client-secret` flag can be substituted with the `INFISICAL_UNIVERSAL_AUTH_CLIENT_SECRET` environment variable.
```bash
infisical login --machine-identity-id= # Optional, required if --method=kubernetes, azure, gcp-id-token, gcp-iam, or aws-iam.
```
#### Description
The ID of the machine identity. This is required if the `--method` flag is set to `kubernetes`, `azure`, `gcp-id-token`, `gcp-iam`, or `aws-iam`.
The `machine-identity-id` flag can be substituted with the `INFISICAL_MACHINE_IDENTITY_ID` environment variable.
```bash
infisical login --service-account-token-path= # Optional Will default to '/var/run/secrets/kubernetes.io/serviceaccount/token'.
```
#### Description
The path to the Kubernetes service account token to use for authentication.
This is optional and will default to `/var/run/secrets/kubernetes.io/serviceaccount/token`.
The `service-account-token-path` flag can be substituted with the `INFISICAL_KUBERNETES_SERVICE_ACCOUNT_TOKEN_PATH` environment variable.
```bash
infisical login --service-account-key-file-path= # Optional, but required if --method=gcp-iam.
```
#### Description
The path to your GCP service account key file. This is required if the `--method` flag is set to `gcp-iam`.
The `service-account-key-path` flag can be substituted with the `INFISICAL_GCP_IAM_SERVICE_ACCOUNT_KEY_FILE_PATH` environment variable.
```bash
infisical login --oidc-jwt=
```
#### Description
The JWT provided by an identity provider for OIDC authentication.
The `oidc-jwt` flag can be substituted with the `INFISICAL_OIDC_AUTH_JWT` environment variable.
### Machine Identity Authentication Quick Start
In this example we'll be using the `universal-auth` method to login to obtain an Infisical access token, which we will then use to fetch secrets with.
```bash
export INFISICAL_TOKEN=$(infisical login --method=universal-auth --client-id= --client-secret= --silent --plain) # silent and plain is important to ensure only the token itself is printed, so we can easily set it as an environment variable.
```
Now that we've set the `INFISICAL_TOKEN` environment variable, we can use the CLI to interact with Infisical. The CLI will automatically check for the presence of the `INFISICAL_TOKEN` environment variable and use it for authentication.
Alternatively, if you would rather use the `--token` flag to pass the token directly, you can do so by running the following command:
```bash
infisical [command] --token= # The token output from the login command.
```
```bash
infisical secrets --projectId=
The `--recursive`, and `--env` flag is optional and will fetch all secrets in subfolders. The default environment is `dev` if no `--env` flag is provided.
# infisical reset
Reset Infisical
```bash
infisical reset
```
## Description
This command provides a way to clear all Infisical-generated configuration data, effectively resetting the software to its default settings. This can be an effective way to address any persistent issues that arise while using the CLI.
# infisical run
The command that injects your secrets into local environment
```bash
infisical run [options] -- [your application start command]
# Example
infisical run [options] -- npm run dev
```
```bash
infisical run [options] --command [string command]
# Example
infisical run [options] --command "npm run bootstrap && npm run dev start; other-bash-command"
```
## Description
Inject secrets from Infisical into your application process.
## Subcommands & flags
Use this command to inject secrets into your applications process
```bash
$ infisical run --
# Example
$ infisical run -- npm run dev
```
### Environment variables
Used to fetch secrets via a [machine identity](/documentation/platform/identities/machine-identities) apposed to logged in credentials. Simply, export this variable in the terminal before running this command.
```bash
# Example
export INFISICAL_TOKEN=$(infisical login --method=universal-auth --client-id= --client-secret= --silent --plain) # --plain flag will output only the token, so it can be fed to an environment variable. --silent will disable any update messages.
```
Alternatively, you may use service tokens.
```bash
# Example
export INFISICAL_TOKEN=
```
Used to disable the check for new CLI versions. This can improve the time it takes to run this command. Recommended for production environments.
To use, simply export this variable in the terminal before running this command.
```bash
# Example
export INFISICAL_DISABLE_UPDATE_CHECK=true
```
### Flags
By passing the `watch` flag, you are telling the CLI to watch for changes that happen in your Infisical project.
If secret changes happen, the command you provided will automatically be restarted with the new environment variables attached.
```bash
# Example
infisical run --watch -- printenv
```
Explicitly set the directory where the .infisical.json resides. This is useful for some monorepo setups.
```bash
# Example
infisical run --project-config-dir=/some-dir -- printenv
```
Pass secrets into multiple commands at once
```bash
# Example
infisical run --command="npm run build && npm run dev; more-commands..."
```
The project ID to fetch secrets from. This is required when using a machine identity to authenticate.
```bash
# Example
infisical run --projectId= -- npm run dev
```
If you are using a [machine identity](/documentation/platform/identities/machine-identities) to authenticate, you can pass the token as a flag
```bash
# Example
infisical run --token="" --projectId= -- npm run start
```
You may also expose the token to the CLI by setting the environment variable `INFISICAL_TOKEN` before executing the run command. This will have the same effect as setting the token with `--token` flag
Turn on or off the shell parameter expansion in your secrets. If you have used shell parameters in your secret(s), activating this feature will populate them before injecting them into your application process.
Default value: `true`
By default imported secrets are available, you can disable it by setting this option to false.
Default value: `true`
{" "}
This is used to specify the environment from which secrets should be
retrieved. The accepted values are the environment slugs defined for your
project, such as `dev`, `staging`, `test`, and `prod`. Default value: `dev`
Prioritizes personal secrets with the same name over shared secrets
Default value: `true`
When working with tags, you can use this flag to filter and retrieve only secrets that are associated with a specific tag(s).
```bash
# Example
infisical run --tags=tag1,tag2,tag3 -- npm run dev
```
Note: you must reference the tag by its slug name not its fully qualified name. Go to project settings to view all tag slugs.
By default, all secrets are fetched
The `--path` flag indicates which project folder secrets will be injected from.
```bash
# Example
infisical run --path="/nextjs" -- npm run dev
```
## Automatically reload command when secrets change
To automatically reload your command when secrets change, use the `--watch` flag.
```bash
infisical run --watch -- npm run dev
```
This will watch for changes in your secrets and automatically restart your command with the new secrets.
When your command restarts, it will have the new environment variables injeceted into it.
Please note that this feature is intended for development purposes. It is not recommended to use this in production environments. Generally it's not recommended to automatically reload your application in production when remote changes are made.
# scan
Scan git history, directories, and files for secrets
```bash
infisical scan
# Display the full secret findings
infisical scan --verbose
```
## Description
The `infisical scan` command serves to scan repositories, directories, and files. It's compatible with both individual developer machines and Continuous Integration (CI) environments.
When you run `infisical scan` on a Git repository, Infisical will parses the output of a `git log -p` command. This command generates [patches](https://stackoverflow.com/questions/8279602/what-is-a-patch-in-git-version-control) that Infisical uses to identify secrets in your code.
You can configure the range of commits that `git log` will cover using the `--log-opts` flag.
Any options you can use with `git log -p` are valid for `--log-opts`.
For instance, to instruct Infisical to scan a specific range of commits, use the following command: `infisical scan --log-opts="--all commitA..commitB"`. For more details, refer to the [Git log documentation](https://git-scm.com/docs/git-log).
To scan individual files and directories, use the `--no-git` flag.
### Flags
**Description**
git log options
**Description**
treat git repo as a regular directory and scan those files, --log-opts has no effect on the scan when --no-git is set
Default value: `false`
Short hand: `-b`
**Description**
scan input from stdin, ex: `cat some_file | infisical scan --pipe`
Default value: `false`
Short hand: `-b`
**Description**
scan files that are symlinks to other files
Default value: `false`
Short hand: `-b`
**Description**
path to baseline with issues that can be ignored
Short hand: `-c`
**Description**
config file path
order of precedence:
1. \--config flag
2. env var INFISICAL\_SCAN\_CONFIG
3. (--source/-s)/.infisical-scan.toml
If none of the three options are used, then Infisical will use the default config
**Description**
exit code when leaks have been encountered (default 1)
**Description**
files larger than this will be skipped
**Description**
turn off color for verbose output
**Description**
redact secrets from logs and stdout
**Description**
output format (json, csv, sarif) (default "json")
**Description**
report file
**Description**
path to source (default ".")
**Description**
show verbose output from scan
# scan git-changes
Scan for secrets in your uncommitted code
```bash
infisical scan git-changes
# Display the full secret findings
infisical scan git-changes --verbose
```
## Description
Scanning for secrets before you commit your changes is great way to prevent leaks. Infisical makes this easy with the sub command `git-changes`.
The `git-changes` scans for uncommitted changes in a Git repository, and is especially designed for use on developer machines, aligning with the ['shift left'](https://cloud.google.com/architecture/devops/devops-tech-shifting-left-on-security) security approach.
When `git-changes` is run on a Git repository, Infisical parses the output from a `git diff` command.
To scan changes in commits that have been staged via `git add`, you can add the `--staged` flag to the sub command. This flag is particularly useful when using Infisical CLI as a pre-commit tool.
### Flags
**Description**
detect secrets in a --staged state
Default value: `false`
**Description**
git log options
Short hand: `-b`
**Description**
path to baseline with issues that can be ignored
Short hand: `-c`
**Description**
config file path
order of precedence:
1. \--config flag
2. env var INFISICAL\_SCAN\_CONFIG
3. (--source/-s)/.infisical-scan.toml
If none of the three options are used, then Infisical will use the default config
**Description**
exit code when leaks have been encountered (default 1)
**Description**
files larger than this will be skipped
**Description**
turn off color for verbose output
**Description**
redact secrets from logs and stdout
**Description**
output format (json, csv, sarif) (default "json")
**Description**
report file
**Description**
path to source (default ".")
**Description**
show verbose output from scan
# scan install
Add various scanning tools seamlessly into your development lifecycle
```bash
infisical scan install --pre-commit-hook
```
## Description
The command `infisical scan install` is designed to incorporate various scanning tools seamlessly into your development lifecycle.
Initially, we are offering users the ability to install a pre-commit hook. This hook conducts an automatic scan for any exposed secrets in your commits before they are pushed.
### Flags
```bash
infisical scan install --pre-commit-hook
```
**Description**
Installs a git pre-commit hook that triggers Infisical to scan your staged changes for any exposed secrets prior to pushing.
# infisical secrets
Perform CRUD operations with Infisical secrets
```
infisical secrets
```
## Description
This command enables you to perform CRUD (create, read, update, delete) operations on secrets within your Infisical project. With it, you can view, create, update, and delete secrets in your environment.
### Sub-commands
Use this command to print out all of the secrets in your project
```bash
$ infisical secrets
```
### Environment variables
Used to fetch secrets via a [machine identity](/documentation/platform/identities/machine-identities) apposed to logged in credentials. Simply, export this variable in the terminal before running this command.
```bash
# Example
export INFISICAL_TOKEN=$(infisical login --method=universal-auth --client-id= --client-secret= --silent --plain) # --plain flag will output only the token, so it can be fed to an environment variable. --silent will disable any update messages.
```
Alternatively, you may use service tokens.
```bash
# Example
export INFISICAL_TOKEN=
```
Used to disable the check for new CLI versions. This can improve the time it takes to run this command. Recommended for production environments.
To use, simply export this variable in the terminal before running this command.
```bash
# Example
export INFISICAL_DISABLE_UPDATE_CHECK=true
```
### Flags
Parse shell parameter expansions in your secrets
Default value: `true`
The project ID to fetch secrets from. This is required when using a machine identity to authenticate.
```bash
# Example
infisical secrets --projectId=
```
Used to select the environment name on which actions should be taken on
Default value: `dev`
The `--path` flag indicates which project folder secrets will be injected from.
```bash
# Example
infisical secrets --path="/" --env=dev
```
The `--plain` flag will output all your secret values without formatting, one per line.
```bash
# Example
infisical secrets --plain --silent
```
The `--silent` flag disables output of tip/info messages. Useful when running in scripts or CI/CD pipelines.
```bash
# Example
infisical secrets --silent
```
Can be used inline to replace `INFISICAL_DISABLE_UPDATE_CHECK`
This command allows you selectively print the requested secrets by name
```bash
$ infisical secrets get ...
# Example
$ infisical secrets get DOMAIN
$ infisical secrets get DOMAIN PORT
```
### Flags
Used to select the environment name on which actions should be taken on
Default value: `dev`
The `--plain` flag will output all your requested secret values without formatting, one per line.
Default value: `false`
```bash
# Example
infisical secrets get FOO --plain
infisical secrets get FOO BAR --plain
# Fetch a single value and assign it to a variable
API_KEY=$(infisical secrets get FOO --plain --silent)
```
When running in CI/CD environments or in a script, set `INFISICAL_DISABLE_UPDATE_CHECK=true` or add the `--silent` flag. This will help hide any CLI info/debug output and only show the secret value.
The `--silent` flag disables output of tip/info messages. Useful when running in scripts or CI/CD pipelines.
```bash
# Example
infisical secrets get FOO --plain --silent
```
Can be used inline to replace `INFISICAL_DISABLE_UPDATE_CHECK`
Use `--plain` instead, as it supports single and multiple secrets.
Used to print the plain value of a single requested secret without any table style.
Default value: `false`
Example: `infisical secrets get DOMAIN --raw-value`
When running in CI/CD environments or in a script, set `INFISICAL_DISABLE_UPDATE_CHECK=true` or add the `--silent` flag. This will help hide any CLI info/debug output and only show the secret value.
This command allows you to set or update secrets in your environment. If the secret key provided already exists, its value will be updated with the new value.
If the secret key does not exist, a new secret will be created using both the key and value provided.
```bash
$ infisical secrets set ...
## Example
$ infisical secrets set STRIPE_API_KEY=sjdgwkeudyjwe DOMAIN=example.com HASH=jebhfbwe
```
### Flags
Used to select the environment name on which actions should be taken on
Default value: `dev`
Used to select the project folder in which the secrets will be set. This is useful when creating new secrets under a particular path.
```bash
# Example
infisical secrets set DOMAIN=example.com --path="common/backend"
```
Used to select the type of secret to create. This could be either personal or shared (defaults to shared)
```bash
# Example
infisical secrets set DOMAIN=example.com --type=personal
```
This command allows you to delete secrets by their name(s).
```bash
$ infisical secrets delete ...
## Example
$ infisical secrets delete STRIPE_API_KEY DOMAIN HASH
```
### Flags
Used to select the environment name on which actions should be taken on
Default value: `dev`
The `--path` flag indicates which project folder secrets will be injected from.
```bash
# Example
infisical secrets delete ... --path="/"
```
This command allows you to fetch, create and delete folders from within a path from a given project.
```bash
$ infisical secrets folders
```
### sub commands
Used to fetch all folders within a path in a given project
```
infisical secrets folders get --path=/some/path/to/folder
```
#### Flags
The path from where folders should be fetched from
Default value: `/`
Fetch folders using a [machine identity](/documentation/platform/identities/machine-identities) access token.
Default value: \`\`
Used to create a folder by name within a path.
```
infisical secrets folders create --path=/some/path/to/folder --name=folder-name
```
### Flags
Path to where the folder should be created
Default value: `/`
Name of the folder to be created in selected `--path`
Default value: \`\`
Used to delete a folder by name within a path.
```
infisical secrets folders delete --path=/some/path/to/folder --name=folder-name
```
### Flags
Path to where the folder should be created
Default value: `/`
Name of the folder to be deleted within selected `--path`
Default value: \`\`
This command allows you to generate an example .env file from your secrets and with their associated comments and tags. This is useful when you would like to let
others who work on the project but do not use Infisical become aware of the required environment variables and their intended values.
To place default values in your example .env file, you can simply include the syntax `DEFAULT:` within your secret's comment in Infisical. This will result in the specified value being extracted and utilized as the default.
```bash
$ infisical secrets generate-example-env
## Example
$ infisical secrets generate-example-env > .example-env
```
### Flags
Used to select the environment name on which actions should be taken on
Default value: `dev`
# infisical service-token
Manage Infisical service tokens
This command is deprecated and will be removed in the near future. Please
switch to using [Machine
Identities](/documentation/platform/identities/machine-identities) for
authenticating with Infisical.
```bash
infisical service-token create --scope=dev:/global --scope=dev:/backend --access-level=read --access-level=write
```
## Description
The Infisical `service-token` command allows you to manage service tokens for a given Infisical project.
With this command, you can create, view, and delete service tokens.
Use this command to create a service token
```bash
$ infisical service-token create --scope=dev:/backend/** --access-level=read --access-level=write
```
### Flags
```bash
infisical service-token create --scope=dev:/global --scope=dev:/backend/** --access-level=read
```
Use the scope flag to define which environments and paths your service token should be authorized to access.
The value of your scope flag should be in the following `:`.
Here, `environment slug` refers to the slug name of the environment, and `path` indicates the folder path where your secrets are stored.
For specifying multiple scopes, you can use multiple --scope flags.
The `path` can be a Glob pattern
```bash
infisical service-token create --scope=dev:/global --access-level=read --projectId=63cefb15c8d3175601cfa989
```
The project ID you'd like to create the service token for.
By default, the CLI will attempt to use the linked Infisical project in `.infisical.json` generated by `infisical init` command.
```bash
infisical service-token create --scope=dev:/global --access-level=read --name service-token-name
```
Service token name
Default: `Service token generated via CLI`
```bash
infisical service-token create --scope=dev:/global --access-level=read --expiry-seconds 120
```
Set the service token's expiration time in seconds from now. To never expire set to zero.
Default: `1 day`
```bash
infisical service-token create --scope=dev:/global --access-level=read --access-level=write
```
The type of access the service token should have. Can be `read` and or `write`
```bash
infisical service-token create --scope=dev:/global --access-level=read --access-level=write --token-only
```
When true, only the service token will be printed
Default: `false`
# infisical token
Manage your Infisical identity access tokens
```bash
infisical token renew
```
## Description
The Infisical `token` command allows you to manage your universal auth access tokens.
With this command, you can renew your access tokens. In the future more subcommands will be added to better help you manage your tokens through the CLI.
Use this command to renew your access token. This command will renew your access token and output a renewed access token to the console.
```bash
$ infisical token renew
```
# infisical user
Manage logged in users
```bash
infisical user
```
## Description
This command allows you to manage the current logged in users on the CLI
### Sub-commands
Use this command to switch between profiles that are currently logged into the CLI
```bash
infisical user switch
```
With this command, you can modify the backend API that is utilized for all requests associated with a specific profile.
For instance, you have the option to point the profile to use either the Infisical Cloud or your own self-hosted Infisical instance.
```bash
infisical user update domain
```
# infisical vault
Change the vault type in Infisical
```bash
infisical vault
# Example output
Vaults are used to securely store your login details locally. Available vaults:
- auto (automatically select native vault on system)
- file (encrypted file vault)
You are currently using [file] vault to store your login credentials
```
```bash
infisical vault set
# Example
infisical vault set keychain
```
## Description
To safeguard your login details when using the CLI, Infisical attempts to store them in a system keyring. If a system keyring cannot be found on your machine, the data is stored in a config file.
# FAQ
Frequently Asked Questions about Infisical CLI
Frequently asked questions about the CLI can be found on this page.
If you can't find the answer you are looking for, please create an issue on our GitHub repository or join our Slack channel for additional support.
By default, the CLI will choose the most suitable store available on your system.
If you experience issues with the default store, you can switch to a different one.
If none of the available stores work for you, you can try using the `file` store type by running `infisical vault set file`, which should work in most cases.
If you are still experiencing trouble, please seek support.
[Learn more about vault command](./commands/vault)
Yes. If you have previously retrieved secrets for a specific project and environment (such as dev, staging, or prod), the `run`/`secret` command will utilize the saved secrets, even when offline, on subsequent fetch attempts.
Yes. This is simply a configuration file and contains no sensitive data.
Visit the Infisical website and navigate to a project of your choice. Once on the project page, access the **Project Settings** from the sidebar. Within the Project name section, click the "Copy Project ID" button for copying the current Project ID to clipboard, or simply obtain it from the URL of the current page.
```
https://app.infisical.com/project//settings
```
# Install
Infisical's CLI is one of the best way to manage environments and secrets. Install it here
The Infisical CLI is powerful command line tool that can be used to retrieve, modify, export and inject secrets into any process or application as environment variables.
You can use it across various environments, whether it's local development, CI/CD, staging, or production.
## Installation
Use [brew](https://brew.sh/) package manager
```bash
brew install infisical/get-cli/infisical
```
### Updates
```bash
brew update && brew upgrade infisical
```
Use [Scoop](https://scoop.sh/) package manager
```bash
scoop bucket add org https://github.com/Infisical/scoop-infisical.git
```
```bash
scoop install infisical
```
### Updates
```bash
scoop update infisical
```
Use [NPM](https://www.npmjs.com/) package manager
```bash
npm install -g @infisical/cli
```
### Updates
```bash
npm update -g @infisical/cli
```
Install prerequisite
```bash
apk add --no-cache bash sudo
```
Add Infisical repository
```bash
curl -1sLf \
'https://dl.cloudsmith.io/public/infisical/infisical-cli/setup.alpine.sh' \
| bash
```
Then install CLI
```bash
apk update && sudo apk add infisical
```
###
If you are installing the CLI in production environments, we highly recommend to set the version of the CLI to a specific version. This will help keep your CLI version consistent across reinstalls. [View versions](https://cloudsmith.io/~infisical/repos/infisical-cli/packages/)
Add Infisical repository
```bash
curl -1sLf \
'https://dl.cloudsmith.io/public/infisical/infisical-cli/setup.rpm.sh' \
| sudo -E bash
```
Then install CLI
```bash
sudo yum install infisical
```
###
If you are installing the CLI in production environments, we highly recommend to set the version of the CLI to a specific version. This will help keep your CLI version consistent across reinstalls. [View versions](https://cloudsmith.io/~infisical/repos/infisical-cli/packages/)
Add Infisical repository
```bash
curl -1sLf \
'https://dl.cloudsmith.io/public/infisical/infisical-cli/setup.deb.sh' \
| sudo -E bash
```
Then install CLI
```bash
sudo apt-get update && sudo apt-get install -y infisical
```
###
If you are installing the CLI in production environments, we highly recommend to set the version of the CLI to a specific version. This will help keep your CLI version consistent across reinstalls. [View versions](https://cloudsmith.io/~infisical/repos/infisical-cli/packages/)
Use the `yay` package manager to install from the [Arch User Repository](https://aur.archlinux.org/packages/infisical-bin)
```bash
yay -S infisical-bin
```
###
If you are installing the CLI in production environments, we highly recommend to set the version of the CLI to a specific version. This will help keep your CLI version consistent across reinstalls. [View versions](https://cloudsmith.io/~infisical/repos/infisical-cli/packages/)
## Quick Usage Guide
Now that you have the CLI installed on your system, follow this guide to make the best use of it
# Project config file
Project config file & customization options
To link your local project on your machine with an Infisical project, we suggest using the infisical init CLI command. This will generate a `.infisical.json` file in the root directory of your project.
The `.infisical.json` file specifies various parameters, such as the Infisical project to retrieve secrets from, along with other configuration options. Furthermore, you can define additional properties in the file to further tailor your local development experience.
## Set default environment
If you need to change environments while using the CLI, you can do so by including the `--env` flag in your command.
However, this can be inconvenient if you typically work in just one environment.
To simplify the process, you can establish a default environment, which will be used for every command unless you specify otherwise.
```json .infisical.json
{
"workspaceId": "63ee5410a45f7a1ed39ba118",
"defaultEnvironment": "test",
"gitBranchToEnvironmentMapping": null
}
```
### How it works
If both `defaultEnvironment` and `gitBranchToEnvironmentMapping` are configured, `gitBranchToEnvironmentMapping` will take precedence over `defaultEnvironment`.
However, if `gitBranchToEnvironmentMapping` is not set and `defaultEnvironment` is, then the `defaultEnvironment` will be used to execute your Infisical CLI commands.
If you wish to override the `defaultEnvironment`, you can do so by using the `--env` flag explicitly.
## Set Infisical environment based on GitHub branch
When fetching your secrets from Infisical, you can switch between environments by using the `--env` flag. However, in certain cases, you may prefer the environment to be automatically mapped based on the current GitHub branch you are working on.
To achieve this, simply add the `gitBranchToEnvironmentMapping` property to your configuration file, as shown below.
```json .infisical.json
{
"workspaceId": "63ee5410a45f7a1ed39ba118",
"gitBranchToEnvironmentMapping": {
"branchName": "dev",
"anotherBranchName": "staging"
}
}
```
### How it works
After configuring this property, every time you use the CLI with the specified configuration file, it will automatically verify if there is a corresponding environment mapping for the current Github branch you are on.
If it exists, the CLI will use that environment to retrieve secrets. You can override this behavior by explicitly using the `--env` flag while interacting with the CLI.
# Secret scanning
Scan and prevent secret leaks in your code base
Building upon its core functionality of fetching and injecting secrets into your applications, Infisical now takes a significant step forward in bolstering your code security.
We've enhanced our CLI tool to include a powerful scanning feature, capable of identifying more than 140 different types of secret leaks in your codebase.
In addition to scanning for past leaks, this new addition also actively aids in preventing future leaks.
# Scanning
```bash
infisical scan
# Display the full secret findings
infisical scan --verbose
```
The `infisical scan` command serves to scan repositories, directories, and files. It's compatible with both individual developer machines and Continuous Integration (CI) environments.
When you run `infisical scan` on a Git repository, Infisical will parses the output of a `git log -p` command. This command generates [patches](https://stackoverflow.com/questions/8279602/what-is-a-patch-in-git-version-control) that Infisical uses to identify secrets in your code.
You can configure the range of commits that `git log` will cover using the `--log-opts` flag.
Any options you can use with `git log -p` are valid for `--log-opts`.
For instance, to instruct Infisical to scan a specific range of commits, use the following command: `infisical scan --log-opts="--all commitA..commitB"`. For more details, refer to the [Git log documentation](https://git-scm.com/docs/git-log).
To scan individual files and directories, use the `--no-git` flag.
**View [full details for this command](./commands/scan)**
```bash
infisical scan git-changes
# Display the full secret findings
infisical scan git-changes --verbose
```
Scanning for secrets before you commit your changes is great way to prevent leaks. Infisical makes this easy with the sub command `git-changes`.
The `git-changes` scans for uncommitted changes in a Git repository, and is especially designed for use on developer machines, aligning with the ['shift left'](https://cloud.google.com/architecture/devops/devops-tech-shifting-left-on-security) security approach.
When `git-changes` is run on a Git repository, Infisical parses the output from a `git diff` command.
To scan changes in commits that have been staged via `git add`, you can add the `--staged` flag to the sub command. This flag is particularly useful when using Infisical CLI as a pre-commit tool.
**View [full details for this command](./commands/scan-git-changes)**
`git-changes` command is only for Git repositories; using it on files or directories will result in an error.
#
#
# Automatically scan changes before you commit
To lower the risk of committing hardcoded secrets to your code repository, we have designed a custom git pre-commit hook.
This hook scans the changes you're about to commit for any exposed secrets. If any hardcoded secrets are detected, it will block your commit.
### Install pre-commit hook
To install this git hook, go into your local git repository and run the following command.
```bash
infisical scan install --pre-commit-hook
```
To disable this hook after installing it, run the command `git config --bool hooks.infisical-scan false`
### Third party hooks management
If you would rather handle your pre-commit hook outside of the standard `.git/hooks` directory, you can quickly achieve this by adding the following command into your pre-commit script.
For instance, if you utilize [Husky](https://typicode.github.io/husky/) for managing your Git hooks, you can insert the command provided below into your `.husky/pre-commit` file.
```bash
infisical scan git-changes --staged --verbose
```
#
#
# Creating a baseline
When scanning large repositories or repositories with a long history, it can be helpful to use a baseline.
A baseline allows Infisical to ignore any old findings that are already present in the baseline findings. You can create a infisical scan report by running `infisical scan` with the `--report-path` flag.
To create a Infisical scan report and save it in a file called leaks-report.json, use the following command:
```
infisical scan --report-path leaks-report.json
```
Once a baseline is created, you can apply it when running the `infisical scan` command again. Use the following command:
```
infisical scan --baseline-path leaks-report.json --report-path findings.json
```
After running the `scan` command with the `--baseline-path` flag, the report output in findings.json will only contain new issues.
#
#
# Configuration file
To customize the scan, such as specifying your own rules or establishing exceptions for certain files or paths that should not be flagged as risks, you can define these specifications in the configuration file.
```toml infisical-scan.toml
# Title for the configuration file
title = "Some title"
# This configuration is the foundation that can be expanded. If there are any overlapping rules
# between this base and the expanded configuration, the rules in this base will take priority.
# Another aspect of extending configurations is the ability to link multiple files, up to a depth of 2.
# "Allowlist" arrays get appended and may have repeated elements.
# "useDefault" and "path" cannot be used simultaneously. Please choose one.
[extend]
# useDefault will extend the base configuration with the default config:
# https://raw.githubusercontent.com/Infisical/infisical/main/cli/config/infisical-scan.toml
useDefault = true
# or you can supply a path to a configuration. Path is relative to where infisical cli
# was invoked, not the location of the base config.
path = "common_config.toml"
# An array of tables that contain information that define instructions
# on how to detect secrets
[[rules]]
# Unique identifier for this rule
id = "some-identifier-for-rule"
# Short human readable description of the rule.
description = "awesome rule 1"
# Golang regular expression used to detect secrets. Note Golang's regex engine
# does not support lookaheads.
regex = '''one-go-style-regex-for-this-rule'''
# Golang regular expression used to match paths. This can be used as a standalone rule or it can be used
# in conjunction with a valid `regex` entry.
path = '''a-file-path-regex'''
# Array of strings used for metadata and reporting purposes.
tags = ["tag","another tag"]
# A regex match may have many groups, this allows you to specify the group that should be used as (which group the secret is contained in)
# its entropy checked if `entropy` is set.
secretGroup = 3
# Float representing the minimum shannon entropy a regex group must have to be considered a secret.
# Shannon entropy measures how random a data is. Since secrets are usually composed of many random characters, they typically have high entropy
entropy = 3.5
# Keywords are used for pre-regex check filtering.
# If rule has keywords but the text fragment being scanned doesn't have at least one of it's keywords, it will be skipped for processing further.
# Ideally these values should either be part of the identifier or unique strings specific to the rule's regex
# (introduced in v8.6.0)
keywords = [
"auth",
"password",
"token",
]
# You can include an allowlist table for a single rule to reduce false positives or ignore commits
# with known/rotated secrets
[rules.allowlist]
description = "ignore commit A"
commits = [ "commit-A", "commit-B"]
paths = [
'''go\.mod''',
'''go\.sum'''
]
# note: (rule) regexTarget defaults to check the _Secret_ in the finding.
# if regexTarget is not specified then _Secret_ will be used.
# Acceptable values for regexTarget are "match" and "line"
regexTarget = "match"
regexes = [
'''process''',
'''getenv''',
]
# note: stopwords targets the extracted secret, not the entire regex match
# if the extracted secret is found in the stopwords list, the finding will be skipped (i.e not included in report)
stopwords = [
'''client''',
'''endpoint''',
]
# This is a global allowlist which has a higher order of precedence than rule-specific allowlists.
# If a commit listed in the `commits` field below is encountered then that commit will be skipped and no
# secrets will be detected for said commit. The same logic applies for regexes and paths.
[allowlist]
description = "global allow list"
commits = [ "commit-A", "commit-B", "commit-C"]
paths = [
'''gitleaks\.toml''',
'''(.*?)(jpg|gif|doc)'''
]
# note: (global) regexTarget defaults to check the _Secret_ in the finding.
# if regexTarget is not specified then _Secret_ will be used.
# Acceptable values for regexTarget are "match" and "line"
regexTarget = "match"
regexes = [
'''219-09-9999''',
'''078-05-1120''',
'''(9[0-9]{2}|666)-\d{2}-\d{4}''',
]
# note: stopwords targets the extracted secret, not the entire regex match
# if the extracted secret is found in the stopwords list, the finding will be skipped (i.e not included in report)
stopwords = [
'''client''',
'''endpoint''',
]
```
#
#
# Ignoring Known Secrets
If you're intentionally committing a test secret that `infisical scan` might flag, you can instruct Infisical to overlook that secret with the methods listed below.
### infisical-scan:ignore
To ignore a secret contained in line of code, simply add `infisical-scan:ignore ` at the end of the line as comment in the given programming.
```js example.js
function helloWorld() {
console.log("8dyfuiRyq=vVc3RRr_edRk-fK__JItpZ"); // infisical-scan:ignore
}
```
### .infisicalignore
An alternative method to exclude specific findings involves creating a .infisicalignore file at your repository's root.
You can then add the fingerprints of the findings you wish to exclude. The Infisical scan report provides a unique Fingerprint for each secret found.
By incorporating these Fingerprints into the .infisicalignore file, Infisical will skip the corresponding secret findings in subsequent scans.
```.ignore .infisicalignore
bea0ff6e05a4de73a5db625d4ae181a015b50855:frontend/components/utilities/attemptLogin.js:stripe-access-token:147
bea0ff6e05a4de73a5db625d4ae181a015b50855:backend/src/json/integrations.json:generic-api-key:5
1961b92340e5d2613acae528b886c842427ce5d0:frontend/components/utilities/attemptLogin.js:stripe-access-token:148
```
# Quickstart
Manage secrets with Infisical CLI
The CLI is designed for a variety of secret management applications ranging from local development to CI/CD and production scenarios.
In the following steps, we explore how to use the Infisical CLI to fetch back environment variables from Infisical
and inject them into your local development process.
Start by running the `infisical login` command to authenticate with Infisical.
```bash
infisical login
```
If you are in a containerized environment such as WSL 2 or Codespaces, run `infisical login -i` to avoid browser based login
Next, navigate to your project and initialize Infisical.
```bash
# navigate to your project
cd /path/to/project
# initialize infisical
infisical init
```
The `infisical init` command creates a `.infisical.json` file, containing [local project settings](./project-config), at the location where the command is executed.
The `.infisical.json` file does not contain any sensitive data, so you may commit it to your git repository.
Finally, pass environment variables from Infisical into your application.
```bash
infisical run --env=dev --path=/apps/firefly -- [your application start command] # e.g. npm run dev
# example with node (nodemon)
infisical run --env=staging --path=/apps/spotify -- nodemon index.js
# example with flask
infisical run --env=prod --path=/apps/backend -- flask run
# example with spring boot - maven
infisical run --env=dev --path=/apps/ -- ./mvnw spring-boot:run --quiet
```
Custom aliases can utilize secrets from Infisical. Suppose there is a custom alias `yd` in `custom.sh` that runs `yarn dev` and needs the secrets provided by Infisical.
```bash
#!/bin/sh
yd() {
yarn dev
}
```
To make the secrets available from Infisical to `yd`, you can run the following command:
```bash
infisical run --env=prod --path=/apps/reddit --command="source custom.sh && yd"
```
View all available options for `run` command [here](./commands/run)
In the following steps, we explore how to use the Infisical CLI in a non-local development scenario
to fetch back environment variables and export them to a file.
Follow the steps listed [here](/documentation/platform/identities/universal-auth) to create a machine identity and obtain a **client ID** and **client secret** for it.
Run the following command to authenticate with Infisical using the **client ID** and **client secret** credentials from step 1 and set the `INFISICAL_TOKEN` environment variable to the retrieved access token.
```bash
export INFISICAL_TOKEN=$(infisical login --method=universal-auth --client-id= --client-secret= --silent --plain) # --plain flag will output only the token, so it can be fed to an environment variable. --silent will disable any update messages.
```
The CLI is configured to look out for the `INFISICAL_TOKEN` environment variable, so going forward any command used will be authenticated.
Alternatively, assuming you have an access token on hand, you can also pass it directly to the CLI using the `--token` flag in conjunction with other CLI commands.
Keep in mind that the machine identity access token has a limited lifetime. It is recommended to use it only for the duration of the task at hand.
You can [refresh the token](./commands/token) if needed.
Finally, export the environment variables from Infisical to a file of choice.
```bash
# export variables to a .env file (with export keyword)
infisical export --format=dotenv-export > .env
# export variables to a YAML file
infisical export --format=yaml > secrets.yaml
```
## History
Your terminal keeps a history with the commands you run. When you create Infisical secrets directly from your terminal, they'll stay there for a while.
For security and privacy concerns, we recommend you to configure your terminal to ignore those specific Infisical commands.
`$HOME/.profile` is pretty common but, you could place it under `$HOME/.profile.d/infisical.sh` or any profile file run at login
```bash
cat <> $HOME/.profile && source $HOME/.profile
# Ignoring specific Infisical CLI commands
DEFAULT_HISTIGNORE=$HISTIGNORE
export HISTIGNORE="*infisical secrets set*:$DEFAULT_HISTIGNORE"
EOF
```
If you're on WSL, then you can use the Unix/Linux method.
Here's some [documentation](https://superuser.com/a/1658331) about how to clear the terminal history, in PowerShell and CMD
## FAQ
Yes. The CLI is set to connect to Infisical Cloud by default, but if you're running your own instance of Infisical, you can direct the CLI to it using one of the methods provided below.
#### Method 1: Use the updated CLI
Beginning with CLI version V0.4.0, it is now possible to choose between logging in through the Infisical cloud or your own self-hosted instance. Simply execute the `infisical login` command and follow the on-screen instructions.
#### Method 2: Export environment variable
You can point the CLI to the self-hosted Infisical instance by exporting the environment variable `INFISICAL_API_URL` in your terminal.
```bash
# set backend host
export INFISICAL_API_URL="https://your-self-hosted-infisical.com/api"
# remove backend host
unset INFISICAL_API_URL
```
```bash
# set backend host
setx INFISICAL_API_URL "https://your-self-hosted-infisical.com/api"
# remove backend host
setx INFISICAL_API_URL ""
# NOTE: Once set or removed, please restart powershell for the change to take effect
```
#### Method 3: Set manually on every command
Another option to point the CLI to your self-hosted Infisical instance is to set it via a flag on every command you run.
```bash
# Example
infisical --domain="https://your-self-hosted-infisical.com/api"
```
To use Infisical for non local development scenarios, please create a service token. The service token will allow you to authenticate and interact with Infisical. Once you have created a service token with the required permissions, you’ll need to feed the token to the CLI.
```bash
infisical export --token=
infisical secrets --token=
infisical run --token= -- npm run dev
```
#### Pass via shell environment variable
The CLI is configured to look for an environment variable named `INFISICAL_TOKEN`. If set, it’ll attempt to use it for authentication.
```bash
export INFISICAL_TOKEN=
```
# Code of Conduct
What you should know before contributing to Infisical?
## Our Pledge
We as members, contributors, and leaders pledge to make participation in our
community a harassment-free experience for everyone, regardless of age, body
size, visible or invisible disability, ethnicity, sex characteristics, gender
identity and expression, level of experience, education, socio-economic status,
nationality, personal appearance, race, caste, color, religion, or sexual
identity and orientation.
We pledge to act and interact in ways that contribute to an open, welcoming,
diverse, inclusive, and healthy community.
## Our Standards
Examples of behavior that contributes to a positive environment for our
community include:
* Demonstrating empathy and kindness toward other people
* Being respectful of differing opinions, viewpoints, and experiences
* Giving and gracefully accepting constructive feedback
* Accepting responsibility and apologizing to those affected by our mistakes,
and learning from the experience
* Focusing on what is best not just for us as individuals, but for the overall
community
Examples of unacceptable behavior include:
* The use of sexualized language or imagery, and sexual attention or advances of
any kind
* Trolling, insulting or derogatory comments, and personal or political attacks
* Public or private harassment
* Publishing others' private information, such as a physical or email address,
without their explicit permission
* Other conduct which could reasonably be considered inappropriate in a
professional setting
## Enforcement Responsibilities
Community leaders are responsible for clarifying and enforcing our standards of
acceptable behavior and will take appropriate and fair corrective action in
response to any behavior that they deem inappropriate, threatening, offensive,
or harmful.
Community leaders have the right and responsibility to remove, edit, or reject
comments, commits, code, wiki edits, issues, and other contributions that are
not aligned to this Code of Conduct, and will communicate reasons for moderation
decisions when appropriate.
## Scope
This Code of Conduct applies within all community spaces, and also applies when
an individual is officially representing the community in public spaces.
Examples of representing our community include using an official e-mail address,
posting via an official social media account, or acting as an appointed
representative at an online or offline event.
## Enforcement
Instances of abusive, harassing, or otherwise unacceptable behavior may be
reported to the community leaders responsible for enforcement at
[team@infisical.com](mailto:team@infisical.com).
All complaints will be reviewed and investigated promptly and fairly.
All community leaders are obligated to respect the privacy and security of the
reporter of any incident.
## Enforcement Guidelines
Community leaders will follow these Community Impact Guidelines in determining
the consequences for any action they deem in violation of this Code of Conduct:
### 1. Correction
**Community Impact**: Use of inappropriate language or other behavior deemed
unprofessional or unwelcome in the community.
**Consequence**: A private, written warning from community leaders, providing
clarity around the nature of the violation and an explanation of why the
behavior was inappropriate. A public apology may be requested.
### 2. Warning
**Community Impact**: A violation through a single incident or series of
actions.
**Consequence**: A warning with consequences for continued behavior. No
interaction with the people involved, including unsolicited interaction with
those enforcing the Code of Conduct, for a specified period of time. This
includes avoiding interactions in community spaces as well as external channels
like social media. Violating these terms may lead to a temporary or permanent
ban.
### 3. Temporary Ban
**Community Impact**: A serious violation of community standards, including
sustained inappropriate behavior.
**Consequence**: A temporary ban from any sort of interaction or public
communication with the community for a specified period of time. No public or
private interaction with the people involved, including unsolicited interaction
with those enforcing the Code of Conduct, is allowed during this period.
Violating these terms may lead to a permanent ban.
### 4. Permanent Ban
**Community Impact**: Demonstrating a pattern of violation of community
standards, including sustained inappropriate behavior, harassment of an
individual, or aggression toward or disparagement of classes of individuals.
**Consequence**: A permanent ban from any sort of public interaction within the
community.
## Attribution
This Code of Conduct is adapted from the [Contributor Covenant][homepage],
version 2.1, available at
[https://www.contributor-covenant.org/version/2/1/code\_of\_conduct.html][v2.1].
Community Impact Guidelines were inspired by
[Mozilla's code of conduct enforcement ladder][mozilla coc].
For answers to common questions about this code of conduct, see the FAQ at
[https://www.contributor-covenant.org/faq][faq]. Translations are available at
[https://www.contributor-covenant.org/translations][translations].
[homepage]: https://www.contributor-covenant.org
[v2.1]: https://www.contributor-covenant.org/version/2/1/code_of_conduct.html
[mozilla coc]: https://github.com/mozilla/diversity
[faq]: https://www.contributor-covenant.org/faq
[translations]: https://www.contributor-covenant.org/translations
# FAQ
Frequently Asked Questions about contributing to Infisical
Frequently asked questions about contributing to Infisical can be found on this page.
If you can't find the answer you are looking for, please create an issue on our GitHub repository or join our Slack channel for additional support.
The Alpine Linux CDN may be unavailable/down in your region infrequently (eg. there is an unplanned outage). One possible fix is to add a retry mechanism and a fallback mirrors array to the Dockerfile. You can also use this as an opportunity to pin the Alpine Linux version for Docker to use in case there are issues with the latest version. Ensure to use https for the mirrors.
#### Make the following changes to the backend Dockerfile
```bash
# Pin Alpine version from list: https://dl-cdn.alpinelinux.org/alpine/
ARG ALPINE_VERSION=3.17
ARG ALPINE_APPEND=v3.17/main
# Specify number of retries for each mirror
ARG MAX_RETRIES=3
# Define base Alpine mirror URLs in attempt order from list: https://dl-cdn.alpinelinux.org/alpine/MIRRORS.txt
ARG BASE_ALPINE_MIRRORS="https://dl-cdn.alpinelinux.org/alpine https://ftp.halifax.rwth-aachen.de/alpine https://uk.alpinelinux.org/alpine"
# Build stage
# Add the Alpine version arg
FROM node:16-alpine$ALPINE_VERSION AS build
WORKDIR /app
COPY package*.json ./
RUN npm ci --only-production
COPY . .
RUN npm run build
# Production stage
# Add the Alpine version arg
FROM node:16-alpine$ALPINE_VERSION
WORKDIR /app
ENV npm_config_cache /home/node/.npm
COPY package*.json ./
RUN npm ci --only-production
COPY --from=build /app .
# Add retry mechanism and loop through the specified mirrors
RUN retries_left=$MAX_RETRIES; \
for mirror in $ALPINE_MIRRORS; do \
full_mirror="$mirror/$ALPINE_APPEND"; \
echo "Trying mirror: $full_mirror"; \
echo >>/etc/apk/repositories "$full_mirror"; \
for i in $(seq $retries_left); do \
echo "Retrying... Attempt $i (Retries Left: $((retries_left - i)))"; \
if apk add --no-cache bash curl git && \
curl -1sLf 'https://dl.cloudsmith.io/public/infisical/infisical-cli/setup.alpine.sh' | bash && \
apk add --no-cache infisical=0.8.1; then \
break; \
fi; \
sleep 10; \
done; \
if [ $? -eq 0 ]; then \
break; \
fi; \
done
HEALTHCHECK --interval=10s --timeout=3s --start-period=10s \
CMD node healthcheck.js
EXPOSE 4000
CMD ["npm", "run", "start"]
```
[Alpine Linux (mirrors) - official site](https://dl-cdn.alpinelinux.org/alpine/MIRRORS.txt)
[Alpine Linux (mirrors) - archived site](https://web.archive.org/web/20230914123159/https://dl-cdn.alpinelinux.org/alpine/MIRRORS.txt)
[Alpine Linux (versions) - official site](https://dl-cdn.alpinelinux.org/alpine/)
[Alpine Linux (versions) - archived site](https://web.archive.org/web/20230914123455/https://dl-cdn.alpinelinux.org/alpine/)
# Overview
Contributing to the Infisical ecosystem.
To set a strong foundation, this section outlines how we, the community and members of Infisical,
should approach the development and contribution process.
## Code-bases
Infisical has two major code-bases. One for the platform code, and one for SDKs. The contribution process has some key differences between the two, so we've split the documentation into two sections:
* The [Infisical Platform](https://github.com/Infisical/infisical), the Infisical platform itself.
* The [Infisical SDK](https://github.com/Infisical/sdk), the official Infisical client SDKs.
The Infisical platform is the core of the Infisical ecosystem.
The SDKs are the official Infisical client libraries, used by developers to easily interact with the Infisical platform.
## Community
We are building an inclusive community, and this means adhering to the [Code of Conduct](/contributing/getting-started/code-of-conduct).
## Bugs and issues
Bug reports help make Infisical a better experience for everyone. When you report a bug, a template will be created automatically containing information we'd like to know.
Before raising a new issue, please search existing ones to make sure you're not creating a duplicate.
If the issue is related to security, please email us directly at
[team@infisical.com](mailto:team@infisical.com).
## Deciding what to work on
You can start by browsing through our list of issues or adding your own that improves on the platform experience. Once you've decided on an issue, leave a comment and wait to get approved; this helps avoid multiple people working on the same issue.
If you're ever in doubt about whether or not a proposed feature aligns with Infisical as a whole, feel free to raise an issue about it and we'll get back to you promptly.
## Writing and submitting code
Anyone can contribute code to Infisical. To get started, check out the local development guides for each language.
* Local development guide for Platform is [here](/contributing/platform/developing).
* Local development guide for SDK is [here](/contributing/sdk/developing).
## Licensing
Most of Infisical's code is under the MIT license, though some paid feature restrictions are covered by a proprietary license.
Any third party components incorporated into our code are licensed under the original license provided by the applicable component owner.
# Pull requests
This guide walks through the code submission process for Infisical.
## Making a pull request
Once you are done making changes in local development, you can submit a pull request (PR) to the main repository branch.
We require a few considerations on your part to ensure that PRs are easy to review and up to standard with the code.
### Title and content
Start by providing a concise title addressing what your PR achieves such as "add pagination to retrieve environment variables for GitLab integration."
You should follow the automatically-generated PR template to fill in the PR description. This includes a more detailed description of the changes in the PR, the type of PR, and an acknowledgement that you've read and agreed to the contributing guidelines.
### Feature PRs
Give a functional overview of how your feature works, including how the user can use the feature. Then share any technical details in an overview of how the PR works.
As of `06-01-2023`, all PRs created after this date are required to attach a video of you performing the described functionality.
### Bug Fix PRs
Give an overview of the bug at hand and how your PR fixes the it at both a high and low level.
Feel free to add a short video or screenshots of what your PR achieves.
## Getting your PR reviewed
Once your PR is reviewed, one or two relevant members of the Infisical team should review and approve the PR before it is merged. You should coordinate and ping the team member closest to the submitted functionality via our [Slack](https://infisical.com/slack) to review your PR.
* Vlad: Frontend, Web UI
* Tony: Backend, SDKs, Security
* Maidul: Backend, CI/CD, CLI, Kubernetes Operator
* Daniel: Frontend, UI/UX, Backend, SDKs
The team member(s) will start by enabling baseline checks to ensure that there are no leaked secrets, new dependencies are clear, and the frontend/backend services start up. Afterward, they will review your PR thoroughly by testing the code and leave any feedback or work in with you to revise the PR up to standard.
Once everything is good, the team member(s) will approve the PR to be merged into the `main` branch; all changes will be tested in CI/CD and our staging environment first before being deployed to production.
Due to the high volume of issues, PRs, etc. from the community, and limited bandwidth on our end to address everything instantly, we prefer
reviewing PRs once they are fully complete and well-tested.
In the past, we've often had to review low-quality PRs up to 10 times which severely restricts our capacity to address other issues, PRs, and initiatives in the pipeline. As such, we ask of the community to submit higher-quality PRs that, for example, don't break existing code; in return we'll prioritize the first 3 reviews for PRs.
# Backend folder structure
```
├── scripts
├── e2e-test
└── src/
├── @types/
│ ├── knex.d.ts
│ └── fastify.d.ts
├── db/
│ ├── migrations
│ ├── schemas
│ └── seed
├── lib/
│ ├── fn
│ ├── date
│ └── config
├── queue
├── server/
│ ├── routes/
│ │ ├── v1
│ │ └── v2
│ ├── plugins
│ └── config
├── services/
│ ├── auth
│ ├── org
│ └── project/
│ ├── project-service.ts
│ ├── project-types.ts
│ └── project-dal.ts
└── ee/
├── routes
└── services
```
### `backend/scripts`
Contains reusable scripts for backend automation, like running migrations and generating SQL schemas.
### `backend/e2e-test`
Integration tests for the APIs.
### `backend/src`
The source code of the backend.
* `@types`: Type definitions for libraries like Fastify and Knex.
* `db`: Knex.js configuration for the database, including migration, seed files, and SQL type schemas.
* `lib`: Stateless, reusable functions used across the codebase.
* `queue`: Infisical's queue system based on BullMQ.
### `src/server`
* Scope anything related to Fastify/service here.
* Includes routes, Fastify plugins, and server configurations.
* The routes folder contains various versions of routes separated into v1, v2, etc.
### `src/services`
* Handles the core business logic for all operations.
* Follows the co-location principle: related components should be kept together.
* Each service component typically contains:
1. **dal**: Database Access Layer functions for database operations
2. **service**: The service layer containing business logic.
3. **type**: Type definitions used within the service component.
4. **fns**: An optional component for sharing reusable functions related to the service.
5. **queue**: An optional component for queue-specific logic, like `secret-queue.ts`.
### `src/ee`
Follows the same pattern as above, with the exception of a license change from MIT to Infisical Proprietary License.
### Guidelines and Best Practices
* All services are interconnected at `/src/server/routes/index.ts`, following the principle of simple dependency injection.
* Files should be named in dash-case.
* Avoid using classes in the codebase; opt for simple functions instead.
* All committed code must be properly linted using `npm run lint:fix` and type-checked with `npm run type:check`.
* Minimize shared logic between services as much as possible.
* Controllers within a router component should ideally call only one service layer, with exceptions for services like `audit-log` that require access to request object data.
# Backend development guide
Suppose you're interested in implementing a new feature in Infisical's backend, let's call it "feature-x." Here are the general steps you should follow.
## Database schema migration
In order to run [schema migrations](https://en.wikipedia.org/wiki/Schema_migration#:~:text=A%20schema%20migration%20is%20performed,some%20newer%20or%20older%20version) you need to expose your database connection string. Create a `.env.migration` file to set the database connection URI for migration scripts, or alternatively, export the `DB_CONNECTION_URI` environment variable.
## Creating new database model
If your feature involves a change in the database, you need to first address this by generating the necessary database schemas.
1. If you're adding a new table, update the `TableName` enum in `/src/db/schemas/models.ts` to include the new table name.
2. Create a new migration file by running `npm run migration:new` and give it a relevant name, such as `feature-x`.
3. Navigate to `/src/db/migrations/_.ts`.
4. Modify both the `up` and `down` functions to create or alter Postgres fields on migration up and to revert these changes on migration down, ensuring idempotency as outlined [here](https://github.com/graphile/migrate/blob/main/docs/idempotent-examples.md).
### Generating TS Schemas
While typically you would need to manually write TS types for Knex type-sense, we have automated this process:
1. Start the server.
2. Run `npm run migration:latest` to apply all database changes.
3. Execute `npm run generate:schema` to automatically generate types and schemas using [zod](https://github.com/colinhacks/zod) in the `/src/db/schemas` folder.
4. Update the barrel export in `schema/index` and include the new tables in `/src/@types/knex.d.ts` to enable type-sensing in Knex.js.
## Business Logic
Once the database changes are in place, it's time to create the APIs for `feature-x`:
1. Execute `npm run generate:component`.
2. Choose option 1 for the service component.
3. Name the service in dash-case, like `feature-x`. This will create a `feature-x` folder in `/src/services` containing three files.
1. `feature-x-dal`: The Database Access Layer functions.
2. `feature-x-service`: The service layer where all the business logic is handled.
3. `feature-x-type`: The types used by `feature-x`.
For reusable shared functions, set up a file named `feature-x-fns`.
Use the custom Infisical function `ormify` in `src/lib/knex` for simple database operations within the DAL.
## Connecting the Service Layer to the Server Layer
Server-related logic is handled in `/src/server`. To connect the service layer to the server layer, we use Fastify plugins for dependency injection:
1. Add the service type in the `fastify.d.ts` file under the `service` namespace of a FastifyServerInstance type.
2. In `/src/server/routes/index.ts`, instantiate the required dependencies for `feature-x`, such as the DAL and service layers, and then pass them to `fastify.register("service,{...dependencies})`.
3. This makes the service layer accessible within all routes under the Fastify service instance, accessed via `server.services..`.
## Writing API Routes
1. To create a route component, run `npm run generate:component`.
2. Select option 3, type the router name in dash-case, and provide the version number. This will generate a router file in `src/server/routes/v/`
1. Implement your logic to connect with the service layer as needed.
2. Import the router component in the version folder's index.ts. For instance, if it's in v1, import it in `v1/index.ts`.
3. Finally, register it under the appropriate prefix for access.
# Local development
This guide will help you set up and run the Infisical platform in local development.
## Fork and clone the repo
[Fork](https://docs.github.com/en/get-started/quickstart/fork-a-repo) the [repository](https://github.com/Infisical/infisical) to your own GitHub account and then [clone](https://docs.github.com/en/repositories/creating-and-managing-repositories/cloning-a-repository) it to your local device.
Once, you've done that, create a new branch:
```console
git checkout -b MY_BRANCH_NAME
```
## Set up environment variables
Start by creating a .env file at the root of the Infisical directory then copy the contents of the file linked [here](https://github.com/Infisical/infisical/blob/main/.env.example). View all available [environment variables](https://infisical.com/docs/self-hosting/configuration/envars) and guidance for each.
## Starting Infisical for development
We use Docker to spin up all required services for Infisical in local development. If you are unfamiliar with Docker, don’t worry, all you have to do is install Docker for your
machine and run the command below to start up the development server.
#### Start local server
```bash
docker compose -f docker-compose.dev.yml up --build --force-recreate
```
#### Access local server
Once all the services have spun up, browse to [http://localhost:8080](http://localhost:8080).
#### Shutdown local server
```bash
# To stop environment use Control+C (on Mac) CTRL+C (on Win) or
docker compose -f docker-compose.dev.yml down
```
## Starting Infisical docs locally
We use [Mintlify](https://mintlify.com/) for our docs.
#### Install Mintlify CLI.
```bash
npm i -g mintlify
```
or
```bash
yarn global add mintlify
```
#### Running the docs
Go to `docs` directory and run `mintlify dev`. This will start up the docs on `localhost:3000`
```bash
# From the root directory
cd docs; mintlify dev;
```
# Local development
This guide will help you contribute to the Infisical SDK.
## Fork and clone the repo
[Fork](https://docs.github.com/en/get-started/quickstart/fork-a-repo) the [repository](https://github.com/Infisical/sdk) to your own GitHub account and then [clone](https://docs.github.com/en/repositories/creating-and-managing-repositories/cloning-a-repository) it to your local device.
Once, you've done that, create a new branch:
```console
git checkout -b MY_BRANCH_NAME
```
## Set up environment variables
Start by creating a .env file at the root of the Infisical directory then copy the contents of the file below into the .env file.
```env
# This is required for running tests locally.
# Rename this file to ".env" and fill in the values below.
# Please make sure that the machine identity has access to the project you are testing in.
# https://infisical.com/docs/documentation/platform/identities/universal-auth
INFISICAL_UNIVERSAL_CLIENT_ID=MACHINE_IDENTITY_CLIENT_ID
INFISICAL_UNIVERSAL_CLIENT_SECRET=MACHINE_IDENTITY_CLIENT_SECRET
# The ID of the Infisical project where we will create the test secrets.
# NOTE: The project must have a dev environment. (This is created by default when you create a project.)
INFISICAL_PROJECT_ID=INFISICAL_TEST_PROJECT_ID
# The Infisical site URL. If you are testing with a local Infisical instance, then this should be set to "http://localhost:8080".
INFISICAL_SITE_URL=https://app.infisical.com
```
The above values are required for running tests locally. Before opening a pull request, make sure to run `cargo test` to ensure that all tests pass.
## Guidelines
### Predictable and consistent
When adding new functionality (such as new functions), it's very important that the functionality is added to *all* the SDK's. This is to ensure that the SDK's are predictable and consistent across all languages. If you are adding new functionality, please make sure to add it to all the SDK's.
### Handling errors
Error handling is very important when writing SDK's. We want to make sure that the SDK's are easy to use, and that the user gets a good understanding of what went wrong when something fails. When adding new functionality, please make sure to add proper error handling. [Read more about error handling here](#error-handling).
### Tests
If you add new functionality or modify existing functionality, please write tests thats properly cover the new functionality. You can run tests locally by running `cargo test` from the root directory. You must always run tests before opening a pull request.
### Code style
Please follow the default rust styling guide when writing code for the base SDK. [Read more about rust code style here](https://doc.rust-lang.org/nightly/style-guide/#the-default-rust-style).
## Prerequisites for contributing
### Understanding the terms
In the guide we use some terms that might be unfamiliar to you. Here's a quick explanation of the terms we use:
* **Base SDK**: The base SDK is the SDK that all other SDK's are built on top of. The base SDK is written in Rust, and is responsible for executing commands and parsing the input and output to and from JSON.
* **Commands**: Commands are what's being sent from the target language to the command handler. The command handler uses the command to execute the corresponding function in the base SDK. Commands are in reality just a JSON string that tells the command handler what function to execute, and what input to use.
* **Command handler**: The command handler is the part of the base SDK that takes care of executing commands. It also takes care of parsing the input and output to and from JSON.
* **Target language**: The target language refers to the actual SDK code. For example, the [Node.js SDK](https://www.npmjs.com/package/@infisical/sdk) is a "target language", and so is the [Python SDK](https://pypi.org/project/infisical-python/).
### Understanding the execution flow
After the target language SDK is initiated, it uses language-specific bindings to interact with the base SDK.
These bindings are instantiated, setting up the interface for command execution. A client within the command handler is created, which issues commands to the base SDK.
When a command is executed, it is first validated. If valid, the command handler locates the corresponding command to perform. If the command executes successfully, the command handler returns the output to the target language SDK, where it is parsed and returned to the user.
If the command handler fails to validate the input, an error will be returned to the target language SDK.
### Rust knowledge
Contributing to the SDK requires intermediate to advanced knowledge of Rust concepts such as lifetimes, traits, generics, and async/await *(futures)*, and more.
### Rust setup
The base SDK is written in rust. Therefore you must have rustc and cargo installed. You can install rustc and cargo by following the instructions [here](https://www.rust-lang.org/tools/install).
You shouldn't have to use the rust cross compilation toolchain, as all compilation is done through a collection of Github Actions. However. If you need to test cross compilation, please do so with Github Actions.
### Tests
If you add new functionality or modify existing functionality, please write tests thats properly cover the new functionality. You can run tests locally by running `cargo test` from the root directory.
### Language-specific crates
The language-specific crates should ideally never have to be modified, as they are simply a wrapper for the `infisical-json` crate, which executes "commands" from the base SDK. If you need to create a new target-language specific crate, please try to create native bindings for the target language. Some languages don't have direct support for native bindings (Java as an example). In those cases we can use the C bindings (`crates/infisical-c`) in the target language.
## Generate types
Having almost seemless type safety from the base SDK to the target language is critical, as writing types for each language has a lot of drawbacks such as duplicated code, and lots of overhead trying to keep the types up-to-date and in sync across a large collection of languages. Therefore we decided to use [QuickType](https://quicktype.io/) and [Serde](https://serde.rs/) to help us generate types for each language. In our Rust base SDK (`crates/infisical`), we define all the inputs/outputs.
If you are interested in reading about QuickType works under the hood, you can [read more here](http://blog.quicktype.io/under-the-hood/).
This is an example of a type defined in Rust (both input and output). For this to become a generated type, you'll need to add it to our schema generator. More on that further down.
```rust
use schemars::JsonSchema;
use serde::{Deserialize, Serialize};
#[derive(Serialize, Deserialize, Debug, JsonSchema)]
#[serde(rename_all = "camelCase")]
// Input:
pub struct CreateSecretOptions {
pub environment: String, // environment
pub secret_comment: Option, // secretComment
pub path: Option, // secretPath
pub secret_value: String, // secretValue
pub skip_multiline_encoding: Option, // skipMultilineEncoding
pub r#type: Option, // shared / personal
pub project_id: String, // workspaceId
pub secret_name: String, // secretName (PASSED AS PARAMETER IN REQUEST)
}
// Output:
#[derive(Serialize, Deserialize, Debug, JsonSchema)]
#[serde(rename_all = "camelCase")]
pub struct CreateSecretResponse {
pub secret: Secret, // "Secret" is defined elsewhere.
}
```
### Adding input types to the schema generator
You will *only* have to define outputs in our schema generator, then QuickType will take care of the rest behind the scenes. You can find the Rust crate that takes care of type generation here: `crates/sdk-schemas/src/main.rs`.
Simply add the output *(also called response)*, to the `write_schema_for_response!` macro. This will let QuickType know that it should generate types for the given structs. The main function will look something like this:
```rust
fn main() -> Result<()> {
// Input types for new Client
write_schema_for!(infisical_json::client::ClientSettings);
// Input types for Client::run_command
write_schema_for!(infisical_json::command::Command);
// Output types for Client::run_command
// Only add structs which are direct results of SDK commands.
write_schema_for_response! {
infisical::manager::secrets::GetSecretResponse,
infisical::manager::secrets::ListSecretsResponse,
infisical::manager::secrets::UpdateSecretResponse,
infisical::manager::secrets::DeleteSecretResponse,
infisical::manager::secrets::CreateSecretResponse, // <-- This is the output from the above example!
infisical::auth::AccessTokenSuccessResponse
};
Ok(())
}
```
### Generating the types for the target language
Once you've added the output to the schema generator, you can generate the types for the target language by running the following command from the root directory:
```console
$ npm install
$ npm run schemas
```
If you change any of the structs defined in the base SDK, you will need to run this script to re-generate the types.
This command will run the `schemas.ts` file found in the `support/scripts` folder. If you are adding a new language, it's important that you add the language to the code.
This is an example of how how we generate types for Node.js:
```ts
const ts = await quicktype({
inputData,
lang: "typescript",
rendererOptions: {}
});
await ensureDir("./languages/node/src/infisical_client");
writeToFile("./languages/node/src/infisical_client/schemas.ts", ts.lines);
```
## Building bindings
We've tried to streamline the building process as much as possible. So you shouldn't have to worry much about building bindings, as it should just be a few commands.
### Node.js
Building bindings for Node.js is very straight foward. The command below will generate NAPI bindings for Node.js, and move the bindings to the correct folder. We use [NAPI-RS](https://napi.rs/) to generate the bindings.
```console
$ cd languages/node
$ npm run build
```
### Python
To generate and use python bindings you will need to run the following commands.
The Python SDK is located inside the crates folder. This is a limitation of the maturin tool, forcing us to structure the project in this way.
```console
$ pip install -U pip maturin
$ cd crates/infisical-py
$ python3 -m venv .venv
$ source .venv/bin/activate
$ maturin develop
```
After running the commands above, it's very important that you rename the generated .so file to `infisical_py.so`. After renaming it you also need to move it into the root of the `crates/infisical-py` folder.
### Java
Java uses the C bindings to interact with the base SDK. To build and use the C bindings in Java, please follow the instructions below.
```console
$ cd crates/infisical-c
$ cargo build --release
$ cd ../../languages/java
```
After generating the C bindings, the generated .so or .dll has been created in the `/target` directory at the root of the project.
You have to manually move the generated file into the `languages/java/src/main/resources` directory.
## Error handling
### Error handling in the base SDK
The base SDK should never panic. If an error occurs, we should return a `Result` with an error message. We have a custom Result type defined in the `error.rs` file in the base SDK.
All our errors are defined in an enum called `Error`. The `Error` enum is defined in the `error.rs` file in the base SDK. The `Error` enum is used in the `Result` type, which is used as the return type for all functions in the base SDK.
```rust
#[derive(Debug, Error)]
pub enum Error {
// Secret not found
#[error("Secret with name '{}' not found.", .secret_name)]
SecretNotFound { secret_name: String },
// .. other errors
// Errors that are not specific to the base SDK.
#[error(transparent)]
Reqwest(#[from] reqwest::Error),
#[error(transparent)]
Serde(#[from] serde_json::Error),
#[error(transparent)]
Io(#[from] std::io::Error),
}
```
### Returning an error
You can find many examples of how we return errors in the SDK code. A relevant example is for creating secrets, which can be found in `crates/infisical/src/api/secrets/create_secret.rs`. When the error happened due to a request error to our API, we have an API error handler. This prevents duplicate code and keeps error handling consistent across the SDK. You can find the api error handler in the `error.rs` file.
### Error handling in the target language SDK's.
All data sent to the target language SDK has the same format. The format is an object with 3 fields: `success (boolean)`, `data (could be anything or nothing)`, and `errorMessage (string or null)`.
The `success` field is used to determine if the request was successful or not. The `data` field is used to return data from the SDK. The `errorMessage` field is used to return an error message if the request was not successful.
This means that if the success if false or if the error message is not null, something went wrong and we should throw an error on the target-language level, with the error message.
## Command handler
### What is the command handler
The command handler (the `infisical-json` crate), takes care of executing commands sent from the target language. It also takes care of parsing the input and output to and from JSON. The command handler is the only part of the base SDK that should be aware of JSON. The rest of the base SDK should be completely unaware of JSON, and only work with the Rust structs defined in the base SDK.
The command handler exposes a function called `run_command`, which is what we use in the target language to execute commands. The function takes a json string as input, and returns a json string as output. We use helper functions generated by QuickType to convert the input and output to and from JSON.
### Creating new SDK methods
Creating new commands is necessary when adding new methods to the SDK's. Defining a new command is a 3-step process in most cases.
#### 1. Define the input and output structs
Earlier in this guide, we defined the input and output structs for the `CreateSecret` command. We will use that as an example here as well.
#### 2. Creating the method in the base SDK
The first step is to create the method in the base SDK. This step will be different depending on what method you are adding. In this example we're going to assume you're adding a function for creating a new secret.
After you created the function for creating the secret, you'll need need to add it to the ClientSecrets implementation. We do it this way to keep the code organized and easy to read. The ClientSecrets struct is located in the `crates/infisical/src/manager/secrets.rs` file.
```rust
pub struct ClientSecrets<'a> {
pub(crate) client: &'a mut crate::Client,
}
impl<'a> ClientSecrets<'a> {
pub async fn create(&mut self, input: &CreateSecretOptions) -> Result {
create_secret(self.client, input).await // <-- This is the function you created!
}
}
impl<'a> Client {
pub fn secrets(&'a mut self) -> ClientSecrets<'a> {
ClientSecrets { client: self }
}
}
```
#### 3. Define a new command
We define new commands in the `crates/infisical-json/src/command.rs` file. The `Command` enum is what we use to define new commands.
In the codesnippet below we define a new command called `CreateSecret`. The `CreateSecret` command takes a `CreateSecretOptions` struct as input. We don't have to define the output, because QuickType's converter helps us with figuring out the return type for each command.
````rust
```rust
use schemars::JsonSchema;
use serde::{Deserialize, Serialize};
#[derive(Serialize, Deserialize, JsonSchema, Debug)]
#[serde(rename_all = "camelCase", deny_unknown_fields)]
pub enum Command {
GetSecret(GetSecretOptions),
ListSecrets(ListSecretsOptions),
CreateSecret(CreateSecretOptions), // <-- The new command!
UpdateSecret(UpdateSecretOptions),
DeleteSecret(DeleteSecretOptions),
}
````
#### 4. Add the command to the command handler
After defining the command, we need to add it to the command handler itself. This takes place in the `crates/infisical-json/src/client.rs` file. The `run_command` function is what we use to execute commands.
In the Client implementation we try to parse the JSON string into a `Command` enum. If the parsing is successful, we match the command and execute the corresponding function.
```rust
match cmd {
Command::GetSecret(req) => self.0.secrets().get(&req).await.into_string(),
Command::ListSecrets(req) => self.0.secrets().list(&req).await.into_string(),
Command::UpdateSecret(req) => self.0.secrets().update(&req).await.into_string(),
Command::DeleteSecret(req) => self.0.secrets().delete(&req).await.into_string(),
// This is the new command:
Command::CreateSecret(req) => self.0.secrets().create(&req).await.into_string(),
}
```
#### 5. Implementing the new command in the target language SDK's
We did it! We've now added a new command to the base SDK. The last step is to implement the new command in the target language SDK's. The process is a little different from language to language, but in this example we're going to assume that we're adding a new command to the Node.js SDK.
First you'll need to generate the new type schemas, we added a new command, input struct, and output struct. [Read more about generating types here](#generating-the-types-for-the-target-language).
Secondly you need to build the new node bindings so we can use the new functionality in the Node.js SDK. You can do this by running the following command from the `languages/node` directory:
```console
$ npm install
$ npm run build
```
The build command will execute a build script in the `infisical-napi` crate, and move the generated bindings to the appropriate folder.
After building the new bindings, you can access the new functionality in the Node.js SDK source.
```ts
// 'binding' is a js file that makes it easier to access the methods in the bindings. (it's auto generated when running npm run build)
import * as rust from "../../binding";
// We can import the newly generated types from the schemas.ts file. (Generated with QuickType!)
import type { CreateSecretOptions, CreateSecretResponse } from "./schemas";
// This is the QuickType converter that we use to create commands with! It takes care of all JSON parsing and serialization.
import { Convert, ClientSettings } from "./schemas";
export class InfisicalClient {
#client: rust.Client;
constructor(settings: ClientSettings) {
const settingsJson = settings == null ? null : Convert.clientSettingsToJson(settings);
this.#client = new rust.InfisicalClient(settingsJson);
}
// ... getSecret
// ... listSecrets
// ... updateSecret
// ... deleteSecret
async createSecret(options: CreateSecretOptions): Promise {
// The runCommand will return a JSON string, which we can parse into a CreateSecretResponse.
const command = await this.#client.runCommand(
Convert.commandToJson({
createSecret: options
})
);
const response = Convert.toResponseForCreateSecretResponse(command); // <-- This is the QuickType converter in action!
// If the response is not successful or the data is null, we throw an error.
if (!response.success || response.data == null) {
throw new Error(response.errorMessage ?? "Something went wrong");
}
// To make it easier to work with the response, we return the secret directly.
return response.data.secret;
}
}
```
And that's it! We've now added a new command to the base SDK, and implemented it in the Node.js SDK. The process is very similar for all other languages, but the code will look a little different.
## Conclusion
The SDK has a lot of moving parts, and it can be a little overwhelming at first. But once you get the hang of it, it's actually quite simple. If you have any questions, feel free to reach out to us on [Slack](https://infisical.com/slack), or [open an issue](https://github.com/Infisical/sdk/issues) on GitHub.
# What is Infisical?
An Introduction to the Infisical secret management platform.
**[Infisical](https://infisical.com)** is the open source secret management platform that developers use to centralize their application configuration and secrets like API keys and database credentials as well as manage their internal PKI. Additionally, developers use Infisical to prevent secrets leaks to git and securely share secrets amongst engineers.
Start managing secrets securely with [Infisical Cloud](https://app.infisical.com) or learn how to [host Infisical](/self-hosting/overview) yourself.
Get started with Infisical Cloud in just a few minutes.
Self-host Infisical on your own infrastructure.
## Why Infisical?
Infisical helps developers achieve secure centralized secret management and provides all the tools to easily manage secrets in various environments and infrastructure components. In particular, here are some of the most common points that developers mention after adopting Infisical:
* Streamlined **local development** processes (switching .env files to [Infisical CLI](/cli/commands/run) and removing secrets from developer machines).
* **Best-in-class developer experience** with an easy-to-use [Web Dashboard](/documentation/platform/project).
* Simple secret management inside **[CI/CD pipelines](/integrations/cicd/githubactions)** and staging environments.
* Secure and compliant secret management practices in **[production environments](/sdks/overview)**.
* **Facilitated workflows** around [secret change management](/documentation/platform/pr-workflows), [access requests](/documentation/platform/access-controls/access-requests), [temporary access provisioning](/documentation/platform/access-controls/temporary-access), and more.
* **Improved security posture** thanks to [secret scanning](/cli/scanning-overview), [granular access control policies](/documentation/platform/access-controls/overview), [automated secret rotation](https://infisical.com/docs/documentation/platform/secret-rotation/overview), and [dynamic secrets](/documentation/platform/dynamic-secrets/overview) capabilities.
## How does Infisical work?
To make secret management effortless and secure, Infisical follows a certain structure for enabling secret management workflows as defined below.
**Identities** in Infisical are users or machine which have a certain set of roles and permissions assigned to them. Such identities are able to manage secrets in various **Clients** throughout the entire infrastructure. To do that, identities have to verify themselves through one of the available **Authentication Methods**.
As a result, the 3 main concepts that are important to understand are:
* **[Identities](/documentation/platform/identities/overview)**: users or machines with a set permissions assigned to them.
* **[Clients](/integrations/platforms/kubernetes)**: Infisical-developed tools for managing secrets in various infrastructure components (e.g., [Kubernetes Operator](/integrations/platforms/kubernetes), [Infisical Agent](/integrations/platforms/infisical-agent), [CLI](/cli/usage), [SDKs](/sdks/overview), [API](/api-reference/overview/introduction), [Web Dashboard](/documentation/platform/organization)).
* **[Authentication Methods](/documentation/platform/identities/universal-auth)**: ways for Identities to authenticate inside different clients (e.g., SAML SSO for Web Dashboard, Universal Auth for Infisical Agent, AWS Auth etc.).
## How to get started with Infisical?
Depending on your use case, it might be helpful to look into some of the resources and guides provided below.
Inject secrets into any application process/environment.
Fetch secrets with any programming language on demand.
Inject secrets into Docker containers.
Fetch and save secrets as native Kubernetes secrets.
Fetch secrets via HTTP request.
Explore integrations for GitHub, Vercel, AWS, and more.
# Introduction
Whether you're running a Node application on Heroku, Next.js application with Vercel, or Kubernetes on AWS, Infisical has a secret management strategy from local development to production ready for you.
## Guides by Language
Manage secrets across your Node stack
Manage secrets across your Python stack
## Guides by Stack
Manage secrets for your Next.js + Vercel stack
Want a guide? [Throw in a request](https://github.com/Infisical/infisical/issues).
# Secret Management in Development Environments
Learn how to manage secrets in local development environments.
## Problem at hand
There is a number of issues that arise with secret management in local development environment:
1. **Getting secrets onto local machines**. When new developers join or a new project is created, the process of getting the development set of secrets onto local machines is often unclear. As a result, developers end up spending a lot of time onboarding and risk potentially following insecure practices when sharing secrets from one developer to another.
2. **Syncing secrets with teammates**. One of the problems with .env files is that they become unsynced when one of the developers updates a secret or configuration. Even if the rest of the team is notified, developers don't make all the right changes immediately, and later on end up spending a lot of time debugging an issue due to missing environment variables. This leads to a lot of inefficiencies and lost time.
3. **Accidentally leaking secrets**. When developing locally, it's common for developers to accidentally leak a hardcoded secret as part of a commit. As soon as the secret is part of the git history, it becomes hard to get it removed and create a security vulnerability.
## Solution
One of the main benefits of Infisical is the facilitation of secret management workflows in local development use cases. In particular, Infisical heavily follows the "Security Shift Left" principle to enable developers to effortlessly follow secure practices when coding.
### CLI
[Infisical CLI](/cli/overview) is the most frequently used Infisical tool for secret management in local development environments. It makes it easy to inject secrets right into the local application environments based on the permissions given to corresponding developers.
### Dashboard
On top of that, Infisical provides a great [Web Dashboard](https://app.infisical.com/signup) that can be used to making quick secret updates.
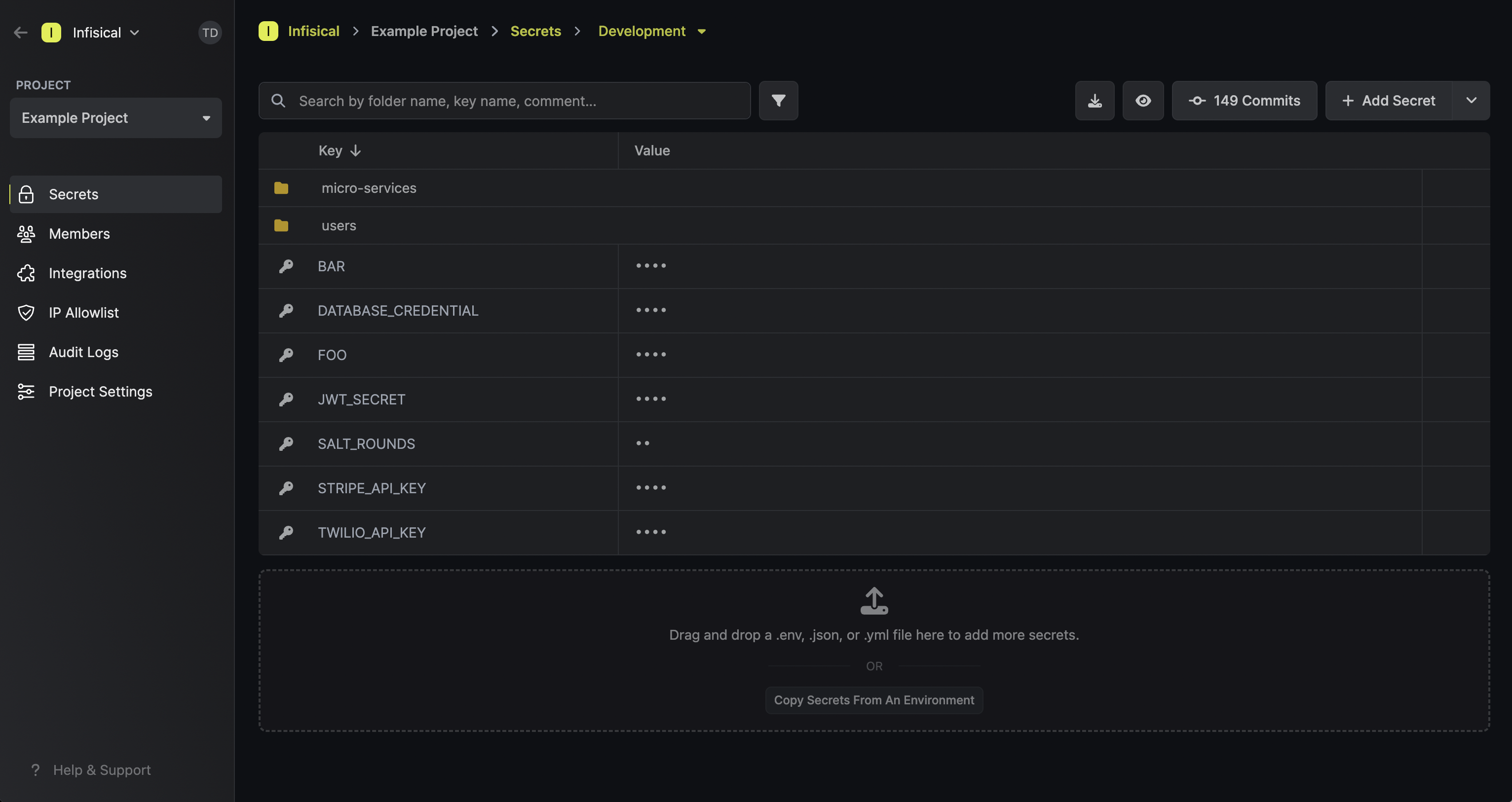
### Personal Overrides
By default, all the secrets in the Infisical environments are shared among project members who have the permission to access those environment. At the same time, when doing local development, it is often desirable to change the value of a certain secret only for a particular self. For such use cases, Infisical supports the functionality of **Personal Overrides** – which allow developers to override values of any secrets without affecting the workflows of the rest of the team. Personal Overrides can be created both in the dashboard or via [Infisical CLI](/cli/overview).
### Secret Scanning
In addition, Infisical also provides a set of tools to automatically prevent secret leaks to git history. This functionality can be set up on the level of [Infisical CLI using pre-commit hooks](/cli/scanning-overview#automatically-scan-changes-before-you-commit) or through a direct integration with platforms like GitHub.
# Microsoft Power Apps
Learn how to manage secrets in Microsoft Power Apps with Infisical.
In recent years, there has been a shift towards so-called low-code and no-code platforms. These platforms are particularly appealing to businesses without internal development capabilities, yet teams often discover that some coding is necessary to fully satisfy their business needs.
Low-code platforms have become increasingly sophisticated and useful, leading to a rise in their adoption by businesses. A prime example is Microsoft Power Apps, which offers a range of data sources and service integrations right out of the box. However, even with advanced tools, you might not always find a ready-made solution for every challenge. This means that low-code doesn't equate to no-code, as some coding and customization are still required to cater to specific needs.
Consider the need for data integrations where an HTTP-based call to a web service might be necessary, typically requiring authentication through an API key or another type of secret.
Importantly, it's crucial to avoid hardcoding these secrets, as they would then be accessible to anyone with collaboration rights to the code. This underscores the importance of using a secret management solution like Infisical.
In this article, we'll demonstrate how to retrieve app secrets from Infisical for use in a Power Apps application. We'll create a simple application with a dedicated data connector to illustrate the ease of integrating Infisical with Power Apps. This tutorial assumes some prior programming experience in C#.
Prerequisites:
* Created Microsoft Power App.
First, let’s create a new Azure Function using the Azure Management Portal. Get the [Function App](https://azuremarketplace.microsoft.com/en-us/marketplace/apps/Microsoft.FunctionApp?tab=Overview) from the [Azure Marketplace](https://azuremarketplace.microsoft.com/en-us/).
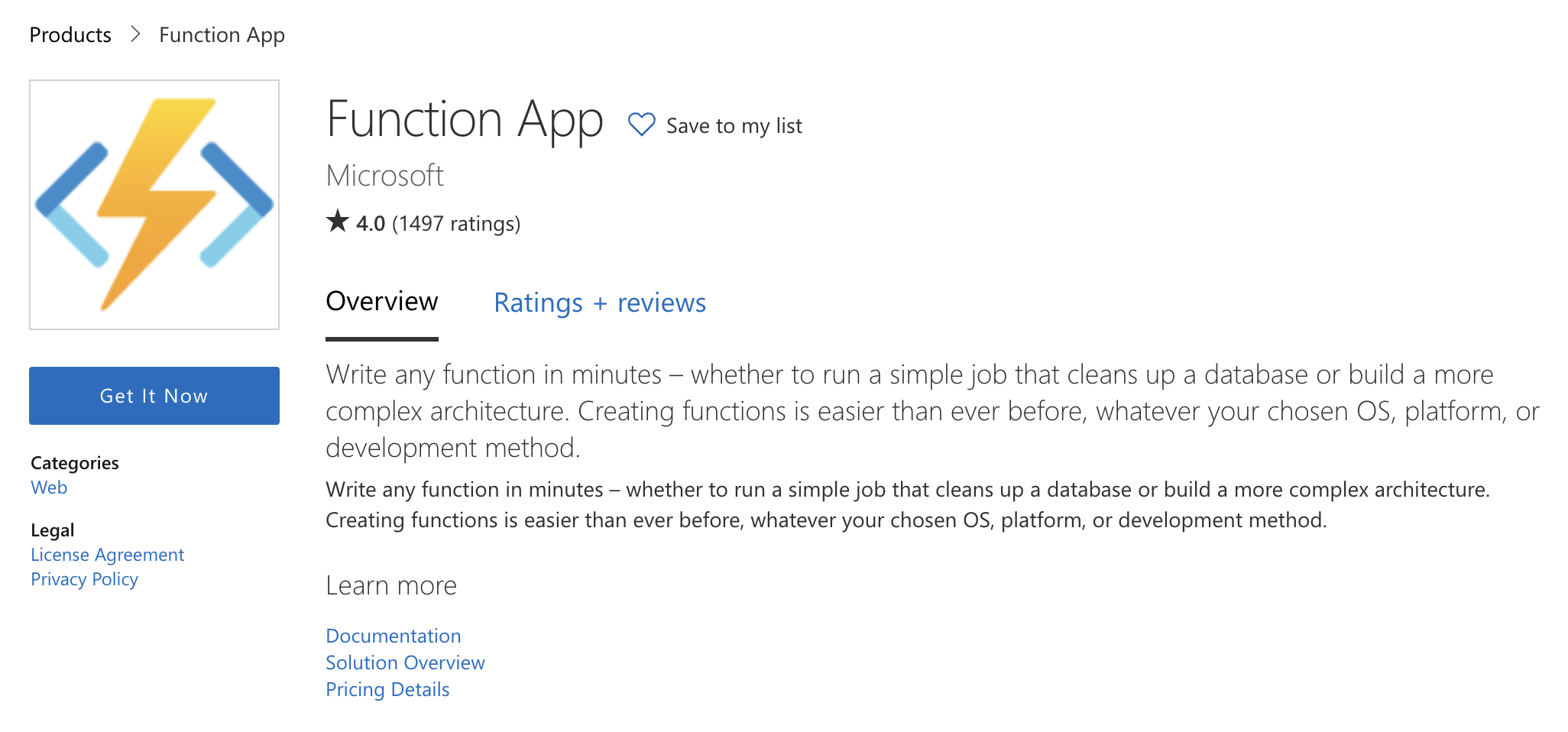
Place it in a subscription using any resource group. The name of the function is arbitrary. We'll use .NET as a runtime stack, but you can use whatever you're most comfortable with. The OS choice is also up to you. While Linux may look like a lightweight solution, Windows actually has more Azure Functions support. For instance, you cannot edit a Linux-based Azure Function within the Azure management portal.
By using a consumption plan, we'll only pay for the resources we use when they are requested. This is the classic “serverless” approach, where you do not pay for running servers, only for interactivity.
Once the new Azure Functions instance is ready, we add a function. In this case, we can do that already from the Azure Management Portal. Use the “HTTP trigger” template and choose the “function” authorization level.
The code for the first function can be as simple as:
```
using System.Net;
public static async Task Run(HttpRequestMessage req, TraceWriter log)
{
log.Info("C# HTTP trigger function processed a request.");
return req.CreateResponse(HttpStatusCode.OK, "Hello World");
}
```
The code above is written for the older runtime. As a result, you may need to change the runtime version to 1 for the Azure Power Apps integration to work. If we start at a newer version (for example, 3) this triggers a warning before the migration.
Finally, we also need to publish the Swagger (or API) definitions and enable cross-origin resource sharing (CORS). While the API definitions are rather easy to set up, the correct CORS value may be tricky. For now, we can use the wildcard option to allow all hosts.
Once we set all this up, it’s time to create the custom connector.
You can create the custom connector via the data pane. When we use “Create from Azure Service (Preview)”, this yields a dialog similar to the following:
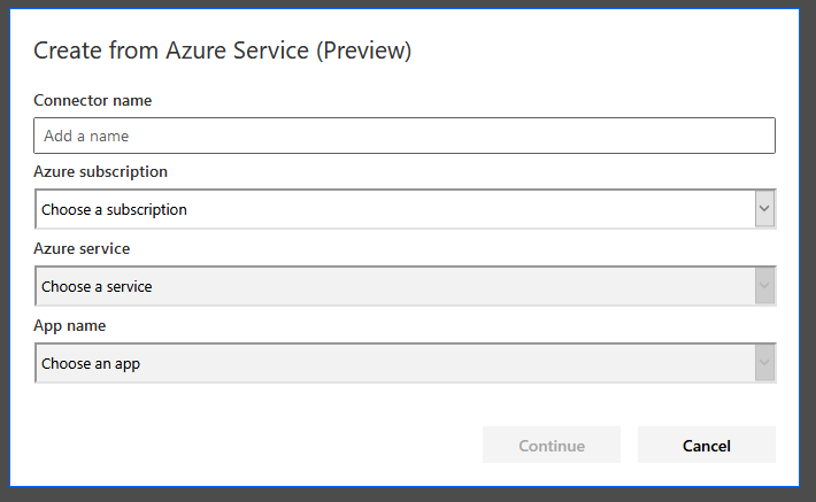
We can now fill out the fields using the information for our created function. The combination boxes are automatically filled in order. Once we select one of the reachable subscriptions (tied to the same account we’ve used to log in to create a Power App), the available services are displayed. Once we select our Azure Functions service, we select the function for retrieving the secret.
You can add Infisical in an Azure Function quite easily using the [Infisical SDK for .NET](https://infisical.com/docs/sdks/languages/csharp) (or other languages). This enables the function to communicate with Infisical to obtain secrets, among other things.
In short, we can simply bring all the necessary classes over and start using the Client class. Essentially, this enables us to write code like this:
```
var settings = new ClientSettings
{
ClientId = "CLIENT_ID",
ClientSecret = "CLIENT_SECRET",
// SiteUrl = "http://localhost:8080", <-- This line can be omitted if you're using Infisical Cloud.
};
var infisical = new InfisicalClient(settings);
var options = new GetSecretOptions
{
SecretName = "TEST",
ProjectId = "PROJECT_ID",
Environment = "dev",
};
var secret = infisical.GetSecret(options);
```
Knowing the URL of Infisical as well as the Client Id and Client Secret, we can now access the desired values.
Now it’s time to actually use the secret within a Power App. There are two ways to request a desired target service with a secret retrieved from the function:
1. Call the function first, retrieve the secret, then call the target service, for example, via another custom connector with the secret as input.
2. Perform the final API request within the function call — not returning a secret at all, just the response from invoking the target service.
While the first option is more flexible (and presumably cheaper!), the second option is definitely easier. In the end, you should mostly decide based on whether the function should be reused for other purposes. If the single Power App is the only consumer of the function, it may make more sense to go with the second option. Otherwise, you should use the first option.
For our simple example, we don’t need to reuse the function. We also don’t want the additional complexity of maintaining two different custom connectors, where we only use one to pass data to the other one.
Based on the previous snippet, we create the following code (for proxying a GET request from an API accessible via the URL specified in the apiEndpoint variable).
```
using (var client = new HttpClient())
{
client.DefaultRequestHeaders
.Accept
.Add(new MediaTypeWithQualityHeaderValue("application/json"));
client.DefaultRequestHeaders.Add("X-API-KEY", secret);
var result = await client.GetAsync(apiEndpoint);
var resultContent = await result.Content.ReadAsStringAsync();
req.CreateResponse(HttpStatusCode.OK, resultContent);
}
```
This creates a request to the resource protected by an API key that is retrieved from Infisical.
# Next.js + Vercel
This guide demonstrates how to use Infisical to manage secrets for your Next.js + Vercel stack from local development to production. It uses:
* Infisical (you can use [Infisical Cloud](https://app.infisical.com) or a [self-hosted instance of Infisical](https://infisical.com/docs/self-hosting/overview)) to store your secrets.
## Project Setup
To begin, we need to set up a project in Infisical and add secrets to an environment in it.
### Create a project
1. Create a new project in [Infisical](https://app.infisical.com/).
2. Add a secret to the development environment of this project so we can pull it back for local development. In the **Secrets Overview** page, press **Explore Development** and add a secret with the key `NEXT_PUBLIC_NAME` and value `YOUR_NAME`.
3. Add a secret to the production environment of this project so we can sync it to Vercel. Switch to the **Production** environment and add a secret with the key `NEXT_PUBLIC_NAME` and value `ANOTHER_NAME`.
## Create a Next.js app
Initialize a new Node.js app.
We can use `create-next-app` to initialize an app called `infisical-nextjs`.
```console
npx create-next-app@latest --use-npm infisical-nextjs
cd infisical-nextjs
```
```console
npx create-next-app@latest --ts --use-npm infisical-nextjs
cd infisical-nextjs
```
Next, inside `pages/_app.js`, lets add a `console.log()` to print out the environment variable in the browser console.
```js
import '@/styles/globals.css'
export default function App({ Component, pageProps }) {
console.log('Hello, ', process.env.NEXT_PUBLIC_NAME);
return
}
```
```tsx
import '@/styles/globals.css'
import type { AppProps } from 'next/app'
export default function App({ Component, pageProps }: AppProps) {
console.log('Hello, ', process.env.NEXT_PUBLIC_NAME);
return
}
```
## Infisical CLI for local development environment variables
We'll now use the Infisical CLI to fetch secrets from Infisical into your Next.js app for local development.
### CLI Installation
Follow the instructions for your operating system to install the Infisical CLI.
Use [brew](https://brew.sh/) package manager
```console
$ brew install infisical/get-cli/infisical
```
Use [Scoop](https://scoop.sh/) package manager
```console
$ scoop bucket add org https://github.com/Infisical/scoop-infisical.git
```
```console
$ scoop install infisical
```
Install prerequisite
```console
$ apk add --no-cache bash sudo
```
Add Infisical repository
```console
$ curl -1sLf \
'https://dl.cloudsmith.io/public/infisical/infisical-cli/setup.alpine.sh' \
| bash
```
Then install CLI
```console
$ apk update && sudo apk add infisical
```
Add Infisical repository
```console
$ curl -1sLf \
'https://dl.cloudsmith.io/public/infisical/infisical-cli/setup.rpm.sh' \
| sudo -E bash
```
Then install CLI
```console
$ sudo yum install infisical
```
Add Infisical repository
```console
$ curl -1sLf \
'https://dl.cloudsmith.io/public/infisical/infisical-cli/setup.deb.sh' \
| sudo -E bash
```
Then install CLI
```console
$ sudo apt-get update && sudo apt-get install -y infisical
```
Use the `yay` package manager to install from the [Arch User Repository](https://aur.archlinux.org/packages/infisical-bin)
```console
$ yay -S infisical-bin
```
### Login
Authenticate the CLI with the Infisical platform using your email and password.
```console
$ infisical login
```
### Initialization
Run the `init` command at the root of the Next.js app. This step connects your local project to the project on the Infisical platform and creates a `infisical.json` file containing a reference to that latter project.
```console
$ infisical init
```
### Start the Next.js app with secrets injected as environment variables
```console
$ infisical run -- npm run dev
```
If you open your browser console, **Hello, YOUR\_NAME** should be printed out.
Here, the CLI fetched the secret from Infisical and injected it into the Next.js app upon starting up. By default,
the CLI fetches secrets from the development environment which has the slug `dev`; you can inject secrets from different
environments by modifying the `env` flag as per the [CLI documentation](/cli/usage).
At this stage, you know how to use the Infisical CLI to inject secrets into your Next.js app for local development.
## Infisical-Vercel integration for production environment variables
We'll now use the Infisical-Vercel integration send secrets from Infisical to Vercel as production environment variables.
### Infisical-Vercel integration
To begin we have to import the Next.js app into Vercel as a project. [Follow these instructions](https://nextjs.org/learn/basics/deploying-nextjs-app/deploy) to deploy the Next.js app to Vercel.
Next, navigate to your project's integrations tab in Infisical and press on the Vercel tile to grant Infisical access to your Vercel account.
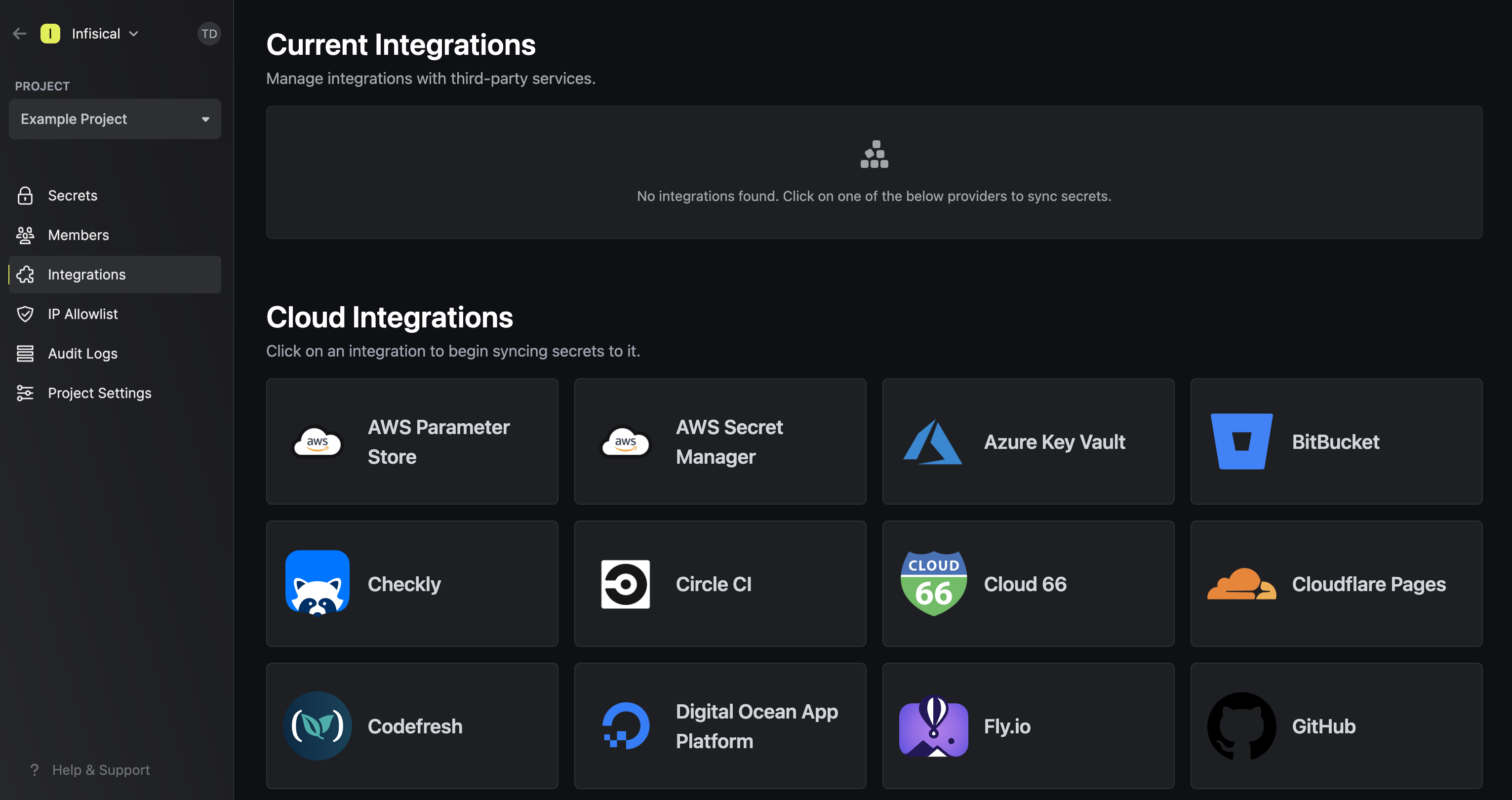
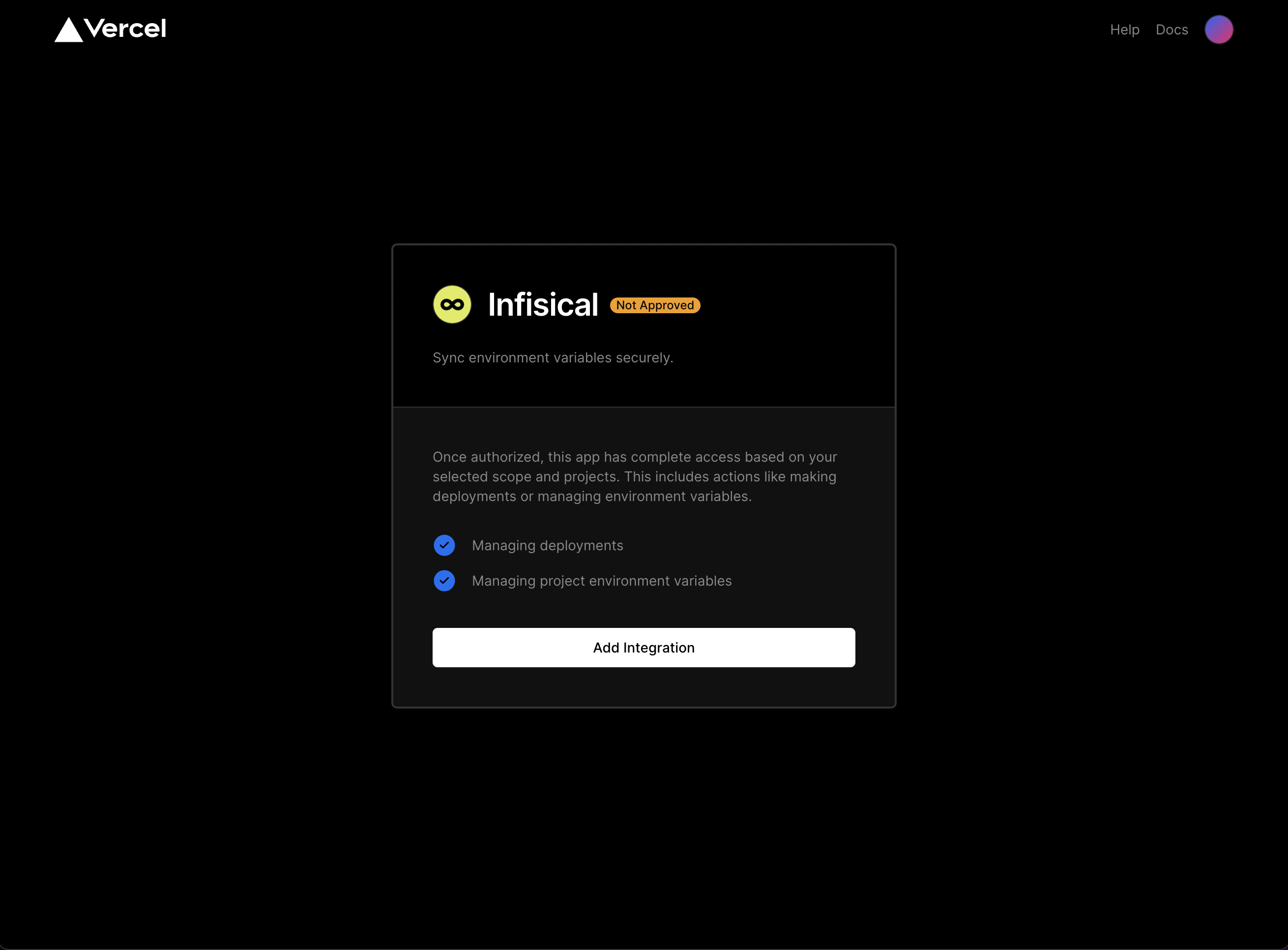
Opting in for the Infisical-Vercel integration will break end-to-end encryption since Infisical will be able to read
your secrets. This is, however, necessary for Infisical to sync the secrets to Vercel.
Your secrets remain encrypted at rest following our [security guide mechanics](/security/mechanics).
Now select **Production** for (the source) **Environment** and sync it to the **Production Environment** of the (target) application in Vercel.
Lastly, press create integration to start syncing secrets to Vercel.
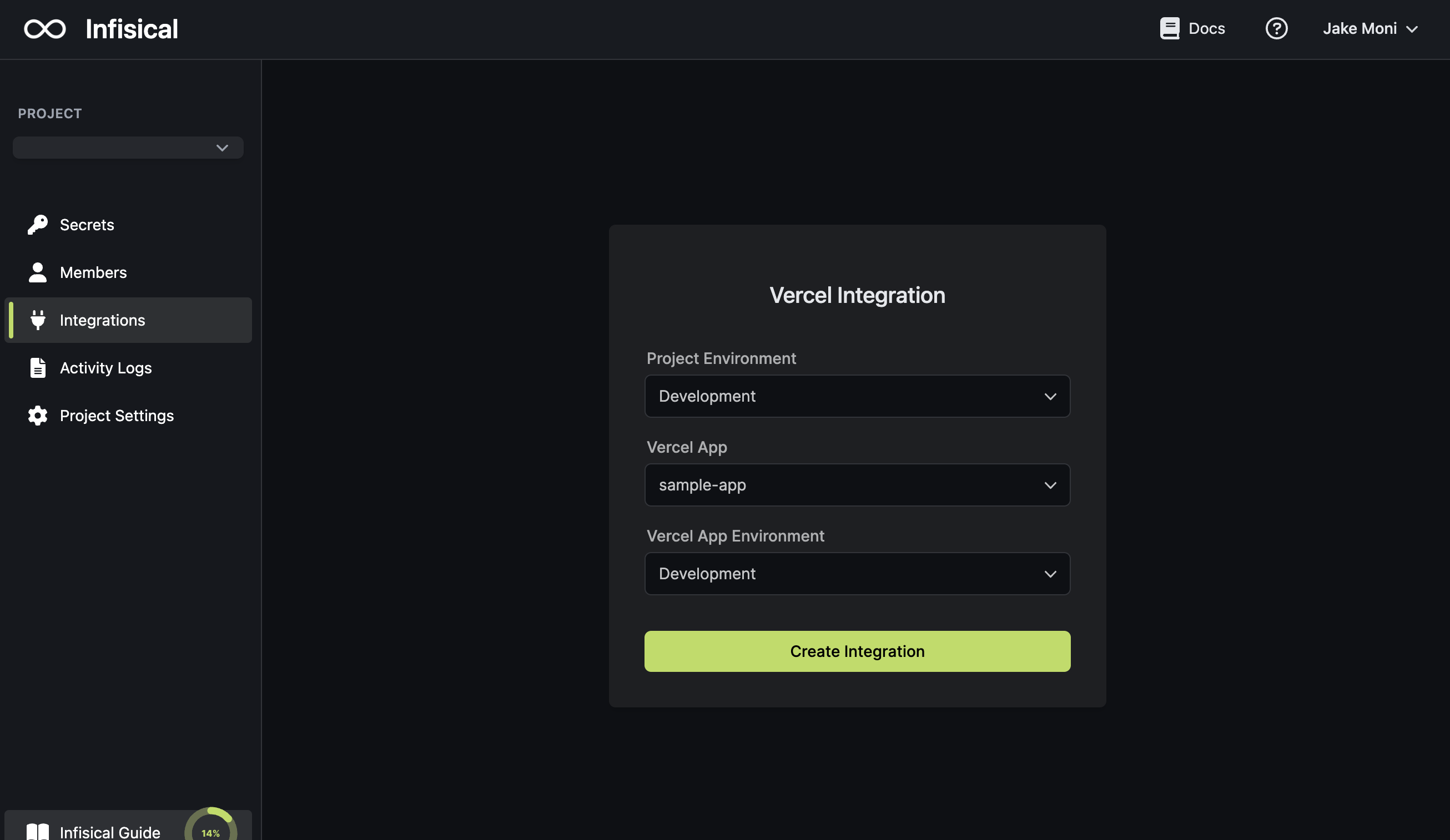
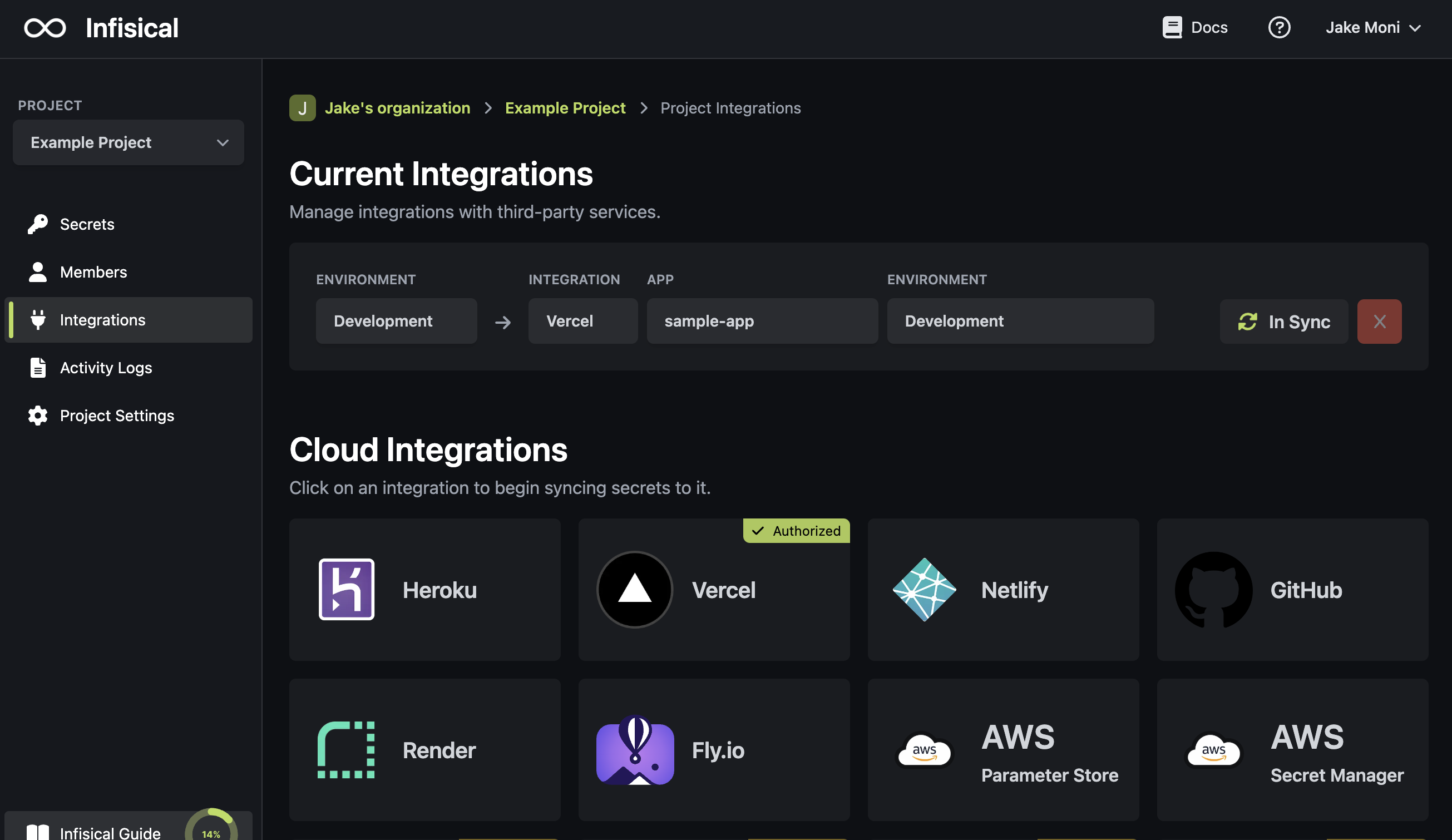
You should now see your secret from Infisical appear as production environment variables in your Vercel project.
At this stage, you know how to use the Infisical-Vercel integration to sync production secrets from Infisical to Vercel.
The following environment variable names are reserved by Vercel and cannot be
synced: `AWS_SECRET_KEY`, `AWS_EXECUTION_ENV`, `AWS_LAMBDA_LOG_GROUP_NAME`,
`AWS_LAMBDA_LOG_STREAM_NAME`, `AWS_LAMBDA_FUNCTION_NAME`,
`AWS_LAMBDA_FUNCTION_MEMORY_SIZE`, `AWS_LAMBDA_FUNCTION_VERSION`,
`NOW_REGION`, `TZ`, `LAMBDA_TASK_ROOT`, `LAMBDA_RUNTIME_DIR`,
`AWS_ACCESS_KEY_ID`, `AWS_SECRET_ACCESS_KEY`, `AWS_SESSION_TOKEN`,
`AWS_REGION`, and `AWS_DEFAULT_REGION`.
## FAQ
Vercel does not specialize in secret management which means it lacks many useful features for effectively managing environment variables.
Here are some features that teams benefit from by using Infisical together with Vercel:
* Audit logs: See which team members are creating, reading, updating, and deleting environment variables across all environments.
* Versioning and point in time recovery: Rolling back secrets and an entire project state.
* Overriding secrets that should be unique amongst team members.
And much more.
Yes. Your secrets are still encrypted at rest. To note, most secret managers actually don't support end-to-end encryption.
Check out the [security guide](/security/overview).
See also:
* [Documentation for the Infisical CLI](/cli/overview)
* [Documentation for the Vercel integration](/integrations/cloud/vercel)
# Node
This guide demonstrates how to use Infisical to manage secrets for your Node stack from local development to production. It uses:
* Infisical (you can use [Infisical Cloud](https://app.infisical.com) or a [self-hosted instance of Infisical](https://infisical.com/docs/self-hosting/overview)) to store your secrets.
* The [@infisical/sdk](https://github.com/Infisical/sdk/tree/main/languages/node) Node.js client SDK to fetch secrets back to your Node application on demand.
## Project Setup
To begin, we need to set up a project in Infisical and add secrets to an environment in it.
### Create a project
1. Create a new project in [Infisical](https://app.infisical.com/).
2. Add a secret to the development environment of this project so we can pull it back for local development. In the **Secrets Overview** page, press **Explore Development** and add a secret with the key `NAME` and value `YOUR_NAME`.
### Create a Machine Identity
Now that we've created a project and added a secret to its development environment, we need to configure an Infisical Machine Identity that our Node application can use to access the secret.
* [How to setup machine identities](/documentation/platform/identities/overview)
## Create a Node app
For this demonstration, we use a minimal Express application. However, the same principles will apply to any Node application such as those built on Koa or Fastify.
### Create an Express app
Initialize a new Node.js project with a default `package.json` file.
```console
npm init -y
```
Install `express` and [@infisical/sdk](https://www.npmjs.com/package/@infisical/sdk), the client Node SDK for Infisical.
```console
npm install express @infisical/sdk
```
Finally, create an index.js file containing the application code.
```js
const express = require('express');
const { InfisicalClient } = require("@infisical/sdk");
const app = express();
const PORT = 3000;
const client = new InfisicalClient({
auth: {
universalAuth: {
clientId: "YOUR_CLIENT_ID",
clientSecret: "YOUR_CLIENT_SECRET",
}
}
});
app.get("/", async (req, res) => {
// access value
const name = await client.getSecret({
environment: "dev",
projectId: "PROJECT_ID",
path: "/",
type: "shared",
secretName: "NAME"
});
res.send(`Hello! My name is: ${name.secretValue}`);
});
app.listen(PORT, async () => {
// initialize client
console.log(`App listening on port ${PORT}`);
});
```
Here, we initialized a `client` instance of the Infisical Node SDK with the Infisical Token
that we created earlier, giving access to the secrets in the development environment of the
project in Infisical that we created earlier.
Finally, start the app and head to `http://localhost:3000` to see the message **Hello, Your Name**.
```console
node index.js
```
The client fetched the secret with the key `NAME` from Infisical that we returned in the response of the endpoint.
At this stage, you know how to fetch secrets from Infisical back to your Node application. By using Infisical Tokens scoped to different environments, you can easily manage secrets across various stages of your project in Infisical, from local development to production.
## FAQ
The client SDK caches every secret and implements a 5-minute waiting period before
re-requesting it. The waiting period can be controlled by setting the `cacheTTL` parameter at
the time of initializing the client.
The SDK caches every secret and falls back to the cached value if a request fails. If no cached
value ever-existed, the SDK falls back to whatever value is on `process.env`.
The token enables the SDK to authenticate with Infisical to fetch back your secrets.
Although the SDK requires you to pass in a token, it enables greater efficiency and security
than if you managed dozens of secrets yourself without it. Here're some benefits:
* You always pull in the right secrets because they're fetched on demand from a centralized source that is Infisical.
* You can use the Infisical which comes with tons of benefits like secret versioning, access controls, audit logs, etc.
* You now risk leaking one token that can be revoked instead of dozens of raw secrets.
And much more.
See also:
* Explore the [Node SDK](https://github.com/Infisical/sdk/tree/main/languages/node)
# Infisical Organizational Structure Blueprint
Learn how to structure your projects, secrets, and other resources within Infisical.
Infisical is designed to provide comprehensive, centralized, and efficient management of secrets, certificates, and encryption keys within organizations. Below is an overview of Infisical's structured components, which developers and administrators can leverage for optimal project management and security posture.
### 1. Projects
* **Definition and Role**: [Projects](/documentation/platform/project) are the highest-level construct within an [organization](/documentation/platform/organization) in Infisical. They serve as the primary container for all functionalities.
* **Correspondence to Code Repositories**: Projects typically align with specific code repositories.
* **Functional Capabilities**: Each project encompasses features for managing secrets, certificates, and encryption keys, serving as the central hub for these resources.
### 2. Environments
* **Purpose**: Environments are designed for organizing and compartmentalizing secrets within projects.
* **Customization Options**: Environments can be tailored to align with existing infrastructure setups of any project. Default options include **Development**, **Staging**, and **Production**.
* **Structure**: Each environment inherently has a root level for storing secrets, but additional sub-organizations can be created through [folders](/documentation/platform/folder) for better secret management.
### 3. Folders
* **Use Case**: Folders are available for more advanced organizational needs, allowing logical separation of secrets.
* **Typical Structure**: Folders can correspond to specific logical units, such as microservices or different layers of an application, providing refined control over secrets.
### 4. Imports
* **Purpose and Benefits**: To promote reusability and avoid redundancy, Infisical supports the use of imports. This allows secrets, folders, or entire environments to be referenced across multiple projects as needed.
* **Best Practice**: Utilizing [secret imports](/documentation/platform/secret-reference#secret-imports) or [references](/documentation/platform/secret-reference#secret-referencing) ensures consistency and minimizes manual overhead.
### 5. Approval Workflows
* **Importance**: Implementing approval workflows is recommended for organizations aiming to enhance efficiency and strengthen their security posture.
* **Types of Workflows**:
* **[Access Requests](/documentation/platform/pr-workflows)**: This workflow allows developers to request access to sensitive resources. Such access can be configured for temporary use, a practice known as "just-in-time" access.
* **[Change Requests](/documentation/platform/access-controls/access-requests)**: Facilitates reviews and approvals when changes are proposed for sensitive environments or specific folders, ensuring proper oversight.
### 6. Access Controls
Infisical’s access control framework is unified for both human users and machine identities, ensuring consistent management across the board.
### 6.1 Roles
* **2 Role Types**:
* **Organization-Level Roles**: Provide broad access across the organization (e.g., ability to manage billing, configure settings, etc.).
* **Project-Level Roles**: Essential for configuring access to specific secrets and other sensitive assets within a project.
* **Granular Permissions**: While default roles are available, [custom roles](/documentation/platform/access-controls/role-based-access-controls#creating-custom-roles) can be created for more tailored access controls.
* **Admin Considerations**: Note that admin users are able to access all projects. This role should be assigned judiciously to prevent unintended overreach.
Project access is defined not via an organization-level role, but rather through specific project memberships of both human and machine identities. Admin roles bypass this by default.
### 6.2 Additional Privileges
[Additional privileges](/documentation/platform/access-controls/additional-privileges) can be assigned to users and machines on an ad-hoc basis for specific scenarios where roles alone are insufficient. If you find yourself using additional privileges too much, it is recommended to create custom roles. Additional privileges can be temporary or permanent.
### 6.3 Attribute-Based Access Control (ABAC)
[Attribute-based Access Controls](/documentation/platform/access-controls/attribute-based-access-controls) allow restrictions based on tags or attributes linked to secrets. These can be integrated with SAML assertions and other security frameworks for dynamic access management.
### 6.4 User Groups
* **Application**: Organizations should use users groups in situations when they have a lot of developers with the same level of access (e.g., separated by team, department, seniority, etc.).
* **Synchronization**: [User groups](/documentation/platform/groups) can be synced with an identity provider to maintain consistency and reduce manual management.
### **Implementation Note**
For larger-scale organizations, automating configurations through **Terraform** or other infrastructure-as-code (IaC) tools is advisable. Manual configurations may lead to errors, so leveraging IaC enhances reliability and consistency in managing Infisical's robust capabilities.
This structured approach ensures that Infisical's functionalities are fully leveraged, providing both flexibility and rigorous control over an organization's sensitive information and access needs.
# Python
This guide demonstrates how to use Infisical to manage secrets for your Python stack from local development to production. It uses:
* Infisical (you can use [Infisical Cloud](https://app.infisical.com) or a [self-hosted instance of Infisical](https://infisical.com/docs/self-hosting/overview)) to store your secrets.
* The [infisical-python](https://pypi.org/project/infisical-python/) Python client SDK to fetch secrets back to your Python application on demand.
## Project Setup
To begin, we need to set up a project in Infisical and add secrets to an environment in it.
### Create a project
1. Create a new project in [Infisical](https://app.infisical.com/).
2. Add a secret to the development environment of this project so we can pull it back for local development. In the **Secrets Overview** page, press **Explore Development** and add a secret with the key `NAME` and value `YOUR_NAME`.
### Create a Machine Identity
Now that we've created a project and added a secret to its development environment, we need to configure an Infisical Machine Identity that our Python application can use to access the secret.
* [How to setup machine identities](/documentation/platform/identities/overview)
## Create a Python app
For this demonstration, we use a minimal Flask application. However, the same principles will apply to any Python application such as those built with Django.
### Create a Flask app
First, create a virtual environment and activate it.
```console
python3 -m venv env
source env/bin/activate
```
Install Flask and [infisical-python](https://pypi.org/project/infisical-python/), the client Python SDK for Infisical.
```console
pip install flask infisical-python
```
Finally, create an `app.py` file containing the application code.
```py
from flask import Flask
from infisical_client import ClientSettings, InfisicalClient, GetSecretOptions, AuthenticationOptions, UniversalAuthMethod
app = Flask(__name__)
client = InfisicalClient(ClientSettings(
auth=AuthenticationOptions(
universal_auth=UniversalAuthMethod(
client_id="CLIENT_ID",
client_secret="CLIENT_SECRET",
)
)
))
@app.route("/")
def hello_world():
# access value
name = client.getSecret(options=GetSecretOptions(
environment="dev",
project_id="PROJECT_ID",
secret_name="NAME"
))
return f"Hello! My name is: {name.secret_value}"
```
Here, we initialized a `client` instance of the Infisical Python SDK with the Infisical Token
that we created earlier, giving access to the secrets in the development environment of the
project in Infisical that we created earlier.
Finally, start the app and head to `http://localhost:5000` to see the message **Hello, Your Name**.
```console
flask run
```
The client fetched the secret with the key `NAME` from Infisical that we returned in the response of the endpoint.
At this stage, you know how to fetch secrets from Infisical back to your Python application. By using Infisical Tokens scoped to different environments, you can easily manage secrets across various stages of your project in Infisical, from local development to production.
## FAQ
The client SDK caches every secret and implements a 5-minute waiting period before
re-requesting it. The waiting period can be controlled by setting the `cacheTTL` parameter at
the time of initializing the client.
The SDK caches every secret and falls back to the cached value if a request fails. If no cached
value ever-existed, the SDK falls back to whatever value is on `process.env`.
The token enables the SDK to authenticate with Infisical to fetch back your secrets.
Although the SDK requires you to pass in a token, it enables greater efficiency and security
than if you managed dozens of secrets yourself without it. Here're some benefits:
* You always pull in the right secrets because they're fetched on demand from a centralized source that is Infisical.
* You can use the Infisical which comes with tons of benefits like secret versioning, access controls, audit logs, etc.
* You now risk leaking one token that can be revoked instead of dozens of raw secrets.
And much more.
See also:
* Explore the [Python SDK](https://github.com/Infisical/sdk/tree/main/crates/infisical-py)
# Access Requests
Learn how to request access to sensitive resources in Infisical.
In certain situations, developers need to expand their access to a certain new project or a sensitive environment. For those use cases, it is helpful to utilize Infisical's **Access Requests** functionality.
This functionality works in the following way:
1. A project administrator sets up an access policy that assigns access managers (also known as eligible approvers) to a certain sensitive folder or environment.
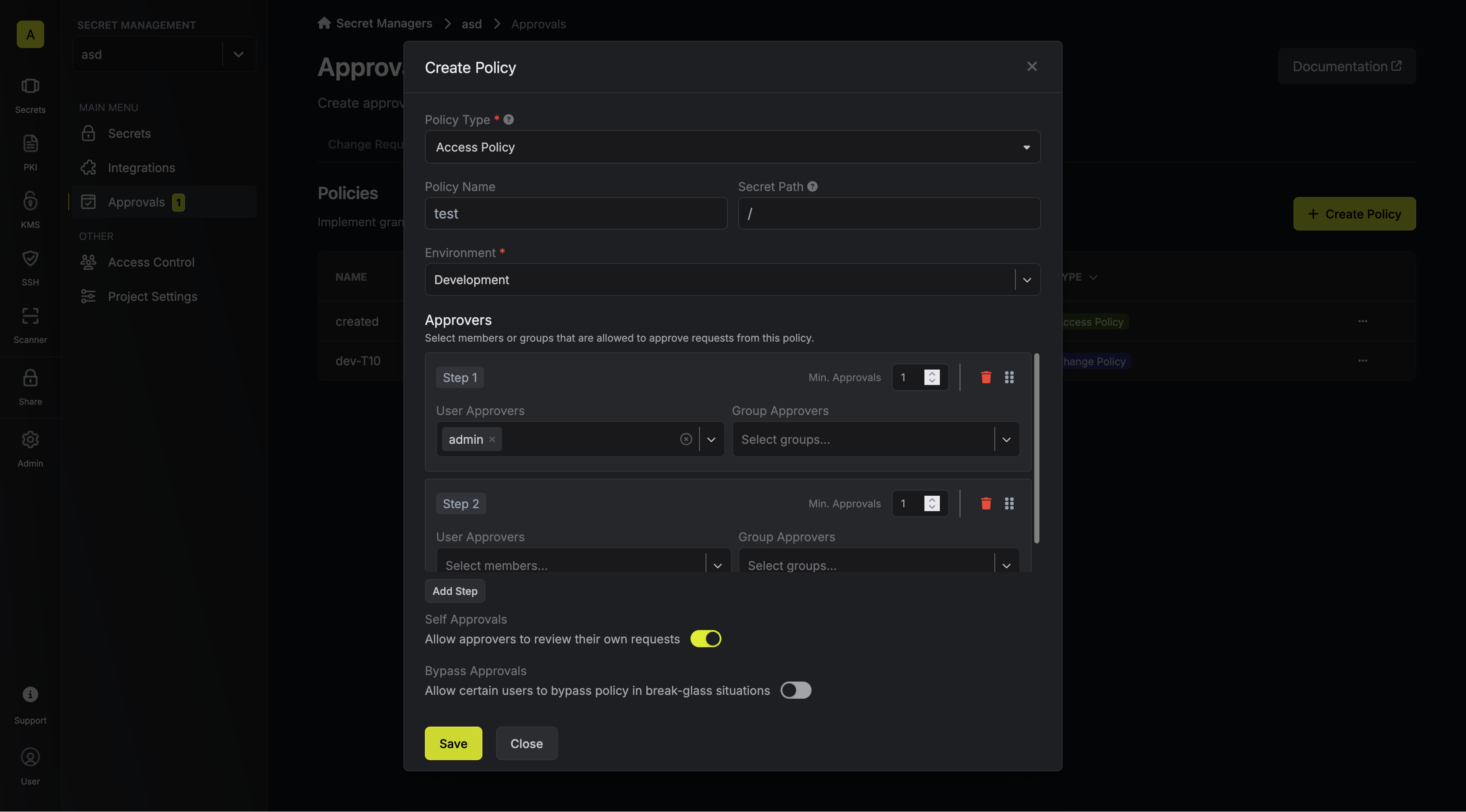
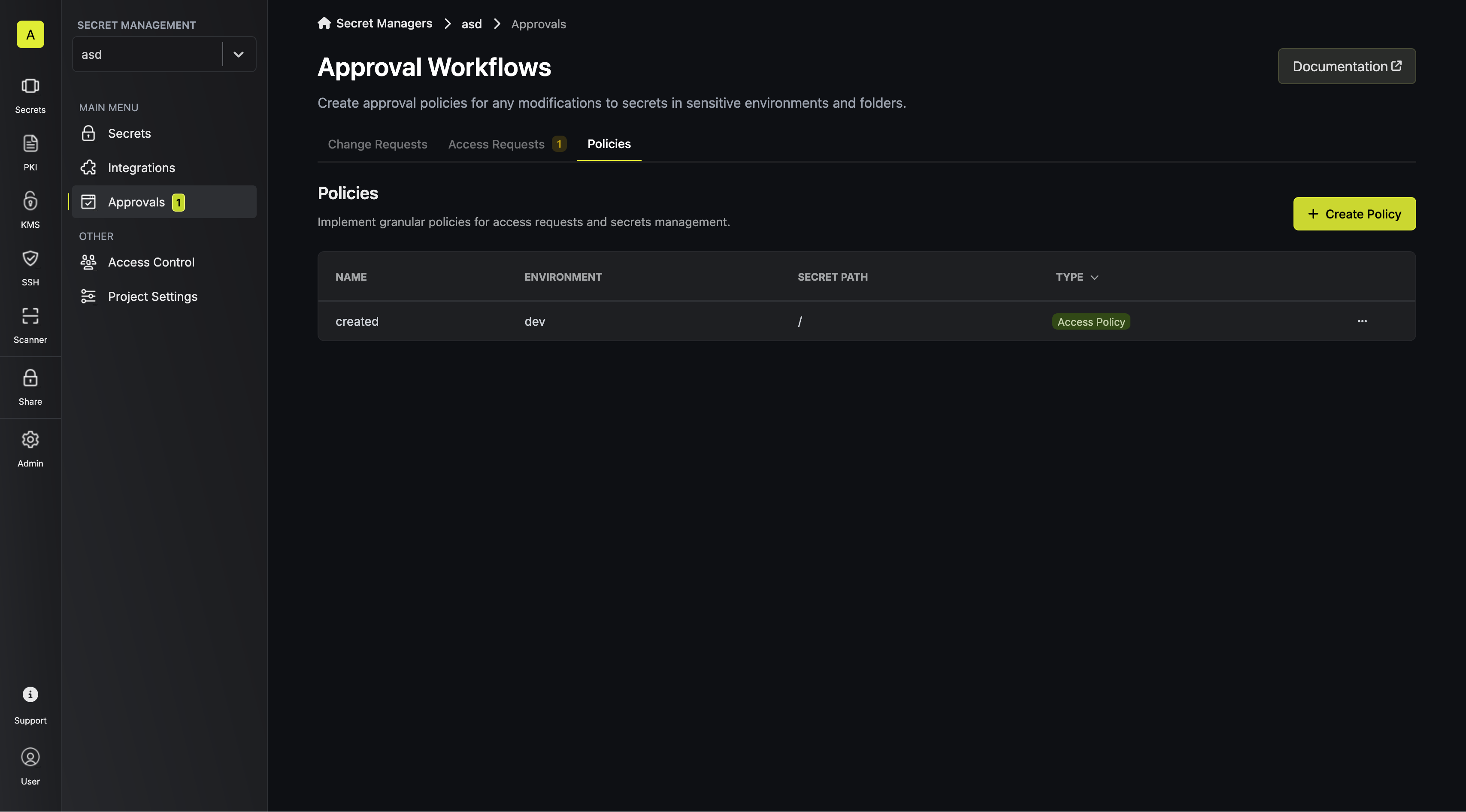
2. When a developer requests access to one of such sensitive resources, the request is visible in the dashboard, and the corresponding eligible approvers get an email notification about it.
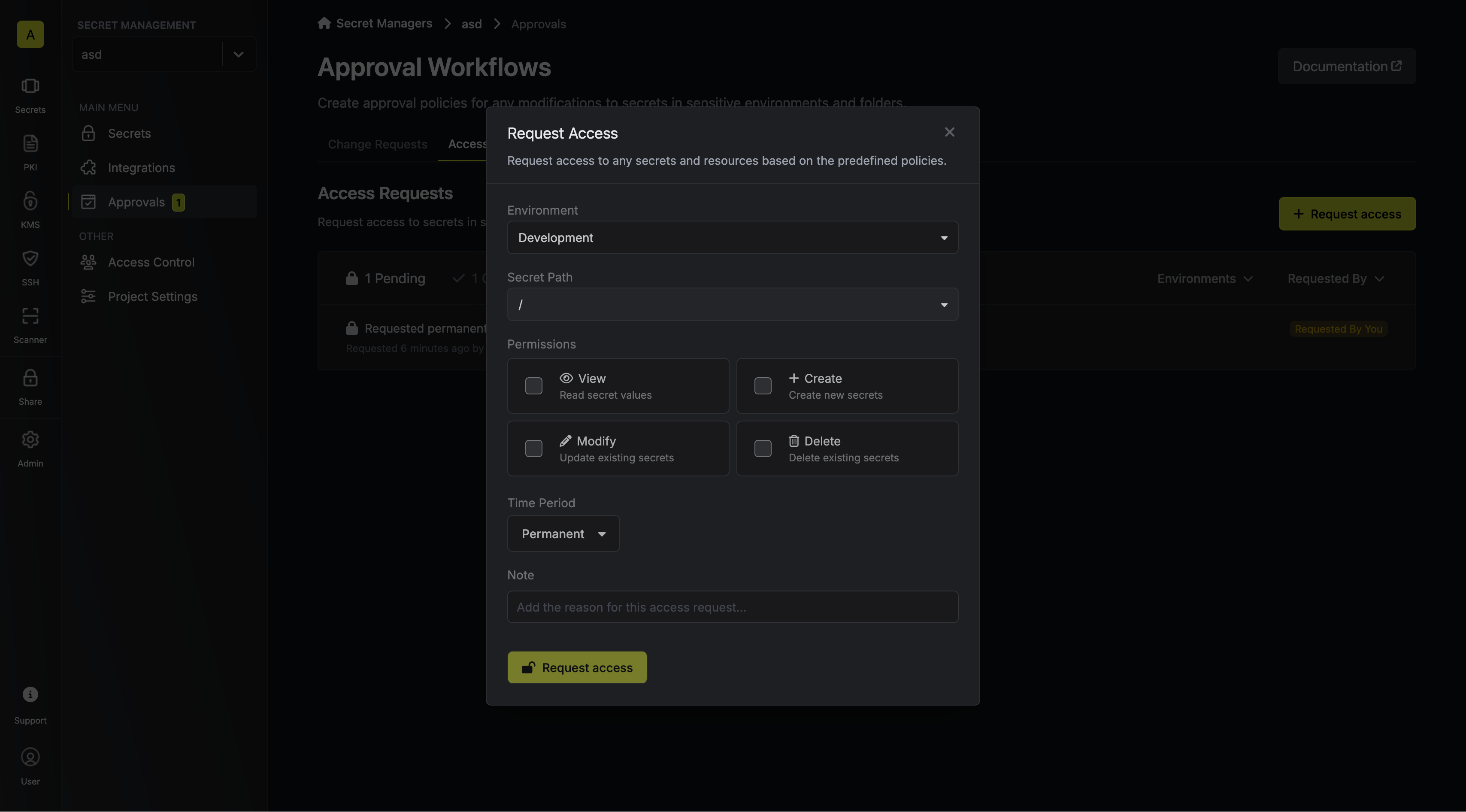
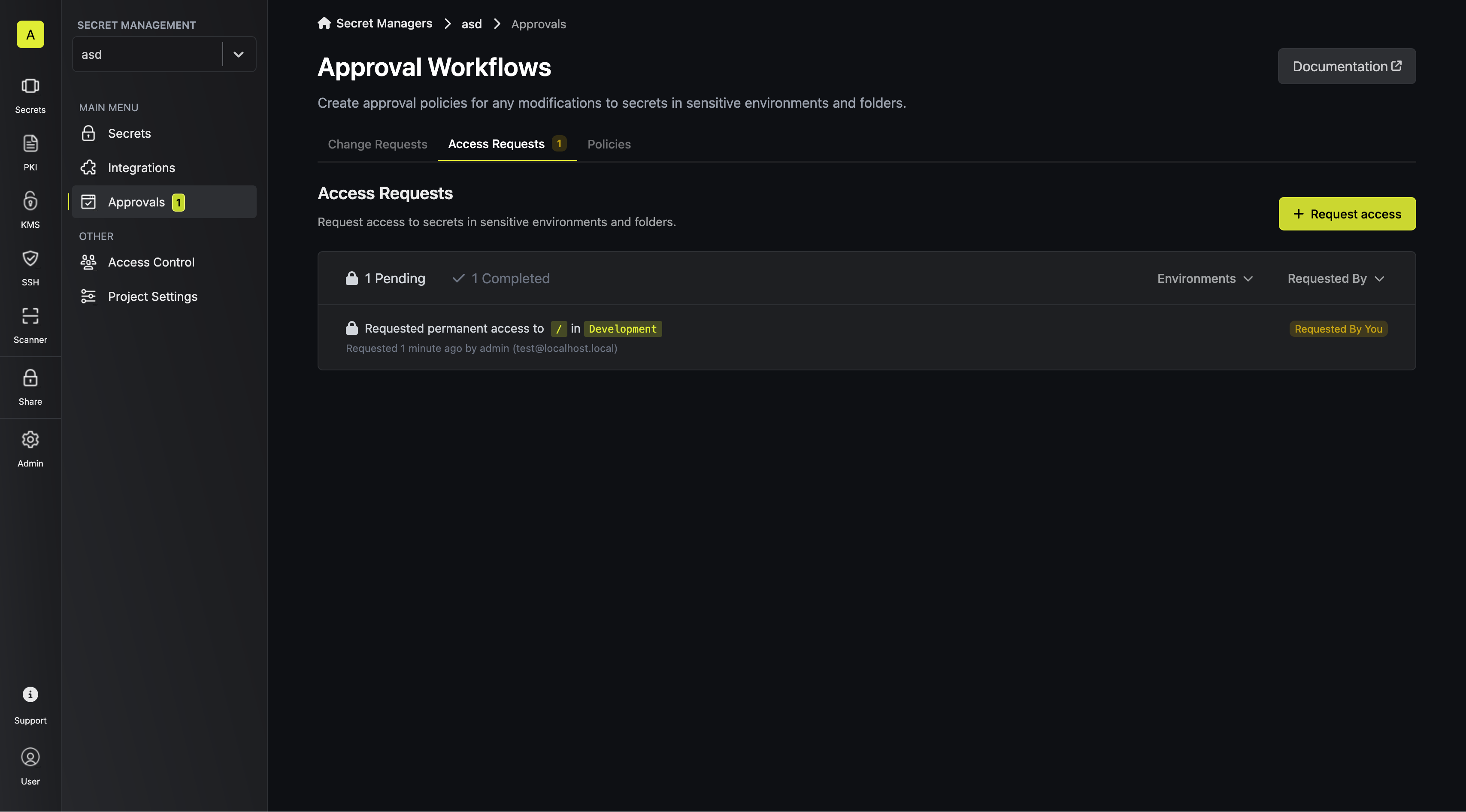
3. An eligible approver can approve or reject the access request.
{/*  */}
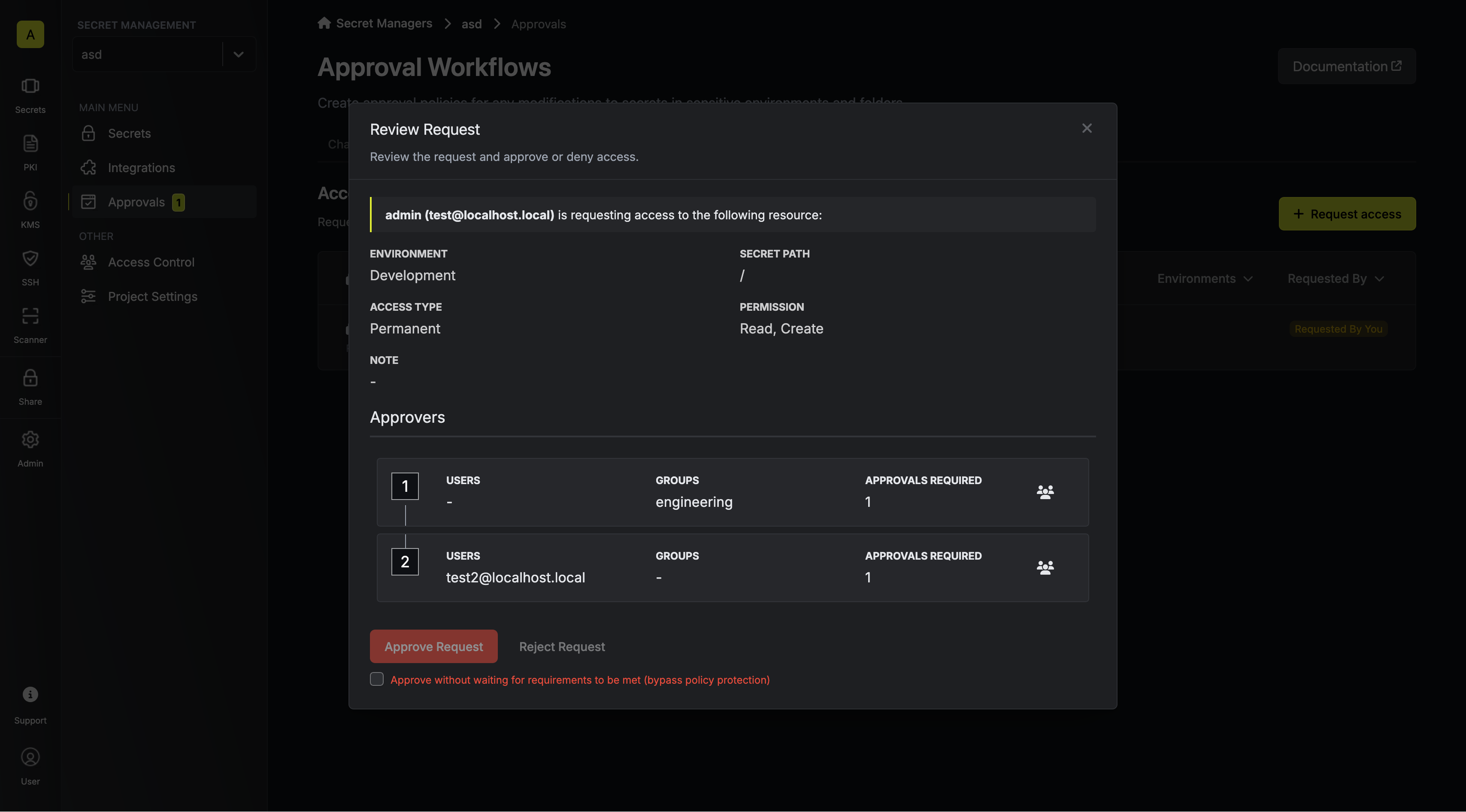
If the access request matches with a policy that has a **Soft** enforcement level, the requester may bypass the policy and get access to the resource without full approval.
5. As soon as the request is approved, developer is able to access the sought resources.
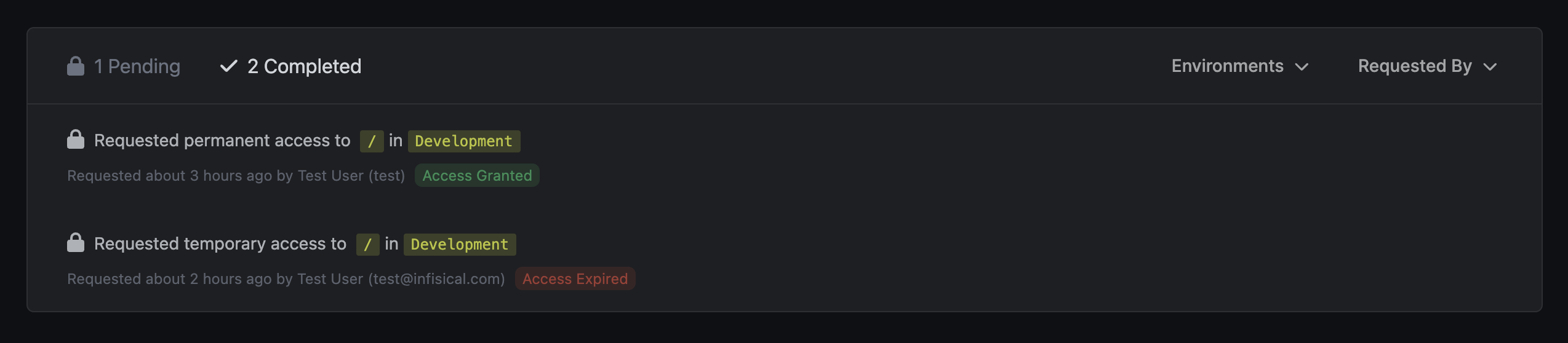
# Additional Privileges
Learn how to add specific privileges on top of predefined roles.
Even though Infisical supports full-fledged [role-base access controls](./role-based-access-controls) with ability to set predefined permissions for user and machine identities, it is sometimes desired to set additional privileges for specific user or machine identities on top of their roles.
Infisical **Additional Privileges** functionality enables specific permissions with access to sensitive secrets/folders by identities within certain projects. It is possible to set up additional privileges through Web UI or API.
To provision specific privileges through Web UI:
1. Click on the `Edit` button next to the set of roles for user or identities.
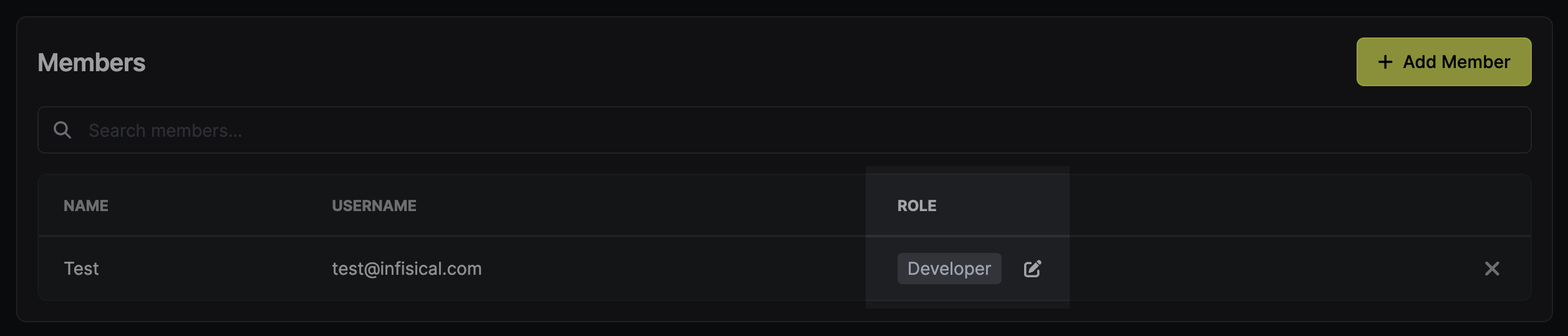
2. Click `Add Additional Privileges` in the corresponding section of the permission management modal.

3. Fill out the necessary parameters in the privilege entry that appears. It is possible to specify the `Environment` and `Secret Path` to which you want to enable access.
It is also possible to define the range of permissions (`View`, `Create`, `Modify`, `Delete`) as well as how long the access should last (e.g., permanent or timed).
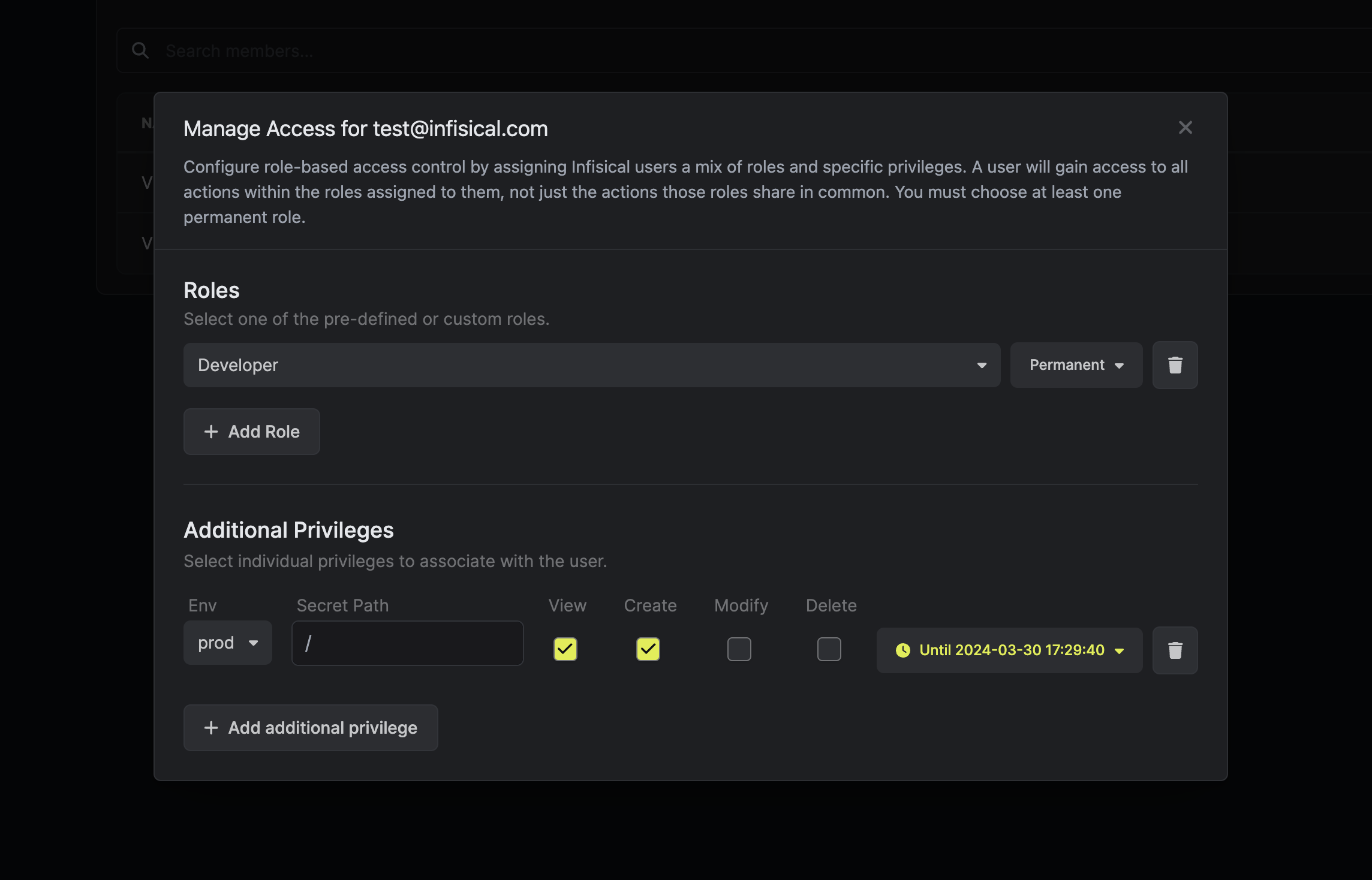
4. Click the `Save` button to enable the additional privilege.
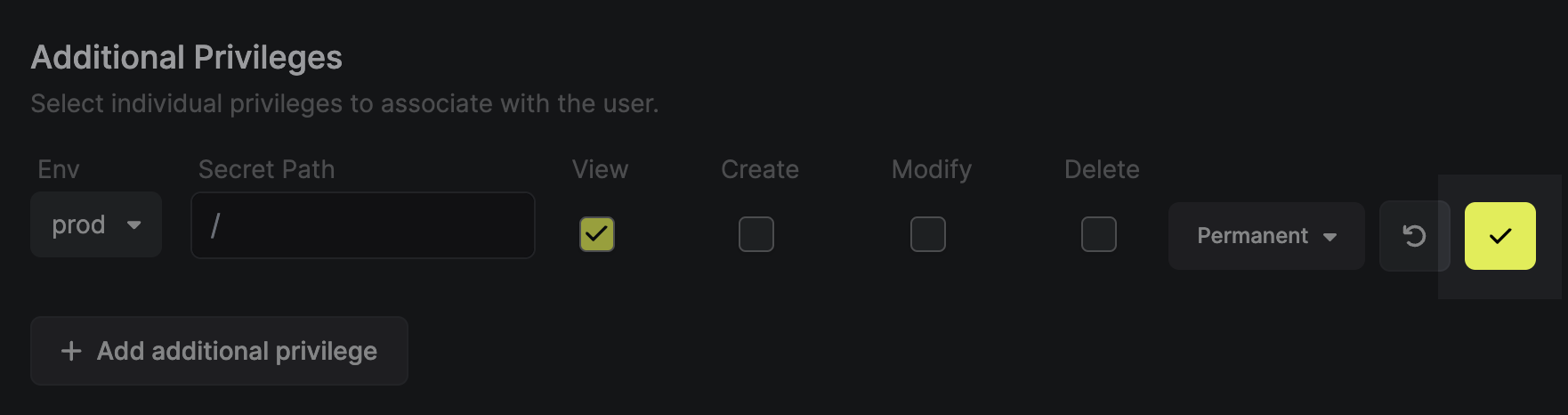
# Attribute-based Access Controls
Learn how to use ABAC to manage permissions based on identity attributes.
Infisical's Attribute-based Access Controls (ABAC) allow for dynamic, attribute-driven permissions for both user and machine identities.
ABAC policies use metadata attributes—stored as key-value pairs on identities—to enforce fine-grained permissions that are context aware.
In ABAC, access controls are defined using metadata attributes, such as location or department, which can be set directly on user or machine identities.
During policy execution, these attributes are evaluated, and determine whether said actor can access the requested resource or perform the requested operation.
## Project-level Permissions
Attribute-based access controls are currently available for polices defined on projects. You can set ABAC permissions to control access to environments, folders, secrets, and secret tags.
### Setting Metadata on Identities
For organizations using SAML for login, Infisical automatically maps metadata attributes from SAML assertions to user identities.
This makes it easy to create policies that dynamically adapt based on the SAML user’s attributes.
## Defining ABAC Policies
ABAC policies make use of identity metadata to define dynamic permissions. Each attribute must start and end with double curly-brackets `{{ }}`.
The following attributes are available within project permissions:
* **User ID**: `{{ identity.id }}`
* **Username**: `{{ identity.username }}`
* **Metadata Attributes**: `{{ identity.metadata. }}`
During policy execution, these placeholders are replaced by their actual values prior to evaluation.
### Example Use Case
#### Location-based Access Control
Suppose you want to restrict access to secrets within a specific folder based on a user's geographic region.
You could assign a `location` attribute to each user (e.g., `identity.metadata.location`).
You could then structure your folders to align with this attribute and define permissions accordingly.
For example, a policy might restrict access to folders matching the user's location attribute in the following pattern:
```
/appA/{{ identity.metadata.location }}
```
Using this structure, users can only access folders that correspond to their configured `location` attribute.
Consequently, if a users attribute changes due to relocation, no policies need to be changed to gain access to the folders associated with their new location.
# Access Controls
Learn about Infisical's access control toolset.
To make sure that users and machine identities are only accessing the resources and performing actions they are authorized to, Infisical supports a wide range of access control tools.
Manage user and machine identitity permissions through predefined roles.
Manage user and machine identitity permissions based on their attributes.
Add specific privileges to users and machines on top of their roles.
Grant timed access to roles and specific privileges.
Enable users to request (temporary) access to sensitive resources.
Set up review policies for secret changes in sensitive environments.
Track every action performed by user and machine identities in Infisical.
# Role-based Access Controls
Learn how to use RBAC to manage user permissions.
Infisical's Role-based Access Controls (RBAC) enable the usage of predefined and custom roles that imply a set of permissions for user and machine identities. Such roles male it possible to restrict access to resources and the range of actions that can be performed.
In general, access controls can be split up across [projects](/documentation/platform/project) and [organizations](/documentation/platform/organization).
## Organization-level access controls
By default, every user and machine identity in a organization is either an **admin** or a **member**.
**Admins** are able to perform every action with the organization, including adding and removing organization members, managing access controls, setting up security settings, and creating new projects.
**Members**, on the other hand, are restricted from removing organization members, modifying billing information, updating access controls, and performing a number of other actions.
Overall, organization-level access controls are significantly of administrative nature. Access to projects, secrets and other sensitive data is specified on the project level.
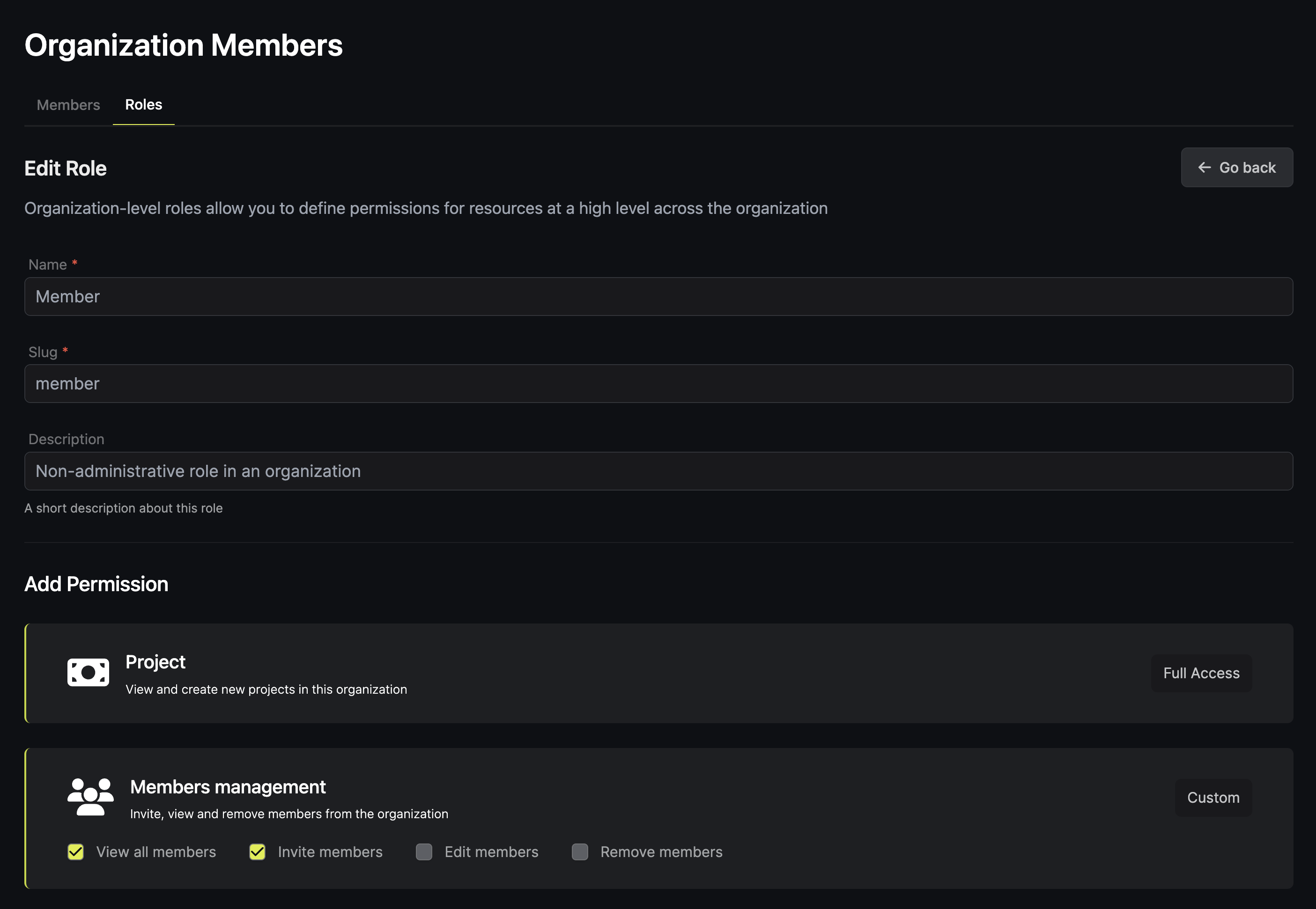
## Project-level access controls
By default, every user in a project is either a **viewer**, **developer**, or an **admin**. Each of these roles comes with a varying access to different features and resources inside projects.
As such:
* **Admin**: This role enables identities to have access to all environments, folders, secrets, and actions within the project.
* **Developers**: This role restricts identities from performing project control actions, updating Approval Workflow policies, managing roles/members, and more.
* **Viewer**: The most limiting bulit-in role on the project level – it forbids user and machine identities to perform any action and rather shows them in the read-only mode.
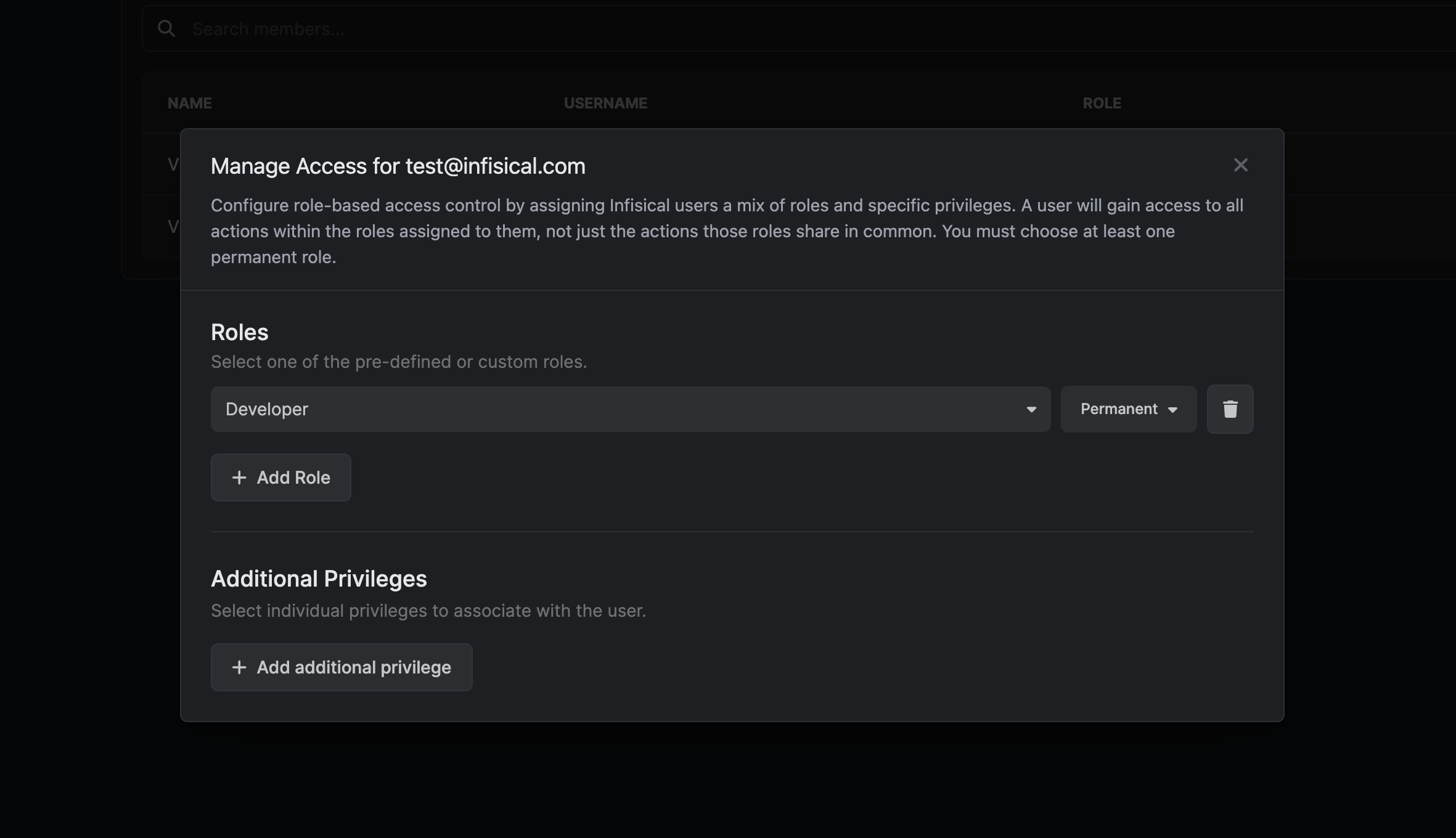
## Creating custom roles
By creating custom roles, you are able to adjust permissions to the needs of your organization. This can be useful for:
* Creating superadmin roles, roles specific to SRE engineers, etc.
* Restricting access of users to specific secrets, folders, and environments.
* Embedding these specific roles into [Approval Workflow policies](/documentation/platform/pr-workflows).
It is worth noting that users are able to assume multiple built-in and custom roles. A user will gain access to all actions within the roles assigned to them, not just the actions those roles share in common.
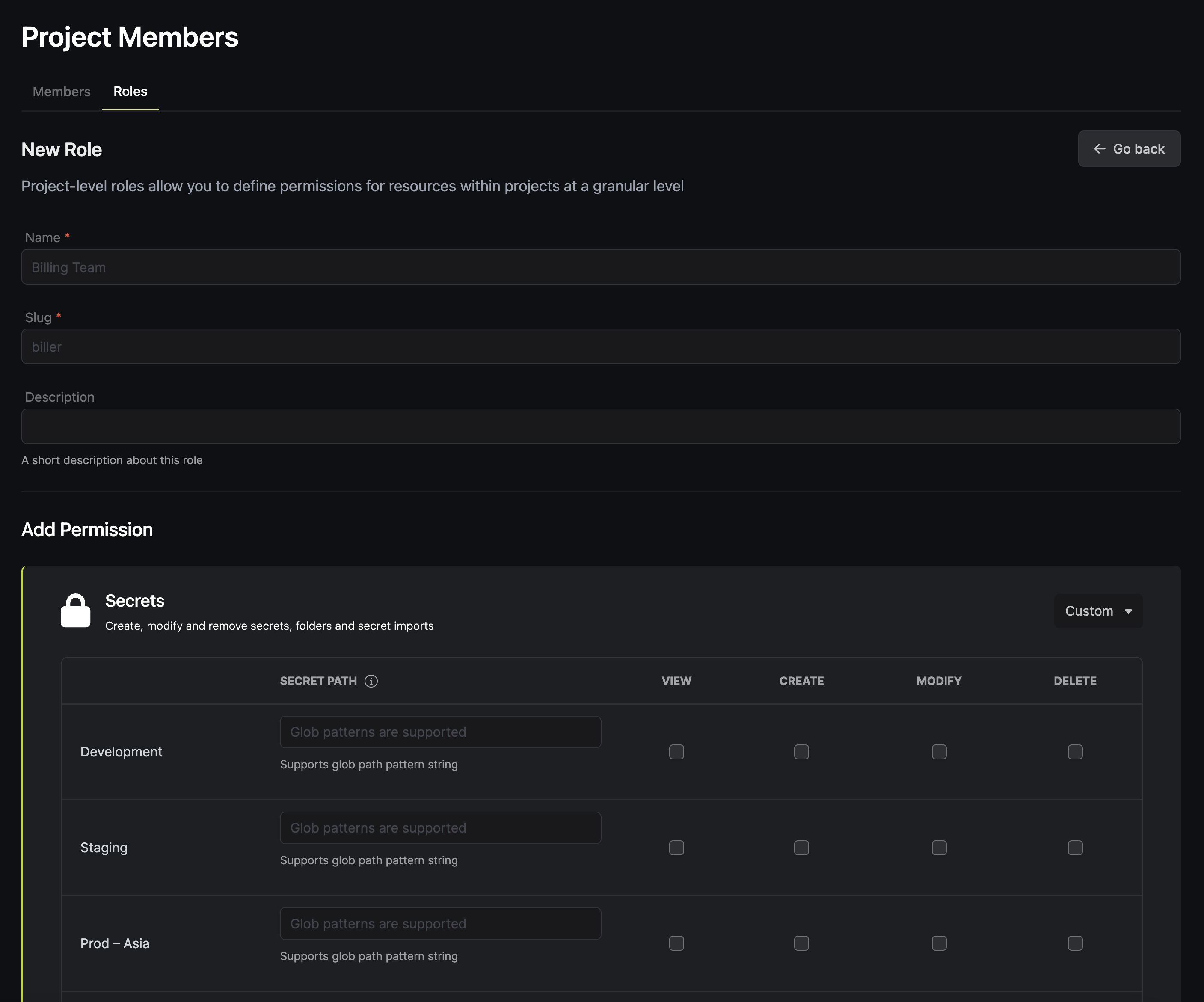
# Temporary Access
Learn how to set up timed access to sensitive resources for user and machine identities.
Certain environments and secrets are so sensitive that it is recommended to not give any user permanent access to those. For such use cases, Infisical supports the functionality of **Temporary Access** provisioning.
To provision temporary access through Web UI:
1. Click on the `Edit` button next to the set of roles for user or identities.
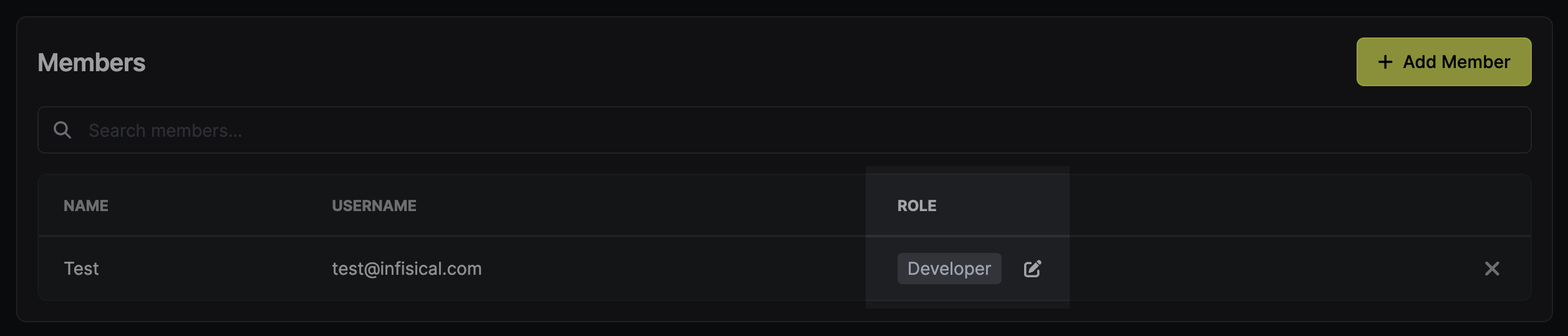
2. Click `Permanent` next to the role or specific privilege that you want to make temporary.
3. Specify the duration of remporary access (e.g., `1m`, `2h`, `3d`).
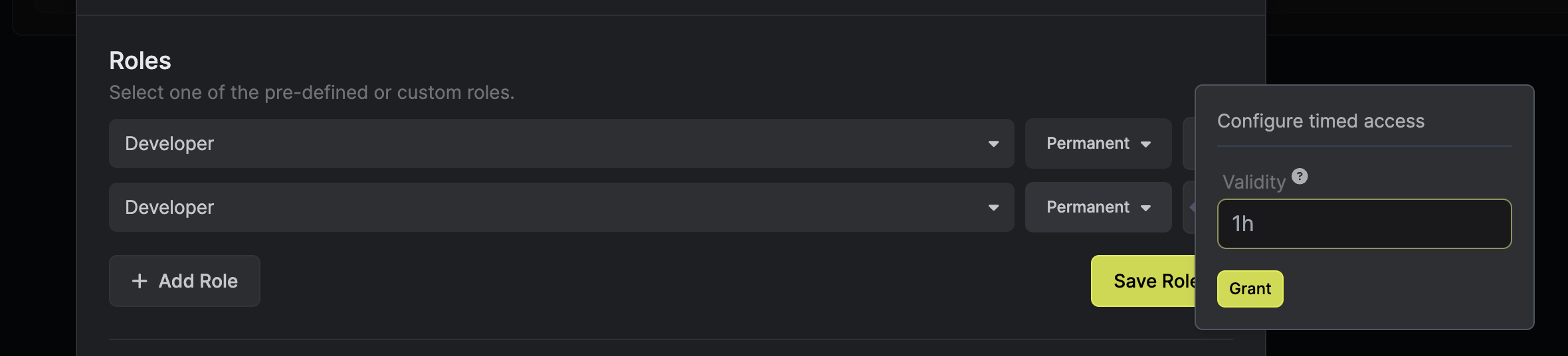
4. Click `Grant`.
5. Click the corresponding `Save` button to enable remporary access.
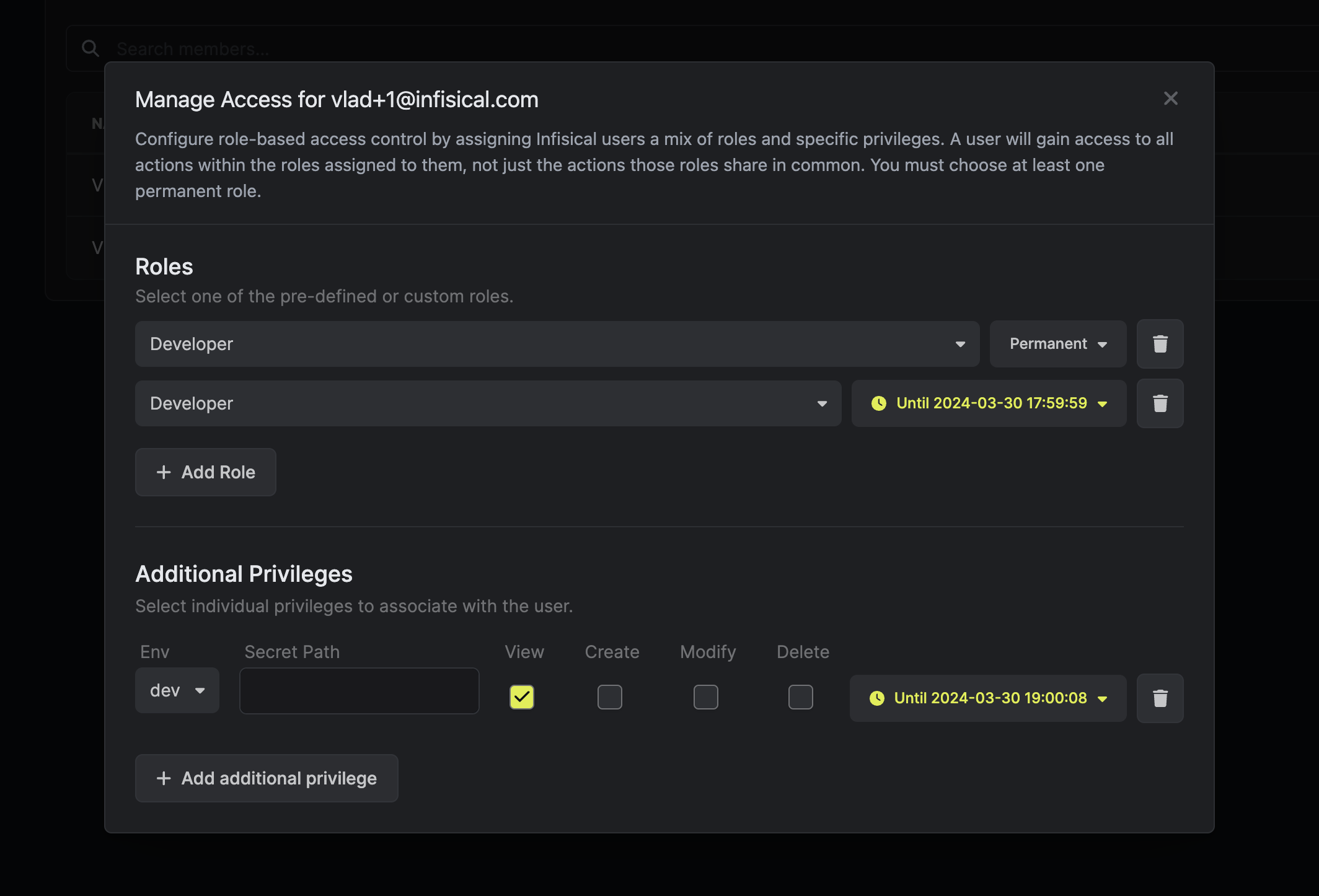
Every user and machine identity should always have at least one permanent role attached to it.
# Organization Admin Console
View and manage resources across your organization
The Organization Admin Console can only be accessed by organization members with admin status.
## Accessing the Organization Admin Console
On the sidebar, tap on your initials to access the settings dropdown and press the **Organization Admin Console** option.
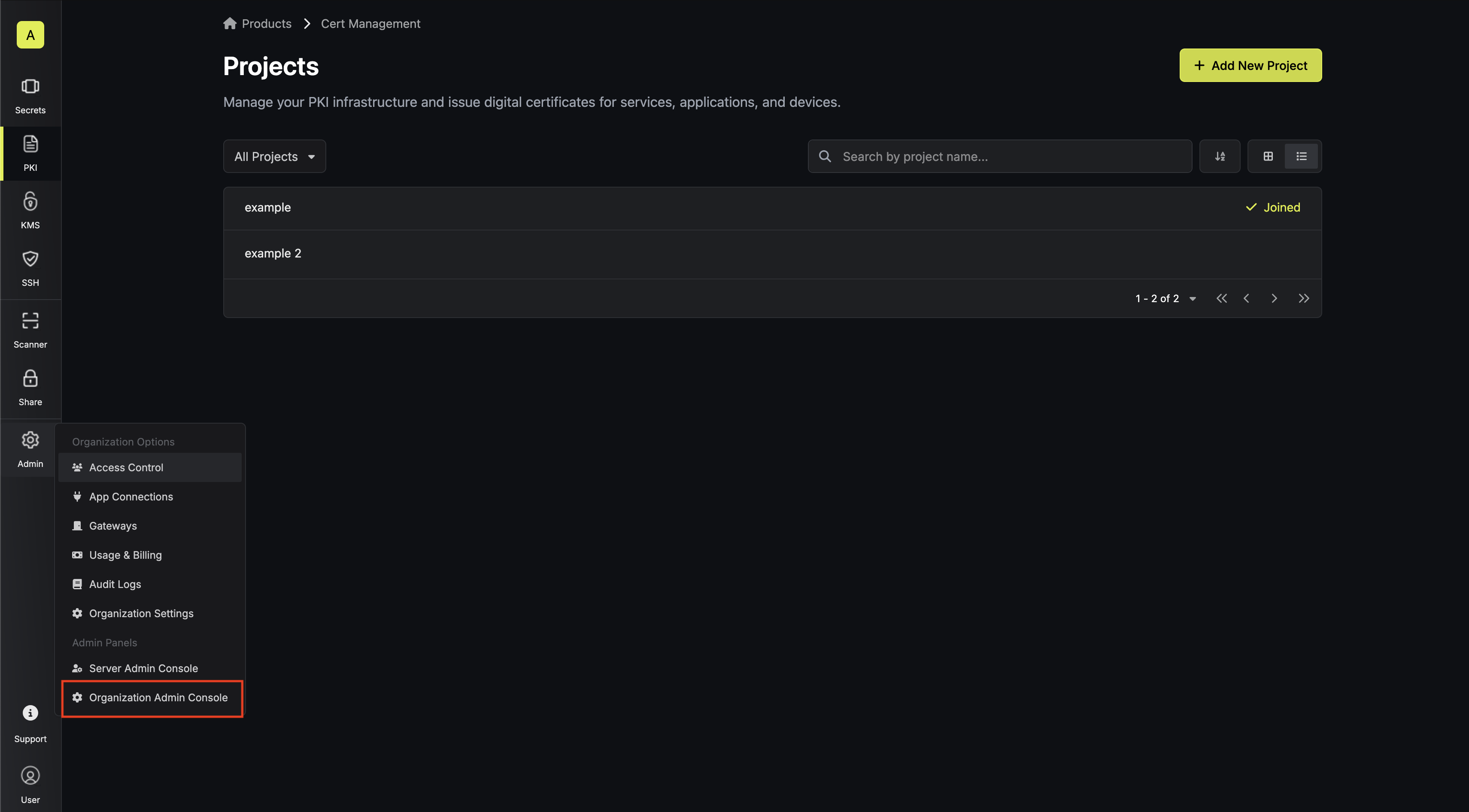
## Projects Tab
The Projects tab lists all the projects within your organization, including those which you are not a member of. You can easily filter projects by name or slug using the search bar.
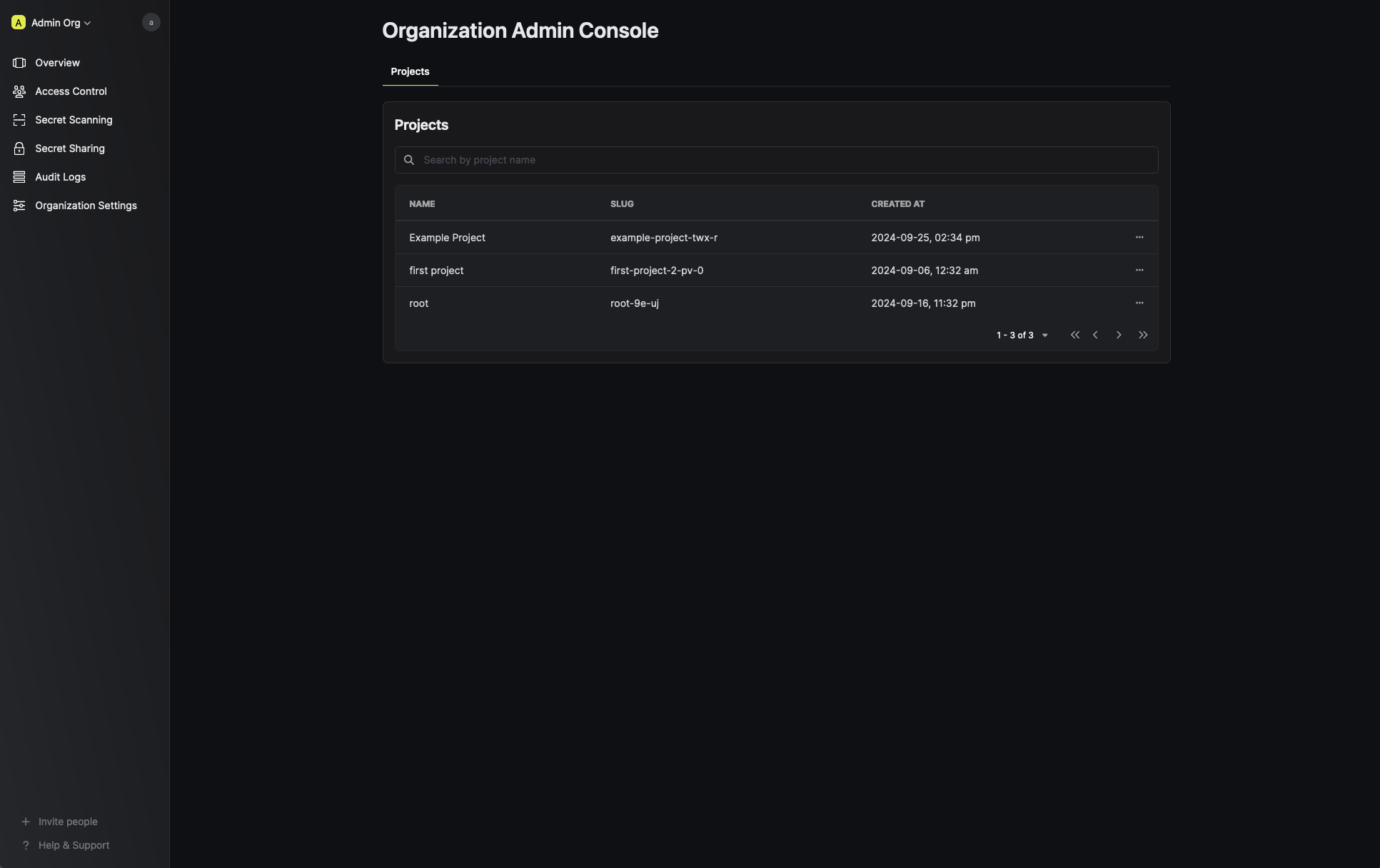
### Accessing a Project in Your Organization
You can access a project that you are not a member of by tapping on the options menu of the project row and pressing the **Access** button.
Doing so will grant you admin permissions for the selected project and add you as a member.
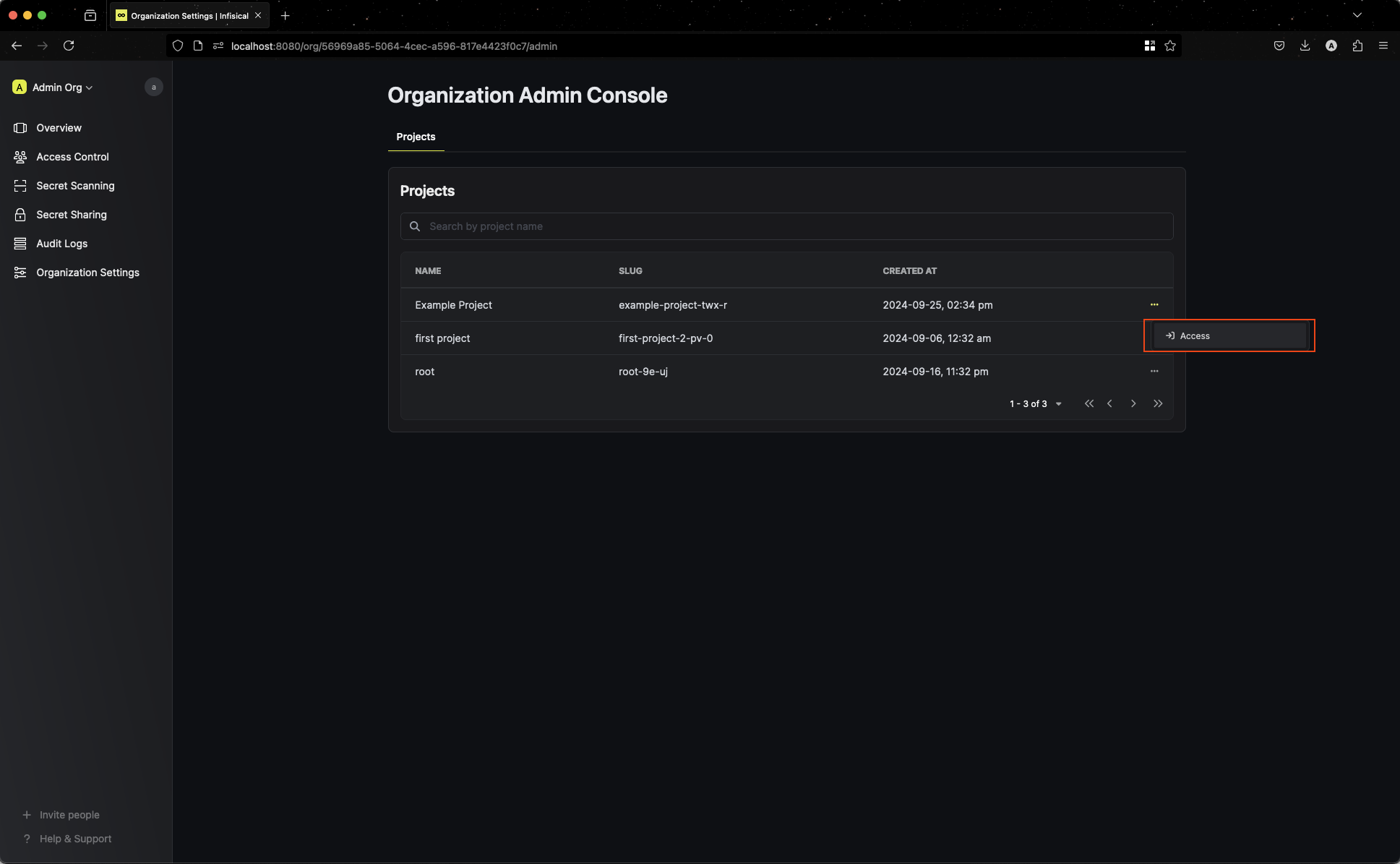
# null
Learn about Infisical's Admin Consoles
Infisical offers a server and organization level console for admins to customize their settings and manage various resources across the platform.
Configure and manage server related features.
View and access resources across your organization.
# Server Admin Console
Configure and manage server related features
The Server Admin Console provides **server administrators** with the ability to
customize settings and manage users for their entire Infisical instance.
The first user to setup an account on your Infisical instance is designated as the server administrator by default.
## Accessing the Server Admin Console
On the sidebar, tap on your initials to access the settings dropdown and press the **Server Admin Console** option.
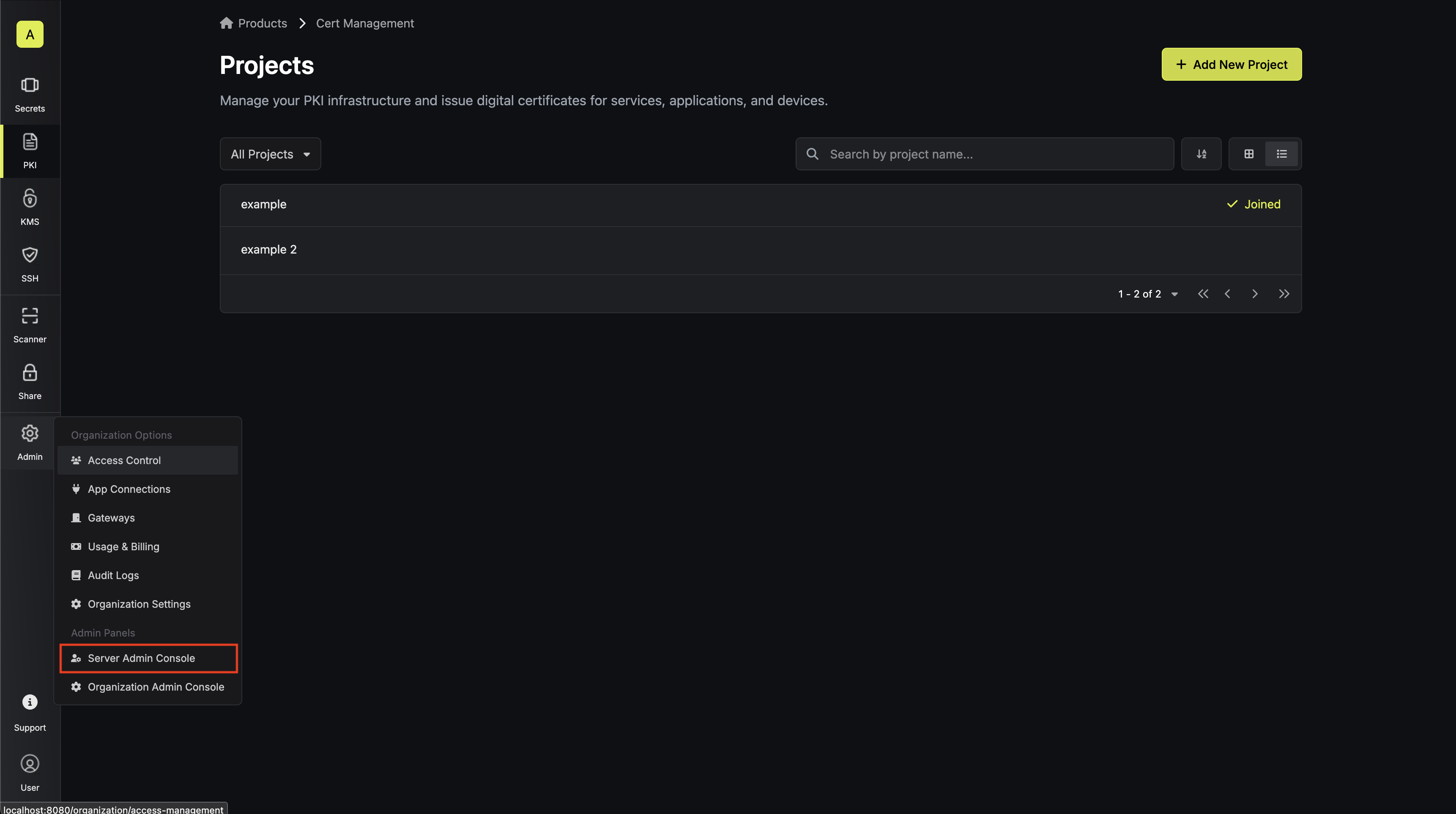
## General Tab
Configure general settings for your instance.
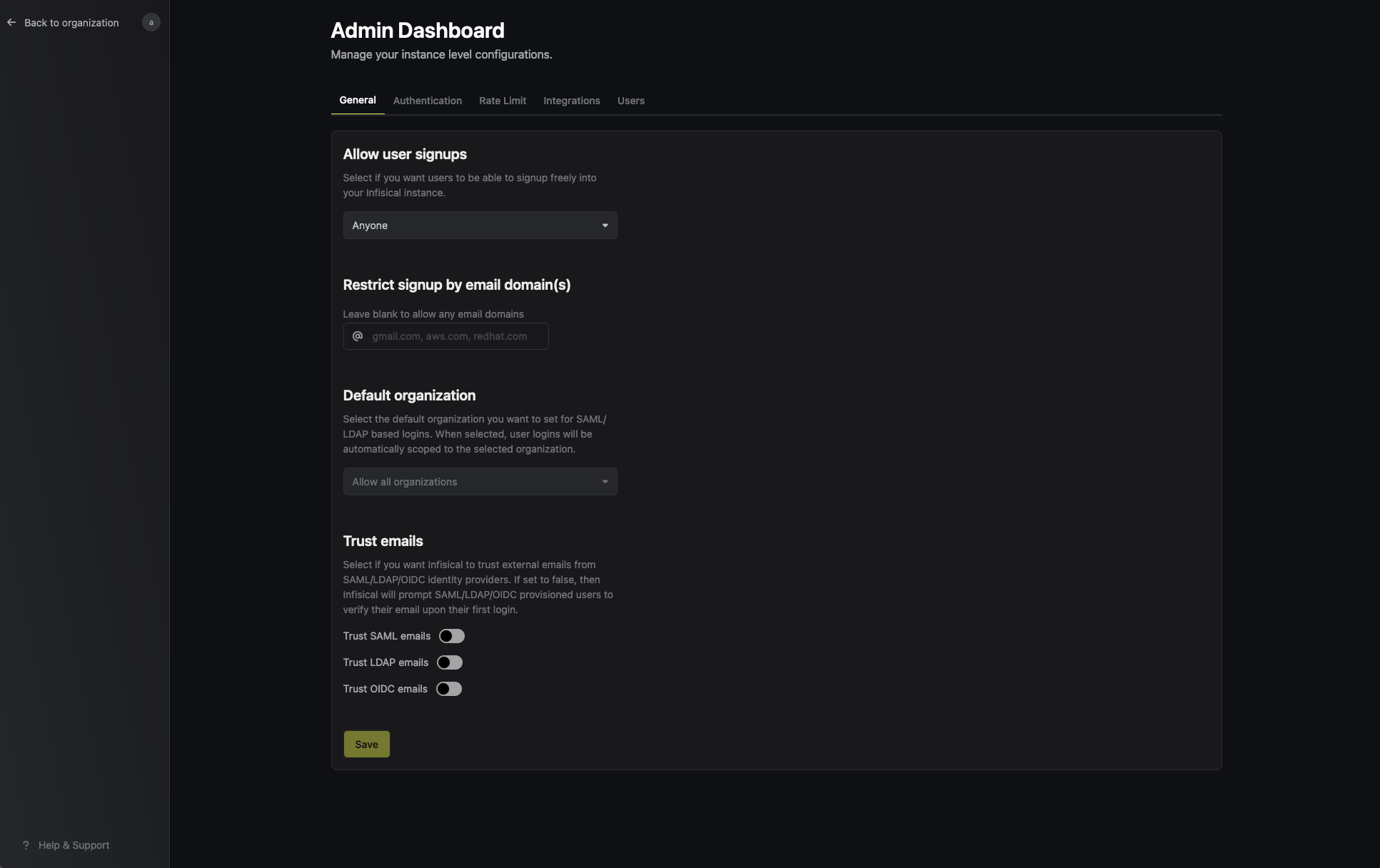
### Allow User Signups
User signups are enabled by default, allowing **Anyone** with access to your instance to sign up. This can alternatively be **Disabled** to prevent any users from signing up.
### Restrict Signup Domain
Signup can be restricted to users matching one or more email domains, such as your organization's domain, to control who has access to your instance.
### Default Organization
If you're using SAML/LDAP/OIDC for only one organization on your instance, you can specify a default organization to use at login to skip requiring users to manually enter the organization slug.
### Trust Emails
By default, users signing up through SAML/LDAP/OIDC will still need to verify their email address to prevent email spoofing. This requirement can be skipped by enabling the switch to trust logins through the respective method.
## Authentication Tab
From this tab, you can configure which login methods are enabled for your instance.
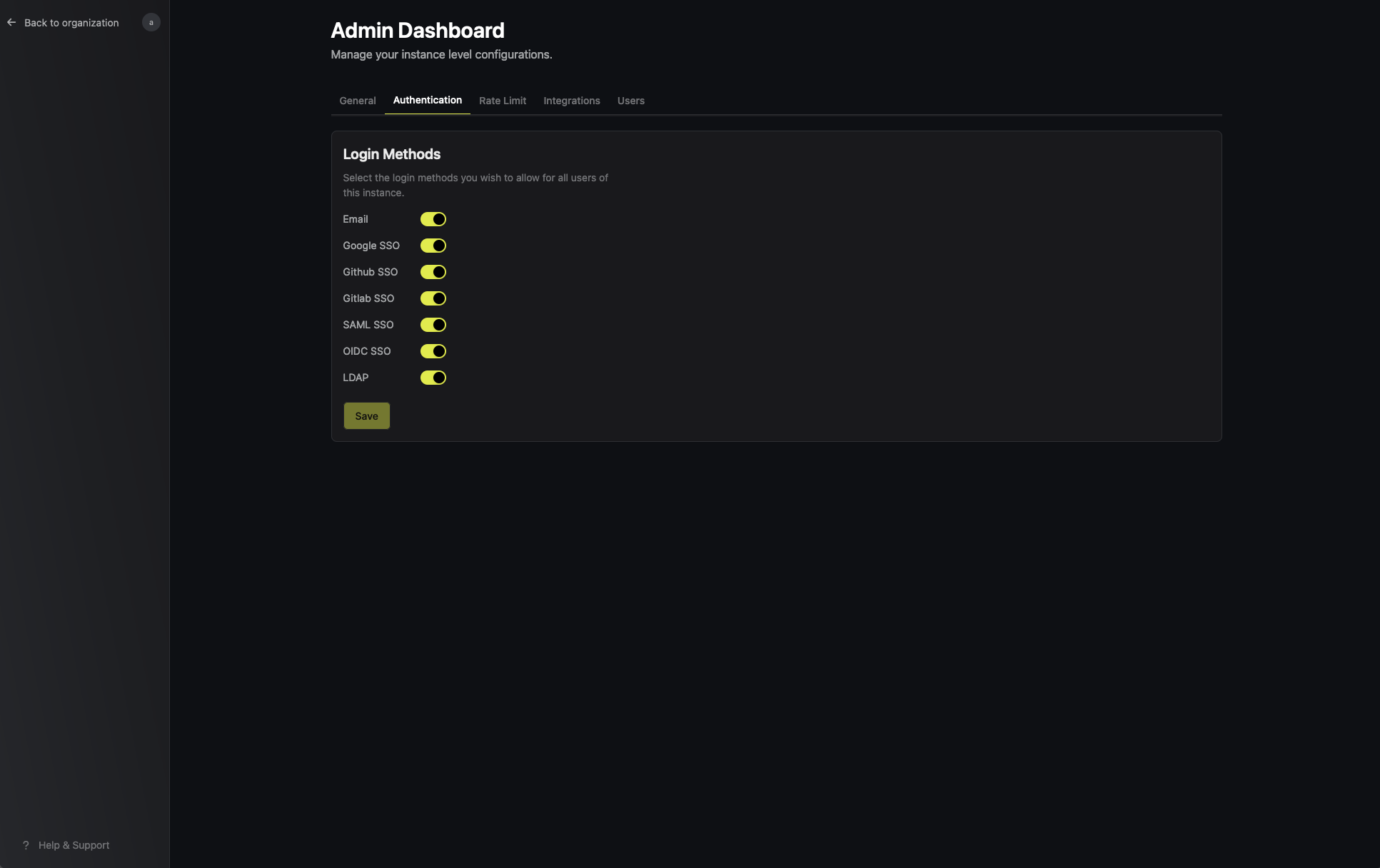
## Rate Limit Tab
This tab allows you to set various rate limits for your Infisical instance. You do not need to redeploy when making changes to rate limits as these will be propagated automatically.
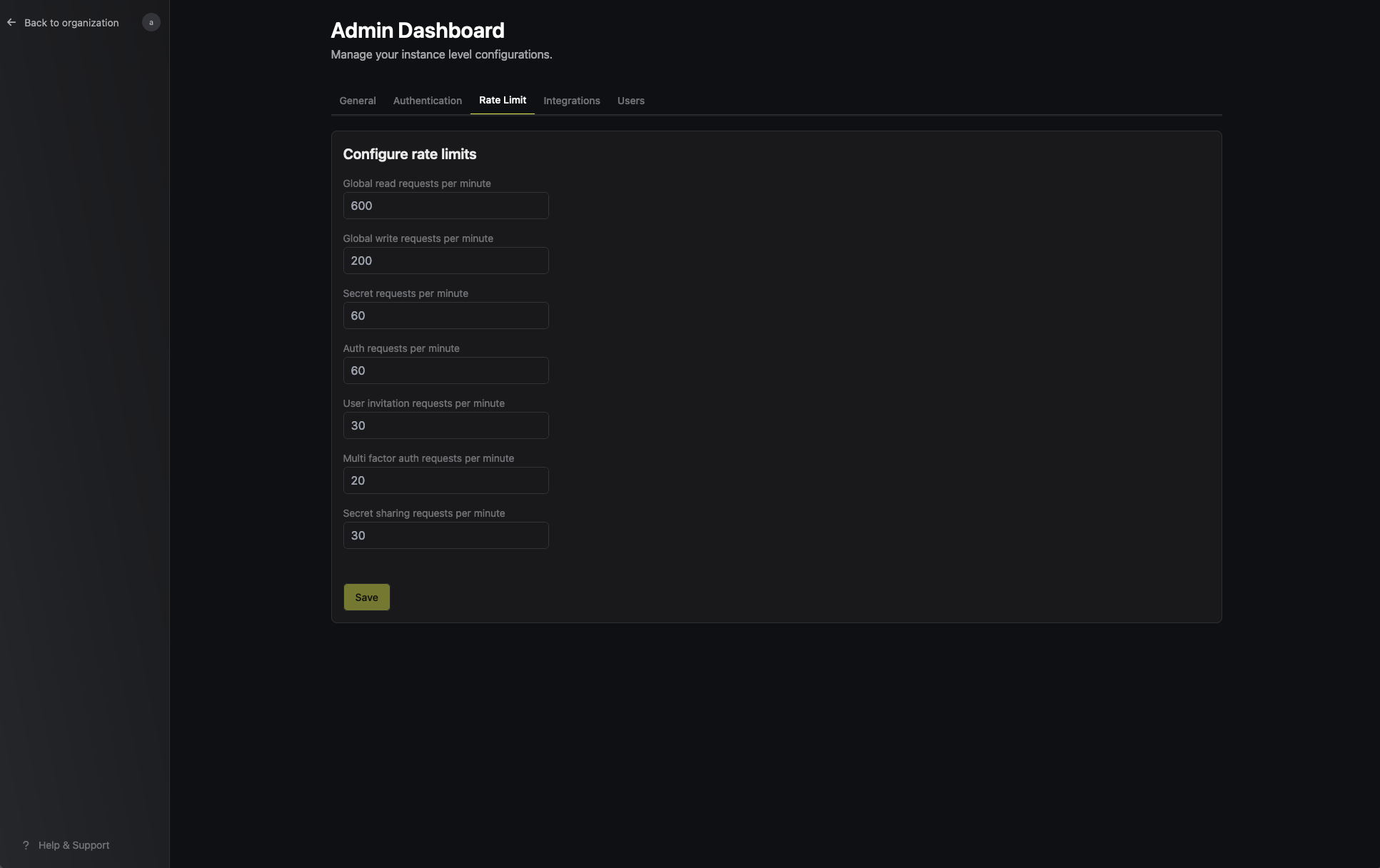
Note that rate limit configuration is a paid feature. Please contact [sales@infisical.com](mailto:sales@infisical.com) to purchase a license for its use.
## User Management Tab
From this tab, you can view all the users who have signed up for your instance. You can search for users using the search bar and remove them from your instance by pressing the **X** button on their respective row.
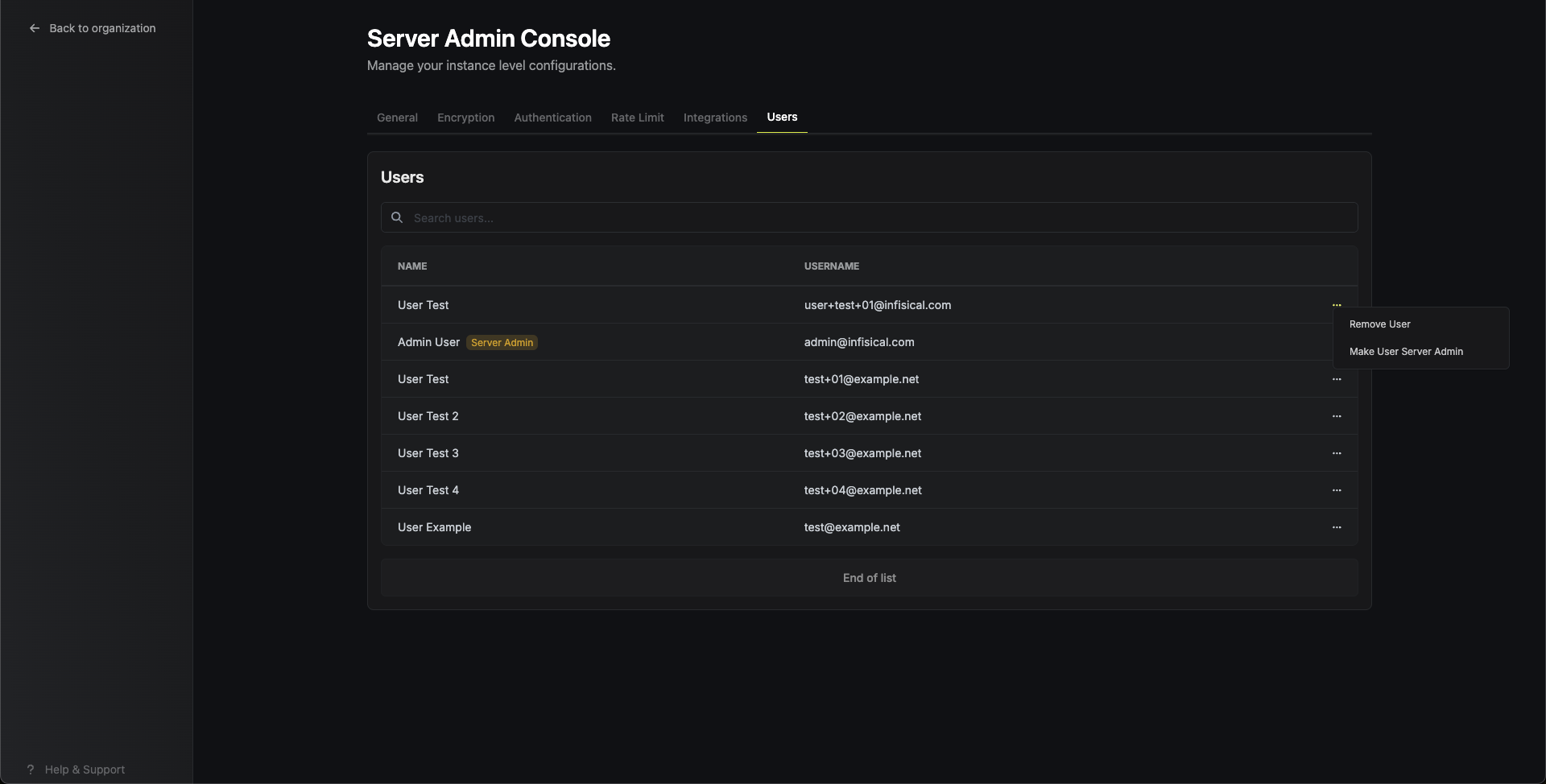
Note that rate limit configuration is a paid feature. Please contact [sales@infisical.com](mailto:sales@infisical.com) to purchase a license for its use.
# Audit Log Streams
Learn how to stream Infisical Audit Logs to external logging providers.
Audit log streams is a paid feature.
If you're using Infisical Cloud, then it is available under the **Enterprise Tier**. If you're self-hosting Infisical,
then you should contact [team@infisical.com](mailto:team@infisical.com) to purchase an enterprise license to use it.
Infisical Audit Log Streaming enables you to transmit your organization's Audit Logs to external logging providers for monitoring and analysis.
The logs are formatted in JSON, requiring your logging provider to support JSON-based log parsing.
## Overview
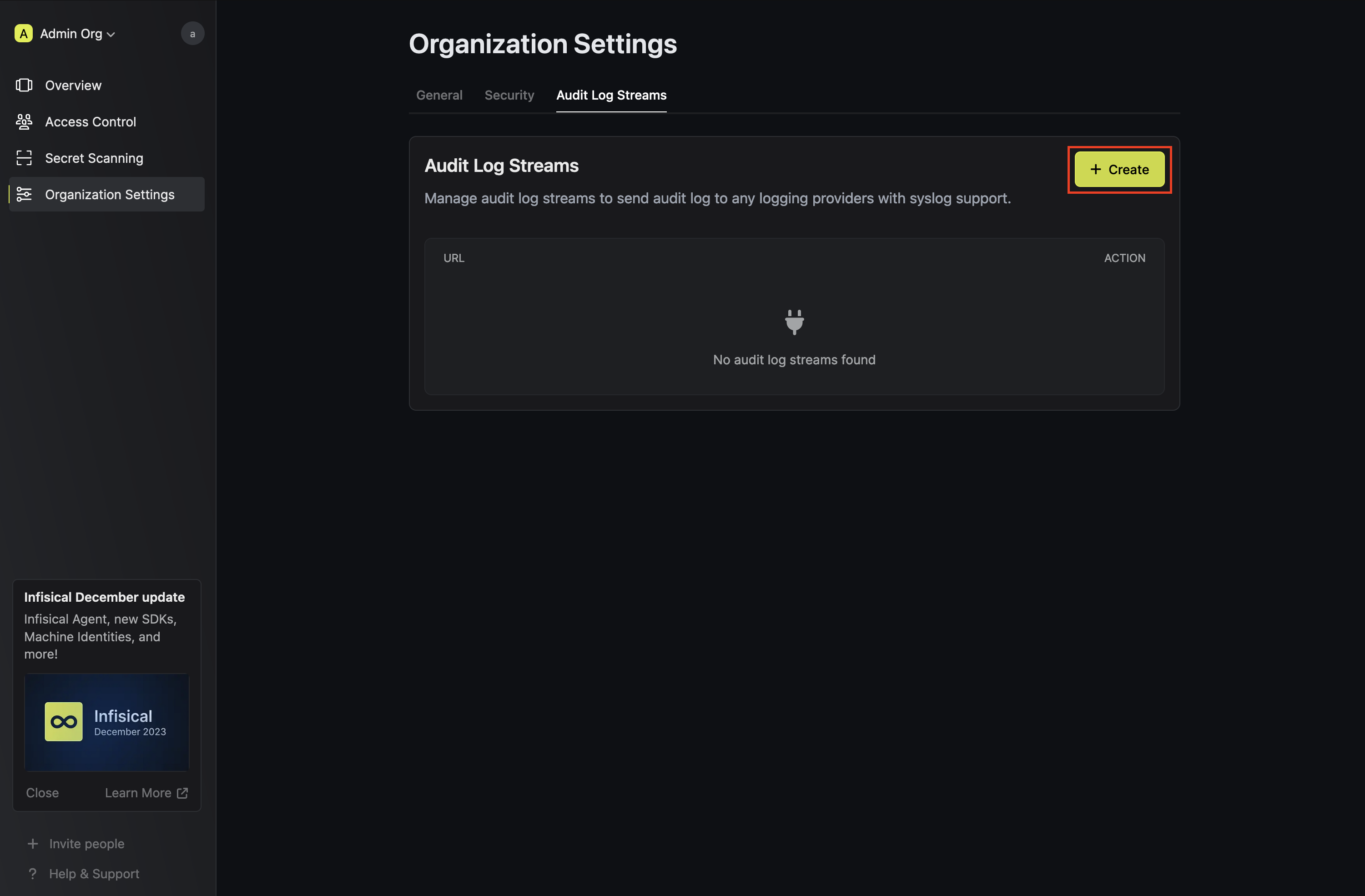
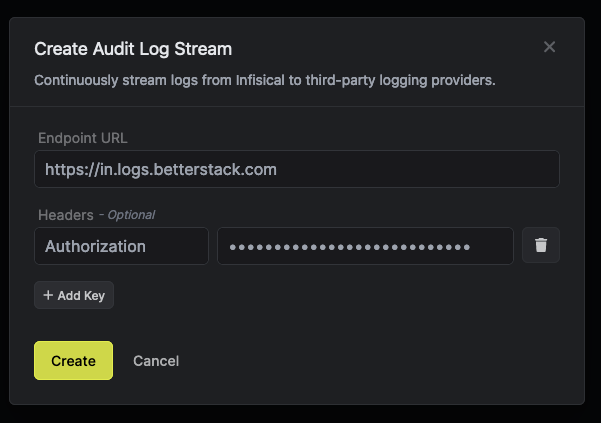
Provide the following values
The HTTPS endpoint URL of the logging provider that collects the JSON stream.
The HTTP headers for the logging provider for identification and authentication.
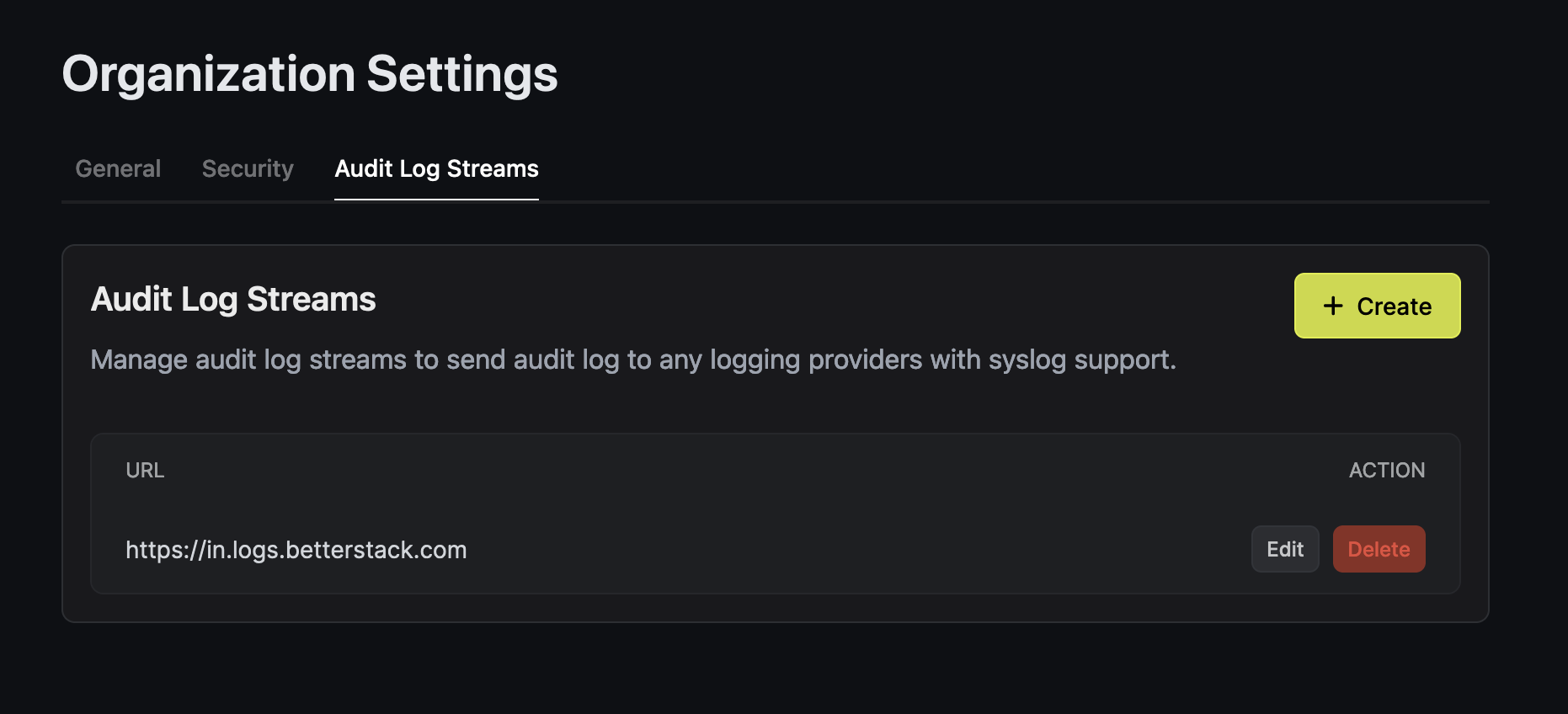
Your Audit Logs are now ready to be streamed.
## Example Providers
### Better Stack
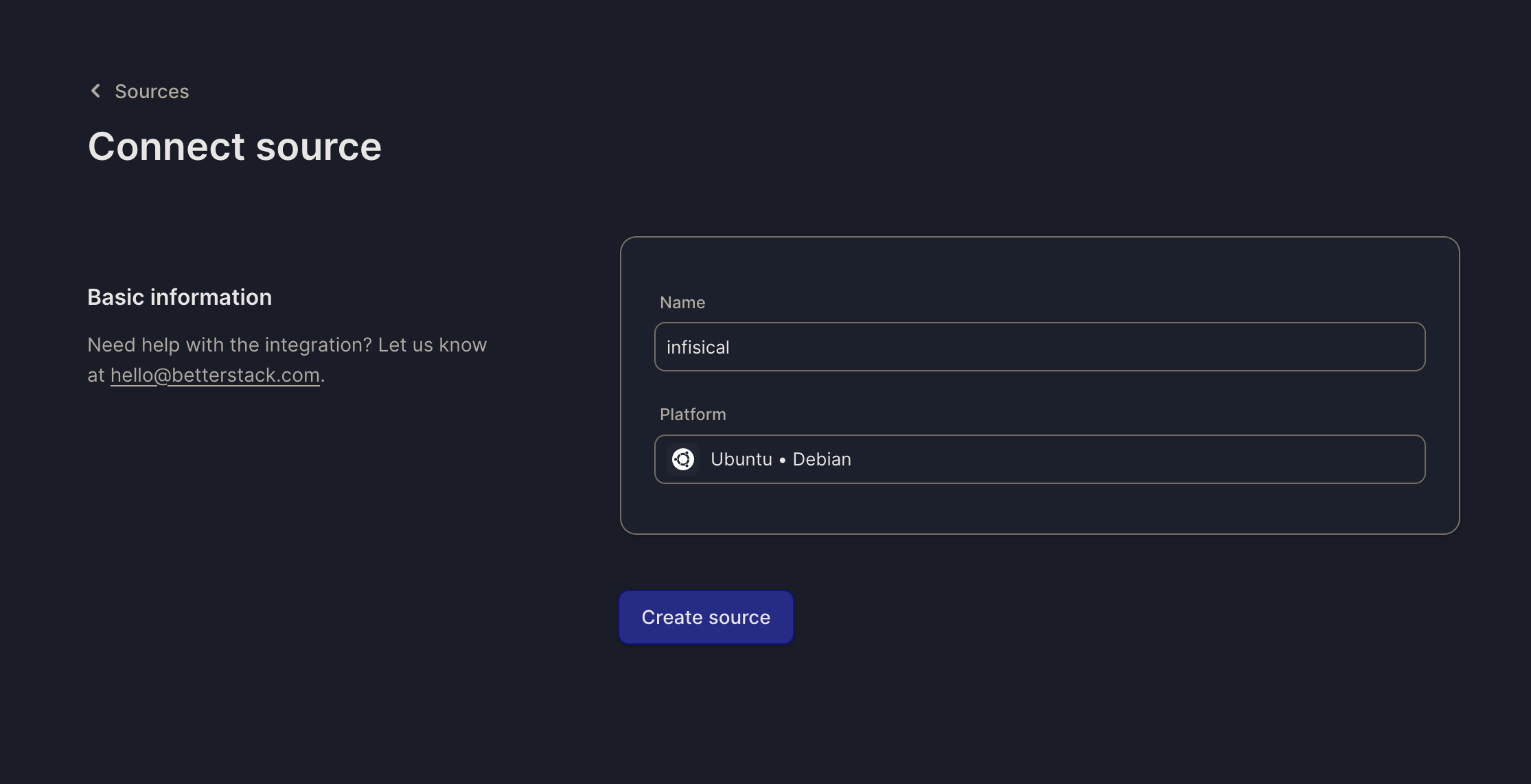
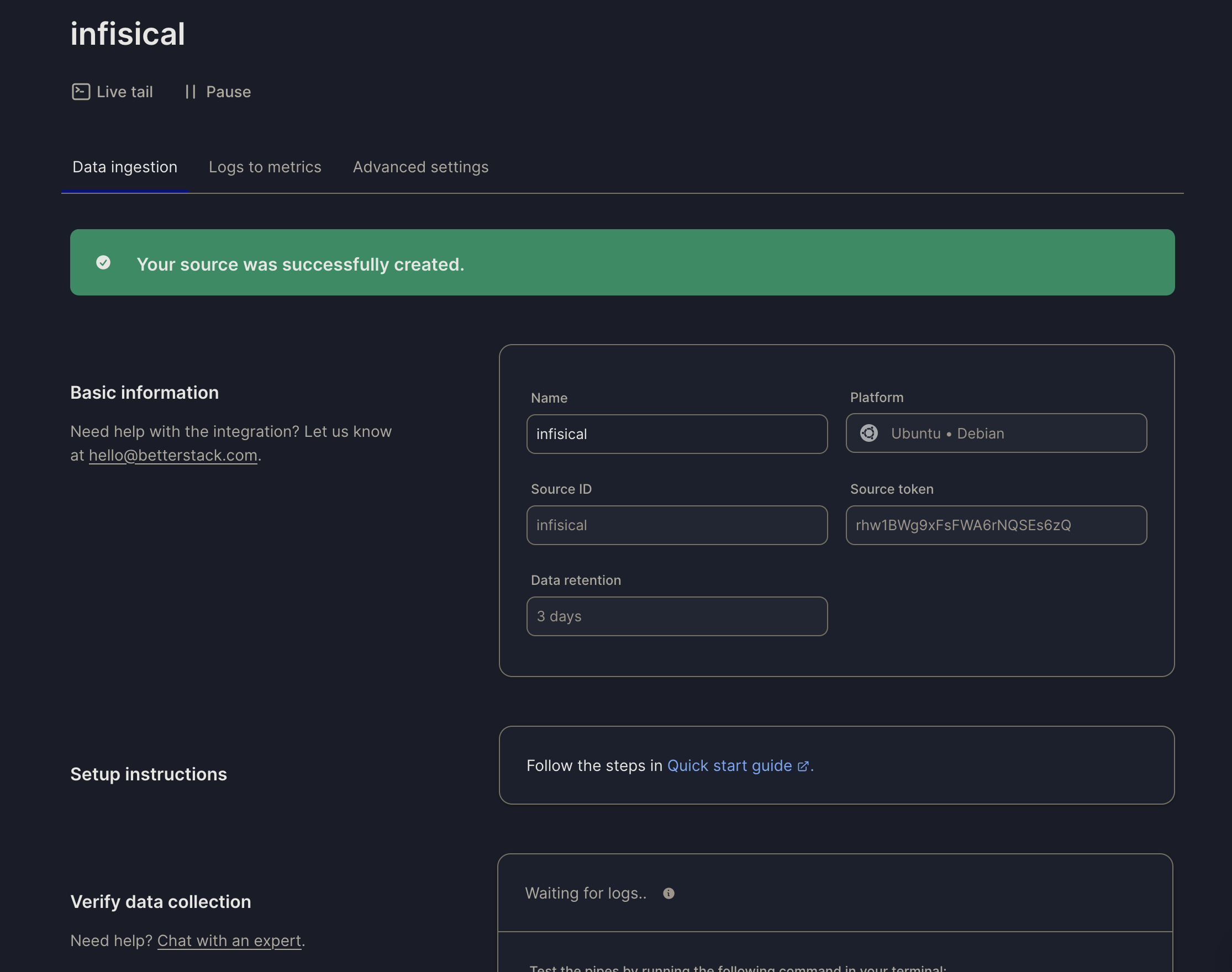
1. Copy the **endpoint** from Better Stack to the **Endpoint URL** field.
2. Create a new header with key **Authorization** and set the value as **Bearer \**.
### Datadog
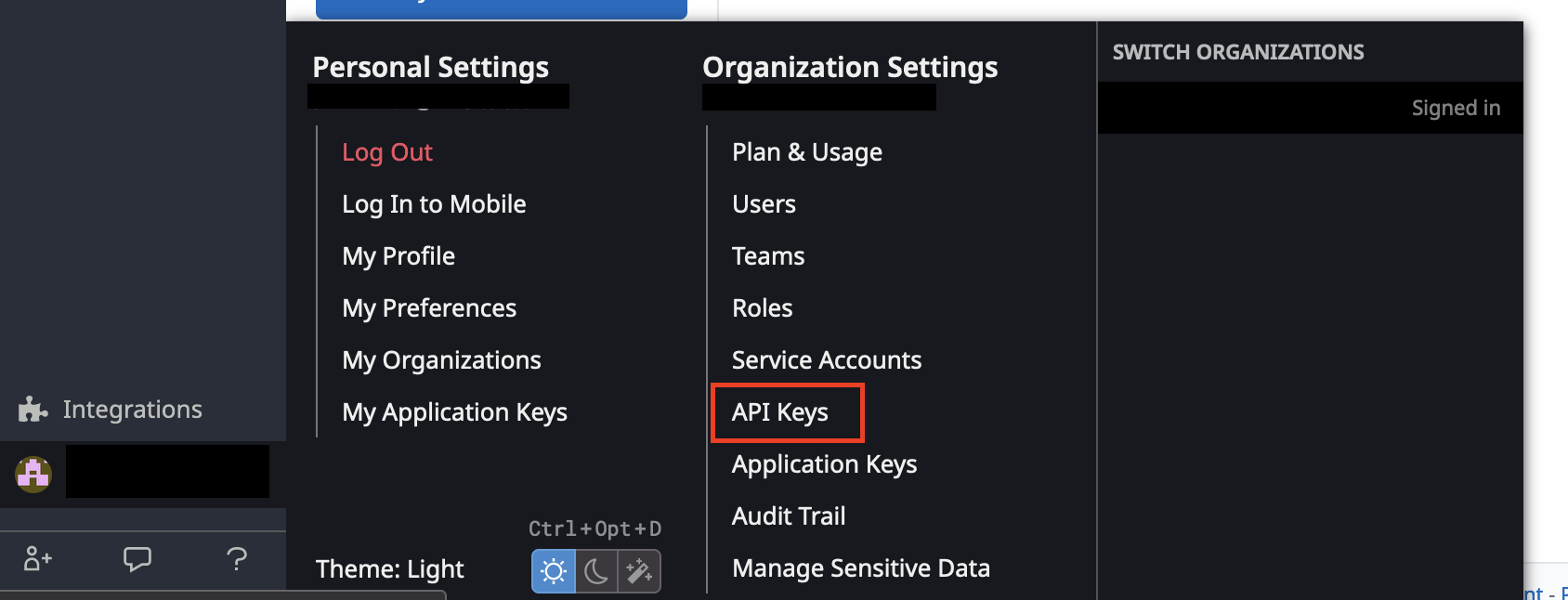
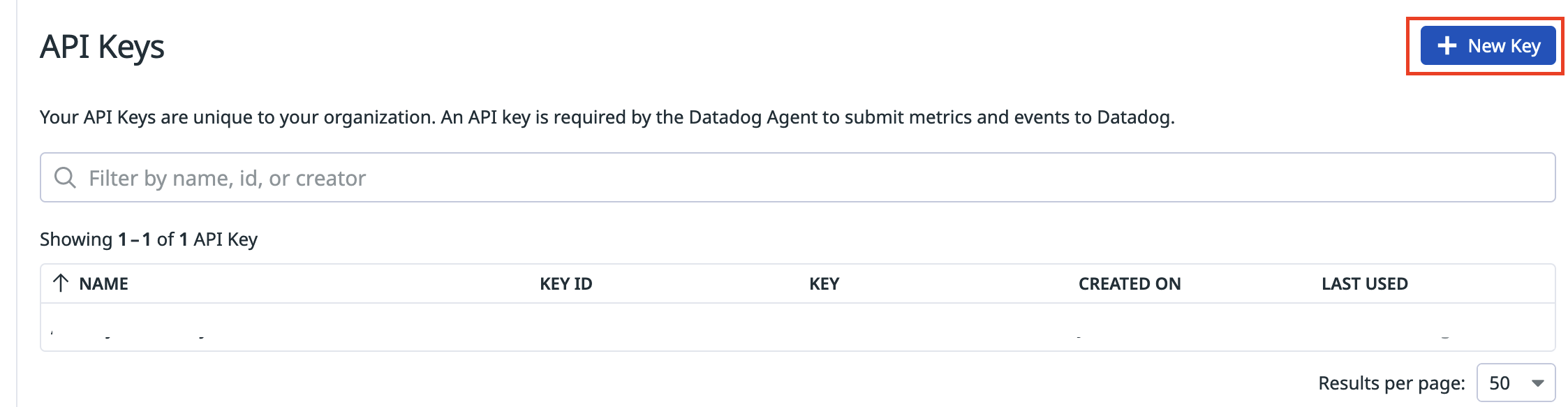
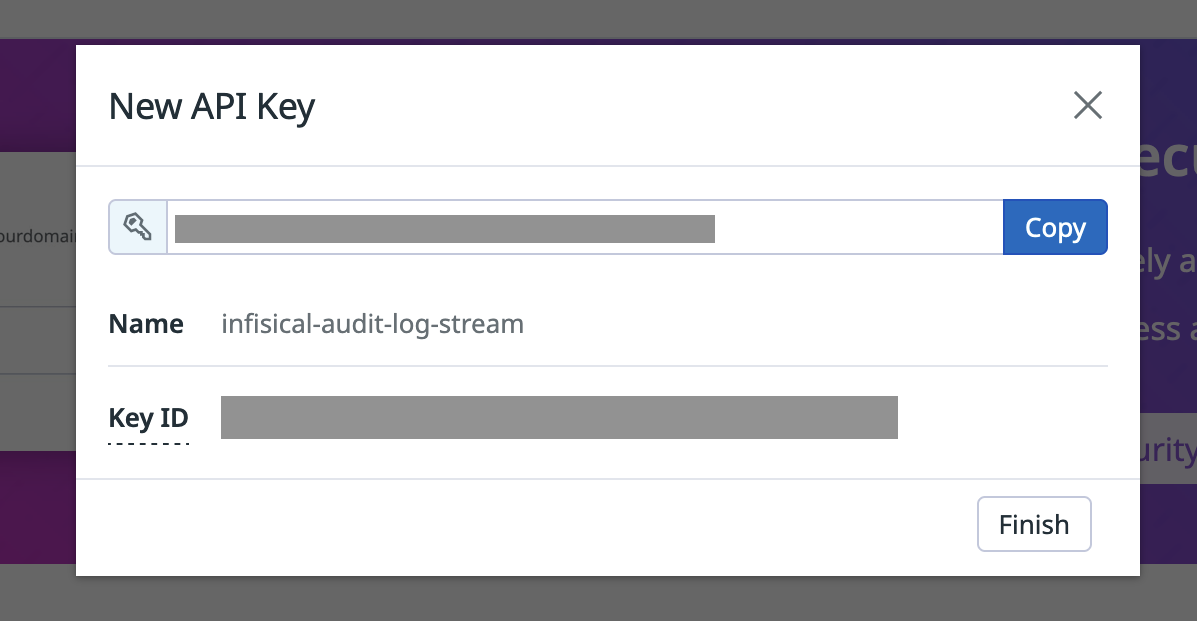
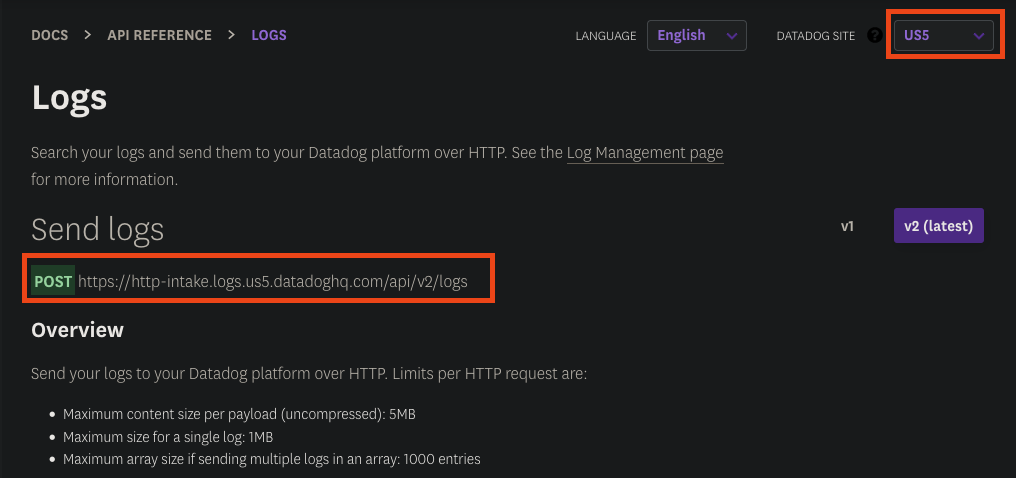
1. Navigate to the [Datadog Send Logs API documentation](https://docs.datadoghq.com/api/latest/logs/?code-lang=curl\&site=us5#send-logs).
2. Pick your Datadog account region.
3. Obtain your Datadog logging endpoint URL.
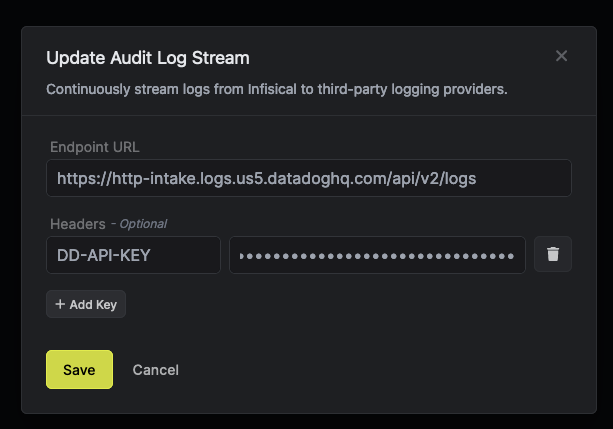
1. Copy the **logging endpoint** from Datadog to the **Endpoint URL** field.
2. Copy the **API Key** from previous step
3. Create a new header with key **DD-API-KEY** and set the value as **API Key**.
# Stream to Non-HTTP providers
How to stream Infisical Audit Logs to Non-HTTP log providers
Audit log streams is a paid feature.
If you're using Infisical Cloud, then it is available under the **Enterprise Tier**. If you're self-hosting Infisical,
then you should contact [team@infisical.com](mailto:team@infisical.com) to purchase an enterprise license to use it.
This guide will demonstrate how you can send Infisical Audit log streams to storage solutions that do not support direct HTTP-based ingestion, such as AWS S3.
To achieve this, you will learn how you can use a log collector like Fluent Bit to capture and forward logs from Infisical to non-HTTP storage options.
In this pattern, Fluent Bit acts as an intermediary, accepting HTTP log streams from Infisical and transforming them into a format that can be sent to your desired storage provider.
## Overview
Log collectors are tools used to collect, analyze, transform, and send logs to storage.
For the purposes of this guide, we will use [Fluent Bit](https://fluentbit.io) as our log collector and send logs from Infisical to AWS S3.
However, this is just a example and you can use any log collector of your choice.
## Deploy Fluent Bit
You can deploy Fluent Bit in one of two ways:
1. As a sidecar to your self-hosted Infisical instance
2. As a standalone service in any deployment/compute service (e.g., AWS EC2, ECS, or GCP Compute Engine)
To view all deployment methods, visit the [Fluent Bit Getting Started guide](https://docs.fluentbit.io/manual/installation/getting-started-with-fluent-bit).
## Configure Fluent Bit
To set up Fluent Bit, you'll need to provide a configuration file that establishes an HTTP listener and configures an output to send JSON data to your chosen storage solution.
The following Fluent Bit configuration sets up an HTTP listener on port `8888` and sends logs to AWS S3:
```ini
[SERVICE]
Flush 1
Log_Level info
Daemon off
[INPUT]
Name http
Listen 0.0.0.0
Port 8888
[OUTPUT]
Name s3
Match *
bucket my-bucket
region us-west-2
total_file_size 50M
use_put_object Off
compression gzip
s3_key_format /$TAG/%Y/%m/%d/%H_%M_%S.gz
```
### Connecting Infisical Audit Log Stream
Once Fluent Bit is set up and configured, you can point the Infisical [audit log stream](/documentation/platform/audit-log-streams/audit-log-streams) to Fluent Bit's HTTP listener, which will then forward the logs to your chosen provider.
Using this pattern, you are able to send Infisical Audit logs to various providers that do not support HTTP based log ingestion by default.
# Overview
Track evert event action performed within Infisical projects.
Note that Audit Logs is a paid feature.
If you're using Infisical Cloud, then it is available under the **Pro**,
and **Enterprise Tier** with varying retention periods. If you're self-hosting Infisical,
then you should contact [sales@infisical.com](mailto:sales@infisical.com) to purchase an enterprise license to use it.
Infisical provides audit logs for security and compliance teams to monitor information access.
With the Audit Log functionality, teams can:
* **Track** 40+ different events;
* **Filter** audit logs by event, actor, source, date or any combination of these filters;
* **Inspect** extensive metadata in the event of any suspicious activity or incident review.
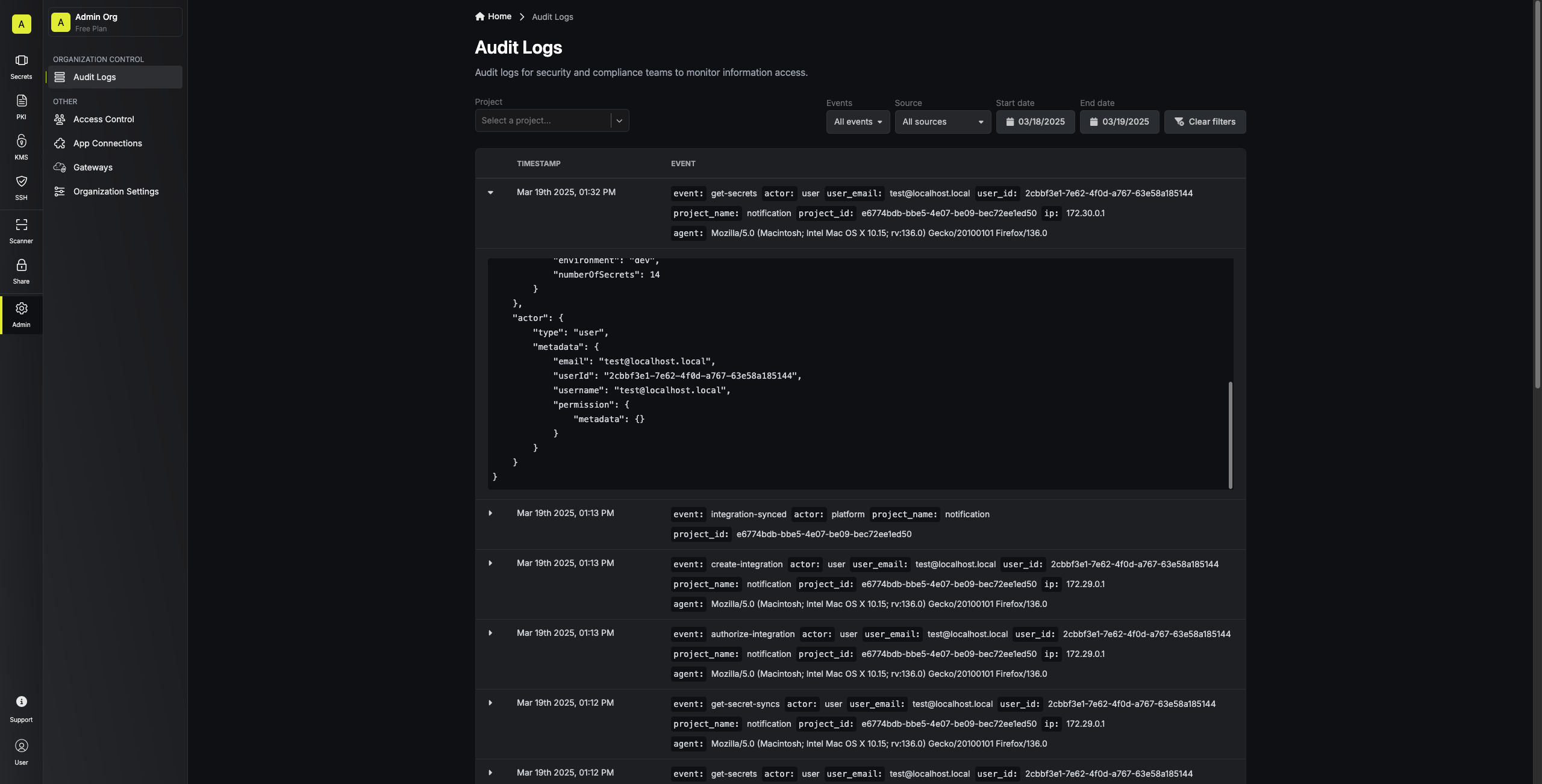
Each log contains the following data:
* **Event**: The underlying action such as create, list, read, update, or delete secret(s).
* **Actor**: The entity responsible for performing or causing the event; this can be a user or service.
* **Timestamp**: The date and time at which point the event occurred.
* **Source** (User agent + IP): The software (user agent) and network address (IP) from which the event was initiated.
* **Metadata**: Additional data to provide context for each event. For example, this could be the path at which a secret was fetched from etc.
# Email and Password
Learn how to authenticate into Infisical with email and password.
**Email and Password** is the most common authentication method that can be used by user identities for authentication into Web Dashboard and Infisical CLI. It is recommended to utilize [Multi-factor Authentication](/documentation/platform/mfa) in addition to it.
It is currently possible to use the **Email and Password** auth method to authenticate into the Web Dashboard and Infisical CLI.
Every **Email and Password** is accompanied by an emergency kit given to users during signup. If the password is lost or forgotten, emergency kit is only way to retrieve the access to your account. It is possible to generate a new emergency kit with the following steps:
1. Open the `Personal Settings` menu.
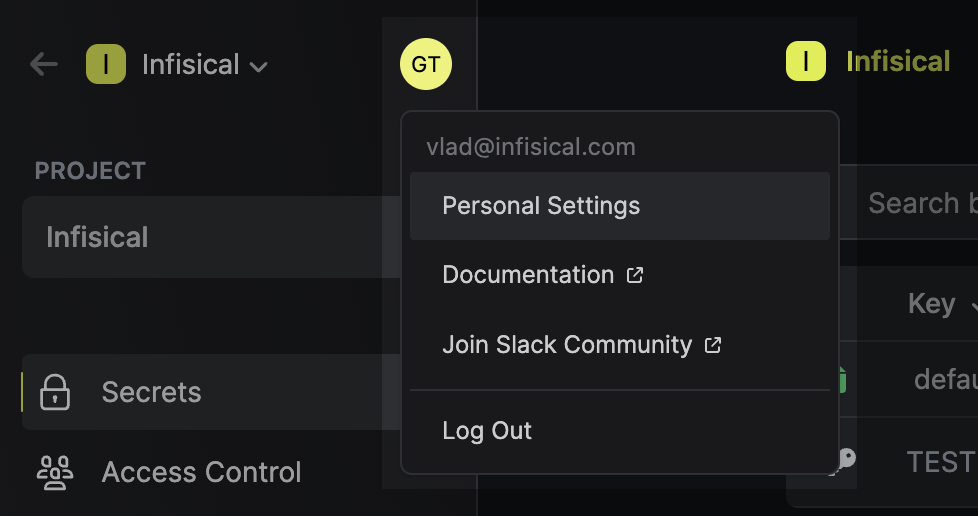
2. Scroll down to the `Emergency Kit` section.
3. Enter your current password and click `Save`.
# AWS ElastiCache
Learn how to dynamically generate AWS ElastiCache user credentials.
The Infisical AWS ElastiCache dynamic secret allows you to generate AWS ElastiCache credentials on demand based on configured role.
## Prerequisites
2. Create an AWS IAM user with the following permissions:
```json
{
"Version": "2012-10-17",
"Statement": [
{
"Sid": "",
"Effect": "Allow",
"Action": [
"elasticache:DescribeUsers",
"elasticache:ModifyUser",
"elasticache:CreateUser",
"elasticache:CreateUserGroup",
"elasticache:DeleteUser",
"elasticache:DescribeReplicationGroups",
"elasticache:DescribeUserGroups",
"elasticache:ModifyReplicationGroup",
"elasticache:ModifyUserGroup"
],
"Resource": "arn:aws:elasticache:::user:*"
}
]
}
```
3. Create an access key ID and secret access key for the user you created in the previous step. You will need these to configure the Infisical dynamic secret.
New leases may take up-to a couple of minutes before ElastiCache has the chance to complete their configuration.
It is recommended to use a retry strategy when establishing new ElastiCache connections.
This may prevent errors when trying to use a password that isn't yet live on the targeted ElastiCache cluster.
While a leasing is being created, you will be unable to create new leases for the same dynamic secret.
Please ensure that your ElastiCache cluster has transit encryption enabled and set to required. This is required for the dynamic secret to work.
## Set up Dynamic Secrets with AWS ElastiCache
Open the Secret Overview dashboard and select the environment in which you would like to add a dynamic secret.
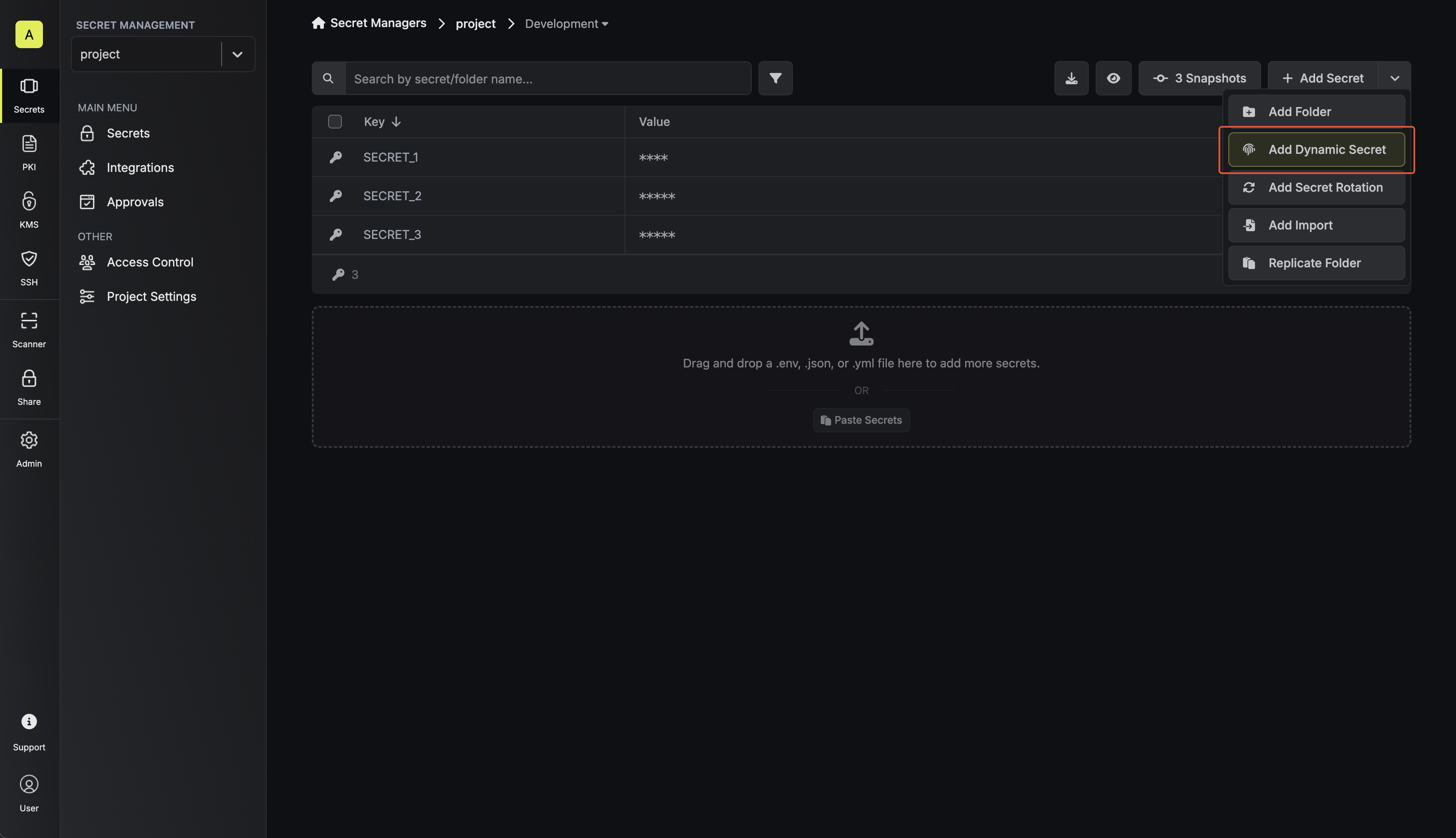
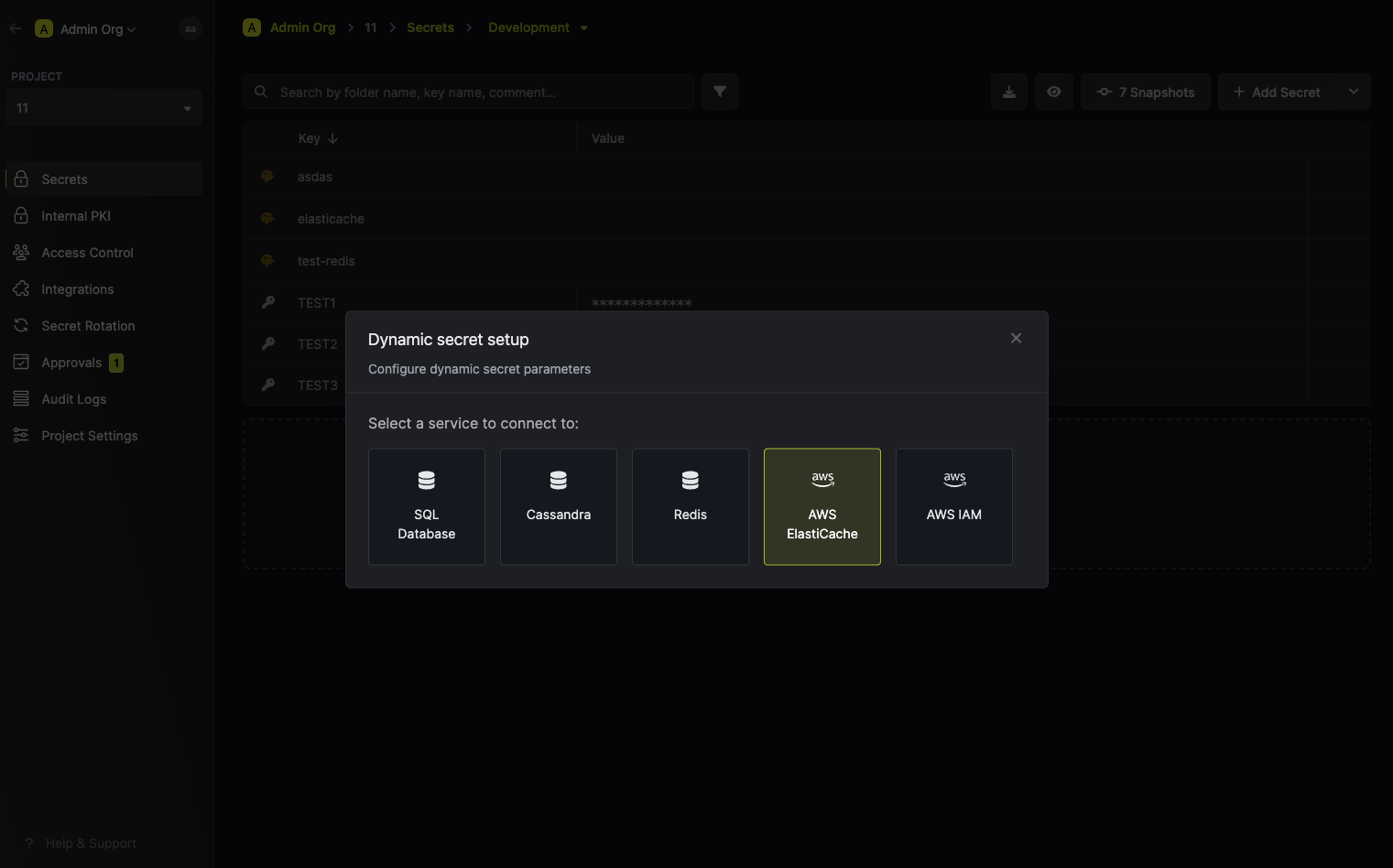
Name by which you want the secret to be referenced
Default time-to-live for a generated secret (it is possible to modify this value when a secret is generate)
Maximum time-to-live for a generated secret.
The region that the ElastiCache cluster is located in. *(e.g. us-east-1)*
This is the access key ID of the AWS IAM user you created in the prerequisites. This will be used to provision and manage the dynamic secret leases.
This is the secret access key of the AWS IAM user you created in the prerequisites. This will be used to provision and manage the dynamic secret leases.
A CA may be required if your DB requires it for incoming connections. This is often the case when connecting to a managed service.
If you want to provide specific privileges for the generated dynamic credentials, you can modify the ElastiCache statement to your needs. This is useful if you want to only give access to a specific table(s).
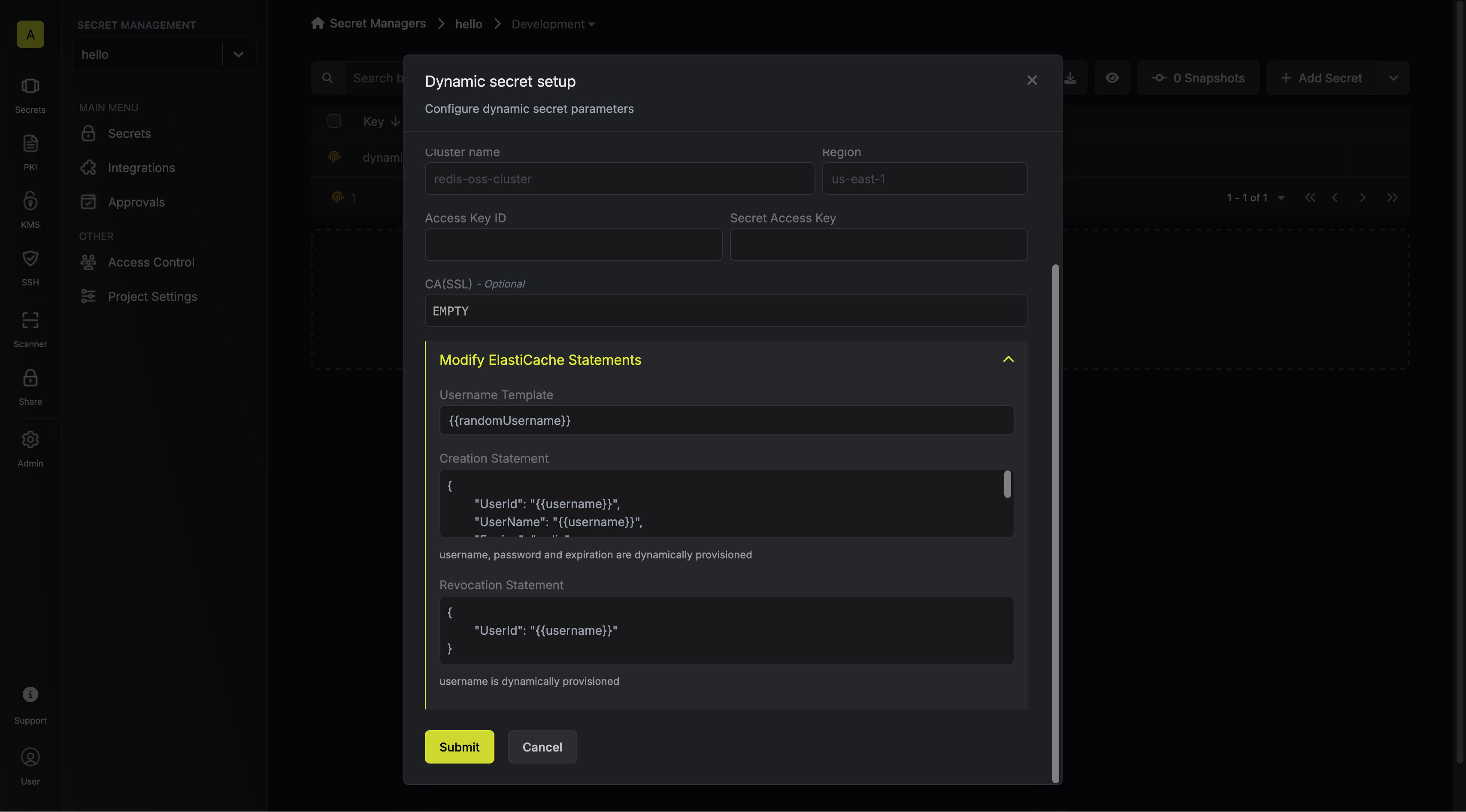
After submitting the form, you will see a dynamic secret created in the dashboard.
If this step fails, you may have to add the CA certificate.
Once you've successfully configured the dynamic secret, you're ready to generate on-demand credentials.
To do this, simply click on the 'Generate' button which appears when hovering over the dynamic secret item.
Alternatively, you can initiate the creation of a new lease by selecting 'New Lease' from the dynamic secret lease list section.


When generating these secrets, it's important to specify a Time-to-Live (TTL) duration. This will dictate how long the credentials are valid for.
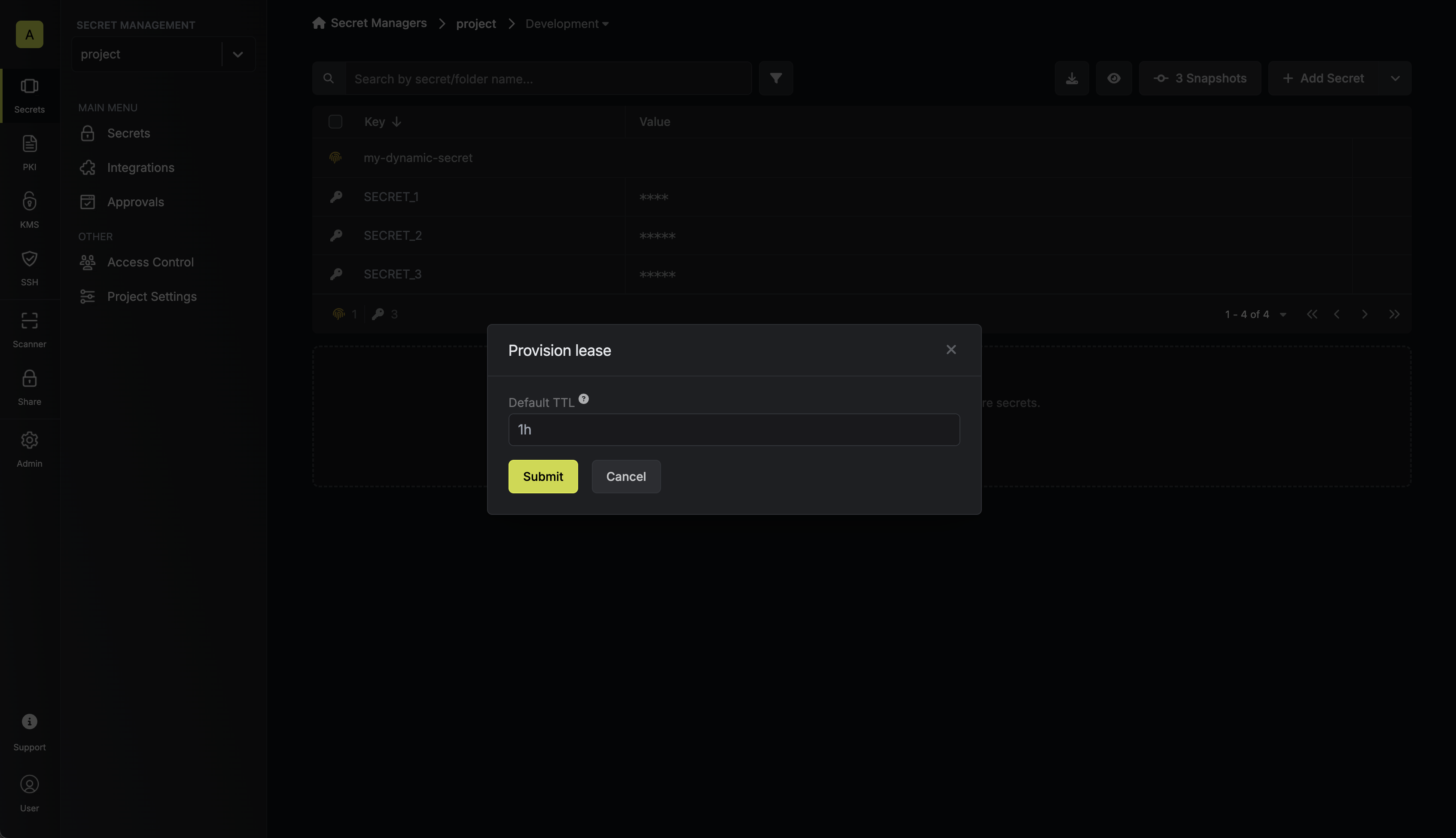
Ensure that the TTL for the lease fall within the maximum TTL defined when configuring the dynamic secret.
Once you click the `Submit` button, a new secret lease will be generated and the credentials from it will be shown to you.
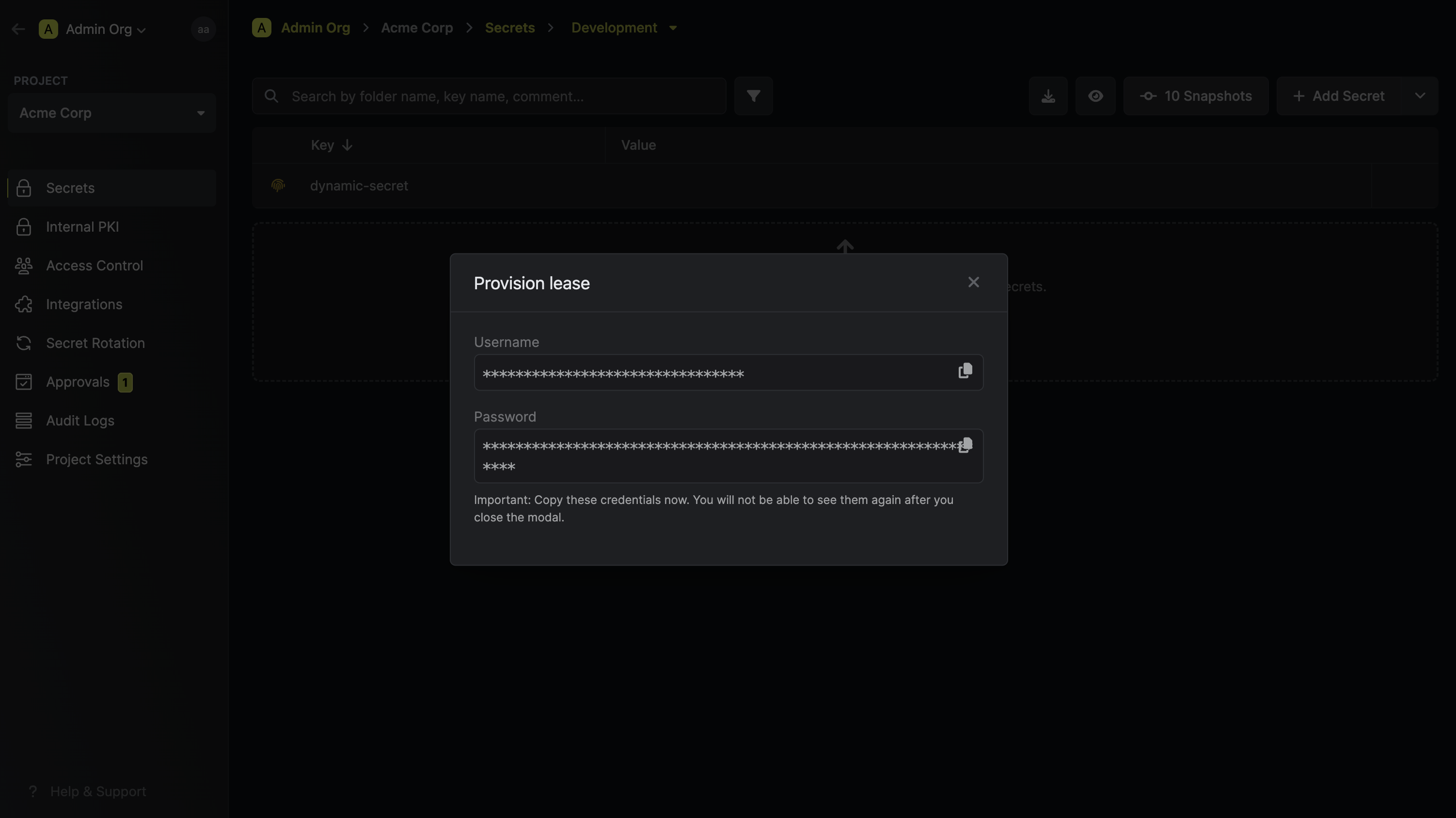
## Audit or Revoke Leases
Once you have created one or more leases, you will be able to access them by clicking on the respective dynamic secret item on the dashboard.
This will allow you see the expiration time of the lease or delete a lease before it's set time to live.
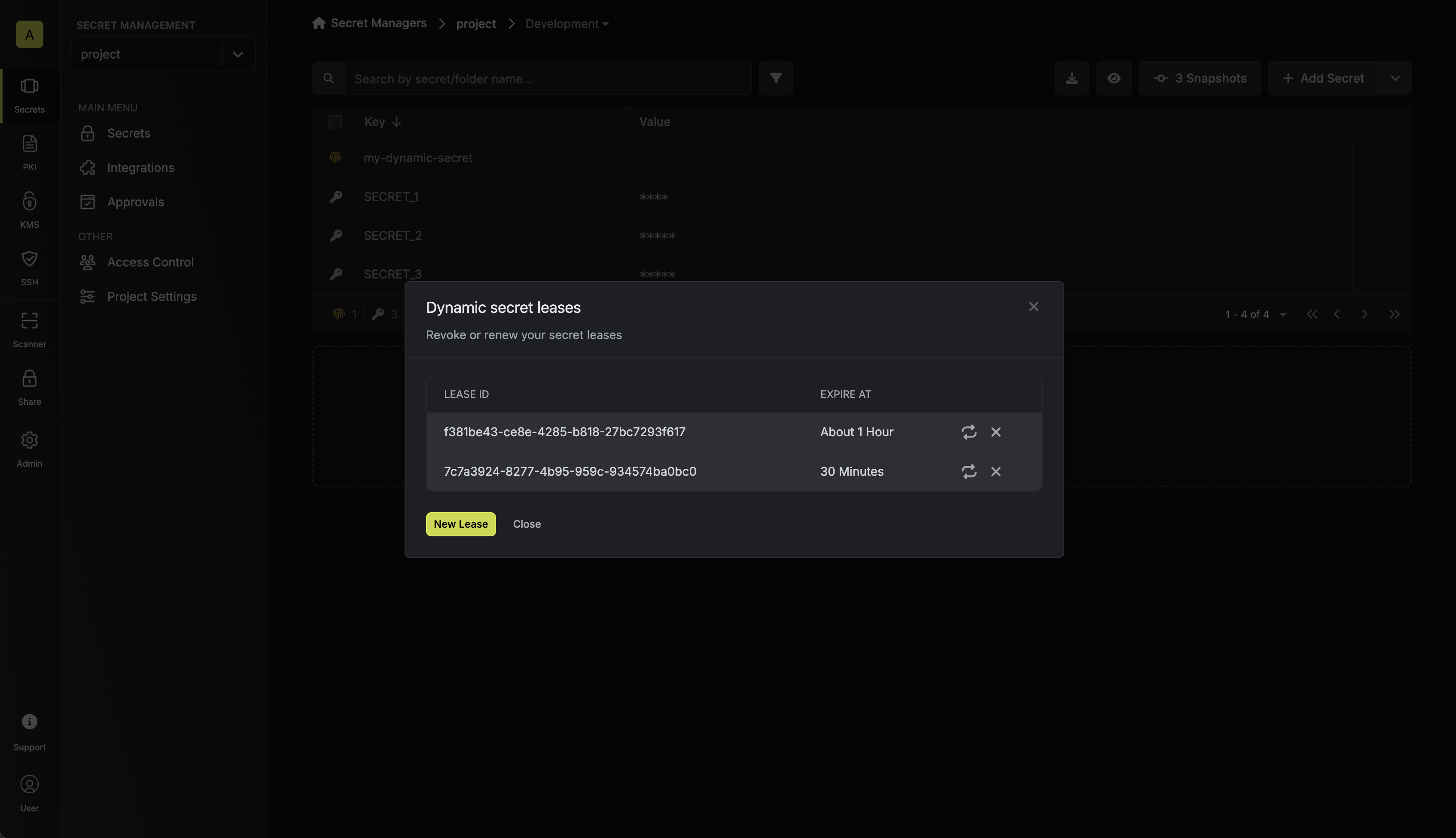
## Renew Leases
To extend the life of the generated dynamic secret leases past its initial time to live, simply click on the **Renew** as illustrated below.
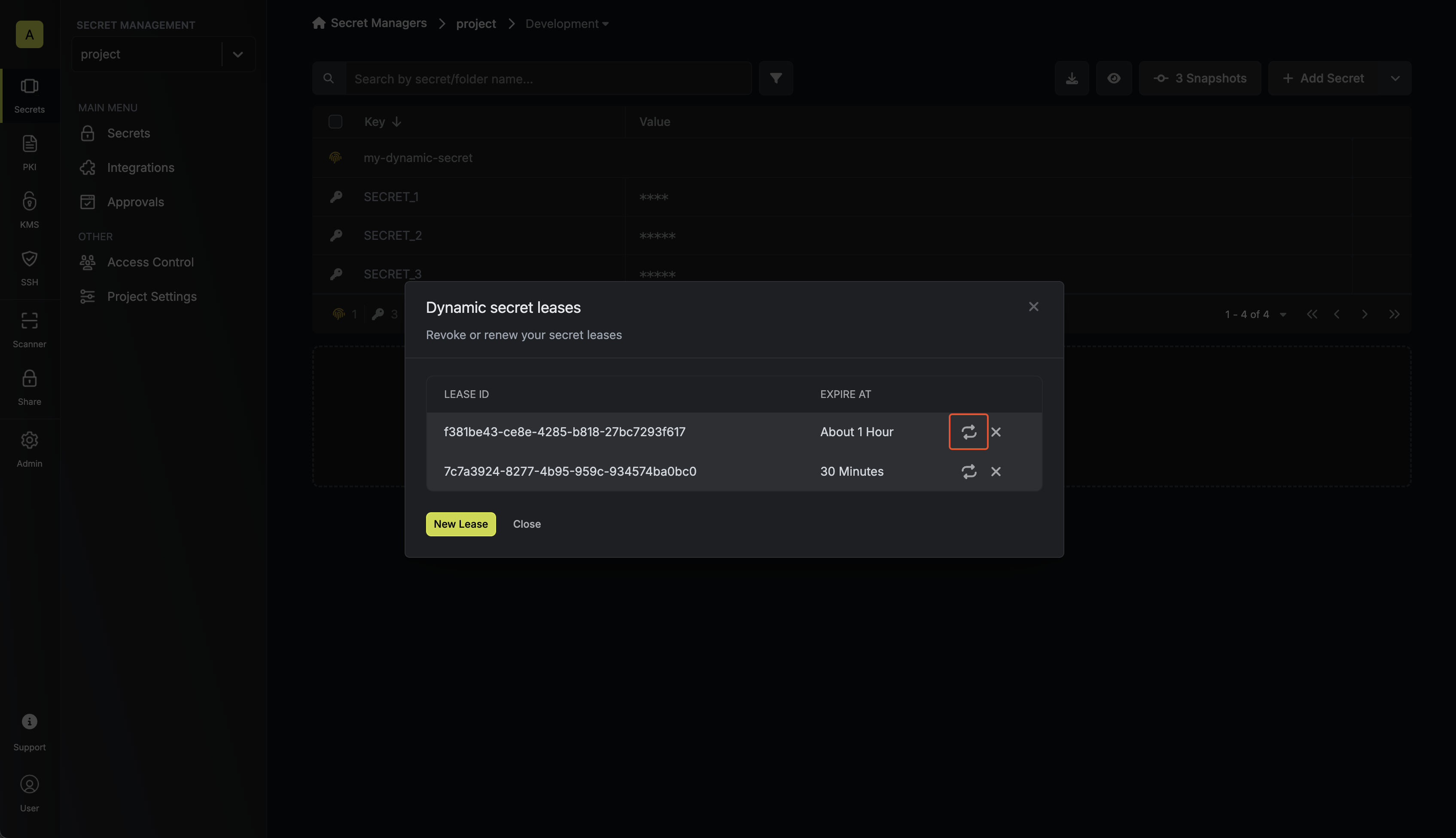
Lease renewals cannot exceed the maximum TTL set when configuring the dynamic secret
# AWS IAM
Learn how to dynamically generate AWS IAM Users.
The Infisical AWS IAM dynamic secret allows you to generate AWS IAM Users on demand based on configured AWS policy.
## Prerequisite
Infisical needs an initial AWS IAM user with the required permissions to create sub IAM users. This IAM user will be responsible for managing the lifecycle of new IAM users.
```json
{
"Version": "2012-10-17",
"Statement": [
{
"Effect": "Allow",
"Action": [
"iam:AttachUserPolicy",
"iam:CreateAccessKey",
"iam:CreateUser",
"iam:DeleteAccessKey",
"iam:DeleteUser",
"iam:DeleteUserPolicy",
"iam:DetachUserPolicy",
"iam:GetUser",
"iam:ListAccessKeys",
"iam:ListAttachedUserPolicies",
"iam:ListGroupsForUser",
"iam:ListUserPolicies",
"iam:PutUserPolicy",
"iam:AddUserToGroup",
"iam:RemoveUserFromGroup"
],
"Resource": ["*"]
}
]
}
```
To minimize managing user access you can attach a resource in format
> arn:aws:iam::\:user/\
Replace **\** with your AWS account id and **\** with a path to minimize managing user access.
## Set up Dynamic Secrets with AWS IAM
Navigate to the Secret Overview dashboard and select the environment in which you would like to add a dynamic secret to.
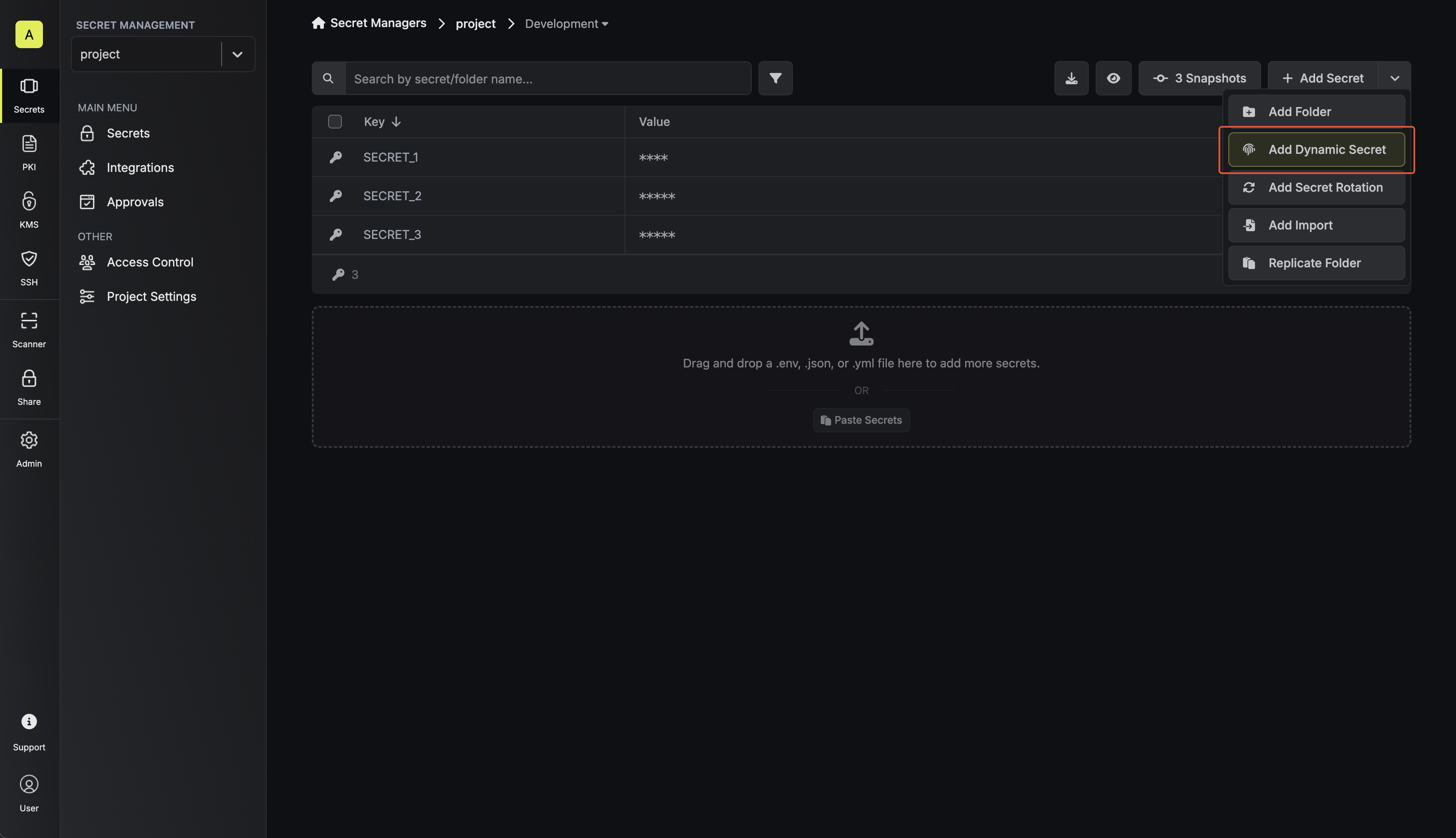
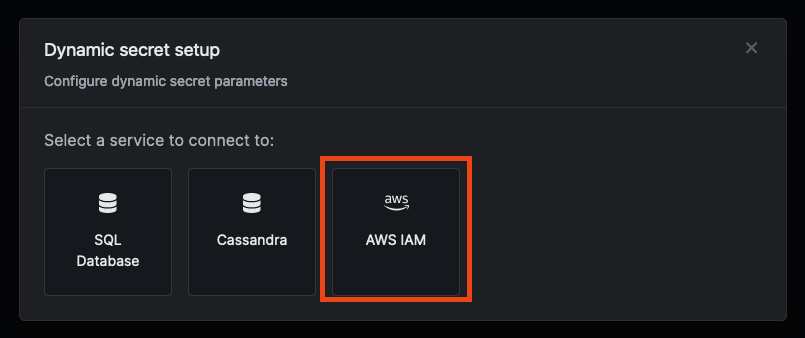
Name by which you want the secret to be referenced
Default time-to-live for a generated secret (it is possible to modify this value when a secret is generate)
Maximum time-to-live for a generated secret
The managing AWS IAM User Access Key
The managing AWS IAM User Secret Key
[IAM AWS Path](https://aws.amazon.com/blogs/security/optimize-aws-administration-with-iam-paths/) to scope created IAM User resource access.
The AWS data center region.
The IAM Policy ARN of the [AWS Permissions Boundary](https://docs.aws.amazon.com/IAM/latest/UserGuide/access_policies_boundaries.html) to attach to IAM users created in the role.
The AWS IAM groups that should be assigned to the created users. Multiple values can be provided by separating them with commas
The AWS IAM managed policies that should be attached to the created users. Multiple values can be provided by separating them with commas
The AWS IAM inline policy that should be attached to the created users. Multiple values can be provided by separating them with commas

After submitting the form, you will see a dynamic secret created in the dashboard.

Once you've successfully configured the dynamic secret, you're ready to generate on-demand credentials.
To do this, simply click on the 'Generate' button which appears when hovering over the dynamic secret item.
Alternatively, you can initiate the creation of a new lease by selecting 'New Lease' from the dynamic secret lease list section.
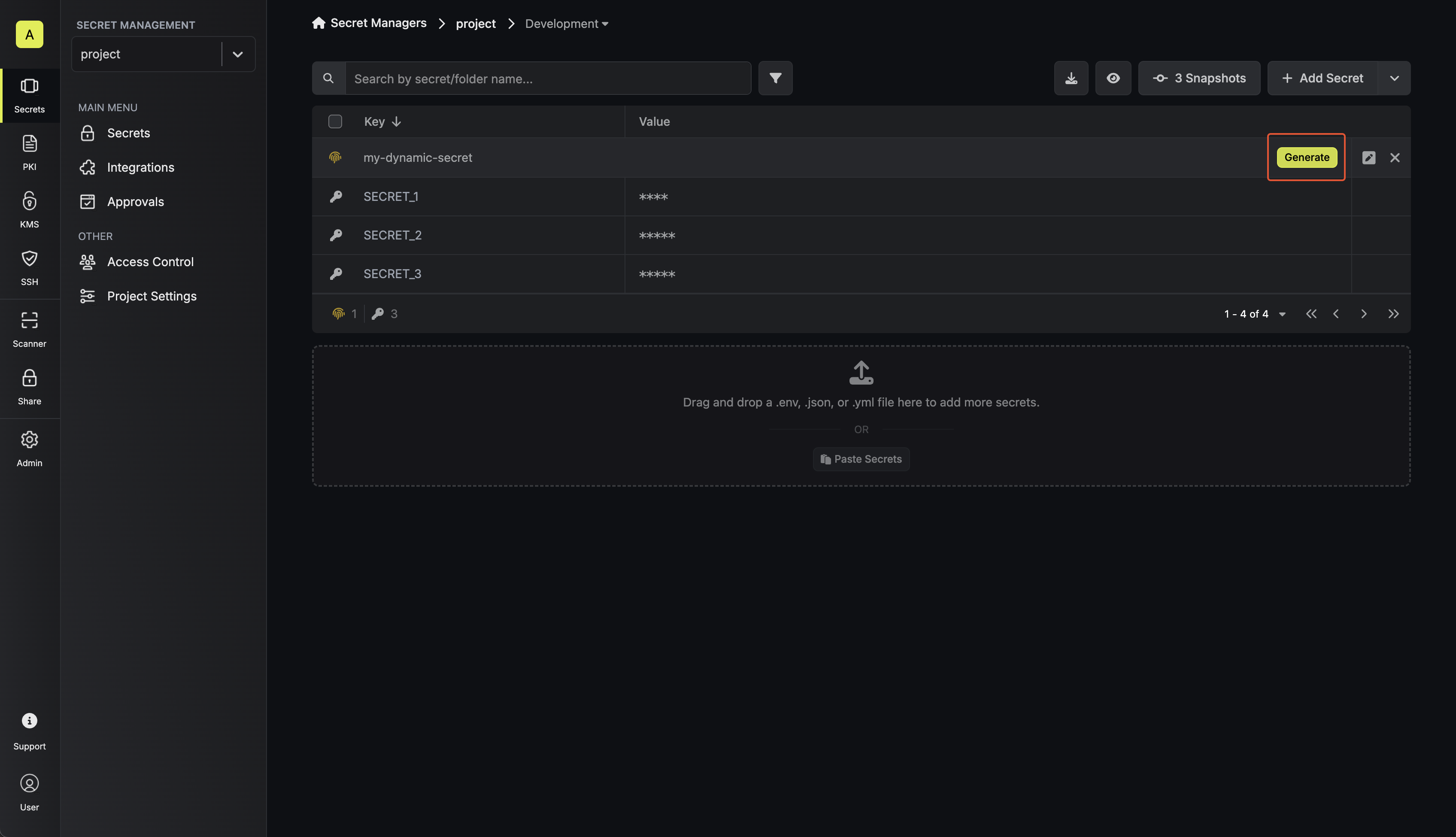
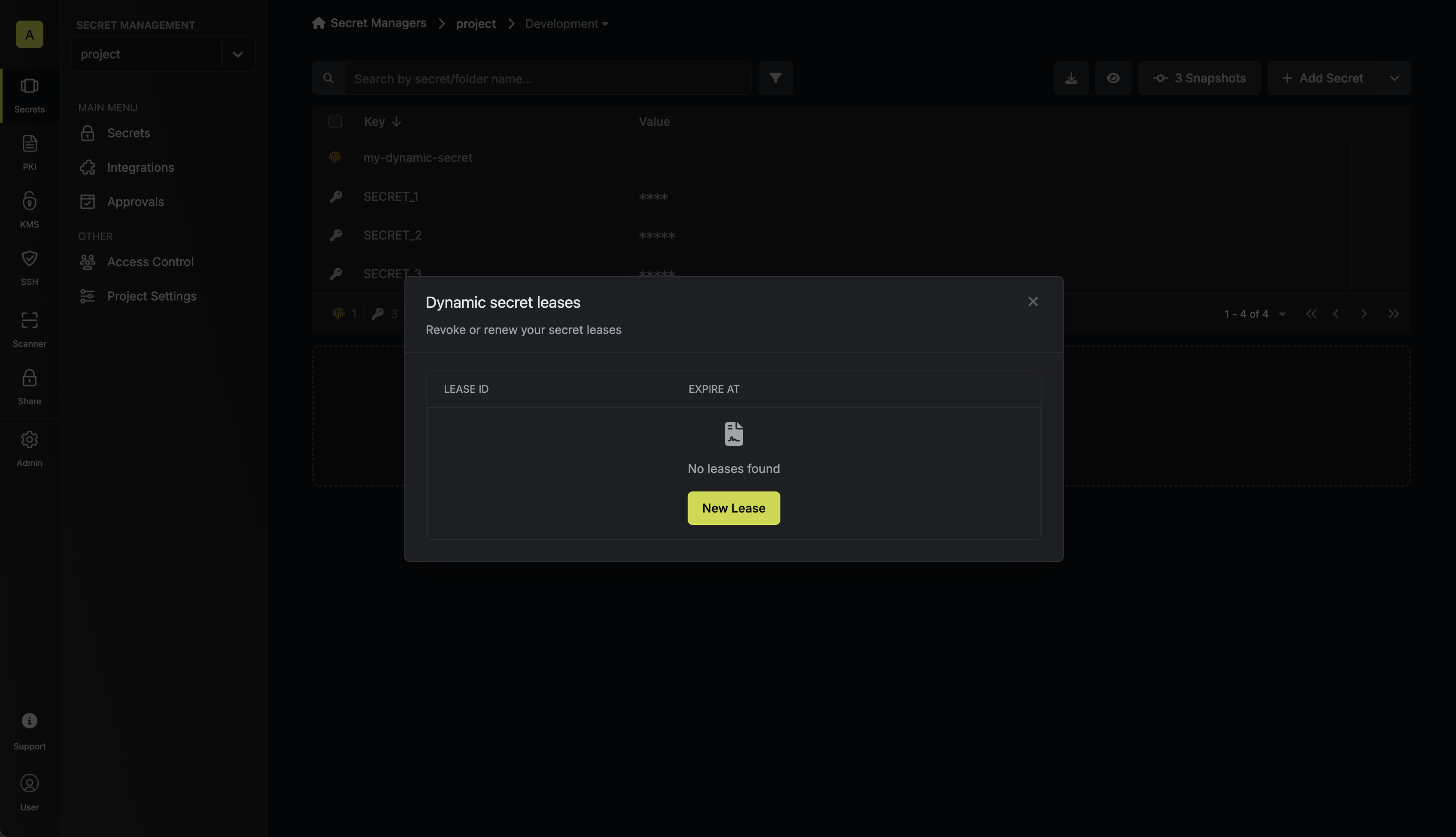
When generating these secrets, it's important to specify a Time-to-Live (TTL) duration. This will dictate how long the credentials are valid for.
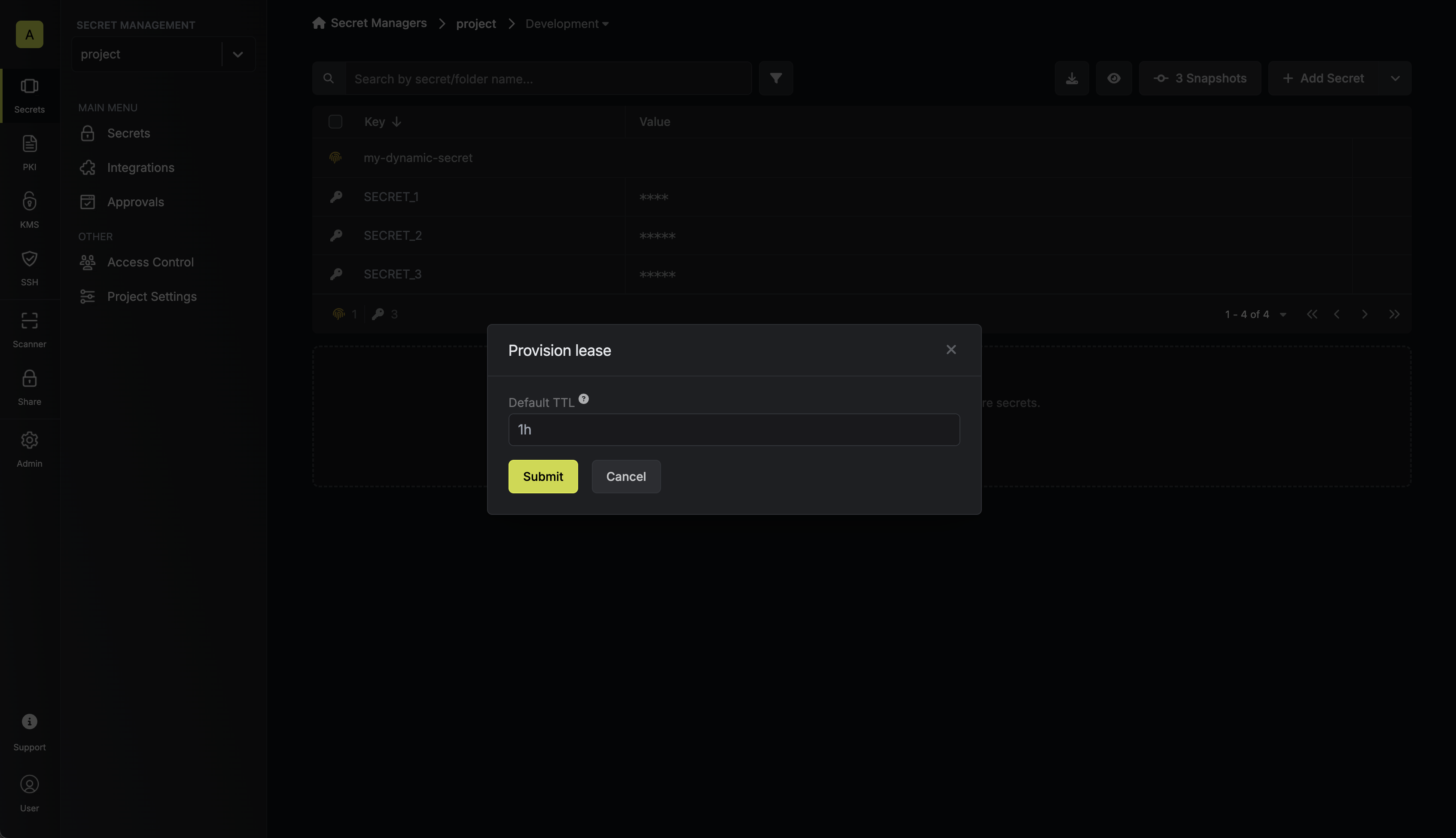
Ensure that the TTL for the lease fall within the maximum TTL defined when configuring the dynamic secret in step 4.
Once you click the `Submit` button, a new secret lease will be generated and the credentials for it will be shown to you.
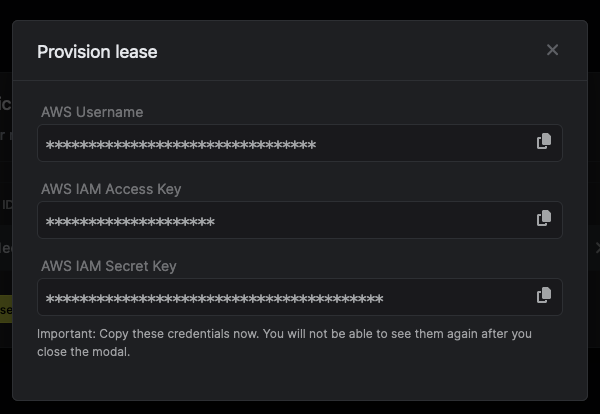
## Audit or Revoke Leases
Once you have created one or more leases, you will be able to access them by clicking on the respective dynamic secret item on the dashboard.
This will allow you see the lease details and delete the lease ahead of its expiration time.
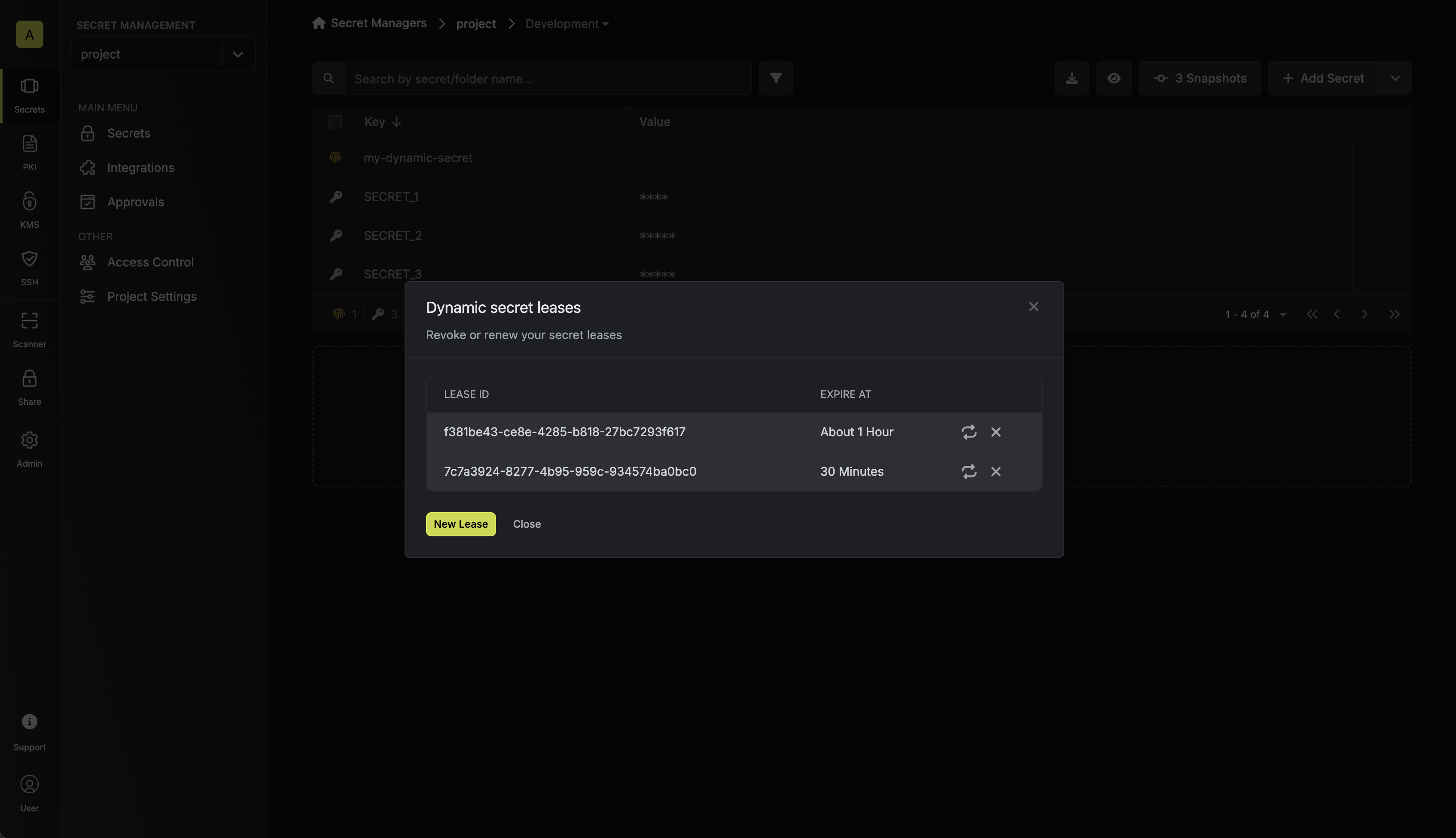
## Renew Leases
To extend the life of the generated dynamic secret lease past its initial time to live, simply click on the **Renew** as illustrated below.
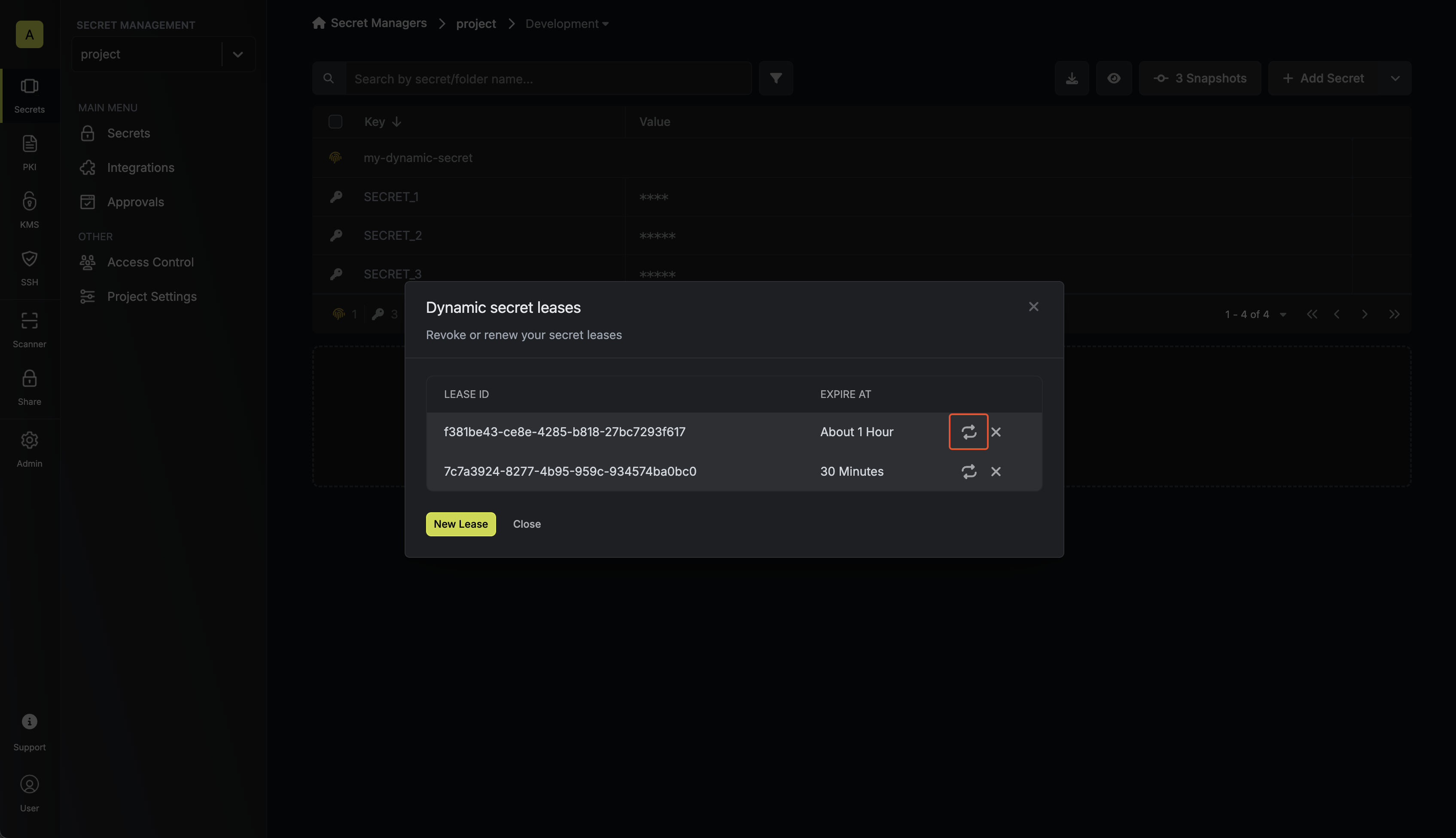
Lease renewals cannot exceed the maximum TTL set when configuring the dynamic secret
# Azure Entra Id
Learn how to dynamically generate Azure Entra Id user credentials.
The Infisical Azure Entra Id dynamic secret allows you to generate Azure Entra Id credentials on demand based on configured role.
## Prerequisites
Login to [Microsoft Entra ID](https://entra.microsoft.com/)
Go to Overview, Copy and store `Tenant Id`
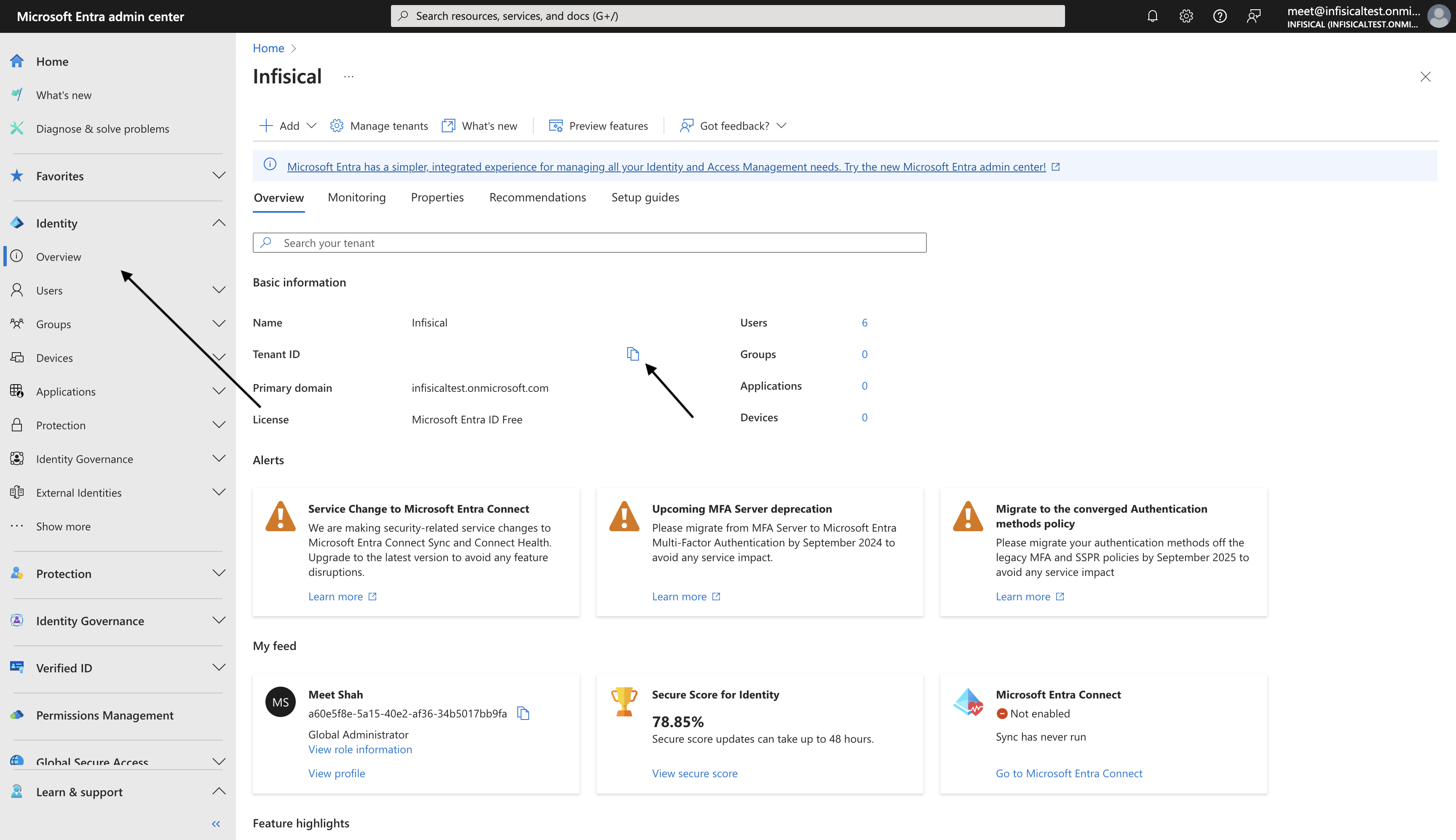
Go to Applications > App registrations. Click on New Registration.
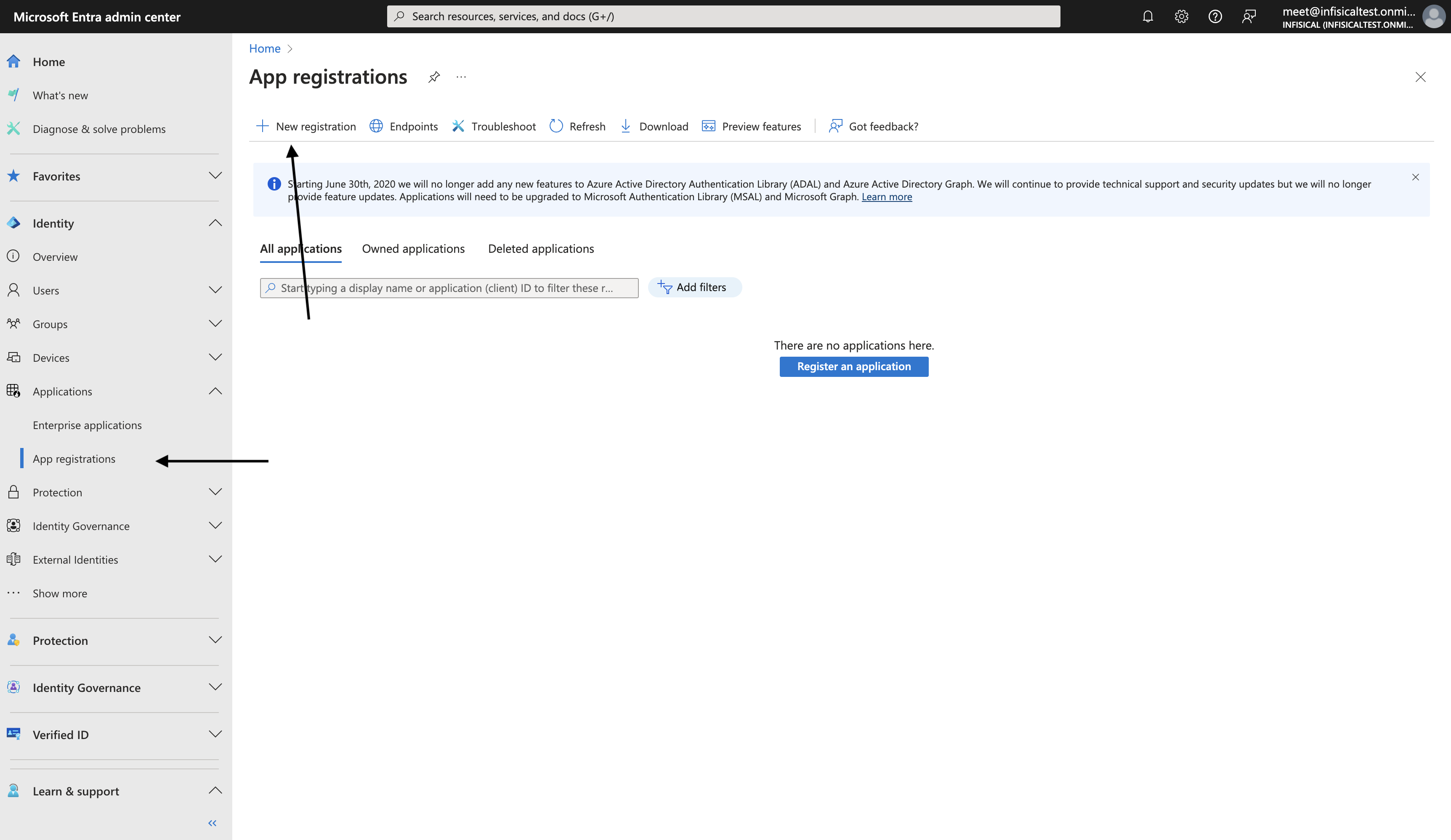
Enter an application name. Click Register.
Copy and store `Application Id`.
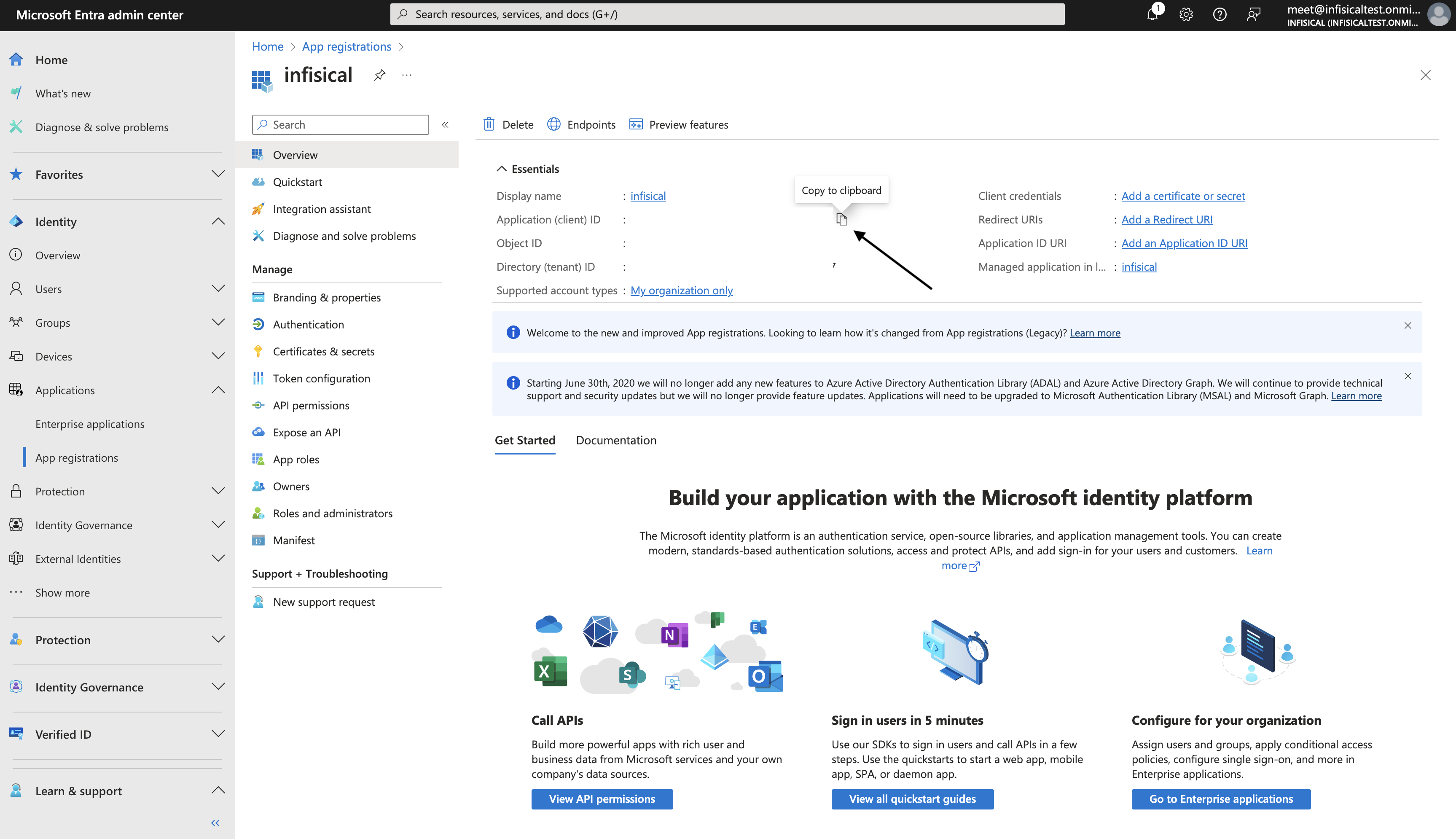
Go to Clients and Secrets. Click on New Client Secret.
Enter a description, select expiry and click Add.
Copy and store `Client Secret` value.
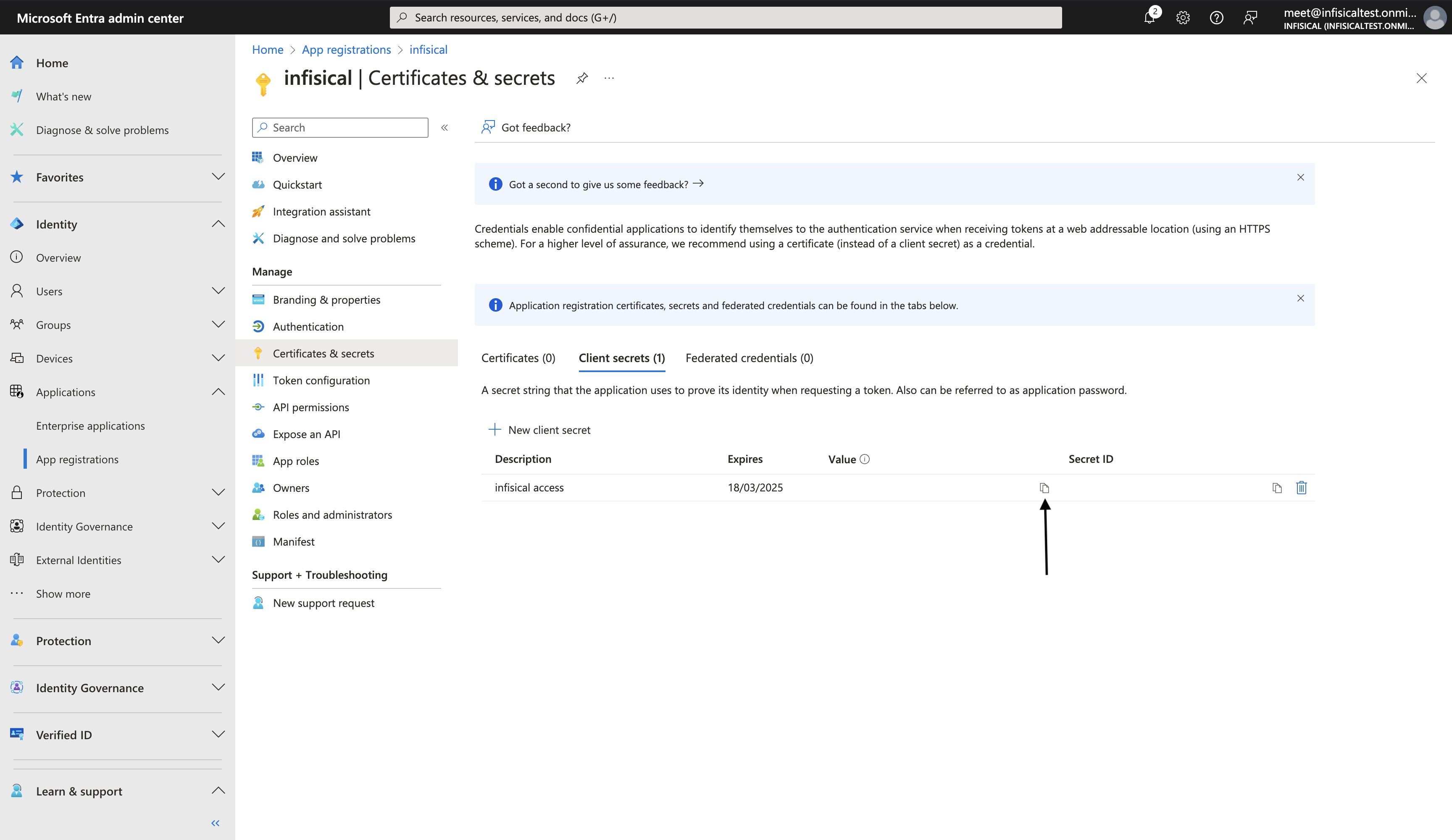
Go to API Permissions. Click on Add a permission.
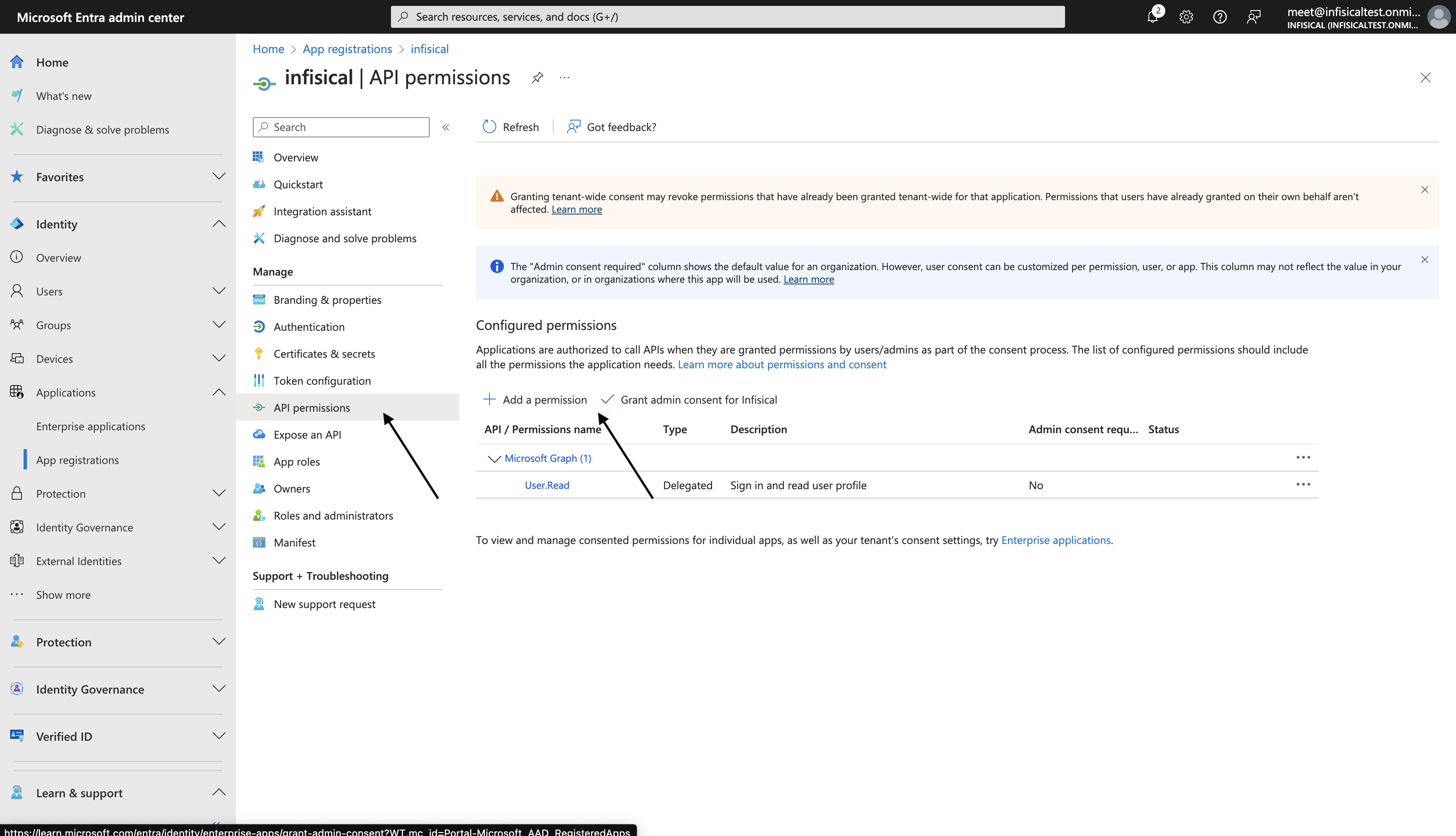
Click on Microsoft Graph.
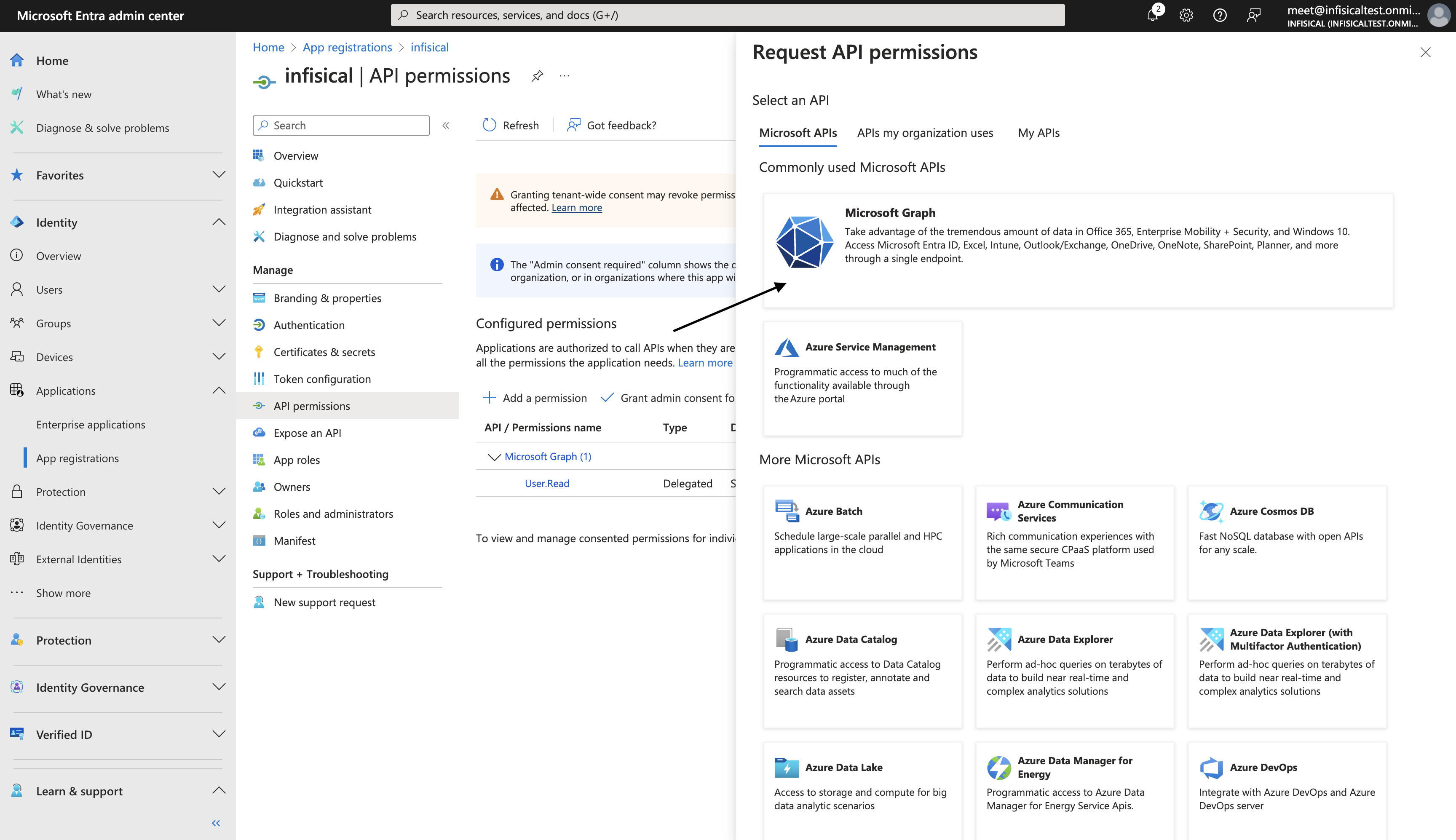
Click on Application Permissions. Search and select `User.ReadWrite.All` and click Add permissions.
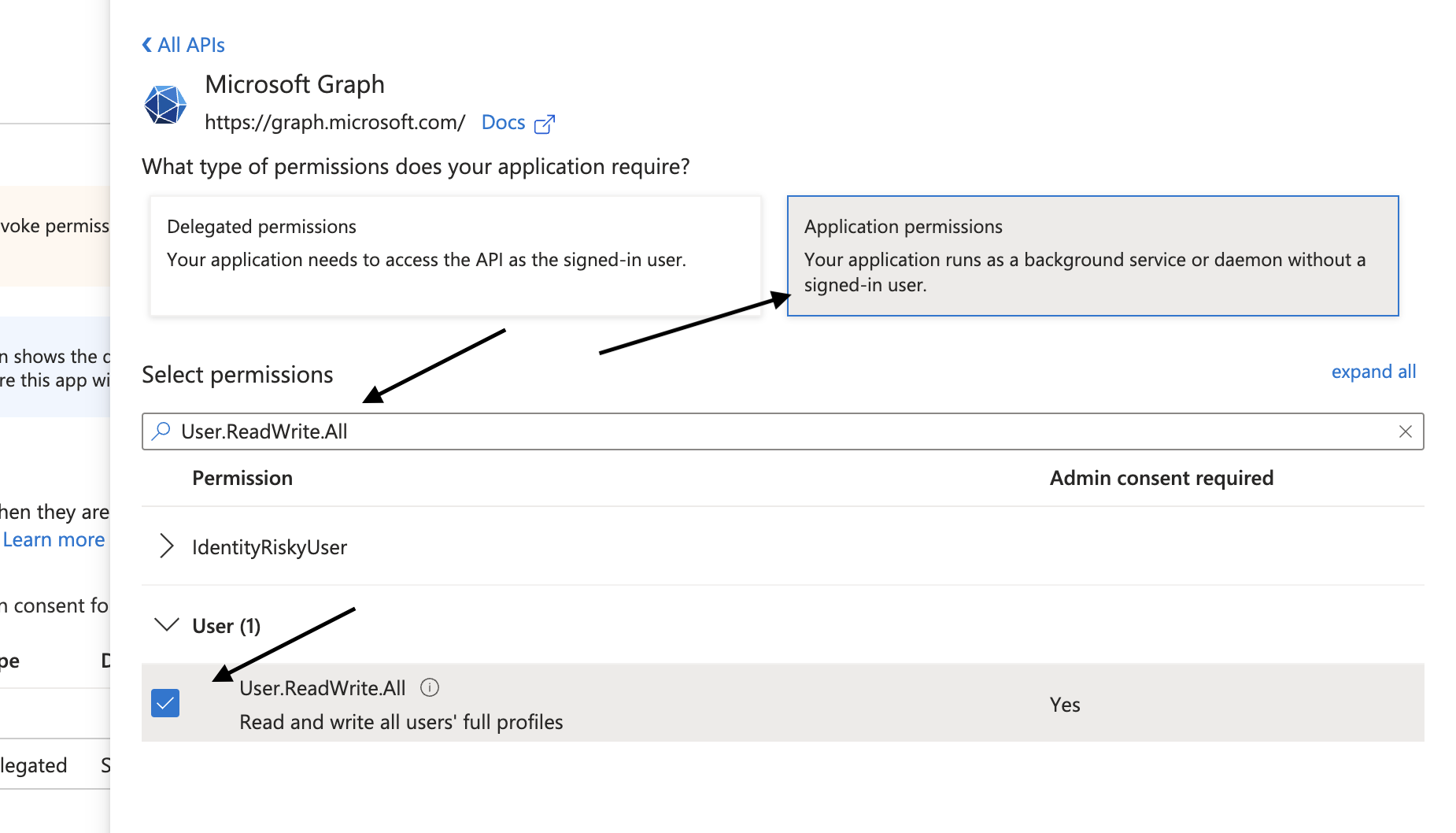
Click on Grant admin consent for app. Click yes to confirm.
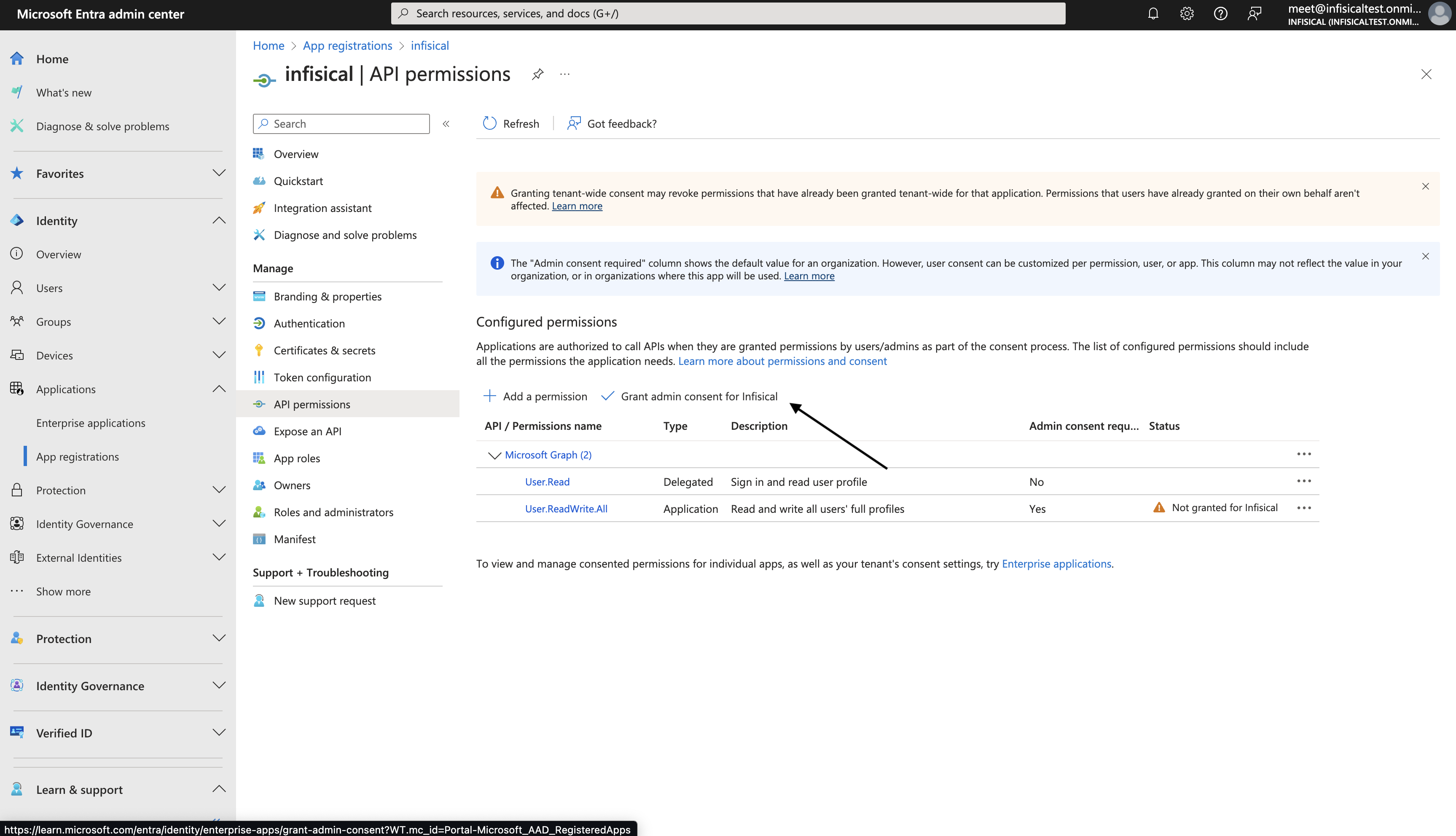
Go to Dashboard. Click on show more.
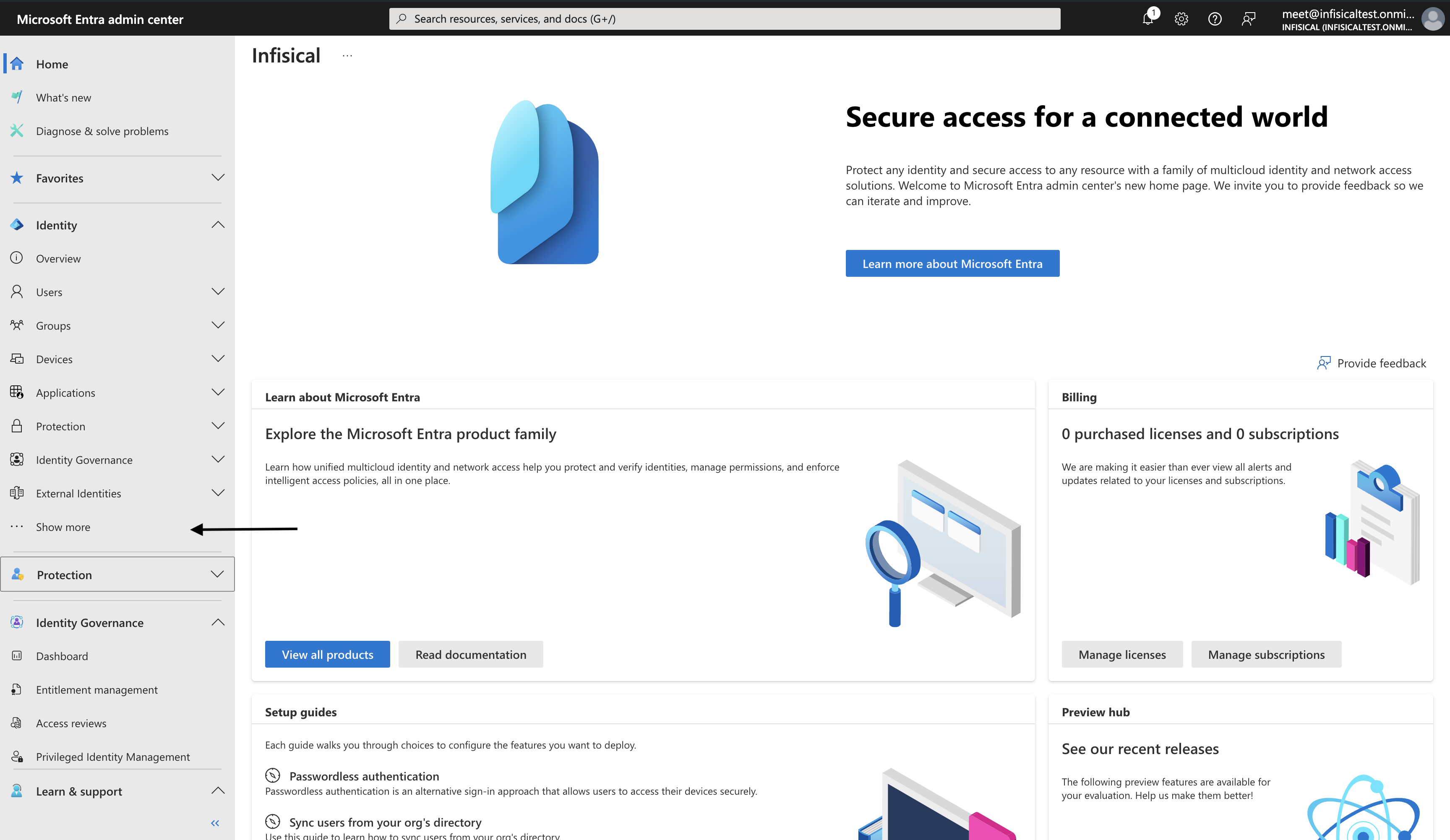
Click on Roles & admins. Search for User Administrator and click on it.
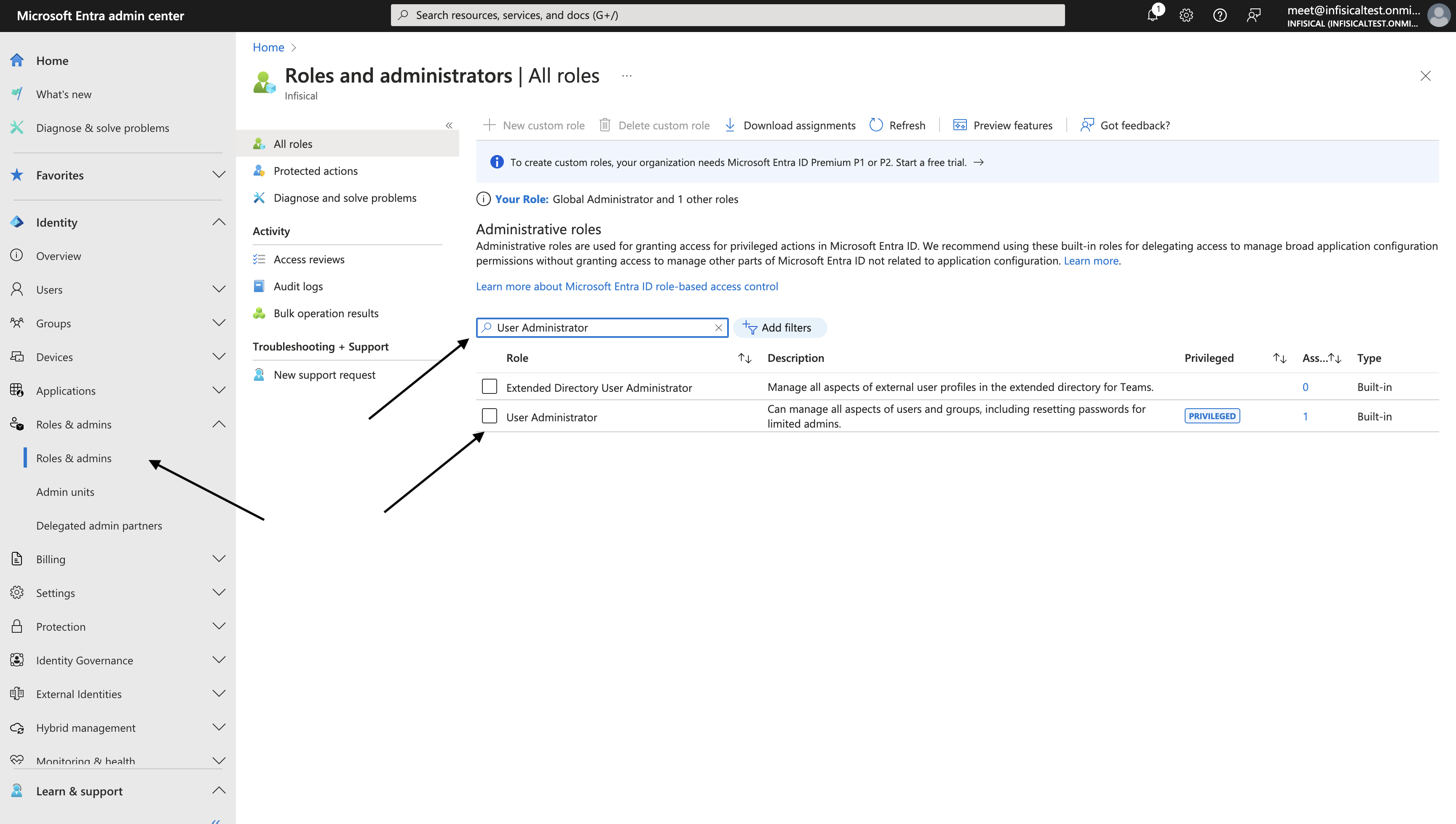
Click on Add assignments. Search for the application name you created and select it. Click on Add.
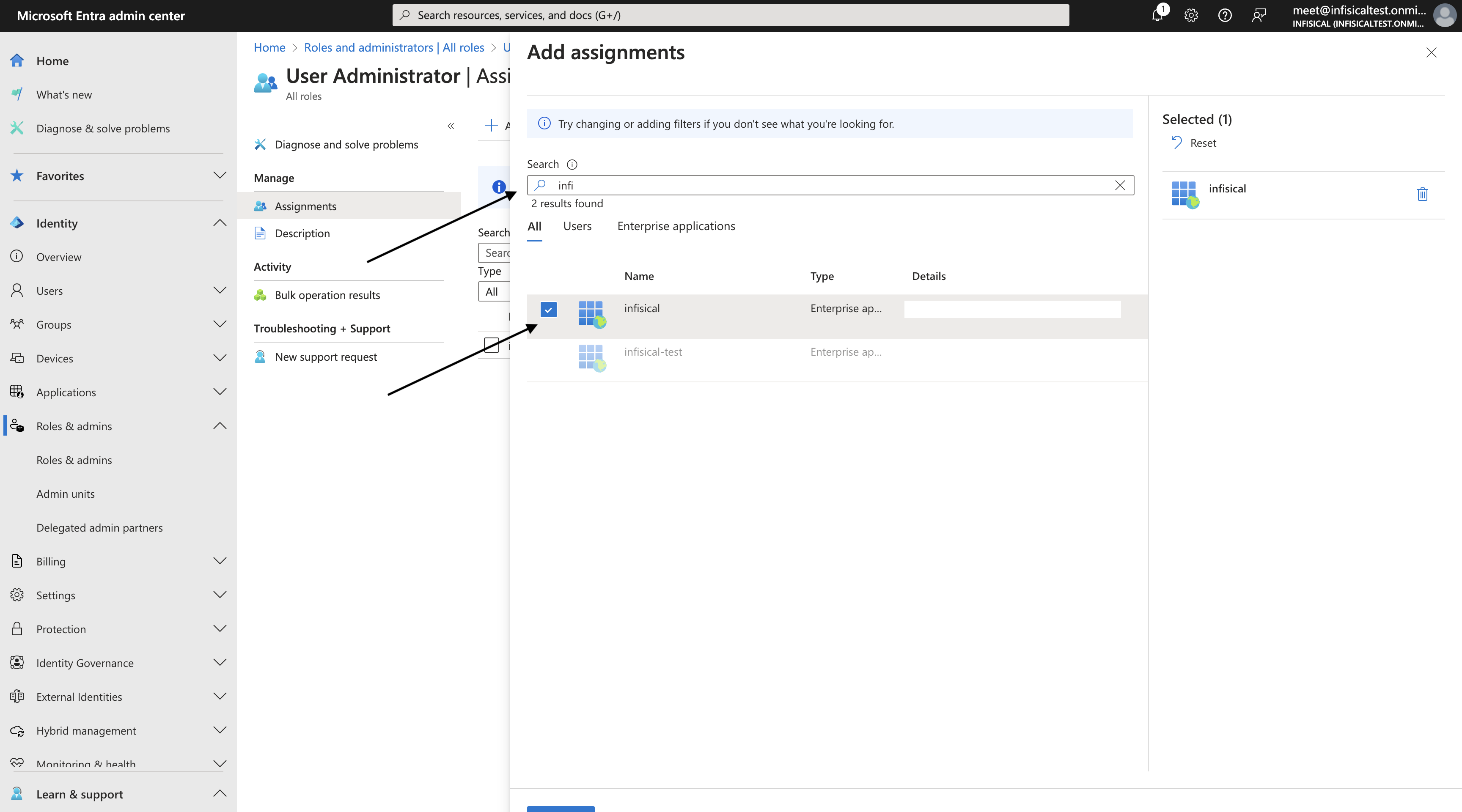
## Set up Dynamic Secrets with Azure Entra ID
Open the Secret Overview dashboard and select the environment in which you would like to add a dynamic secret.
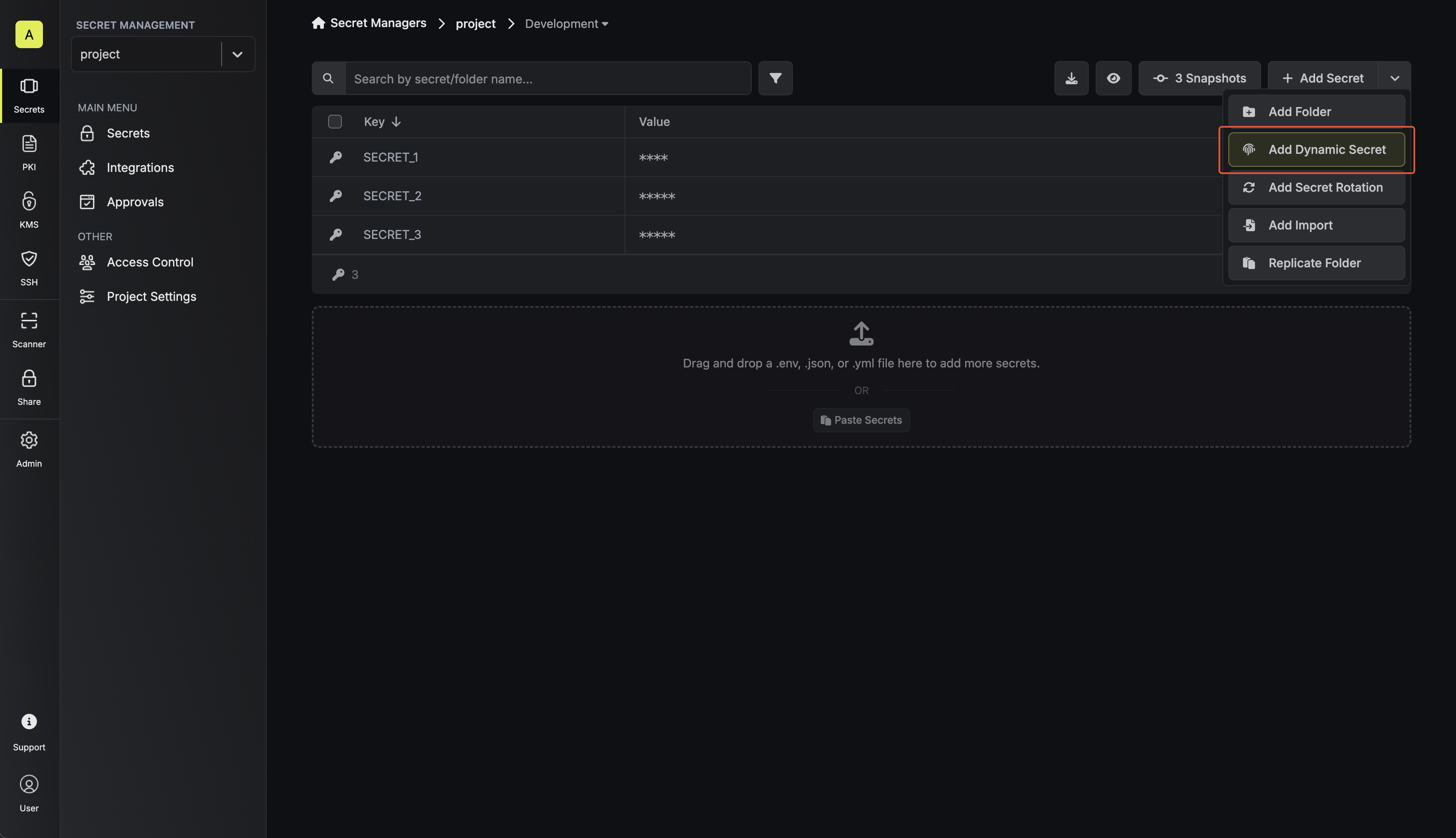
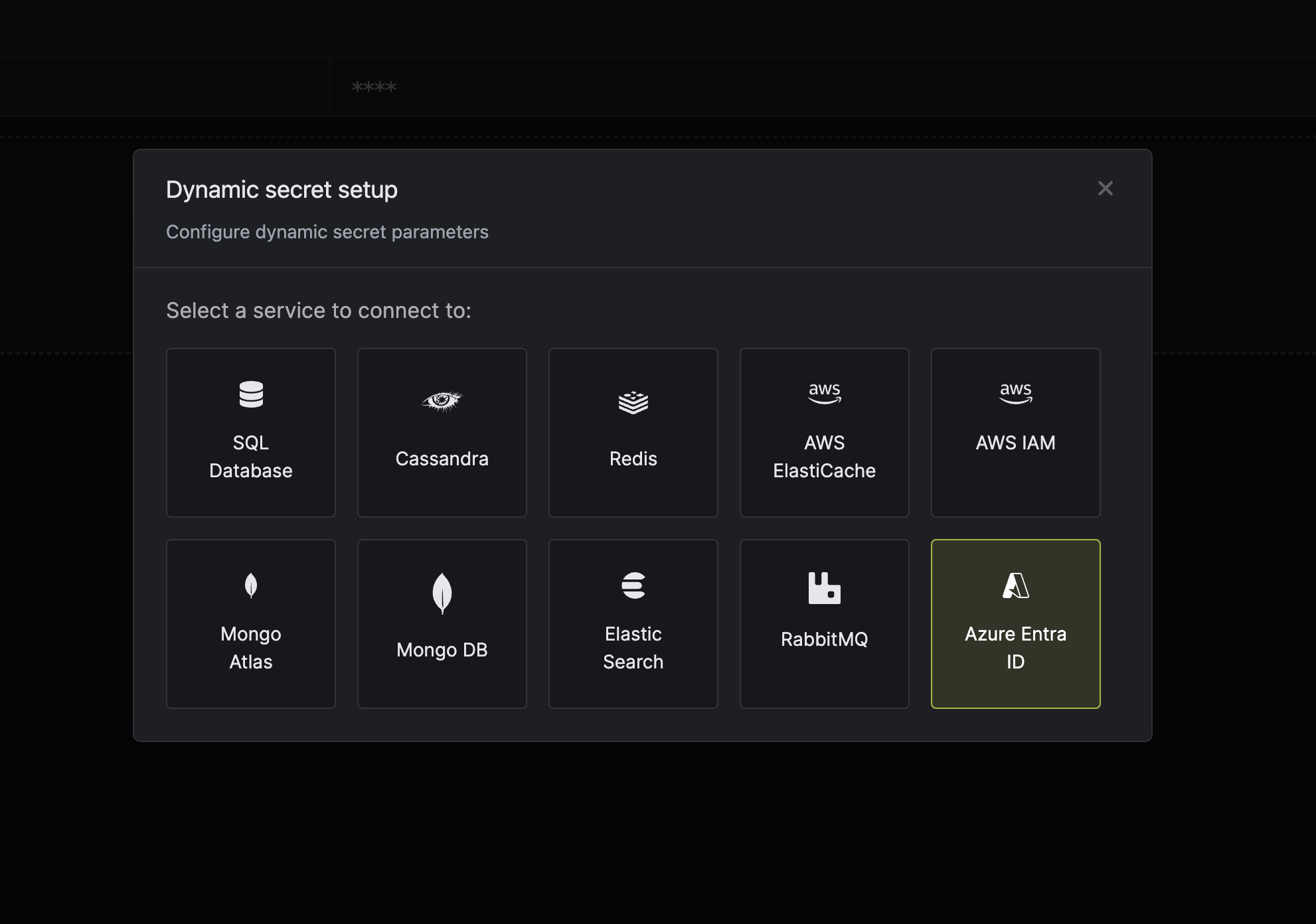
Prefix for the secrets to be created
Default time-to-live for a generated secret (it is possible to modify this value when a secret is generate)
Maximum time-to-live for a generated secret.
The Tenant ID of your Azure Entra ID account.
The Application ID of the application you created in Azure Entra ID.
The Client Secret of the application you created in Azure Entra ID.
Multi select list of users to generate secrets for.
After submitting the form, you will see a dynamic secrets for each user created in the dashboard.
Once you've successfully configured the dynamic secret, you're ready to generate on-demand credentials.
To do this, simply click on the 'Generate' button which appears when hovering over the dynamic secret item.
Alternatively, you can initiate the creation of a new lease by selecting 'New Lease' from the dynamic secret lease list section.


When generating these secrets, it's important to specify a Time-to-Live (TTL) duration. This will dictate how long the credentials are valid for.
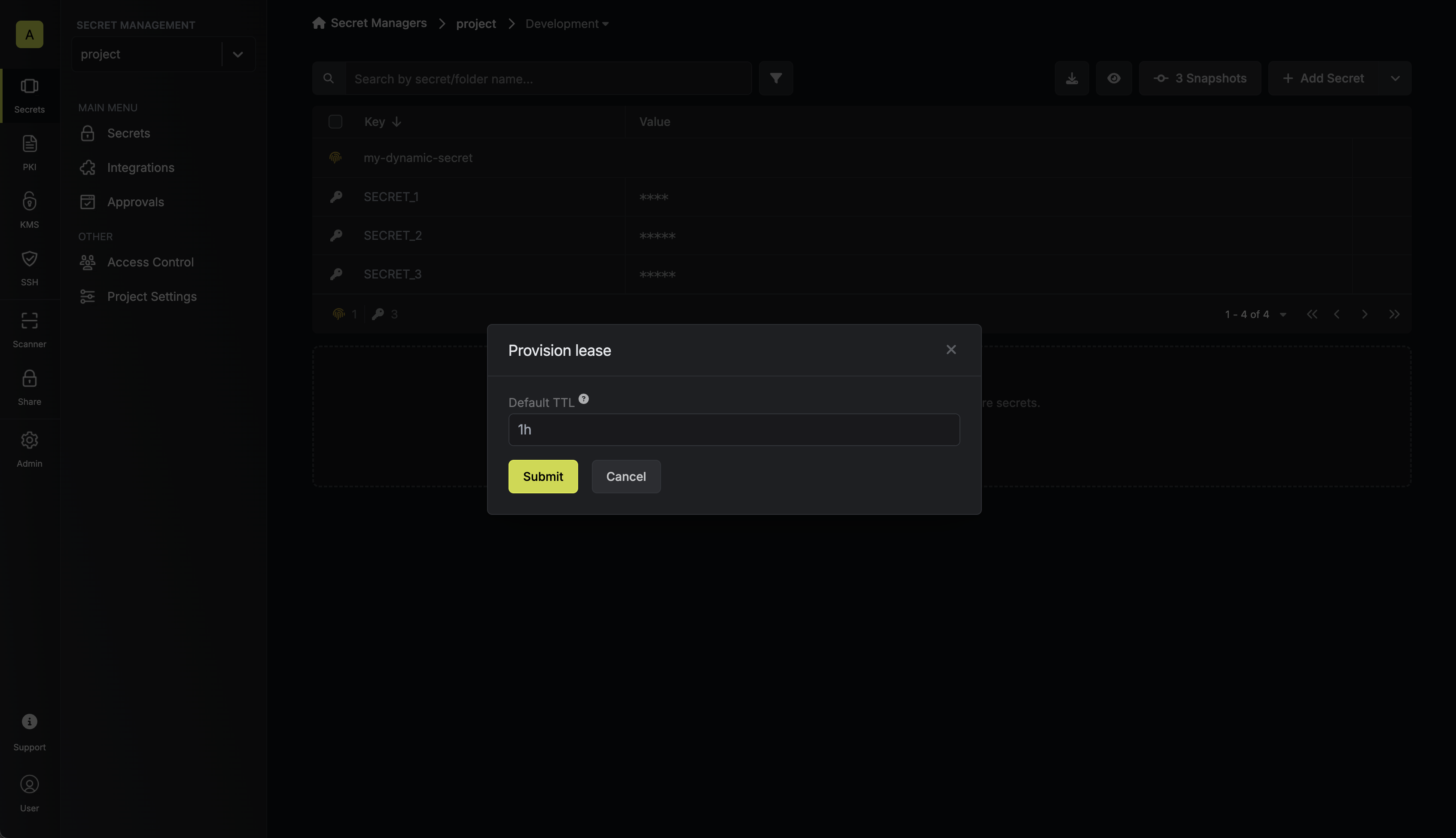
Ensure that the TTL for the lease fall within the maximum TTL defined when configuring the dynamic secret.
Once you click the `Submit` button, a new secret lease will be generated and the credentials from it will be shown to you.
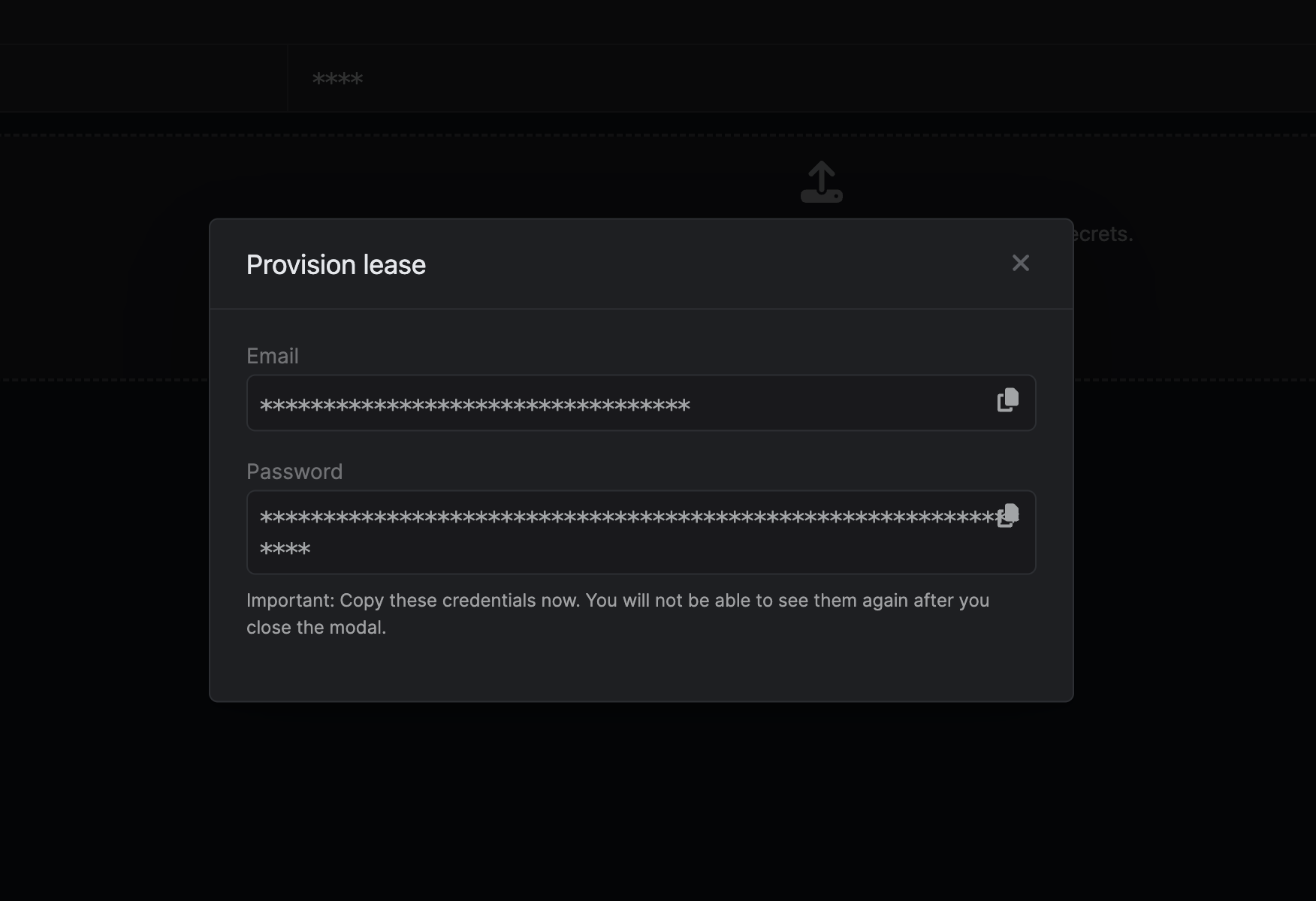
## Audit or Revoke Leases
Once you have created one or more leases, you will be able to access them by clicking on the respective dynamic secret item on the dashboard.
This will allow you see the expiration time of the lease or delete a lease before it's set time to live.
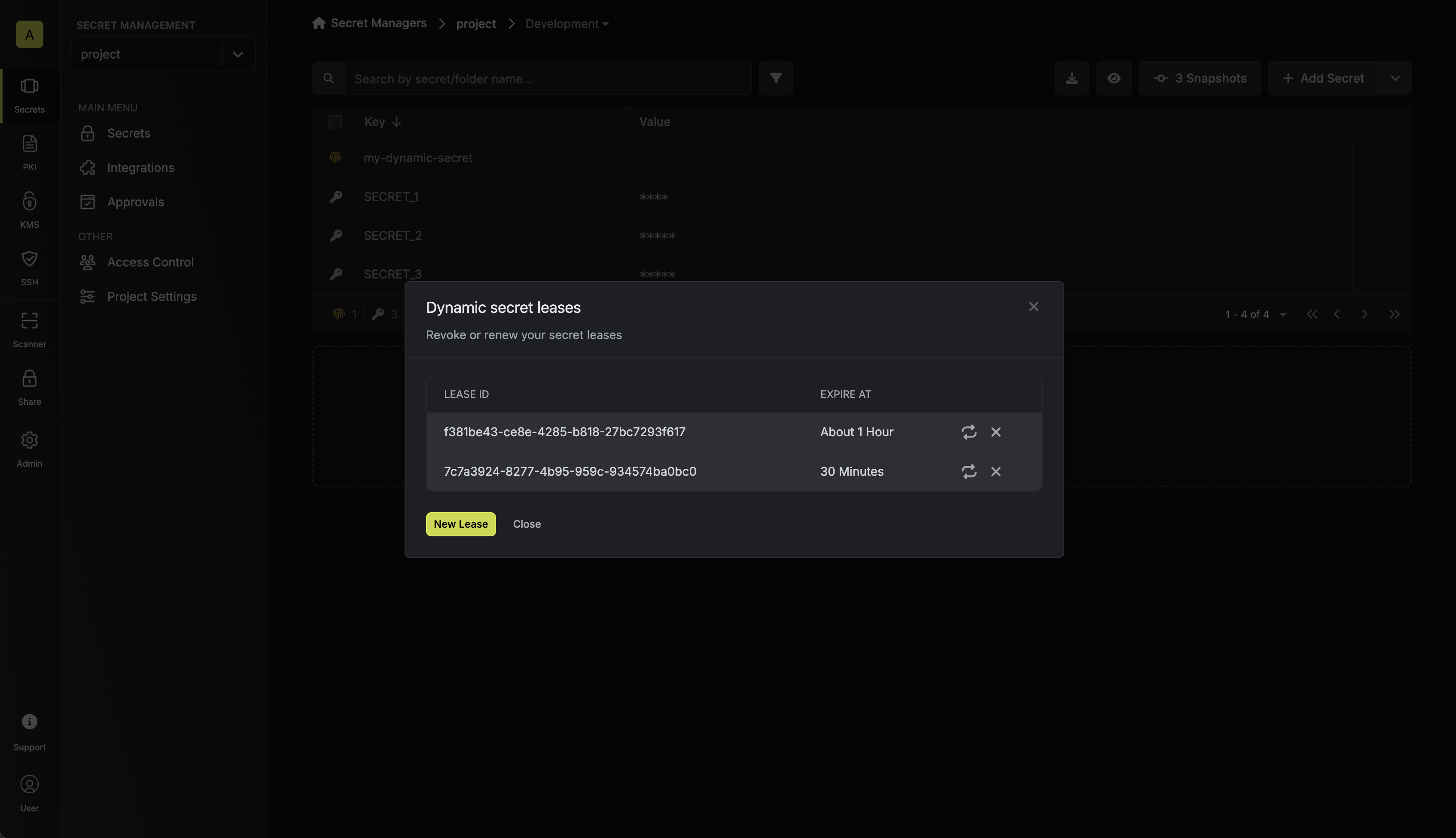
## Renew Leases
To extend the life of the generated dynamic secret leases past its initial time to live, simply click on the **Renew** as illustrated below.
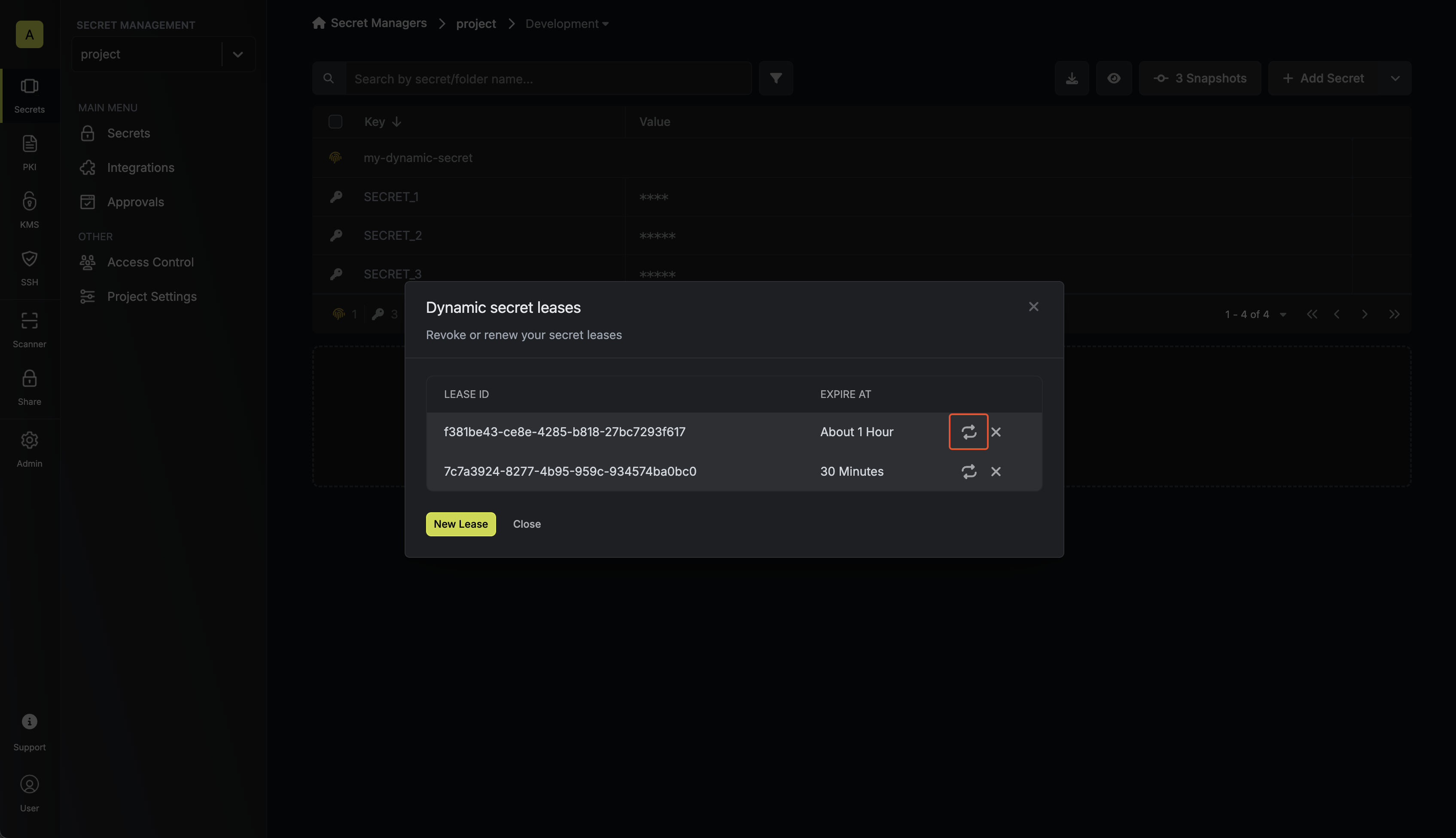
Lease renewals cannot exceed the maximum TTL set when configuring the dynamic secret
# Cassandra
Learn how to dynamically generate Cassandra database user credentials
The Infisical Cassandra dynamic secret allows you to generate Cassandra database credentials on demand based on configured role.
## Prerequisite
Infisical requires a Cassandra user in your instance with the necessary permissions. This user will facilitate the creation of new accounts as needed.
Ensure the user possesses privileges for creating, dropping, and granting permissions to roles for it to be able to create dynamic secrets.
In your Cassandra configuration file `cassandra.yaml`, make sure you have the following settings:
```yaml
authenticator: PasswordAuthenticator
authorizer: CassandraAuthorizer
```
The above configuration allows user creation and granting permissions.
## Set up Dynamic Secrets with Cassandra
Open the Secret Overview dashboard and select the environment in which you would like to add a dynamic secret.
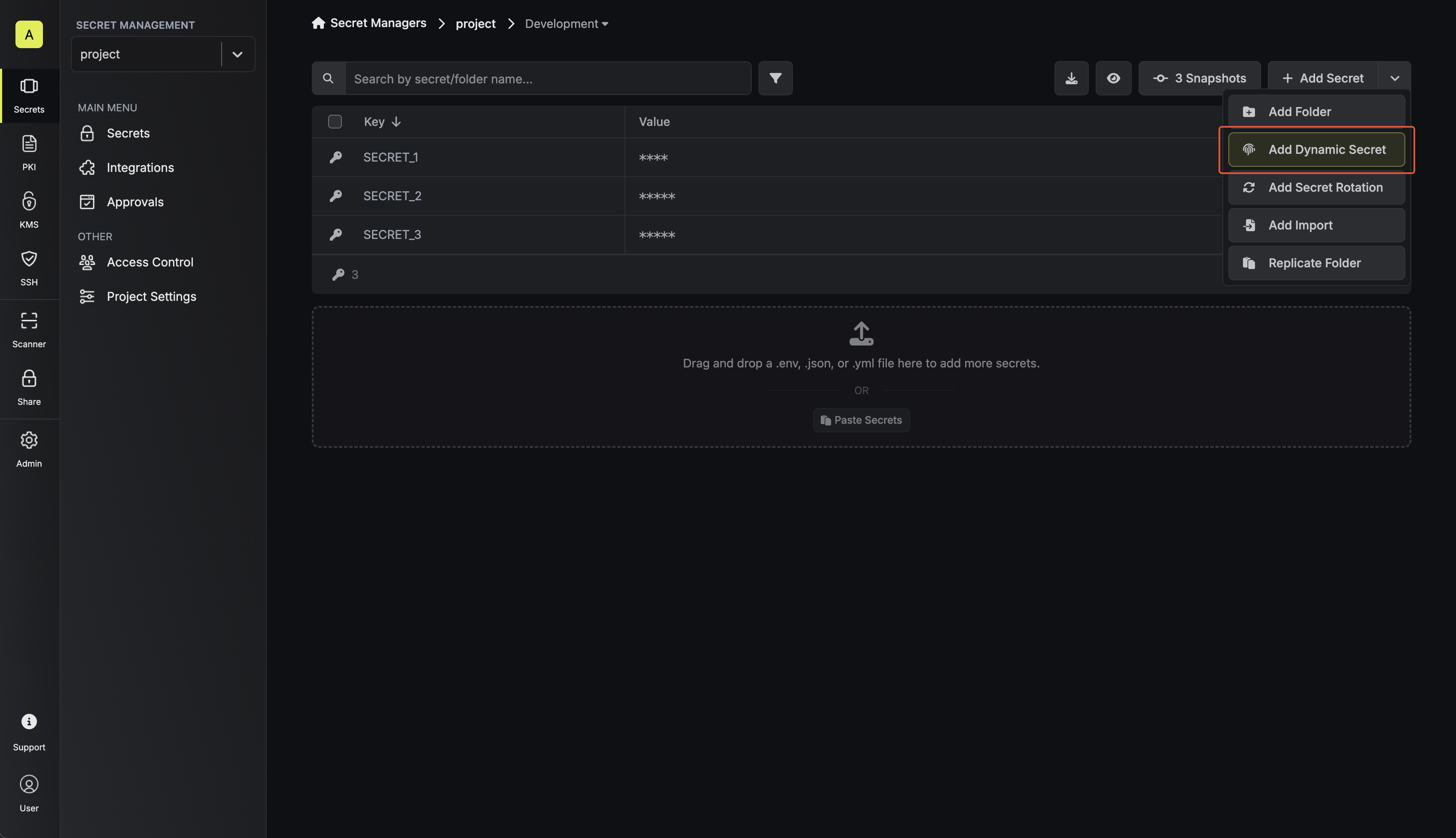
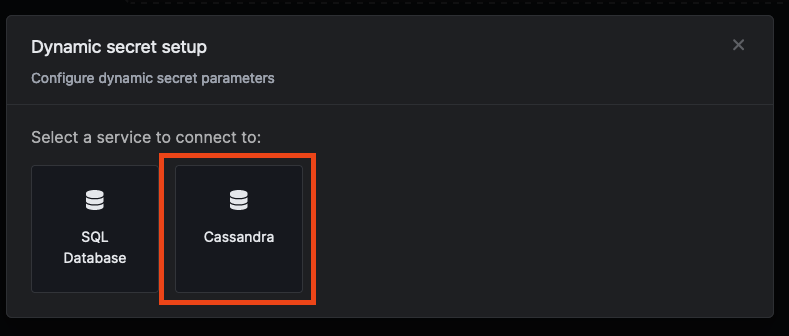
Name by which you want the secret to be referenced
Default time-to-live for a generated secret (it is possible to modify this value when a secret is generate)
Maximum time-to-live for a generated secret
Cassandra Host. You can specify multiple Cassandra hosts by separating them with commas.
Cassandra port
Username that will be used to create dynamic secrets
Password that will be used to create dynamic secrets
Specify the local data center in Cassandra that you want to use. This choice should align with your Cassandra cluster setup.
Keyspace name where you want to create dynamic secrets. This ensures that the user is limited to that keyspace.
A CA may be required if your cassandra requires it for incoming connections.
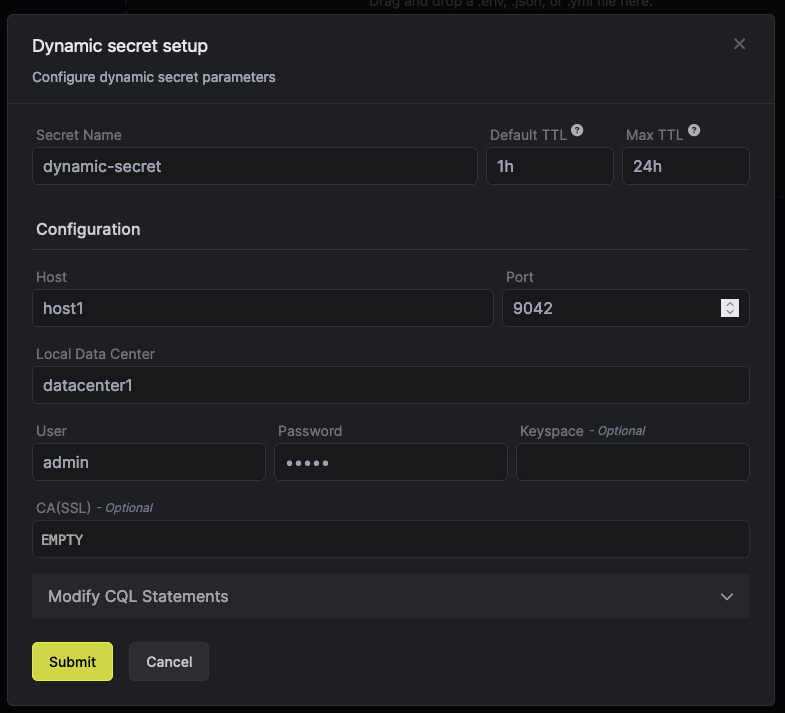
If you want to provide specific privileges for the generated dynamic credentials, you can modify the CQL statement to your needs. This is useful if you want to only give access to a specific key-space(s).
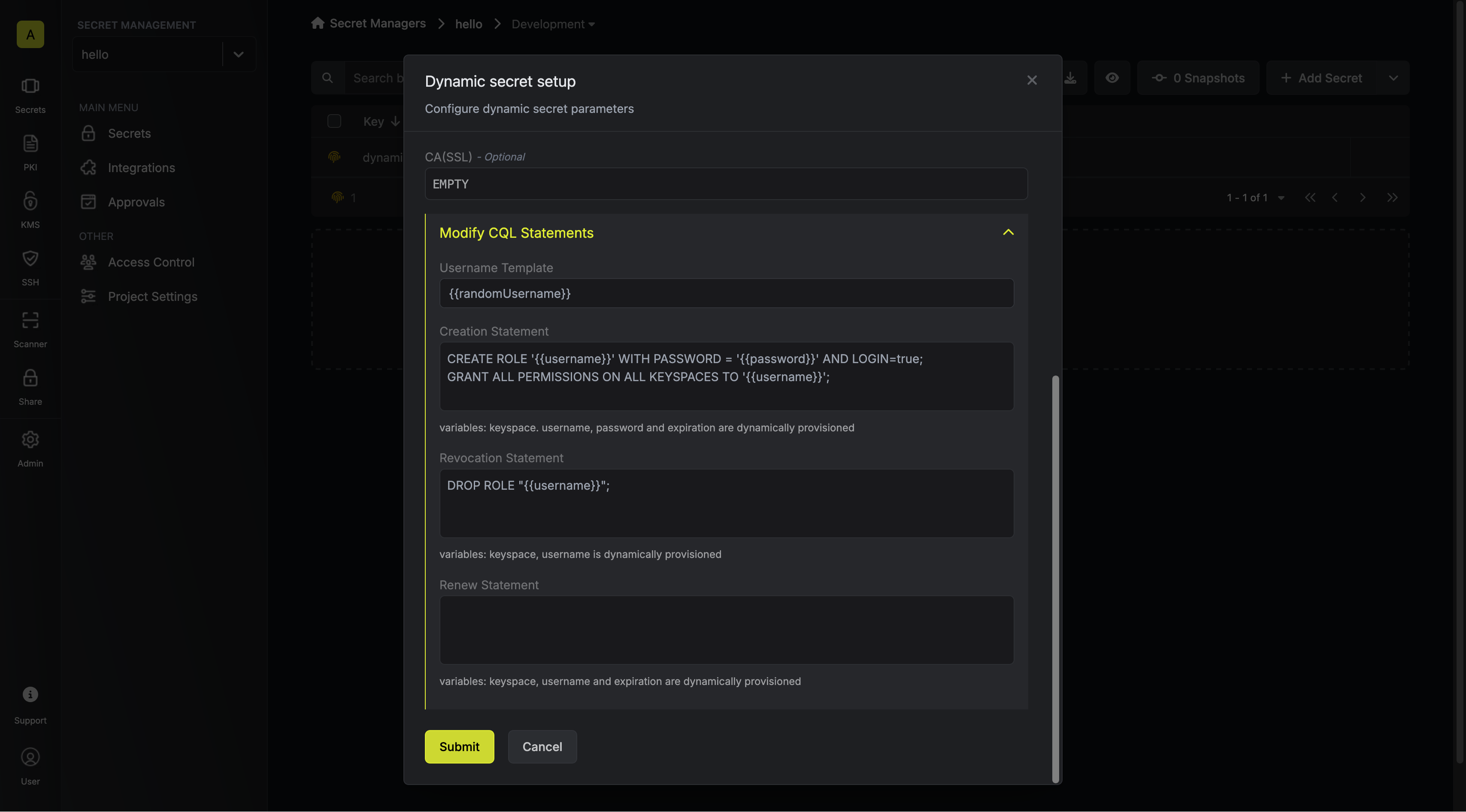
After submitting the form, you will see a dynamic secret created in the dashboard.
If this step fails, you may have to add the CA certficate.

Once you've successfully configured the dynamic secret, you're ready to generate on-demand credentials.
To do this, simply click on the 'Generate' button which appears when hovering over the dynamic secret item.
Alternatively, you can initiate the creation of a new lease by selecting 'New Lease' from the dynamic secret lease list section.
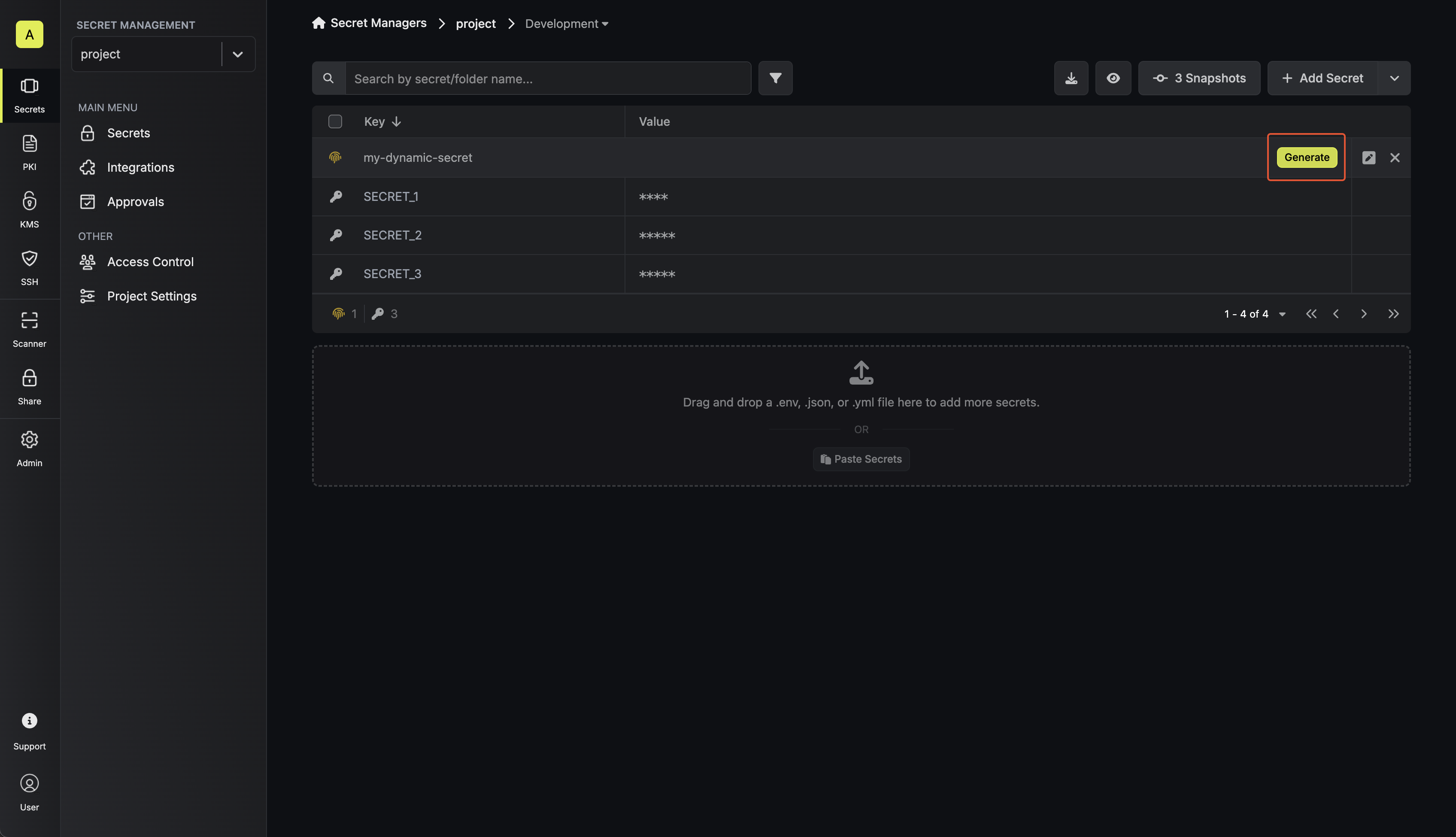
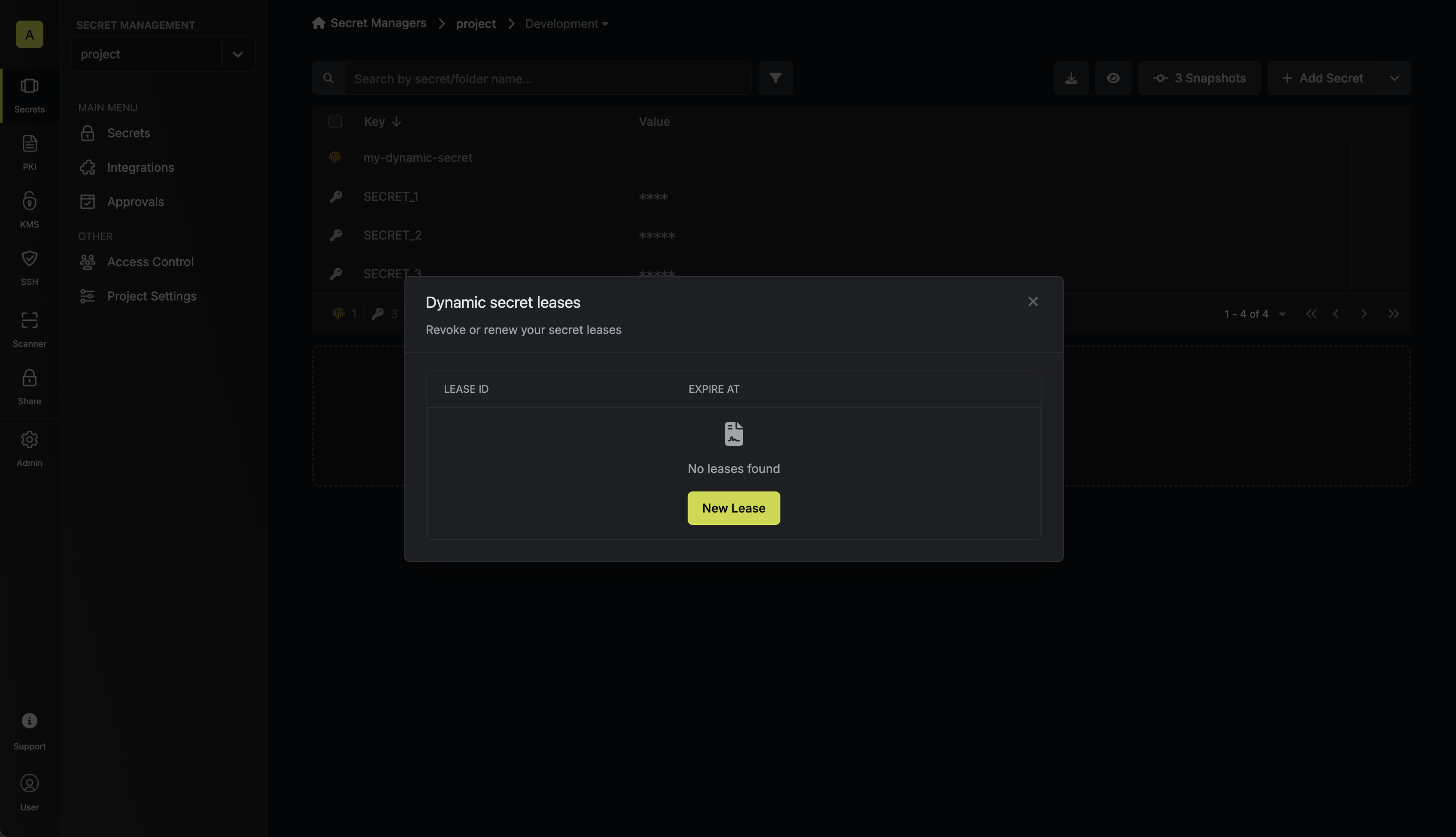
When generating these secrets, it's important to specify a Time-to-Live (TTL) duration. This will dictate how long the credentials are valid for.
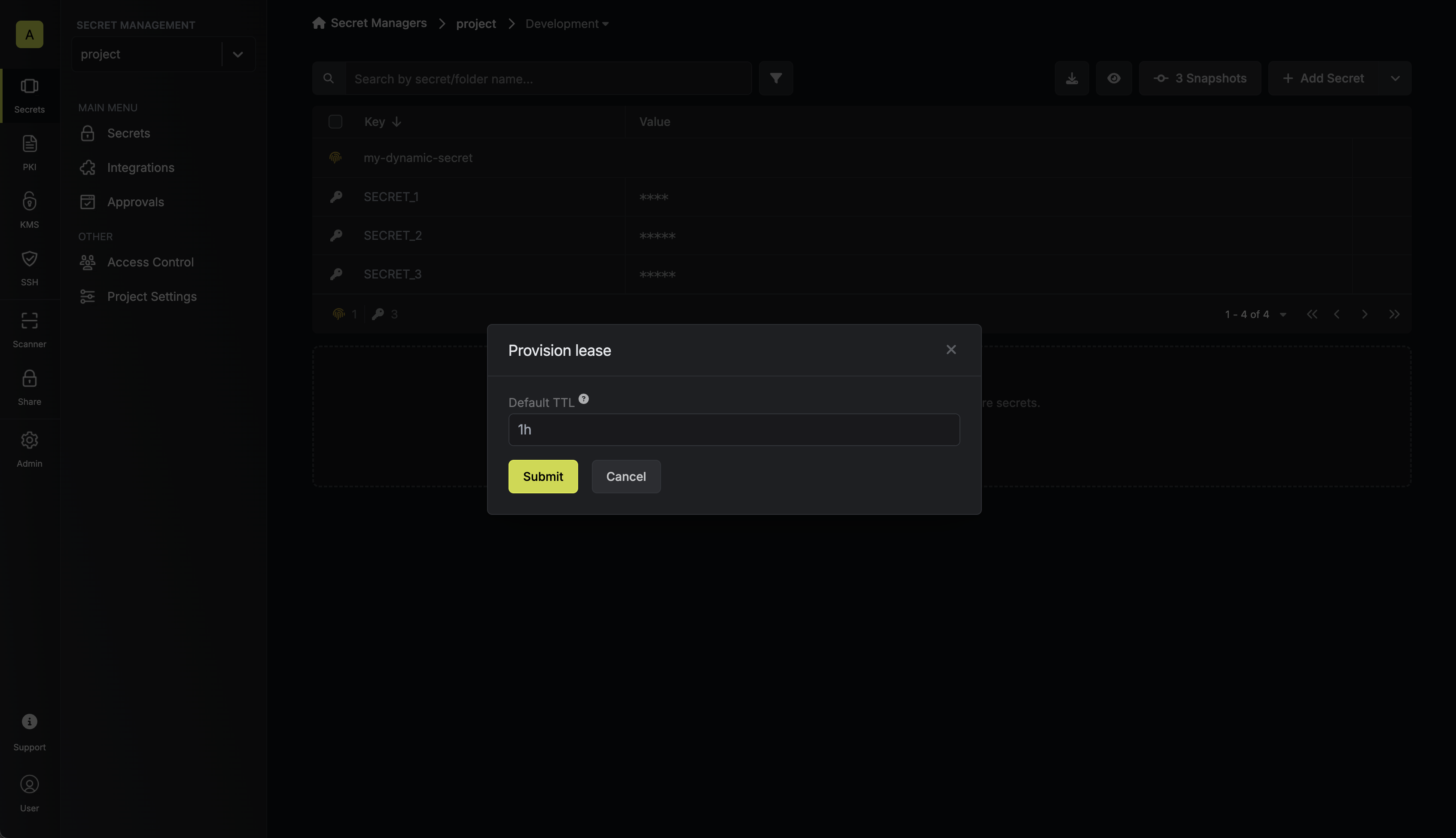
Ensure that the TTL for the lease fall within the maximum TTL defined when configuring the dynamic secret in step 4.
Once you click the `Submit` button, a new secret lease will be generated and the credentials for it will be shown to you.
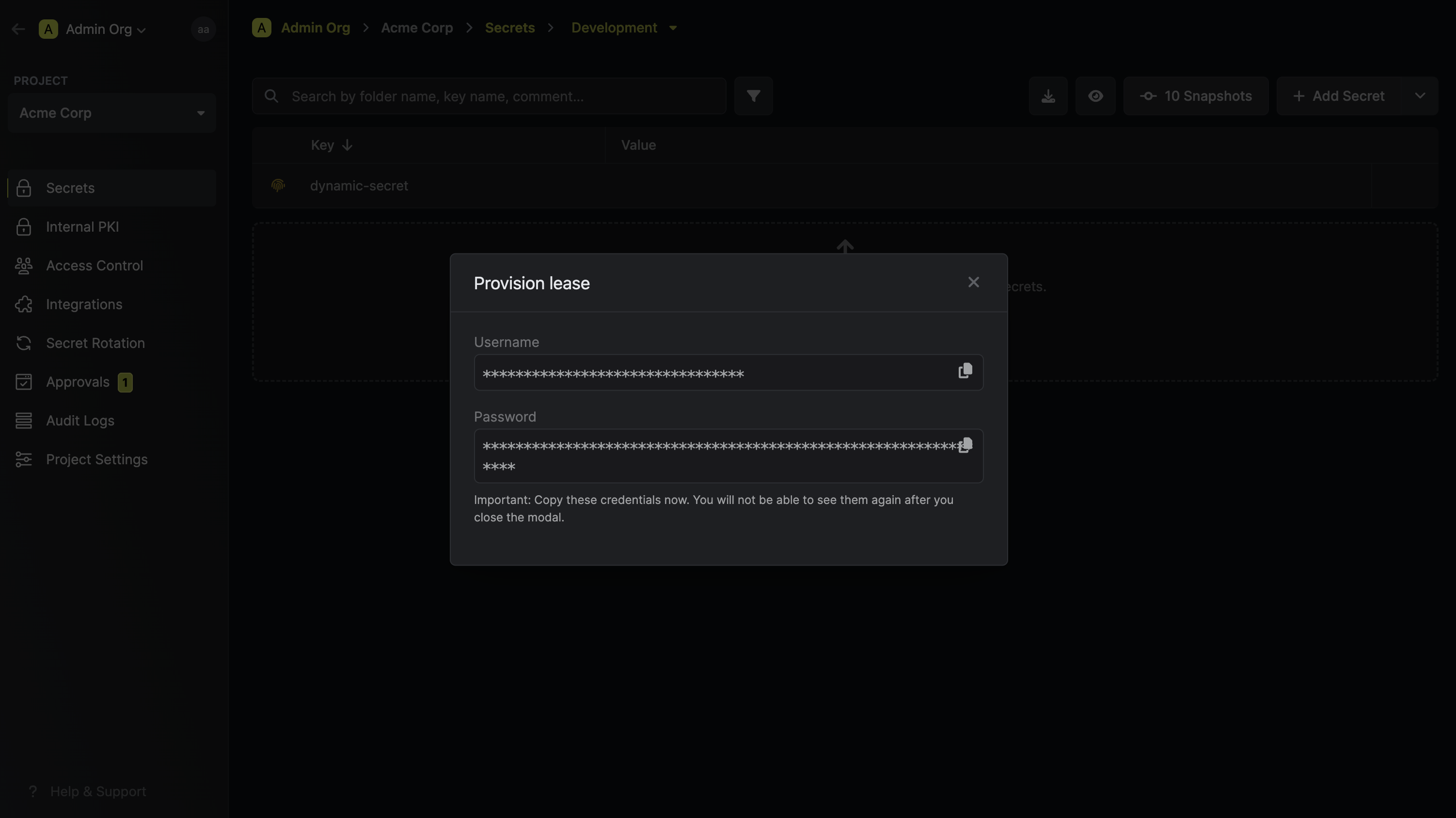
## Audit or Revoke Leases
Once you have created one or more leases, you will be able to access them by clicking on the respective dynamic secret item on the dashboard.
This will allow you see the lease details and delete the lease ahead of its expiration time.
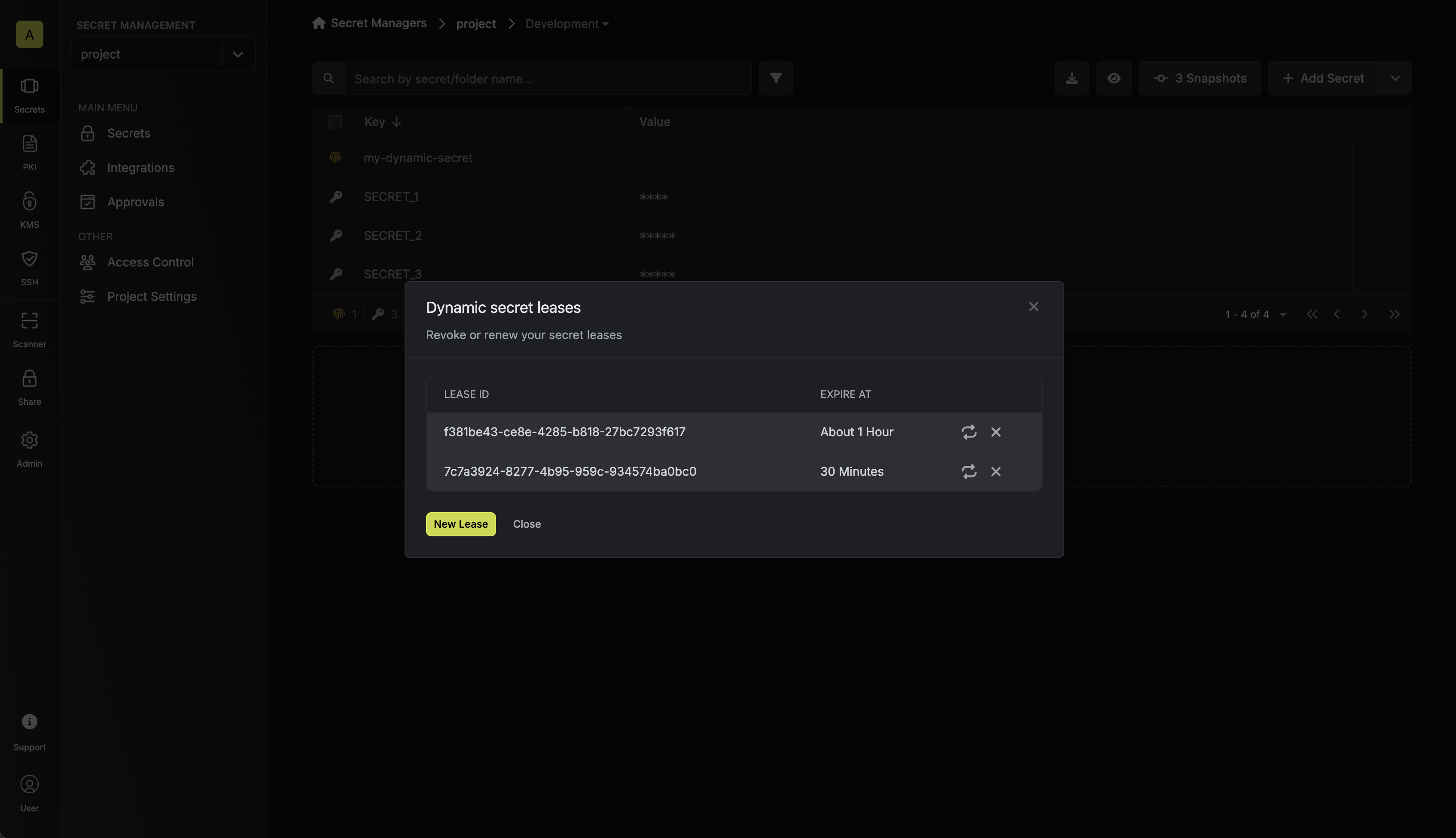
## Renew Leases
To extend the life of the generated dynamic secret lease past its initial time to live, simply click on the **Renew** as illustrated below.
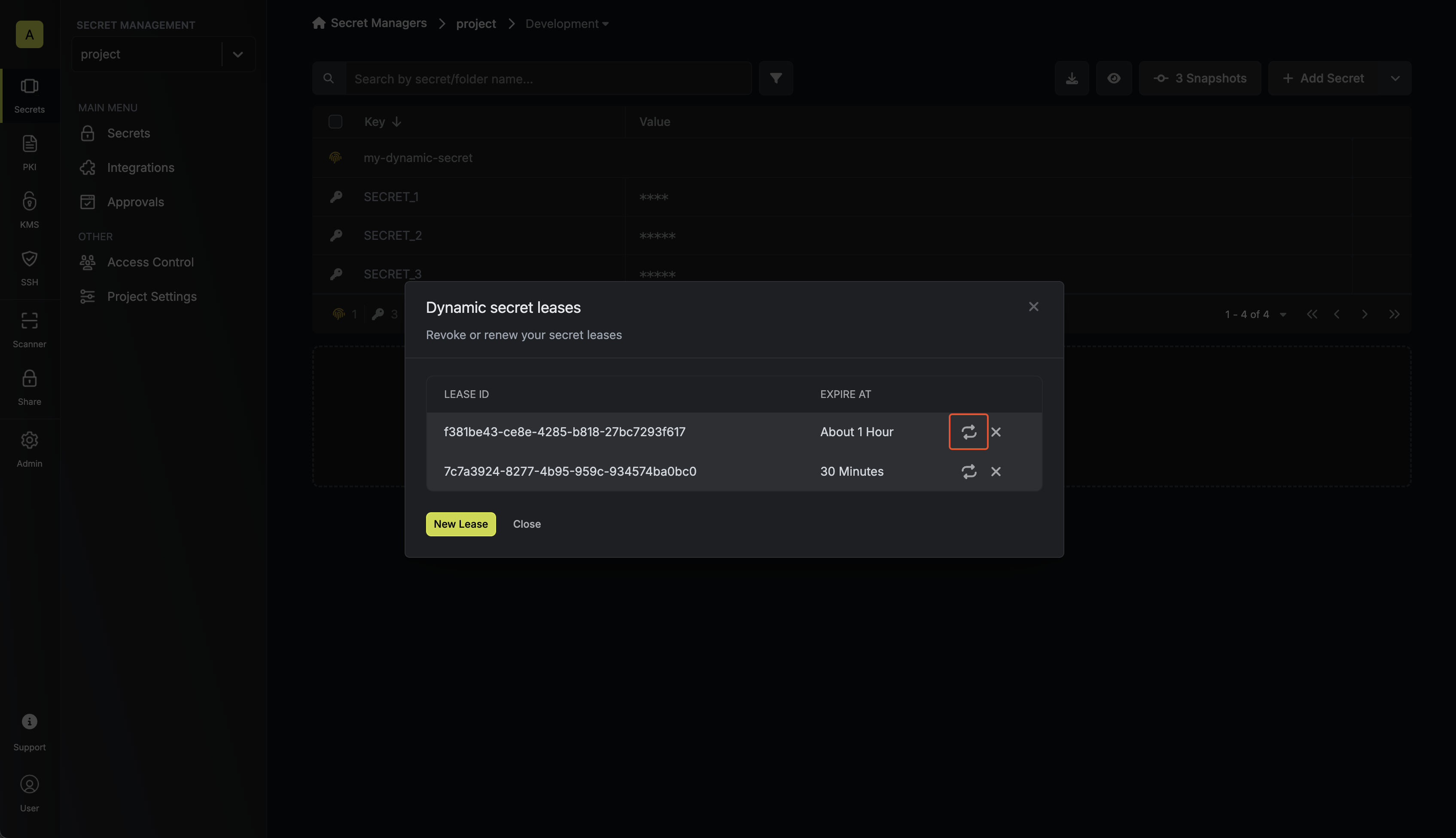
Lease renewals cannot exceed the maximum TTL set when configuring the dynamic secret
# Elasticsearch
Learn how to dynamically generate Elasticsearch user credentials.
The Infisical Elasticsearch dynamic secret allows you to generate Elasticsearch credentials on demand based on configured role.
## Prerequisites
1. Create a role with at least `manage_security` and `monitor` permissions.
2. Assign the newly created role to your API key or user that you'll use later in the dynamic secret configuration.
For testing purposes, you can also use a highly privileged role like `superuser`, that will have full control over the cluster. This is not recommended in production environments following the principle of least privilege.
## Set up Dynamic Secrets with Elasticsearch
Open the Secret Overview dashboard and select the environment in which you would like to add a dynamic secret.
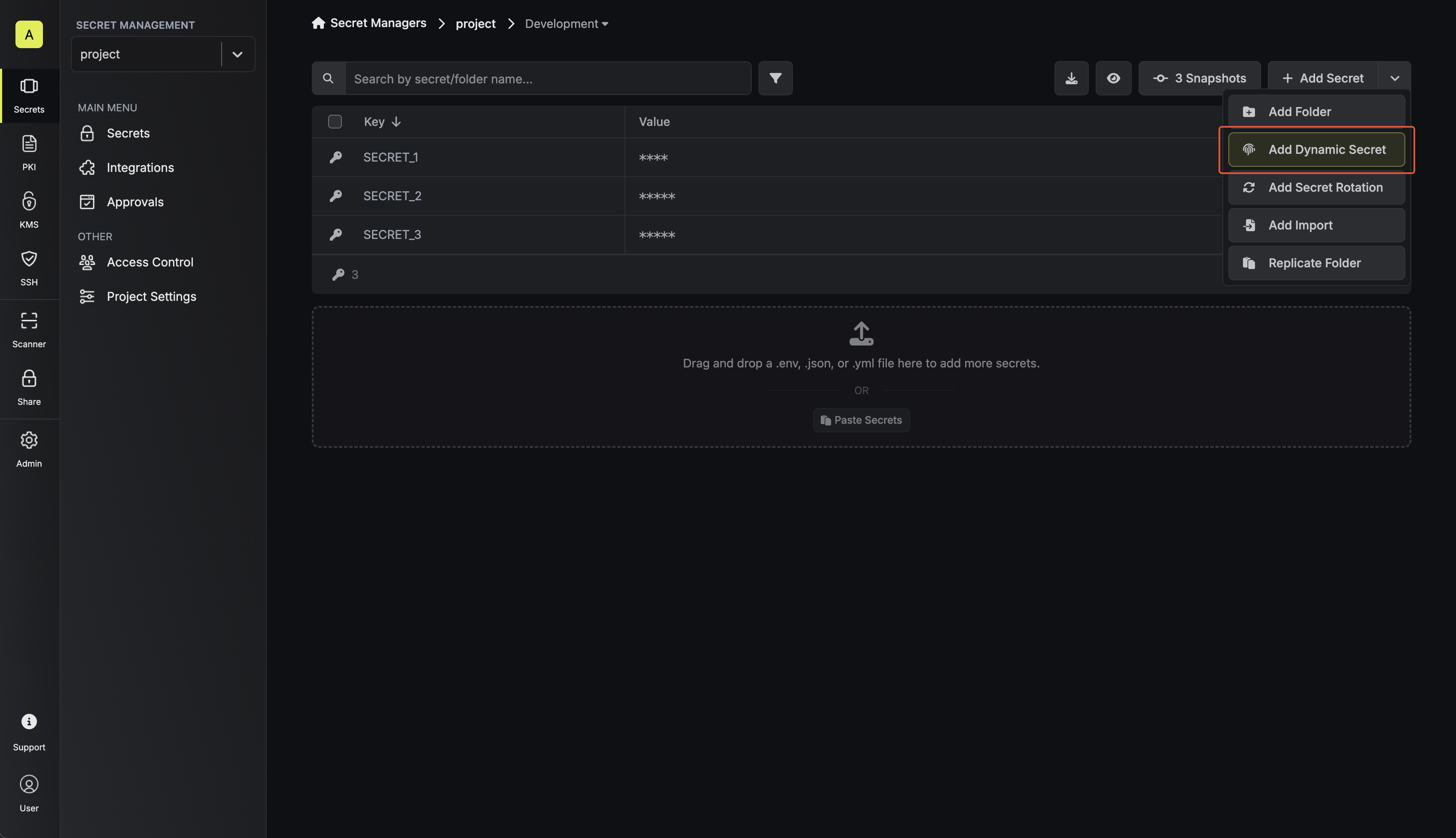
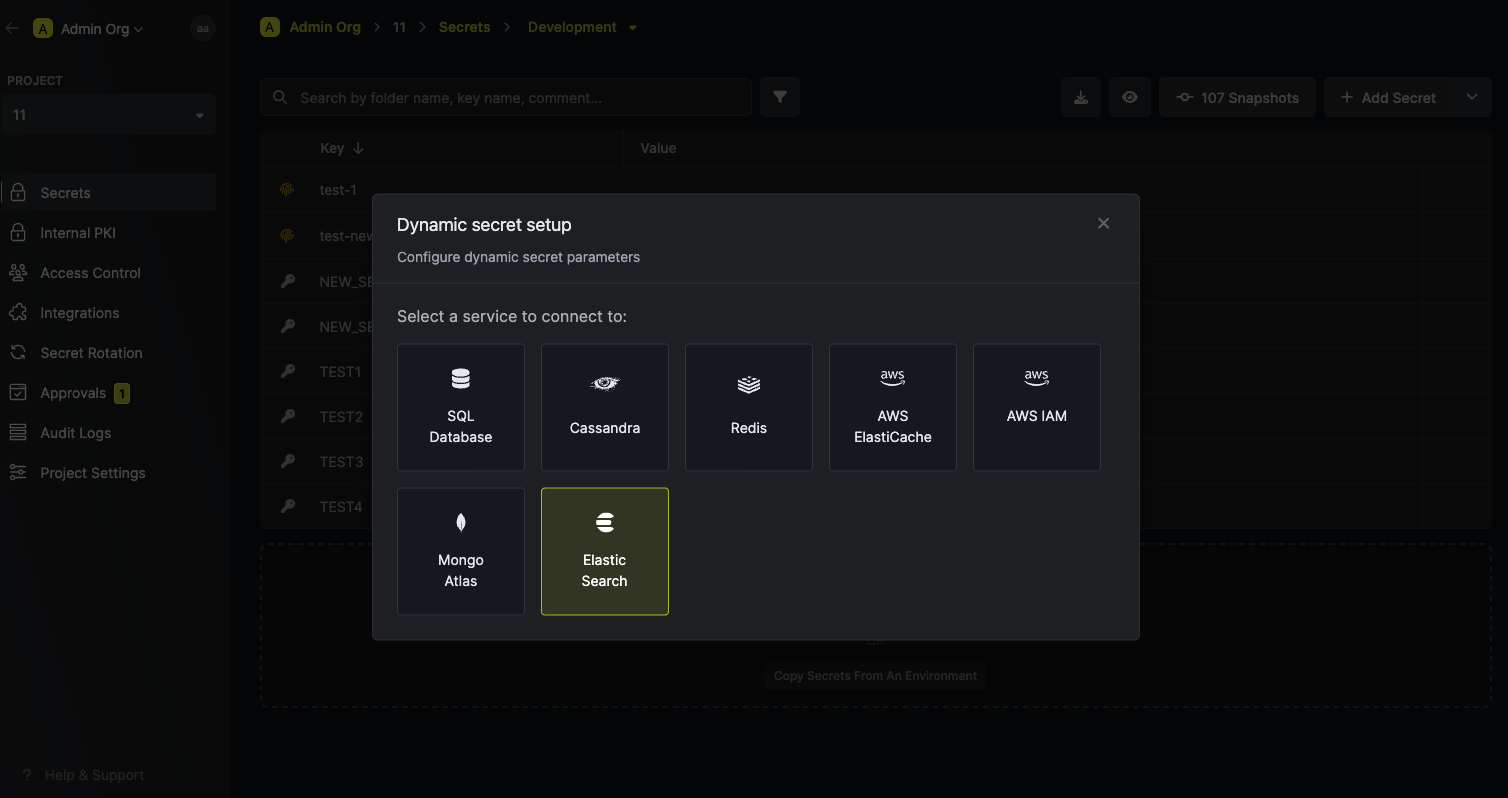
Name by which you want the secret to be referenced
Default time-to-live for a generated secret (it is possible to modify this value when a secret is generate)
Maximum time-to-live for a generated secret.
Your Elasticsearch host. This is the endpoint that your instance runs on. *(Example: [https://your-cluster-ip](https://your-cluster-ip))*
The port that your Elasticsearch instance is running on. *(Example: 9200)*
The roles that the new user that is created when a lease is provisioned will be assigned to. This is a required field. This defaults to `superuser`, which is highly privileged. It is recommended to create a new role with the least privileges required for the lease.
Select the authentication method you want to use to connect to your Elasticsearch instance.
The username of the user that will be used to provision new dynamic secret leases. Only required if you selected the `Username/Password` authentication method.
The password of the user that will be used to provision new dynamic secret leases. Only required if you selected the `Username/Password` authentication method.
The ID of the API key that will be used to provision new dynamic secret leases. Only required if you selected the `API Key` authentication method.
The API key that will be used to provision new dynamic secret leases. Only required if you selected the `API Key` authentication method.
A CA may be required if your DB requires it for incoming connections. This is often the case when connecting to a managed service.
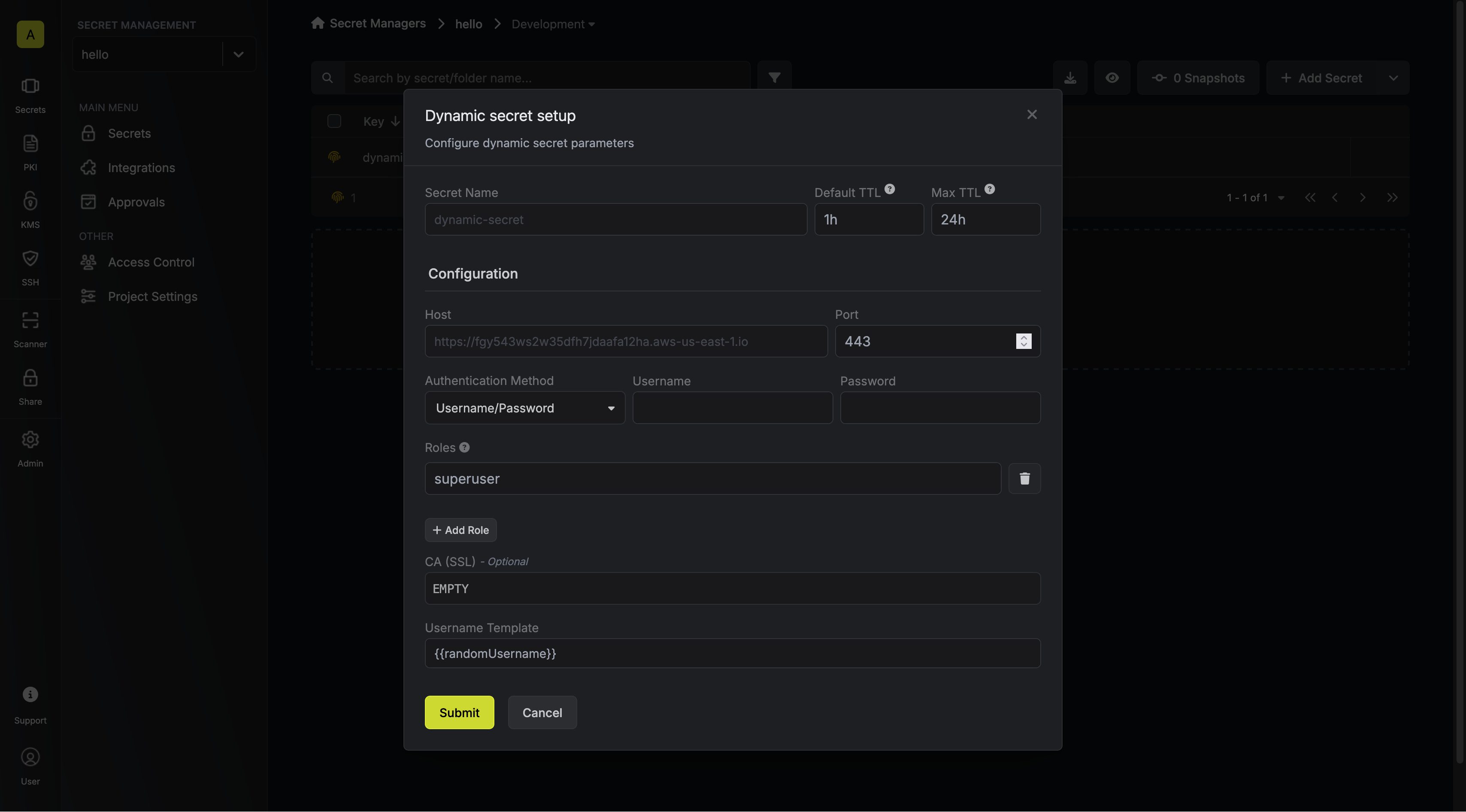
After submitting the form, you will see a dynamic secret created in the dashboard.
If this step fails, you may have to add the CA certificate.
Once you've successfully configured the dynamic secret, you're ready to generate on-demand credentials.
To do this, simply click on the 'Generate' button which appears when hovering over the dynamic secret item.
Alternatively, you can initiate the creation of a new lease by selecting 'New Lease' from the dynamic secret lease list section.


When generating these secrets, it's important to specify a Time-to-Live (TTL) duration. This will dictate how long the credentials are valid for.
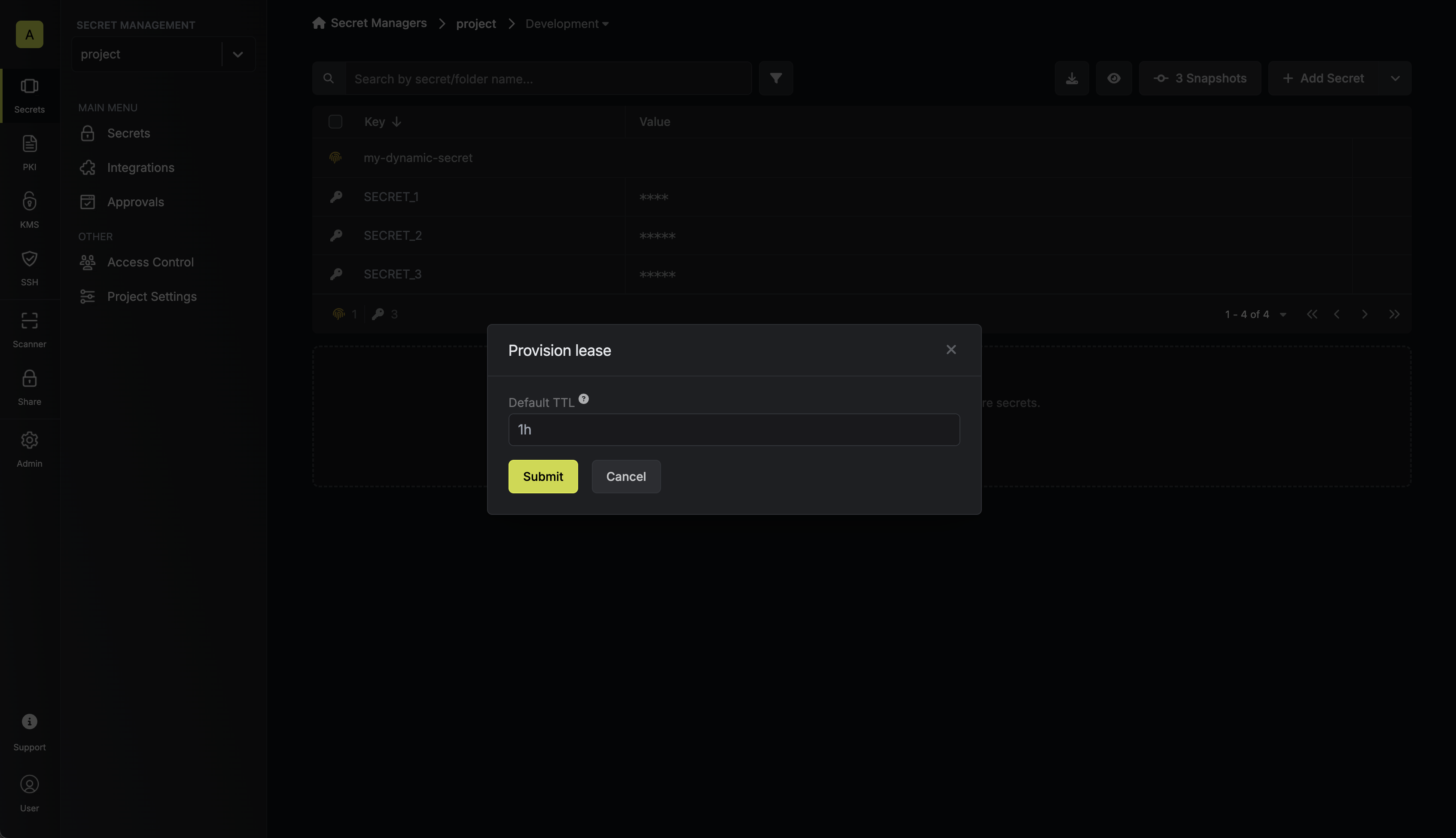
Ensure that the TTL for the lease fall within the maximum TTL defined when configuring the dynamic secret.
Once you click the `Submit` button, a new secret lease will be generated and the credentials from it will be shown to you.
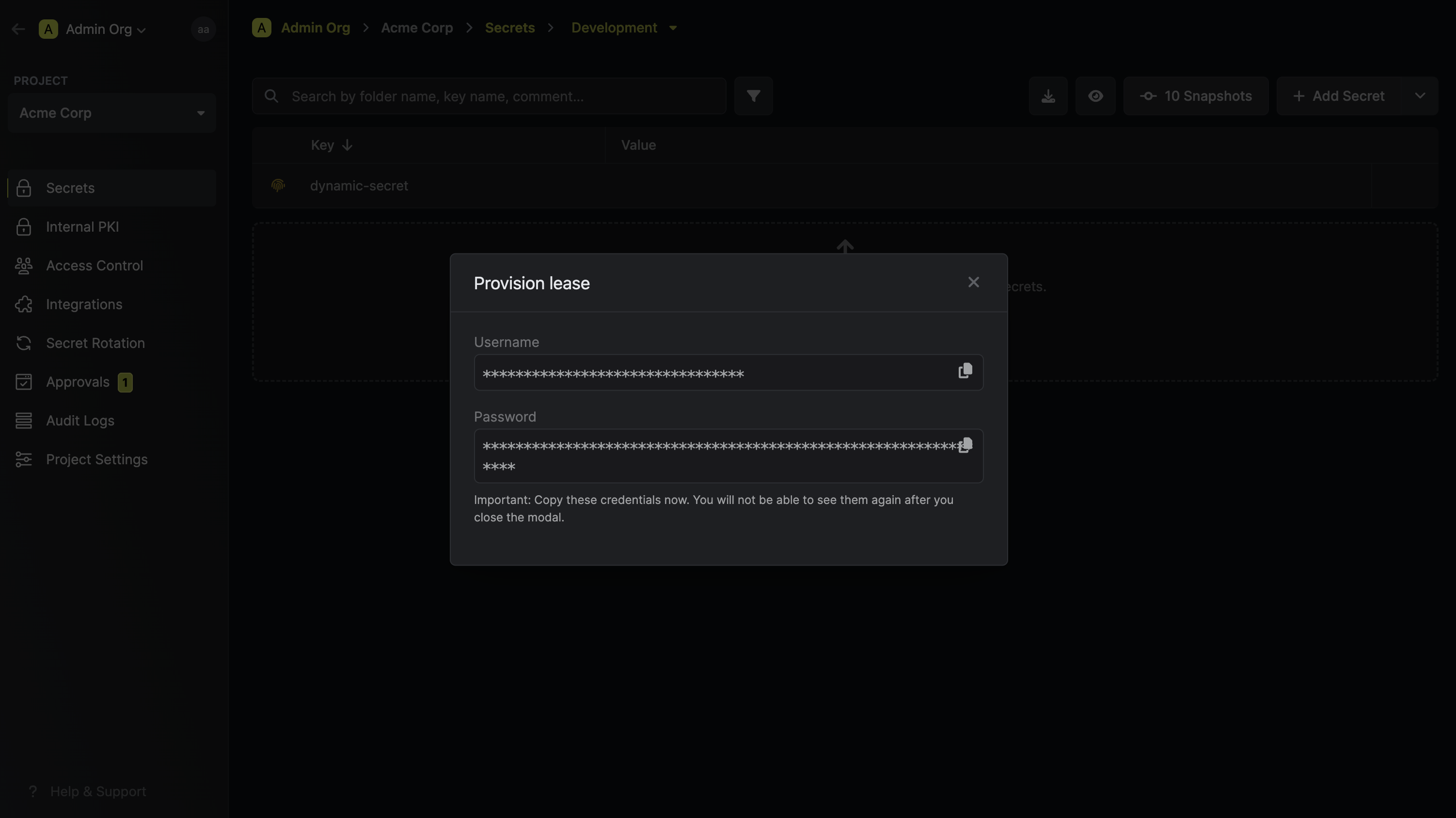
## Audit or Revoke Leases
Once you have created one or more leases, you will be able to access them by clicking on the respective dynamic secret item on the dashboard.
This will allow you see the expiration time of the lease or delete a lease before it's set time to live.
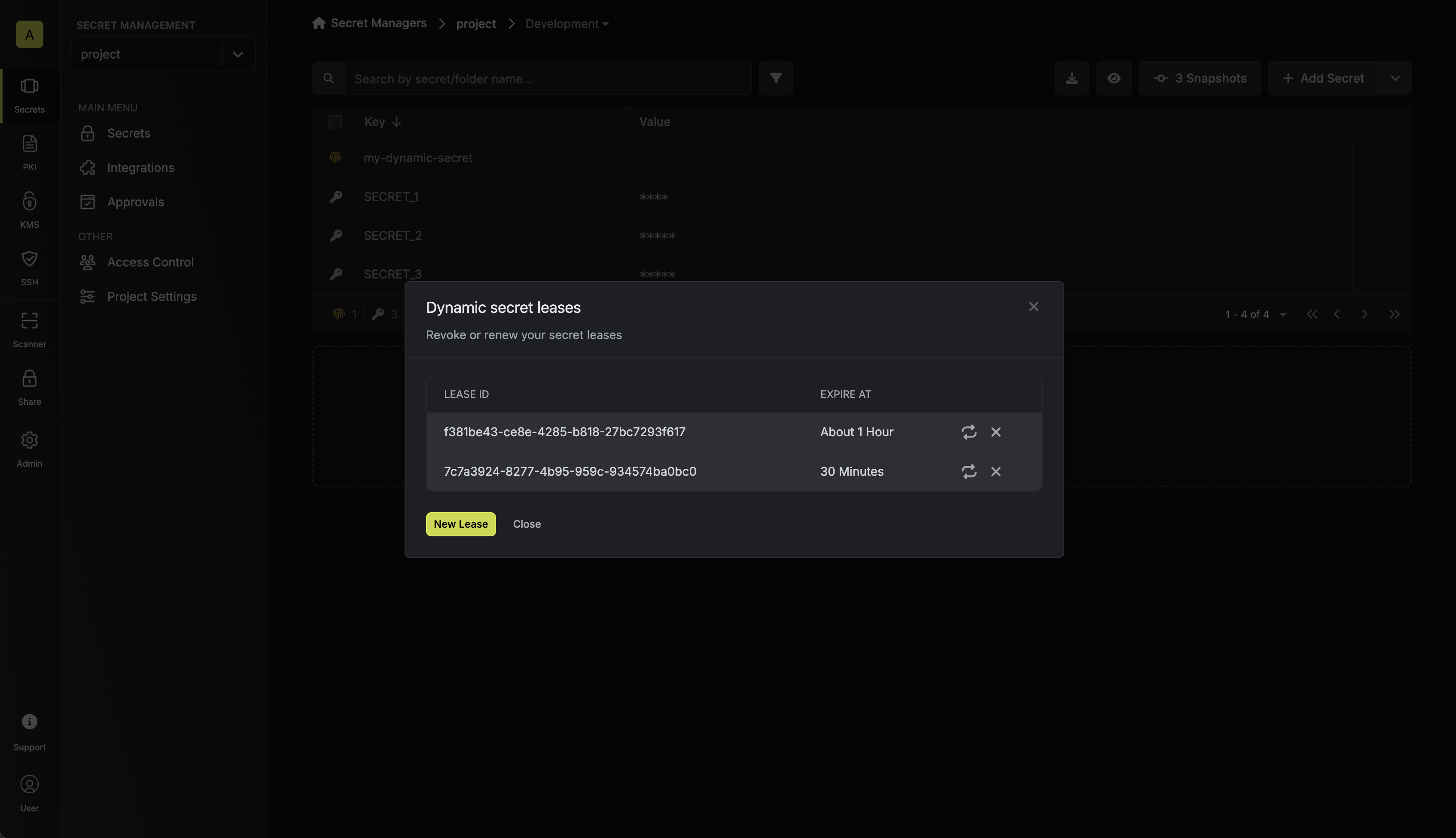
## Renew Leases
To extend the life of the generated dynamic secret leases past its initial time to live, simply click on the **Renew** as illustrated below.
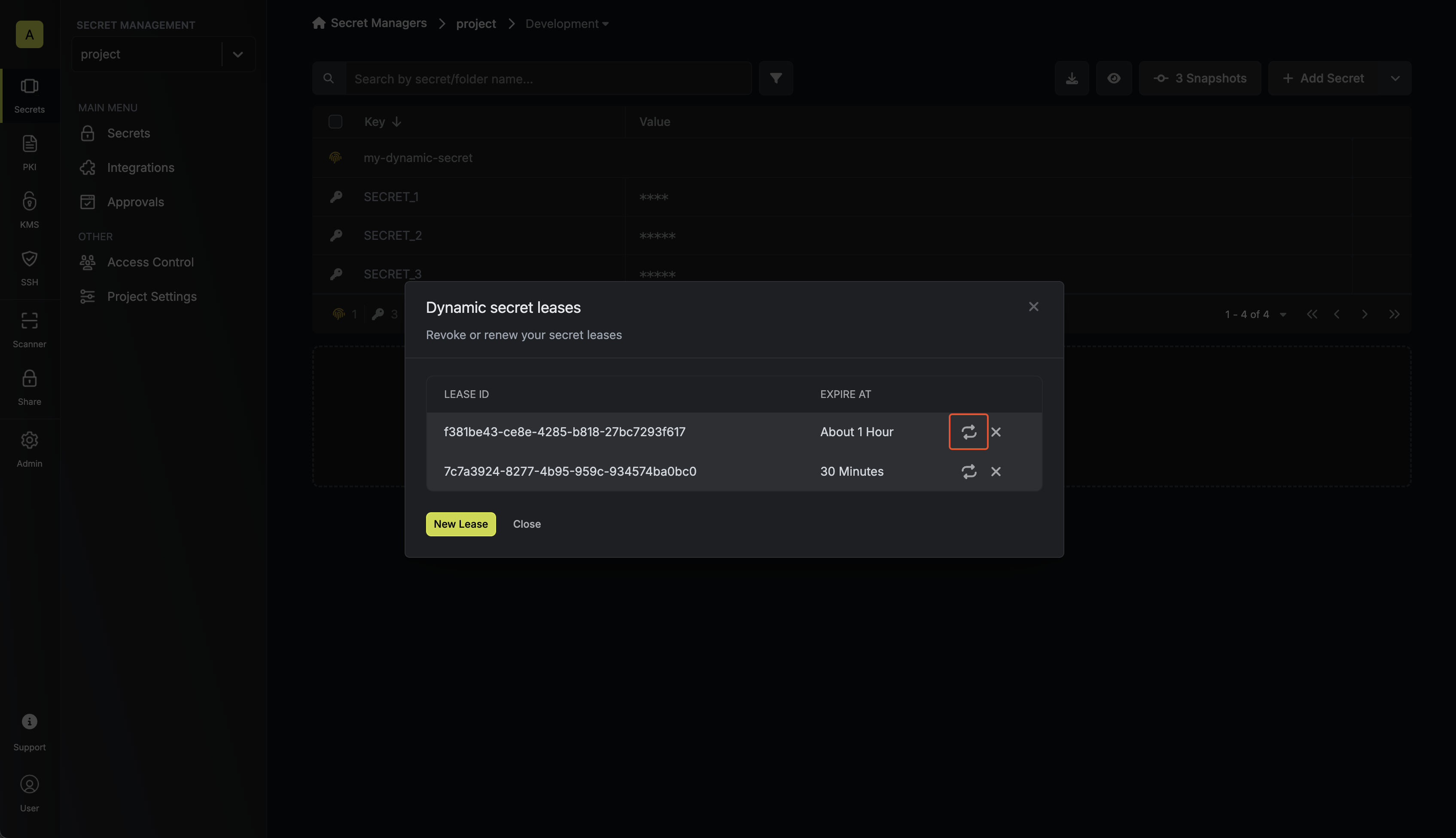
Lease renewals cannot exceed the maximum TTL set when configuring the dynamic secret
# LDAP
Learn how to dynamically generate user credentials via LDAP.
The Infisical LDAP dynamic secret allows you to generate user credentials on demand via LDAP. The integration is general to any LDAP implementation but has been tested with OpenLDAP and Active directory as of now.
## Prerequisites
1. Create a user with the necessary permissions to create users in your LDAP server.
2. Ensure your LDAP server is reachable via Infisical instance.
## Create LDAP Credentials
Open the Secret Overview dashboard and select the environment in which you would like to add a dynamic secret.
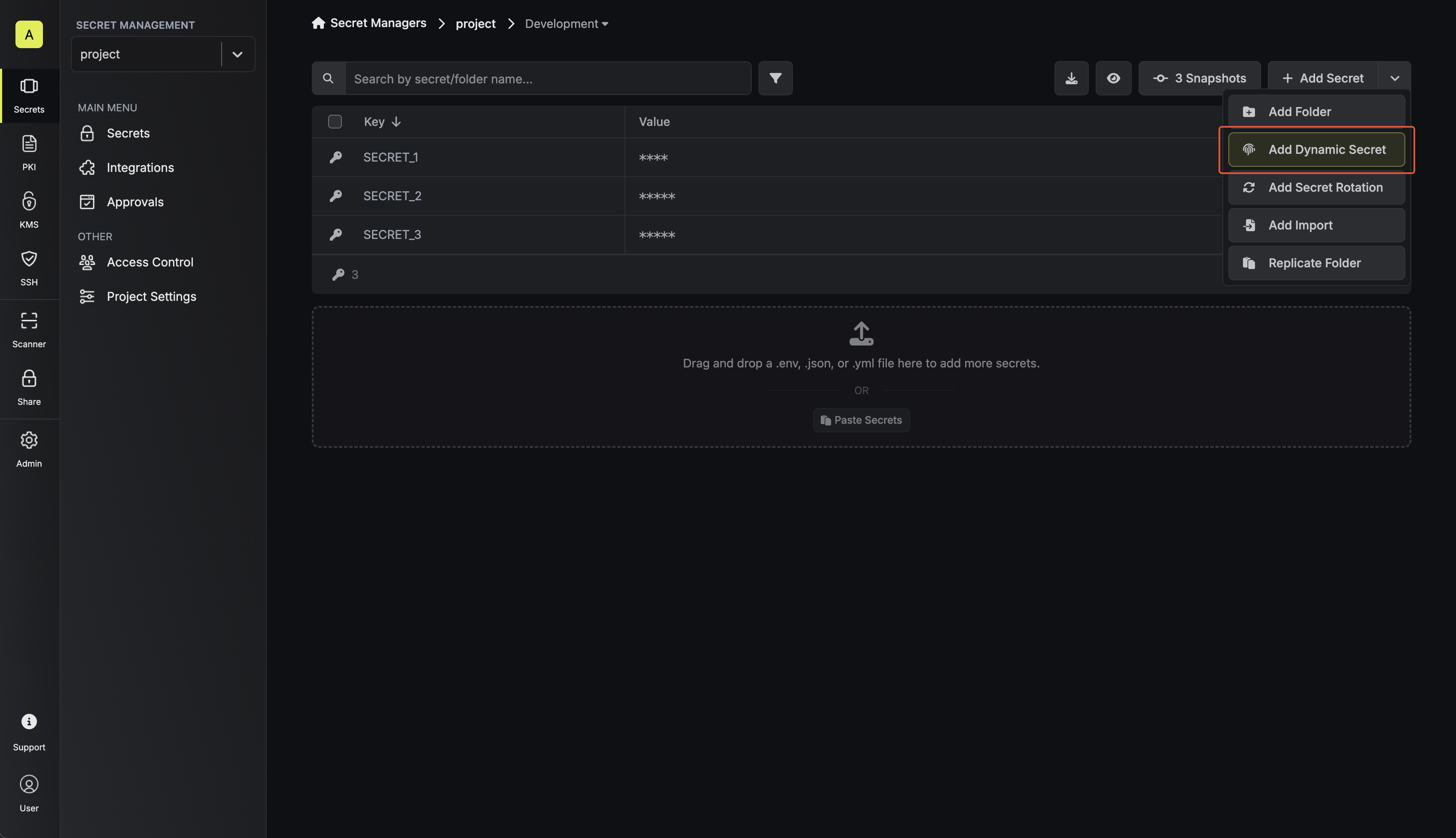
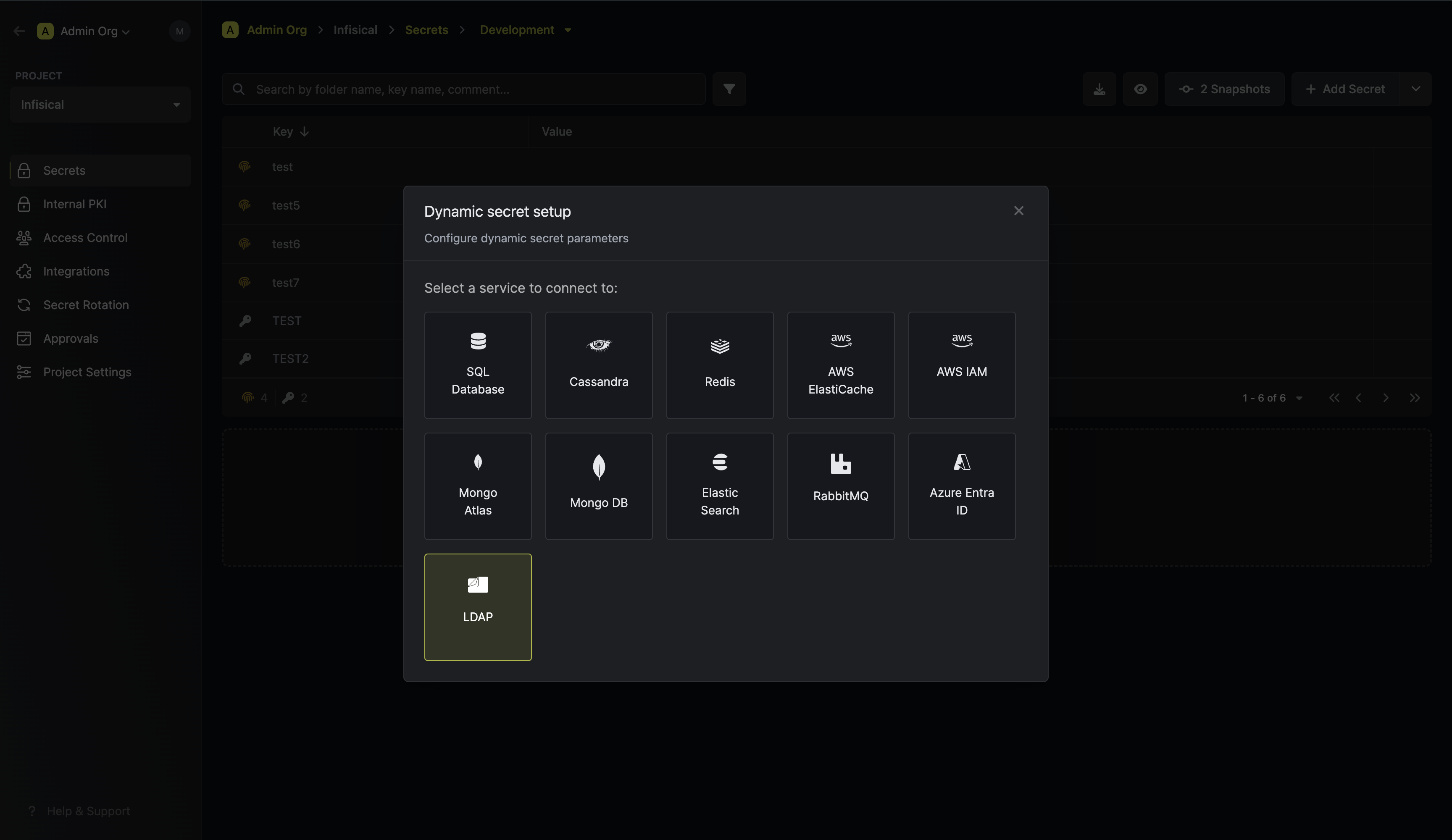
Name by which you want the secret to be referenced
Default time-to-live for a generated secret (it is possible to modify this value when a secret is generate)
Maximum time-to-live for a generated secret.
LDAP url to connect to. *(Example: ldap\://your-ldap-ip:389 or ldaps\://domain:636)*
DN to bind to. This should have permissions to create a new users.
Password for the given DN.
CA certificate to use for TLS in case of a secure connection.
The type of LDAP credential - select Dynamic.
LDIF to run while creating a user in LDAP. This can include extra steps to assign the user to groups or set permissions.
Here `{{Username}}`, `{{Password}}` and `{{EncodedPassword}}` are templatized variables for the username and password generated by the dynamic secret.
`{{EncodedPassword}}` is the encoded password required for the `unicodePwd` field in Active Directory as described [here](https://learn.microsoft.com/en-us/troubleshoot/windows-server/active-directory/change-windows-active-directory-user-password).
**OpenLDAP** Example:
```
dn: uid={{Username}},dc=infisical,dc=com
changetype: add
objectClass: top
objectClass: person
objectClass: organizationalPerson
objectClass: inetOrgPerson
cn: John Doe
sn: Doe
uid: jdoe
mail: jdoe@infisical.com
userPassword: {{Password}}
```
**Active Directory** Example:
```
dn: CN={{Username}},OU=Test Create,DC=infisical,DC=com
changetype: add
objectClass: top
objectClass: person
objectClass: organizationalPerson
objectClass: user
userPrincipalName: {{Username}}@infisical.com
sAMAccountName: {{Username}}
unicodePwd::{{EncodedPassword}}
userAccountControl: 66048
dn: CN=test-group,OU=Test Create,DC=infisical,DC=com
changetype: modify
add: member
member: CN={{Username}},OU=Test Create,DC=infisical,DC=com
-
```
LDIF to run while revoking a user in LDAP. This can include extra steps to remove the user from groups or set permissions.
Here `{{Username}}` is a templatized variable for the username generated by the dynamic secret.
**OpenLDAP / Active Directory** Example:
```
dn: CN={{Username}},OU=Test Create,DC=infisical,DC=com
changetype: delete
```
LDIF to run incase Creation LDIF fails midway.
For the creation example shown above, if the user is created successfully but not added to a group, this LDIF can be used to remove the user.
Here `{{Username}}`, `{{Password}}` and `{{EncodedPassword}}` are templatized variables for the username generated by the dynamic secret.
**OpenLDAP / Active Directory** Example:
```
dn: CN={{Username}},OU=Test Create,DC=infisical,DC=com
changetype: delete
```
After submitting the form, you will see a dynamic secret created in the dashboard.
Once you've successfully configured the dynamic secret, you're ready to generate on-demand credentials.
To do this, simply click on the 'Generate' button which appears when hovering over the dynamic secret item.
Alternatively, you can initiate the creation of a new lease by selecting 'New Lease' from the dynamic secret lease list section.


When generating these secrets, it's important to specify a Time-to-Live (TTL) duration. This will dictate how long the credentials are valid for.
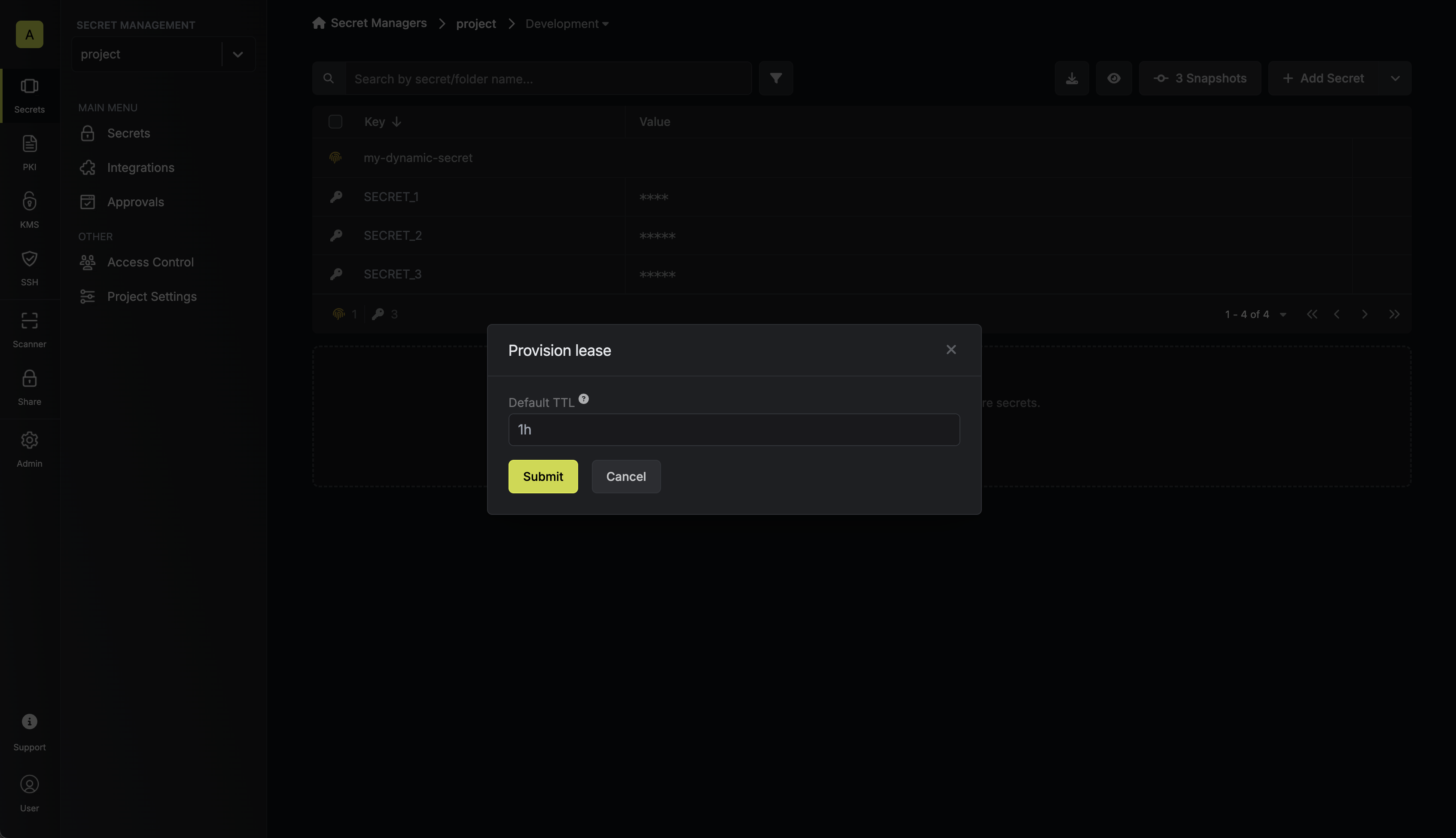
Ensure that the TTL for the lease fall within the maximum TTL defined when configuring the dynamic secret.
Once you click the `Submit` button, a new secret lease will be generated and the credentials from it will be shown to you with an array of DN's altered depending on the Creation LDIF.
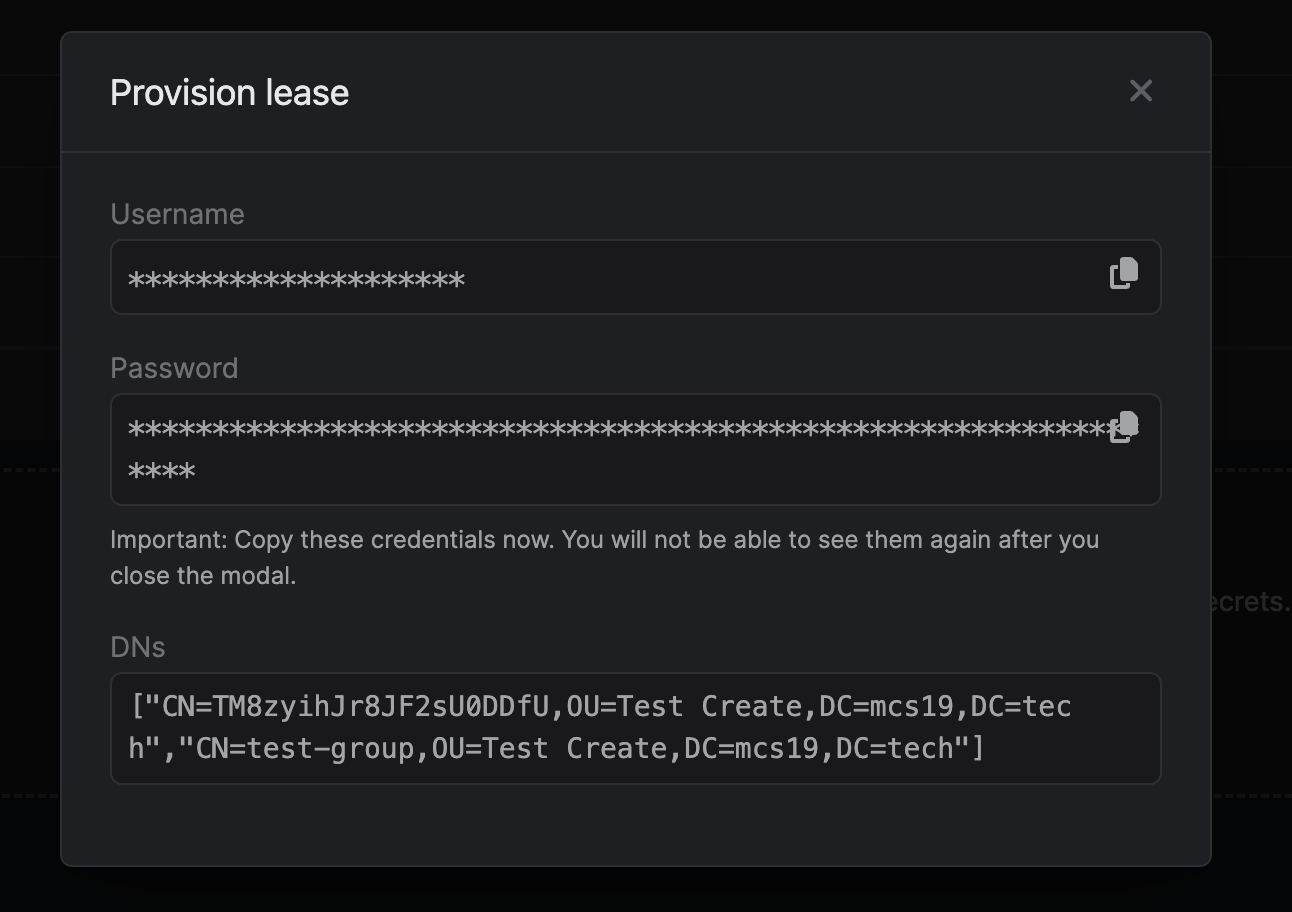
Open the Secret Overview dashboard and select the environment in which you would like to add a dynamic secret.
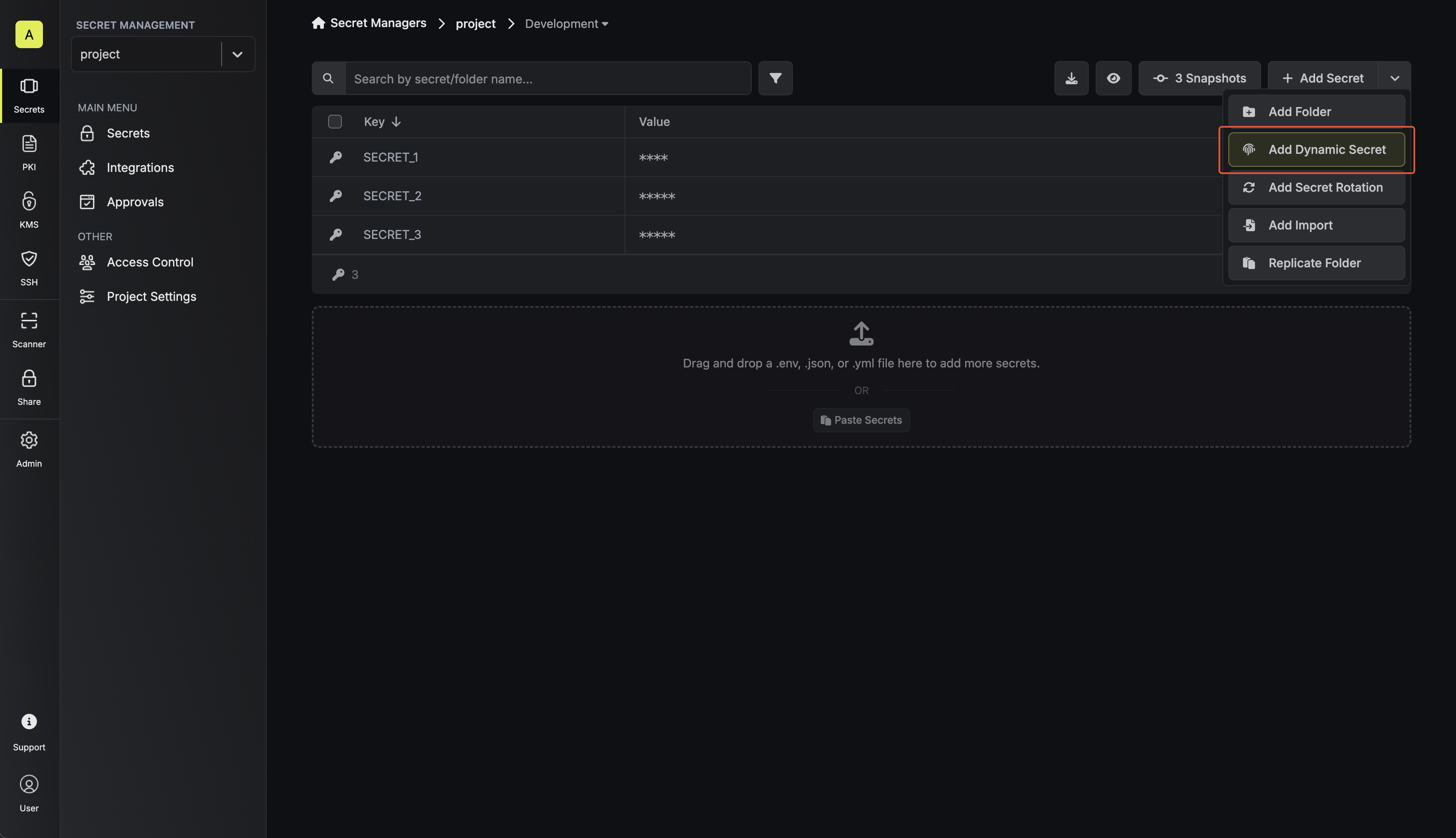
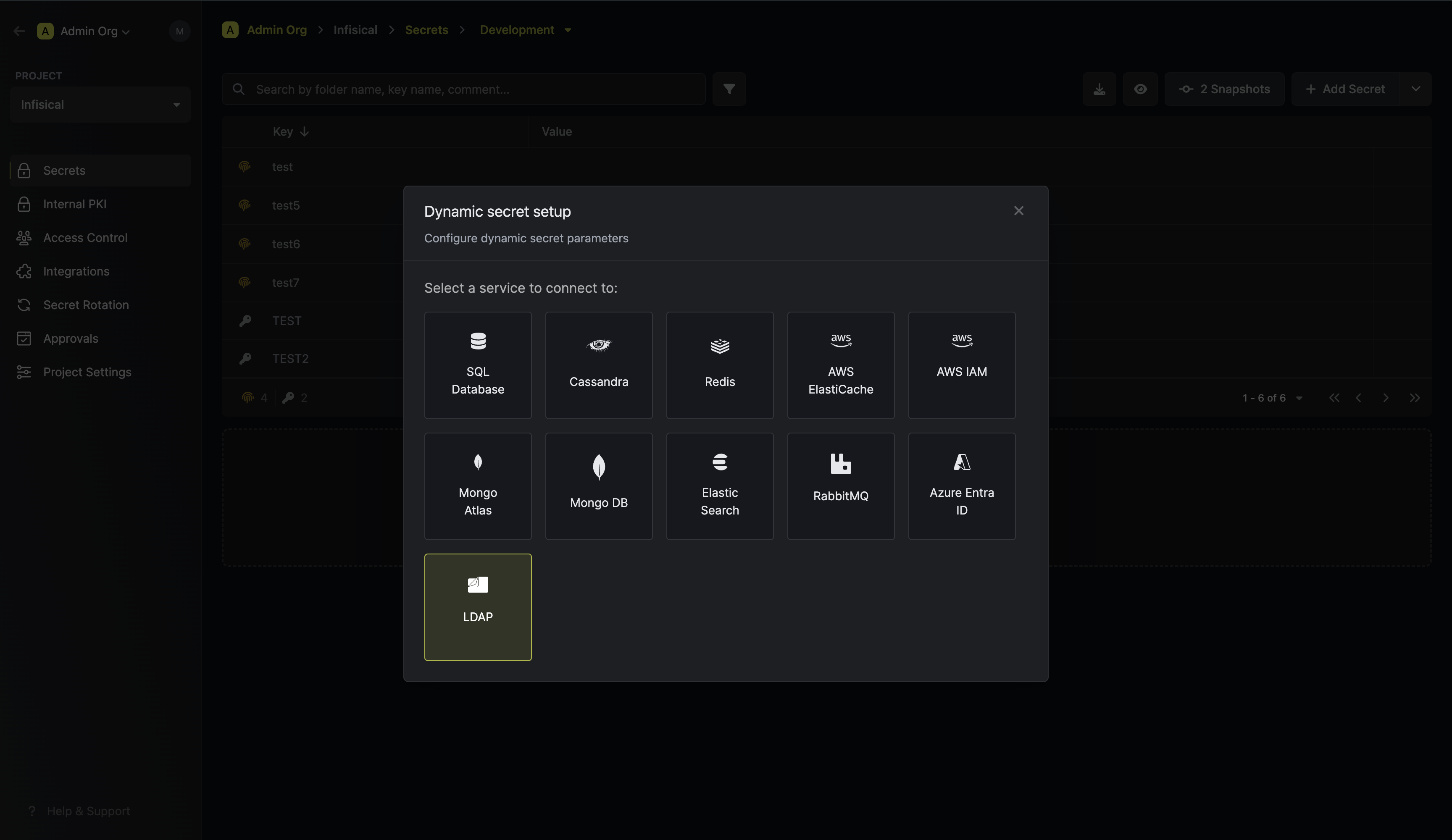
Name by which you want the secret to be referenced
Default time-to-live for a generated secret (it is possible to modify this value when a secret is generate)
Maximum time-to-live for a generated secret.
LDAP url to connect to. *(Example: ldap\://your-ldap-ip:389 or ldaps\://domain:636)*
DN to bind to. This should have permissions to create a new users.
Password for the given DN.
CA certificate to use for TLS in case of a secure connection.
The type of LDAP credential - select Static.
LDIF to run for rotating the credentals of an LDAP user. This can include extra LDAP steps based on your needs.
Here `{{Password}}` and `{{EncodedPassword}}` are templatized variables for the password generated by the dynamic secret.
Note that the `-` characters and the empty lines found at the end of the examples are necessary based on the LDIF format.
**OpenLDAP** Example:
```
dn: cn=sheencaps capadngan,ou=people,dc=acme,dc=com
changetype: modify
replace: userPassword
password: {{Password}}
-
```
**Active Directory** Example:
```
dn: cn=sheencaps capadngan,ou=people,dc=acme,dc=com
changetype: modify
replace: unicodePwd
unicodePwd::{{EncodedPassword}}
-
```
`{{EncodedPassword}}` is the encoded password required for the `unicodePwd` field in Active Directory as described [here](https://learn.microsoft.com/en-us/troubleshoot/windows-server/active-directory/change-windows-active-directory-user-password).
After submitting the form, you will see a dynamic secret created in the dashboard.
Once you've successfully configured the dynamic secret, you're ready to generate on-demand credentials.
To do this, simply click on the 'Generate' button which appears when hovering over the dynamic secret item.
Alternatively, you can initiate the creation of a new lease by selecting 'New Lease' from the dynamic secret lease list section.


When generating these secrets, it's important to specify a Time-to-Live (TTL) duration. This will dictate how long the credentials are valid for.
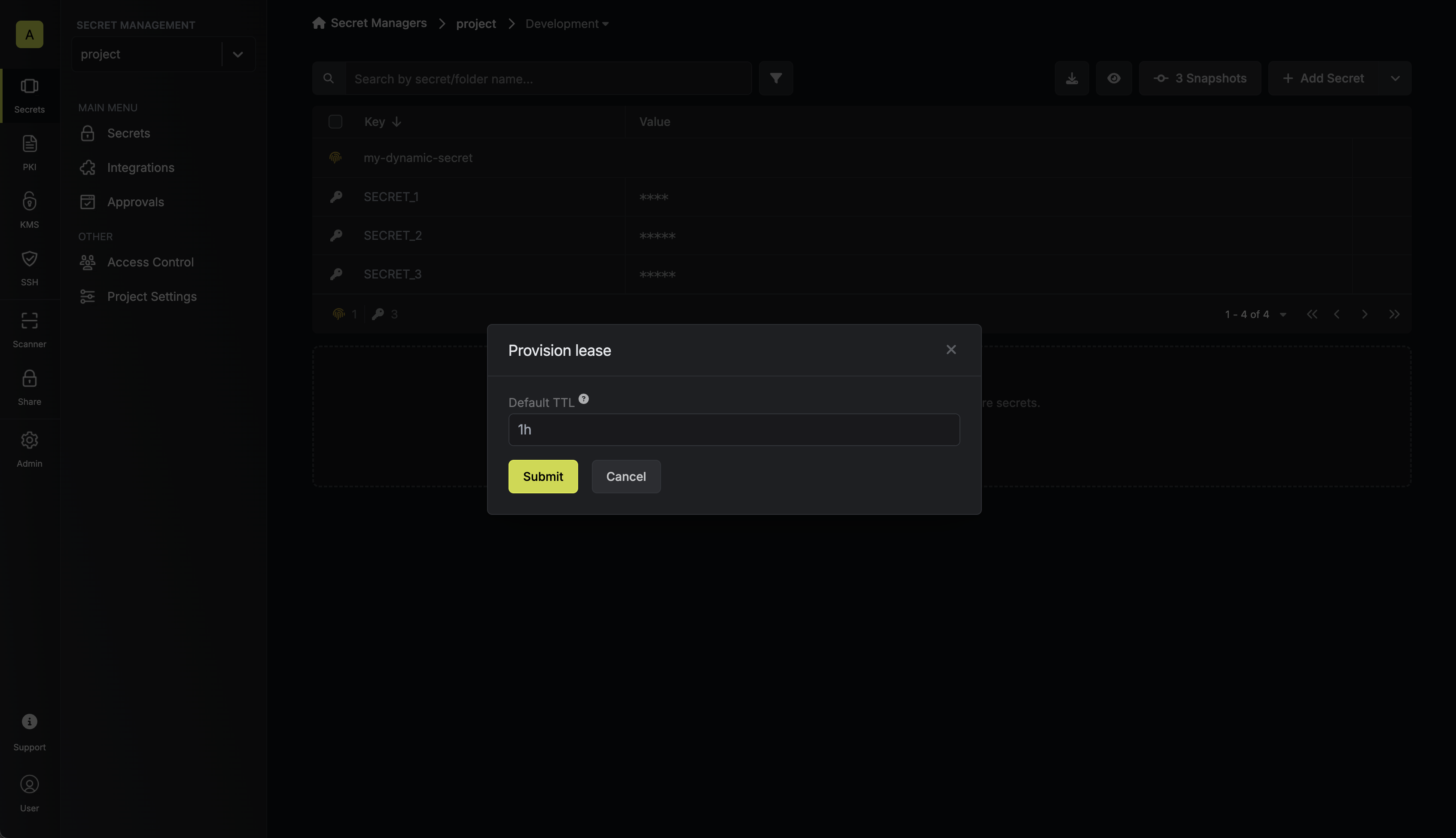
Ensure that the TTL for the lease fall within the maximum TTL defined when configuring the dynamic secret.
Once you click the `Submit` button, a new secret lease will be generated and the credentials from it will be shown to you with an array of DN's altered depending on the Creation LDIF.
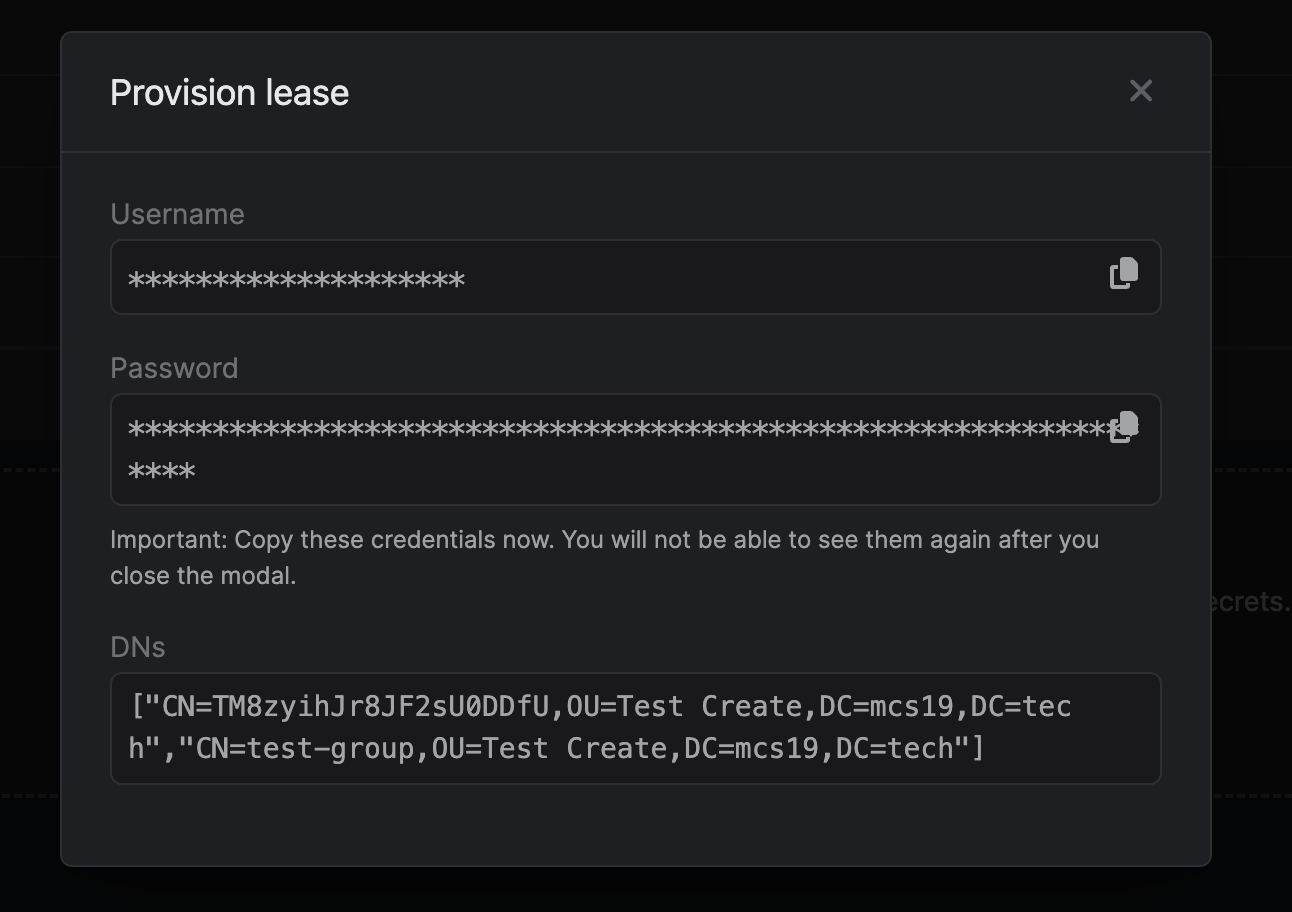
## Active Directory Integration
* Passwords in Active Directory are set using the `unicodePwd` field. This must be proceeded by two colons `::` as shown in the example. [Source](https://learn.microsoft.com/en-us/troubleshoot/windows-server/active-directory/change-windows-active-directory-user-password)
* Active directory uses the `userAccountControl` field to enable account. [Read More](https://learn.microsoft.com/en-us/troubleshoot/windows-server/active-directory/useraccountcontrol-manipulate-account-properties)
* `userAccountControl` set to `512` enables a user.
* To disable AD's password expiration for this dynamic user account. The `userAccountControl` value for this is: `65536`.
* Since `userAccountControl` flag is cumulative set it to `512 + 65536` = `66048` to do both.
* Active Directory does not permit direct modification of a user's `memberOf` attribute. The member attribute of a group and the `memberOf` attribute of a user are [linked attributes](https://learn.microsoft.com/en-us/windows/win32/ad/linked-attributes), where the member attribute represents the forward link, which can be modified. In the context of AD group membership, the group's `member` attribute serves as the forward link. Therefore, to add a newly created dynamic user to a group, a modification request must be issued to the desired group, updating its membership to include the new user.
## LDIF Entries
User account management is handled through **LDIF entries**.
#### Things to Remember
* **No trailing spaces:** Ensure there are no trailing spaces on any line, including blank lines.
* **Empty lines before modify blocks:** Every modify block must be preceded by an empty line.
* **Multiple modifications:** You can define multiple modifications for a DN within a single modify block. Each modification should end with a single dash (`-`).
# Mongo Atlas
Learn how to dynamically generate Mongo Atlas Database user credentials.
The Infisical Mongo Atlas dynamic secret allows you to generate Mongo Atlas Database credentials on demand based on configured role.
## Prerequisite
Create a project scopped API Key with the required permission in your Mongo Atlas following the [official doc](https://www.mongodb.com/docs/atlas/configure-api-access/#grant-programmatic-access-to-a-project).
The API Key must have permission to manage users in the project.
## Set up Dynamic Secrets with Mongo Atlas
Open the Secret Overview dashboard and select the environment in which you would like to add a dynamic secret.
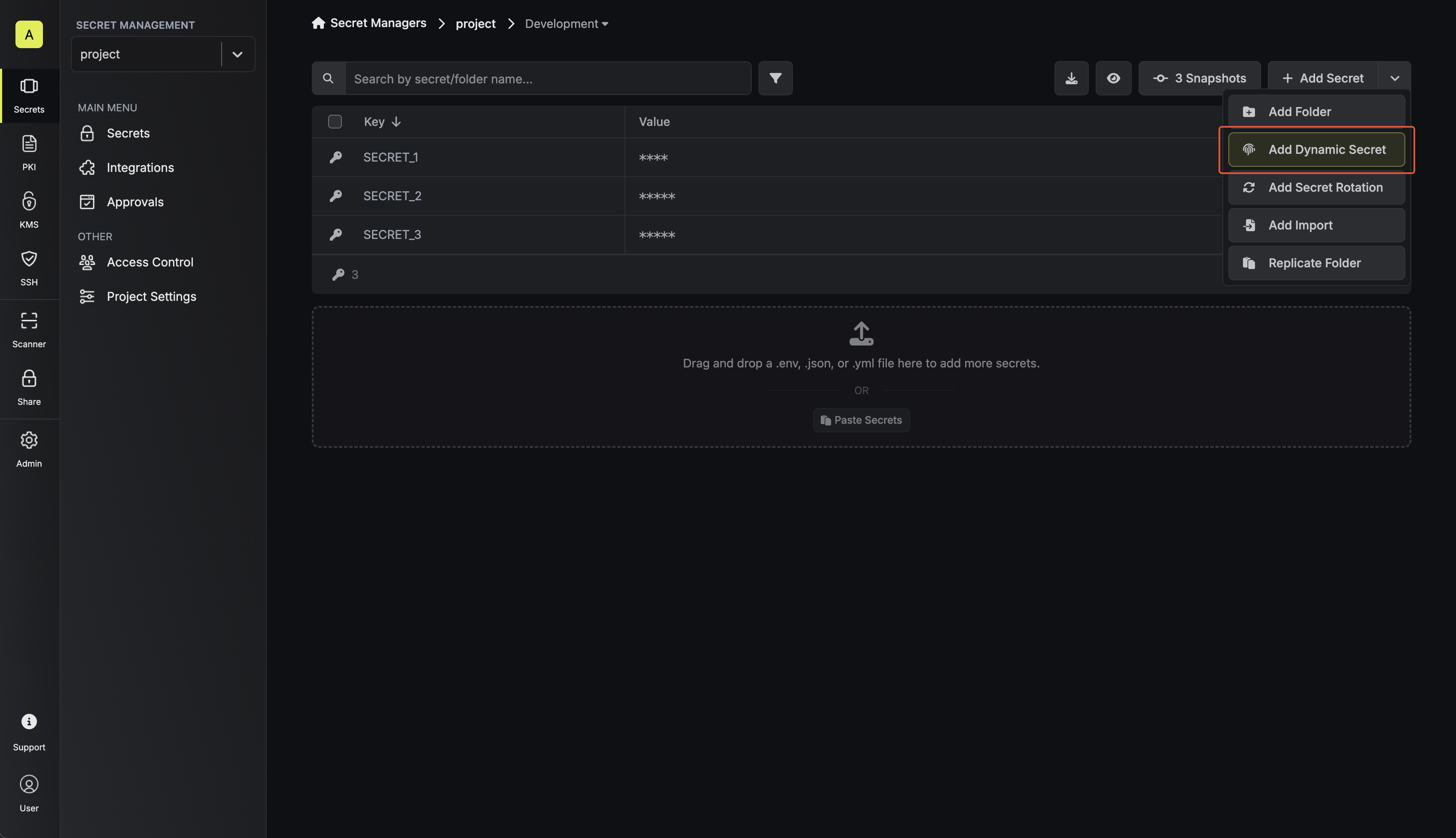
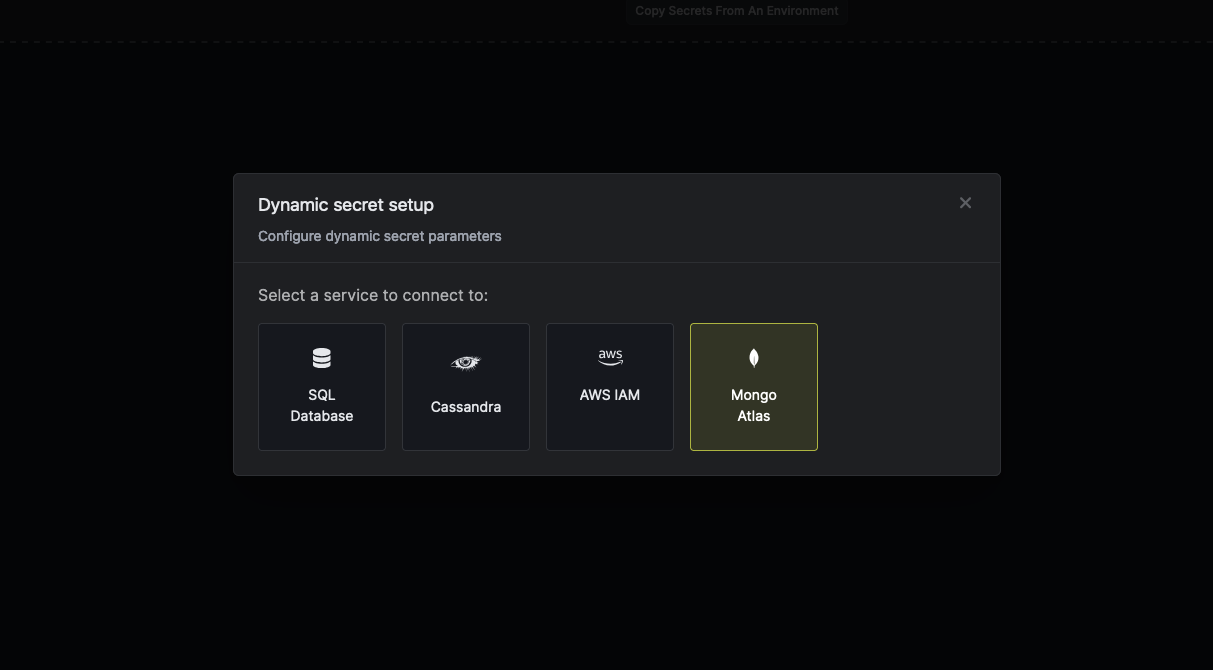
Name by which you want the secret to be referenced
Default time-to-live for a generated secret (it is possible to modify this value when a secret is generate)
Maximum time-to-live for a generated secret
The public key of your generated Atlas API Key. This acts as a username.
The private key of your generated Atlas API Key. This acts as a password.
Unique 24-hexadecimal digit string that identifies your project. This is same as project id
List that provides the pairings of one role with one applicable database.
* **Database Name**: Database to which the user is granted access privileges.
* **Collection**: Collection on which this role applies.
* **Role Name**: Human-readable label that identifies a group of privileges assigned to a database user. This value can either be a built-in role or a custom role.
* Enum: `atlasAdmin` `backup` `clusterMonitor` `dbAdmin` `dbAdminAnyDatabase` `enableSharding` `read` `readAnyDatabase` `readWrite` `readWriteAnyDatabase` ``.
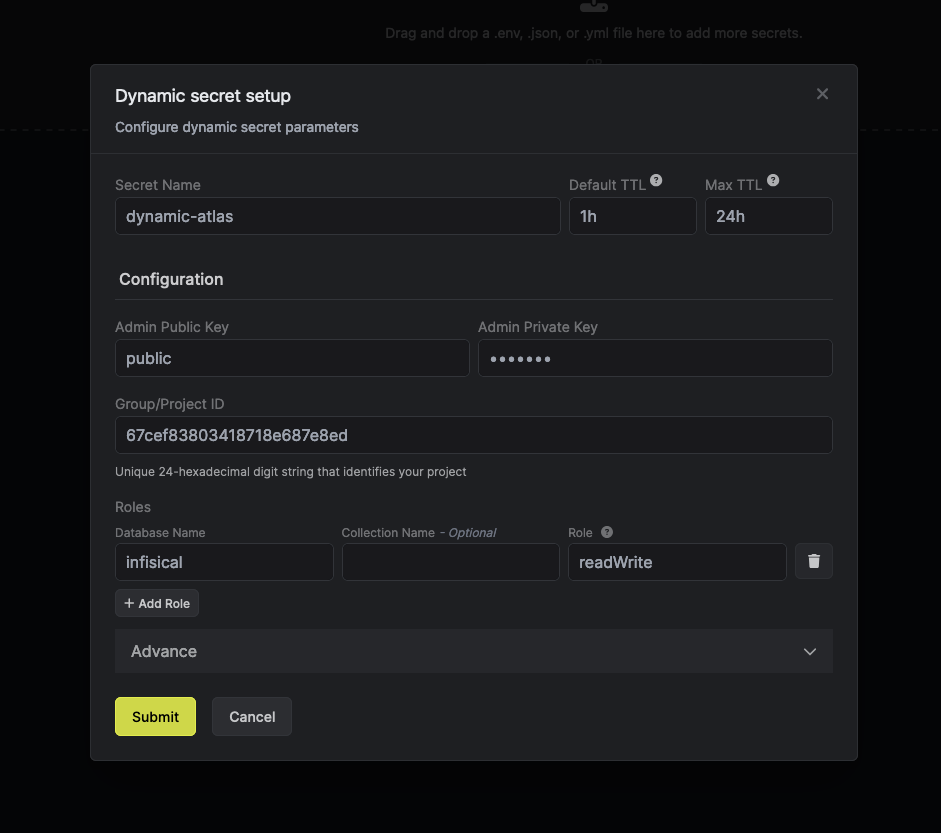
List that contains clusters, MongoDB Atlas Data Lakes, and MongoDB Atlas Streams Instances that this database user can access. If omitted, MongoDB Cloud grants the database user access to all the clusters, MongoDB Atlas Data Lakes, and MongoDB Atlas Streams Instances in the project.
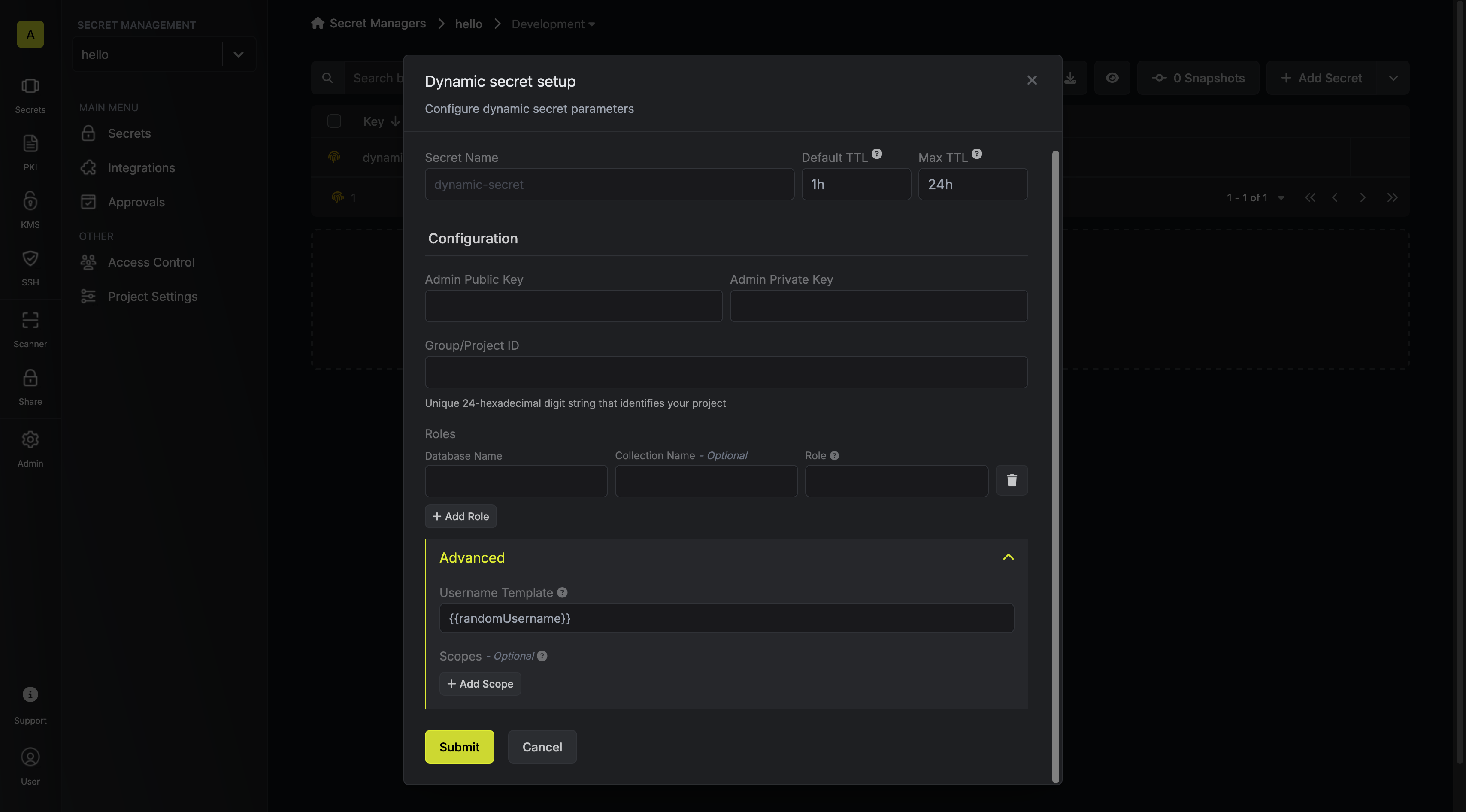
* **Label**: Human-readable label that identifies the cluster or MongoDB Atlas Data Lake that this database user can access.
* **Type**: Category of resource that this database user can access.
After submitting the form, you will see a dynamic secret created in the dashboard.
If this step fails, you may have to add the CA certficate.

Once you've successfully configured the dynamic secret, you're ready to generate on-demand credentials.
To do this, simply click on the 'Generate' button which appears when hovering over the dynamic secret item.
Alternatively, you can initiate the creation of a new lease by selecting 'New Lease' from the dynamic secret lease list section.
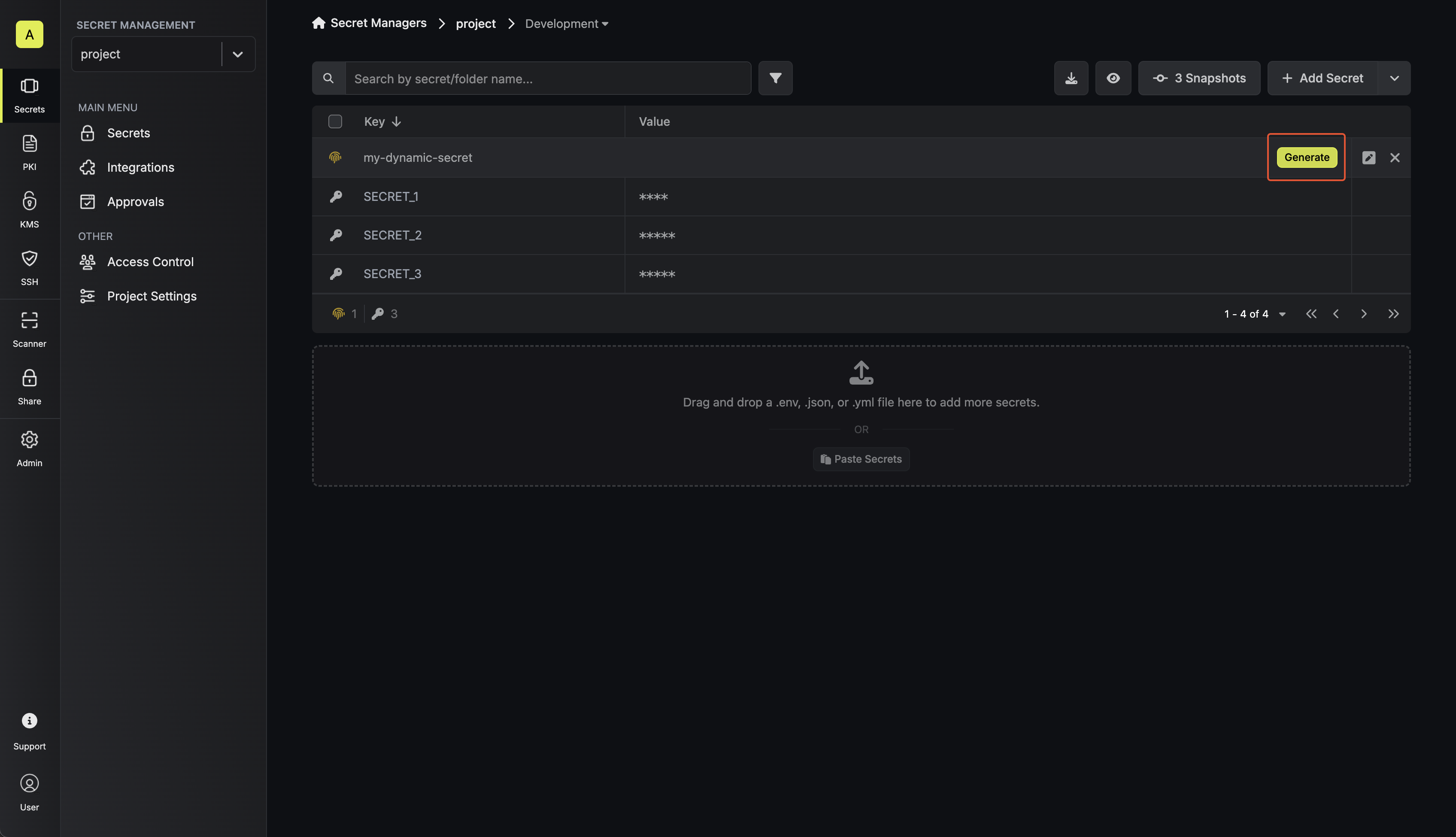
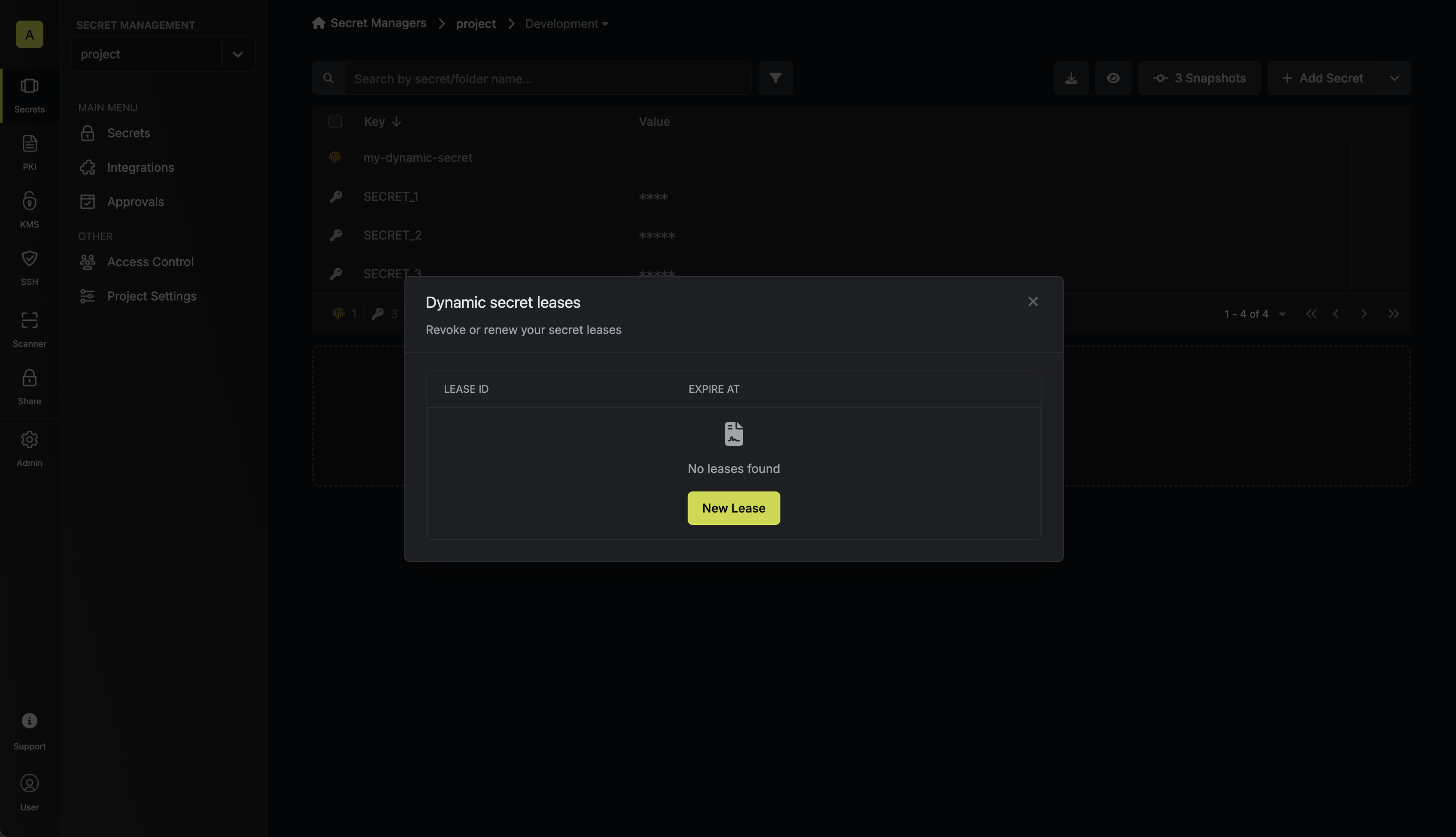
When generating these secrets, it's important to specify a Time-to-Live (TTL) duration. This will dictate how long the credentials are valid for.
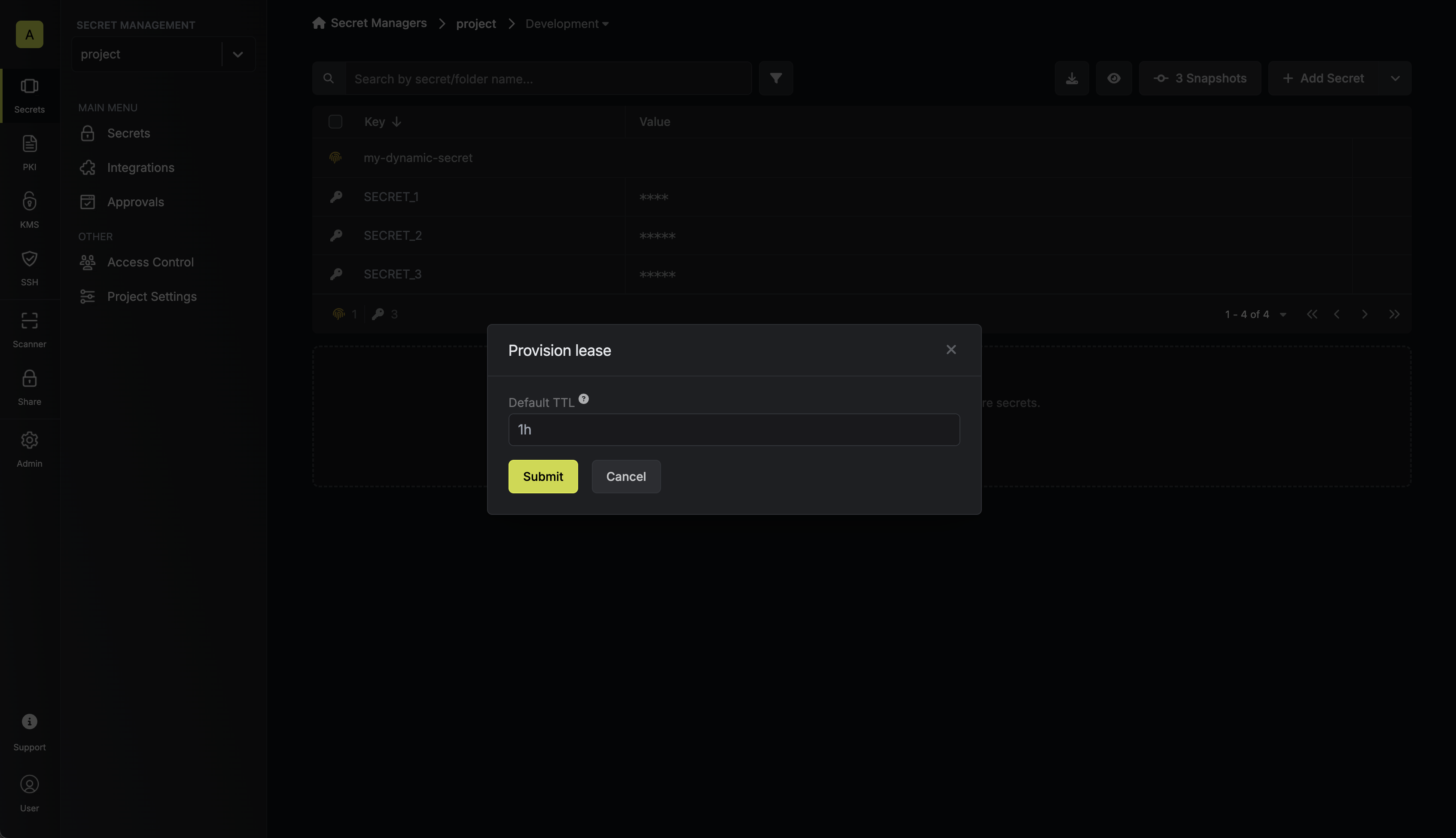
Ensure that the TTL for the lease fall within the maximum TTL defined when configuring the dynamic secret.
Once you click the `Submit` button, a new secret lease will be generated and the credentials for it will be shown to you.
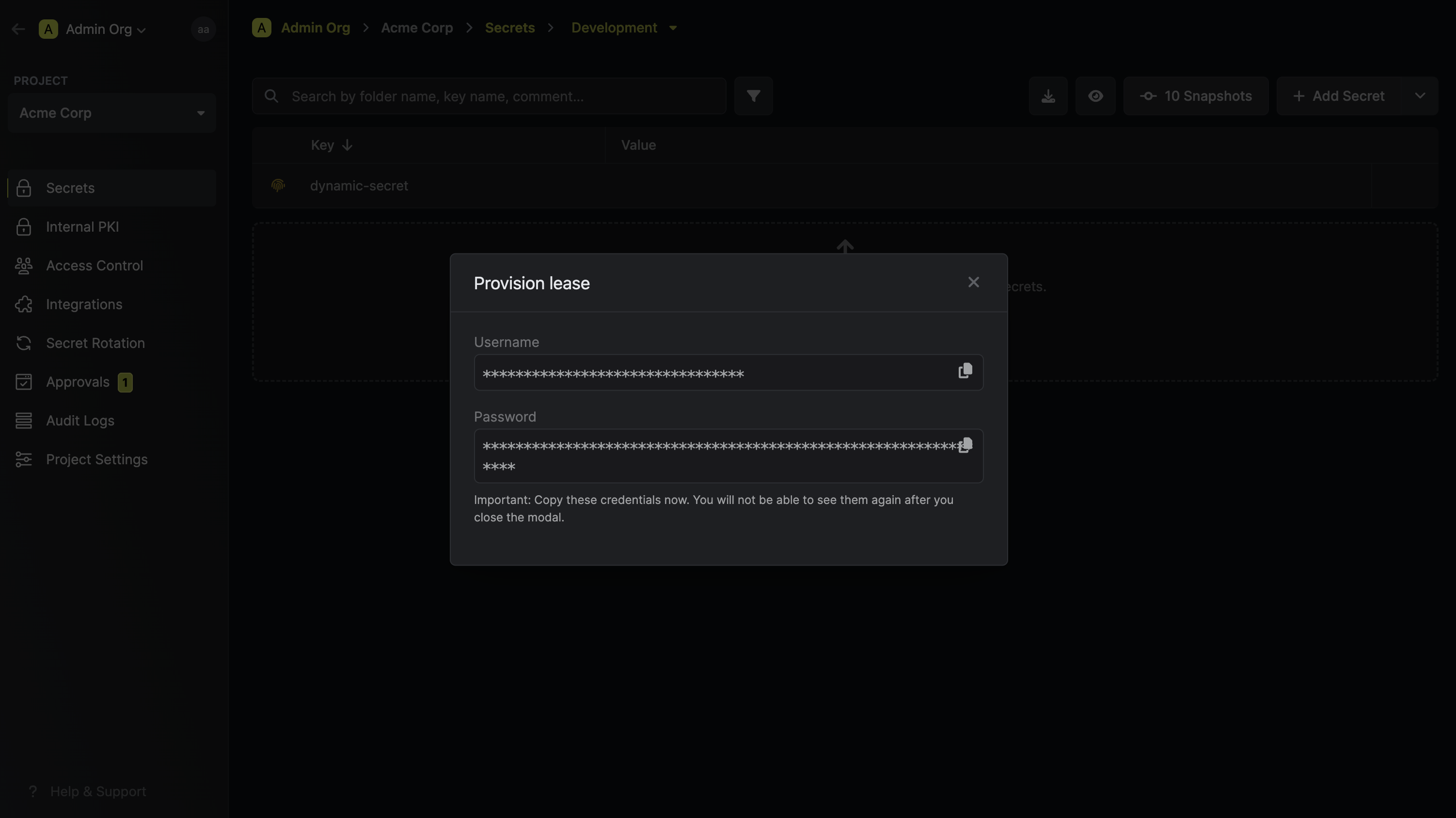
## Audit or Revoke Leases
Once you have created one or more leases, you will be able to access them by clicking on the respective dynamic secret item on the dashboard.
This will allow you see the expiration time of the lease or delete a lease before it's set time to live.
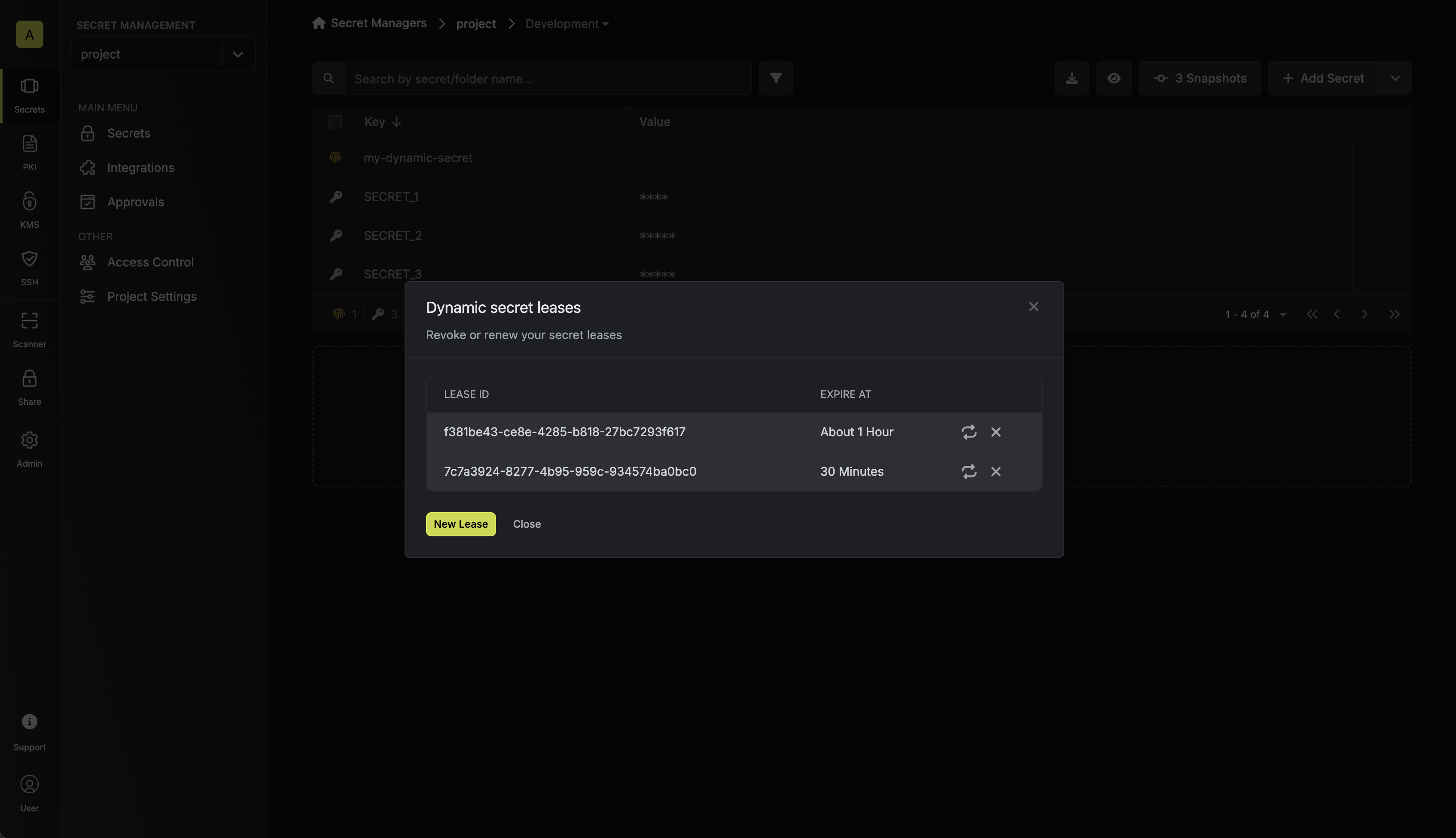
## Renew Leases
To extend the life of the generated dynamic secret leases past its initial time to live, simply click on the **Renew** as illustrated below.
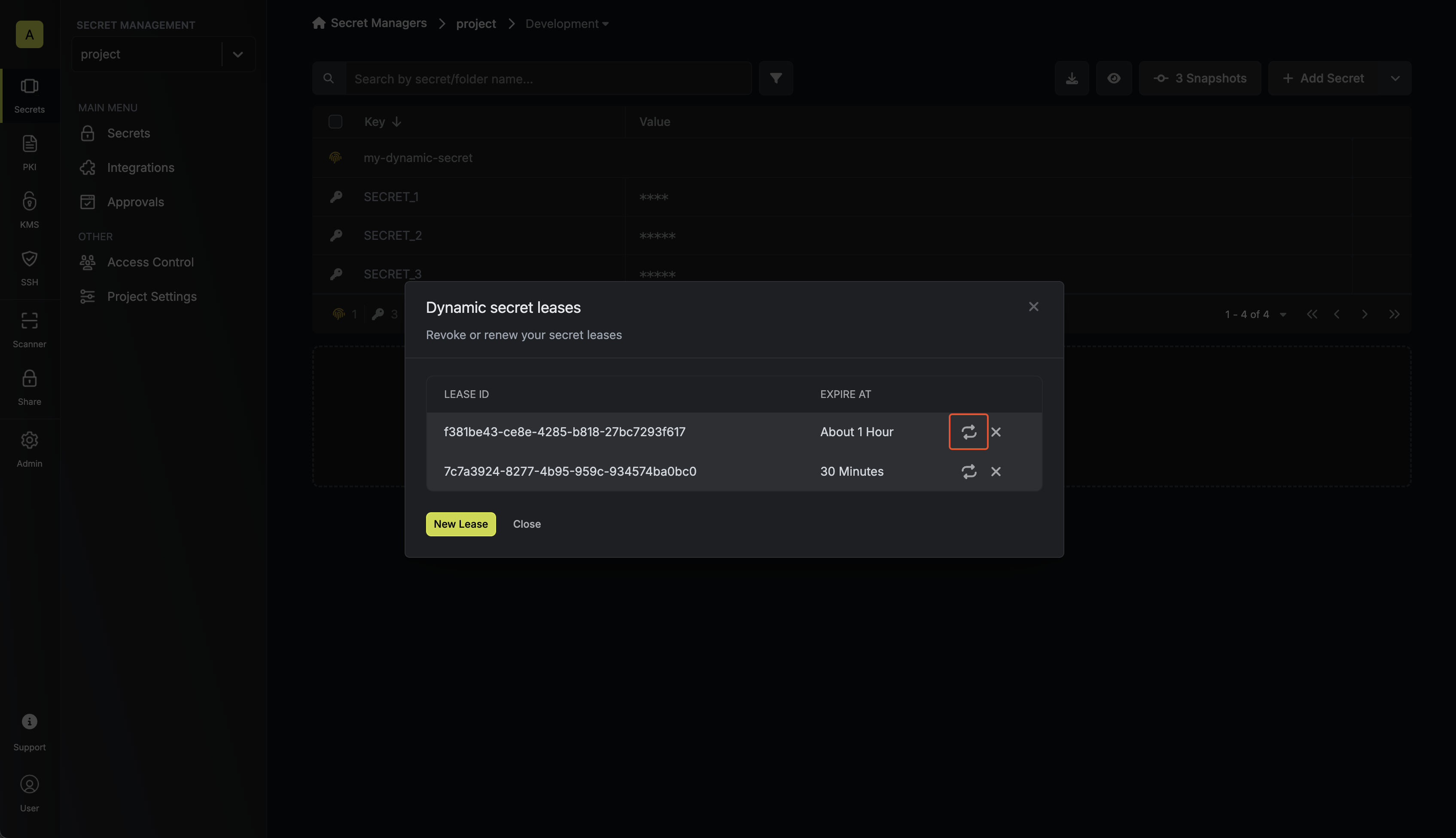
Lease renewals cannot exceed the maximum TTL set when configuring the dynamic secret
# Mongo DB
Learn how to dynamically generate Mongo DB Database user credentials.
The Infisical Mongo DB dynamic secret allows you to generate Mongo DB Database credentials on demand based on configured role.
If your using Mongo Atlas, please use [Atlas Dynamic Secret](./mongo-atlas) as MongoDB commands are not supported by atlas.
## Prerequisite
Create a user with the required permission in your MongoDB instance. This user will be used to create new accounts on-demand.
## Set up Dynamic Secrets with Mongo DB
Open the Secret Overview dashboard and select the environment in which you would like to add a dynamic secret.
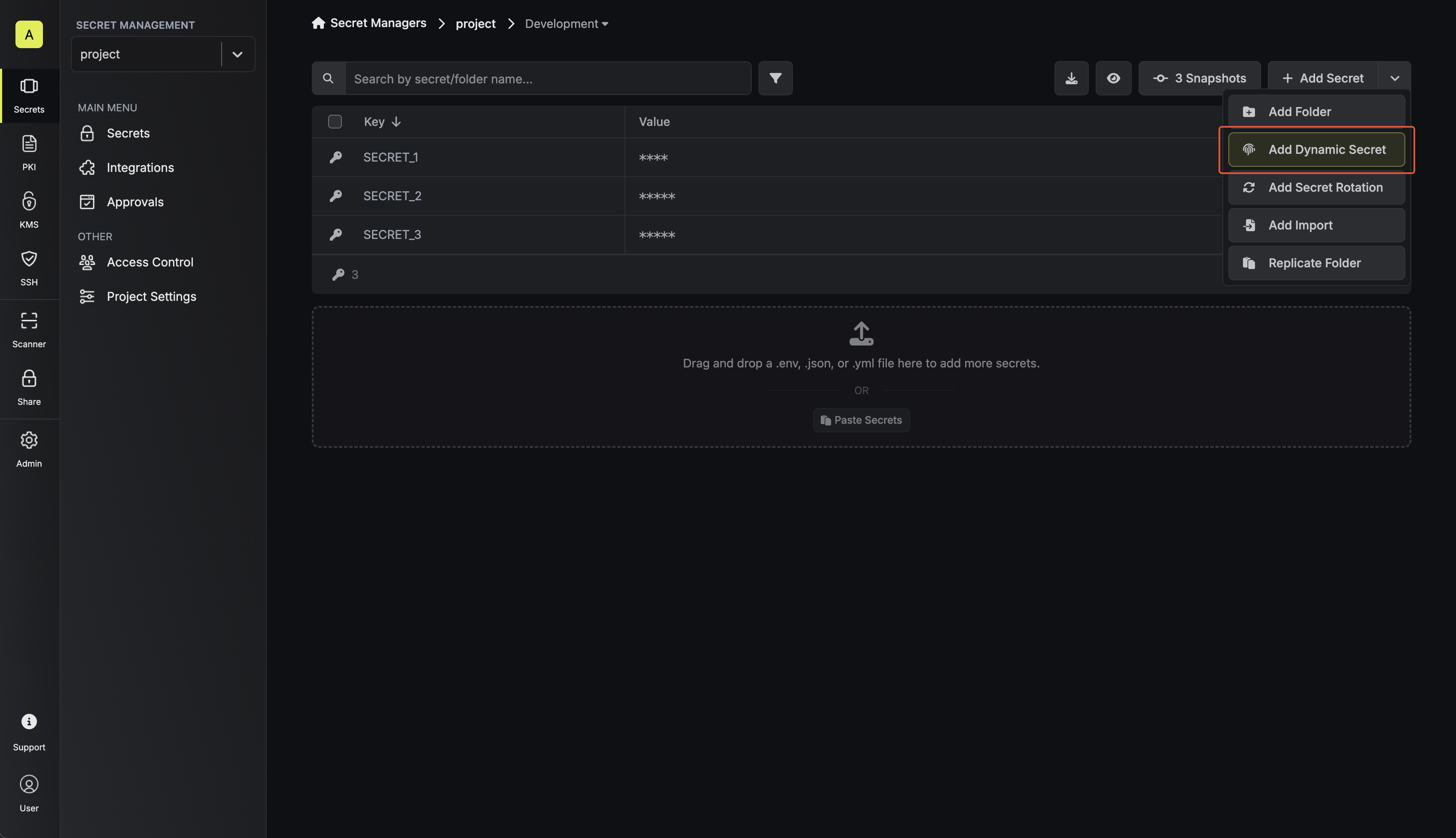
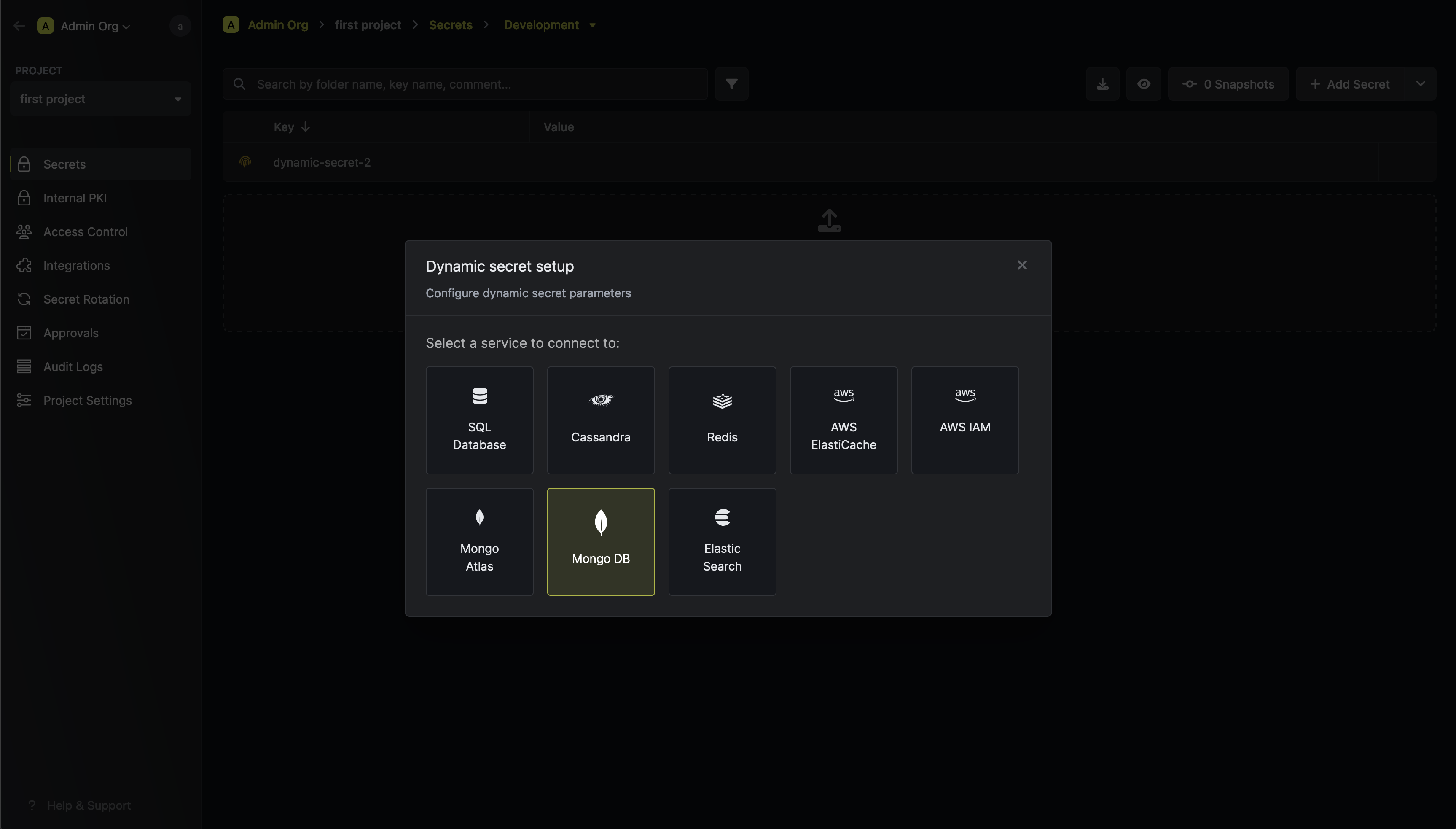
Name by which you want the secret to be referenced
Default time-to-live for a generated secret (it is possible to modify this value when a secret is generate)
Maximum time-to-live for a generated secret
Database host URL.
Database port number. If your Mongo DB is cluster you can omit this.
Username of the admin user that will be used to create dynamic secrets
Password of the admin user that will be used to create dynamic secrets
Name of the database for which you want to create dynamic secrets
Human-readable label that identifies a group of privileges assigned to a database user. This value can either be a built-in role or a custom role.
* Enum: `atlasAdmin` `backup` `clusterMonitor` `dbAdmin` `dbAdminAnyDatabase` `enableSharding` `read` `readAnyDatabase` `readWrite` `readWriteAnyDatabase` ``.
A CA may be required if your DB requires it for incoming connections.
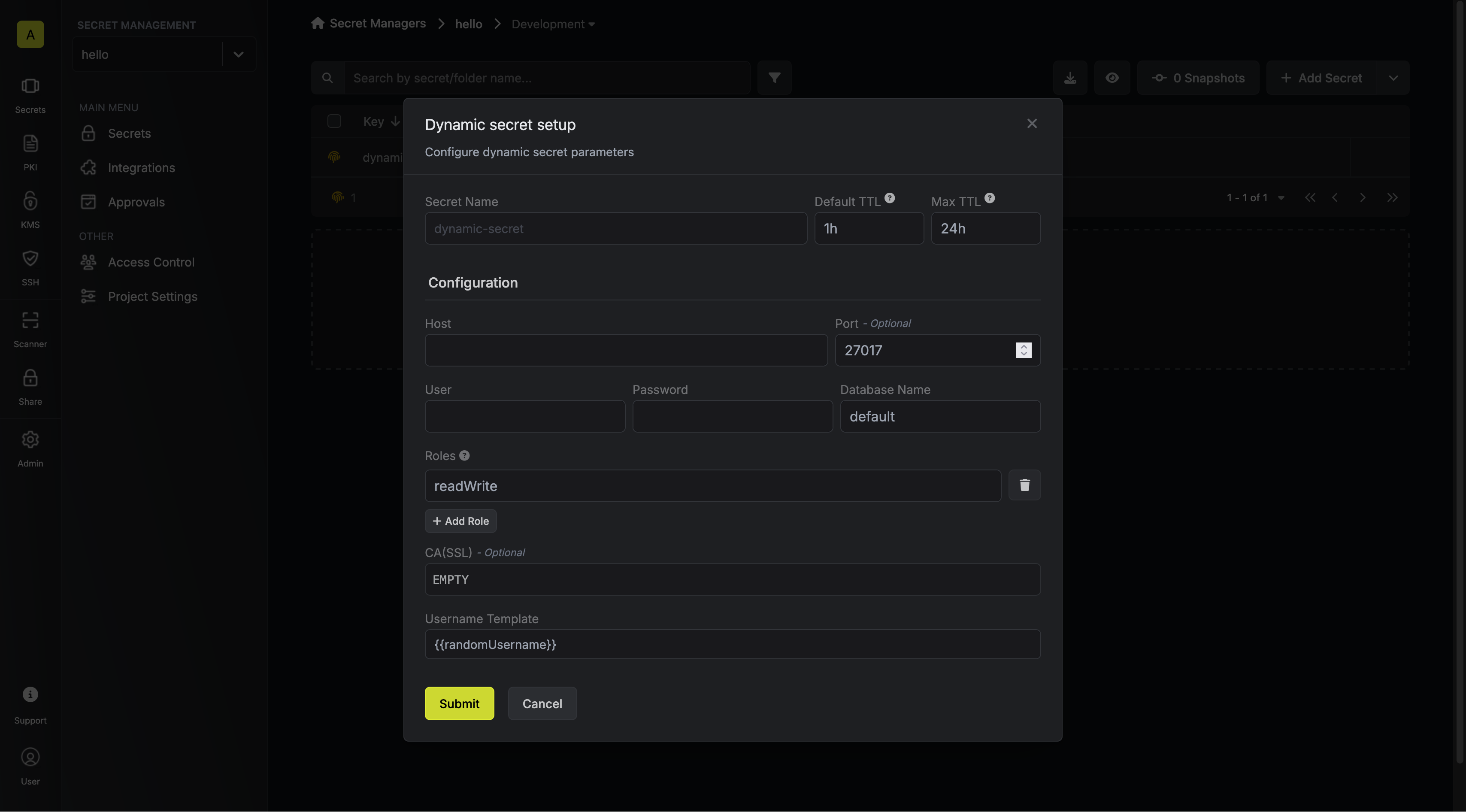
After submitting the form, you will see a dynamic secret created in the dashboard.
If this step fails, you may have to add the CA certificate.
Once you've successfully configured the dynamic secret, you're ready to generate on-demand credentials.
To do this, simply click on the 'Generate' button which appears when hovering over the dynamic secret item.
Alternatively, you can initiate the creation of a new lease by selecting 'New Lease' from the dynamic secret lease list section.
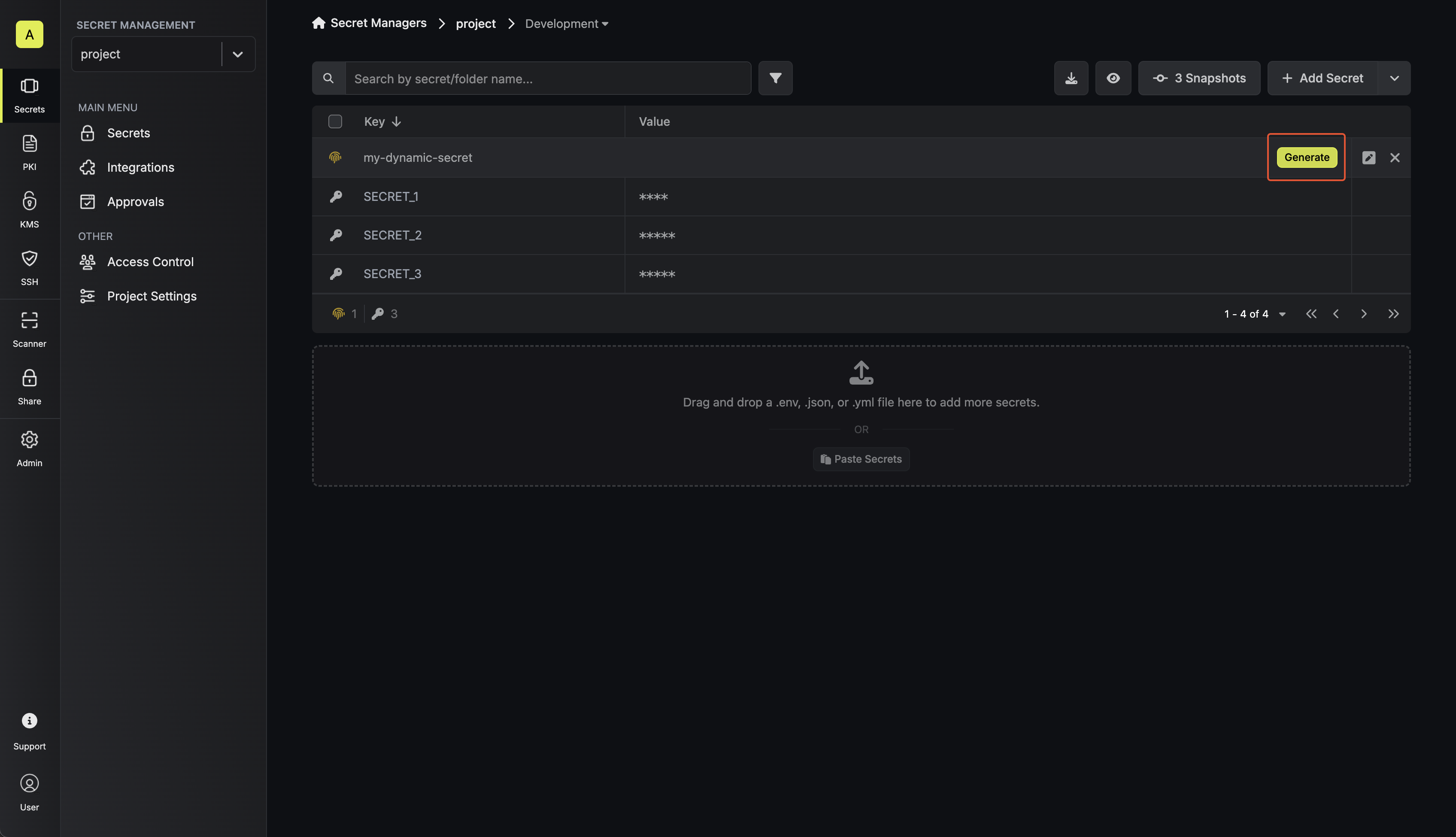
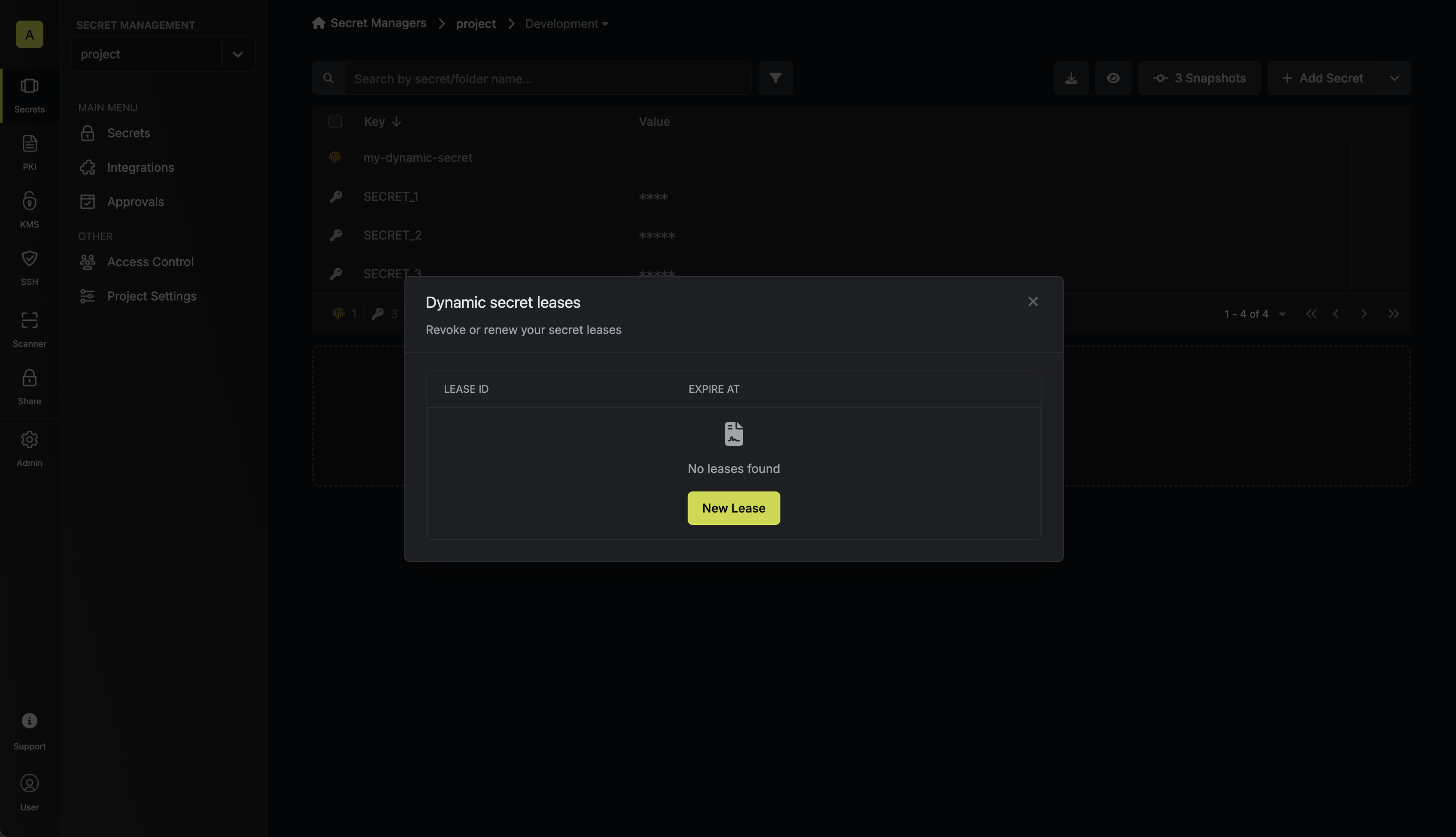
When generating these secrets, it's important to specify a Time-to-Live (TTL) duration. This will dictate how long the credentials are valid for.
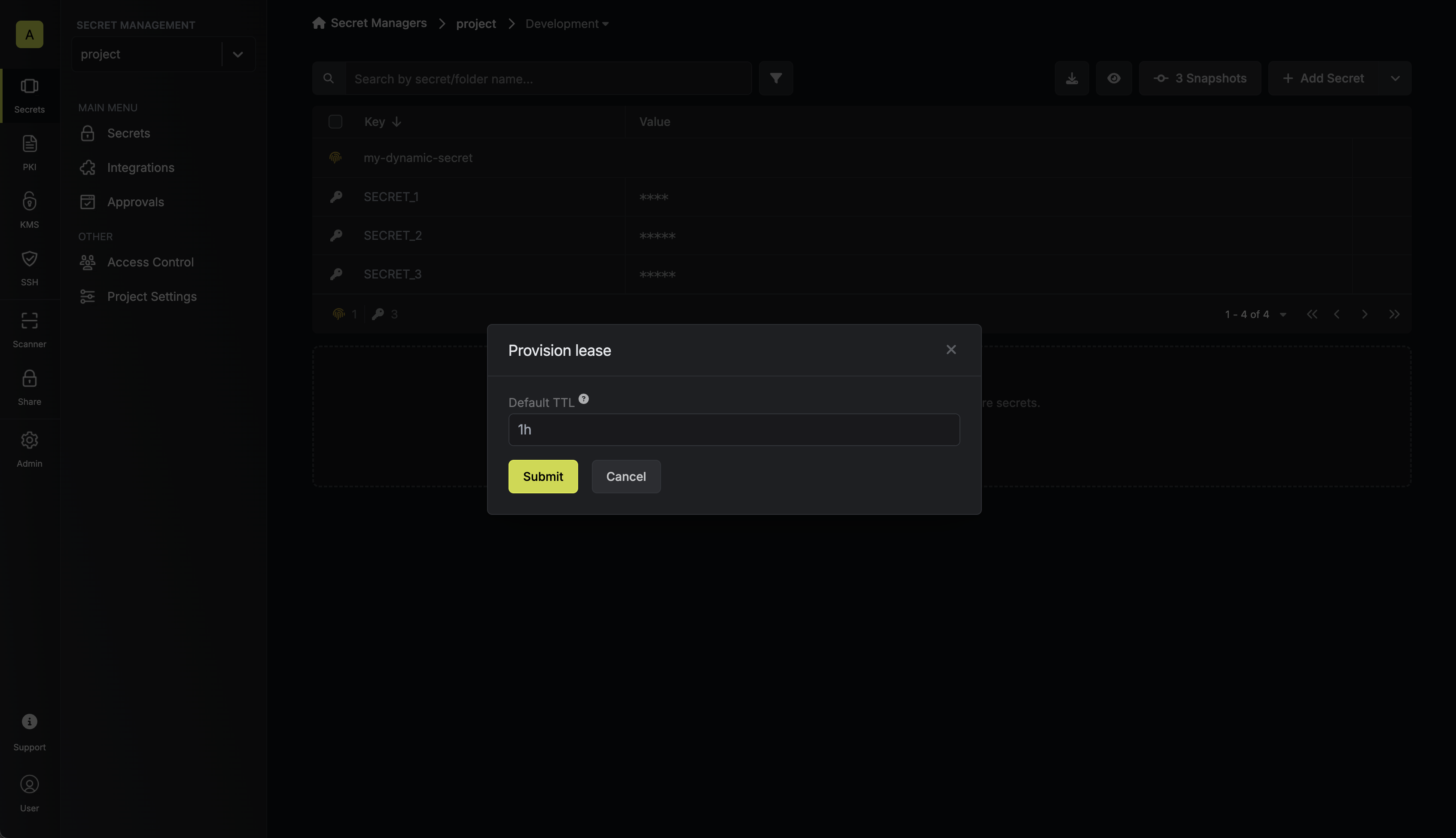
Ensure that the TTL for the lease fall within the maximum TTL defined when configuring the dynamic secret.
Once you click the `Submit` button, a new secret lease will be generated and the credentials from it will be shown to you.
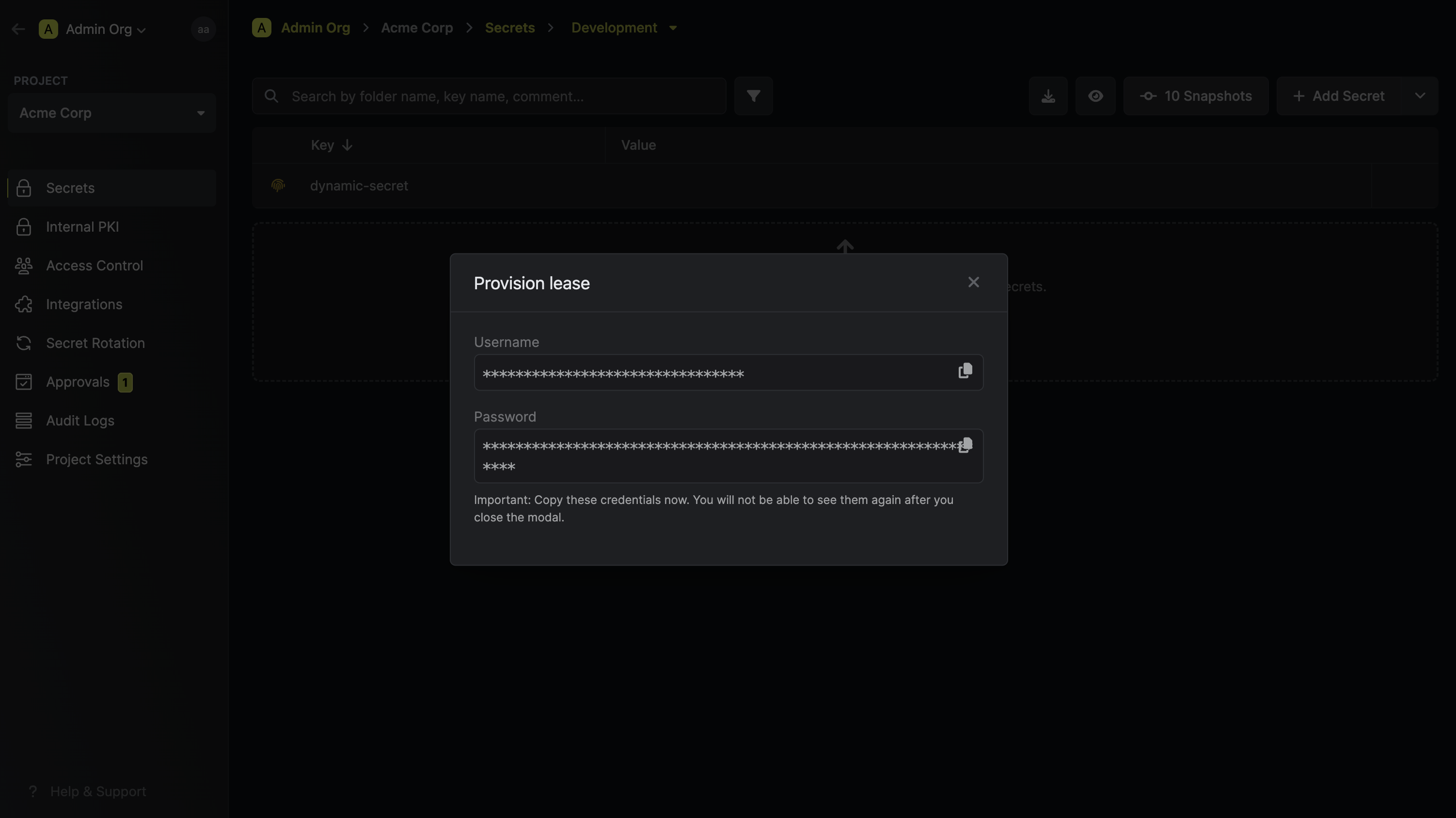
## Audit or Revoke Leases
Once you have created one or more leases, you will be able to access them by clicking on the respective dynamic secret item on the dashboard.
This will allow you see the expiration time of the lease or delete a lease before it's set time to live.
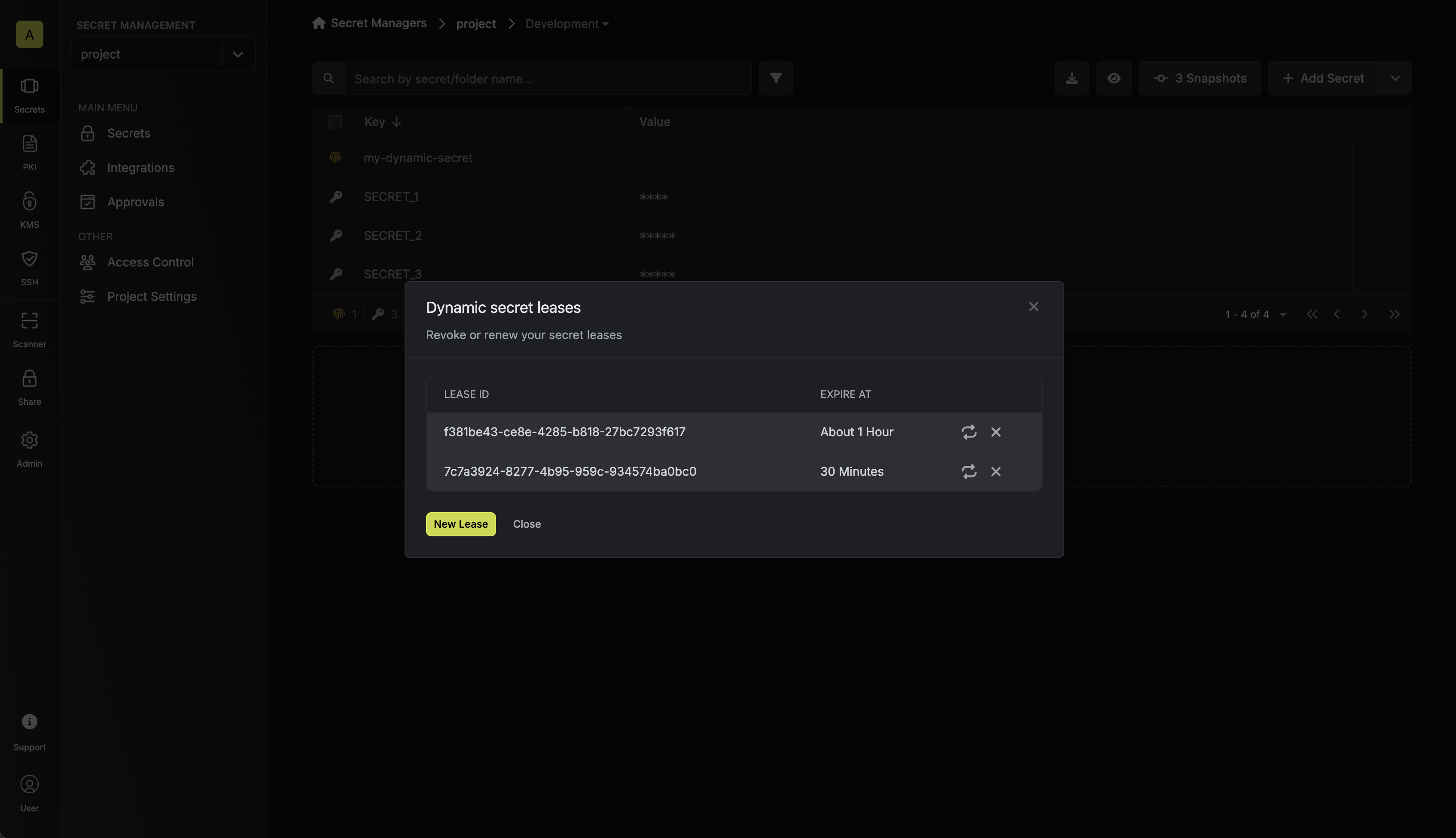
## Renew Leases
To extend the life of the generated dynamic secret leases past its initial time to live, simply click on the **Renew** as illustrated below.
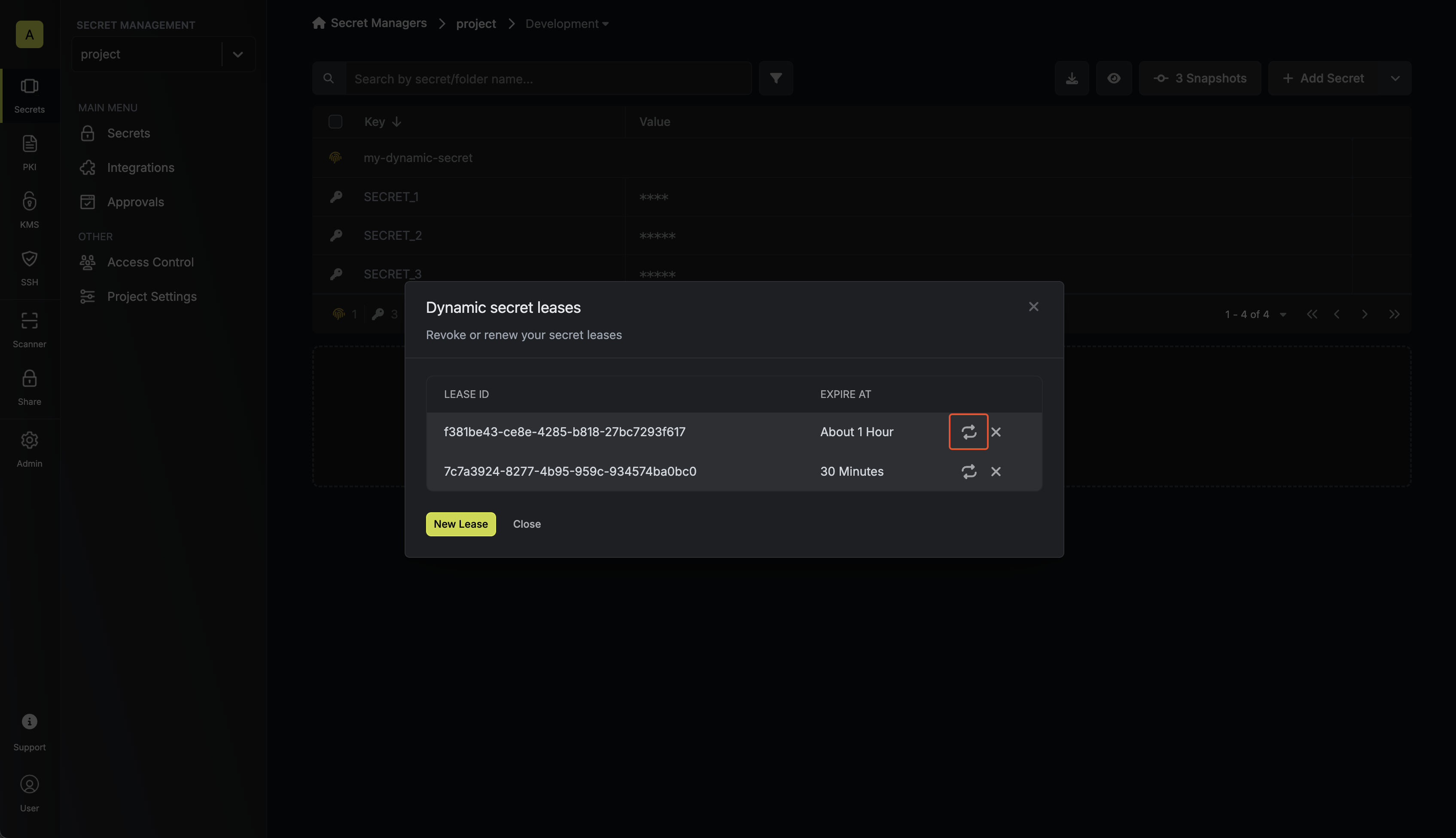
Lease renewals cannot exceed the maximum TTL set when configuring the dynamic secret
# MS SQL
Learn how to dynamically generate MS SQL database user credentials.
The Infisical MS SQL dynamic secret allows you to generate Microsoft SQL server database credentials on demand based on configured role.
## Prerequisite
Create a user with the required permission in your SQL instance. This user will be used to create new accounts on-demand.
## Set up Dynamic Secrets with MS SQL
Open the Secret Overview dashboard and select the environment in which you would like to add a dynamic secret.
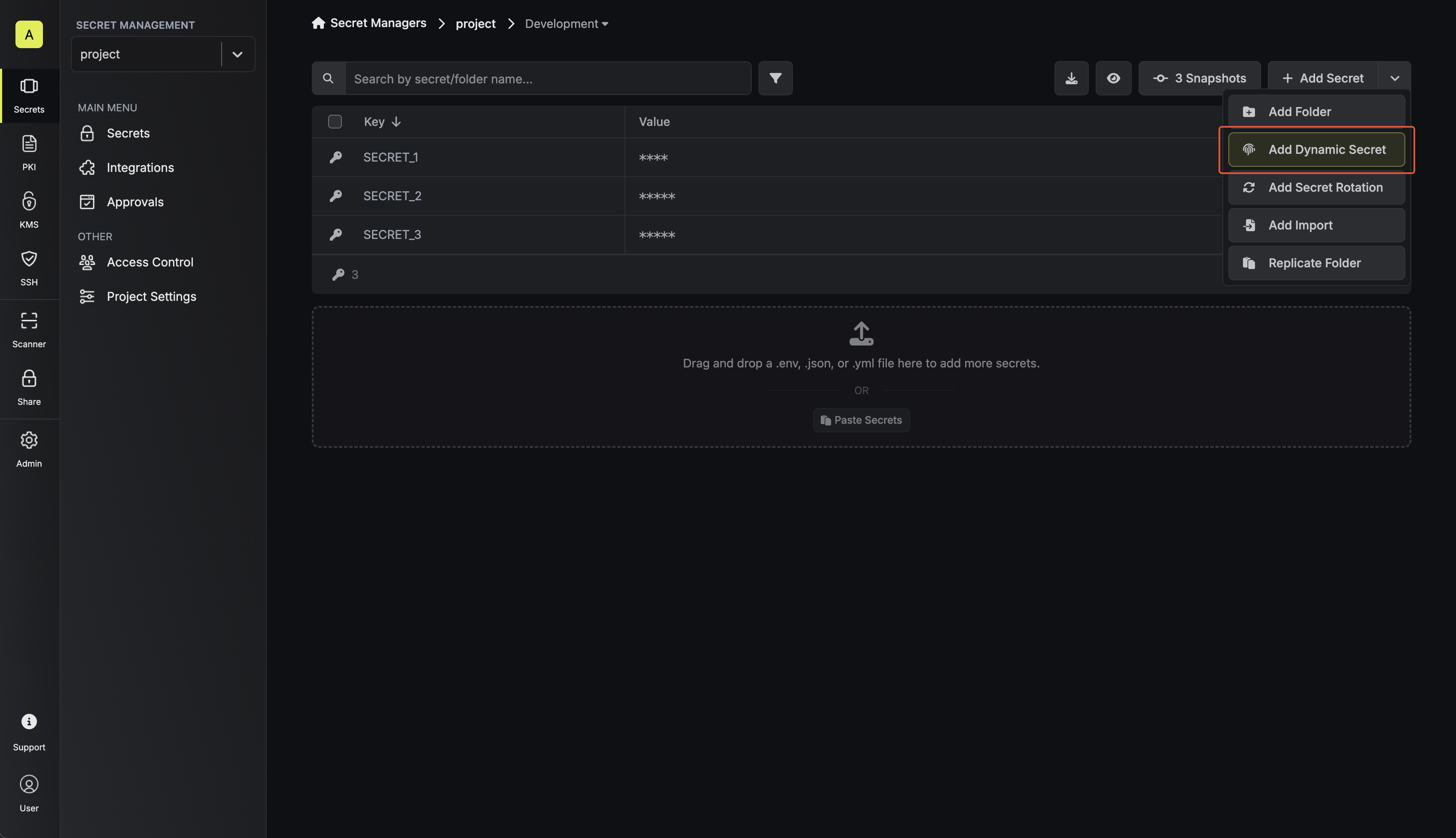
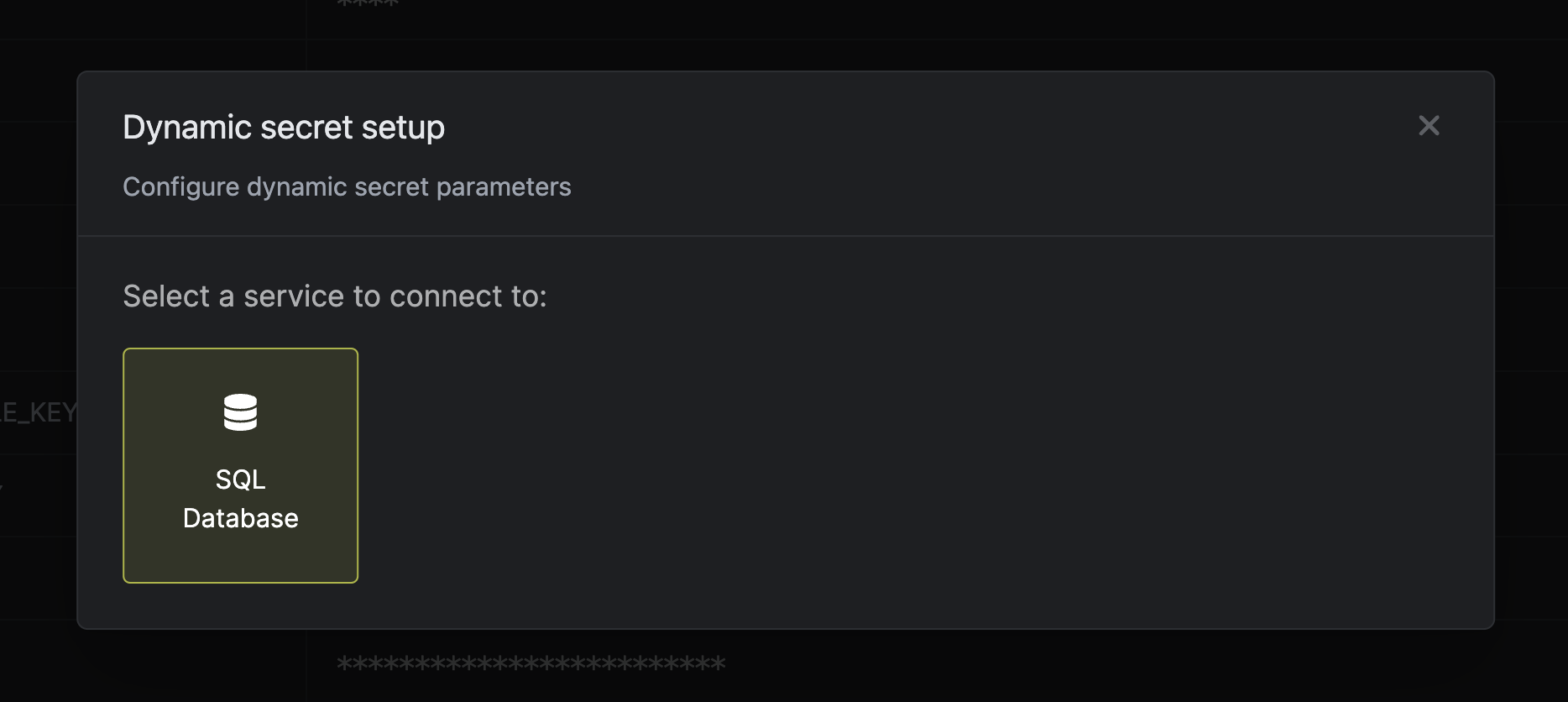
Name by which you want the secret to be referenced
Default time-to-live for a generated secret (it is possible to modify this value when a secret is generate)
Maximum time-to-live for a generated secret
Choose the service you want to generate dynamic secrets for. This must be selected as **MS SQL**.
Database host
Database port
Username that will be used to create dynamic secrets
Password that will be used to create dynamic secrets
Name of the database for which you want to create dynamic secrets
A CA may be required if your DB requires it for incoming connections. AWS RDS instances with default settings will requires a CA which can be downloaded [here](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/UsingWithRDS.SSL.html#UsingWithRDS.SSL.CertificatesAllRegions).
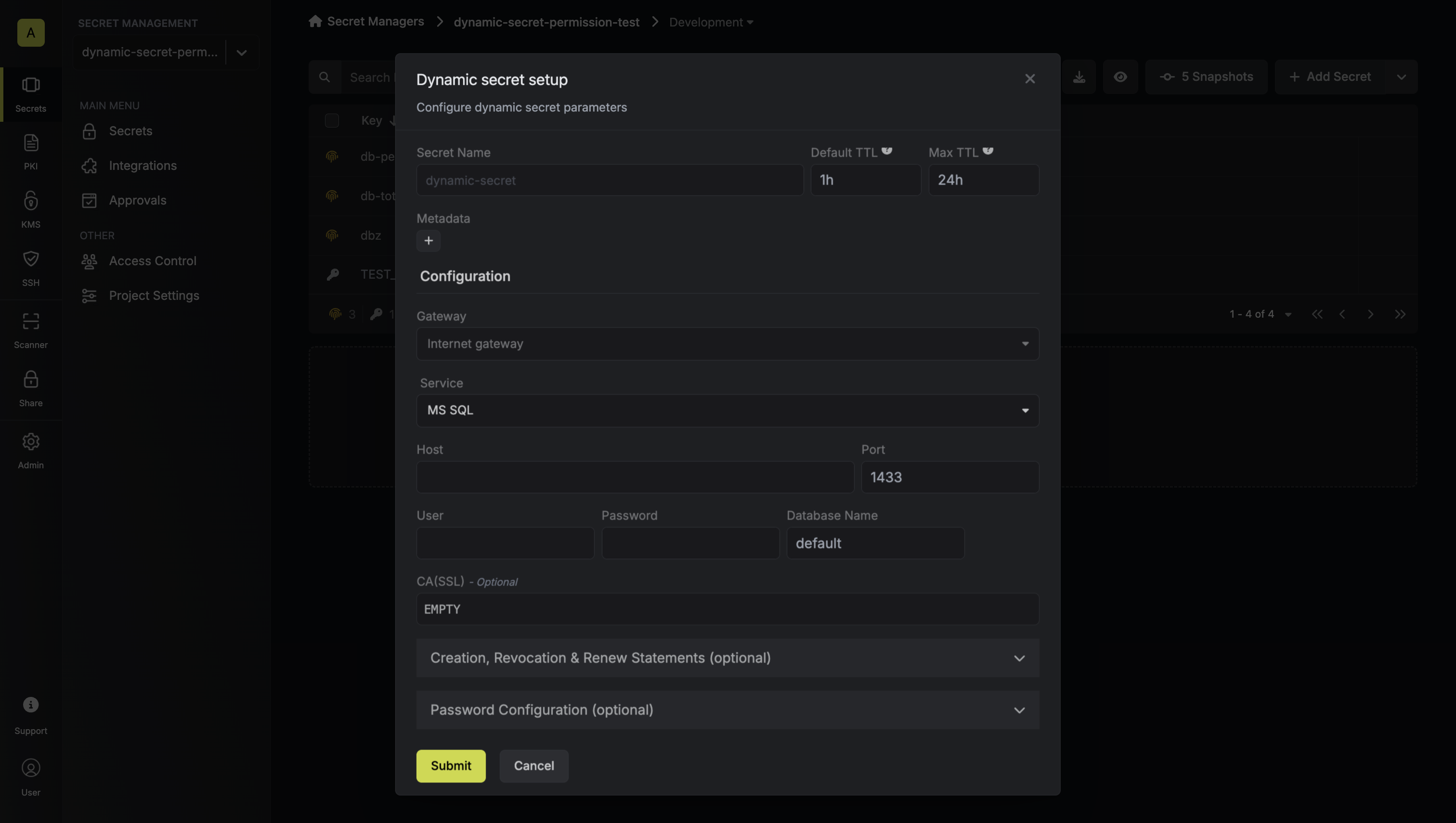
If you want to provide specific privileges for the generated dynamic credentials, you can modify the SQL statement to your needs. This is useful if you want to only give access to a specific table(s).
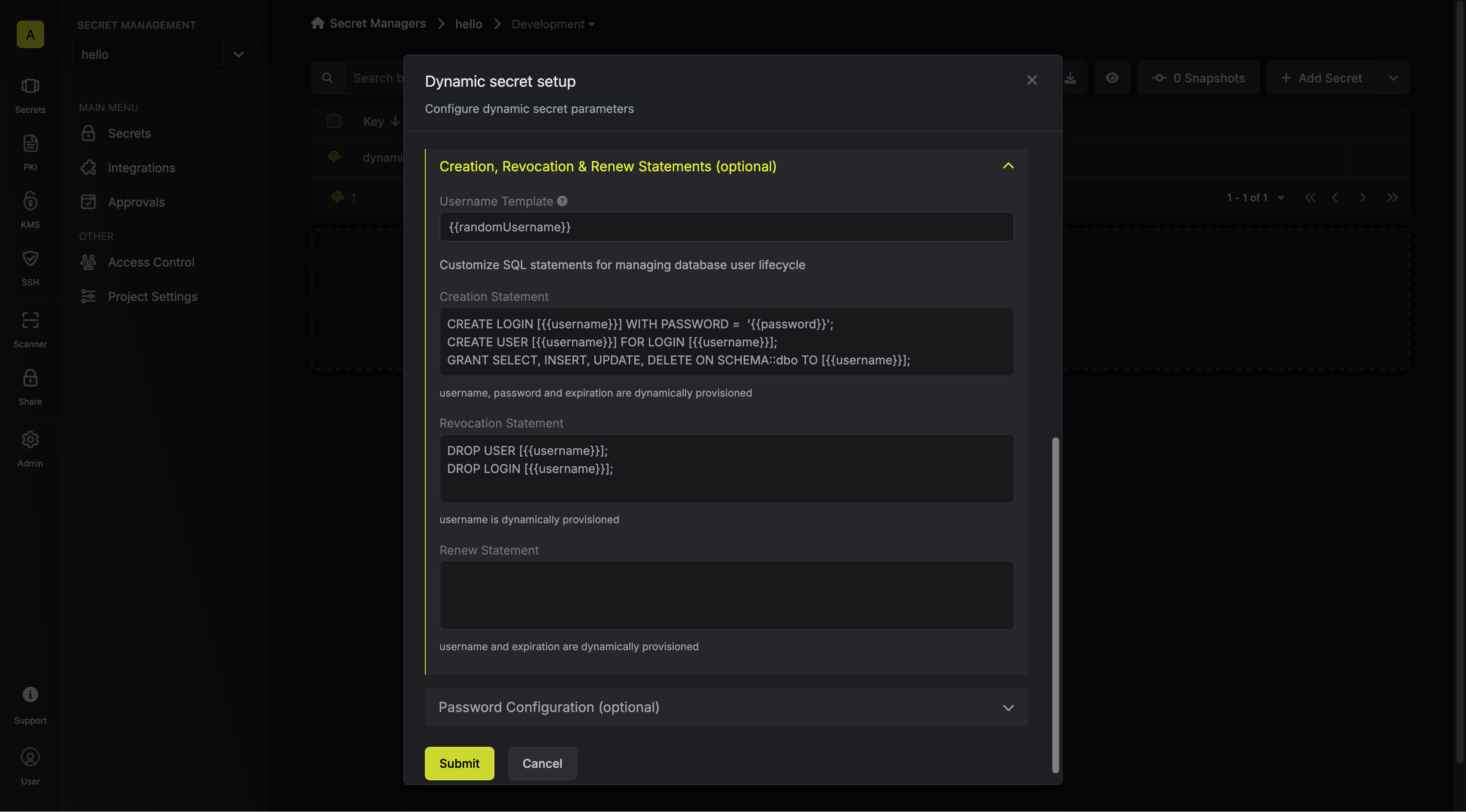
After submitting the form, you will see a dynamic secret created in the dashboard.
If this step fails, you may have to add the CA certficate.

Once you've successfully configured the dynamic secret, you're ready to generate on-demand credentials.
To do this, simply click on the 'Generate' button which appears when hovering over the dynamic secret item.
Alternatively, you can initiate the creation of a new lease by selecting 'New Lease' from the dynamic secret lease list section.
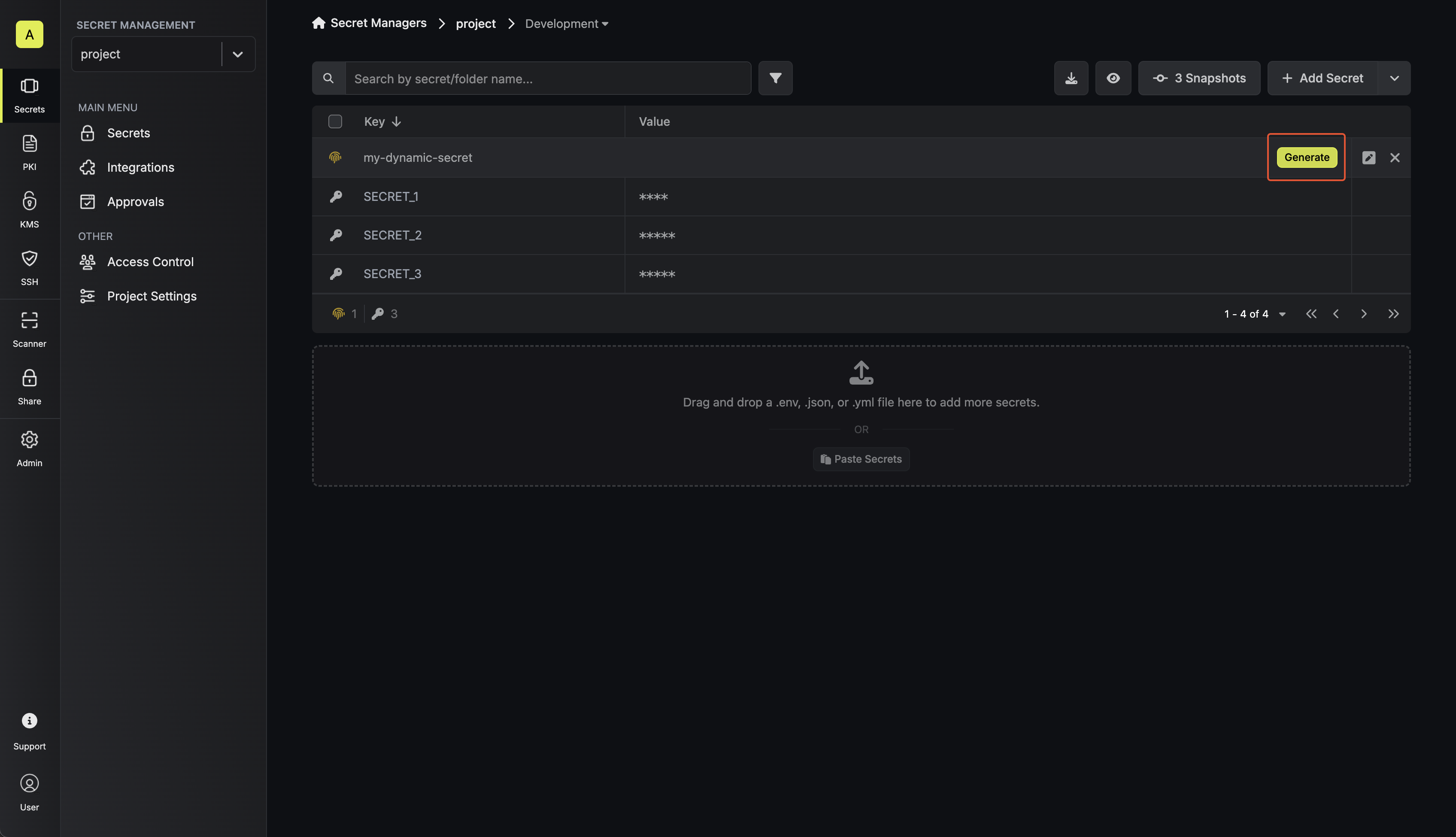
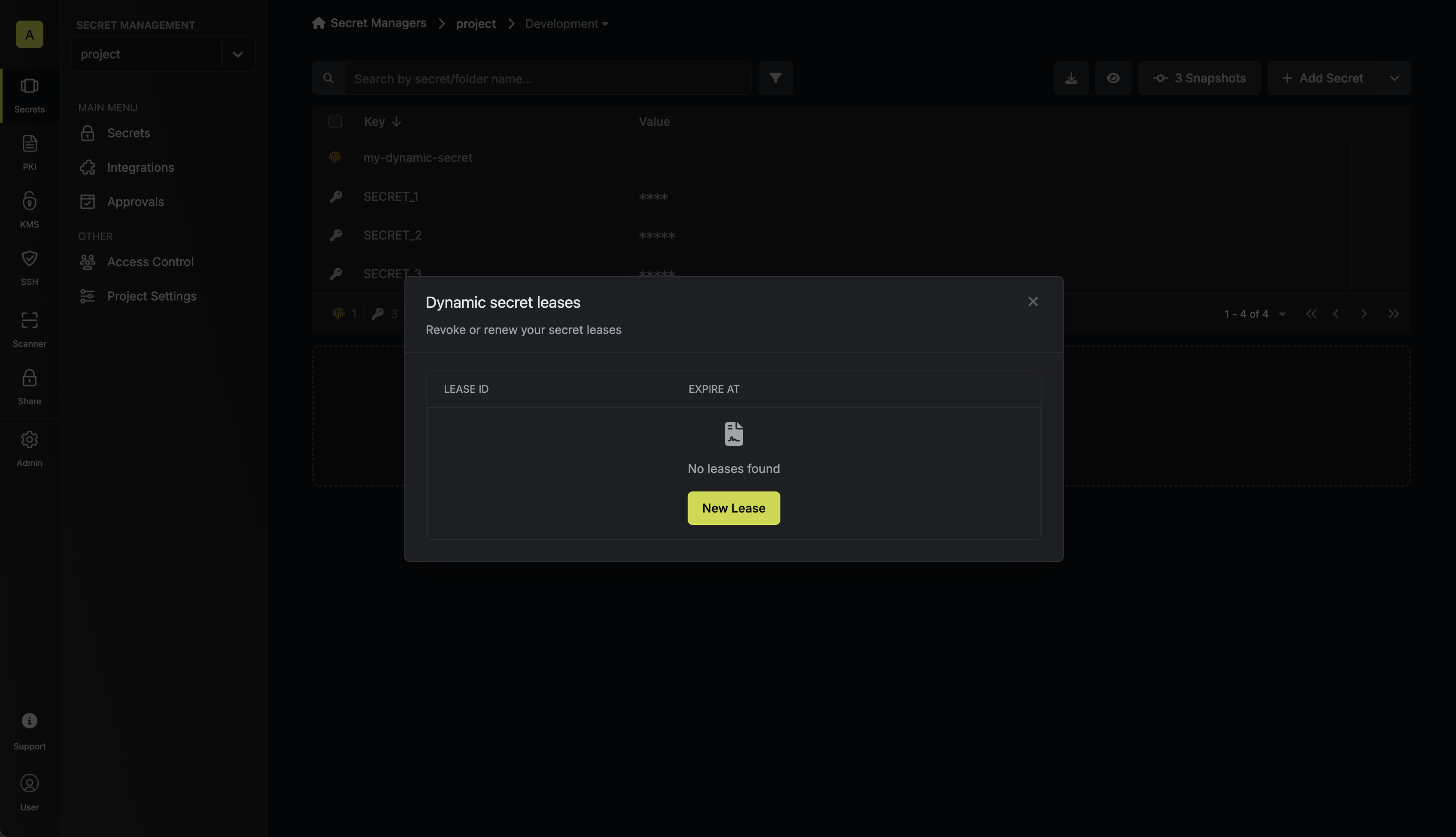
When generating these secrets, it's important to specify a Time-to-Live (TTL) duration. This will dictate how long the credentials are valid for.
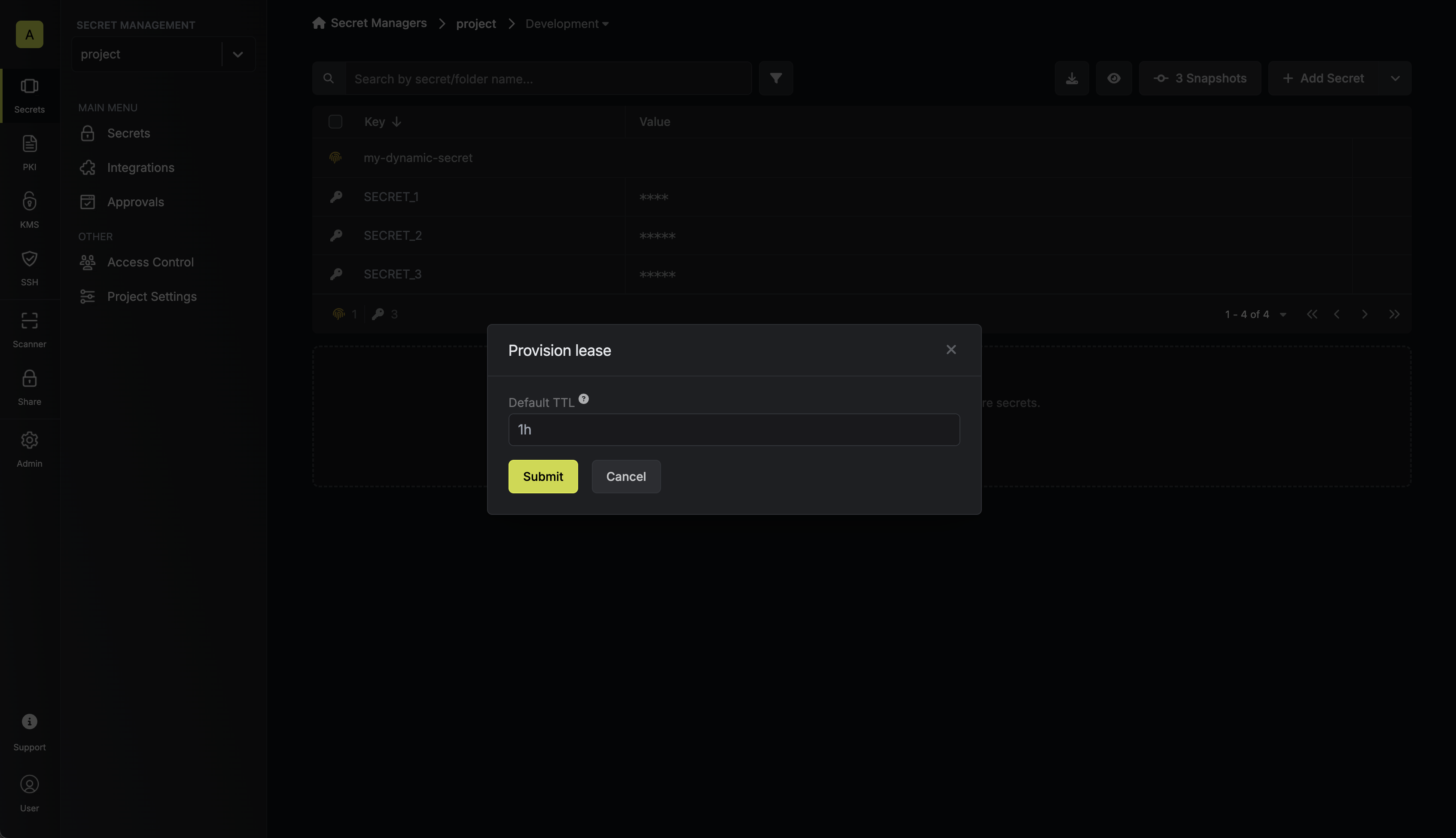
Ensure that the TTL for the lease fall within the maximum TTL defined when configuring the dynamic secret.
Once you click the `Submit` button, a new secret lease will be generated and the credentials for it will be shown to you.
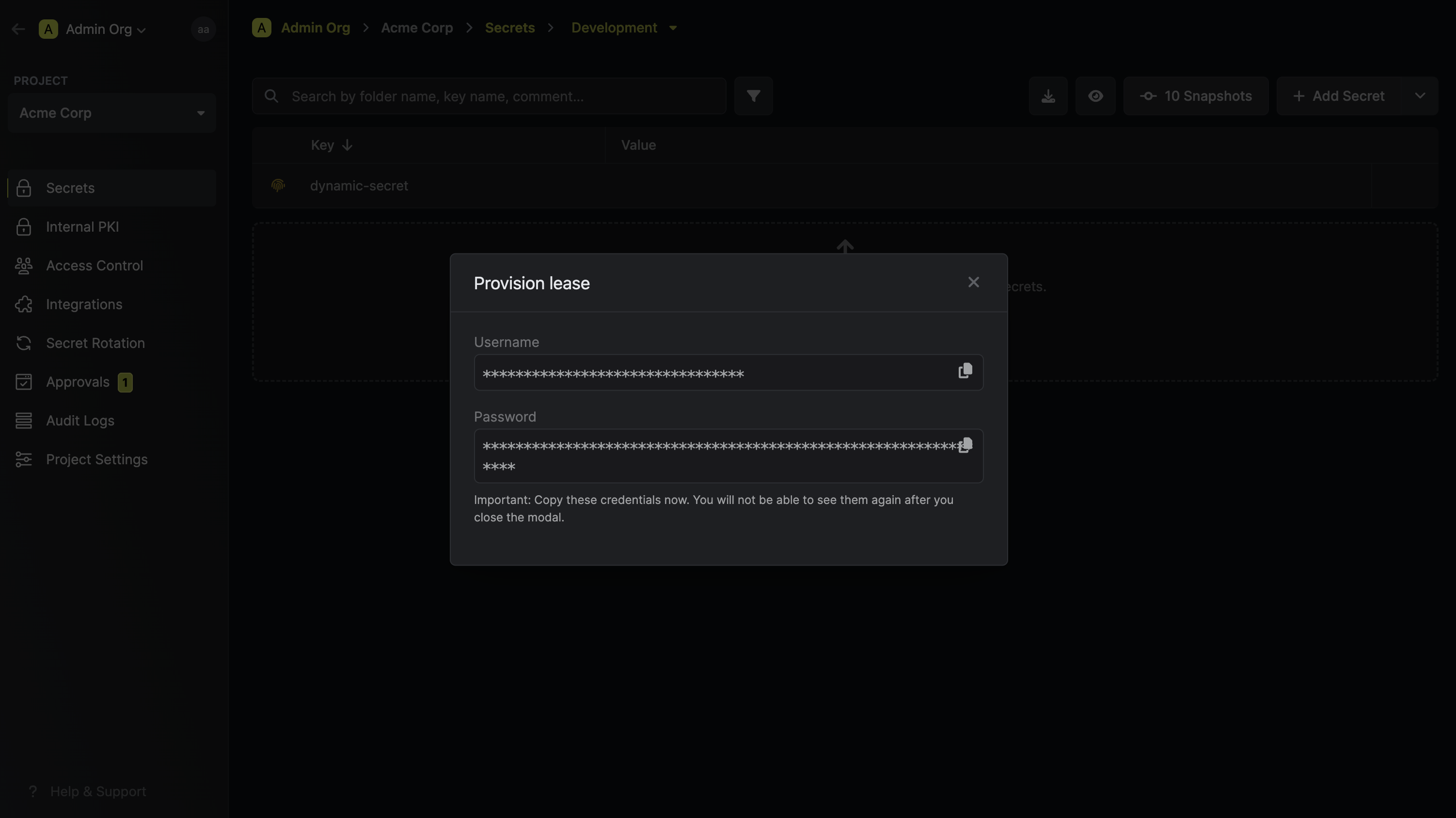
## Audit or Revoke Leases
Once you have created one or more leases, you will be able to access them by clicking on the respective dynamic secret item on the dashboard.
This will allow you see the expiration time of the lease or delete the lease before it's set time to live.
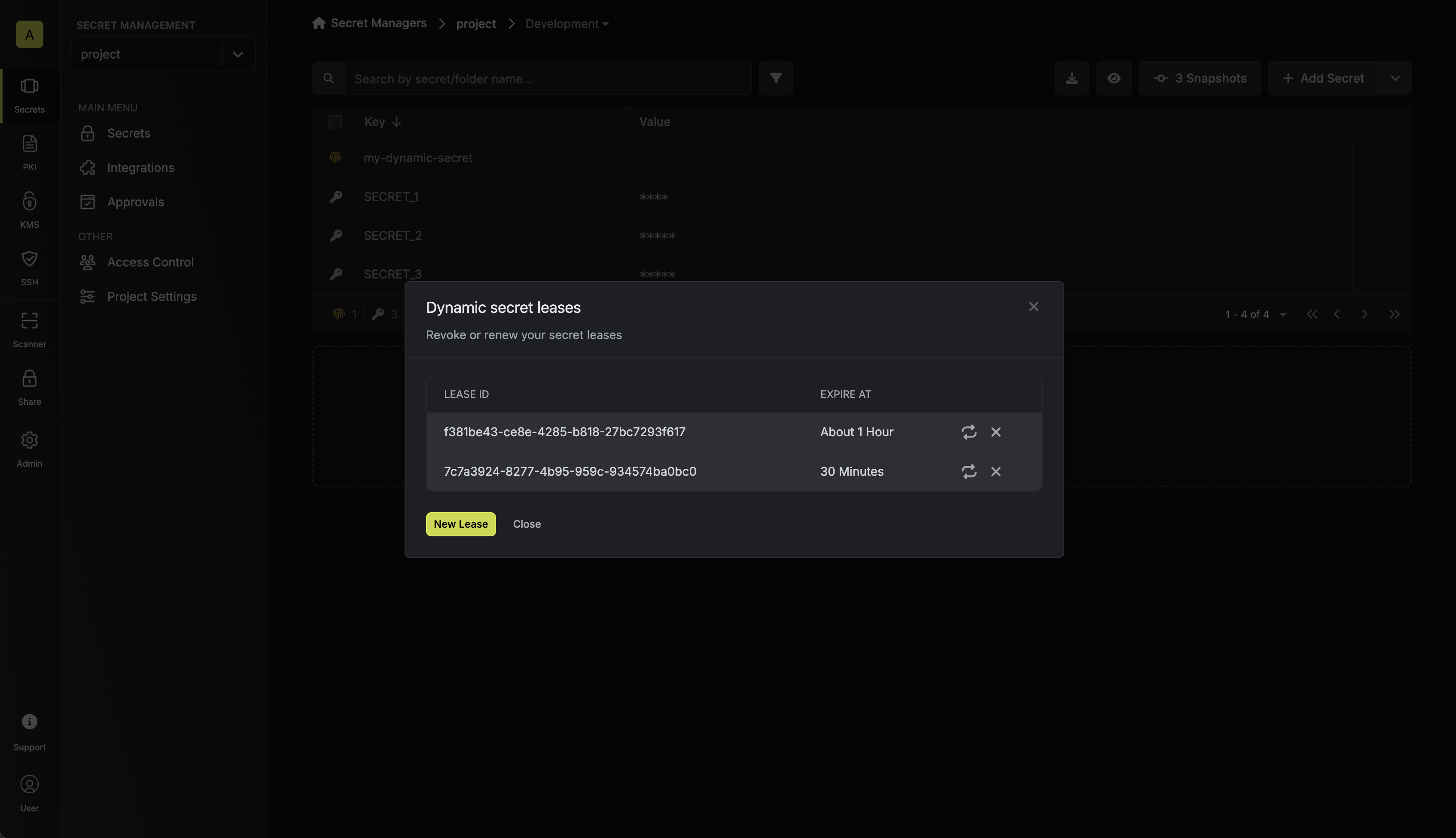
## Renew Leases
To extend the life of the generated dynamic secret leases past its initial time to live, simply click on the **Renew** as illustrated below.
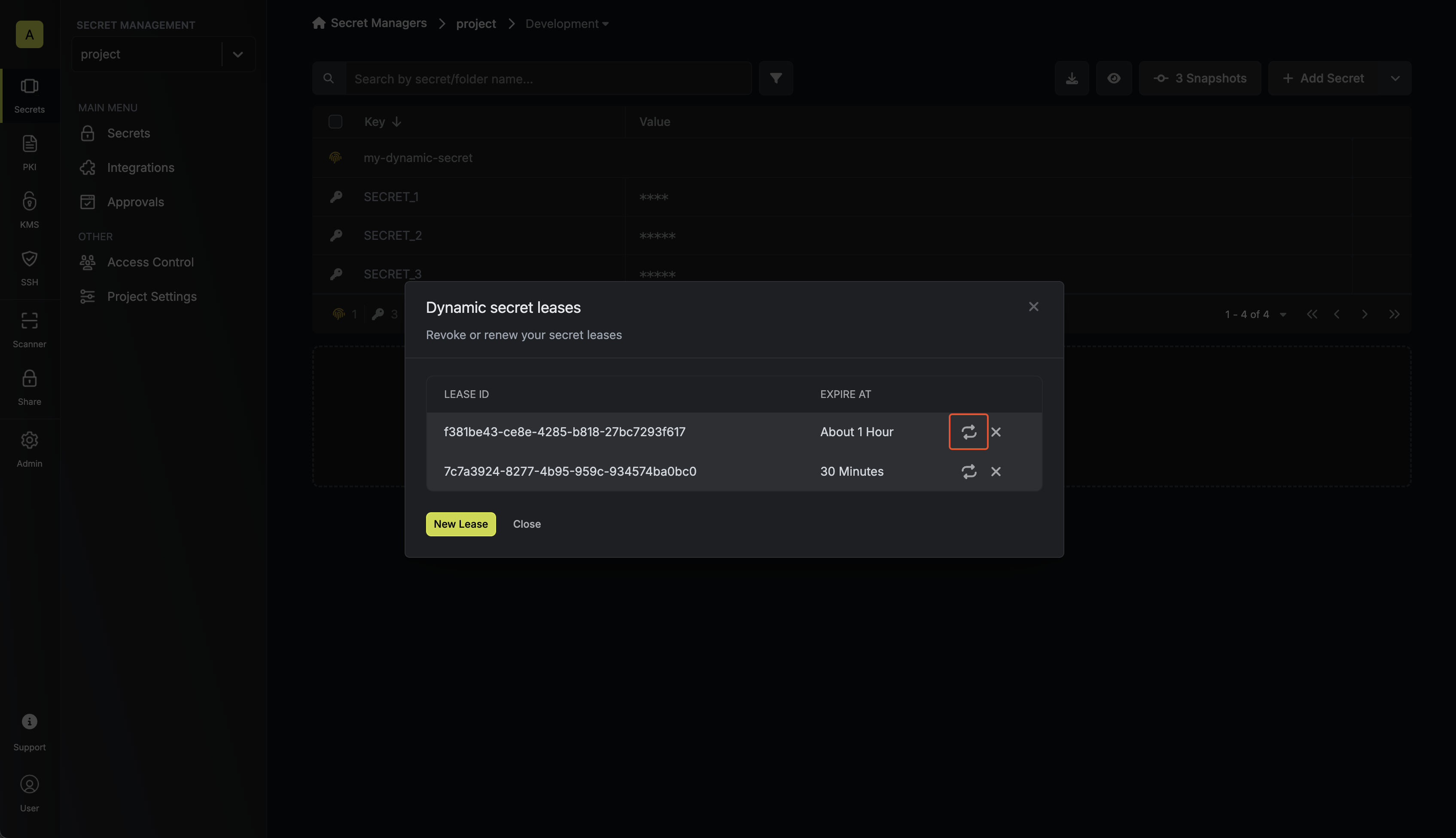
Lease renewals cannot exceed the maximum TTL set when configuring the dynamic secret
# MySQL
Learn how to dynamically generate MySQL Database user credentials.
The Infisical MySQL dynamic secret allows you to generate MySQL Database credentials on demand based on configured role.
## Prerequisite
Create a user with the required permission in your SQL instance. This user will be used to create new accounts on-demand.
## Set up Dynamic Secrets with MySQL
Open the Secret Overview dashboard and select the environment in which you would like to add a dynamic secret.
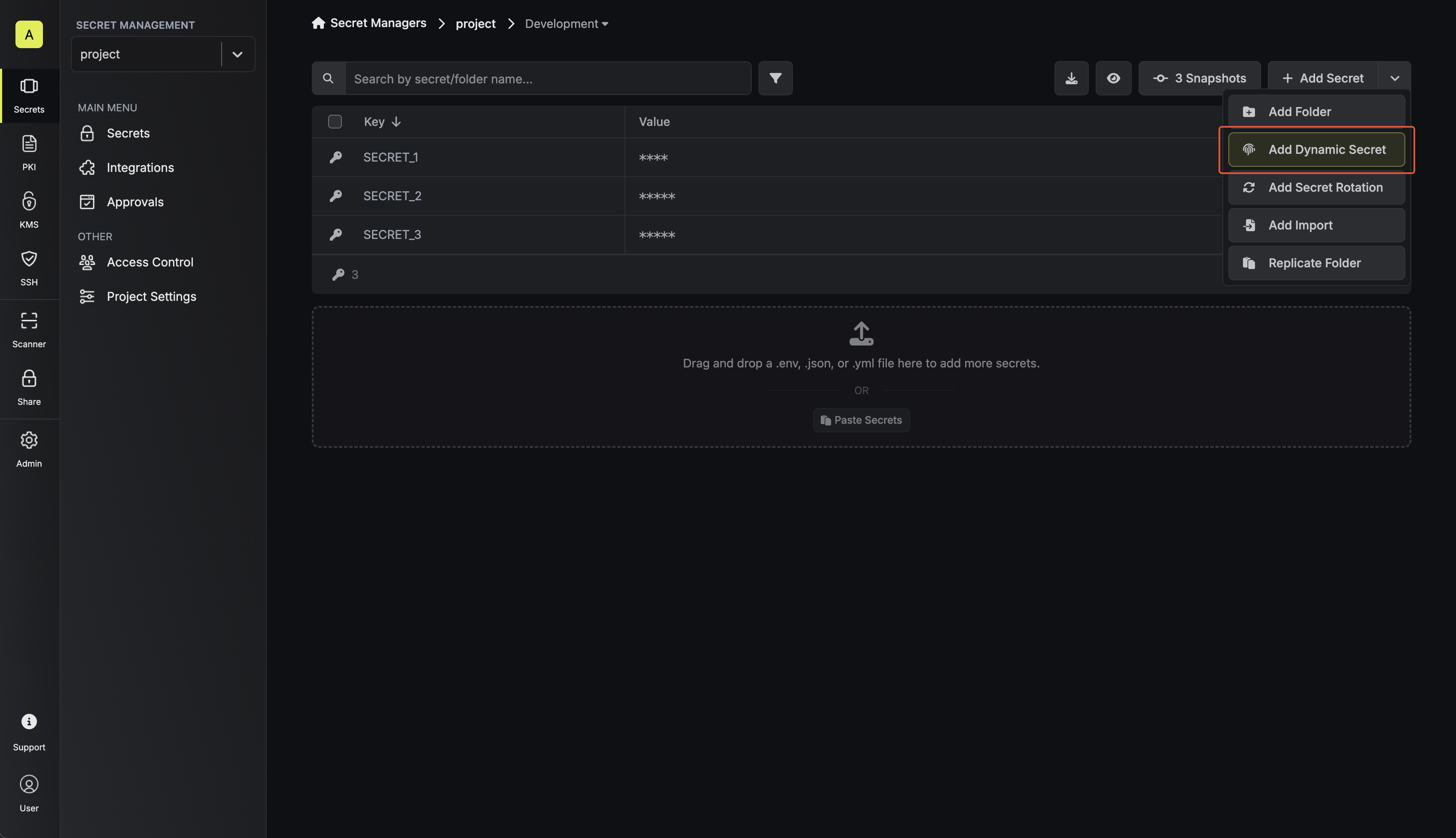
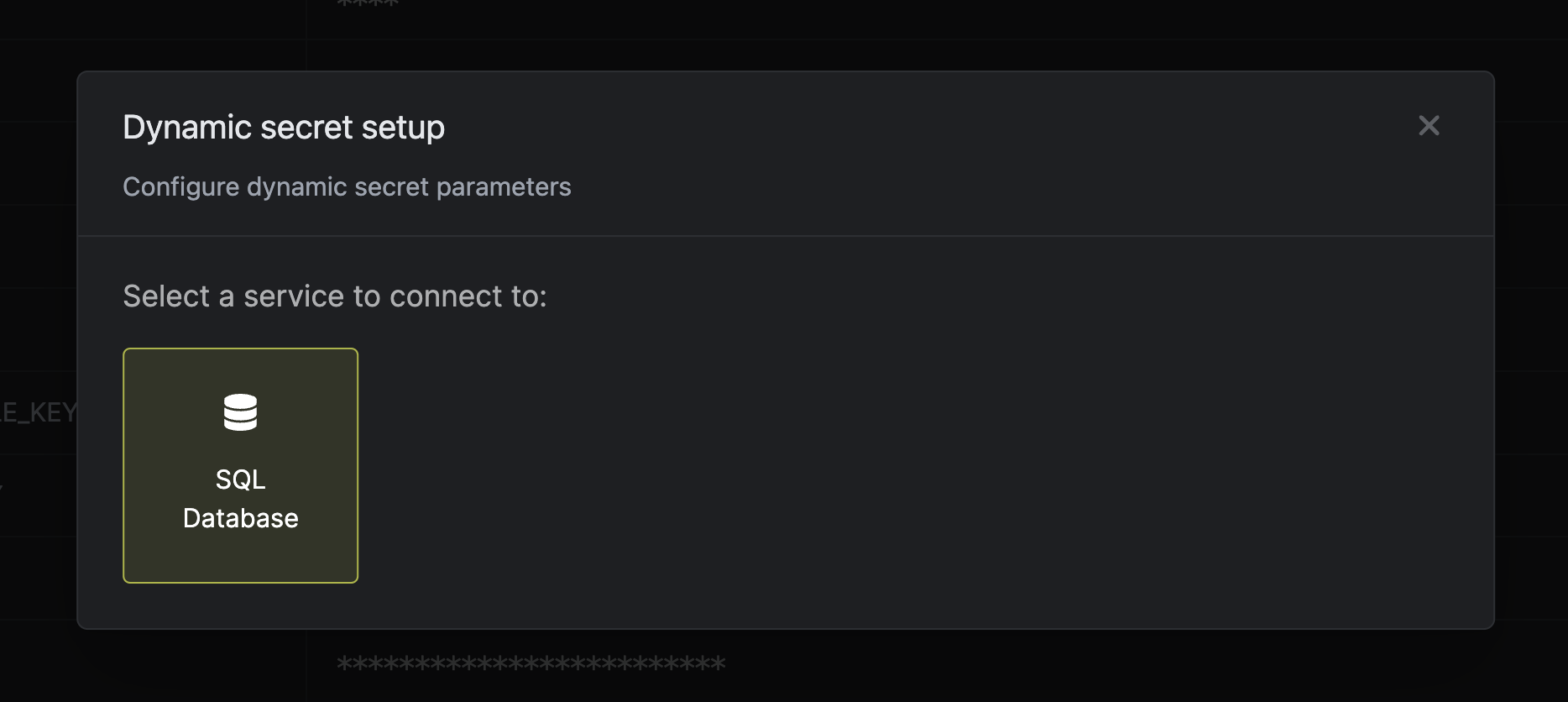
Name by which you want the secret to be referenced
Default time-to-live for a generated secret (it is possible to modify this value when a secret is generate)
Maximum time-to-live for a generated secret
Choose the service you want to generate dynamic secrets for. This must be selected as **MySQL**.
Database host
Database port
Username that will be used to create dynamic secrets
Password that will be used to create dynamic secrets
Name of the database for which you want to create dynamic secrets
A CA may be required if your DB requires it for incoming connections. AWS RDS instances with default settings will requires a CA which can be downloaded [here](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/UsingWithRDS.SSL.html#UsingWithRDS.SSL.CertificatesAllRegions).
If you want to provide specific privileges for the generated dynamic credentials, you can modify the SQL statement to your needs. This is useful if you want to only give access to a specific table(s).
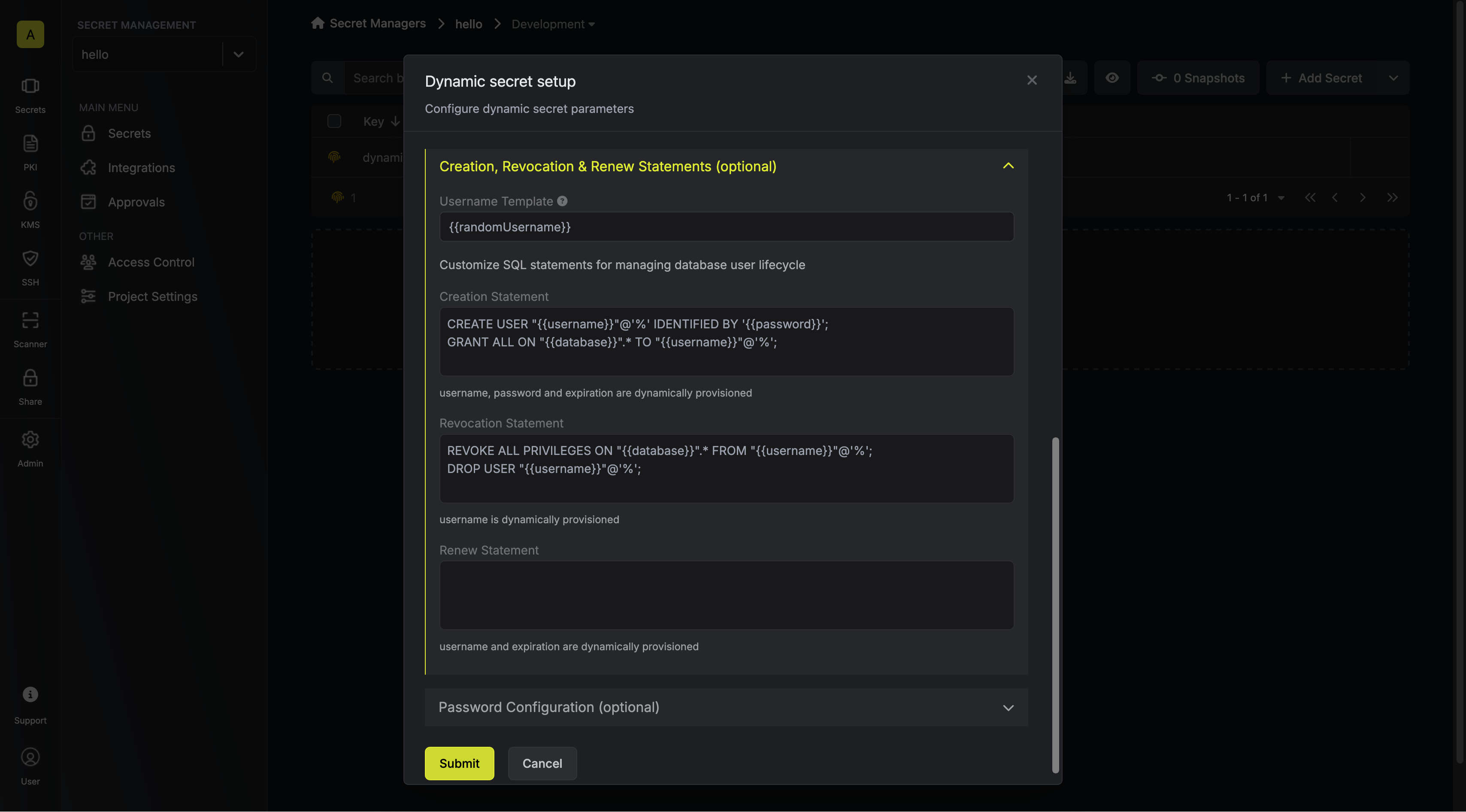
After submitting the form, you will see a dynamic secret created in the dashboard.
If this step fails, you may have to add the CA certificate.

Once you've successfully configured the dynamic secret, you're ready to generate on-demand credentials.
To do this, simply click on the 'Generate' button which appears when hovering over the dynamic secret item.
Alternatively, you can initiate the creation of a new lease by selecting 'New Lease' from the dynamic secret lease list section.
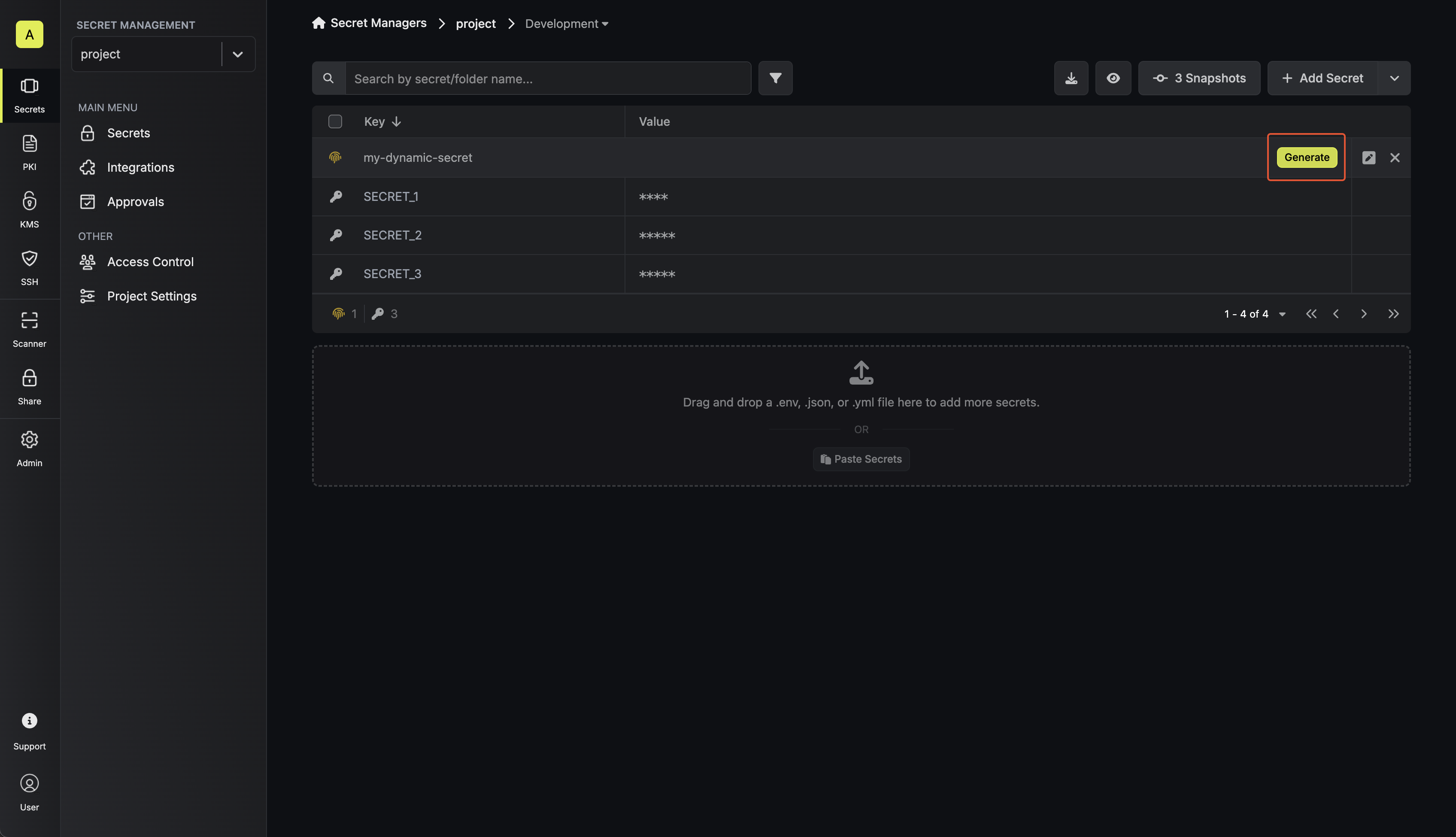
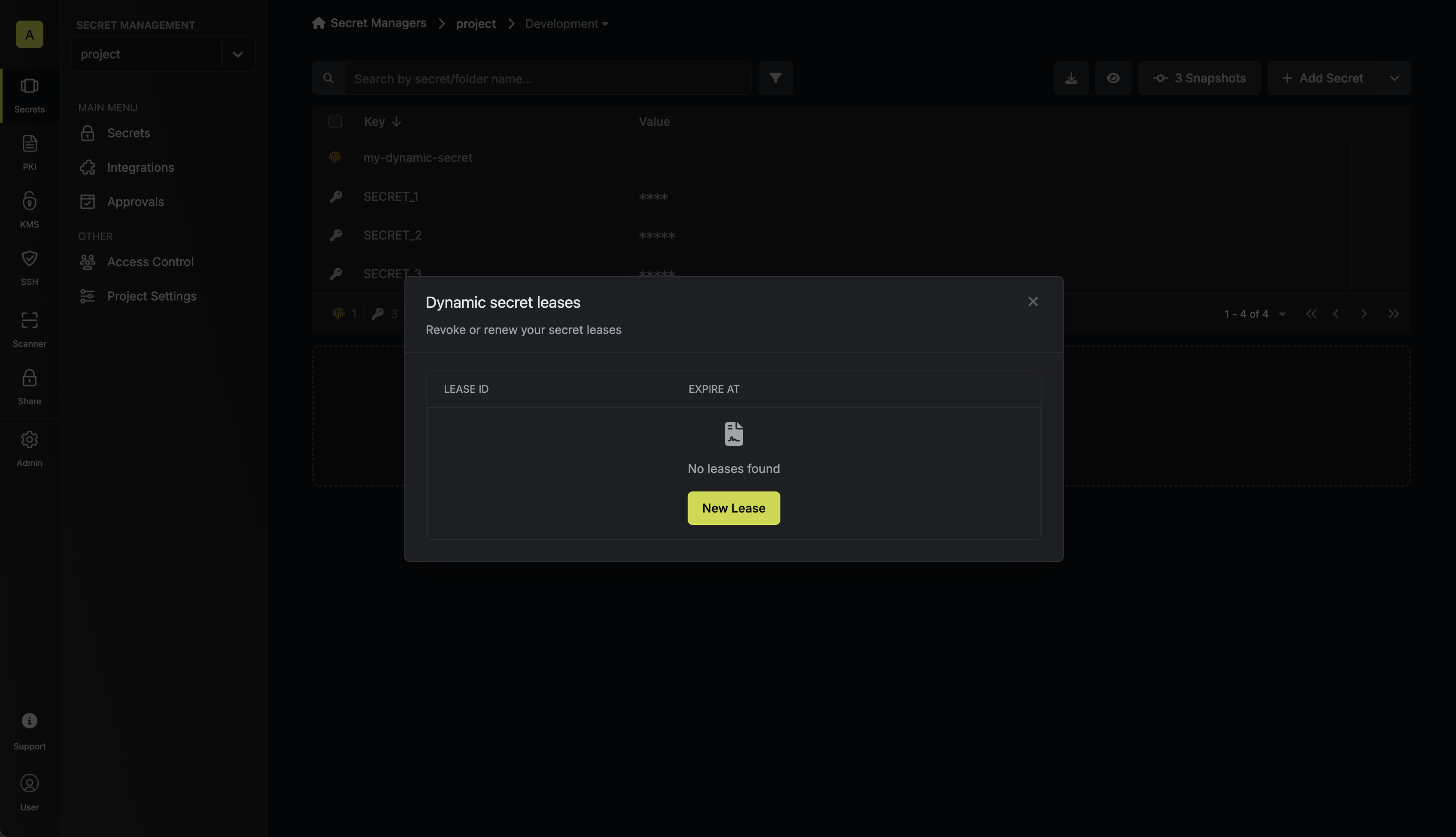
When generating these secrets, it's important to specify a Time-to-Live (TTL) duration. This will dictate how long the credentials are valid for.
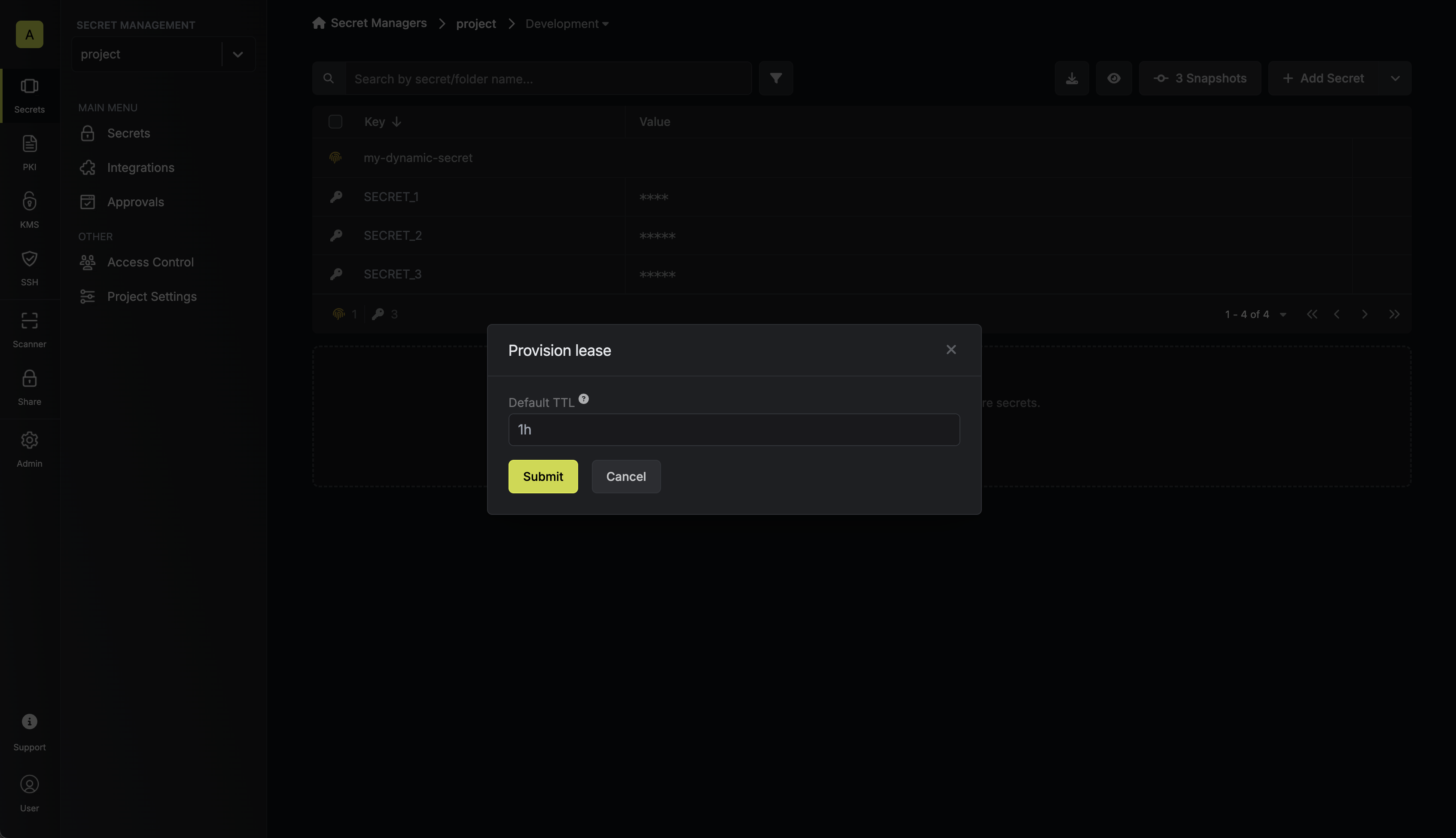
Ensure that the TTL for the lease fall within the maximum TTL defined when configuring the dynamic secret.
Once you click the `Submit` button, a new secret lease will be generated and the credentials from it will be shown to you.
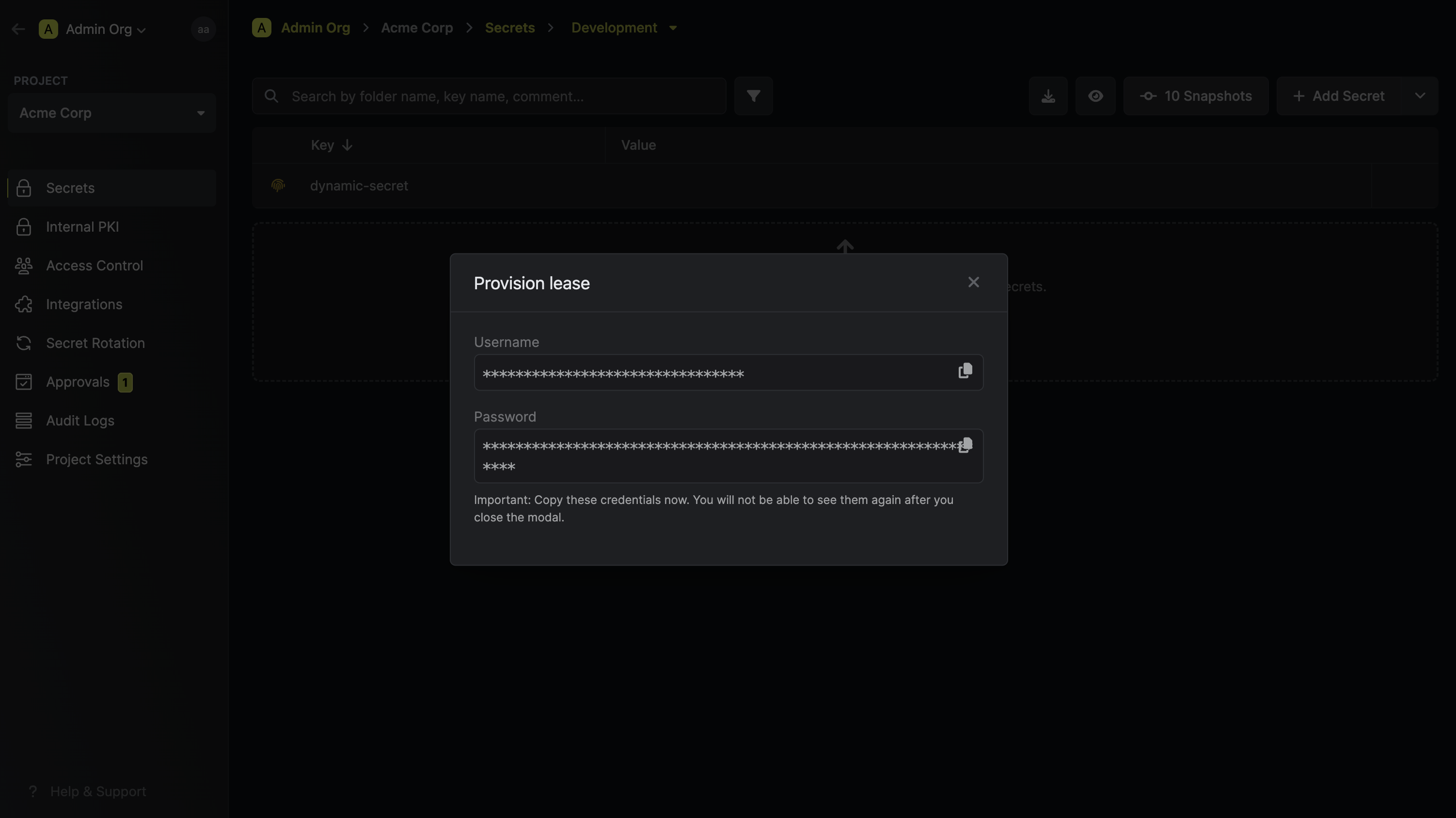
## Audit or Revoke Leases
Once you have created one or more leases, you will be able to access them by clicking on the respective dynamic secret item on the dashboard.
This will allow you see the expiration time of the lease or delete a lease before it's set time to live.
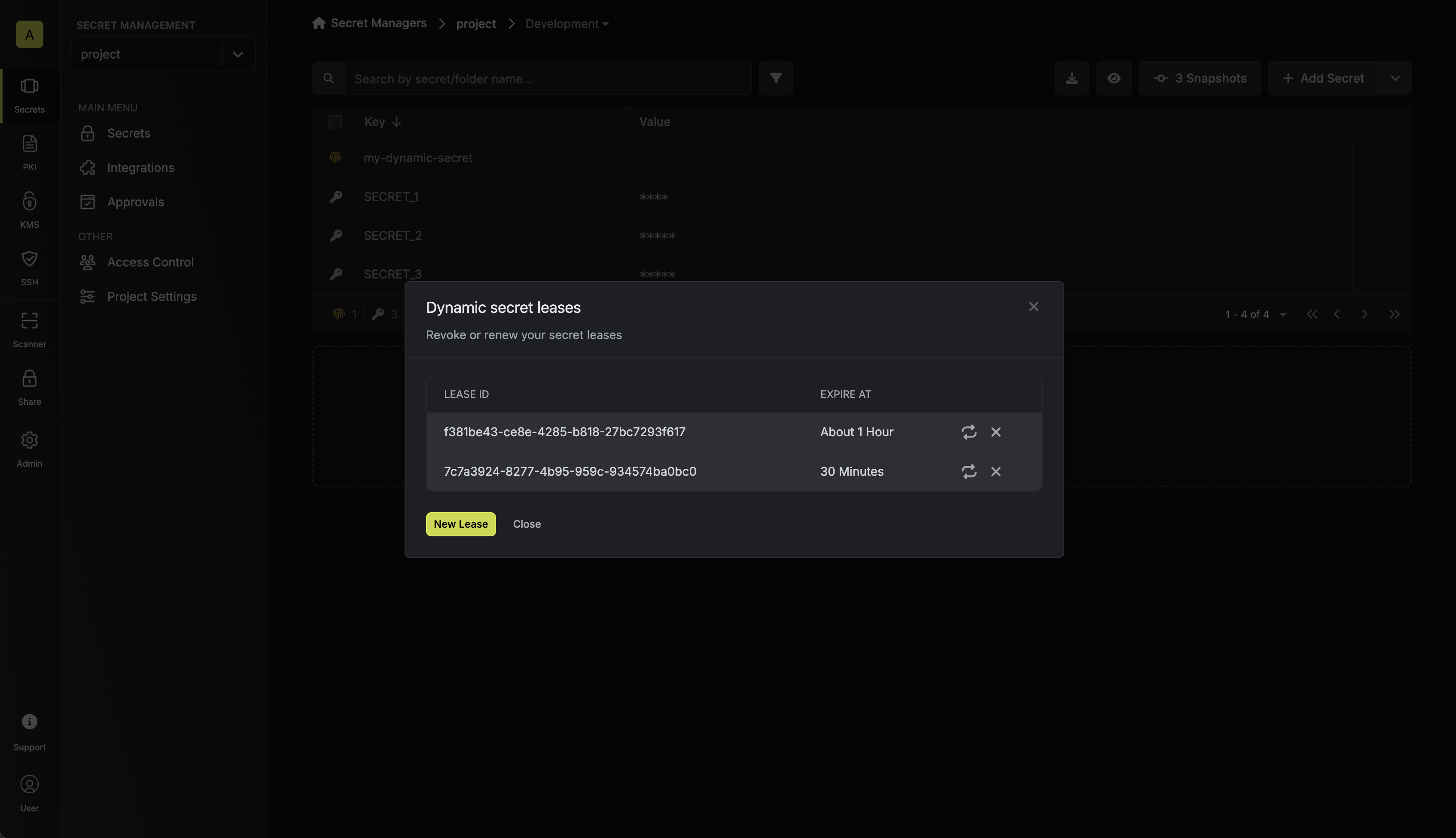
## Renew Leases
To extend the life of the generated dynamic secret leases past its initial time to live, simply click on the **Renew** as illustrated below.
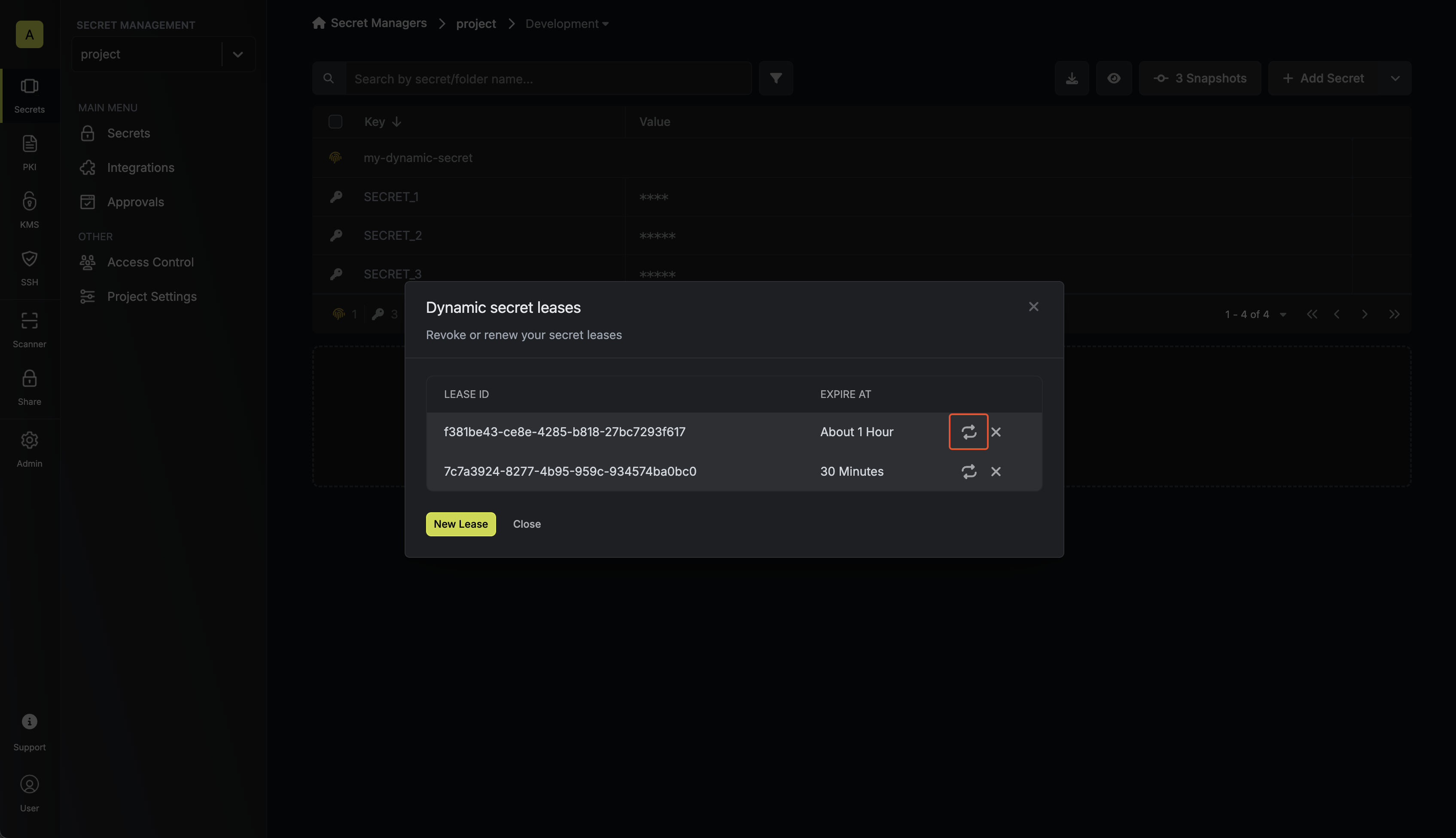
Lease renewals cannot exceed the maximum TTL set when configuring the dynamic secret
# Oracle
Learn how to dynamically generate Oracle Database user credentials.
The Infisical Oracle dynamic secret allows you to generate Oracle Database credentials on demand based on configured role.
## Prerequisite
Create a user with the required permission in your SQL instance. This user will be used to create new accounts on-demand.
## Set up Dynamic Secrets with Oracle
Open the Secret Overview dashboard and select the environment in which you would like to add a dynamic secret.
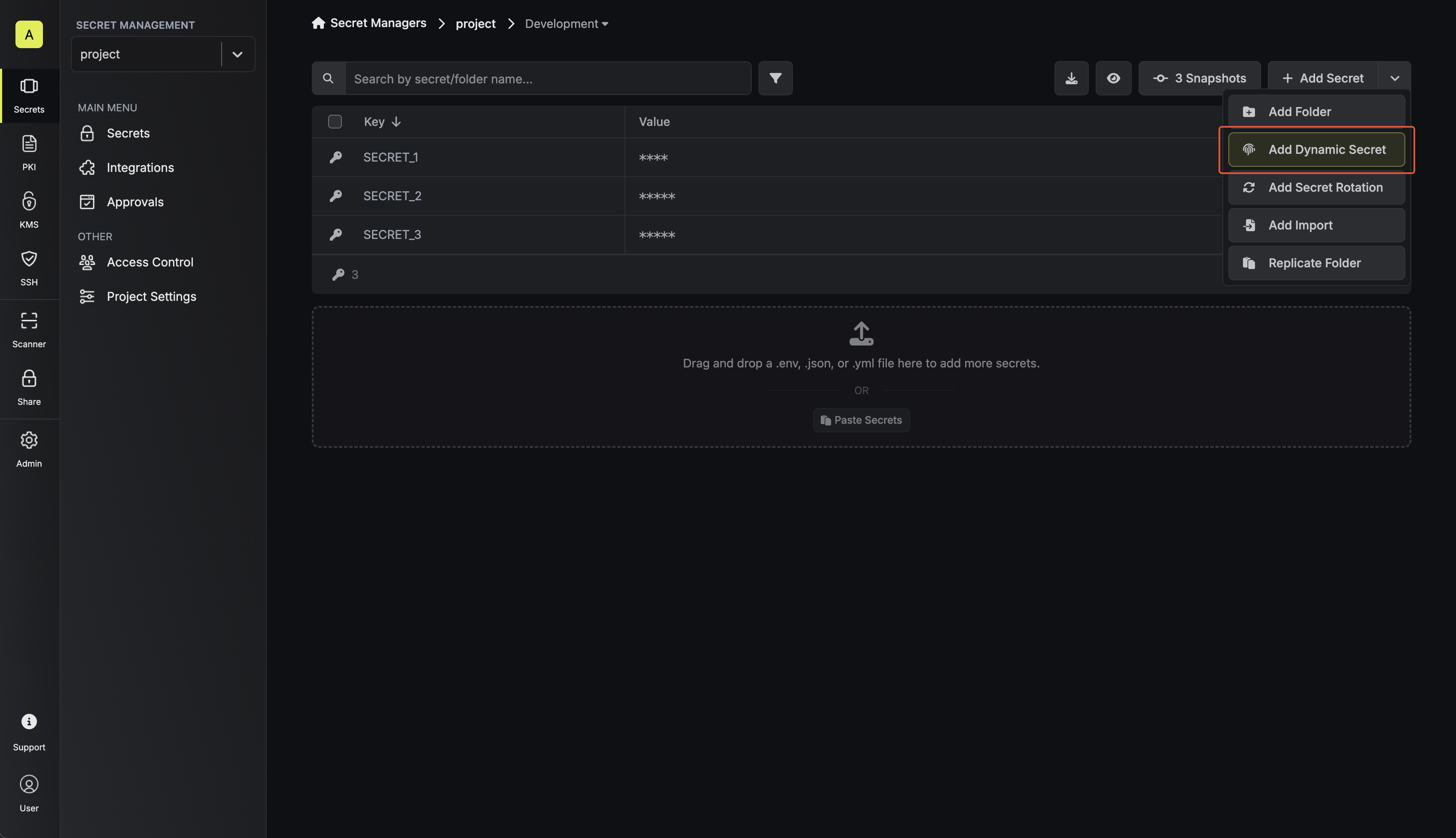
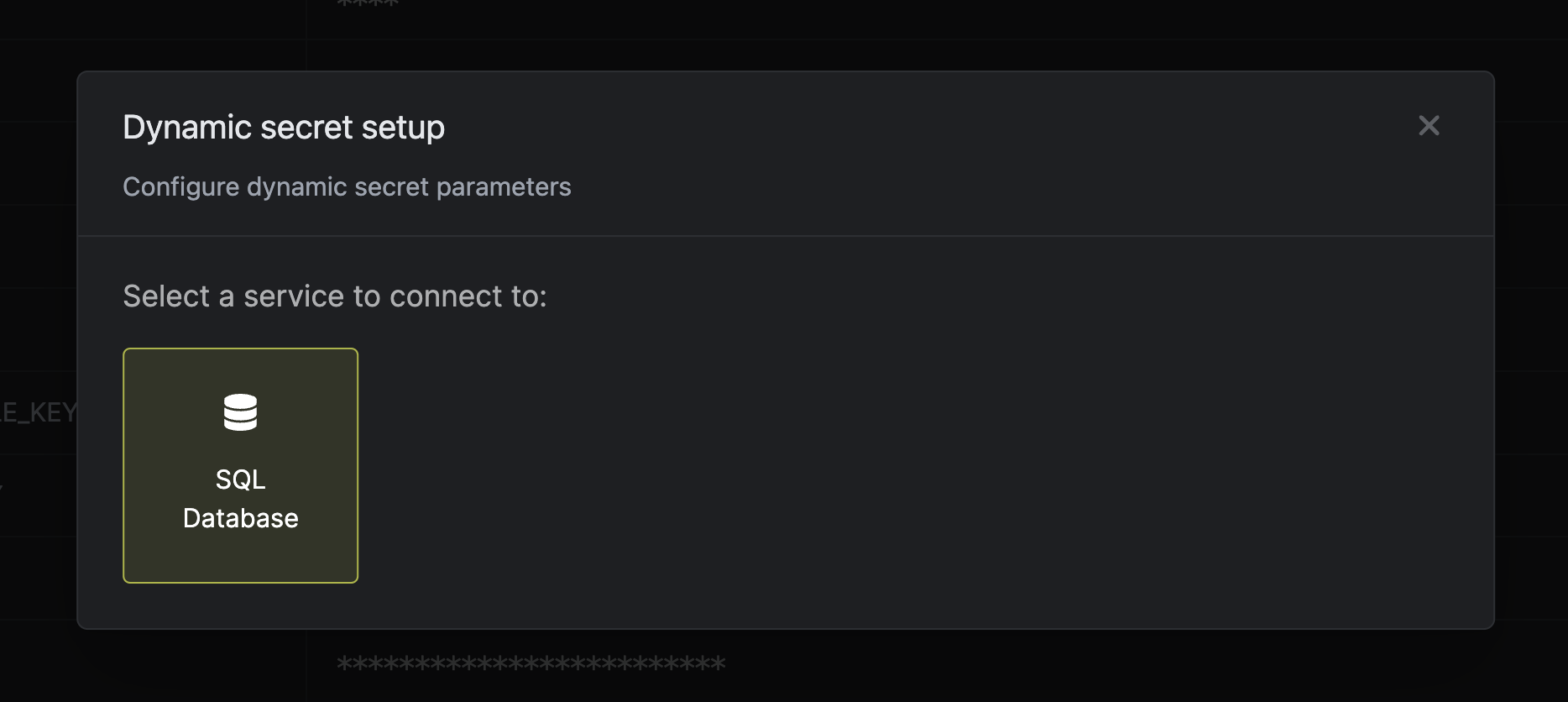
Name by which you want the secret to be referenced
Default time-to-live for a generated secret (it is possible to modify this value when a secret is generate)
Maximum time-to-live for a generated secret
Choose the service you want to generate dynamic secrets for. This must be selected as **Oracle**.
Database host
Database port
Username that will be used to create dynamic secrets
Password that will be used to create dynamic secrets
Name of the database for which you want to create dynamic secrets
A CA may be required if your DB requires it for incoming connections. AWS RDS instances with default settings will requires a CA which can be downloaded [here](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/UsingWithRDS.SSL.html#UsingWithRDS.SSL.CertificatesAllRegions).

If you want to provide specific privileges for the generated dynamic credentials, you can modify the SQL statement to your needs. This is useful if you want to only give access to a specific table(s).
After submitting the form, you will see a dynamic secret created in the dashboard.
If this step fails, you may have to add the CA certficate.

Once you've successfully configured the dynamic secret, you're ready to generate on-demand credentials.
To do this, simply click on the 'Generate' button which appears when hovering over the dynamic secret item.
Alternatively, you can initiate the creation of a new lease by selecting 'New Lease' from the dynamic secret lease list section.
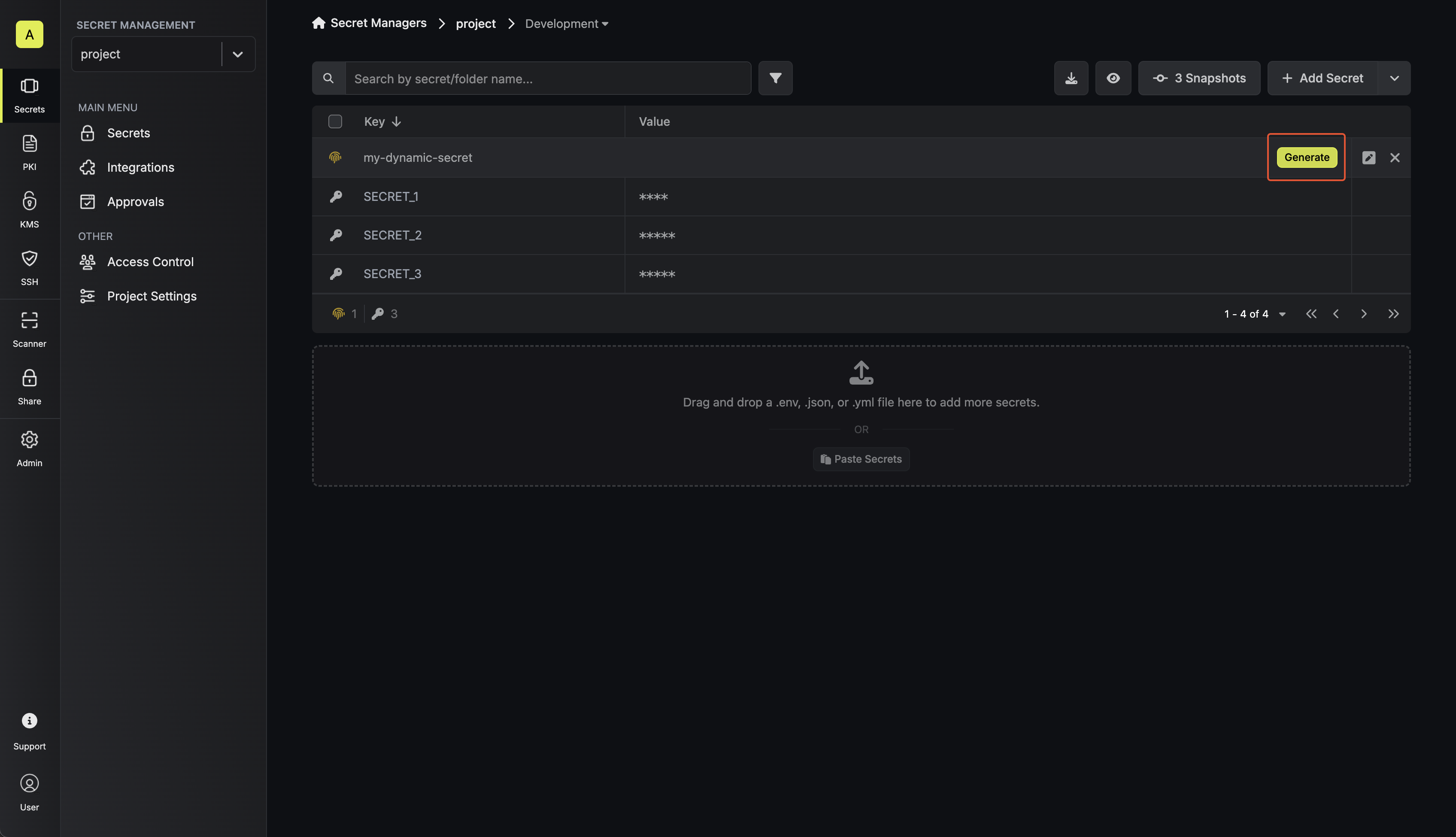
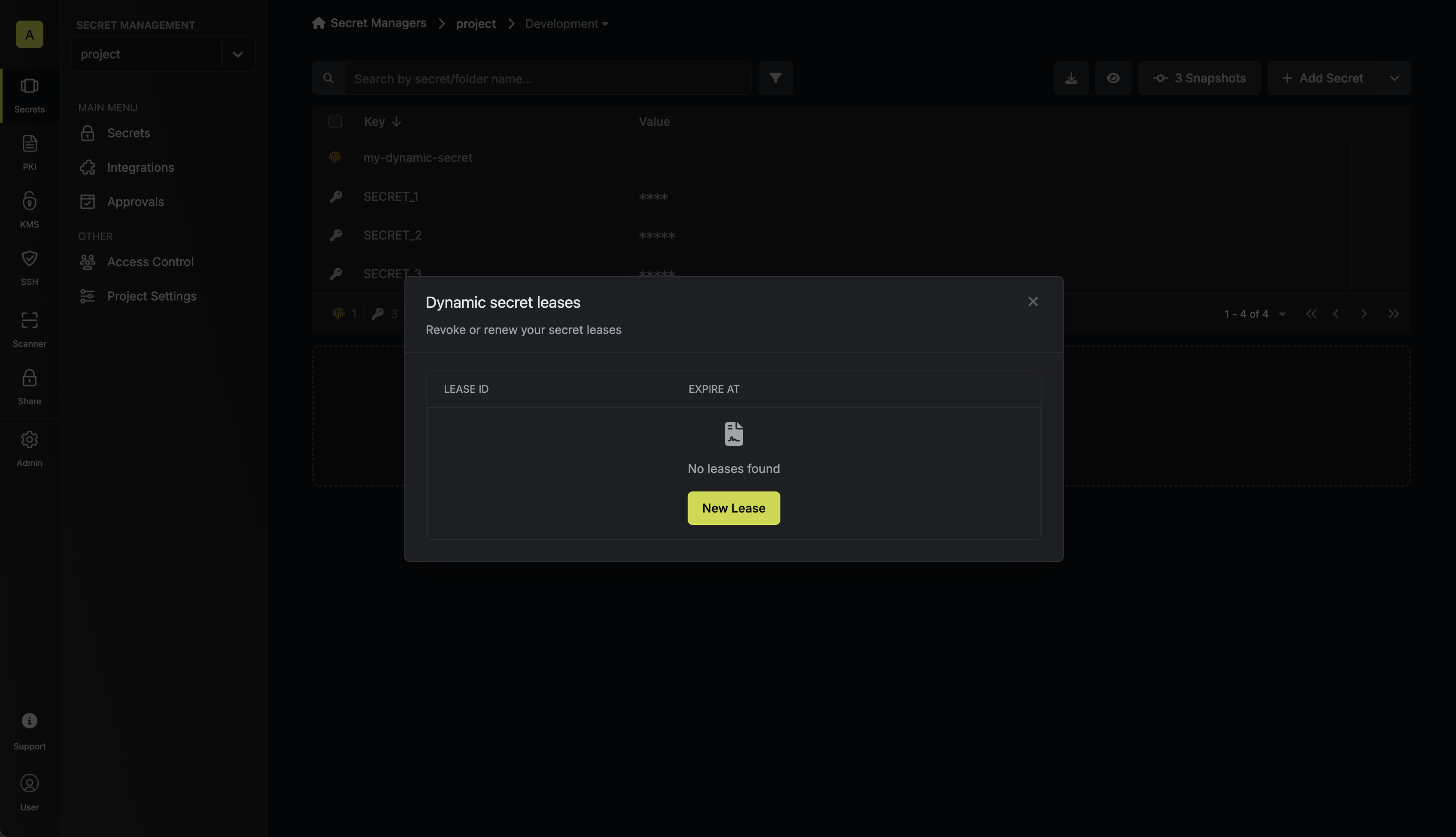
When generating these secrets, it's important to specify a Time-to-Live (TTL) duration. This will dictate how long the credentials are valid for.
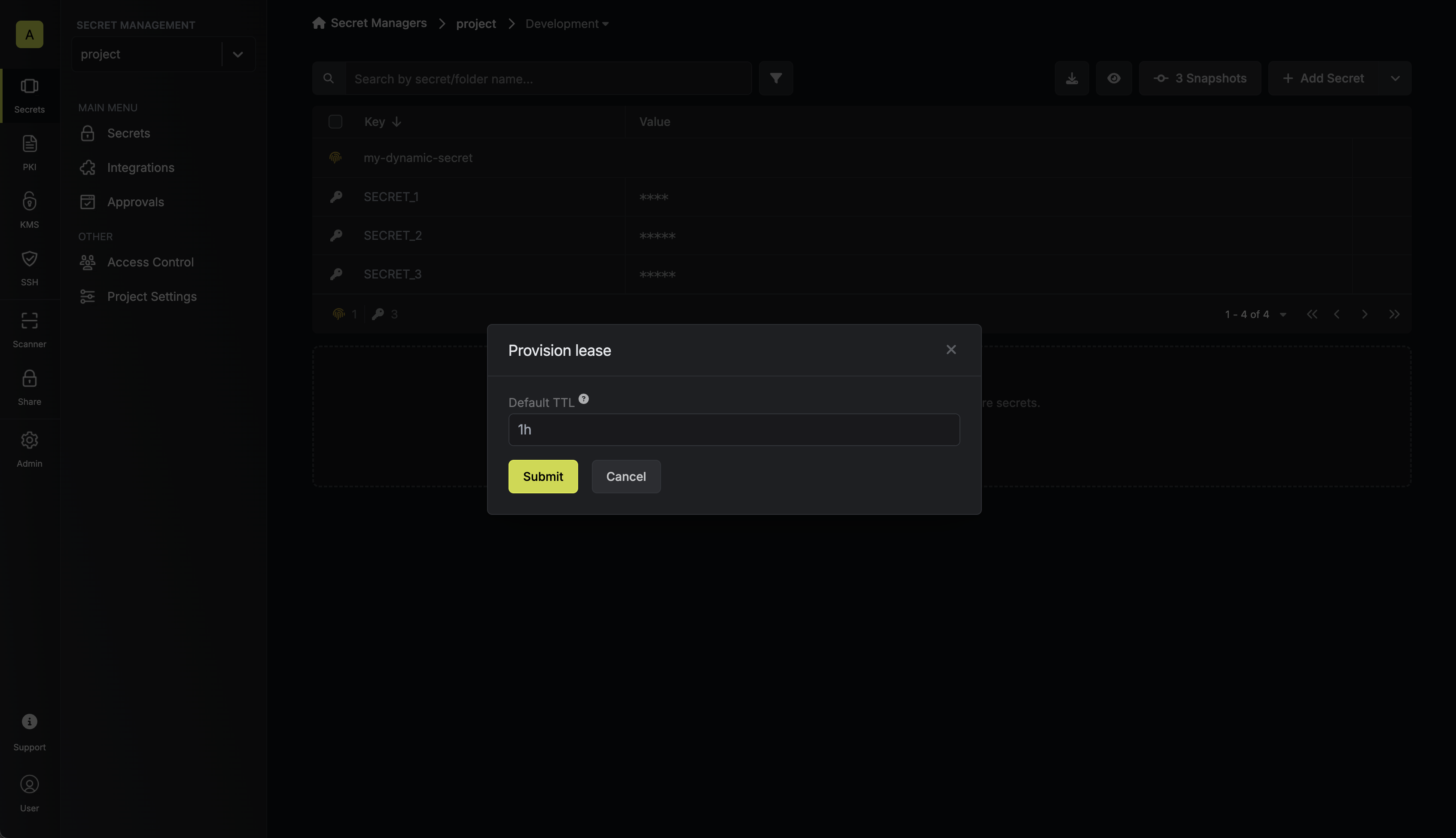
Ensure that the TTL for the lease fall within the maximum TTL defined when configuring the dynamic secret.
Once you click the `Submit` button, a new secret lease will be generated and the credentials for it will be shown to you.
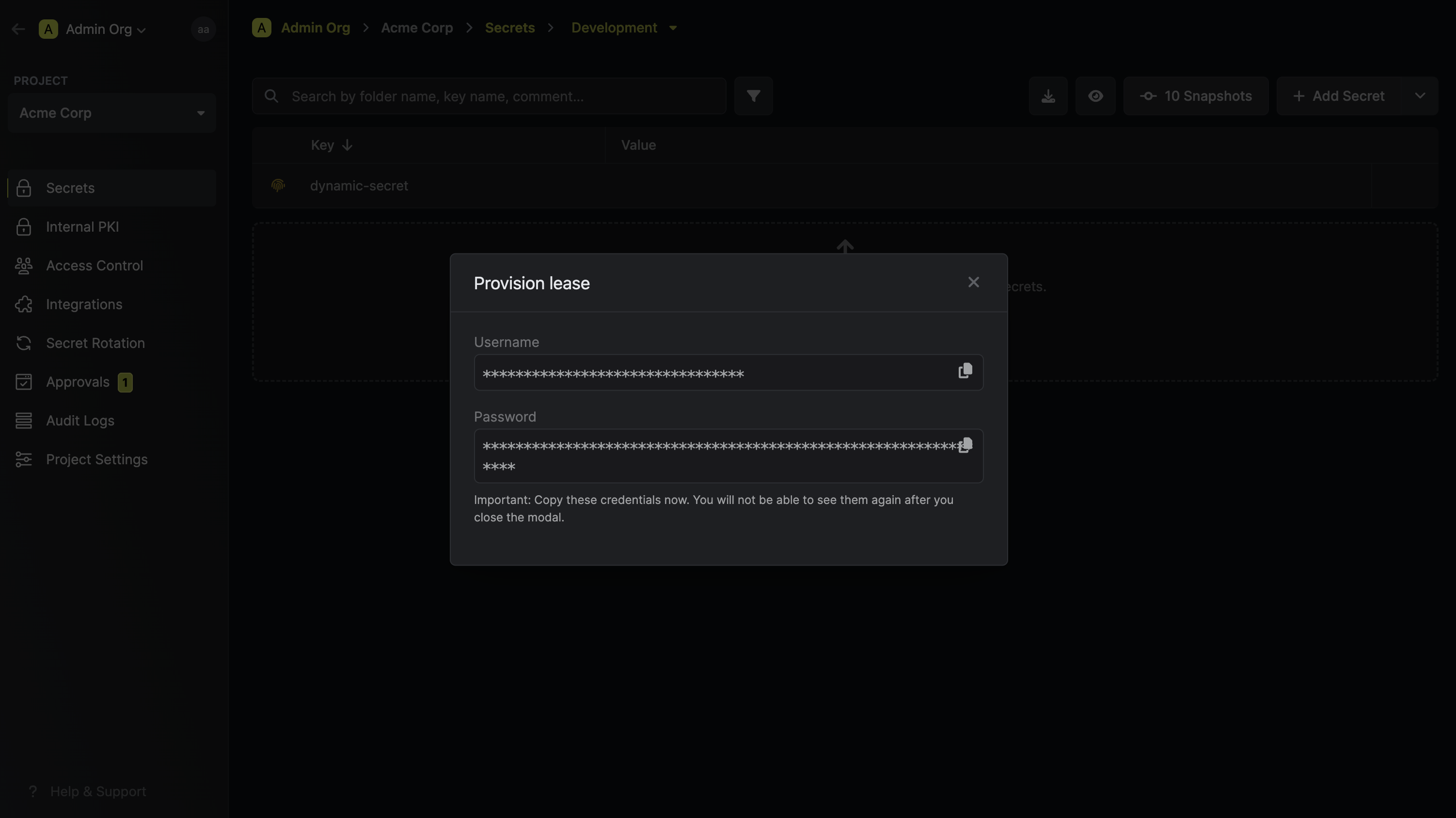
## Audit or Revoke Leases
Once you have created one or more leases, you will be able to access them by clicking on the respective dynamic secret item on the dashboard.
This will allow you see the expiration time of the lease or delete a lease before it's set time to live.
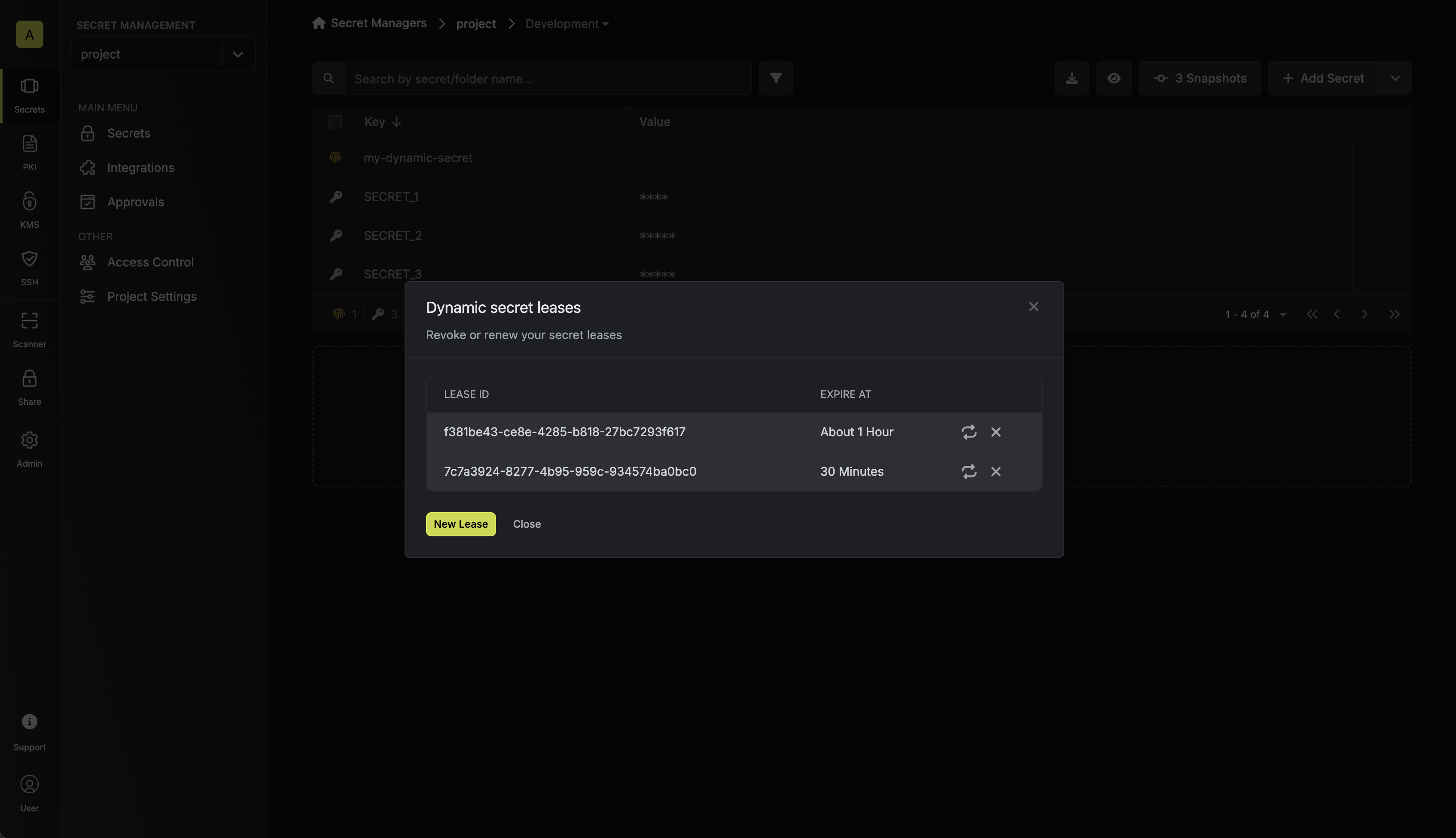
## Renew Leases
To extend the life of the generated dynamic secret leases past its initial time to live, simply click on the **Renew** as illustrated below.
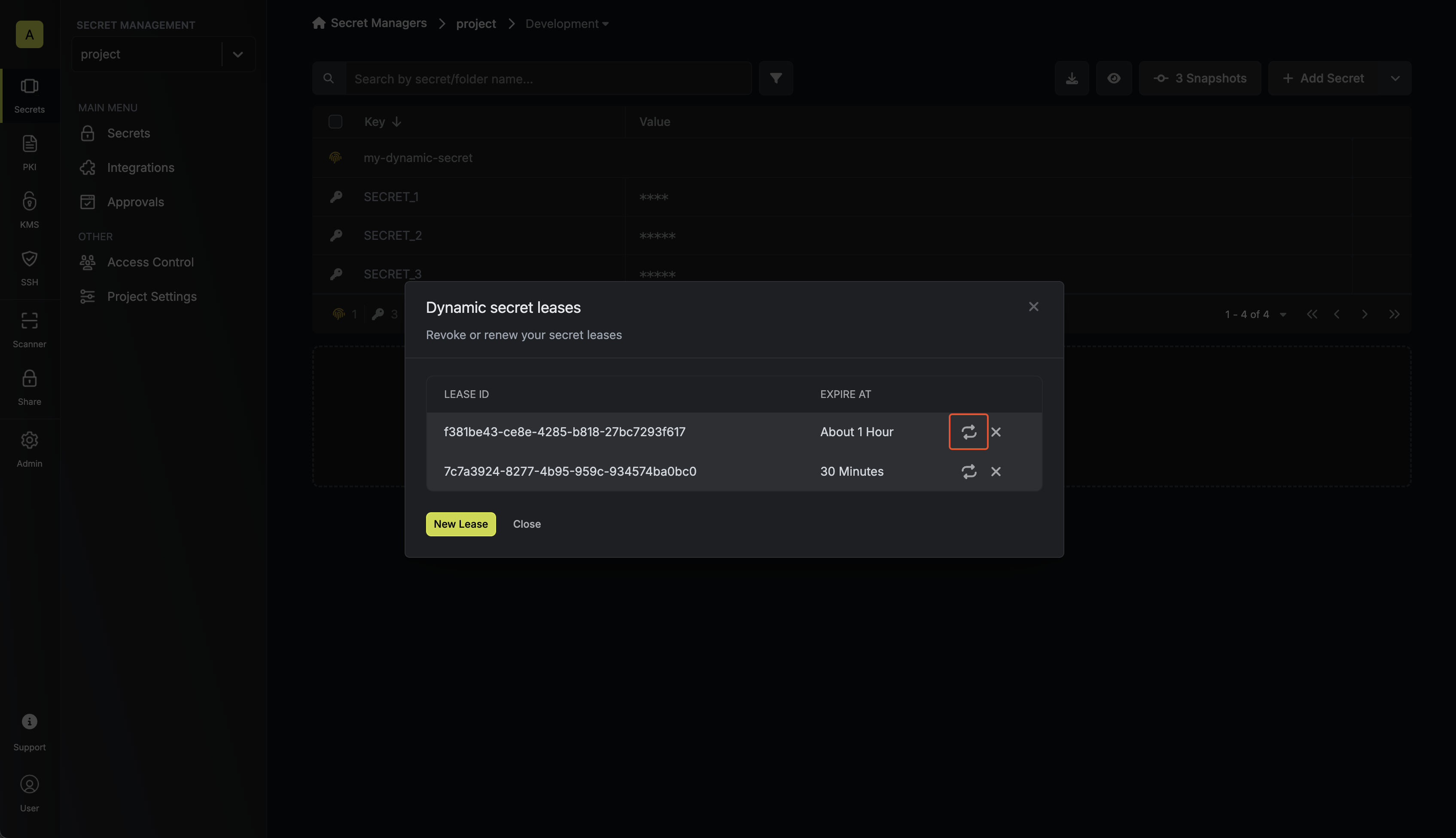
Lease renewals cannot exceed the maximum TTL set when configuring the dynamic secret
# Dynamic Secrets
Learn how to generate secrets dynamically on-demand.
## Introduction
Contrary to static key-value secrets, which require manual input of data into the secure Infisical storage, **dynamic secrets are generated on-demand upon access**.
**Dynamic secrets are unique to every identity using them**. Such secrets come are generated only at the moment they are retrieved, eliminating the possibility of theft or reuse by another identity. Thanks to Infisical's integrated revocation capabilities, dynamic secrets can be promptly invalidated post-use, significantly reducing their lifespan.
## Benefits of Dynamic Secrets
This approach offers several advantages in terms of security and management:
* **Enhanced Security**: By frequently changing secrets, dynamic secrets minimize the risk associated with secret compromise. Even if an attacker manages to obtain a secret, it would likely be invalid by the time they attempt to use it.
* **Reduced Secret Lifetime**: The limited validity period of dynamic secrets means that they are less valuable targets for attackers. This inherently reduces the time window during which a secret can be exploited.
* **Automated Management**: Dynamic secrets enable automated systems to handle the generation, distribution, revocation, and rotation of secrets without human intervention, thus reducing the risk of human error.
* **Auditing and Traceability**: The generation of dynamic secrets can be tightly controlled and monitored. This allows for detailed auditing of who accessed what secret and when, improving overall security posture and compliance with regulatory standards.
* **Scalability**: Dynamic secret management systems can scale more effectively to handle a large number of services and applications, as they automate much of the overhead associated with manual secret management.
Dynamic secrets are particularly useful in environments with stringent security requirements, such as cloud environments, distributed systems, and microservices architectures, where they help to manage database credentials, API keys, tokens, and other types of secrets.
## Infisical Dynamic Secret Templates
1. [PostgreSQL](./postgresql)
2. [MySQL](./mysql)
3. [Cassandra](./cassandra)
4. [Oracle](./oracle)
5. [Redis](./redis)
6. [AWS IAM](./aws-iam)
# PostgreSQL
Learn how to dynamically generate PostgreSQL database users.
The Infisical PostgreSQL dynamic secret allows you to generate PostgreSQL database credentials on demand based on configured role.
## Prerequisite
Create a user with the required permission in your SQL instance. This user will be used to create new accounts on-demand.
## Set up Dynamic Secrets with PostgreSQL
Open the Secret Overview dashboard and select the environment in which you would like to add a dynamic secret.
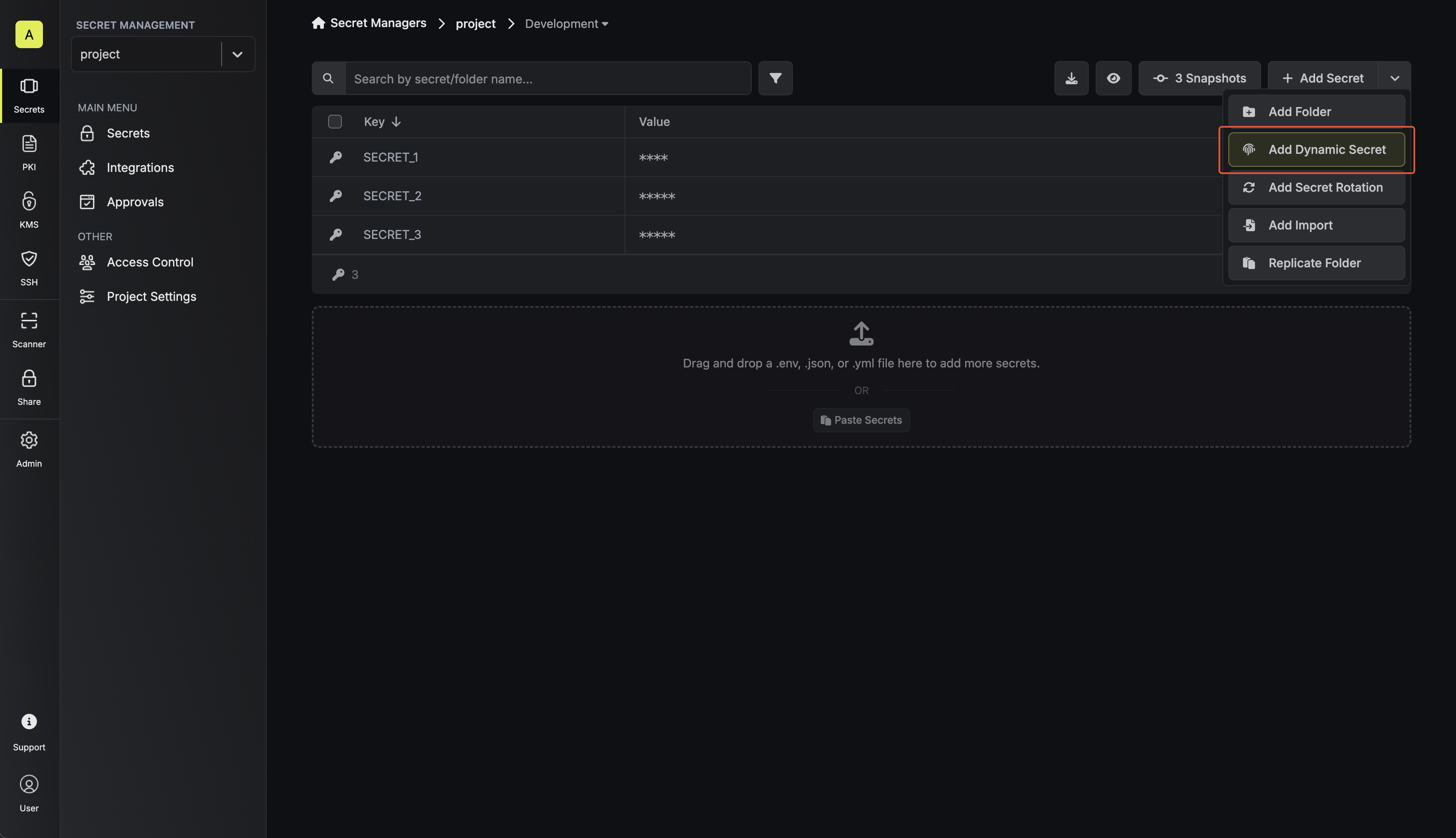
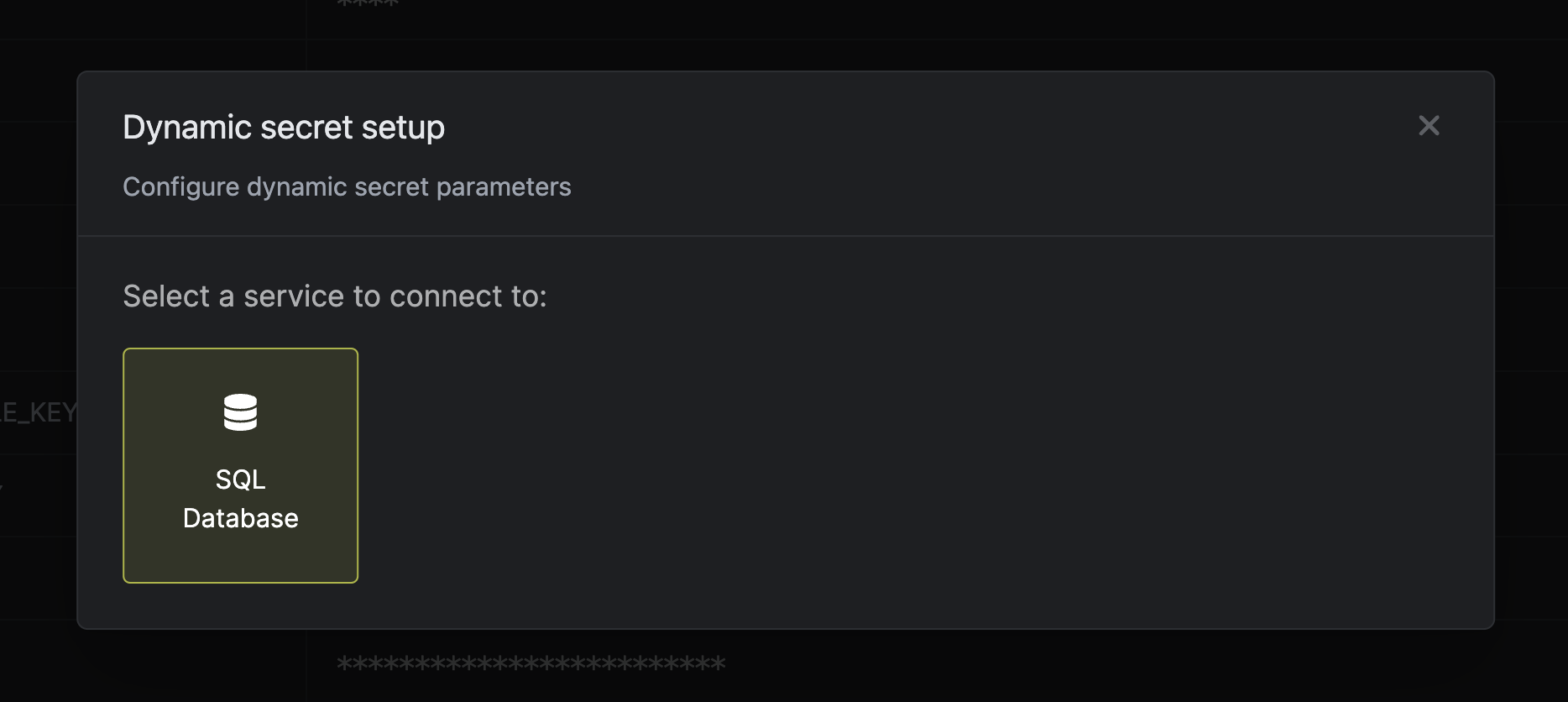
Name by which you want the secret to be referenced
Default time-to-live for a generated secret (it is possible to modify this value when a secret is generate)
Maximum time-to-live for a generated secret
Choose the service you want to generate dynamic secrets for. This must be selected as **PostgreSQL**.
Database host
Database port
Username that will be used to create dynamic secrets
Password that will be used to create dynamic secrets
Name of the database for which you want to create dynamic secrets
A CA may be required if your DB requires it for incoming connections. AWS RDS instances with default settings will requires a CA which can be downloaded [here](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/UsingWithRDS.SSL.html#UsingWithRDS.SSL.CertificatesAllRegions).
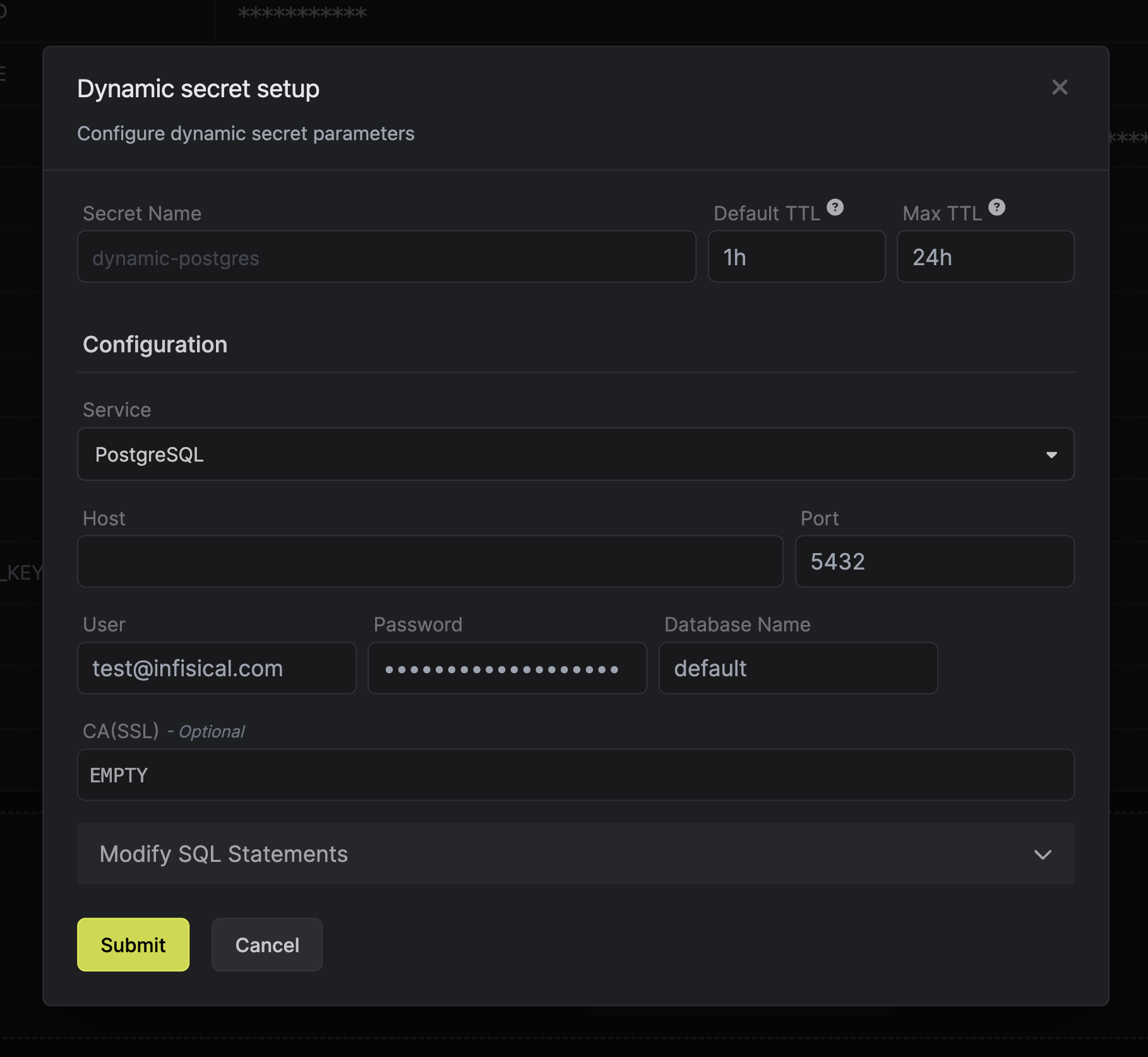
If you want to provide specific privileges for the generated dynamic credentials, you can modify the SQL statement to your needs. This is useful if you want to only give access to a specific table(s).
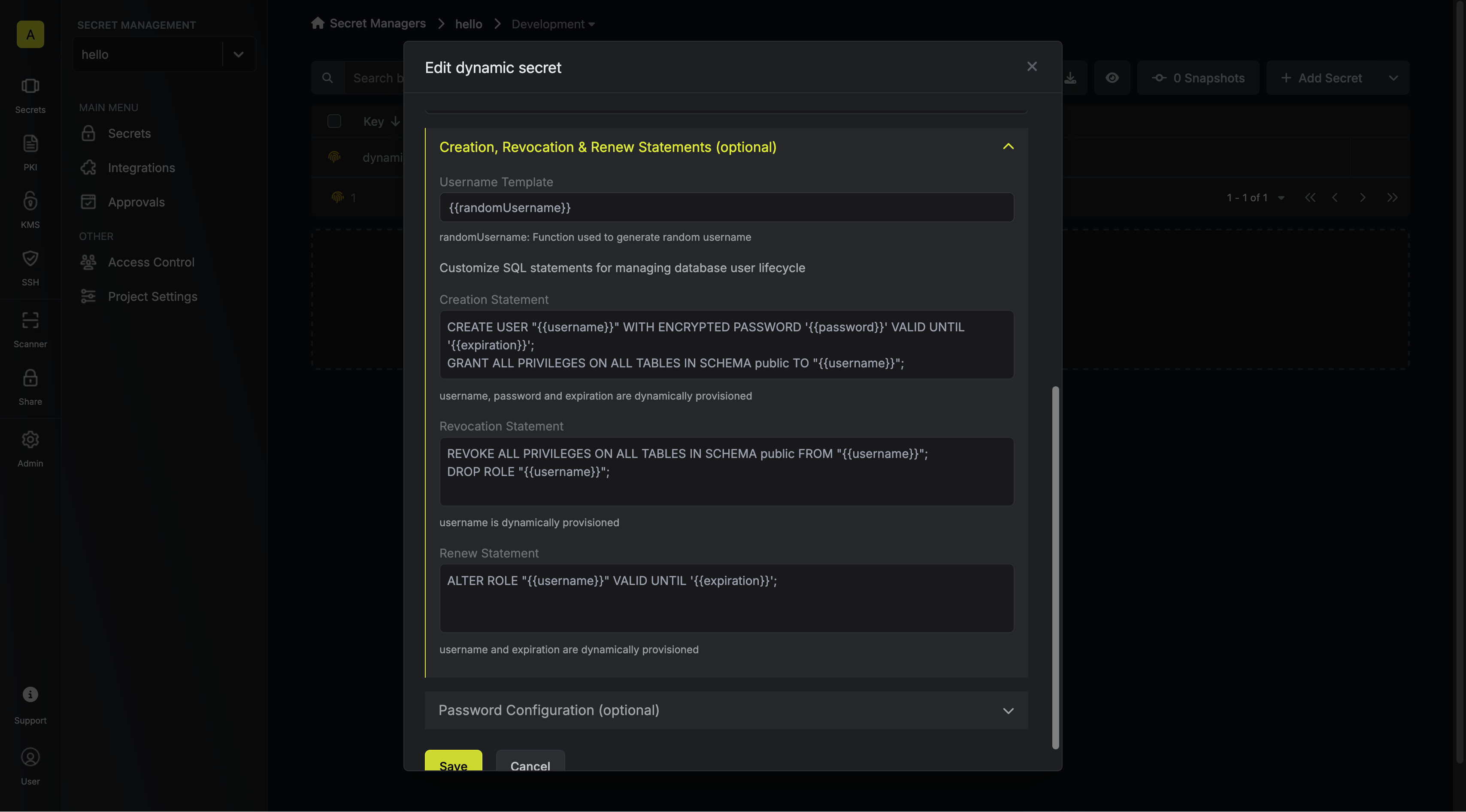
After submitting the form, you will see a dynamic secret created in the dashboard.
If this step fails, you may have to add the CA certficate.

Once you've successfully configured the dynamic secret, you're ready to generate on-demand credentials.
To do this, simply click on the 'Generate' button which appears when hovering over the dynamic secret item.
Alternatively, you can initiate the creation of a new lease by selecting 'New Lease' from the dynamic secret lease list section.
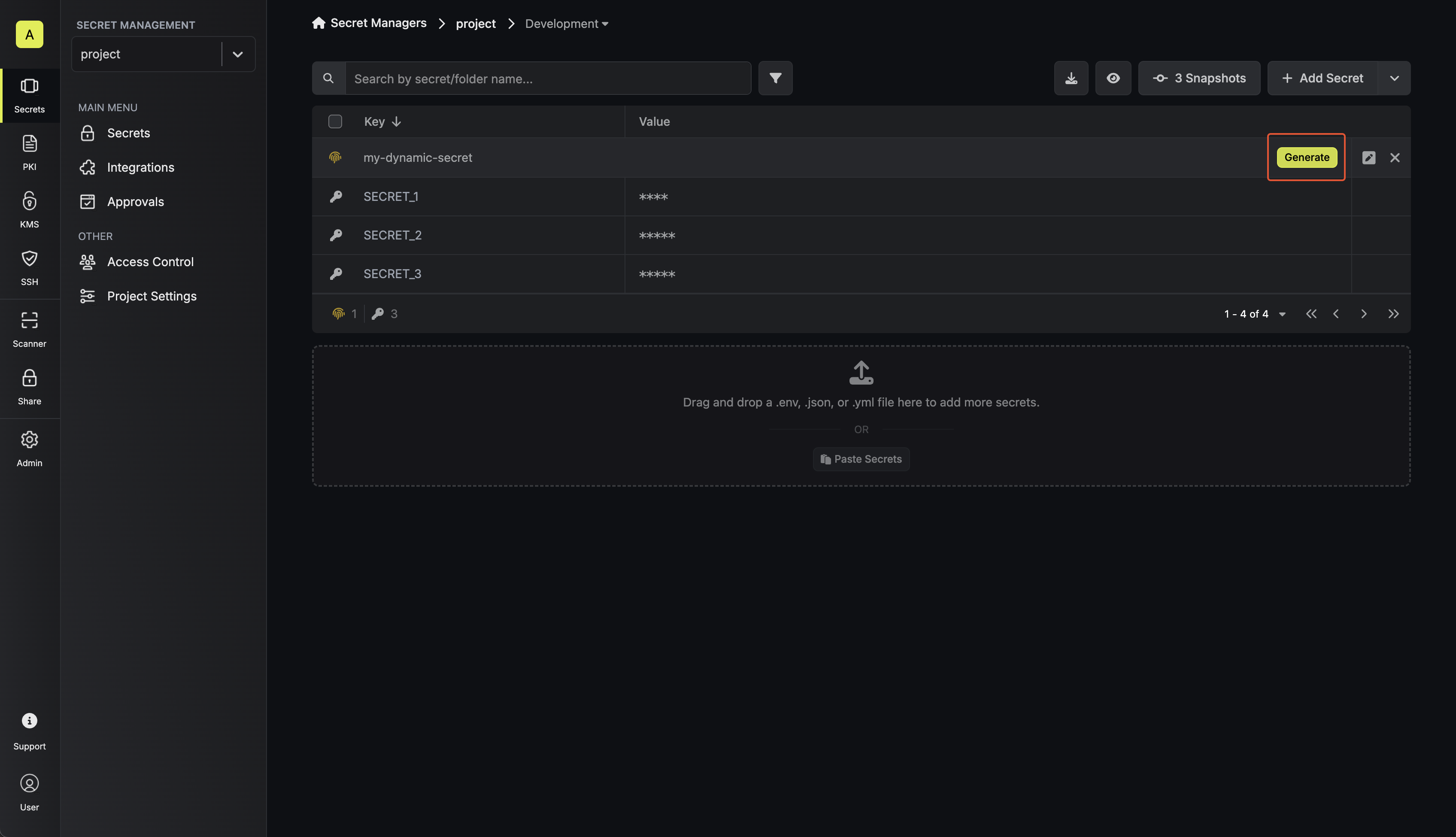
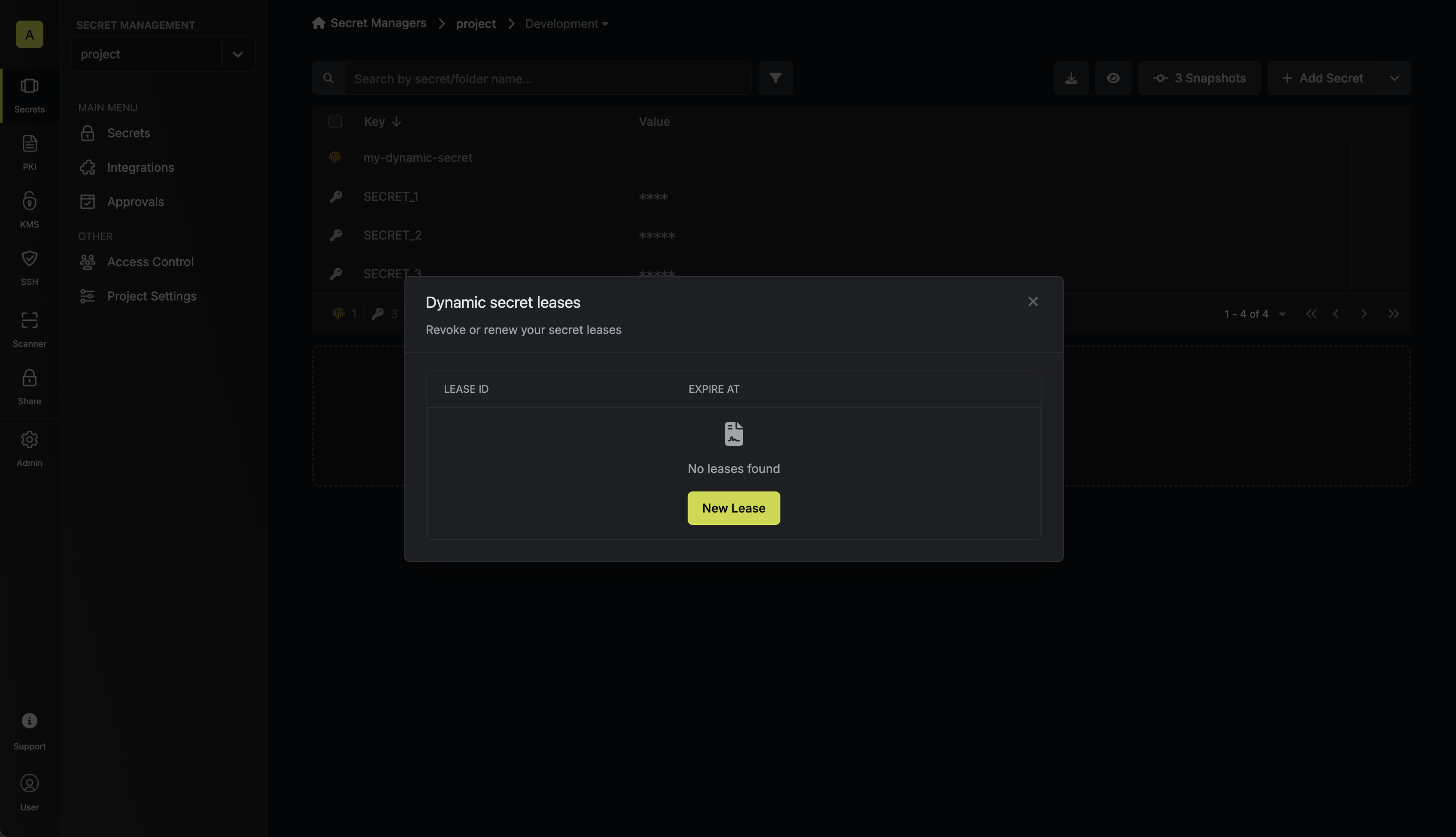
When generating these secrets, it's important to specify a Time-to-Live (TTL) duration. This will dictate how long the credentials are valid for.
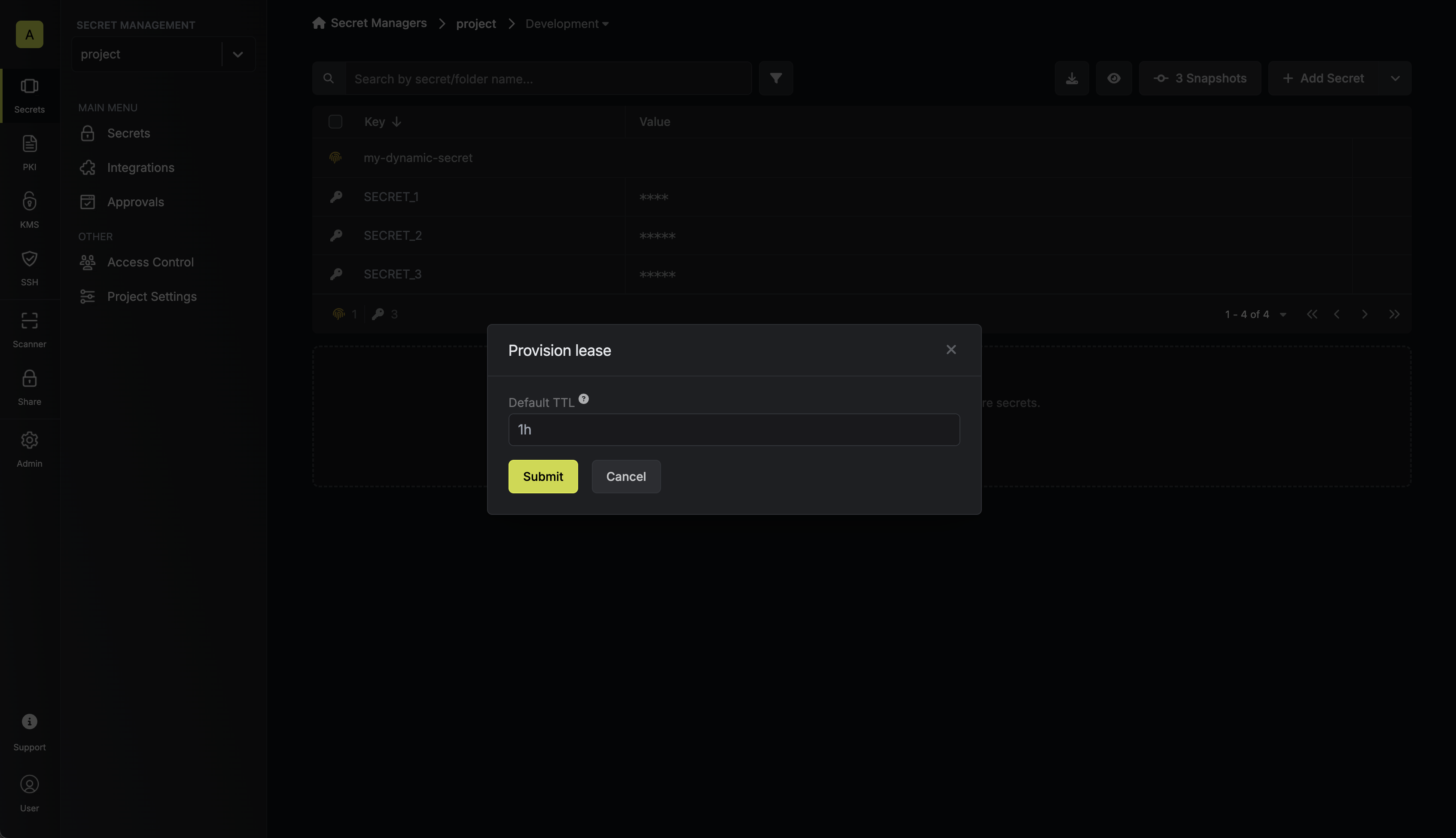
Ensure that the TTL for the lease fall within the maximum TTL defined when configuring the dynamic secret.
Once you click the `Submit` button, a new secret lease will be generated and the credentials for it will be shown to you.
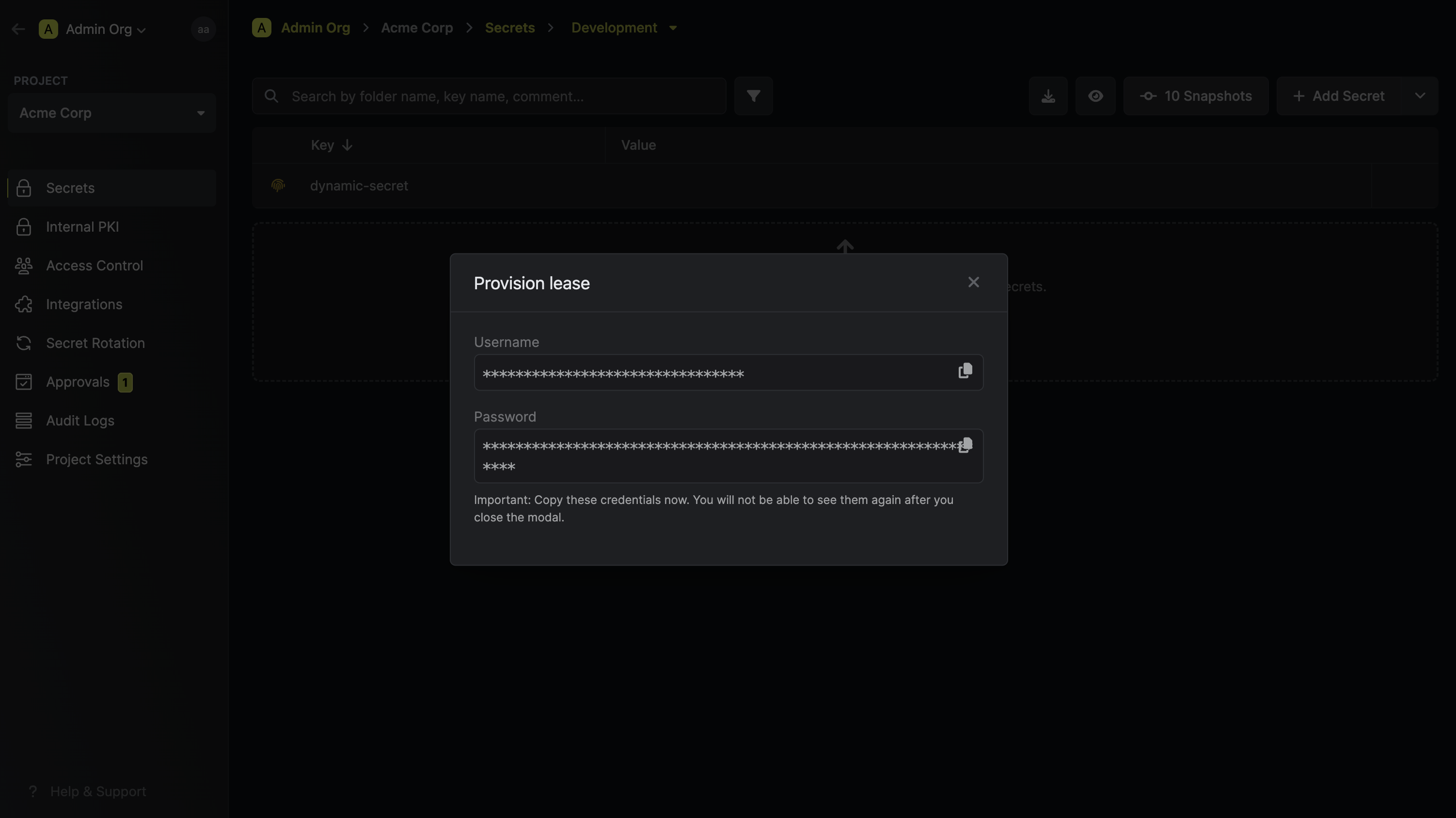
## Audit or Revoke Leases
Once you have created one or more leases, you will be able to access them by clicking on the respective dynamic secret item on the dashboard.
This will allow you see the expiration time of the lease or delete the lease before it's set time to live.
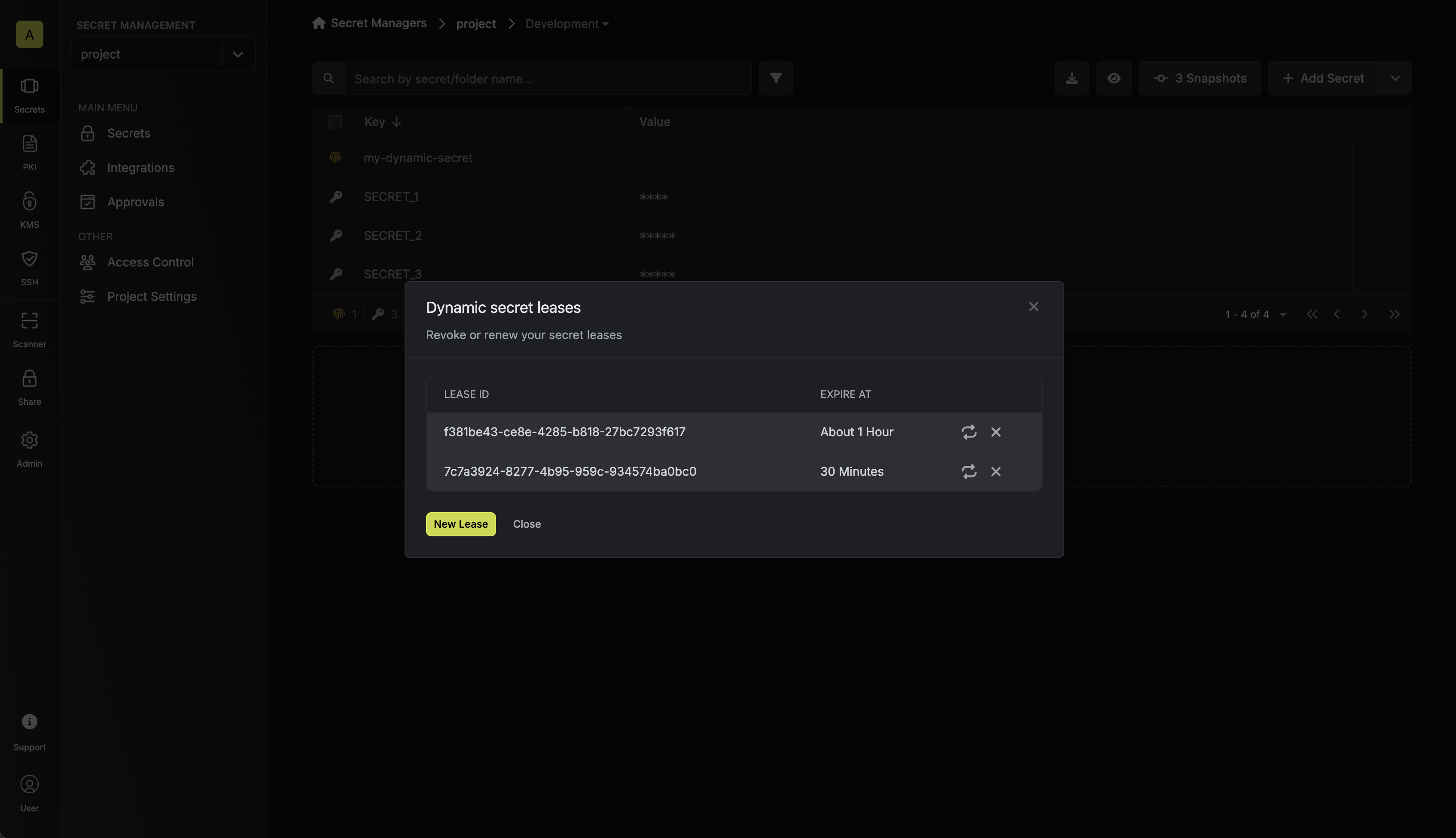
## Renew Leases
To extend the life of the generated dynamic secret leases past its initial time to live, simply click on the **Renew** as illustrated below.
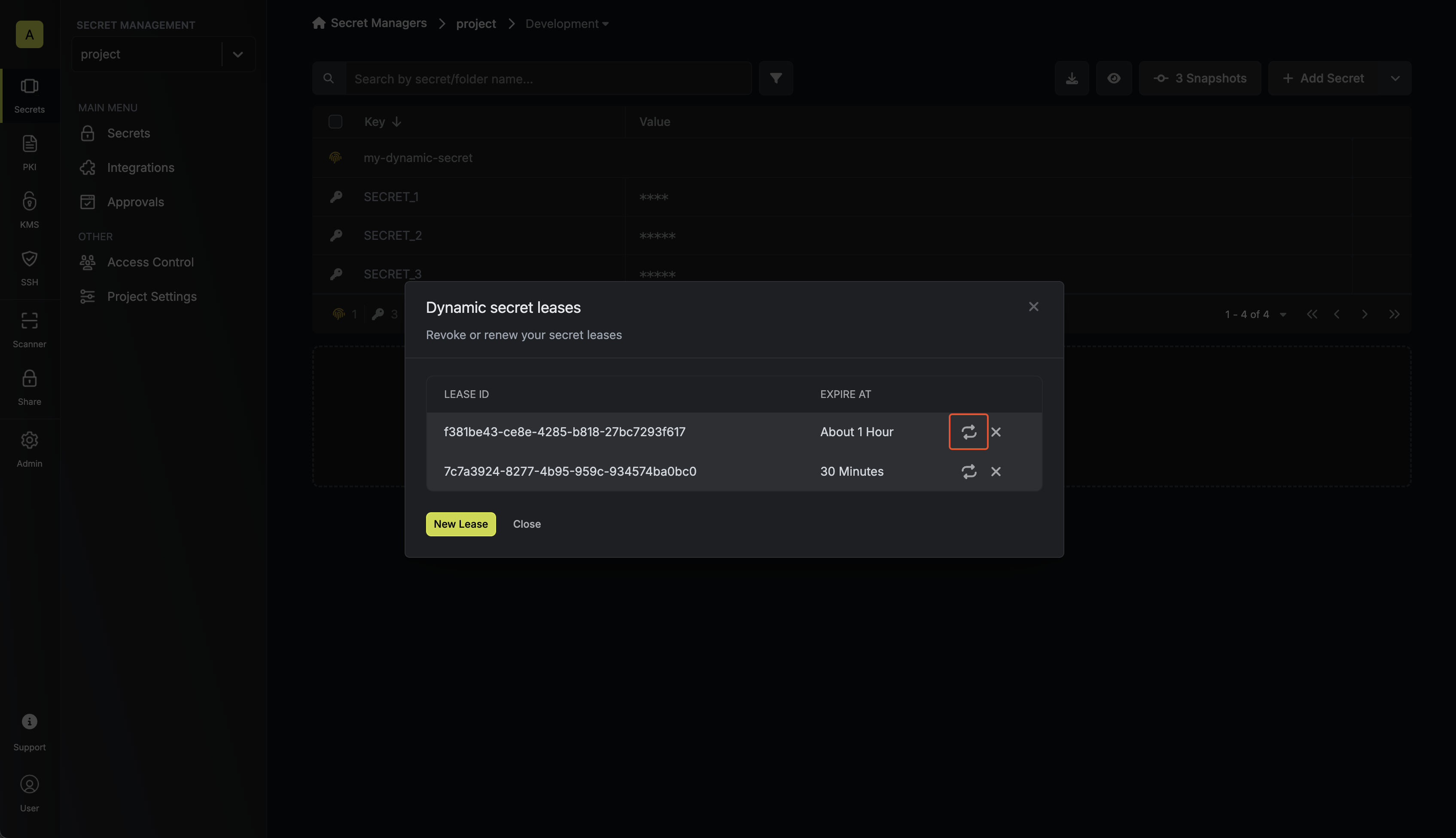
Lease renewals cannot exceed the maximum TTL set when configuring the dynamic secret
# RabbitMQ
Learn how to dynamically generate RabbitMQ user credentials.
The Infisical RabbitMQ dynamic secret allows you to generate RabbitMQ credentials on demand based on configured role.
## Prerequisites
1. Ensure that the `management` plugin is enabled on your RabbitMQ instance. This is required for the dynamic secret to work.
## Set up Dynamic Secrets with RabbitMQ
Open the Secret Overview dashboard and select the environment in which you would like to add a dynamic secret.
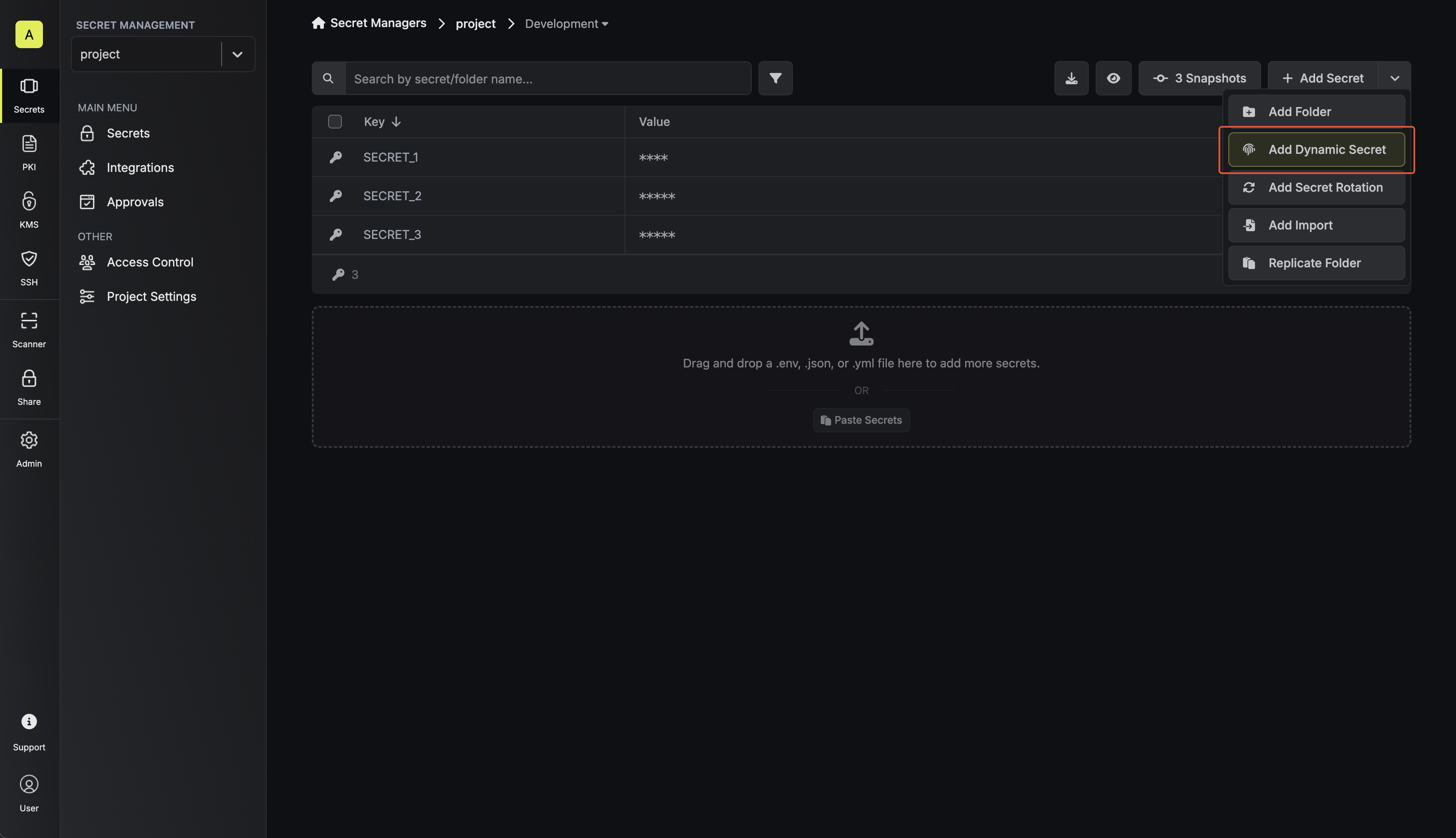

Name by which you want the secret to be referenced
Default time-to-live for a generated secret (it is possible to modify this value when a secret is generate)
Maximum time-to-live for a generated secret.
Your RabbitMQ host. This must be in HTTP format. *(Example: [http://your-cluster-ip](http://your-cluster-ip))*
The port that the RabbitMQ management plugin is listening on. This is `15672` by default.
The name of the virtual host that the user will be assigned to. This defaults to `/`.
The permissions that the user will have on the virtual host. This defaults to `.*`.
The three permission fields all take a regular expression *(regex)*, that should match resource names for which the user is granted read / write / configuration permissions
The username of the user that will be used to provision new dynamic secret leases.
The password of the user that will be used to provision new dynamic secret leases.
A CA may be required if your DB requires it for incoming connections. This is often the case when connecting to a managed service.
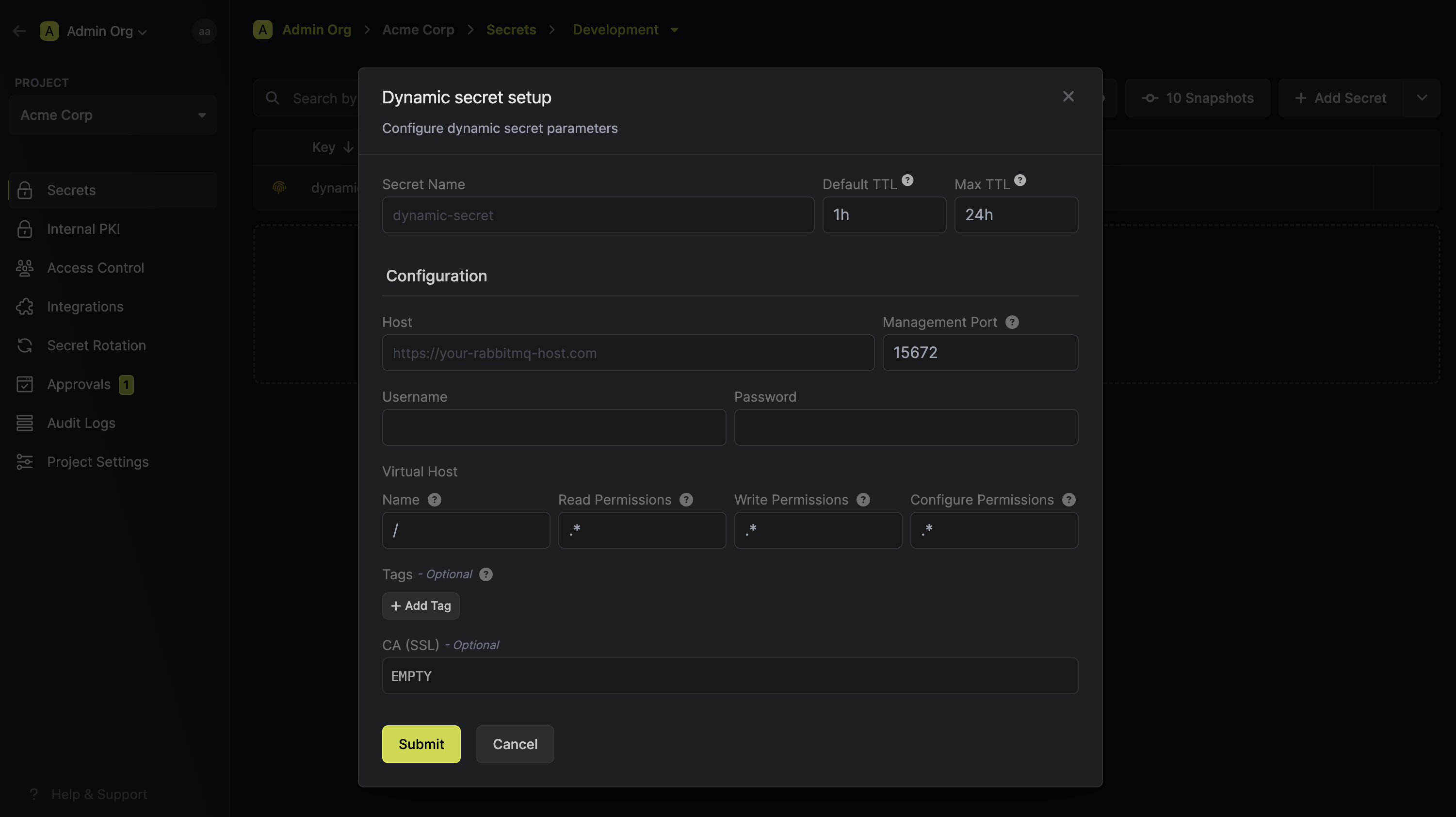
After submitting the form, you will see a dynamic secret created in the dashboard.
If this step fails, you may have to add the CA certificate.
Once you've successfully configured the dynamic secret, you're ready to generate on-demand credentials.
To do this, simply click on the 'Generate' button which appears when hovering over the dynamic secret item.
Alternatively, you can initiate the creation of a new lease by selecting 'New Lease' from the dynamic secret lease list section.


When generating these secrets, it's important to specify a Time-to-Live (TTL) duration. This will dictate how long the credentials are valid for.
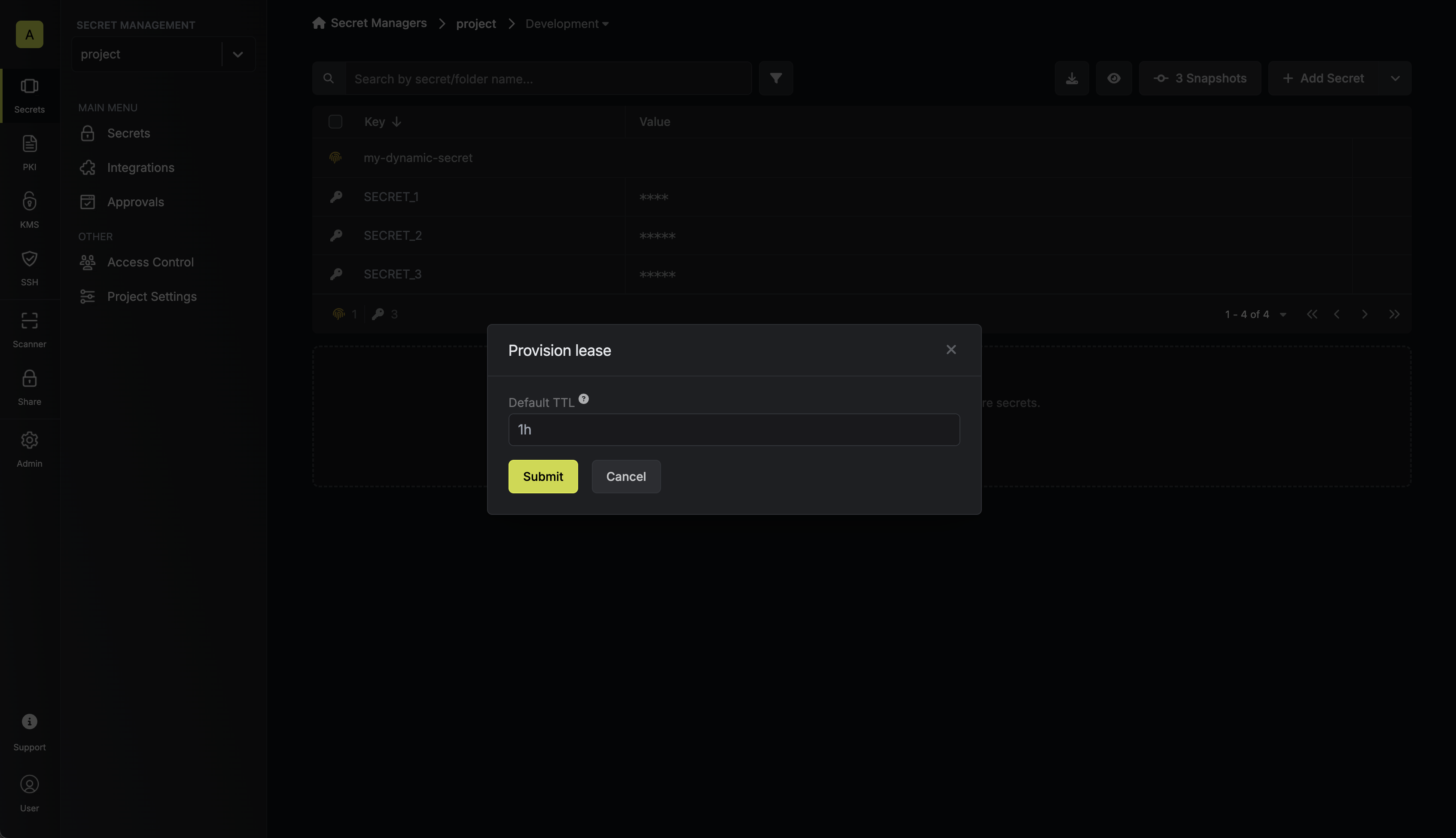
Ensure that the TTL for the lease fall within the maximum TTL defined when configuring the dynamic secret.
Once you click the `Submit` button, a new secret lease will be generated and the credentials from it will be shown to you.
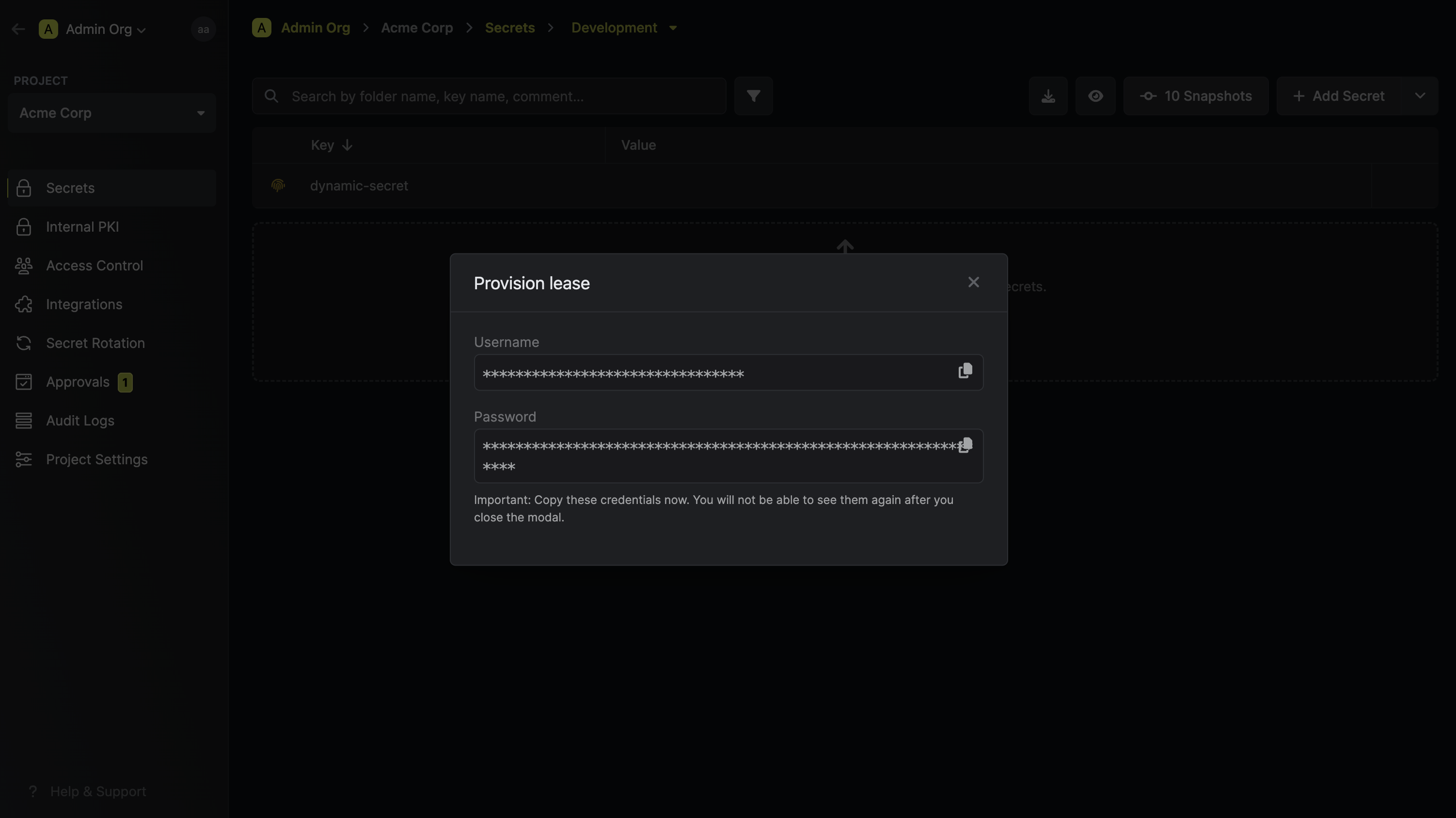
## Audit or Revoke Leases
Once you have created one or more leases, you will be able to access them by clicking on the respective dynamic secret item on the dashboard.
This will allow you see the expiration time of the lease or delete a lease before it's set time to live.
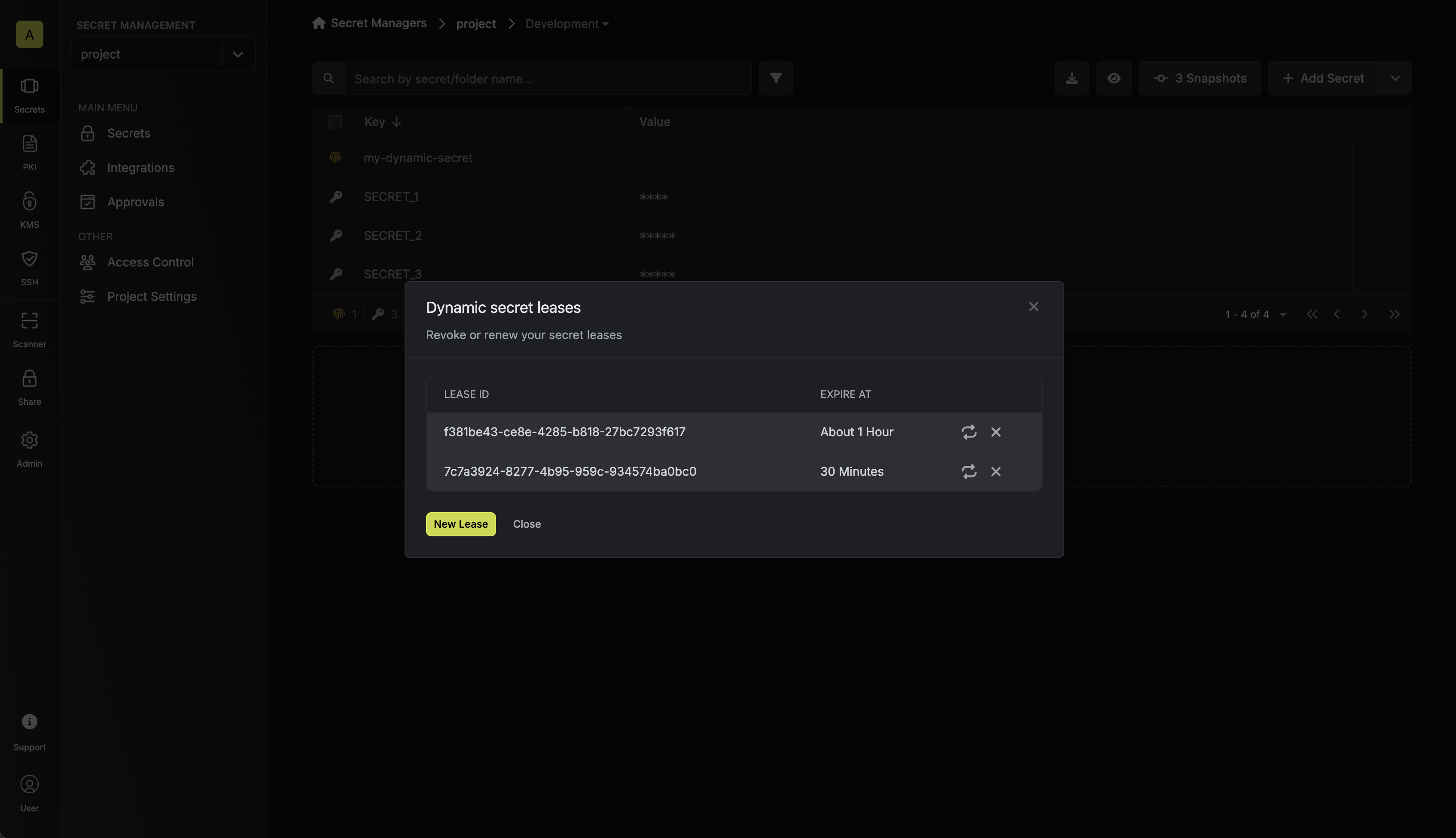
## Renew Leases
To extend the life of the generated dynamic secret leases past its initial time to live, simply click on the **Renew** as illustrated below.
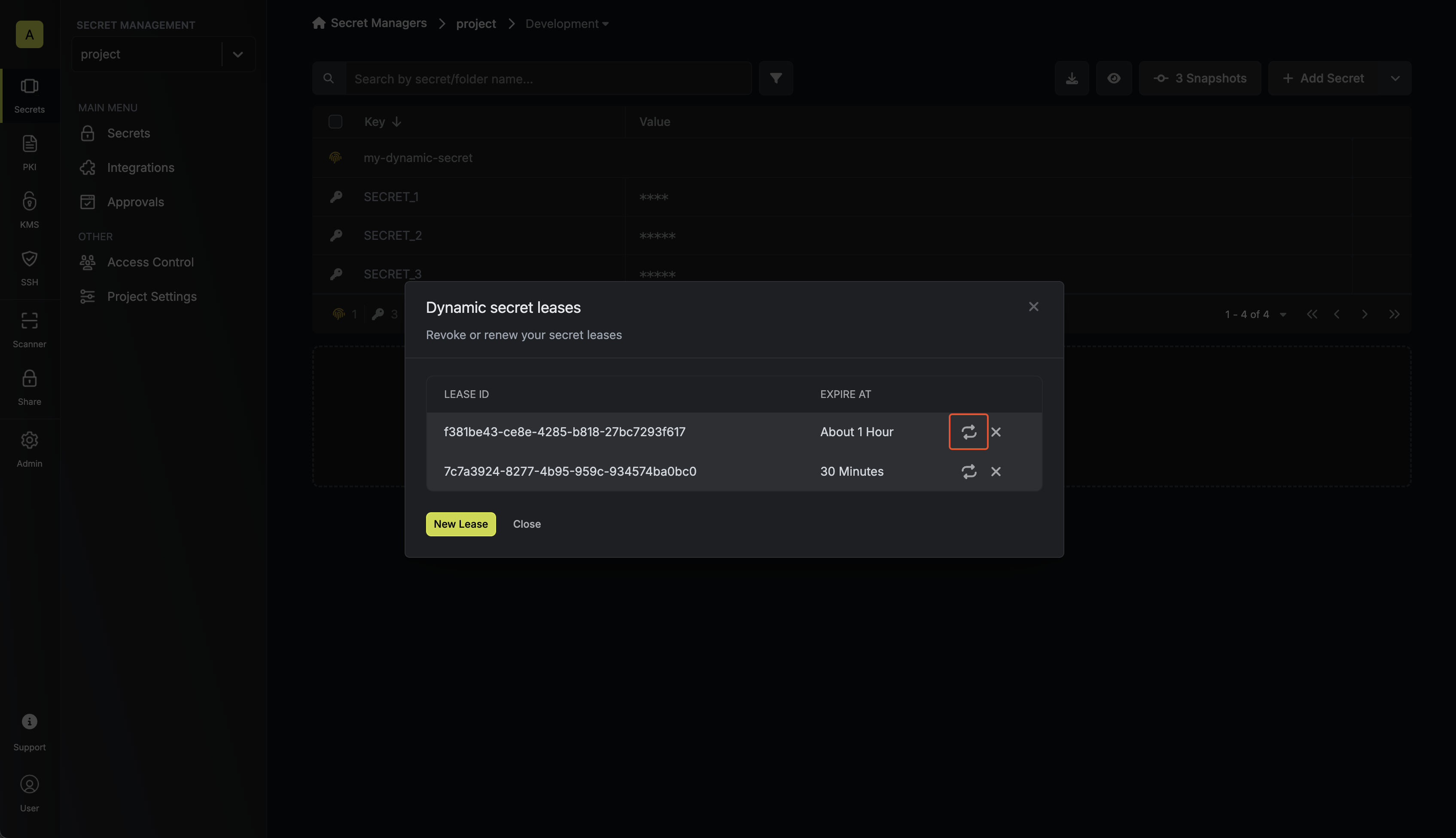
Lease renewals cannot exceed the maximum TTL set when configuring the dynamic secret
# Redis
Learn how to dynamically generate Redis Database user credentials.
The Infisical Redis dynamic secret allows you to generate Redis Database credentials on demand based on configured role.
## Prerequisite
Create a user with the required permission in your Redis instance. This user will be used to create new accounts on-demand.
## Set up Dynamic Secrets with Redis
Open the Secret Overview dashboard and select the environment in which you would like to add a dynamic secret.
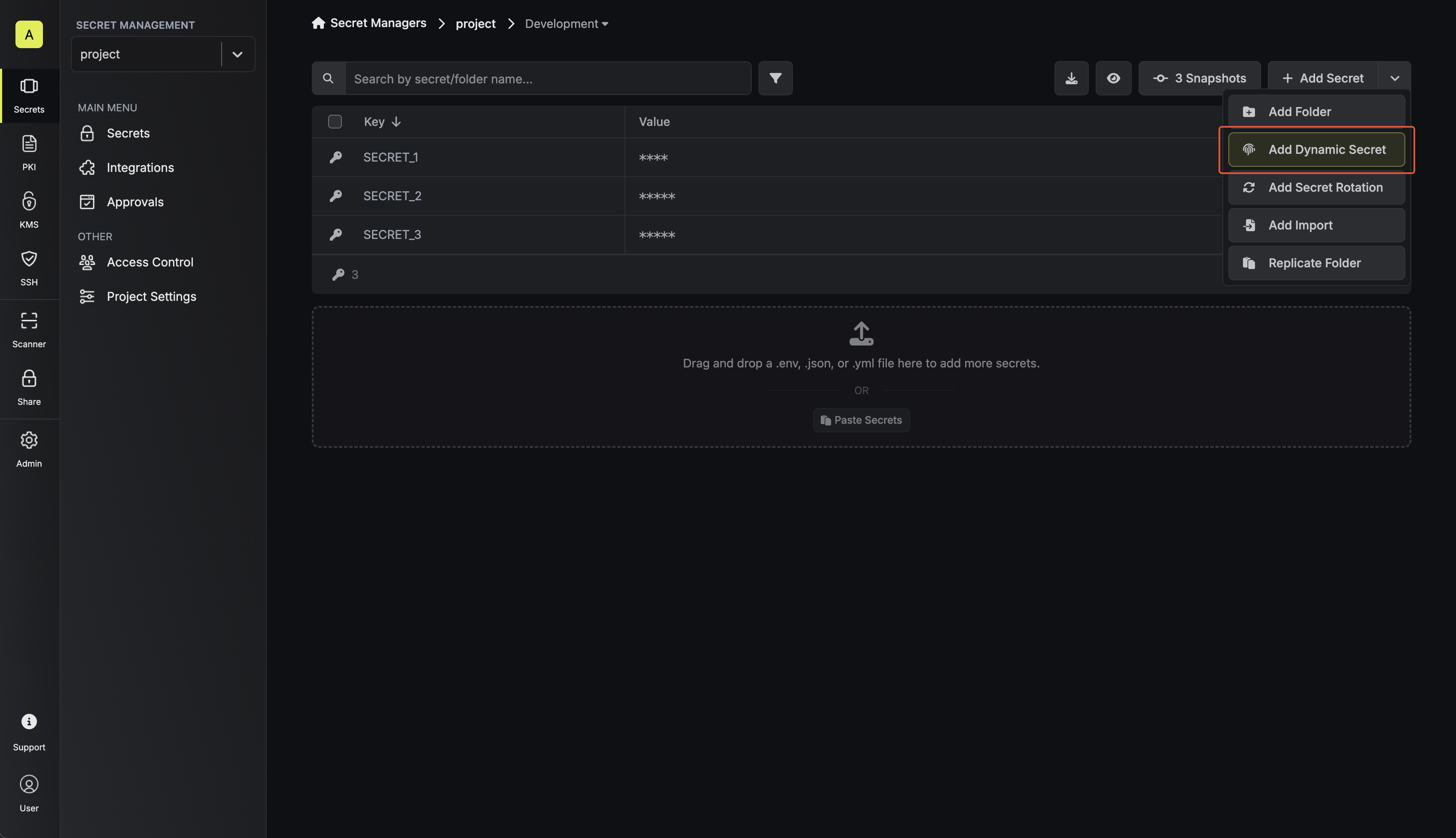
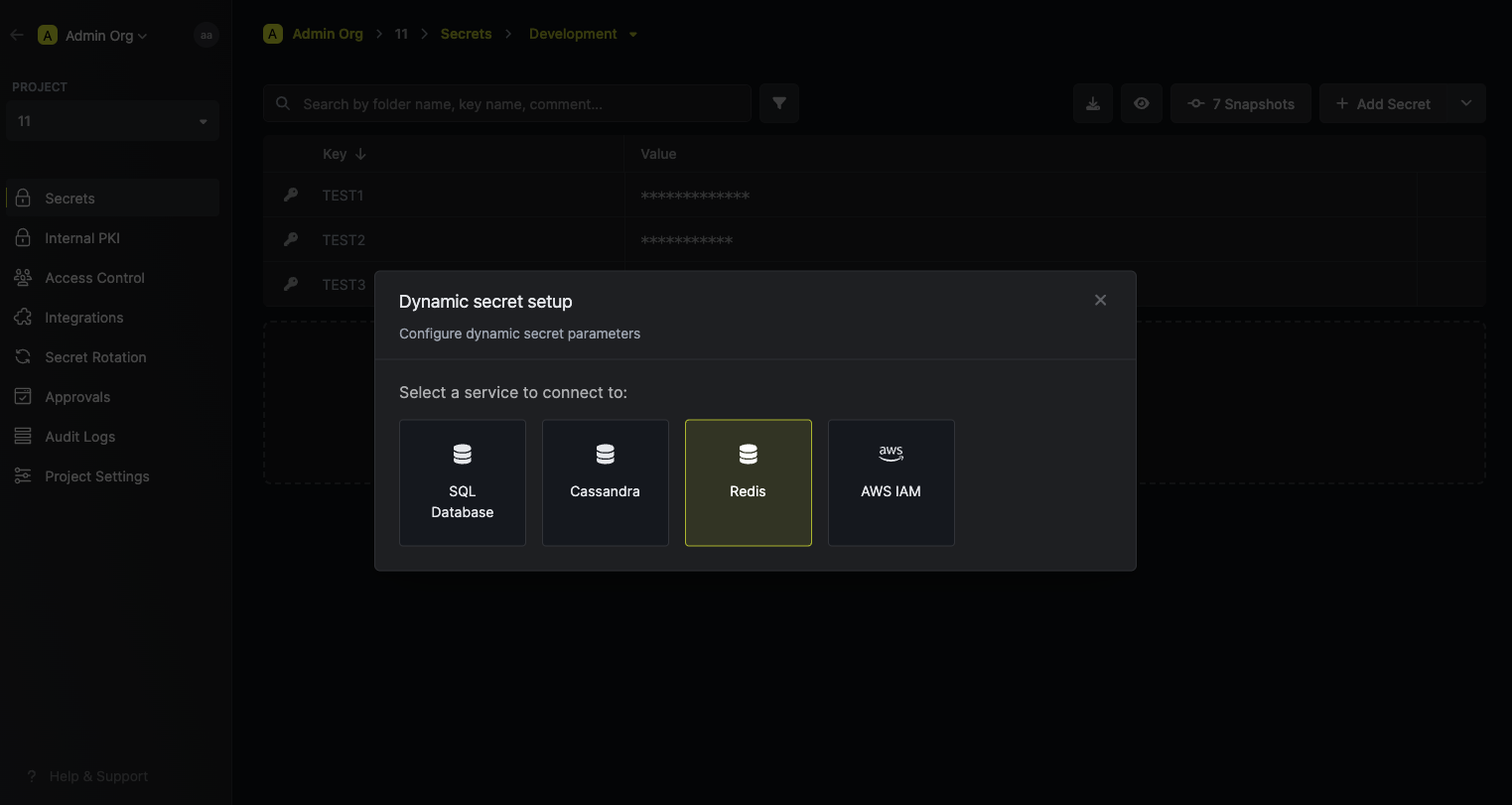
Name by which you want the secret to be referenced
Default time-to-live for a generated secret (it is possible to modify this value when a secret is generate)
Maximum time-to-live for a generated secret.
The database host, this can be an IP address or a domain name as long as Infisical can reach it.
The database port, this is the port that the Redis instance is listening on.
Redis username that will be used to create new users on-demand. This is often 'default' or 'admin'.
Password that will be used to create dynamic secrets. This is required if your Redis instance is password protected.
A CA may be required if your DB requires it for incoming connections. This is often the case when connecting to a managed service.
If you want to provide specific privileges for the generated dynamic credentials, you can modify the Redis statement to your needs. This is useful if you want to only give access to a specific table(s).
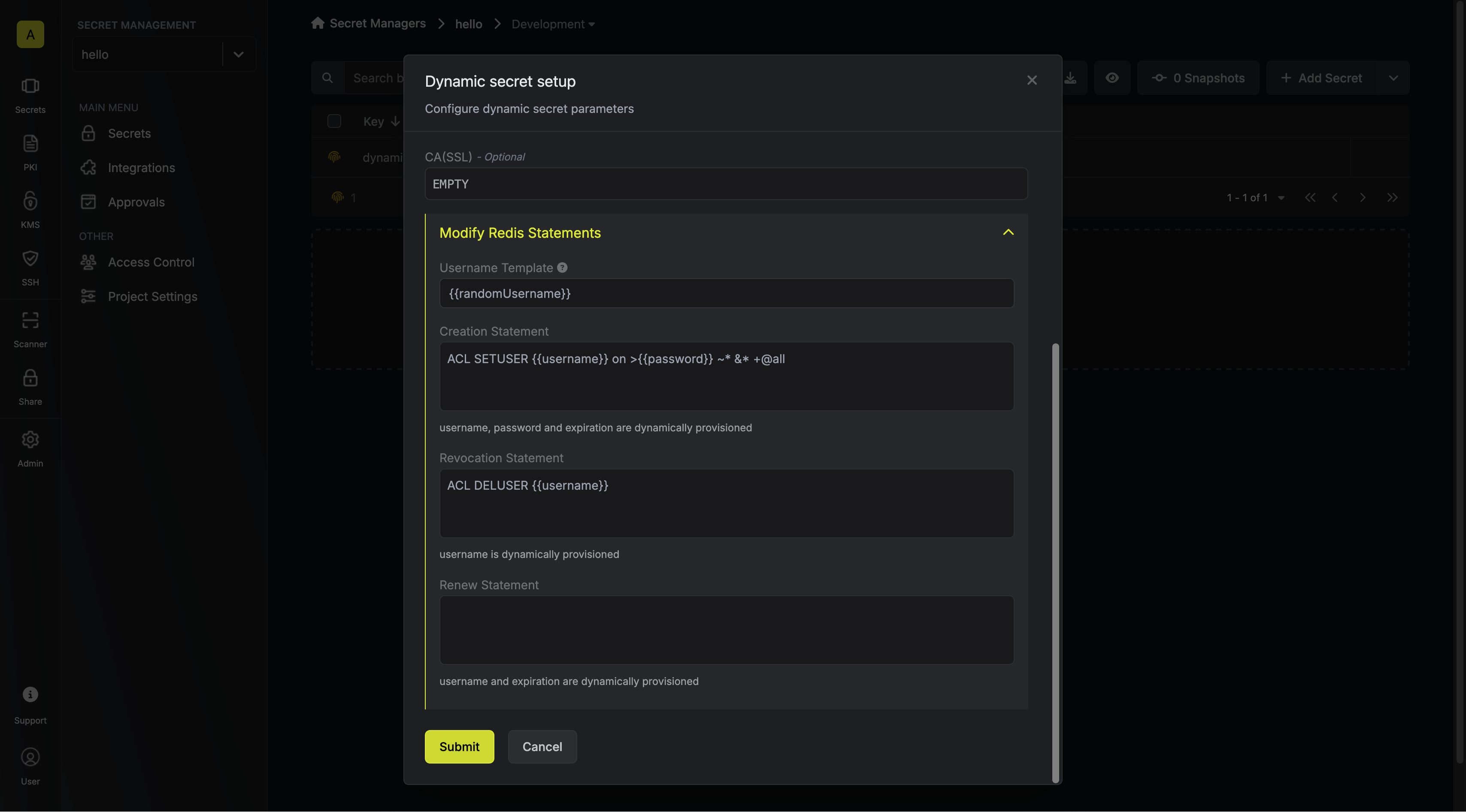
After submitting the form, you will see a dynamic secret created in the dashboard.
If this step fails, you may have to add the CA certificate.
Once you've successfully configured the dynamic secret, you're ready to generate on-demand credentials.
To do this, simply click on the 'Generate' button which appears when hovering over the dynamic secret item.
Alternatively, you can initiate the creation of a new lease by selecting 'New Lease' from the dynamic secret lease list section.


When generating these secrets, it's important to specify a Time-to-Live (TTL) duration. This will dictate how long the credentials are valid for.
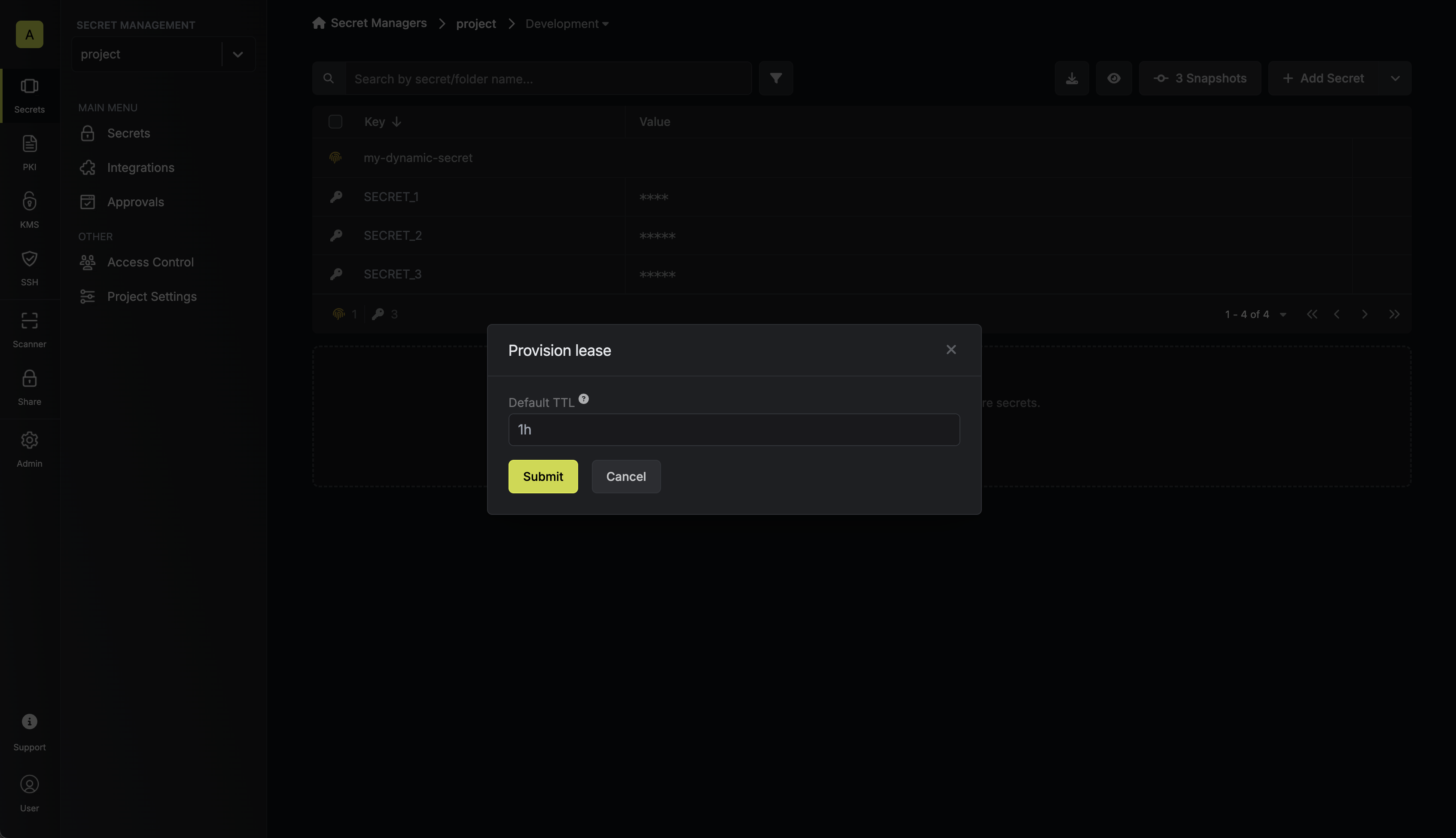
Ensure that the TTL for the lease fall within the maximum TTL defined when configuring the dynamic secret.
Once you click the `Submit` button, a new secret lease will be generated and the credentials from it will be shown to you.
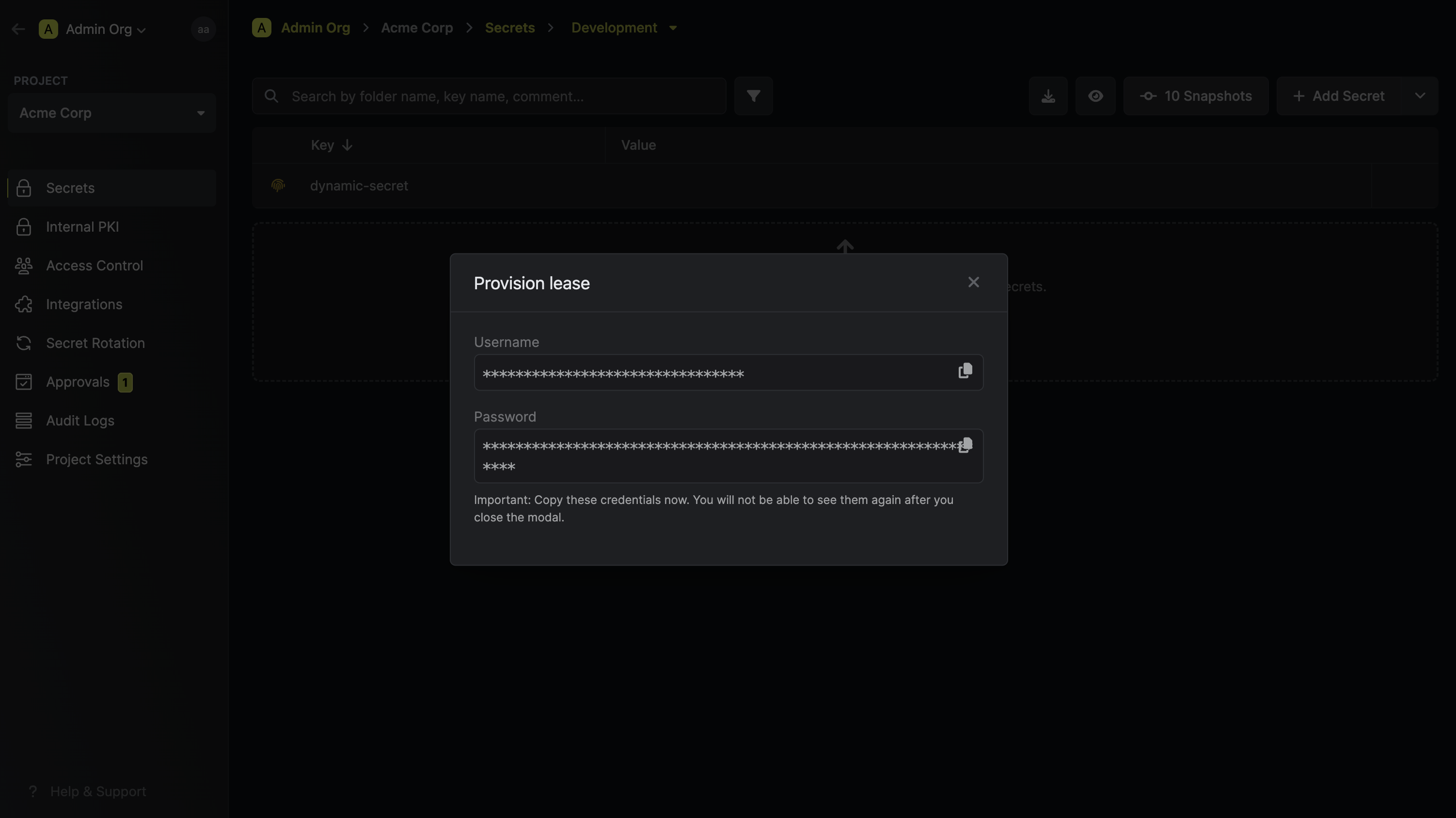
## Audit or Revoke Leases
Once you have created one or more leases, you will be able to access them by clicking on the respective dynamic secret item on the dashboard.
This will allow you see the expiration time of the lease or delete a lease before it's set time to live.
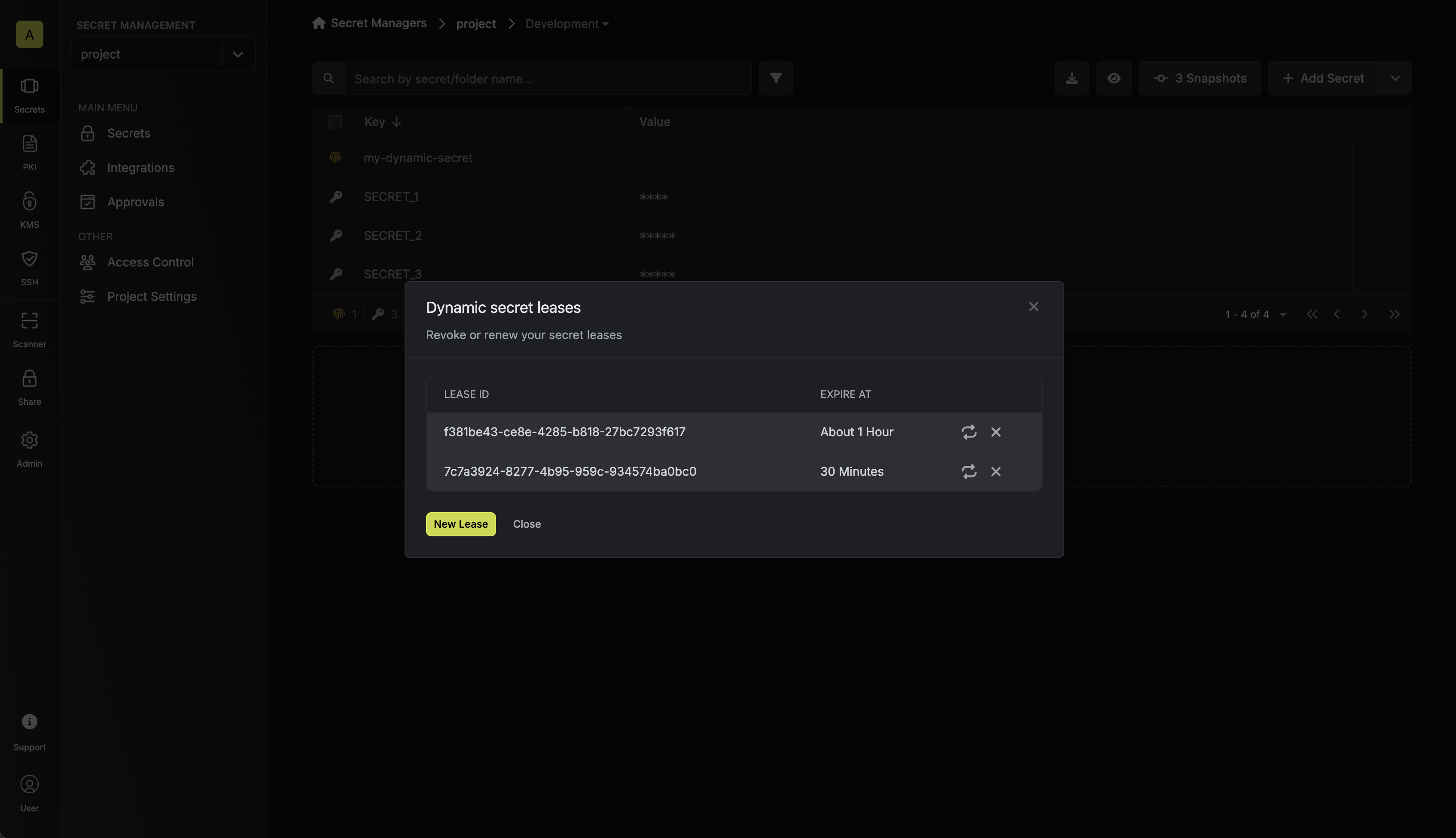
## Renew Leases
To extend the life of the generated dynamic secret leases past its initial time to live, simply click on the **Renew** as illustrated below.
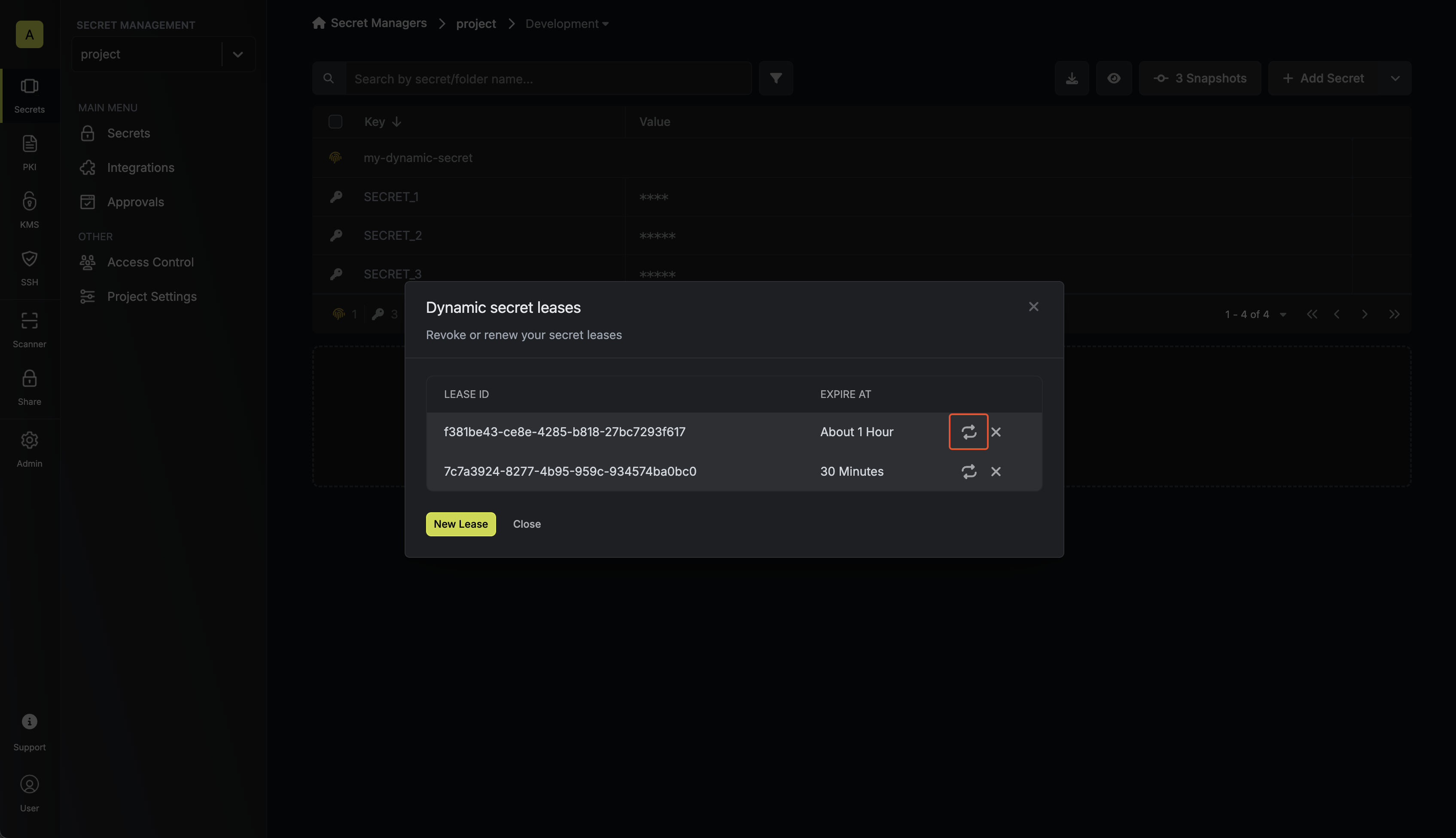
Lease renewals cannot exceed the maximum TTL set when configuring the dynamic secret
# SAP HANA
Learn how to dynamically generate SAP HANA database account credentials.
The Infisical SAP HANA dynamic secret allows you to generate SAP HANA database credentials on demand.
## Prerequisite
* Infisical requires a SAP HANA database user in your instance with the necessary permissions. This user will facilitate the creation of new accounts as needed.
Ensure the user possesses privileges for creating, dropping, and granting permissions to roles for it to be able to create dynamic secrets.
* The SAP HANA instance should be reachable by Infisical.
## Set up Dynamic Secrets with SAP HANA
Open the Secret Overview dashboard and select the environment in which you would like to add a dynamic secret.
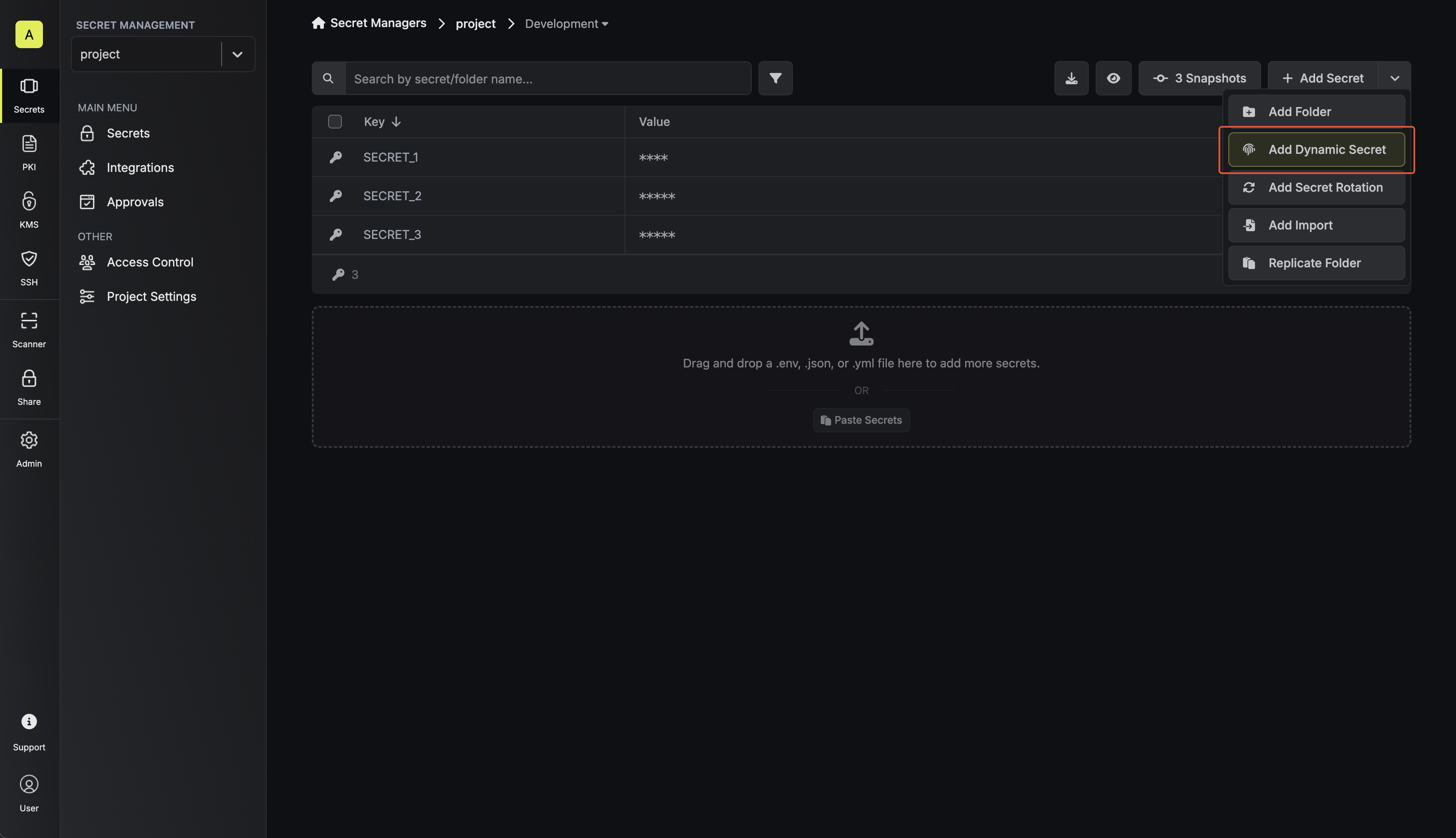
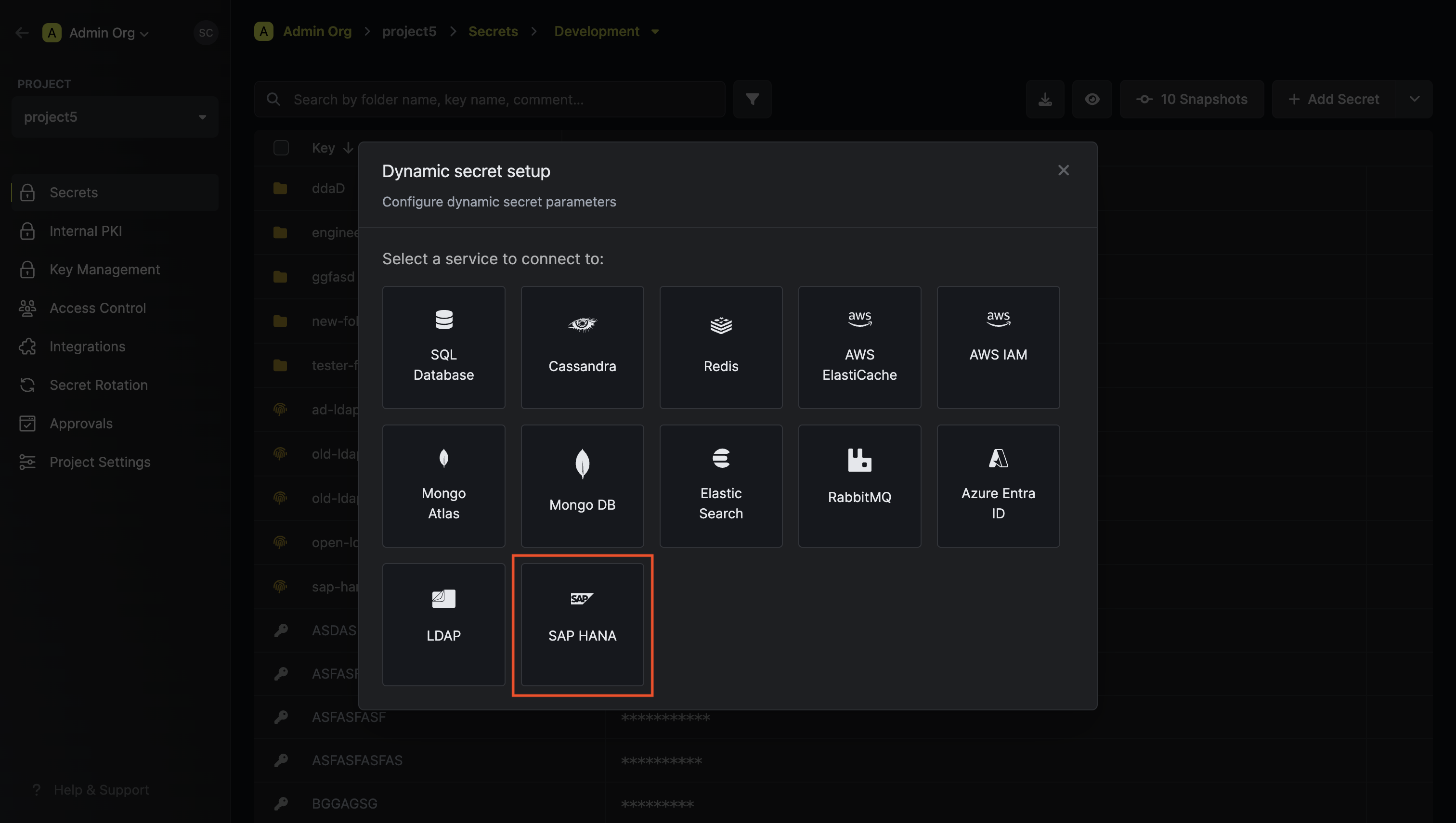
Name by which you want the secret to be referenced
Default time-to-live for a generated secret (it is possible to modify this value when a secret is generate)
Maximum time-to-live for a generated secret
SAP HANA Host
SAP HANA Port
Username that will be used to create dynamic secrets
Password that will be used to create dynamic secrets
A CA may be required for SSL if you are self-hosting SAP HANA
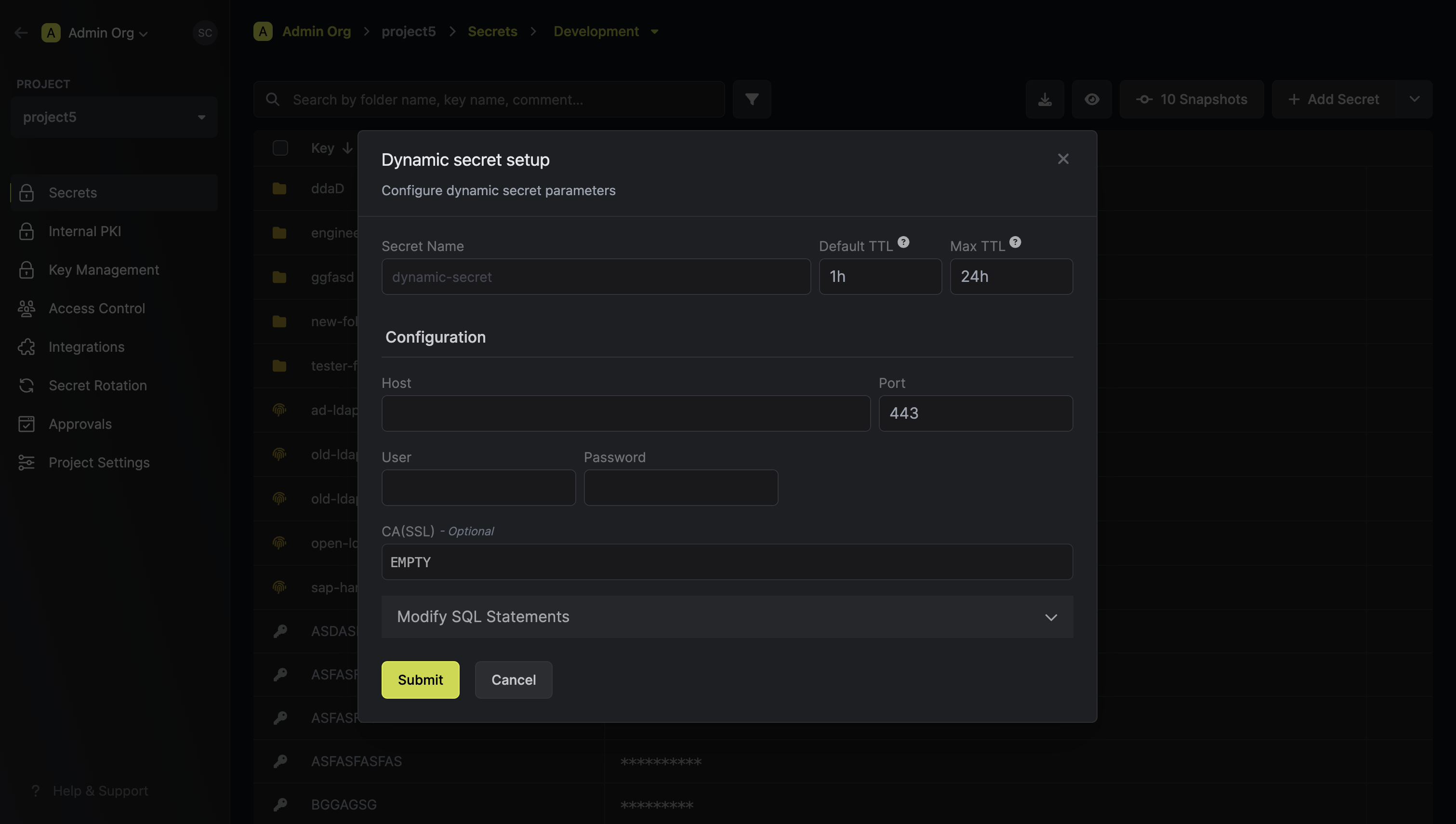
If you want to provide specific privileges for the generated dynamic credentials, you can modify the SQL statement to your needs.
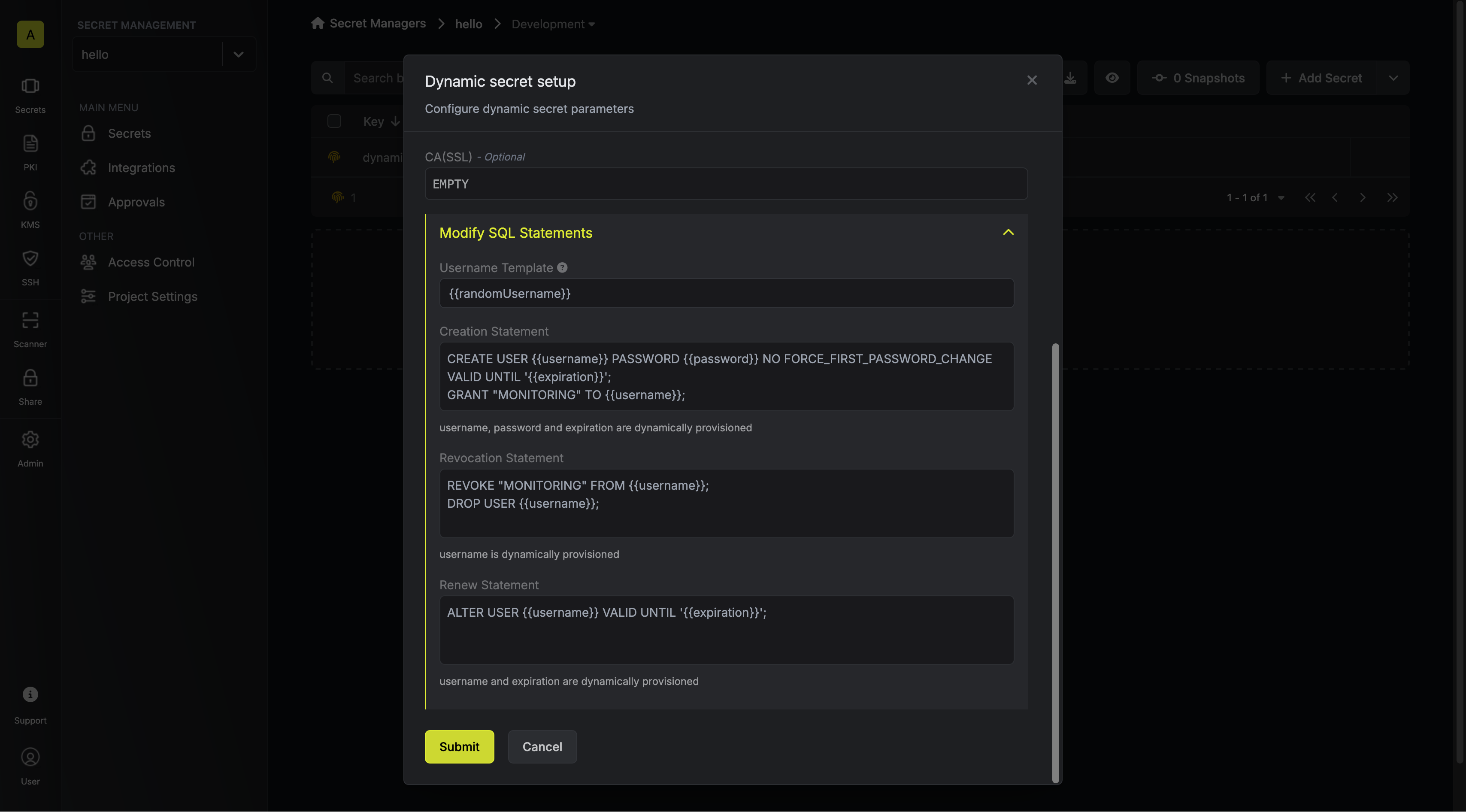
Due to SAP HANA limitations, the attached SQL statements are not executed as a transaction.
After submitting the form, you will see a dynamic secret created in the dashboard.
If this step fails, you may have to add the CA certficate.
Once you've successfully configured the dynamic secret, you're ready to generate on-demand credentials.
To do this, simply click on the 'Generate' button which appears when hovering over the dynamic secret item.
Alternatively, you can initiate the creation of a new lease by selecting 'New Lease' from the dynamic secret lease list section.
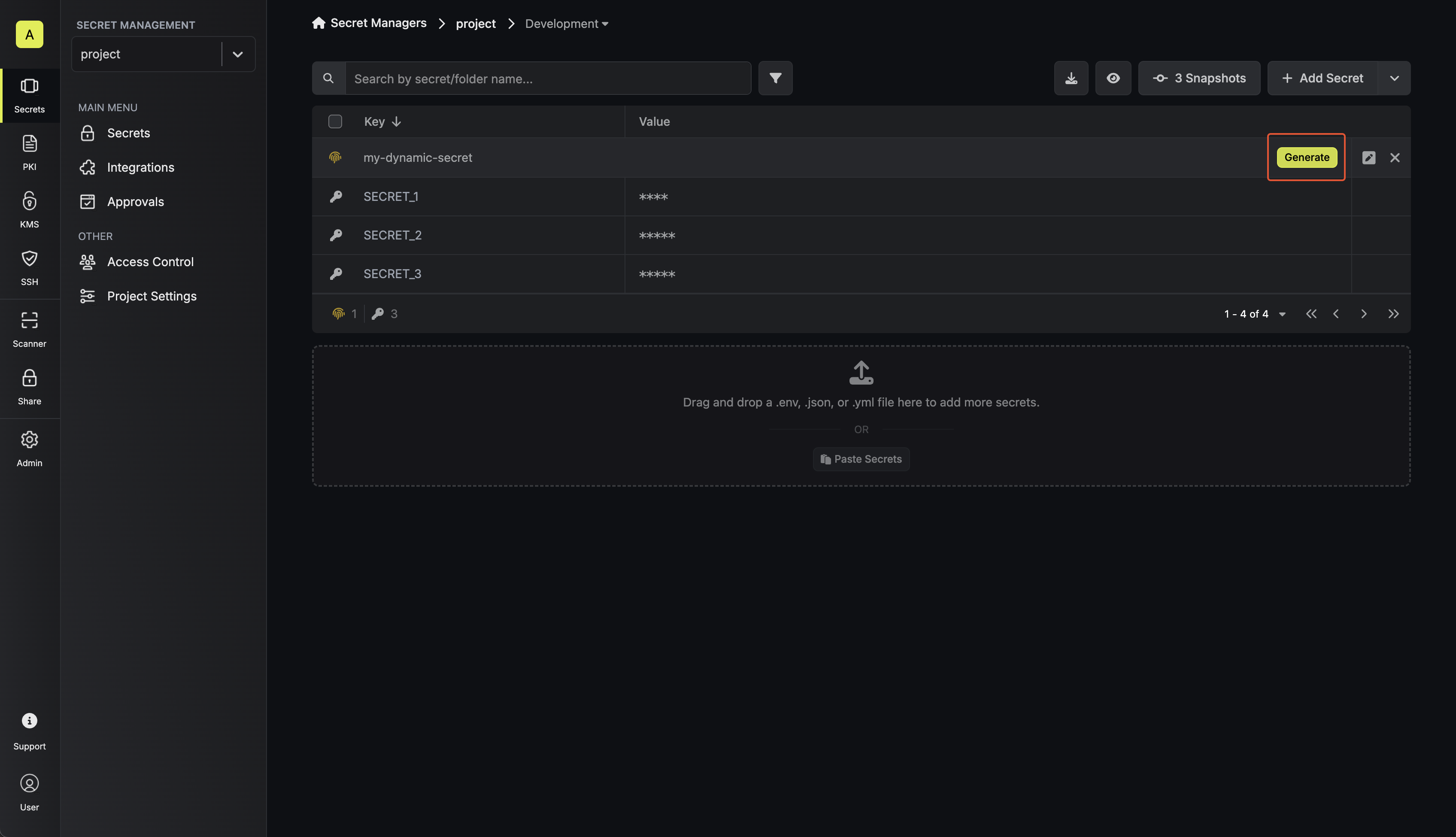
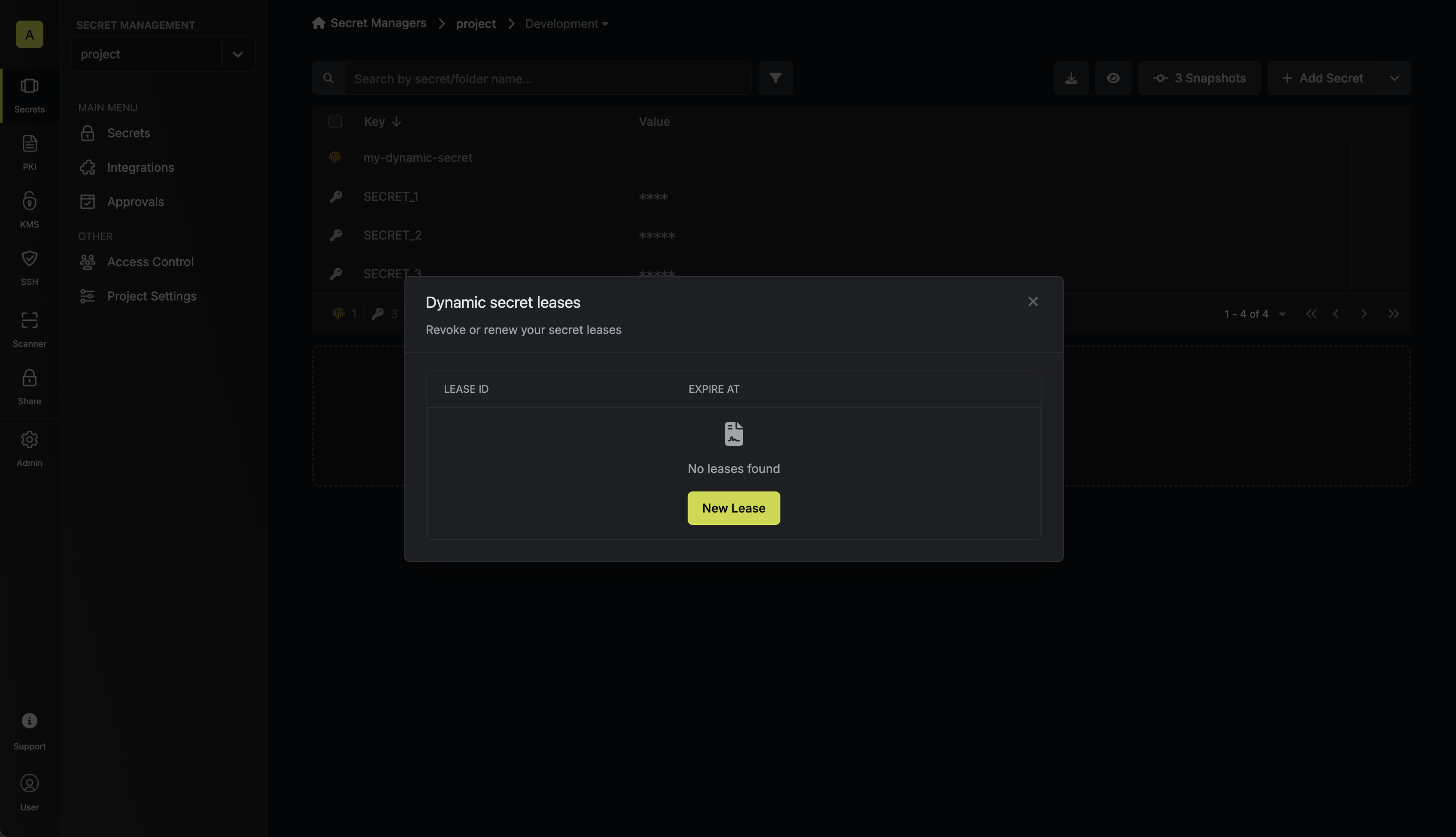
When generating these secrets, it's important to specify a Time-to-Live (TTL) duration. This will dictate how long the credentials are valid for.
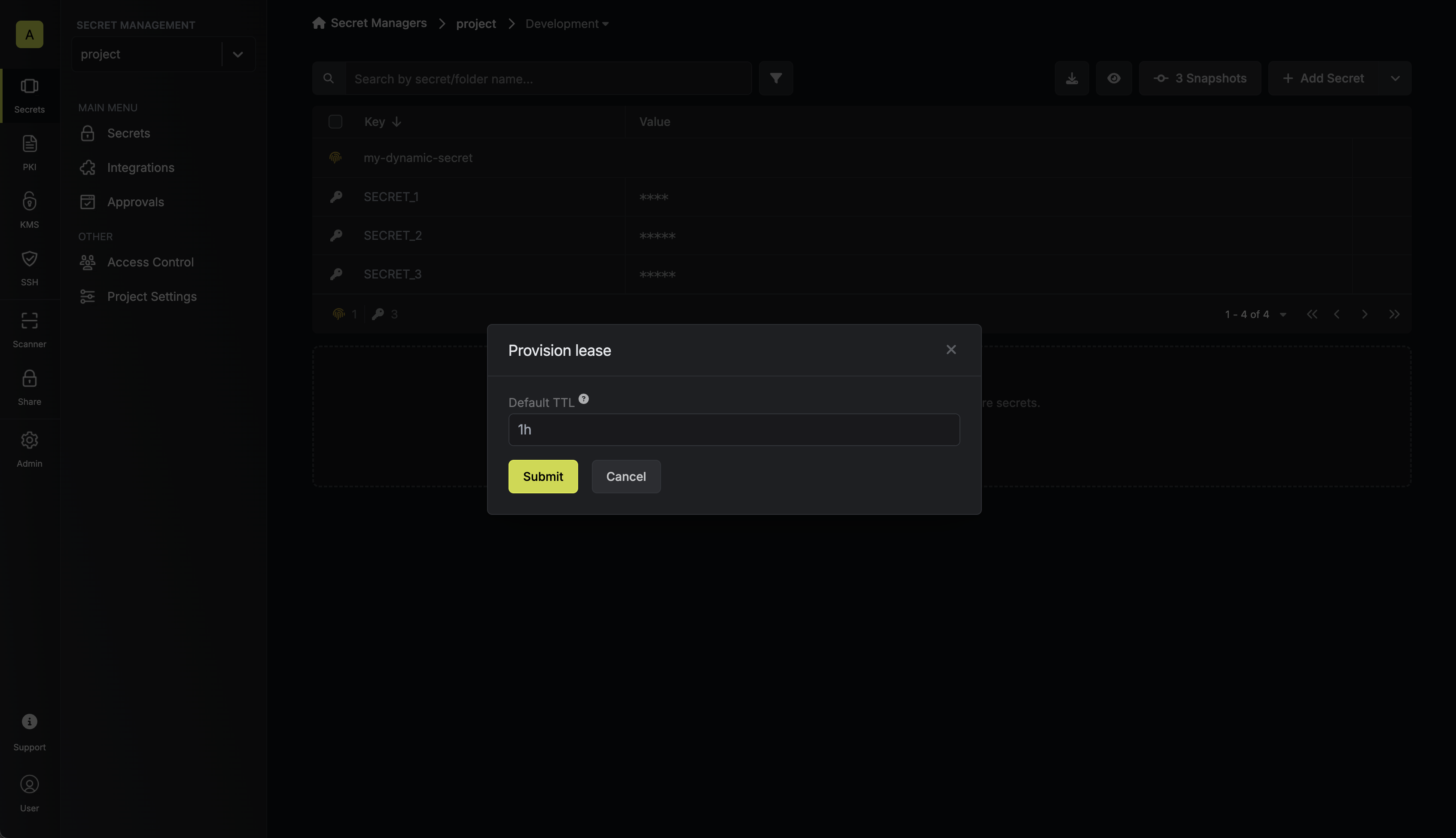
Ensure that the TTL for the lease fall within the maximum TTL defined when configuring the dynamic secret in step 4.
Once you click the `Submit` button, a new secret lease will be generated and the credentials for it will be shown to you.
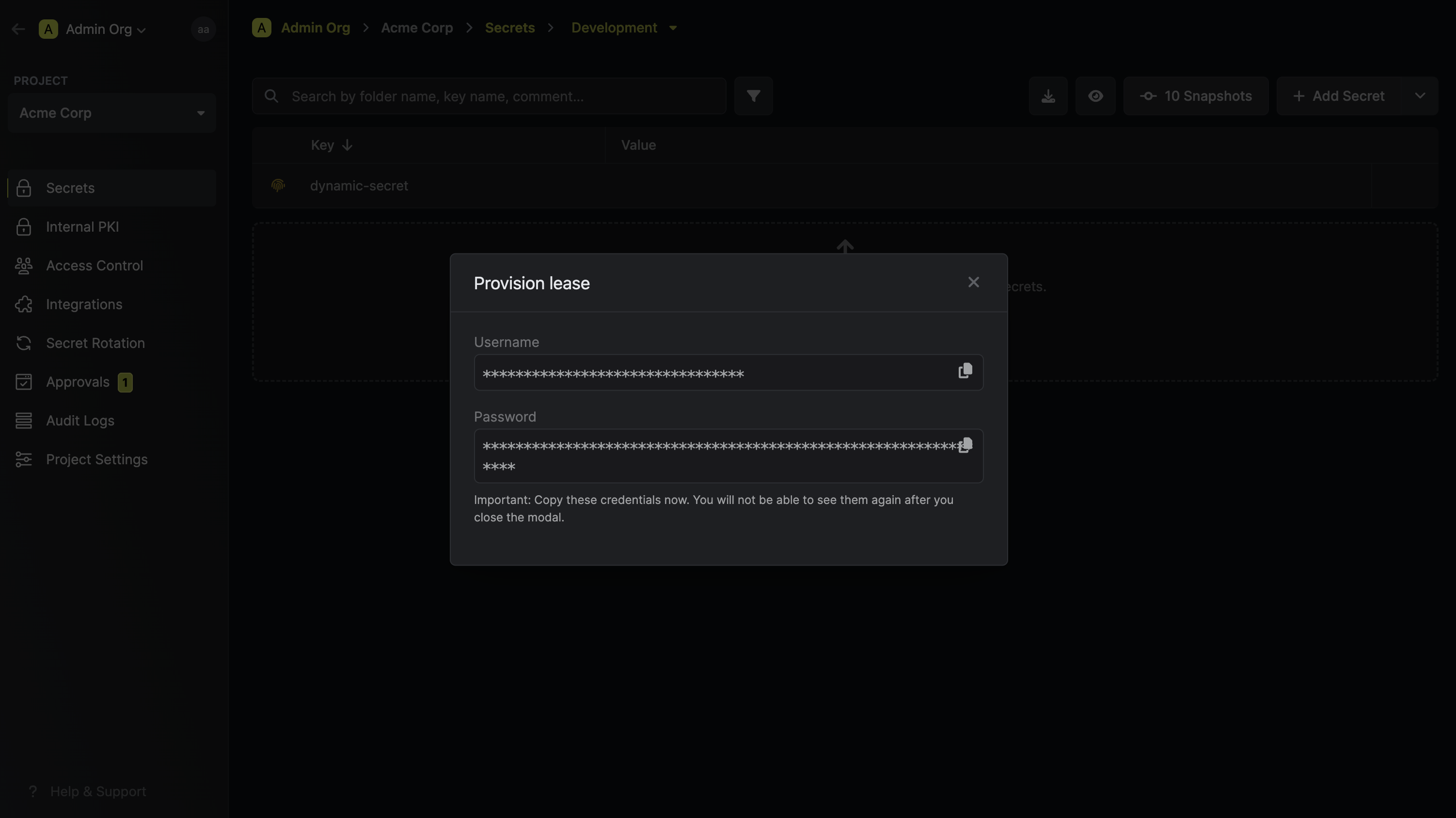
## Audit or Revoke Leases
Once you have created one or more leases, you will be able to access them by clicking on the respective dynamic secret item on the dashboard.
This will allow you see the lease details and delete the lease ahead of its expiration time.
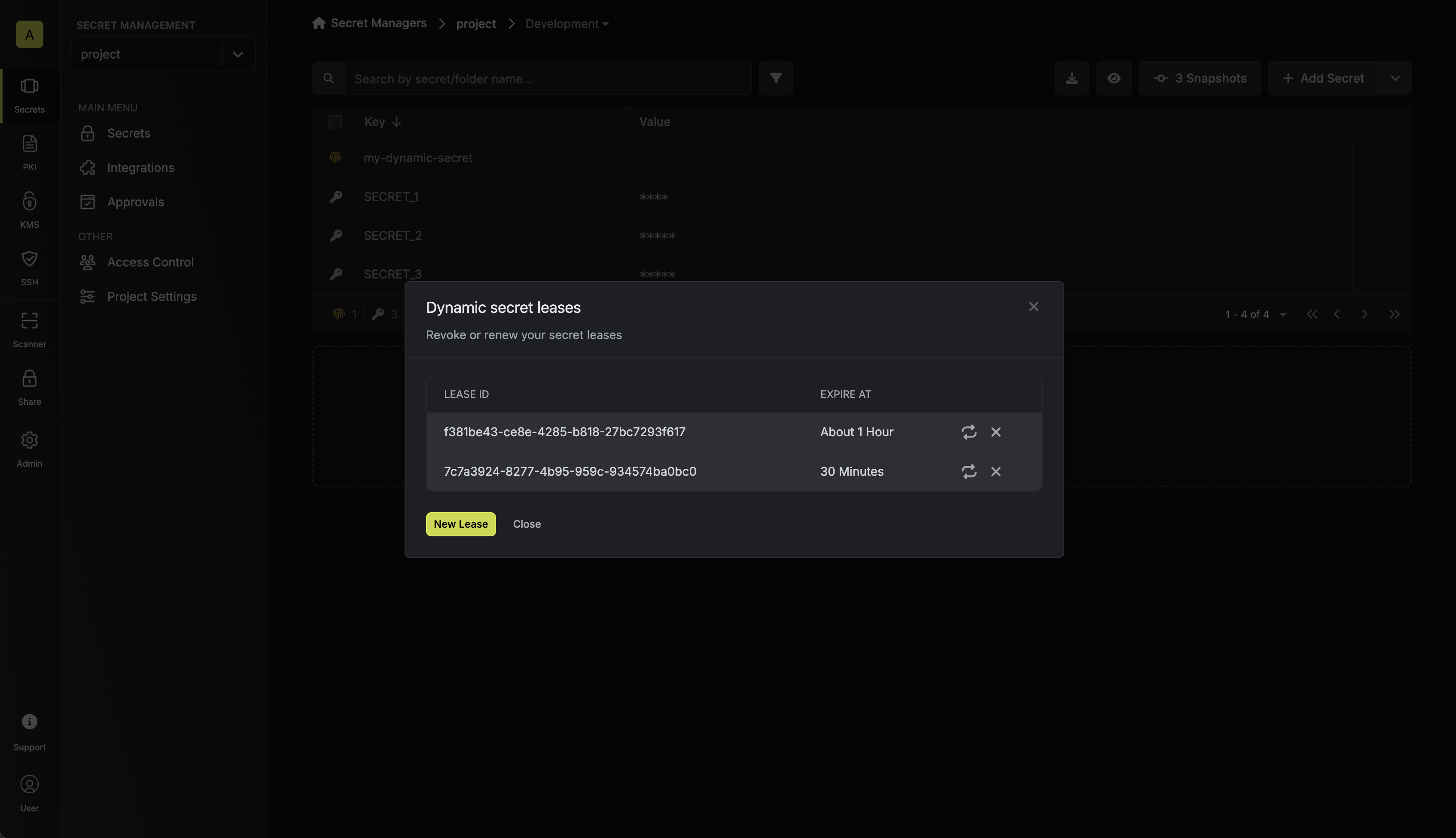
## Renew Leases
To extend the life of the generated dynamic secret lease past its initial time to live, simply click on the **Renew** as illustrated below.
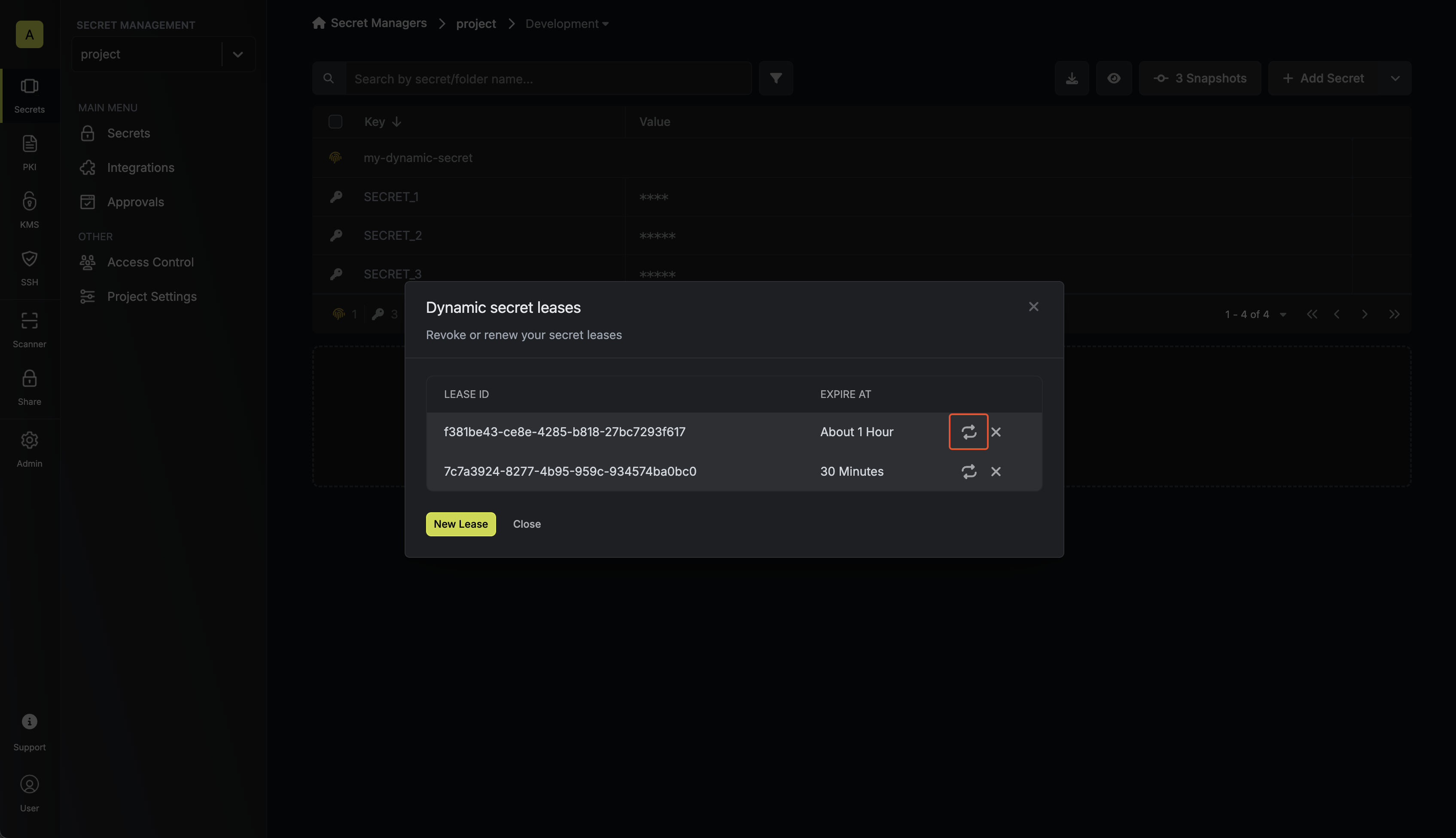
Lease renewals cannot exceed the maximum TTL set when configuring the dynamic
secret.
# Snowflake
Learn how to dynamically generate Snowflake user credentials.
Infisical's Snowflake dynamic secrets allow you to generate Snowflake user credentials on demand.
## Snowflake Prerequisites
Infisical requires a Snowflake user in your account with the USERADMIN role. This user will act as a service account for Infisical and facilitate the creation of new users as needed.
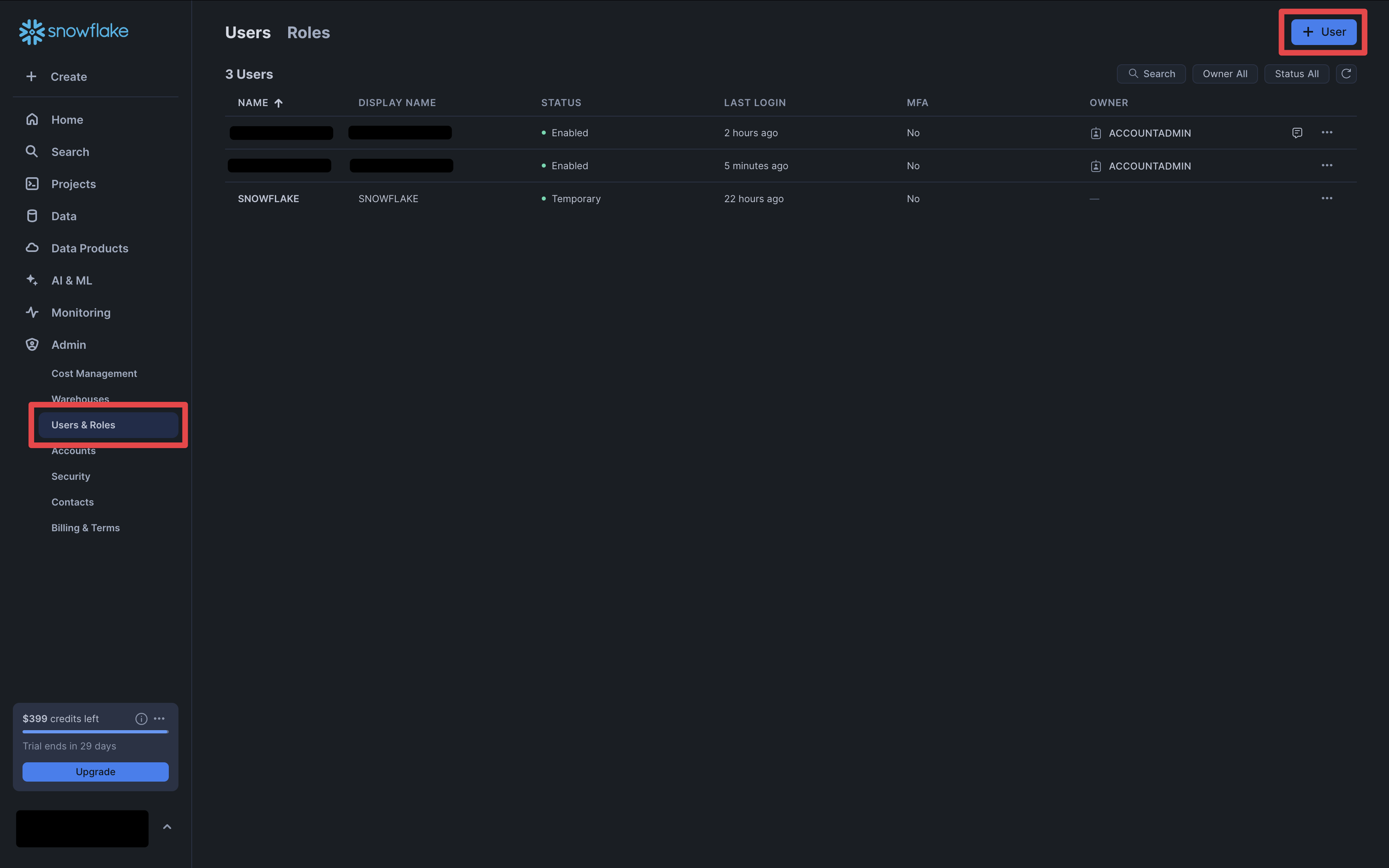
Be sure to uncheck "Force user to change password on first time login"
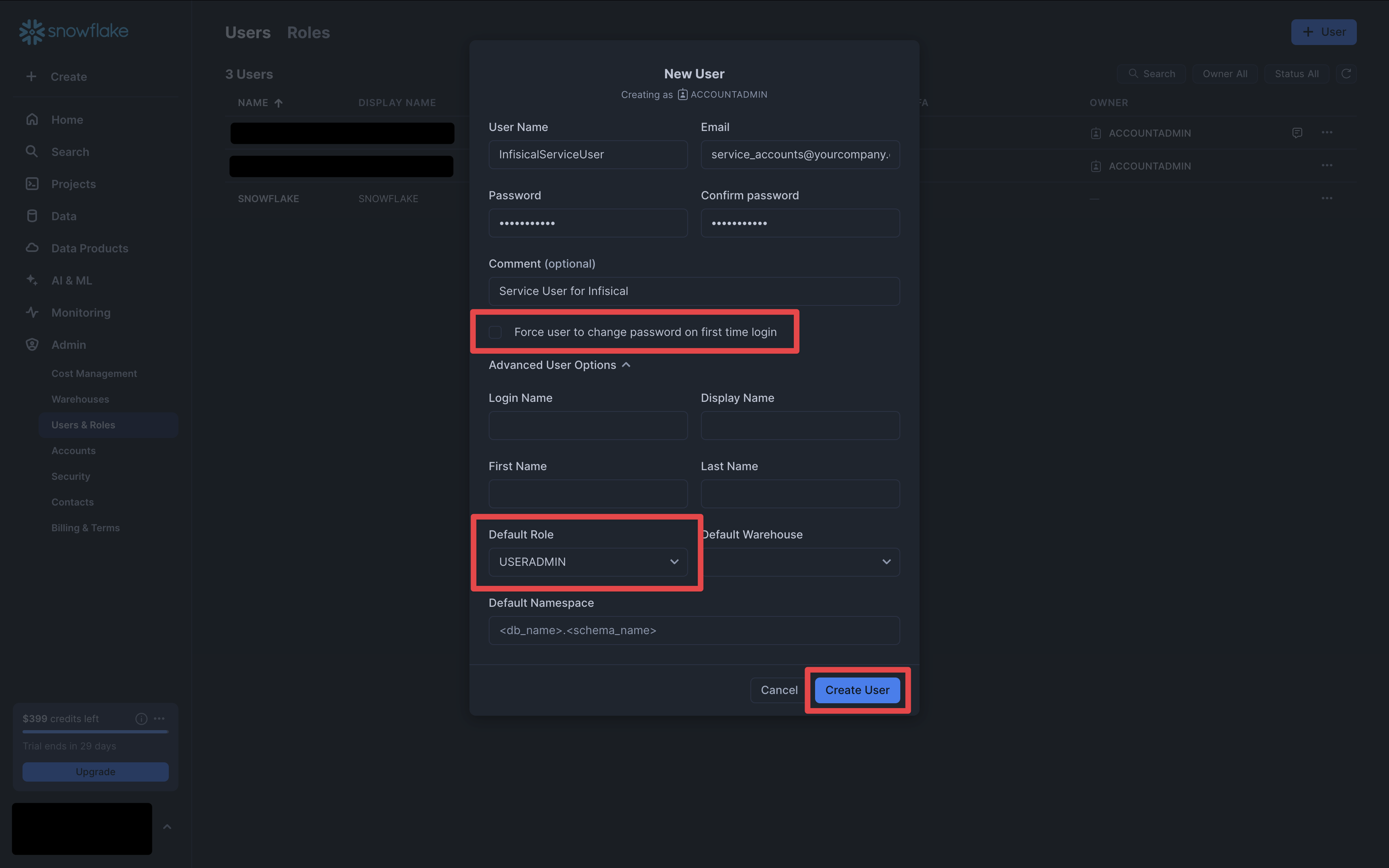
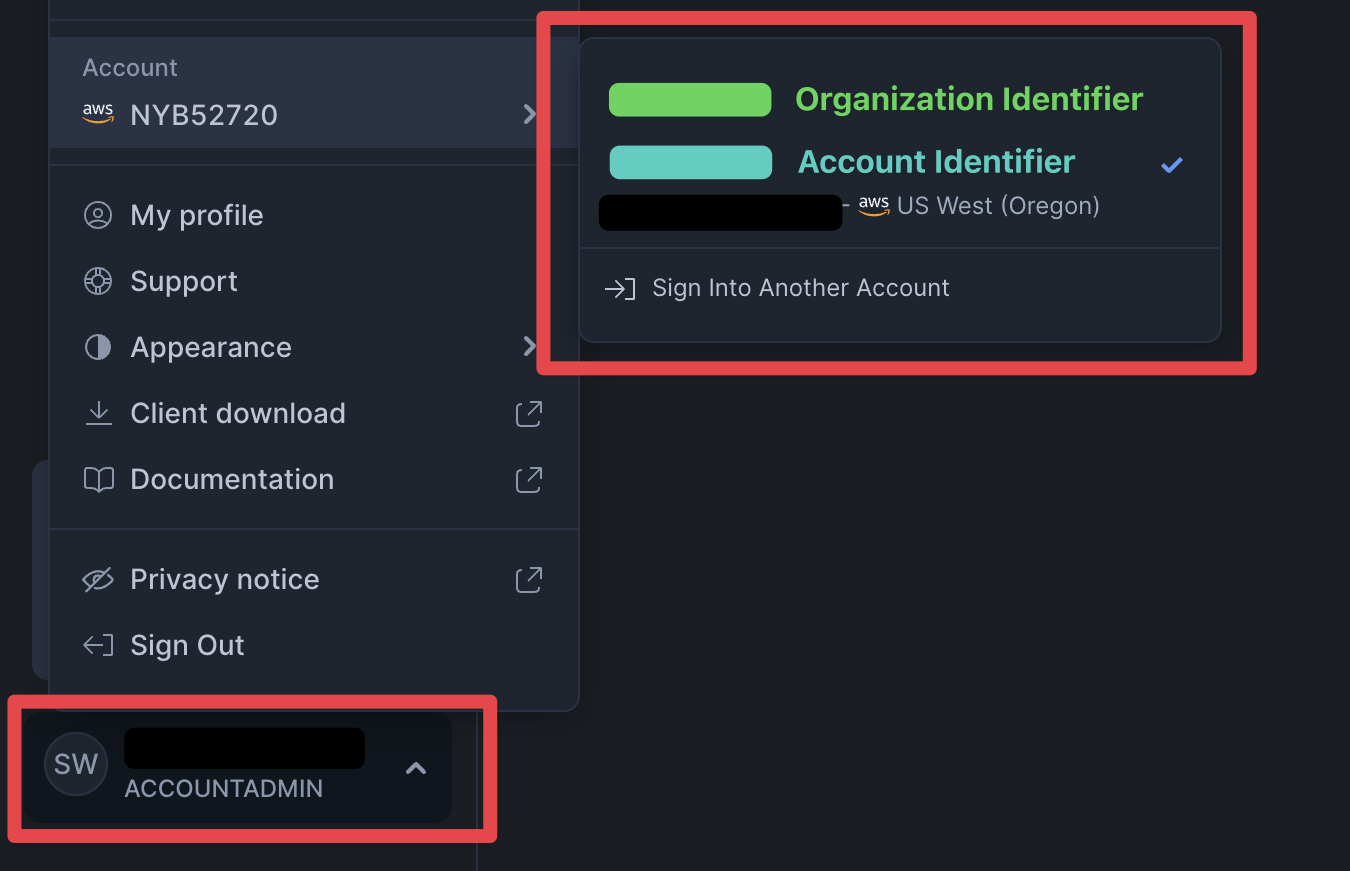
## Set up Dynamic Secrets with Snowflake
Open the Secret Overview dashboard and select the environment in which you would like to add a dynamic secret.
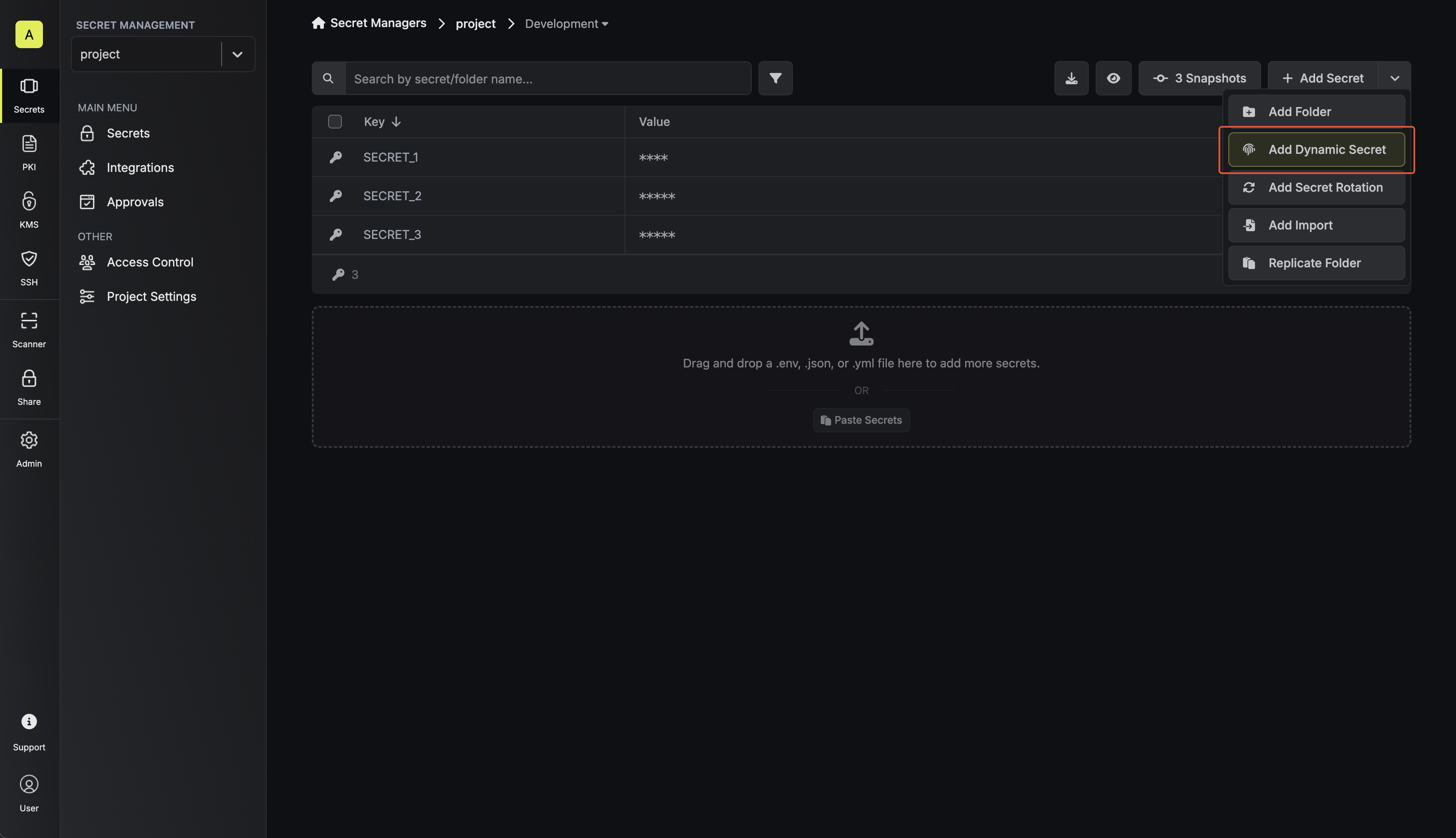
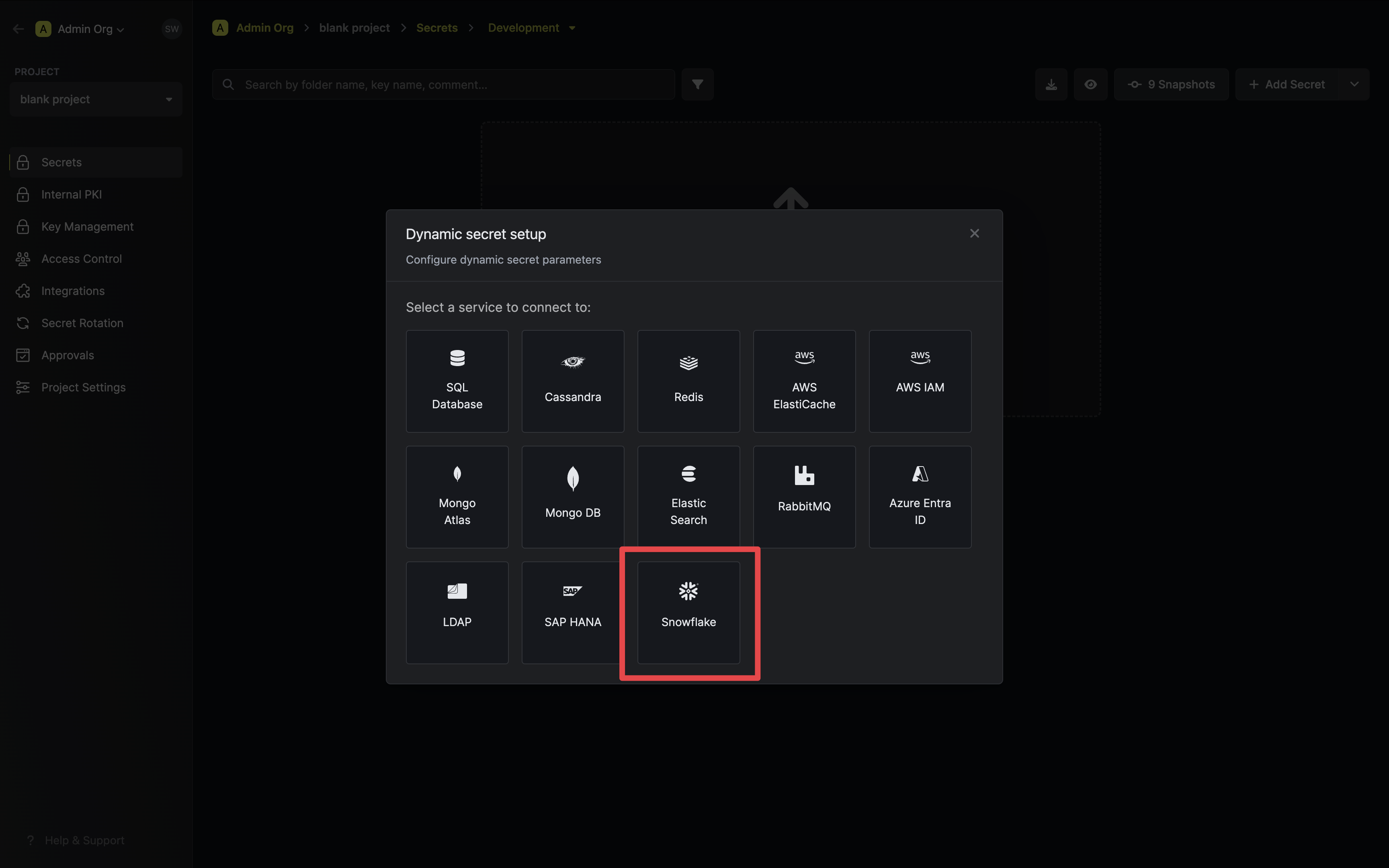
The name you want to reference this secret by
Default time-to-live for a generated secret (it is possible to modify this value when generating a secret)
Maximum time-to-live for a generated secret
Snowflake account identifier
Snowflake organization identifier
Username of the Infisical Service User
Password of the Infisical Service User
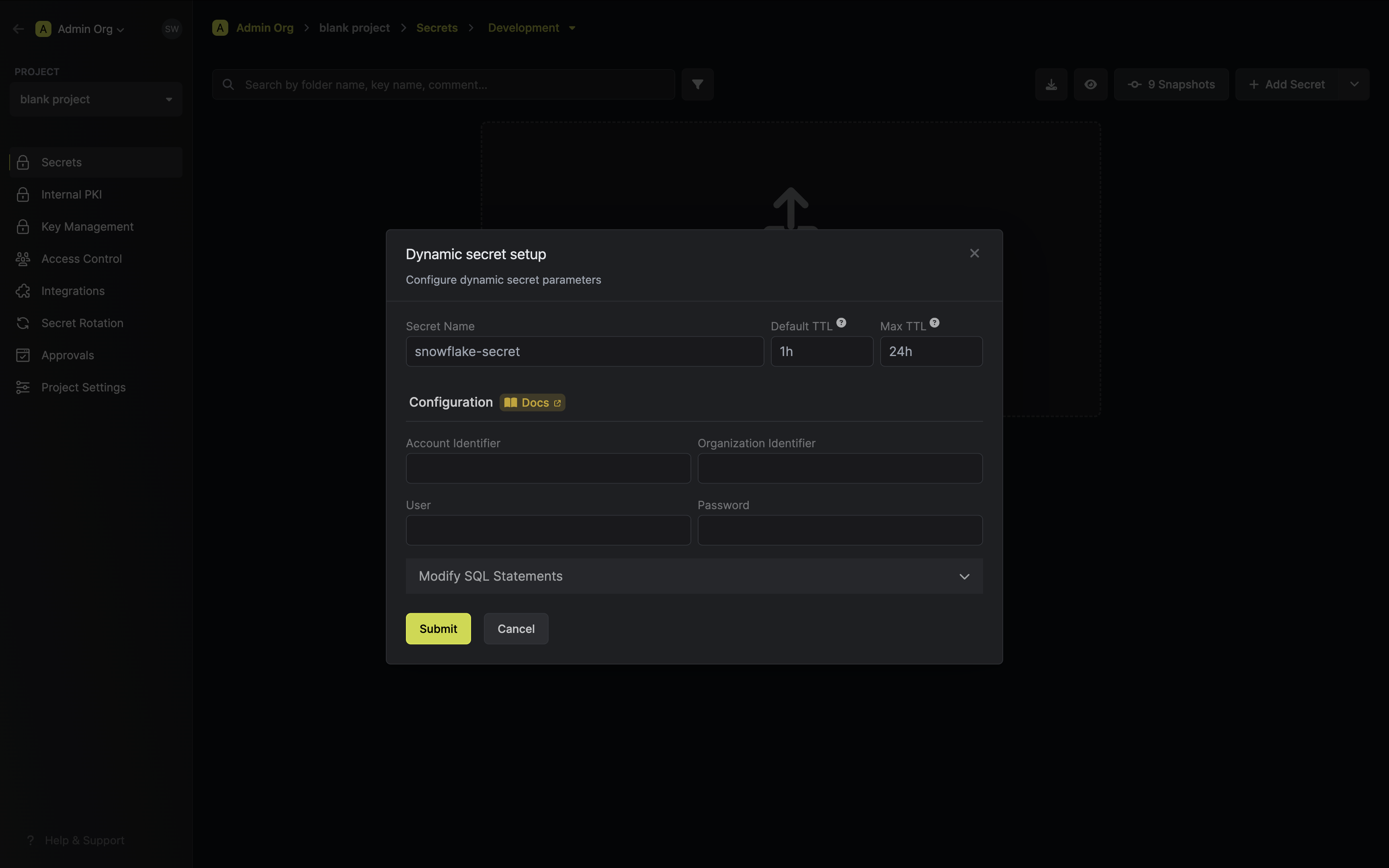
If you want to provide specific privileges for the generated dynamic credentials, you can modify the SQL
statement to your needs.
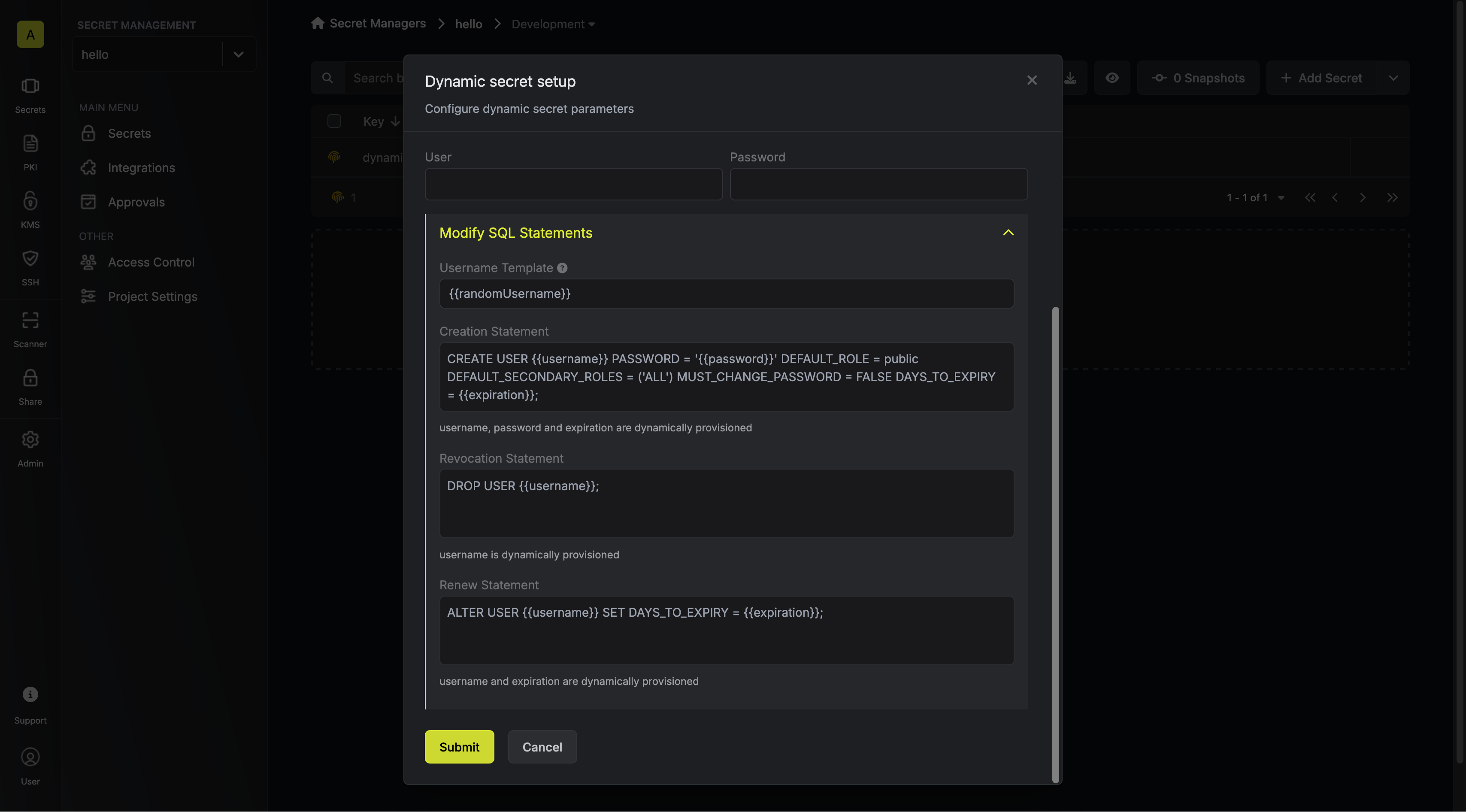
After submitting the form, you will see a dynamic secret created in the dashboard.
Once you've successfully configured the dynamic secret, you're ready to generate on-demand credentials.
To do this, simply click on the 'Generate' button which appears when hovering over the dynamic secret item.
Alternatively, you can initiate the creation of a new lease by selecting 'New Lease' from the dynamic secret
lease list section.
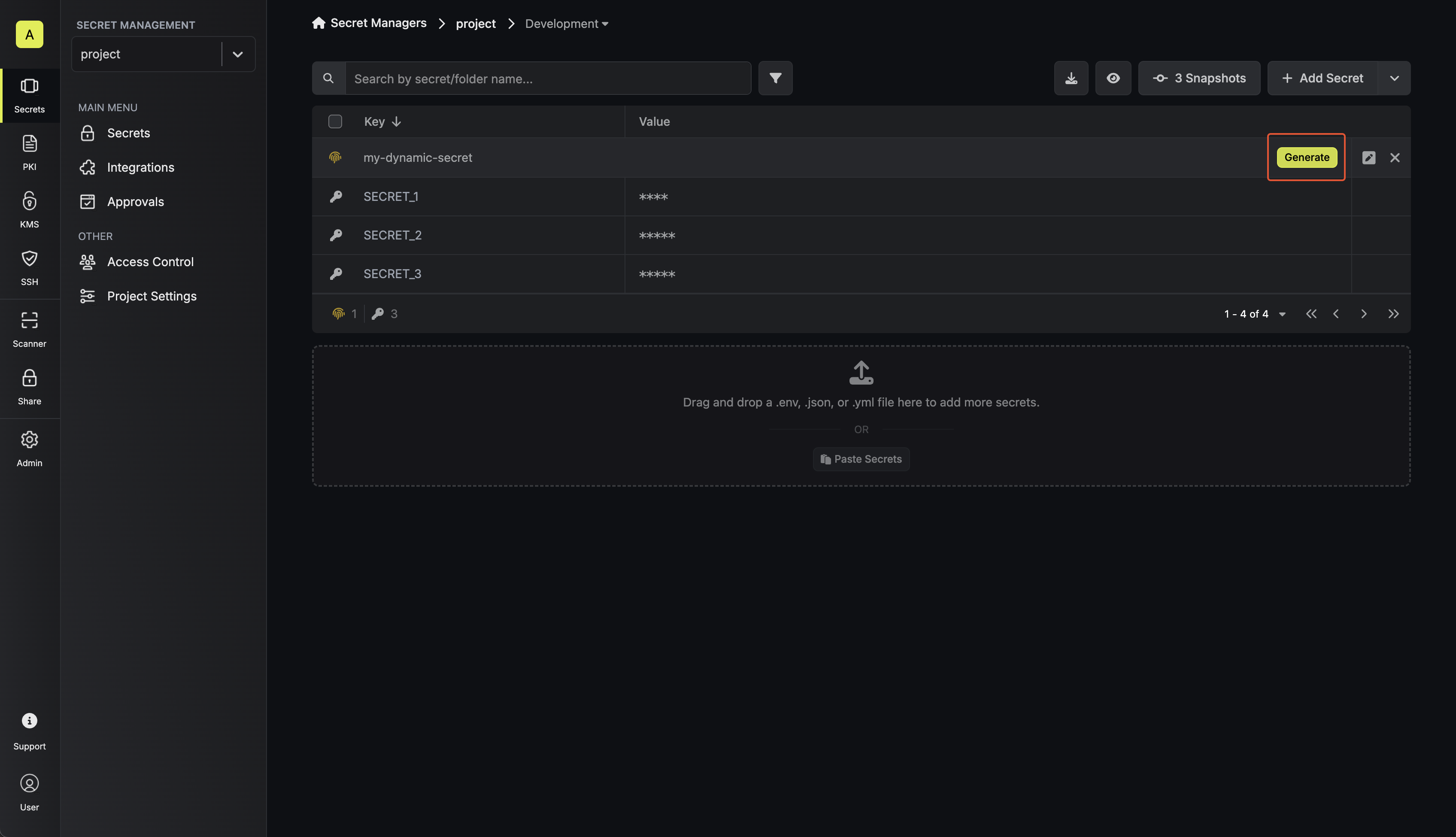
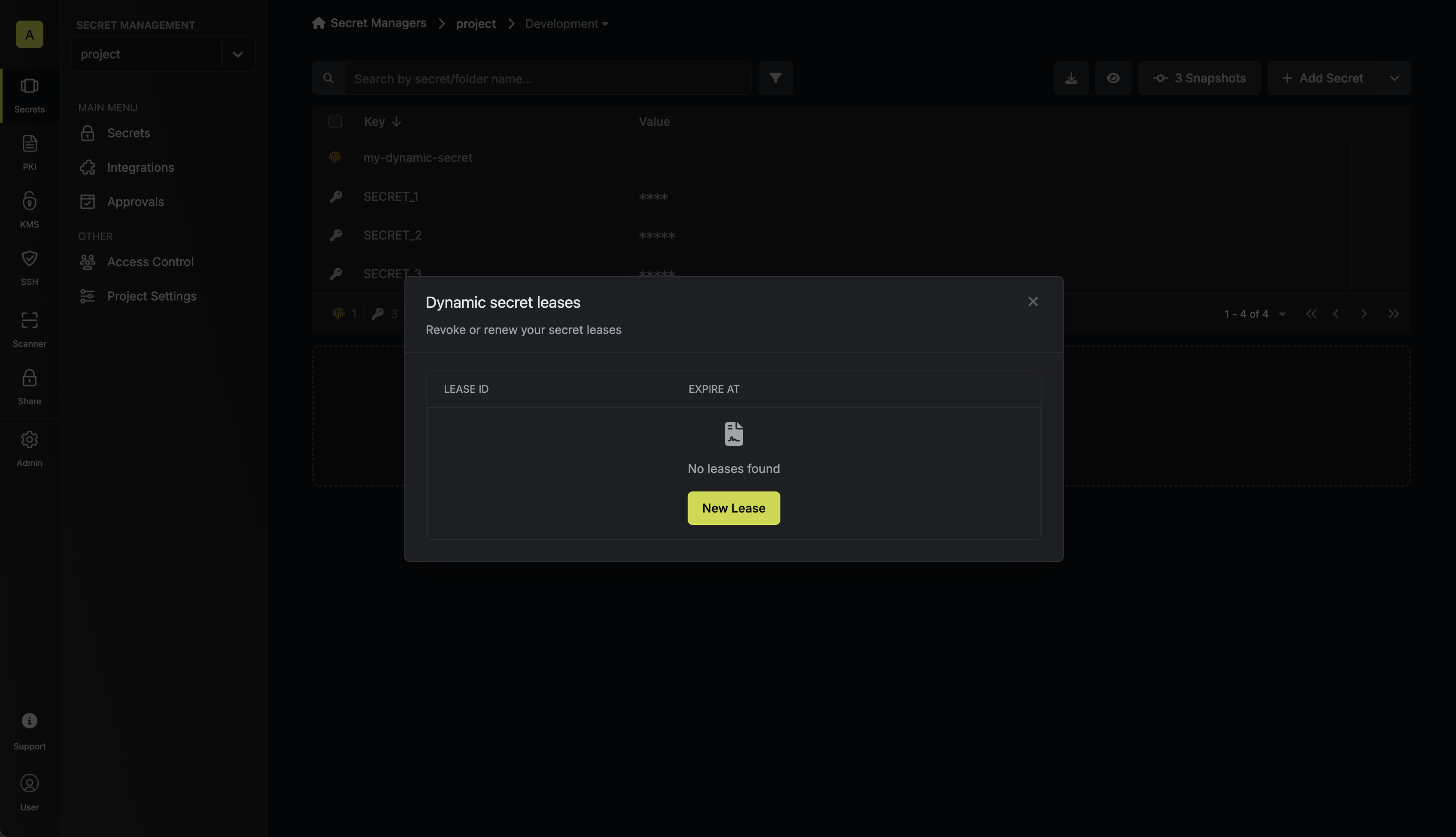
When generating these secrets, it's important to specify a Time-to-Live (TTL) duration. This will dictate how
long the credentials are valid for.
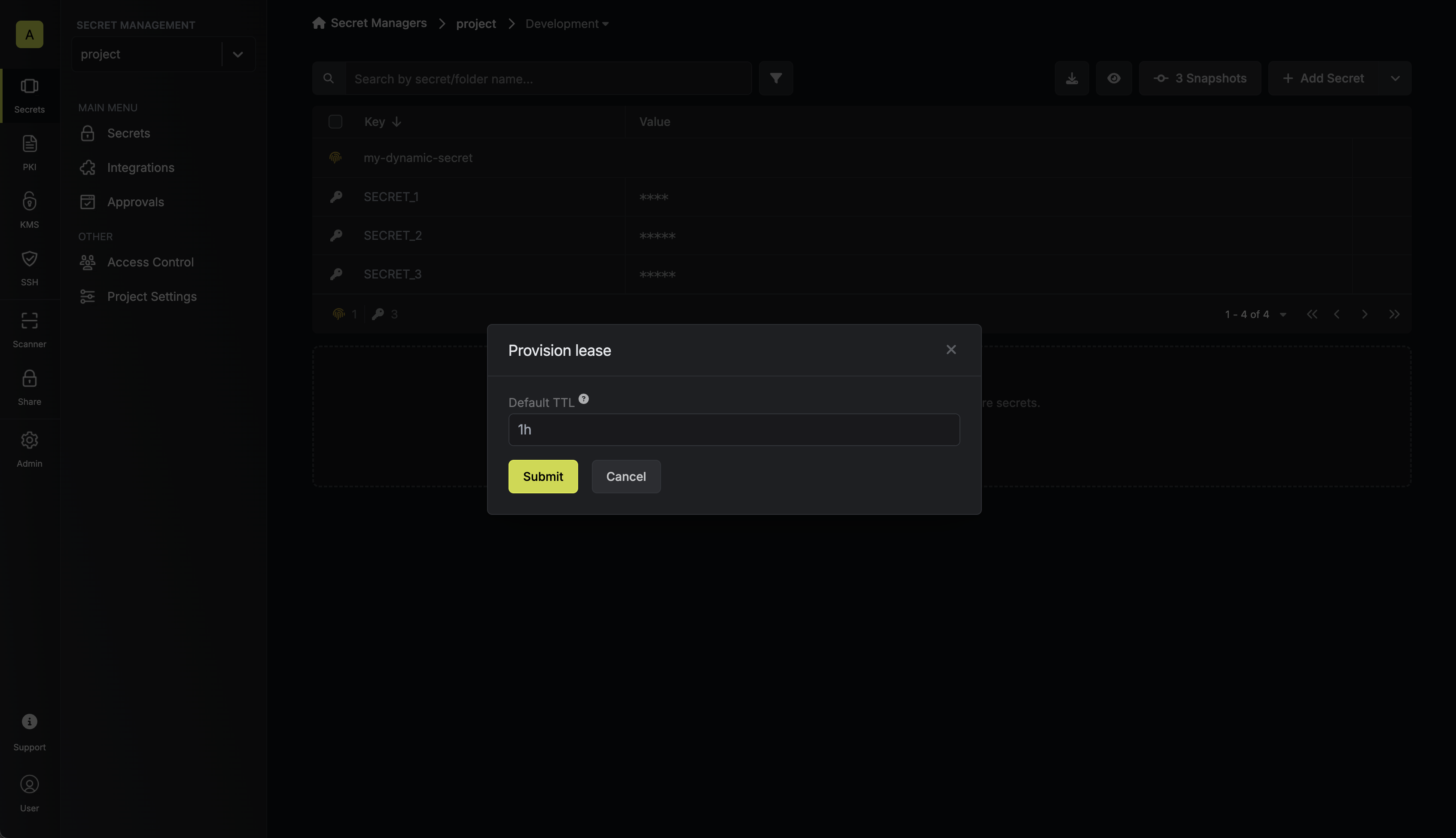
Ensure that the TTL for the lease fall within the maximum TTL defined when configuring the dynamic secret in
step 4.
Once you click the `Submit` button, a new secret lease will be generated and the credentials for it will be
shown to you.
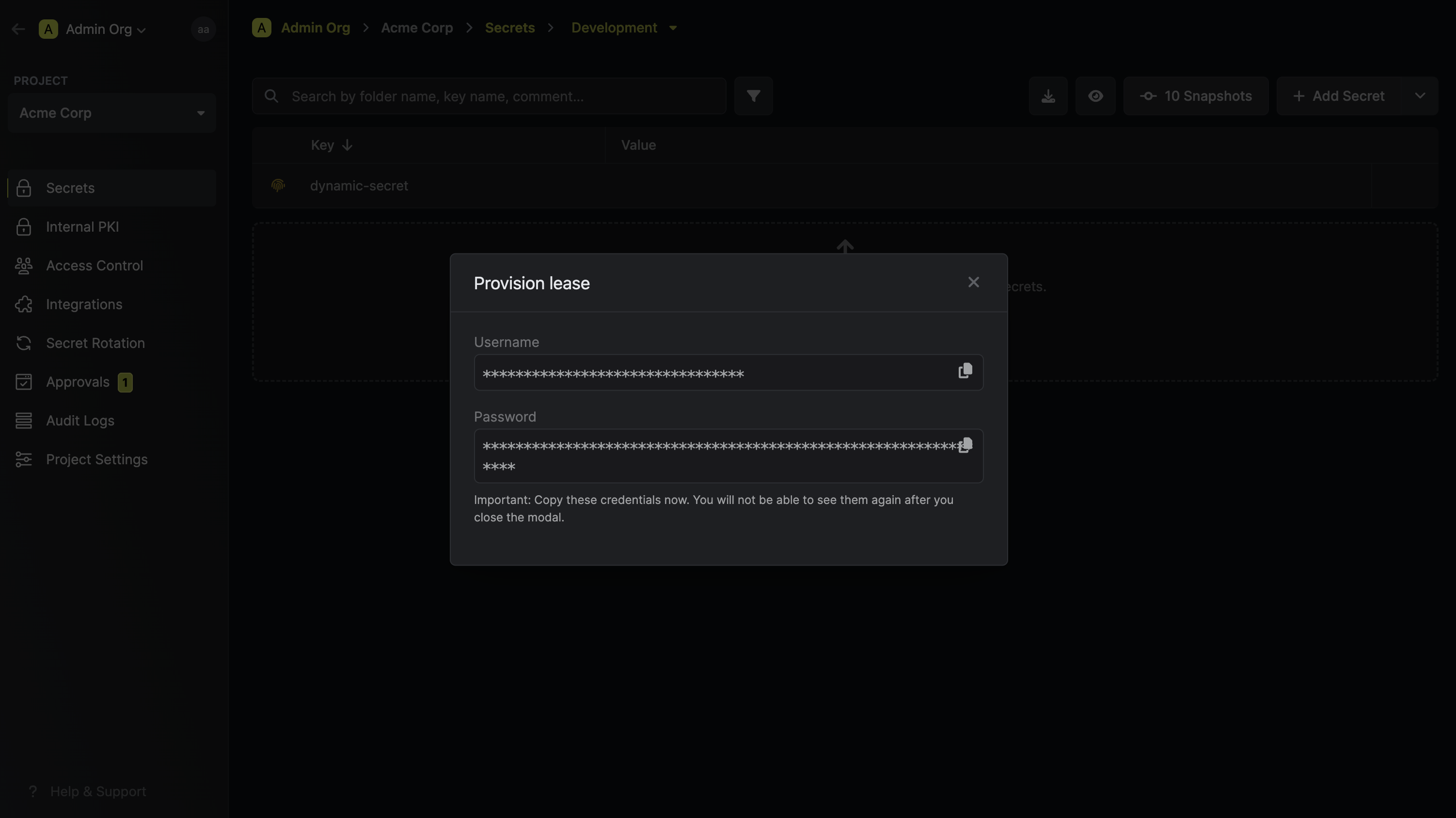
## Audit or Revoke Leases
Once you have created one or more leases, you will be able to access them by clicking on the respective dynamic secret item on the dashboard.
This will allow you see the lease details and delete the lease ahead of its expiration time.
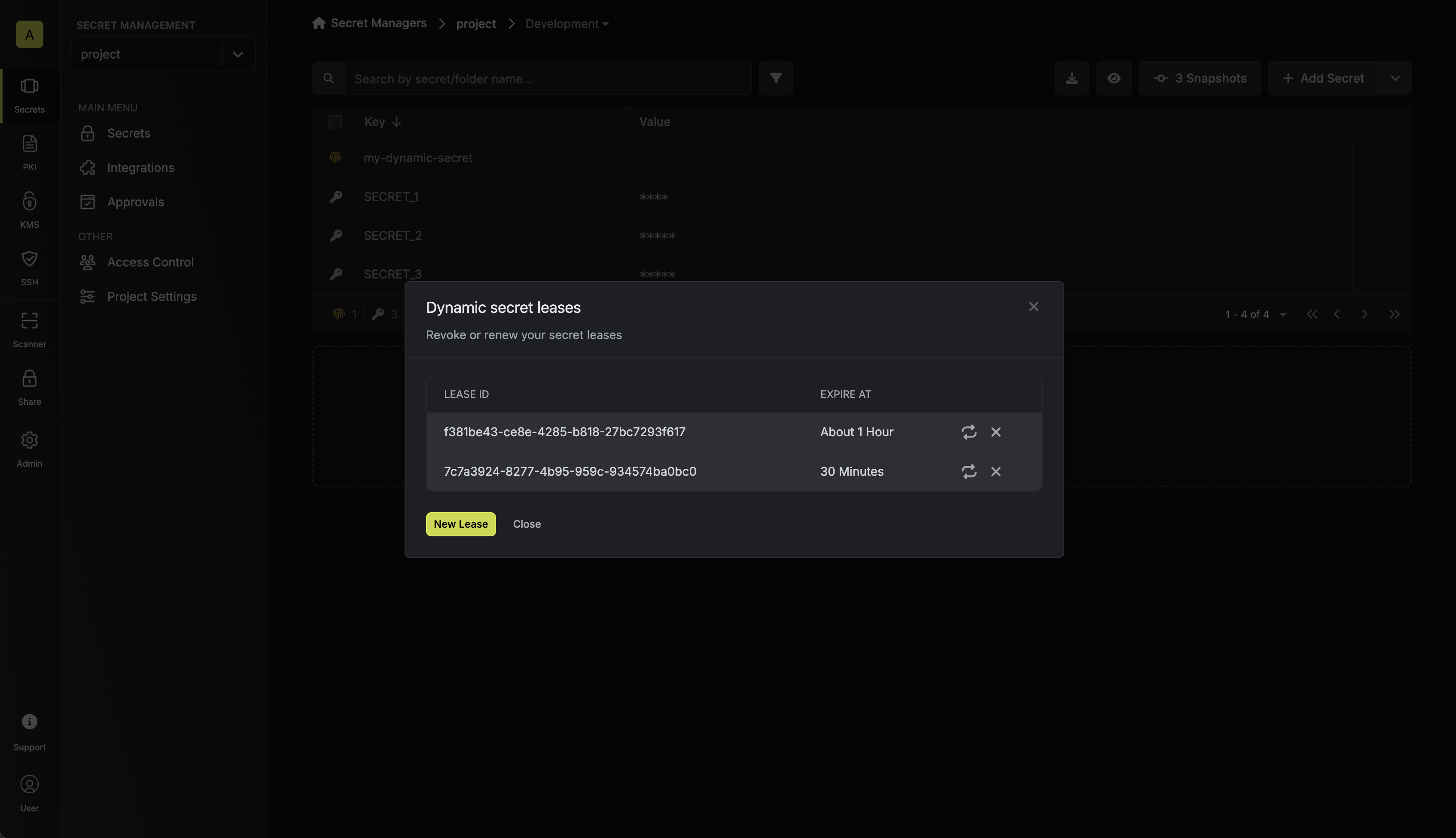
## Renew Leases
To extend the life of the generated dynamic secret lease past its initial time to live, simply click on the **Renew** button as illustrated below.
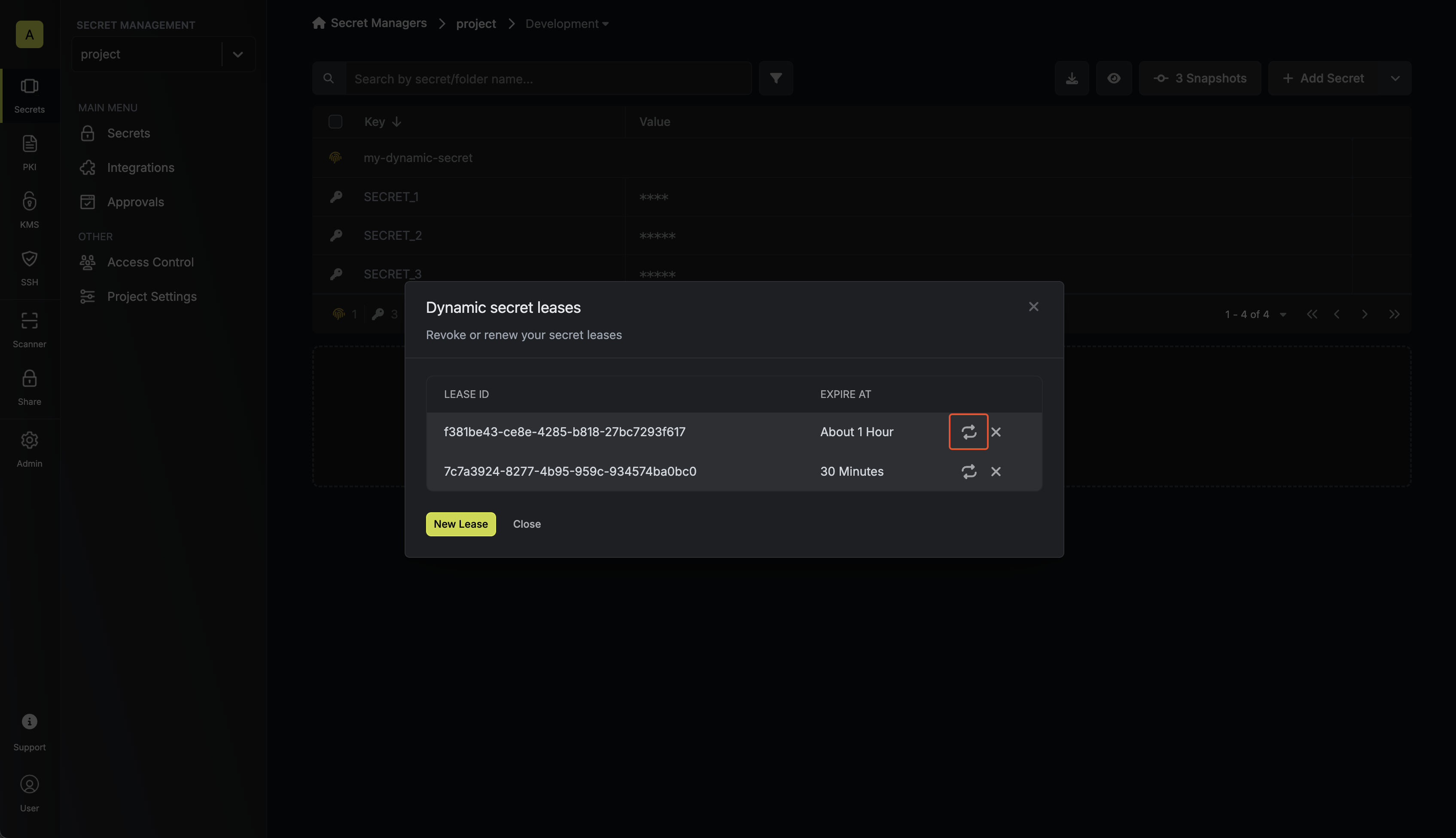
Lease renewals cannot exceed the maximum TTL set when configuring the dynamic
secret.
# Folders
Learn how to organize secrets with folders.
Infisical Folders enable users to organize secrets using custom structures dependent on the intended use case (also known as **path-based secret storage**).
It is great for organizing secrets around hierarchies with multiple services or types of secrets involved at large quantities.
Infisical Folders can be infinitely nested to mirror your application architecture – whether it's microservices, monorepos,
or any logical grouping that best suits your needs.
Consider the following structure for a microservice architecture:
```
| service1
|---- envars
|---- users
|-------- tokens1
|-------- tokens2
| service2
|---- envars
...
```
In this example, we store environment variables for each microservice under each respective `/envars` folder.
We also store user-specific secrets for micro-service 1 under `/service1/users`. With this folder structure in place, your applications only need to specify a path like `/microservice1/envars` to fetch secrets from there.
By extending this example, you can see how path-based secret storage provides a versatile approach to manage secrets for any architecture.
## Managing folders
To add a folder, press the downward chevron to the right of the **Add Secret** button; then press on the **Add Folder** button.
Folder names can only contain alphabets, numbers, and dashes
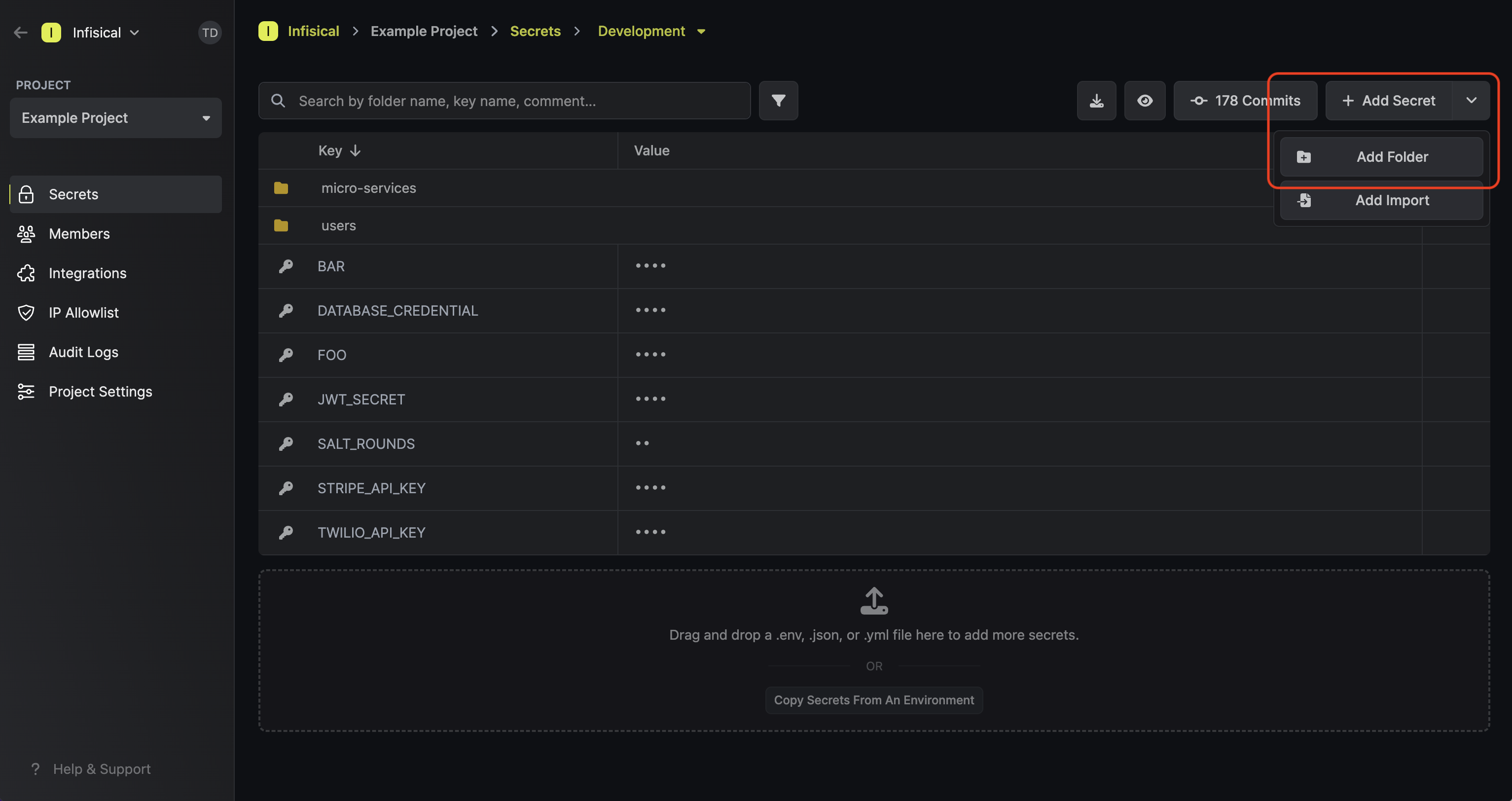
To delete a folder, hover over it and press the **X** button that appears on the right side.
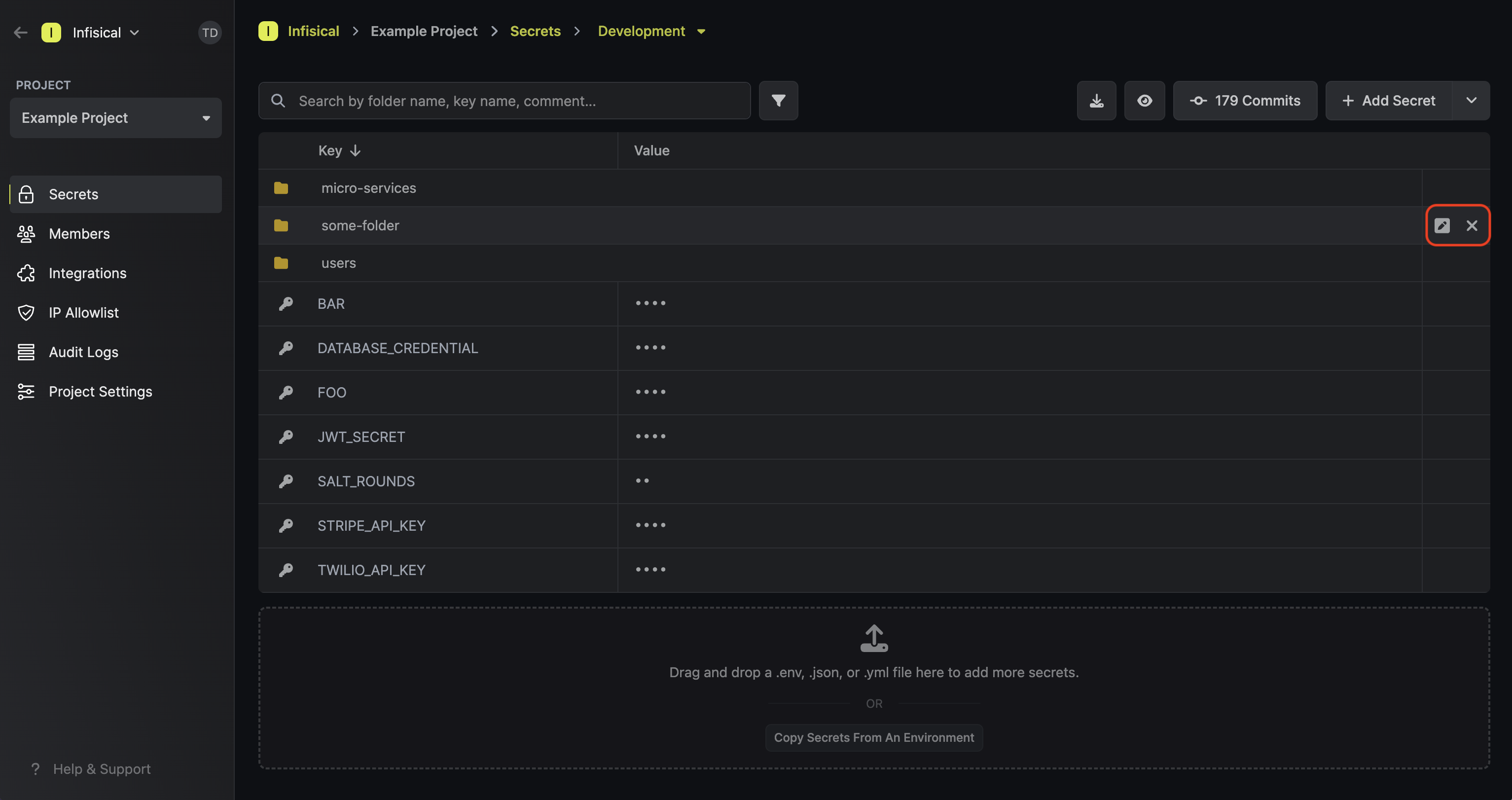
### Comparing folders
It's possible to compare the contents of folders across environments in the **Secrets Overview** page.
When you click on a folder, the table will display the items within it across environments.
In the image below, you can see that the **Development** environment is the only one that contains items
in the `/users` folder, being other folders `/user-a`, `/user-b`, ... `/user-f`.
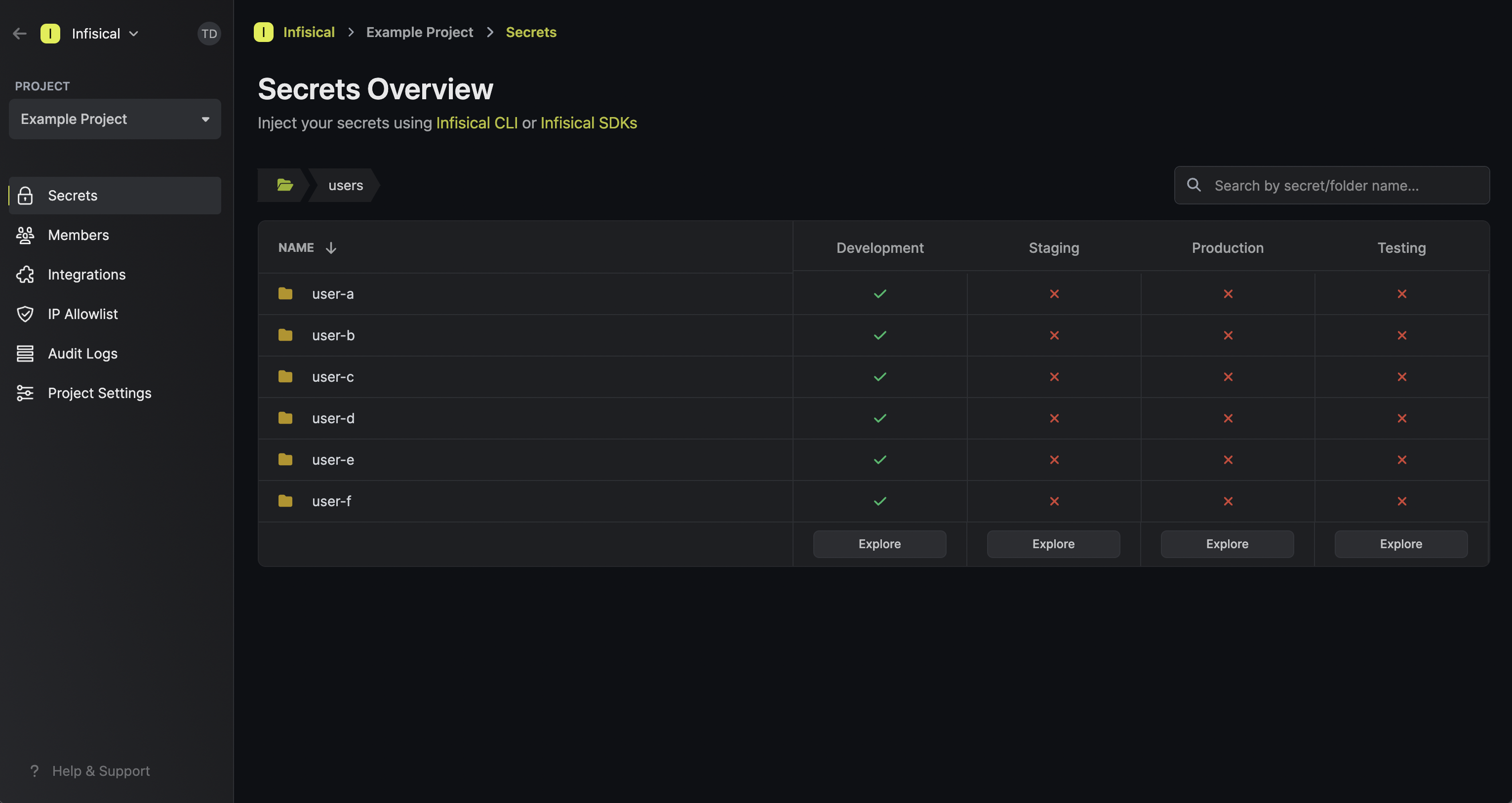
# User Groups
Manage user groups in Infisical.
User Groups is a paid feature.
If you're using Infisical Cloud, then it is available under the **Enterprise Tier**. If you're self-hosting Infisical,
then you should contact [team@infisical.com](mailto:team@infisical.com) to purchase an enterprise license to use it.
## Concept
A (user) group is a collection of users that you can create in an Infisical organization to more efficiently manage permissions and access control for multiple users together. For example, you can have a group called `Developers` with the `Developer` role containing all the developers in your organization.
User groups have the following properties:
* If a group is added to a project under specific role(s), all users in the group will be provisioned access to the project with the role(s). Conversely, if a group is removed from a project, all users in the group will lose access to the project.
* If a user is added to a group, they will inherit the access control properties of the group including access to project(s) under the role(s) assigned to the group. Conversely, if a user is removed from a group, they will lose access to project(s) that the group has access to.
* If a user was previously added to a project under a role and is later added to a group that has access to the same project under a different role, then the user will now have access to the project under the composite permissions of the two roles. If the group is subsequently removed from the project, the user will not lose access to the project as they were previously added to the project separately.
* A user can be part of multiple groups. If a user is part of multiple groups, they will inherit the composite permissions of all the groups that they are part of.
## Workflow
In the following steps, we explore how to create and use user groups to provision user access to projects in Infisical.
To create a group, head to your Organization Settings > Access Control > Groups and press **Create group**.
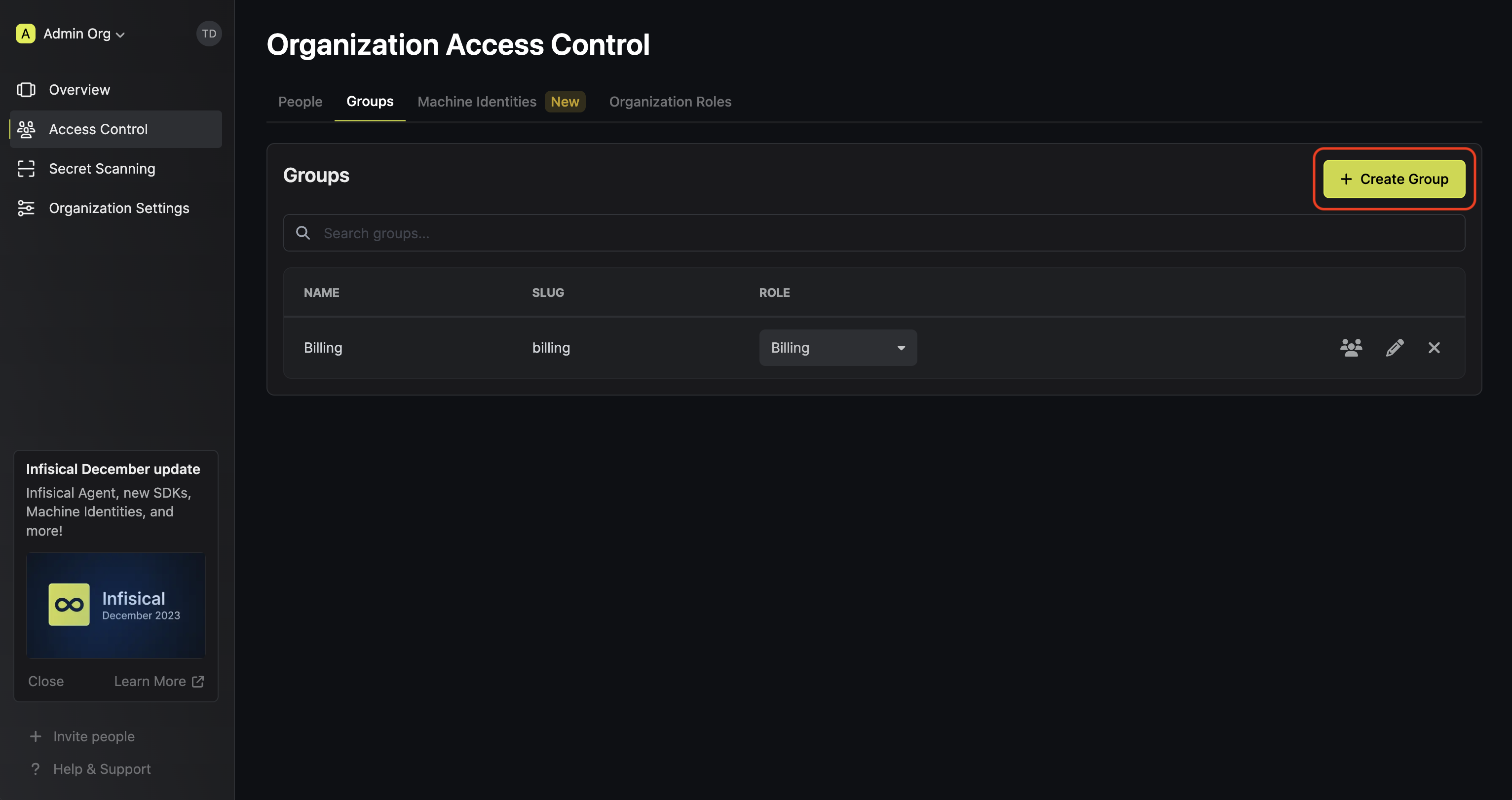
When creating a group, you specify an organization level [role](/documentation/platform/role-based-access-controls) for it to assume; you can configure roles in Organization Settings > Access Control > Organization Roles.
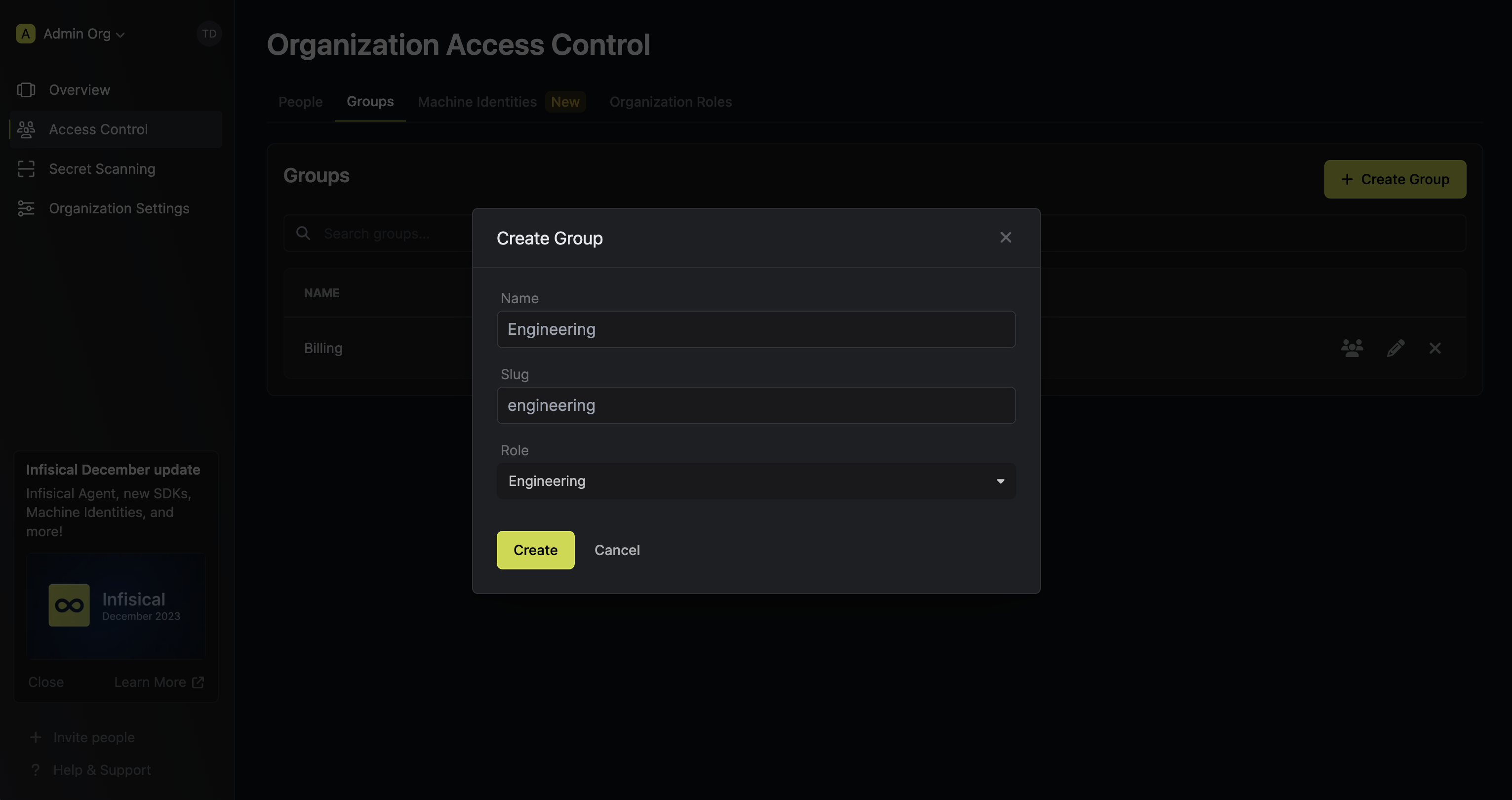
Now input a few details for your new group. Here’s some guidance for each field:
* Name (required): A friendly name for the group like `Engineering`.
* Slug (required): A unique identifier for the group like `engineering`.
* Role (required): A role from the Organization Roles tab for the group to assume. The organization role assigned will determine what organization level resources this group can have access to.
Next, you'll want to assign users to the group. To do this, press on the users icon on the group and start assigning users to the group.
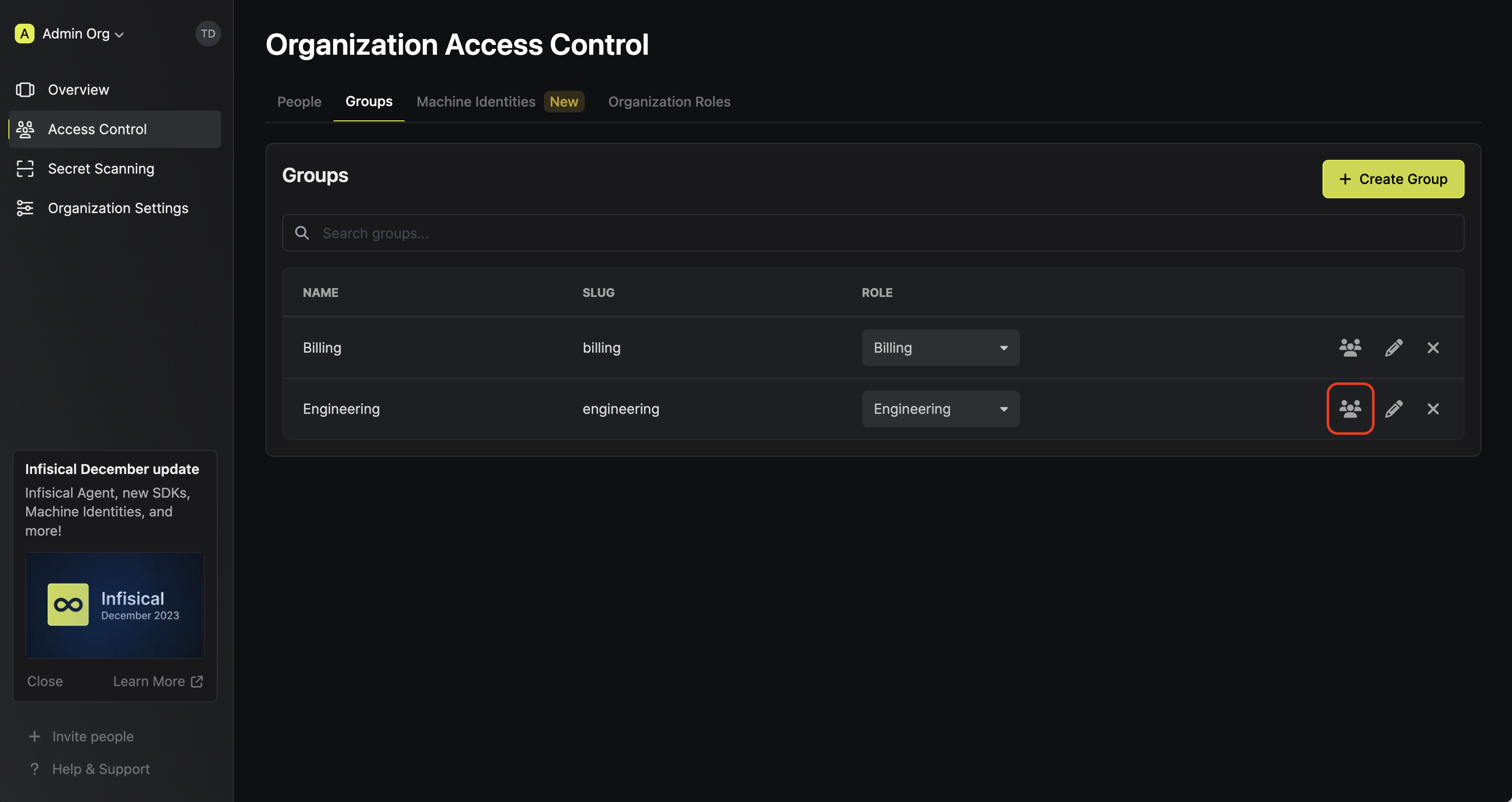
In this example, we're assigning **Alan Turing** and **Ada Lovelace** to the group **Engineering**.
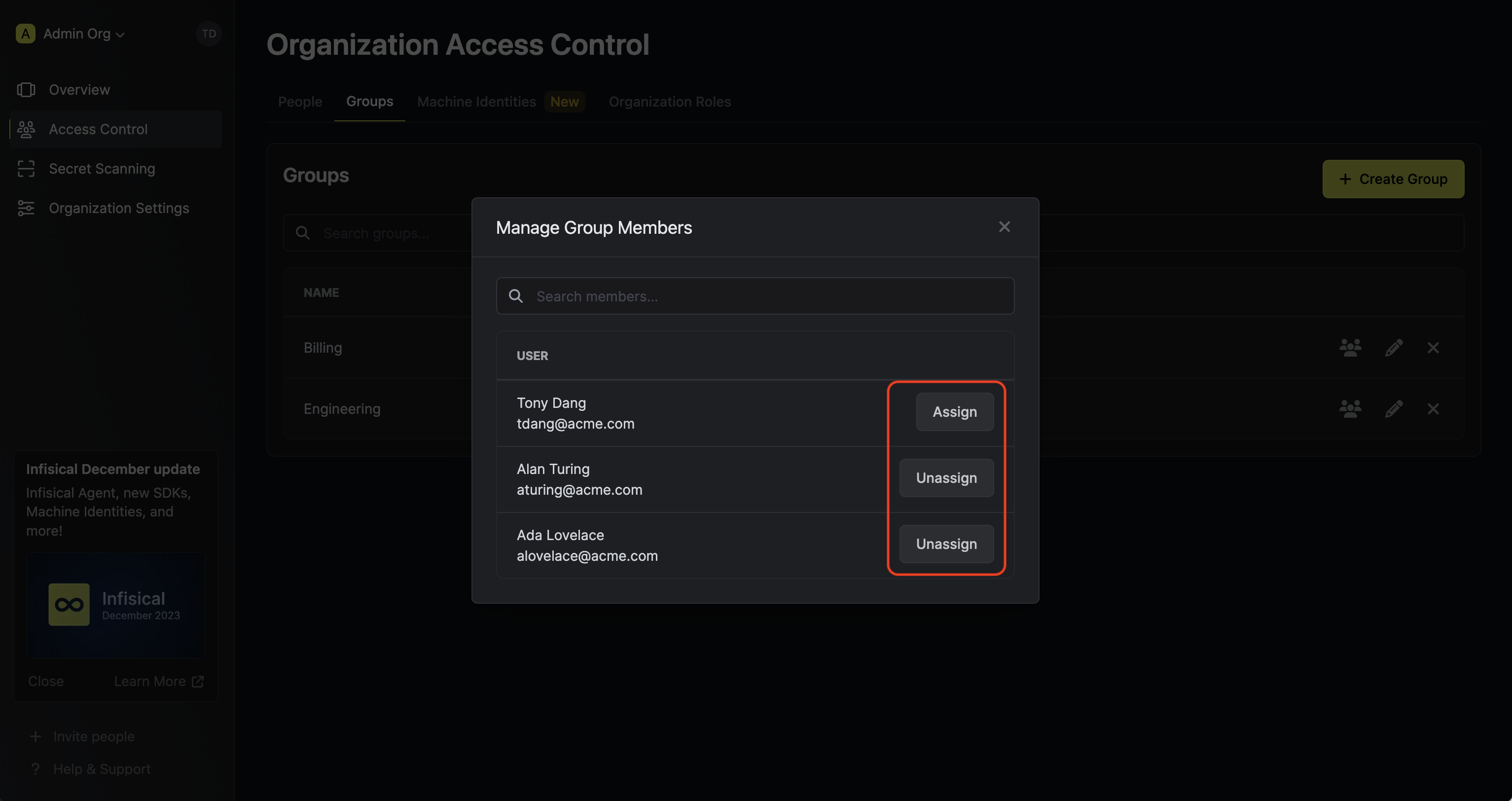
To enable the group to access project-level resources such as secrets within a specific project, you should add it to that project.
To do this, head over to the project you want to add the group to and go to Project Settings > Access Control > Groups and press **Add group**.
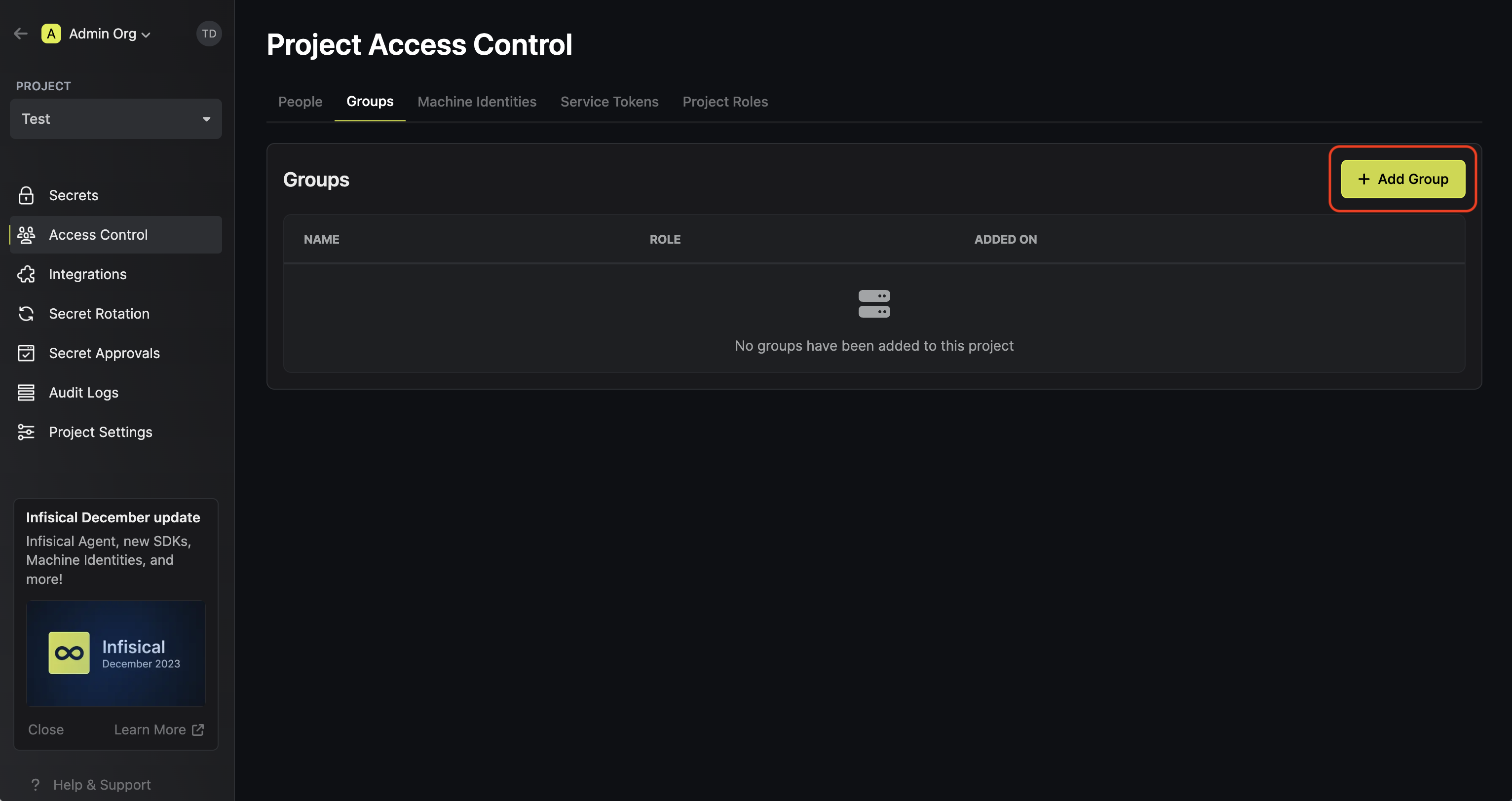
Next, select the group you want to add to the project and the project level role you want to allow it to assume. The project role assigned will determine what project level resources this group can have access to.
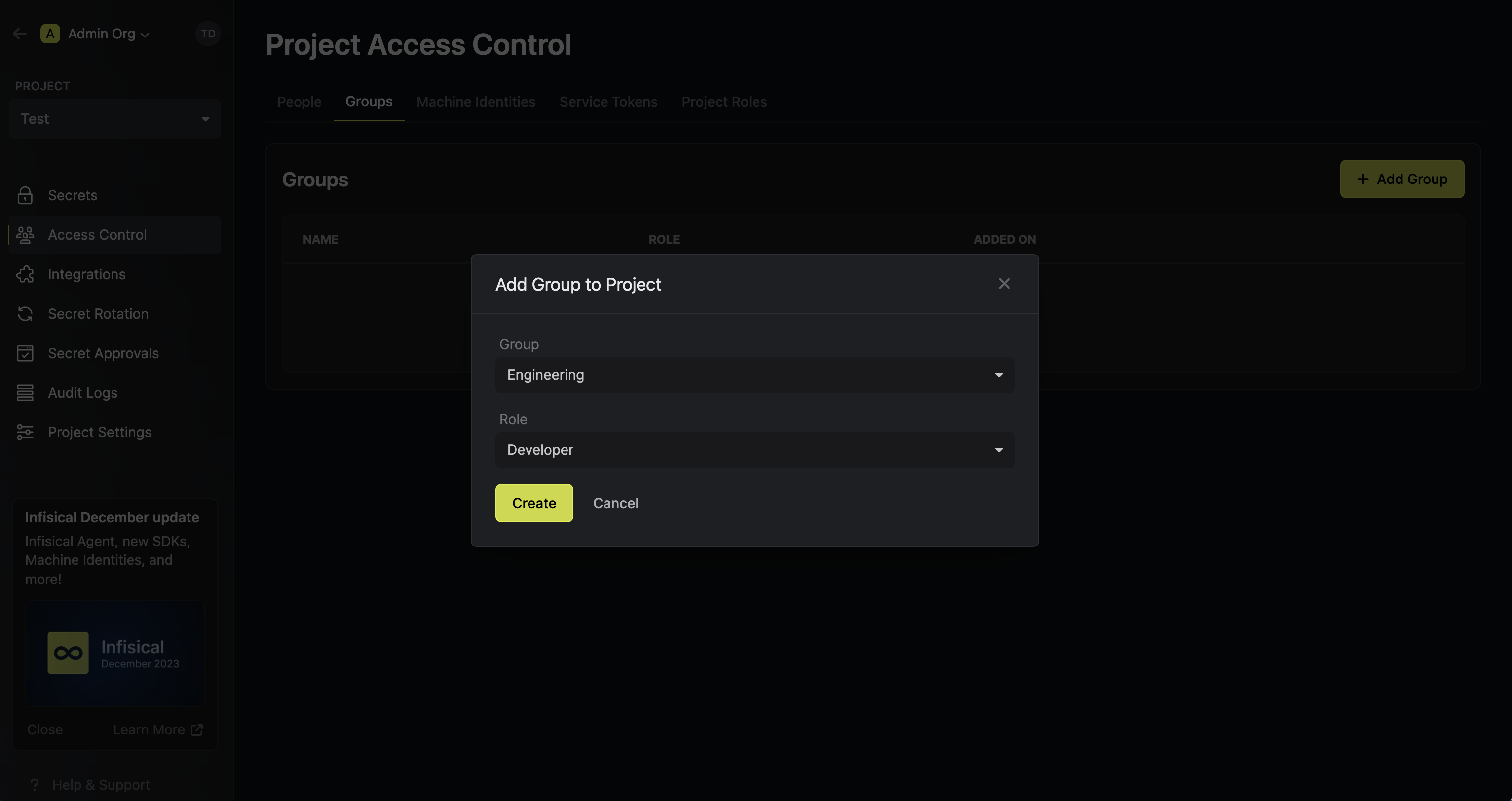
That's it!
The users of the group now have access to the project under the role you assigned to the group.
# AWS Auth
Learn how to authenticate with Infisical for EC2 instances, Lambda functions, and other IAM principals.
**AWS Auth** is an AWS-native authentication method for IAM principals like EC2 instances or Lambda functions to access Infisical.
## Diagram
The following sequence diagram illustrates the AWS Auth workflow for authenticating AWS IAM principals with Infisical.
```mermaid
sequenceDiagram
participant Client as Client
participant Infis as Infisical
participant AWS as AWS STS
Note over Client,Client: Step 1: Sign GetCallerIdentityQuery
Note over Client,Infis: Step 2: Login Operation
Client->>Infis: Send signed query details /api/v1/auth/aws-auth/login
Note over Infis,AWS: Step 3: Query verification
Infis->>AWS: Forward signed GetCallerIdentity query
AWS-->>Infis: Return IAM user/role details
Note over Infis: Step 4: Identity Property Validation
Infis->>Client: Return short-lived access token
Note over Client,Infis: Step 5: Access Infisical API with Token
Client->>Infis: Make authenticated requests using the short-lived access token
```
## Concept
At a high-level, Infisical authenticates an IAM principal by verifying its identity and checking that it meets specific requirements (e.g. it is an allowed IAM principal ARN) at the `/api/v1/auth/aws-auth/login` endpoint. If successful,
then Infisical returns a short-lived access token that can be used to make authenticated requests to the Infisical API.
To be more specific:
1. The client IAM principal signs a `GetCallerIdentity` query using the [AWS Signature v4 algorithm](https://docs.aws.amazon.com/IAM/latest/UserGuide/create-signed-request.html); this is done using the credentials from the AWS environment where the IAM principal is running.
2. The client sends the signed query data to Infisical including the request method, request body, and request headers at the `/api/v1/auth/aws-auth/login` endpoint.
3. Infisical reconstructs the query and sends it to AWS STS API via the [sts:GetCallerIdentity](https://docs.aws.amazon.com/STS/latest/APIReference/API_GetCallerIdentity.html) method for verification and obtains the identity associated with the IAM principal.
4. Infisical checks the identity's properties against set criteria such **Allowed Principal ARNs**.
5. If all is well, Infisical returns a short-lived access token that the IAM principal can use to make authenticated requests to the Infisical API.
We recommend using one of Infisical's clients like SDKs or the Infisical Agent
to authenticate with Infisical using AWS Auth as they handle the
authentication process including the signed `GetCallerIdentity` query
construction for you.
Also, note that Infisical needs network-level access to send requests to the AWS STS API
as part of the AWS Auth workflow.
## Guide
In the following steps, we explore how to create and use identities for your workloads and applications on AWS to
access the Infisical API using the AWS Auth authentication method.
To create an identity, head to your Organization Settings > Access Control > Machine Identities and press **Create identity**.
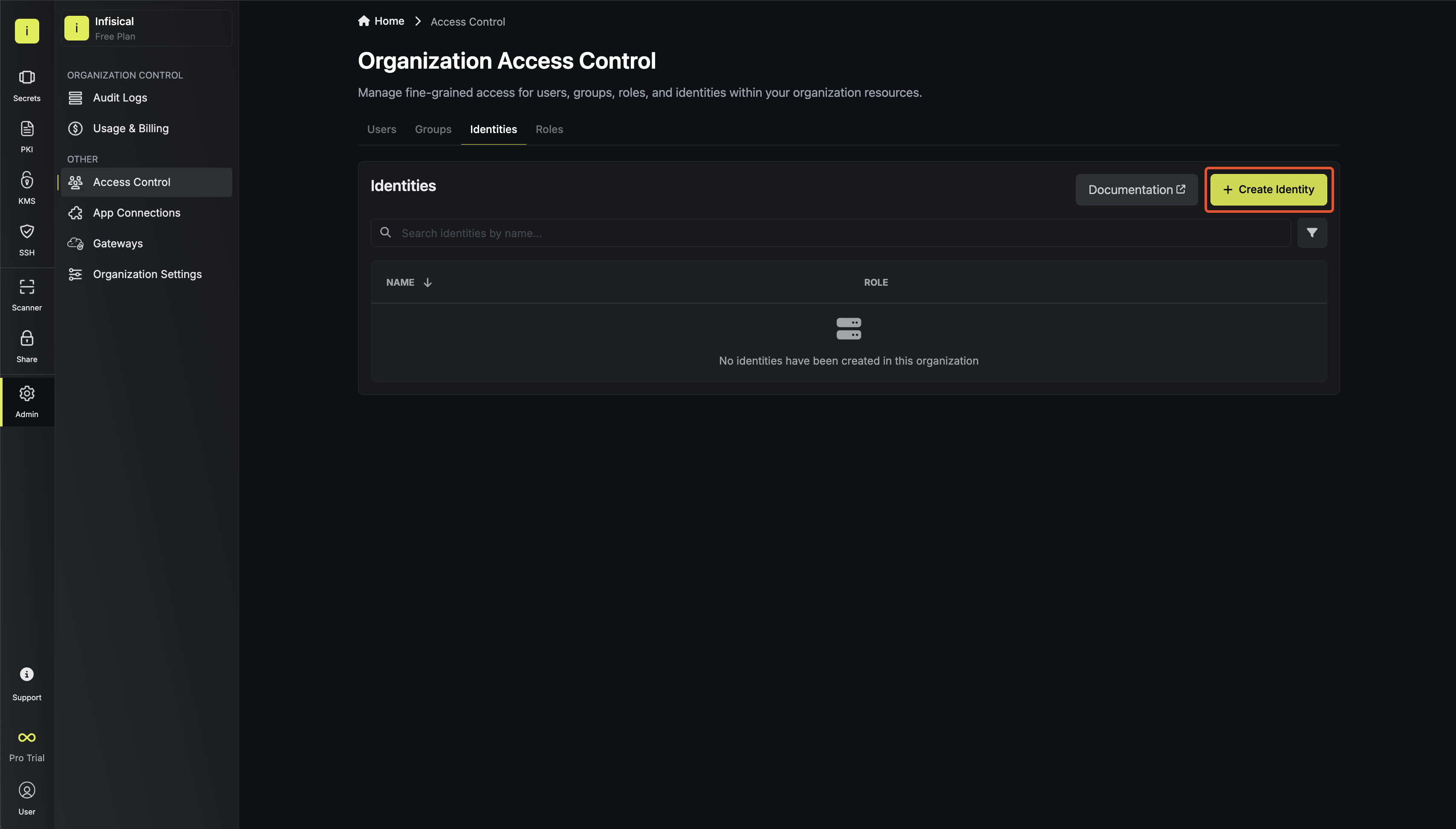
When creating an identity, you specify an organization level [role](/documentation/platform/role-based-access-controls) for it to assume; you can configure roles in Organization Settings > Access Control > Organization Roles.
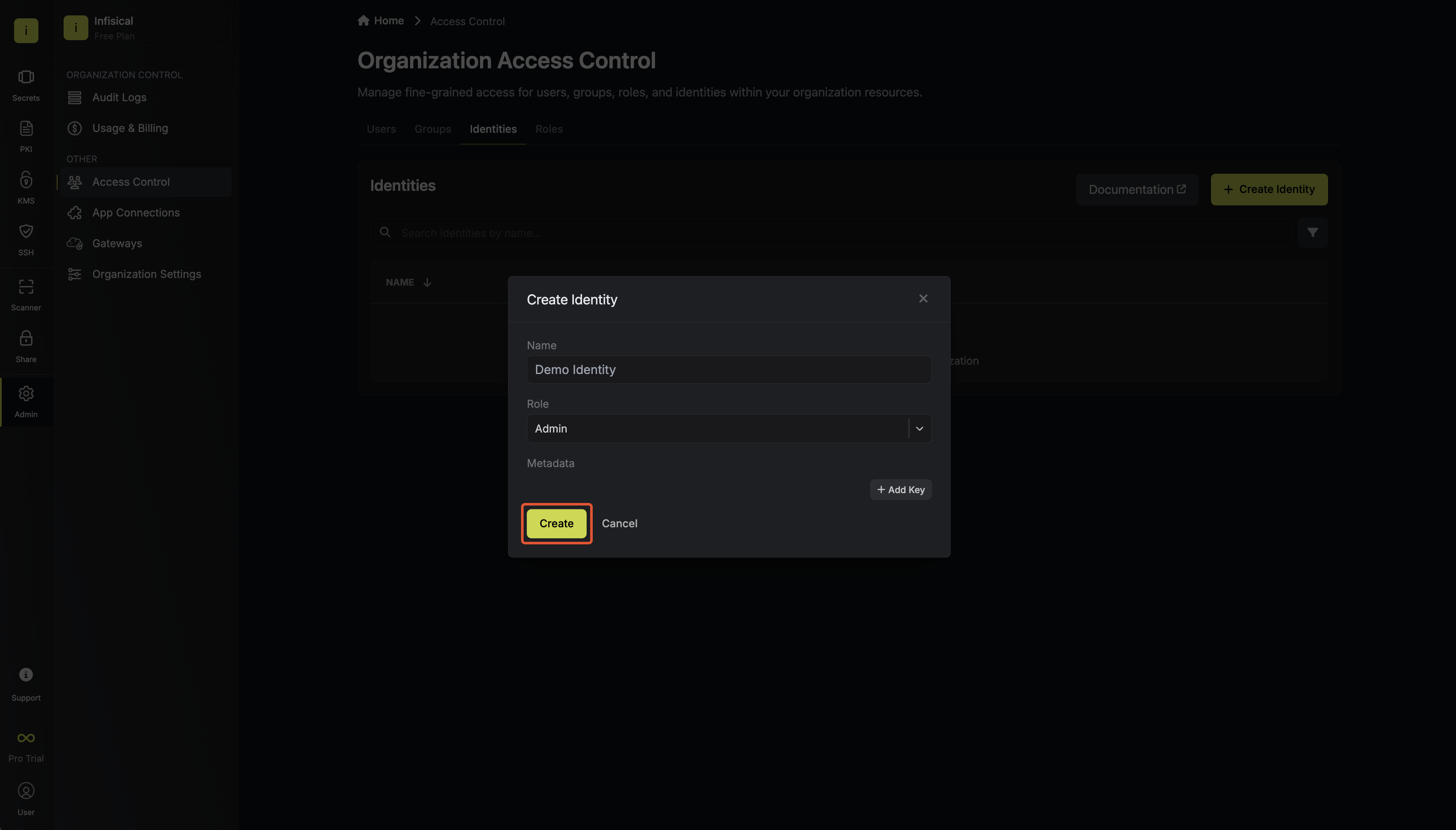
Now input a few details for your new identity. Here's some guidance for each field:
* Name (required): A friendly name for the identity.
* Role (required): A role from the **Organization Roles** tab for the identity to assume. The organization role assigned will determine what organization level resources this identity can have access to.
Once you've created an identity, you'll be redirected to a page where you can manage the identity.
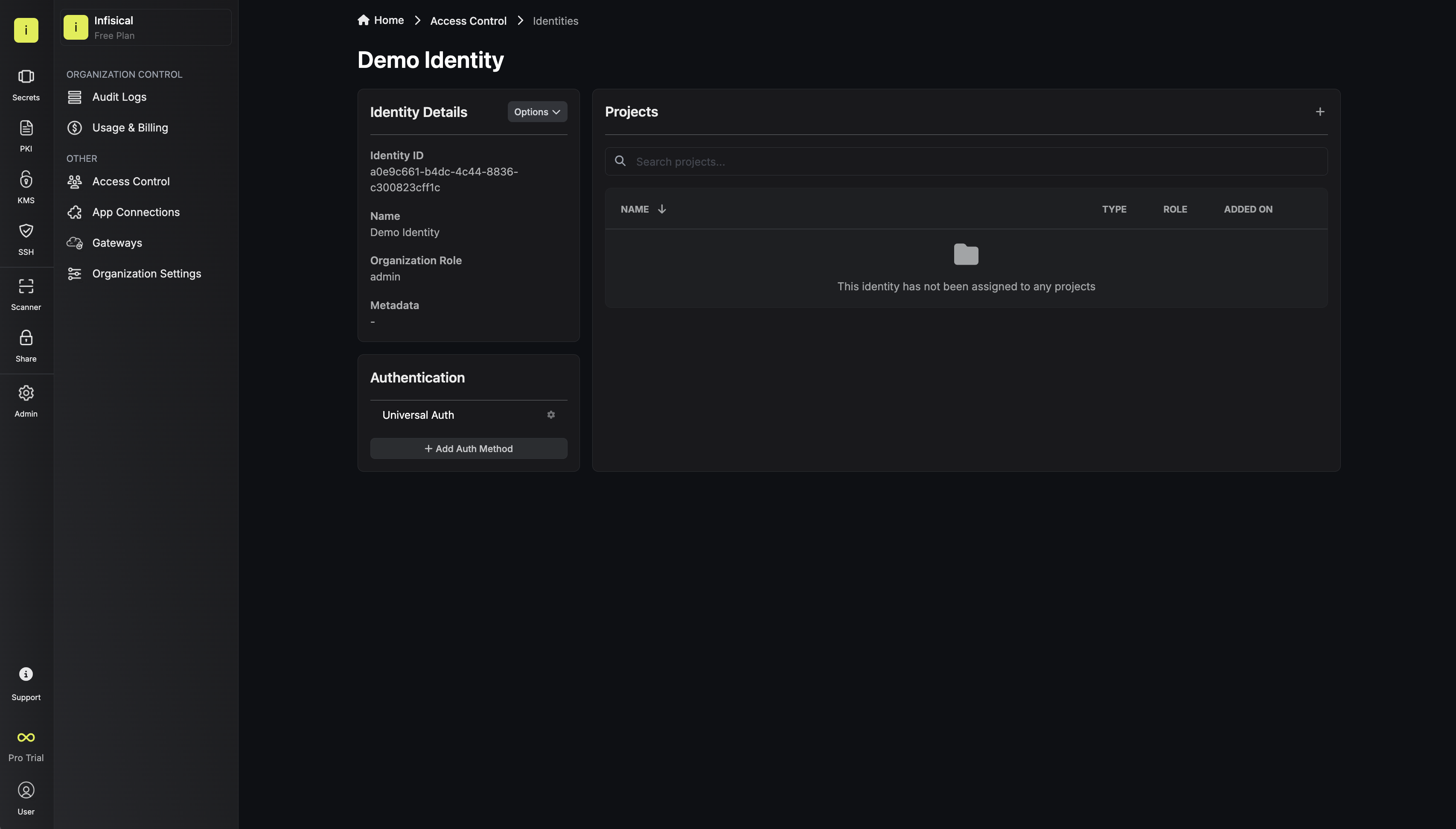
Since the identity has been configured with Universal Auth by default, you should re-configure it to use AWS Auth instead. To do this, press to edit the **Authentication** section,
remove the existing Universal Auth configuration, and add a new AWS Auth configuration onto the identity.
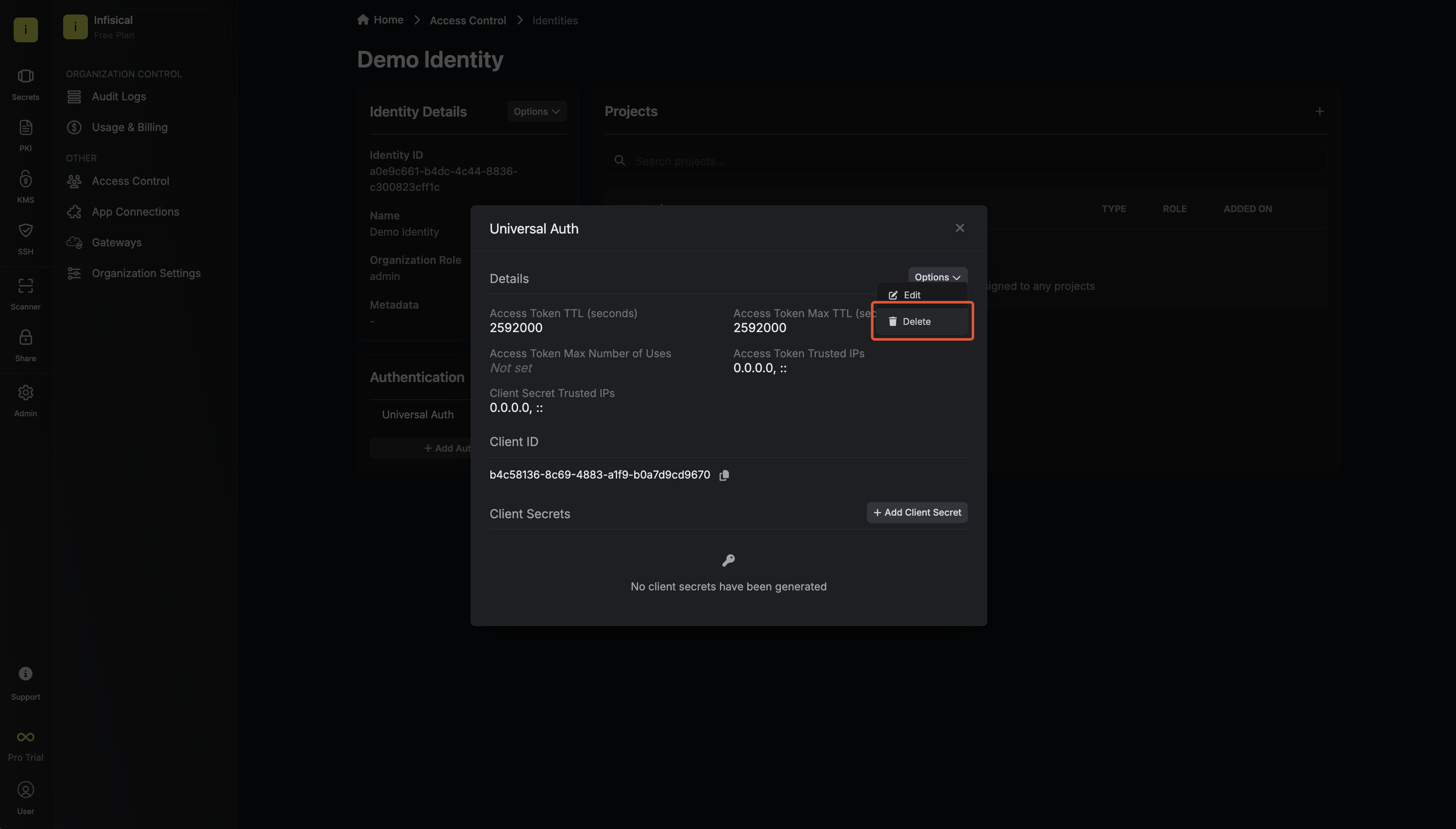
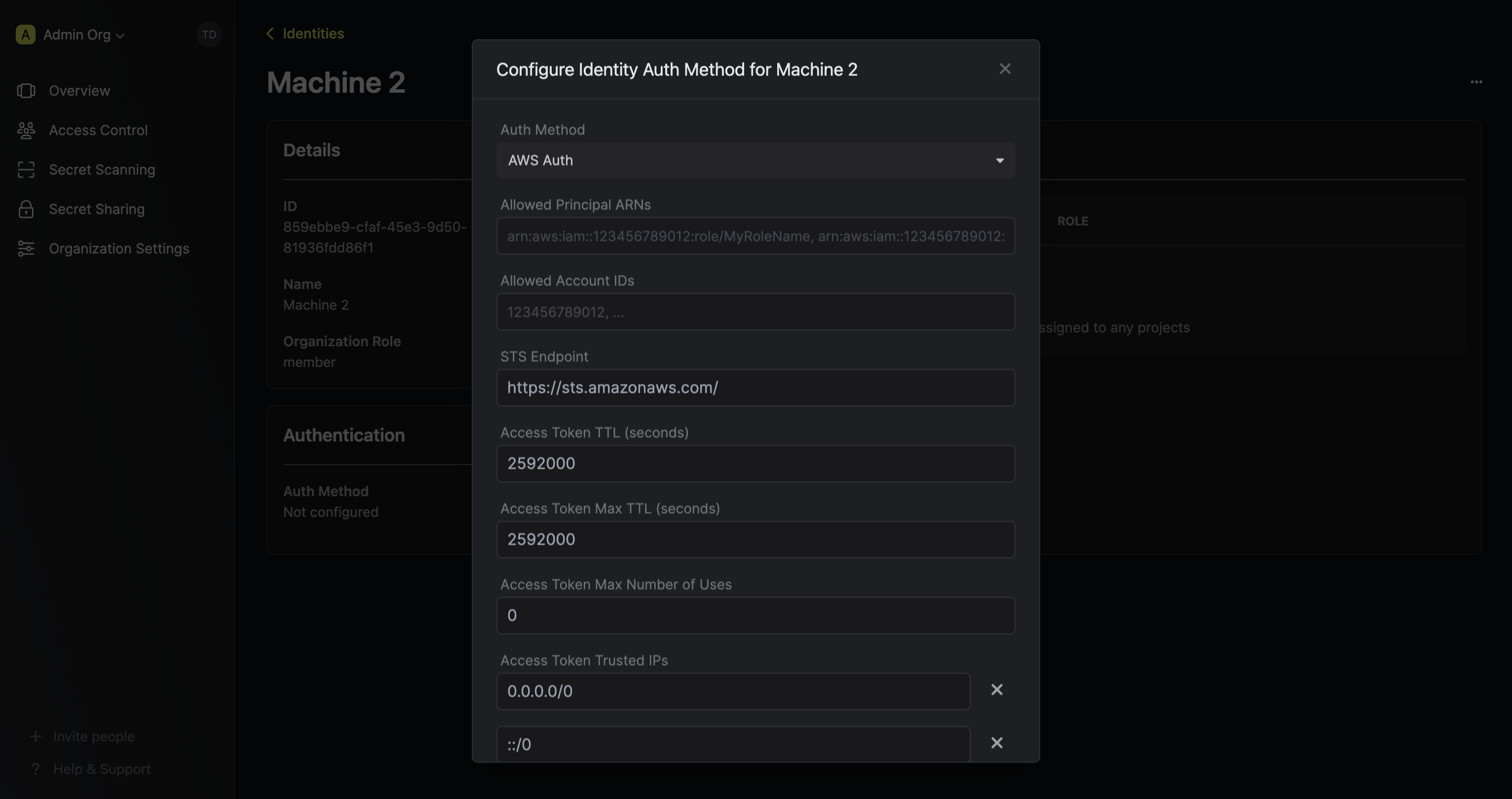
Here's some more guidance on each field:
* Allowed Principal ARNs: A comma-separated list of trusted IAM principal ARNs that are allowed to authenticate with Infisical. The values should take one of three forms: `arn:aws:iam::123456789012:user/MyUserName`, `arn:aws:iam::123456789012:role/MyRoleName`, or `arn:aws:iam::123456789012:*`. Using a wildcard in this case allows any IAM principal in the account `123456789012` to authenticate with Infisical under the identity.
* Allowed Account IDs: A comma-separated list of trusted AWS account IDs that are allowed to authenticate with Infisical.
* STS Endpoint (default is `https://sts.amazonaws.com/`): The endpoint URL for the AWS STS API. This value should be adjusted based on the AWS region you are operating in (e.g. `https://sts.us-east-1.amazonaws.com/`); refer to the list of regional STS endpoints [here](https://docs.aws.amazon.com/general/latest/gr/sts.html).
* Access Token TTL (default is `2592000` equivalent to 30 days): The lifetime for an acccess token in seconds. This value will be referenced at renewal time.
* Access Token Max TTL (default is `2592000` equivalent to 30 days): The maximum lifetime for an acccess token in seconds. This value will be referenced at renewal time.
* Access Token Max Number of Uses (default is `0`): The maximum number of times that an access token can be used; a value of `0` implies infinite number of uses.
* Access Token Trusted IPs: The IPs or CIDR ranges that access tokens can be used from. By default, each token is given the `0.0.0.0/0`, allowing usage from any network address.
To enable the identity to access project-level resources such as secrets within a specific project, you should add it to that project.
To do this, head over to the project you want to add the identity to and go to Project Settings > Access Control > Machine Identities and press **Add identity**.
Next, select the identity you want to add to the project and the project level role you want to allow it to assume. The project role assigned will determine what project level resources this identity can have access to.
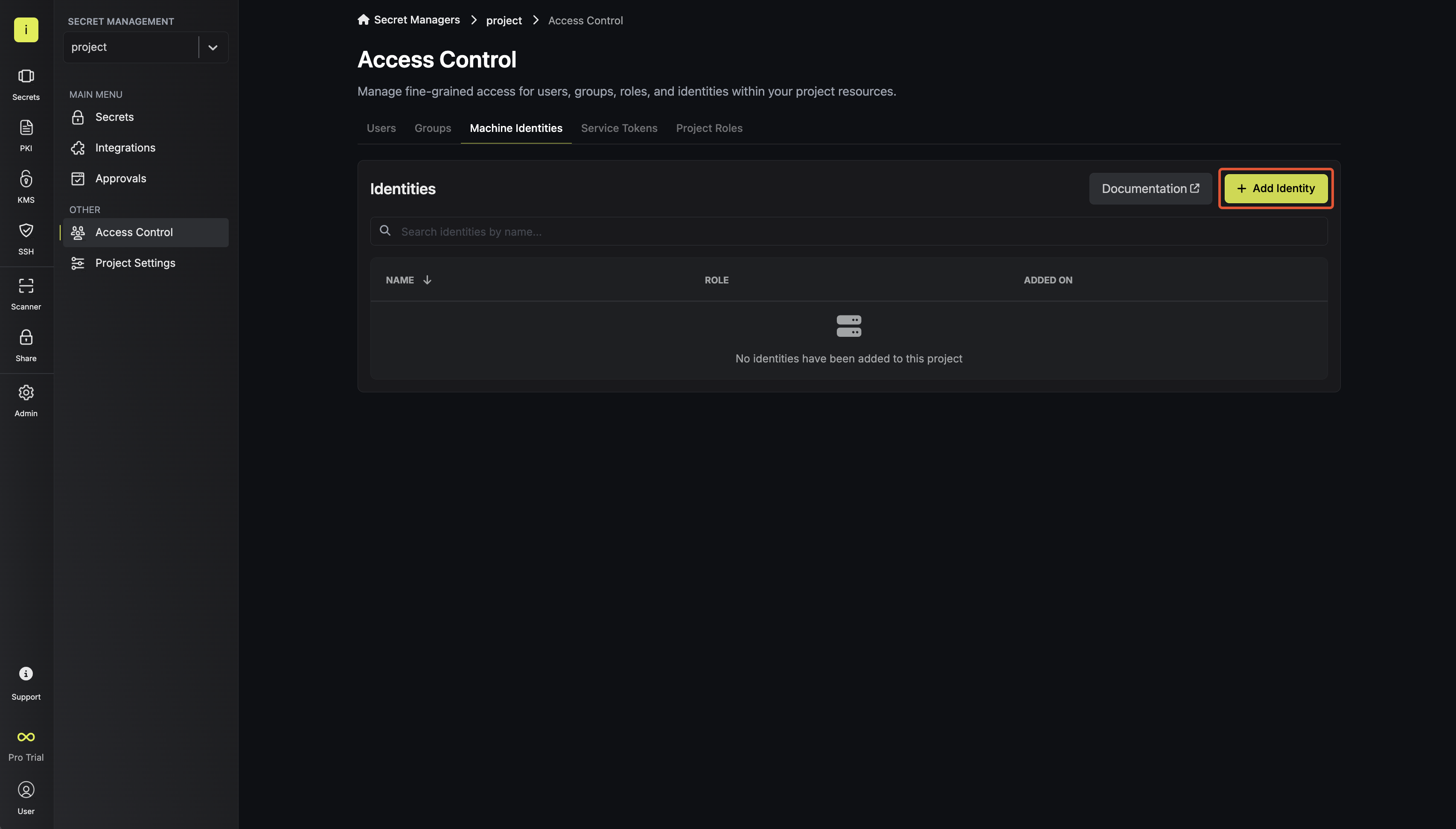
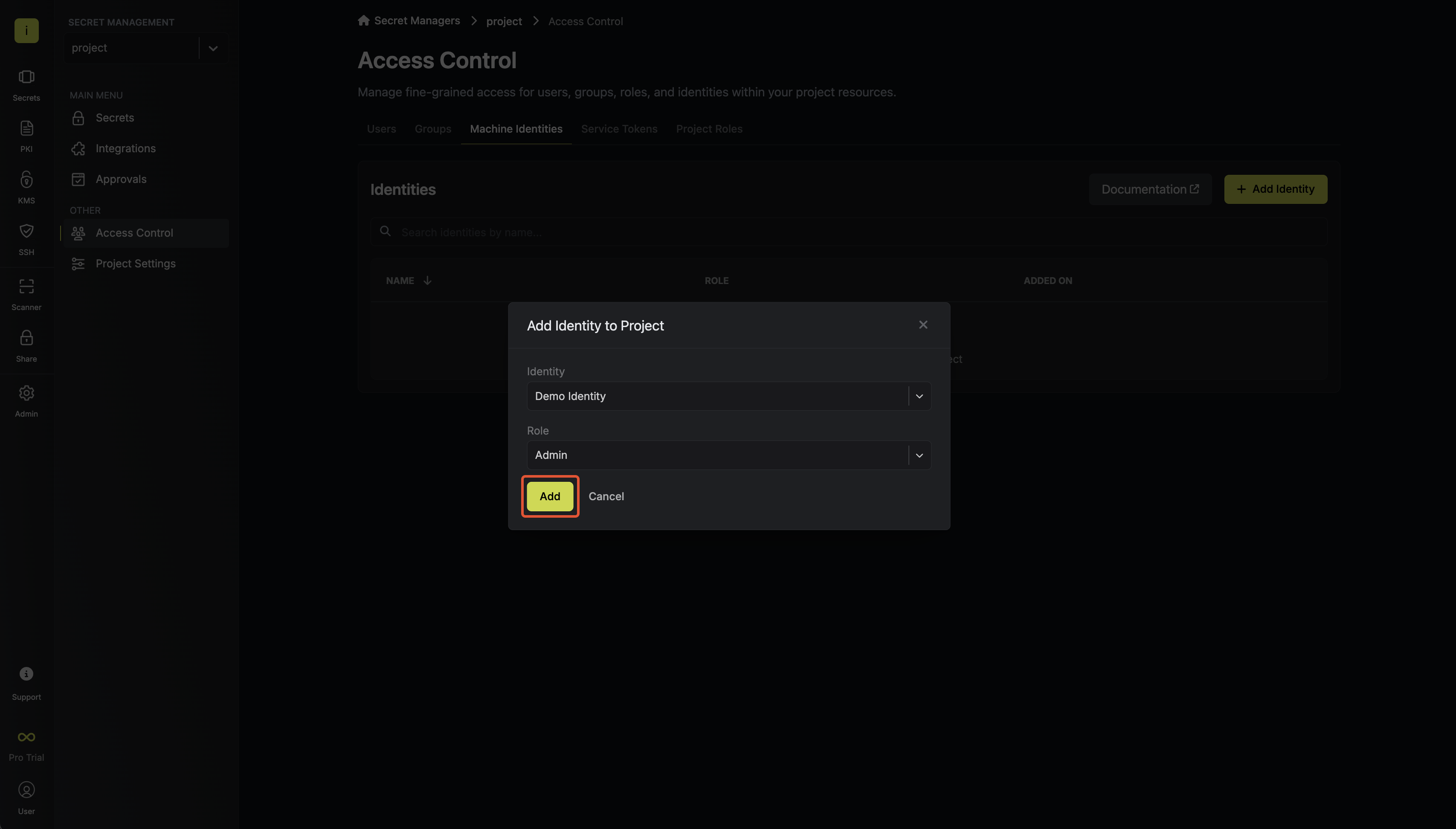
To access the Infisical API as the identity, you need to construct a signed `GetCallerIdentity` query using the [AWS Signature v4 algorithm](https://docs.aws.amazon.com/IAM/latest/UserGuide/create-signed-request.html) and make a request to the `/api/v1/auth/aws-auth/login` endpoint containing the query data
in exchange for an access token.
We provide a few code examples below of how you can authenticate with Infisical from inside a Lambda function, EC2 instance, etc. and obtain an access token to access the [Infisical API](/api-reference/overview/introduction).
The following query construction is an example of how you can authenticate with Infisical from inside a Lambda function.
The shown example uses Node.js but you can use other languages supported by AWS Lambda.
```javascript
import AWS from "aws-sdk";
import axios from "axios";
export const handler = async (event, context) => {
try {
const region = process.env.AWS_REGION;
AWS.config.update({ region });
const iamRequestURL = `https://sts.${region}.amazonaws.com/`;
const iamRequestBody = "Action=GetCallerIdentity&Version=2011-06-15";
const iamRequestHeaders = {
"Content-Type": "application/x-www-form-urlencoded; charset=utf-8",
Host: `sts.${region}.amazonaws.com`,
};
// Create the request
const request = new AWS.HttpRequest(iamRequestURL, region);
request.method = "POST";
request.headers = iamRequestHeaders;
request.headers["X-Amz-Date"] = AWS.util.date
.iso8601(new Date())
.replace(/[:-]|\.\d{3}/g, "");
request.body = iamRequestBody;
request.headers["Content-Length"] =
Buffer.byteLength(iamRequestBody).toString();
// Sign the request
const signer = new AWS.Signers.V4(request, "sts");
signer.addAuthorization(AWS.config.credentials, new Date());
const infisicalUrl = "https://app.infisical.com"; // or your self-hosted Infisical URL
const identityId = "";
const { data } = await axios.post(
`${infisicalUrl}/api/v1/auth/aws-auth/login`,
{
identityId,
iamHttpRequestMethod: "POST",
iamRequestUrl: Buffer.from(iamRequestURL).toString("base64"),
iamRequestBody: Buffer.from(iamRequestBody).toString("base64"),
iamRequestHeaders: Buffer.from(
JSON.stringify(iamRequestHeaders)
).toString("base64"),
}
);
console.log("result data: ", data); // access token here
} catch (err) {
console.error(err);
}
};
```
The following query construction is an example of how you can authenticate with Infisical from inside a EC2 instance.
The shown example uses Node.js but you can use other language you wish.
```javascript
import AWS from "aws-sdk";
import axios from "axios";
const main = async () => {
try {
// obtain region from EC2 instance metadata
const tokenResponse = await axios.put("http://169.254.169.254/latest/api/token", null, {
headers: {
"X-aws-ec2-metadata-token-ttl-seconds": "21600"
}
});
const url = "http://169.254.169.254/latest/dynamic/instance-identity/document";
const response = await axios.get(url, {
headers: {
"X-aws-ec2-metadata-token": tokenResponse.data
}
});
const region = response.data.region;
AWS.config.update({
region
});
const iamRequestURL = `https://sts.${region}.amazonaws.com/`;
const iamRequestBody = "Action=GetCallerIdentity&Version=2011-06-15";
const iamRequestHeaders = {
"Content-Type": "application/x-www-form-urlencoded; charset=utf-8",
Host: `sts.${region}.amazonaws.com`
};
const request = new AWS.HttpRequest(new AWS.Endpoint(iamRequestURL), AWS.config.region);
request.method = "POST";
request.headers = iamRequestHeaders;
request.headers["X-Amz-Date"] = AWS.util.date.iso8601(new Date()).replace(/[:-]|\.\d{3}/g, "");
request.body = iamRequestBody;
request.headers["Content-Length"] = Buffer.byteLength(iamRequestBody);
const signer = new AWS.Signers.V4(request, "sts");
signer.addAuthorization(AWS.config.credentials, new Date());
const infisicalUrl = "https://app.infisical.com"; // or your self-hosted Infisical URL
const identityId = "";
const { data } = await axios.post(`${infisicalUrl}/api/v1/auth/aws-auth/login`, {
identityId,
iamHttpRequestMethod: "POST",
iamRequestUrl: Buffer.from(iamRequestURL).toString("base64"),
iamRequestBody: Buffer.from(iamRequestBody).toString("base64"),
iamRequestHeaders: Buffer.from(JSON.stringify(iamRequestHeaders)).toString("base64")
});
console.log("result data: ", data); // access token here
} catch (err) {
console.error(err);
}
}
main();
```
The following query construction provides a generic example of how you can construct a signed `GetCallerIdentity` query and obtain the required payload components.
The shown example uses Node.js but you can use any language you wish.
```javascript
const AWS = require("aws-sdk");
const region = "";
const infisicalUrl = "https://app.infisical.com"; // or your self-hosted Infisical URL
const iamRequestURL = `https://sts.${region}.amazonaws.com/`;
const iamRequestBody = "Action=GetCallerIdentity&Version=2011-06-15";
const iamRequestHeaders = {
"Content-Type": "application/x-www-form-urlencoded; charset=utf-8",
Host: `sts.${region}.amazonaws.com`
};
const request = new AWS.HttpRequest(new AWS.Endpoint(iamRequestURL), region);
request.method = "POST";
request.headers = iamRequestHeaders;
request.headers["X-Amz-Date"] = AWS.util.date.iso8601(new Date()).replace(/[:-]|\.\d{3}/g, "");
request.body = iamRequestBody;
request.headers["Content-Length"] = Buffer.byteLength(iamRequestBody);
const signer = new AWS.Signers.V4(request, "sts");
signer.addAuthorization(AWS.config.credentials, new Date());
```
#### Sample request
```bash Request
curl --location --request POST 'https://app.infisical.com/api/v1/auth/aws-auth/login' \
--header 'Content-Type: application/x-www-form-urlencoded' \
--data-urlencode 'identityId=...' \
--data-urlencode 'iamHttpRequestMethod=...' \
--data-urlencode 'iamRequestBody=...' \
--data-urlencode 'iamRequestHeaders=...'
```
Note that you should replace `` with the ID of the identity you created in step 1.
#### Sample response
```bash Response
{
"accessToken": "...",
"expiresIn": 7200,
"accessTokenMaxTTL": 43244
"tokenType": "Bearer"
}
```
Next, you can use the access token to access the [Infisical API](/api-reference/overview/introduction)
We recommend using one of Infisical's clients like SDKs or the Infisical Agent to authenticate with Infisical using AWS Auth as they handle the authentication process including the signed `GetCallerIdentity` query construction for you.
Each identity access token has a time-to-live (TLL) which you can infer from the response of the login operation;
the default TTL is `7200` seconds which can be adjusted.
If an identity access token expires, it can no longer authenticate with the Infisical API. In this case,
a new access token should be obtained by performing another login operation.
# Azure Auth
Learn how to authenticate with Infisical for services on Azure
**Azure Auth** is an Azure-native authentication method for Azure resources like Azure VMs, Azure App Services, Azure Functions, Azure Kubernetes Service, etc. to access Infisical.
## Diagram
The following sequence diagram illustrates the Azure Auth workflow for authenticating Azure [service principals](https://learn.microsoft.com/en-us/entra/identity-platform/app-objects-and-service-principals?tabs=browser) with Infisical.
```mermaid
sequenceDiagram
participant Client as Client
participant Infis as Infisical
participant Azure as Azure AD OpenID
Note over Client,Azure: Step 1: Instance Identity Token Retrieval
Client->>Azure: Request managed identity access token
Azure-->>Client: Return managed identity access token
Note over Client,Infis: Step 2: Identity Token Login Operation
Client->>Infis: Send managed identity access token to /api/v1/auth/azure-auth/login
Infis->>Azure: Request public key
Azure-->>Infis: Return public key
Note over Infis: Step 3: Identity Token Verification
Note over Infis: Step 4: Identity Property Validation
Infis->>Client: Return short-lived access token
Note over Client,Infis: Step 4: Access Infisical API with Token
Client->>Infis: Make authenticated requests using the short-lived access token
```
## Concept
At a high-level, Infisical authenticates an Azure service by verifying its identity and checking that it meets specific requirements (e.g. it is bound to an allowed service principal) at the `/api/v1/auth/azure-auth/login` endpoint. If successful,
then Infisical returns a short-lived access token that can be used to make authenticated requests to the Infisical API.
To be more specific:
1. The client running on an Azure service obtains an [access token](https://learn.microsoft.com/en-us/entra/identity/managed-identities-azure-resources/how-to-use-vm-token#get-a-token-using-http) that is a JWT token representing the managed identity for the Azure resource such as a Virtual Machine; the managed identity is associated with a service principal in Azure AD.
2. The client sends the access token to Infisical.
3. Infisical verifies the token against the corresponding public key at the [public Azure AD OpenID configuration endpoint](https://learn.microsoft.com/en-us/answers/questions/793793/azure-ad-validate-access-token).
4. Infisical checks if the entity behind the access token is allowed to authenticate with Infisical based on set criteria such as **Allowed Service Principal IDs**.
5. If all is well, Infisical returns a short-lived access token that the client can use to make authenticated requests to the Infisical API.
We recommend using one of Infisical's clients like SDKs or the Infisical Agent
to authenticate with Infisical using Azure Auth as they handle the
authentication process including generating the client access token for you.
Also, note that Infisical needs network-level access to send requests to the Google Cloud API
as part of the Azure Auth workflow.
## Guide
In the following steps, we explore how to create and use identities for your applications in Azure to
access the Infisical API using the Azure Auth authentication method.
To create an identity, head to your Organization Settings > Access Control > Machine Identities and press **Create identity**.
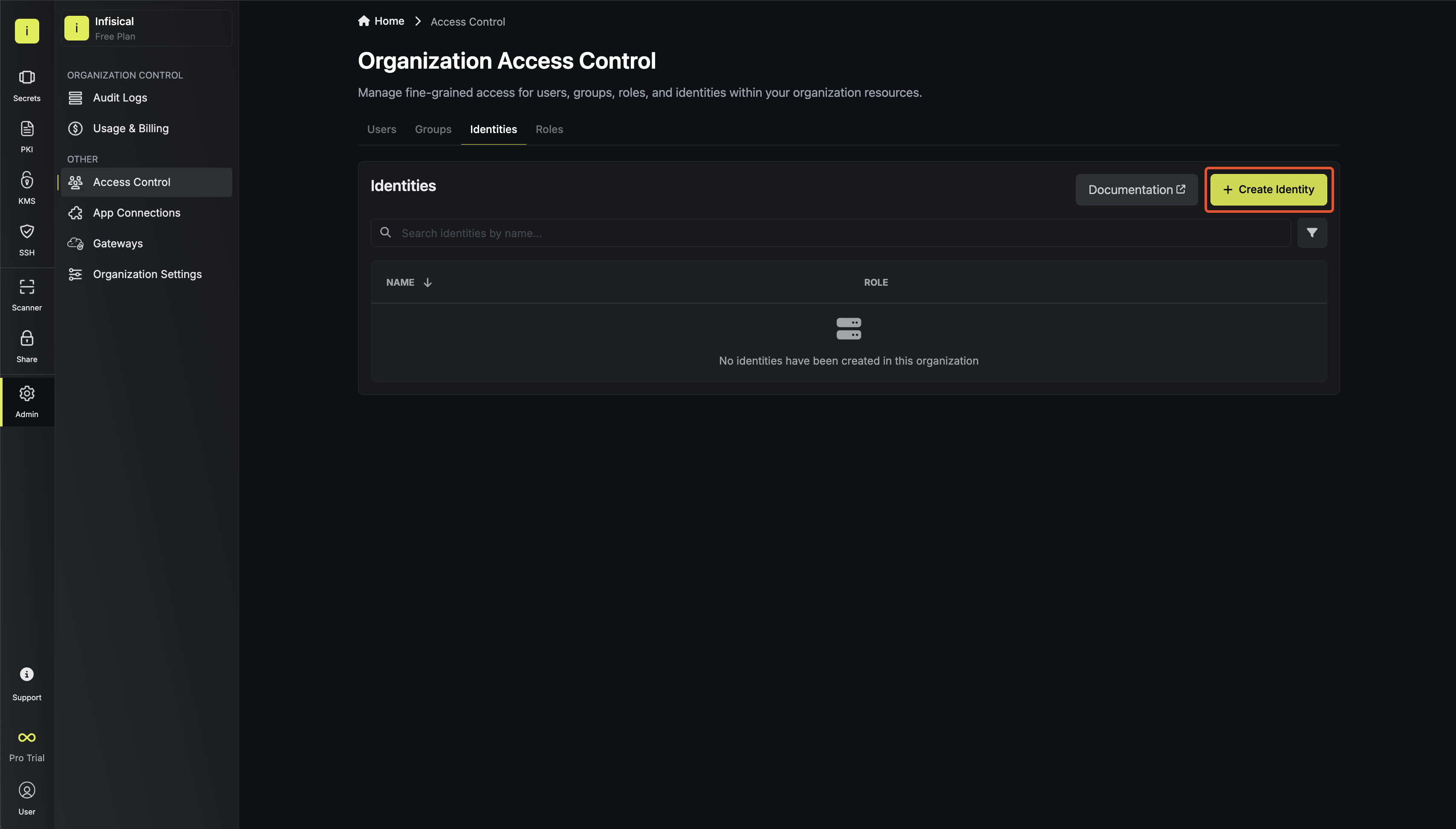
When creating an identity, you specify an organization level [role](/documentation/platform/role-based-access-controls) for it to assume; you can configure roles in Organization Settings > Access Control > Organization Roles.
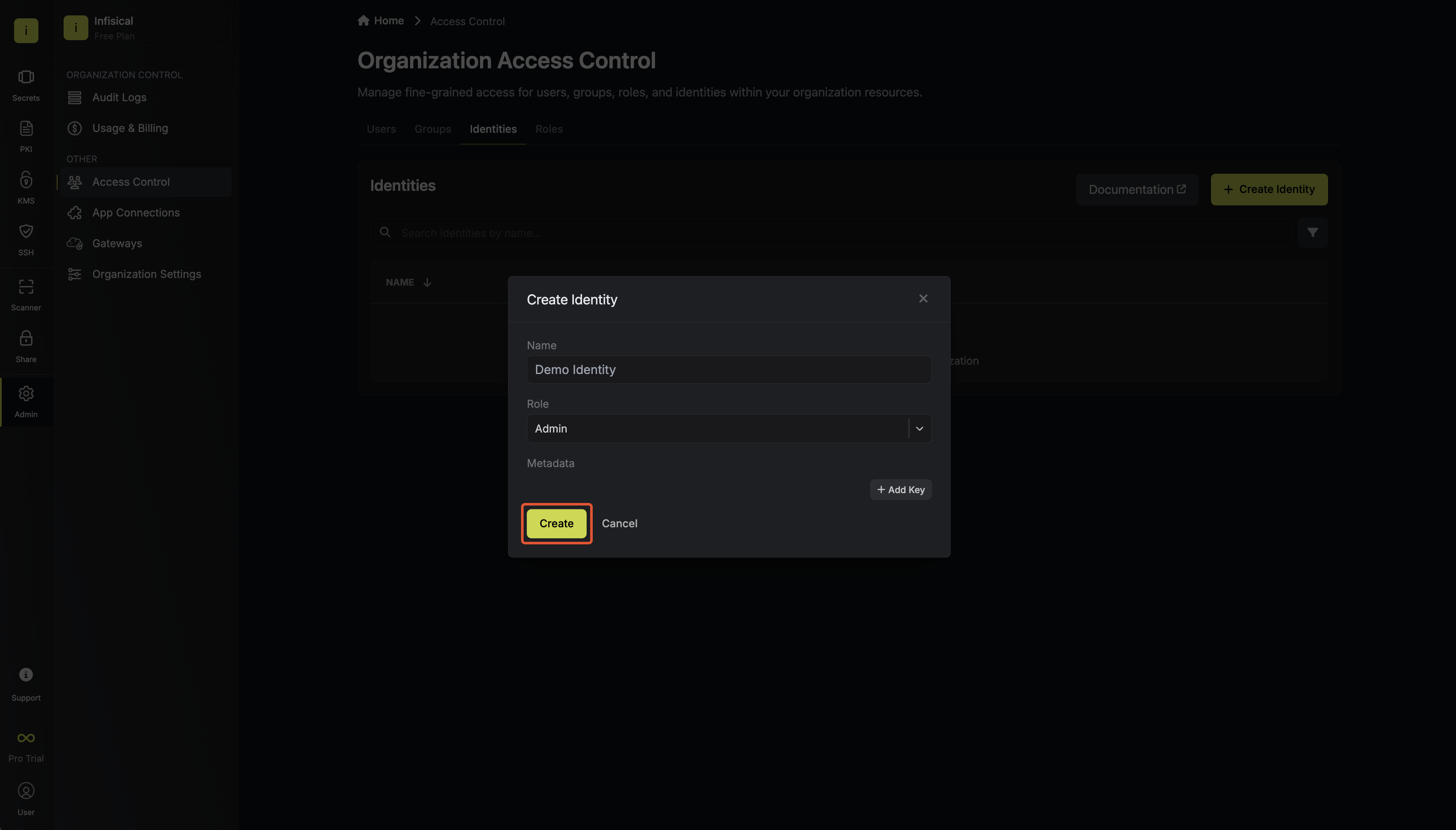
Now input a few details for your new identity. Here's some guidance for each field:
* Name (required): A friendly name for the identity.
* Role (required): A role from the **Organization Roles** tab for the identity to assume. The organization role assigned will determine what organization level resources this identity can have access to.
Once you've created an identity, you'll be redirected to a page where you can manage the identity.
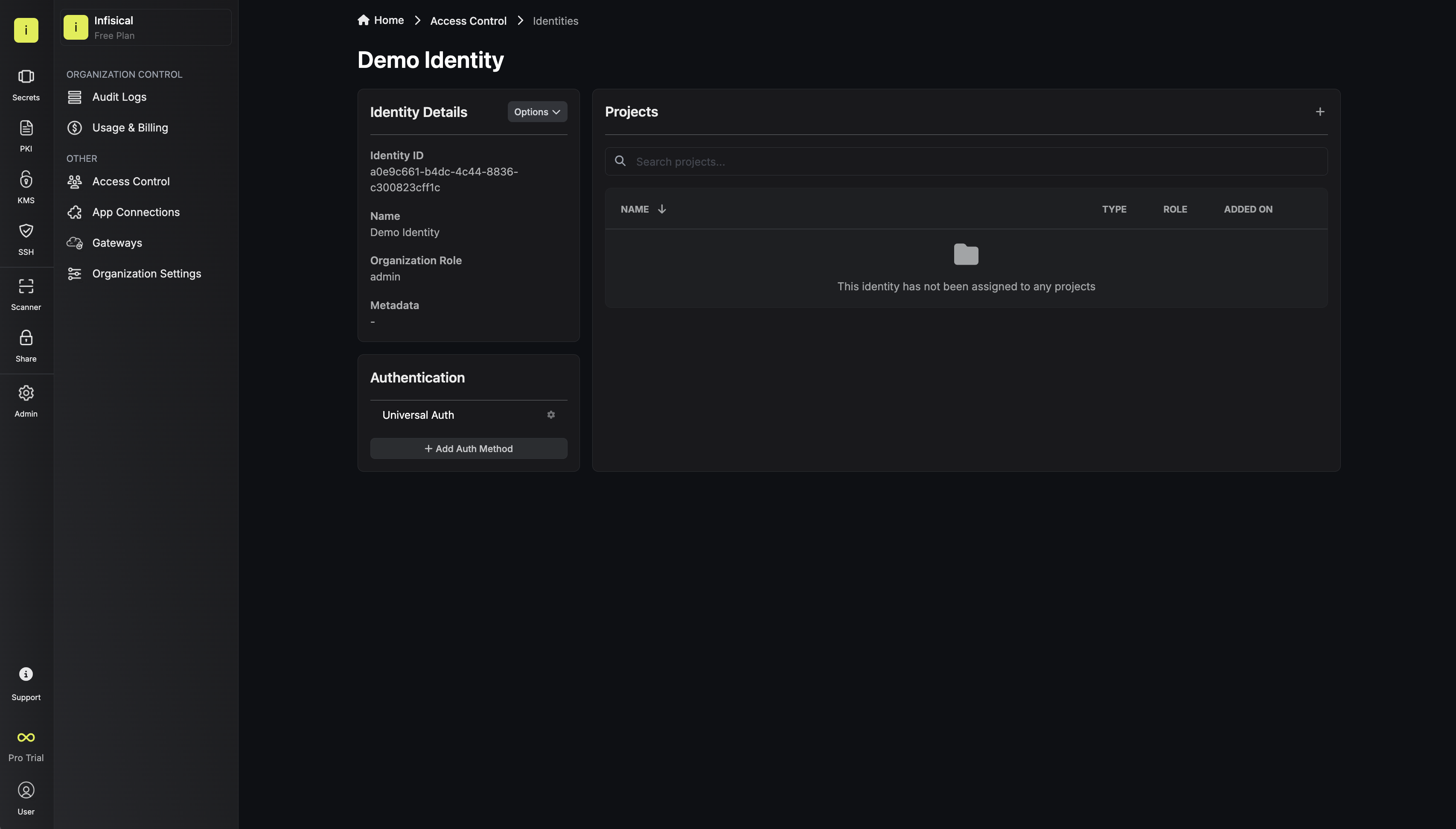
Since the identity has been configured with Universal Auth by default, you should re-configure it to use Azure Auth instead. To do this, press to edit the **Authentication** section,
remove the existing Universal Auth configuration, and add a new Azure Auth configuration onto the identity.
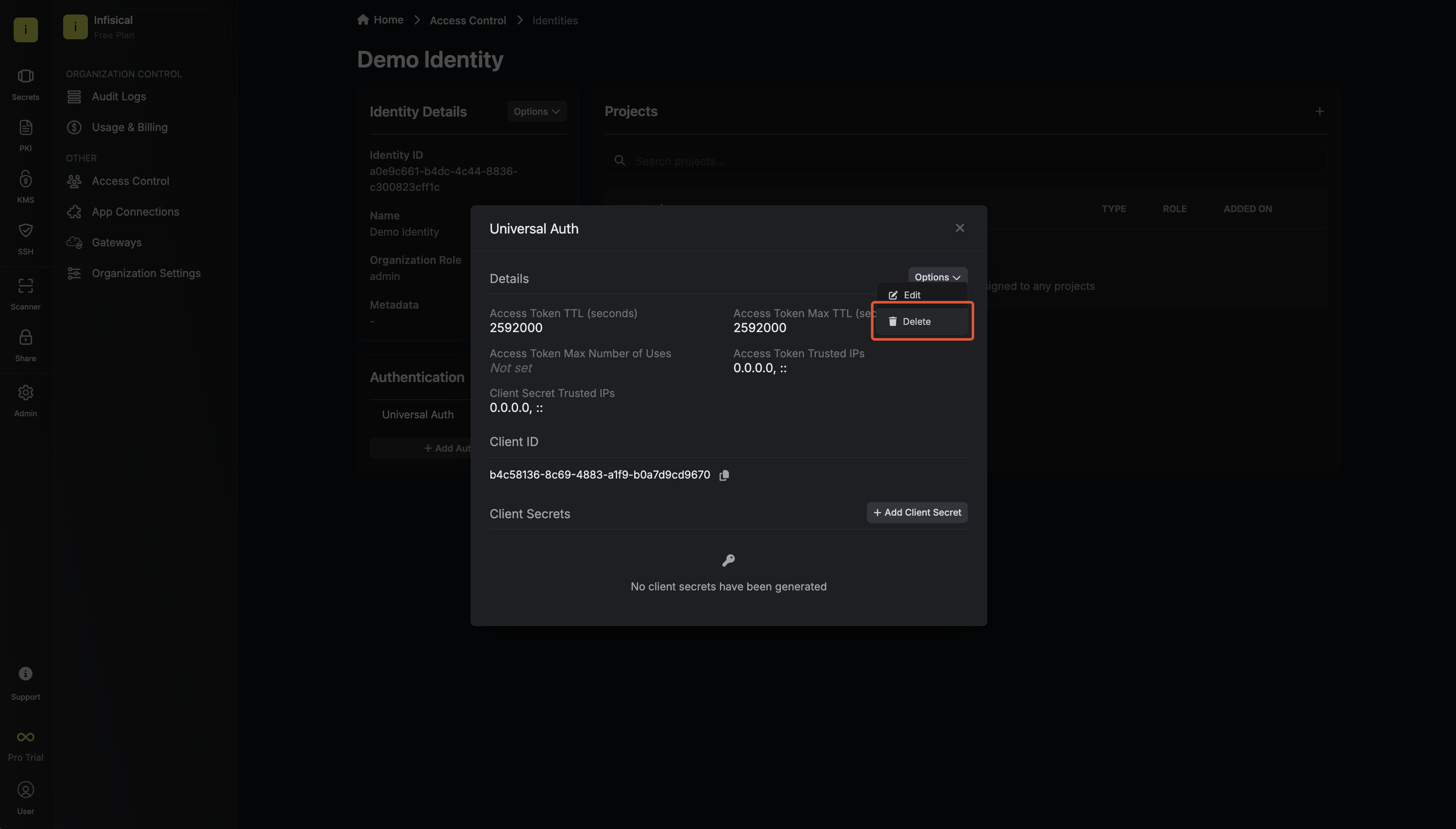
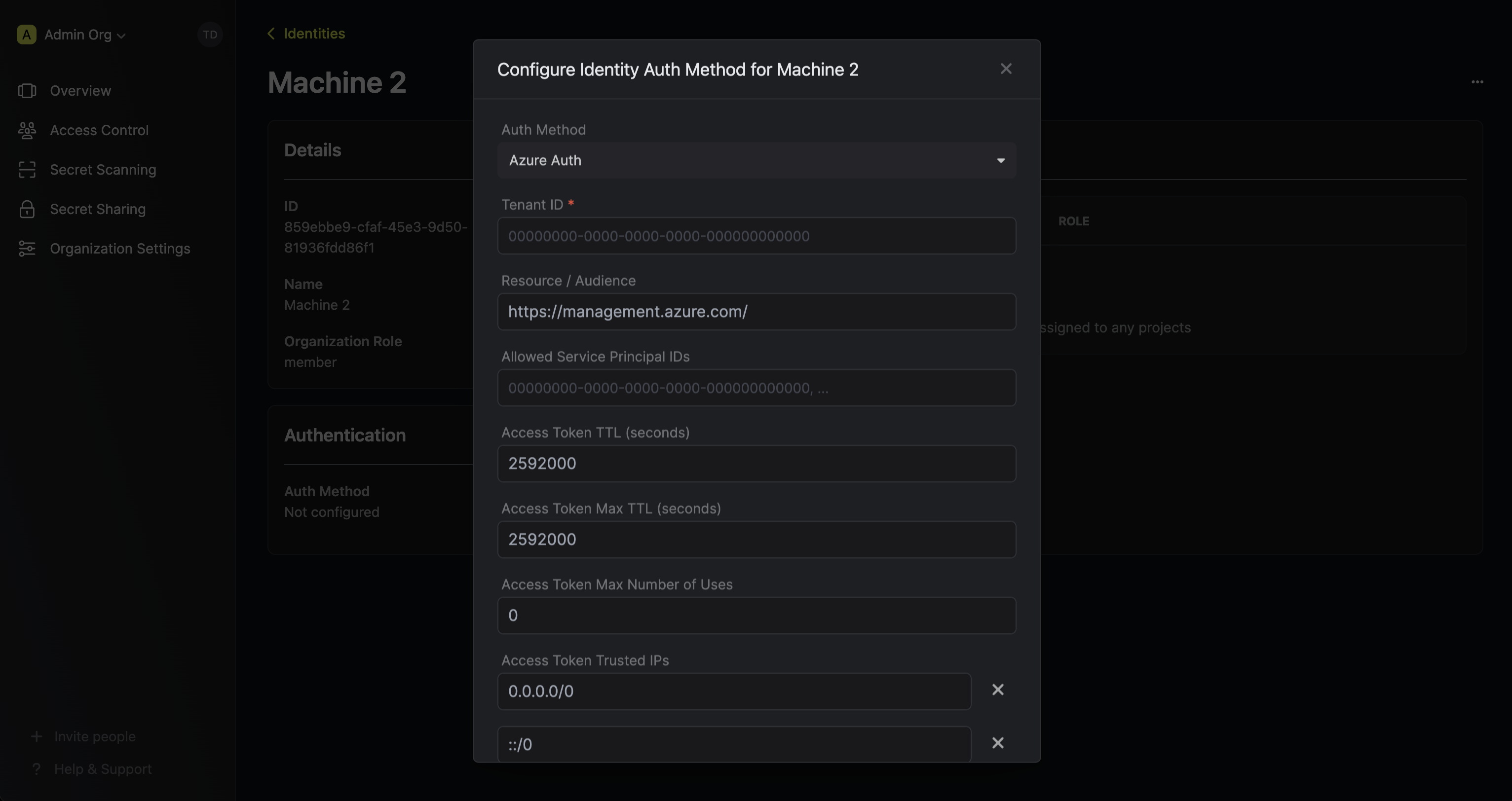
Here's some more guidance on each field:
* Tenant ID: The [tenant ID](https://learn.microsoft.com/en-us/entra/fundamentals/how-to-find-tenant) for the Azure AD organization.
* Resource / Audience: The resource URL for the application registered in Azure AD. The value is expected to match the `aud` claim of the access token JWT later used in the login operation against Infisical. See the [resource](https://learn.microsoft.com/en-us/entra/identity/managed-identities-azure-resources/how-to-use-vm-token#get-a-token-using-http) parameter for how the audience is set when requesting a JWT access token from the Azure Instance Metadata Service (IMDS) endpoint. In most cases, this value should be `https://management.azure.com/` which is the default.
* Allowed Service Principal IDs: A comma-separated list of Azure AD service principal IDs that are allowed to authenticate with Infisical.
* Access Token TTL (default is `2592000` equivalent to 30 days): The lifetime for an acccess token in seconds. This value will be referenced at renewal time.
* Access Token Max TTL (default is `2592000` equivalent to 30 days): The maximum lifetime for an acccess token in seconds. This value will be referenced at renewal time.
* Access Token Max Number of Uses (default is `0`): The maximum number of times that an access token can be used; a value of `0` implies infinite number of uses.
* Access Token Trusted IPs: The IPs or CIDR ranges that access tokens can be used from. By default, each token is given the `0.0.0.0/0`, allowing usage from any network address.
To enable the identity to access project-level resources such as secrets within a specific project, you should add it to that project.
To do this, head over to the project you want to add the identity to and go to Project Settings > Access Control > Machine Identities and press **Add identity**.
Next, select the identity you want to add to the project and the project level role you want to allow it to assume. The project role assigned will determine what project level resources this identity can have access to.
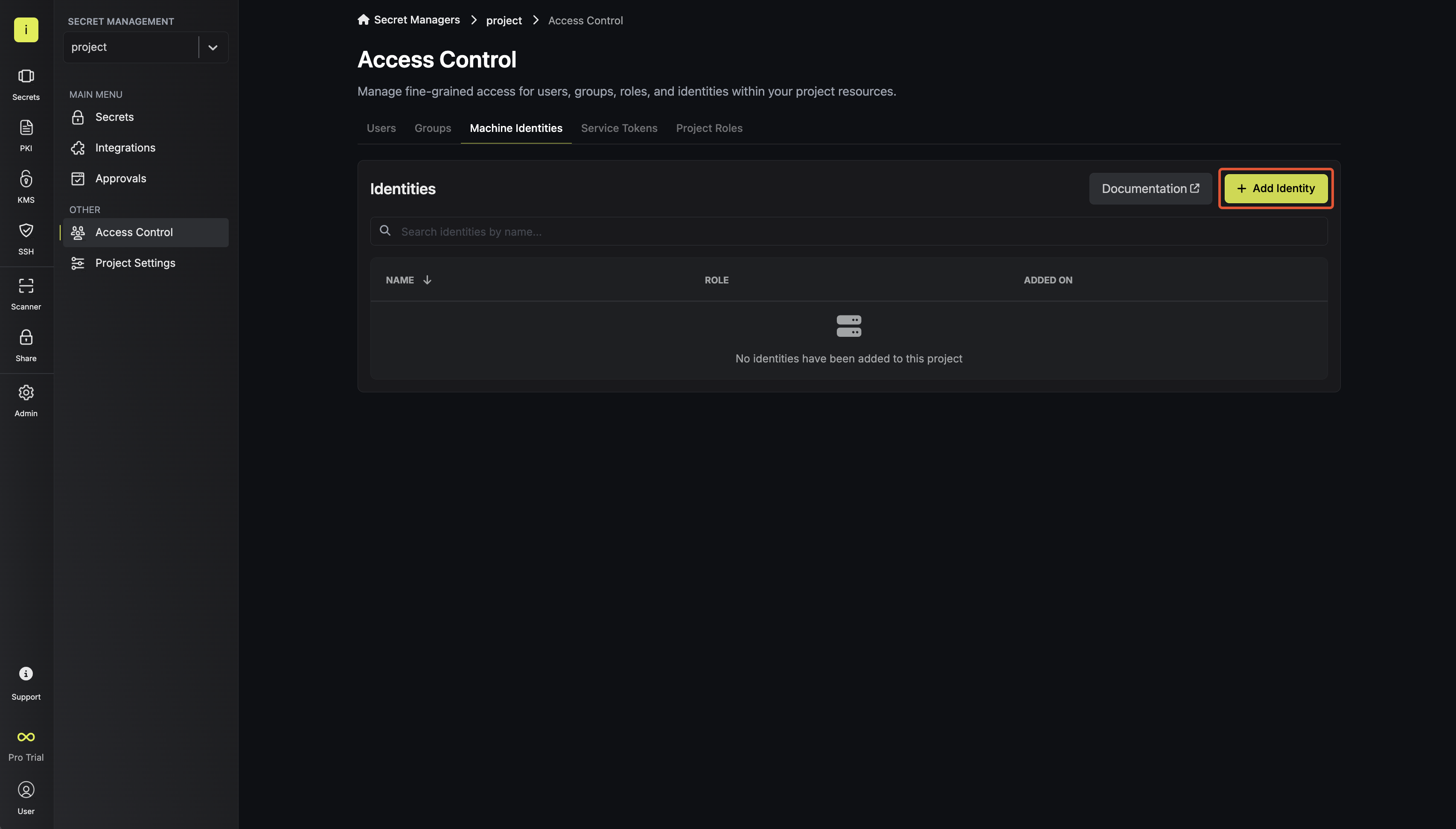
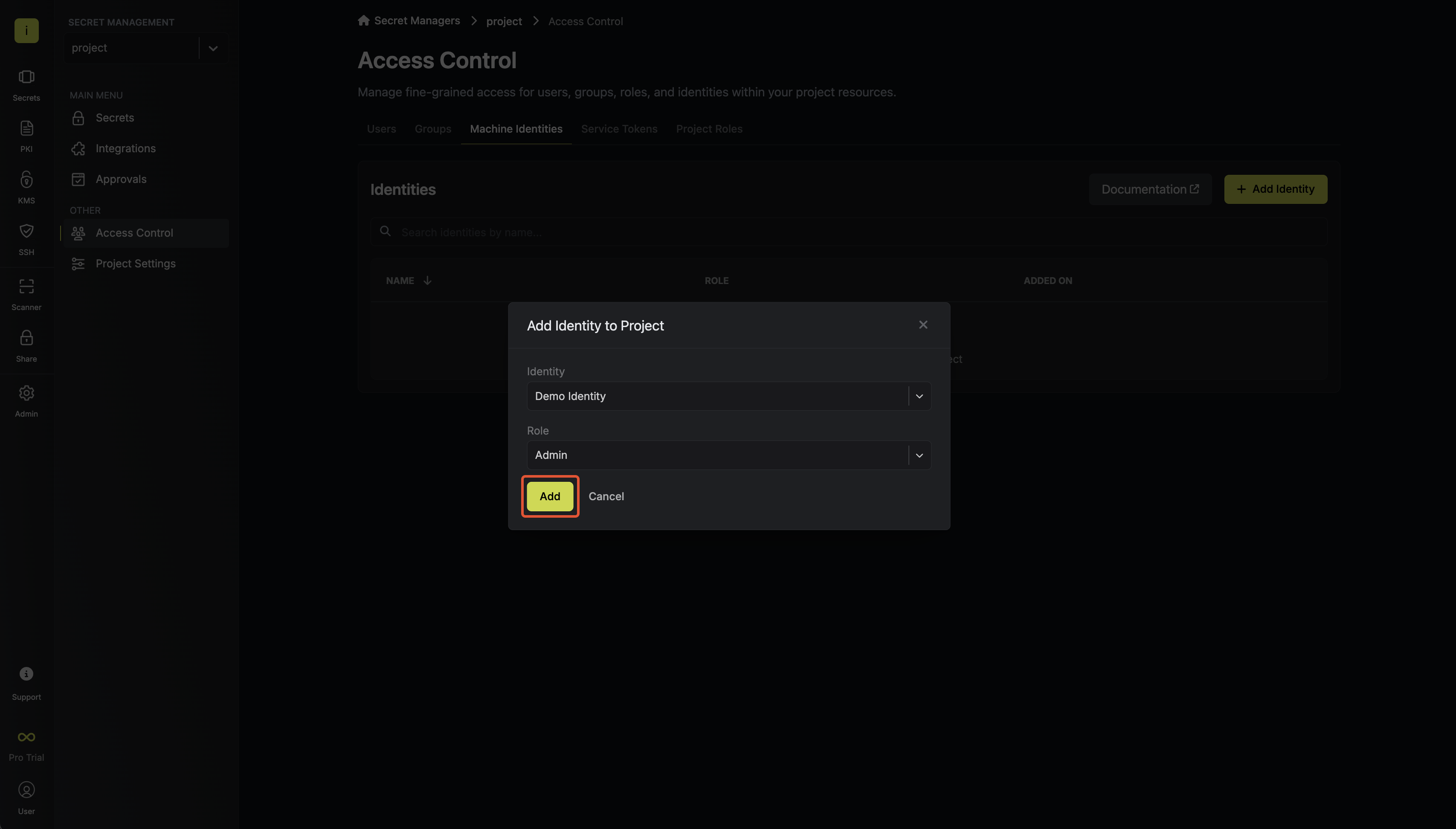
To access the Infisical API as the identity, you need to generate a managed identity [access token](https://learn.microsoft.com/en-us/entra/identity/managed-identities-azure-resources/how-to-use-vm-token#get-a-token-using-http) that is a JWT token representing the managed identity for the Azure resource such as a Virtual Machine. The client token must be sent to the `/api/v1/auth/azure-auth/login` endpoint in exchange for a separate access token to access the Infisical API.
We provide a few code examples below of how you can authenticate with Infisical to access the [Infisical API](/api-reference/overview/introduction).
Start by making a request from your Azure client such as Virtual Machine to obtain a managed identity access token.
For more examples of how to obtain the managed identity access token, refer to the [official documentation](https://learn.microsoft.com/en-us/entra/identity/managed-identities-azure-resources/how-to-use-vm-token#get-a-token-using-http).
#### Sample request
```bash curl
curl 'http://169.254.169.254/metadata/identity/oauth2/token?api-version=2018-02-01&resource=https%3A%2F%2Fmanagement.azure.com%2F' -H Metadata:true -s
```
#### Sample response
```bash
{
"access_token": "eyJ0eXAi...",
"refresh_token": "",
"expires_in": "3599",
"expires_on": "1506484173",
"not_before": "1506480273",
"resource": "https://management.azure.com/",
"token_type": "Bearer"
}
```
Next use send the obtained managed identity access token (i.e. the token from the `access_token` field above) to authenticate with Infisical and obtain a separate access token.
#### Sample request
```bash Request
curl --location --request POST 'https://app.infisical.com/api/v1/auth/gcp-auth/login' \
--header 'Content-Type: application/x-www-form-urlencoded' \
--data-urlencode 'identityId=...' \
--data-urlencode 'jwt=...'
```
Note that you should replace `` with the ID of the identity you created in step 1.
#### Sample response
```bash Response
{
"accessToken": "...",
"expiresIn": 7200,
"accessTokenMaxTTL": 43244
"tokenType": "Bearer"
}
```
Next, you can use this access token to access the [Infisical API](/api-reference/overview/introduction)
We recommend using one of Infisical's clients like SDKs or the Infisical Agent to authenticate with Infisical using Azure Auth as they handle the authentication process including retrieving the client access token.
Each identity access token has a time-to-live (TLL) which you can infer from the response of the login operation;
the default TTL is `7200` seconds which can be adjusted.
If an identity access token expires, it can no longer authenticate with the Infisical API. In this case,
a new access token should be obtained by performing another login operation.
# GCP Auth
Learn how to authenticate with Infisical for services on Google Cloud Platform
**GCP Auth** is a GCP-native authentication method for GCP resources to access Infisical. It consists of two sub-methods/approaches:
* GCP ID Token Auth: For GCP services including [Compute Engine](https://cloud.google.com/compute/docs/instances/verifying-instance-identity#request_signature), [App Engine standard environment](https://cloud.google.com/appengine/docs/standard/python3/runtime#metadata_server), [App Engine flexible environment](https://cloud.google.com/appengine/docs/flexible/python/runtime#metadata_server), [Cloud Functions](https://cloud.google.com/functions/docs/securing/function-identity#using_the_metadata_server_to_acquire_tokens), [Cloud Run](https://cloud.google.com/run/docs/container-contract#metadata-server), [Google Kubernetes Engine](https://cloud.google.com/kubernetes-engine/docs/concepts/workload-identity#instance_metadata), and [Cloud Build](https://cloud.google.com/kubernetes-engine/docs/concepts/workload-identity#instance_metadata) to authenticate with Infisical.
* GCP IAM Auth: For Google Cloud Platform (GCP) service accounts to authenticate with Infisical.
## Diagram
The following sequence diagram illustrates the GCP ID Token Auth workflow for authenticating GCP resources with Infisical.
```mermaid
sequenceDiagram
participant GCE as GCP Service
participant Infis as Infisical
participant Google as OAuth2 API
Note over GCE,Google: Step 1: Instance Identity Token Retrieval
GCE->>Google: Request instance identity metadata token
Google-->>GCE: Return JWT token with RS256 signature
Note over GCE,Infis: Step 2: Identity Token Login Operation
GCE->>Infis: Send JWT token to /api/v1/auth/gcp-auth/login
Infis->>Google: Request OAuth2 certificates
Google-->>Infis: Return certificates
Note over Infis: Step 3: Identity Token Verification
Note over Infis: Step 4: Identity Property Validation
Infis->>GCE: Return short-lived access token
Note over GCE,Infis: Step 4: Access Infisical API with Token
GCE->>Infis: Make authenticated requests using the short-lived access token
```
## Concept
At a high-level, Infisical authenticates a GCP resource by verifying its identity and checking that it meets specific requirements (e.g. it is an allowed GCE instance) at the `/api/v1/auth/gcp-auth/login` endpoint. If successful,
then Infisical returns a short-lived access token that can be used to make authenticated requests to the Infisical API.
To be more specific:
1. The client running on a GCP service obtains an [ID token](https://cloud.google.com/docs/authentication/get-id-token) constituting the identity for a GCP resource such as a GCE instance or Cloud Function; this is a unique JWT token that includes details about the instance as well as Google's [RS256 signature](https://datatracker.ietf.org/doc/html/rfc7518#section-3.3).
2. The client sends the ID token to Infisical at the `/api/v1/auth/gcp-auth/login` endpoint.
3. Infisical verifies the token against Google's [public OAuth2 certificates](https://www.googleapis.com/oauth2/v3/certs).
4. Infisical checks if the entity behind the ID token is allowed to authenticate with Infisical based on set criteria such as **Allowed Service Account Emails**.
5. If all is well, Infisical returns a short-lived access token that the client can use to make authenticated requests to the Infisical API.
We recommend using one of Infisical's clients like SDKs or the Infisical Agent
to authenticate with Infisical using GCP ID Token Auth as they handle the
authentication process including generating the instance ID token for you.
Also, note that Infisical needs network-level access to send requests to the Google Cloud API
as part of the GCP Auth workflow.
## Guide
In the following steps, we explore how to create and use identities for your workloads and applications on GCP to
access the Infisical API using the GCP ID Token authentication method.
To create an identity, head to your Organization Settings > Access Control > Machine Identities and press **Create identity**.
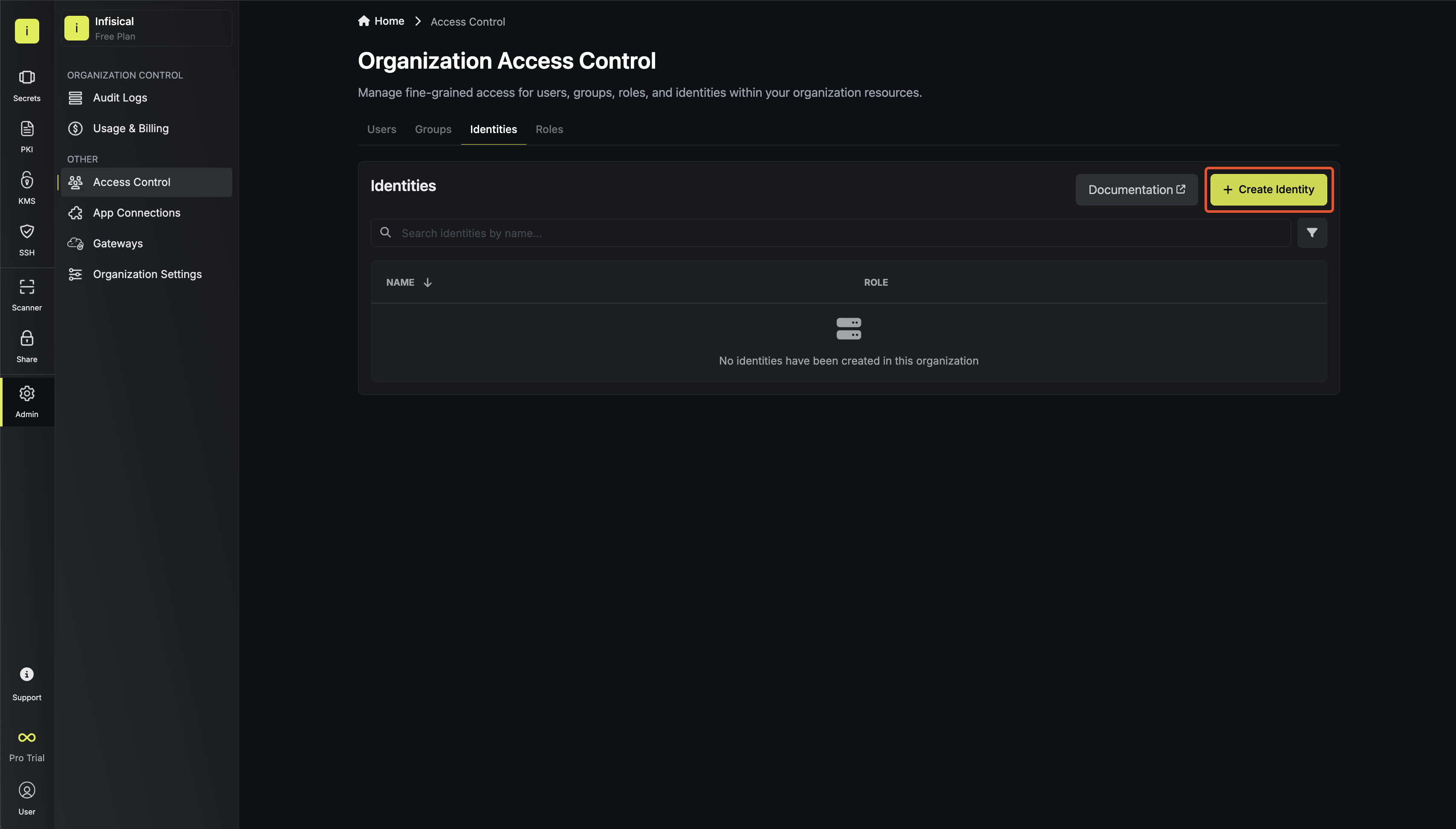
When creating an identity, you specify an organization level [role](/documentation/platform/role-based-access-controls) for it to assume; you can configure roles in Organization Settings > Access Control > Organization Roles.
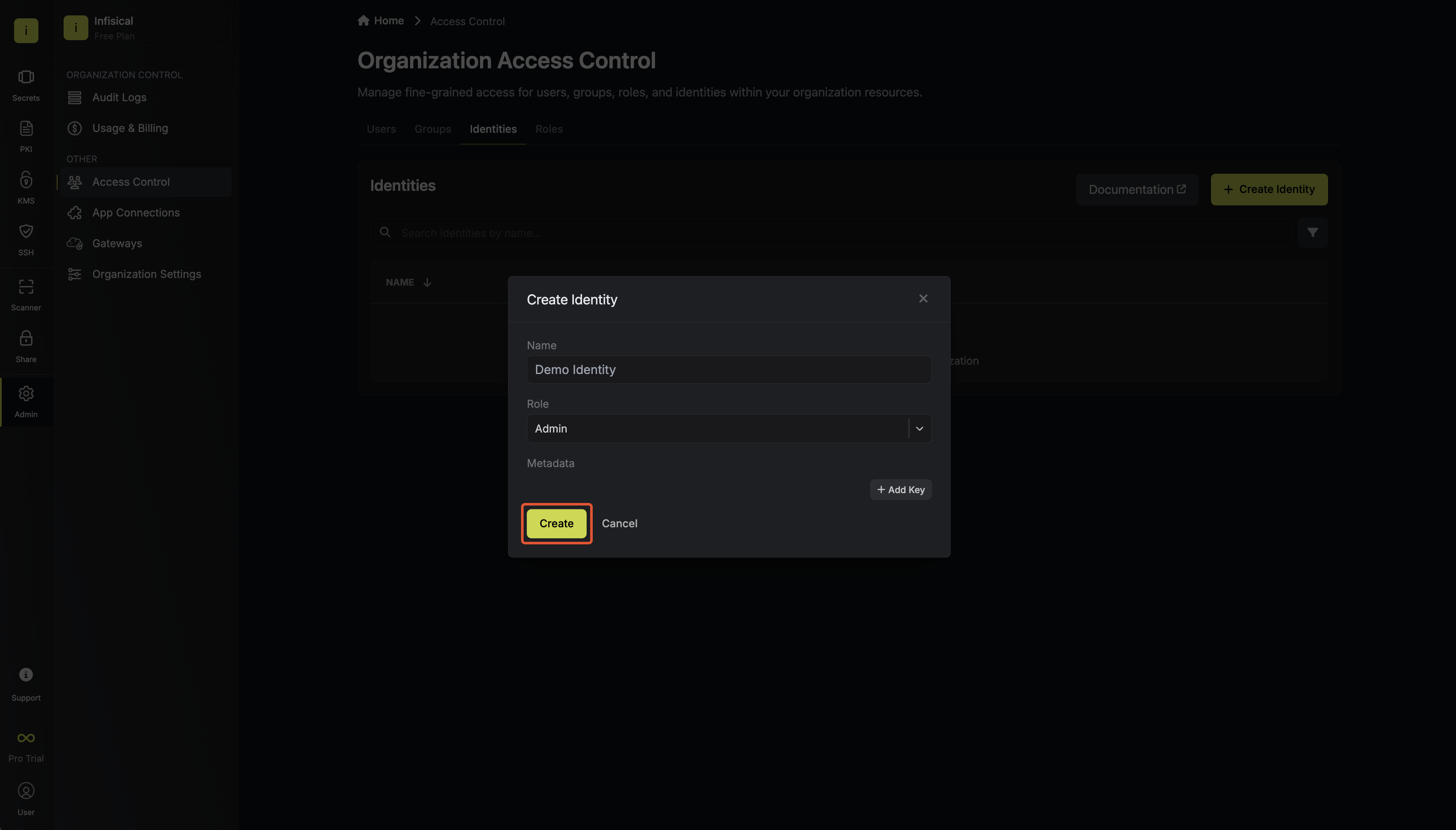
Now input a few details for your new identity. Here's some guidance for each field:
* Name (required): A friendly name for the identity.
* Role (required): A role from the **Organization Roles** tab for the identity to assume. The organization role assigned will determine what organization level resources this identity can have access to.
Once you've created an identity, you'll be redirected to a page where you can manage the identity.
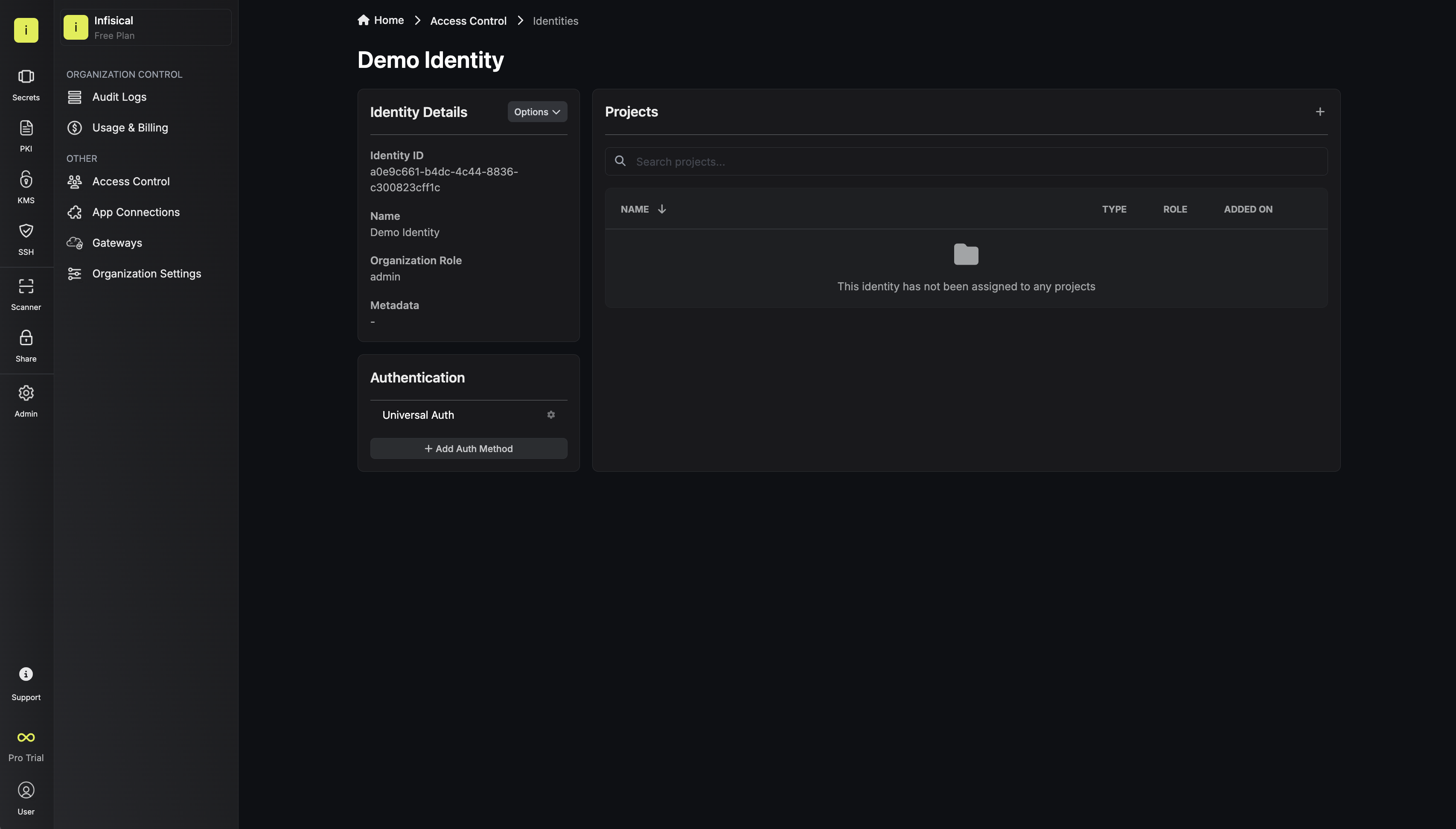
Since the identity has been configured with Universal Auth by default, you should re-configure it to use GCP Auth instead. To do this, press to edit the **Authentication** section,
remove the existing Universal Auth configuration, and add a new GCP Auth configuration onto the identity; set the **Type** field to **GCP ID Token Auth**.
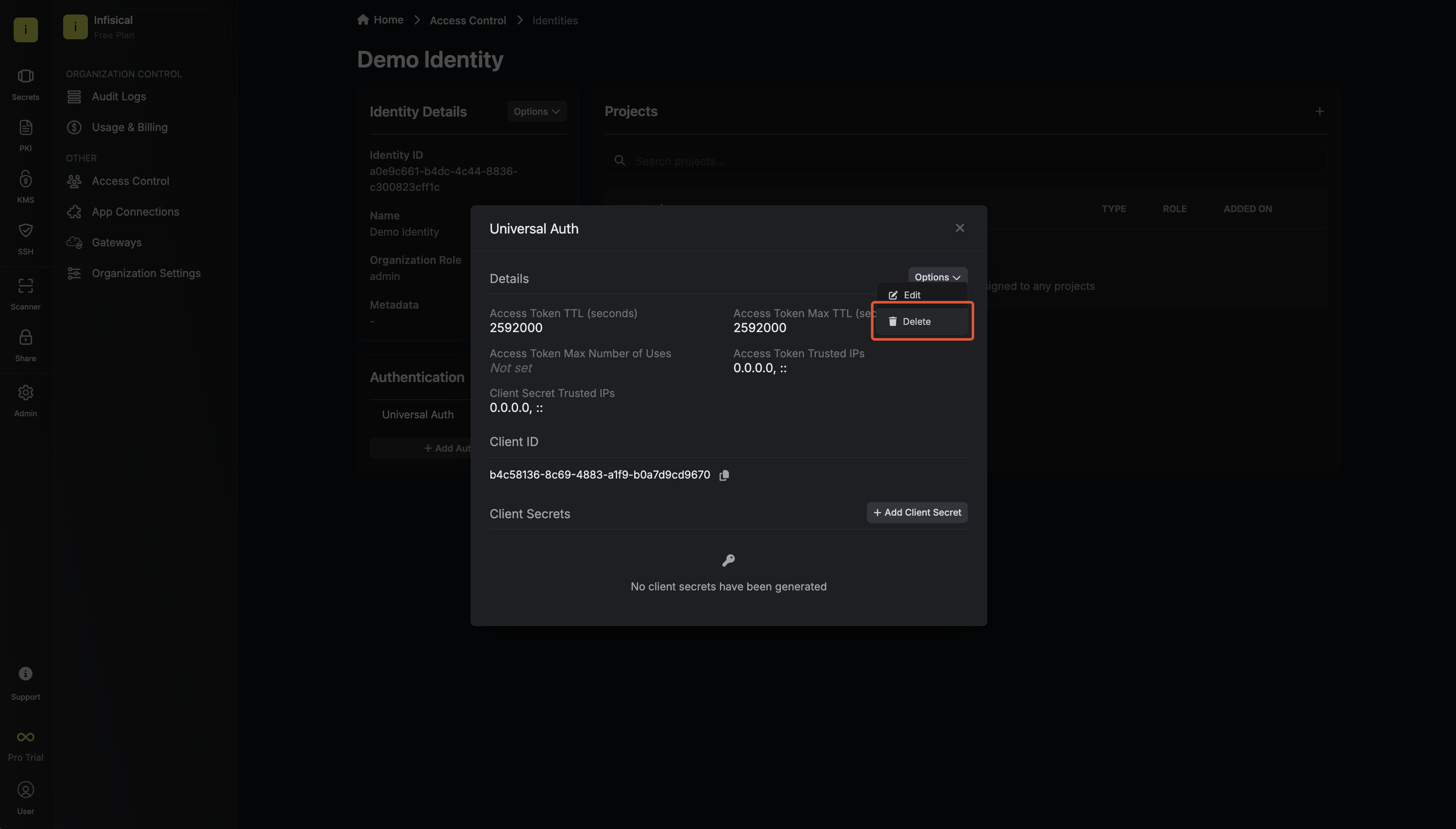
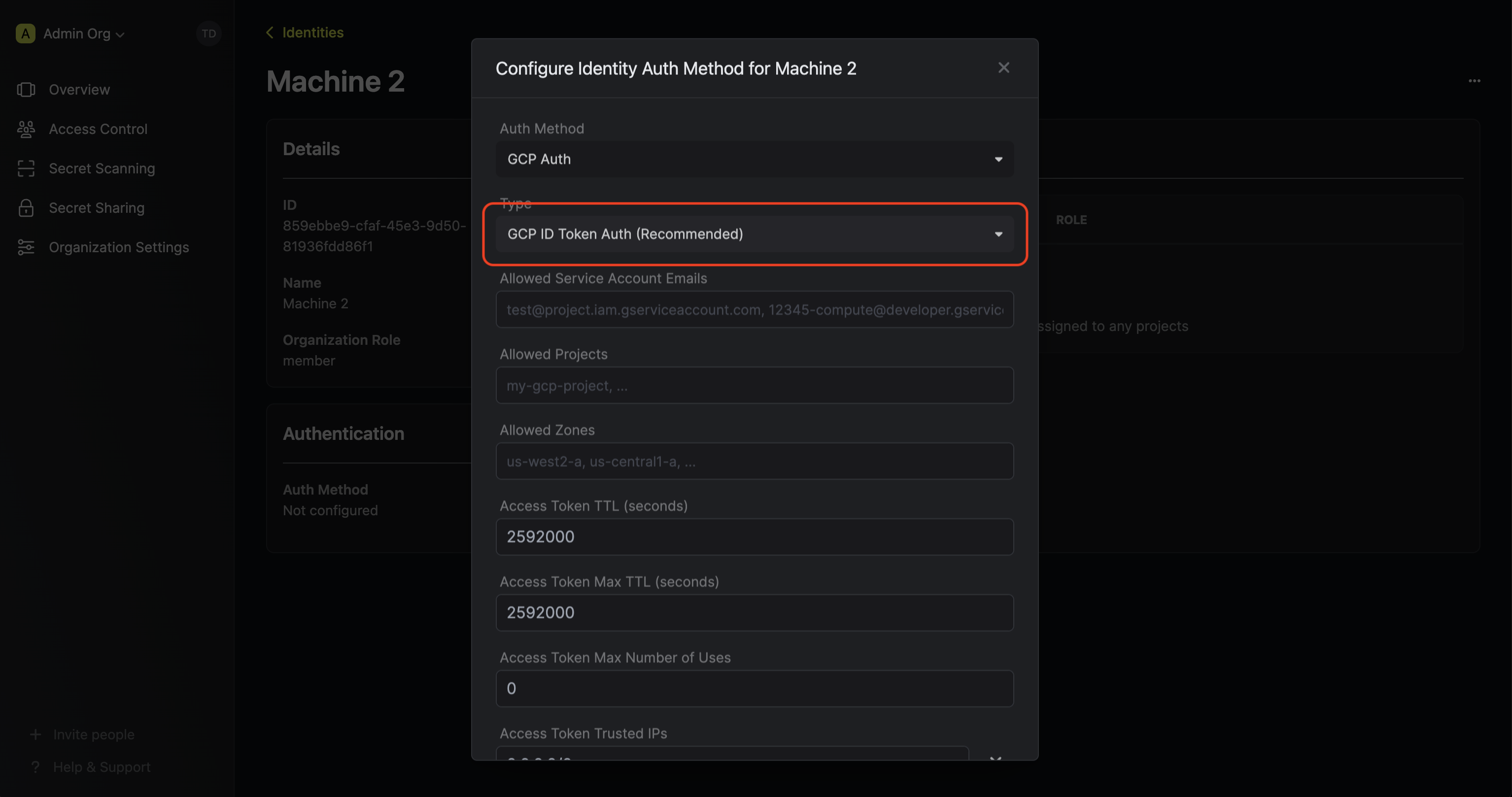
Here's some more guidance on each field:
* Allowed Service Account Emails: A comma-separated list of trusted service account emails corresponding to the GCE resource(s) allowed to authenticate with Infisical; this could be something like `test@project.iam.gserviceaccount.com`, `12345-compute@developer.gserviceaccount.com`, etc.
* Allowed Projects: A comma-separated list of trusted GCP projects that the GCE instance must belong to authenticate with Infisical. Note that this validation property will only work for GCE instances.
* Allowed Zones: A comma-separated list of trusted zones that the GCE instances must belong to authenticate with Infisical; this should be the fully-qualified zone name in the format `-`like `us-central1-a`, `us-west1-b`, etc. Note that this validation property will only work for GCE instances.
* Access Token TTL (default is `2592000` equivalent to 30 days): The lifetime for an acccess token in seconds. This value will be referenced at renewal time.
* Access Token Max TTL (default is `2592000` equivalent to 30 days): The maximum lifetime for an acccess token in seconds. This value will be referenced at renewal time.
* Access Token Max Number of Uses (default is `0`): The maximum number of times that an access token can be used; a value of `0` implies infinite number of uses.
* Access Token Trusted IPs: The IPs or CIDR ranges that access tokens can be used from. By default, each token is given the `0.0.0.0/0`, allowing usage from any network address.
To enable the identity to access project-level resources such as secrets within a specific project, you should add it to that project.
To do this, head over to the project you want to add the identity to and go to Project Settings > Access Control > Machine Identities and press **Add identity**.
Next, select the identity you want to add to the project and the project level role you want to allow it to assume. The project role assigned will determine what project level resources this identity can have access to.
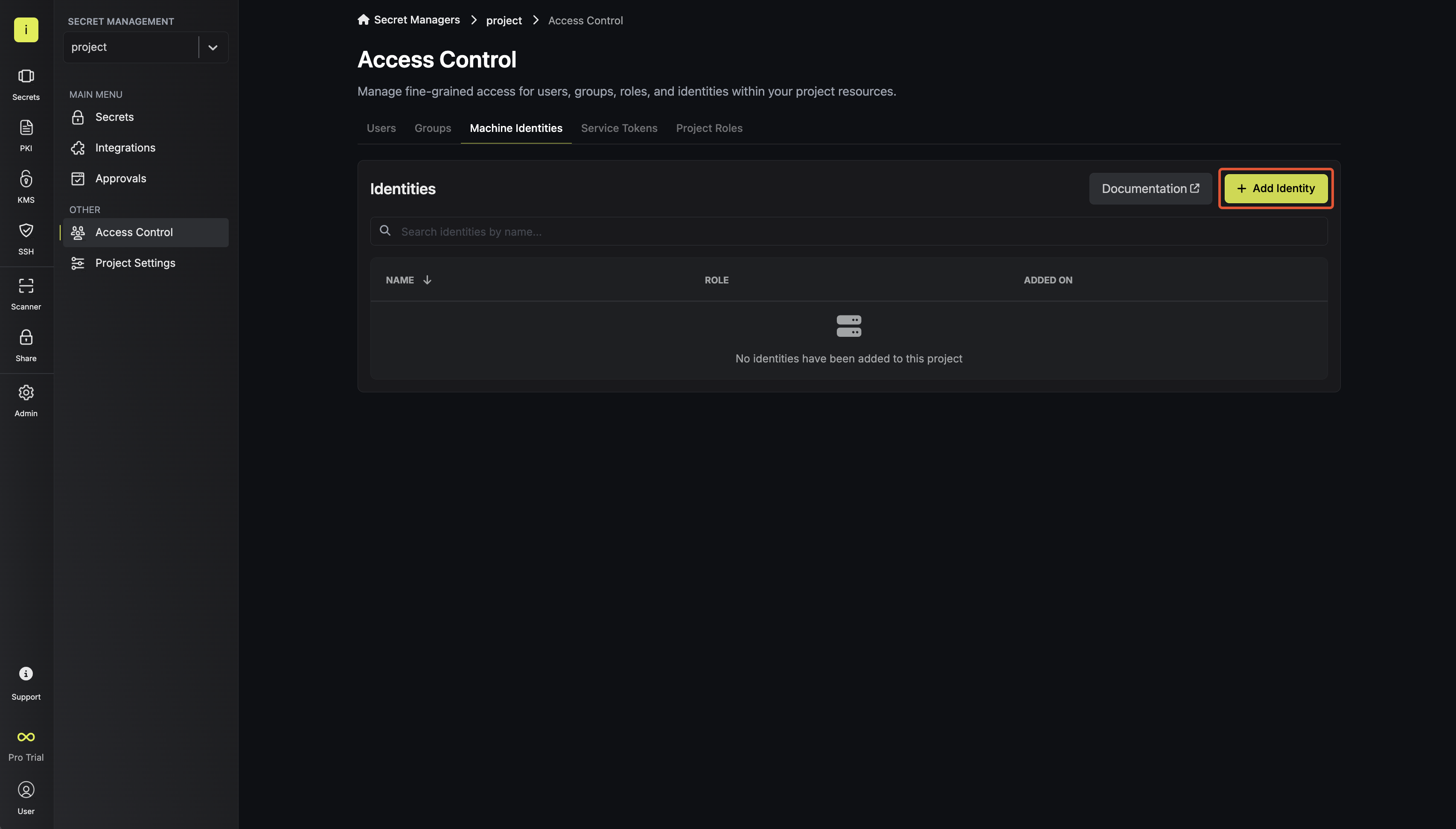
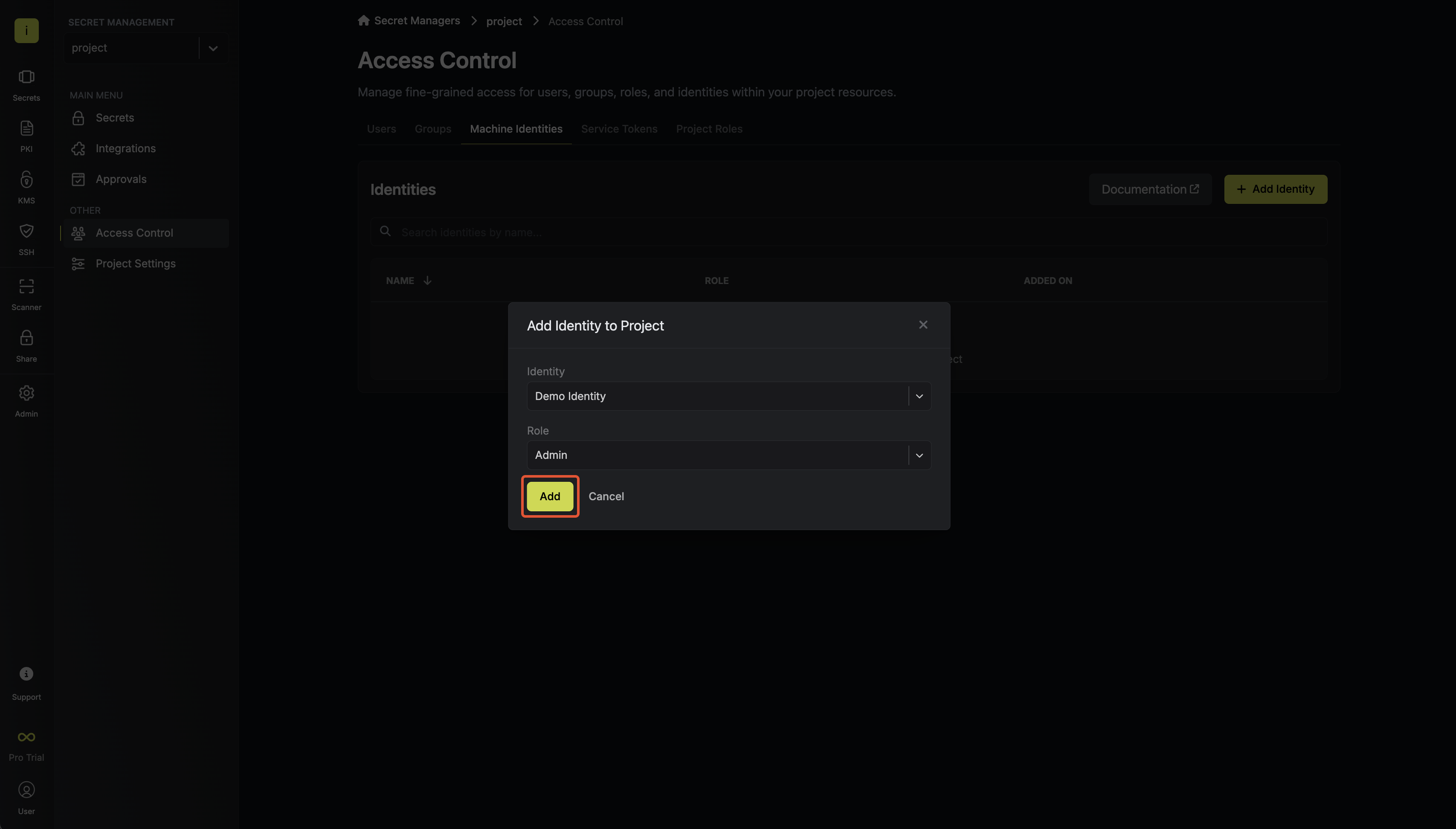
To access the Infisical API as the identity, you need to generate an [ID token](https://cloud.google.com/docs/authentication/get-id-token) constituting the identity of the present GCE instance and make a request to the `/api/v1/auth/gcp-auth/login` endpoint containing the token in exchange for an access token.
We provide a few code examples below of how you can authenticate with Infisical to access the [Infisical API](/api-reference/overview/introduction).
Start by making a request from the GCE instance to obtain the ID token.
For more examples of how to obtain the token in Java, Go, Node.js, etc. refer to the [official documentation](https://cloud.google.com/docs/authentication/get-id-token#curl).
#### Sample request
```bash curl
curl -H "Metadata-Flavor: Google" \
'http://metadata/computeMetadata/v1/instance/service-accounts/default/identity?audience=&format=full'
```
Note that you should replace `` with the ID of the identity you created in step 1.
Next use send the obtained JWT token along to authenticate with Infisical and obtain an access token.
#### Sample request
```bash Request
curl --location --request POST 'https://app.infisical.com/api/v1/auth/gcp-auth/login' \
--header 'Content-Type: application/x-www-form-urlencoded' \
--data-urlencode 'identityId=...' \
--data-urlencode 'jwt=...'
```
#### Sample response
```bash Response
{
"accessToken": "...",
"expiresIn": 7200,
"accessTokenMaxTTL": 43244
"tokenType": "Bearer"
}
```
Next, you can use the access token to access the [Infisical API](/api-reference/overview/introduction)
We recommend using one of Infisical's clients like SDKs or the Infisical Agent to authenticate with Infisical using GCP IAM Auth as they handle the authentication process including generating the signed JWT token.
Each identity access token has a time-to-live (TLL) which you can infer from the response of the login operation;
the default TTL is `7200` seconds which can be adjusted.
If an identity access token expires, it can no longer authenticate with the Infisical API. In this case,
a new access token should be obtained by performing another login operation.
## Diagram
The following sequence diagram illustrates the GCP IAM Auth workflow for authenticating GCP IAM service accounts with Infisical.
```mermaid
sequenceDiagram
participant GCE as Client
participant Infis as Infisical
participant Google as Cloud IAM
Note over GCE,Google: Step 1: Signed JWT Token Generation
GCE->>Google: Request to generate signed JWT token
Google-->>GCE: Return signed JWT token
Note over GCE,Infis: Step 2: JWT Token Login Operation
GCE->>Infis: Send signed JWT token to /api/v1/auth/gcp-auth/login
Infis->>Google: Request public key
Google-->>Infis: Return public key
Note over Infis: Step 3: JWT Token Verification
Note over Infis: Step 4: JWT Property Validation
Infis->>GCE: Return short-lived access token
Note over GCE,Infis: Step 5: Access Infisical API with Token
GCE->>Infis: Make authenticated requests using the short-lived access token
```
## Concept
At a high-level, Infisical authenticates an IAM service account by verifying its identity and checking that it meets specific requirements (e.g. it is an allowed service account) at the `/api/v1/auth/gcp-auth/login` endpoint. If successful,
then Infisical returns a short-lived access token that can be used to make authenticated requests to the Infisical API.
To be more specific:
1. The client generates a signed JWT token using the `projects.serviceAccounts.signJwt` [API method](https://cloud.google.com/iam/docs/reference/credentials/rest/v1/projects.serviceAccounts/signJwt); this is done using the service account credentials associated with the client.
2. The client sends the signed JWT token to Infisical at the `/api/v1/auth/gcp-auth/login` endpoint.
3. Infisical verifies the signed JWT token.
4. Infisical checks if the service account behind the JWT token is allowed to authenticate with Infisical based **Allowed Service Account Emails**.
5. If all is well, Infisical returns a short-lived access token that the client can use to make authenticated requests to the Infisical API.
We recommend using one of Infisical's clients like SDKs or the Infisical Agent
to authenticate with Infisical using GCP IAM Auth as they handle the
authentication process including generating the signed JWT token.
Also, note that Infisical needs network-level access to send requests to the Google Cloud API
as part of the GCP Auth workflow.
## Guide
In the following steps, we explore how to create and use identities for your workloads and applications on GCP to
access the Infisical API using the GCP IAM authentication method.
To create an identity, head to your Organization Settings > Access Control > Machine Identities and press **Create identity**.
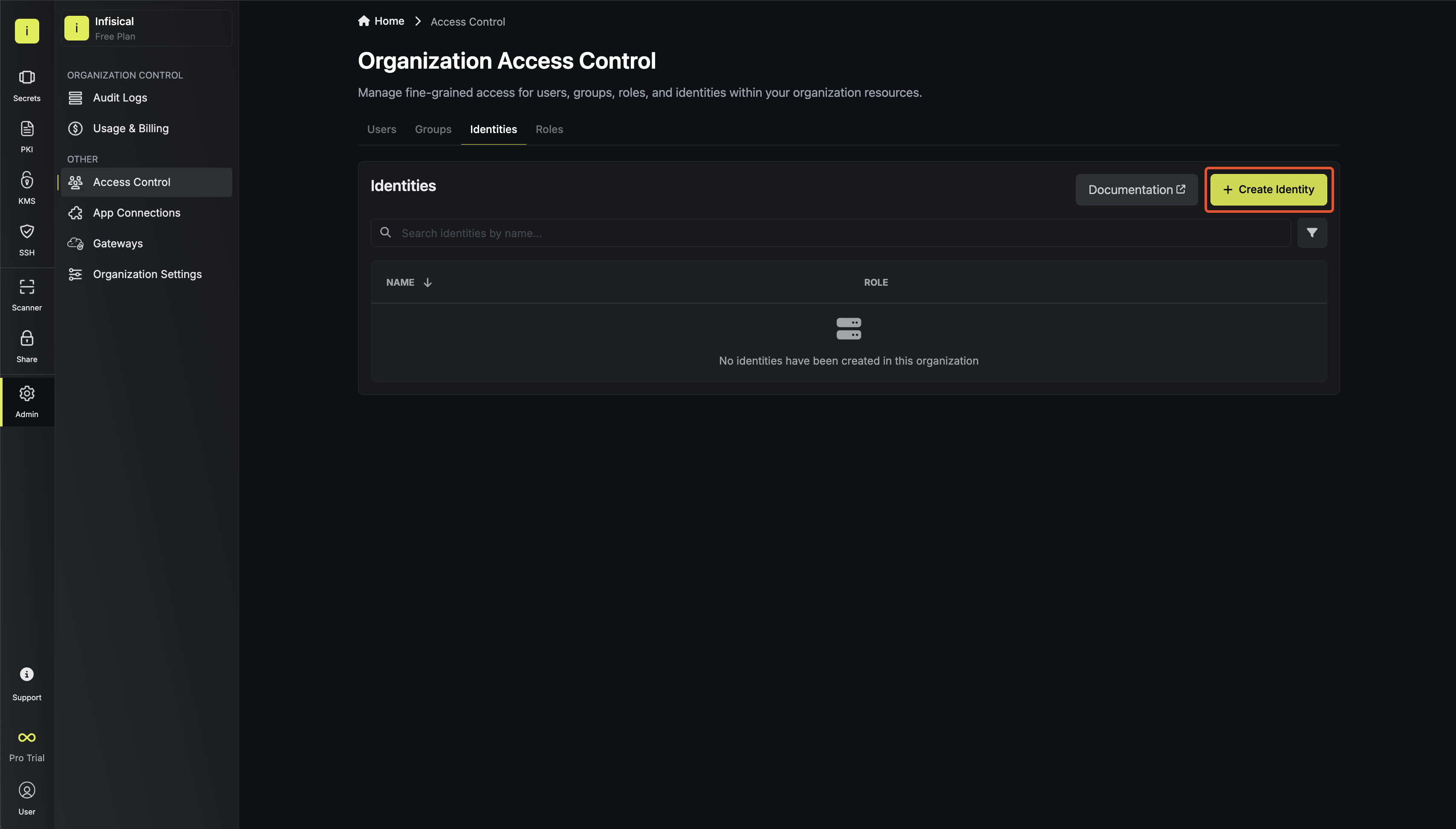
When creating an identity, you specify an organization level [role](/documentation/platform/role-based-access-controls) for it to assume; you can configure roles in Organization Settings > Access Control > Organization Roles.
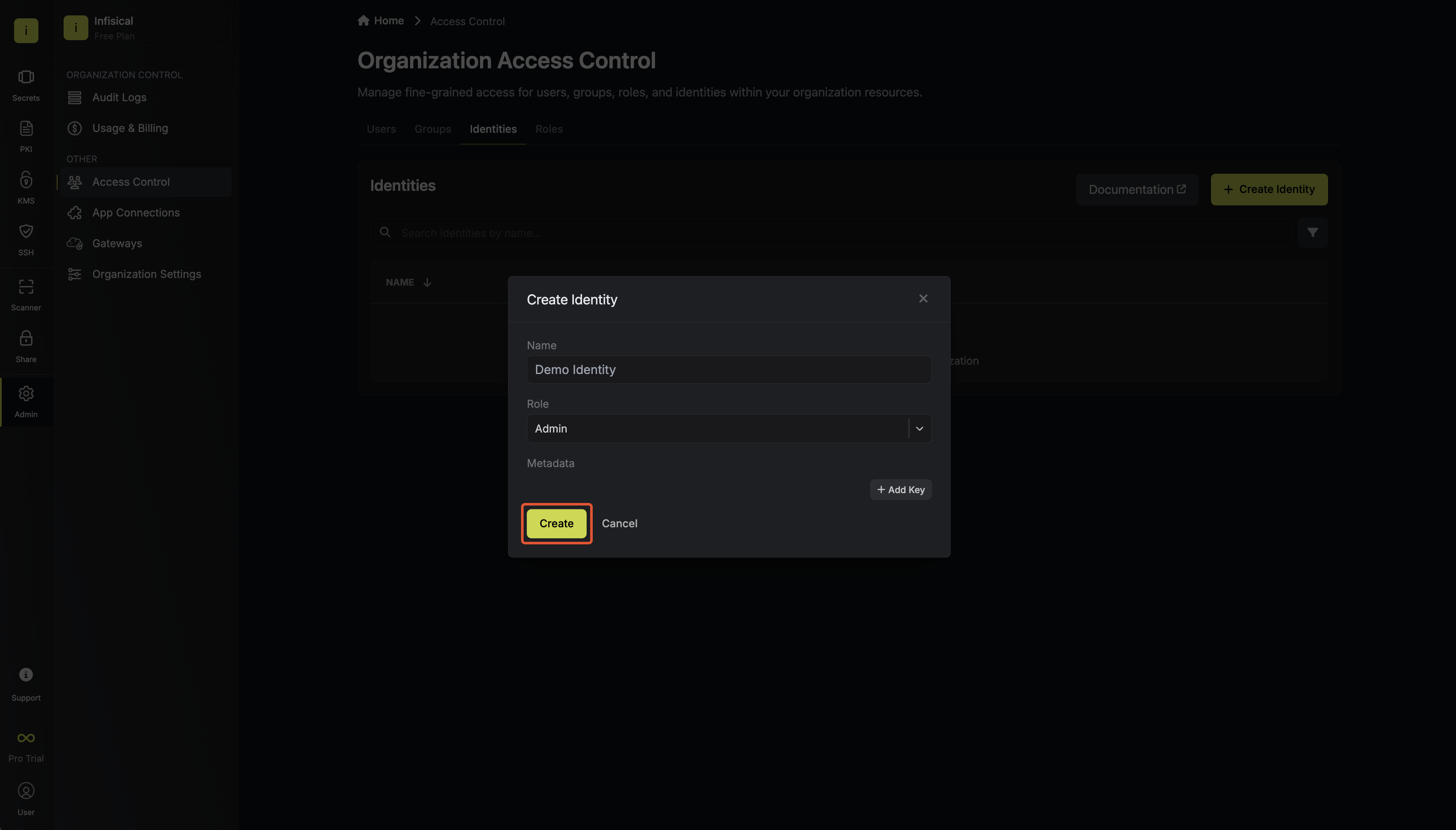
Now input a few details for your new identity. Here's some guidance for each field:
* Name (required): A friendly name for the identity.
* Role (required): A role from the **Organization Roles** tab for the identity to assume. The organization role assigned will determine what organization level resources this identity can have access to.
Once you've created an identity, you'll be redirected to a page where you can manage the identity.
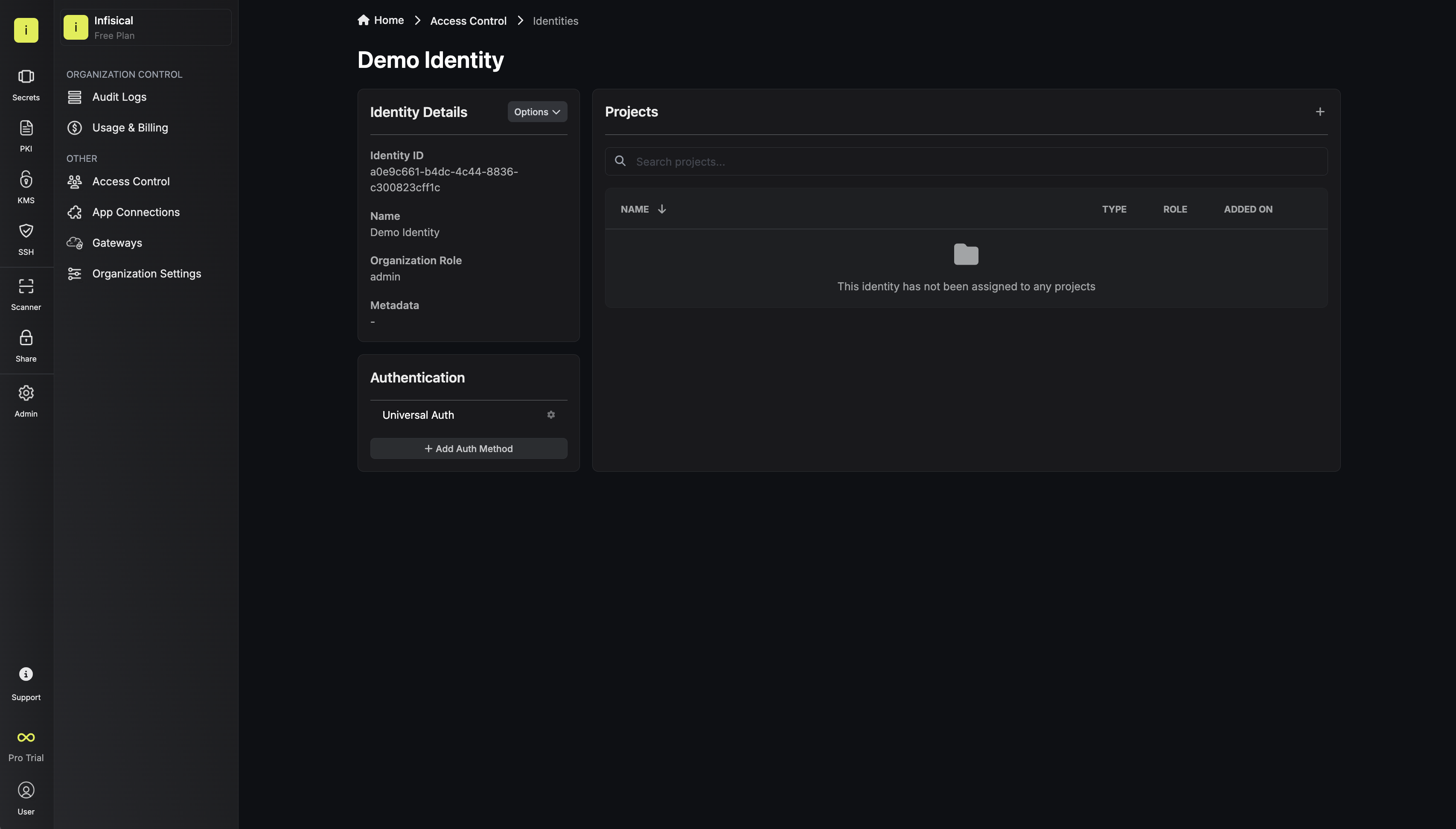
Since the identity has been configured with Universal Auth by default, you should re-configure it to use GCP Auth instead. To do this, press to edit the **Authentication** section,
remove the existing Universal Auth configuration, and add a new GCP Auth configuration onto the identity; set the **Type** field to **GCP IAM Auth**.
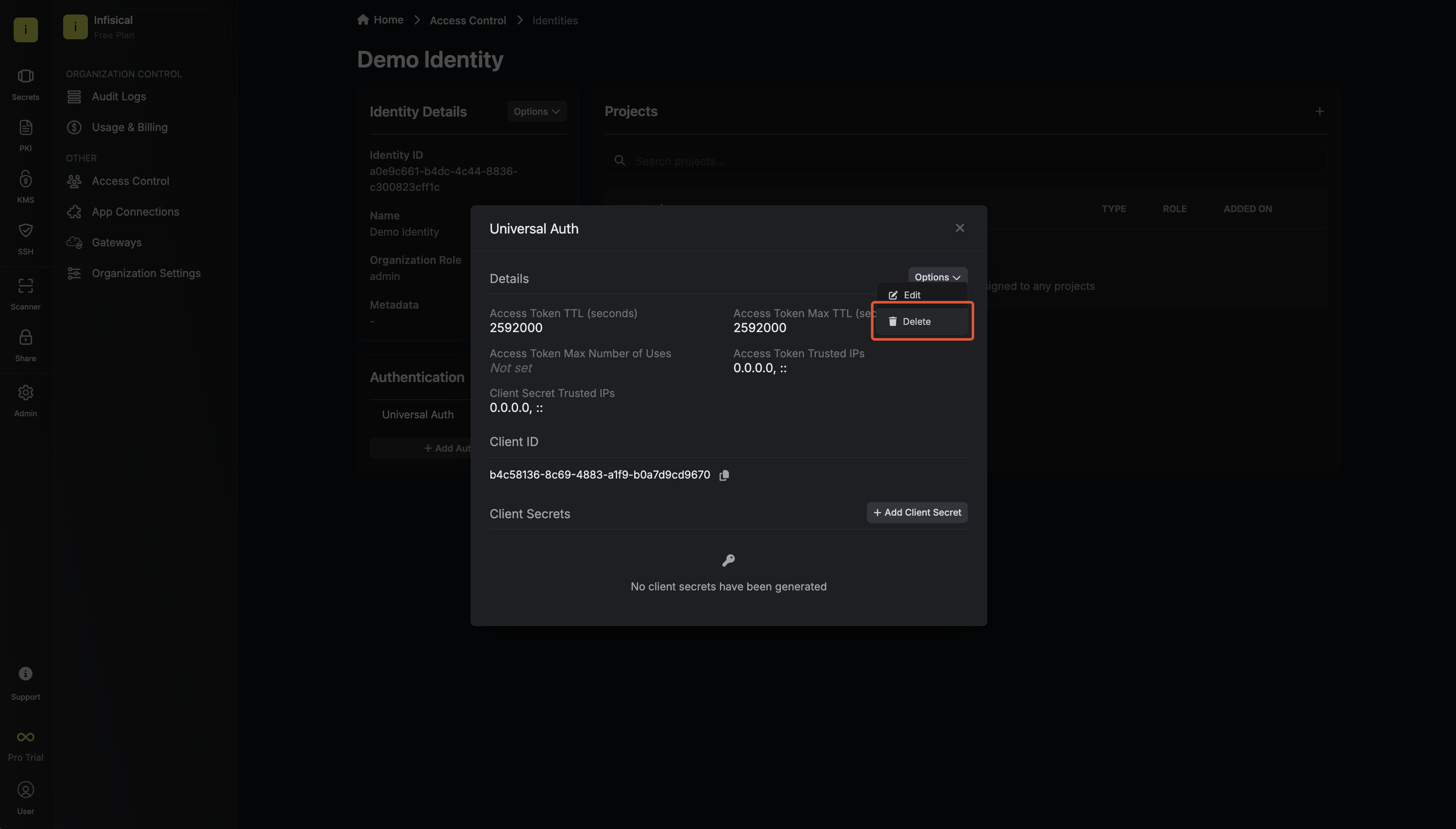
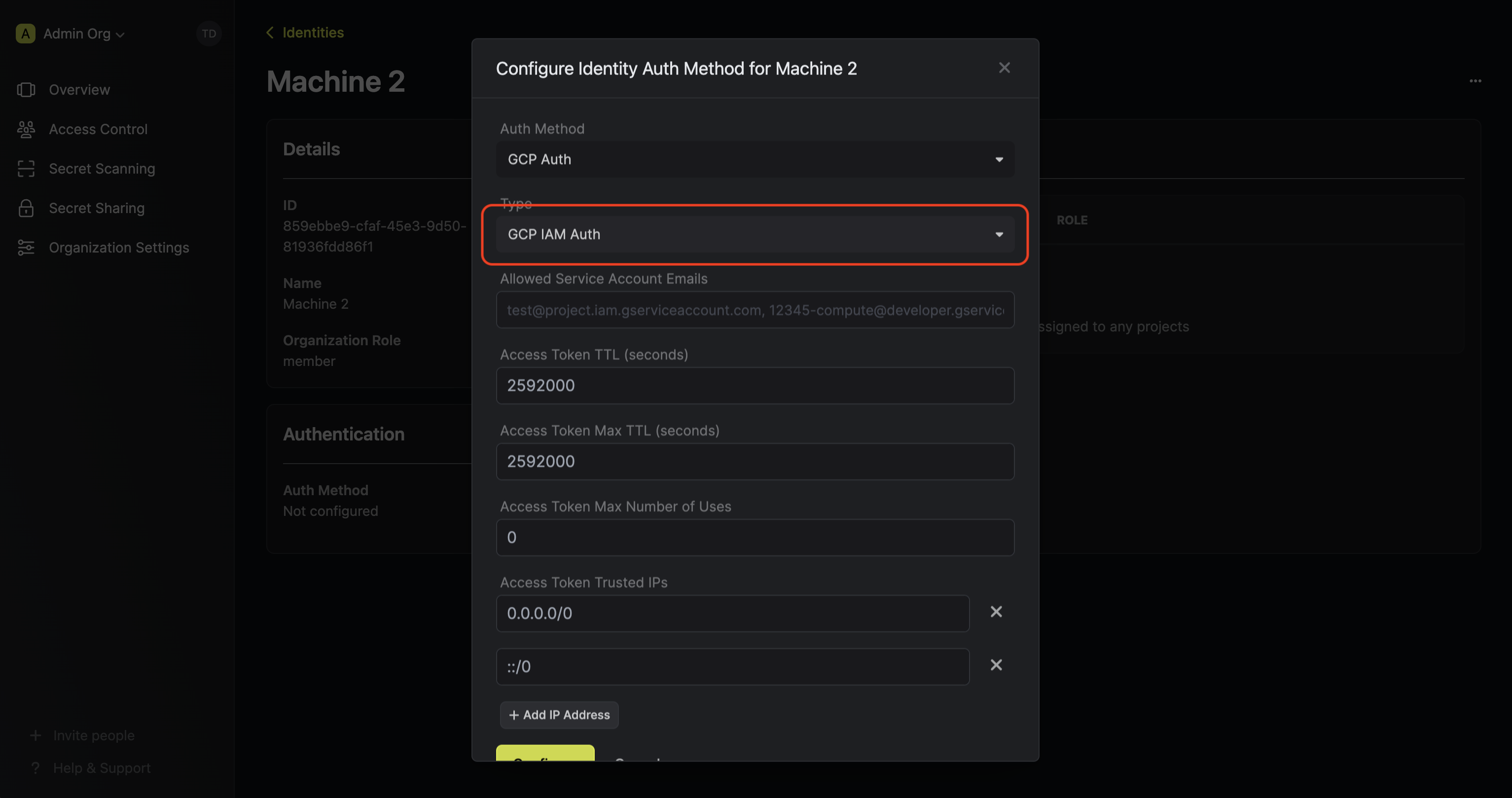
Here's some more guidance on each field:
* Allowed Service Account Emails: A comma-separated list of trusted IAM service account emails that are allowed to authenticate with Infisical; this could be something like `test@project.iam.gserviceaccount.com`, `12345-compute@developer.gserviceaccount.com`, etc.
* Access Token TTL (default is `2592000` equivalent to 30 days): The lifetime for an acccess token in seconds. This value will be referenced at renewal time.
* Access Token Max TTL (default is `2592000` equivalent to 30 days): The maximum lifetime for an acccess token in seconds. This value will be referenced at renewal time.
* Access Token Max Number of Uses (default is `0`): The maximum number of times that an access token can be used; a value of `0` implies infinite number of uses.
* Access Token Trusted IPs: The IPs or CIDR ranges that access tokens can be used from. By default, each token is given the `0.0.0.0/0`, allowing usage from any network address.
To enable the identity to access project-level resources such as secrets within a specific project, you should add it to that project.
To do this, head over to the project you want to add the identity to and go to Project Settings > Access Control > Machine Identities and press **Add identity**.
Next, select the identity you want to add to the project and the project level role you want to allow it to assume. The project role assigned will determine what project level resources this identity can have access to.
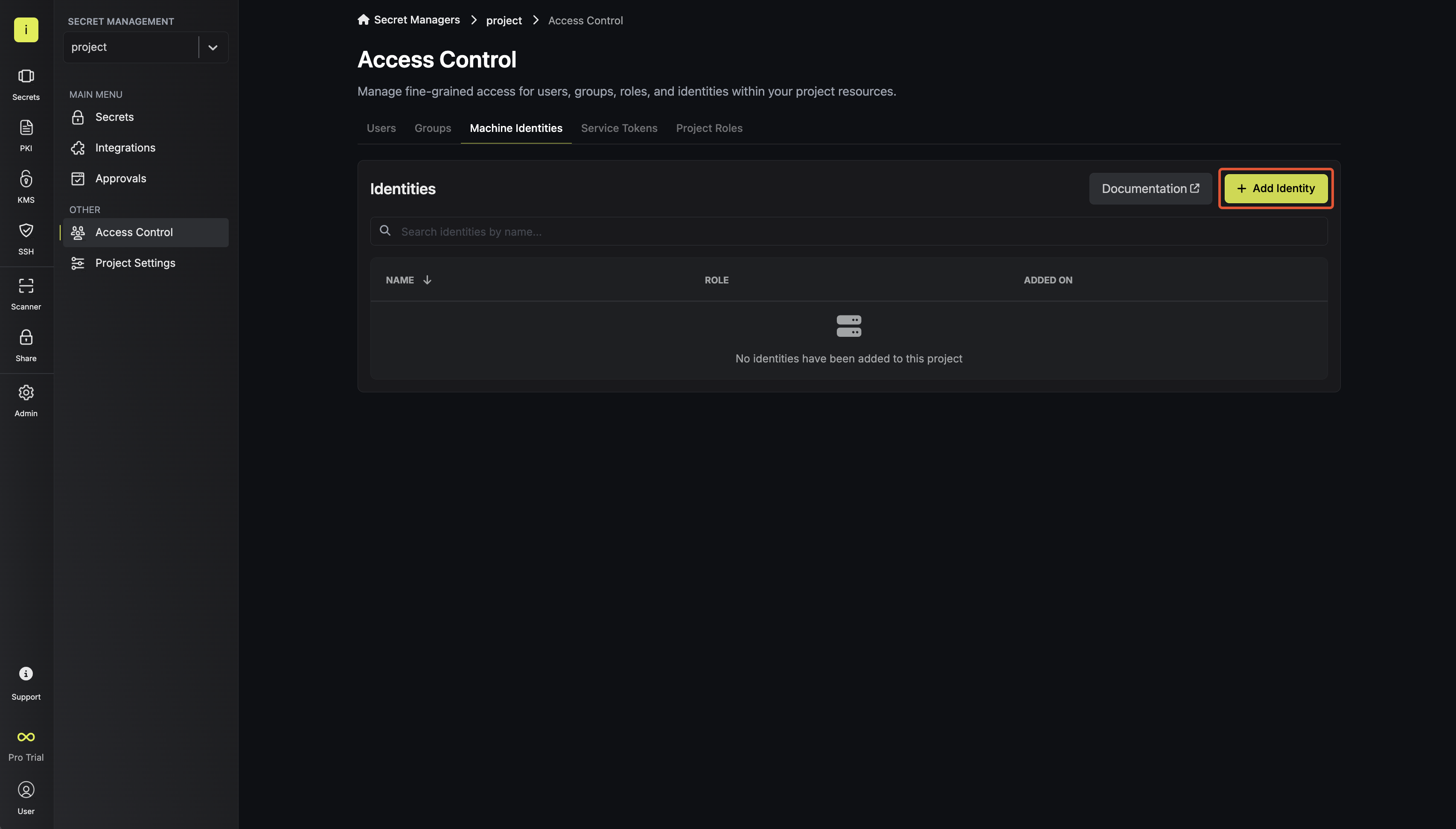
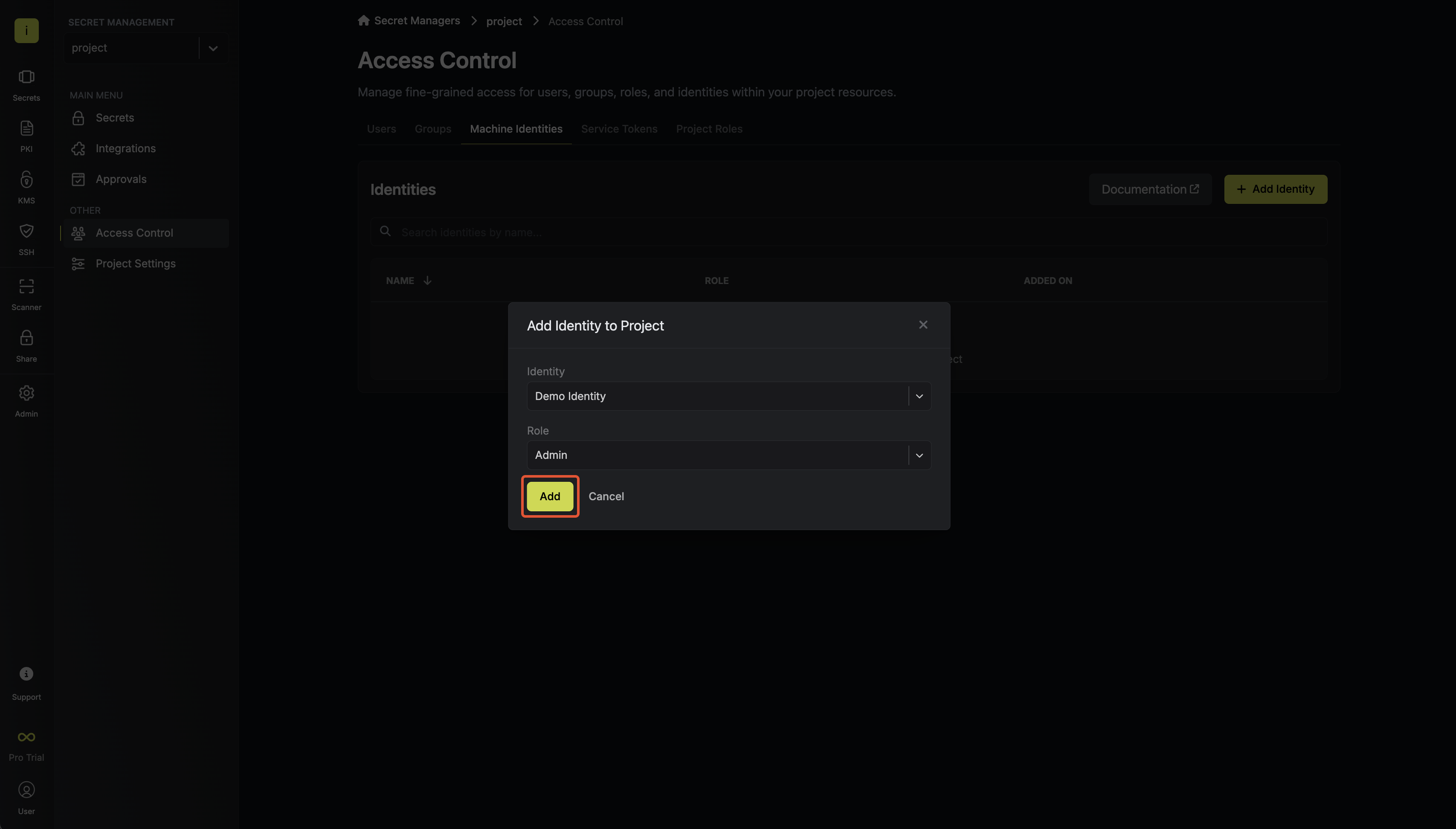
To access the Infisical API as the identity, you need to generate a signed JWT token using the `projects.serviceAccounts.signJwt` [API method](https://cloud.google.com/iam/docs/reference/credentials/rest/v1/projects.serviceAccounts/signJwt) and make a request to the `/api/v1/auth/gcp-auth/login` endpoint containing the signed JWT token in exchange for an access token.
Make sure that the service account has the `iam.serviceAccounts.signJwt` permission or the `roles/iam.serviceAccountTokenCreator` role.
We provide a few code examples below of how you can authenticate with Infisical to access the [Infisical API](/api-reference/overview/introduction).
The following code provides a generic example of how you can generate a signed JWT token against the `projects.serviceAccounts.signJwt` API method.
The shown example uses Node.js and the official [google-auth-library](https://github.com/googleapis/google-auth-library-nodejs#readme) package but you can use any language you wish.
```javascript
const { GoogleAuth } = require("google-auth-library");
const auth = new GoogleAuth({
scopes: "https://www.googleapis.com/auth/cloud-platform",
});
const credentials = await auth.getCredentials();
const identityId = "";
const jwtPayload = {
sub: credentials.client_email,
aud: identityId,
};
const { data } = await client.request({
url: `https://iamcredentials.googleapis.com/v1/projects/-/serviceAccounts/${credentials.client_email}:signJwt`,
method: "POST",
data: { payload: JSON.stringify(jwtPayload) },
});
const jwt = data.signedJwt // send this jwt to Infisical in the next step
```
#### Sample request
```bash Request
curl --location --request POST 'https://app.infisical.com/api/v1/auth/gcp-auth/login' \
--header 'Content-Type: application/x-www-form-urlencoded' \
--data-urlencode 'identityId=...' \
--data-urlencode 'jwt=...'
```
#### Sample response
```bash Response
{
"accessToken": "...",
"expiresIn": 7200,
"accessTokenMaxTTL": 43244
"tokenType": "Bearer"
}
```
Next, you can use the access token to access the [Infisical API](/api-reference/overview/introduction)
We recommend using one of Infisical's clients like SDKs or the Infisical Agent to authenticate with Infisical using GCP IAM Auth as they handle the authentication process including generating the signed JWT token.
Each identity access token has a time-to-live (TLL) which you can infer from the response of the login operation;
the default TTL is `7200` seconds which can be adjusted.
If an identity access token expires, it can no longer authenticate with the Infisical API. In this case,
a new access token should be obtained by performing another login operation.
# Kubernetes Auth
Learn how to authenticate with Infisical in Kubernetes
**Kubernetes Auth** is a Kubernetes-native authentication method for applications (e.g. pods) to access Infisical.
## Diagram
The following sequence diagram illustrates the Kubernetes Auth workflow for authenticating applications running in pods with Infisical.
```mermaid
sequenceDiagram
participant Pod as Pod
participant Infis as Infisical
participant KubernetesServer as K8s API Server
Note over Pod: Step 1: Service Account JWT Token Retrieval
Note over Pod,Infis: Step 2: JWT Token Login Operation
Pod->>Infis: Send JWT token to /api/v1/auth/kubernetes-auth/login
Infis->>KubernetesServer: Forward JWT token for validation
KubernetesServer-->>Infis: Return identity info for JWT
Note over Infis: Step 3: Identity Property Verification
Infis->>Pod: Return short-lived access token
Note over Pod,Infis: Step 4: Access Infisical API with Token
Pod->>Infis: Make authenticated requests using the short-lived access token
```
## Concept
At a high-level, Infisical authenticates an application in Kubernetes by verifying its identity and checking that it meets specific requirements (e.g. it is bound to an allowed service account) at the `/api/v1/auth/kubernetes-auth/login` endpoint. If successful,
then Infisical returns a short-lived access token that can be used to make authenticated requests to the Infisical API.
To be more specific:
1. The application deployed on Kubernetes retrieves its [service account credential](https://kubernetes.io/docs/tasks/configure-pod-container/configure-service-account/#opt-out-of-api-credential-automounting) that is a JWT token at the `/var/run/secrets/kubernetes.io/serviceaccount/token` pod path.
2. The application sends the JWT token to Infisical at the `/api/v1/auth/kubernetes-auth/login` endpoint after which Infisical forwards the JWT token to the Kubernetes API Server at the [TokenReview API](https://kubernetes.io/docs/reference/kubernetes-api/authentication-resources/token-review-v1/) for verification and to obtain the service account information associated with the JWT token. Infisical is able to authenticate and interact with the TokenReview API by using a long-lived service account JWT token itself (referred to onward as the token reviewer JWT token).
3. Infisical checks the service account properties against set criteria such **Allowed Service Account Names** and **Allowed Namespaces**.
4. If all is well, Infisical returns a short-lived access token that the application can use to make authenticated requests to the Infisical API.
We recommend using one of Infisical's clients like SDKs or the Infisical Agent
to authenticate with Infisical using Kubernetes Auth as they handle the
authentication process including service account credential retrieval for you.
## Guide
In the following steps, we explore how to create and use identities for your applications in Kubernetes to access the Infisical API using the Kubernetes Auth authentication method.
1.1. Start by creating a service account in your Kubernetes cluster that will be used by Infisical to authenticate with the Kubernetes API Server.
```yaml infisical-service-account.yaml
apiVersion: v1
kind: ServiceAccount
metadata:
name: infisical-auth
namespace: default
```
```
kubectl apply -f infisical-service-account.yaml
```
1.2. Bind the service account to the `system:auth-delegator` cluster role. As described [here](https://kubernetes.io/docs/reference/access-authn-authz/rbac/#other-component-roles), this role allows delegated authentication and authorization checks, specifically for Infisical to access the [TokenReview API](https://kubernetes.io/docs/reference/kubernetes-api/authentication-resources/token-review-v1/). You can apply the following configuration file:
```yaml cluster-role-binding.yaml
apiVersion: rbac.authorization.k8s.io/v1
kind: ClusterRoleBinding
metadata:
name: role-tokenreview-binding
namespace: default
roleRef:
apiGroup: rbac.authorization.k8s.io
kind: ClusterRole
name: system:auth-delegator
subjects:
- kind: ServiceAccount
name: infisical-auth
namespace: default
```
```
kubectl apply -f cluster-role-binding.yaml
```
1.3. Next, create a long-lived service account JWT token (i.e. the token reviewer JWT token) for the service account using this configuration file for a new `Secret` resource:
```yaml service-account-token.yaml
apiVersion: v1
kind: Secret
type: kubernetes.io/service-account-token
metadata:
name: infisical-auth-token
annotations:
kubernetes.io/service-account.name: "infisical-auth"
```
```
kubectl apply -f service-account-token.yaml
```
1.4. Link the secret in step 1.3 to the service account in step 1.1:
```bash
kubectl patch serviceaccount infisical-auth -p '{"secrets": [{"name": "infisical-auth-token"}]}' -n default
```
1.5. Finally, retrieve the token reviewer JWT token from the secret.
```bash
kubectl get secret infisical-auth-token -n default -o=jsonpath='{.data.token}' | base64 --decode
```
Keep this JWT token handy as you will need it for the **Token Reviewer JWT** field when configuring the Kubernetes Auth authentication method for the identity in step 2.
To create an identity, head to your Organization Settings > Access Control > Machine Identities and press **Create identity**.
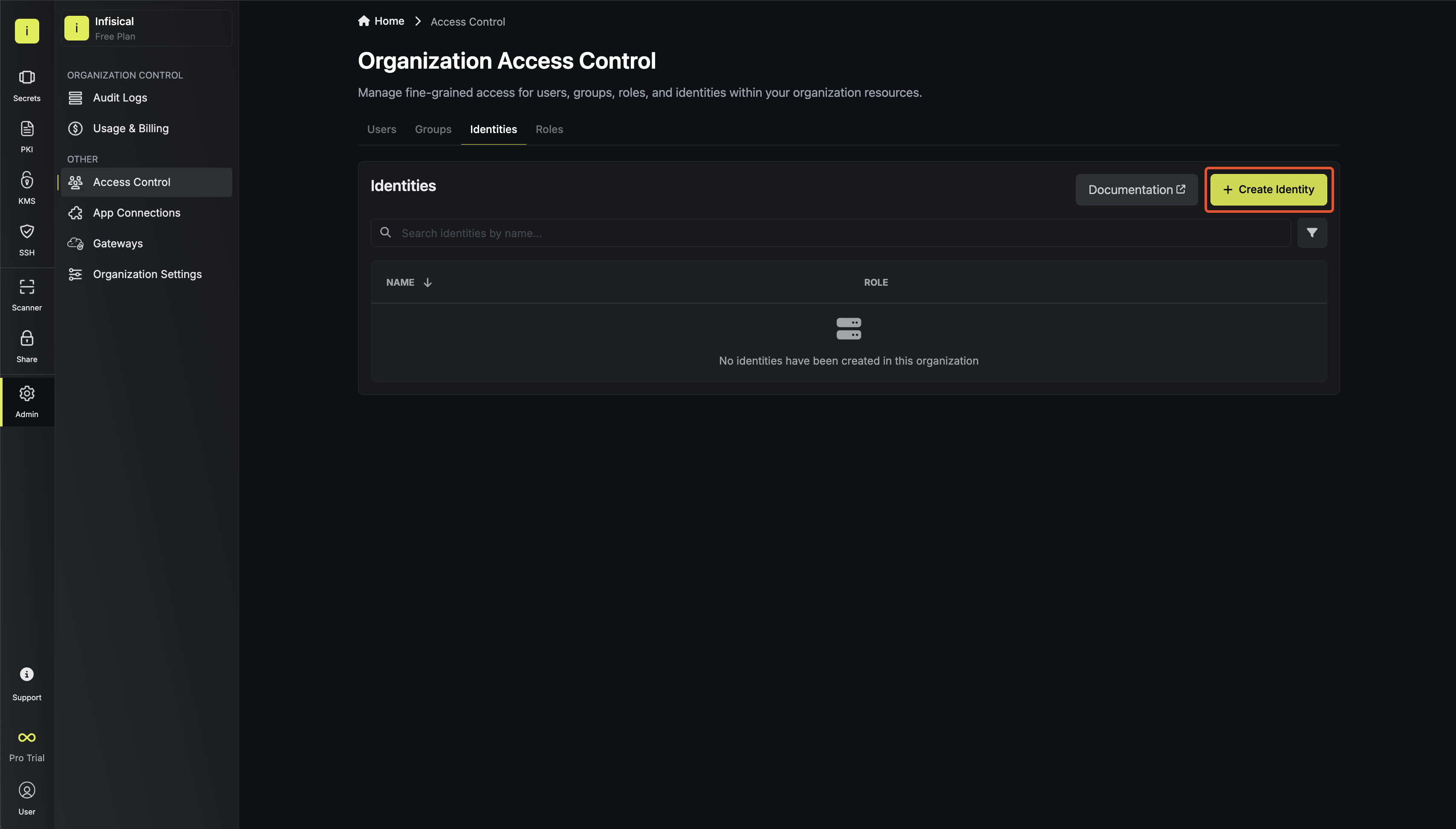
When creating an identity, you specify an organization level [role](/documentation/platform/role-based-access-controls) for it to assume; you can configure roles in Organization Settings > Access Control > Organization Roles.
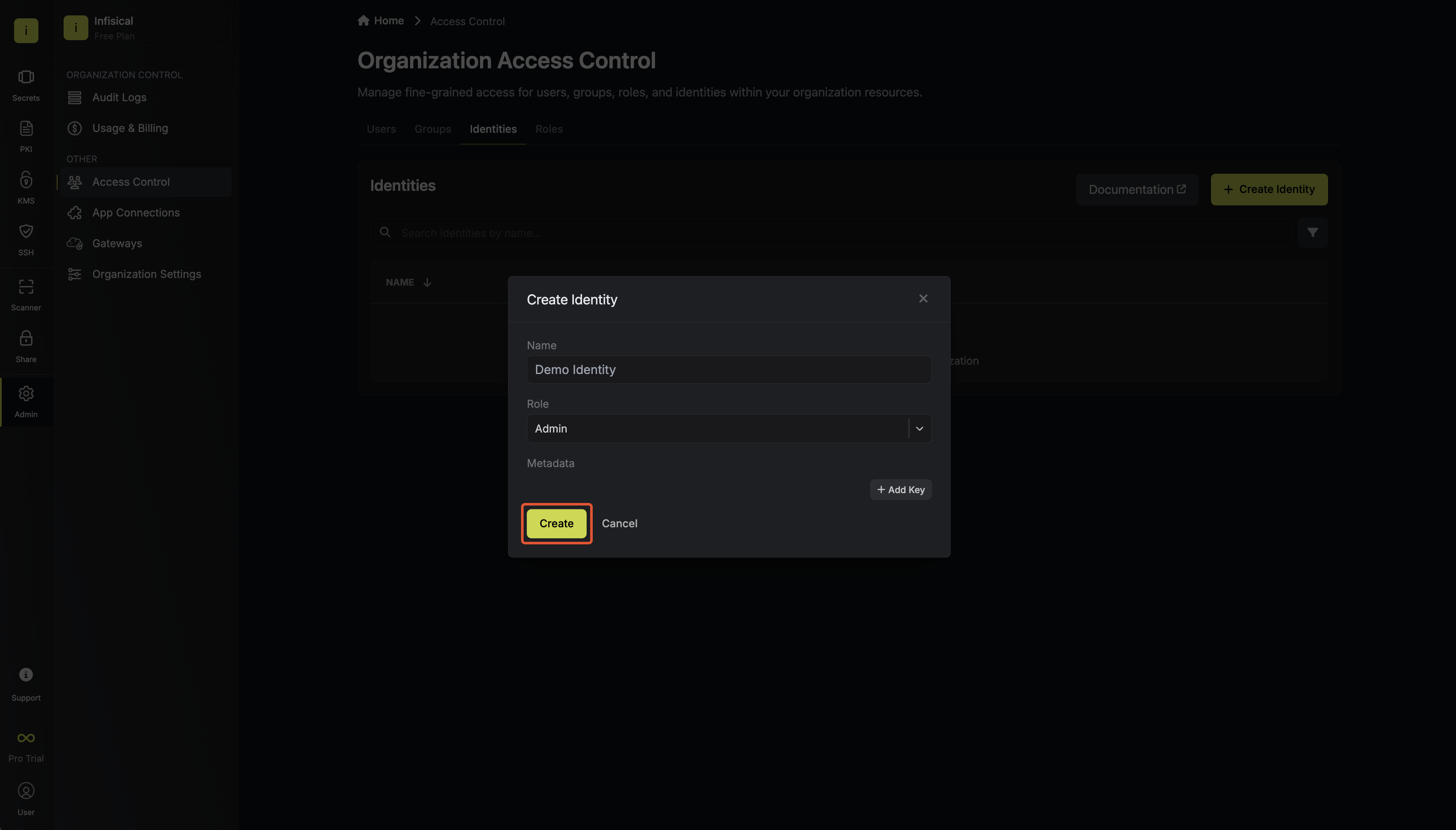
Now input a few details for your new identity. Here's some guidance for each field:
* Name (required): A friendly name for the identity.
* Role (required): A role from the **Organization Roles** tab for the identity to assume. The organization role assigned will determine what organization level resources this identity can have access to.
Once you've created an identity, you'll be redirected to a page where you can manage the identity.
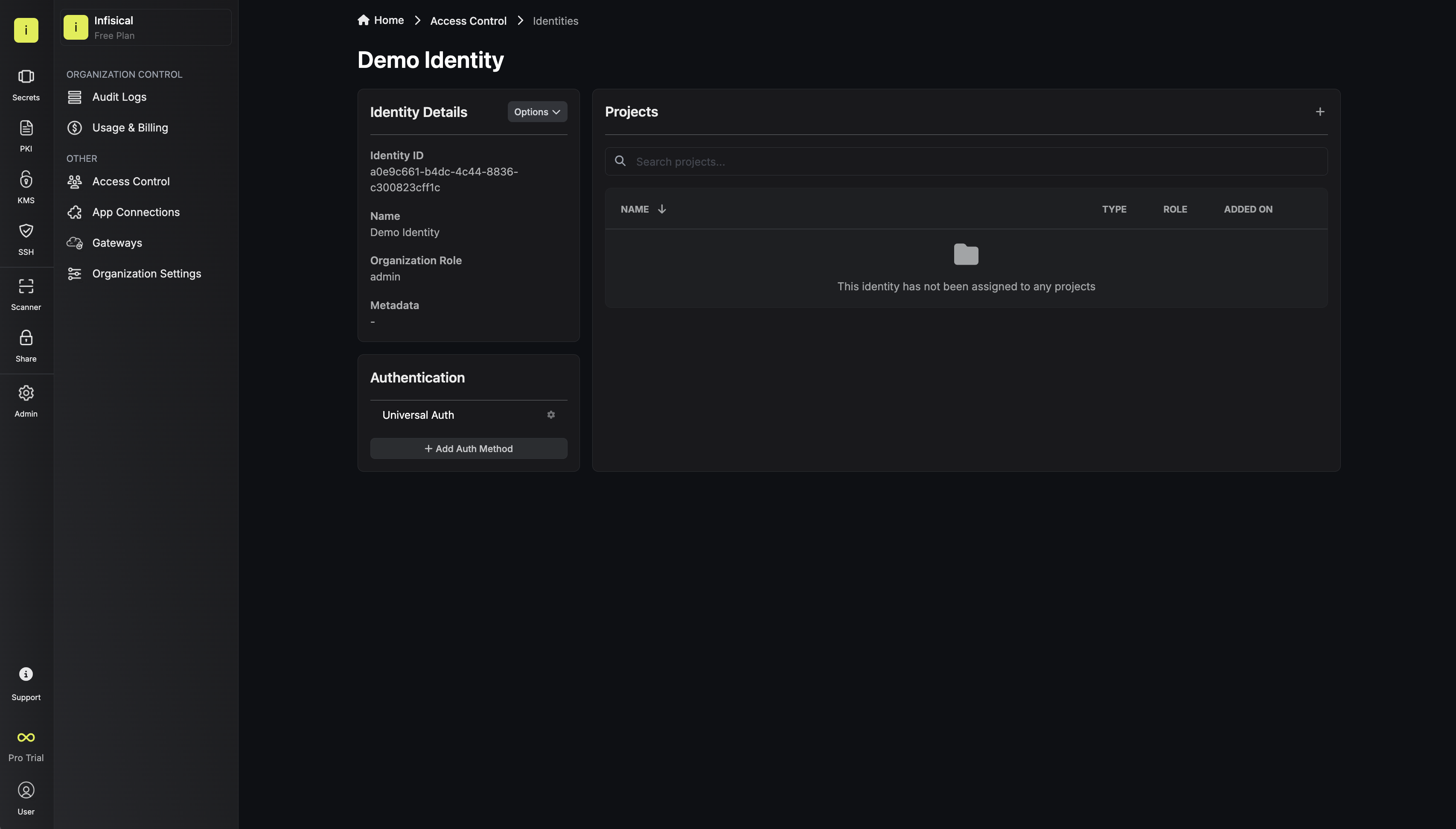
Since the identity has been configured with Universal Auth by default, you should re-configure it to use Kubernetes Auth instead. To do this, press to edit the **Authentication** section,
remove the existing Universal Auth configuration, and add a new Kubernetes Auth configuration onto the identity.
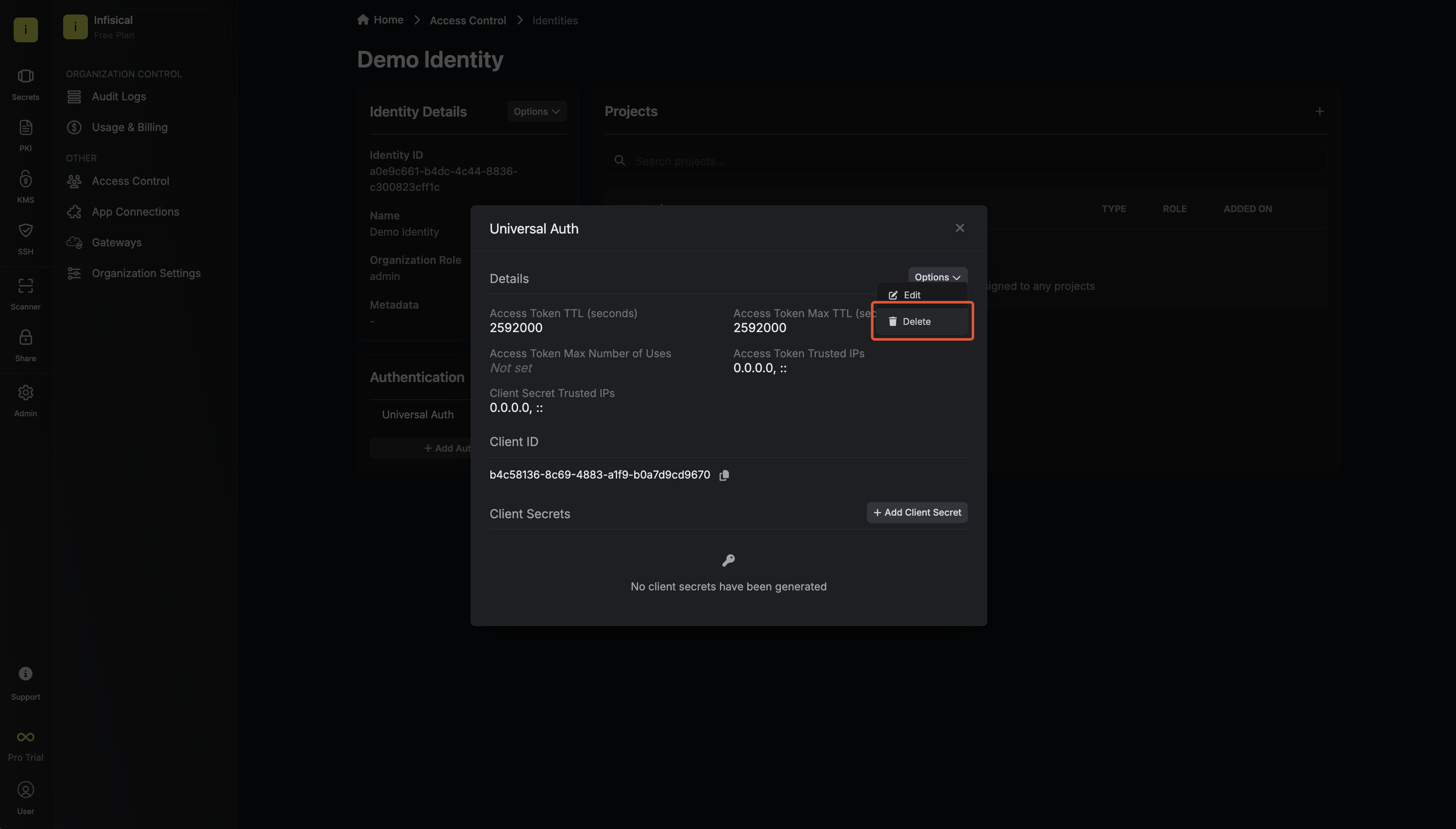
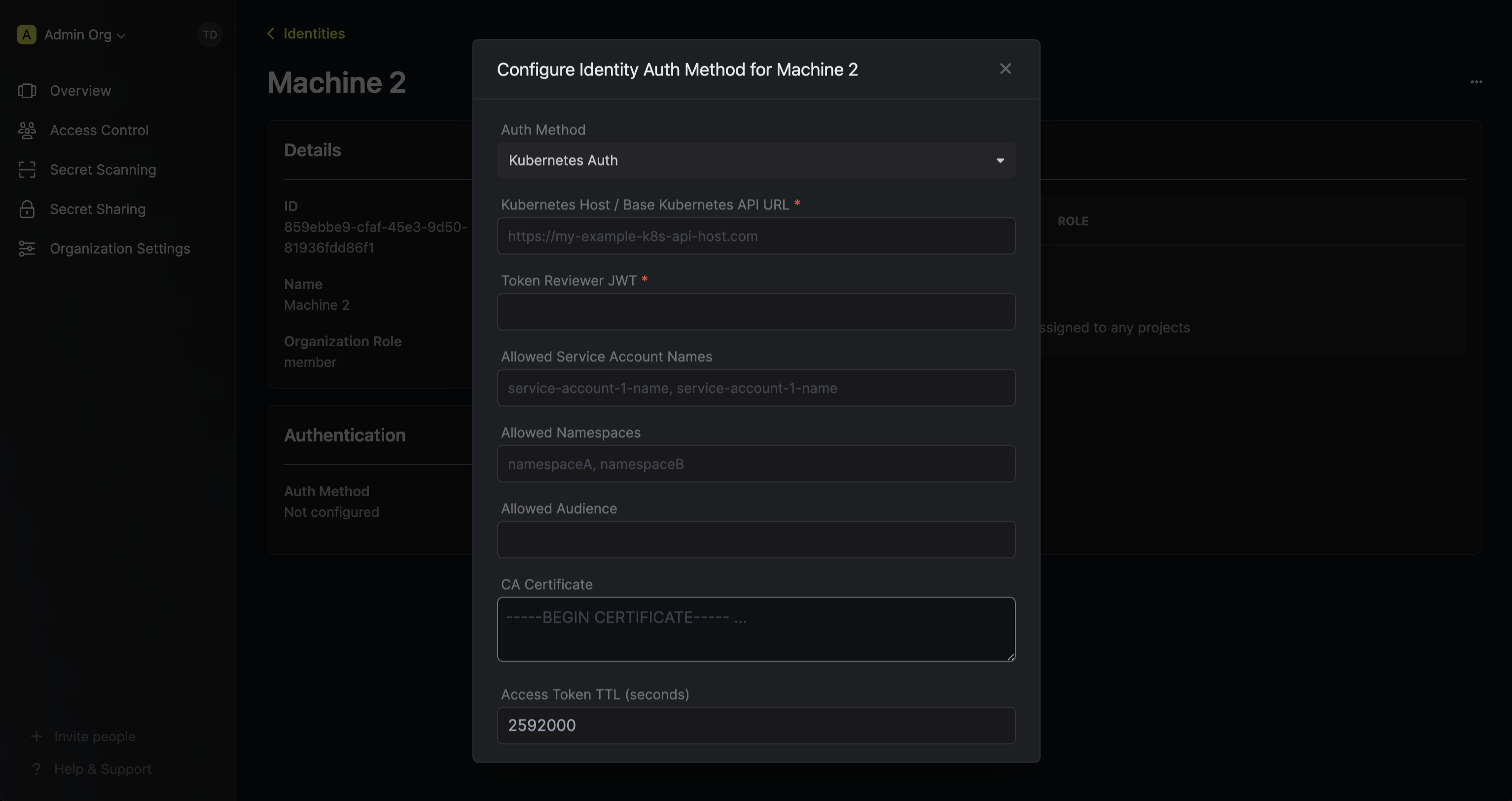
Here's some more guidance on each field:
* Kubernetes Host / Base Kubernetes API URL: The host string, host:port pair, or URL to the base of the Kubernetes API server. This can usually be obtained by running `kubectl cluster-info`.
* Token Reviewer JWT: A long-lived service account JWT token for Infisical to access the [TokenReview API](https://kubernetes.io/docs/reference/kubernetes-api/authentication-resources/token-review-v1/) to validate other service account JWT tokens submitted by applications/pods. This is the JWT token obtained from step 1.5.
* Allowed Service Account Names: A comma-separated list of trusted service account names that are allowed to authenticate with Infisical.
* Allowed Namespaces: A comma-separated list of trusted namespaces that service accounts must belong to authenticate with Infisical.
* Allowed Audience: An optional audience claim that the service account JWT token must have to authenticate with Infisical.
* CA Certificate: The PEM-encoded CA cert for the Kubernetes API server. This is used by the TLS client for secure communication with the Kubernetes API server.
* Access Token TTL (default is `2592000` equivalent to 30 days): The lifetime for an acccess token in seconds. This value will be referenced at renewal time.
* Access Token Max TTL (default is `2592000` equivalent to 30 days): The maximum lifetime for an acccess token in seconds. This value will be referenced at renewal time.
* Access Token Max Number of Uses (default is `0`): The maximum number of times that an access token can be used; a value of `0` implies infinite number of uses.
* Access Token Trusted IPs: The IPs or CIDR ranges that access tokens can be used from. By default, each token is given the `0.0.0.0/0`, allowing usage from any network address.
To enable the identity to access project-level resources such as secrets within a specific project, you should add it to that project.
To do this, head over to the project you want to add the identity to and go to Project Settings > Access Control > Machine Identities and press **Add identity**.
Next, select the identity you want to add to the project and the project level role you want to allow it to assume. The project role assigned will determine what project level resources this identity can have access to.
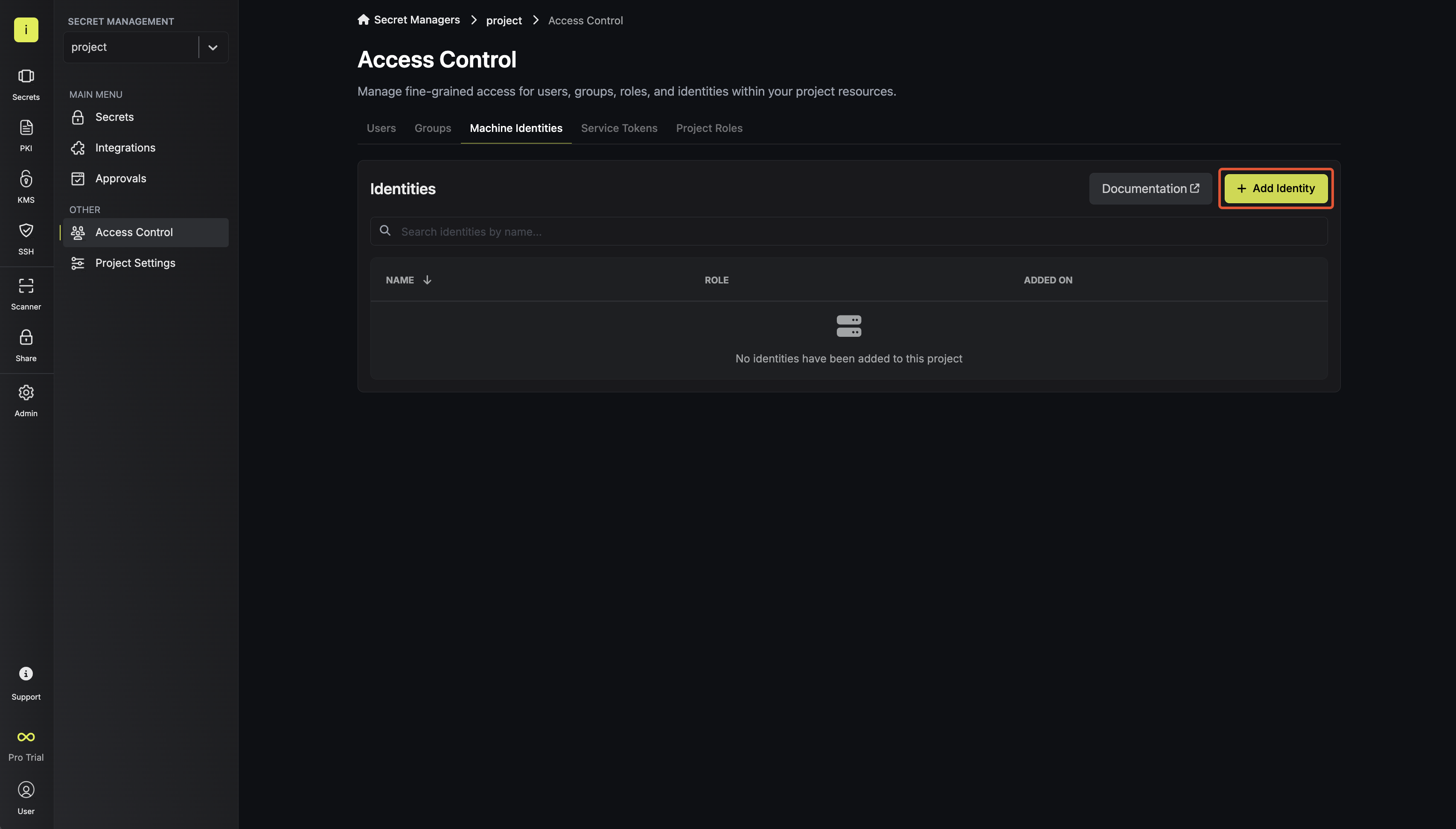
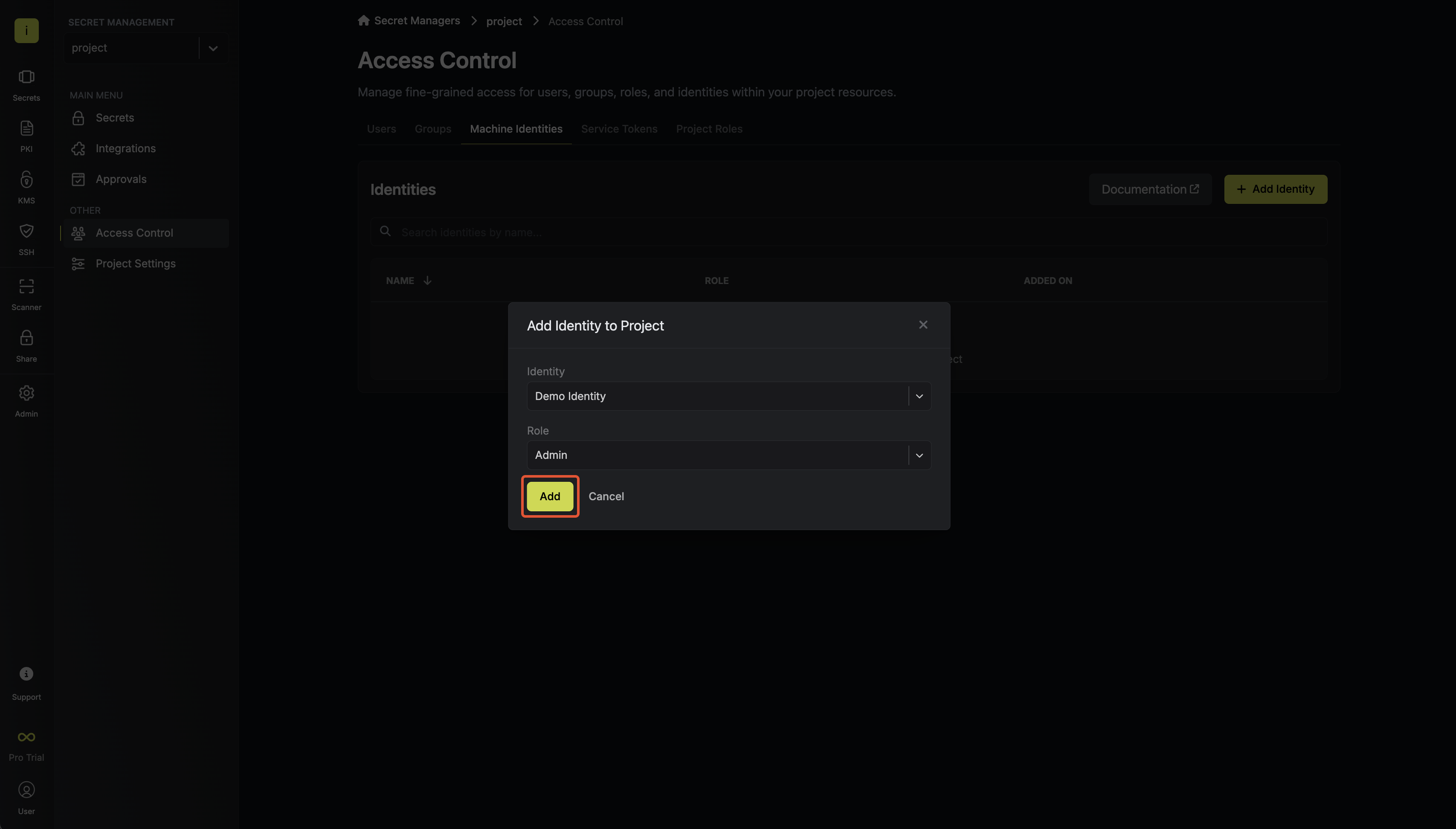
To access the Infisical API as the identity, you should first make sure that the pod running your application is bound to a service account specified in the **Allowed Service Account Names** field of the identity's Kubernetes Auth authentication method configuration in step 2.
Once bound, the pod will receive automatically mounted service account credentials that is a JWT token at the `/var/run/secrets/kubernetes.io/serviceaccount/token` path. This token should be used to authenticate with Infisical at the `/api/v1/auth/kubernetes-auth/login` endpoint.
For information on how to configure sevice accounts for pods, refer to the guide [here](https://kubernetes.io/docs/tasks/configure-pod-container/configure-service-account/).
We provide a code example below of how you might retrieve the JWT token and use it to authenticate with Infisical to gain access to the [Infisical API](/api-reference/overview/introduction).
The shown example uses Node.js but you can use any other language to retrieve the service account JWT token and use it to authenticate with Infisical.
```javascript
const fs = require("fs");
try {
const tokenPath = "/var/run/secrets/kubernetes.io/serviceaccount/token";
const jwtToken = fs.readFileSync(tokenPath, "utf8");
const infisicalUrl = "https://app.infisical.com"; // or your self-hosted Infisical URL
const identityId = "";
const { data } = await axios.post(
`{infisicalUrl}/api/v1/auth/kubernetes-auth/login`,
{
identityId,
jwt,
}
);
console.log("result data: ", data); // access token here
} catch(err) {
console.error(err);
}
```
We recommend using one of Infisical's clients like SDKs or the Infisical Agent to authenticate with Infisical using Kubernetes Auth as they handle the authentication process including service account credential retrieval for you.
Each identity access token has a time-to-live (TLL) which you can infer from the response of the login operation;
the default TTL is `7200` seconds which can be adjusted.
If an identity access token exceeds its max ttl, it can no longer authenticate with the Infisical API. In this case,
a new access token should be obtained by performing another login operation.
**FAQ**
There are a few reasons for why this might happen:
* The Kubernetes Auth authentication method configuration is invalid.
* The service account JWT token has expired is malformed or invalid.
* The service account associated with the JWT token does not meet the criteria set forth in the Kubernetes Auth authentication method configuration such as **Allowed Service Account Names** and **Allowed Namespaces**.
There are a few reasons for why this might happen:
* The access token has expired.
* The identity is insufficently permissioned to interact with the resources you wish to access.
* The client access token is being used from an untrusted IP.
A identity access token can have a time-to-live (TTL) or incremental lifetime after which it expires.
In certain cases, you may want to extend the lifespan of an access token; to do so, you must set a max TTL parameter.
A token can be renewed any number of times where each call to renew it can extend the token's lifetime by increments of the access token's TTL.
Regardless of how frequently an access token is renewed, its lifespan remains bound to the maximum TTL determined at its creation.
# Machine Identities
Learn how to use Machine Identities to programmatically interact with Infisical.
## Concept
An Infisical machine identity is an entity that represents a workload or application that require access to various resources in Infisical. This is conceptually similar to an IAM user in AWS or service account in Google Cloud Platform (GCP).
Each identity must authenticate with the Infisical API using a supported authentication method like [Token Auth](/documentation/platform/identities/token-auth), [Universal Auth](/documentation/platform/identities/universal-auth), [Kubernetes Auth](/documentation/platform/identities/kubernetes-auth), [AWS Auth](/documentation/platform/identities/aws-auth), [Azure Auth](/documentation/platform/identities/azure-auth), or [GCP Auth](/documentation/platform/identities/gcp-auth) to get back a short-lived access token to be used in subsequent requests.
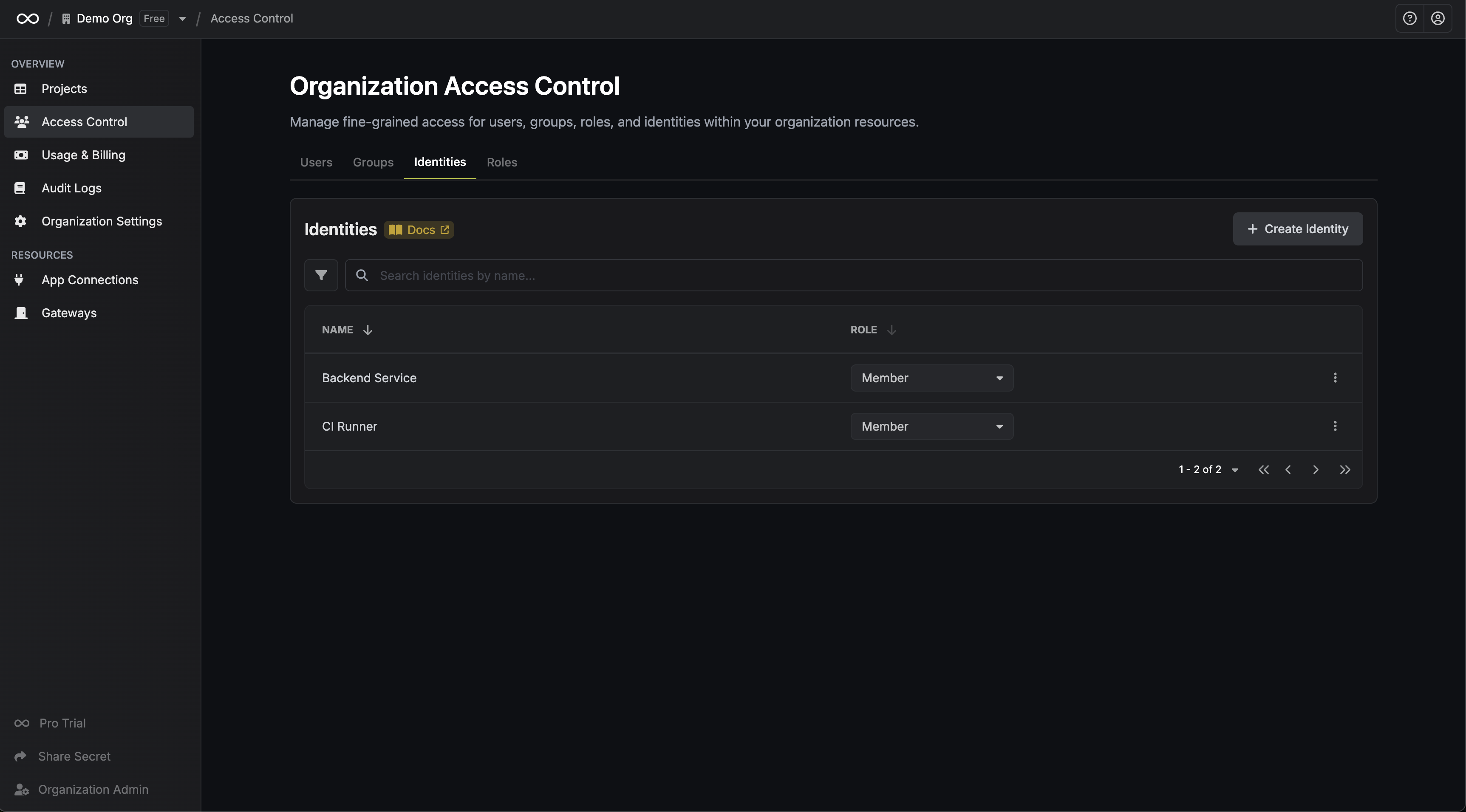
Key Features:
* Role Assignment: Identities must be assigned [roles](/documentation/platform/role-based-access-controls). These roles determine the scope of access to resources, either at the organization level or project level.
* Auth/Token Configuration: Identities must be configured with corresponding authentication methods and access token properties to securely interact with the Infisical API.
## Workflow
A typical workflow for using identities consists of four steps:
1. Creating the identity with a name and [role](/documentation/platform/access-controls/role-based-access-controls) in Organization Access Control > Machine Identities.
This step also involves configuring an authentication method for it.
2. Adding the identity to the project(s) you want it to have access to.
3. Authenticating the identity with the Infisical API based on the configured authentication method on it and receiving a short-lived access token back.
4. Authenticating subsequent requests with the Infisical API using the short-lived access token.
## Authentication Methods
To interact with various resources in Infisical, Machine Identities can authenticate with the Infisical API using:
* [Token Auth](/documentation/platform/identities/token-auth): A platform-agnostic, simple authentication method suitable to authenticate with Infisical using a token.
* [Universal Auth](/documentation/platform/identities/universal-auth): A platform-agnostic authentication method suitable to authenticate with Infisical using a Client ID and Client Secret.
* [Kubernetes Auth](/documentation/platform/identities/kubernetes-auth): A Kubernetes-native authentication method for applications (e.g. pods).
* [AWS Auth](/documentation/platform/identities/aws-auth): An AWS-native authentication method for AWS services (e.g. EC2, Lambda functions, etc.).
* [Azure Auth](/documentation/platform/identities/azure-auth): An Azure-native authentication method for Azure resources (e.g. Azure VMs, Azure App Services, Azure Functions, Azure Kubernetes Service, etc.).
* [GCP Auth](/documentation/platform/identities/gcp-auth): A GCP-native authentication method for GCP resources (e.g. Compute Engine, App Engine, Cloud Run, Google Kubernetes Engine, IAM service accounts, etc.).
* [OIDC Auth](/documentation/platform/identities/oidc-auth): A platform-agnostic, JWT-based authentication method for workloads using an OpenID Connect identity provider.
## FAQ
Yes - Identities can be used with the CLI.
You can learn more about how to do this in the CLI quickstart [here](/cli/usage).
A service token is a project-level authentication method that is being deprecated in favor of identities. The service token method will be removed in the future in accordance with the deprecation notice and timeline stated [here](https://infisical.com/blog/deprecating-api-keys).
Amongst many differences, identities provide broader access over the Infisical API, utilizes the same
permission system as user identities, and come with a significantly larger number of configurable authentication and security features.
If you're looking for a simple authentication method, similar to service tokens, that can be bound onto an identity, we recommend checking out [Token Auth](/documentation/platform/identities/token-auth).
There are a few reasons for why this might happen:
* You have insufficient organization permissions to create, read, update, delete identities.
* The identity you are trying to read, update, or delete is more privileged than yourself.
* The role you are trying to create an identity for or update an identity to is more privileged than yours.
# CircleCI
Learn how to authenticate CircleCI jobs with Infisical using OpenID Connect (OIDC).
**OIDC Auth** is a platform-agnostic JWT-based authentication method that can be used to authenticate from any platform or environment using an identity provider with OpenID Connect.
## Diagram
The following sequence diagram illustrates the OIDC Auth workflow for authenticating CircleCI jobs with Infisical.
```mermaid
sequenceDiagram
participant Client as CircleCI Job
participant Idp as CircleCI Identity Provider
participant Infis as Infisical
Idp->>Client: Step 1: Inject JWT with verifiable claims
Note over Client,Infis: Step 2: Login Operation
Client->>Infis: Send signed JWT to /api/v1/auth/oidc-auth/login
Note over Infis,Idp: Step 3: Query verification
Infis->>Idp: Request JWT public key using OIDC Discovery
Idp-->>Infis: Return public key
Note over Infis: Step 4: JWT validation
Infis->>Client: Return short-lived access token
Note over Client,Infis: Step 5: Access Infisical API with Token
Client->>Infis: Make authenticated requests using the short-lived access token
```
## Concept
At a high-level, Infisical authenticates a client by verifying the JWT and checking that it meets specific requirements (e.g. it is issued by a trusted identity provider) at the `/api/v1/auth/oidc-auth/login` endpoint. If successful,
then Infisical returns a short-lived access token that can be used to make authenticated requests to the Infisical API.
To be more specific:
1. CircleCI provides the running job with a valid OIDC token specific to the execution.
2. The CircleCI OIDC token is sent to Infisical at the `/api/v1/auth/oidc-auth/login` endpoint.
3. Infisical fetches the public key that was used to sign the identity token provided by CircleCI.
4. Infisical validates the JWT using the public key provided by the identity provider and checks that the subject, audience, and claims of the token matches with the set criteria.
5. If all is well, Infisical returns a short-lived access token that CircleCI jobs can use to make authenticated requests to the Infisical API.
Infisical needs network-level access to the CircleCI servers.
## Guide
In the following steps, we explore how to create and use identities to access the Infisical API using the OIDC Auth authentication method.
To create an identity, head to your Organization Settings > Access Control > Machine Identities and press **Create identity**.
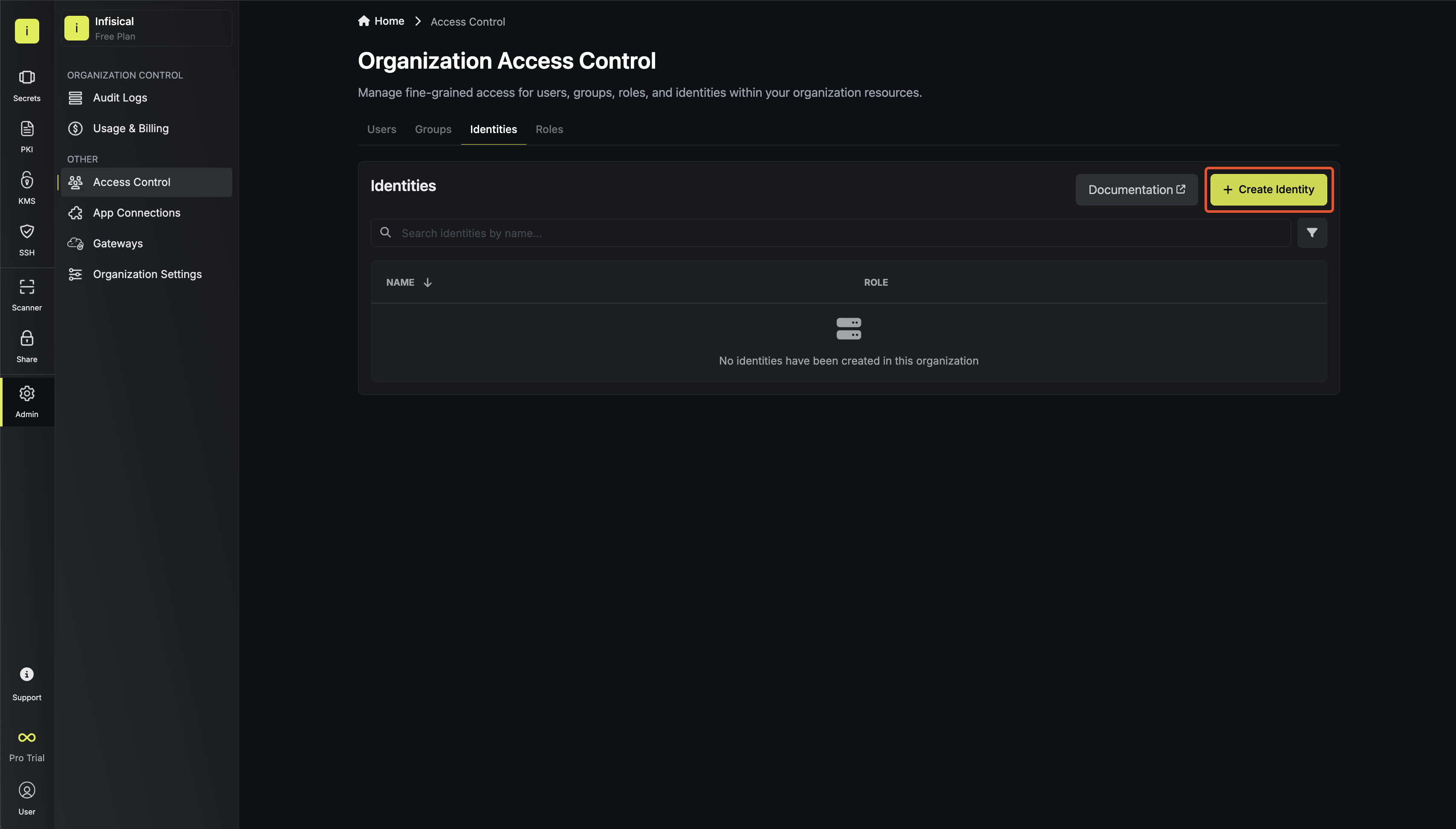
When creating an identity, you specify an organization level [role](/documentation/platform/role-based-access-controls) for it to assume; you can configure roles in Organization Settings > Access Control > Organization Roles.
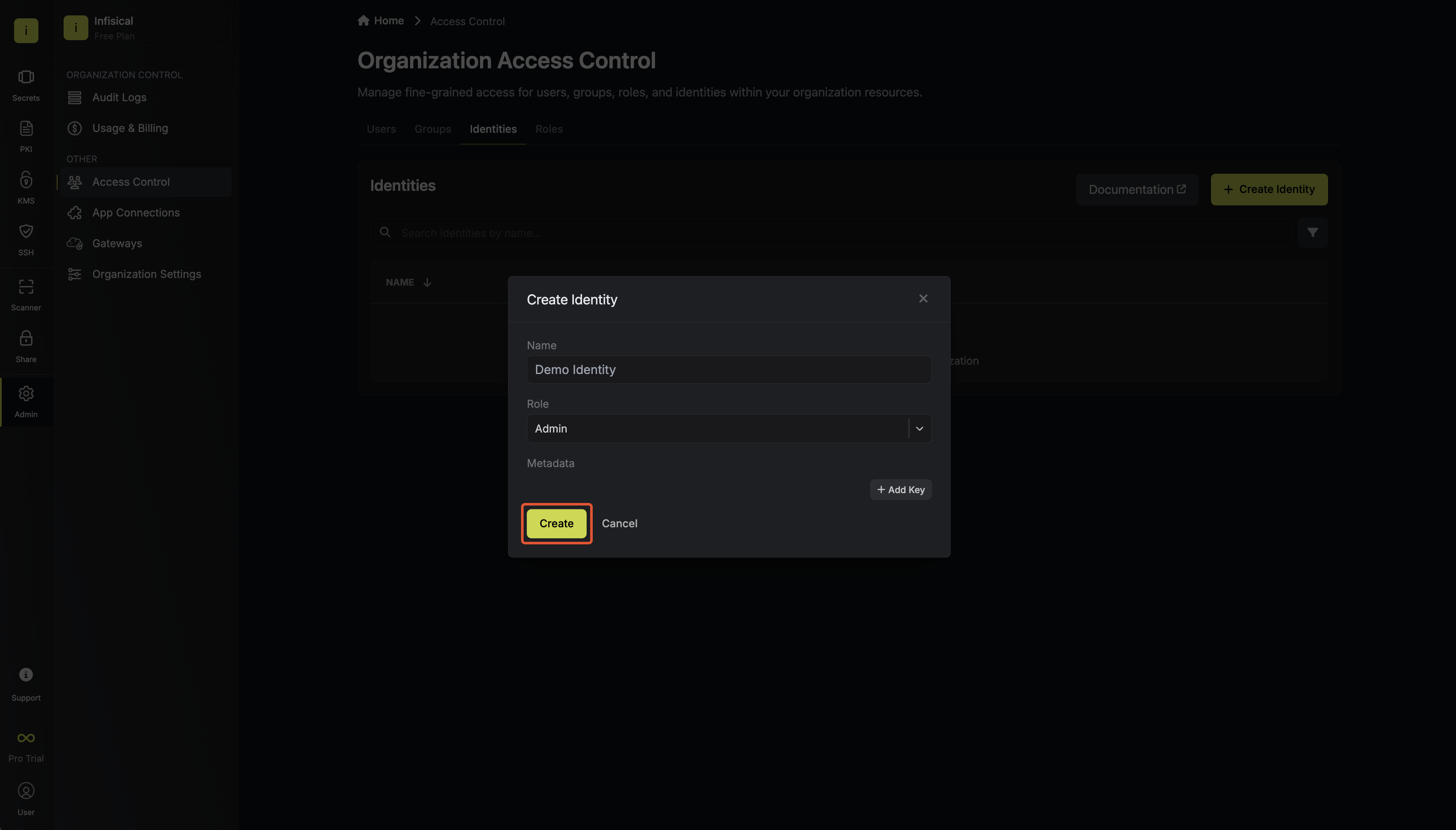
Now input a few details for your new identity. Here's some guidance for each field:
* Name (required): A friendly name for the identity.
* Role (required): A role from the **Organization Roles** tab for the identity to assume. The organization role assigned will determine what organization level resources this identity can have access to.
Once you've created an identity, you'll be redirected to a page where you can manage the identity.
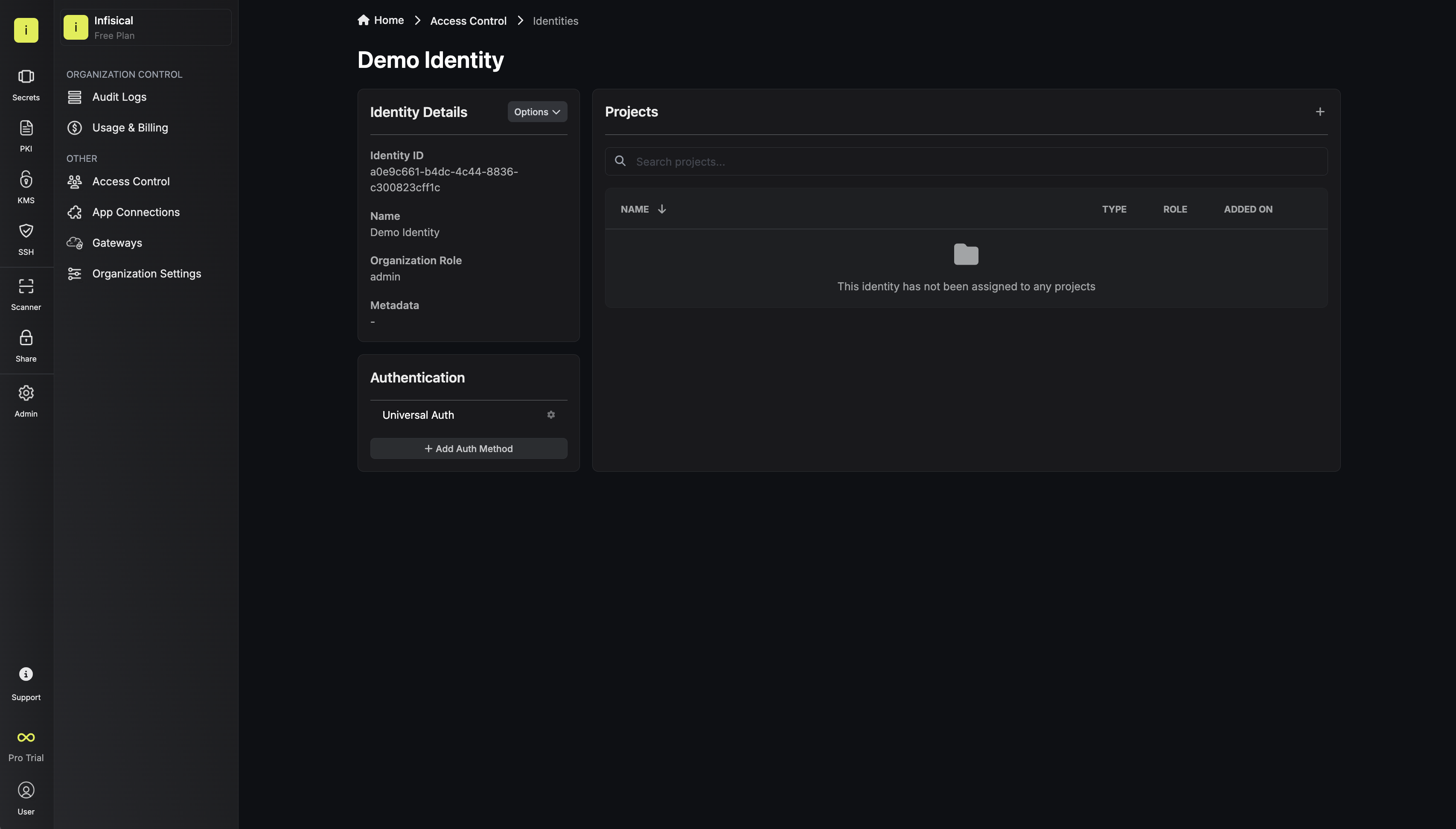
Since the identity has been configured with Universal Auth by default, you should re-configure it to use OIDC Auth instead. To do this, press to edit the **Authentication** section,
remove the existing Universal Auth configuration, and add a new OIDC Auth configuration onto the identity.
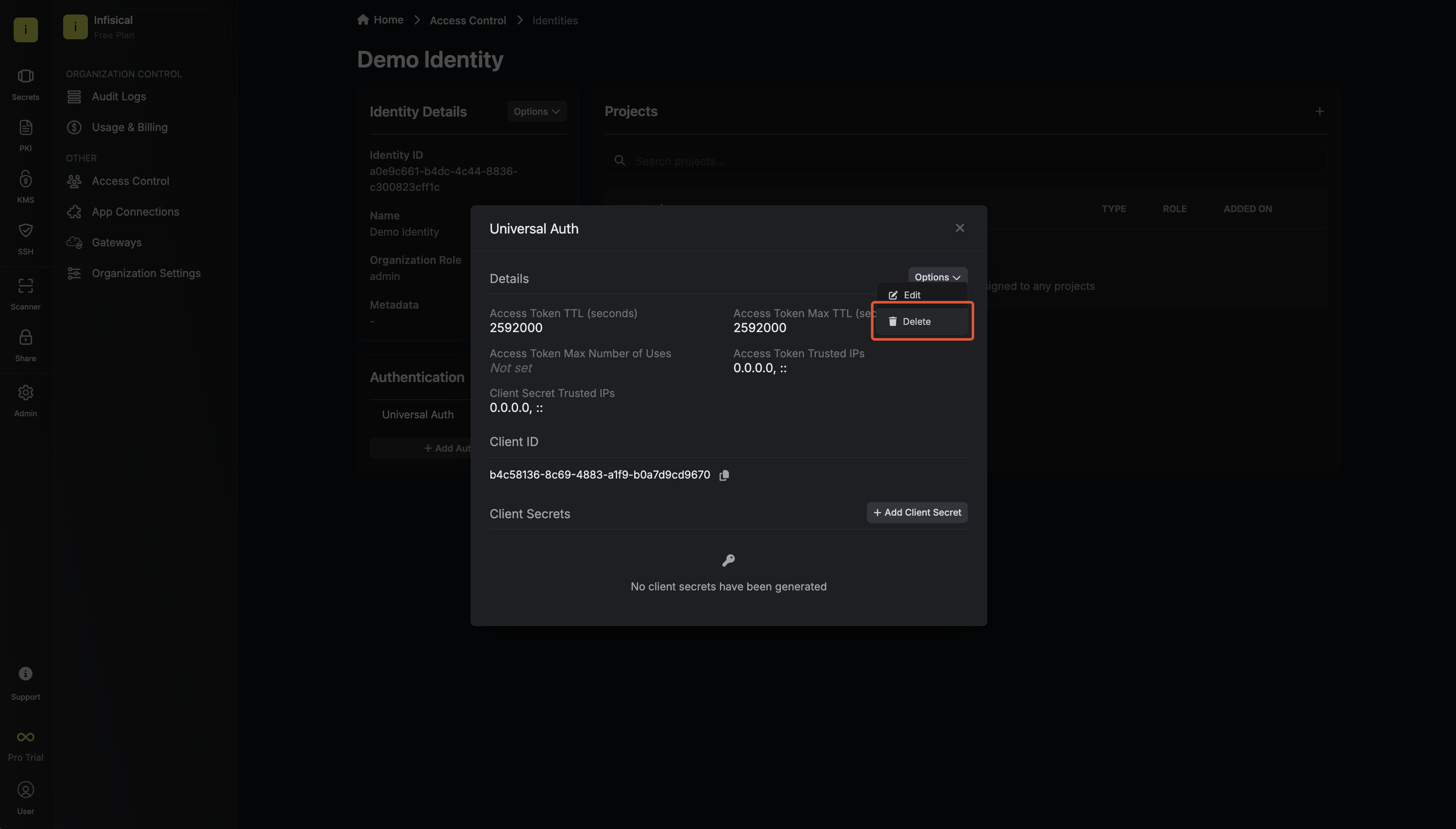
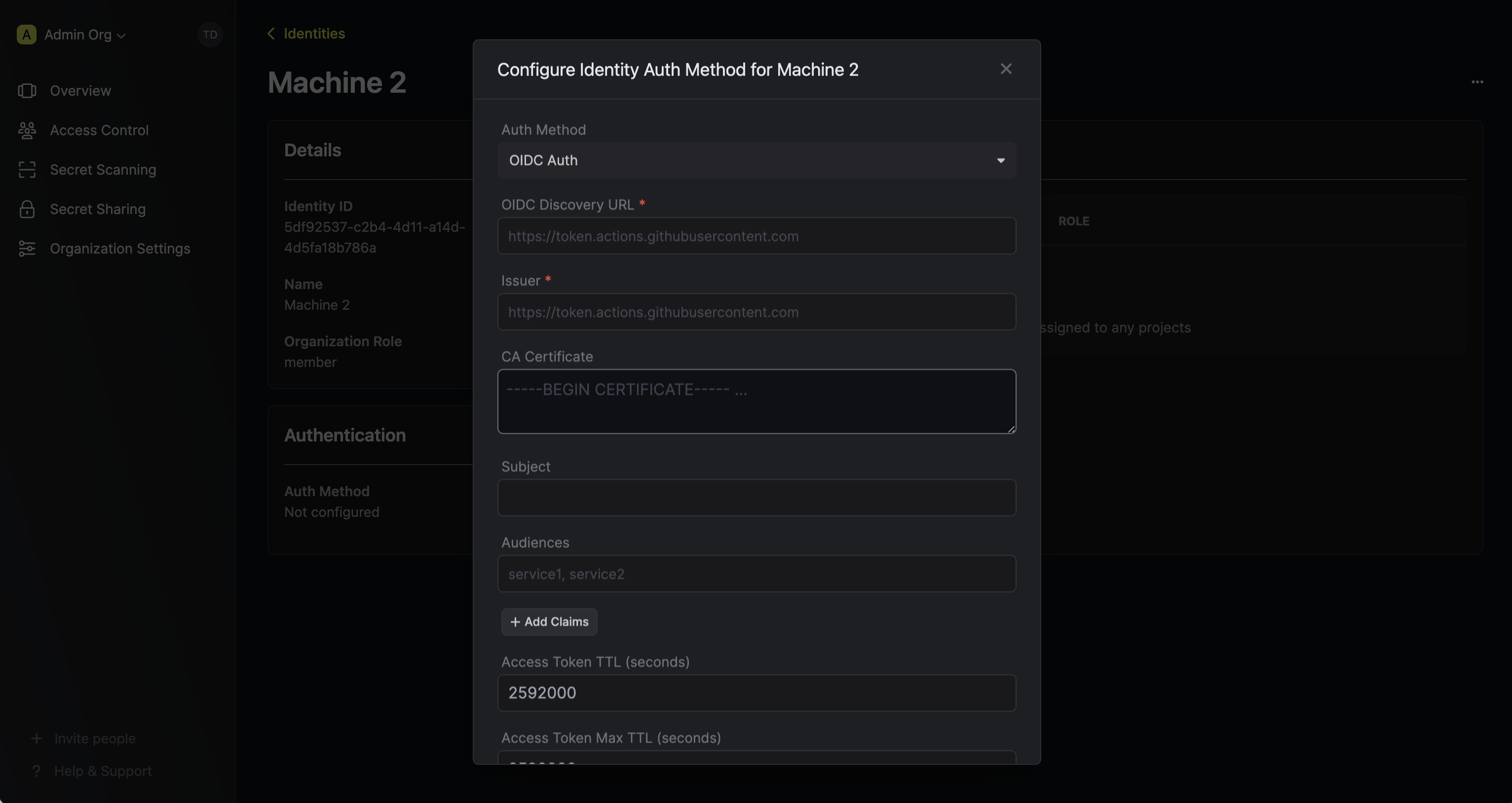
Restrict access by configuring the Subject, Audiences, and Claims fields
Here's some more guidance on each field:
* OIDC Discovery URL: The URL used to retrieve the OpenID Connect configuration from the identity provider. This will be used to fetch the public key needed for verifying the provided JWT. This should be set to `https://oidc.circleci.com/org/` where `organization_id` refers to the CircleCI organization where the job is being run.
* Issuer: The unique identifier of the identity provider issuing the JWT. This value is used to verify the iss (issuer) claim in the JWT to ensure the token is issued by a trusted provider. This should be set to `https://oidc.circleci.com/org/` as well.
* CA Certificate: The PEM-encoded CA cert for establishing secure communication with the Identity Provider endpoints. This can be left as blank.
* Subject: The expected principal that is the subject of the JWT. The format of the sub field for CircleCI OIDC tokens is `org//project//user/` where organization\_id, project\_id, and user\_id are UUIDs that identify the CircleCI organization, project, and user, respectively. The user is the CircleCI user that caused this job to run.
* Audiences: A list of intended recipients. This value is checked against the aud (audience) claim in the token. Set this to the CircleCI `organization_id` corresponding to where the job is running.
* Claims: Additional information or attributes that should be present in the JWT for it to be valid. Refer to CircleCI's [documentation](https://circleci.com/docs/openid-connect-tokens) for the complete list of supported claims.
* Access Token TTL (default is `2592000` equivalent to 30 days): The lifetime for an acccess token in seconds. This value will be referenced at renewal time.
* Access Token Max TTL (default is `2592000` equivalent to 30 days): The maximum lifetime for an acccess token in seconds. This value will be referenced at renewal time.
* Access Token Max Number of Uses (default is `0`): The maximum number of times that an access token can be used; a value of `0` implies infinite number of uses.
* Access Token Trusted IPs: The IPs or CIDR ranges that access tokens can be used from. By default, each token is given the `0.0.0.0/0`, allowing usage from any network address.
For more details on the appropriate values for the OIDC fields, refer to CircleCI's [documentation](https://circleci.com/docs/openid-connect-tokens). The `subject`, `audiences`, and `claims` fields support glob pattern matching; however, we highly recommend using hardcoded values whenever possible.
To enable the identity to access project-level resources such as secrets within a specific project, you should add it to that project.
To do this, head over to the project you want to add the identity to and go to Project Settings > Access Control > Machine Identities and press **Add identity**.
Next, select the identity you want to add to the project and the project level role you want to allow it to assume. The project role assigned will determine what project level resources this identity can have access to.
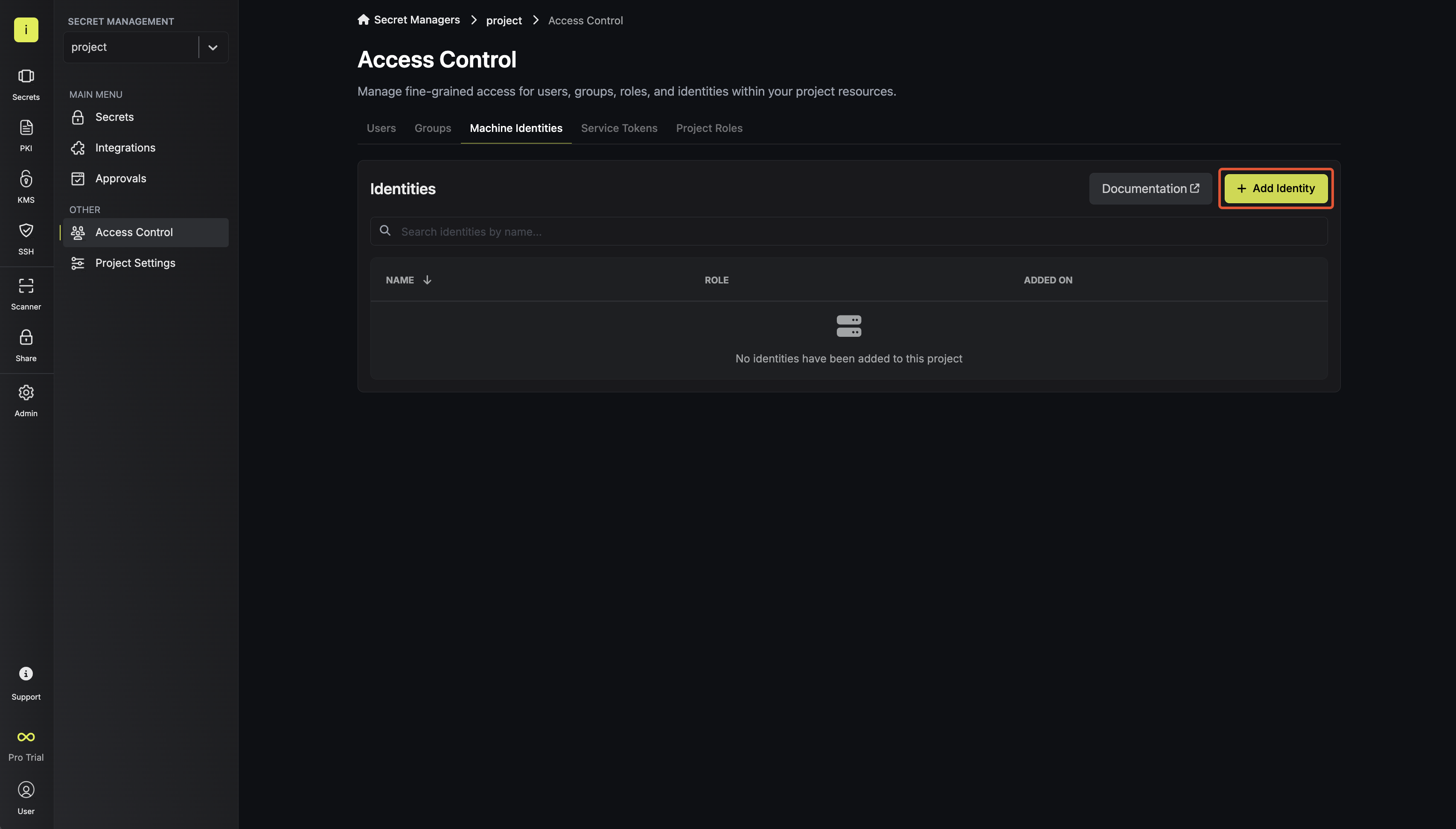
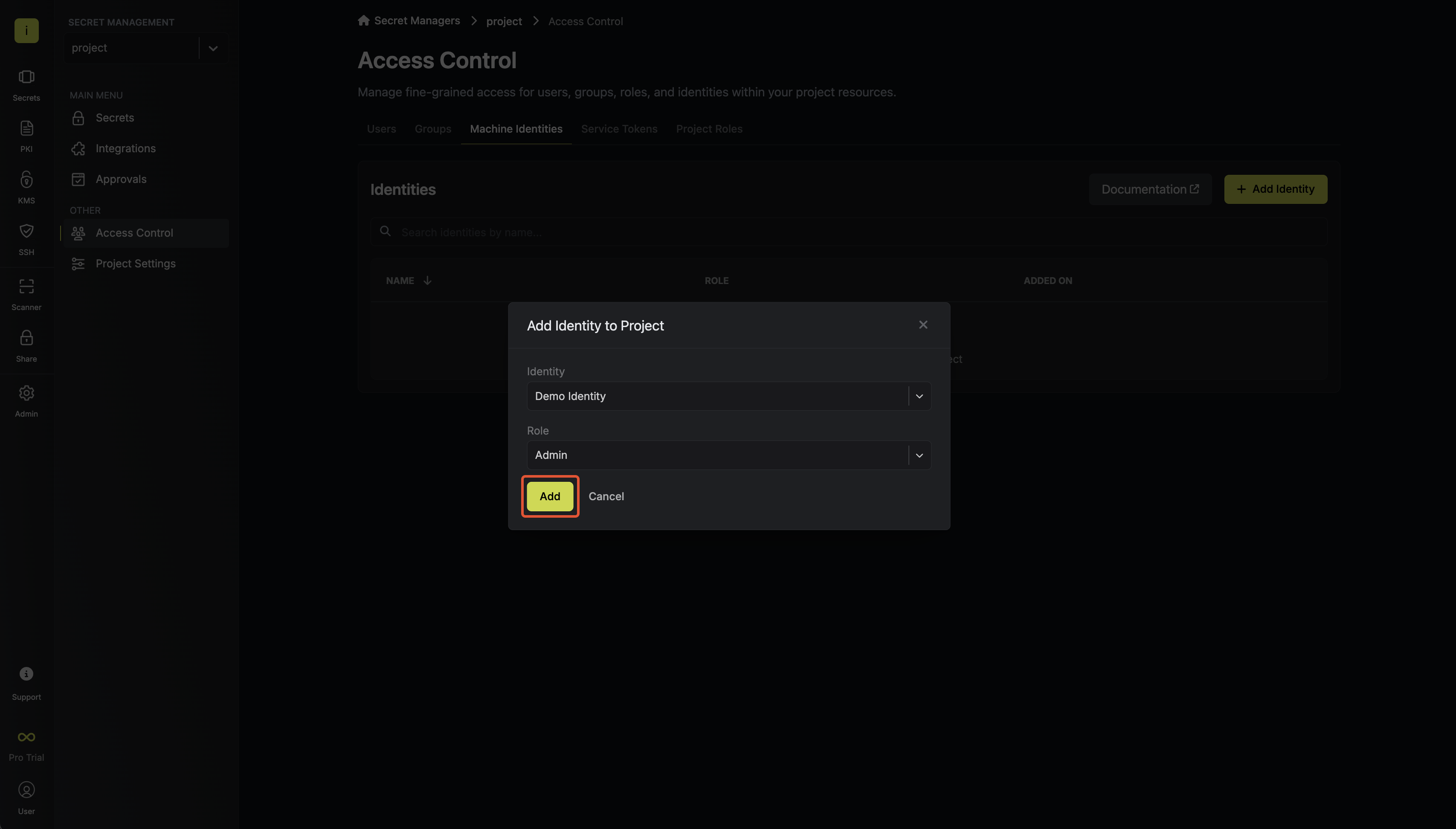
The following is an example of how to use the `$CIRCLE_OIDC_TOKEN` with the Infisical [terraform provider](https://registry.terraform.io/providers/Infisical/infisical/latest/docs) to manage resources in a CircleCI pipeline.
```yml config.yml
version: 2.1
jobs:
terraform-apply:
docker:
- image: hashicorp/terraform:latest
steps:
- checkout
- run:
command: |
export INFISICAL_AUTH_JWT="$CIRCLE_OIDC_TOKEN"
terraform init
terraform apply -auto-approve
workflows:
version: 2
build-and-test:
jobs:
- terraform-apply
```
The Infisical terraform provider expects the `INFISICAL_AUTH_JWT` environment variable to be set to the CircleCI OIDC token.
```hcl main.tf
terraform {
required_providers {
infisical = {
source = "infisical/infisical"
}
}
}
provider "infisical" {
host = "https://app.infisical.com"
auth = {
oidc = {
identity_id = "f2f5ee4c-6223-461a-87c3-406a6b481462"
}
}
}
resource "infisical_access_approval_policy" "prod-access-approval" {
project_id = "09eda1f8-85a3-47a9-8a6f-e27f133b2a36"
name = "my-approval-policy"
environment_slug = "prod"
secret_path = "/"
approvers = [
{
type = "user"
username = "sheen+200@infisical.com"
},
]
required_approvals = 1
enforcement_level = "soft"
}
```
Each identity access token has a time-to-live (TLL) which you can infer from the response of the login operation;
the default TTL is `7200` seconds which can be adjusted.
If an identity access token expires, it can no longer authenticate with the Infisical API. In this case,
a new access token should be obtained by performing another login operation.
# General
Learn how to authenticate with Infisical from any platform or environment using OpenID Connect (OIDC).
**OIDC Auth** is a platform-agnostic JWT-based authentication method that can be used to authenticate from any platform or environment using an identity provider with OpenID Connect.
## Diagram
The following sequence diagram illustrates the OIDC Auth workflow for authenticating clients with Infisical.
```mermaid
sequenceDiagram
participant Client as Client
participant Idp as Identity Provider
participant Infis as Infisical
Client->>Idp: Step 1: Request identity token
Idp-->>Client: Return JWT with verifiable claims
Note over Client,Infis: Step 2: Login Operation
Client->>Infis: Send signed JWT to /api/v1/auth/oidc-auth/login
Note over Infis,Idp: Step 3: Query verification
Infis->>Idp: Request JWT public key using OIDC Discovery
Idp-->>Infis: Return public key
Note over Infis: Step 4: JWT validation
Infis->>Client: Return short-lived access token
Note over Client,Infis: Step 5: Access Infisical API with Token
Client->>Infis: Make authenticated requests using the short-lived access token
```
## Concept
At a high-level, Infisical authenticates a client by verifying the JWT and checking that it meets specific requirements (e.g. it is issued by a trusted identity provider) at the `/api/v1/auth/oidc-auth/login` endpoint. If successful,
then Infisical returns a short-lived access token that can be used to make authenticated requests to the Infisical API.
To be more specific:
1. The client requests an identity token from its identity provider.
2. The client sends the identity token to Infisical at the `/api/v1/auth/oidc-auth/login` endpoint.
3. Infisical fetches the public key that was used to sign the identity token from the identity provider using OIDC Discovery.
4. Infisical validates the JWT using the public key provided by the identity provider and checks that the subject, audience, and claims of the token matches with the set criteria.
5. If all is well, Infisical returns a short-lived access token that the client can use to make authenticated requests to the Infisical API.
Infisical needs network-level access to the identity provider configuration
endpoints.
## Guide
In the following steps, we explore how to create and use identities to access the Infisical API using the OIDC Auth authentication method.
To create an identity, head to your Organization Settings > Access Control > Machine Identities and press **Create identity**.
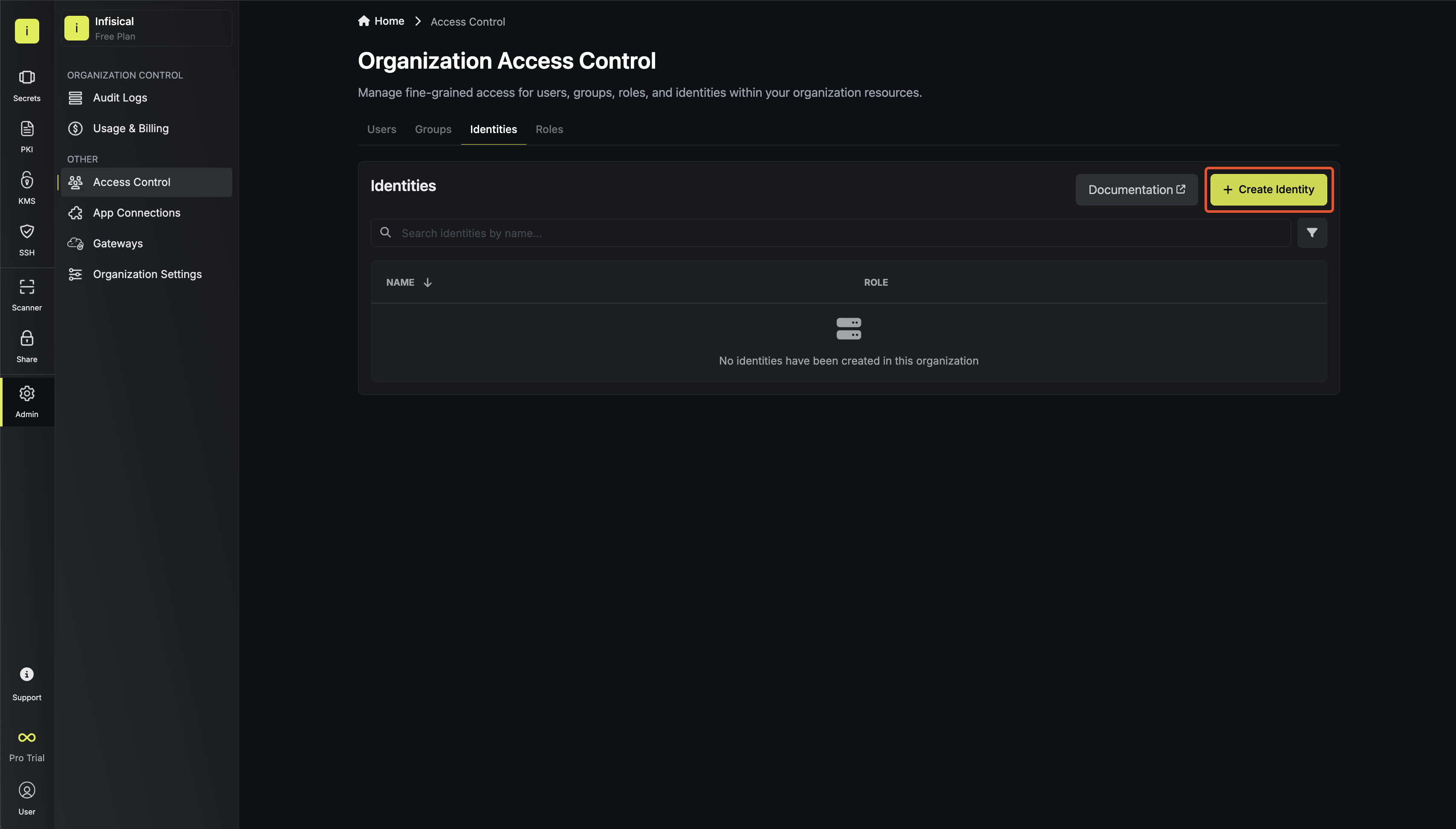
When creating an identity, you specify an organization level [role](/documentation/platform/role-based-access-controls) for it to assume; you can configure roles in Organization Settings > Access Control > Organization Roles.
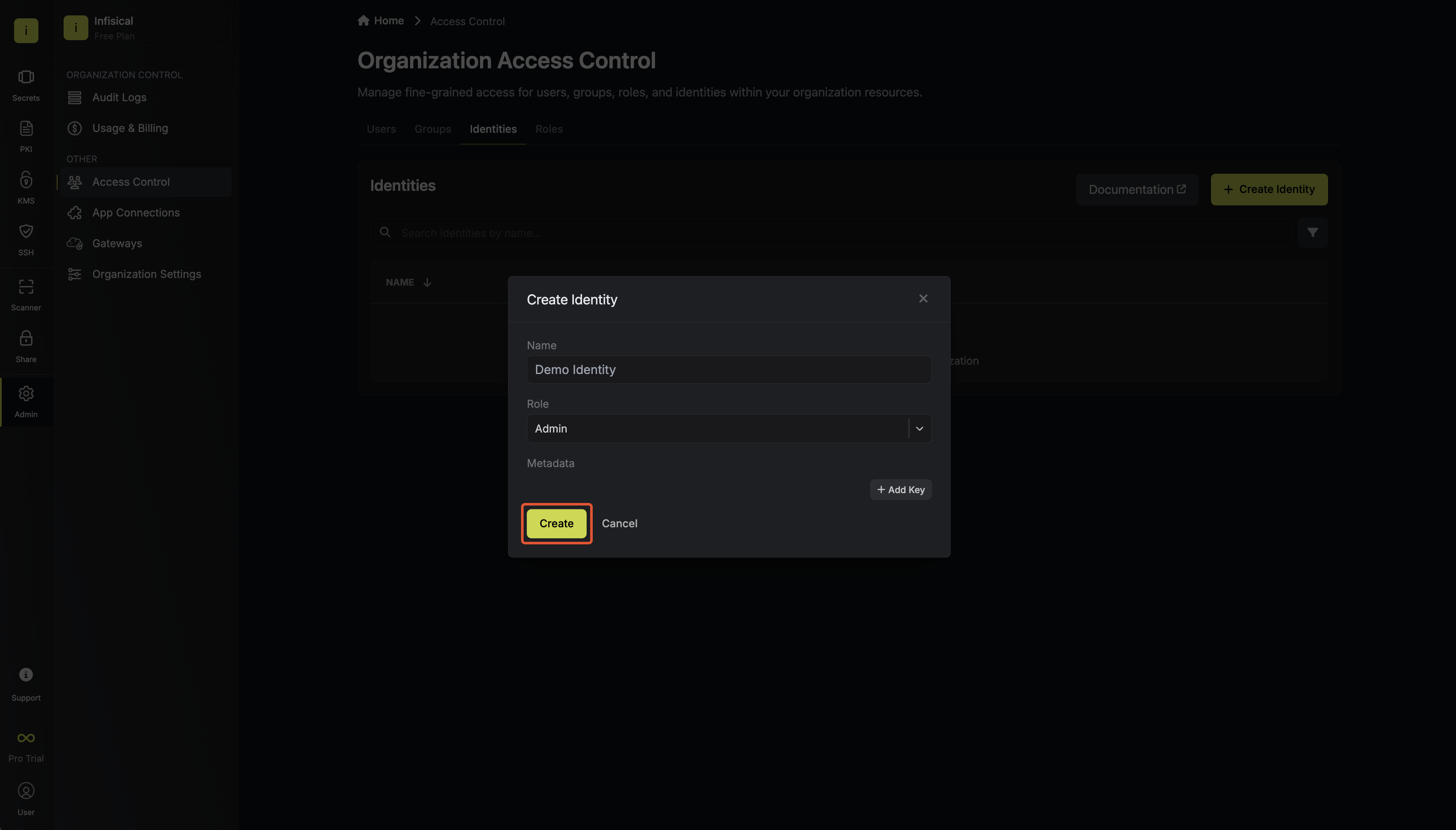
Now input a few details for your new identity. Here's some guidance for each field:
* Name (required): A friendly name for the identity.
* Role (required): A role from the **Organization Roles** tab for the identity to assume. The organization role assigned will determine what organization level resources this identity can have access to.
Once you've created an identity, you'll be redirected to a page where you can manage the identity.
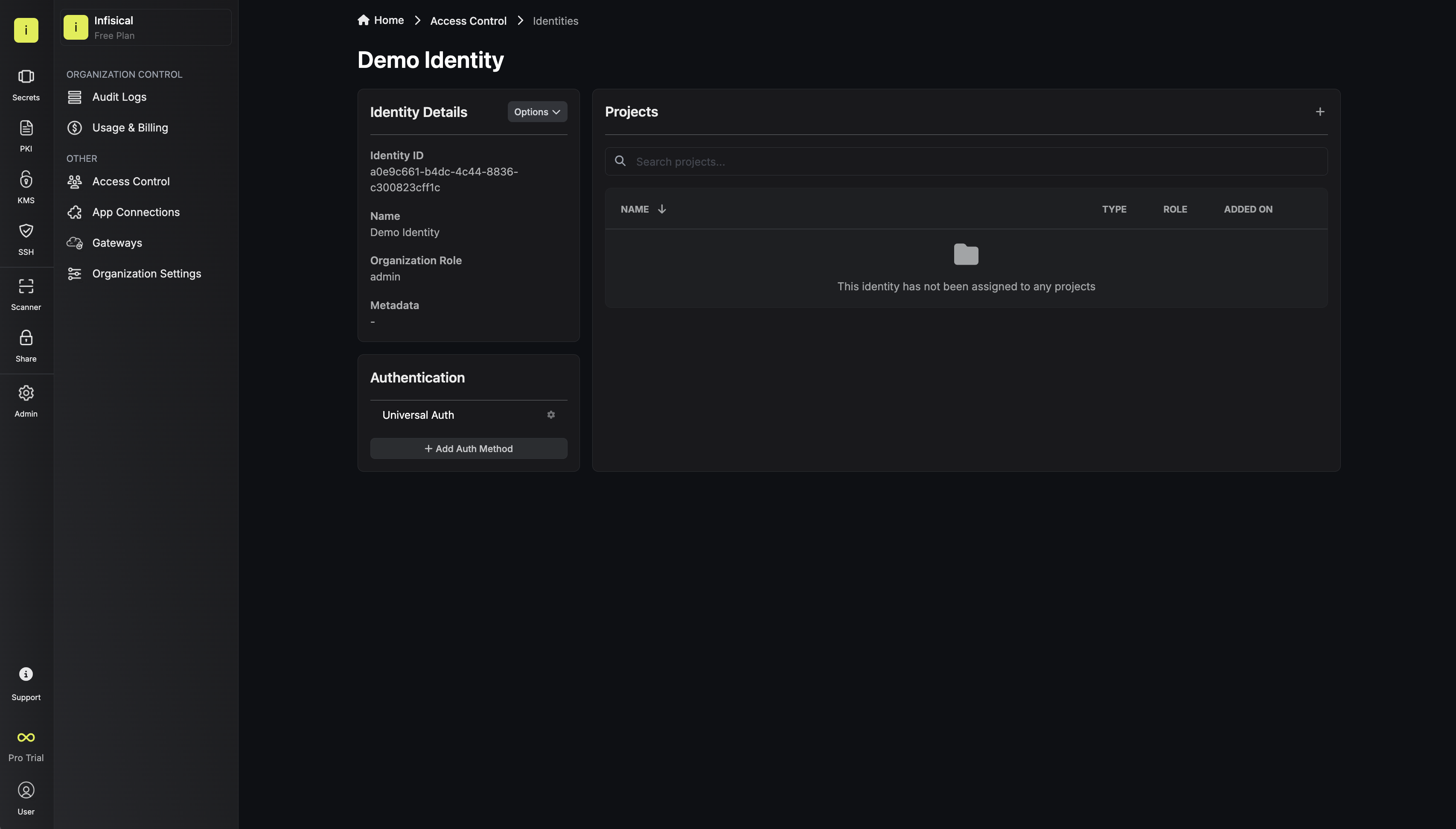
Since the identity has been configured with Universal Auth by default, you should re-configure it to use OIDC Auth instead. To do this, press to edit the **Authentication** section,
remove the existing Universal Auth configuration, and add a new OIDC Auth configuration onto the identity.
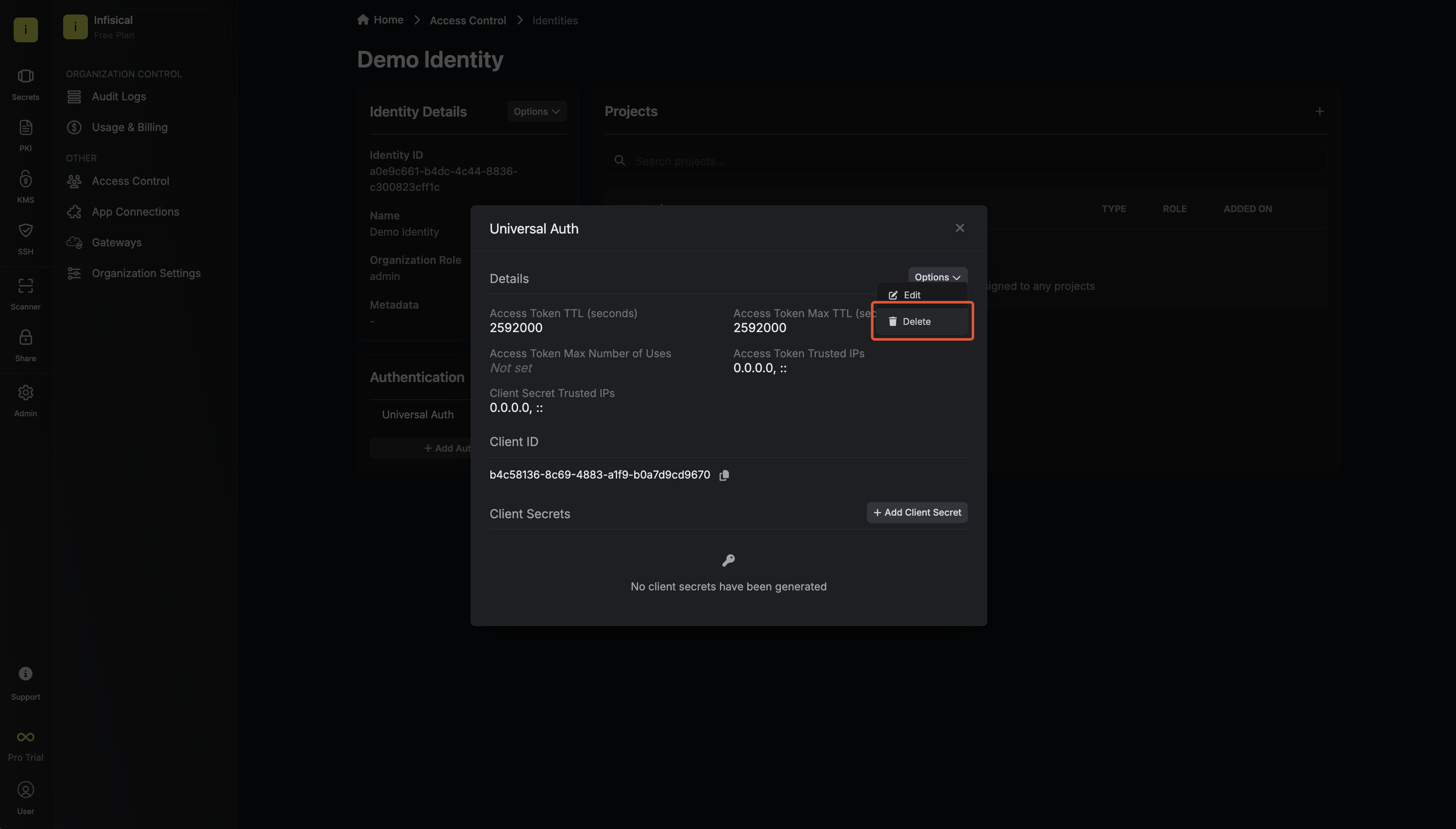
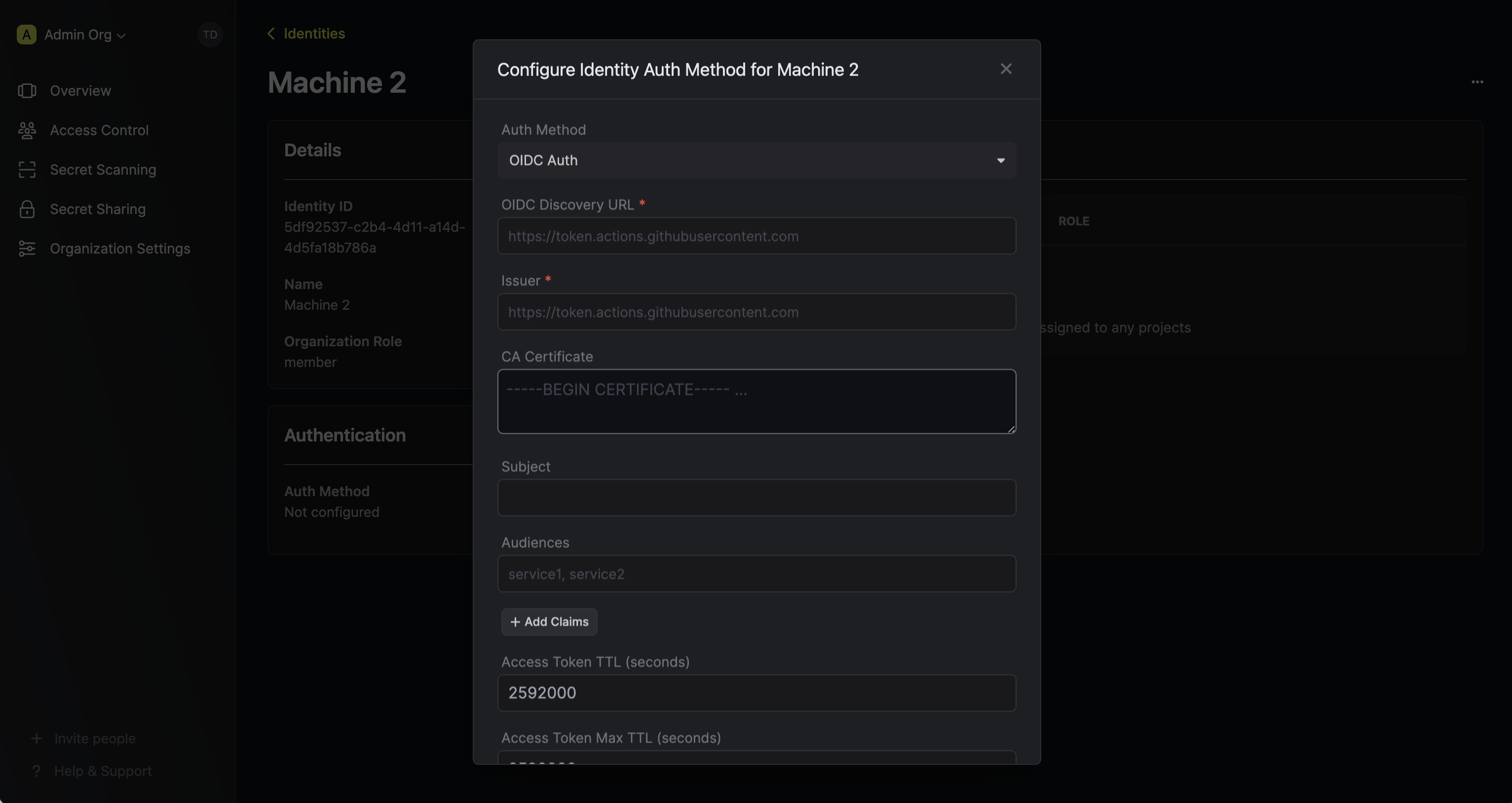
Restrict access by configuring the Subject, Audiences, and Claims fields
Here's some more guidance on each field:
* OIDC Discovery URL: The URL used to retrieve the OpenID Connect configuration from the identity provider. This will be used to fetch the public key needed for verifying the provided JWT.
* Issuer: The unique identifier of the identity provider issuing the JWT. This value is used to verify the iss (issuer) claim in the JWT to ensure the token is issued by a trusted provider.
* CA Certificate: The PEM-encoded CA cert for establishing secure communication with the Identity Provider endpoints.
* Subject: The expected principal that is the subject of the JWT. The `sub` (subject) claim in the JWT should match this value.
* Audiences: A list of intended recipients. This value is checked against the aud (audience) claim in the token. The token's aud claim should match at least one of the audiences for it to be valid.
* Claims: Additional information or attributes that should be present in the JWT for it to be valid.
* Access Token TTL (default is `2592000` equivalent to 30 days): The lifetime for an acccess token in seconds. This value will be referenced at renewal time.
* Access Token Max TTL (default is `2592000` equivalent to 30 days): The maximum lifetime for an acccess token in seconds. This value will be referenced at renewal time.
* Access Token Max Number of Uses (default is `0`): The maximum number of times that an access token can be used; a value of `0` implies infinite number of uses.
* Access Token Trusted IPs: The IPs or CIDR ranges that access tokens can be used from. By default, each token is given the `0.0.0.0/0`, allowing usage from any network address.
The `subject`, `audiences`, and `claims` fields support glob pattern matching; however, we highly recommend using hardcoded values whenever possible.
To enable the identity to access project-level resources such as secrets within a specific project, you should add it to that project.
To do this, head over to the project you want to add the identity to and go to Project Settings > Access Control > Machine Identities and press **Add identity**.
Next, select the identity you want to add to the project and the project level role you want to allow it to assume. The project role assigned will determine what project level resources this identity can have access to.
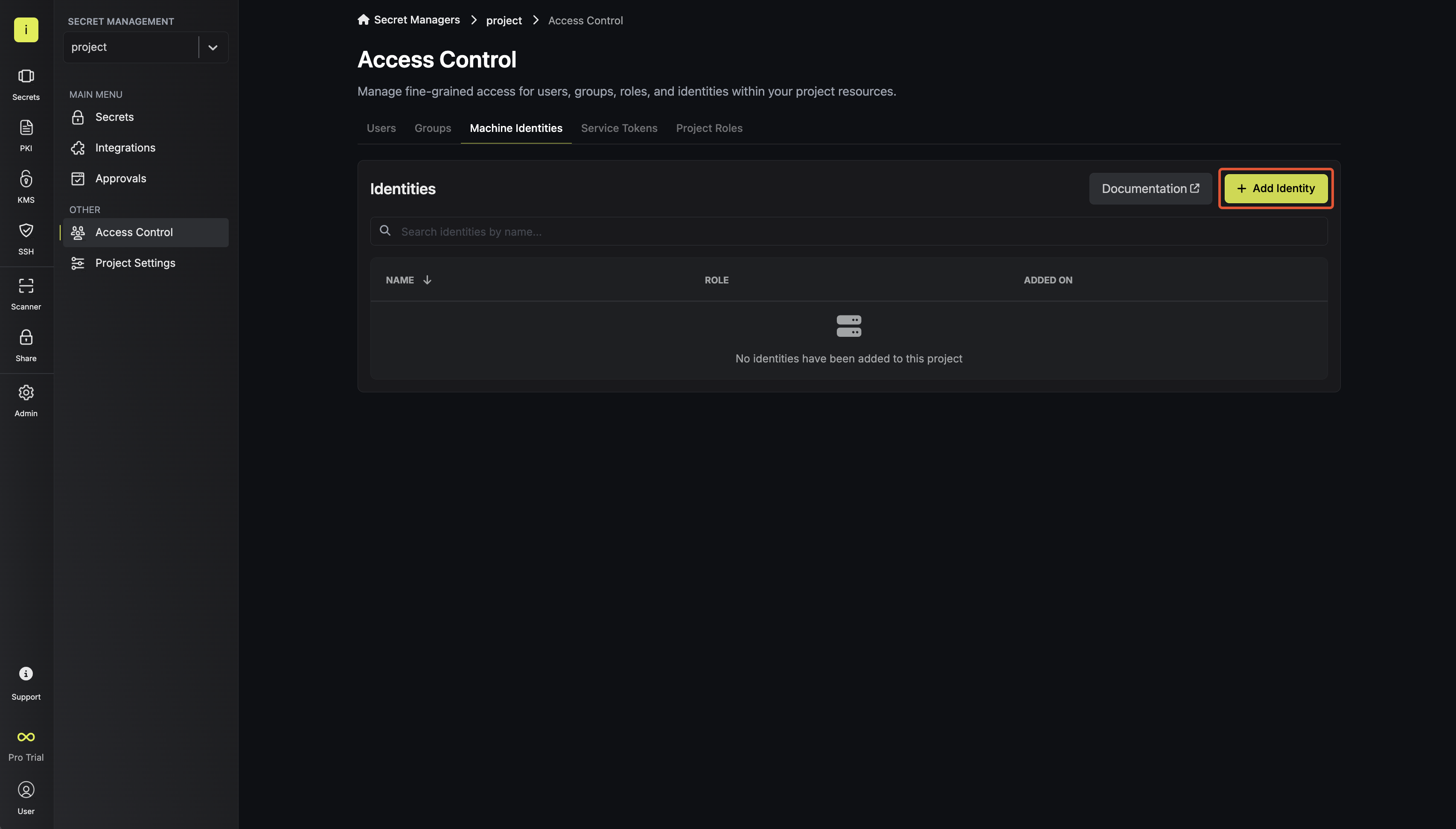
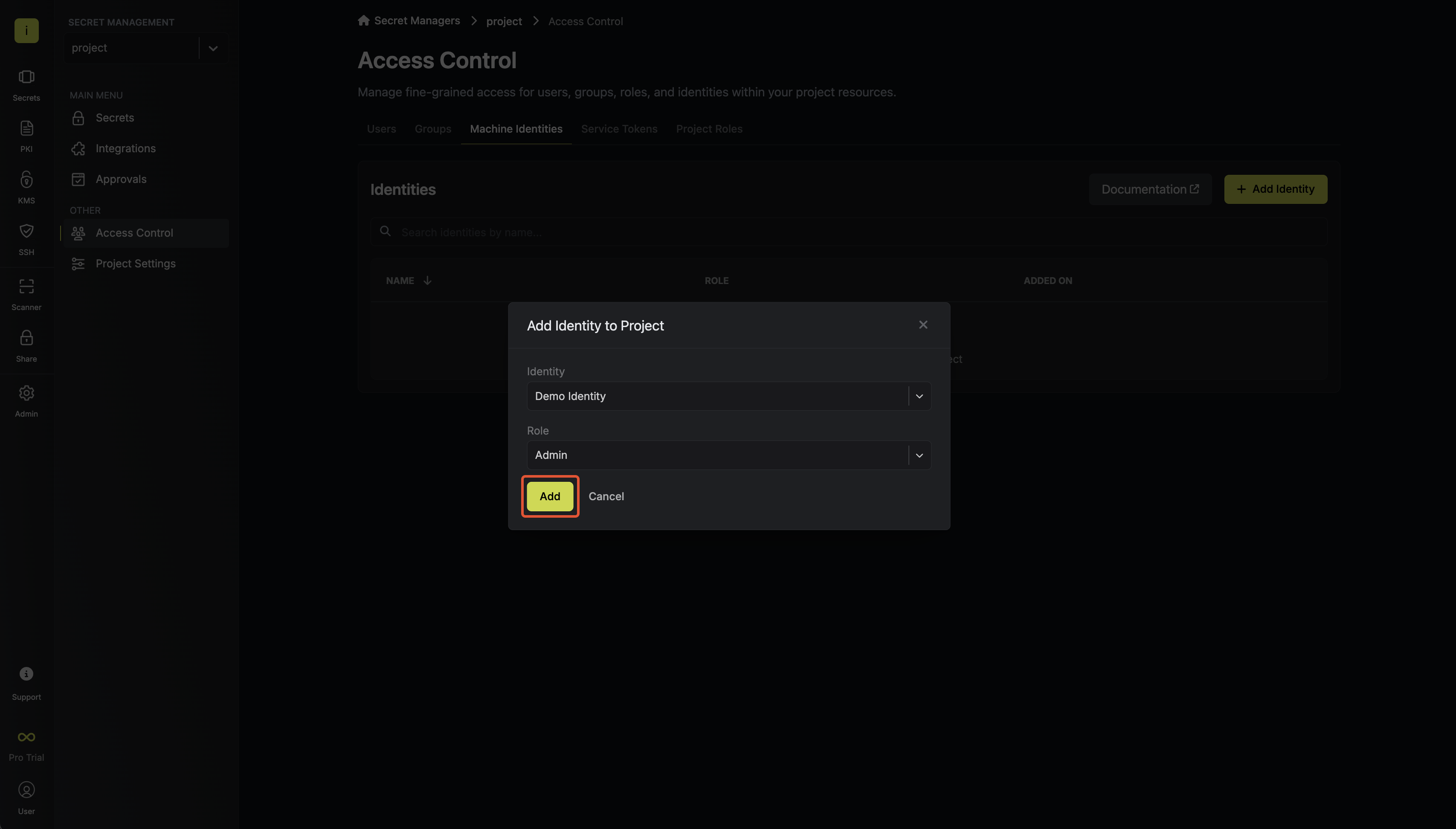
To access the Infisical API as the identity, you need to fetch an identity token from an identity provider and make a request to the `/api/v1/auth/oidc-auth/login` endpoint in exchange for an access token.
We provide an example below of how authentication is done with Infisical using OIDC. It is a snippet from the [official Github secrets action](https://github.com/Infisical/secrets-action).
#### Sample usage
```javascript
export const oidcLogin = async ({ identityId, domain, oidcAudience }) => {
const idToken = await core.getIDToken(oidcAudience);
const loginData = querystring.stringify({
identityId,
jwt: idToken,
});
try {
const response = await axios({
method: "post",
url: `${domain}/api/v1/auth/oidc-auth/login`,
headers: {
"Content-Type": "application/x-www-form-urlencoded",
},
data: loginData,
});
return response.data.accessToken;
} catch (err) {
core.error("Error:", err.message);
throw err;
}
};
```
#### Sample OIDC login response
```bash Response
{
"accessToken": "...",
"expiresIn": 7200,
"accessTokenMaxTTL": 43244
"tokenType": "Bearer"
}
```
We recommend using one of Infisical's clients like SDKs or the Infisical Agent to authenticate with Infisical using OIDC Auth as they handle the authentication process including the fetching of identity tokens for you.
Each identity access token has a time-to-live (TLL) which you can infer from the response of the login operation;
the default TTL is `7200` seconds which can be adjusted.
If an identity access token expires, it can no longer authenticate with the Infisical API. In this case,
a new access token should be obtained by performing another login operation.
# Github
Learn how to authenticate Github workflows with Infisical using OpenID Connect (OIDC).
**OIDC Auth** is a platform-agnostic JWT-based authentication method that can be used to authenticate from any platform or environment using an identity provider with OpenID Connect.
## Diagram
The following sequence diagram illustrates the OIDC Auth workflow for authenticating Github workflows with Infisical.
```mermaid
sequenceDiagram
participant Client as Github Workflow
participant Idp as Identity Provider
participant Infis as Infisical
Client->>Idp: Step 1: Request identity token
Idp-->>Client: Return JWT with verifiable claims
Note over Client,Infis: Step 2: Login Operation
Client->>Infis: Send signed JWT to /api/v1/auth/oidc-auth/login
Note over Infis,Idp: Step 3: Query verification
Infis->>Idp: Request JWT public key using OIDC Discovery
Idp-->>Infis: Return public key
Note over Infis: Step 4: JWT validation
Infis->>Client: Return short-lived access token
Note over Client,Infis: Step 5: Access Infisical API with Token
Client->>Infis: Make authenticated requests using the short-lived access token
```
## Concept
At a high-level, Infisical authenticates a client by verifying the JWT and checking that it meets specific requirements (e.g. it is issued by a trusted identity provider) at the `/api/v1/auth/oidc-auth/login` endpoint. If successful,
then Infisical returns a short-lived access token that can be used to make authenticated requests to the Infisical API.
To be more specific:
1. The Github workflow requests an identity token from Github's identity provider.
2. The fetched identity token is sent to Infisical at the `/api/v1/auth/oidc-auth/login` endpoint.
3. Infisical fetches the public key that was used to sign the identity token from Github's identity provider using OIDC Discovery.
4. Infisical validates the JWT using the public key provided by the identity provider and checks that the subject, audience, and claims of the token matches with the set criteria.
5. If all is well, Infisical returns a short-lived access token that the Github workflow can use to make authenticated requests to the Infisical API.
Infisical needs network-level access to Github's identity provider endpoints.
## Guide
In the following steps, we explore how to create and use identities to access the Infisical API using the OIDC Auth authentication method.
To create an identity, head to your Organization Settings > Access Control > Machine Identities and press **Create identity**.
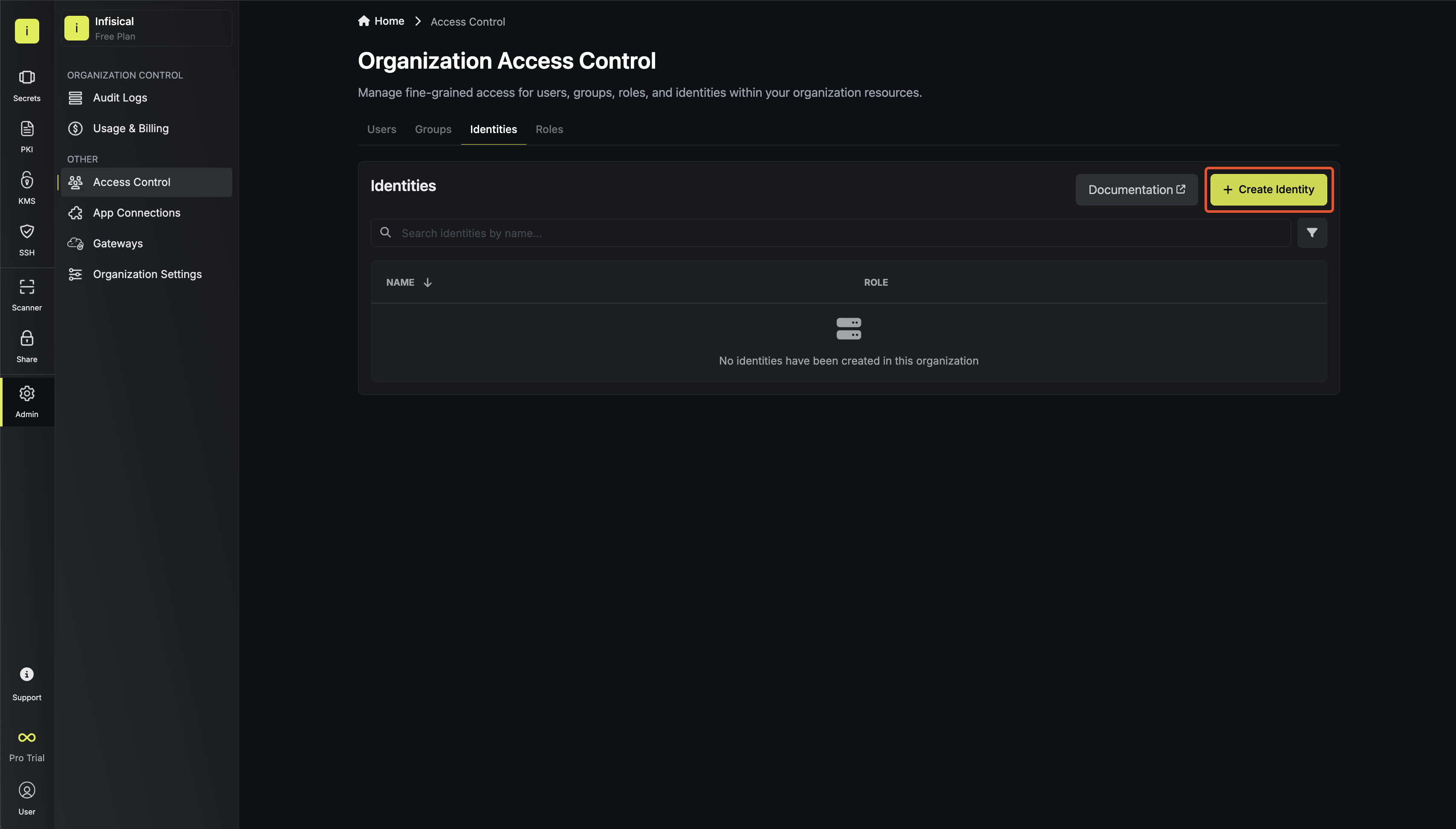
When creating an identity, you specify an organization level [role](/documentation/platform/role-based-access-controls) for it to assume; you can configure roles in Organization Settings > Access Control > Organization Roles.
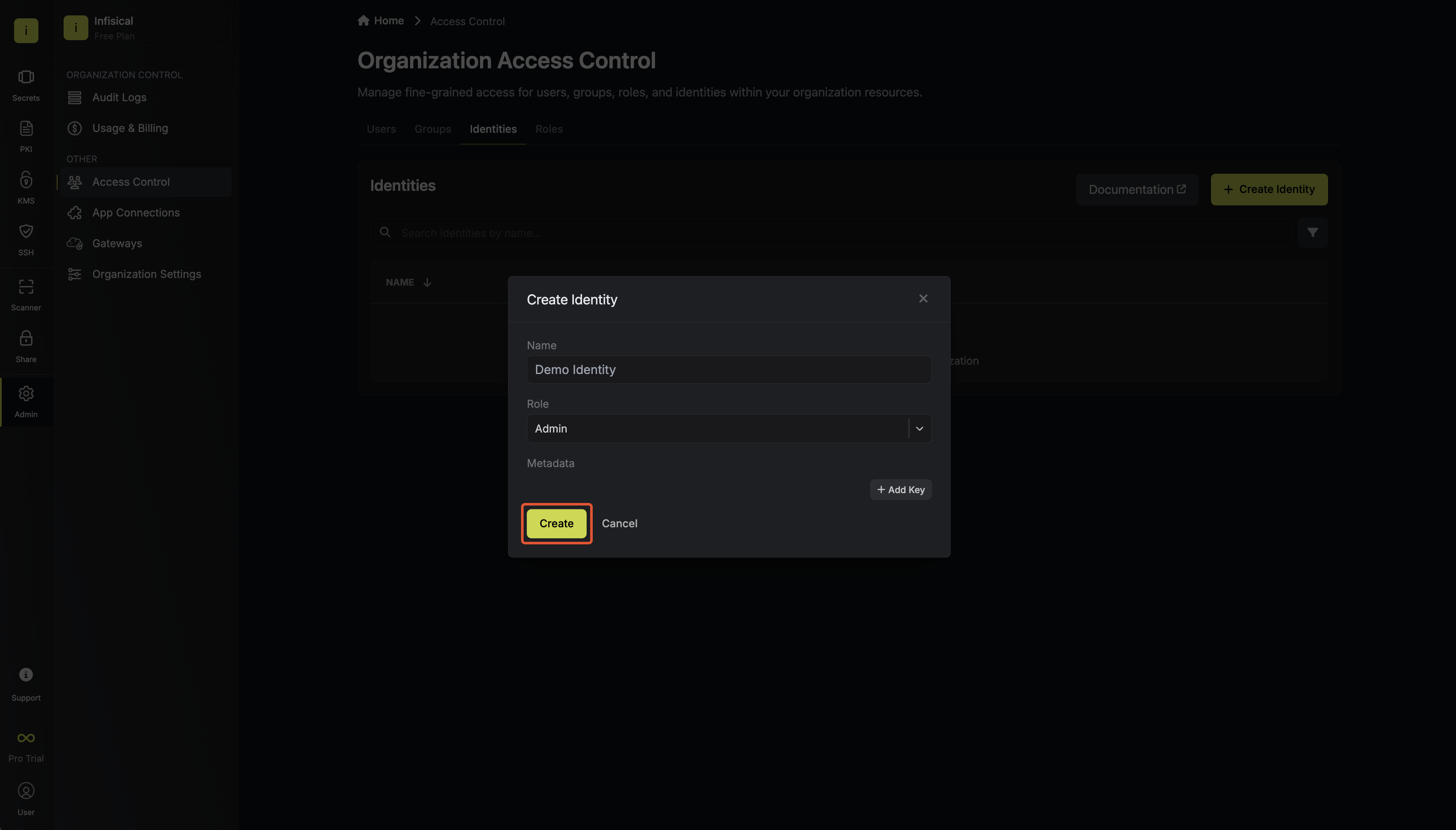
Now input a few details for your new identity. Here's some guidance for each field:
* Name (required): A friendly name for the identity.
* Role (required): A role from the **Organization Roles** tab for the identity to assume. The organization role assigned will determine what organization level resources this identity can have access to.
Once you've created an identity, you'll be redirected to a page where you can manage the identity.
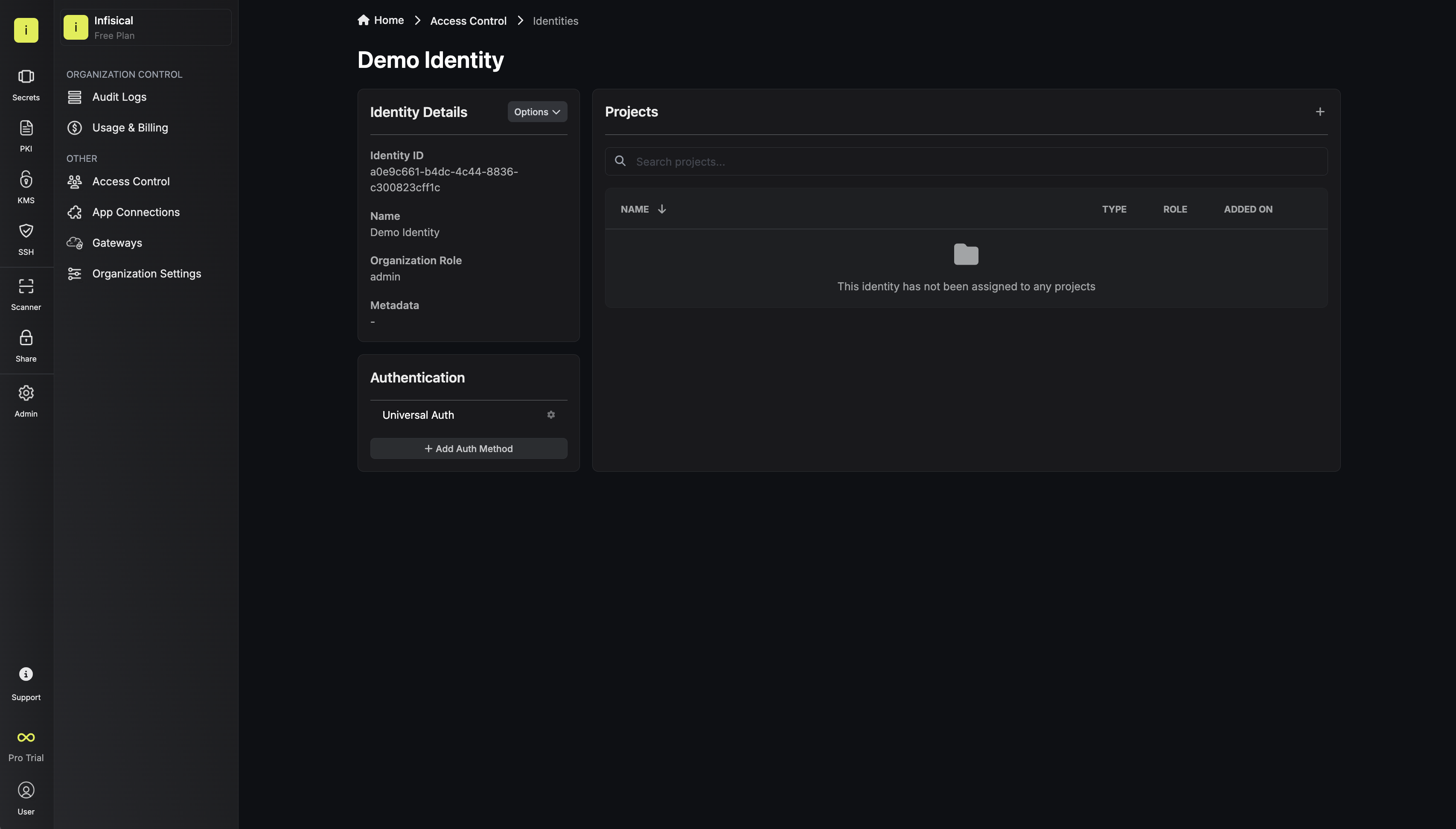
Since the identity has been configured with Universal Auth by default, you should re-configure it to use OIDC Auth instead. To do this, press to edit the **Authentication** section,
remove the existing Universal Auth configuration, and add a new OIDC Auth configuration onto the identity.
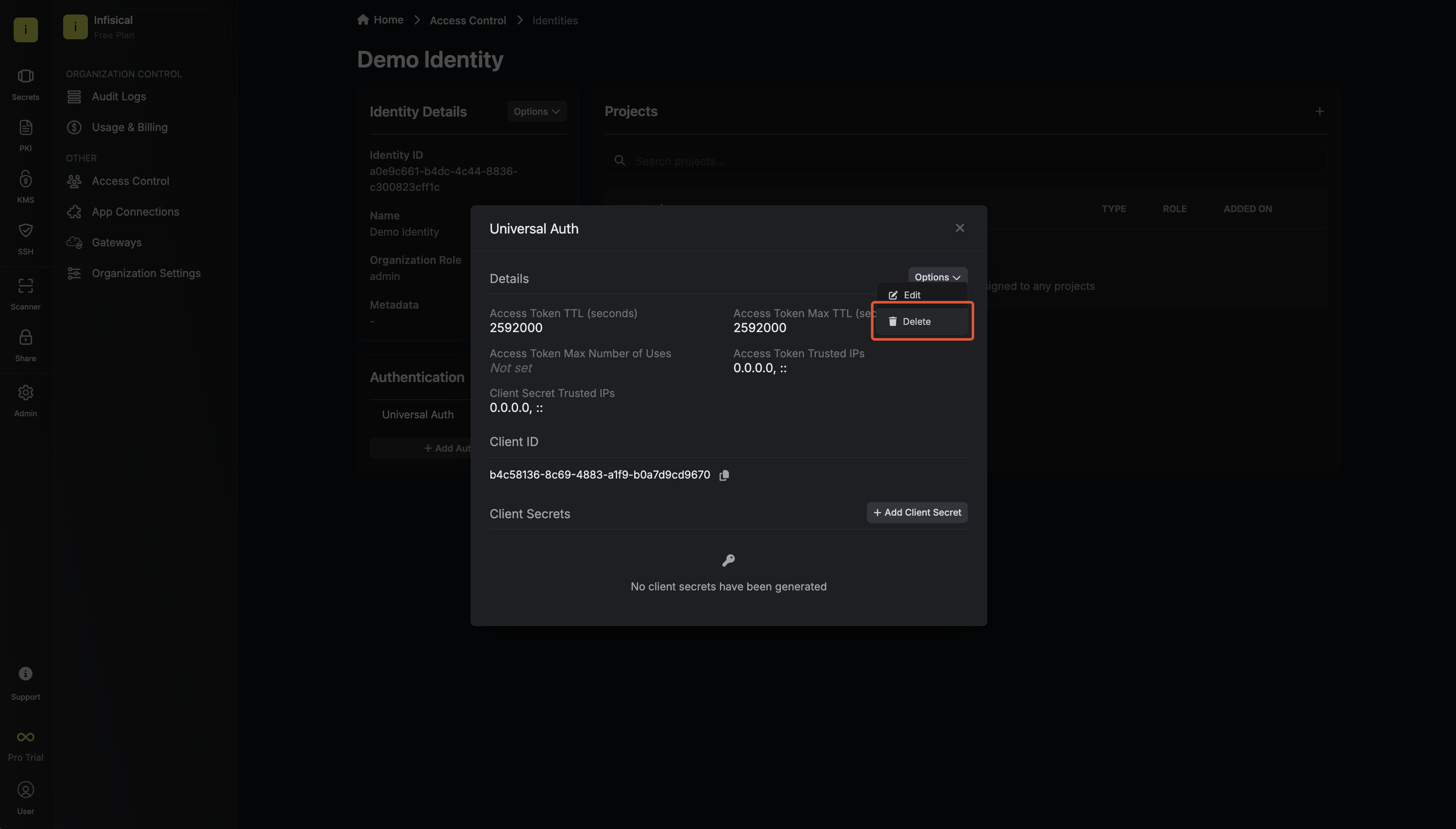
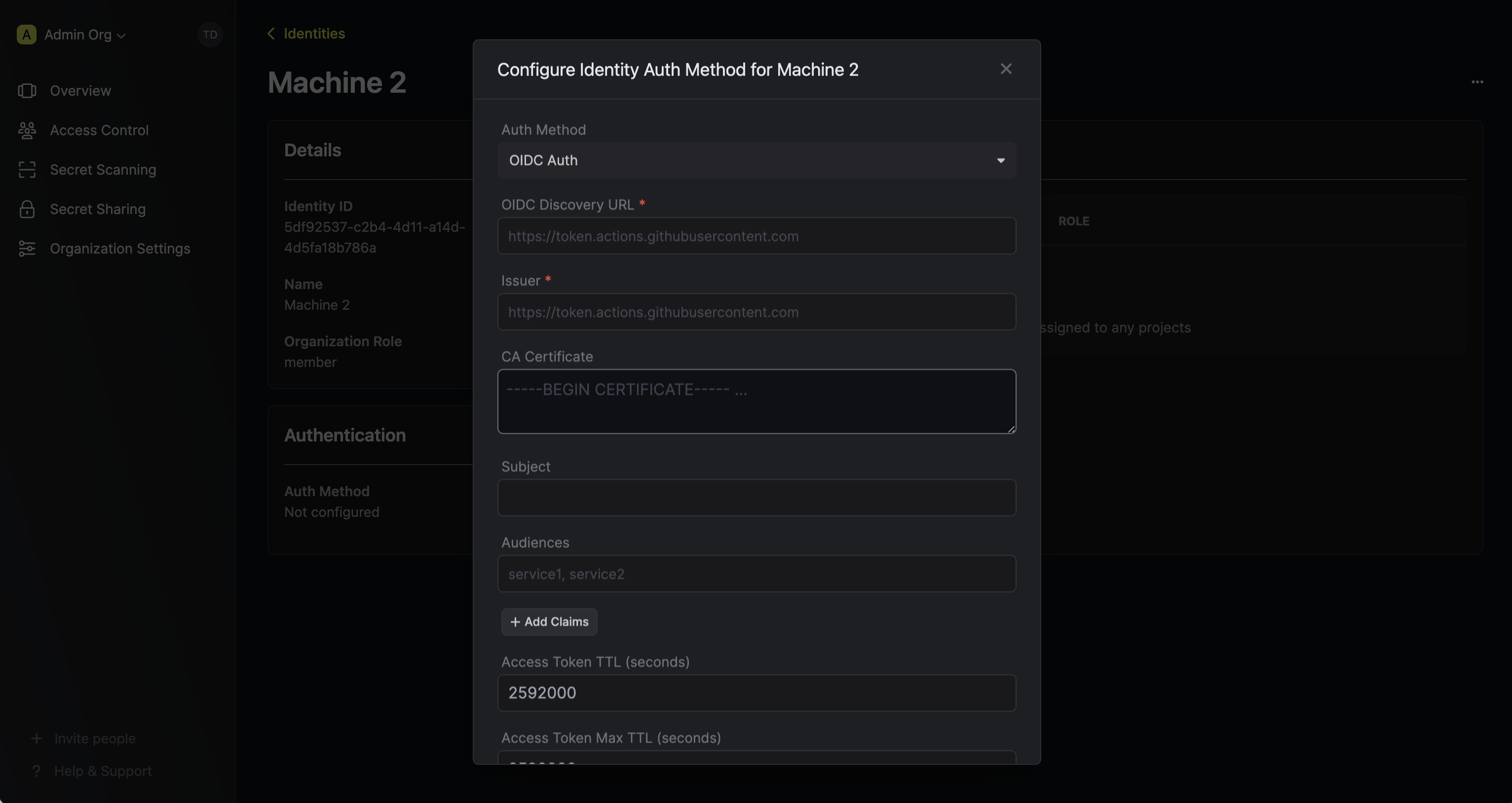
Restrict access by configuring the Subject, Audiences, and Claims fields
Here's some more guidance on each field:
* OIDC Discovery URL: The URL used to retrieve the OpenID Connect configuration from the identity provider. This will be used to fetch the public key needed for verifying the provided JWT. This should be set to `https://token.actions.githubusercontent.com`
* Issuer: The unique identifier of the identity provider issuing the JWT. This value is used to verify the iss (issuer) claim in the JWT to ensure the token is issued by a trusted provider. This should be set to `https://token.actions.githubusercontent.com`
* CA Certificate: The PEM-encoded CA cert for establishing secure communication with the Identity Provider endpoints. For Github workflows, this can be left as blank.
* Subject: The expected principal that is the subject of the JWT. The format of the sub field for GitHub workflow OIDC tokens is as follows: `"repo:/:"`. The environment can be where the GitHub workflow is running, such as `environment`, `ref`, or `job_workflow_ref`. For example, if you have a repository owned by octocat named example-repo, and the GitHub workflow is running on the main branch, the subject field might look like this: `repo:octocat/example-repo:ref:refs/heads/main`
* Audiences: A list of intended recipients. This value is checked against the aud (audience) claim in the token. By default, set this to the URL of the repository owner, such as the organization that owns the repository (e.g. `https://github.com/octo-org`).
* Claims: Additional information or attributes that should be present in the JWT for it to be valid. You can refer to Github's [documentation](https://docs.github.com/en/actions/deployment/security-hardening-your-deployments/about-security-hardening-with-openid-connect#understanding-the-oidc-token) for the complete list of supported claims.
* Access Token TTL (default is `2592000` equivalent to 30 days): The lifetime for an acccess token in seconds. This value will be referenced at renewal time.
* Access Token Max TTL (default is `2592000` equivalent to 30 days): The maximum lifetime for an acccess token in seconds. This value will be referenced at renewal time.
* Access Token Max Number of Uses (default is `0`): The maximum number of times that an access token can be used; a value of `0` implies infinite number of uses.
* Access Token Trusted IPs: The IPs or CIDR ranges that access tokens can be used from. By default, each token is given the `0.0.0.0/0`, allowing usage from any network address.
If you are unsure about what to configure for the subject, audience, and claims fields you can use [github/actions-oidc-debugger](https://github.com/github/actions-oidc-debugger) to get the appropriate values. Alternatively, you can fetch the JWT from the workflow and inspect the fields manually.The `subject`, `audiences`, and `claims` fields support glob pattern matching; however, we highly recommend using hardcoded values whenever possible.
To enable the identity to access project-level resources such as secrets within a specific project, you should add it to that project.
To do this, head over to the project you want to add the identity to and go to Project Settings > Access Control > Machine Identities and press **Add identity**.
Next, select the identity you want to add to the project and the project level role you want to allow it to assume. The project role assigned will determine what project level resources this identity can have access to.
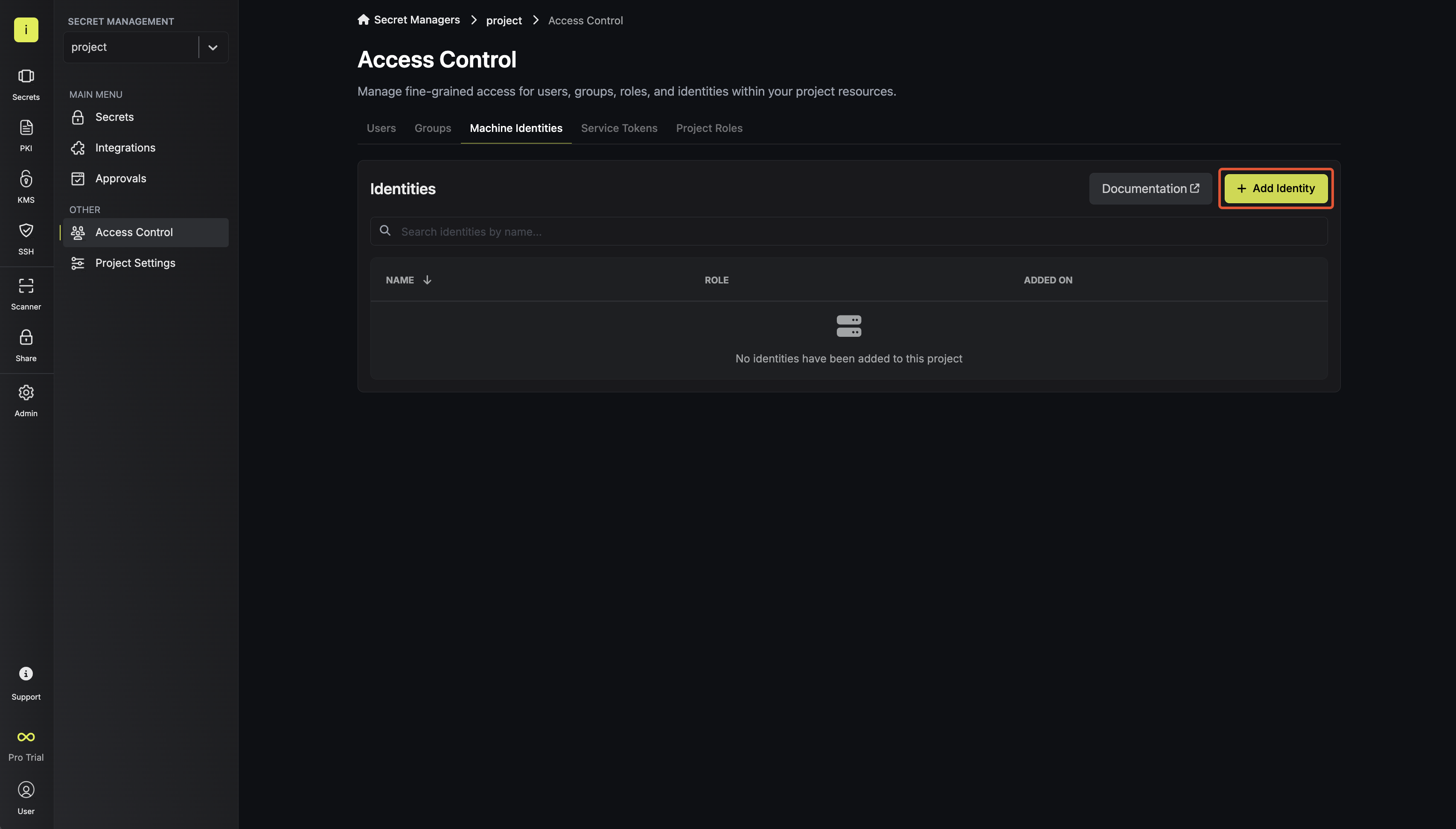
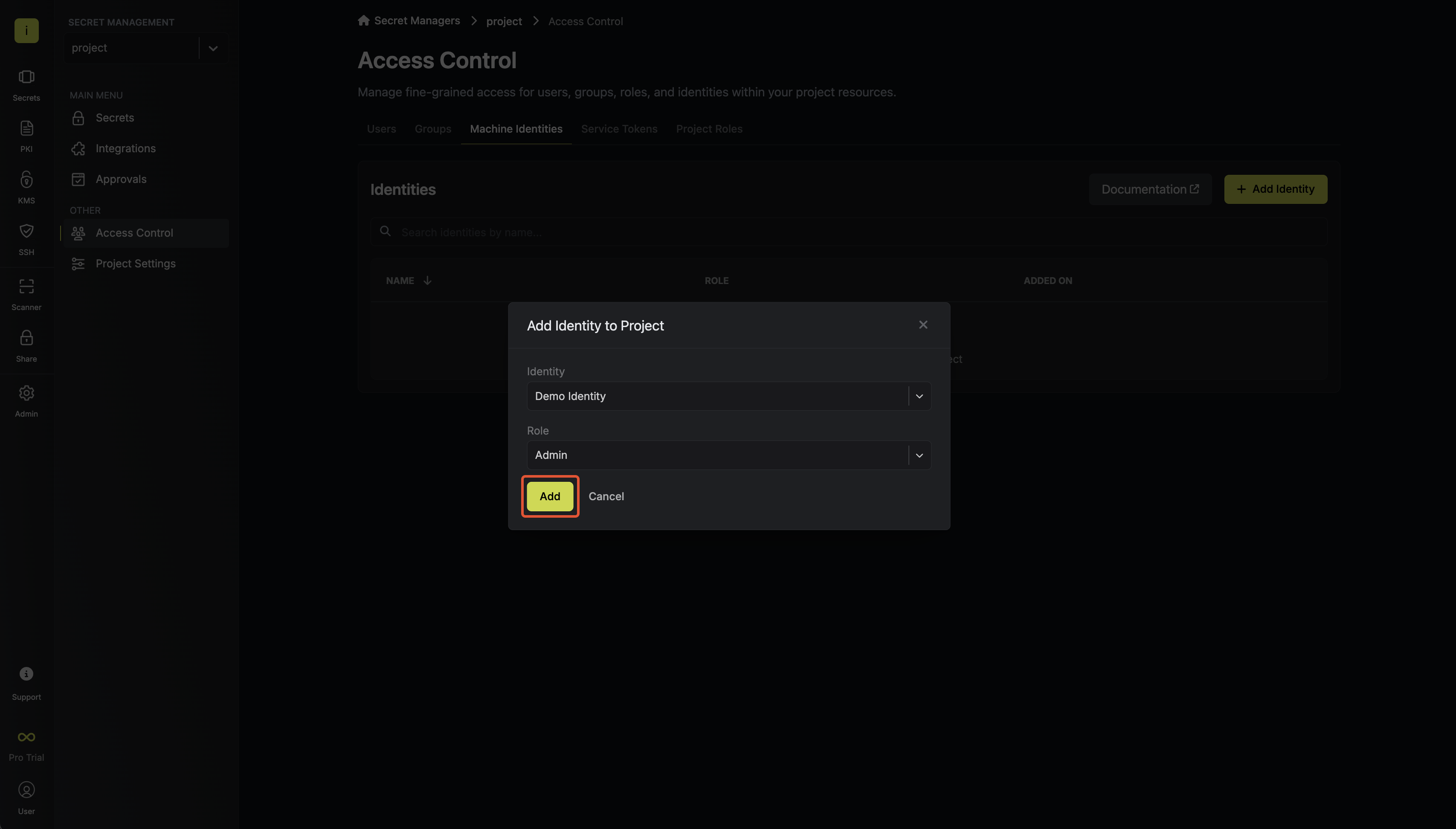
As a prerequisite, you will need to set `id-token:write` permissions for the Github workflow. This setting allows the JWT to be requested from Github's OIDC provider.
```yaml
permissions:
id-token: write # This is required for requesting the JWT
...
```
To access the Infisical API as the identity, you need to fetch an identity token from Github's identity provider and make a request to the `/api/v1/auth/oidc-auth/login` endpoint in exchange for an access token.
The identity token can be fetched using either of the following approaches:
* Using environment variables on the runner (`ACTIONS_ID_TOKEN_REQUEST_URL` and `ACTIONS_ID_TOKEN_REQUEST_TOKEN`).
```yaml
steps:
- name: Request OIDC Token
run: |
echo "Requesting OIDC token..."
TOKEN=$(curl -s -H "Authorization: Bearer $ACTIONS_ID_TOKEN_REQUEST_TOKEN" "$ACTIONS_ID_TOKEN_REQUEST_URL" | jq -r '.value')
echo "TOKEN=$TOKEN" >> $GITHUB_ENV
```
* Using `getIDToken()` from the Github Actions toolkit.
Below is an example of how a Github workflow can be configured to fetch secrets from Infisical using the [Infisical Secrets Action](https://github.com/Infisical/secrets-action) with OIDC Auth.
```yaml
name: Manual workflow
on:
workflow_dispatch:
permissions:
id-token: write # This is required for requesting the JWT
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: Infisical/secrets-action@v1.0.7
with:
method: "oidc"
env-slug: "dev"
project-slug: "ggggg-9-des"
identity-id: "6b579c00-5c85-4b44-aabe-f8a
...
```
Preceding steps can then use the secret values injected onto the workflow's environment.
We recommend using [Infisical Secrets Action](https://github.com/Infisical/secrets-action) to authenticate with Infisical using OIDC Auth as it handles the authentication process including the fetching of identity tokens for you.
Each identity access token has a time-to-live (TLL) which you can infer from the response of the login operation;
the default TTL is `7200` seconds which can be adjusted.
If an identity access token expires, it can no longer authenticate with the Infisical API. In this case,
a new access token should be obtained by performing another login operation.
# GitLab
Learn how to authenticate GitLab pipelines with Infisical using OpenID Connect (OIDC).
**OIDC Auth** is a platform-agnostic JWT-based authentication method that can be used to authenticate from any platform or environment using an identity provider with OpenID Connect.
## Diagram
The following sequence diagram illustrates the OIDC Auth workflow for authenticating GitLab pipelines with Infisical.
```mermaid
sequenceDiagram
participant Client as GitLab Pipeline
participant Idp as GitLab Identity Provider
participant Infis as Infisical
Client->>Idp: Step 1: Request identity token
Idp-->>Client: Return JWT with verifiable claims
Note over Client,Infis: Step 2: Login Operation
Client->>Infis: Send signed JWT to /api/v1/auth/oidc-auth/login
Note over Infis,Idp: Step 3: Query verification
Infis->>Idp: Request JWT public key using OIDC Discovery
Idp-->>Infis: Return public key
Note over Infis: Step 4: JWT validation
Infis->>Client: Return short-lived access token
Note over Client,Infis: Step 5: Access Infisical API with Token
Client->>Infis: Make authenticated requests using the short-lived access token
```
## Concept
At a high-level, Infisical authenticates a client by verifying the JWT and checking that it meets specific requirements (e.g. it is issued by a trusted identity provider) at the `/api/v1/auth/oidc-auth/login` endpoint. If successful,
then Infisical returns a short-lived access token that can be used to make authenticated requests to the Infisical API.
To be more specific:
1. The GitLab pipeline requests an identity token from GitLab's identity provider.
2. The fetched identity token is sent to Infisical at the `/api/v1/auth/oidc-auth/login` endpoint.
3. Infisical fetches the public key that was used to sign the identity token from GitLab's identity provider using OIDC Discovery.
4. Infisical validates the JWT using the public key provided by the identity provider and checks that the subject, audience, and claims of the token matches with the set criteria.
5. If all is well, Infisical returns a short-lived access token that the GitLab pipeline can use to make authenticated requests to the Infisical API.
Infisical needs network-level access to GitLab's identity provider endpoints.
## Guide
In the following steps, we explore how to create and use identities to access the Infisical API using the OIDC Auth authentication method.
To create an identity, head to your Organization Settings > Access Control > Machine Identities and press **Create identity**.
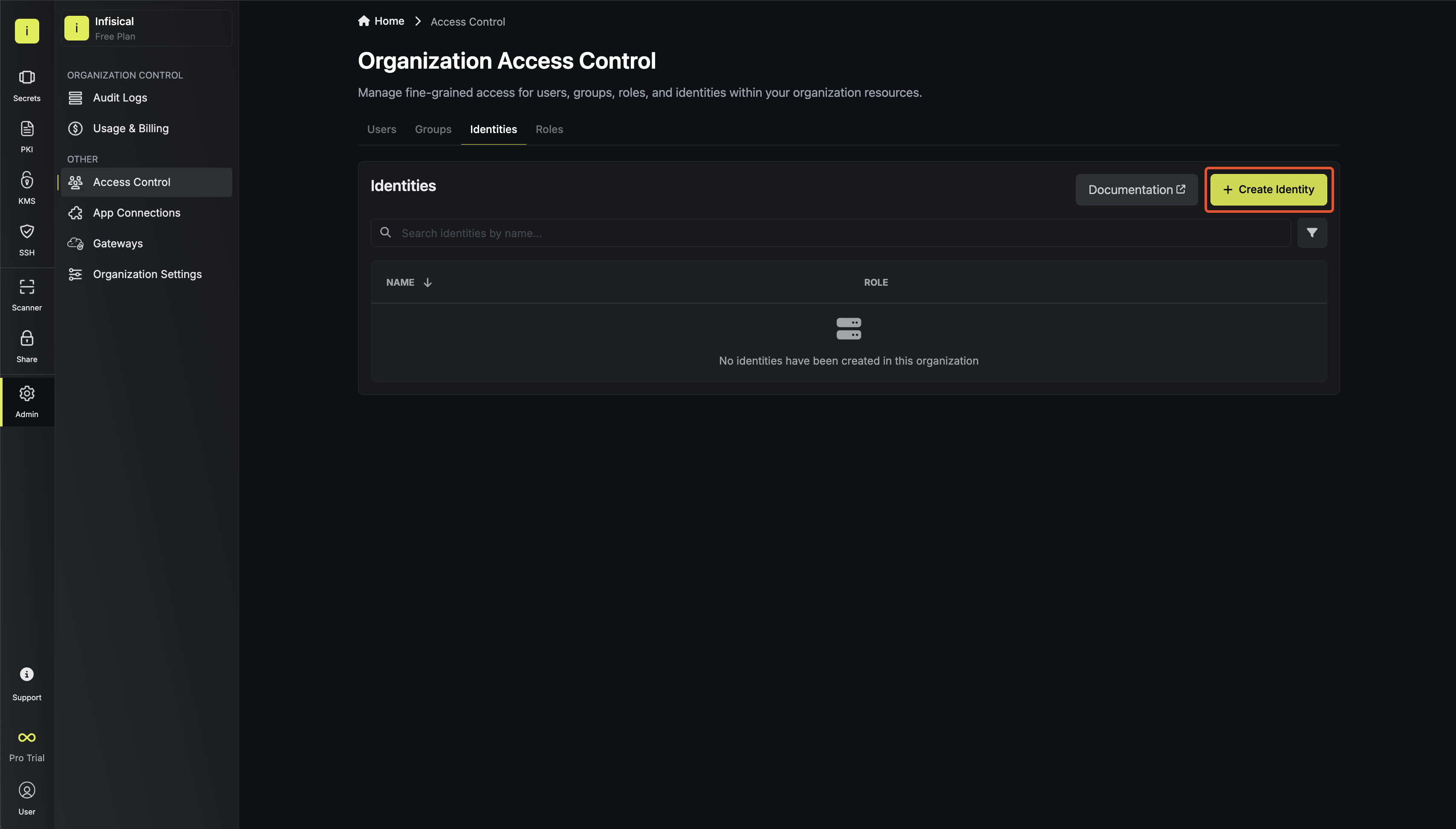
When creating an identity, you specify an organization level [role](/documentation/platform/role-based-access-controls) for it to assume; you can configure roles in Organization Settings > Access Control > Organization Roles.
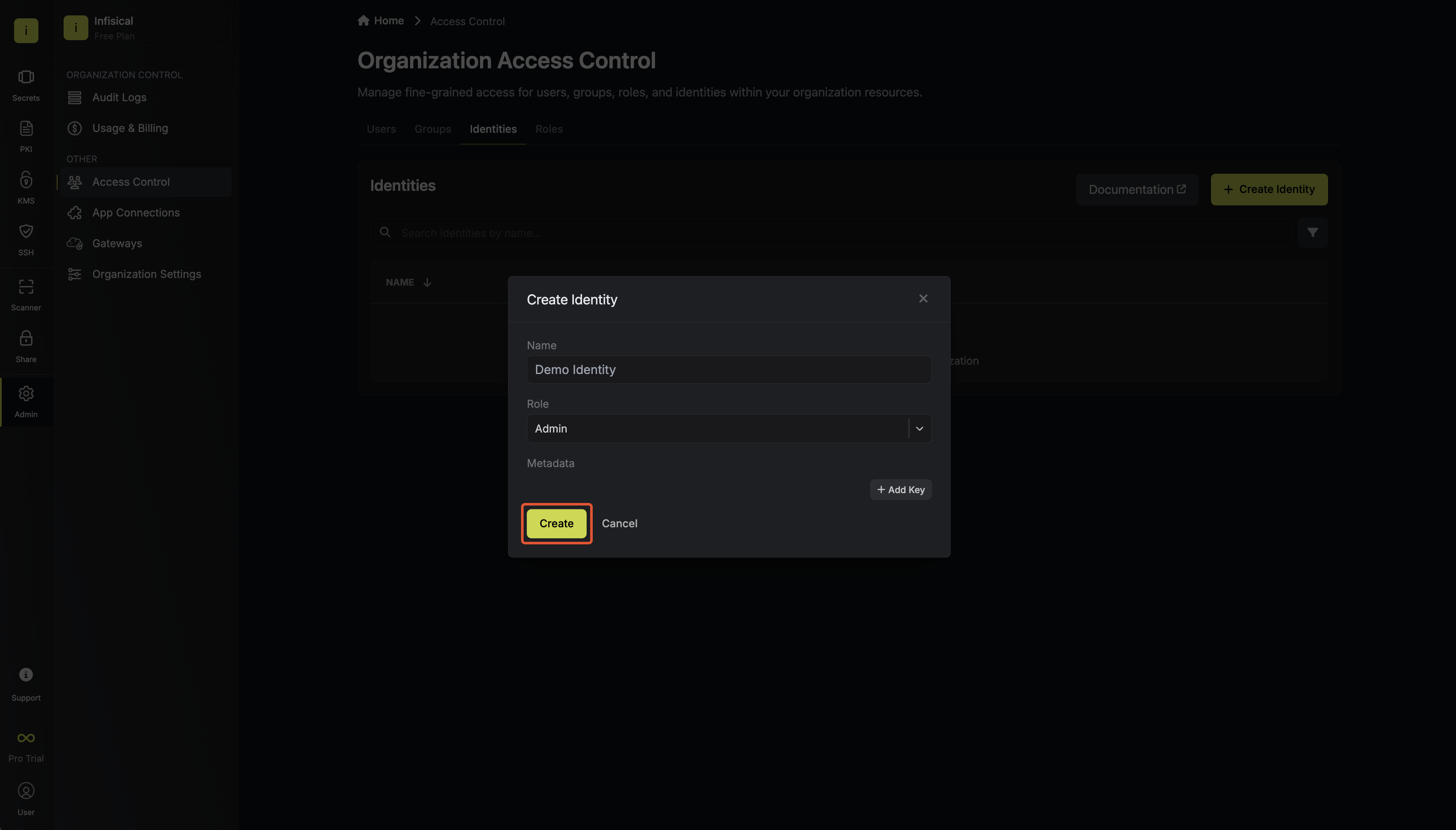
Now input a few details for your new identity. Here's some guidance for each field:
* Name (required): A friendly name for the identity.
* Role (required): A role from the **Organization Roles** tab for the identity to assume. The organization role assigned will determine what organization level resources this identity can have access to.
Once you've created an identity, you'll be redirected to a page where you can manage the identity.
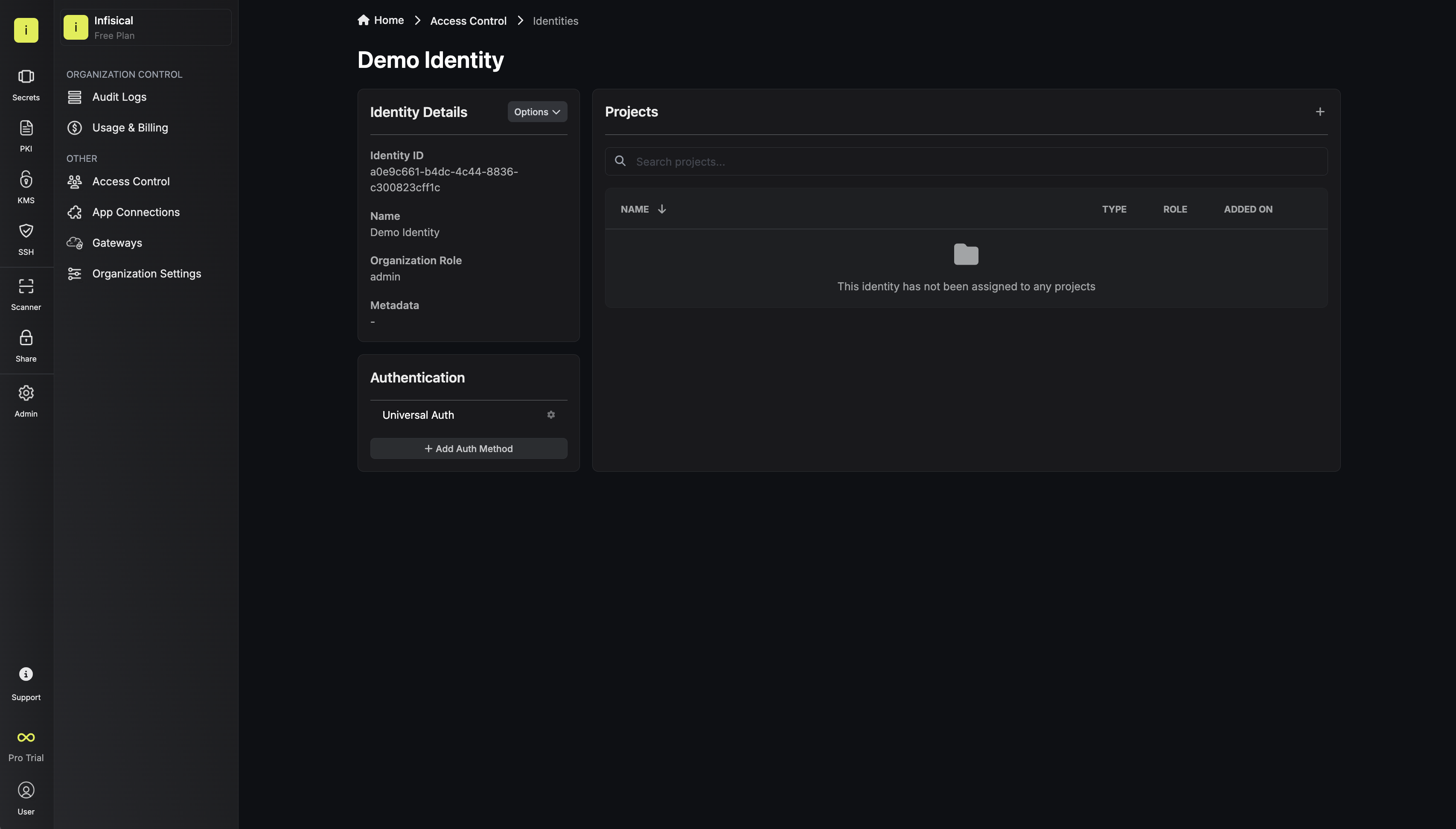
Since the identity has been configured with Universal Auth by default, you should re-configure it to use OIDC Auth instead. To do this, press to edit the **Authentication** section,
remove the existing Universal Auth configuration, and add a new OIDC Auth configuration onto the identity.
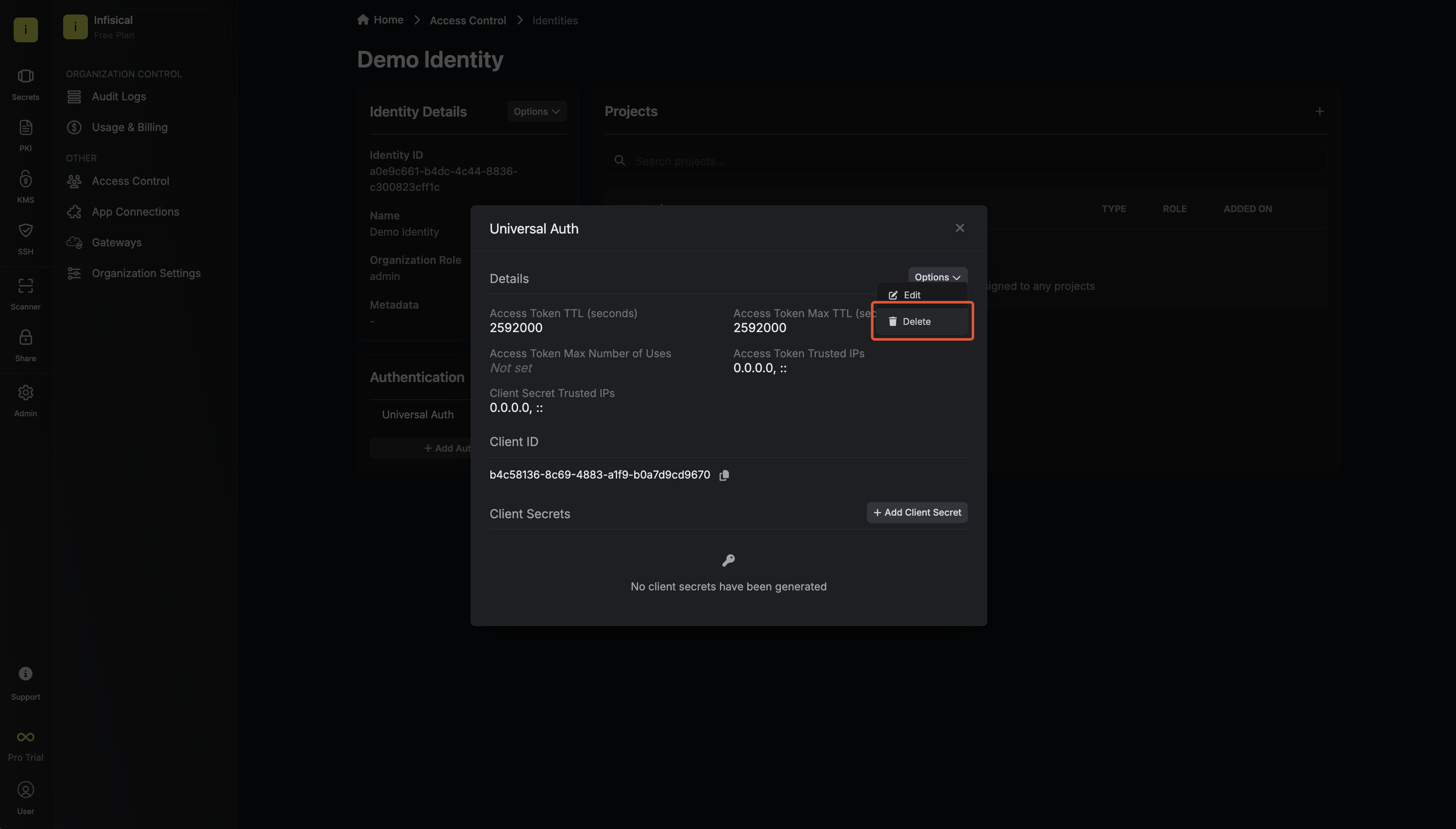
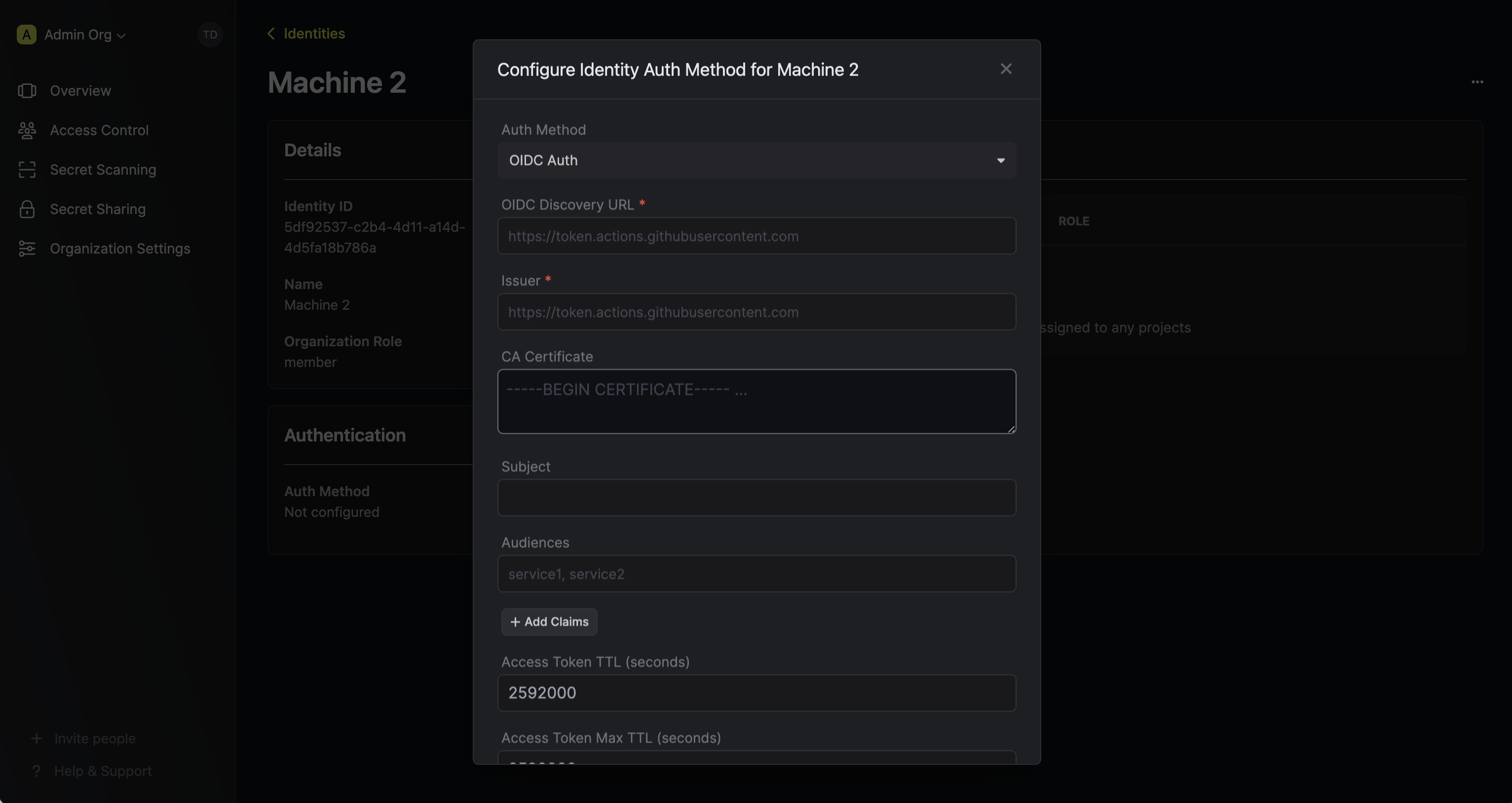
Restrict access by configuring the Subject, Audiences, and Claims fields
Here's some more guidance on each field:
* OIDC Discovery URL: The URL used to retrieve the OpenID Connect configuration from the identity provider. This will be used to fetch the public key needed for verifying the provided JWT. For GitLab SaaS (GitLab.com), this should be set to `https://gitlab.com`. For self-hosted GitLab instances, use the domain of your GitLab instance.
* Issuer: The unique identifier of the identity provider issuing the JWT. This value is used to verify the iss (issuer) claim in the JWT to ensure the token is issued by a trusted provider. This should also be set to the domain of the Gitlab instance.
* CA Certificate: The PEM-encoded CA cert for establishing secure communication with the Identity Provider endpoints. For GitLab.com, this can be left blank.
* Subject: The expected principal that is the subject of the JWT. For GitLab pipelines, this should be set to a string that uniquely identifies the pipeline and its context, in the format `project_path:{group}/{project}:ref_type:{type}:ref:{branch_name}` (e.g., `project_path:example-group/example-project:ref_type:branch:ref:main`).
* Claims: Additional information or attributes that should be present in the JWT for it to be valid. You can refer to GitLab's [documentation](https://docs.gitlab.com/ee/ci/secrets/id_token_authentication.html#token-payload) for the list of supported claims.
* Access Token TTL (default is `2592000` equivalent to 30 days): The lifetime for an acccess token in seconds. This value will be referenced at renewal time.
* Access Token Max TTL (default is `2592000` equivalent to 30 days): The maximum lifetime for an acccess token in seconds. This value will be referenced at renewal time.
* Access Token Max Number of Uses (default is `0`): The maximum number of times that an access token can be used; a value of `0` implies infinite number of uses.
* Access Token Trusted IPs: The IPs or CIDR ranges that access tokens can be used from. By default, each token is given the `0.0.0.0/0`, allowing usage from any network address.
For more details on the appropriate values for the OIDC fields, refer to GitLab's [documentation](https://docs.gitlab.com/ee/ci/secrets/id_token_authentication.html#token-payload). The `subject`, `audiences`, and `claims` fields support glob pattern matching; however, we highly recommend using hardcoded values whenever possible.
To enable the identity to access project-level resources such as secrets within a specific project, you should add it to that project.
To do this, head over to the project you want to add the identity to and go to Project Settings > Access Control > Machine Identities and press **Add identity**.
Next, select the identity you want to add to the project and the project level role you want to allow it to assume. The project role assigned will determine what project level resources this identity can have access to.
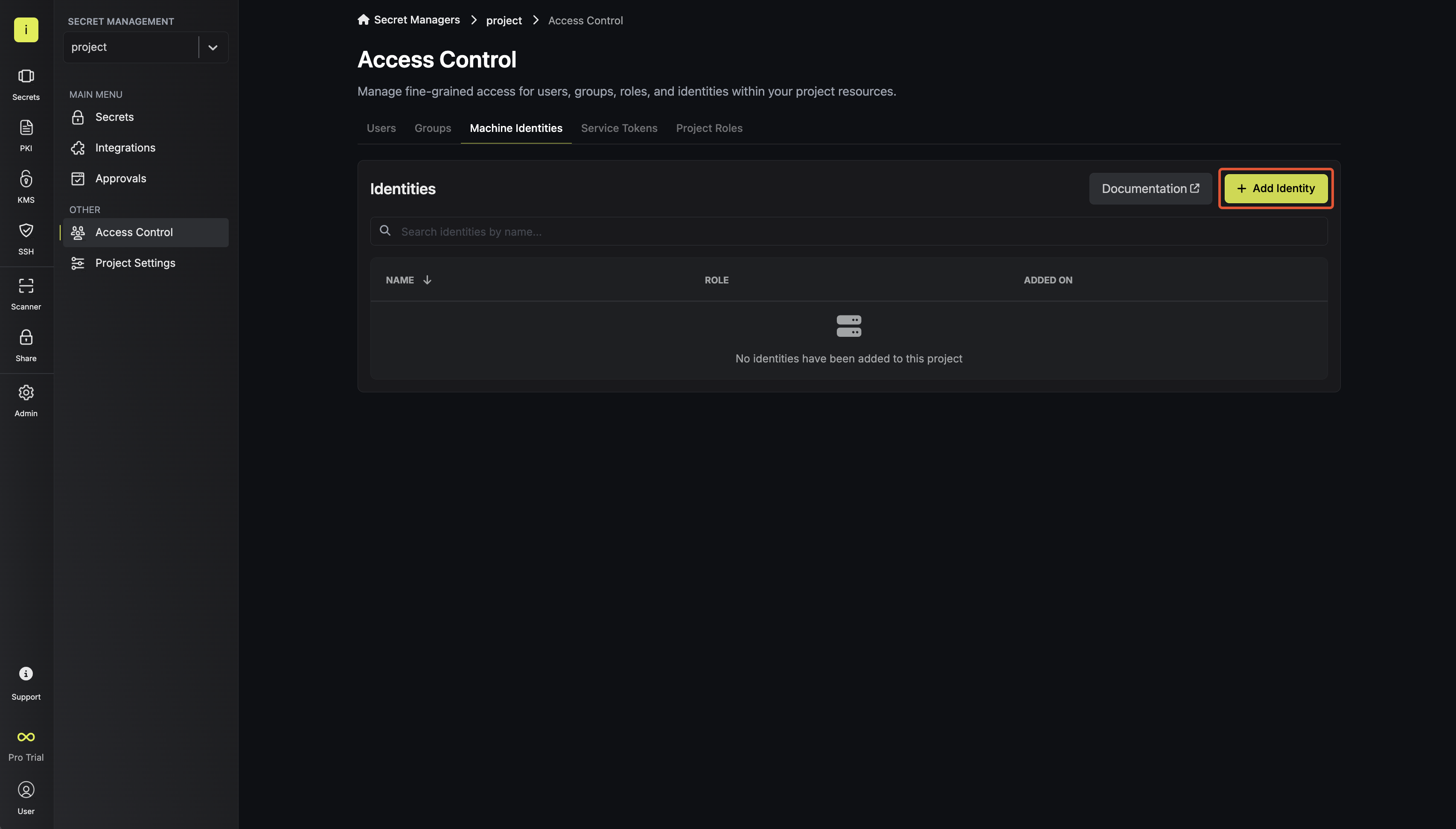
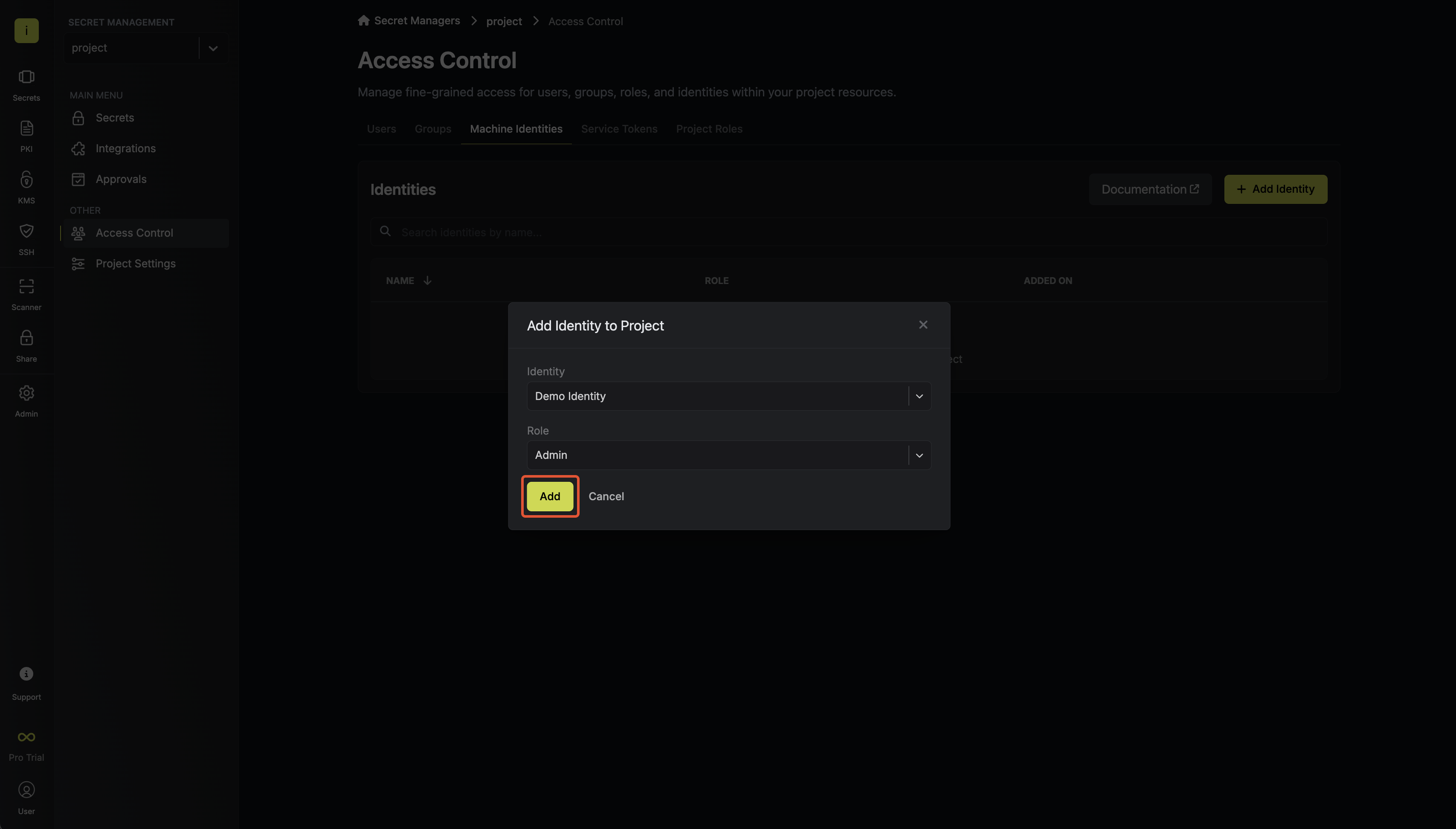
As demonstration, we will be using the Infisical CLI to fetch Infisical secrets and utilize them within a GitLab pipeline.
To access Infisical secrets as the identity, you need to use an identity token from GitLab which matches the OIDC configuration defined for the machine identity.
This can be done by defining the `id_tokens` property. The resulting token would then be used to login with OIDC like the following: `infisical login --method=oidc-auth --oidc-jwt=$GITLAB_TOKEN`
Below is a complete example of how a GitLab pipeline can be configured to work with secrets from Infisical using the Infisical CLI with OIDC Auth:
```yaml
image: ubuntu
stages:
- build
build-job:
stage: build
id_tokens:
INFISICAL_ID_TOKEN:
aud: infisical-aud-test
script:
- apt update && apt install -y curl
- curl -1sLf 'https://dl.cloudsmith.io/public/infisical/infisical-cli/setup.deb.sh' | bash
- apt-get update && apt-get install -y infisical
- export INFISICAL_TOKEN=$(infisical login --method=oidc-auth --machine-identity-id=4e807a78-1b1c-4bd6-9609-ef2b0cf4fd54 --oidc-jwt=$INFISICAL_ID_TOKEN --silent --plain)
- infisical run --projectId=1d0443c1-cd43-4b3a-91a3-9d5f81254a89 --env=dev -- npm run build
```
The `id_tokens` keyword is used to request an ID token for the job. In this example, an ID token named `INFISICAL_ID_TOKEN` is requested with the audience (`aud`) claim set to "infisical-aud-test". This ID token will be used to authenticate with Infisical.
Each identity access token has a time-to-live (TTL) which you can infer from the response of the login operation; the default TTL is `7200` seconds, which can be adjusted.
If an identity access token expires, it can no longer authenticate with the Infisical API. In this case, a new access token should be obtained by performing another login operation.
# User and Machine Identities
Learn more about identities to interact with resources in Infisical.
To interact with secrets and resource with Infisical, it is important to understand the concept of identities.
Identities can be of two types:
* **People** (e.g., developers, platform engineers, administrators)
* **Machines** (e.g., machine entities for managing secrets in CI/CD pipelines, production applications, and more)
Both people and machines are able to utilize corresponding clients (e.g., Dashboard UI, CLI, SDKs, API, Kubernetes Operator) together with allowed authentication methods (e.g., email & password, SAML SSO, LDAP, OIDC, Universal Auth).
Learn more about the concept on user identities in Infisical.
Understand the concept of machine identities in Infisical.
# Token Auth
Learn how to authenticate to Infisical from any platform or environment using an access token.
**Token Auth** is a platform-agnostic, simple authentication method that can be configured for a [machine identity](/documentation/platform/identities/machine-identities) to authenticate from any platform/environment using a token.
## Diagram
The following sequence diagram illustrates the Token Auth workflow for authenticating clients with Infisical.
```mermaid
sequenceDiagram
participant Client as Client
participant Infis as Infisical
Note over Client,Infis: Access Infisical API with Token
Client->>Infis: Make authenticated requests using the token
```
## Concept
Token Auth is the simplest authentication method that a client can use to authenticate with Infisical.
Unlike other authentication methods where a client must exchange credential(s) for a short-lived access token to access the Infisical API,
Token Auth allows a client to make authenticated requests to the Infisical API directly using a token. Conceptually, this is similar to using an API Key.
To be more specific:
1. An operator creates an access token in the Infisical UI.
2. The operator shares the access token with the client which it can then use to make authenticated requests to the Infisical API.
## Guide
In the following steps, we explore how to create and use identities for your workloads and applications to access the Infisical API
using the Token Auth authentication method.
To create an identity, head to your Organization Settings > Access Control > Machine Identities and press **Create identity**.
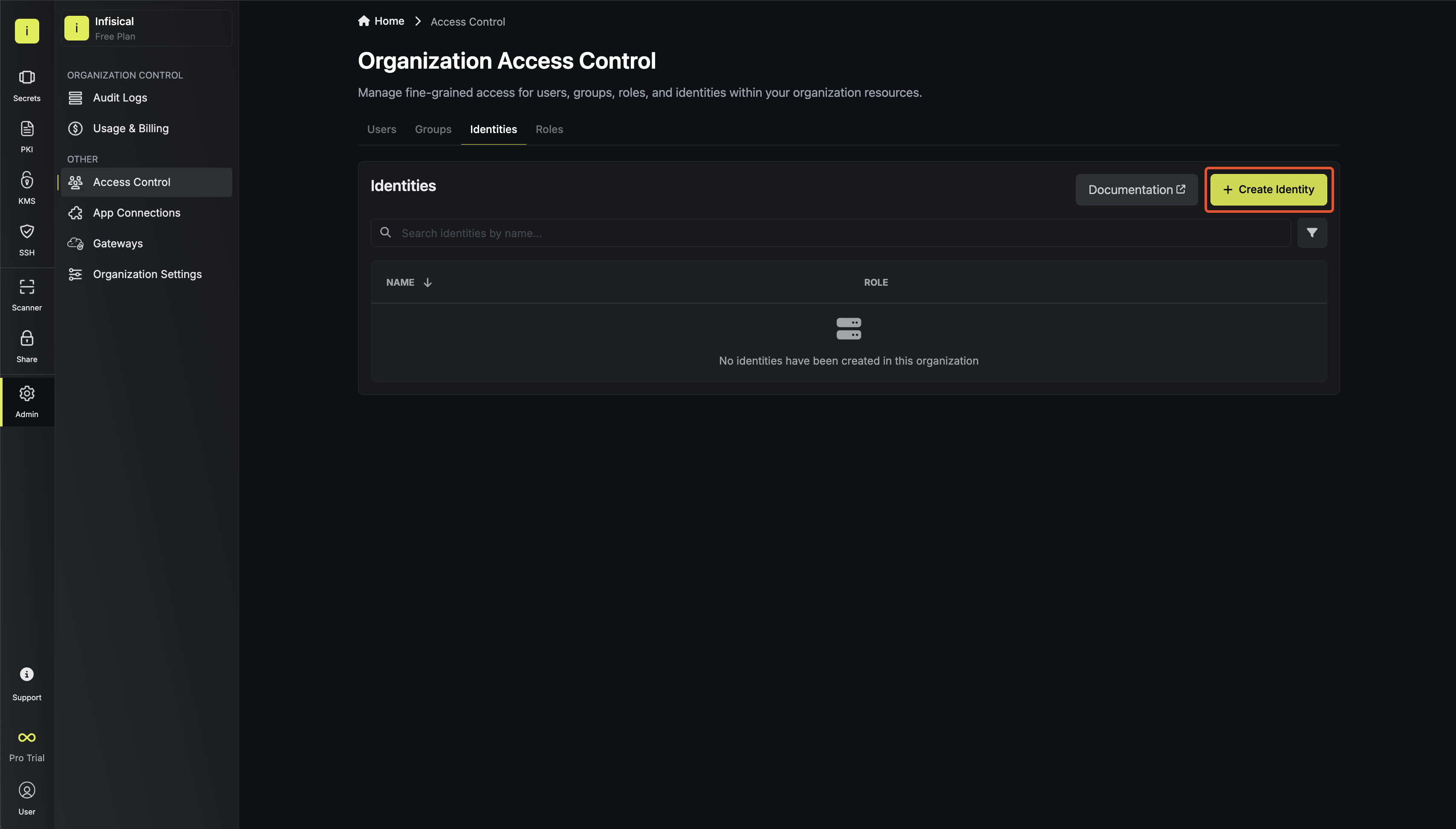
When creating an identity, you specify an organization level [role](/documentation/platform/role-based-access-controls) for it to assume; you can configure roles in Organization Settings > Access Control > Organization Roles.
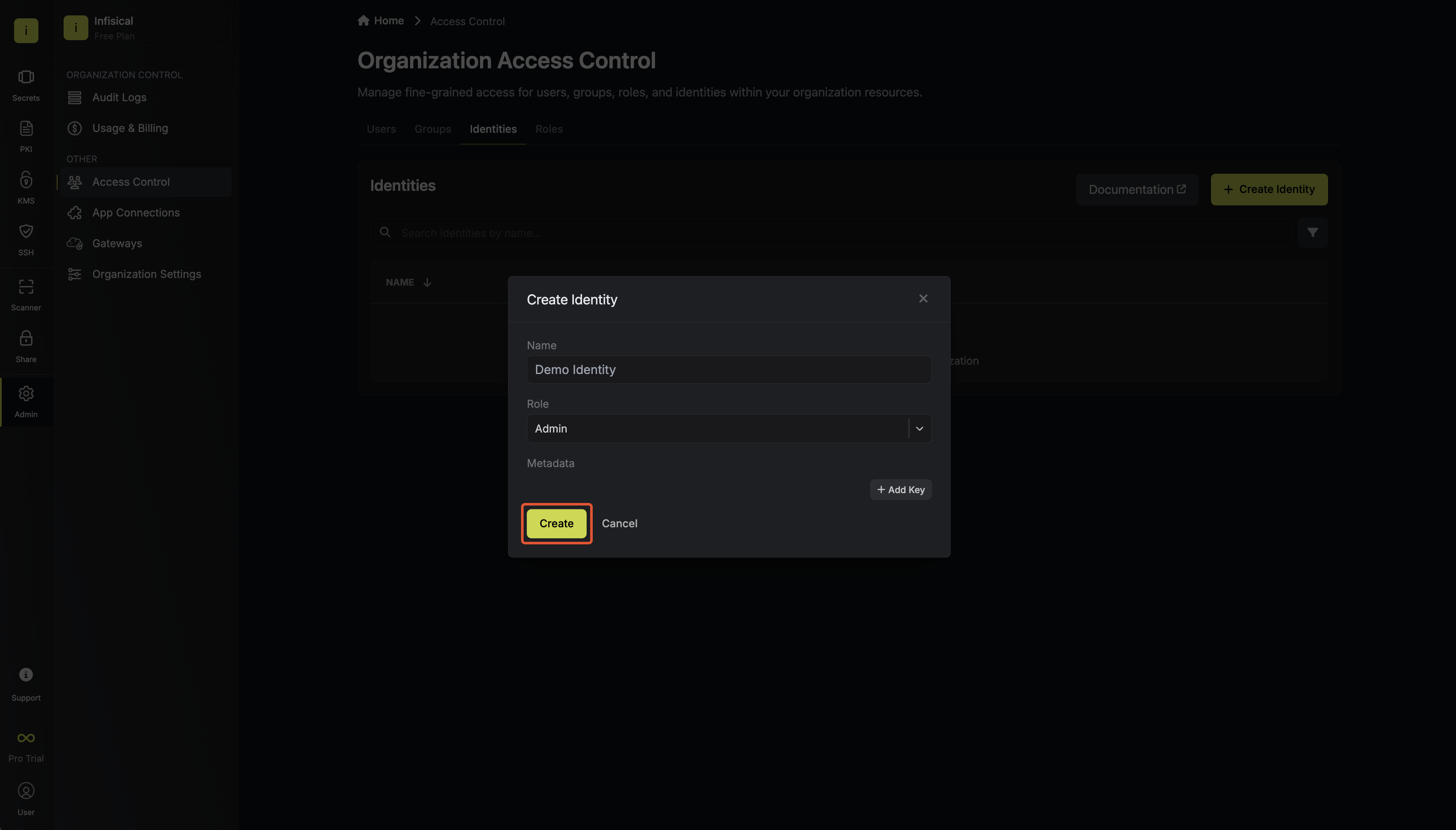
Now input a few details for your new identity. Here's some guidance for each field:
* Name (required): A friendly name for the identity.
* Role (required): A role from the **Organization Roles** tab for the identity to assume. The organization role assigned will determine what organization level resources this identity can have access to.
Once you've created an identity, you'll be redirected to a page where you can manage the identity.
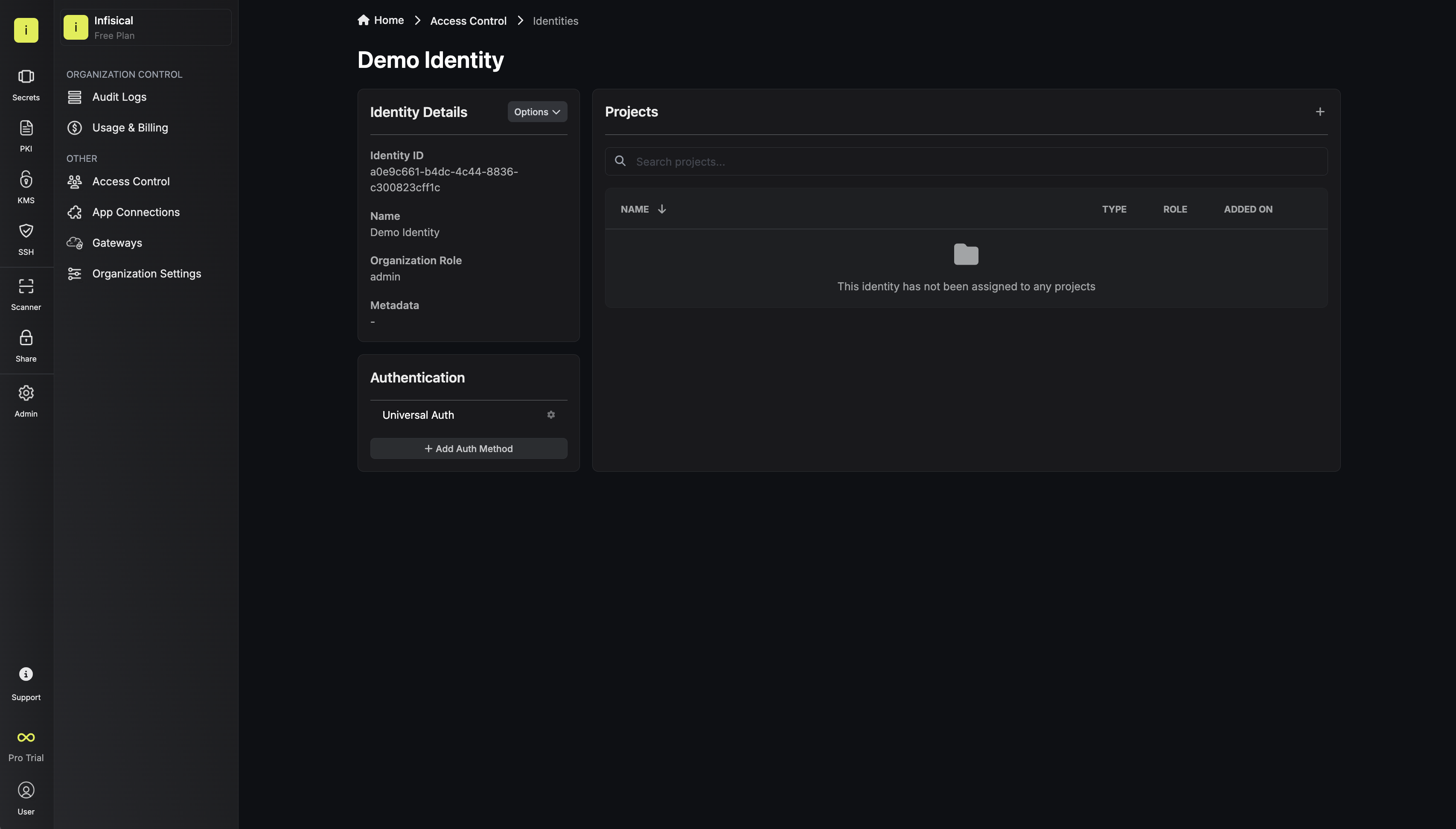
Since the identity has been configured with Universal Auth by default, you should re-configure it to use Token Auth instead. To do this, press to edit the **Authentication** section,
remove the existing Universal Auth configuration, and add a new Token Auth configuration onto the identity.
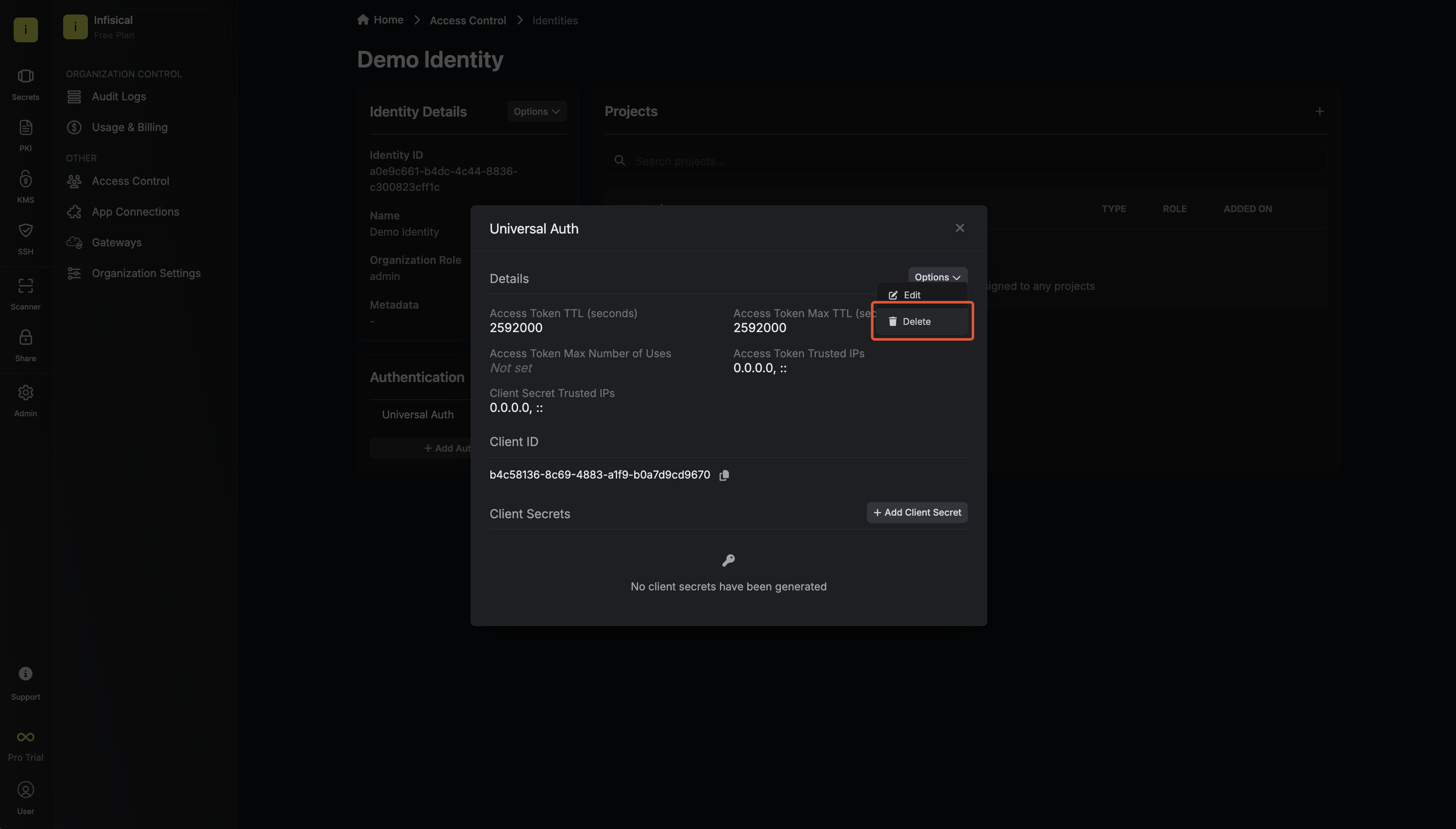
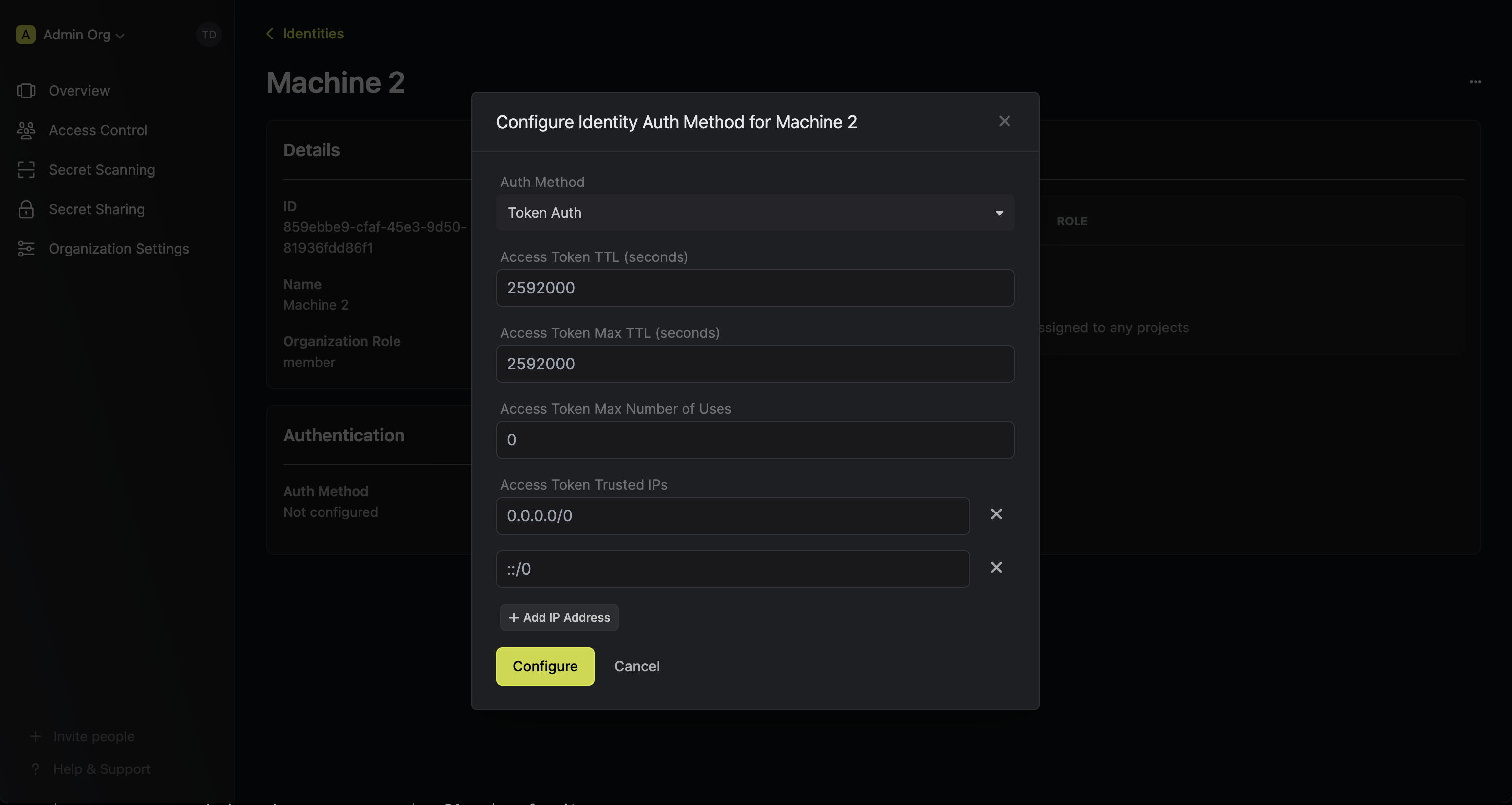
Here's some more guidance on each field:
* Access Token TTL (default is `2592000` equivalent to 30 days): The lifetime for an acccess token in seconds. This value will be referenced at renewal time.
* Access Token Max TTL (default is `2592000` equivalent to 30 days): The maximum lifetime for an acccess token in seconds. This value will be referenced at renewal time.
* Access Token Max Number of Uses (default is `0`): The maximum number of times that an access token can be used; a value of `0` implies infinite number of uses.
* Access Token Trusted IPs: The IPs or CIDR ranges that access tokens can be used from. By default, each token is given the `0.0.0.0/0`, allowing usage from any network address.
Restricting access token usage to specific trusted IPs is a paid feature.
If you’re using Infisical Cloud, then it is available under the Pro Tier. If you’re self-hosting Infisical, then you should contact [sales@infisical.com](mailto:sales@infisical.com) to purchase an enterprise license to use it.
In order to use the identity with Token Auth, you'll need to create an (access) token; you can think of this token akin
to an API Key used to authenticate with the Infisical API. With that, press **Create Token**.
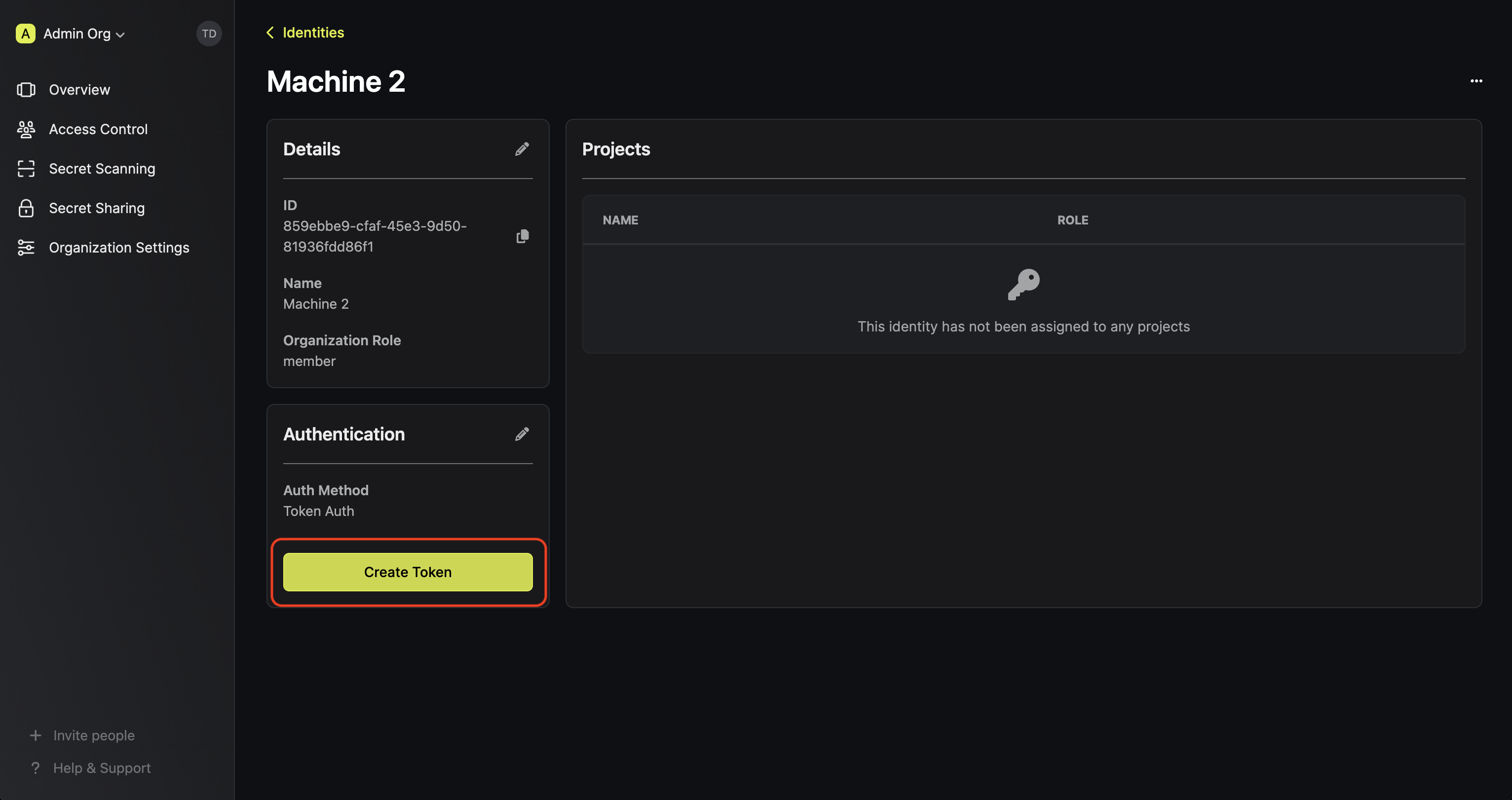
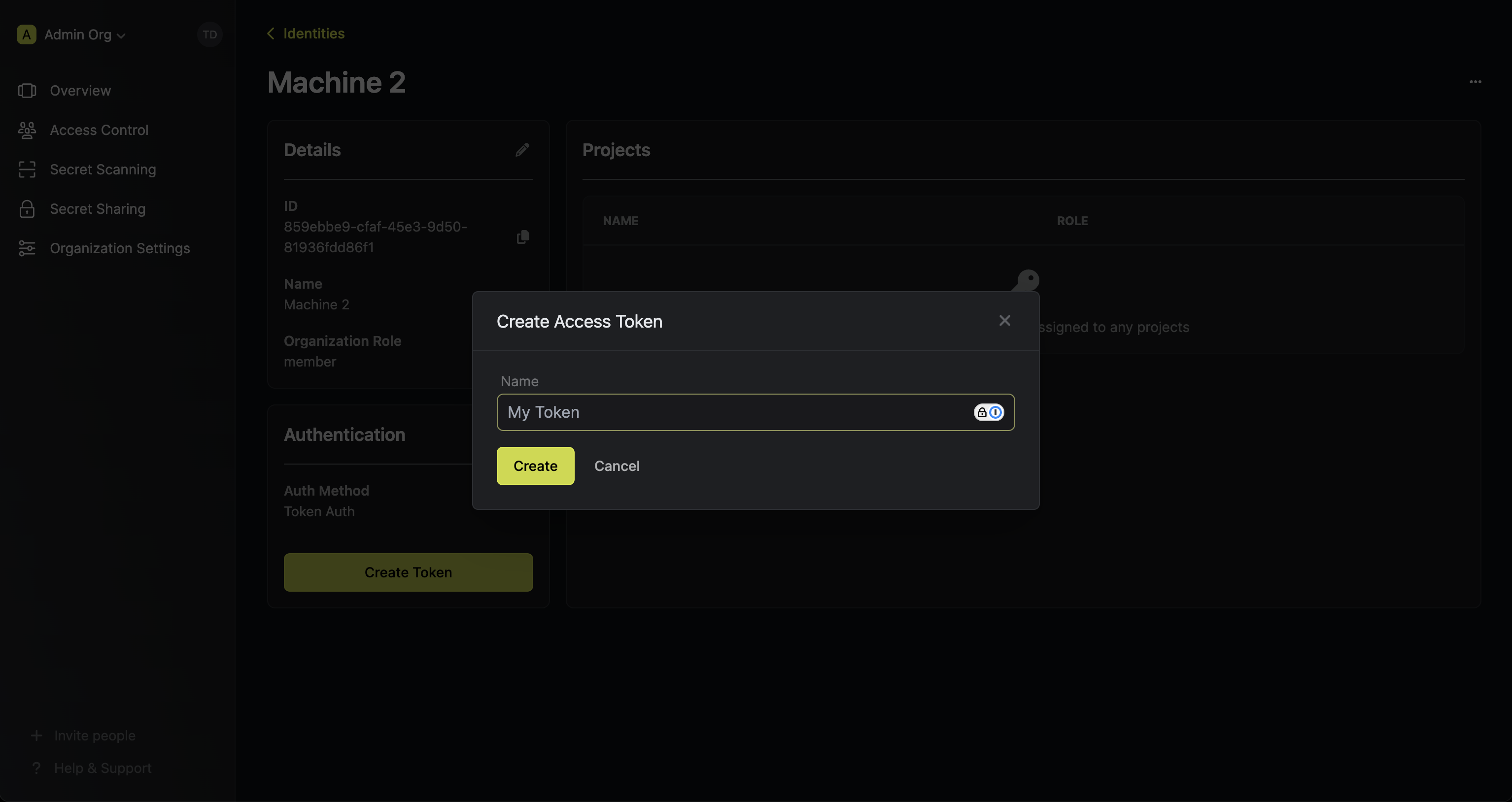
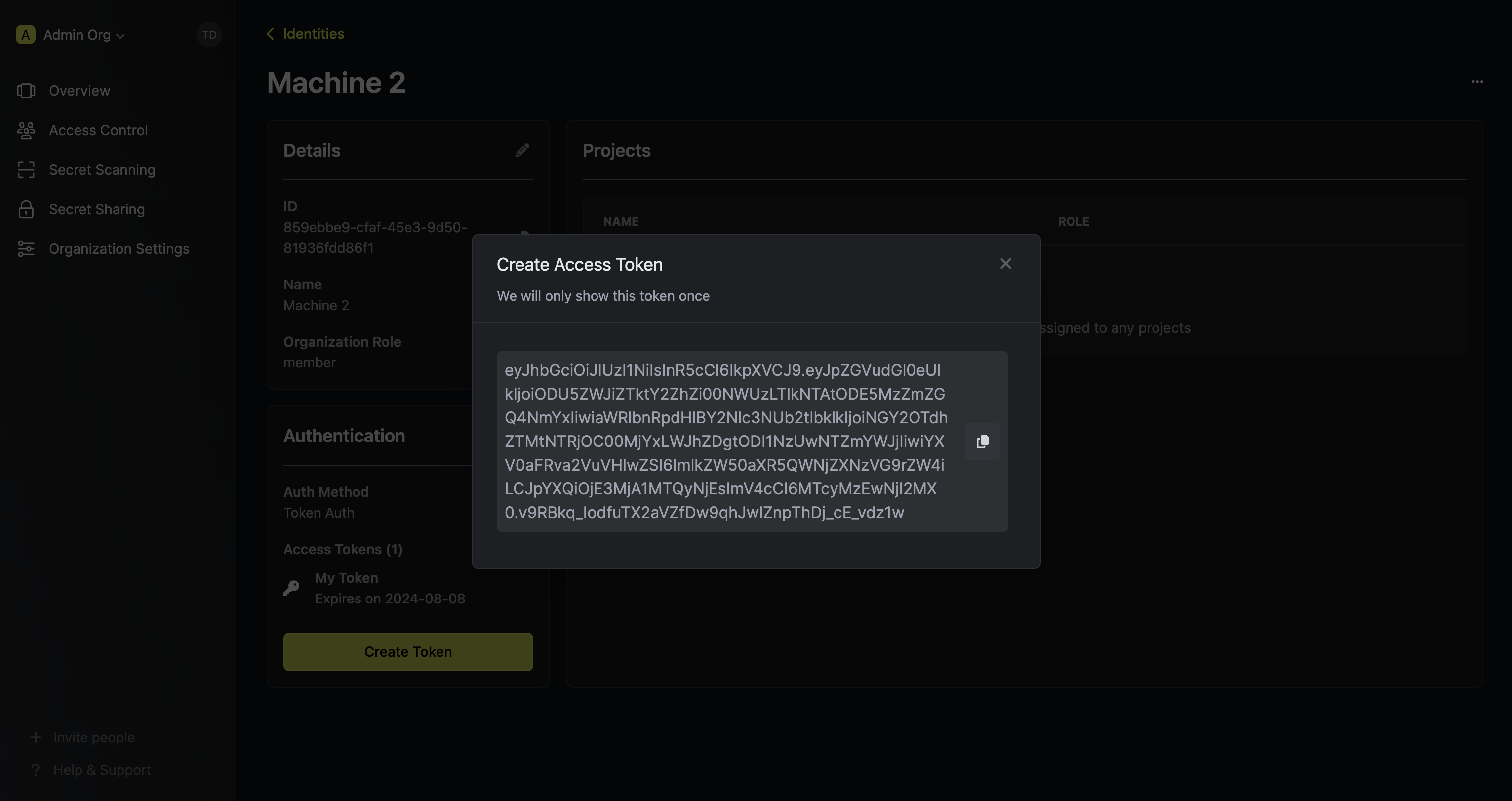
Copy the token and keep it handy as you'll need it to authenticate with the Infisical API.
To enable the identity to access project-level resources such as secrets within a specific project, you should add it to that project.
To do this, head over to the project you want to add the identity to and go to Project Settings > Access Control > Machine Identities and press **Add identity**.
Next, select the identity you want to add to the project and the project level role you want to allow it to assume. The project role assigned will determine what project level resources this identity can have access to.
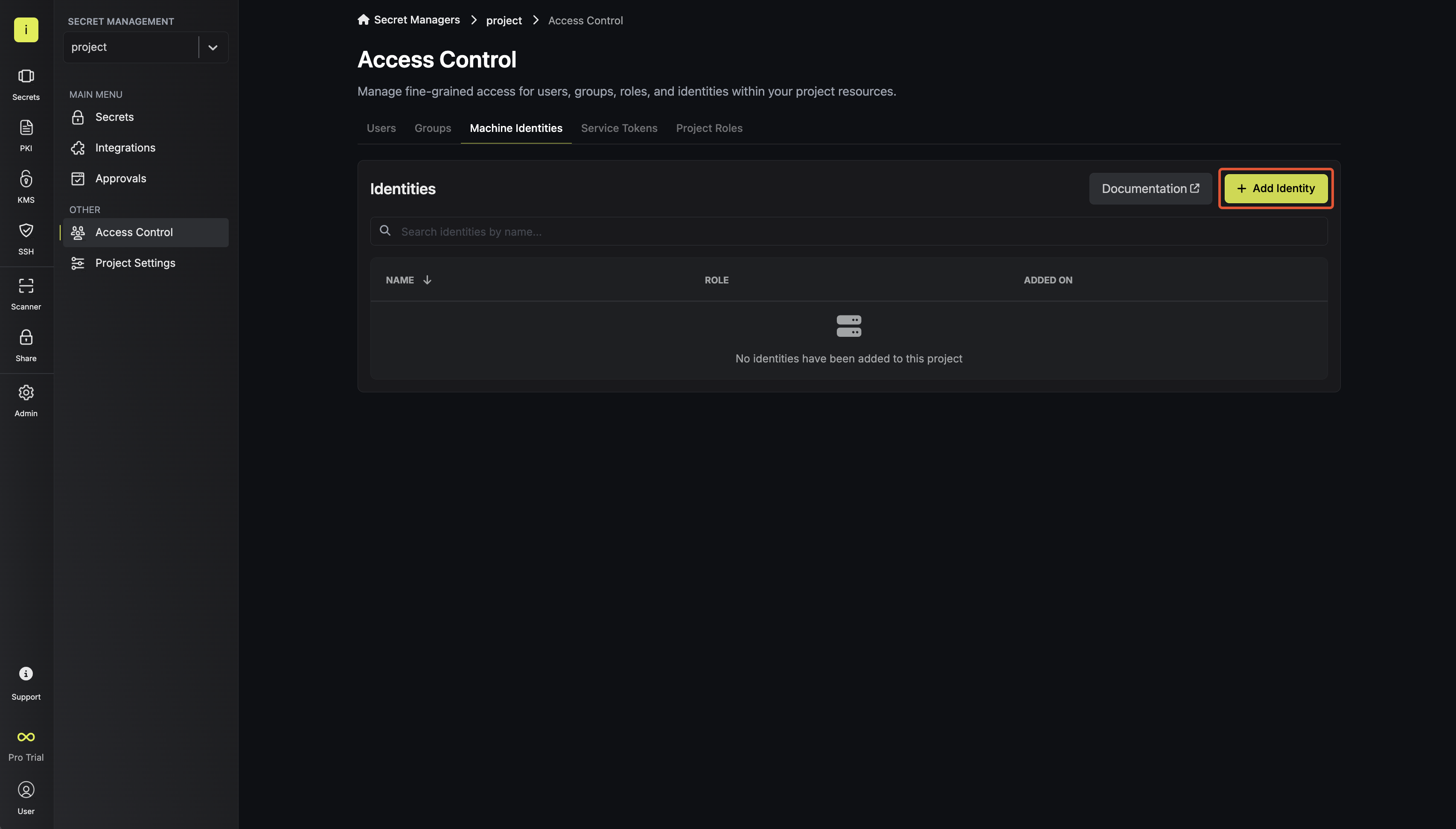
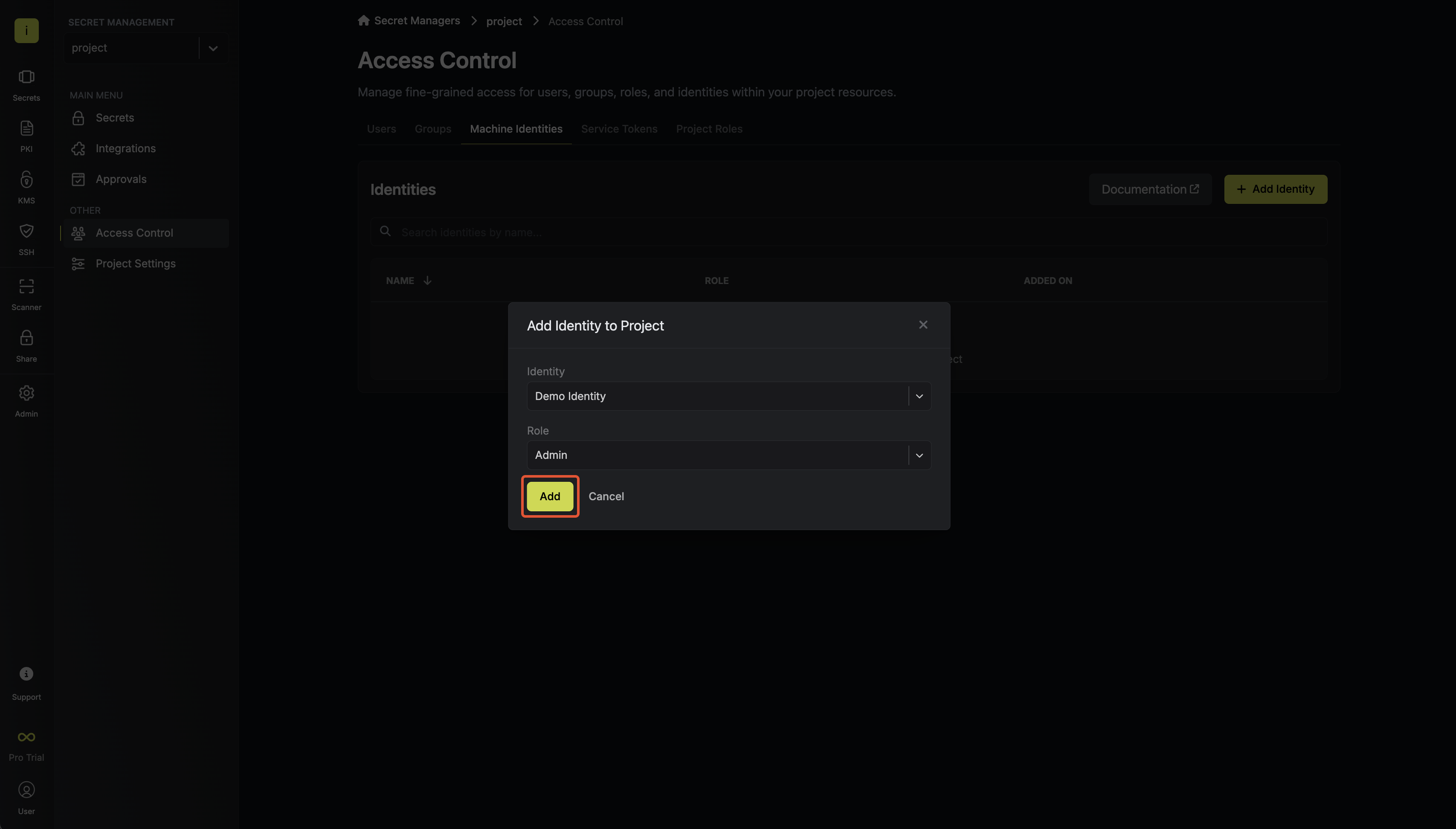
To access the Infisical API as the identity, you can use the generated access token from step 2
to authenticate with the [Infisical API](/api-reference/overview/introduction).
Each identity access token has a time-to-live (TLL) which you can infer from the response of the login operation;
the default TTL is `7200` seconds which can be adjusted in the Token Auth configuration.
If an identity access token expires, it can no longer authenticate with the Infisical API. In this case,
a new access token should be obtained.
**FAQ**
There are a few reasons for why this might happen:
* The access token has expired. If this is the case, you should obtain a new access token or consider extending the token's TTL.
* The identity is insufficently permissioned to interact with the resources you wish to access.
* The access token is being used from an untrusted IP.
A identity access token can have a time-to-live (TTL) or incremental lifetime after which it expires.
In certain cases, you may want to extend the lifespan of an access token; to do so, you must set a max TTL parameter.
A token can be renewed any number of times where each call to renew it can extend the token's lifetime by increments of the access token's TTL.
Regardless of how frequently an access token is renewed, its lifespan remains bound to the maximum TTL determined at its creation.
# Universal Auth
Learn how to authenticate to Infisical from any platform or environment.
**Universal Auth** is a platform-agnostic authentication method that can be configured for a [machine identity](/documentation/platform/identities/machine-identities) to authenticate from any platform/environment using a Client ID and Client Secret.
## Diagram
The following sequence diagram illustrates the Universal Auth workflow for authenticating clients with Infisical.
```mermaid
sequenceDiagram
participant Client as Client
participant Infis as Infisical
Note over Client,Infis: Step 1: Login Operation
Client->>Infis: Send Client ID and Client Secret
Note over Infis: Step 2: Client ID and Client Secret validation
Infis->>Client: Return short-lived access token
Note over Client,Infis: Step 3: Access Infisical API with Token
Client->>Infis: Make authenticated requests using the short-lived access token
```
## Concept
In this method, Infisical authenticates a client by verifying the credentials issued for it at the `/api/v1/auth/universal-auth/login` endpoint. If successful,
then Infisical returns a short-lived access token that can be used to make authenticated requests to the Infisical API.
To be more specific:
1. The client submits a **Client ID** and **Client Secret** to Infisical at the `/api/v1/auth/universal-auth/login` endpoint.
2. Infisical verifies the credential pair.
3. If all is well, Infisical returns a short-lived access token that the client can use to make authenticated requests to the Infisical API.
## Guide
In the following steps, we explore how to create and use identities for your workloads and applications to access the Infisical API
using the Universal Auth authentication method.
To create an identity, head to your Organization Settings > Access Control > Machine Identities and press **Create identity**.
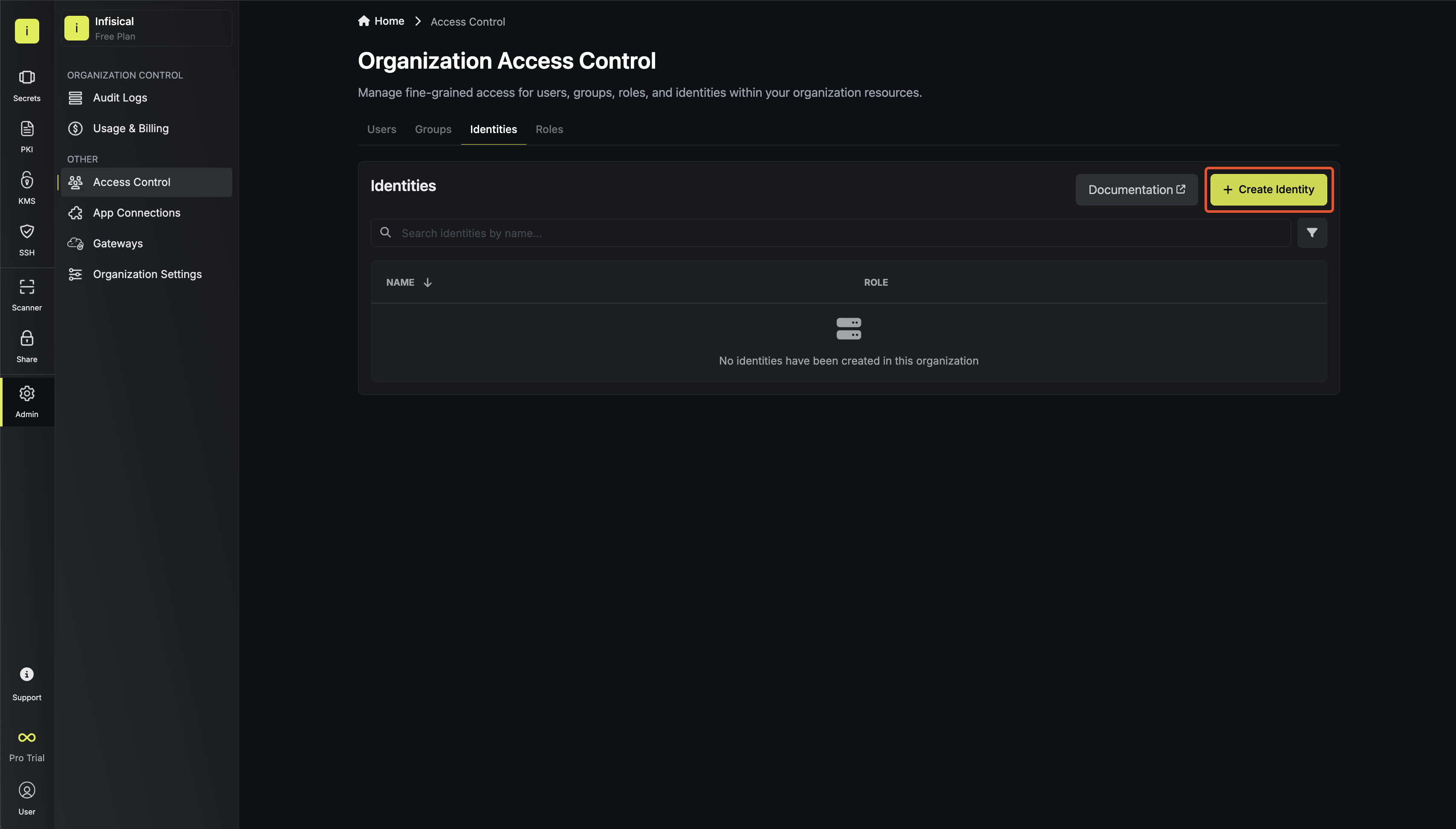
When creating an identity, you specify an organization level [role](/documentation/platform/role-based-access-controls) for it to assume; you can configure roles in Organization Settings > Access Control > Organization Roles.
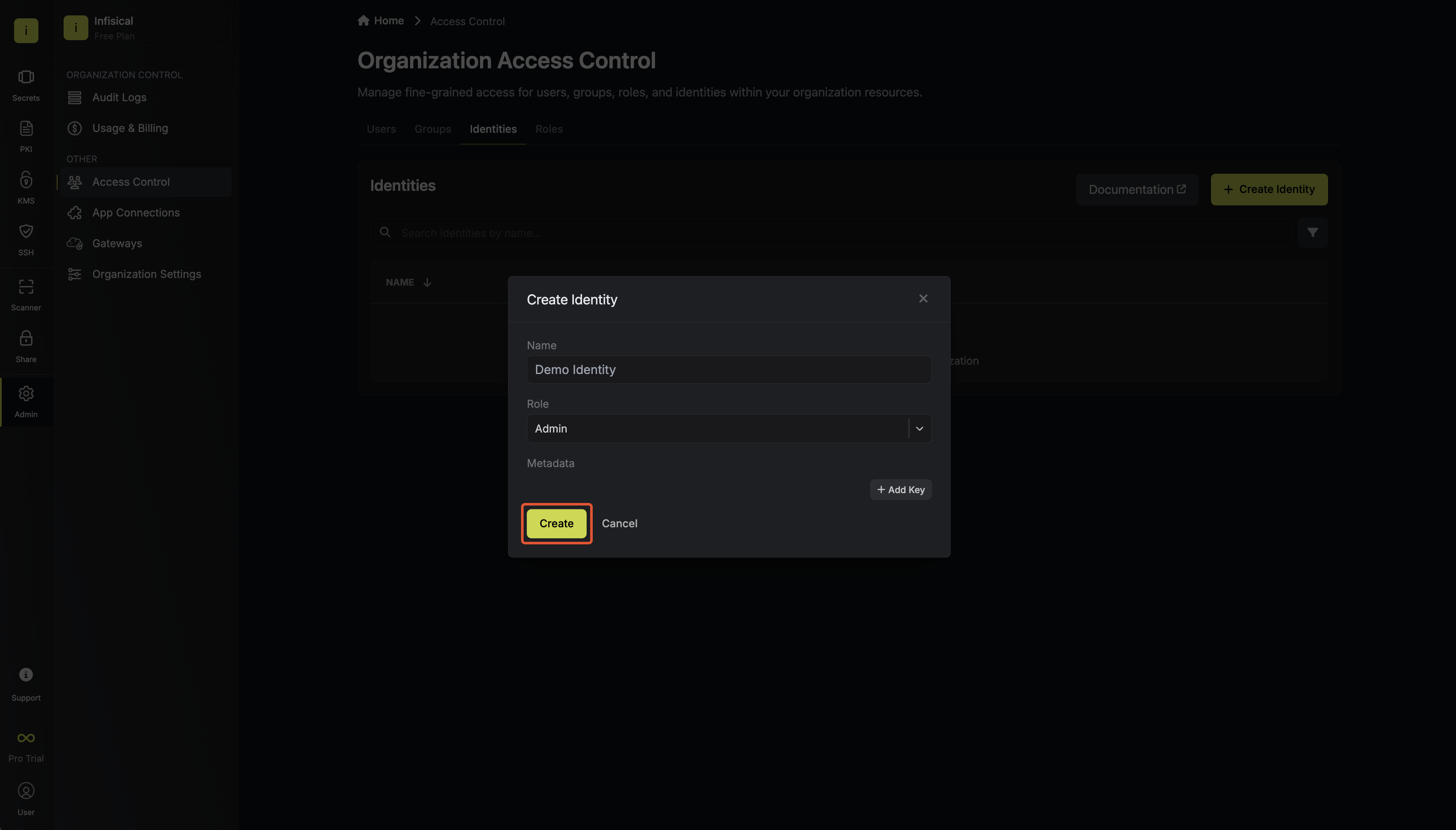
Now input a few details for your new identity. Here's some guidance for each field:
* Name (required): A friendly name for the identity.
* Role (required): A role from the **Organization Roles** tab for the identity to assume. The organization role assigned will determine what organization level resources this identity can have access to.
Once you've created an identity, you'll be redirected to a page where you can manage the identity.
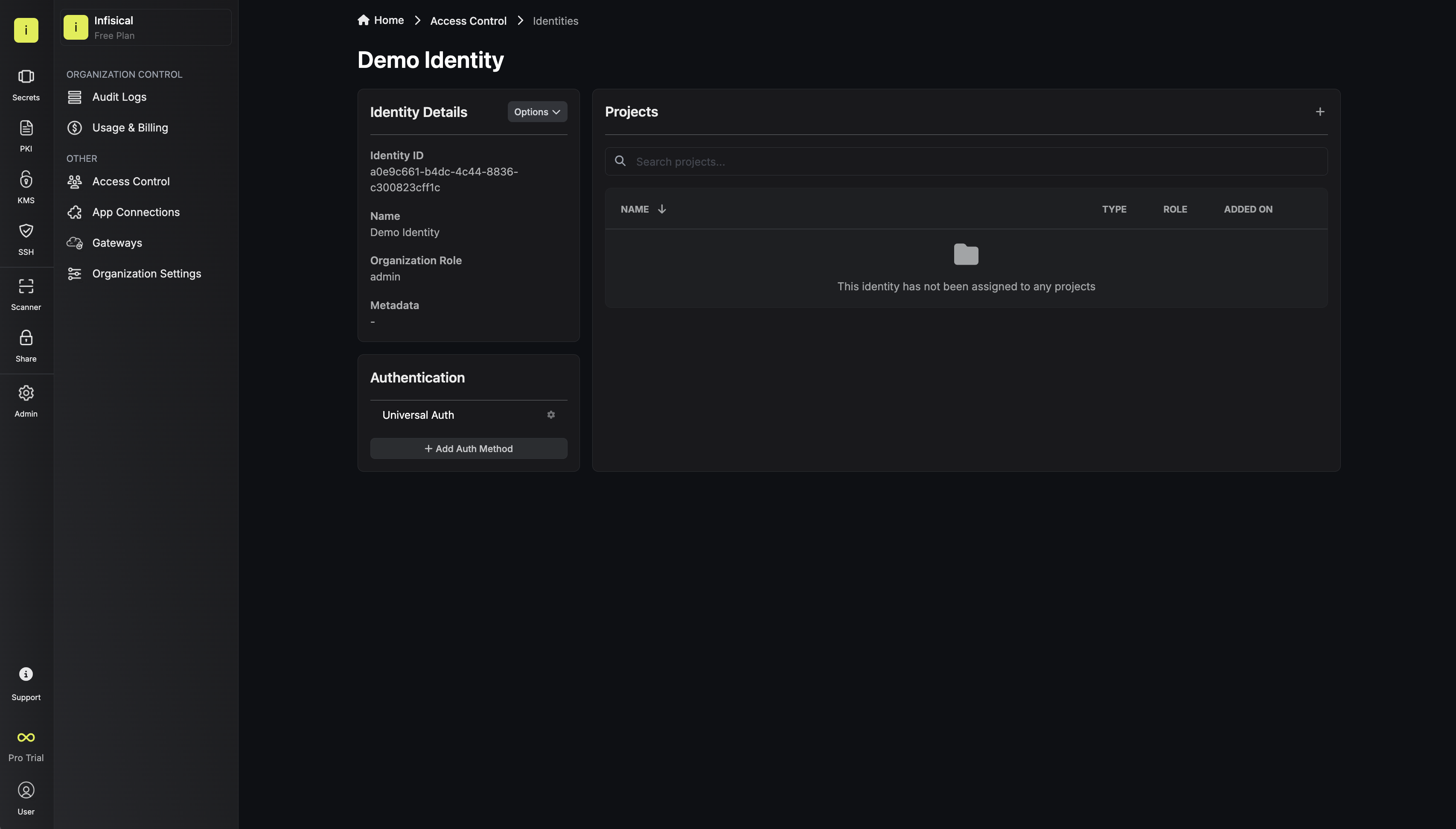
Once you've created an identity, you'll be prompted to configure the **Universal Auth** authentication method for it.
By default, the identity has been configured with Universal Auth. If you wish, you can edit the Universal Auth configuration
details by pressing to edit the **Authentication** section.

Here's some more guidance on each field:
* Access Token TTL (default is `2592000` equivalent to 30 days): The lifetime for an acccess token in seconds. This value will be referenced at renewal time.
* Access Token Max TTL (default is `2592000` equivalent to 30 days): The maximum lifetime for an acccess token in seconds. This value will be referenced at renewal time.
* Access Token Max Number of Uses (default is `0`): The maximum number of times that an access token can be used; a value of `0` implies infinite number of uses.
* Client Secret Trusted IPs: The IPs or CIDR ranges that the **Client Secret** can be used from together with the **Client ID** to get back an access token. By default, **Client Secrets** are given the `0.0.0.0/0`, allowing usage from any network address.
* Access Token Trusted IPs: The IPs or CIDR ranges that access tokens can be used from. By default, each token is given the `0.0.0.0/0`, allowing usage from any network address.
Restricting **Client Secret** and access token usage to specific trusted IPs is a paid feature.
If you’re using Infisical Cloud, then it is available under the Pro Tier. If you’re self-hosting Infisical, then you should contact [sales@infisical.com](mailto:sales@infisical.com) to purchase an enterprise license to use it.
In order to use the identity, you'll need the non-sensitive **Client ID**
of the identity and a **Client Secret** for it; you can think of these credentials akin to a username
and password used to authenticate with the Infisical API.
With that, press **Create Client Secret**.
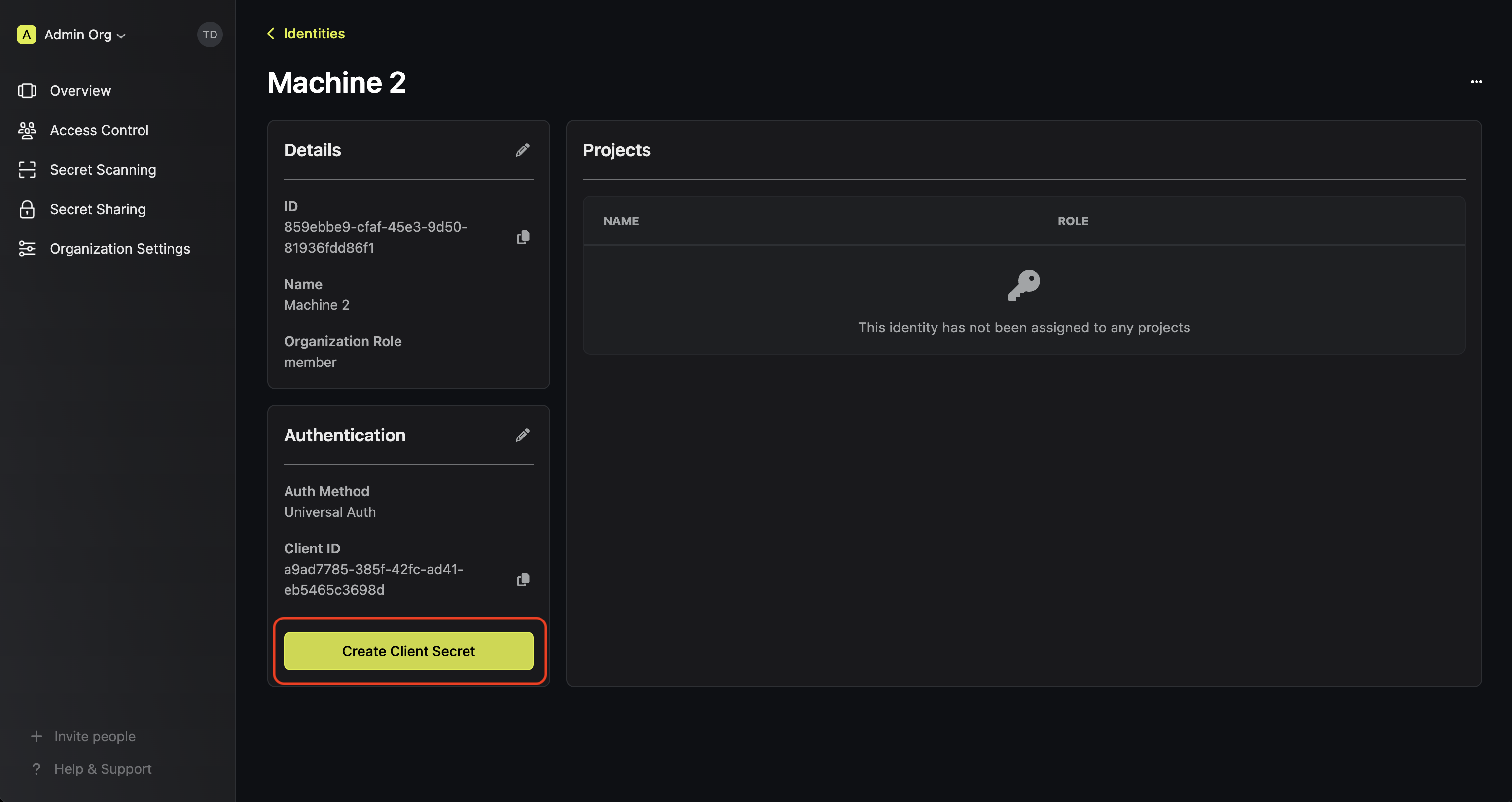
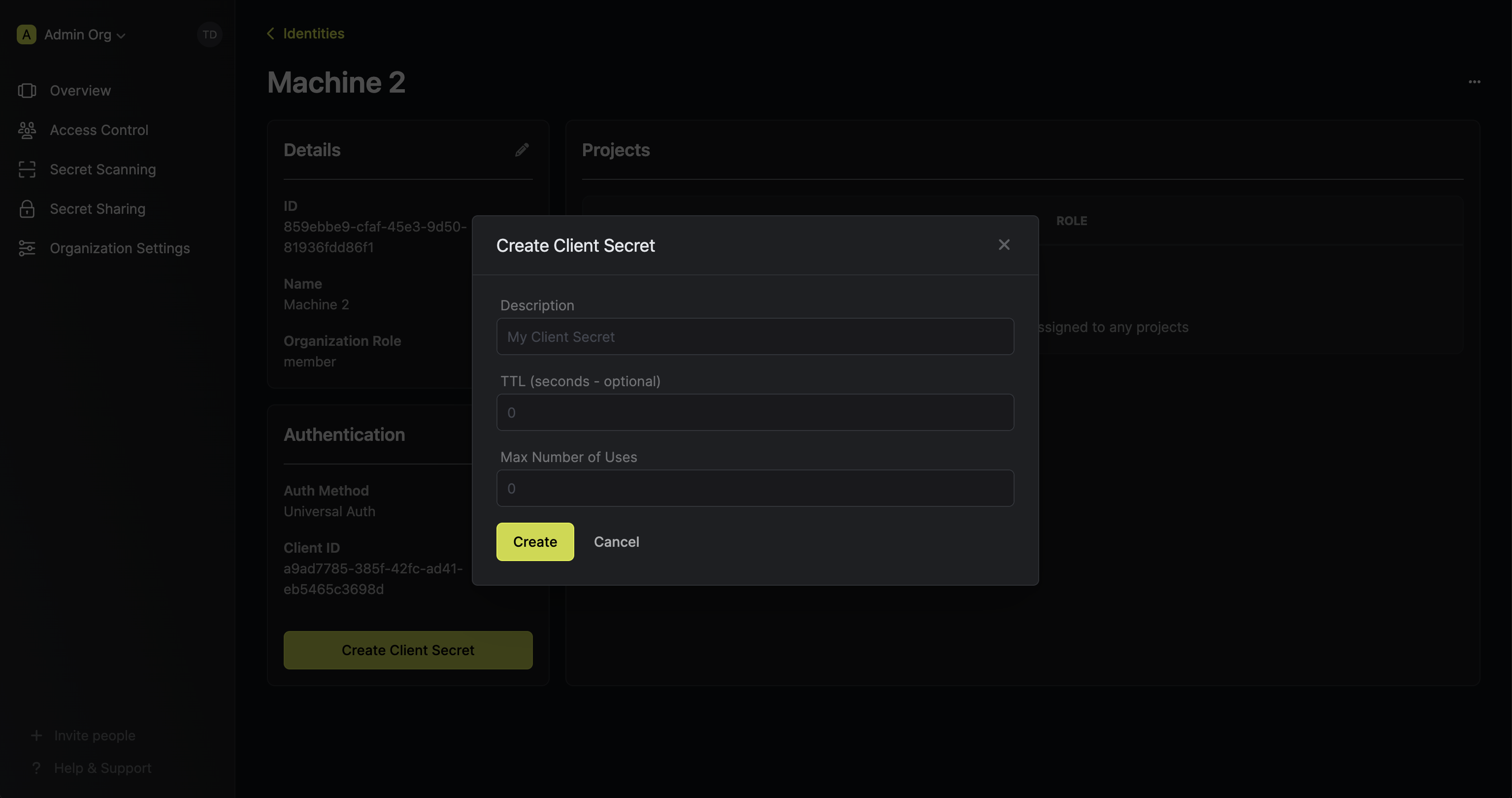
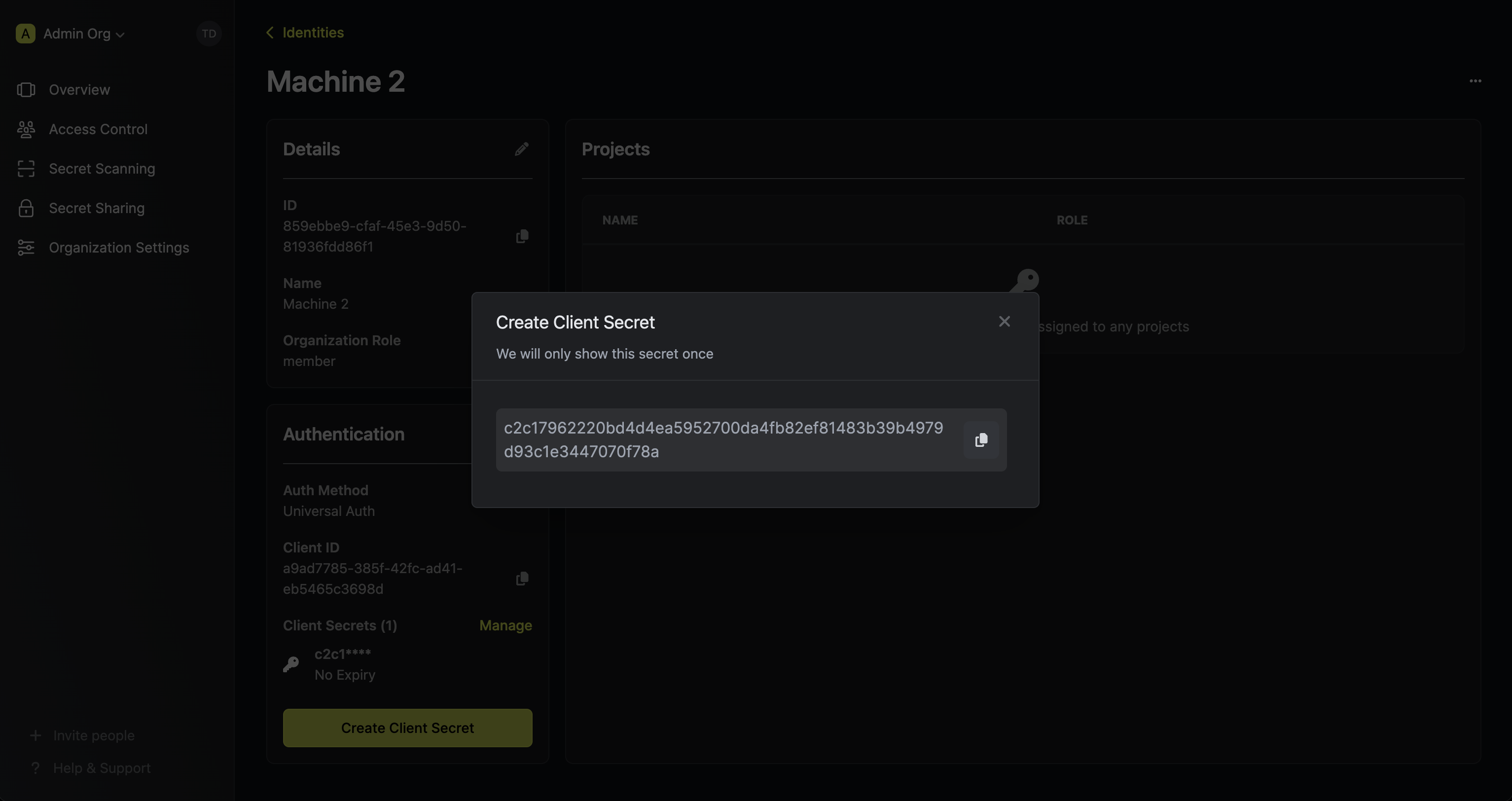
Feel free to input any (optional) details for the **Client Secret** configuration:
* Description: A description for the **Client Secret**.
* TTL (default is `0`): The time-to-live for the **Client Secret**. By default, the TTL will be set to 0 which implies that the **Client Secret** will never expire; a value of `0` implies an infinite lifetime.
* Max Number of Uses (default is `0`): The maximum number of times that the **Client Secret** can be used together with the **Client ID** to get back an access token; a value of `0` implies infinite number of uses.
To enable the identity to access project-level resources such as secrets within a specific project, you should add it to that project.
To do this, head over to the project you want to add the identity to and go to Project Settings > Access Control > Machine Identities and press **Add identity**.
Next, select the identity you want to add to the project and the project level role you want to allow it to assume. The project role assigned will determine what project level resources this identity can have access to.
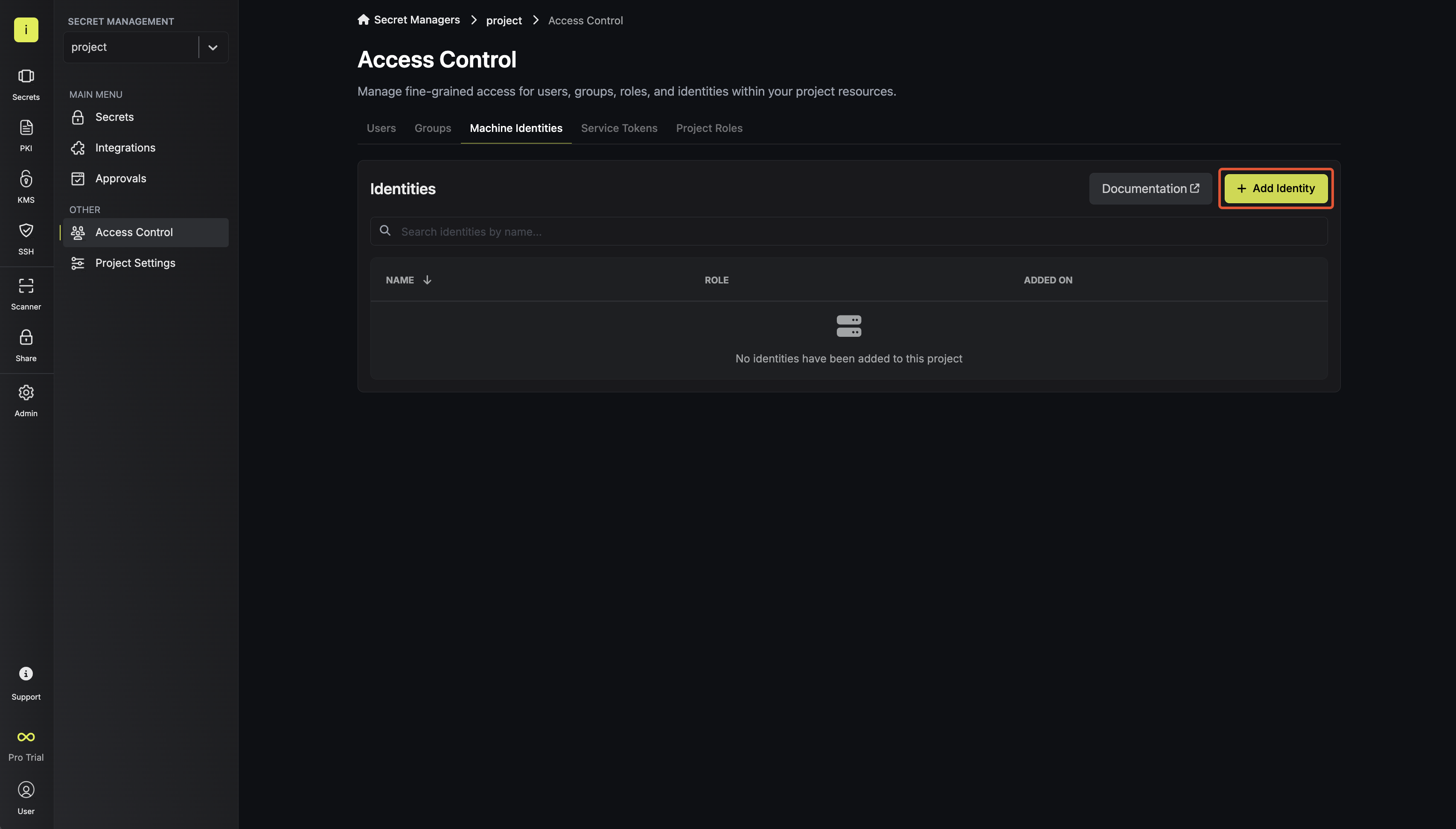
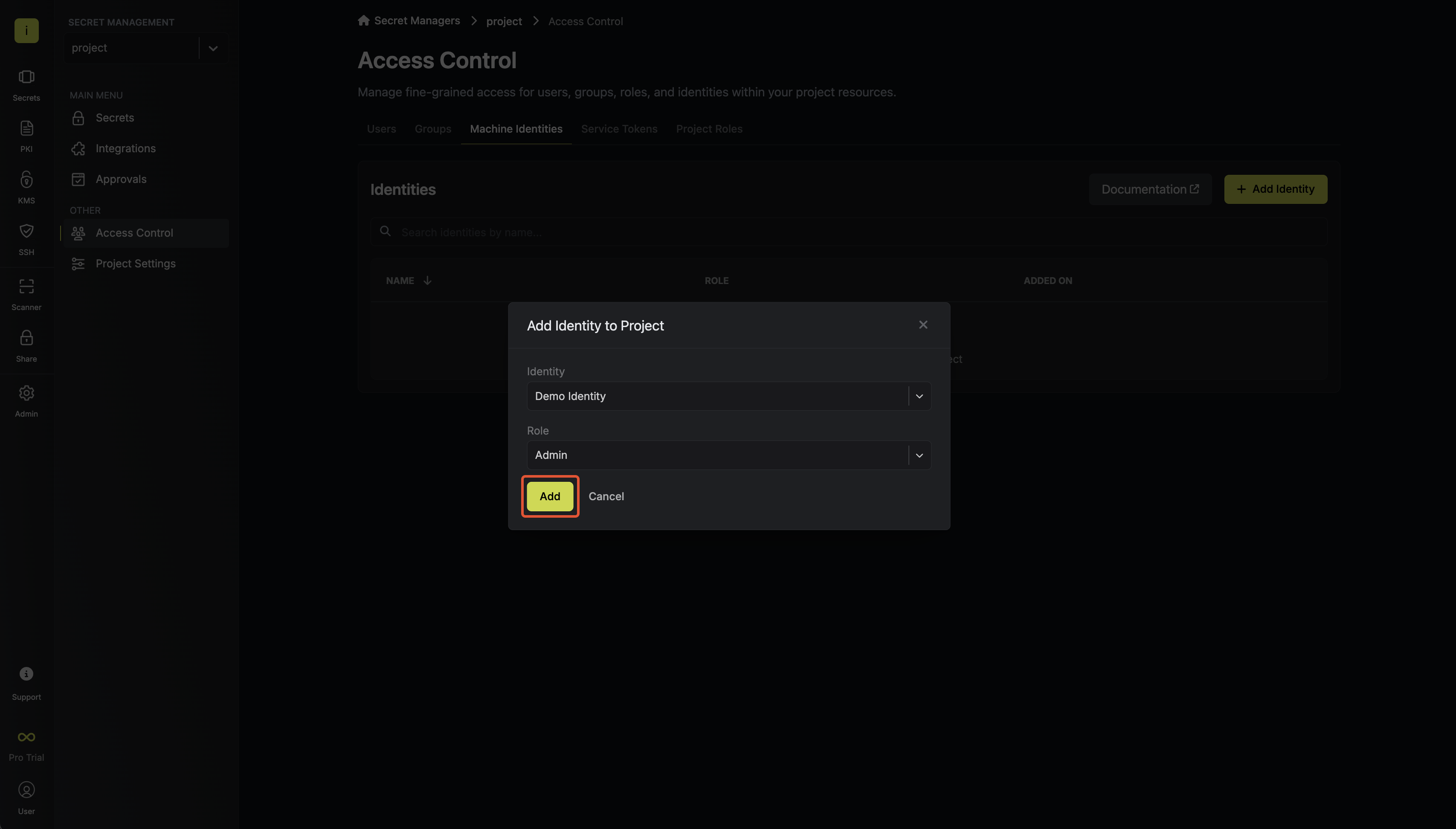
To access the Infisical API as the identity, you should first perform a login operation
that is to exchange the **Client ID** and **Client Secret** of the identity for an access token
by making a request to the `/api/v1/auth/universal-auth/login` endpoint.
#### Sample request
```bash Request
curl --location --request POST 'https://app.infisical.com/api/v1/auth/universal-auth/login' \
--header 'Content-Type: application/x-www-form-urlencoded' \
--data-urlencode 'clientId=...' \
--data-urlencode 'clientSecret=...'
```
#### Sample response
```bash Response
{
"accessToken": "...",
"expiresIn": 7200,
"accessTokenMaxTTL": 43244
"tokenType": "Bearer"
}
```
Next, you can use the access token to authenticate with the [Infisical API](/api-reference/overview/introduction)
Each identity access token has a time-to-live (TLL) which you can infer from the response of the login operation;
the default TTL is `7200` seconds which can be adjusted in the Universal Auth configuration.
If an identity access token expires, it can no longer authenticate with the Infisical API. In this case,
a new access token should be obtained by performing another login operation.
**FAQ**
There are a few reasons for why this might happen:
* The client secret or access token has expired.
* The identity is insufficently permissioned to interact with the resources you wish to access.
* The client secret/access token is being used from an untrusted IP.
A identity access token can have a time-to-live (TTL) or incremental lifetime after which it expires.
In certain cases, you may want to extend the lifespan of an access token; to do so, you must set a max TTL parameter.
A token can be renewed any number of times where each call to renew it can extend the token's lifetime by increments of the access token's TTL.
Regardless of how frequently an access token is renewed, its lifespan remains bound to the maximum TTL determined at its creation.
# User Identities
Read more about the concept of user identities in Infisical.
## Concept
A **user identity** (also known as **user**) represents a developer, admin, or any other human entity interacting with resources in Infisical.
Users can be added manually (through Web UI) or programmatically (e.g., API) to [organizations](../organization) and [projects](../projects).
Upon being added to an organization and projects, users assume a certain set of roles and permissions that represents their identity.

## Authentication methods
To interact with various resources in Infisical, users are able to utilize a number of authentication methods:
* **Email & Password**: the most common authentication method that is used for authentication into Web Dashboard and Infisical CLI. It is recommended to utilize [Multi-factor Authentication](/documentation/platform/mfa) in addition to it.
* **SSO**: Infisical natively integrates with a number of SSO identity providers like [Google](/documentation/platform/sso/google), [GitHub](/documentation/platform/sso/github), and [GitLab](/documentation/platform/sso/gitlab).
* **SAML SSO**: It is also possible to set up SAML SSO integration with identity providers like [Okta](/documentation/platform/sso/okta), [Microsoft Entra ID](/documentation/platform/sso/azure) (formerly known as Azure AD), [JumpCloud](/documentation/platform/sso/jumpcloud), [Google](/documentation/platform/sso/google-saml), and more.
* **LDAP**: For organizations with more advanced needs, Infisical also provides user authentication with [LDAP](/documentation/platform/ldap/overview) that includes a number of LDAP providers.
# AWS CloudHSM
Learn how to manage encryption using AWS CloudHSM
This guide provides instructions on securing Infisical project secrets using AWS CloudHSM.
Integration with AWS CloudHSM is achieved by configuring it as a custom key store for AWS KMS.
Follow the steps below to set up AWS KMS with AWS CloudHSM as the custom key store.
## Prepare AWS CloudHSM Cluster
Before you get started, you'll need to configure a AWS CloudHSM cluster which meets the following criteria:
* The cluster must be active.
* The cluster must not be associated with any other AWS KMS custom key store.
* The cluster must be configured with private subnets in at least two Availability Zones in the Region.
* The security group for the cluster must include inbound and outbound rules that allow TCP traffic on ports 2223-2225.
* The cluster must contain at least two active HSMs in different Availability Zones.
For more details on setting up your cluster, refer to the following [AWS documentation](https://docs.aws.amazon.com/kms/latest/developerguide/create-keystore.html#before-keystore).
## Set Up AWS KMS Custom Key Store
To set up an AWS KMS custom key store with AWS CloudHSM, you will need the following:
* The trust anchor certificate of your AWS CloudHSM cluster.
* A `kmsuser` user in the AWS CloudHSM cluster with the crypto-user role.
In the AWS console, head over to `AWS KMS` > `AWS CloudHSM key stores` and click **Create key store**.
Input the custom key store name. 
Select the AWS CloudHSM cluster. You should be able to select the cluster if it meets the required criteria mentioned above.

Upload your CloudHSM's cluster trust anchor certificate file.
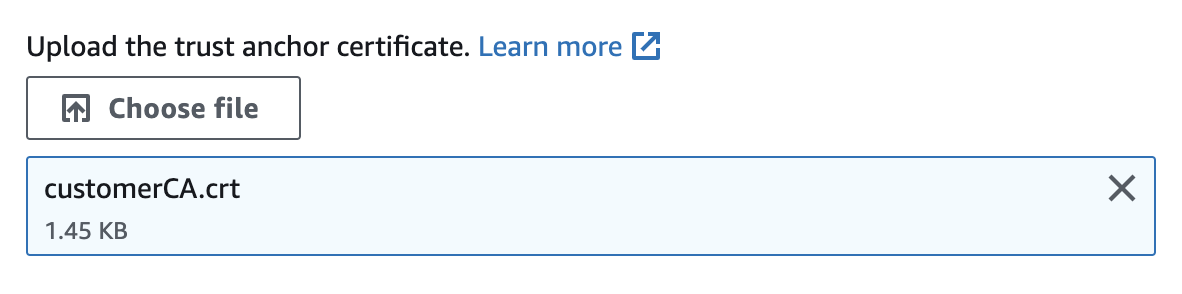
Input the password of the `kmsuser` crypto-user in your cluster.
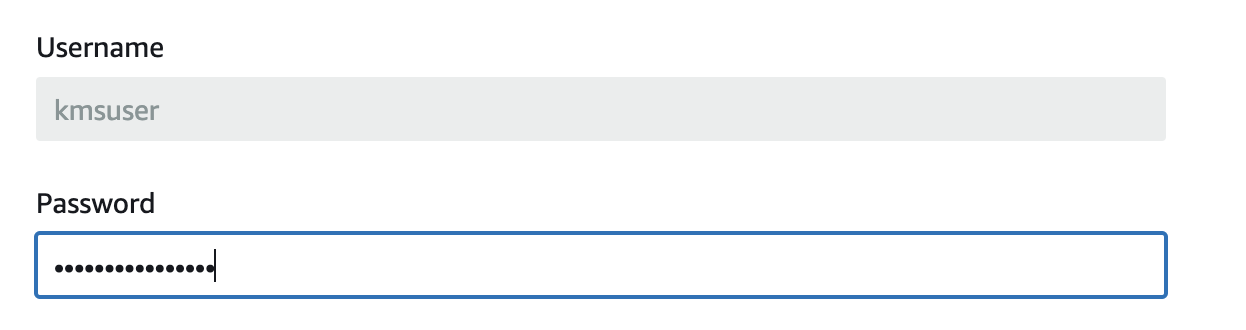
Proceed with creating the AWS CloudHSM key store.
For more details, refer to the following [AWS documentation](https://docs.aws.amazon.com/kms/latest/developerguide/create-keystore.html#create-keystore-console).
## Create AWS KMS Key
Next, you'll need to create a AWS KMS key where you will set the key store you created previously.
In your AWS console, proceed to `AWS KMS` > `Customer managed keys` and click **Create**.
Set Key type to `Symmetric` and Key usage to `Encrypt and decrypt`.
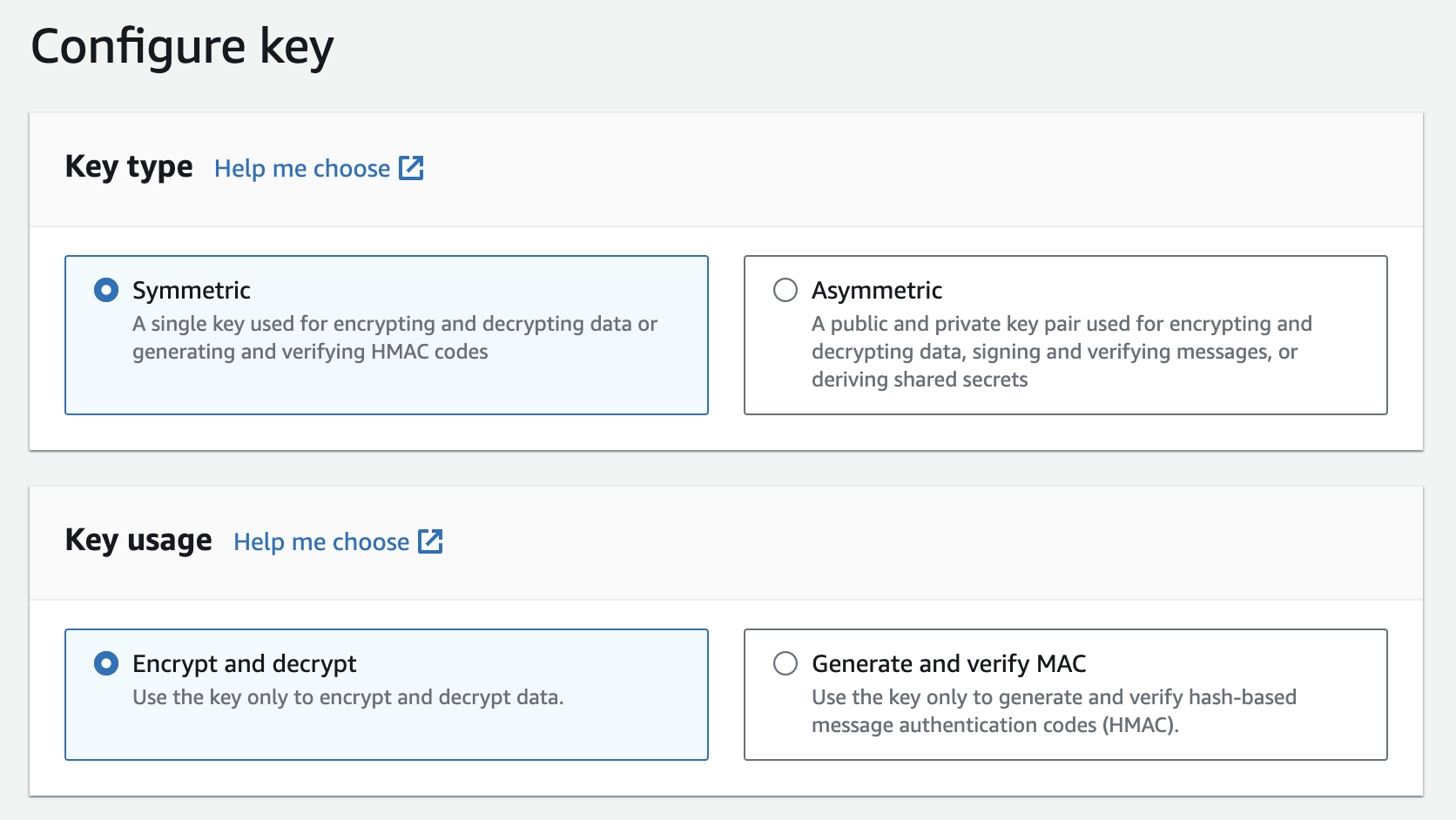
In the advanced options, for the Key material origin field, select `AWS CloudHSM key store`. Then, click next.
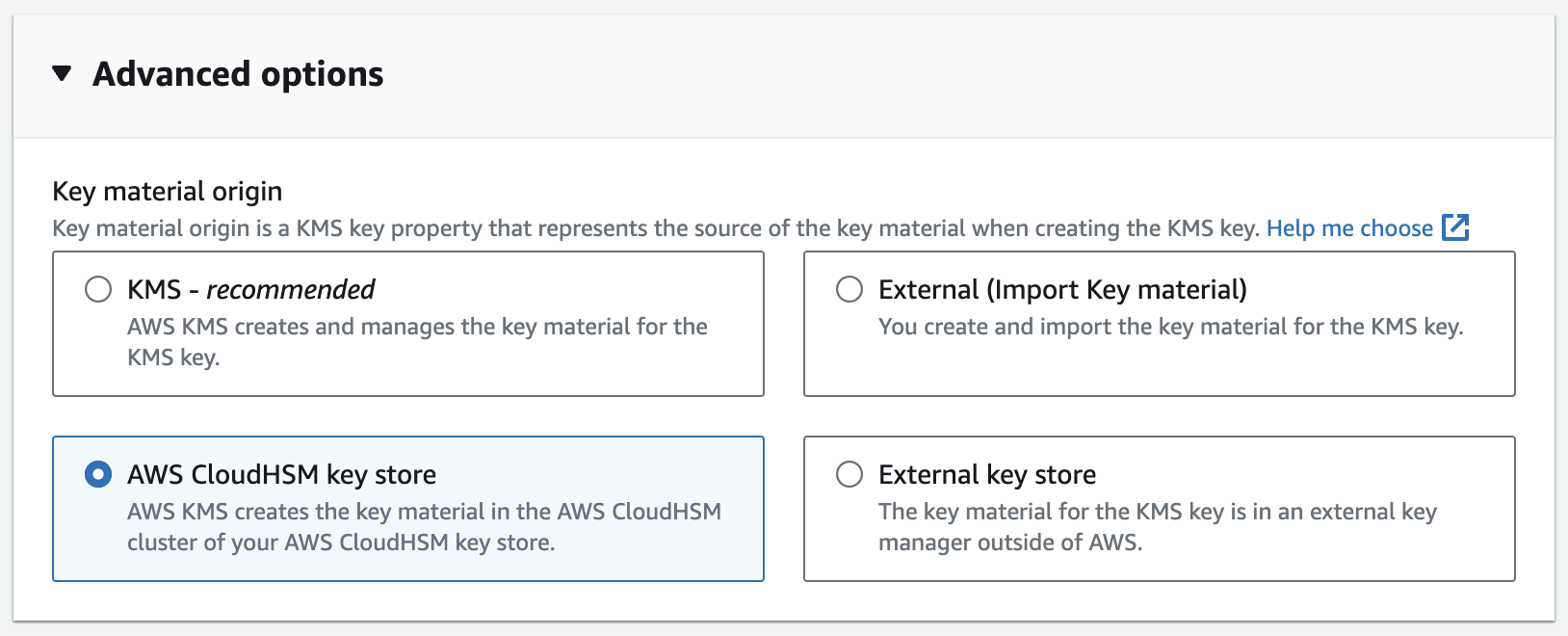
Select the AWS CloudHSM key store you created earlier.
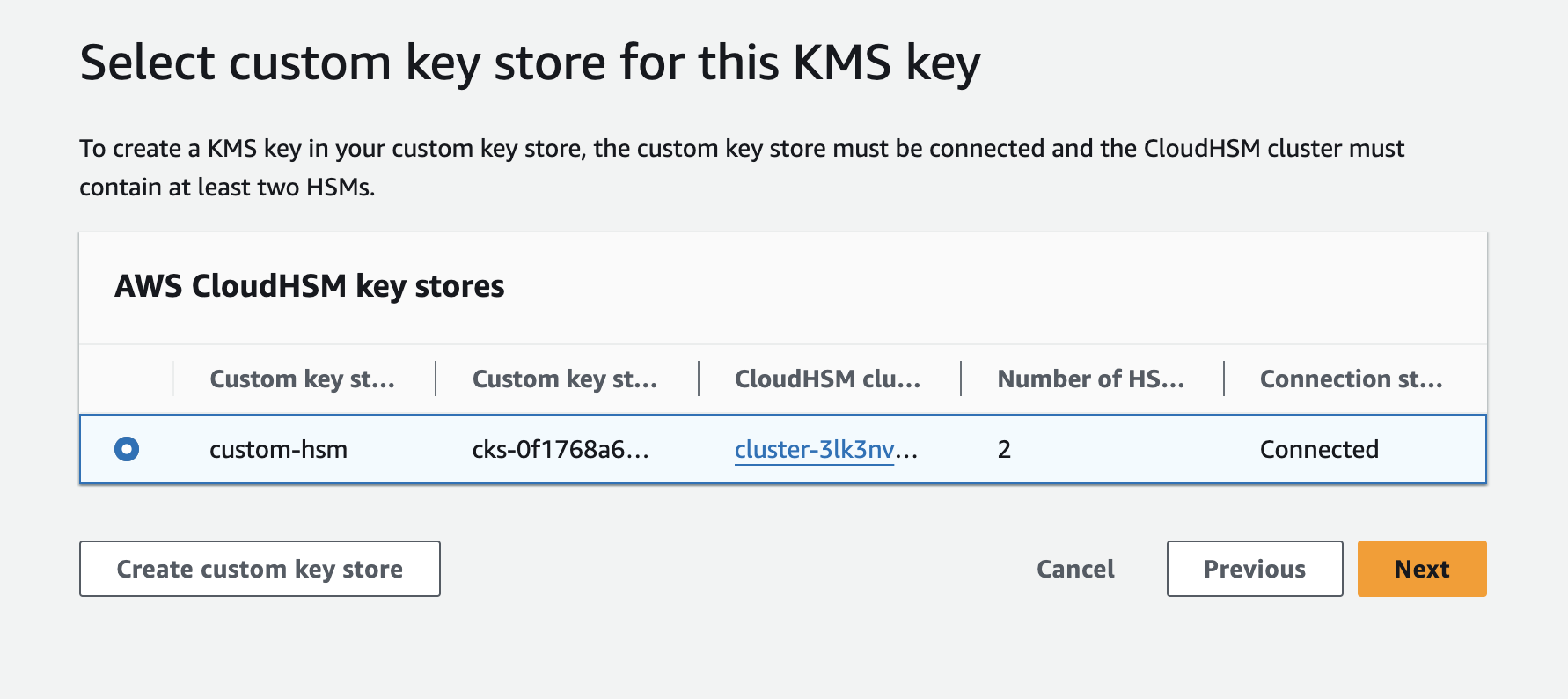
Proceed with creating the AWS KMS Key.
## Connect Infisical to AWS KMS Key
You should now have an AWS KMS that has a custom key store set to AWS CloudHSM.
To secure project resources, you will need to add this AWS KMS to your Infisical organization. To learn how, refer to the documentation [here](./aws-kms).
# AWS Key Management Service
Learn how to manage encryption using AWS KMS
To enhance the security of your Infisical projects, you can now encrypt your secrets using an external Key Management Service (KMS).
When external KMS is configured for your project, all encryption and decryption operations will be handled by the chosen KMS.
This guide will walk you through the steps needed to configure external KMS support with AWS KMS.
## Prerequisites
Before you begin, you'll first need to choose a method of authentication with AWS from below.
1. Navigate to the [Create IAM Role](https://console.aws.amazon.com/iamv2/home#/roles/create?step=selectEntities) page in your AWS Console.
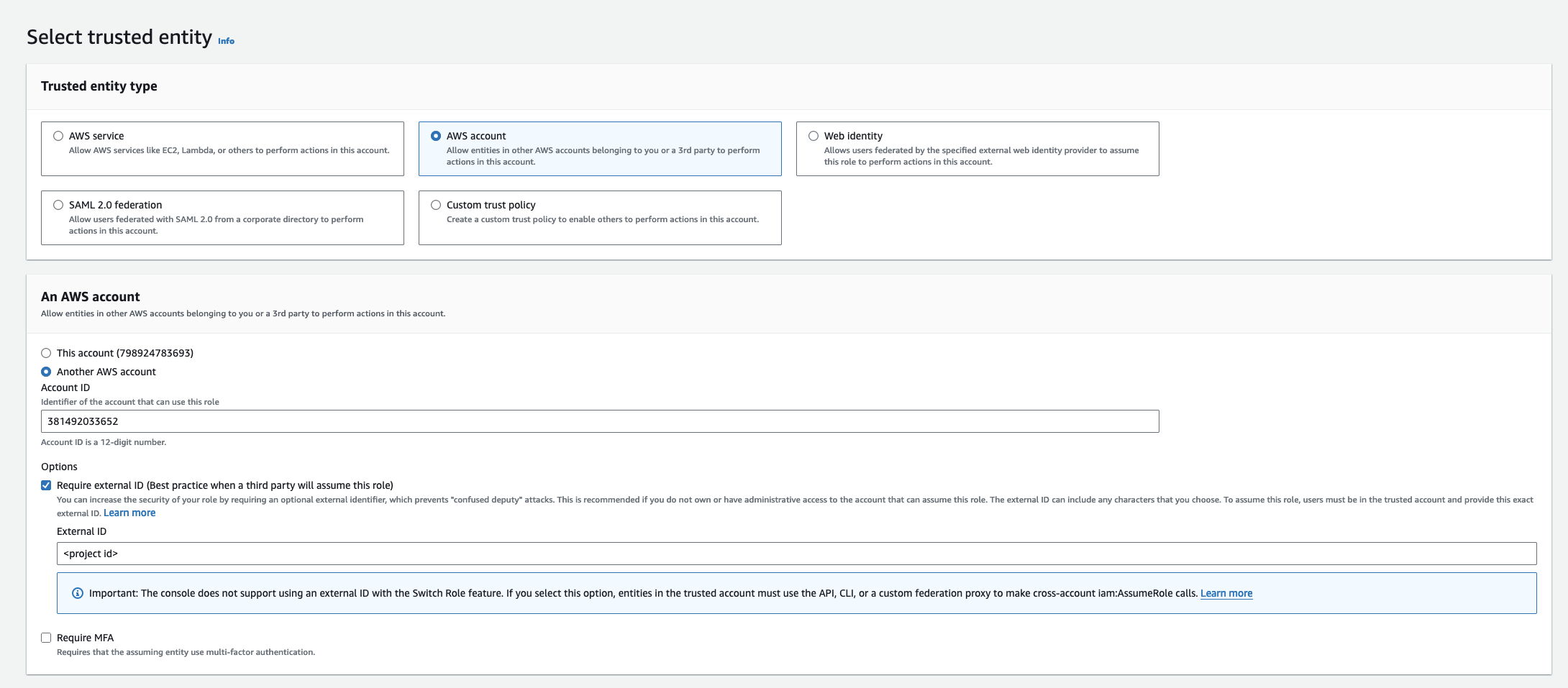
2. Select **AWS Account** as the **Trusted Entity Type**.
3. Choose **Another AWS Account** and enter **381492033652** (Infisical AWS Account ID). This restricts the role to be assumed only by Infisical. If you are self-hosting, provide the AWS account number where Infisical is hosted.
4. Optionally, enable **Require external ID** and enter your Infisical **project ID** to further enhance security.
Use the following custom policy to grant the minimum permissions required by Infisical to integrate with AWS KMS
```json
{
"Version": "2012-10-17",
"Statement": [
{
"Sid": "AllowKMSAccess",
"Effect": "Allow",
"Action": [
"kms:Decrypt",
"kms:Encrypt",
"kms:DescribeKey"
],
"Resource": "*"
}
]
}
```
Navigate to your IAM user and add a policy to grant the following permissions:
```json
{
"Version": "2012-10-17",
"Statement": [
{
"Sid": "AllowKMSAccess",
"Effect": "Allow",
"Action": [
"kms:Decrypt",
"kms:Encrypt",
"kms:DescribeKey"
],
"Resource": "*"
}
]
}
```
## Setup AWS KMS in the Organization Settings
Next, you will need to follow the steps listed below to add AWS KMS for your organization.


Click the 'Add' button to begin adding a new external KMS.

Choose 'AWS KMS' from the list of encryption providers.
Selecting AWS as the provider will require you input the following fields.
Name for referencing the AWS KMS key within the organization.
Short description of the AWS KMS key.
Authentication mode for AWS, either "AWS Assume Role" or "Access Key".
ARN of the AWS role to assume for providing Infisical access to the AWS KMS Key (required if Authentication Mode is "AWS Assume Role")
Custom identifier for additional validation during role assumption.
AWS IAM Access Key ID for authentication (required if Authentication Mode is "Access Key").
AWS IAM Secret Access Key for authentication (required if Authentication Mode is "Access Key").
AWS region where the AWS KMS Key is located.
Key ID of the AWS KMS Key. If left blank, Infisical will generate and use a new AWS KMS Key in the specified region.
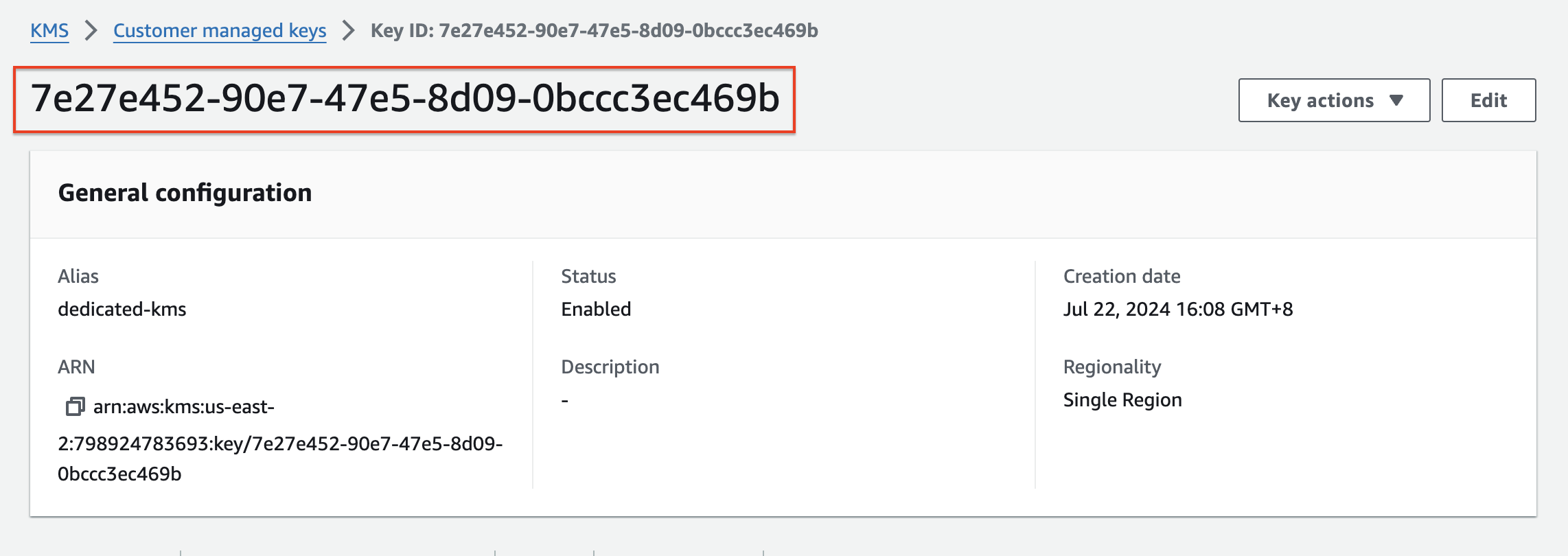
Save your configuration to apply the settings.
You now have an AWS KMS Key configured at the organization level. You can assign these AWS KMS keys to existing Infisical projects by visiting the 'Project Settings' page.
## Assign AWS KMS Key to an Existing Project
To assign the AWS KMS key you added to your organization, follow the steps below.
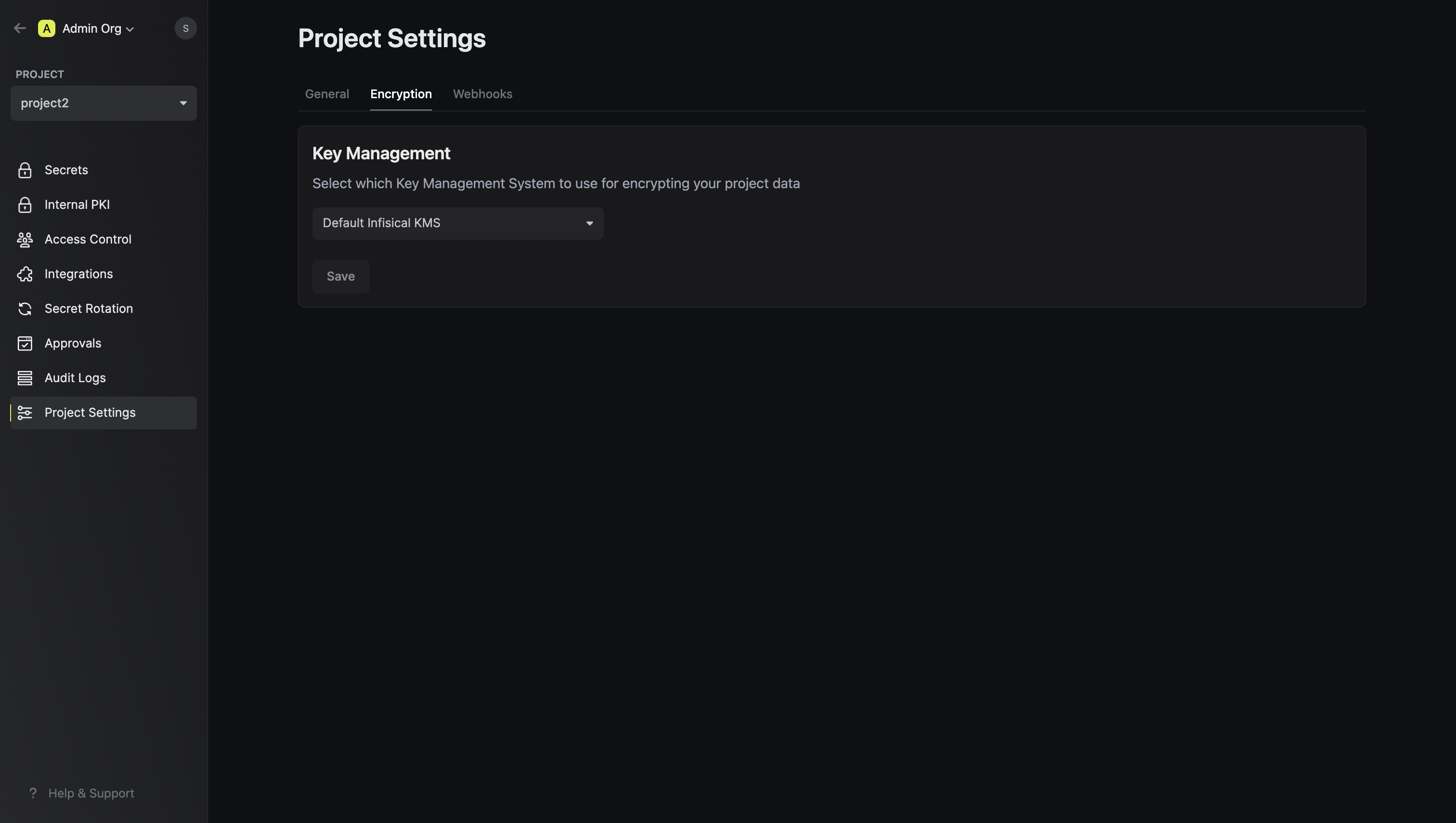
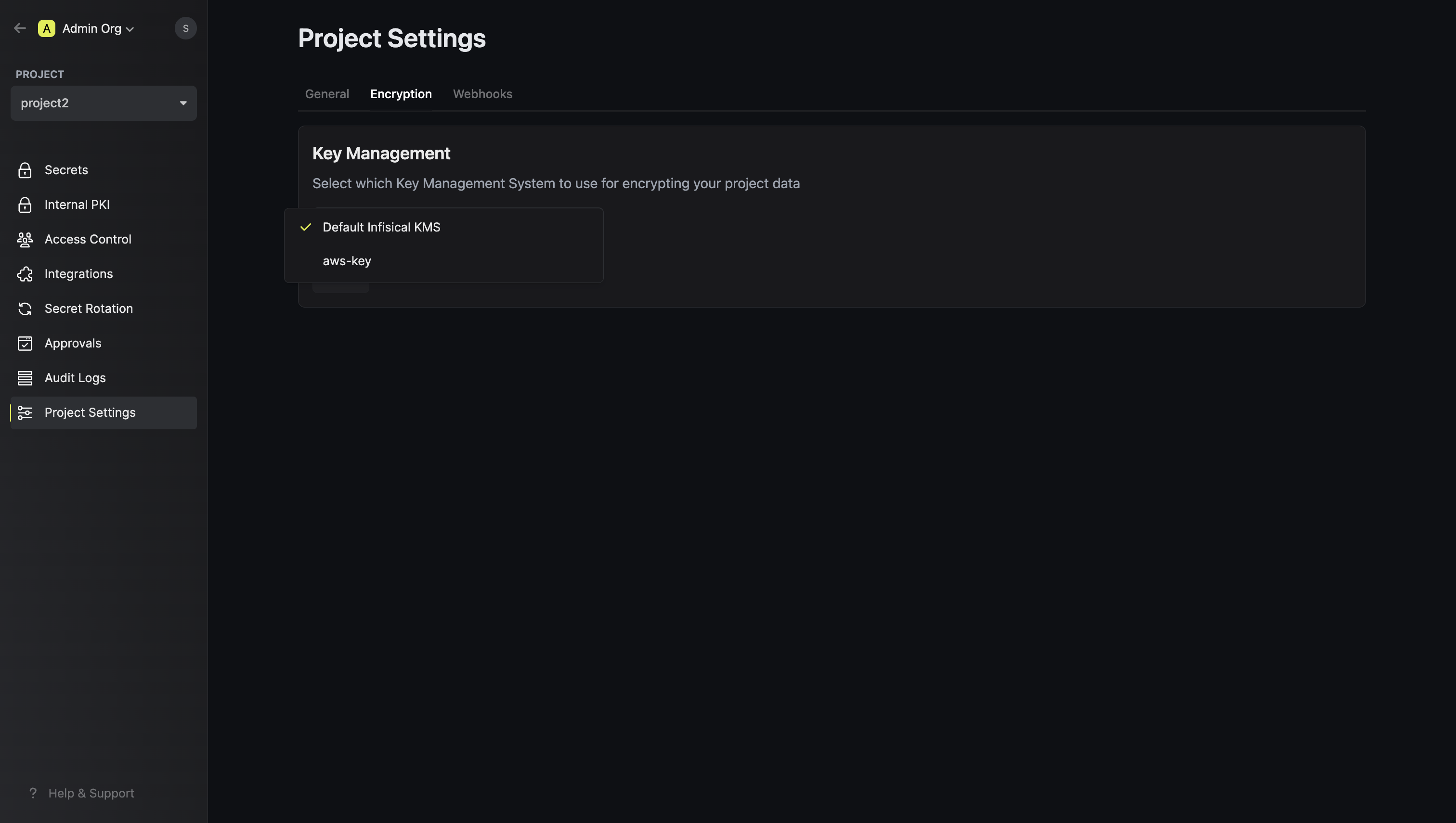
Choose the AWS KMS key you configured earlier.
Once you have selected the KMS of choice, click save.
# Key Management Service (KMS) Configuration
Learn how to configure your project's encryption
## Introduction
Infisical leverages a Key Management Service (KMS) to securely encrypt and decrypt secrets in your projects.
## Overview
Infisical's KMS ensures the security of your project's secrets through the following mechanisms:
* Each project is assigned a unique workspace key, which is responsible for encrypting and decrypting secret values.
* The workspace key itself is encrypted using the project's configured KMS.
* When secrets are requested, the workspace key is derived from the configured KMS. This key is then used to decrypt the secret values on-demand before sending them to the requesting client.
## Configuration
You can set the KMS for new projects during project creation.
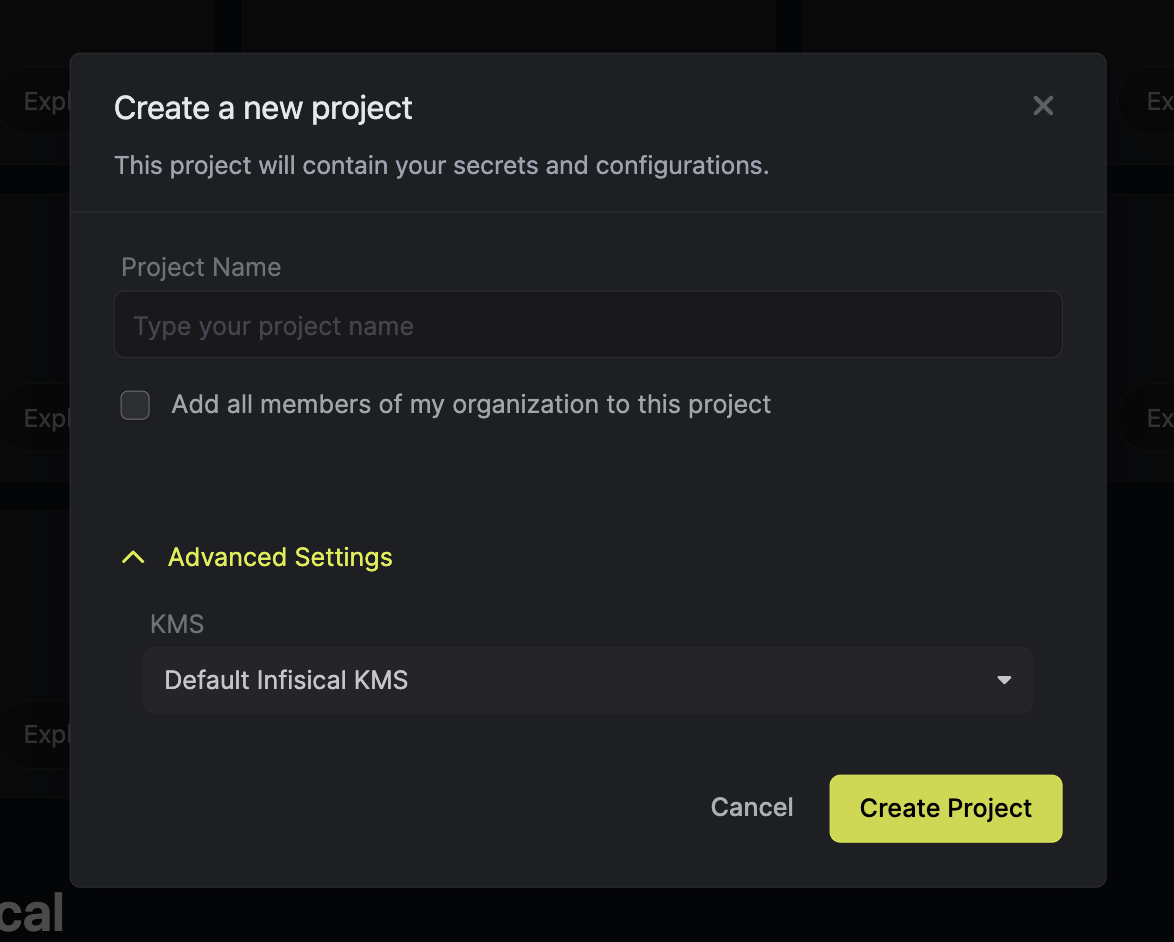
For existing projects, you can configure the KMS from the Project Settings page.
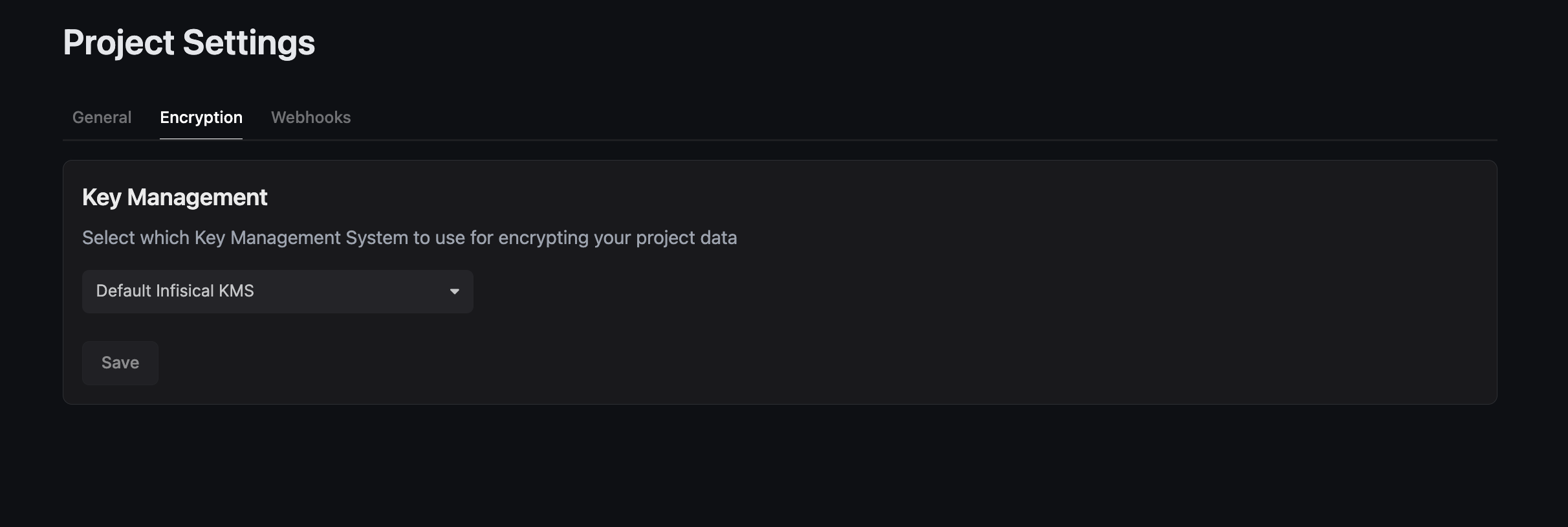
## External KMS
Infisical supports the use of external KMS solutions to enhance security and compliance. You can configure your project to use services like [AWS Key Management Service](./aws-kms) for managing encryption.
# HSM Integration
Learn more about integrating an HSM with Infisical KMS.
Changing the encryption strategy for your instance is an Enterprise-only feature.
This section is intended for users who have obtained an Enterprise license and are on-premise.
Please reach out to [sales@infisical.com](mailto:sales@infisical.com) if you have any questions.
## Overview
Infisical KMS currently supports two encryption strategies:
1. **Standard Encryption**: This is the default encryption strategy used by Infisical KMS. It uses a software-protected encryption key to encrypt KMS keys within your Infisical instance. The root encryption key is defined by setting the `ENCRYPTION_KEY` environment variable.
2. **Hardware Security Module (HSM)**: This encryption strategy uses a Hardware Security Module (HSM) to create a root encryption key that is stored on a physical device to encrypt the KMS keys within your instance.
## Hardware Security Module (HSM)
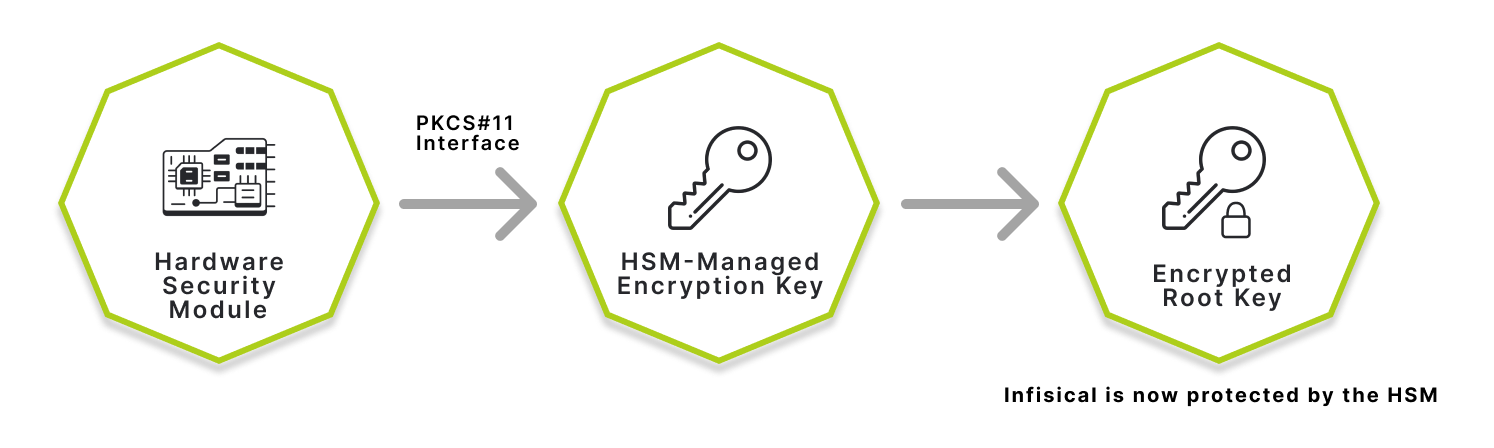
Using a hardware security module comes with the added benefit of having a secure and tamper-proof device to store your encryption keys. This ensures that your data is protected from unauthorized access.
All encryption keys used for cryptographic operations are stored within the HSM. This means that if the HSM is lost or destroyed, you will no longer be able to decrypt your data stored within Infisical. Most providers offer recovery options for HSM devices, which you should consider when setting up an HSM device.
Enabling HSM encryption has a set of key benefits:
1. **Root Key Wrapping**: The root KMS encryption key that is used to secure your Infisical instance will be encrypted using the HSM device rather than the standard software-protected key.
2. **FIPS 140-2/3 Compliance**: Using an HSM device ensures that your Infisical instance is FIPS 140-2 or FIPS 140-3 compliant. For FIPS 140-3, ensure that your HSM is FIPS 140-3 validated.
#### Caveats
* **Performance**: Using an HSM device can have a performance impact on your Infisical instance. This is due to the additional latency introduced by the HSM device. This is however only noticeable when your instance(s) start up or when the encryption strategy is changed.
* **Key Recovery**: If the HSM device is lost or destroyed, you will no longer be able to decrypt your data stored within Infisical. Most HSM providers offer recovery options, which you should consider when setting up an HSM device.
### Requirements
* An Infisical instance with a version number that is equal to or greater than `v0.91.0`.
* If you are using Docker, your instance must be using the `infisical/infisical-fips` image.
* An HSM device from a provider such as [Thales Luna HSM](https://cpl.thalesgroup.com/encryption/data-protection-on-demand/services/luna-cloud-hsm), [AWS CloudHSM](https://aws.amazon.com/cloudhsm/), or others.
### FIPS Compliance
FIPS, also known as the Federal Information Processing Standard, is a set of standards that are used to accredit cryptographic modules. FIPS 140-2 and FIPS 140-3 are the two most common standards used for cryptographic modules. If your HSM uses FIPS 140-3 validated hardware, Infisical will automatically be FIPS 140-3 compliant. If your HSM uses FIPS 140-2 validated hardware, Infisical will be FIPS 140-2 compliant.
HSM devices are especially useful for organizations that operate in regulated industries such as healthcare, finance, and government, where data security and compliance are of the utmost importance.
For organizations that work with US government agencies, FIPS compliance is almost always a requirement when dealing with sensitive information. FIPS compliance ensures that the cryptographic modules used by the organization meet the security requirements set by the US government.
## Setup Instructions
To set up HSM encryption, you need to configure an HSM provider and HSM key. The HSM provider is used to connect to the HSM device, and the HSM key is used to encrypt Infisical's KMS keys. We recommend using a Cloud HSM provider such as [Thales Luna HSM](https://cpl.thalesgroup.com/encryption/data-protection-on-demand/services/luna-cloud-hsm) or [AWS CloudHSM](https://aws.amazon.com/cloudhsm/).
You need to follow the instructions provided by the HSM provider to set up the HSM device. Once the HSM device is set up, the HSM device can be used within Infisical.
After setting up the HSM from your provider, you will have a set of files that you can use to access the HSM. These files need to be present on the machine where Infisical is running.
If you are using containers, you will need to mount the folder where these files are stored as a volume in the container.
The setup process for an HSM device varies depending on the provider. We have created a guide for Thales Luna Cloud HSM, which you can find below.
Are you using Docker? If you are using Docker, please follow the instructions in the [Using HSM's with Docker](#using-hsms-with-docker) section.
Configuring the HSM on Infisical requires setting a set of environment variables:
* `HSM_LIB_PATH`: The path to the PKCS#11 library provided by the HSM provider. This usually comes in the form of a `.so` for Linux and MacOS, or a `.dll` file for Windows. For Docker, you need to mount the library path as a volume. Further instructions can be found below. If you are using Docker, make sure to set the HSM\_LIB\_PATH environment variable to the path where the library is mounted in the container.
* `HSM_PIN`: The PKCS#11 PIN to use for authentication with the HSM device.
* `HSM_SLOT`: The slot number to use for the HSM device. This is typically between `0` and `5` for most HSM devices.
* `HSM_KEY_LABEL`: The label of the key to use for encryption. **Please note that if no key is found with the provided label, the HSM will create a new key with the provided label.**
You can read more about the [default instance configurations](/self-hosting/configuration/envars) here.
After setting up the HSM, you need to restart the Infisical instance for the changes to take effect.
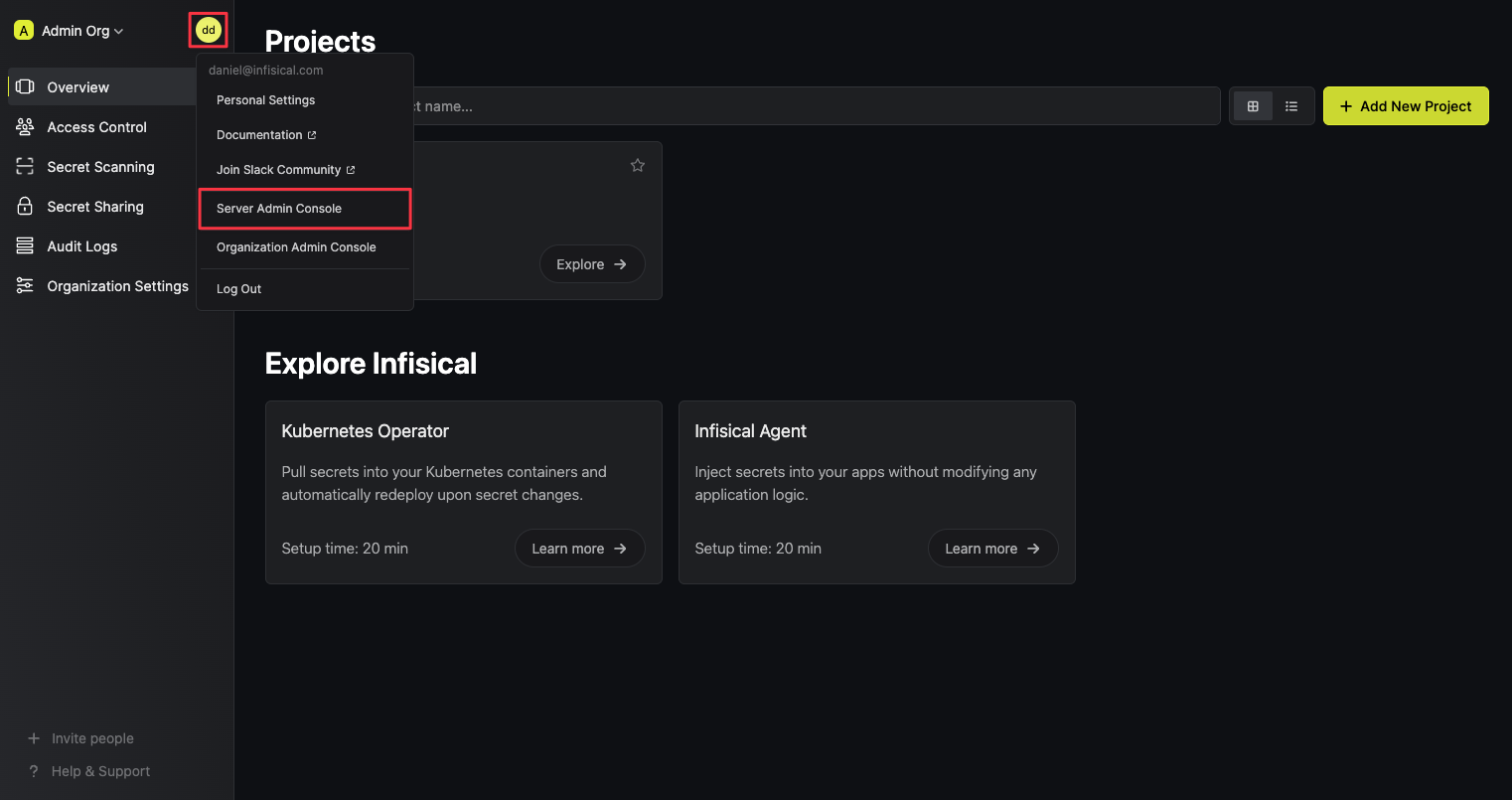
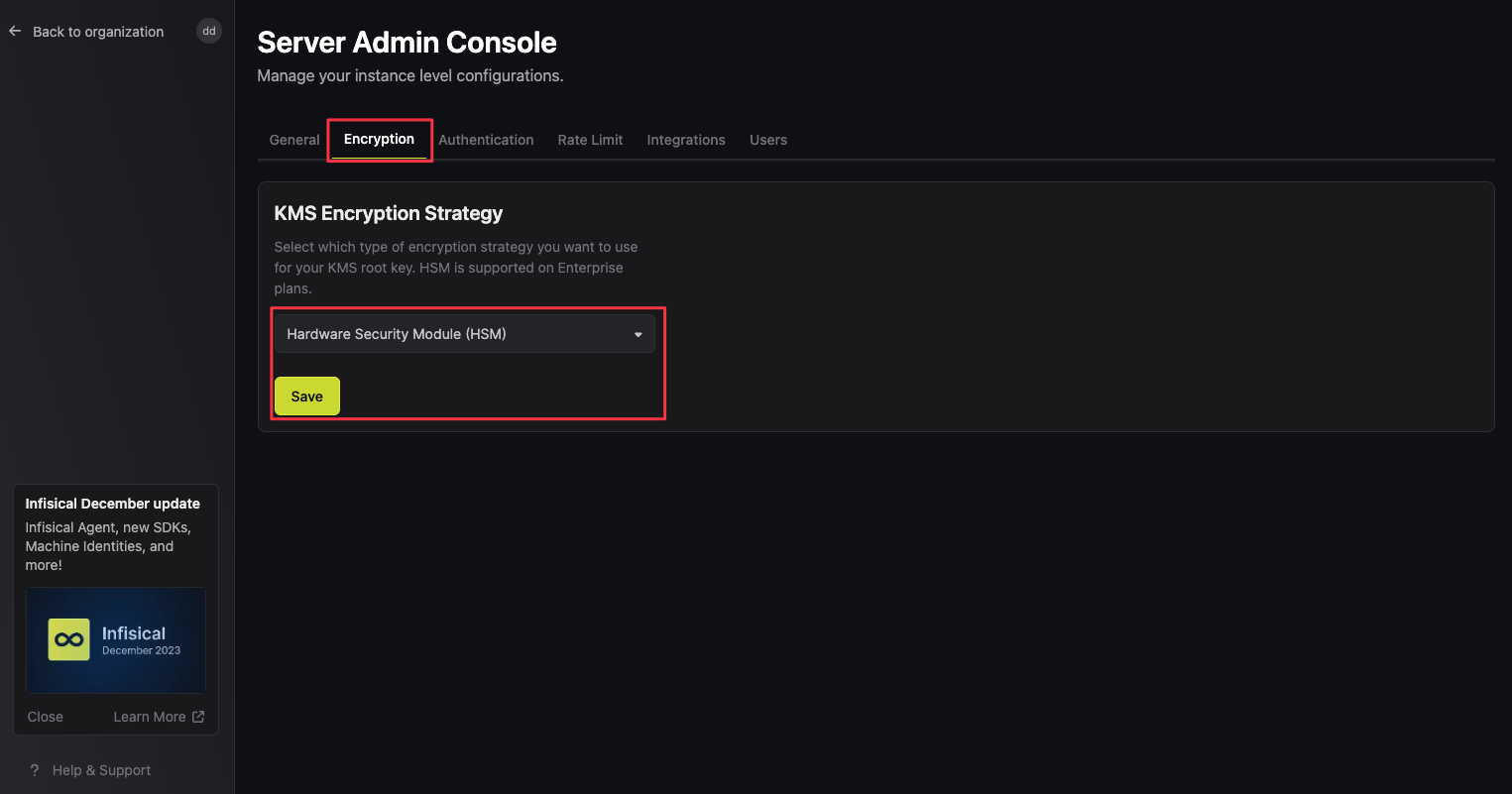
Once you press the 'Save' button, your Infisical instance will immediately switch to the HSM encryption strategy. This will re-encrypt your KMS key with keys from the HSM device.
To verify that the HSM was correctly configured, you can try creating a new secret in one of your projects. If the secret is created successfully, the HSM is now being used for encryption.
## Using HSMs with Docker
When using Docker, you need to mount the path containing the HSM client files. This section covers how to configure your Infisical instance to use an HSM with Docker.
When using Docker, you are able to set your HSM library path to any location on your machine. In this example, we are going to be using `/etc/luna-docker`.
```bash
mkdir /etc/luna-docker
```
After [setting up your Luna Cloud HSM client](https://thalesdocs.com/gphsm/luna/7/docs/network/Content/install/client_install/add_dpod.htm), you should have a set of files, referred to as the HSM client. You don't need all the files, but for simplicity we recommend copying all the files from the client.
A folder structure of a client folder will often look like this:
```
partition-ca-certificate.pem
partition-certificate.pem
server-certificate.pem
Chrystoki.conf
/plugins
libcloud.plugin
/lock
/libs
/64
libCryptoki2.so
/jsp
LunaProvider.jar
/64
libLunaAPI.so
/etc
openssl.cnf
/bin
/64
ckdemo
lunacm
multitoken
vtl
```
The most important parts of the client folder is the `Chrystoki.conf` file, and the `libs`, `plugins`, and `jsp` folders. You need to copy these files to the folder you created in the first step.
```bash
cp -r / /etc/luna-docker
```
The `Chrystoki.conf` file is used to configure the HSM client. You need to update the `Chrystoki.conf` file to point to the correct file paths.
In this example, we will be mounting the `/etc/luna-docker` folder to the Docker container under a different path. The path we will use in this example is `/usr/safenet/lunaclient`. This means `/etc/luna-docker` will be mounted to `/usr/safenet/lunaclient` in the Docker container.
An example config file will look like this:
```Chrystoki.conf
Chrystoki2 = {
# This path points to the mounted path, /usr/safenet/lunaclient
LibUNIX64 = /usr/safenet/lunaclient/libs/64/libCryptoki2.so;
}
Luna = {
DefaultTimeOut = 500000;
PEDTimeout1 = 100000;
PEDTimeout2 = 200000;
PEDTimeout3 = 20000;
KeypairGenTimeOut = 2700000;
CloningCommandTimeOut = 300000;
CommandTimeOutPedSet = 720000;
}
CardReader = {
LunaG5Slots = 0;
RemoteCommand = 1;
}
Misc = {
# Update the paths to point to the mounted path if your folder structure is different from the one mentioned in the previous step.
PluginModuleDir = /usr/safenet/lunaclient/plugins;
MutexFolder = /usr/safenet/lunaclient/lock;
PE1746Enabled = 1;
ToolsDir = /usr/bin;
}
Presentation = {
ShowEmptySlots = no;
}
LunaSA Client = {
ReceiveTimeout = 20000;
# Update the paths to point to the mounted path if your folder structure is different from the one mentioned in the previous step.
SSLConfigFile = /usr/safenet/lunaclient/etc/openssl.cnf;
ClientPrivKeyFile = ./etc/ClientNameKey.pem;
ClientCertFile = ./etc/ClientNameCert.pem;
ServerCAFile = ./etc/CAFile.pem;
NetClient = 1;
TCPKeepAlive = 1;
}
REST = {
AppLogLevel = error
ServerName = ;
ServerPort = 443;
AuthTokenConfigURI = ;
AuthTokenClientId = ;
AuthTokenClientSecret = ;
RestClient = 1;
ClientTimeoutSec = 120;
ClientPoolSize = 32;
ClientEofRetryCount = 15;
ClientConnectRetryCount = 900;
ClientConnectIntervalMs = 1000;
}
XTC = {
Enabled = 1;
TimeoutSec = 600;
}
```
Save the file after updating the paths.
Running Docker with HSM encryption requires setting the HSM-related environment variables as mentioned previously in the [HSM setup instructions](#setup-instructions). You can set these environment variables in your Docker run command.
We are setting the environment variables for Docker via the command line in this example, but you can also pass in a `.env` file to set these environment variables.
If no key is found with the provided key label, the HSM will create a new key with the provided label.
Infisical depends on an AES and HMAC key to be present in the HSM. If these keys are not present, Infisical will create them. The AES key label will be the value of the `HSM_KEY_LABEL` environment variable, and the HMAC key label will be the value of the `HSM_KEY_LABEL` environment variable with the suffix `_HMAC`.
```bash
docker run -p 80:8080 \
-v /etc/luna-docker:/usr/safenet/lunaclient \
-e HSM_LIB_PATH="/usr/safenet/lunaclient/libs/64/libCryptoki2.so" \
-e HSM_PIN="" \
-e HSM_SLOT= \
-e HSM_KEY_LABEL="" \
# The rest are unrelated to HSM setup...
-e ENCRYPTION_KEY="<>" \
-e AUTH_SECRET="<>" \
-e DB_CONNECTION_URI="<>" \
-e REDIS_URL="<>" \
-e SITE_URL="<>" \
infisical/infisical-fips: # Replace with the version you want to use
```
We recommend reading further about [using Infisical with Docker](/self-hosting/deployment-options/standalone-infisical).
After following these steps, your Docker setup will be ready to use HSM encryption.
## Disabling HSM Encryption
To disable HSM encryption, navigate to Infisical's Server Admin Console and set the KMS encryption strategy to `Software-based Encryption`. This will revert the encryption strategy back to the default software-based encryption.
In order to disable HSM encryption, the Infisical instance must be able to access the HSM device. If the HSM device is no longer accessible, you will not be able to disable HSM encryption.
# Kubernetes Encryption with KMS
# Key Management Service (KMS)
Learn how to manage and use cryptographic keys with Infisical.
## Concept
Infisical can be used as a Key Management System (KMS), referred to as Infisical KMS, to centralize management of keys to be used for cryptographic operations like encryption/decryption.
By default your Infisical data such as projects and the data within them are encrypted at rest using Infisical's own KMS. This ensures that your data is secure and protected from unauthorized access.
If you are on-premise, your KMS root key will be created at random with the `ROOT_ENCRYPTION_KEY` environment variable. You can also use a Hardware Security Module (HSM), to create the root key. Read more about [HSM](/docs/documentation/platform/kms/encryption-strategies).
Keys managed in KMS are not extractable from the platform. Additionally, data
is never stored when performing cryptographic operations.
## Workflow
The typical workflow for using Infisical KMS consists of the following steps:
1. Creating a KMS key. As part of this step, you specify a name for the key and the encryption algorithm meant to be used for it (e.g. `AES-GCM-128`, `AES-GCM-256`).
2. Encryption: To encrypt data, you would make a request to the Infisical KMS API endpoint, specifying the base64-encoded plaintext and the intended key to use for encryption; the API would return the base64-encoded ciphertext.
3. Decryption: To decrypt data, you would make a request to the Infisical KMS API endpoint, specifying the base64-encoded ciphertext and the intended key to use for decryption; the API would return the base64-encoded plaintext.
Note that this workflow can be executed via the Infisical UI or manually such
as via API.
## Guide to Encrypting Data
In the following steps, we explore how to generate a key and use it to encrypt data.
Navigate to Project > Key Management and tap on the **Add Key** button.
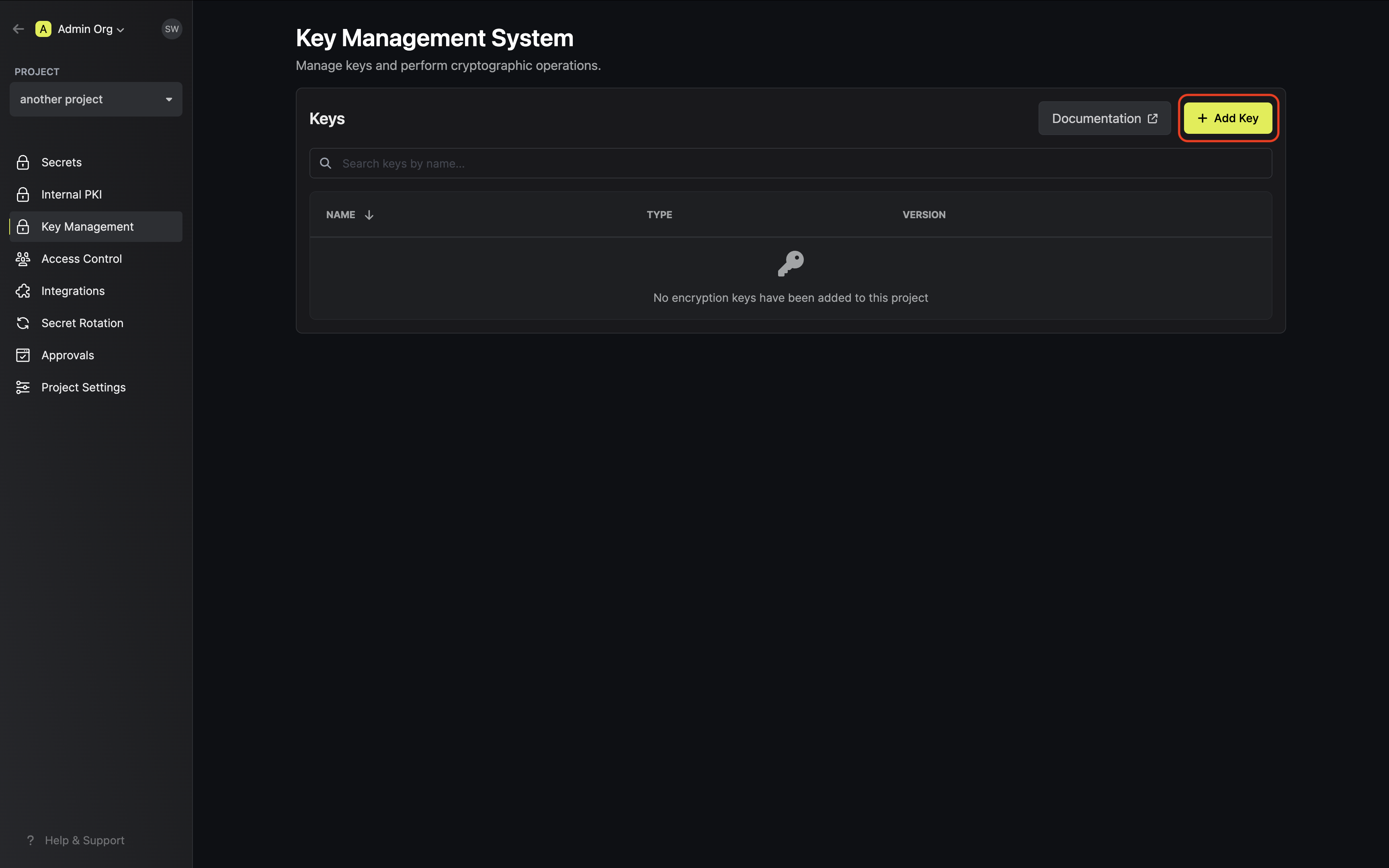
Specify your key details. Here's some guidance on each field:
* Name: A slug-friendly name for the key.
* Type: The encryption algorithm associated with the key (e.g. `AES-GCM-256`).
* Description: An optional description of what the intended usage is for the key.
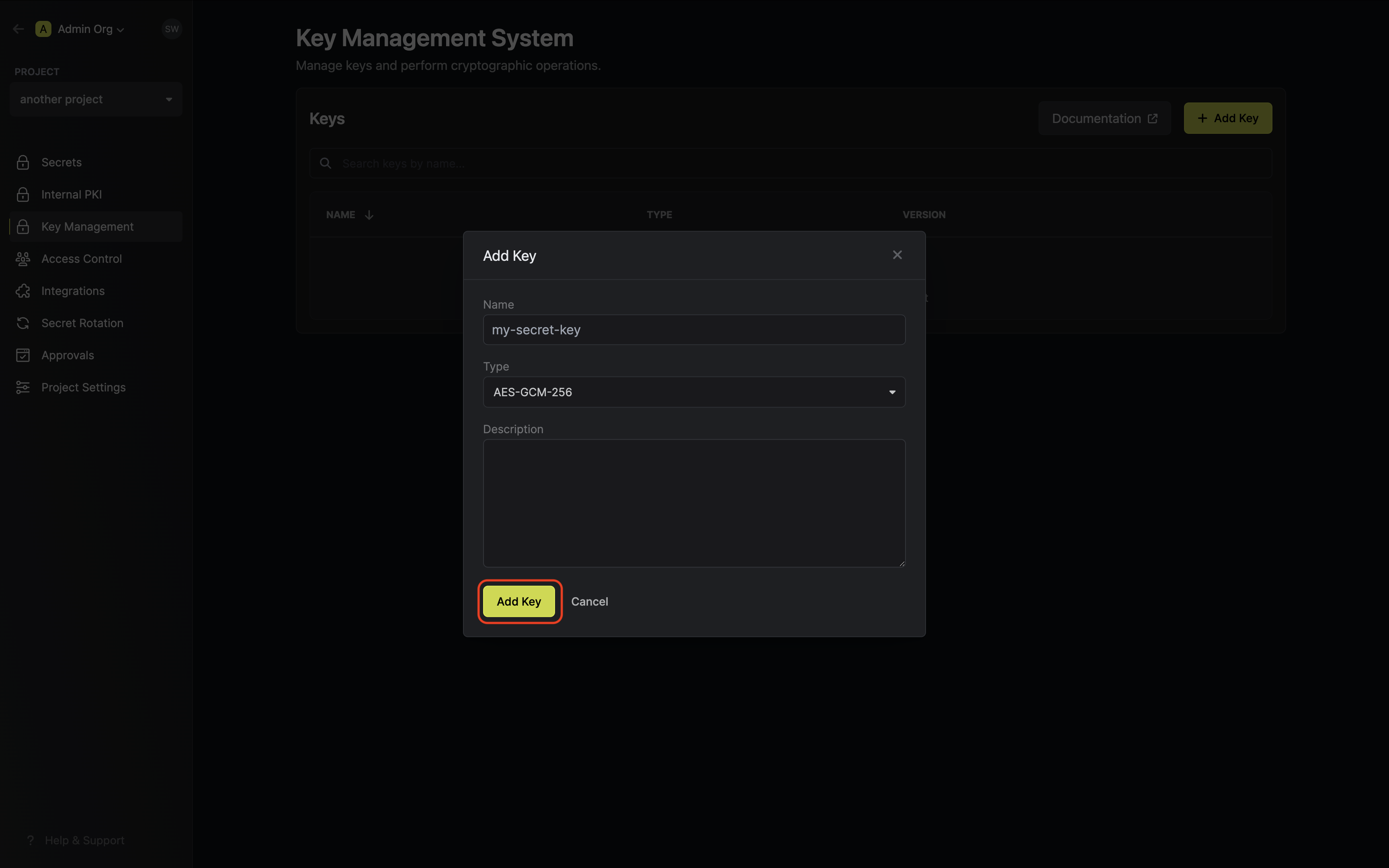
Once your key is generated, open the options menu for the newly created key and select encrypt data.
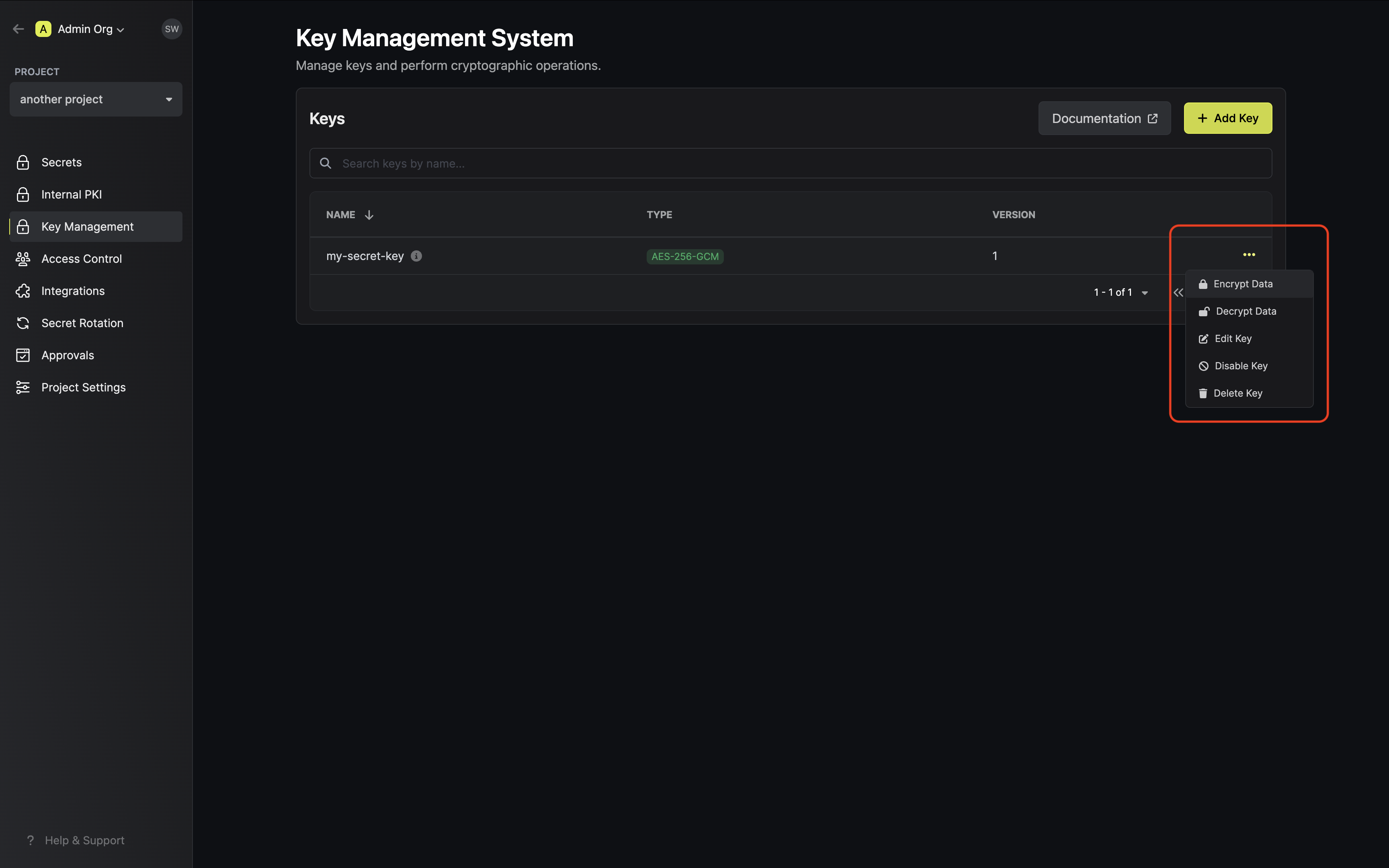
Populate the text area with your data and tap on the Encrypt button.
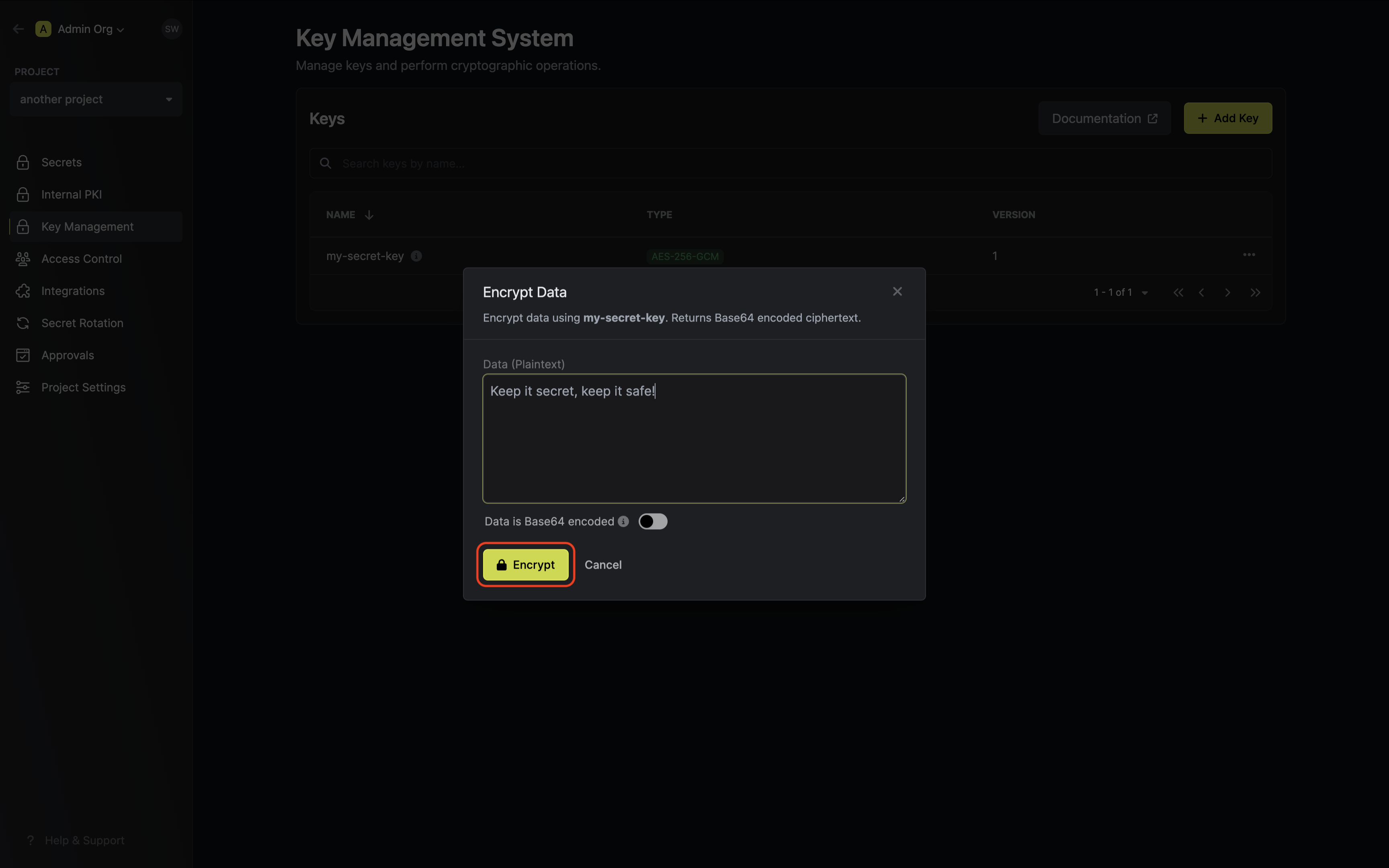
If your data is already Base64 encoded make sure to toggle the respective switch on to avoid
redundant encoding.
Copy and store the encrypted data.
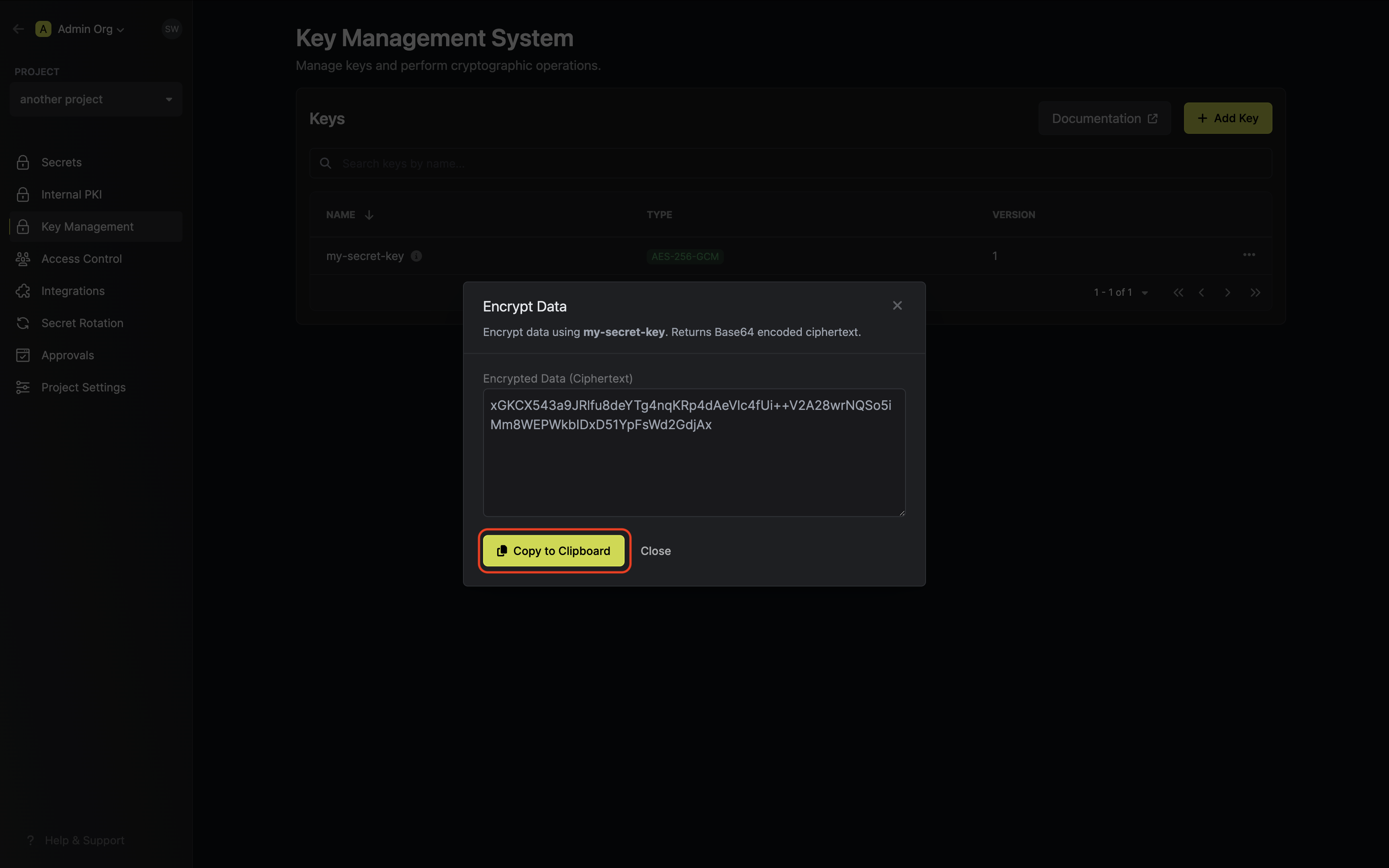
To create a cryptographic key, make an API request to the [Create KMS
Key](/api-reference/endpoints/kms/keys/create) API endpoint.
### Sample request
```bash Request
curl --request POST \
--url https://app.infisical.com/api/v1/kms/keys \
--header 'Content-Type: application/json' \
--data '{
"projectId": "",
"name": "my-secret-key",
"description": "...",
"encryptionAlgorithm": "aes-256-gcm"
}'
```
### Sample response
```bash Response
{
"key": {
"id": "",
"description": "...",
"isDisabled": false,
"isReserved": false,
"orgId": "",
"name": "my-secret-key",
"createdAt": "2023-11-07T05:31:56Z",
"updatedAt": "2023-11-07T05:31:56Z",
"projectId": ""
}
}
```
To encrypt data, make an API request to the [Encrypt
Data](/api-reference/endpoints/kms/keys/encrypt) API endpoint,
specifying the key to use.
Make sure your data is Base64 encoded
### Sample request
```bash Request
curl --request POST \
--url https://app.infisical.com/api/v1/kms/keys//encrypt \
--header 'Content-Type: application/json' \
--data '{
"plaintext": "lUFHM5Ggwo6TOfpuN1S==" // base64 encoded plaintext
}'
```
### Sample response
```bash Response
{
"ciphertext": "HwFHwSFHwlMF6TOfp==" // base64 encoded ciphertext
}
```
## Guide to Decrypting Data
In the following steps, we explore how to use decrypt data using an existing key in Infisical KMS.
Navigate to Project > Key Management and open the options menu for the key used to encrypt the data
you want to decrypt.
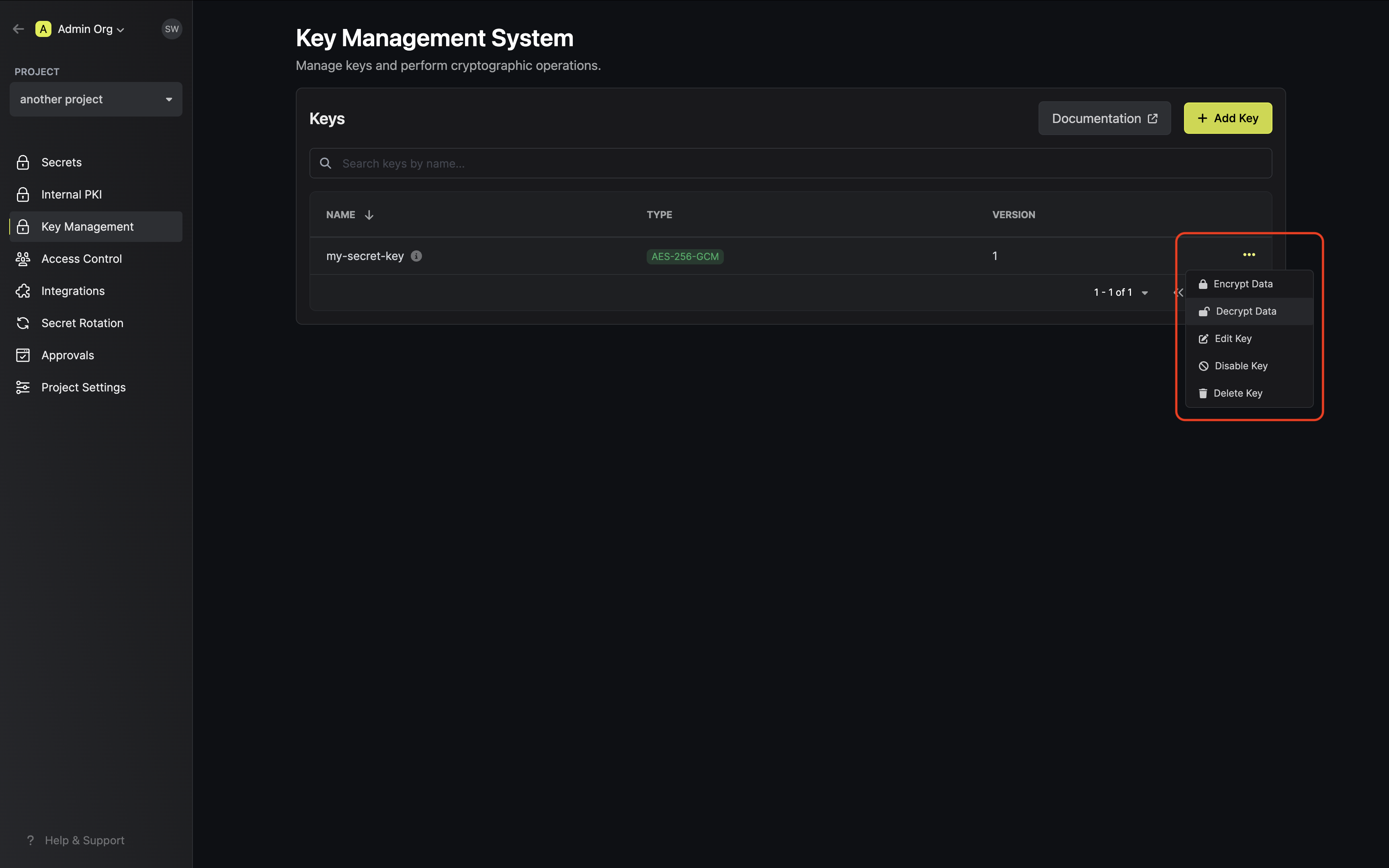
Paste your encrypted data into the text area and tap on the Decrypt button. Optionally, if your data was
originally plain text, enable the decode Base64 switch.
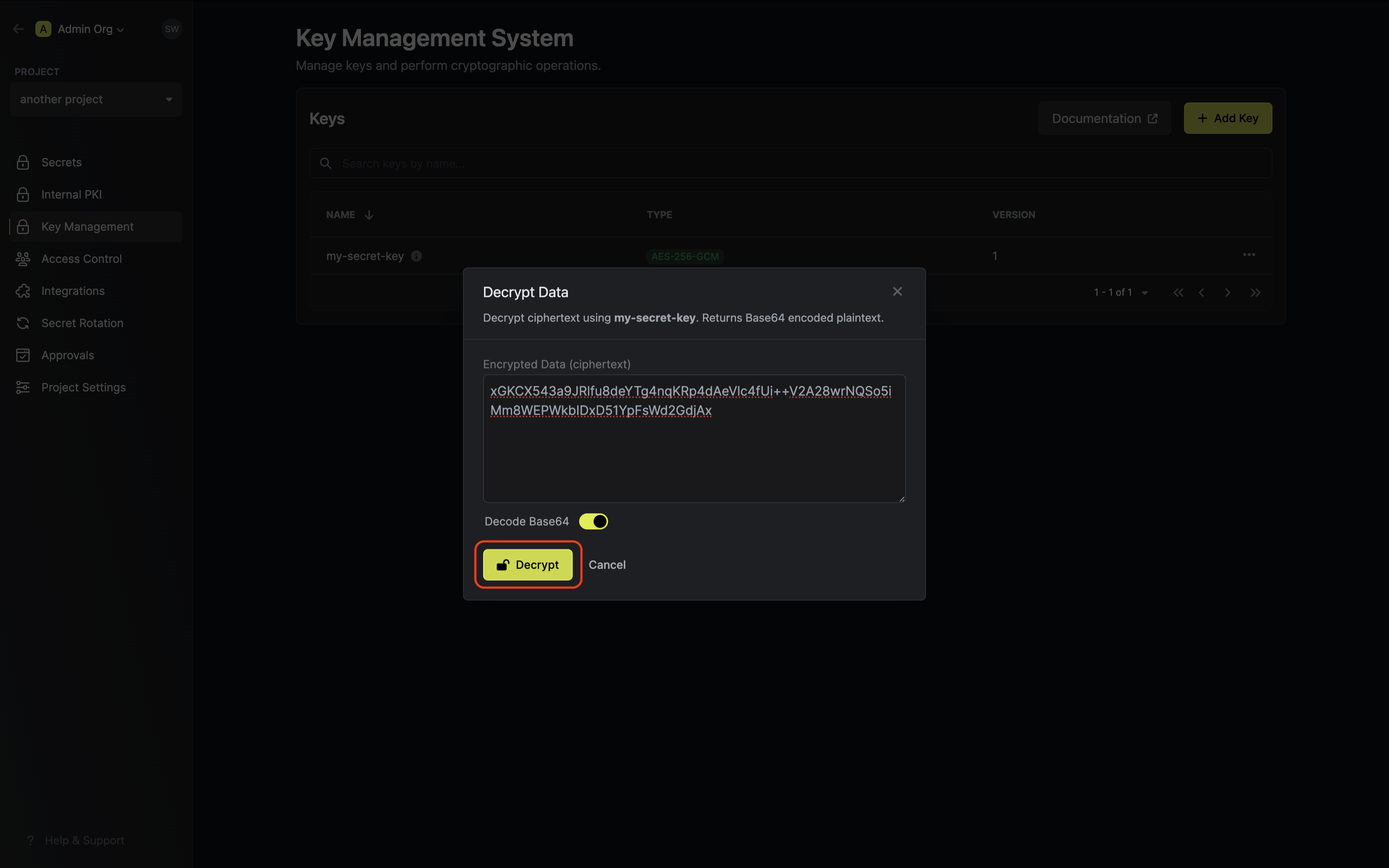
Your decrypted data will be displayed and can be copied for use.
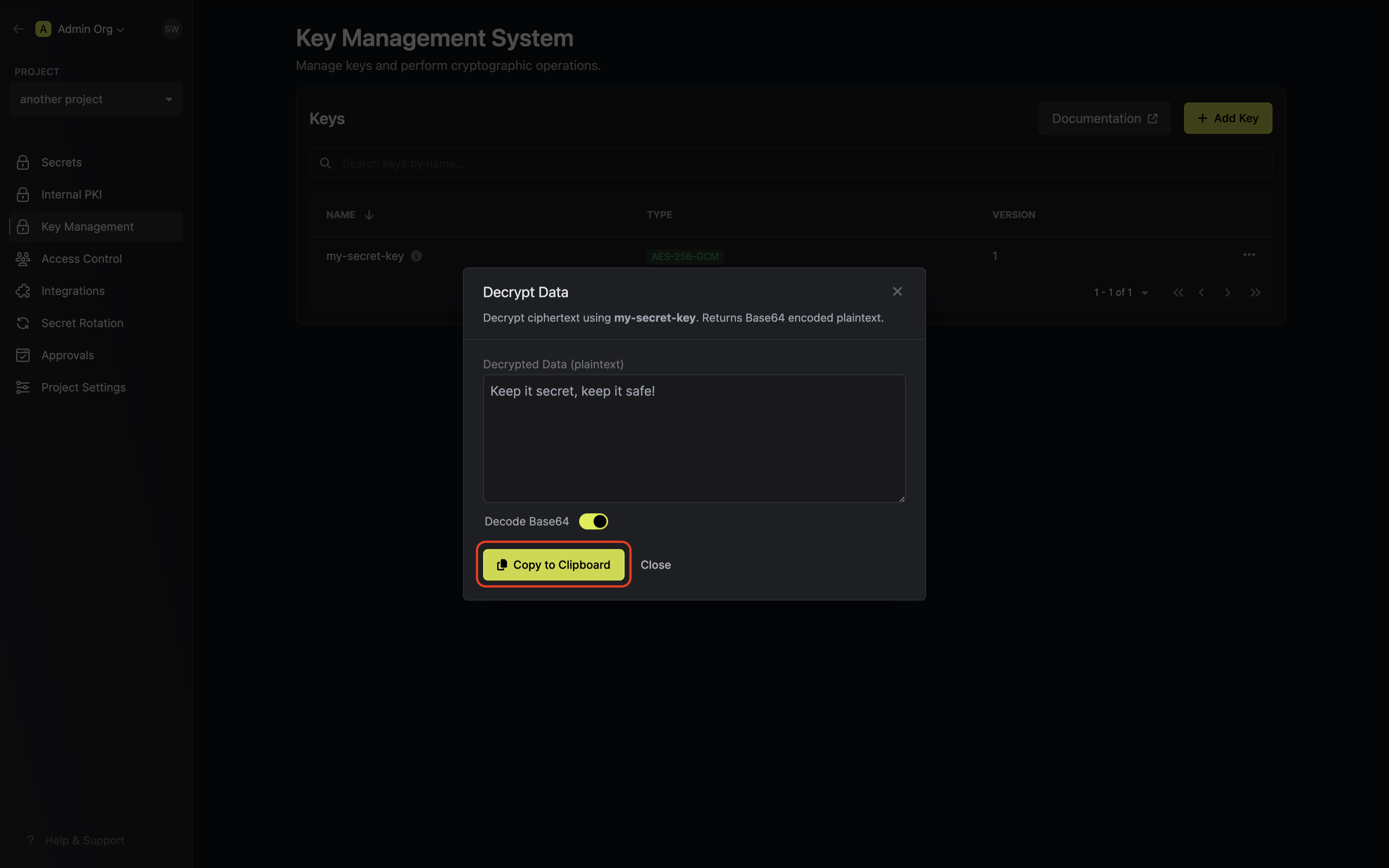
To decrypt data, make an API request to the [Decrypt
Data](/api-reference/endpoints/kms/keys/decrypt) API endpoint,
specifying the key to use.
### Sample request
```bash Request
curl --request POST \
--url https://app.infisical.com/api/v1/kms/keys//decrypt \
--header 'Content-Type: application/json' \
--data '{
"ciphertext": "HwFHwSFHwlMF6TOfp==" // base64 encoded ciphertext
}'
```
### Sample response
```bash Response
{
"plaintext": "lUFHM5Ggwo6TOfpuN1S==" // base64 encoded plaintext
}
```
## FAQ
No. Infisical's KMS only provides cryptographic services and does not store
any encrypted or decrypted data.
No. Infisical's KMS will never expose your keys, encrypted or decrypted, to
external sources.
Currently, Infisical only supports `AES-128-GCM` and `AES-256-GCM` for
encryption operations. We anticipate supporting more algorithms and
cryptographic operations in the coming months.
# General LDAP
Learn how to log in to Infisical with LDAP.
LDAP is a paid feature. If you're using Infisical Cloud, then it is available
under the **Enterprise Tier**. If you're self-hosting Infisical, then you
should contact [sales@infisical.com](mailto:sales@infisical.com) to purchase an enterprise license to use
it.
You can configure your organization in Infisical to have members authenticate with the platform via [LDAP](https://en.wikipedia.org/wiki/Lightweight_Directory_Access_Protocol)
Prerequisites:
* You must have an email address to use LDAP, regardless of whether or not you use that email address to sign in.
In Infisical, head to your Organization Settings > Security > LDAP and select **Manage**.
Next, input your LDAP server settings.
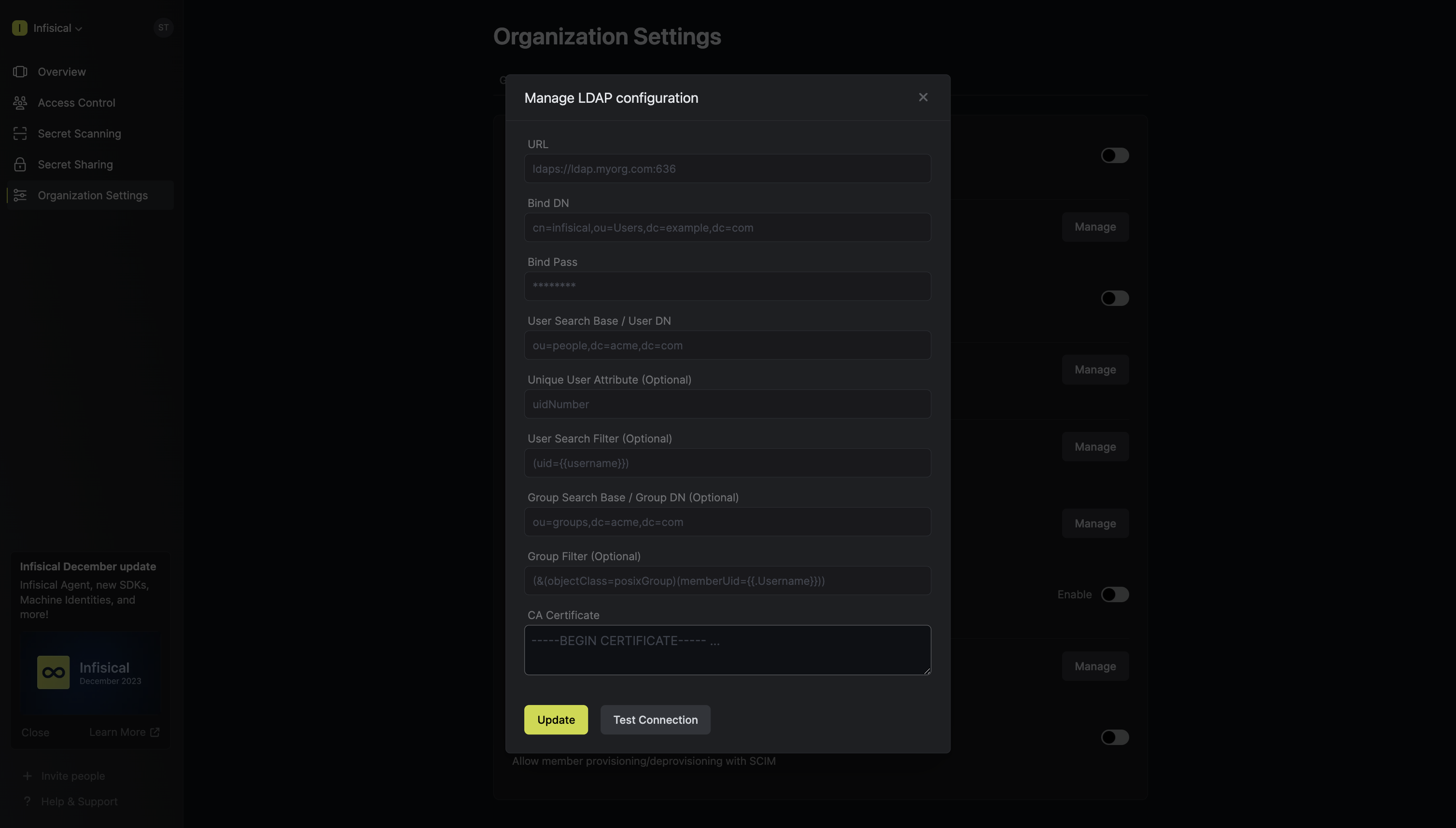
Here's some guidance for each field:
* URL: The LDAP server to connect to such as `ldap://ldap.your-org.com`, `ldaps://ldap.myorg.com:636` (for connection over SSL/TLS), etc.
* Bind DN: The distinguished name of object to bind when performing the user search such as `cn=infisical,ou=Users,dc=acme,dc=com`.
* Bind Pass: The password to use along with `Bind DN` when performing the user search.
* User Search Base / User DN: Base DN under which to perform user search such as `ou=Users,dc=acme,dc=com`.
* Unique User Attribute: The attribute to use as the unique identifier of LDAP users such as `sAMAccountName`, `cn`, `uid`, `objectGUID` ... If left blank, defaults to `uidNumber`
* User Search Filter (optional): Template used to construct the LDAP user search filter such as `(uid={{username}})`; use literal `{{username}}` to have the given username used in the search. The default is `(uid={{username}})` which is compatible with several common directory schemas.
* Group Search Base / Group DN (optional): LDAP search base to use for group membership search such as `ou=Groups,dc=acme,dc=com`.
* Group Filter (optional): Template used when constructing the group membership query such as `(&(objectClass=posixGroup)(memberUid={{.Username}}))`. The template can access the following context variables: \[`UserDN`, `UserName`]. The default is `(|(memberUid={{.Username}})(member={{.UserDN}})(uniqueMember={{.UserDN}}))` which is compatible with several common directory schemas.
* CA Certificate: The CA certificate to use when verifying the LDAP server certificate.
The **Group Search Base / Group DN** and **Group Filter** fields are both required if you wish to sync LDAP groups to Infisical.
Once you've filled out the LDAP configuration, you can test that part of the configuration is correct by pressing the **Test Connection** button.
Infisical will attempt to bind to the LDAP server using the provided **URL**, **Bind DN**, and **Bind Pass**. If the operation is successful, then Infisical will display a success message; if not, then Infisical will display an error message and provide a fuller error in the server logs.
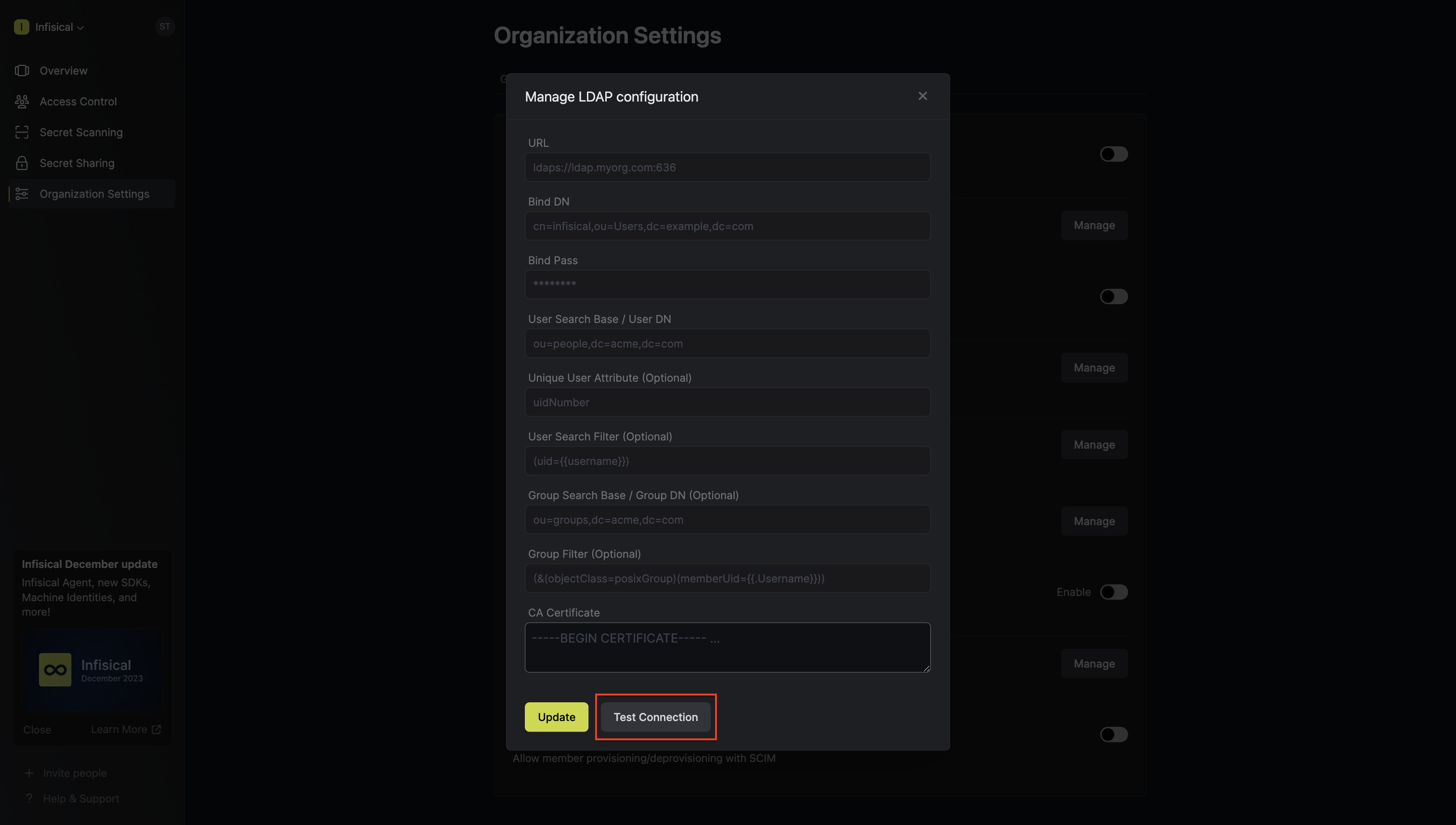
In order to sync LDAP groups to Infisical, head to the **LDAP Group Mappings** section to define mappings from LDAP groups to groups in Infisical.
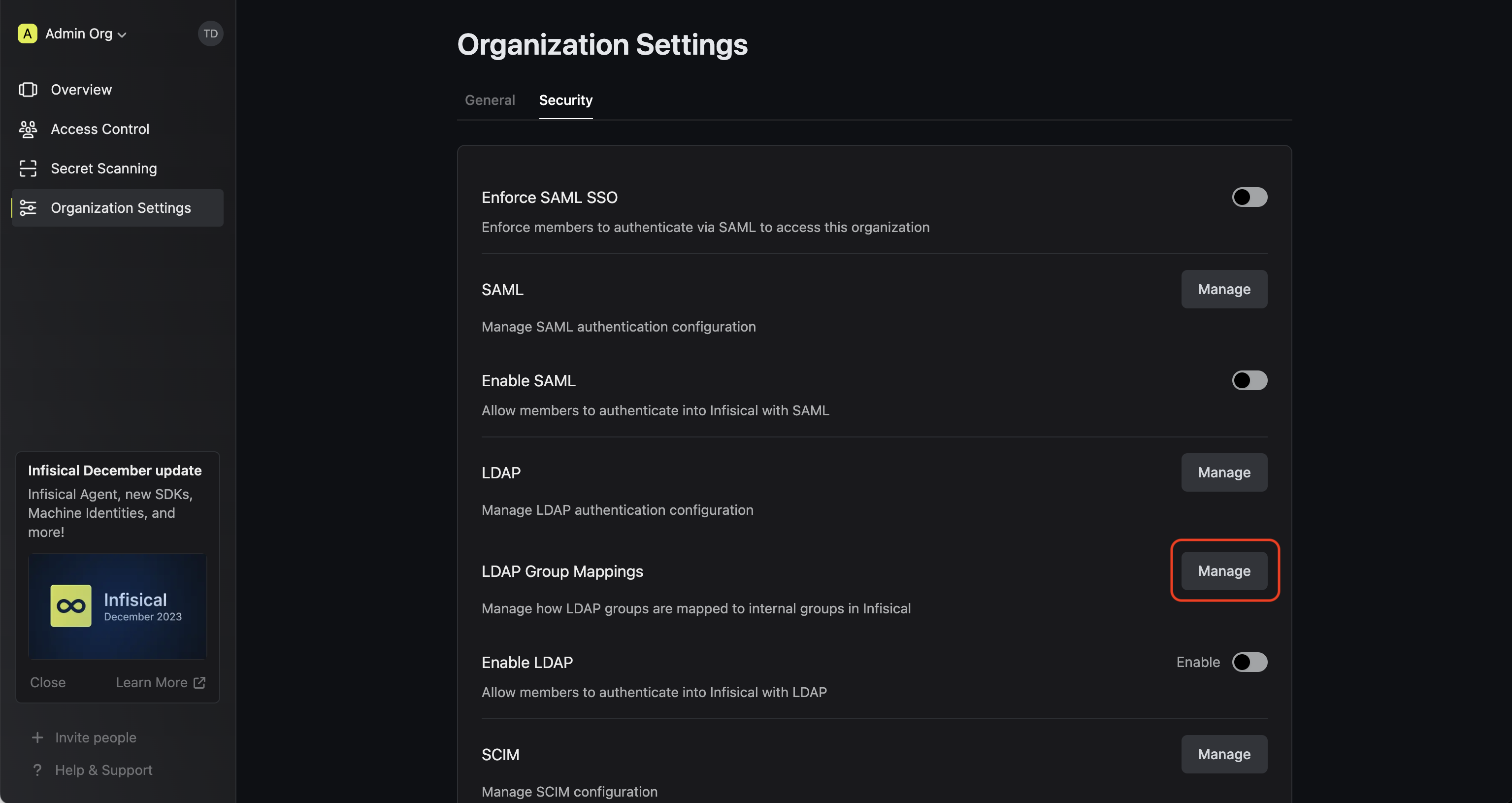
Group mappings ensure that users who log into Infisical via LDAP are added to or removed from the Infisical group(s) that corresponds to the LDAP group(s) they are a member of.
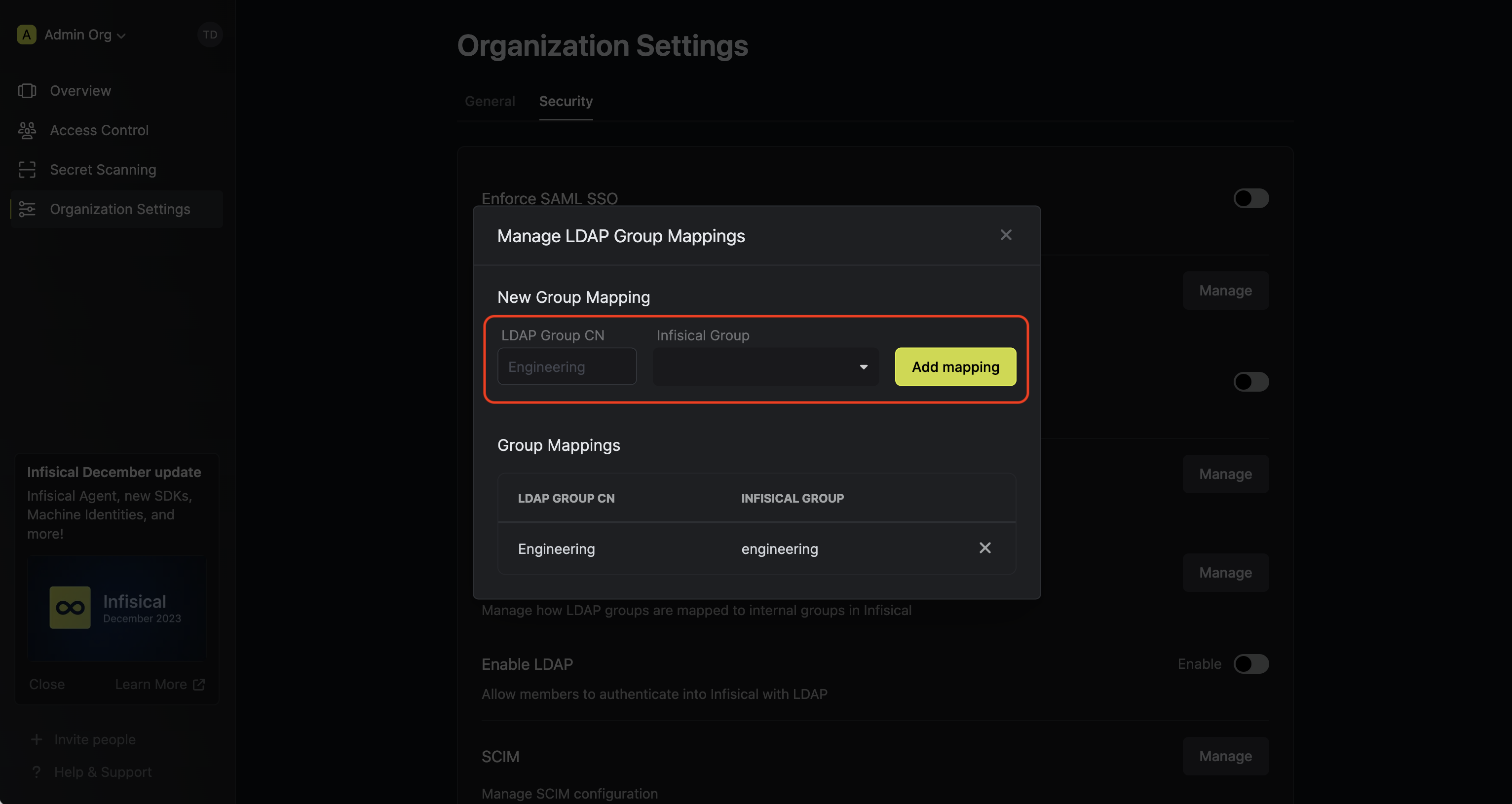
Each group mapping consists of two parts:
* LDAP Group CN: The common name of the LDAP group to map.
* Infisical Group: The Infisical group to map the LDAP group to.
For example, suppose you want to automatically add a user who is part of the LDAP group with CN `Engineers` to the Infisical group `Engineers` when the user sets up their account with Infisical.
In this case, you would specify a mapping from the LDAP group with CN `Engineers` to the Infisical group `Engineers`.
Now when the user logs into Infisical via LDAP, Infisical will check the LDAP groups that the user is a part of whilst referencing the group mappings you created earlier. Since the user is a member of the LDAP group with CN `Engineers`, they will be added to the Infisical group `Engineers`.
In the future, if the user is no longer part of the LDAP group with CN `Engineers`, they will be removed from the Infisical group `Engineers` upon their next login.
Prior to defining any group mappings, ensure that you've created the Infisical groups that you want to map the LDAP groups to.
You can read more about creating (user) groups in Infisical [here](/documentation/platform/groups).
Enabling LDAP allows members in your organization to log into Infisical via LDAP.
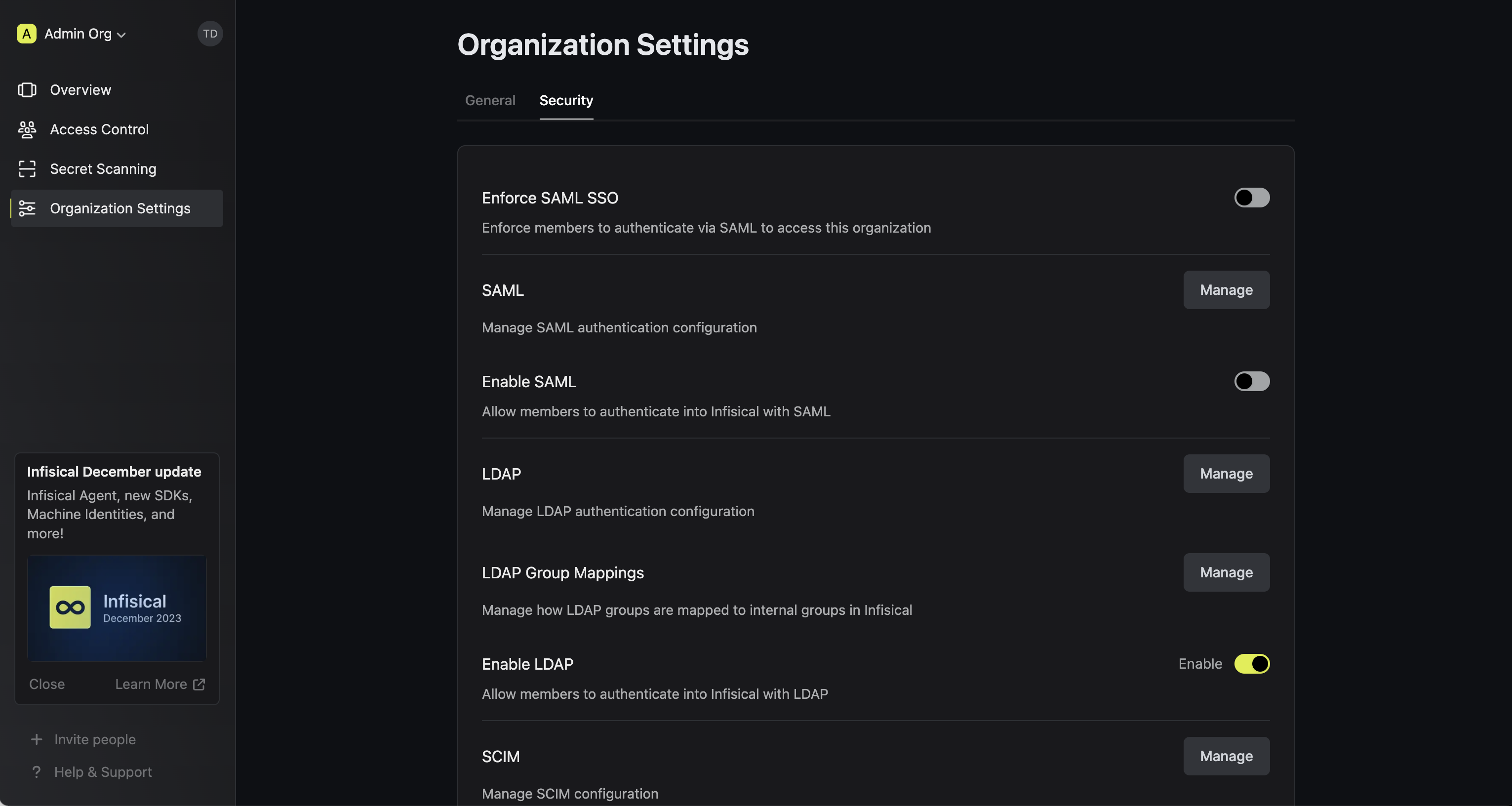
# JumpCloud LDAP
Learn how to configure JumpCloud LDAP for authenticating into Infisical.
LDAP is a paid feature. If you're using Infisical Cloud, then it is available
under the **Enterprise Tier**. If you're self-hosting Infisical, then you
should contact [sales@infisical.com](mailto:sales@infisical.com) to purchase an enterprise license to use
it.
Prerequisites:
* You must have an email address to use LDAP, regardless of whether or not you use that email address to sign in.
In JumpCloud, head to USER MANAGEMENT > Users and create a new user via the **Manual user entry** option. This user
will be used as a privileged service account to facilitate Infisical's ability to bind/search the LDAP directory.
When creating the user, input their **First Name**, **Last Name**, **Username** (required), **Company Email** (required), and **Description**.
Also, create a password for the user.
Next, under User Security Settings and Permissions > Permission Settings, check the box next to **Enable as LDAP Bind DN**.
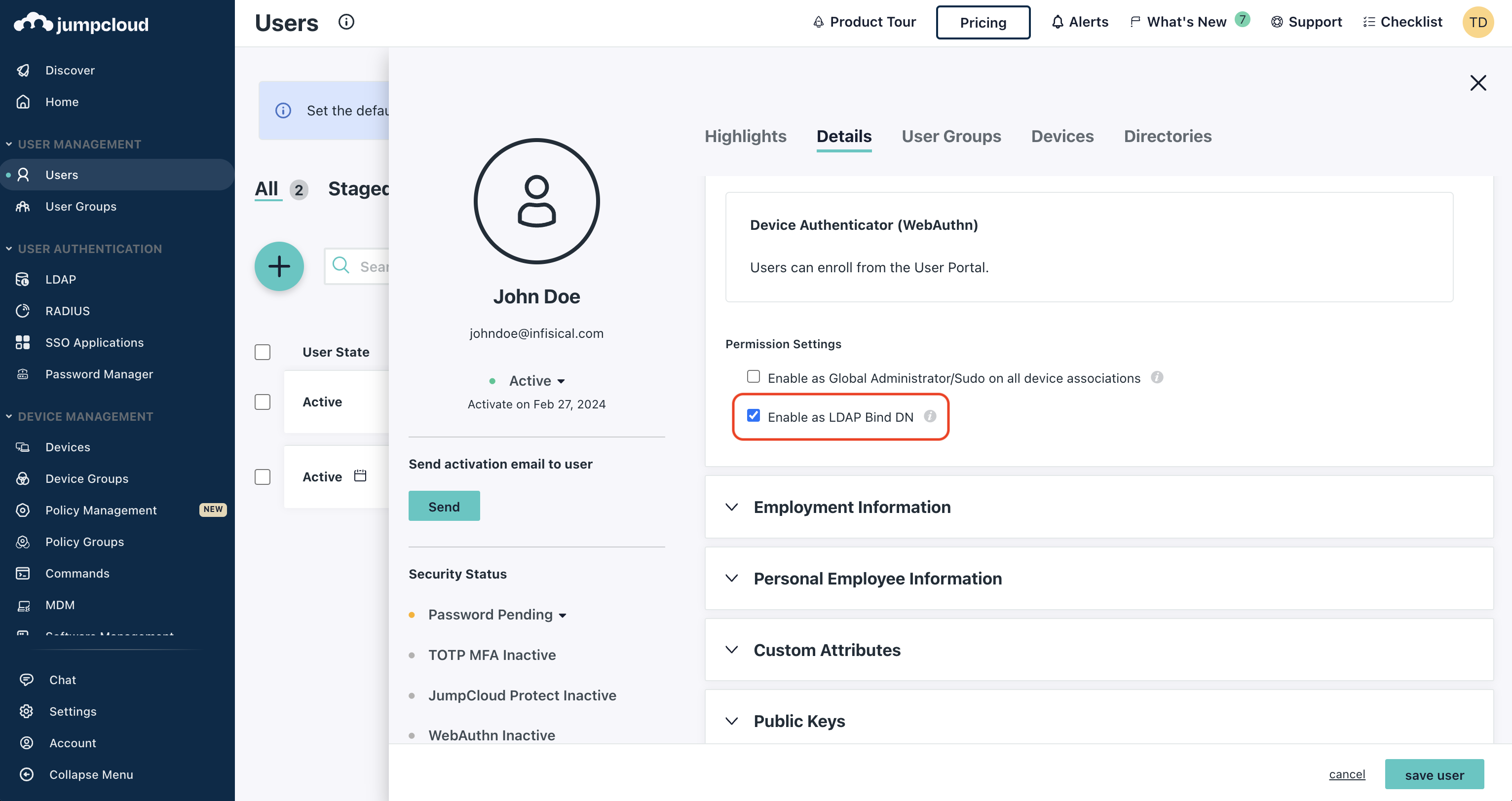
In Infisical, head to your Organization Settings > Security > LDAP and select **Manage**.
Next, input your JumpCloud LDAP server settings.
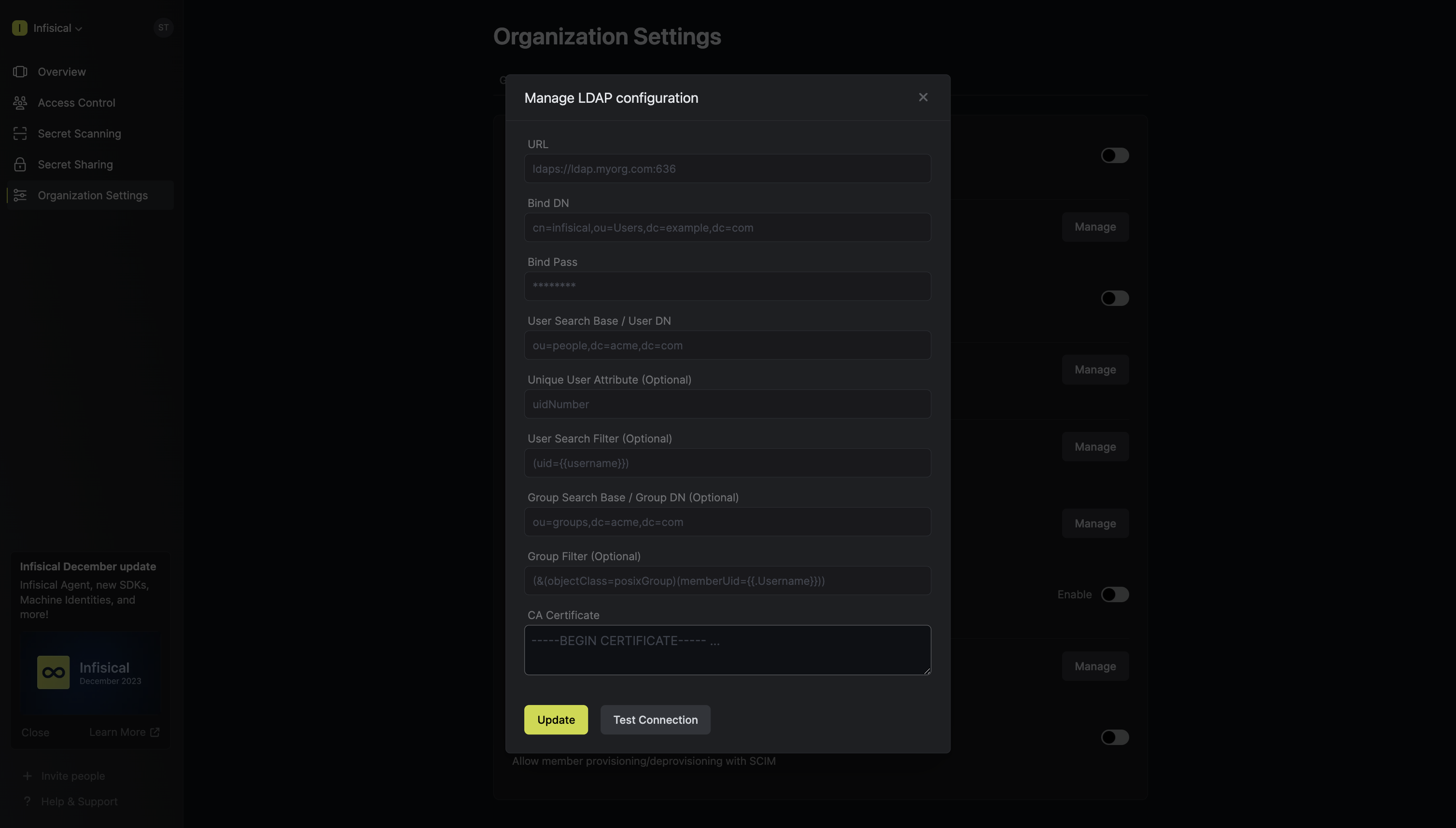
Here's some guidance for each field:
* URL: The LDAP server to connect to (`ldaps://ldap.jumpcloud.com:636`).
* Bind DN: The distinguished name of object to bind when performing the user search (`uid=,ou=Users,o=,dc=jumpcloud,dc=com`).
* Bind Pass: The password to use along with `Bind DN` when performing the user search.
* User Search Base / User DN: Base DN under which to perform user search (`ou=Users,o=,dc=jumpcloud,dc=com`).
* Unique User Attribute: The attribute to use as the unique identifier of LDAP users such as `sAMAccountName`, `cn`, `uid`, `objectGUID` ... If left blank, defaults to `uidNumber`
* User Search Filter (optional): Template used to construct the LDAP user search filter (`(uid={{username}})`).
* Group Search Base / Group DN (optional): LDAP search base to use for group membership search (`ou=Users,o=,dc=jumpcloud,dc=com`).
* Group Filter (optional): Template used when constructing the group membership query (`(&(objectClass=groupOfNames)(member=uid={{.Username}},ou=Users,o=,dc=jumpcloud,dc=com))`)
* CA Certificate: The CA certificate to use when verifying the LDAP server certificate (instructions to obtain the certificate for JumpCloud [here](https://jumpcloud.com/support/connect-to-ldap-with-tls-ssl)).
When filling out the **Bind DN** and **Bind Pass** fields, refer to the username and password of the user created in Step 1.
Also, for the **Bind DN** and **Search Base / User DN** fields, you'll want to use the organization ID that appears
in your LDAP instance **ORG DN**.
Once you've filled out the LDAP configuration, you can test that part of the configuration is correct by pressing the **Test Connection** button.
Infisical will attempt to bind to the LDAP server using the provided **URL**, **Bind DN**, and **Bind Pass**. If the operation is successful, then Infisical will display a success message; if not, then Infisical will display an error message and provide a fuller error in the server logs.
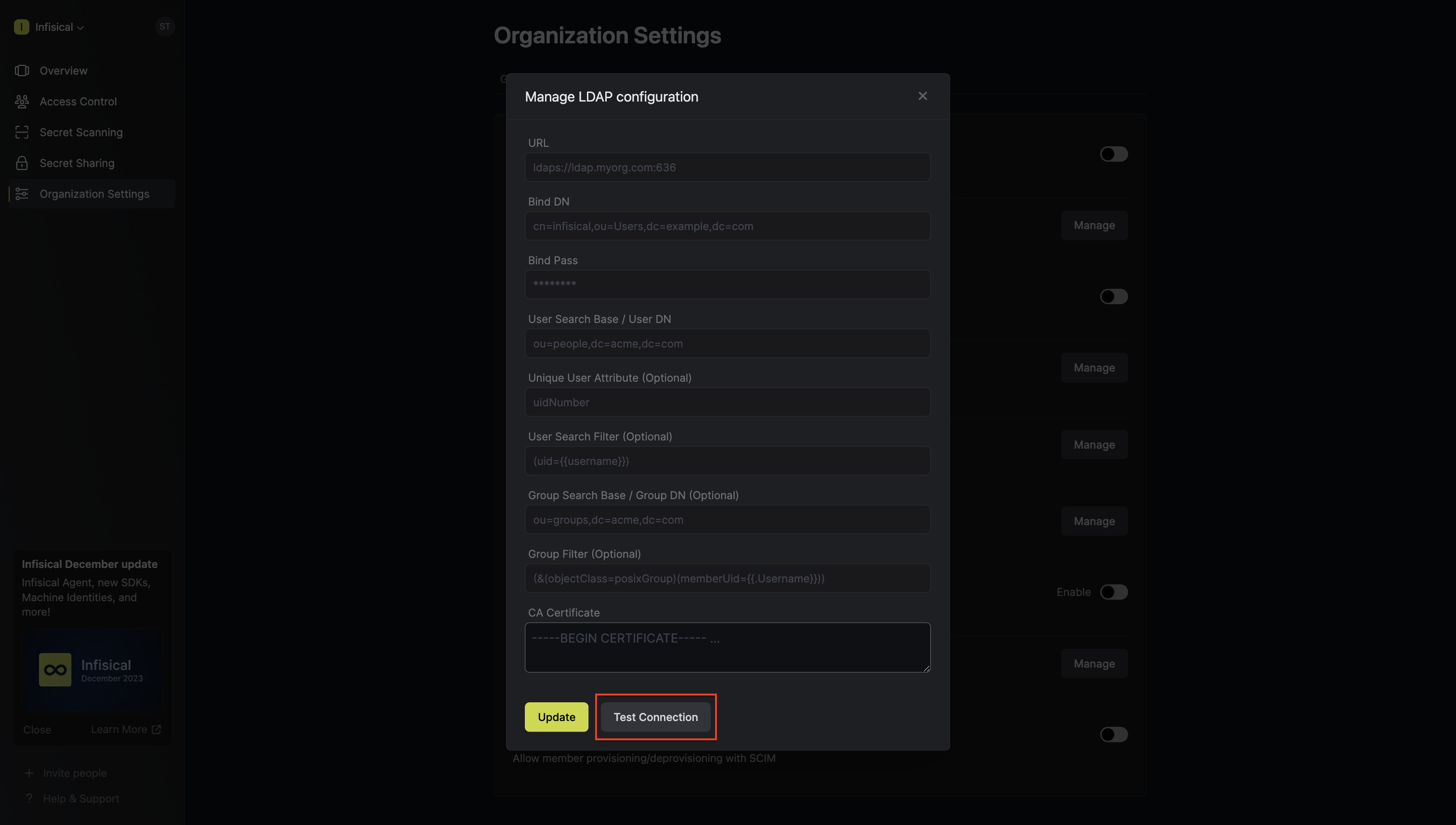
In order to sync LDAP groups to Infisical, head to the **LDAP Group Mappings** section to define mappings from LDAP groups to groups in Infisical.
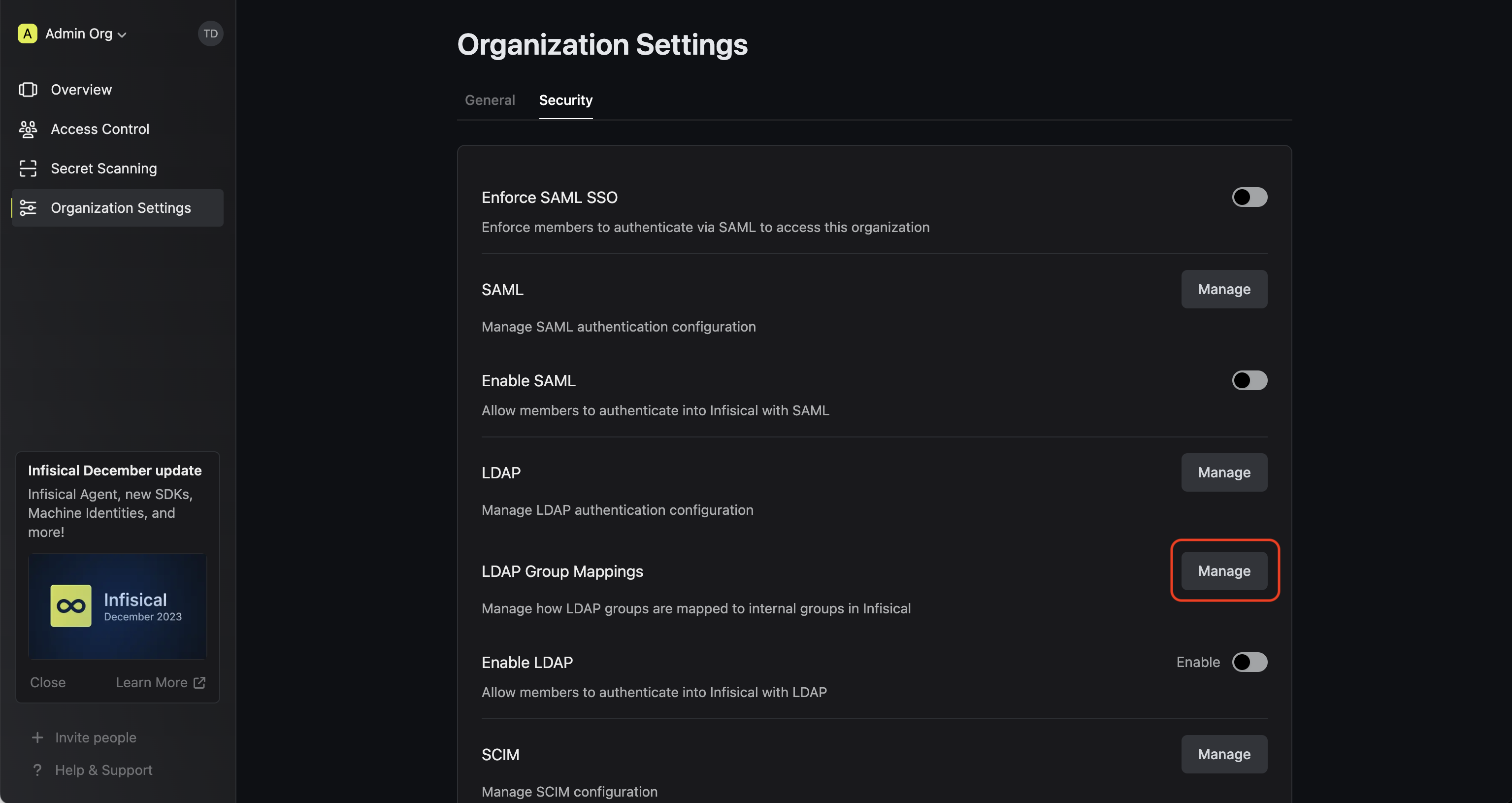
Group mappings ensure that users who log into Infisical via LDAP are added to or removed from the Infisical group(s) that corresponds to the LDAP group(s) they are a member of.
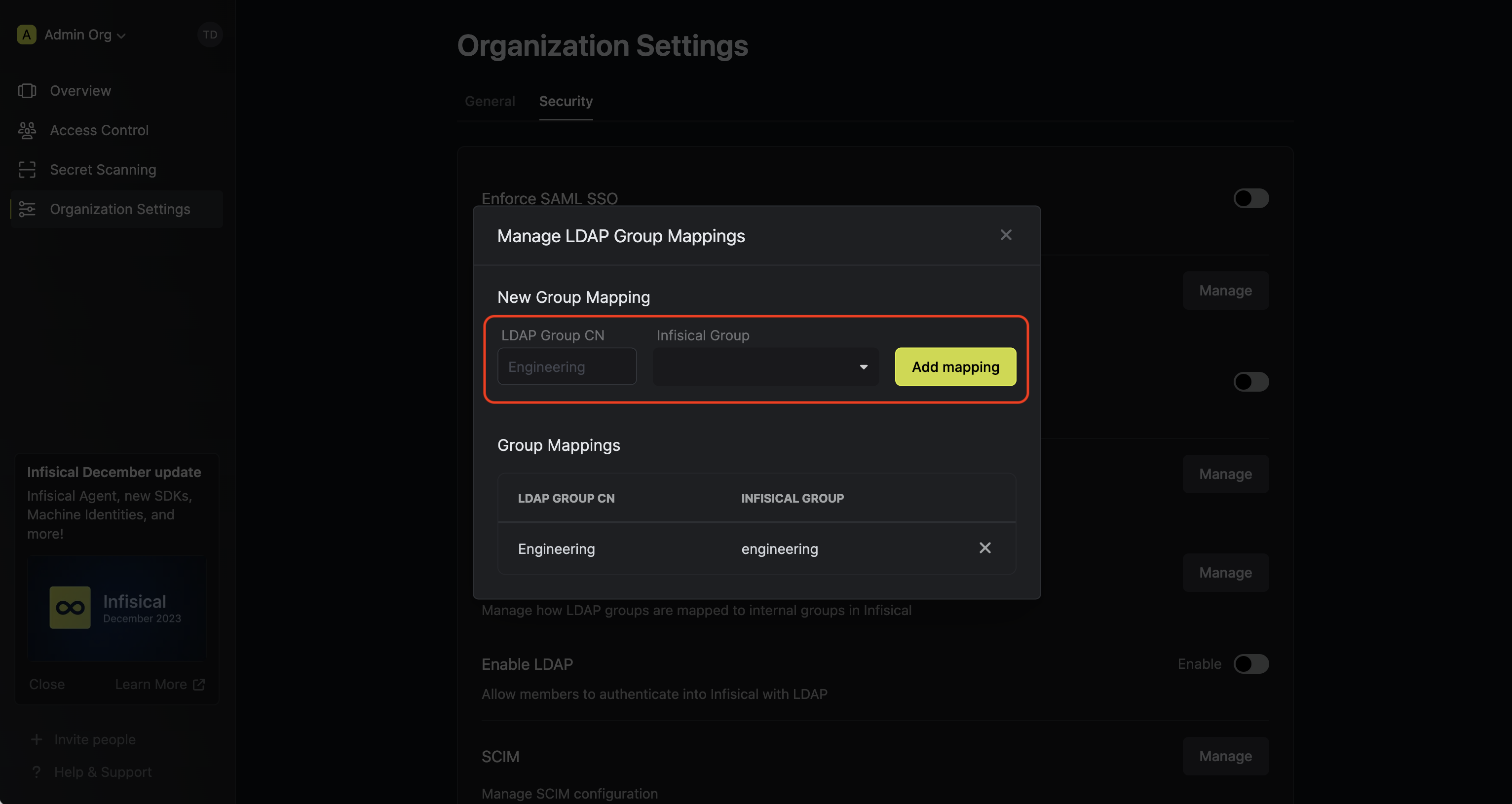
Each group mapping consists of two parts:
* LDAP Group CN: The common name of the LDAP group to map.
* Infisical Group: The Infisical group to map the LDAP group to.
For example, suppose you want to automatically add a user who is part of the LDAP group with CN `Engineers` to the Infisical group `Engineers` when the user sets up their account with Infisical.
In this case, you would specify a mapping from the LDAP group with CN `Engineers` to the Infisical group `Engineers`.
Now when the user logs into Infisical via LDAP, Infisical will check the LDAP groups that the user is a part of whilst referencing the group mappings you created earlier. Since the user is a member of the LDAP group with CN `Engineers`, they will be added to the Infisical group `Engineers`.
In the future, if the user is no longer part of the LDAP group with CN `Engineers`, they will be removed from the Infisical group `Engineers` upon their next login.
Prior to defining any group mappings, ensure that you've created the Infisical groups that you want to map the LDAP groups to.
You can read more about creating (user) groups in Infisical [here](/documentation/platform/groups).
Enabling LDAP allows members in your organization to log into Infisical via LDAP.
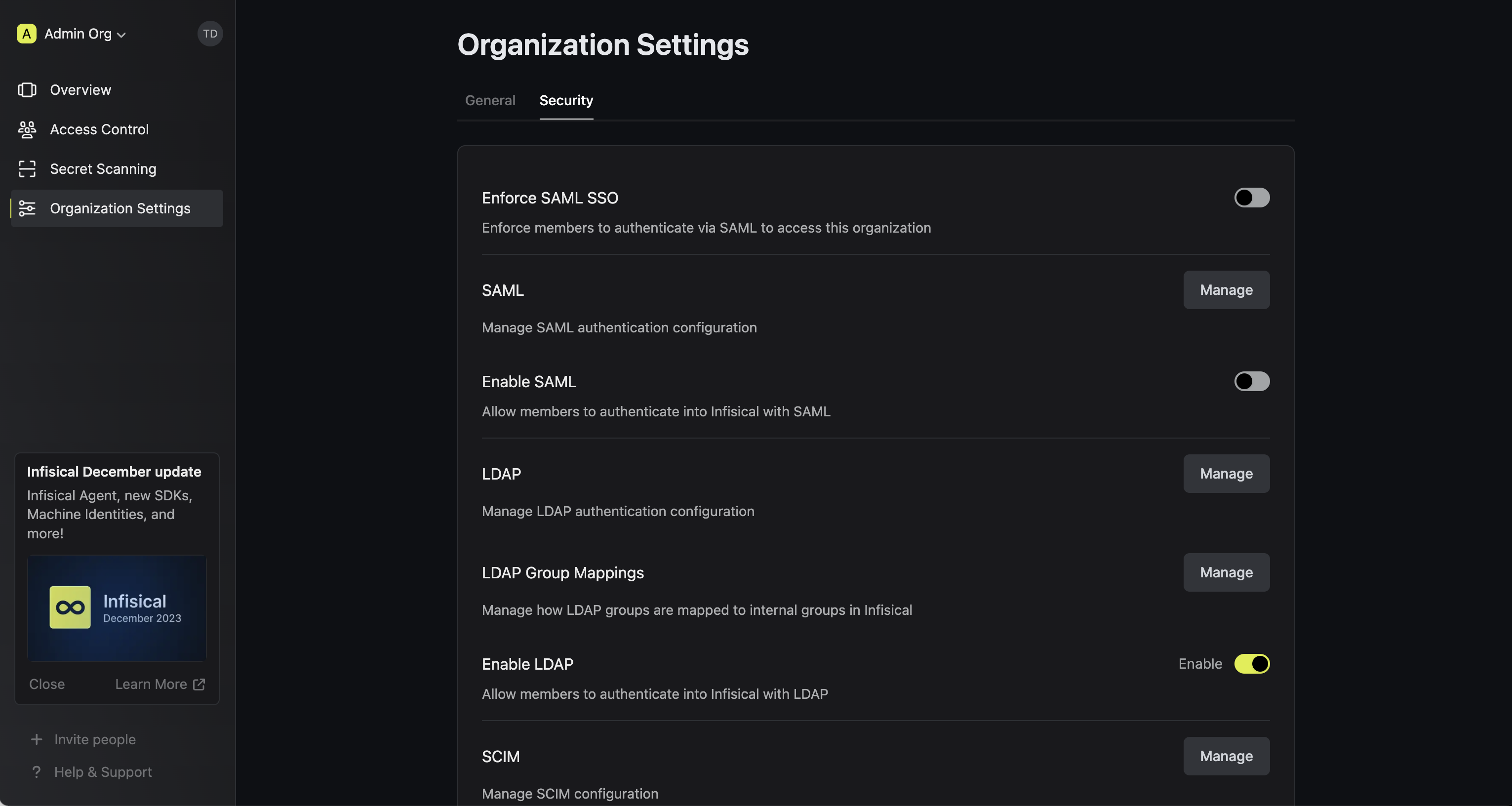
Resources:
* [JumpCloud Cloud LDAP Guide](https://jumpcloud.com/support/use-cloud-ldap)
# LDAP Overview
Learn how to authenticate into Infisical with LDAP.
LDAP is a paid feature.
If you're using Infisical Cloud, then it is available under the **Enterprise Tier**. If you're self-hosting Infisical,
then you should contact [sales@infisical.com](mailto:sales@infisical.com) to purchase an enterprise license to use it.
You can configure your organization in Infisical to have members authenticate with the platform via [LDAP](https://en.wikipedia.org/wiki/Lightweight_Directory_Access_Protocol).
LDAP providers:
* Active Directory
* [JumpCloud LDAP](/documentation/platform/ldap/jumpcloud)
* AWS Directory Service
* Foxpass
Read the general instructions for configuring LDAP [here](/documentation/platform/ldap/general).
If the documentation for your required identity provider is not shown in the list above, please reach out to [team@infisical.com](mailto:team@infisical.com) for assistance.
## FAQ
By default, Infisical Cloud is configured to not trust emails from external
identity providers to prevent any malicious account takeover attempts via
email spoofing. Accordingly, Infisical creates a new user for anyone provisioned
through an external identity provider and requires an additional email
verification step upon their first login.
If you're running a self-hosted instance of Infisical and would like it to trust emails from external identity providers,
you can configure this behavior in the Server Admin Console.
# Multi-factor Authentication
Learn how to secure your Infisical account with MFA.
MFA requires users to provide multiple forms of identification to access their account.
## Email 2FA
If 2-factor authentication is enabled in the Personal settings page, email will be used for MFA by default.
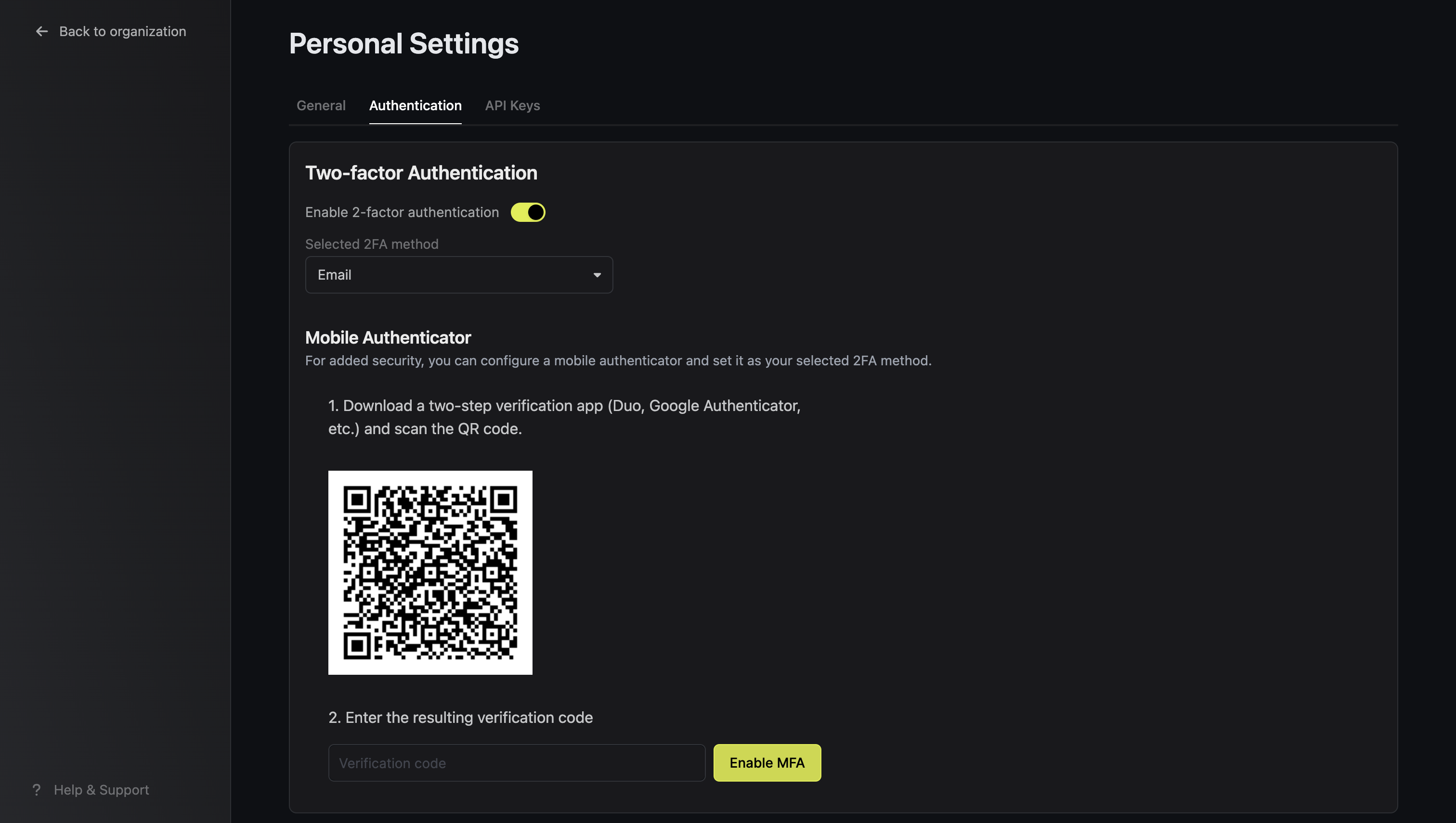
## Mobile Authenticator 2FA
You can use any mobile authenticator app (Authy, Google Authenticator, Duo, etc.) to secure your account. After registration with an authenticator, select **Mobile Authenticator** as your 2FA method.
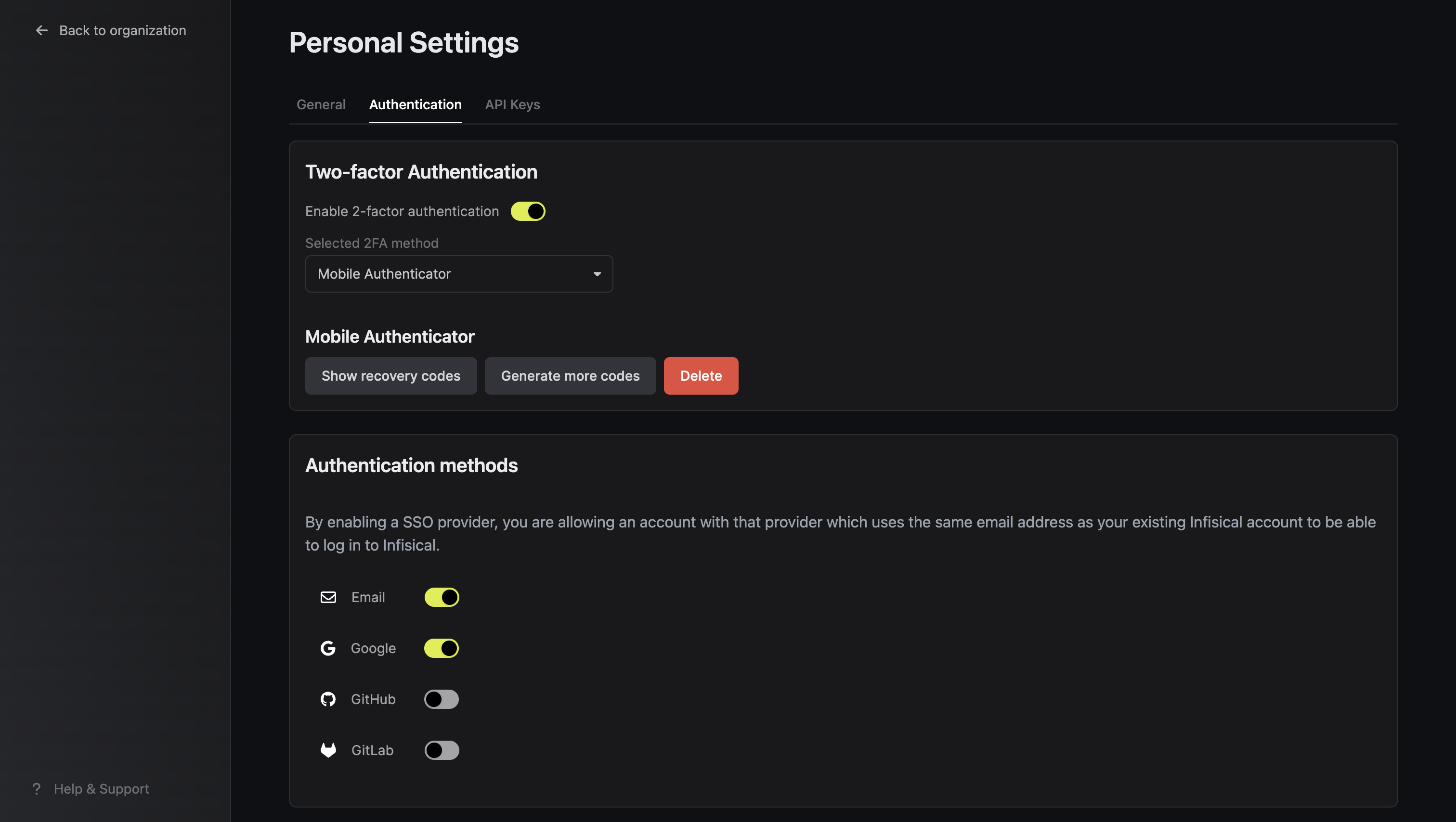
## Entra ID / Azure AD MFA
Before proceeding make sure you've enabled [SAML SSO for Entra ID / Azure AD](./sso/azure).
We also encourage you to have your team download and setup the
[Microsoft Authenticator App](https://www.microsoft.com/en-us/security/mobile-authenticator-app) prior to enabling MFA.
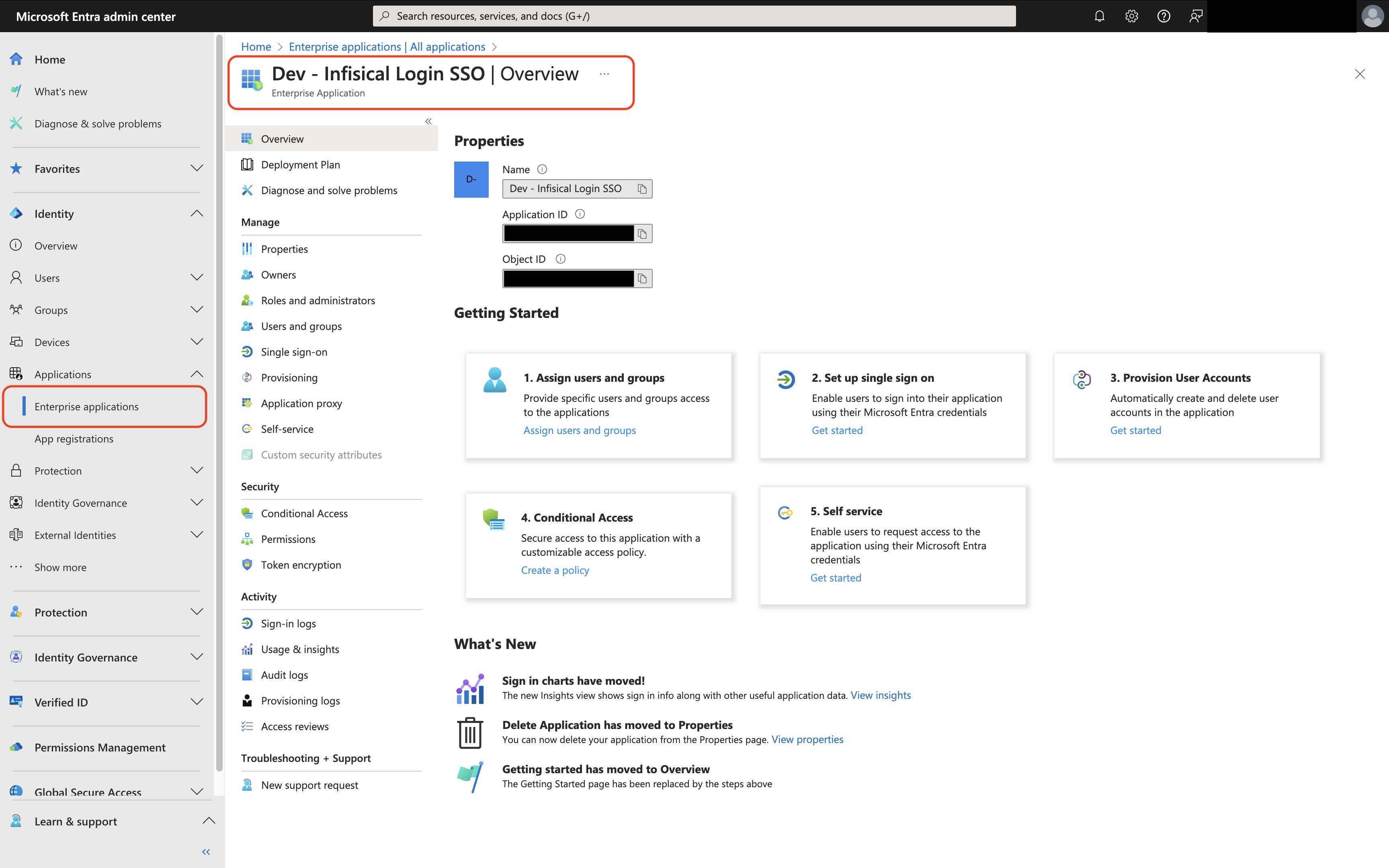
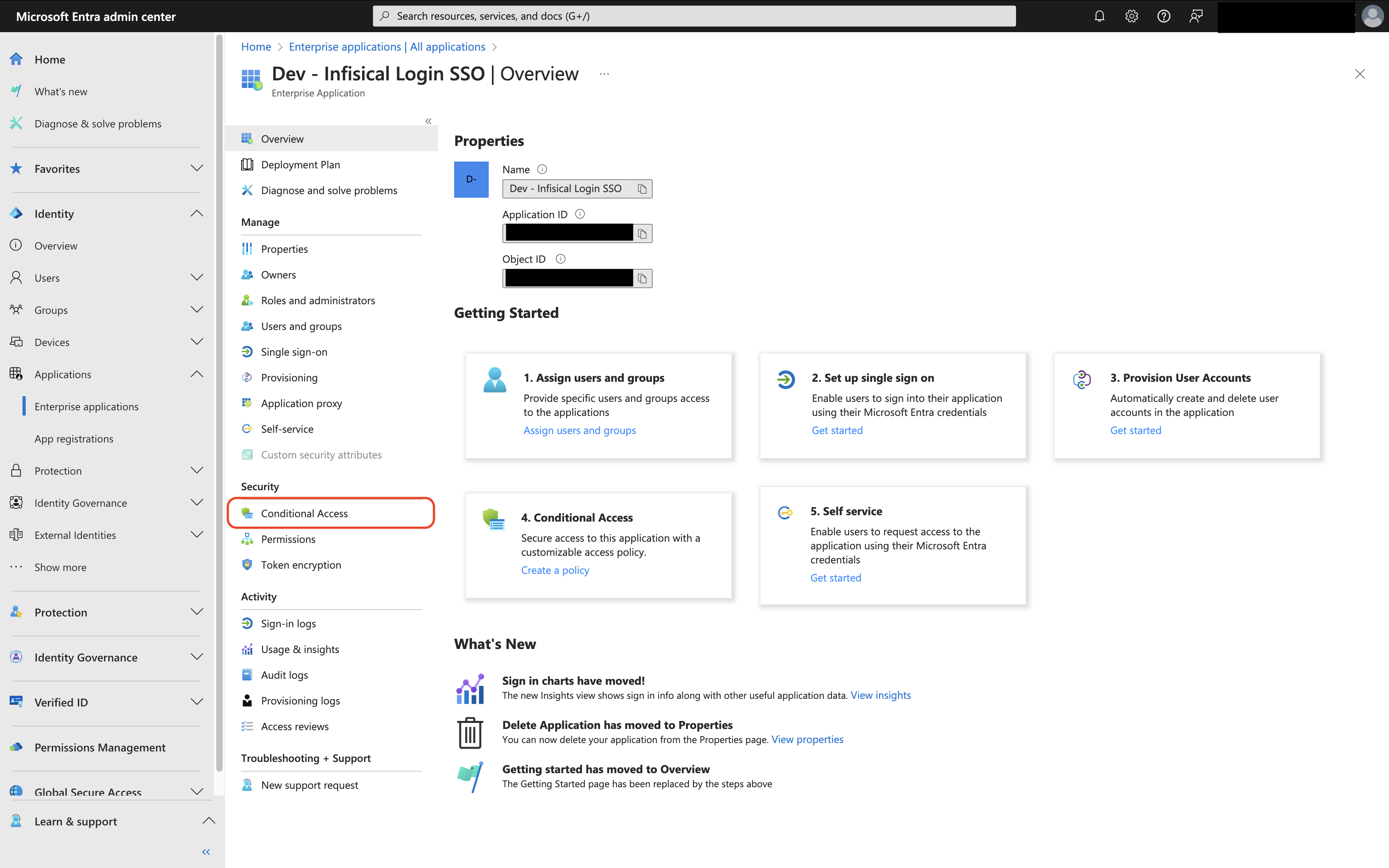
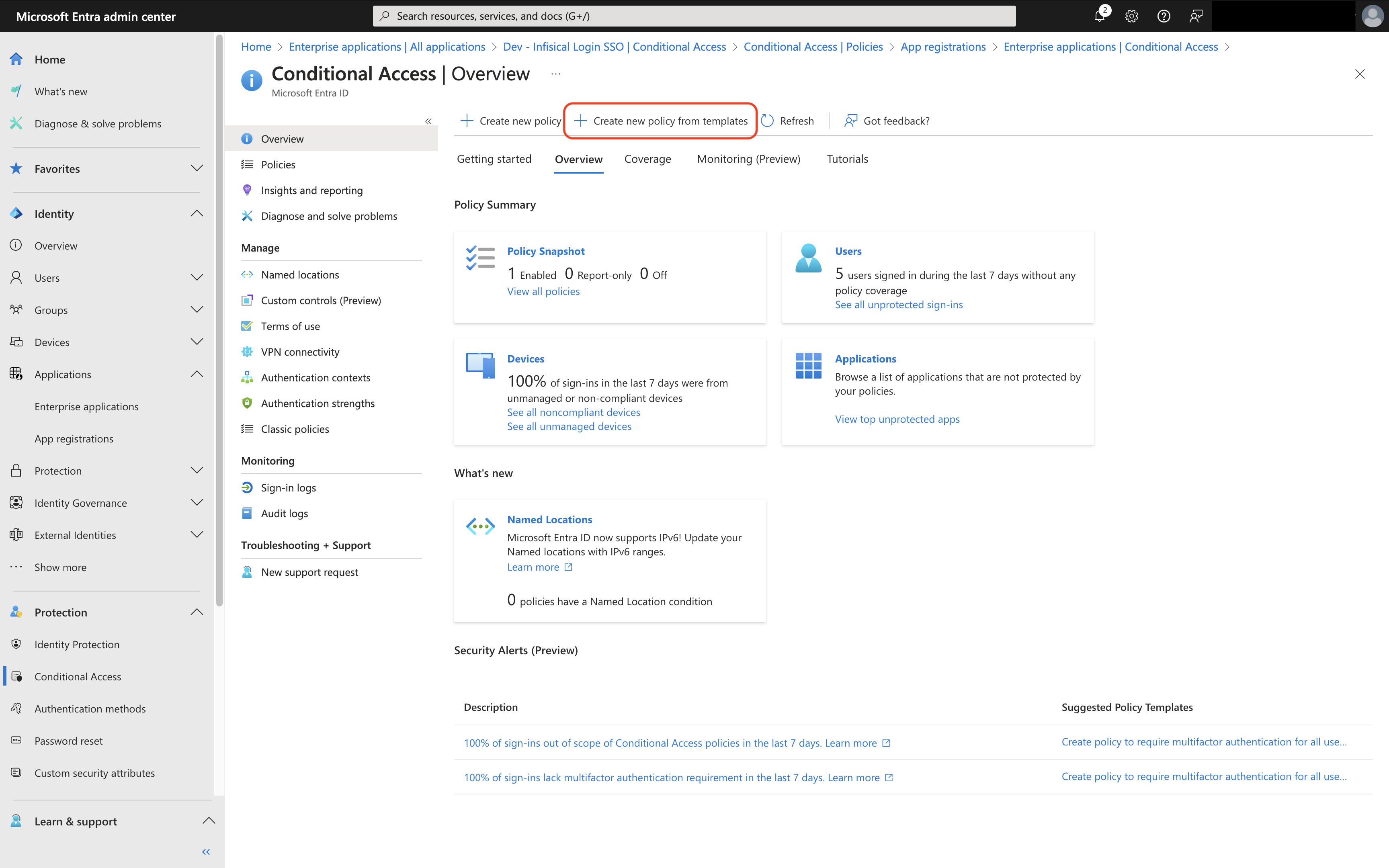
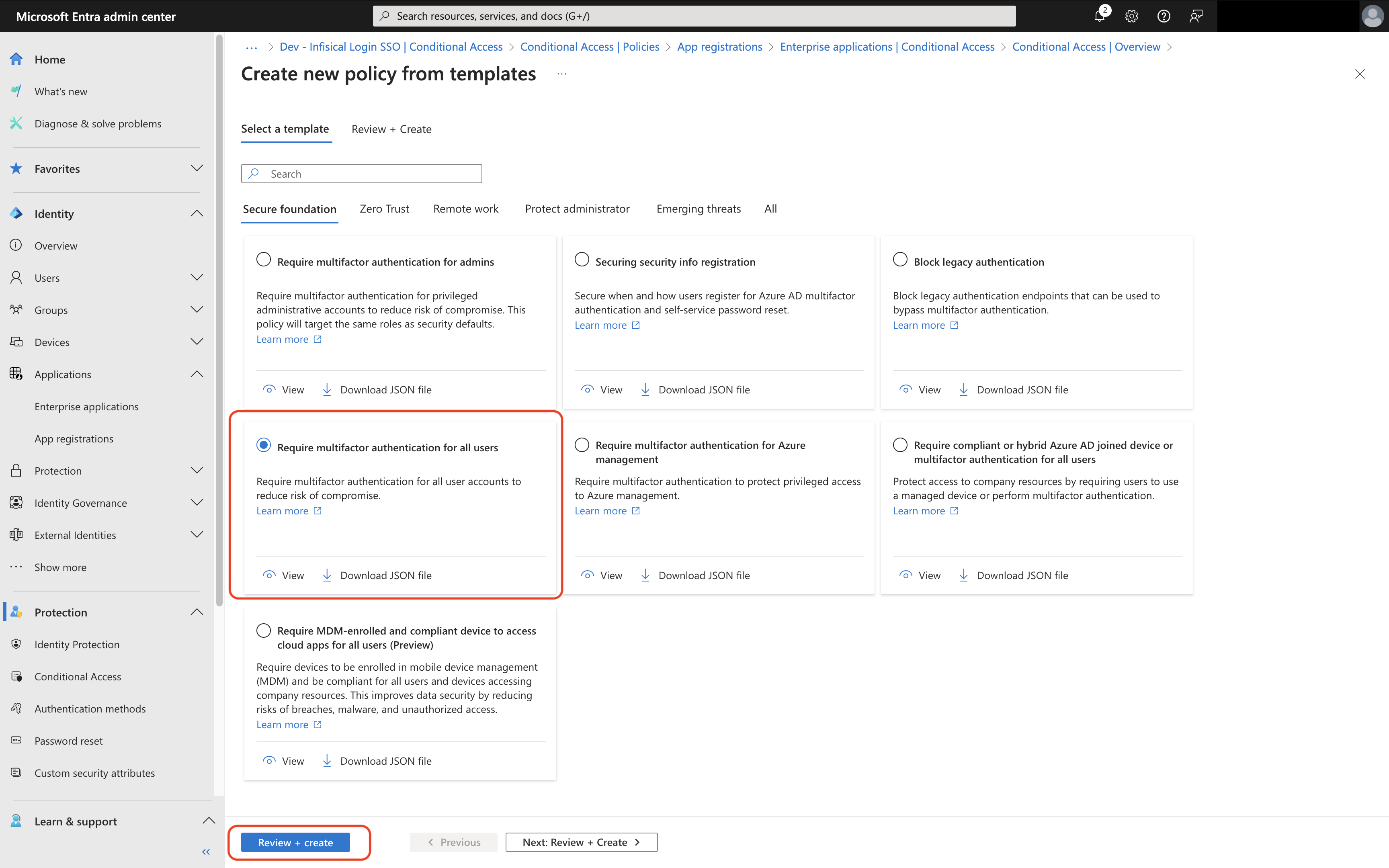
By default all users except the configuring admin will be setup to require
MFA. Microsoft encourages keeping at least one admin excluded from MFA to
prevent accidental lockout.
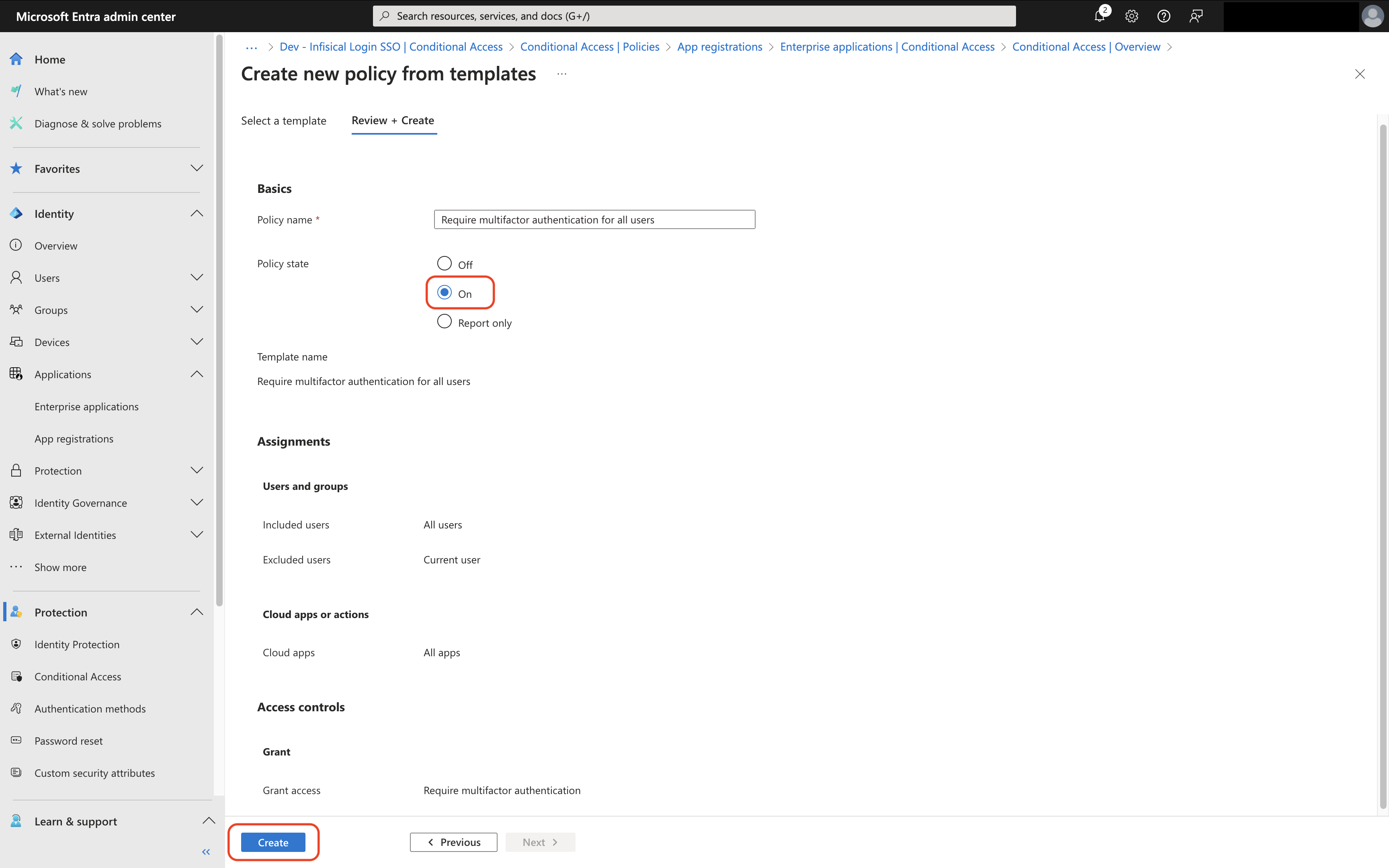
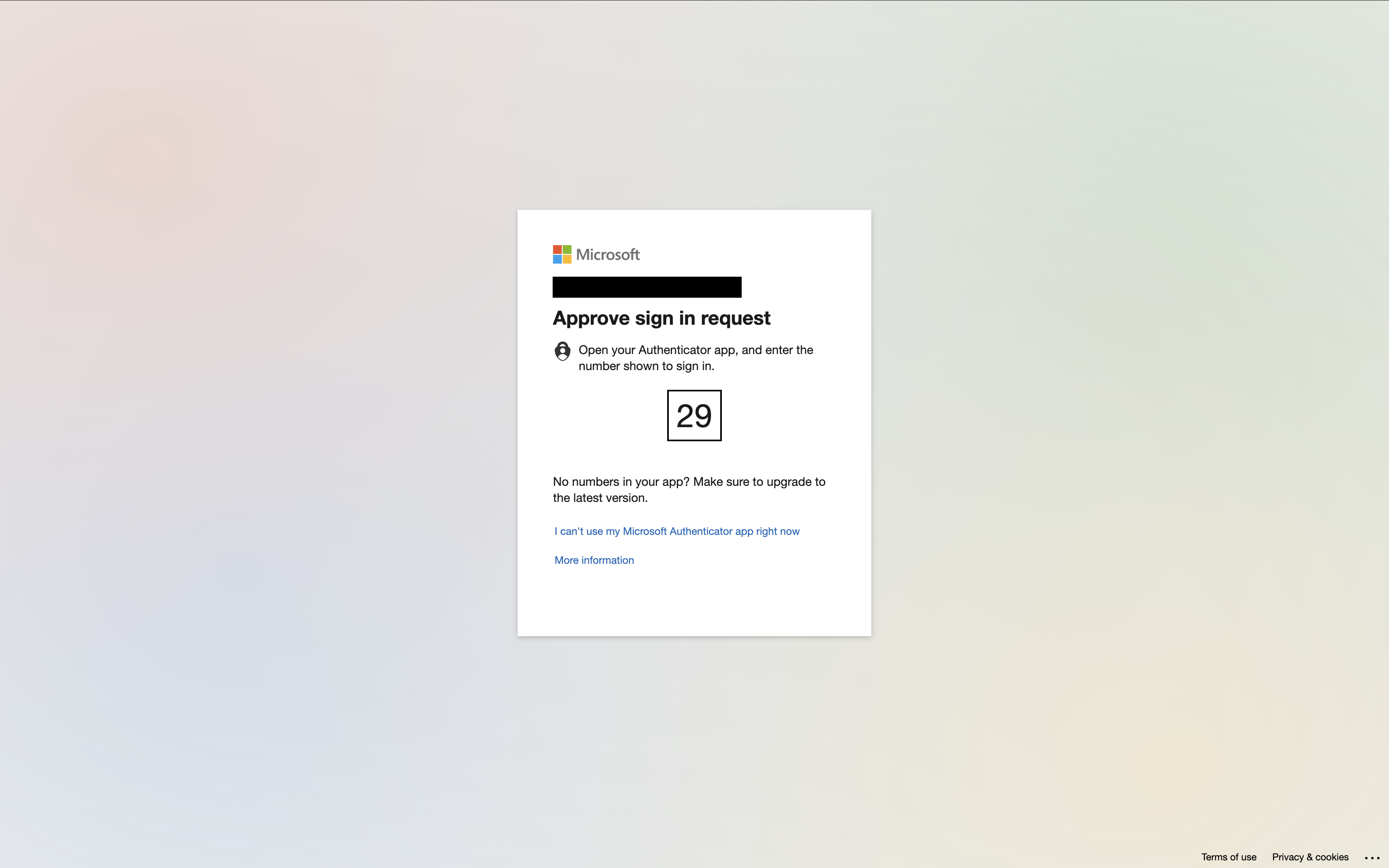
If users have not setup MFA for Entra / Azure they will be prompted to do
so at this time.
# Organizations
Learn more and understand the concept of Infisical organizations.
An Infisical organization is a set of [projects](./project) that use the same billing. Organizations allow one or more users to control billing and project permissions for all of the projects belonging to the organization. Each project belongs to an organization.
## Projects
The **Projects** page is where you can view the projects that you have access to within your organization
as well as create a new project.
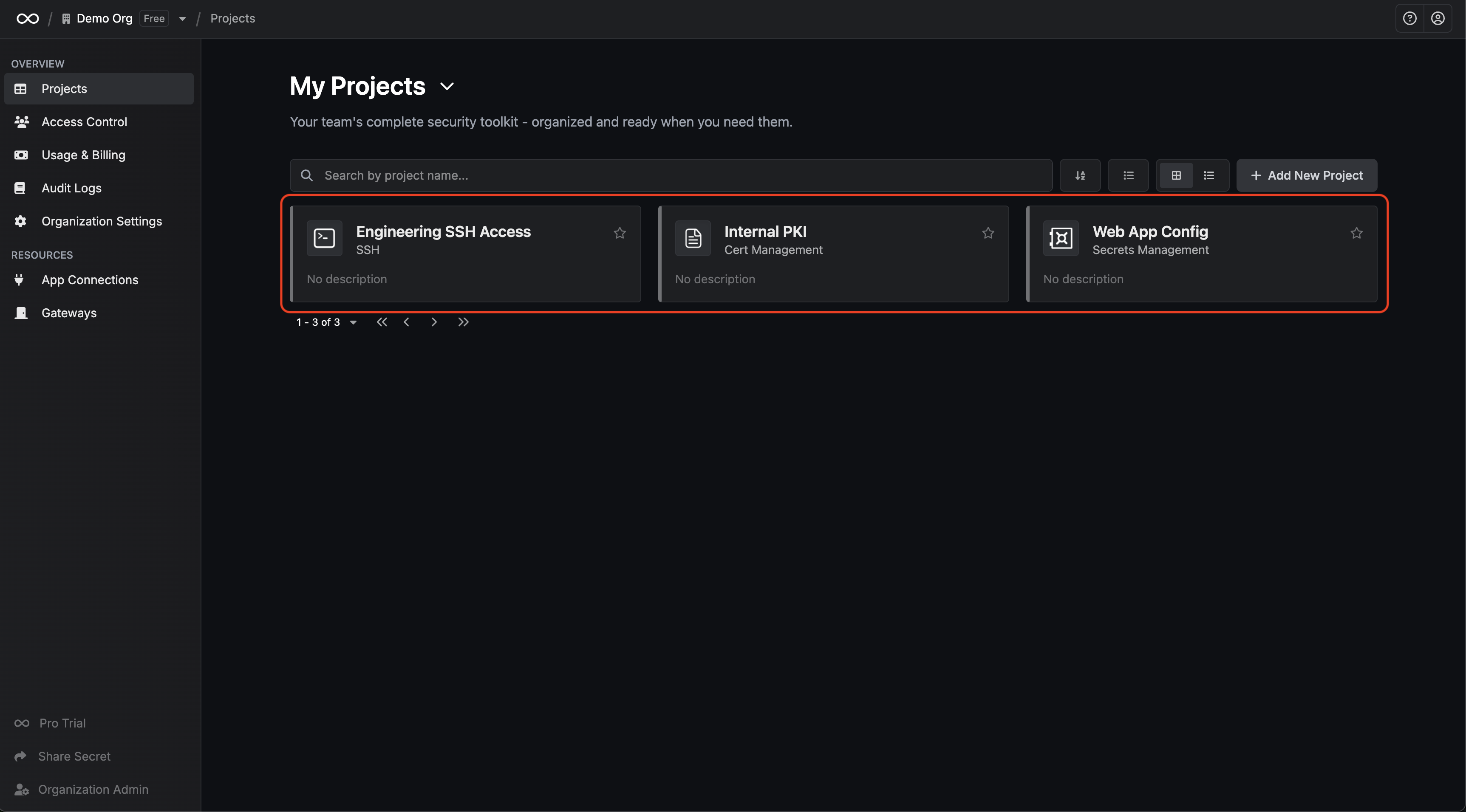
## Settings
The **Settings** page lets you manage information about your organization including:
* **Name**: The name of your organization.
* **Slug**: The slug of your organization.
* **Default Organization Member Role**: The role assigned to users when joining your organization unless otherwise specified.
* **Incident Contacts**: Emails that should be alerted if anything abnormal is detected within the organization.

* Security and Authentication: A set of setting to enforce or manage [SAML](/documentation/platform/sso/overview), [OIDC](/documentation/platform/sso/overview), [SCIM](/documentation/platform/scim/overview), [LDAP](/documentation/platform/ldap/overview), and other authentication configurations.

## Access Control
The **Access Control** page is where you can manage identities (both people and machines) that are part of your organization.
You can add or remove additional members as well as modify their permissions.

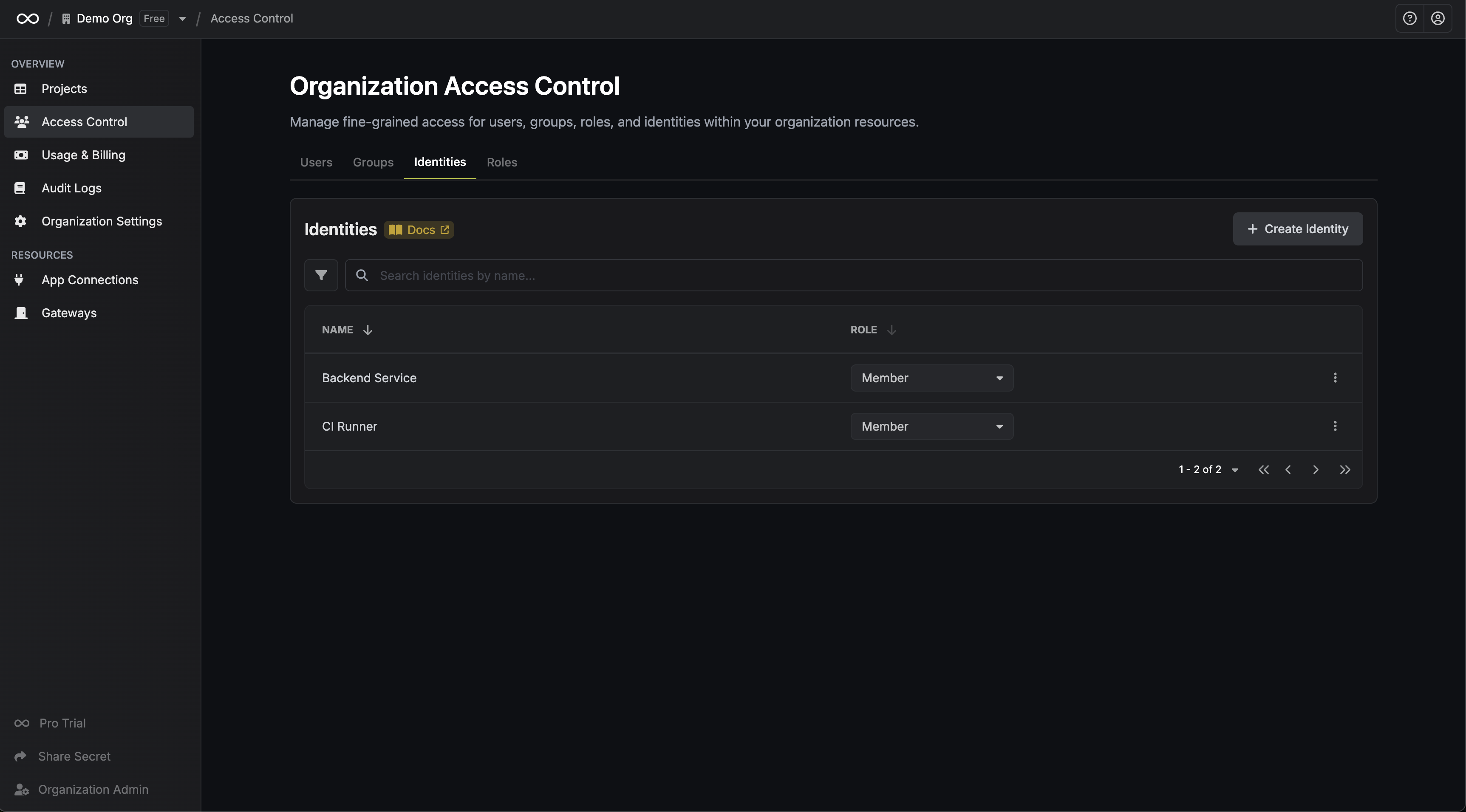
In the **Organization Roles** tab, you can edit current or create new custom roles for members within the organization.
Note that Role-Based Access Management (RBAC) is partly a paid feature.
Infisical provides immutable roles like `admin`, `member`, etc.
at the organization and project level for free.
If you're using Infisical Cloud, the ability to create custom roles is available under the **Pro Tier**.
If you're self-hosting Infisical, then you should contact [sales@infisical.com](mailto:sales@infisical.com) to purchase an enterprise license to use it.

As you can see next, Infisical supports granular permissions that you can tailor to each role.
If you need certain members to only be able to access billing details, for example, then you can
assign them that permission only.

## Usage & Billing
The **Usage & Billing** page applies only to [Infisical Cloud](https://app.infisical.com) and is where you can
manage your plan and billing information.
This includes the following items:
* Current plan: The current plan information such as what tier your organization is on and what features/limits apply to this tier.
* Licenses: The license keys for self-hosted instances of Infisical (if applicable).
* Receipts: The receipts of monthly/annual invoices.
* Billing: The billing details of your organization including payment methods on file, tax IDs (if applicable), etc.

# Point-in-Time Recovery
Learn how to rollback secrets and configurations to any snapshot with Infisical.
Point-in-Time Recovery is a paid feature.
If you're using Infisical Cloud, then it is available under the **Pro Tier**. If you're self-hosting Infisical,
then you should contact [sales@infisical.com](mailto:sales@infisical.com) to purchase an enterprise license to use it.
Infisical's point-in-time recovery functionality allows secrets to be rolled back to any point in time for any given [folder](./folder) or [environment](/documentation/platform/project#project-environments).
Every time a secret is updated, a new snapshot is taken – capturing the state of the folder and environment at that point of time.
## Snapshots
Similar to Git, a commit (also known as snapshot) in Infisical is the state of your project's secrets at a specific point in time scoped to
an environment and [folder](./folder) within it.
To view a list of snapshots for the current folder, press the **Commits** button.
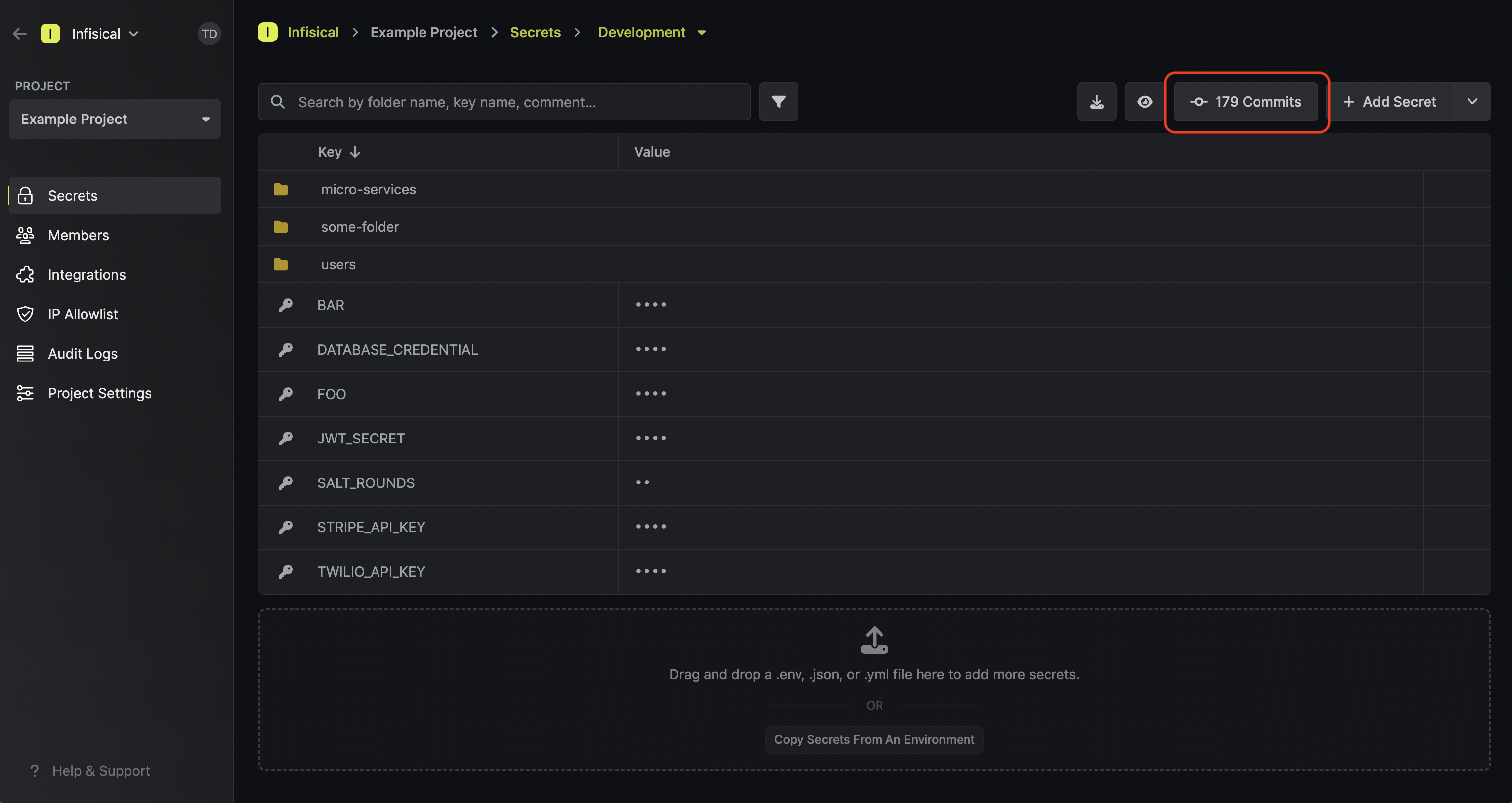
This opens up a sidebar from which you can select to view a particular snapshot:
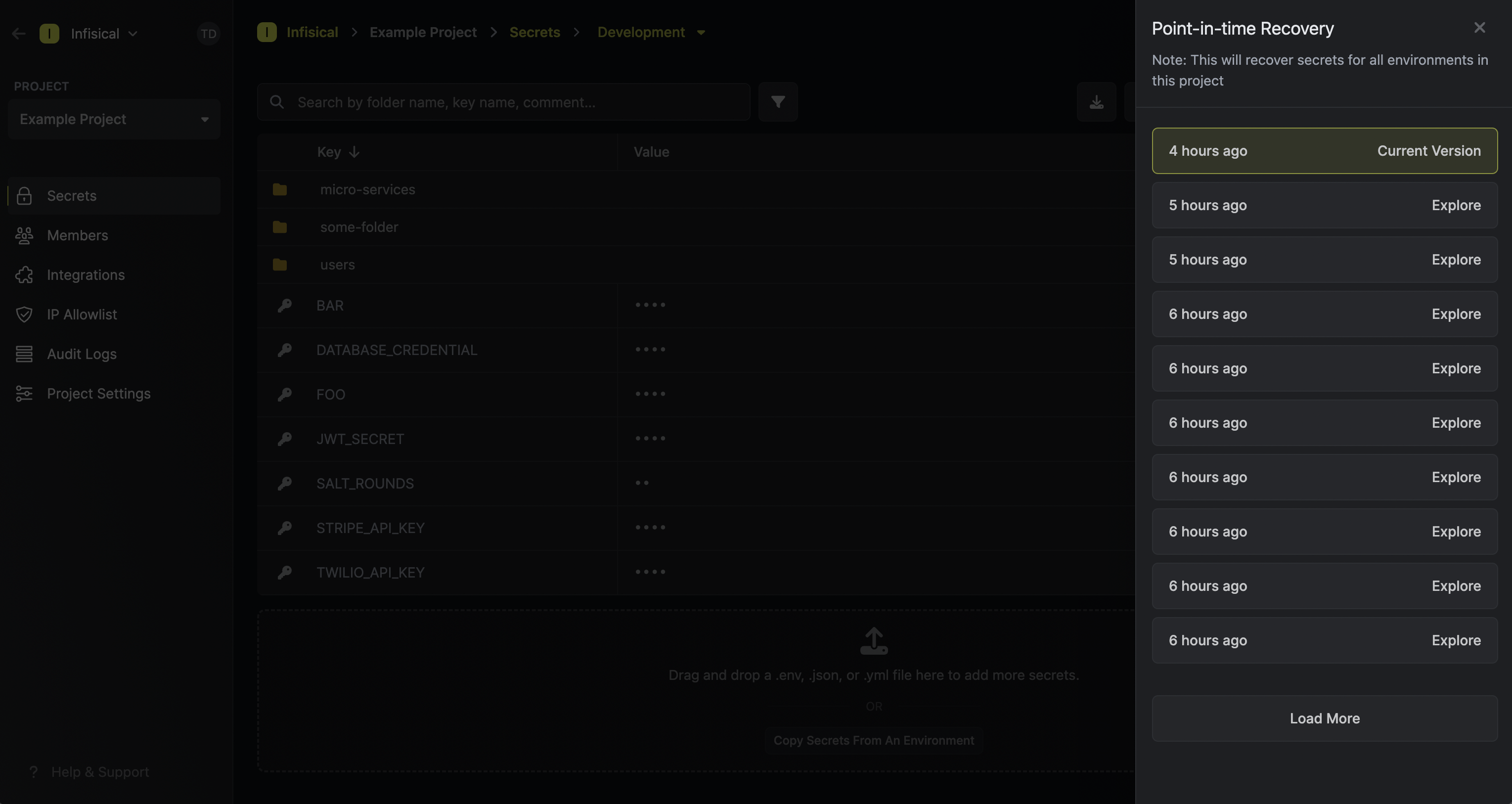
## Rolling back
After pressing on a snapshot from the sidebar, you can view it and roll back the state
of the folder to that point in time by pressing the **Rollback** button.
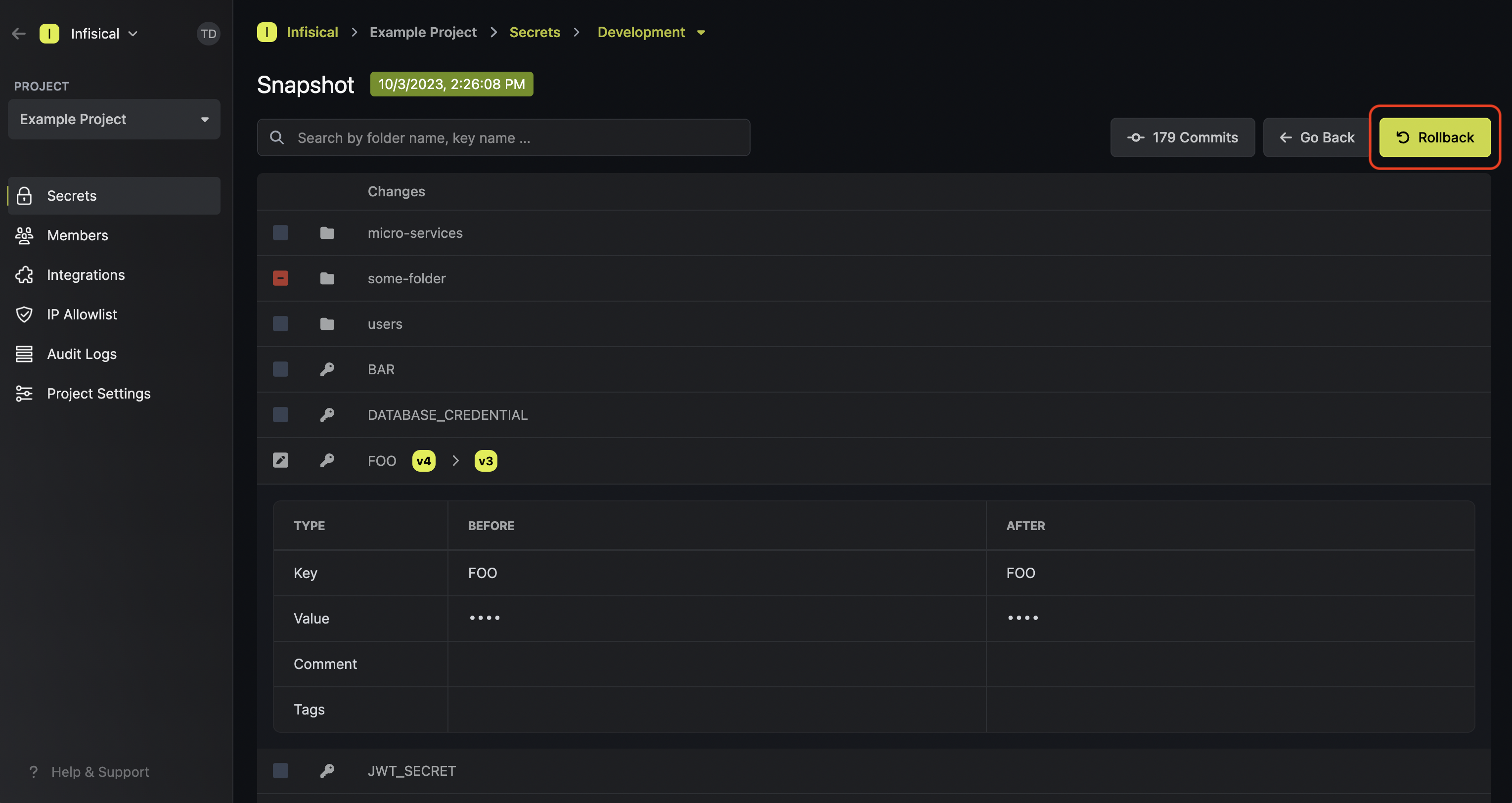
Rolling back secrets to a past snapshot creates a creates a snapshot at the top of the stack and updates secret versions.
Rollbacks are localized to not affect other folders within the same environment. This means each [folder](./folder) maintains its own independent history of changes, offering precise and isolated control over rollback actions.
Put differently, every [folder](./folder) possesses a distinct and separate timeline, providing granular control when managing your secrets.
# Alerting
Learn how to set up alerting for expiring certificates with Infisical
## Concept
In order to ensure that your certificates are always up-to-date and not expired, you can set up alerting for expiring CA and leaf certificates in Infisical.
## Workflow
A typical alerting workflow for expiring certificates consists of the following steps:
1. Creating a PKI/Certificate collection and adding certificates that you wish to monitor for expiration to it.
2. Creating an alert and binding it to the PKI/Certificate collection. As part of the configuration, you specify when the alert should trigger based on the number of days before certificate expiration and the email addresses of the recipients to notify.
## Guide to Creating an Alert
To create a PKI/Certificate collection, head to your Project > Internal
PKI > Alerting > Certificate Collection and press **Create**.
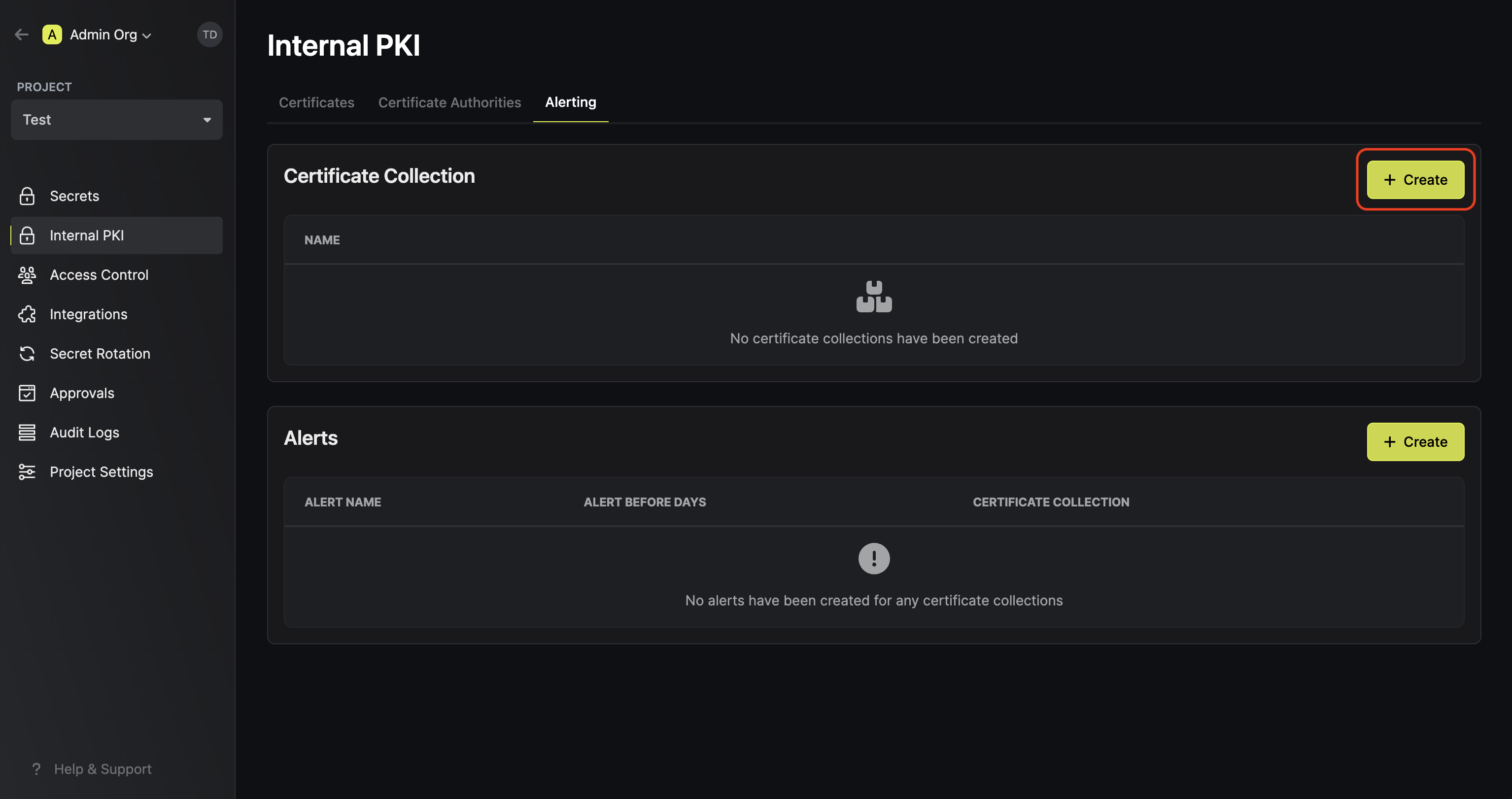
Give the collection a name and proceed to create the empty collection.
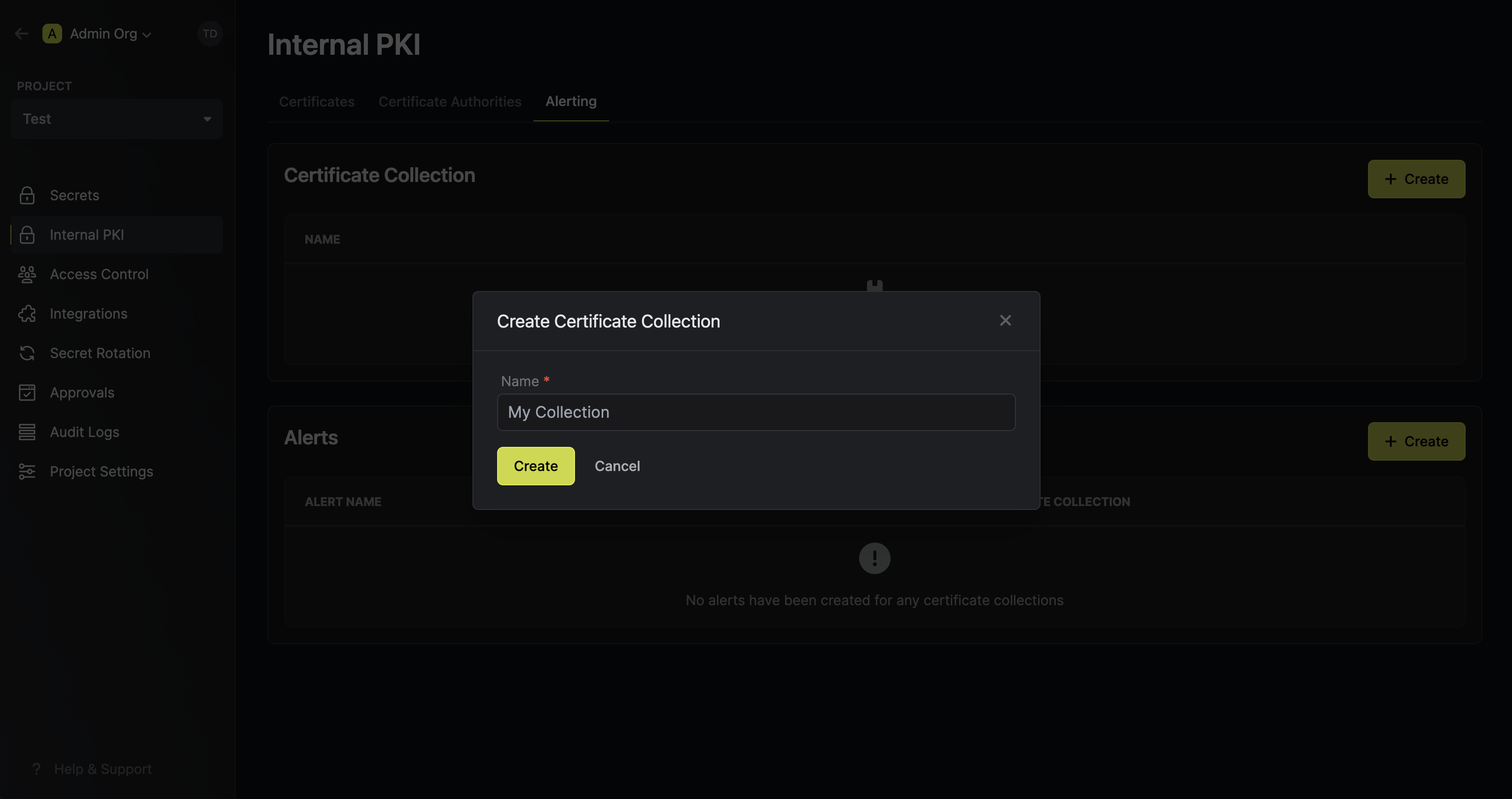
Next, in the Collection Page, add the certificate authorities and leaf certificates
that you wish to monitor for expiration to the collection.
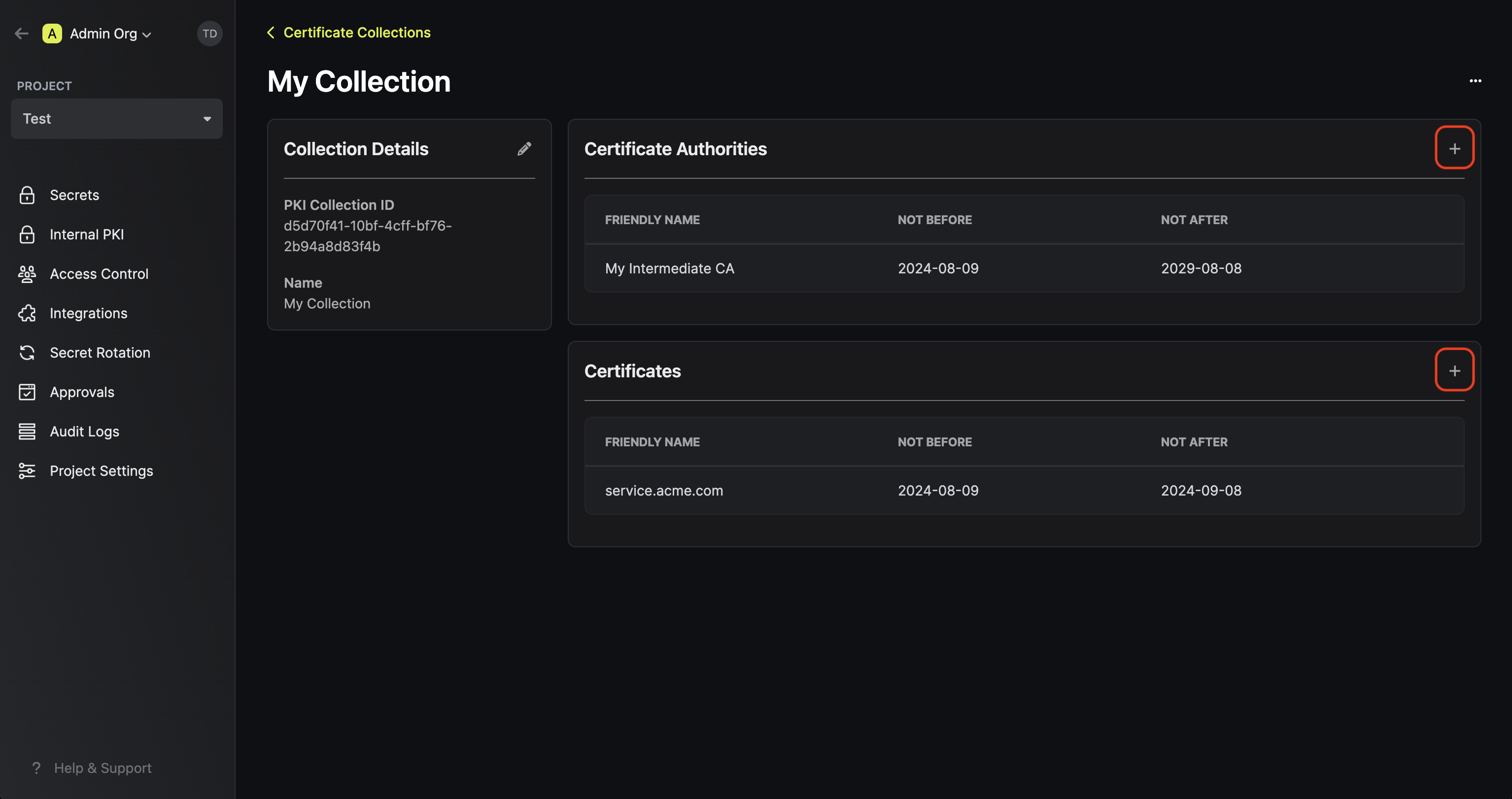
To create an alert, head to your Project > Internal PKI > Alerting > Alerts and press **Create**.
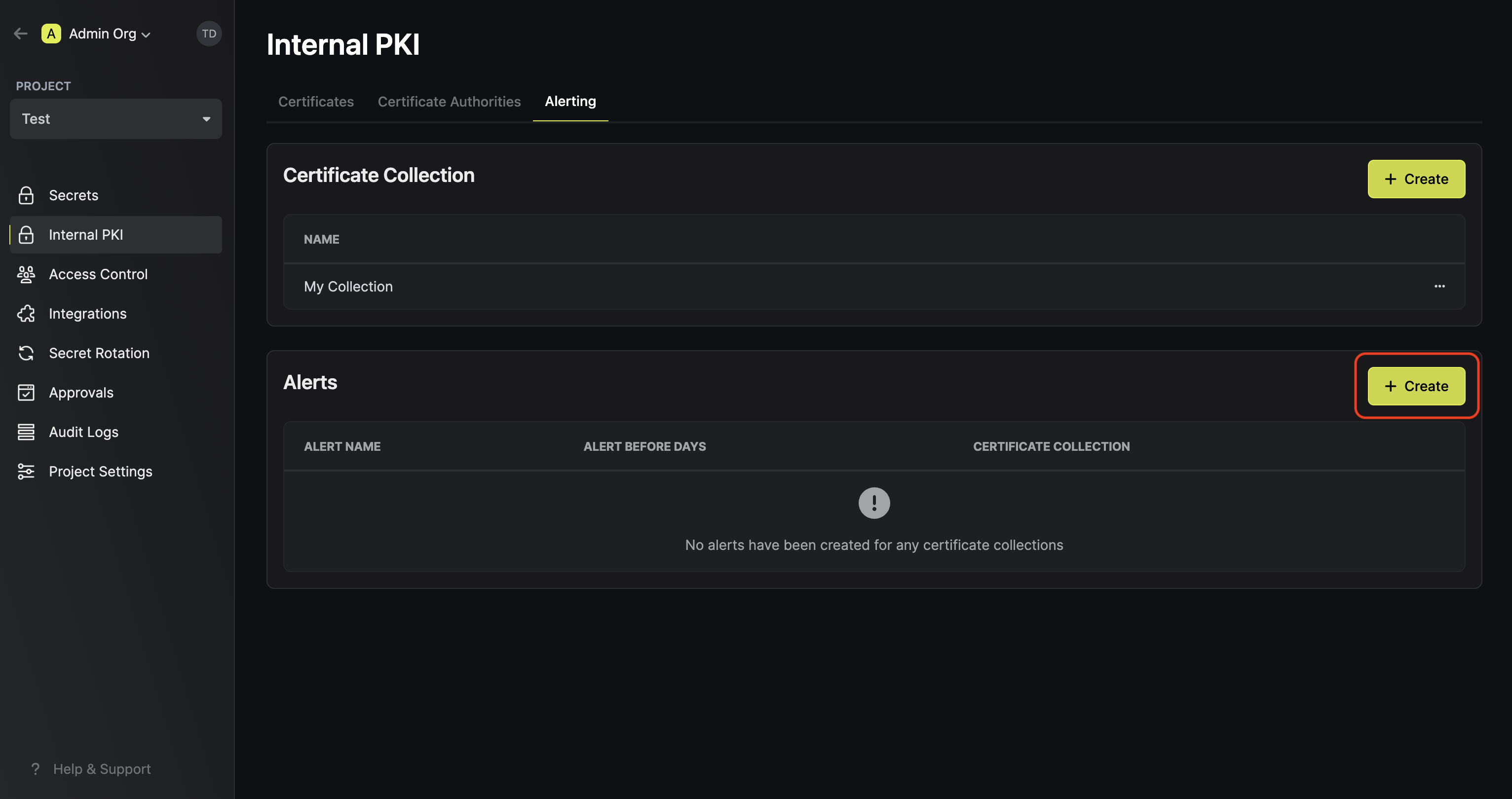
Here, set the **Certificate Collection** to the PKI/Certificate collection you created in the previous step and fill out details for the alert.
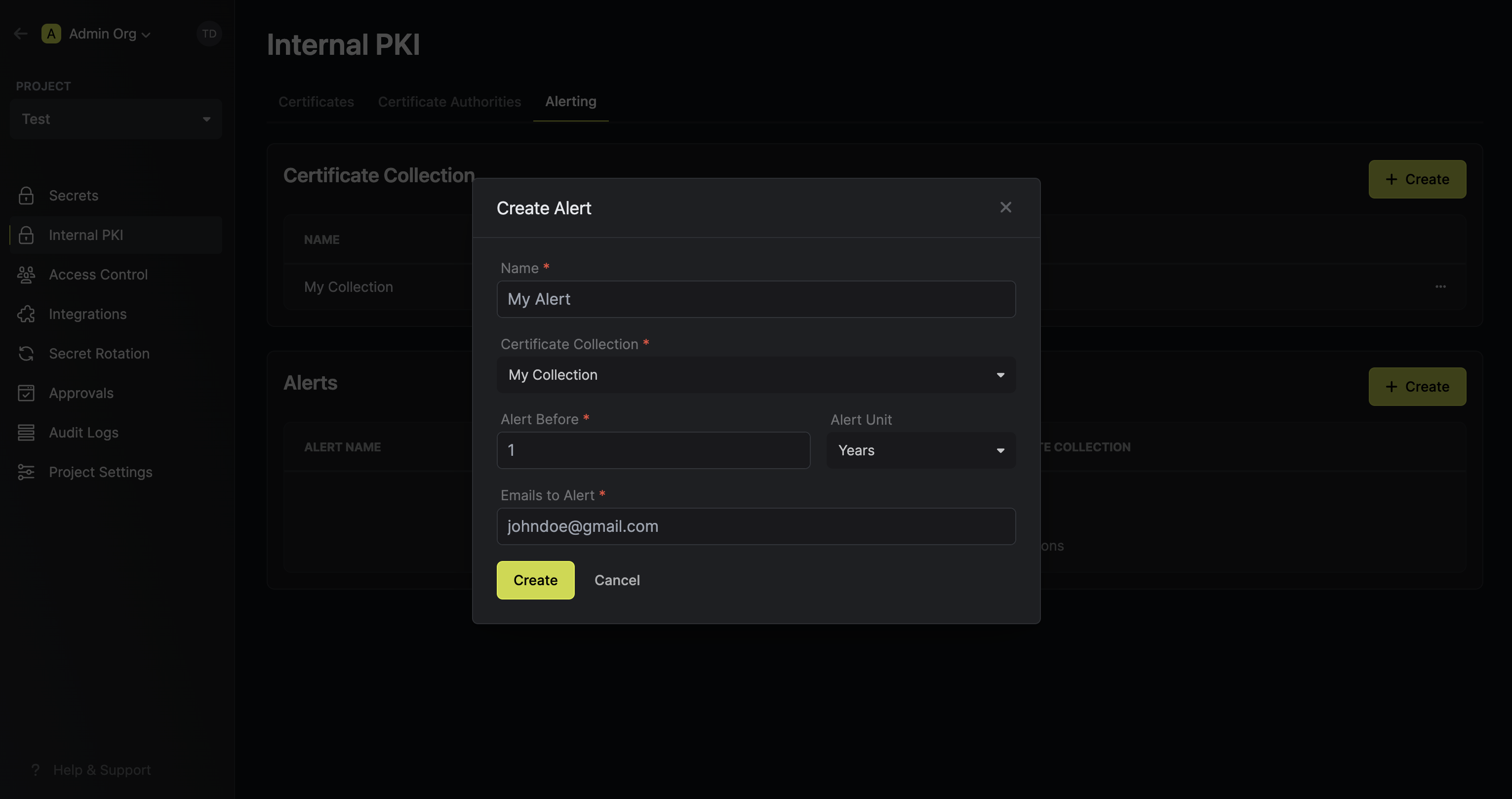
Here's some guidance on each field:
* Name: A name for the alert.
* Collection Collection: The PKI/Certificate collection to bind the alert to from the previous step.
* Alert Before / Unit: The time before certificate expiration to trigger the alert.
* Emails to Alert: A comma-delimited list of email addresses to notify when the alert triggers.
Finally, press **Create** to create the alert.
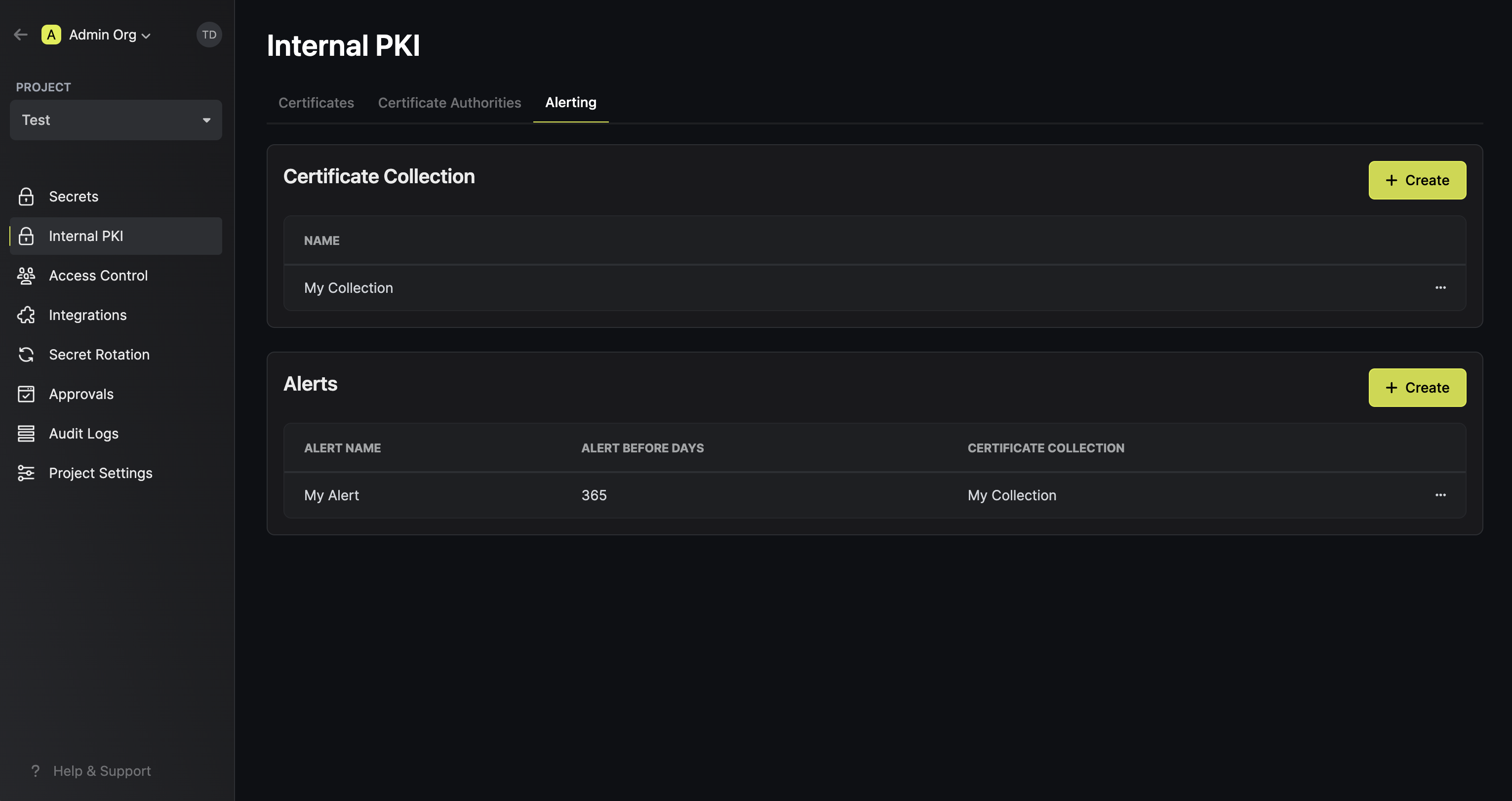
Great! You've successfully created a PKI/Certificate collection and an alert to monitor the expiring certificates in the collection. Once the alert triggers, the specified email addresses will be notified.
1.1. To create a PKI/Certificate collection, make an API request to the [Create PKI Collection](/api-reference/endpoints/pki-collections/create) API endpoint.
### Sample request
```bash Request
curl --location --request POST 'https://app.infisical.com/api/v1/pki/collections' \
--header 'Authorization: Bearer ' \
--header 'Content-Type: application/json' \
--data-raw '{
"projectId": "",
"name": "My Certificate Collection"
}'
```
### Sample response
```bash Response
{
id: "",
name: "My Certificate Collection",
...
}
```
1.2. Next, make an API request to the [Add Collection Item](/api-reference/endpoints/pki-collections/add-item) API endpoint to add a certificate to the collection.
### Sample request
```bash Request
curl --location --request POST 'https://app.infisical.com/api/v1/pki/collections//items' \
--header 'Authorization: Bearer ' \
--header 'Content-Type: application/json' \
--data-raw '{
"type": "certificate",
"itemId": "id-of-certificate"
}'
```
### Sample response
```bash Response
{
id: "",
type: "certificate",
itemId: "id-of-certificate"
...
}
```
To create an alert, make an API request to the [Create Alert](/api-reference/endpoints/pki-alerts/create) API endpoint, specifying the PKI/Certificate collection to bind the alert to, the alert configuration, and the email addresses to notify.
### Sample request
```bash Request
curl --location --request POST 'https://app.infisical.com/api/v1/pki/alerts' \
--header 'Authorization: Bearer ' \
--header 'Content-Type: application/json' \
--data-raw '{
"projectId": "",
"pkiCollectionId": "",
"name": "My Alert",
"alertBeforeDays": 30,
"emails": ["johndoe@gmail.com", "janedoe@gmail.com"]
}'
```
### Sample response
```bash Response
{
id: "",
name: "My Alert",
alertBeforeDays: 30,
recipientEmails: "johndoe@gmail.com,janedoe@gmail.com"
...
}
```
Great! You've successfully created a PKI/Certificate collection and an alert to monitor the expiring certificate in the collection. Once the alert triggers, the specified email addresses will be notified.
# Certificates
Learn how to issue X.509 certificates with Infisical.
## Concept
Assuming that you've created a Private CA hierarchy with a root CA and an intermediate CA, you can now issue/revoke X.509 certificates using the intermediate CA.
```mermaid
graph TD
A[Root CA]
A --> B[Intermediate CA]
A --> C[Intermediate CA]
B --> D[Leaf Certificate]
C --> E[Leaf Certificate]
```
## Workflow
The typical workflow for managing certificates consists of the following steps:
1. Issuing a certificate under an intermediate CA with details like name and validity period. As part of certificate issuance, you can either issue a certificate directly from a CA or do it via a certificate template.
2. Managing certificate lifecycle events such as certificate renewal and revocation. As part of the certificate revocation flow,
you can also query for a Certificate Revocation List [CRL](https://en.wikipedia.org/wiki/Certificate_revocation_list), a time-stamped, signed
data structure issued by a CA containing a list of revoked certificates to check if a certificate has been revoked.
Note that this workflow can be executed via the Infisical UI or manually such
as via API.
## Guide to Issuing Certificates
In the following steps, we explore how to issue a X.509 certificate under a CA.
A certificate template is a set of policies for certificates issued under that template; each template is bound to a specific CA and can also be bound to a certificate collection for alerting such that any certificate issued under the template is automatically added to the collection.
With certificate templates, you can specify, for example, that issued certificates must have a common name (CN) adhering to a specific format like `.*.acme.com` or perhaps that the max TTL cannot be more than 1 year.
Head to your Project > Certificate Authorities > Your Issuing CA and create a certificate template.
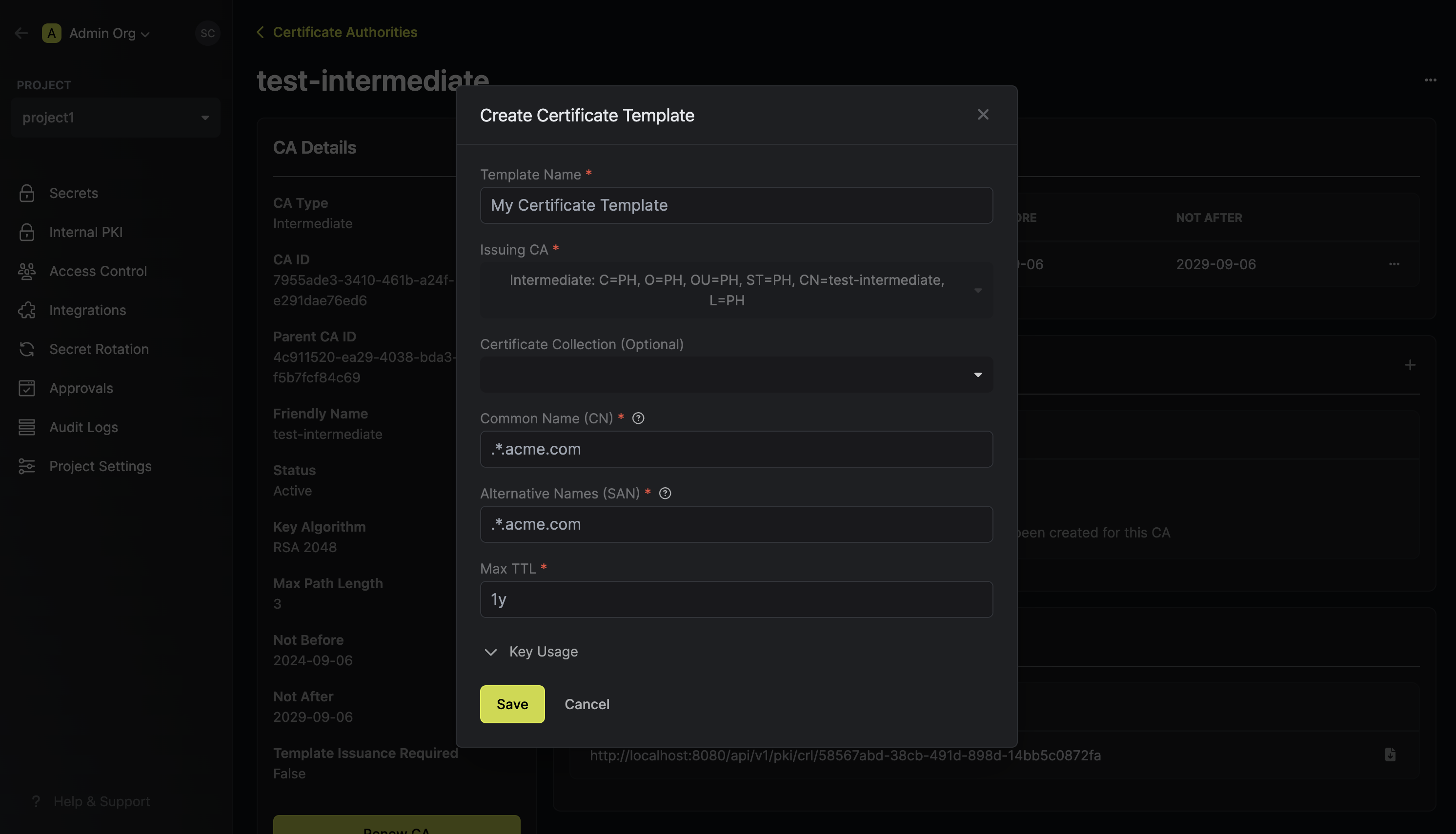
Here's some guidance on each field:
* Template Name: A name for the certificate template.
* Issuing CA: The Certificate Authority (CA) that will issue certificates based on this template.
* Certificate Collection (Optional): The certificate collection that certificates should be added to when issued under the template.
* Common Name (CN): A regular expression used to validate the common name in certificate requests.
* Alternative Names (SANs): A regular expression used to validate subject alternative names in certificate requests.
* TTL: The maximum Time-to-Live (TTL) for certificates issued using this template.
* Key Usage: The key usage constraint or default value for certificates issued using this template.
* Extended Key Usage: The extended key usage constraint or default value for certificates issued using this template.
To create a certificate, head to your Project > Internal PKI > Certificates and press **Issue** under the Certificates section.
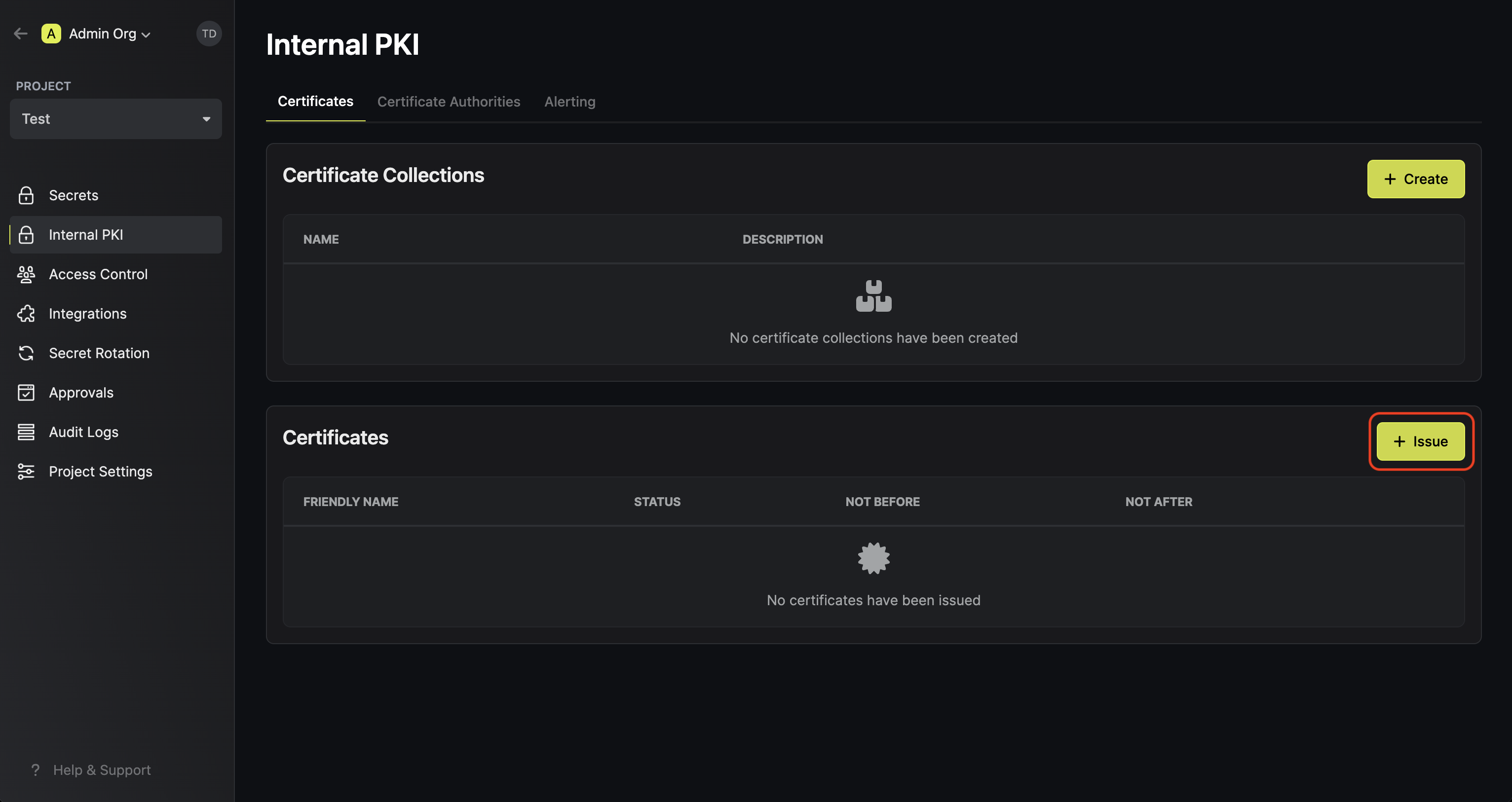
Here, set the **Certificate Template** to the template from step 1 and fill out the rest of the details for the certificate to be issued.
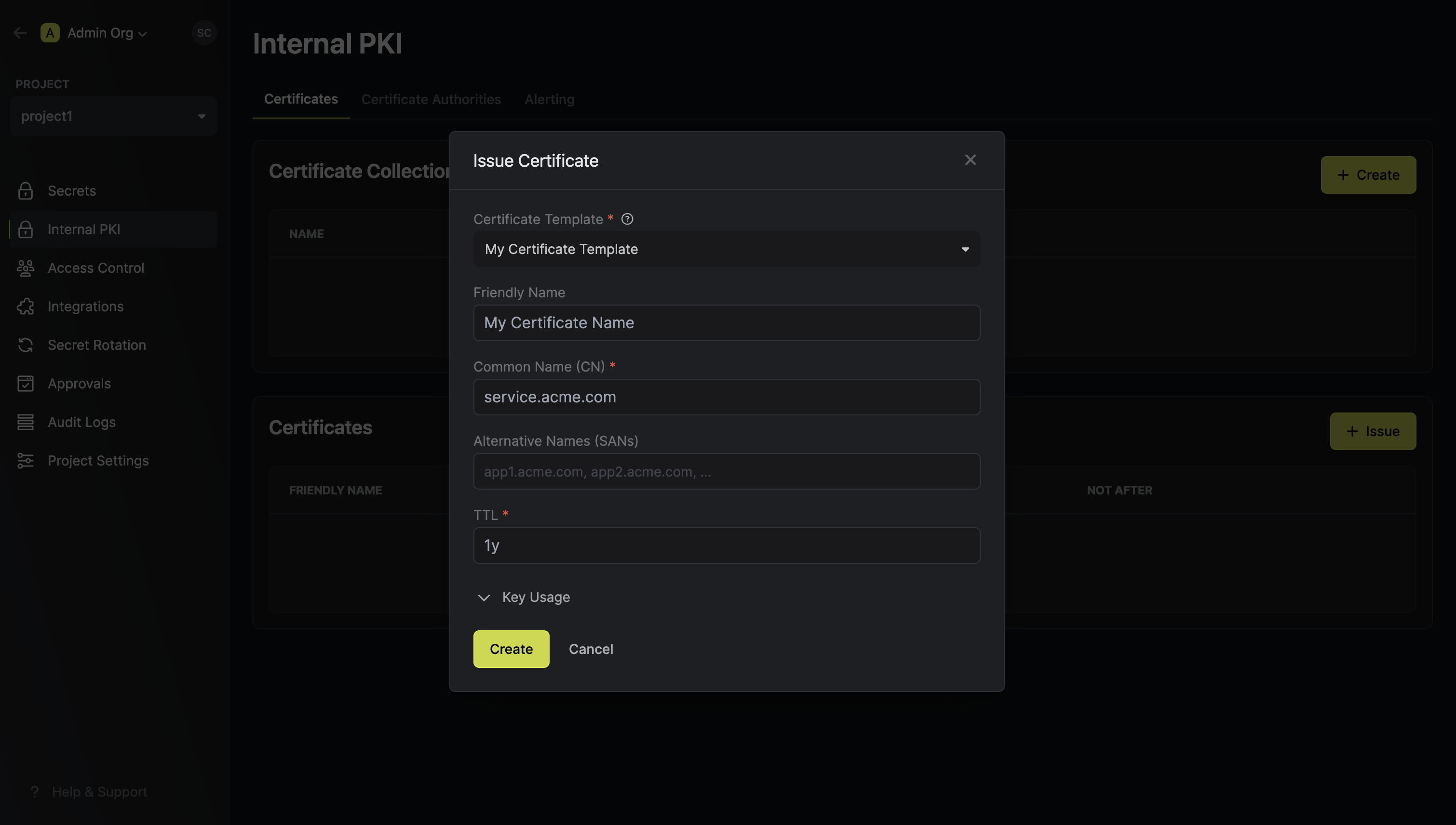
Here's some guidance on each field:
* Friendly Name: A friendly name for the certificate; this is only for display and defaults to the common name of the certificate if left empty.
* Common Name (CN): The (common) name for the certificate like `service.acme.com`.
* Alternative Names (SANs): A comma-delimited list of Subject Alternative Names (SANs) for the certificate; these can be host names or email addresses like `app1.acme.com, app2.acme.com`.
* TTL: The lifetime of the certificate in seconds.
* Key Usage: The key usage extension of the certificate.
* Extended Key Usage: The extended key usage extension of the certificate.
Note that Infisical PKI supports issuing certificates without certificate templates as well. If this is desired, then you can set the **Certificate Template** field to **None**
and specify the **Issuing CA** and optional **Certificate Collection** fields; the rest of the fields for the issued certificate remain the same.
That said, we recommend using certificate templates to enforce policies and attach expiration monitoring on issued certificates.
Once you have created the certificate from step 1, you'll be presented with the certificate details including the **Certificate Body**, **Certificate Chain**, and **Private Key**.
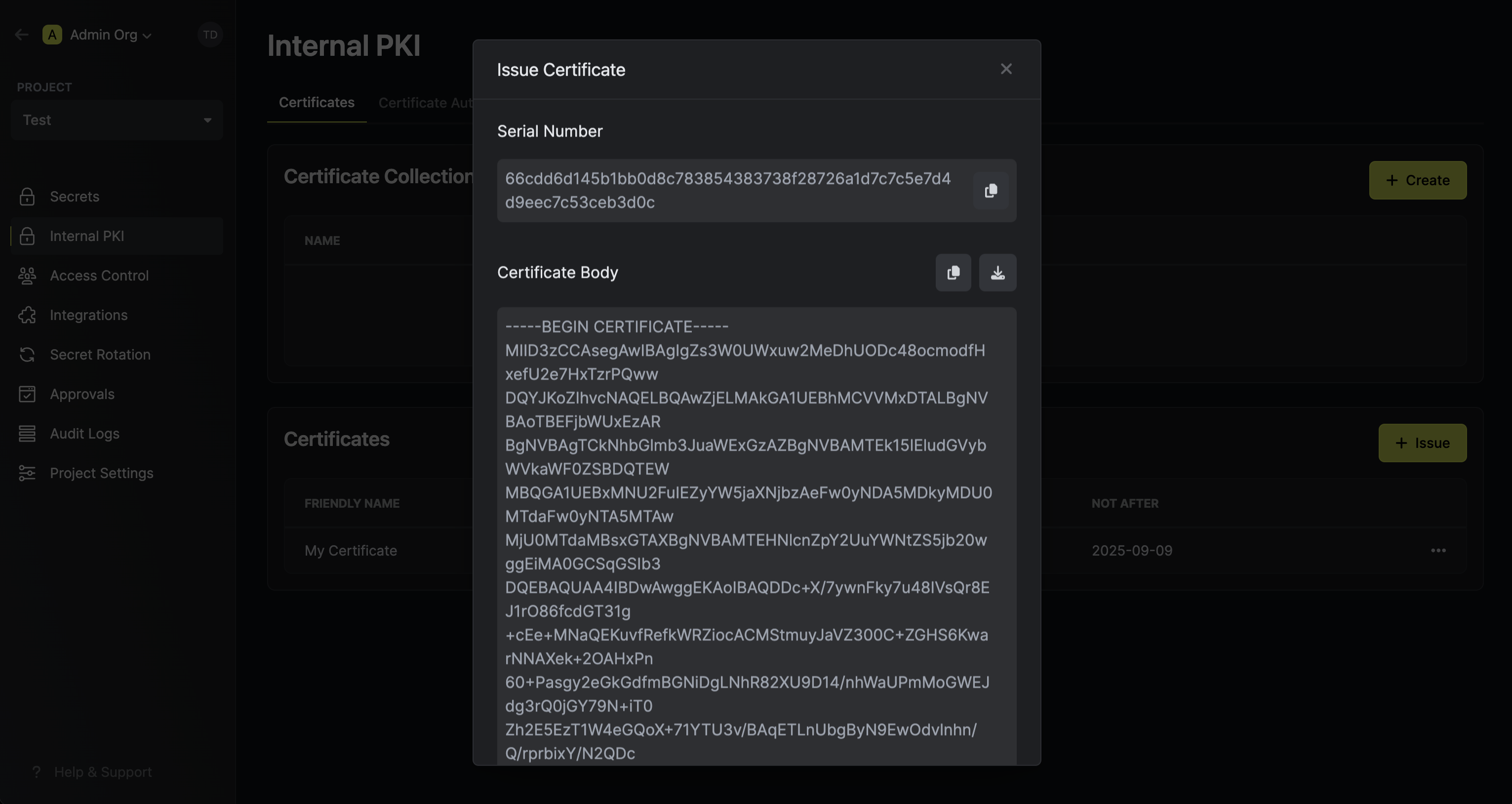
Make sure to download and store the **Private Key** in a secure location as it will only be displayed once at the time of certificate issuance.
The **Certificate Body** and **Certificate Chain** will remain accessible and can be copied at any time.
A certificate template is a set of policies for certificates issued under that template; each template is bound to a specific CA and can also be bound to a certificate collection for alerting such that any certificate issued under the template is automatically added to the collection.
With certificate templates, you can specify, for example, that issued certificates must have a common name (CN) adhering to a specific format like .\*.acme.com or perhaps that the max TTL cannot be more than 1 year.
To create a certificate template, make an API request to the [Create Certificate Template](/api-reference/endpoints/certificate-templates/create) API endpoint, specifying the issuing CA.
### Sample request
```bash Request
curl --location --request POST 'https://app.infisical.com/api/v1/pki/certificate-templates' \
--header 'Content-Type: application/json' \
--data-raw '{
"caId": "",
"name": "My Certificate Template",
"commonName": ".*.acme.com",
"subjectAlternativeName": ".*.acme.com",
"ttl": "1y",
}'
```
### Sample response
```bash Response
{
id: "...",
caId: "...",
name: "...",
commonName: "...",
subjectAlternativeName: "...",
ttl: "...",
}
```
To create a certificate under the certificate template, make an API request to the [Issue Certificate](/api-reference/endpoints/certificates/issue-cert) API endpoint,
specifying the issuing CA.
### Sample request
```bash Request
curl --location --request POST 'https://app.infisical.com/api/v1/pki/certificates/issue-certificate' \
--header 'Content-Type: application/json' \
--data-raw '{
"certificateTemplateId": "",
"commonName": "service.acme.com",
"ttl": "1y",
}'
```
### Sample response
```bash Response
{
certificate: "...",
certificateChain: "...",
issuingCaCertificate: "...",
privateKey: "...",
serialNumber: "..."
}
```
Note that Infisical PKI supports issuing certificates without certificate templates as well. If this is desired, then you can set the **Certificate Template** field to **None**
and specify the **Issuing CA** and optional **Certificate Collection** fields; the rest of the fields for the issued certificate remain the same.
That said, we recommend using certificate templates to enforce policies and attach expiration monitoring on issued certificates.
Make sure to store the `privateKey` as it is only returned once here at the time of certificate issuance. The `certificate` and `certificateChain` will remain accessible and can be retrieved at any time.
If you have an external private key, you can also create a certificate by making an API request containing a pem-encoded CSR (Certificate Signing Request) to the [Sign Certificate](/api-reference/endpoints/certificates/sign-certificate) API endpoint, specifying the issuing CA.
### Sample request
```bash Request
curl --location --request POST 'https://app.infisical.com/api/v1/pki/certificates/sign-certificate' \
--header 'Content-Type: application/json' \
--data-raw '{
"certificateTemplateId": "",
"csr": "...",
"ttl": "1y",
}'
```
### Sample response
```bash Response
{
certificate: "...",
certificateChain: "...",
issuingCaCertificate: "...",
privateKey: "...",
serialNumber: "..."
}
```
## Guide to Revoking Certificates
In the following steps, we explore how to revoke a X.509 certificate under a CA and obtain a Certificate Revocation List (CRL) for a CA.
Assuming that you've issued a certificate under a CA, you can revoke it by
selecting the **Revoke Certificate** option for it and specifying the reason
for revocation.
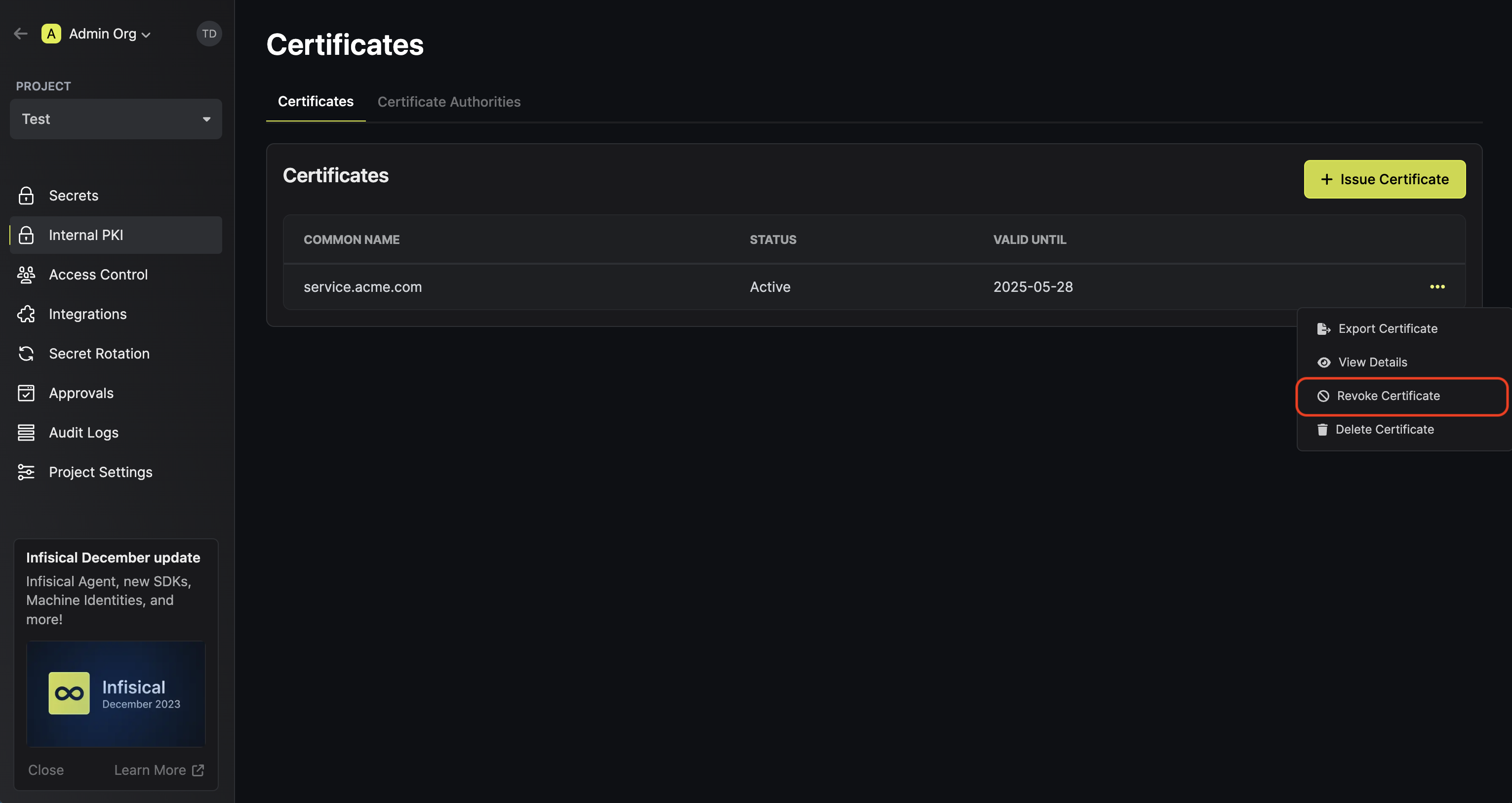
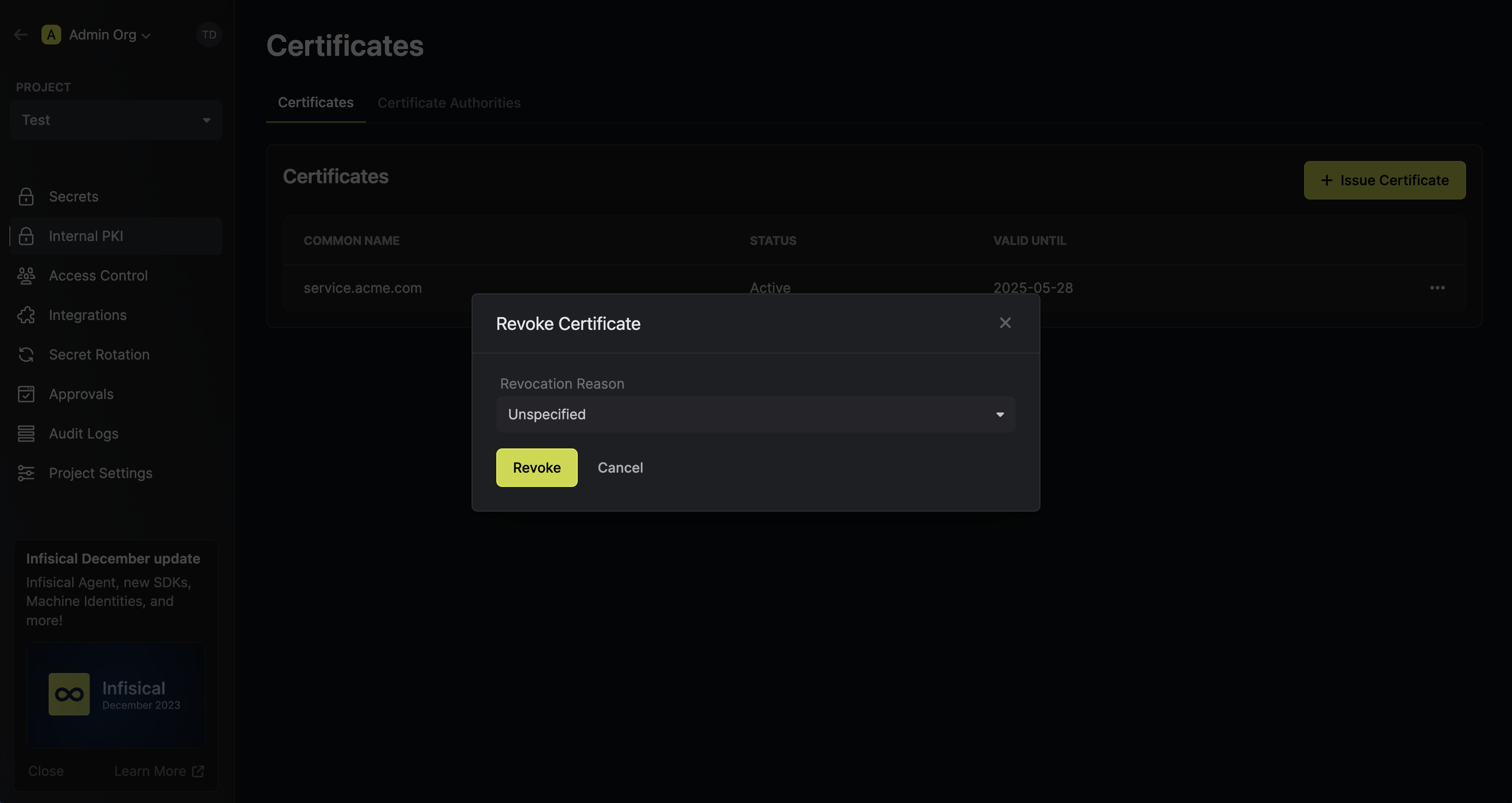
In order to check the revocation status of a certificate, you can check it
against the CRL of a CA by heading to its Issuing CA and downloading the CRL.
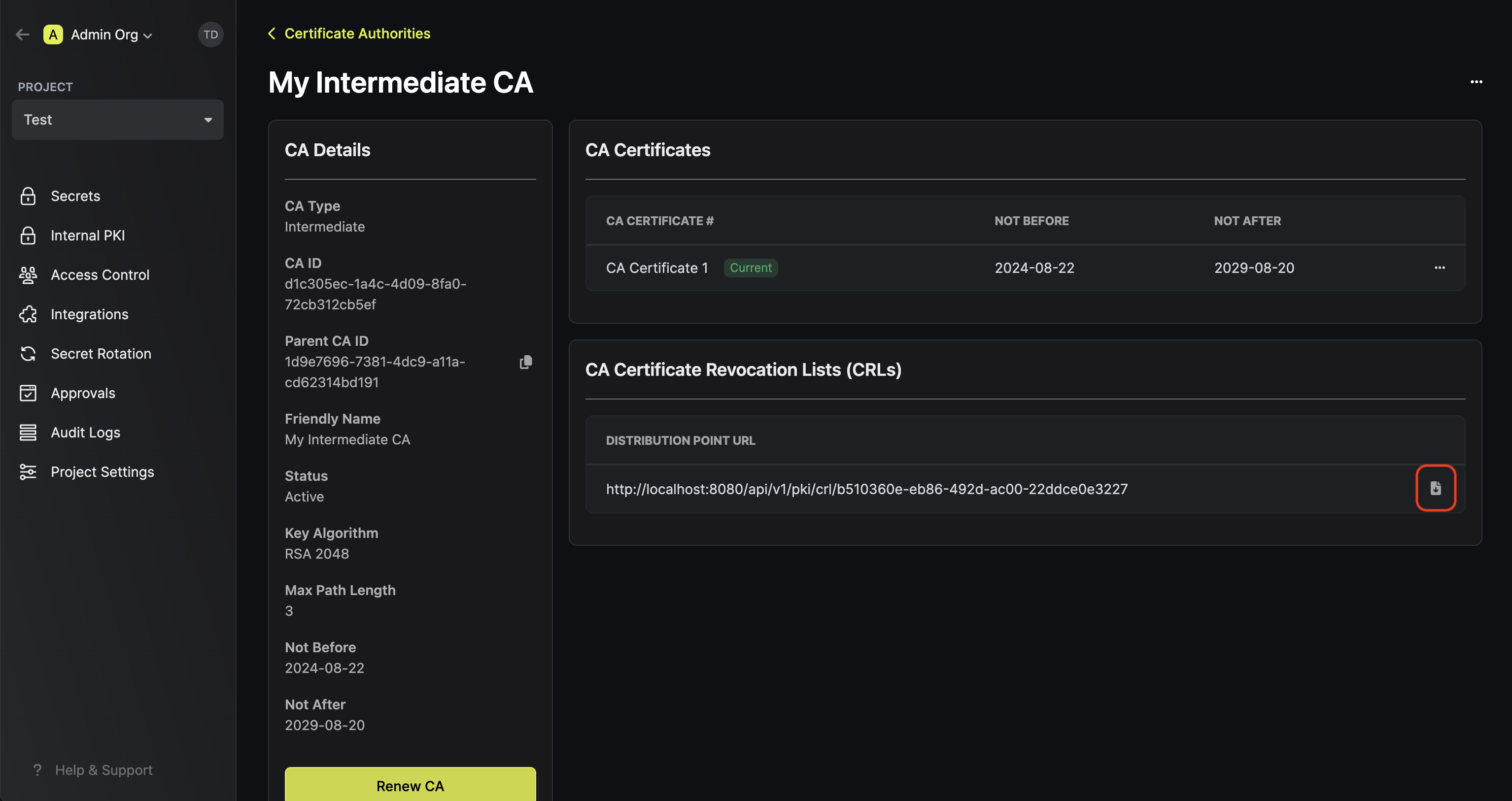
To verify a certificate against the
downloaded CRL with OpenSSL, you can use the following command:
```bash
openssl verify -crl_check -CAfile chain.pem -CRLfile crl.pem cert.pem
```
Note that you can also obtain the CRL from the certificate itself by
referencing the CRL distribution point extension on the certificate itself.
To check a certificate against the CRL distribution point specified within it with OpenSSL, you can use the following command:
```bash
openssl verify -verbose -crl_check -crl_download -CAfile chain.pem cert.pem
```
Assuming that you've issued a certificate under a CA, you can revoke it by making an API request to the [Revoke Certificate](/api-reference/endpoints/certificate-authorities/revoke) API endpoint,
specifying the serial number of the certificate and the reason for revocation.
### Sample request
```bash Request
curl --location --request POST 'https://app.infisical.com/api/v1/pki/certificates//revoke' \
--header 'Authorization: Bearer ' \
--header 'Content-Type: application/json' \
--data-raw '{
"revocationReason": "UNSPECIFIED"
}'
```
### Sample response
```bash Response
{
message: "Successfully revoked certificate",
serialNumber: "...",
revokedAt: "..."
}
```
In order to check the revocation status of a certificate, you can check it against the CRL of the issuing CA.
To obtain the CRLs of the CA, make an API request to the [List CRLs](/api-reference/endpoints/certificate-authorities/crls) API endpoint.
### Sample request
```bash Request
curl --location --request GET 'https://app.infisical.com/api/v1/pki/ca//crls' \
--header 'Authorization: Bearer '
```
### Sample response
```bash Response
[
{
id: "...",
crl: "..."
},
...
]
```
To verify a certificate against the CRL with OpenSSL, you can use the following command:
```bash
openssl verify -crl_check -CAfile chain.pem -CRLfile crl.pem cert.pem
```
## FAQ
To renew a certificate, you have to issue a new certificate from the same CA
with the same common name as the old certificate. The original certificate
will continue to be valid through its original TTL unless explicitly
revoked.
# Enrollment over Secure Transport (EST)
Learn how to manage certificate enrollment of clients using EST
## Concept
Enrollment over Secure Transport (EST) is a protocol used to automate the secure provisioning of digital certificates for devices and applications over a secure HTTPS connection. It is primarily used when a client device needs to obtain or renew a certificate from a Certificate Authority (CA) on Infisical in a secure and standardized manner. EST is commonly employed in environments requiring strong authentication and encrypted communication, such as in IoT, enterprise networks, and secure web services.
Infisical's EST service is based on [RFC 7030](https://datatracker.ietf.org/doc/html/rfc7030) and implements the following endpoints:
* **cacerts** - provides the necessary CA chain for the client to validate certificates issued by the CA.
* **simpleenroll** - allows an EST client to request a new certificate from Infisical's EST server
* **simplereenroll** - similar to the /simpleenroll endpoint but is used for renewing an existing certificate.
These endpoints are exposed on port 8443 under the .well-known/est path e.g.
`https://app.infisical.com:8443/.well-known/est/estLabel/cacerts`
## Prerequisites
* You need to have an existing [CA hierarchy](/documentation/platform/pki/private-ca).
* The client devices need to have a bootstrap/pre-installed certificate.
* The client devices must trust the server certificates used by Infisical's EST server. If the devices are new or lack existing trust configurations, you need to manually establish trust for the appropriate certificates.
* For Infisical Cloud users, the devices must be configured to trust the [Amazon root CA certificates](https://www.amazontrust.com/repository).
## Guide to configuring EST
1. Set up a certificate template with your selected issuing CA. This template will define the policies and parameters for certificates issued through EST. For detailed instructions on configuring a certificate template, refer to the certificate templates [documentation](/documentation/platform/pki/certificates#guide-to-issuing-certificates).
2. Proceed to the certificate template's enrollment settings
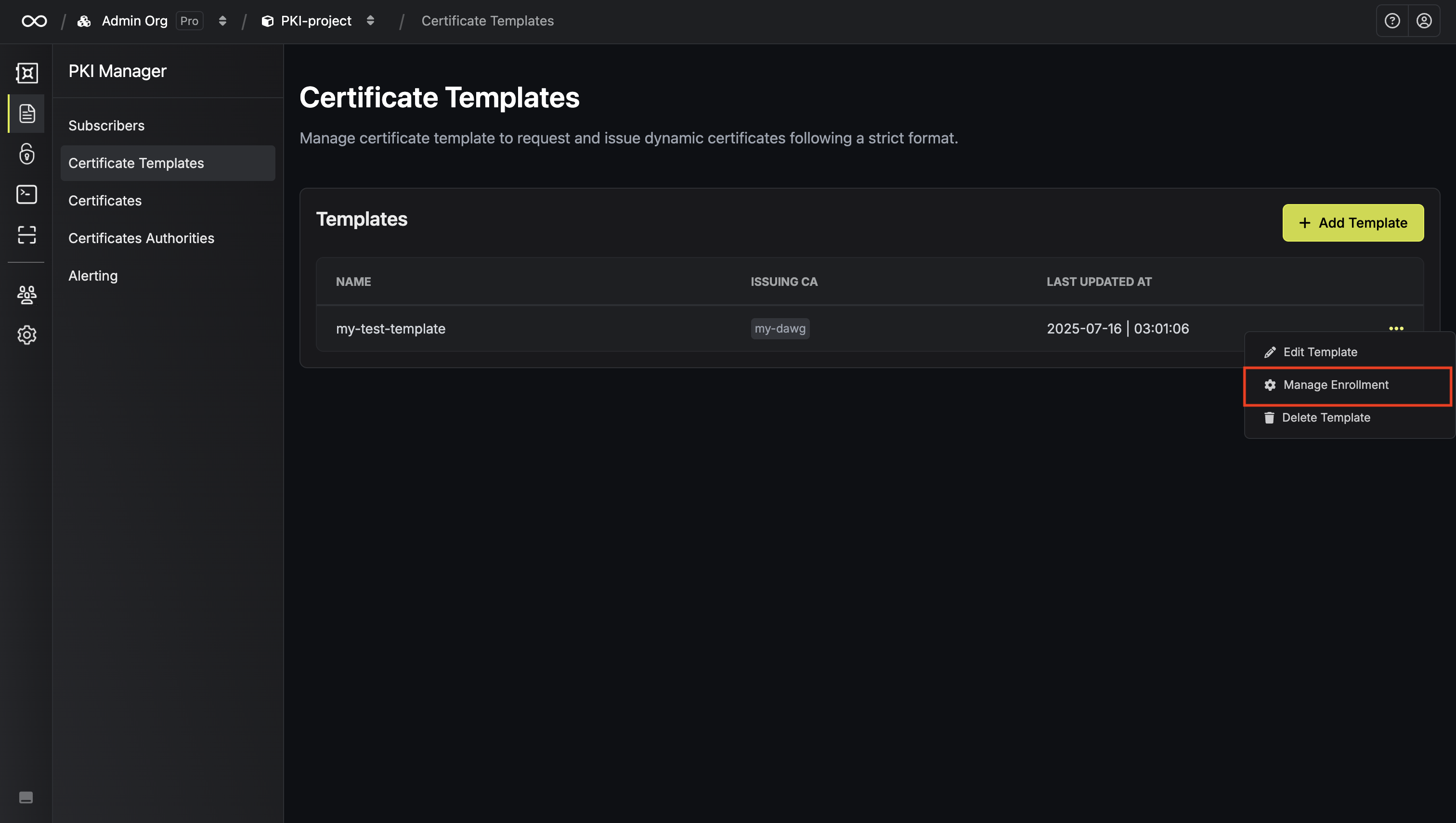
3. Select **EST** as the client enrollment method and fill up the remaining fields.
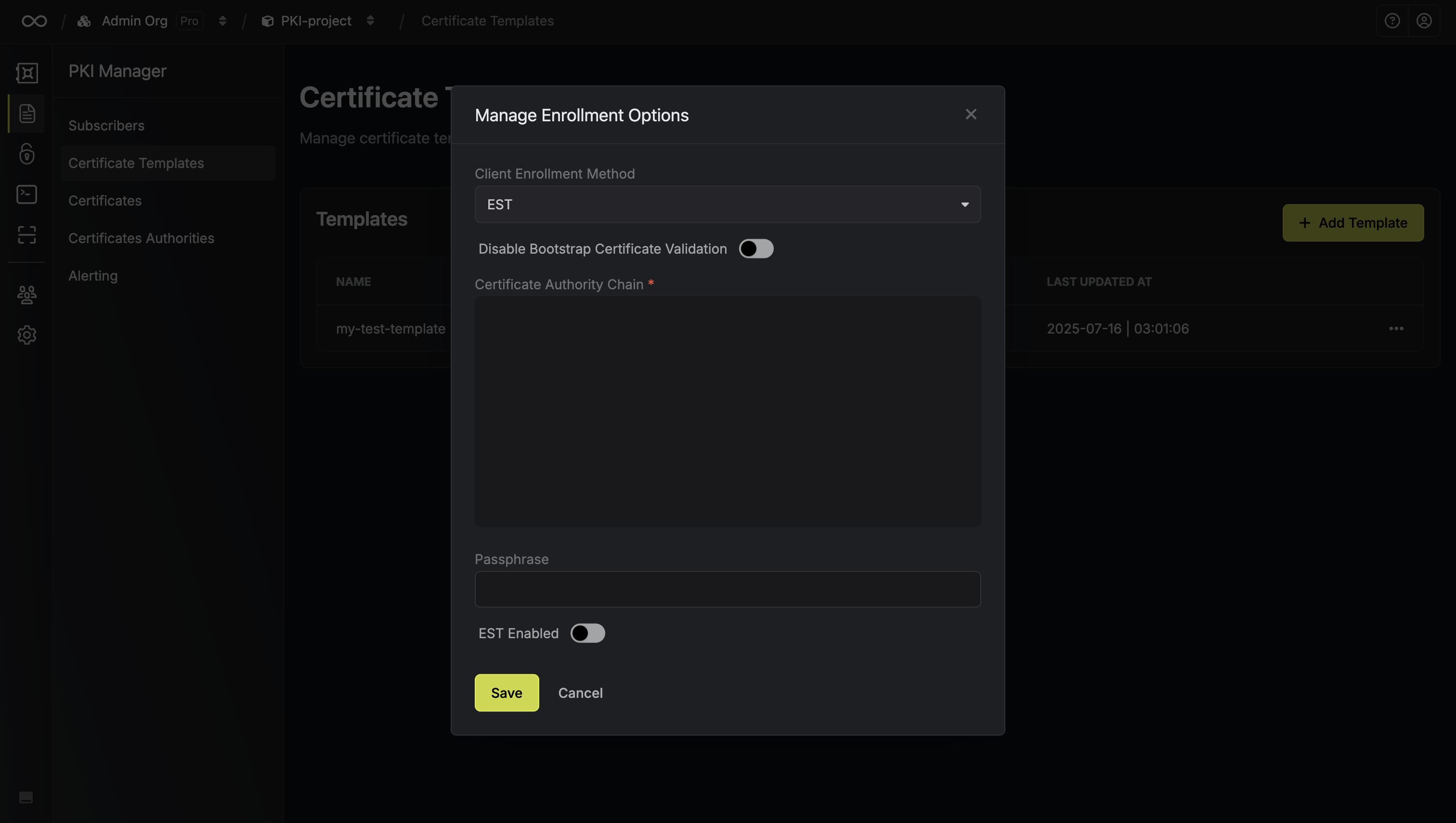
* **Disable Bootstrap Certificate Validation** - Enable this if your devices are not configured with a bootstrap certificate.
* **Certificate Authority Chain** - This is the certificate chain used to validate your devices' manufacturing/pre-installed certificates. This will be used to authenticate your devices with Infisical's EST server.
* **Passphrase** - This is also used to authenticate your devices with Infisical's EST server. When configuring the clients, use the value defined here as the EST password.
For security reasons, Infisical authenticates EST clients using both client certificate and passphrase.
4. Once the configuration of enrollment options is completed, a new **EST Label** field appears in the enrollment settings. This is the value to use as label in the URL when configuring the connection of EST clients to Infisical.
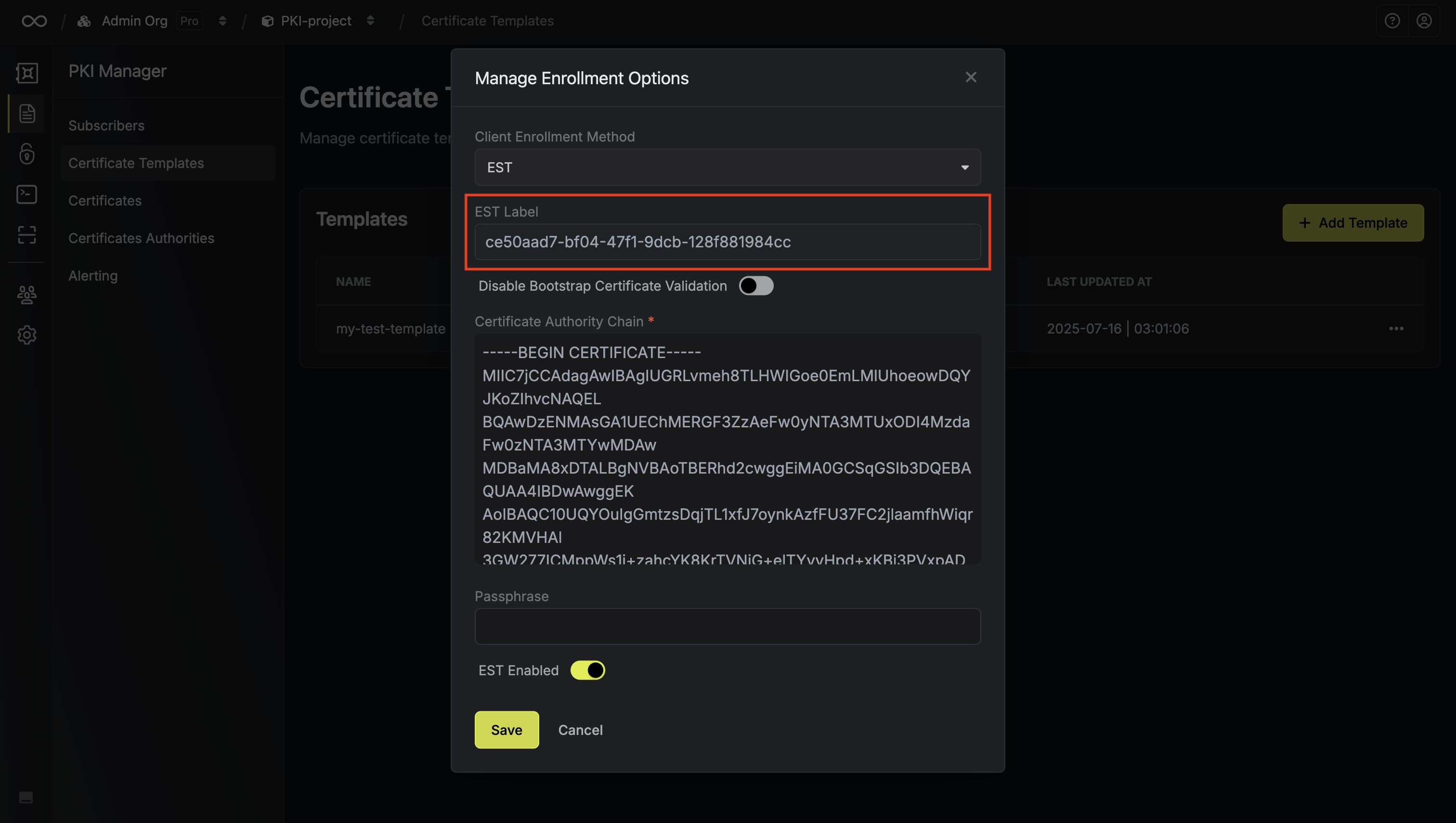
The complete URL of the supported EST endpoints will look like the following:
* [https://app.infisical.com:8443/.well-known/est/f110f308-9888-40ab-b228-237b12de8b96/cacerts](https://app.infisical.com:8443/.well-known/est/f110f308-9888-40ab-b228-237b12de8b96/cacerts)
* [https://app.infisical.com:8443/.well-known/est/f110f308-9888-40ab-b228-237b12de8b96/simpleenroll](https://app.infisical.com:8443/.well-known/est/f110f308-9888-40ab-b228-237b12de8b96/simpleenroll)
* [https://app.infisical.com:8443/.well-known/est/f110f308-9888-40ab-b228-237b12de8b96/simplereenroll](https://app.infisical.com:8443/.well-known/est/f110f308-9888-40ab-b228-237b12de8b96/simplereenroll)
## Setting up EST clients
* To use the EST passphrase in your clients, configure it as the EST password. The EST username can be set to any arbitrary value.
* Use the appropriate client certificates for invoking the EST endpoints.
* For `simpleenroll`, use the bootstrapped/manufacturer client certificate.
* For `simplereenroll`, use a valid EST-issued client certificate.
* When configuring the PKCS#12 objects for the client certificates, only include the leaf certificate and the private key.
# Internal PKI
Learn how to create a Private CA hierarchy and issue X.509 certificates.
Infisical can be used to create a Private Certificate Authority (CA) hierarchy and issue X.509 certificates for internal use. This allows you to manage your own PKI infrastructure and issue digital certificates for services, applications, and devices.
Infisical's internal PKI offering is split into two modules:
* [Private CA](/documentation/platform/pki/private-ca): Infisical lets you create private CAs, including root and intermediary CAs.
* [Certificates](/documentation/platform/pki/certificates): Infisical allows you to issue X.509 certificates using the private CAs you create.
# Kubernetes Issuer
Learn how to automatically provision and manage TLS certificates for in Kubernetes using Infisical PKI
## Concept
The Infisical PKI Issuer is an installable Kubernetes [cert-manager](https://cert-manager.io/) controller that uses Infisical PKI to sign certificate requests. The issuer is perfect for getting X.509 certificates for ingresses and other Kubernetes resources and capable of automatically renewing certificates as needed.
As part of the workflow, you install `cert-manager`, the Infisical PKI Issuer, and configure resources to represent the connection details to your Infisical PKI and the certificates you wish to issue. Each issued certificate and corresponding private key is made available in a Kubernetes secret.
We recommend reading the [cert-manager documentation](https://cert-manager.io/docs/) for a fuller understanding of all the moving parts.
## Workflow
A typical workflow for using the Infisical PKI Issuer to issue certificates for your Kubernetes resources consists of the following steps:
1. Creating a machine identity in Infisical.
2. Creating a Kubernetes secret to store the credentials of the machine identity.
3. Installing `cert-manager` into your Kubernetes cluster.
4. Installing the Infisical PKI Issuer controller into your Kubernetes cluster.
5. Creating an `Issuer` or `ClusterIssuer` resource in your Kubernetes cluster to represent the Infisical PKI issuer you wish to use.
6. Creating a `Certificate` resource in your Kubernetes cluster to represent a certificate you wish to issue. As part of this step, you specify the Kubernetes `Secret` to create and store the issued certificate and private key.
7. Consuming the issued certificate across your Kubernetes resources from the specified Kubernetes `Secret`.
## Guide
In the following steps, we explore how to install the Infisical PKI Issuer using [kubectl](https://github.com/kubernetes/kubectl) and use it to obtain certificates for your Kubernetes resources.
Follow the instructions [here](/documentation/platform/identities/universal-auth) to configure a [machine identity](/documentation/platform/identities/machine-identities) in Infisical with Universal Auth.
By the end of this step, you should have a **Client ID** and **Client Secret** on hand as part of the Universal Auth configuration for the Infisical PKI Issuer to authenticate with Infisical; this will be useful in steps 4 and 5.
Currently, the Infisical PKI Issuer only supports authenticating with Infisical via the [Universal Auth](/documentation/platform/identities/universal-auth) authentication method.
We're planning to add support for [Kubernetes Auth](/documentation/platform/identities/kubernetes-auth) in the near future.
Install `cert-manager` into your Kubernetes cluster by following the instructions [here](https://cert-manager.io/docs/installation/) or by running the following command:
```bash
kubectl apply -f https://github.com/cert-manager/cert-manager/releases/download/v1.15.3/cert-manager.yaml
```
Install the Infisical PKI Issuer controller into your Kubernetes cluster by running the following command:
```bash
kubectl apply -f https://raw.githubusercontent.com/Infisical/infisical-issuer/main/build/install.yaml
```
Start by creating a Kubernetes `Secret` containing the **Client Secret** from step 1. As mentioned previously, this will be used by the Infisical PKI issuer to authenticate with Infisical.
```bash
kubectl create secret generic issuer-infisical-client-secret \
--namespace \
--from-literal=clientSecret=
```
```yaml secret-issuer.yaml
apiVersion: v1
kind: Secret
metadata:
name: issuer-infisical-client-secret
namespace:
data:
clientSecret:
```
```bash
kubectl apply -f secret-issuer.yaml
```
Next, create the Infisical PKI Issuer by filling out `url`, `clientId`, either `caId` or `certificateTemplateId`, and applying the following configuration file for the `Issuer` resource.
This configuration file specifies the connection details to your Infisical PKI CA to be used for issuing certificates.
```yaml infisical-issuer.yaml
apiVersion: infisical-issuer.infisical.com/v1alpha1
kind: Issuer
metadata:
name: issuer-infisical
namespace:
spec:
url: "https://app.infisical.com" # the URL of your Infisical instance
caId: # the ID of the CA you want to use to issue certificates
certificateTemplateId: # the ID of the certificate template you want to use to issue certificates against
authentication:
universalAuth:
clientId: # the Client ID from step 1
secretRef: # reference to the Secret created in step 4
name: "issuer-infisical-client-secret"
key: "clientSecret"
```
```
kubectl apply -f infisical-issuer.yaml
```
The Infisical PKI Issuer supports issuing certificates against a specific CA or a specific certificate template.
For this reason, you should only fill in the `caId` or the `certificateTemplateId` field but not both.
We recommend using the `certificateTemplateId` field to issue certificates against a specific [certificate template](/documentation/platform/pki/certificate-templates)
since templates let you enforce constraints on issued certificates and may have alerting policies bound to them.
You can check that the issuer was created successfully by running the following command:
```bash
kubectl get issuers.infisical-issuer.infisical.com -n -o wide
```
```bash
NAME AGE
issuer-infisical 21h
```
An `Issuer` is a namespaced resource, and it is not possible to issue certificates from an `Issuer` in a different namespace.
This means you will need to create an `Issuer` in each namespace you wish to obtain `Certificates` in.
If you want to create a single `Issuer` that can be consumed in multiple namespaces, you should consider creating a `ClusterIssuer` resource. This is almost identical to the `Issuer` resource, however is non-namespaced so it can be used to issue `Certificates` across all namespaces.
You can read more about the `Issuer` and `ClusterIssuer` resources [here](https://cert-manager.io/docs/configuration/).
Finally, create a `Certificate` by applying the following configuration file.
This configuration file specifies the details of the (end-entity/leaf) certificate to be issued.
```yaml certificate-issuer.yaml
apiVersion: cert-manager.io/v1
kind: Certificate
metadata:
name: certificate-by-issuer
namespace:
spec:
commonName: certificate-by-issuer.example.com # the common name for the certificate
secretName: certificate-by-issuer # the name of the Kubernetes Secret to create and store the certificate and private key in
issuerRef:
name: issuer-infisical
group: infisical-issuer.infisical.com
kind: Issuer
privateKey: # the algorithm and key size to use
algorithm: ECDSA
size: 256
duration: 48h # the ttl for the certificate
renewBefore: 12h # the time before the certificate expiry that the certificate should be automatically renewed
```
The above sample configuration file specifies a certificate to be issued with the common name `certificate-by-issuer.example.com` and ECDSA private key using the P-256 curve, valid for 48 hours; the certificate will be automatically renewed by `cert-manager` 12 hours before expiry.
The certificate is issued by the issuer `issuer-infisical` created in the previous step and the resulting certificate and private key will be stored in a secret named `certificate-by-issuer`.
Note that the full list of the fields supported on the `Certificate` resource can be found in the API reference documentation [here](https://cert-manager.io/docs/reference/api-docs/#cert-manager.io/v1.CertificateSpec).
You can check that the certificate was created successfully by running the following command:
```bash
kubectl get certificates -n -o wide
```
```bash
NAME READY SECRET ISSUER STATUS AGE
certificate-by-issuer True certificate-by-issuer issuer-infisical Certificate is up to date and has not expired 20h
```
Since the actual certificate and private key are stored in a Kubernetes secret, we can check that the secret was created successfully by running the following command:
```bash
kubectl get secret certificate-by-issuer -n
```
```bash
NAME TYPE DATA AGE
certificate-by-issuer kubernetes.io/tls 2 26h
```
We can `describe` the secret to get more information about it:
```bash
kubectl describe secret certificate-by-issuer -n default
```
```bash
Name: certificate-by-issuer
Namespace: default
Labels: controller.cert-manager.io/fao=true
Annotations: cert-manager.io/alt-names:
cert-manager.io/certificate-name: certificate-by-issuer
cert-manager.io/common-name: certificate-by-issuer.example.com
cert-manager.io/ip-sans:
cert-manager.io/issuer-group: infisical-issuer.infisical.com
cert-manager.io/issuer-kind: Issuer
cert-manager.io/issuer-name: issuer-infisical
cert-manager.io/uri-sans:
Type: kubernetes.io/tls
Data
====
ca.crt: 1306 bytes
tls.crt: 2380 bytes
tls.key: 227 bytes
```
Here, `ca.crt` is the Root CA certificate, `tls.crt` is the requested certificate followed by the certificate chain, and `tls.key` is the private key for the certificate.
We can decode the certificate and print it out using `openssl`:
```bash
kubectl get secret certificate-by-issuer -n default -o jsonpath='{.data.tls\.crt}' | base64 --decode | openssl x509 -text -noout
```
In any case, the certificate is ready to be used as Kubernetes Secret by your Kubernetes resources.
## FAQ
The full list of the fields supported on the `Certificate` resource can be found in the API reference documentation [here](https://cert-manager.io/docs/reference/api-docs/#cert-manager.io/v1.CertificateSpec).
Currently, not all fields are supported by the Infisical PKI Issuer.
Yes. `cert-manager` will automatically renew certificates according to the `renewBefore` threshold of expiry as
specified in the corresponding `Certificate` resource.
You can read more about the `renewBefore` field [here](https://cert-manager.io/docs/reference/api-docs/#cert-manager.io/v1.CertificateSpec).
# Private CA
Learn how to create a Private CA hierarchy with Infisical.
## Concept
The first step to creating your Internal PKI is to create a Private Certificate Authority (CA) hierarchy that is a structure of entities
used to issue digital certificates for services, applications, and devices.
```mermaid
graph TD
A[Root CA]
A --> B[Intermediate CA]
A --> C[Intermediate CA]
```
## Workflow
A typical workflow for setting up a Private CA hierarchy consists of the following steps:
1. Configuring an Infisical root CA with details like name, validity period, and path length — This step is optional if you wish to use an external root CA.
2. Configuring and chaining intermediate CA(s) with details like name, validity period, path length, and imported certificate to your Root CA.
3. Managing the CA lifecycle events such as CA succession.
Note that this workflow can be executed via the Infisical UI or manually such
as via API. If manually executing the workflow, you may have to create a
Certificate Signing Request (CSR) for the intermediate CA, create an
intermediate certificate using the root CA private key and CSR, and import the
intermediate certificate back to the intermediate CA as part of Step 2.
## Guide to Creating a CA Hierarchy
In the following steps, we explore how to create a simple Private CA hierarchy
consisting of an (optional) root CA and an intermediate CA.
If you wish to use an external root CA, you can skip this step and head to step 2 to create an intermediate CA.
To create a root CA, head to your Project > Internal PKI > Certificate Authorities and press **Create CA**.
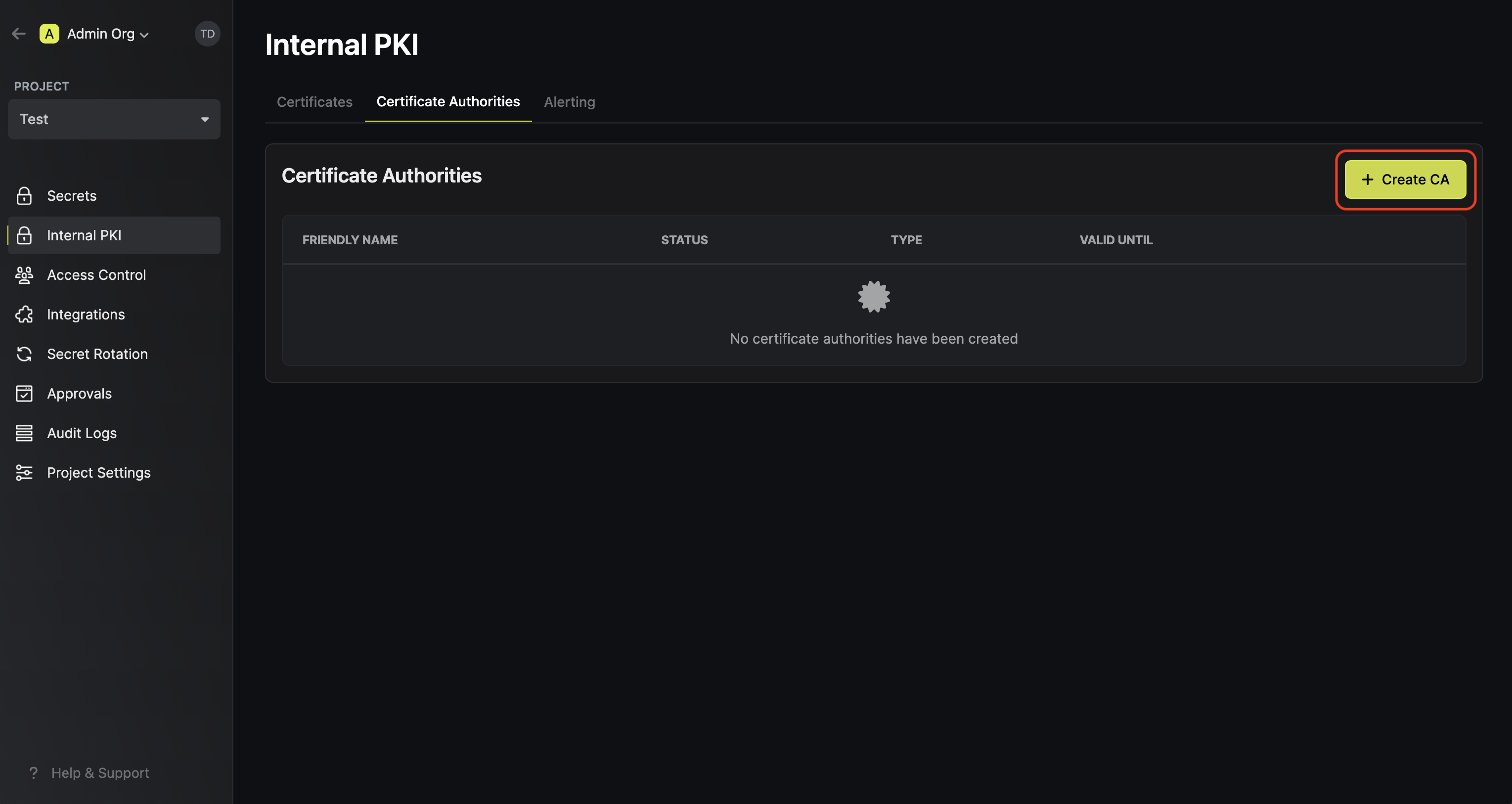
Here, set the **CA Type** to **Root** and fill out details for the root CA.
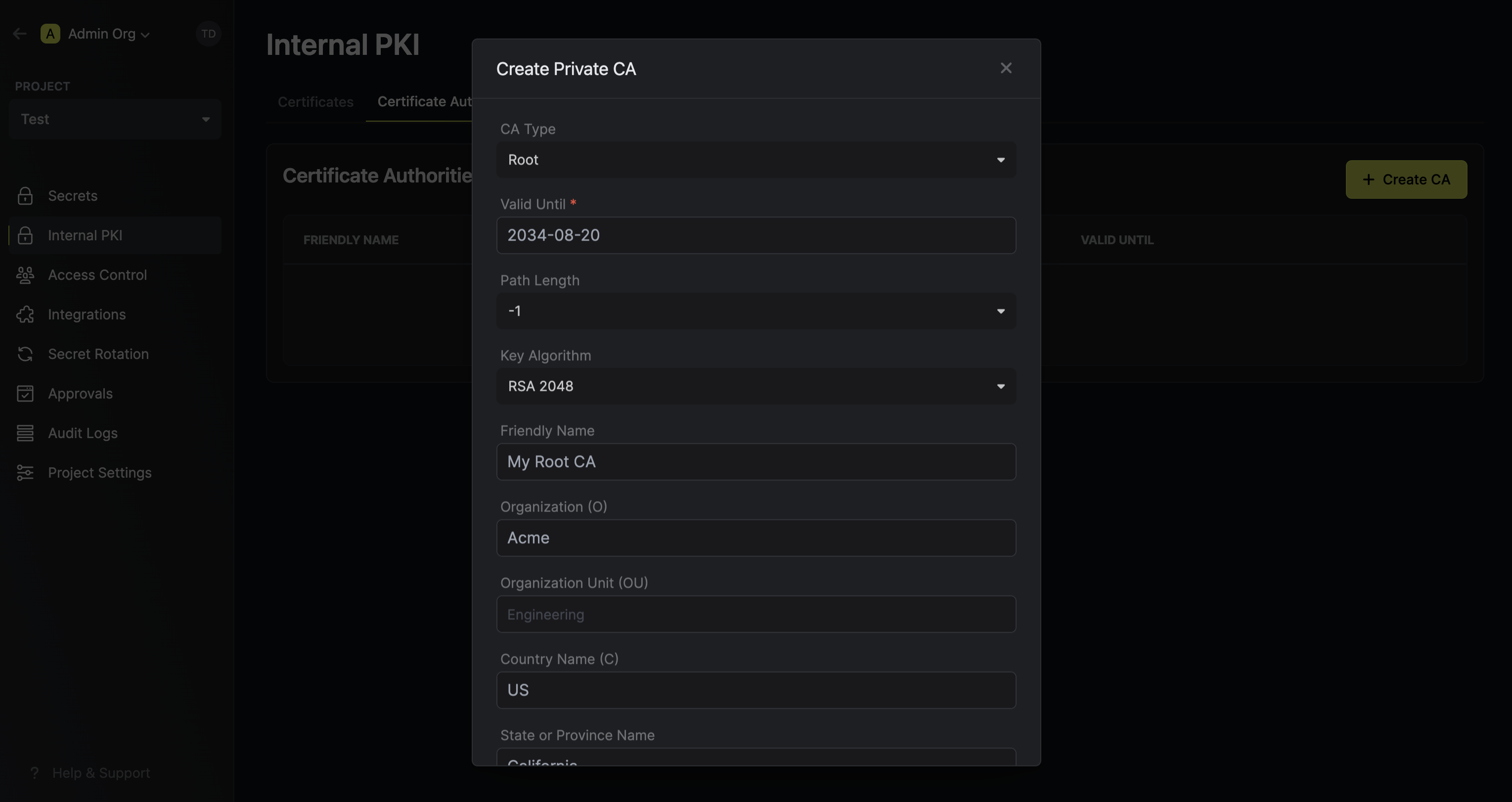
Here's some guidance on each field:
* Valid Until: The date until which the CA is valid in the date time string format specified [here](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Date#date_time_string_format). For example, the following formats would be valid: `YYYY`, `YYYY-MM`, `YYYY-MM-DD`, `YYYY-MM-DDTHH:mm:ss.sssZ`.
* Path Length: The maximum number of intermediate CAs that can be chained to this CA. A path of `-1` implies no limit; a path of `0` implies no intermediate CAs can be chained.
* Key Algorithm: The type of public key algorithm and size, in bits, of the key pair that the CA creates when it issues a certificate. Supported key algorithms are `RSA 2048`, `RSA 4096`, `ECDSA P-256`, and `ECDSA P-384` with the default being `RSA 2048`.
* Friendly Name: A friendly name for the CA; this is only for display and defaults to the subject of the CA if left empty.
* Organization (O): The organization name.
* Country (C): The country code.
* State or Province Name: The state or province.
* Locality Name: The city or locality.
* Common Name: The name of the CA.
* Require Template for Certificate Issuance: Whether or not certificates for this CA can only be issued through certificate templates (recommended).
The Organization, Country, State or Province Name, Locality Name, and Common Name make up the **Distinguished Name (DN)** or **subject** of the CA.
At least one of these fields must be filled out.
2.1. To create an intermediate CA, press **Create CA** again but this time specifying the **CA Type** to be **Intermediate**. Fill out the details for the intermediate CA.
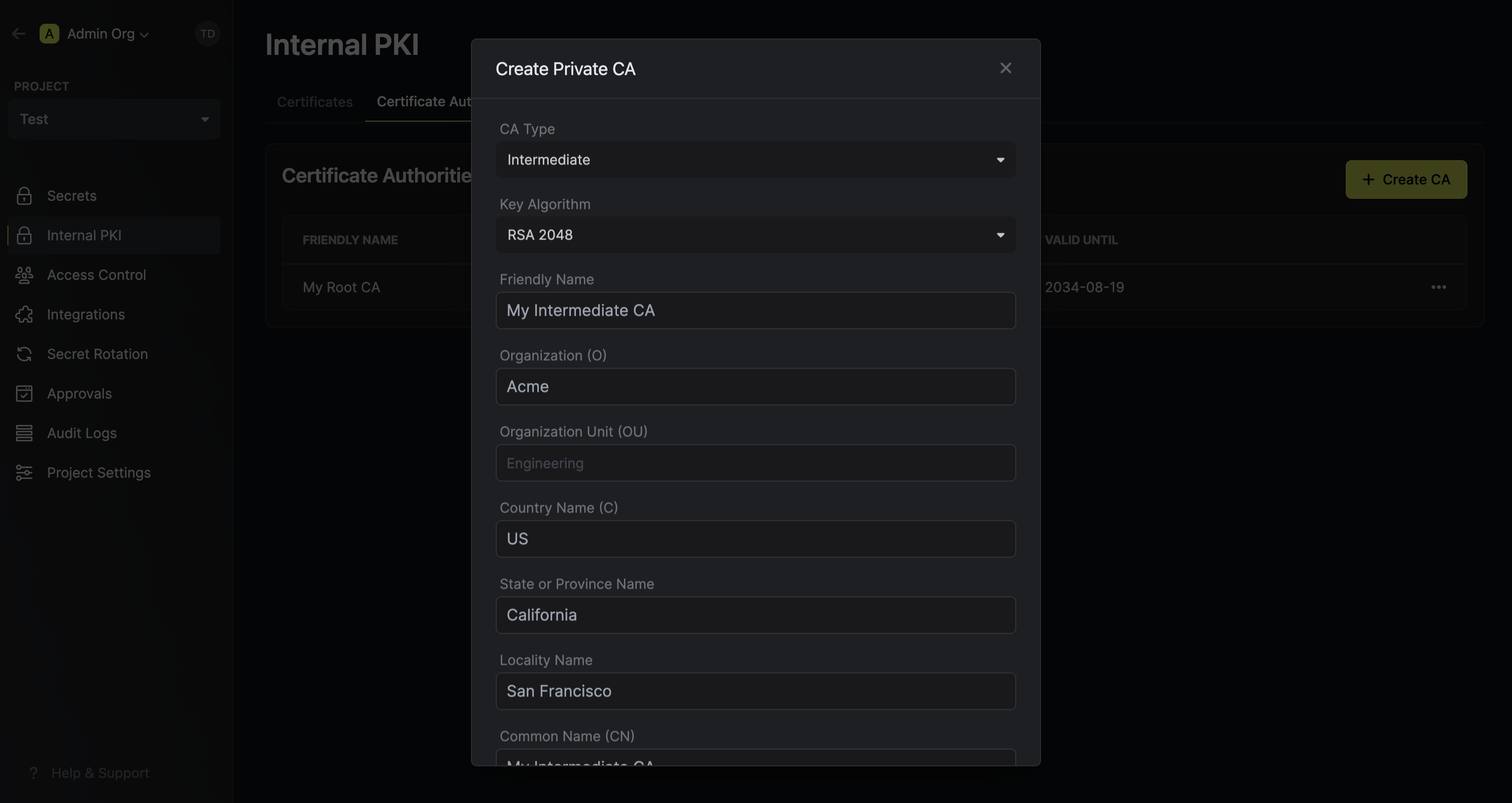
2.2. Next, press the **Install Certificate** option on the intermediate CA from step 1.1.
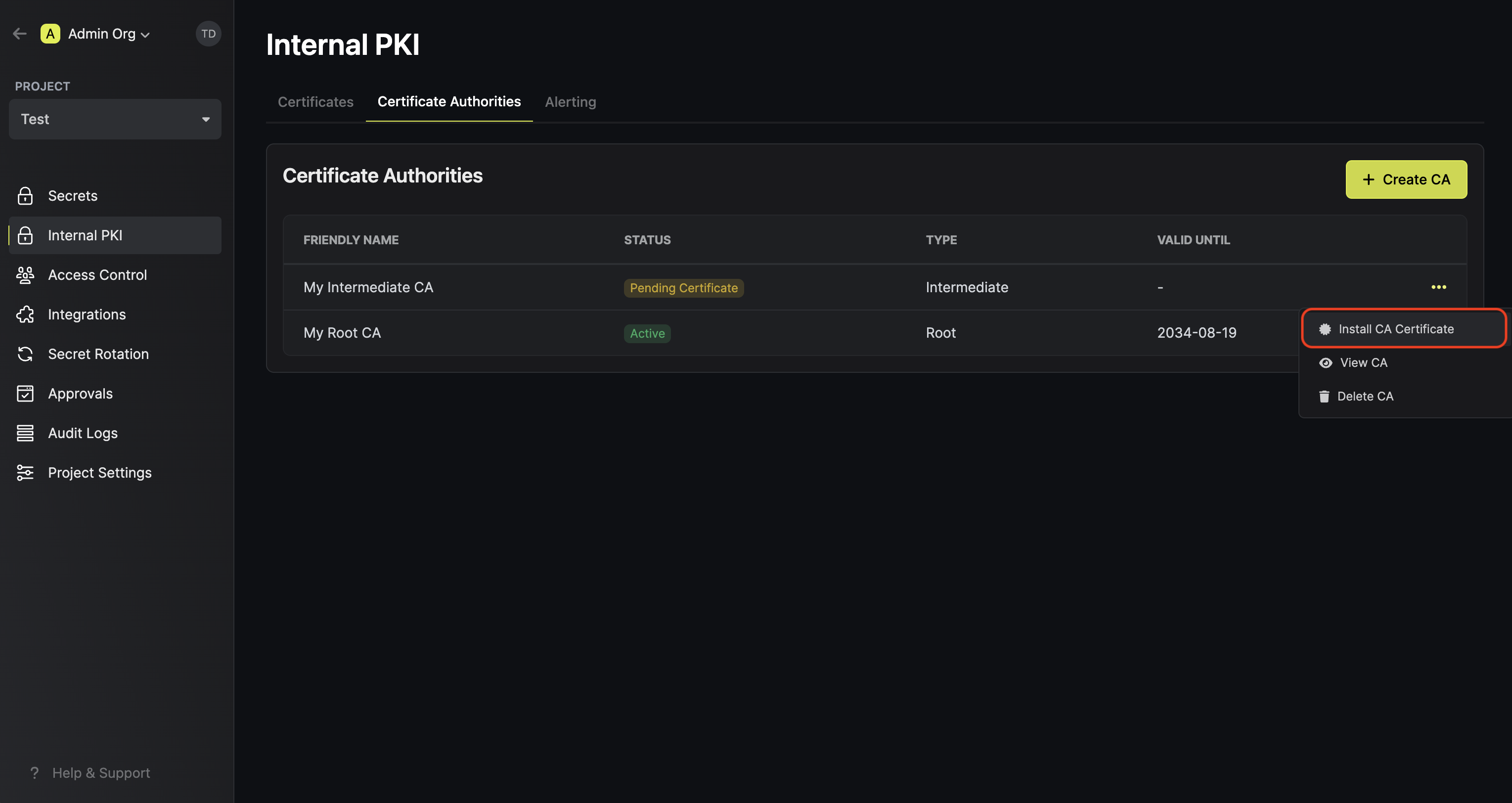
2.3a. If you created a root CA in step 1, select **Infisical CA** for the **Parent CA Type** field.
Next, set the **Parent CA** to the root CA created in step 1 and configure the intended **Valid Until** and **Path Length** fields on the intermediate CA; feel free to use the prefilled values.
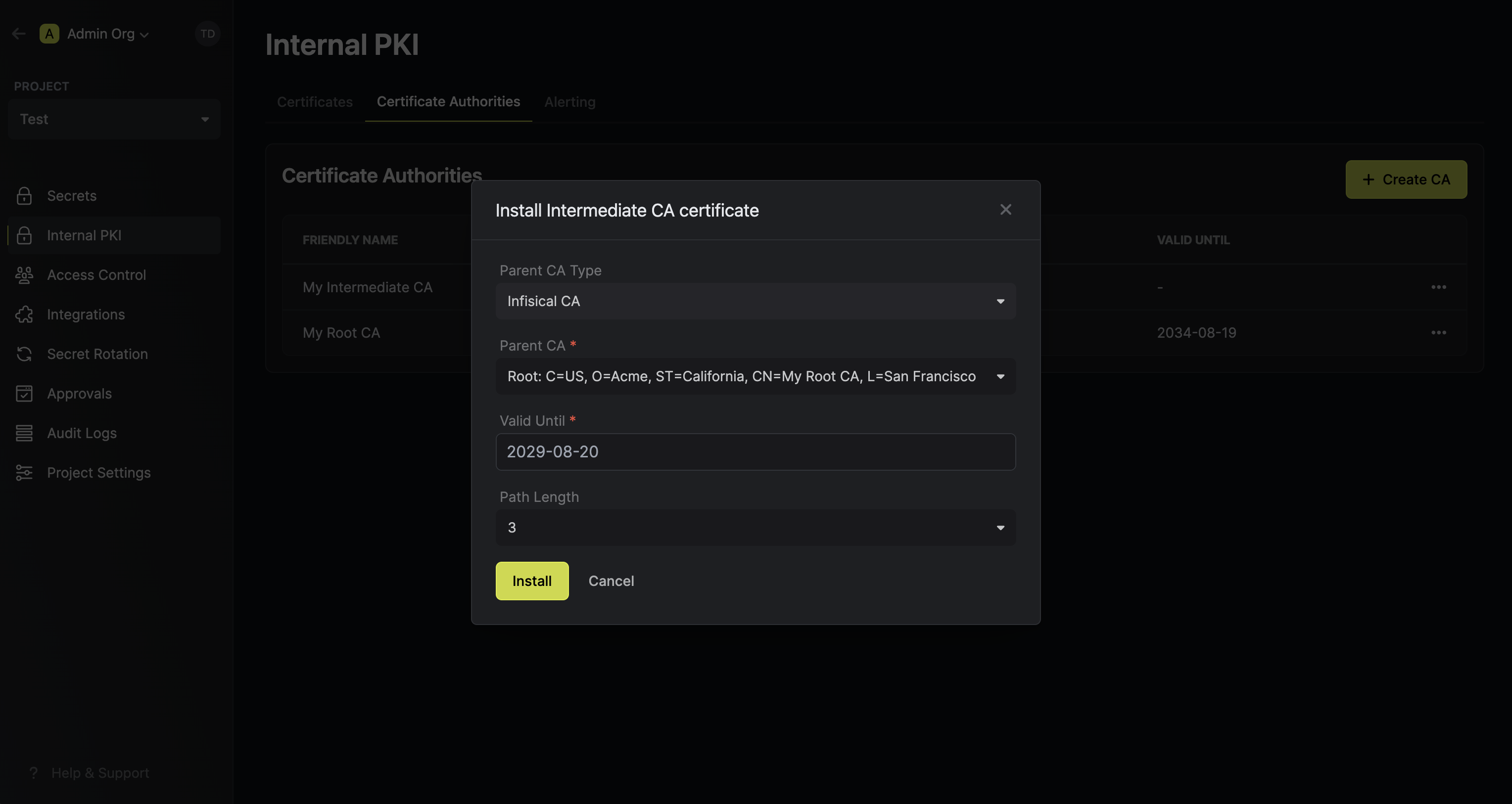
Here's some guidance on each field:
* Parent CA: The parent CA to which this intermediate CA will be chained. In this case, it should be the root CA created in step 1.
* Valid Until: The date until which the CA is valid in the date time string format specified [here](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Date#date_time_string_format). The date must be within the validity period of the parent CA.
* Path Length: The maximum number of intermediate CAs that can be chained to this CA. The path length must be less than the path length of the parent CA.
Finally, press **Install** to chain the intermediate CA to the root CA; this creates a Certificate Signing Request (CSR) for the intermediate CA, creates an intermediate certificate using the root CA private key and CSR, and imports the signed certificate back to the intermediate CA.
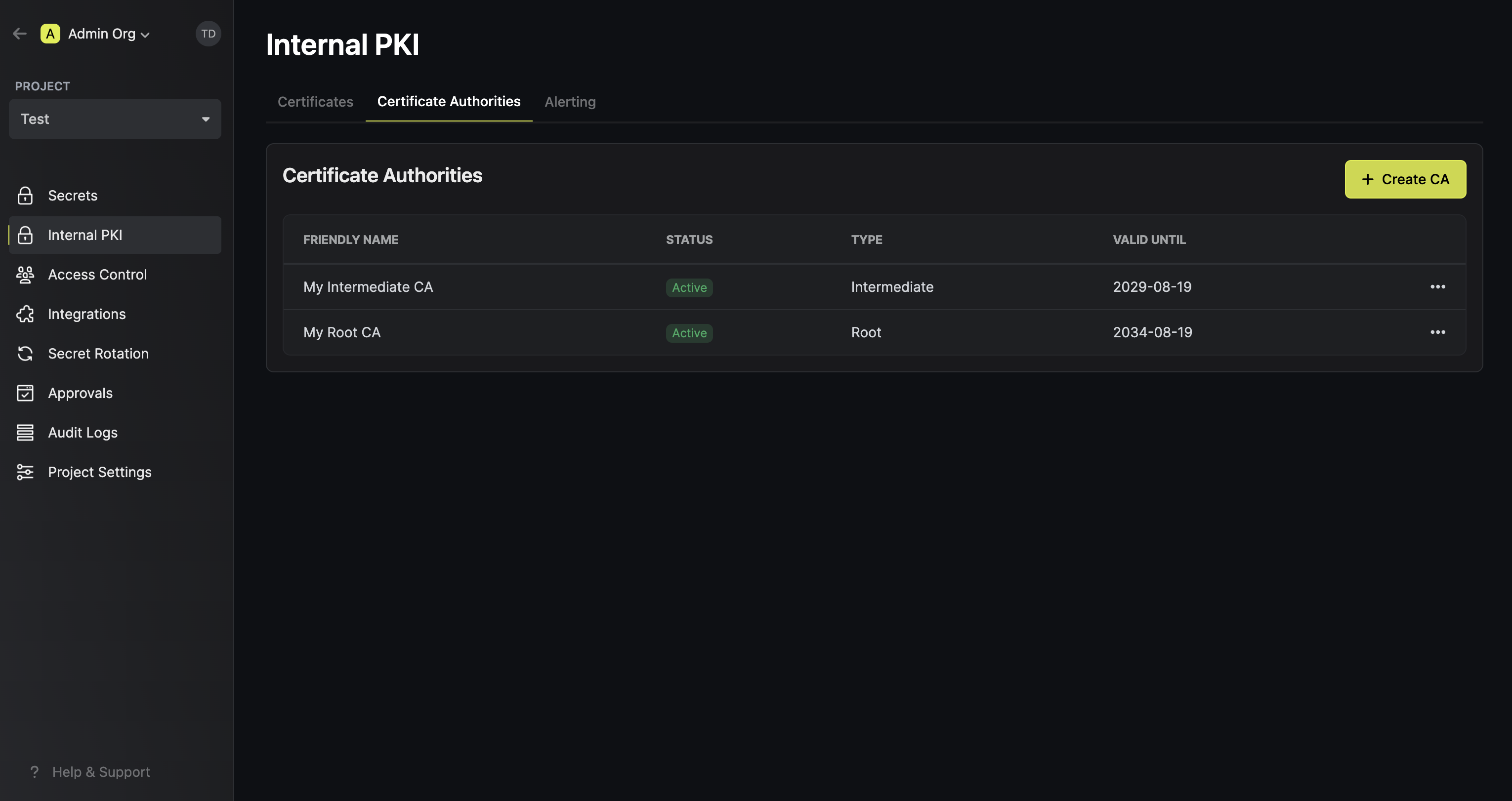
Great! You've successfully created a Private CA hierarchy with a root CA and an intermediate CA.
Now check out the [Certificates](/documentation/platform/pki/certificates) page to learn more about how to issue X.509 certificates using the intermediate CA.
2.3b. If you have an external root CA, select **External CA** for the **Parent CA Type** field.
Next, use the provided intermediate CSR to generate a certificate from your external root CA and paste the PEM-encoded certificate back into the **Certificate Body** field; the PEM-encoded external root CA certificate should be pasted under the **Certificate Chain** field.
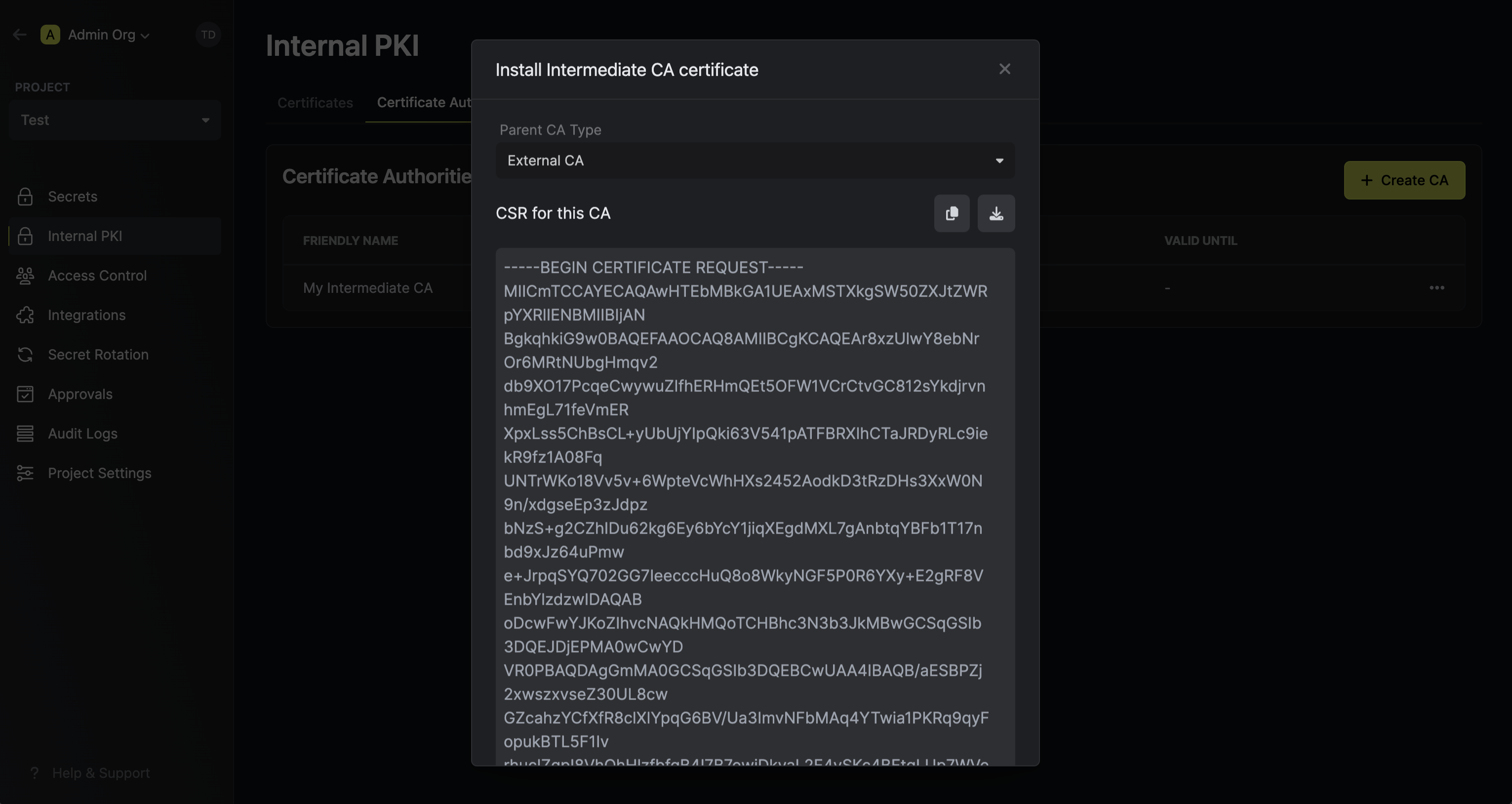
Finally, press **Install** to import the certificate and certificate chain as part of the installation step for the intermediate CA
Great! You've successfully created a Private CA hierarchy with an intermediate CA chained to an external root CA.
Now check out the [Certificates](/documentation/platform/pki/certificates) page to learn more about how to issue X.509 certificates using the intermediate CA.
If you wish to use an external root CA, you can skip this step and head to step 2 to create an intermediate CA.
To create a root CA, make an API request to the [Create CA](/api-reference/endpoints/certificate-authorities/create) API endpoint, specifying the `type` as `root`.
### Sample request
```bash Request
curl --location --request POST 'https://app.infisical.com/api/v1/pki/ca' \
--header 'Authorization: Bearer ' \
--header 'Content-Type: application/json' \
--data-raw '{
"projectSlug": "",
"type": "root",
"commonName": "My Root CA"
}'
```
### Sample response
```bash Response
{
ca: {
id: "",
type: "root",
commonName: "My Root CA",
...
}
}
```
By default, Infisical creates a root CA with the `RSA_2048` key algorithm, validity period of 10 years, with no restrictions on path length;
you may override these defaults by specifying your own options when making the API request.
2.1. To create an intermediate CA, make an API request to the [Create CA](/api-reference/endpoints/certificate-authorities/create) API endpoint, specifying the `type` as `intermediate`.
### Sample request
```bash Request
curl --location --request POST 'https://app.infisical.com/api/v1/pki/ca' \
--header 'Authorization: Bearer ' \
--header 'Content-Type: application/json' \
--data-raw '{
"projectSlug": "",
"type": "intermediate",
"commonName": "My Intermediate CA"
}'
```
### Sample response
```bash Response
{
ca: {
id: "",
type: "intermediate",
commonName: "My Intermediate CA",
...
}
}
```
2.2. Next, get a certificate signing request from the intermediate CA by making an API request to the [Get CSR](/api-reference/endpoints/certificate-authorities/csr) API endpoint.
### Sample request
```bash Request
curl --location --request GET 'https://app.infisical.com/api/v1/pki/ca//csr' \
--header 'Authorization: Bearer ' \
--data-raw ''
```
### Sample response
```bash Response
{
csr: "..."
}
```
If using an external root CA, then use the CSR to generate a certificate for the intermediate CA using your external root CA and skip to step 2.4.
2.3. Next, create an intermediate certificate by making an API request to the [Sign Intermediate](/api-reference/endpoints/certificate-authorities/sign-intermediate) API endpoint
containing the CSR from step 2.2, referencing the root CA created in step 1.
### Sample request
```bash Request
curl --location --request POST 'https://app.infisical.com/api/v1/pki/ca//sign-intermediate' \
--header 'Content-Type: application/json' \
--data-raw '{
"csr": "",
"notAfter": "2029-06-12"
}'
```
### Sample response
```bash Response
{
certificate: "...",
certificateChain: "...",
issuingCaCertificate: "...",
serialNumber: "...",
}
```
The `notAfter` value must be within the validity period of the root CA that is if the root CA is valid until `2029-06-12`, the intermediate CA must be valid until a date before `2029-06-12`.
2.4. Finally, import the intermediate certificate and certificate chain from step 2.3 back to the intermediate CA by making an API request to the [Import Certificate](/api-reference/endpoints/certificate-authorities/import-cert) API endpoint.
If using an external root CA, then import the generated certificate and root CA certificate under certificate chain back into the intermediate CA.
### Sample request
```bash Request
curl --location --request POST 'https://app.infisical.com/api/v1/pki/ca//import-certificate' \
--header 'Authorization: Bearer ' \
--header 'Content-Type: application/json' \
--data-raw '{
"certificate": "",
"certificateChain": ""
}'
```
### Sample response
```bash Response
{
message: "Successfully imported certificate to CA",
caId: "..."
}
```
Great! You’ve successfully created a Private CA hierarchy with a root CA and an intermediate CA. Now check out the Certificates page to learn more about how to issue X.509 certificates using the intermediate CA.
## Guide to CA Renewal
In the following steps, we explore how to renew a CA certificate.
If renewing an intermediate CA chained to an Infisical CA, then Infisical will
automate the process of generating a new certificate for the intermediate CA for you.
If renewing an intermediate CA chained to an external parent CA, you'll be
required to generate a new certificate from the external parent CA and manually import
the certificate back to the intermediate CA.
Head to the CA Page of the CA you wish you renew and press **Renew CA** on
the left side. 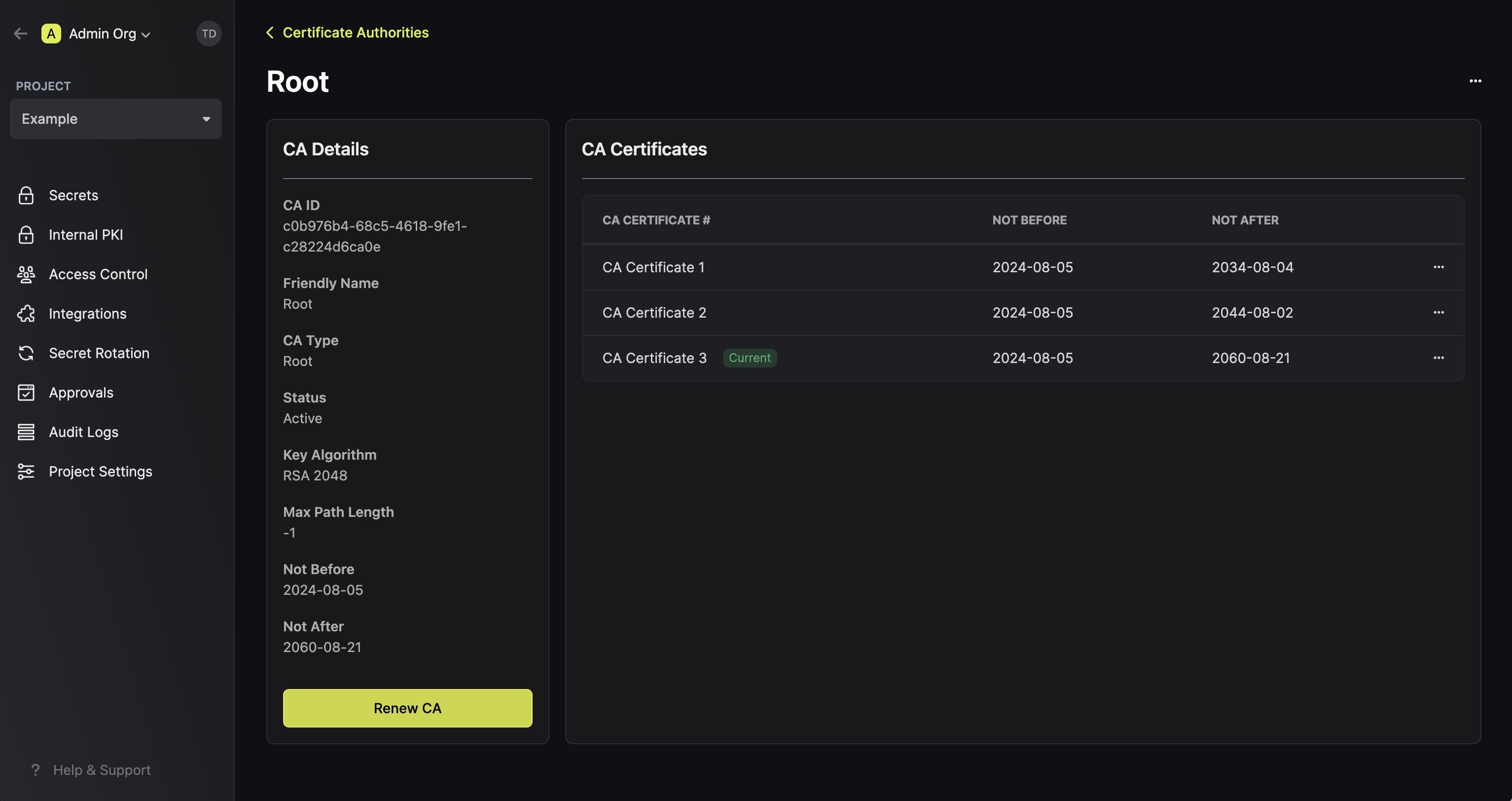 Input a new **Valid Until**
date to be used for the renewed CA certificate and press **Renew** to renew
the CA. 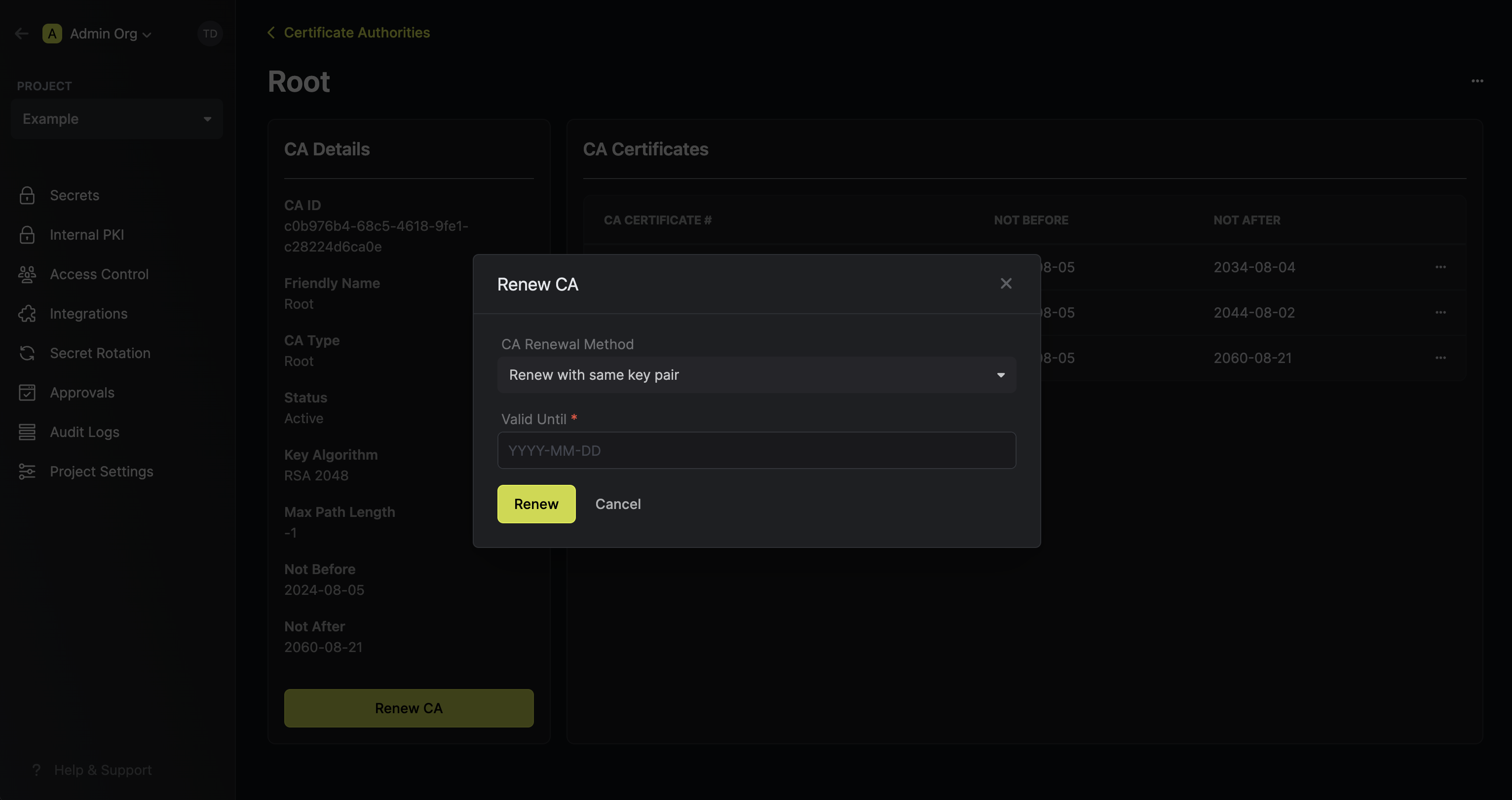
The new **Valid Until** date must be within the validity period of the
parent CA.
To renew a CA certificate, make an API request to the [Renew CA](/api-reference/endpoints/certificate-authorities/renew) API endpoint, specifying the new `notAfter` date for the CA.
### Sample request
```bash Request
curl --location --request POST 'https://app.infisical.com/api/v1/pki/ca//renew' \
--header 'Authorization: Bearer ' \
--header 'Content-Type: application/json' \
--data-raw '{
"type": "existing",
"notAfter": "2029-06-12"
}'
```
### Sample response
```bash Response
{
certificate: "...",
certificateChain: "...",
serialNumber: "..."
}
```
## FAQ
Infisical supports `RSA 2048`, `RSA 4096`, `ECDSA P-256`, `ECDSA P-384` key
algorithms specified at the time of creating a CA.
At the moment, Infisical only supports CA renewal via same key pair. We
anticipate supporting CA renewal via new key pair in the coming month.
Yes. You may obtain a CSR from the Intermediate CA and use it to generate a
certificate from your external CA. The certificate, along with the external
CA certificate chain, can be imported back to the Intermediate CA as part of
the CA installation step.
# Approval Workflows
Learn how to enable a set of policies to manage changes to sensitive secrets and environments.
## Problem at hand
Updating secrets in high-stakes environments (e.g., production) can have a number of problematic issues:
* Most developers should not have access to secrets in production environments. Yet, they are the ones who often need to add new secrets or change the existing ones. Many organizations have in-house policies with regards to what person should be contacted in the case of needing to make changes to secrets. This slows down software development lifecycle and distracts engineers from working on things that matter the most.
* As a general rule, before making changes in production environments, those changes have to be looked over by at least another person. An extra pair of eyes can help reduce the risk of human error and make sure that the change will not affect the application in an unintended way.
* After making updates to secrets, the corresponding applications need to be redeployed with the right set of secrets and configurations. This process is often not automated and hence prone to human error.
## Solution
As a wide-spread software engineering practice, developers have to submit their code as a PR that needs to be approved before the code is merged into the main branch.
In a similar way, to solve the above-mentioned issues, Infisical provides a feature called `Approval Workflows` for secret management. This is a set of policies and workflows that help advance access controls, compliance procedures, and stability of a particular environment. In other words, **Approval Workflows** help you secure, stabilize, and streamline the change of secrets in high-stakes environments.
### Setting a policy
First, you would need to create a set of policies for a certain environment. In the example below, a generic change policy for a production environment is shown. In this case, any user who submits a change to `prod` would first have to get an approval by a predefined approver (or multiple approvers).
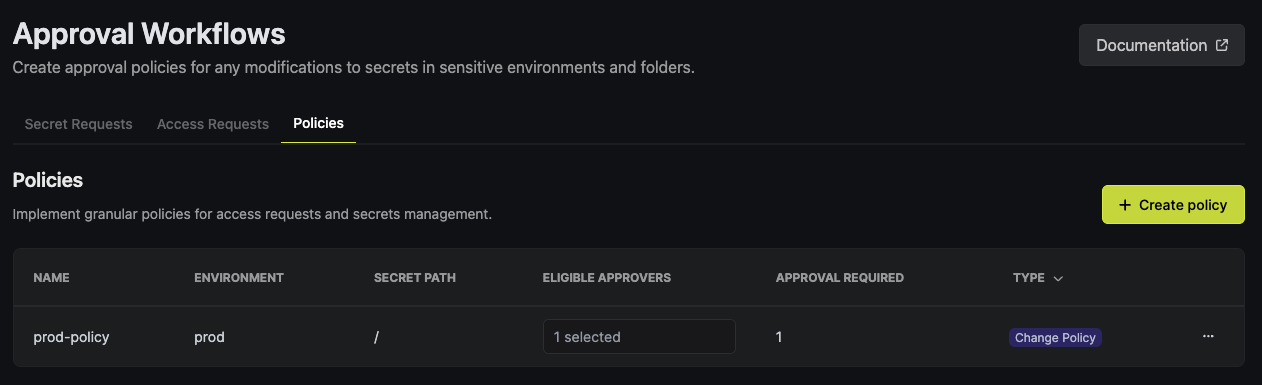
### Policy enforcement levels
The enforcement level determines how strict the policy is. A **Hard** enforcement level means that any change that matches the policy will need full approval prior merging. A **Soft** enforcement level allows for break glass functionality on the request. If a change request is bypassed, the approvers will be notified via email.
### Example of creating a change policy
When creating a policy, you can choose the type of policy you want to create. In this case, we will be creating a `Change Policy`. Other types of policies include `Access Policy` that creates policies for **[Access Requests](/documentation/platform/access-controls/access-requests)**.
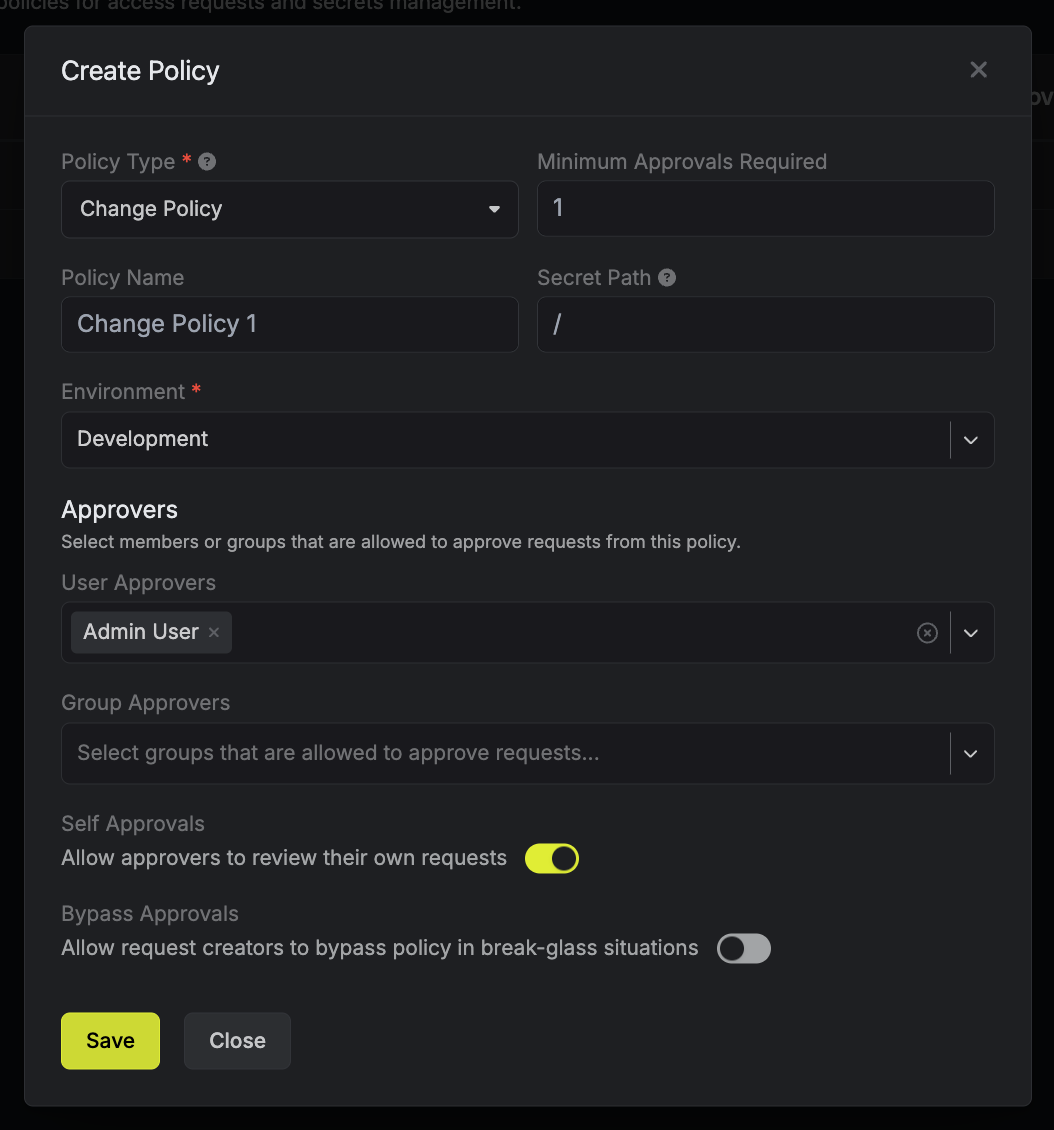
### Example of updating secrets with Approval workflows
When a user submits a change to an enviropnment that is under a particular policy, a corresponsing change request will go to a predefined approver (or multiple approvers).
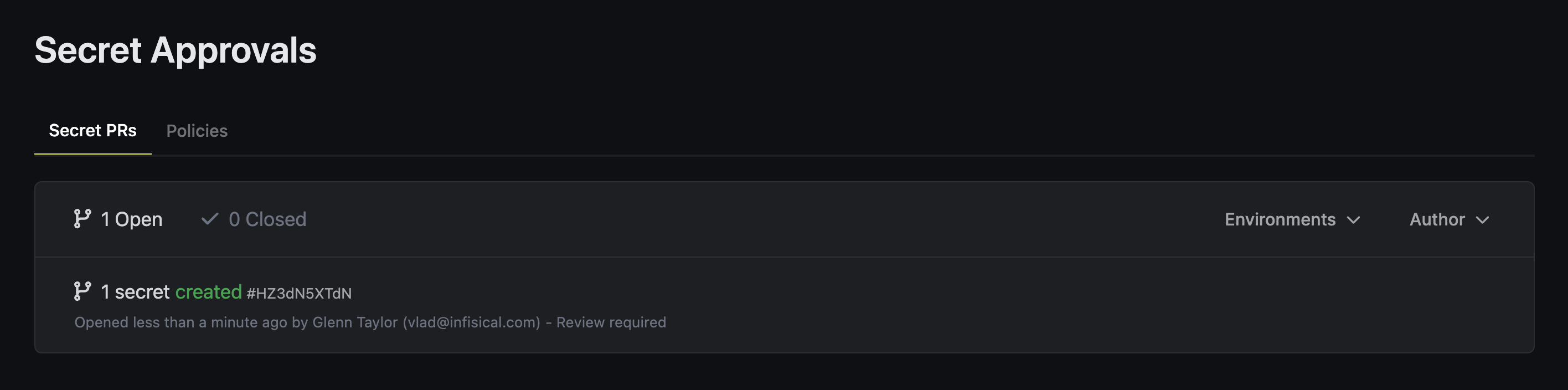
Approvers are notified by email and/or Slack as soon as the request is initiated. In the Infisical Dashboard, they will be able to `approve` and `merge` (or `deny`) a request for a change in a particular environment. After that, depending on the workflows setup, the change will be automatically propagated to the right applications (e.g., using [Infisical Kubernetes Operator](https://infisical.com/docs/integrations/platforms/kubernetes)).
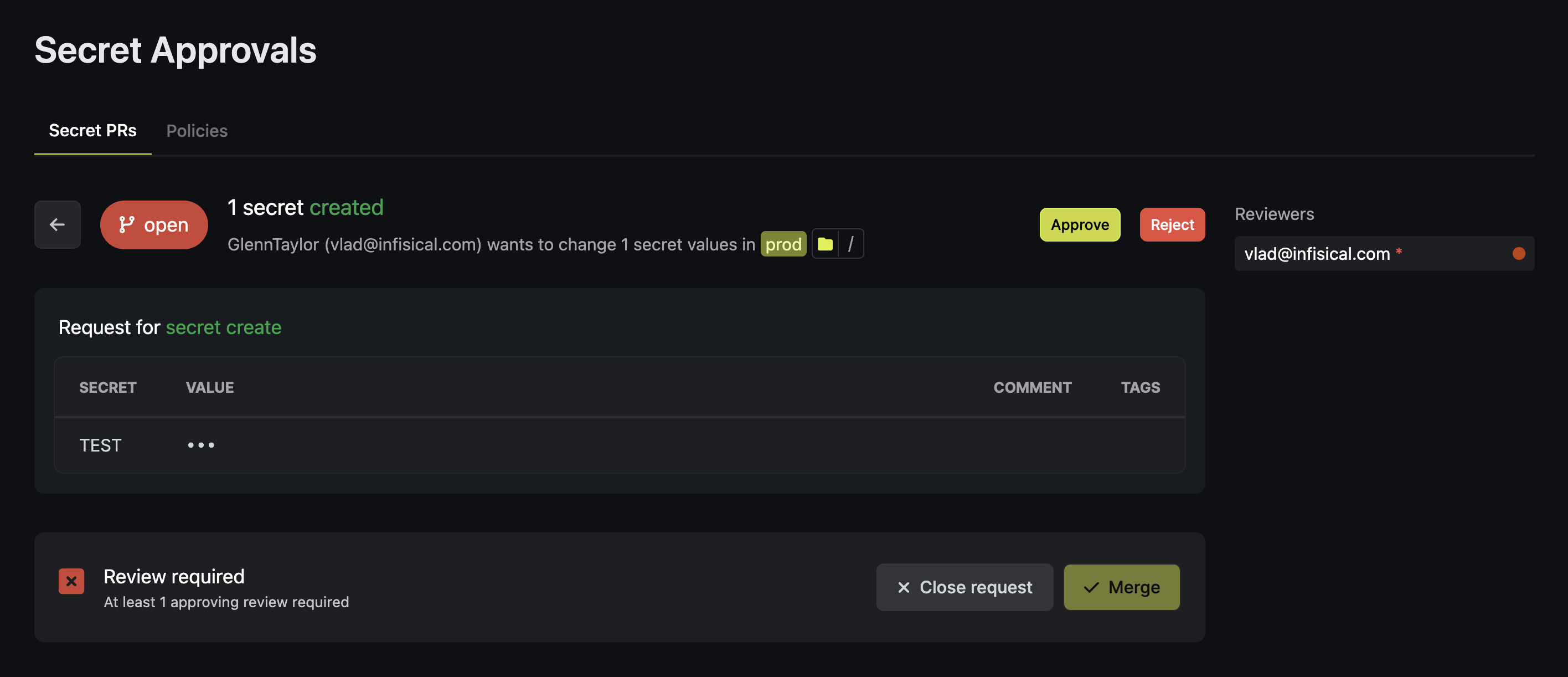
# Projects
Learn more and understand the concept of Infisical projects.
A project in Infisical belongs to an [organization](./organization) and contains a number of environments, folders, and secrets.
Only users and machine identities who belong to a project can access resources inside of it according to predefined permissions.
## Project environments
For both visual and organizational structure, Infisical allows splitting up secrets into environments (e.g., development, staging, production). In project settings, such environments can be
customized depending on the intended use case.
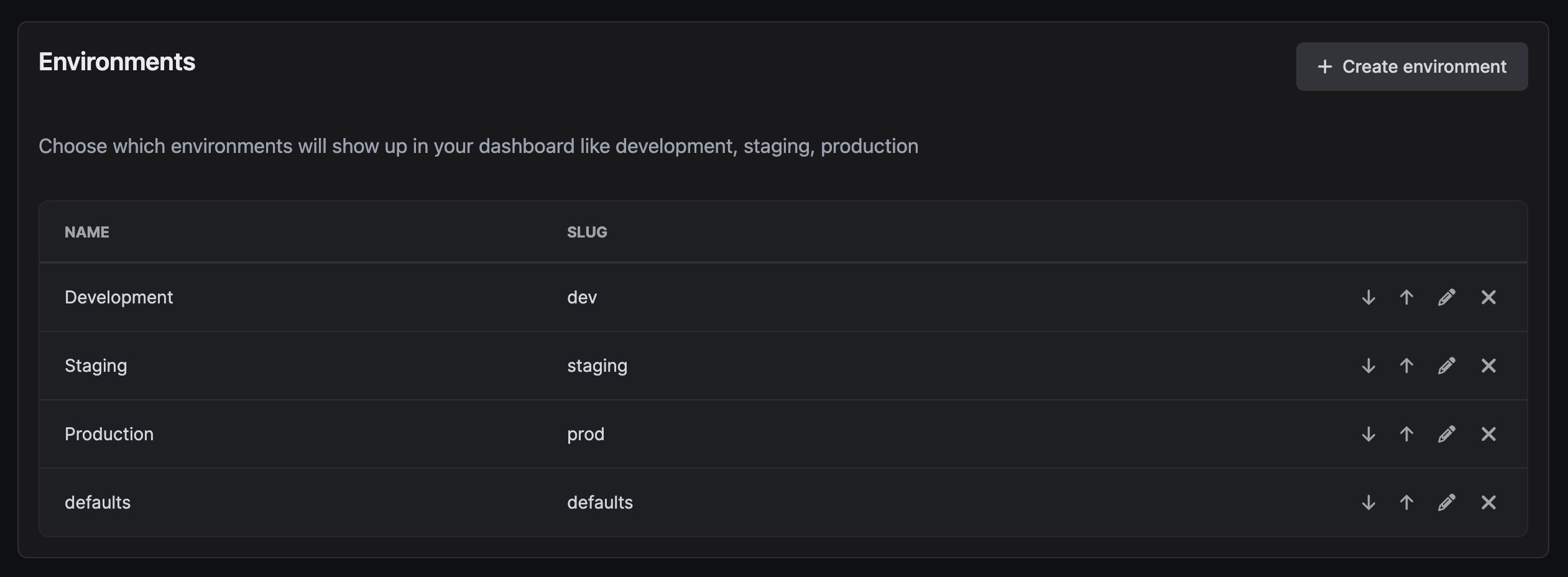
## Secrets Overview
The **Secrets Overview** page captures a birds-eye-view of secrets and [folders](./folder) across environments.
This is useful for comparing secrets, identifying if anything is missing, and making quick changes.
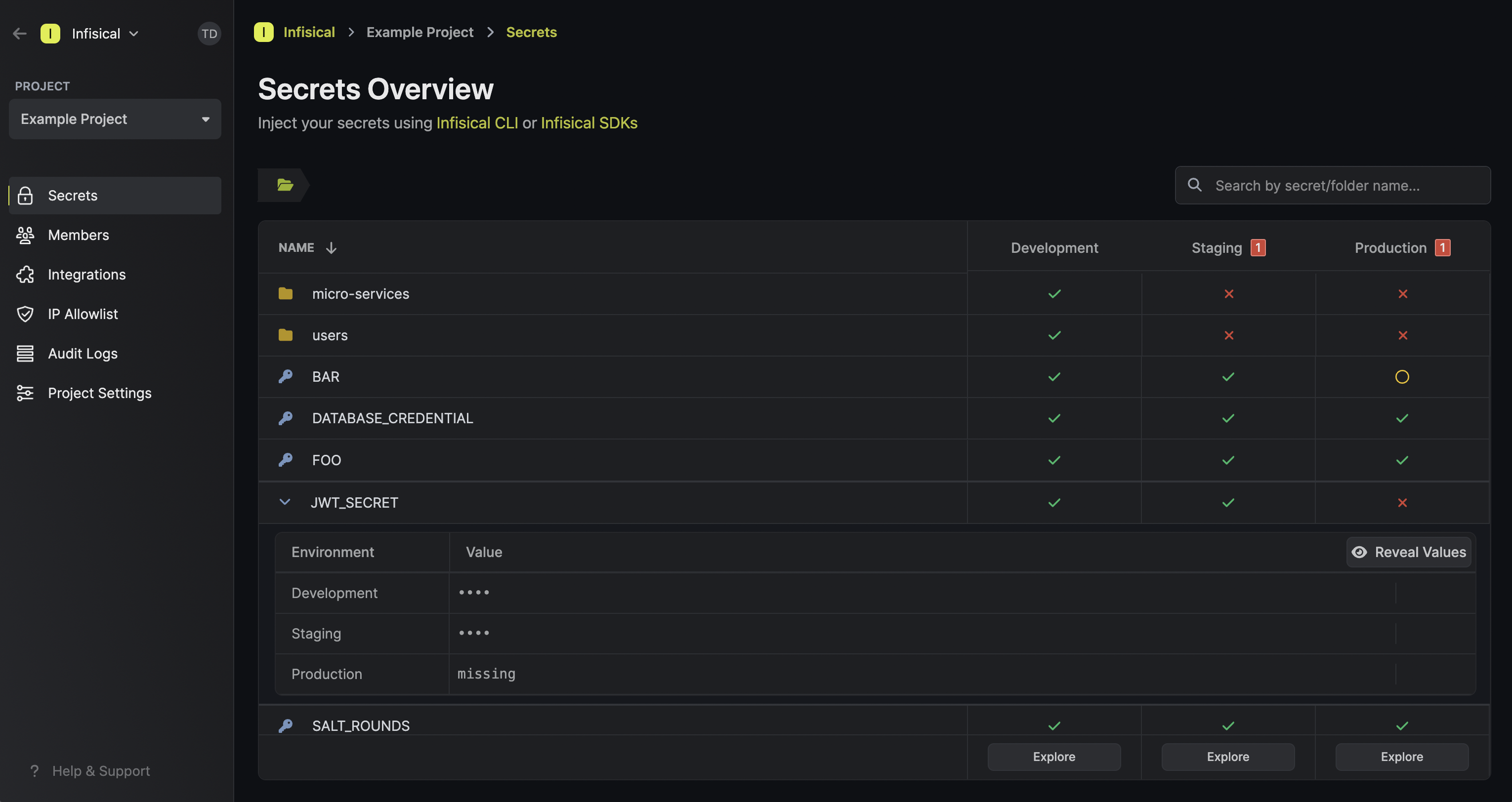
## Secrets Dashboard
The **Secrets Dashboard** page appears when you press to manage the secrets of a specific environment.
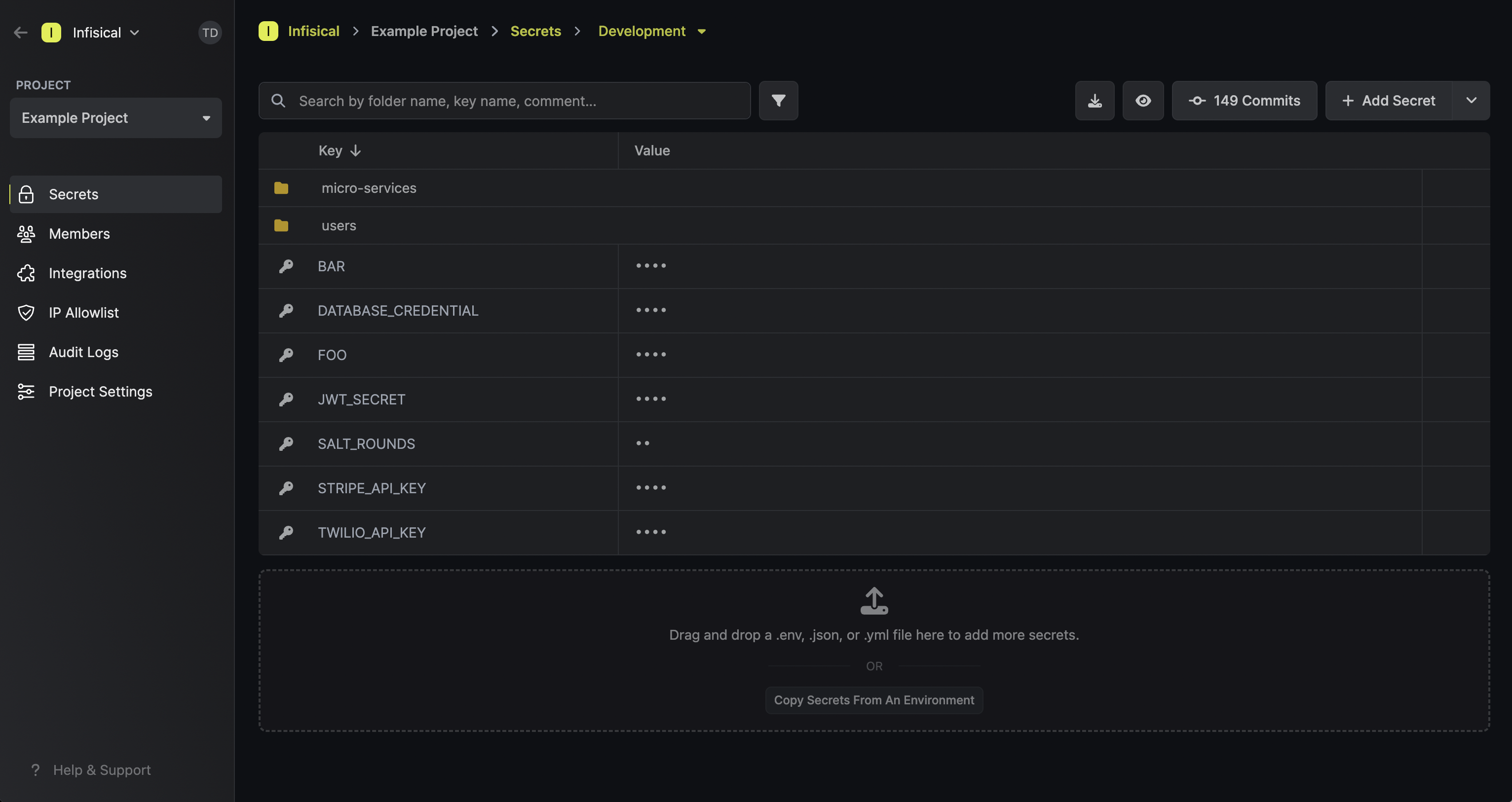
### Secrets
To add a secret, press **Add Secret** button at the top of the dashboard.
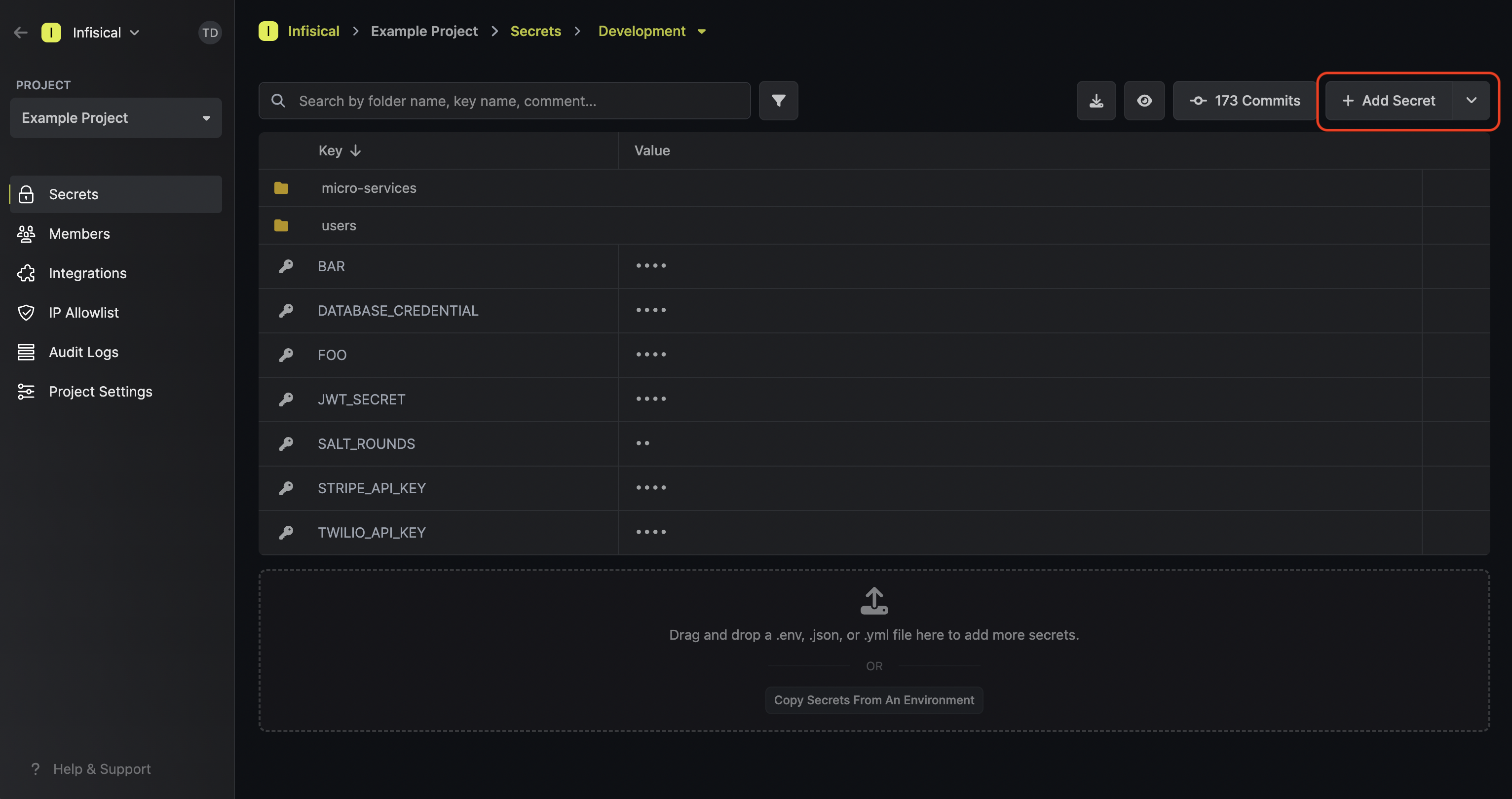
For a new project, it can be convenient to populate the dashboard by dropping a `.env` file into the provided pane as shown below:
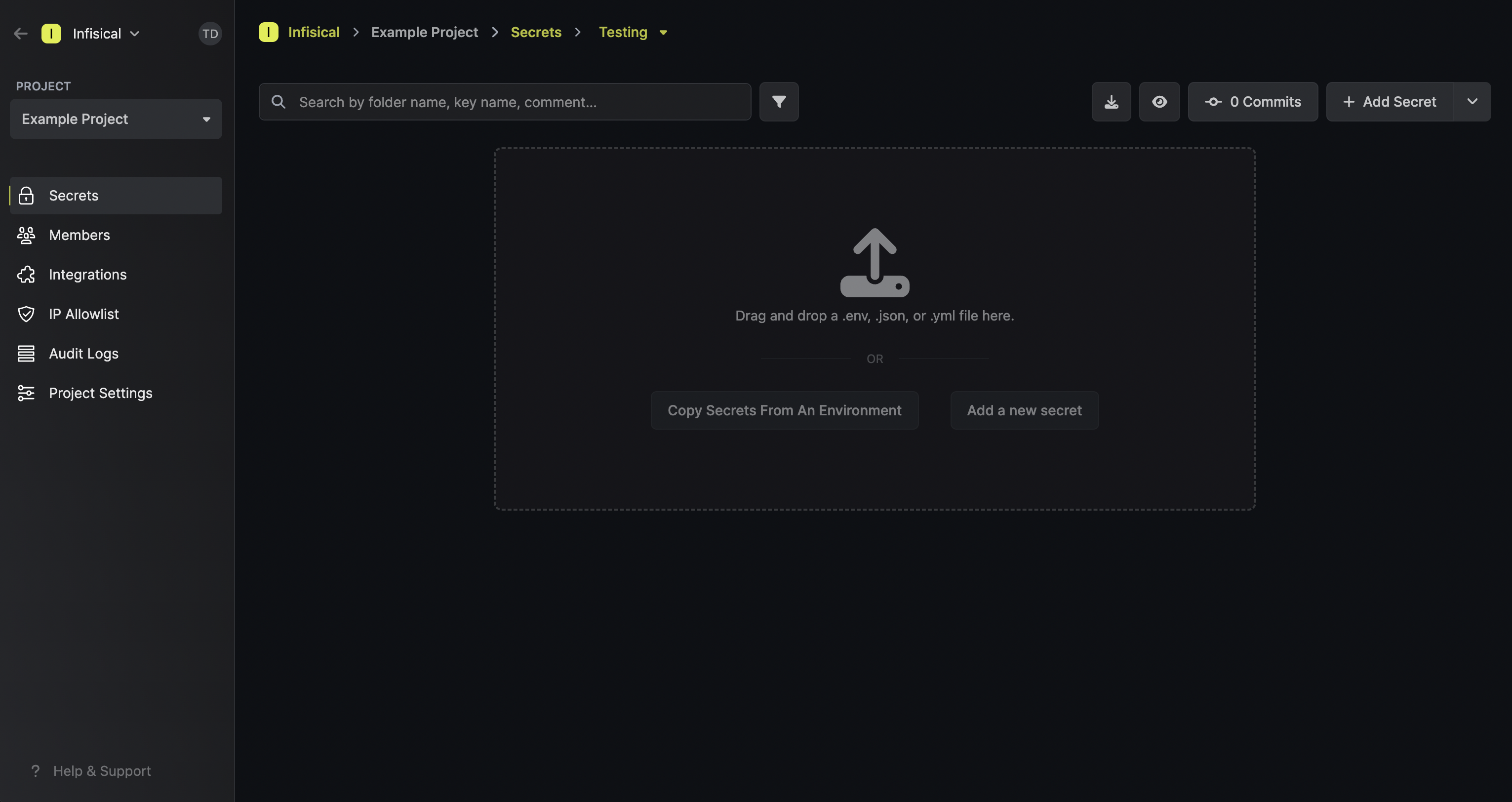
To delete a secret, hover over it and press the **X** button that appears on the right side.
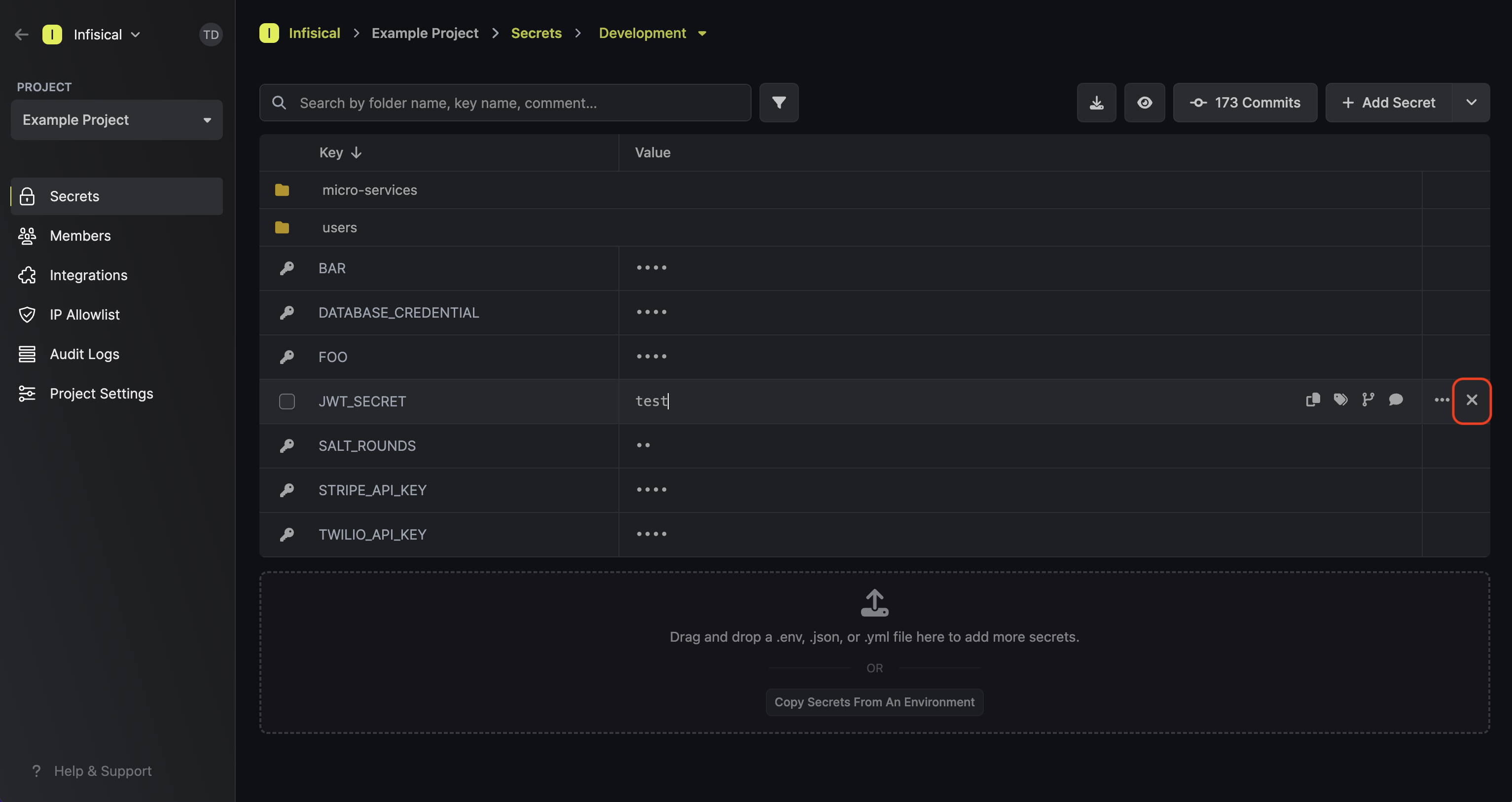
To delete multiple secrets at once, hover over and select the secrets you'd like to delete
and press the **Delete** button that appears at the top.
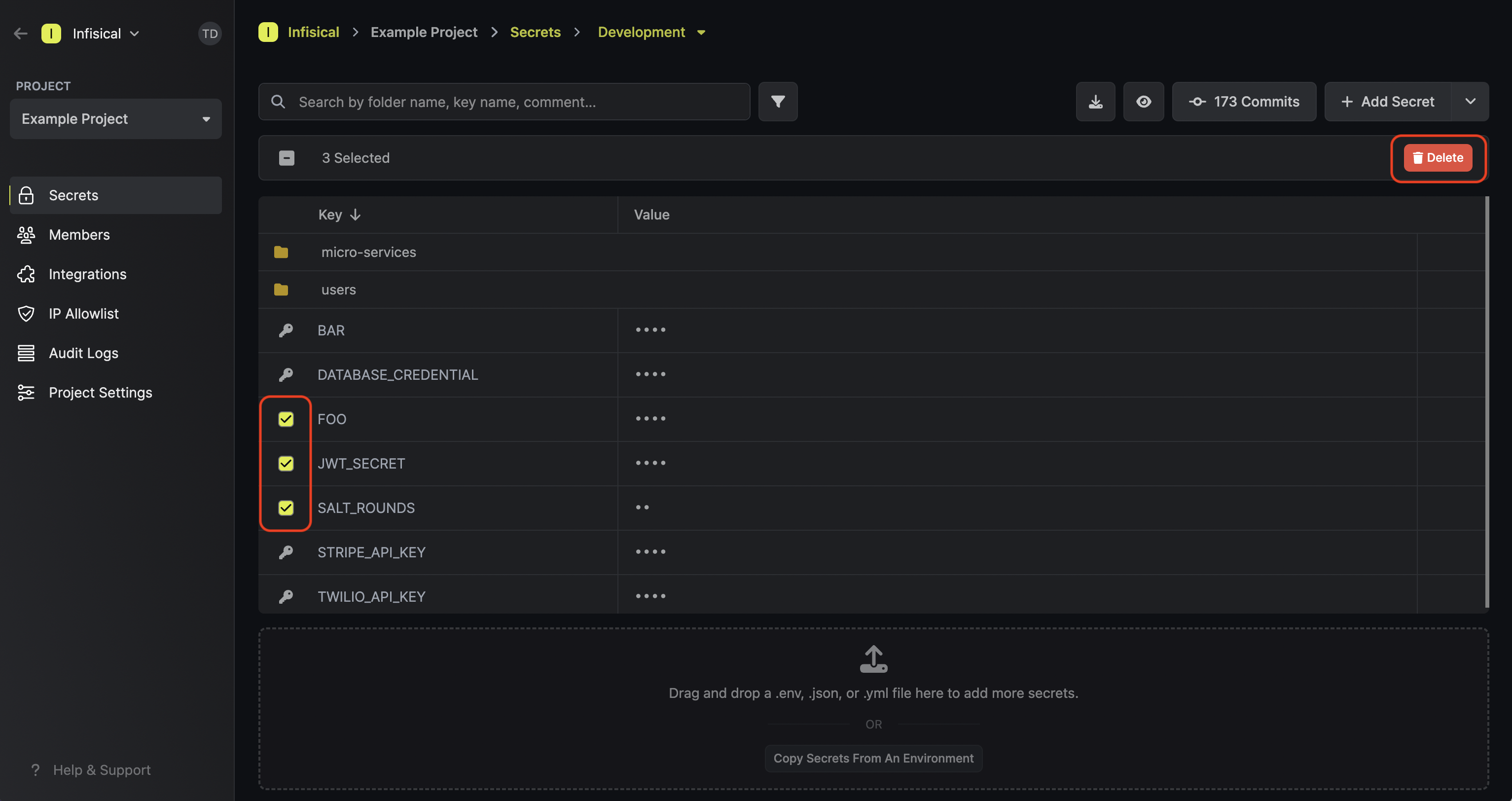
### Search
To search for specific secrets by their key name, you can use the search bar.
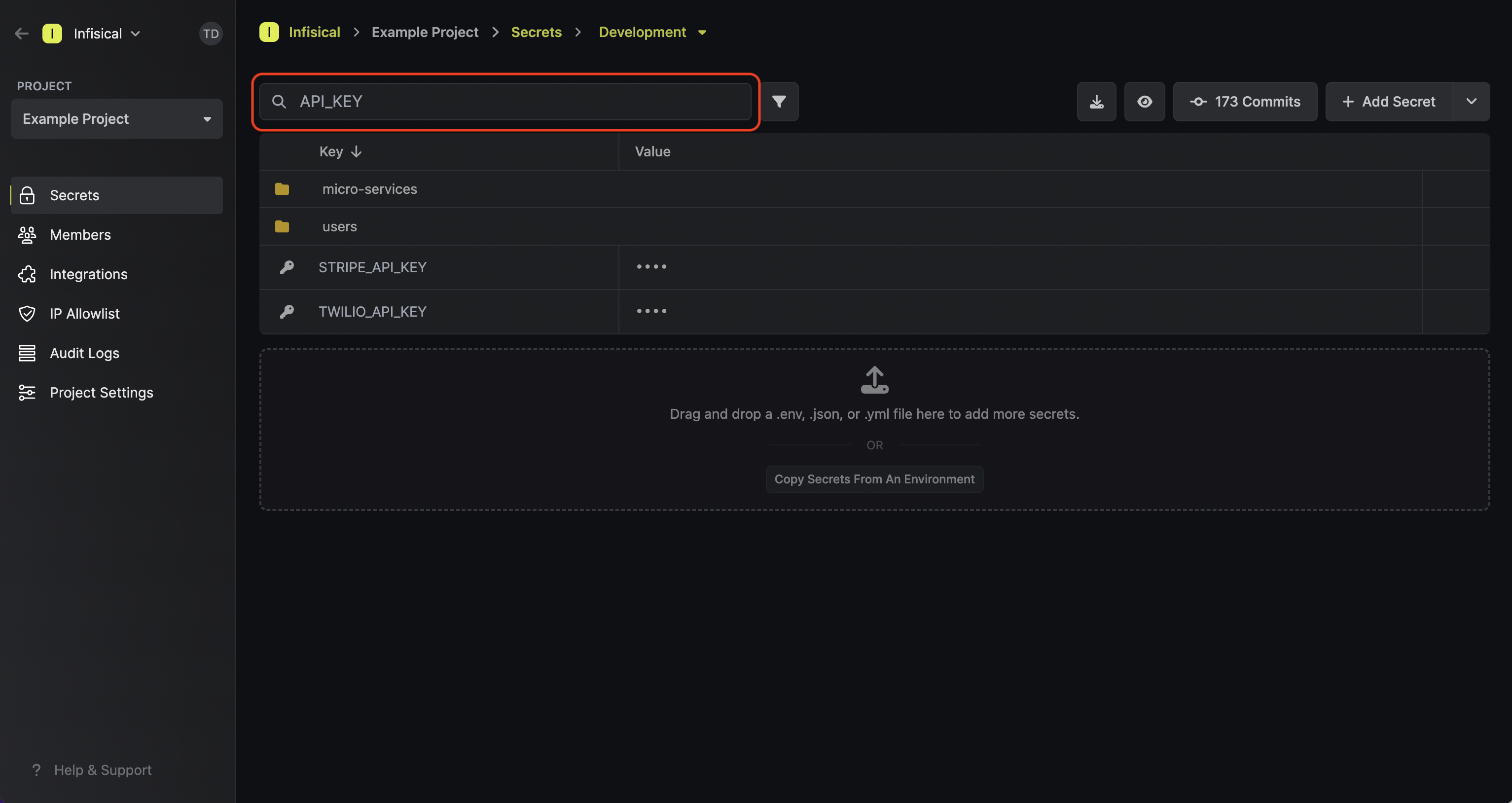
To assist you with finding secrets, you can also group them by similar prefixes and filter them by tags (if applicable).
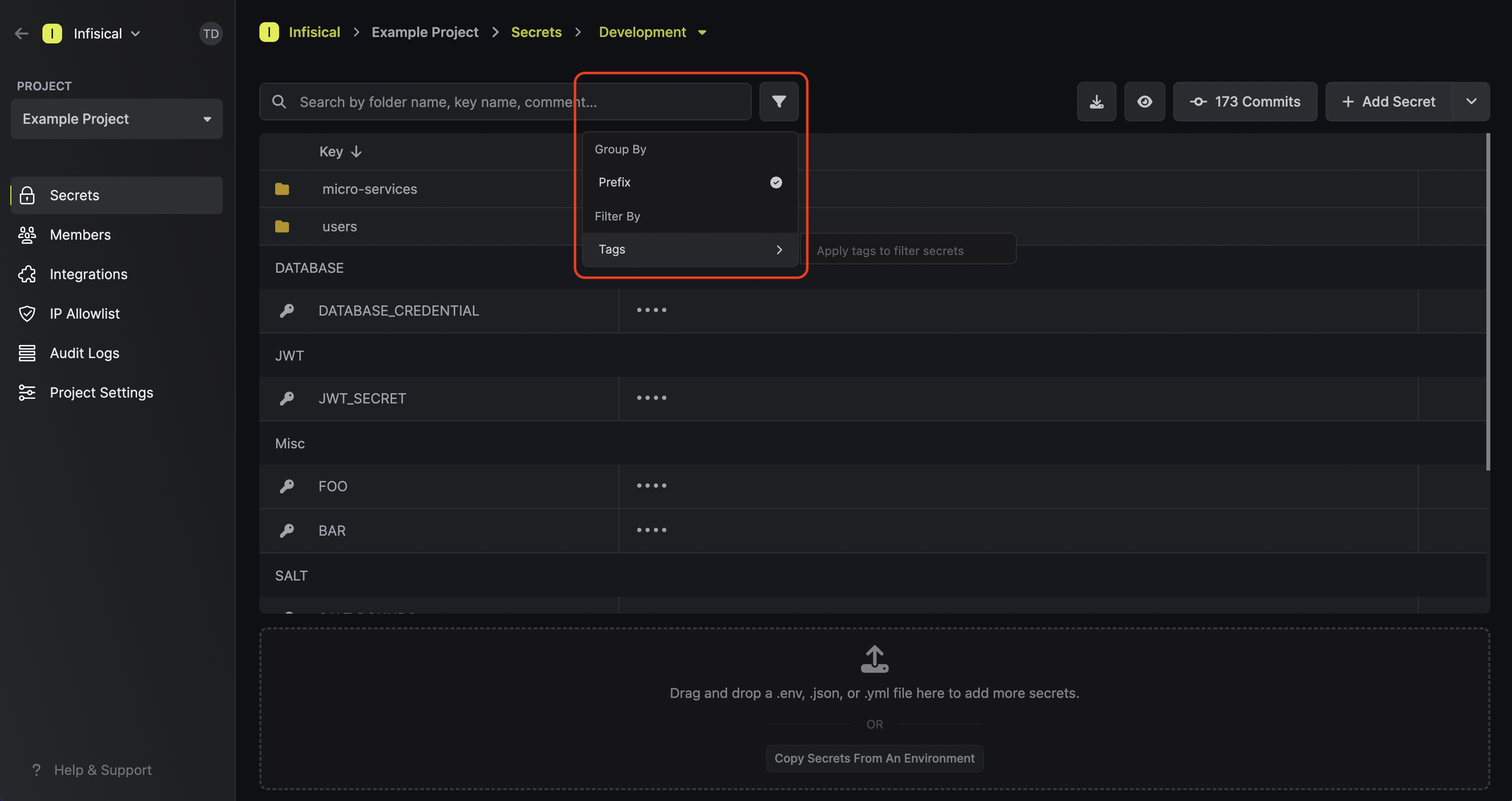
### Hide/Un-hide
To view/hide all secrets at once, toggle the hide or un-hide button.
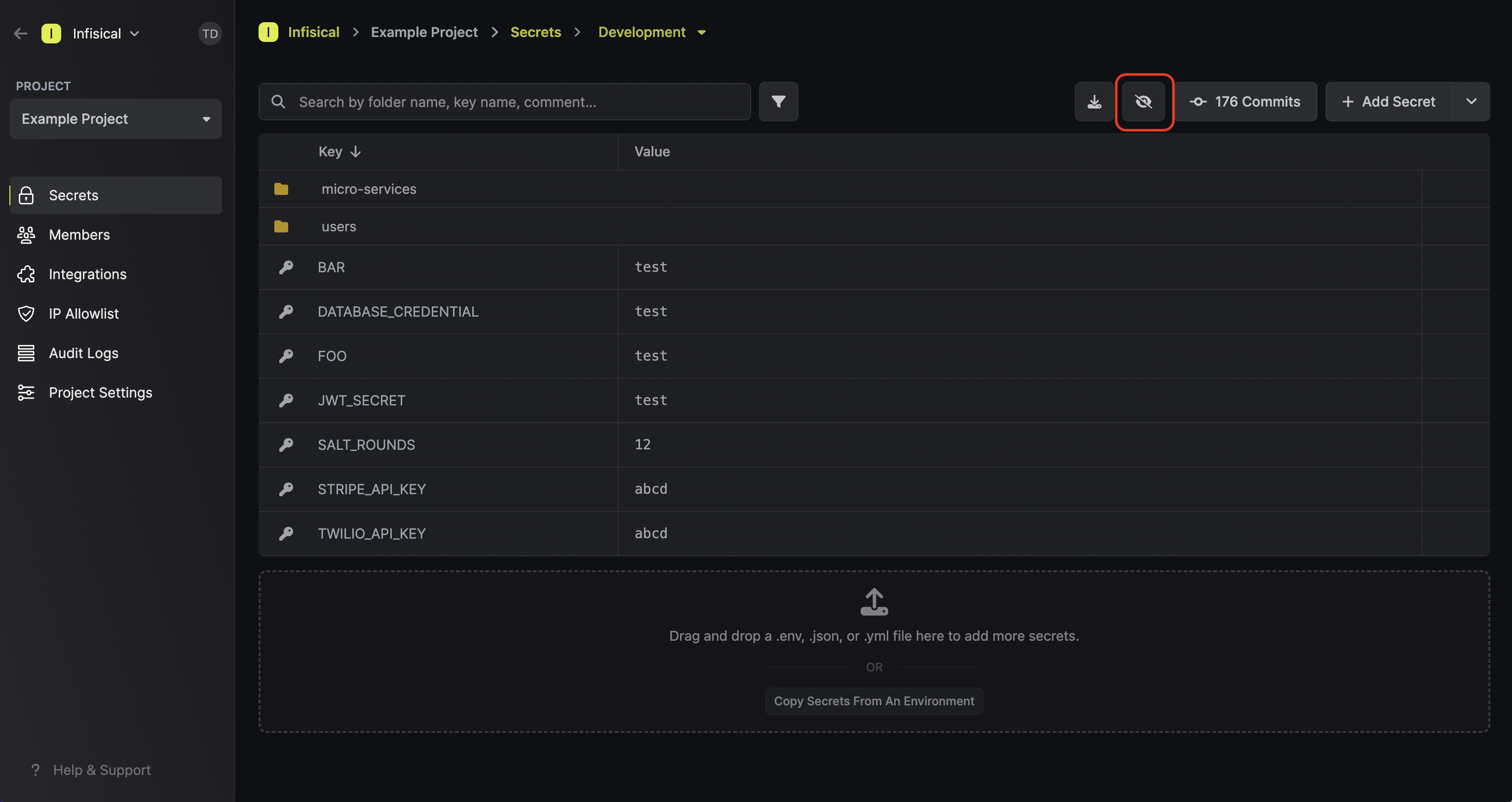
### Download as .env
To download/export secrets back into a `.env` file, press the download button.
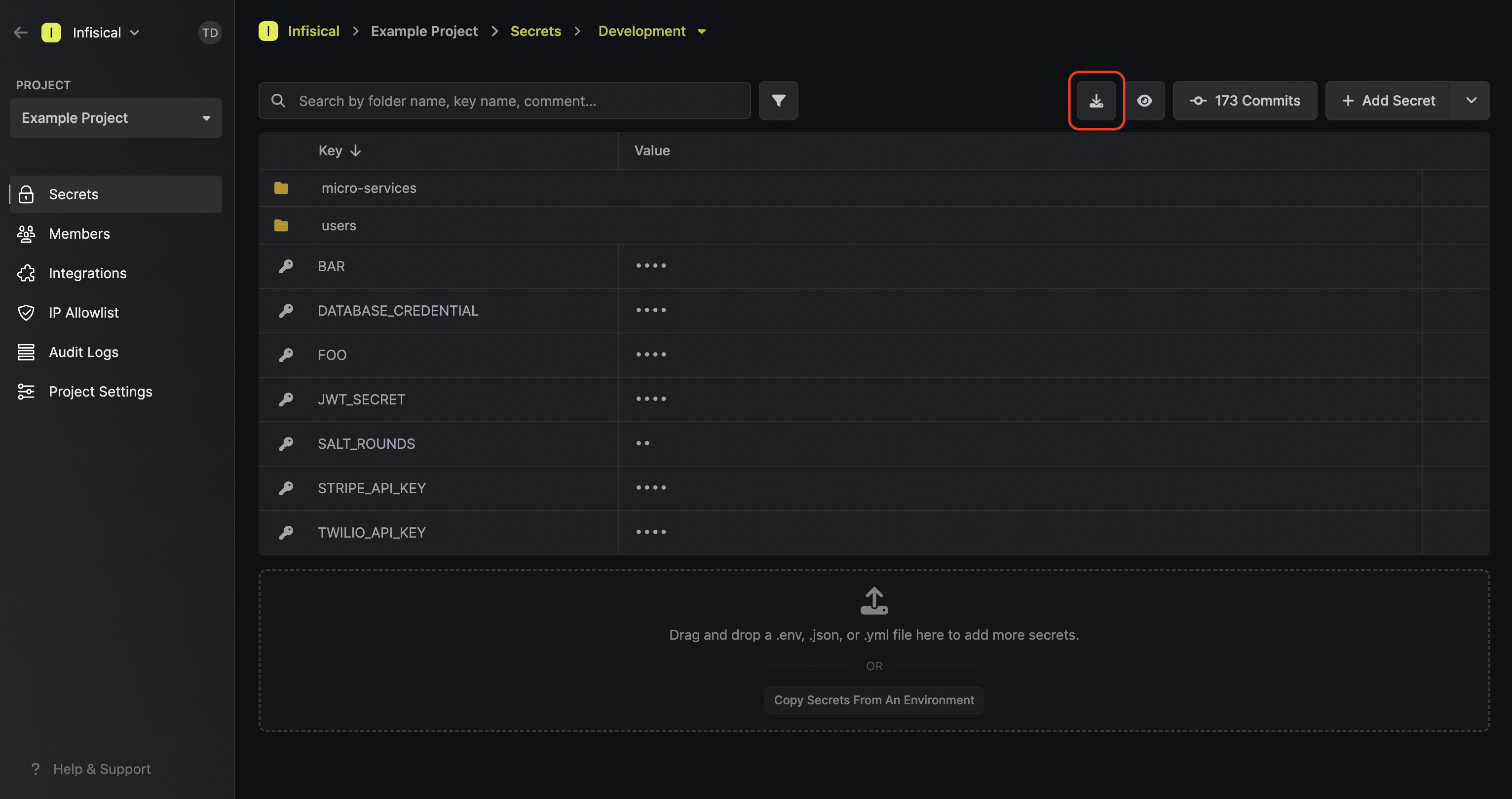
### Tags
To better organize similar secrets, hover over them and label them with a tag.
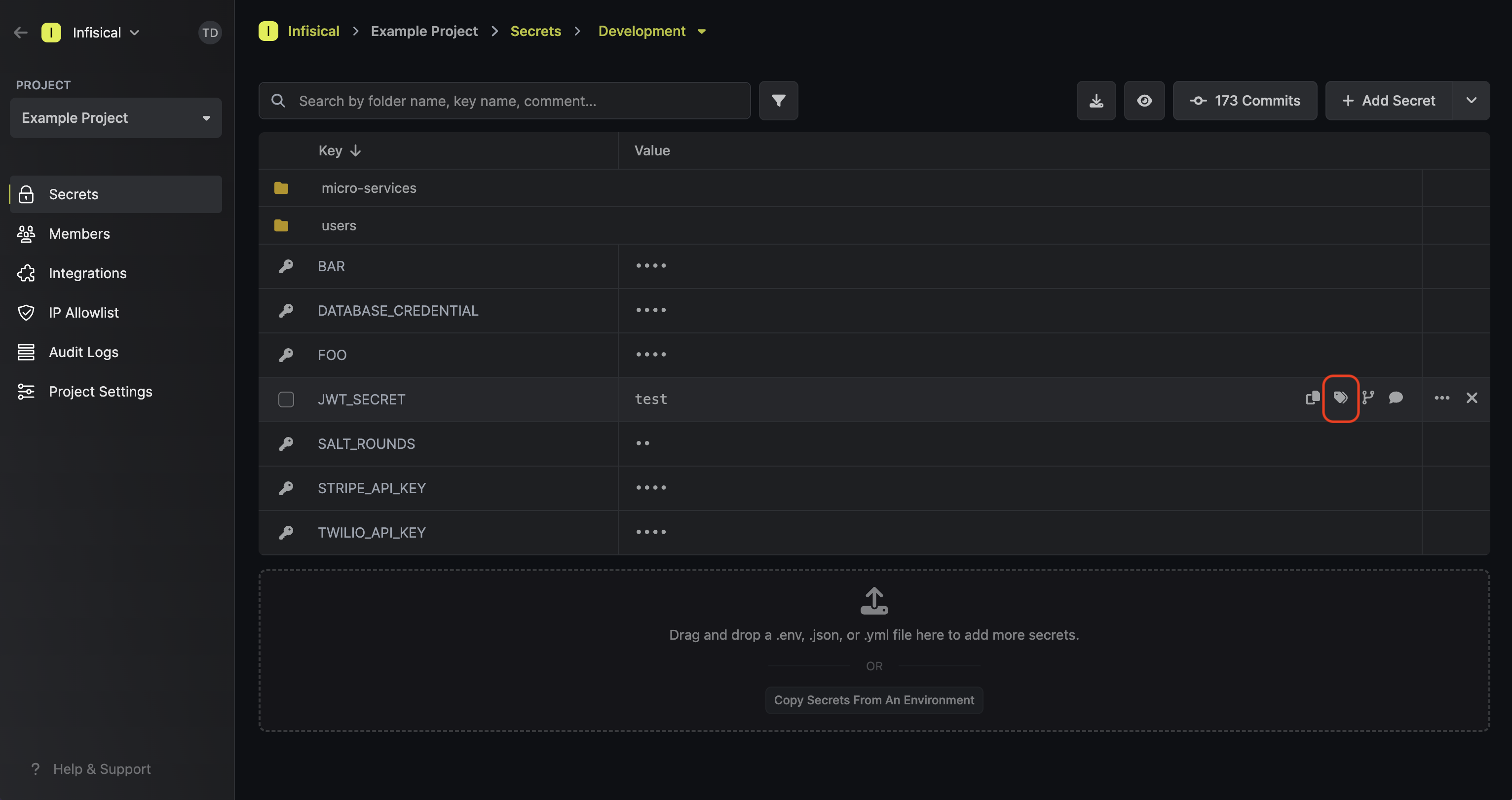
### Comments
To provide more context about a given secret, especially for your team, hover over it and press the comment button.
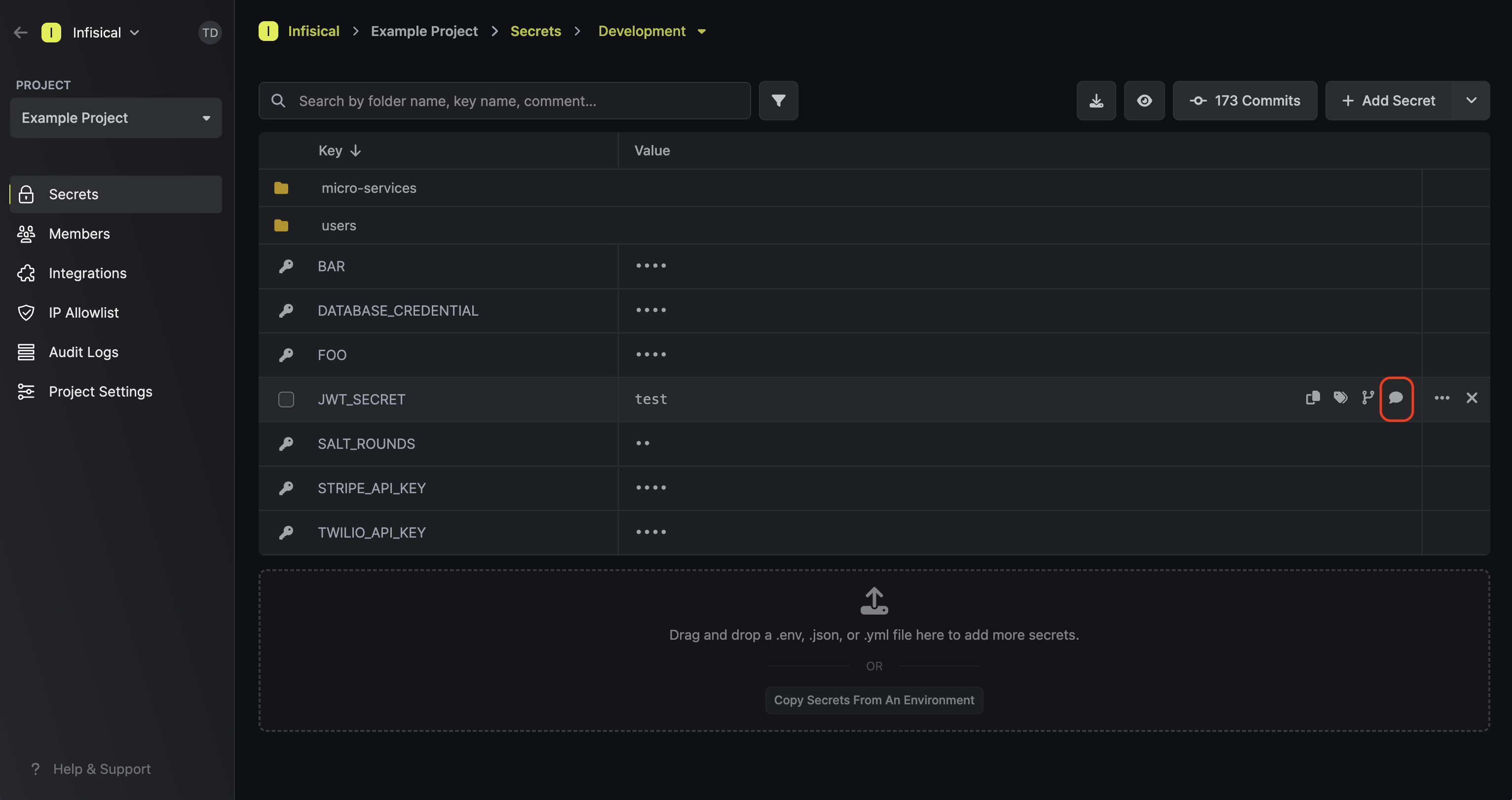
### Personal overrides
Infisical employs the concept of **shared** and **personal** secrets to address the need
for common and custom secret values, or branching, amongst members of a team during software development.
To provide a helpful analogy: A shared value is to a `main` branch as a personal value is to a custom branch.
Consider:
* A team with users A, B, user C.
* A project with an environment containing a shared secret called D with the value E.
Suppose user A overrides the value of secret D with the value F.
Then:
* If user A fetches the secret D back, they get the value F.
* If users B and C fetch the secret D back, they both get the value E.
Please keep in mind that secret reminders won't work with personal overrides.
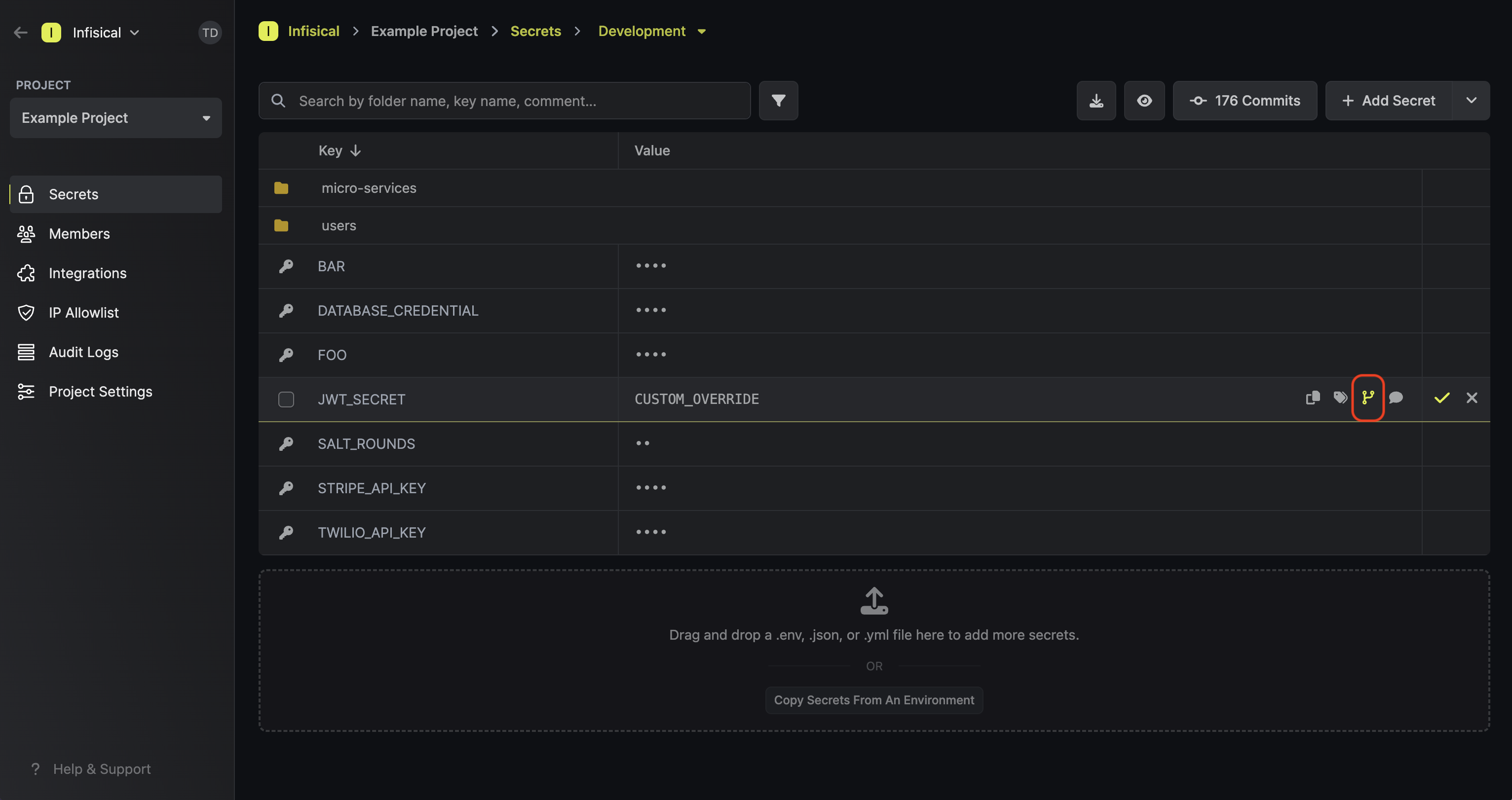
### Drawer
To view the full details of each secret, you can hover over it and press on the ellipses button.
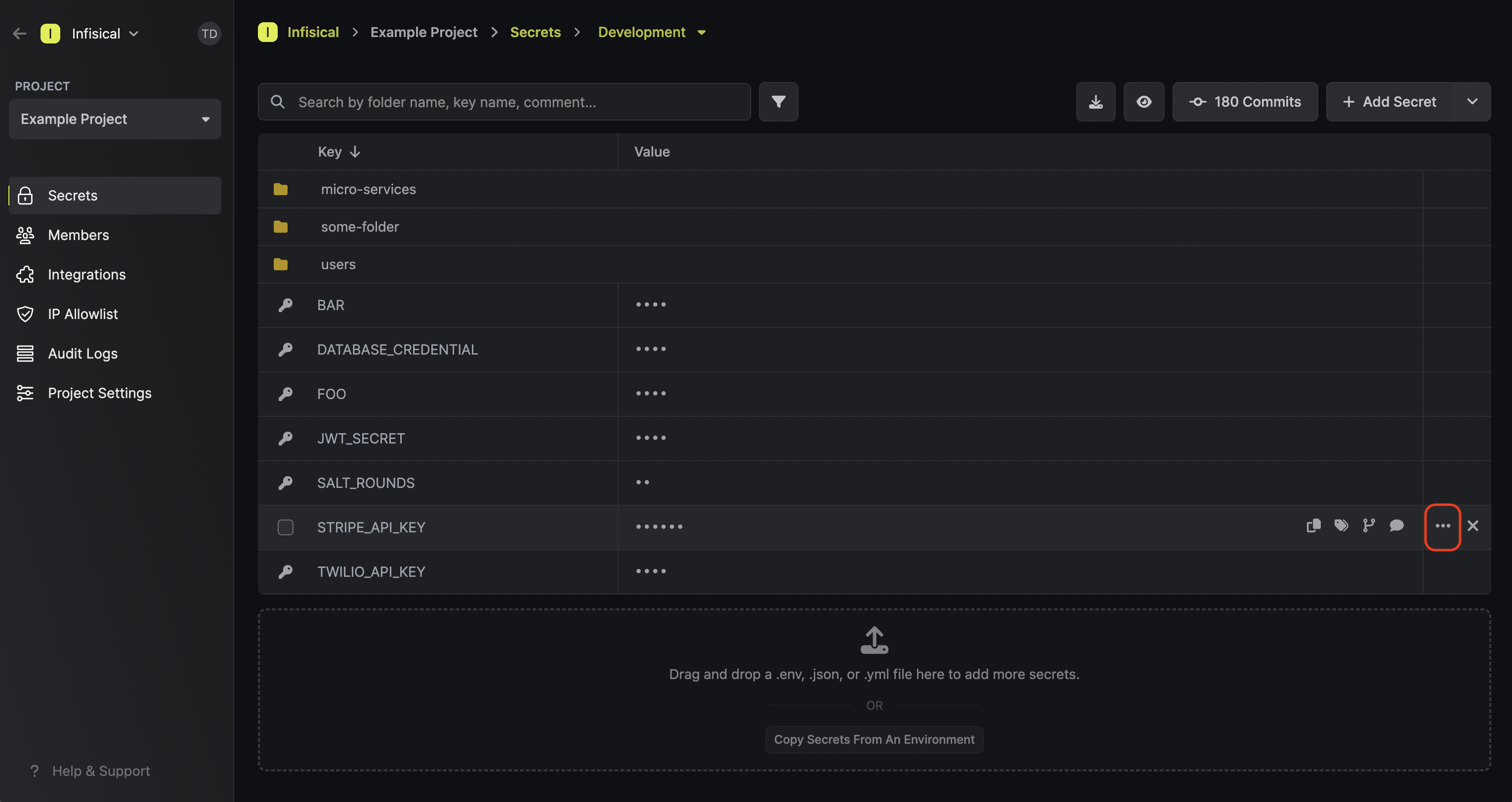
This opens up a side-drawer:
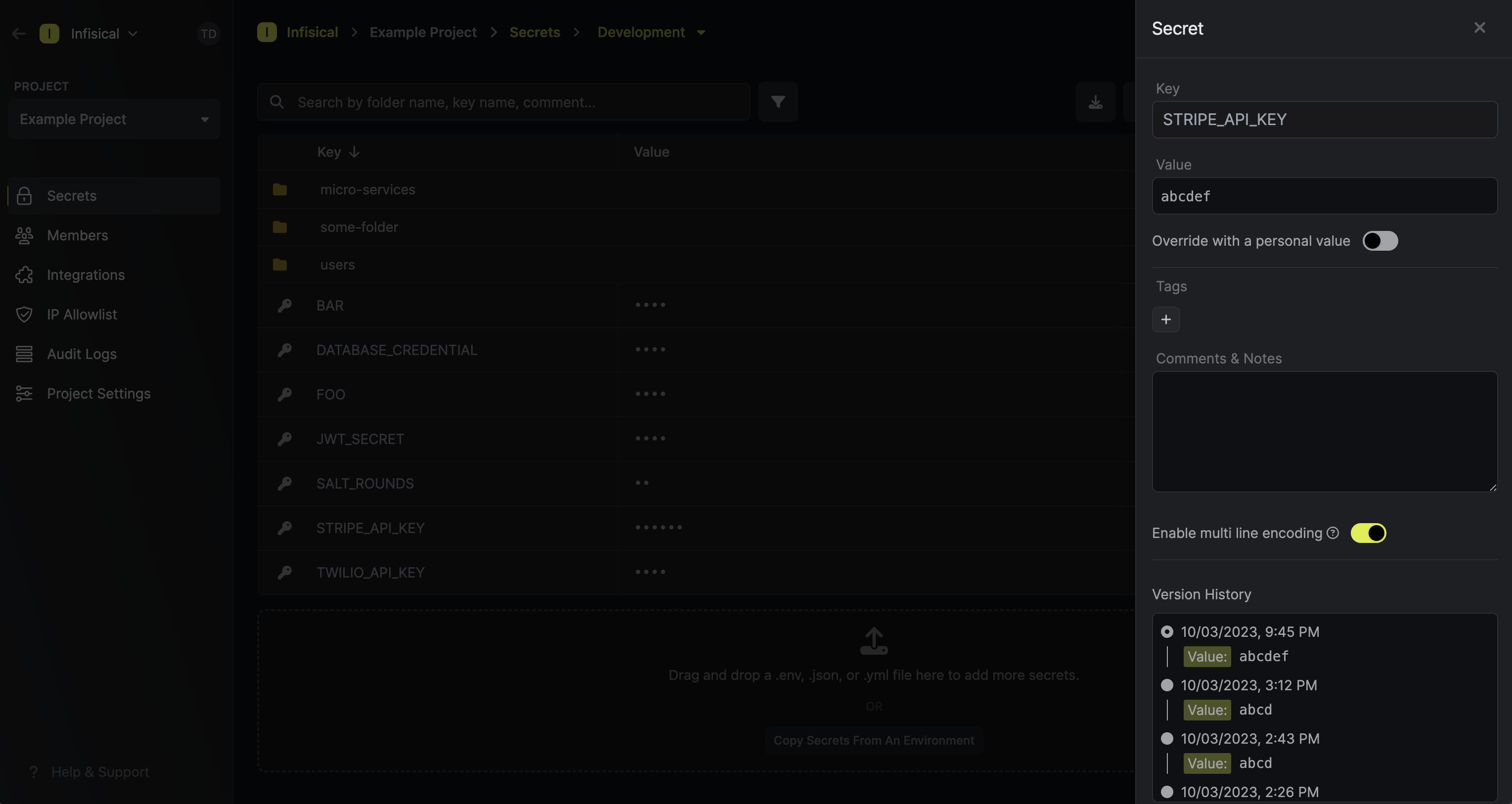
# Project Templates
Learn how to manage and apply project templates
## Concept
Project Templates streamline your ability to set up projects by providing customizable templates to configure projects quickly with a predefined set of environments and roles.
Project Templates is a paid feature.
If you're using Infisical Cloud, then it is available under the **Enterprise Tier**. If you're self-hosting Infisical,
then you should contact [team@infisical.com](mailto:team@infisical.com) to purchase an enterprise license to use it.
## Workflow
The typical workflow for using Project Templates consists of the following steps:
1. Creating a project template: As part of this step, you will configure a set of environments and roles to be created when applying this template to a project.
2. Using a project template: When creating new projects, optionally specify a project template to provision the project with the configured roles and environments.
Note that this workflow can be executed via the Infisical UI or through the API.
## Guide to Creating a Project Template
In the following steps, we'll explore how to set up a project template.
Navigate to the Project Templates tab on the Organization Settings page and tap on the **Add Template** button.
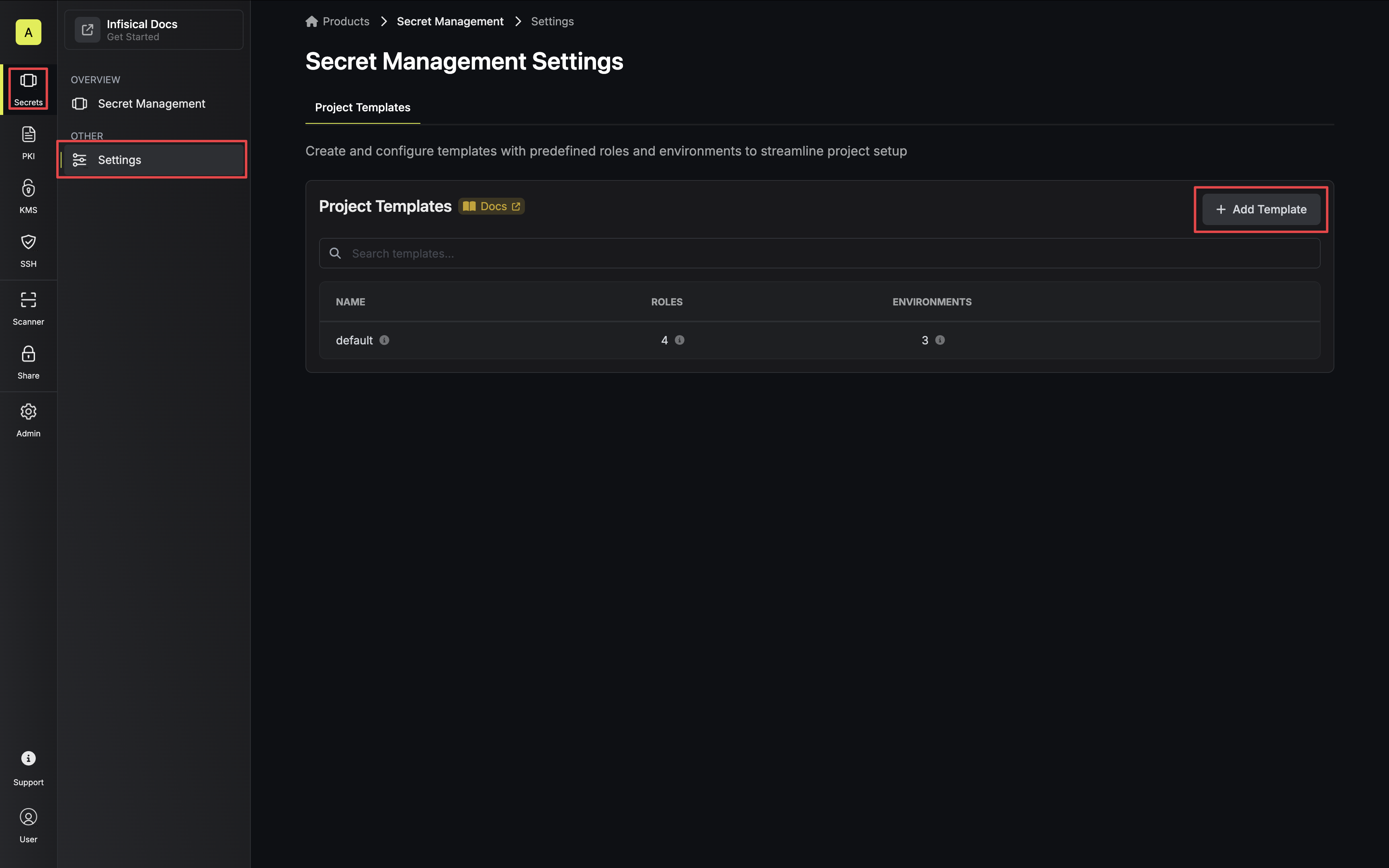
Specify your template details. Here's some guidance on each field:
* Name: A slug-friendly name for the template.
* Description: An optional description of the intended usage of this template.
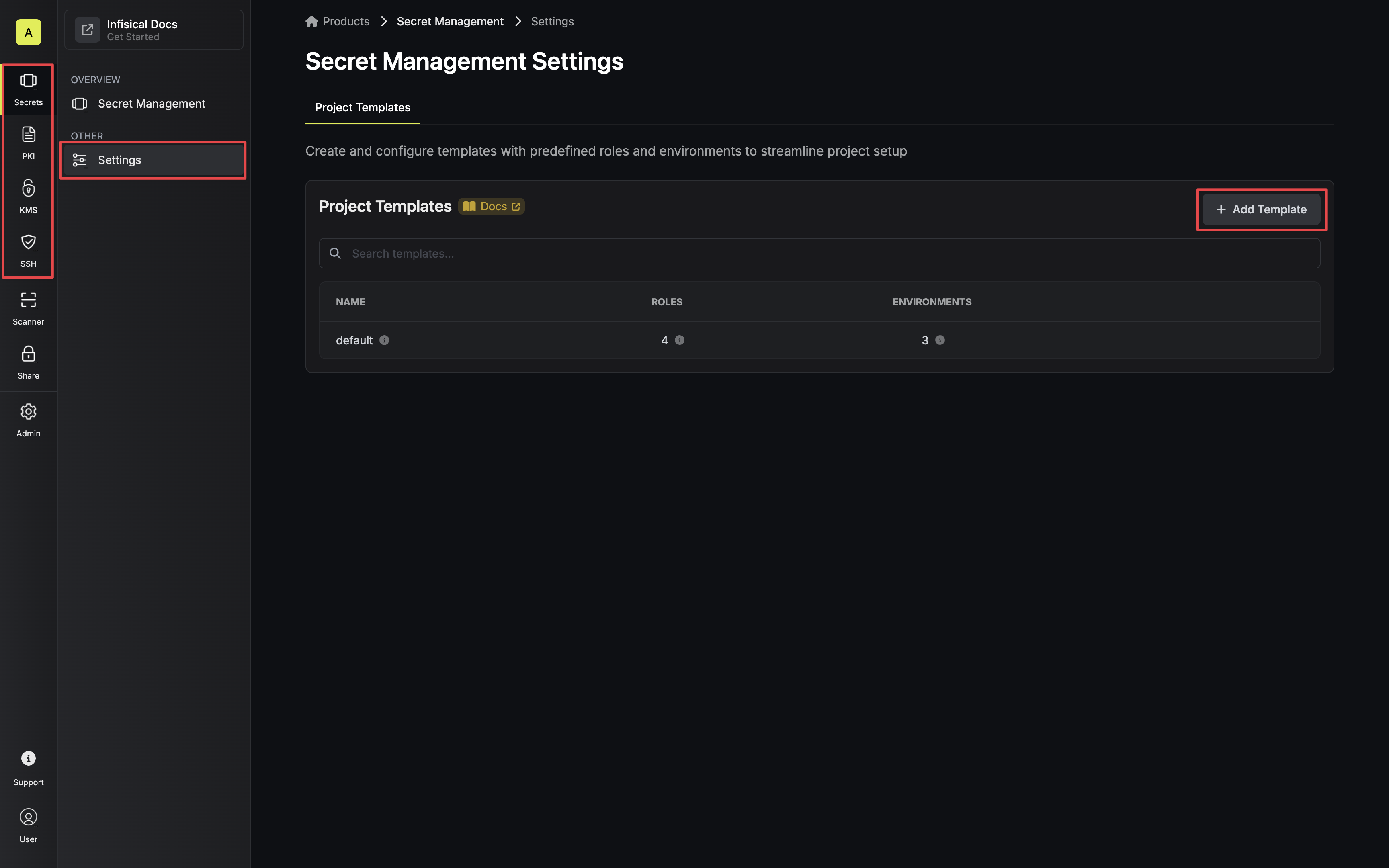
Once your template is created, you'll be directed to the configuration section.
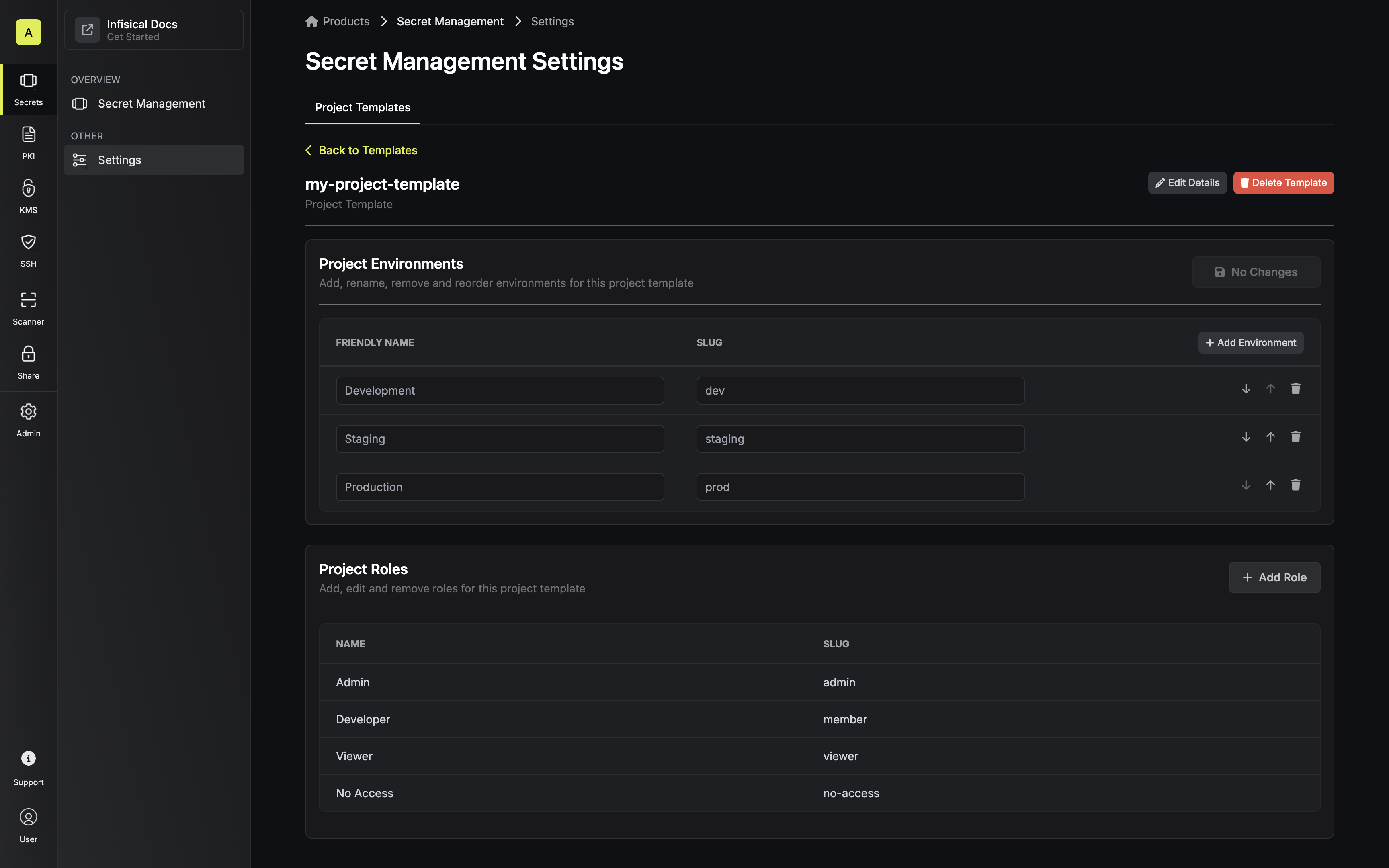
Customize the environments and roles to your needs.
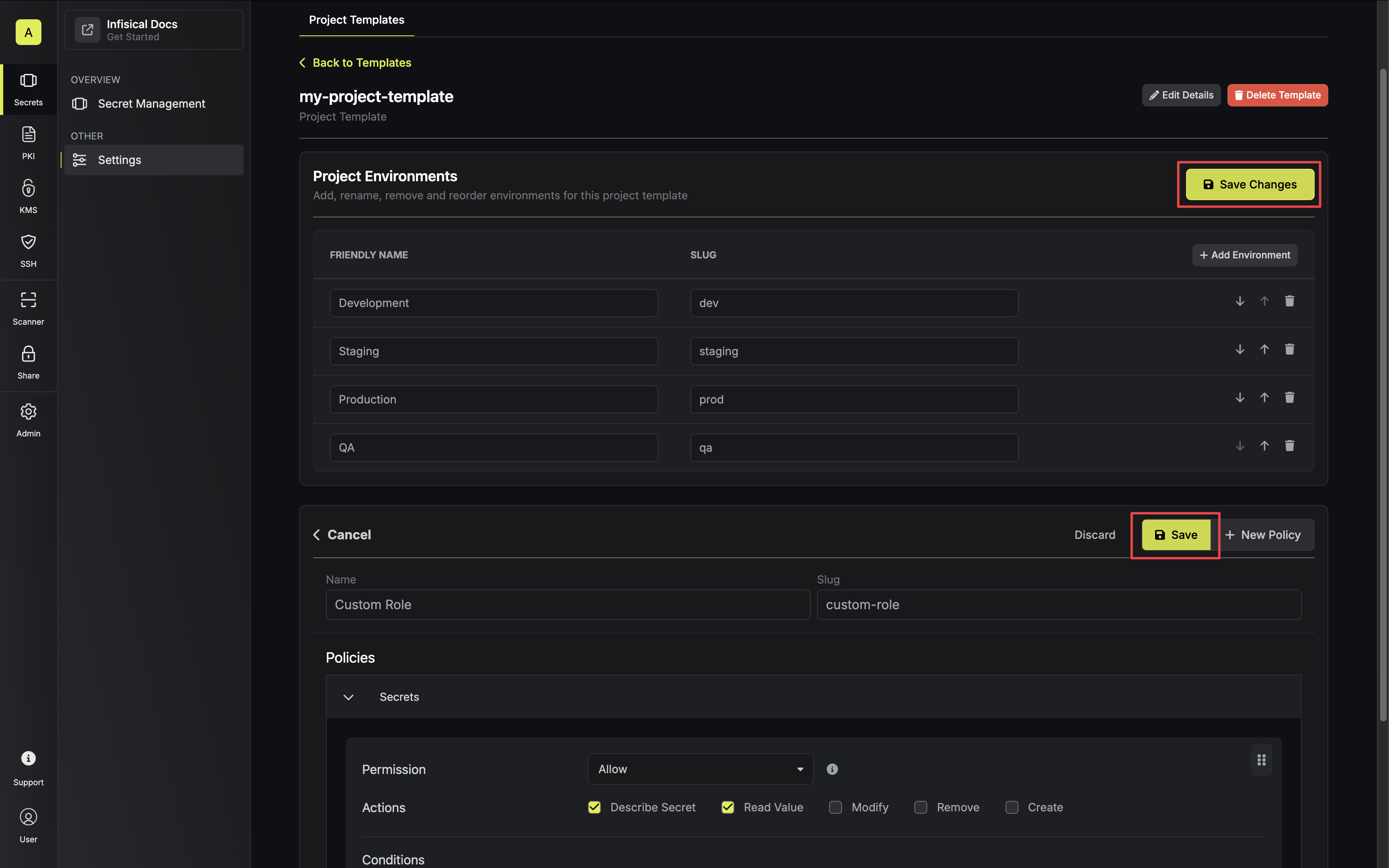
Be sure to save your environment and role changes.
To create a project template, make an API request to the [Create Project Template](/api-reference/endpoints/project-templates/create) API endpoint.
### Sample request
```bash Request
curl --request POST \
--url https://app.infisical.com/api/v1/project-templates \
--header 'Content-Type: application/json' \
--data '{
"name": "my-project-template",
"description": "...",
"environments": "[...]",
"roles": "[...]",
}'
```
### Sample response
```bash Response
{
"projectTemplate": {
"id": "",
"name": "my-project-template",
"description": "...",
"environments": "[...]",
"roles": "[...]",
"orgId": "",
"createdAt": "2023-11-07T05:31:56Z",
"updatedAt": "2023-11-07T05:31:56Z",
}
}
```
## Guide to Using a Project Template
In the following steps, we'll explore how to use a project template when creating a project.
When creating a new project, select the desired template from the dropdown menu in the create project modal.
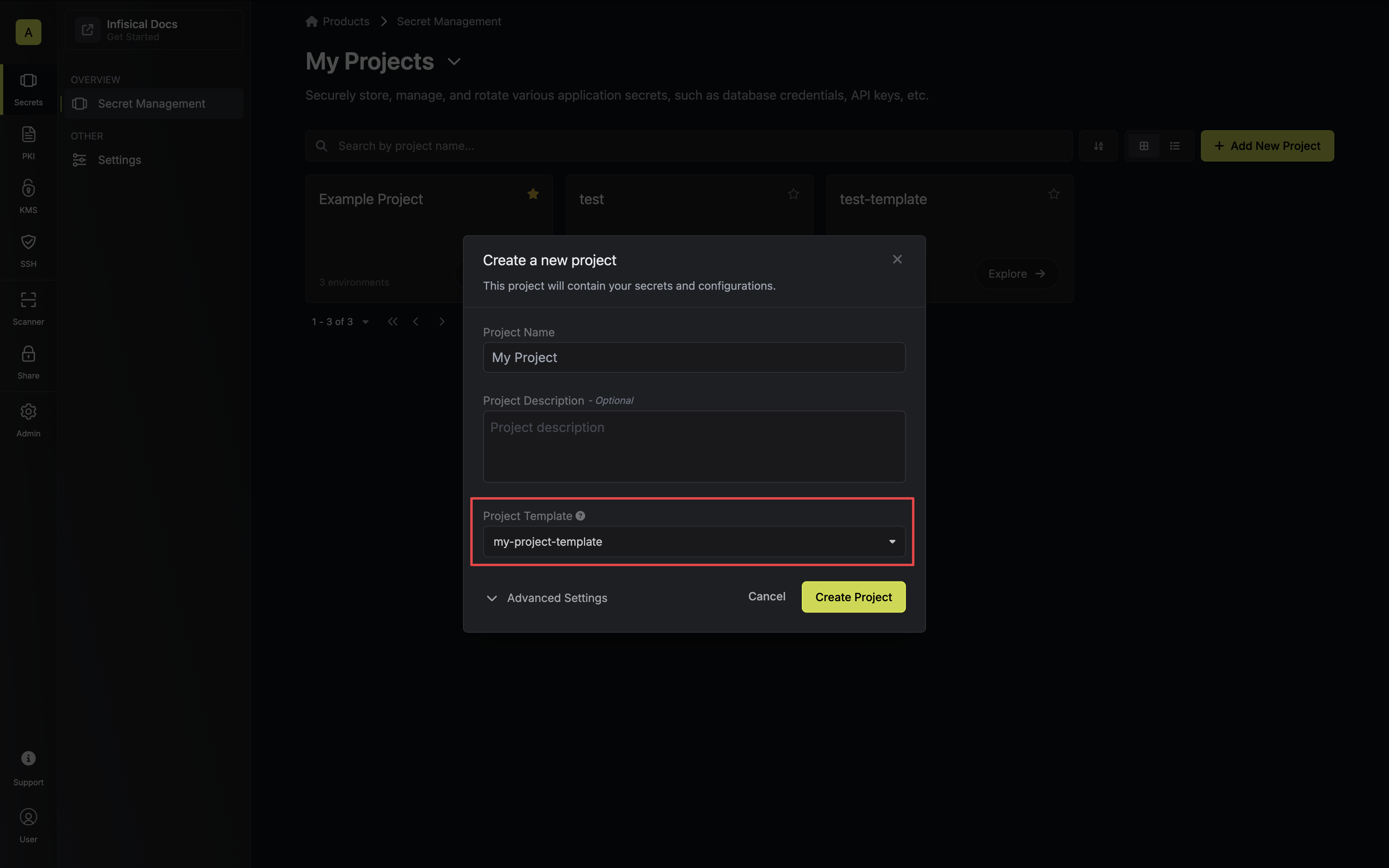
Your project will be provisioned with the configured template roles and environments.
To use a project template, make an API request to the [Create Project](/api-reference/endpoints/workspaces/create-workspace) API endpoint with the specified template name included.
### Sample request
```bash Request
curl --request POST \
--url https://app.infisical.com/api/v2/workspace \
--header 'Content-Type: application/json' \
--data '{
"projectName": "My Project",
"template": "", // defaults to "default"
}'
```
### Sample response
```bash Response
{
"project": {
"id": "",
"environments": "[...]", // configured environments
...
}
}
```
Note that configured roles are not included in the project response.
## FAQ
No. Project templates only apply at the time of project creation.
# Azure SCIM
Learn how to configure SCIM provisioning with Azure for Infisical.
Azure SCIM provisioning is a paid feature.
If you're using Infisical Cloud, then it is available under the **Enterprise Tier**. If you're self-hosting Infisical,
then you should contact [sales@infisical.com](mailto:sales@infisical.com) to purchase an enterprise license to use it.
Prerequisites:
* [Configure Azure SAML for Infisical](/documentation/platform/sso/azure)
In Infisical, head to your Organization Settings > Authentication > SCIM Configuration and
press the **Enable SCIM provisioning** toggle to allow Azure to provision/deprovision users for your organization.
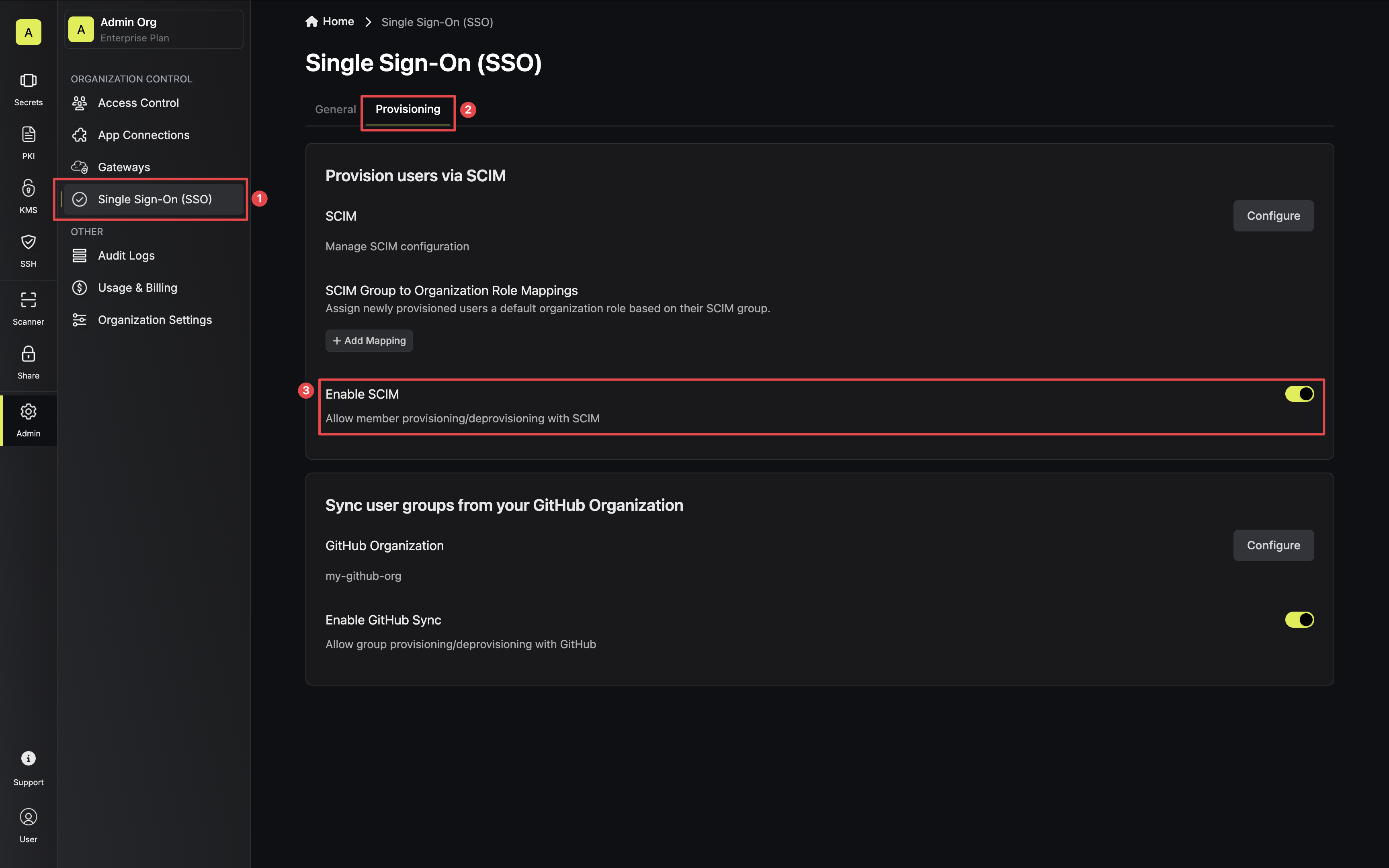
Next, press **Manage SCIM Tokens** and then **Create** to generate a SCIM token for Azure.
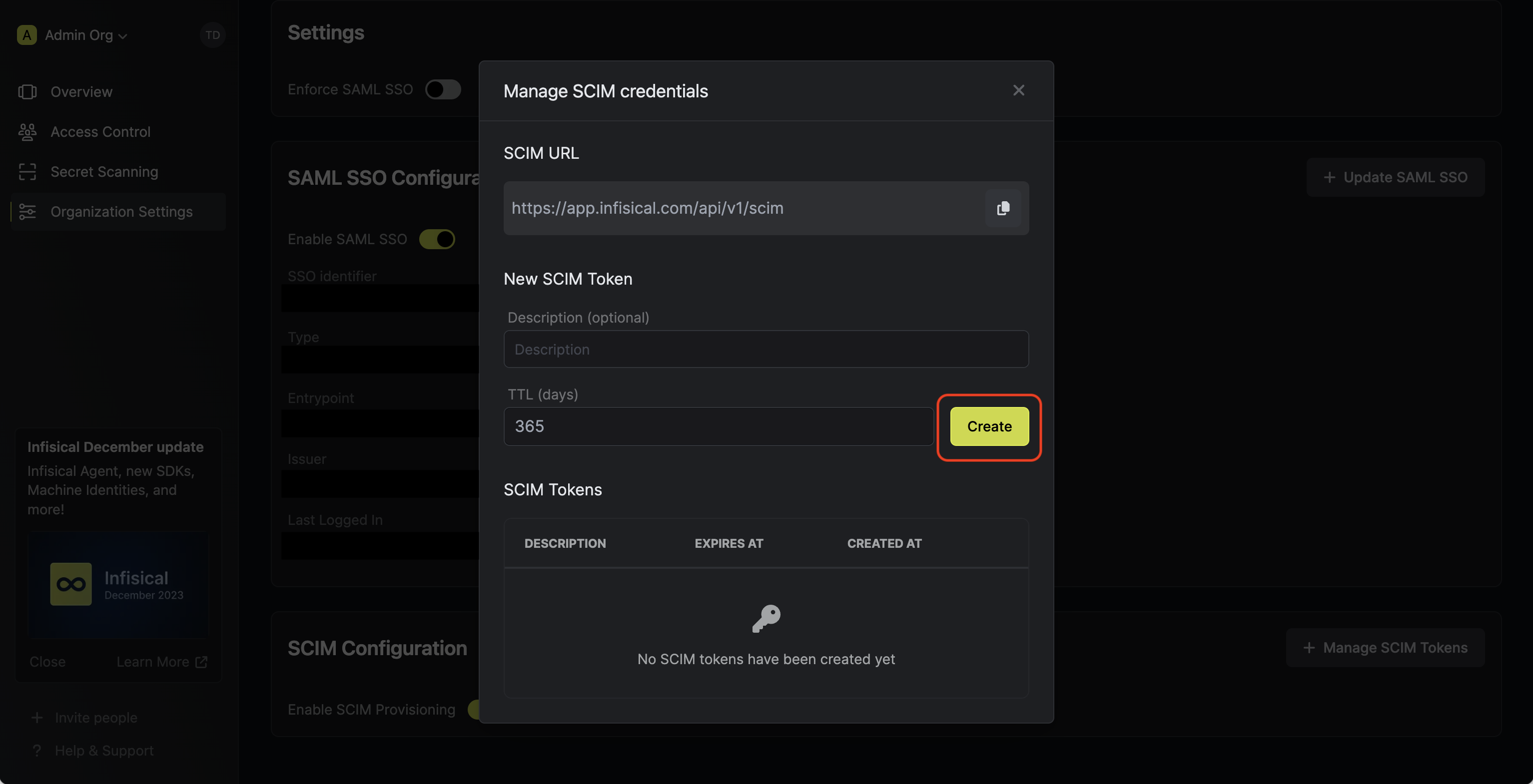
Next, copy the **SCIM URL** and **New SCIM Token** to use when configuring SCIM in Azure.
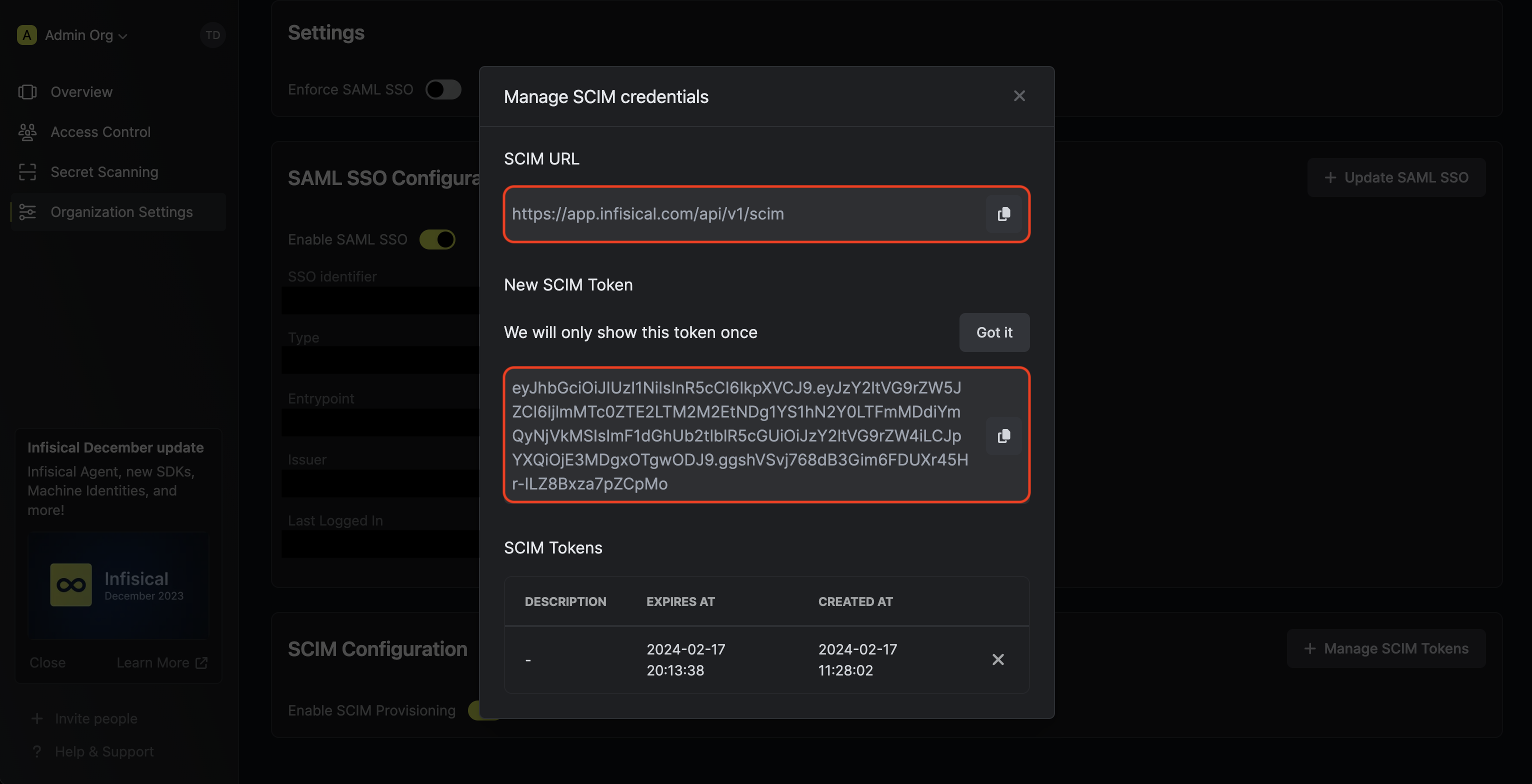
In Azure, navigate to Enterprise Application > Users and Groups. Add any users and/or groups to your application that you would like
to be provisioned over to Infisical.
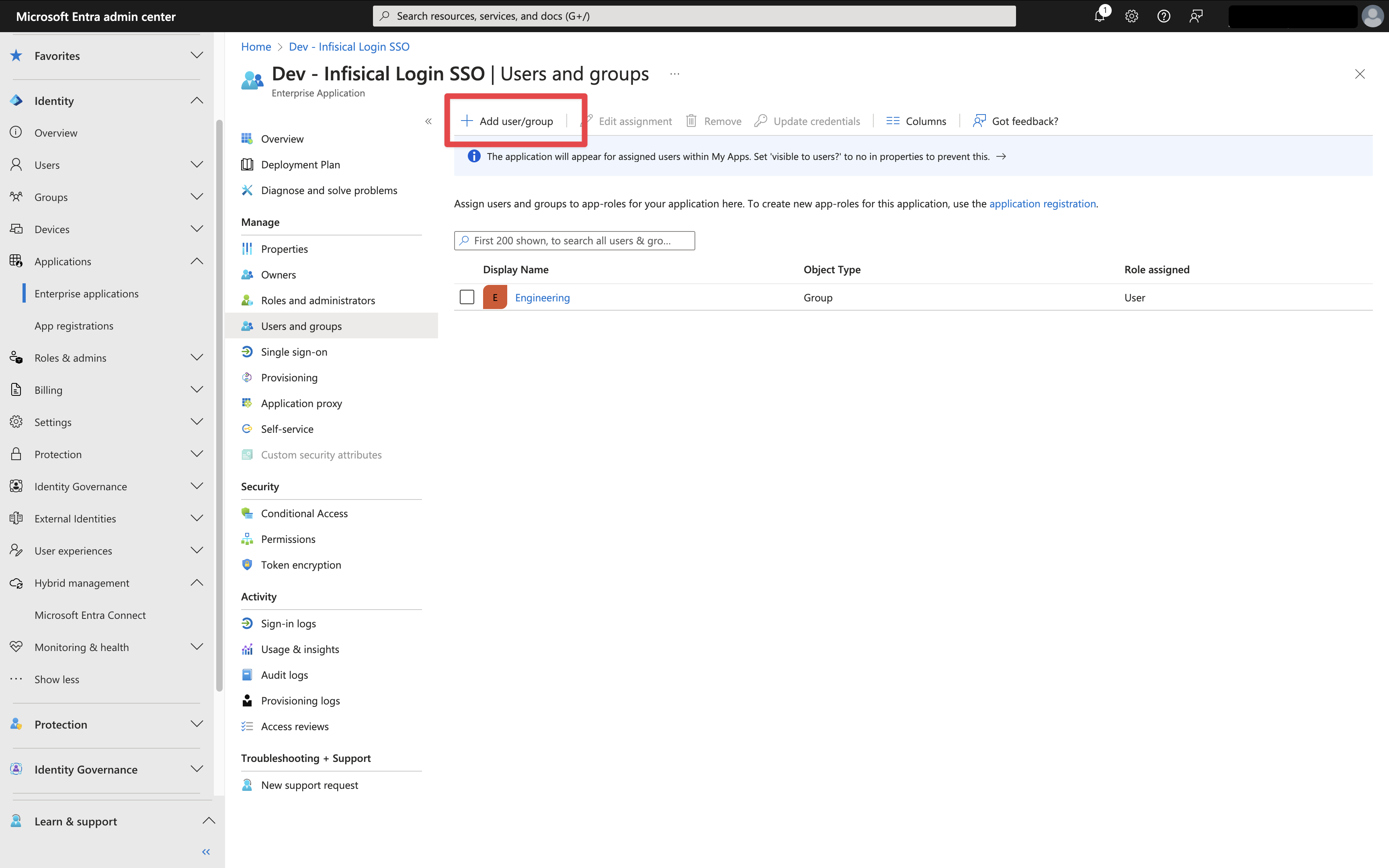
In Azure, head to your Enterprise Application > Provisioning > Overview and press **Get started**.
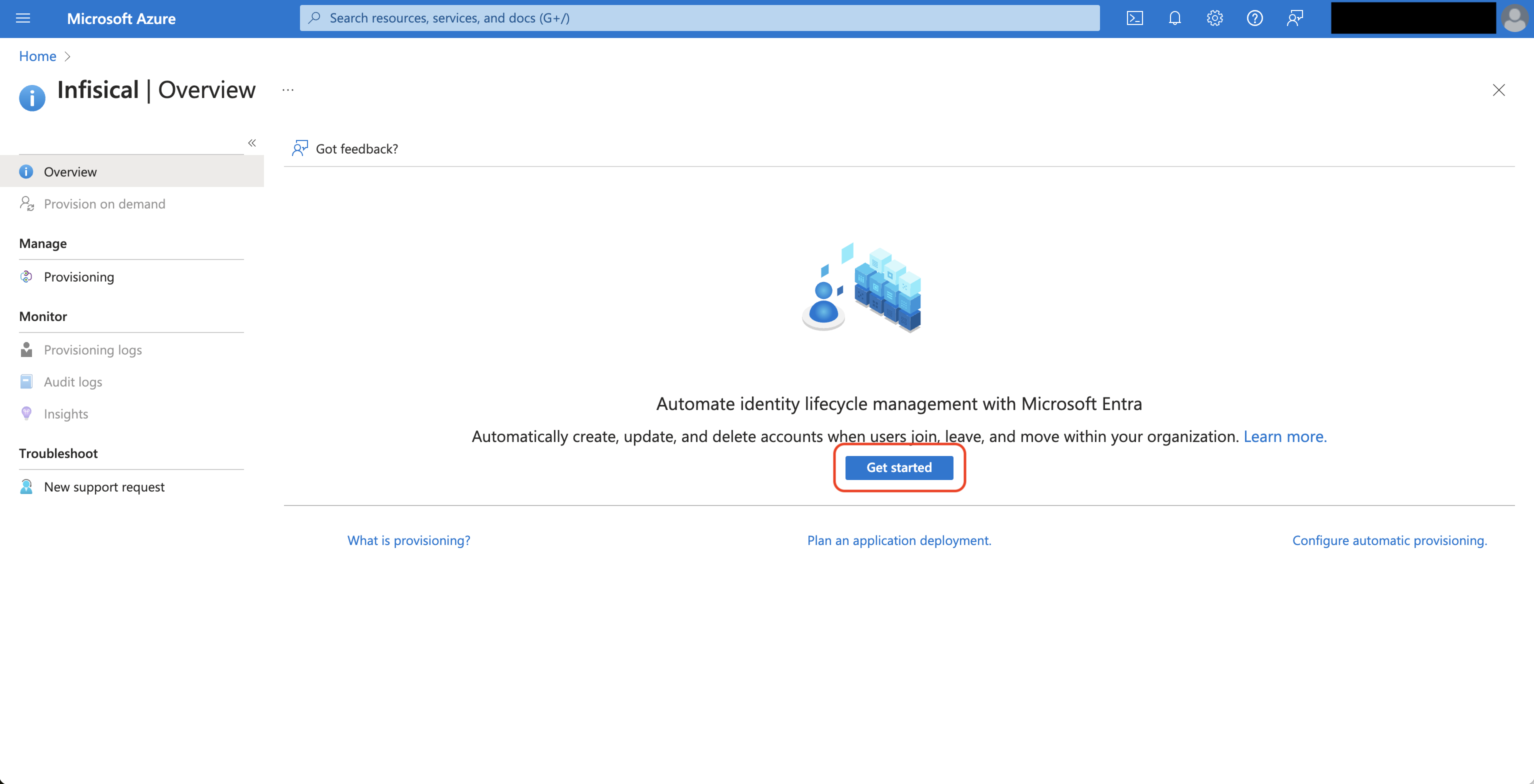
Next, set the following fields:
* Provisioning Mode: Select **Automatic**.
* Tenant URL: Input **SCIM URL** from Step 1.
* Secret Token: Input the **New SCIM Token** from Step 1.
Afterwards, click **Enable SCIM** and press the **Test Connection** button to check that SCIM is configured properly.
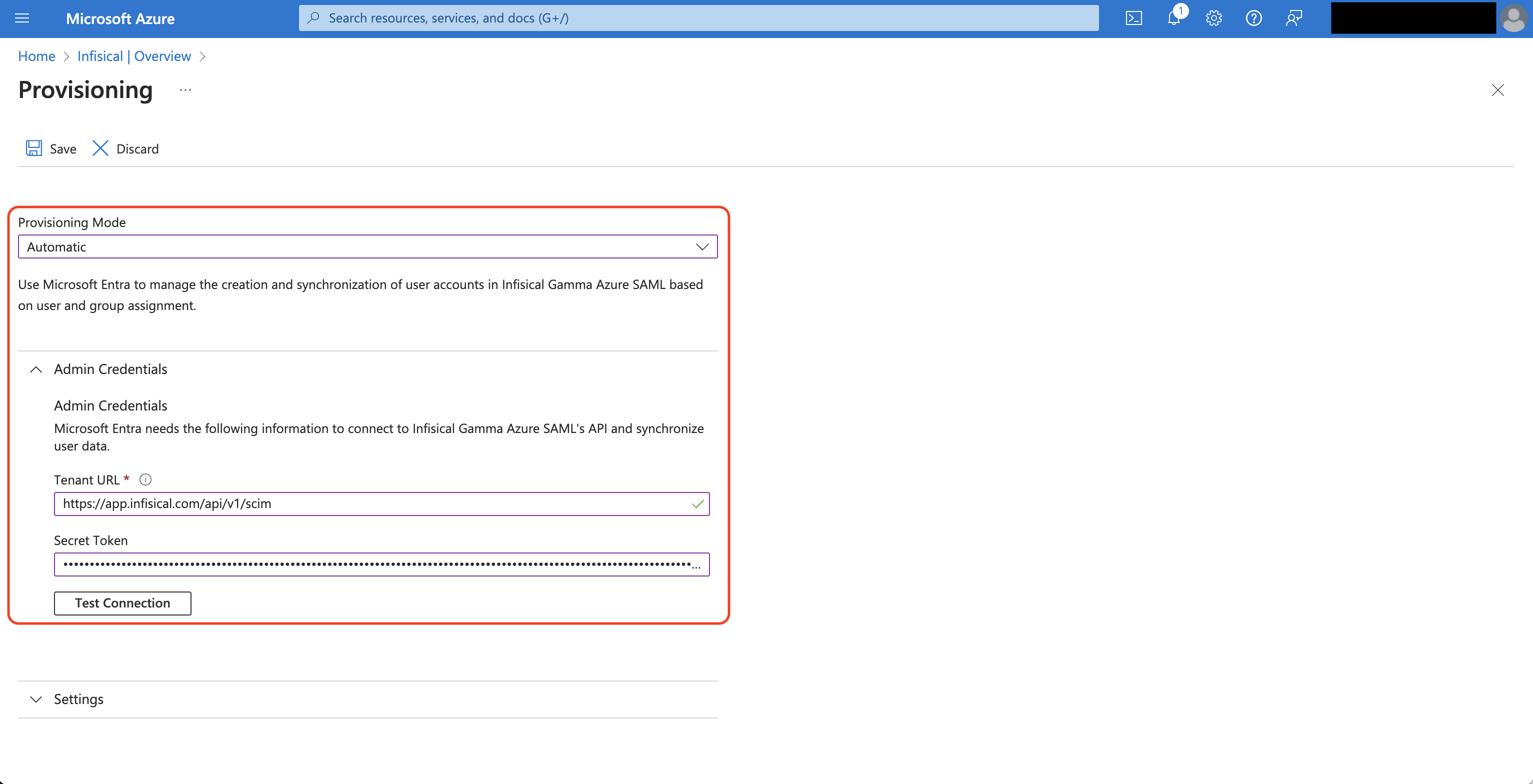
After you hit **Save**, select **Provision Microsoft Entra ID Users** under the **Mappings** subsection.
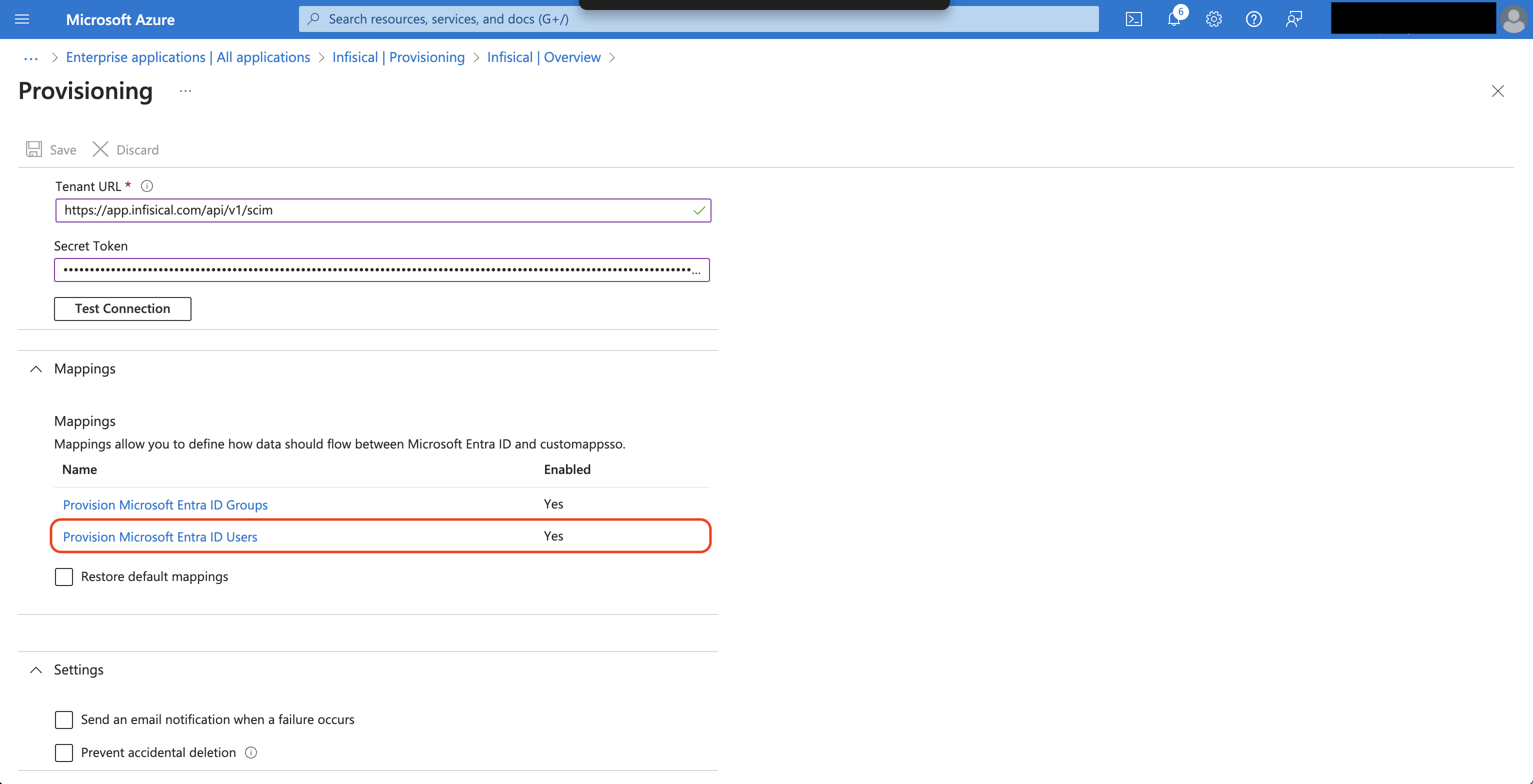
Next, adjust the mappings so you have them configured as below:
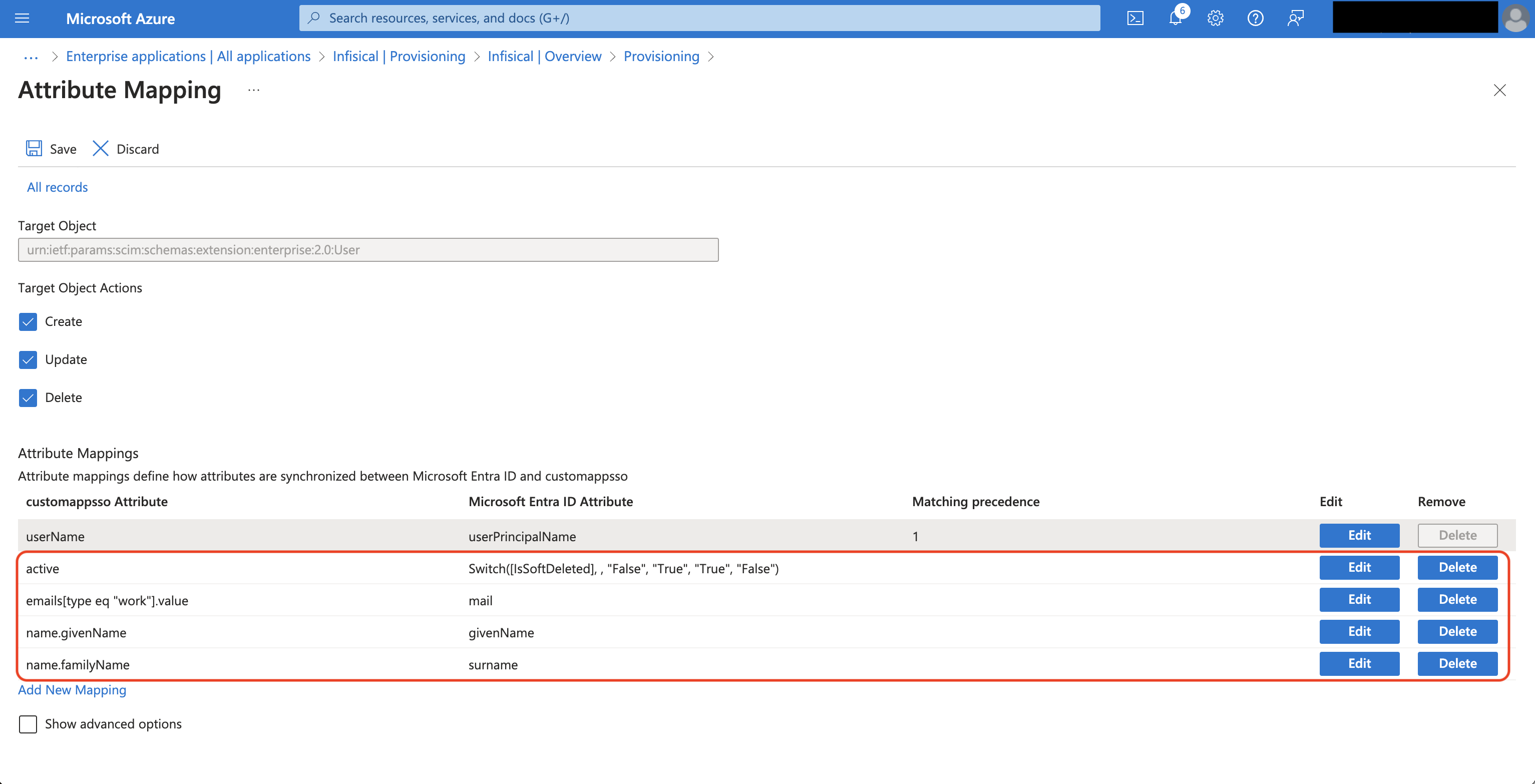
Finally, head to your Enterprise Application > Provisioning and set the **Provisioning Status** to **On**.
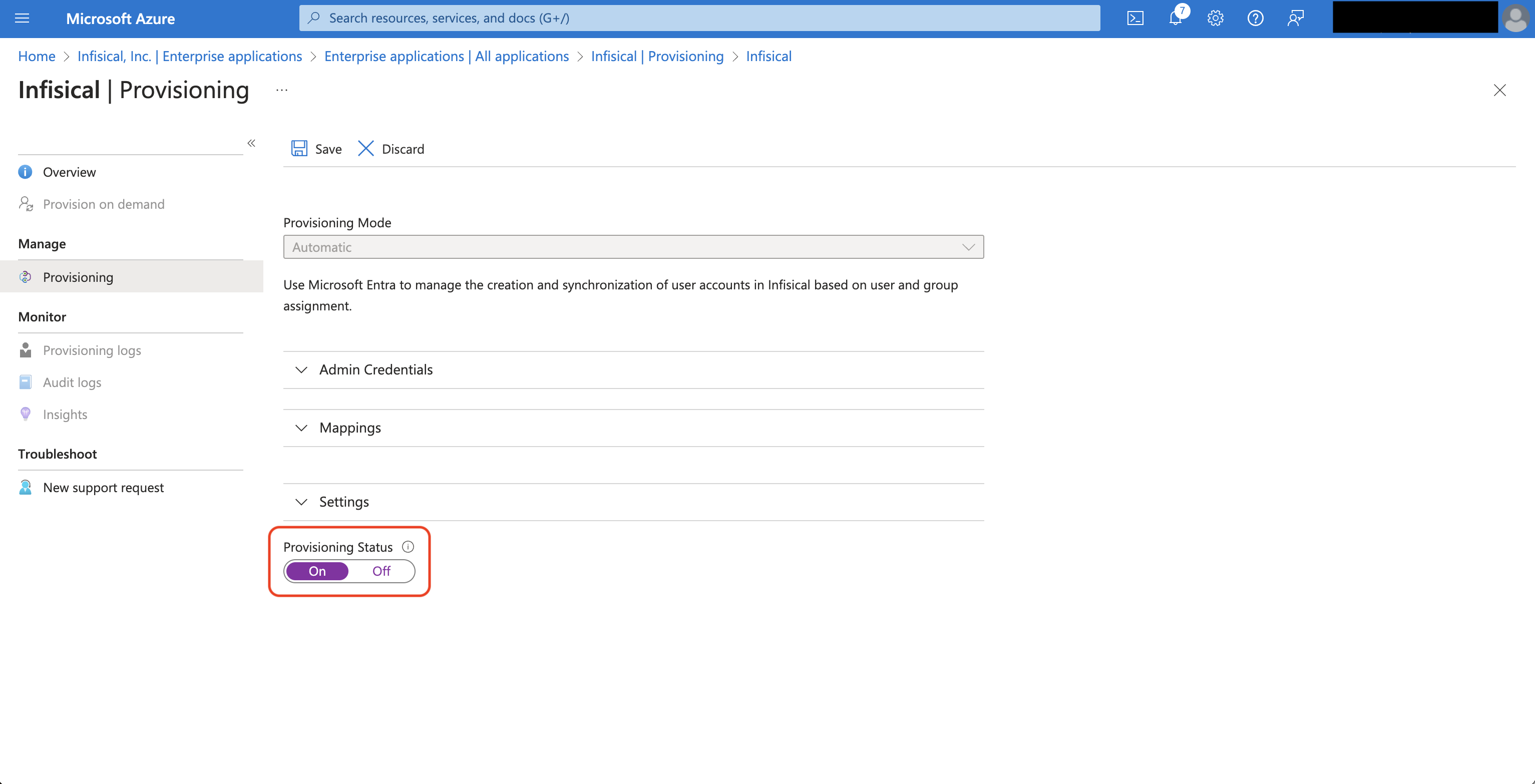
Alternatively, you can go to **Overview** and press **Start provisioning** to have Azure start provisioning/deprovisioning users to Infisical.
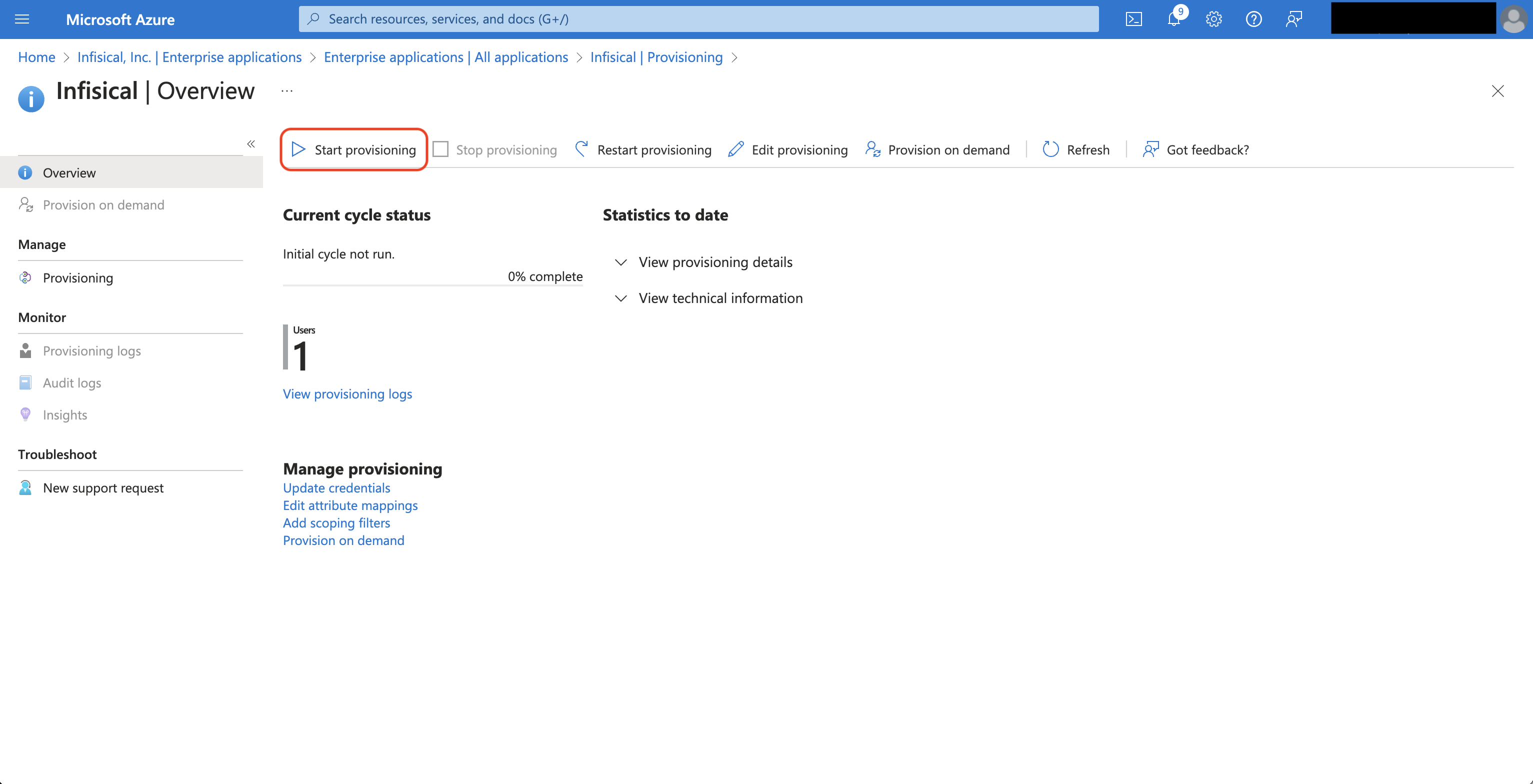
Now Azure can provision/deprovision users to/from your organization in Infisical.
**FAQ**
Infisical's SCIM implmentation accounts for retaining the end-to-end encrypted architecture of Infisical because we decouple the **authentication** and **decryption** steps in the platform.
For this reason, SCIM-provisioned users are initialized but must finish setting up their account when logging in the first time by creating a master encryption/decryption key. With this implementation, IdPs and SCIM providers cannot and will not have access to the decryption key needed to decrypt your secrets.
# SCIM Group Mappings
Learn how to enhance your SCIM implementation using group mappings
SCIM provisioning, and by extension group mapping, is a paid feature.
If you're using Infisical Cloud, then it is available under the **Enterprise Tier**. If you're self-hosting Infisical,
then you should contact [sales@infisical.com](mailto:sales@infisical.com) to purchase an enterprise license to use it.
## SCIM Group to Organization Role Mapping
By default, when users are provisioned via SCIM, they will be assigned the default organization role configured in [Organization General Settings](/documentation/platform/organization#settings).
For more precise control over membership roles, you can set up SCIM Group to Organization Role Mappings. This enables you to assign specific roles based on the group from which a user is provisioned.
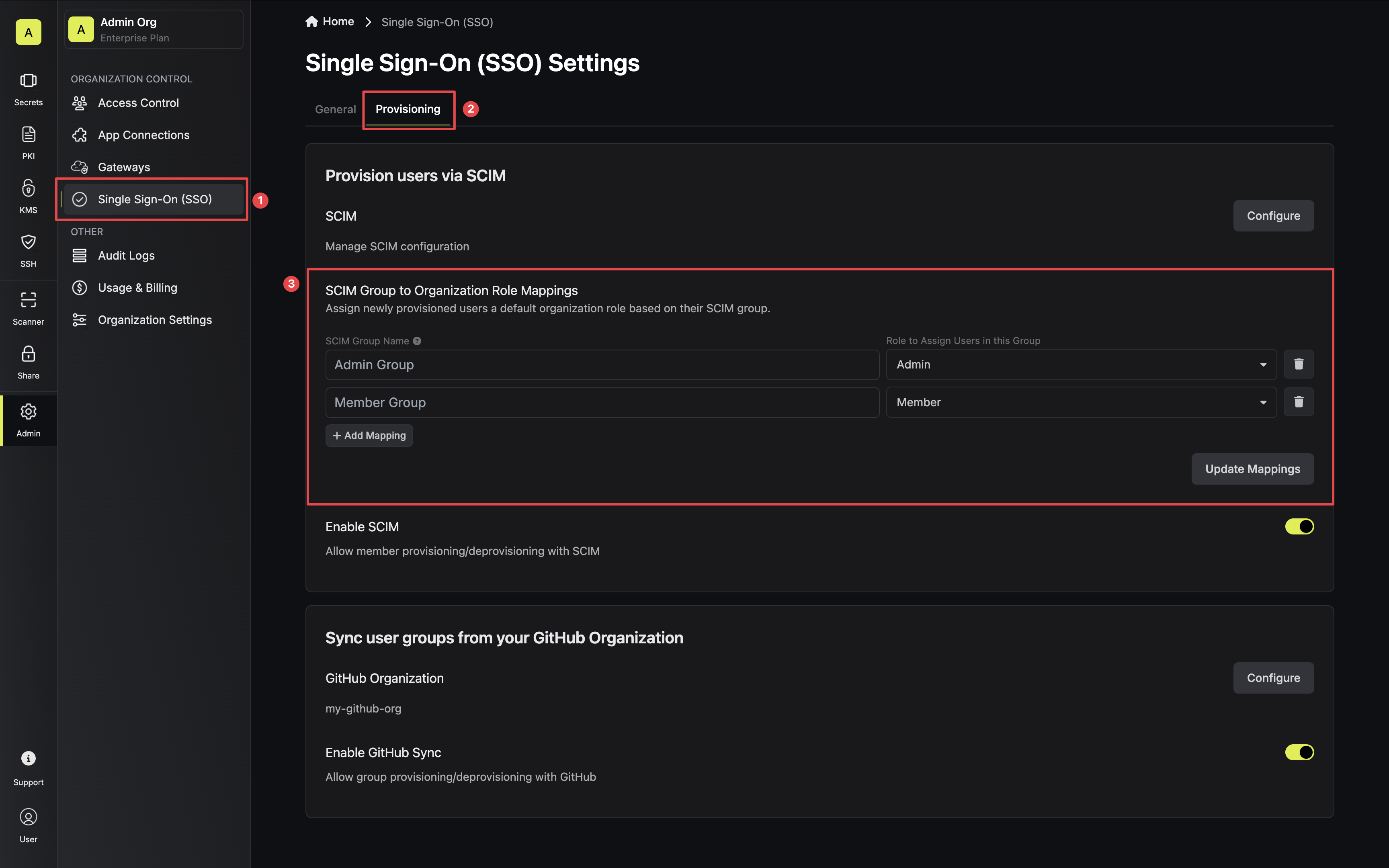
To configure a mapping, simply enter the SCIM group's name and select the role you would like users to be assigned from this group. Be sure
to tap **Update Mappings** once complete.
SCIM Group Mappings only apply when users are first provisioned. Previously provisioned users will not be affected, allowing you to customize user roles after they are added.
# JumpCloud SCIM
Learn how to configure SCIM provisioning with JumpCloud for Infisical.
JumpCloud SCIM provisioning is a paid feature.
If you're using Infisical Cloud, then it is available under the **Enterprise Tier**. If you're self-hosting Infisical,
then you should contact [sales@infisical.com](mailto:sales@infisical.com) to purchase an enterprise license to use it.
Prerequisites:
* [Configure JumpCloud SAML for Infisical](/documentation/platform/sso/jumpcloud)
In Infisical, head to your Organization Settings > Authentication > SCIM Configuration and
press the **Enable SCIM provisioning** toggle to allow JumpCloud to provision/deprovision users and user groups for your organization.
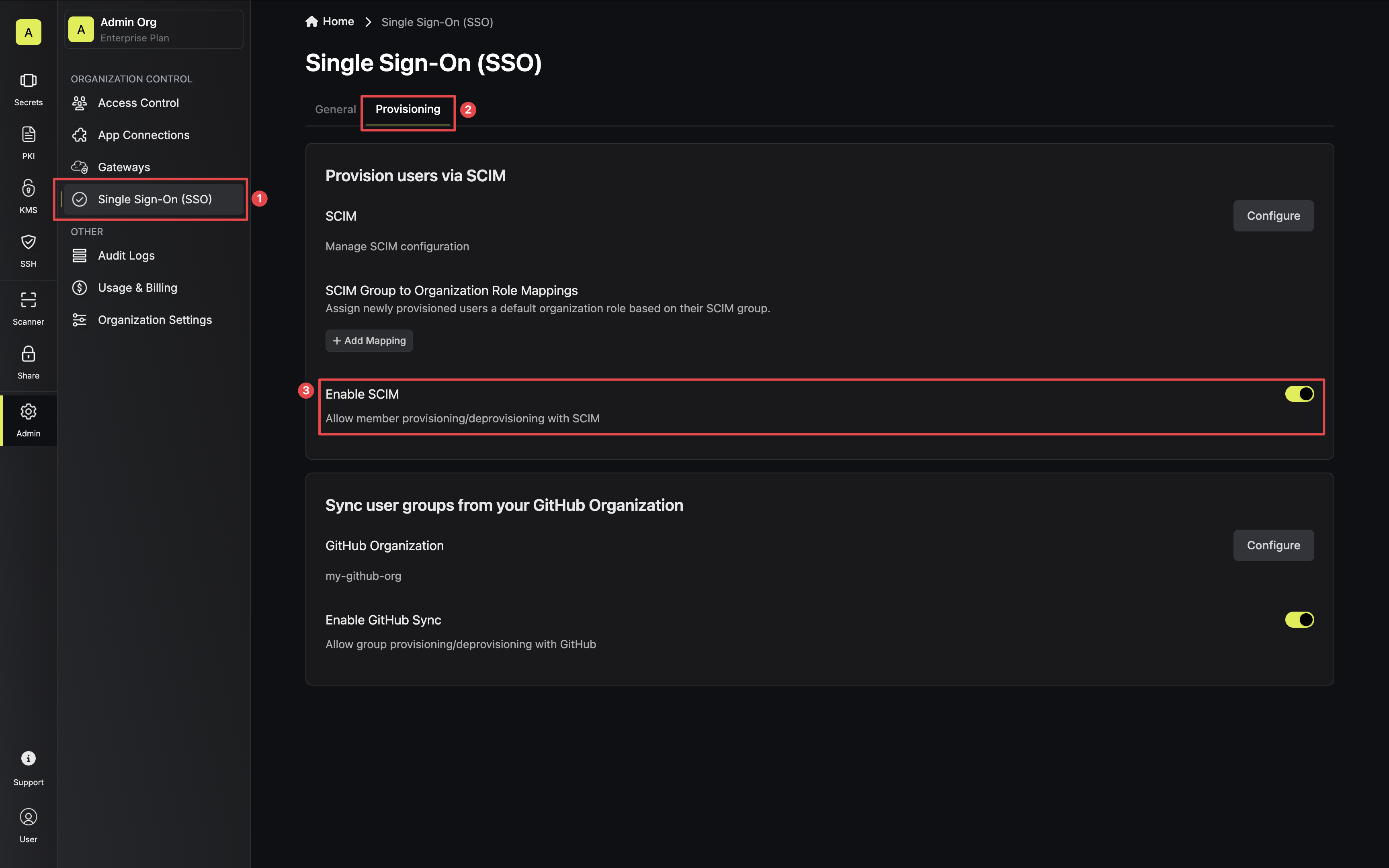
Next, press **Manage SCIM Tokens** and then **Create** to generate a SCIM token for JumpCloud.
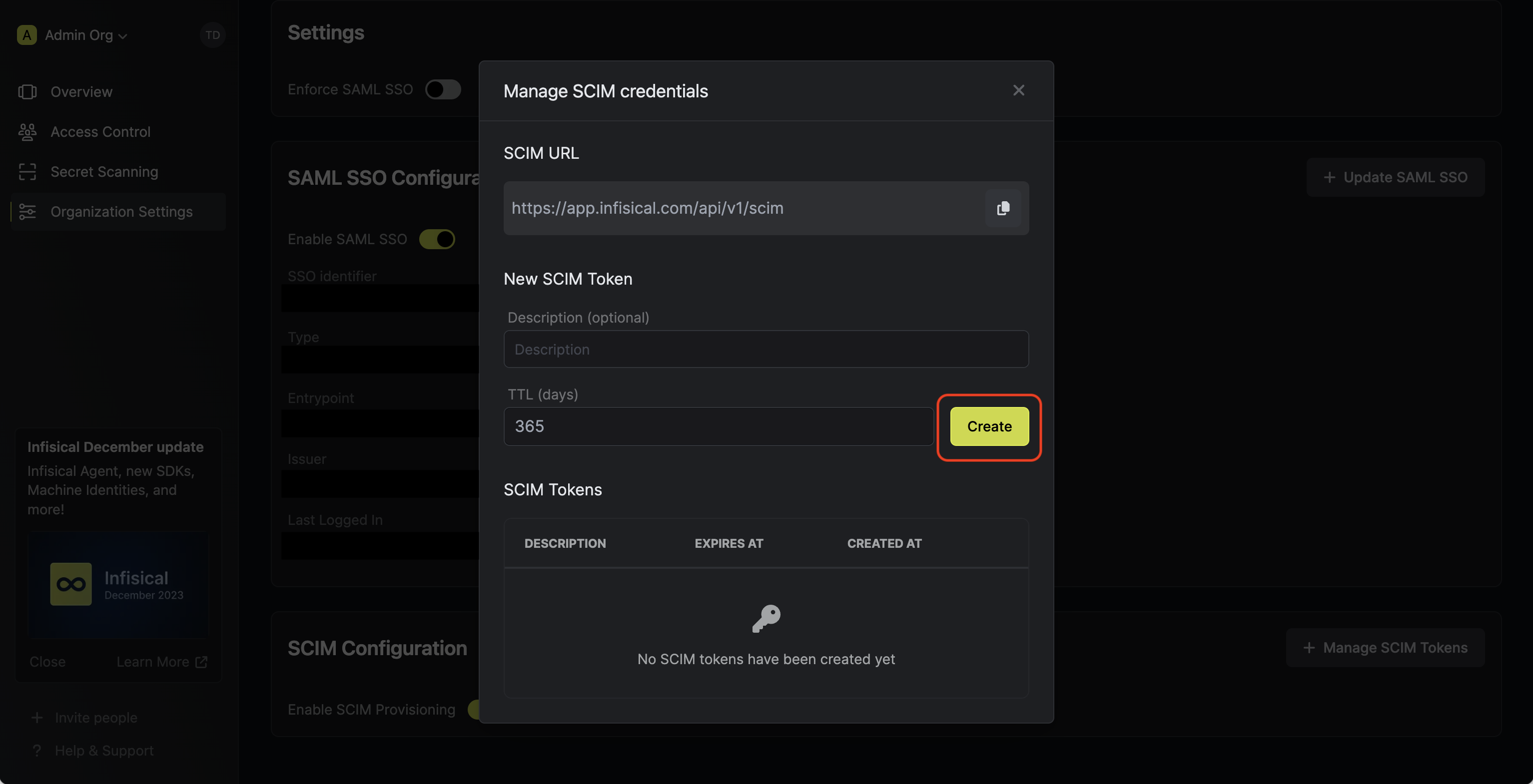
Next, copy the **SCIM URL** and **New SCIM Token** to use when configuring SCIM in JumpCloud.
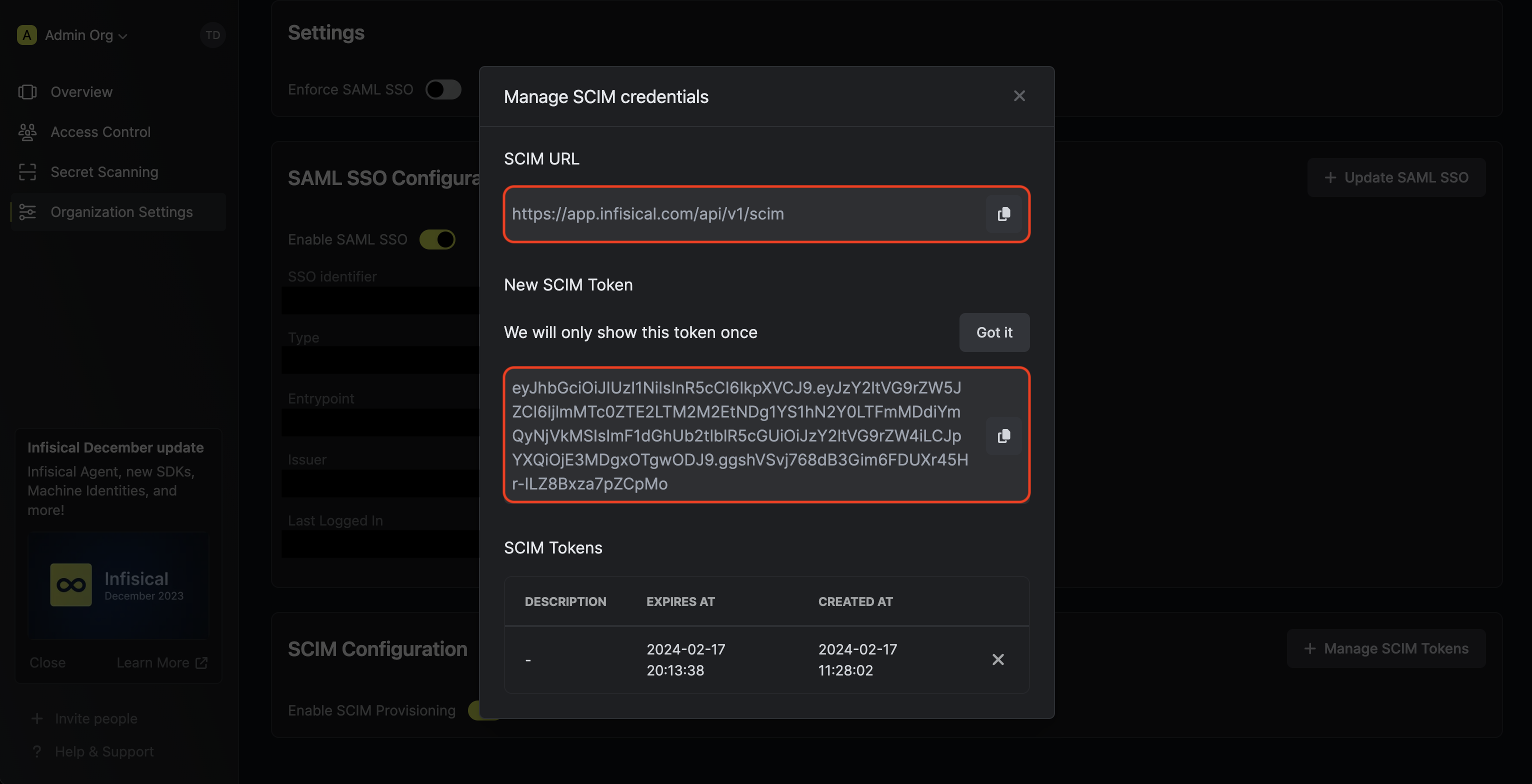
In JumpCloud, head to your Application > Identity Management > Configuration settings and make sure that
**API Type** is set to **SCIM API** and **SCIM Version** is set to **SCIM 2.0**.
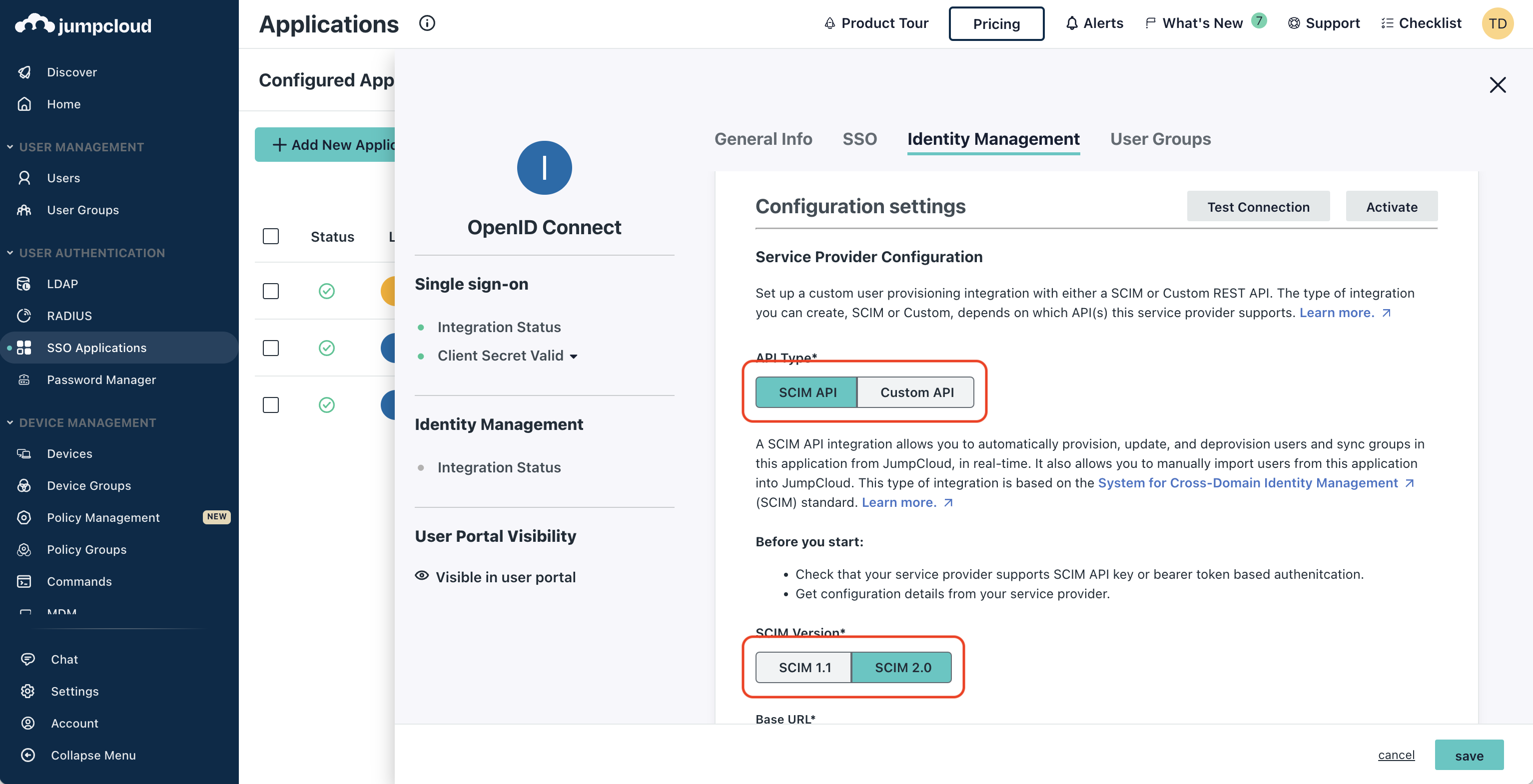
Next, set the following SCIM connection fields:
* Base URL: Input the **SCIM URL** from Step 1.
* Token Key: Input the **New SCIM Token** from Step 1.
* Test User Email: Input a test user email to be used by JumpCloud for testing the SCIM connection.
Alos, under HTTP Header > Authorization: Bearer, input the **New SCIM Token** from Step 1.
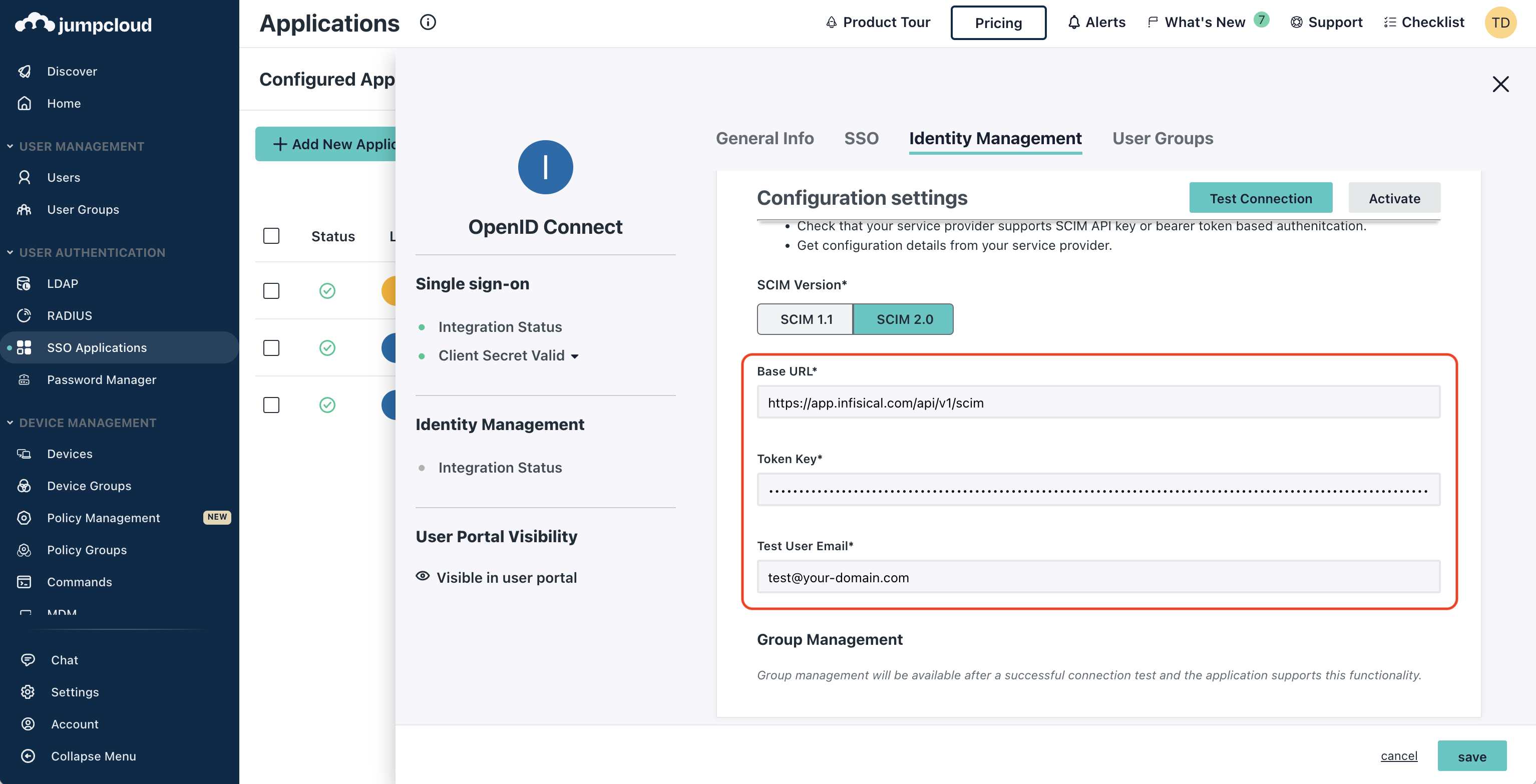
Next, press **Test Connection** to check that SCIM is configured properly. Finally, press **Activate**
to have JumpCloud start provisioning/deprovisioning users to Infisical.
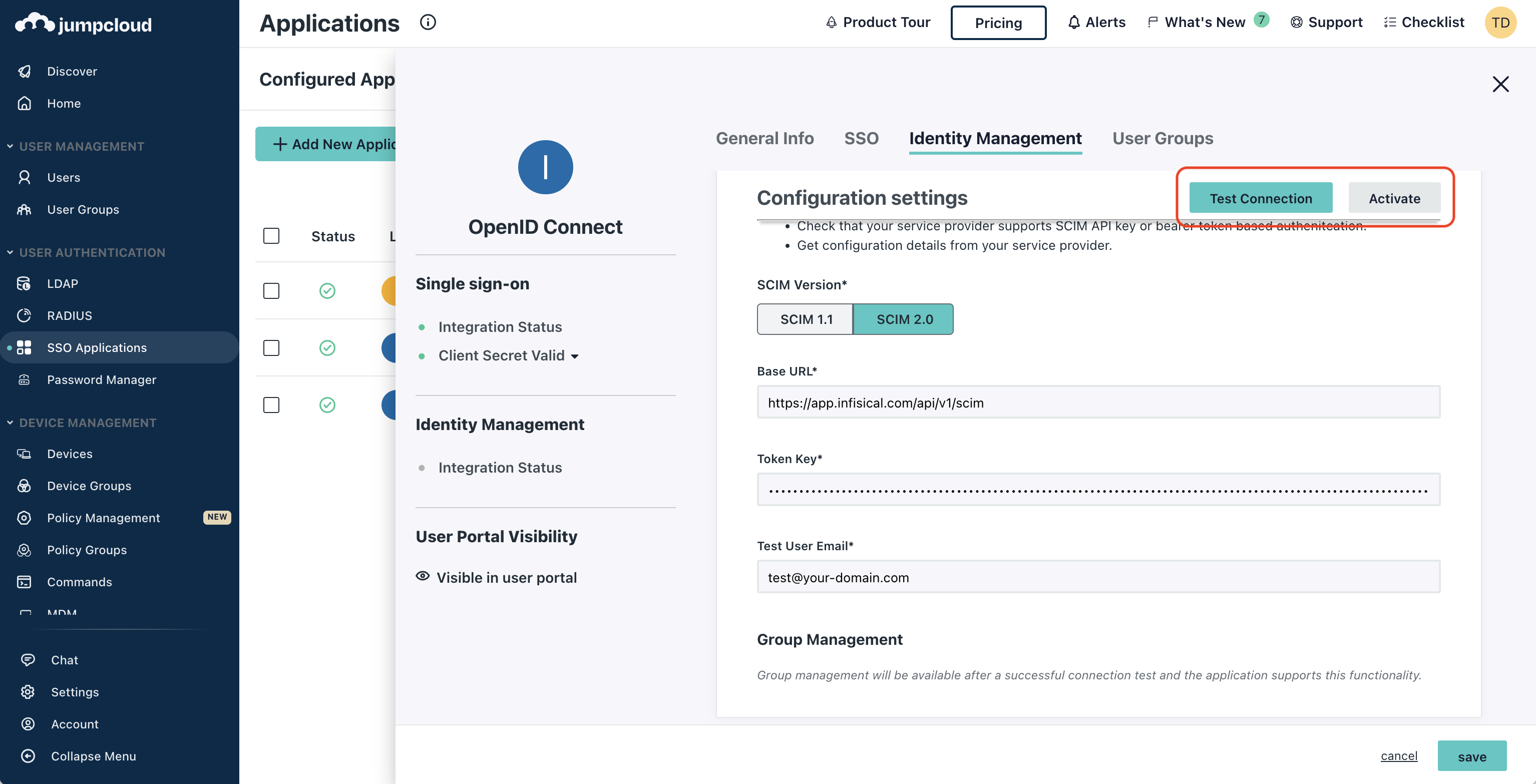
Now JumpCloud can provision/deprovision users and user groups to/from your organization in Infisical.
**FAQ**
Infisical's SCIM implmentation accounts for retaining the end-to-end encrypted architecture of Infisical because we decouple the **authentication** and **decryption** steps in the platform.
For this reason, SCIM-provisioned users are initialized but must finish setting up their account when logging in the first time by creating a master encryption/decryption key. With this implementation, IdPs and SCIM providers cannot and will not have access to the decryption key needed to decrypt your secrets.
# Okta SCIM
Learn how to configure SCIM provisioning with Okta for Infisical.
Okta SCIM provisioning is a paid feature.
If you're using Infisical Cloud, then it is available under the **Enterprise Tier**. If you're self-hosting Infisical,
then you should contact [sales@infisical.com](mailto:sales@infisical.com) to purchase an enterprise license to use it.
Prerequisites:
* [Configure Okta SAML for Infisical](/documentation/platform/sso/okta)
In Infisical, head to your Organization Settings > Authentication > SCIM Configuration and
press the **Enable SCIM provisioning** toggle to allow Okta to provision/deprovision users and user groups for your organization.
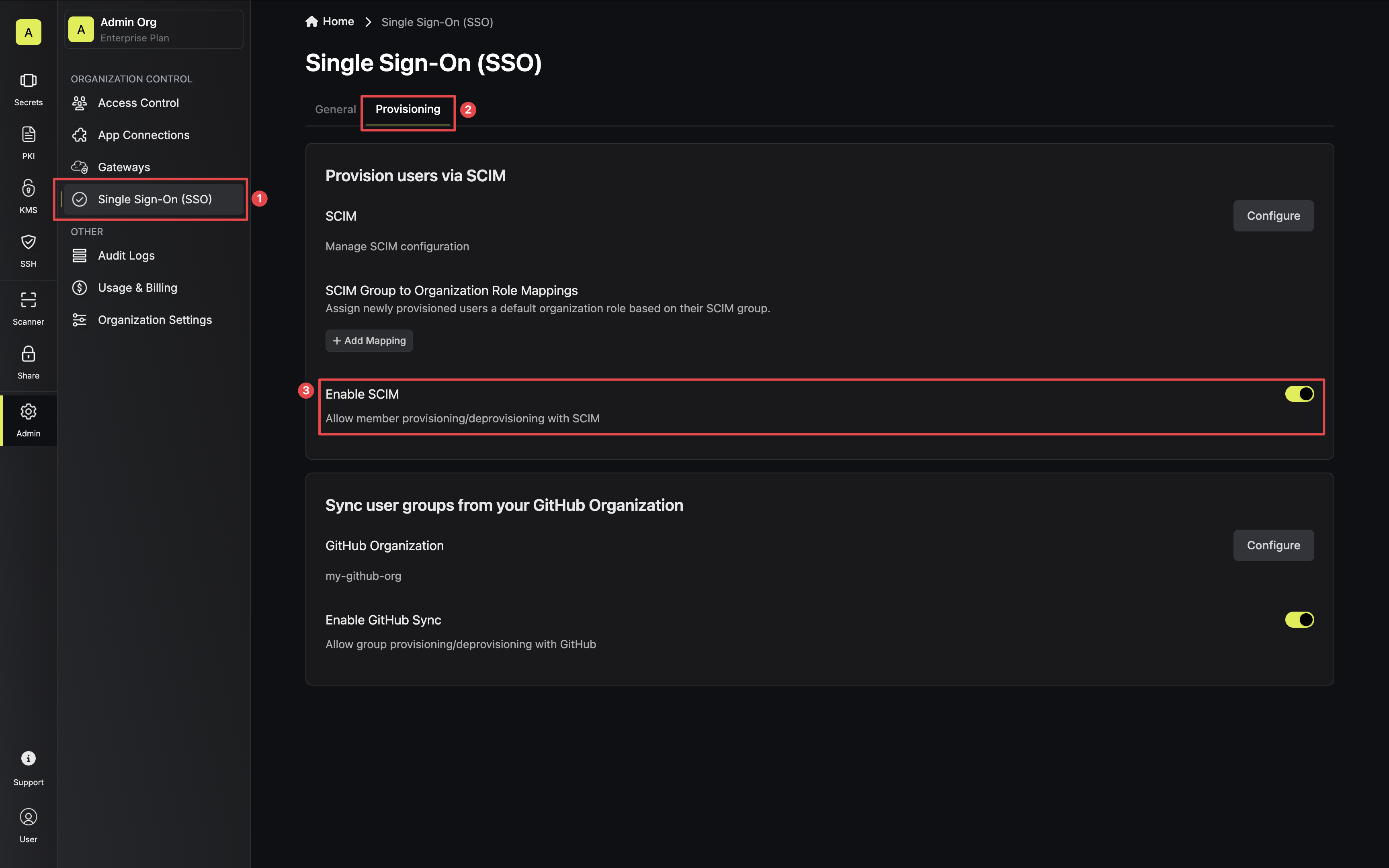
Next, press **Manage SCIM Tokens** and then **Create** to generate a SCIM token for Okta.
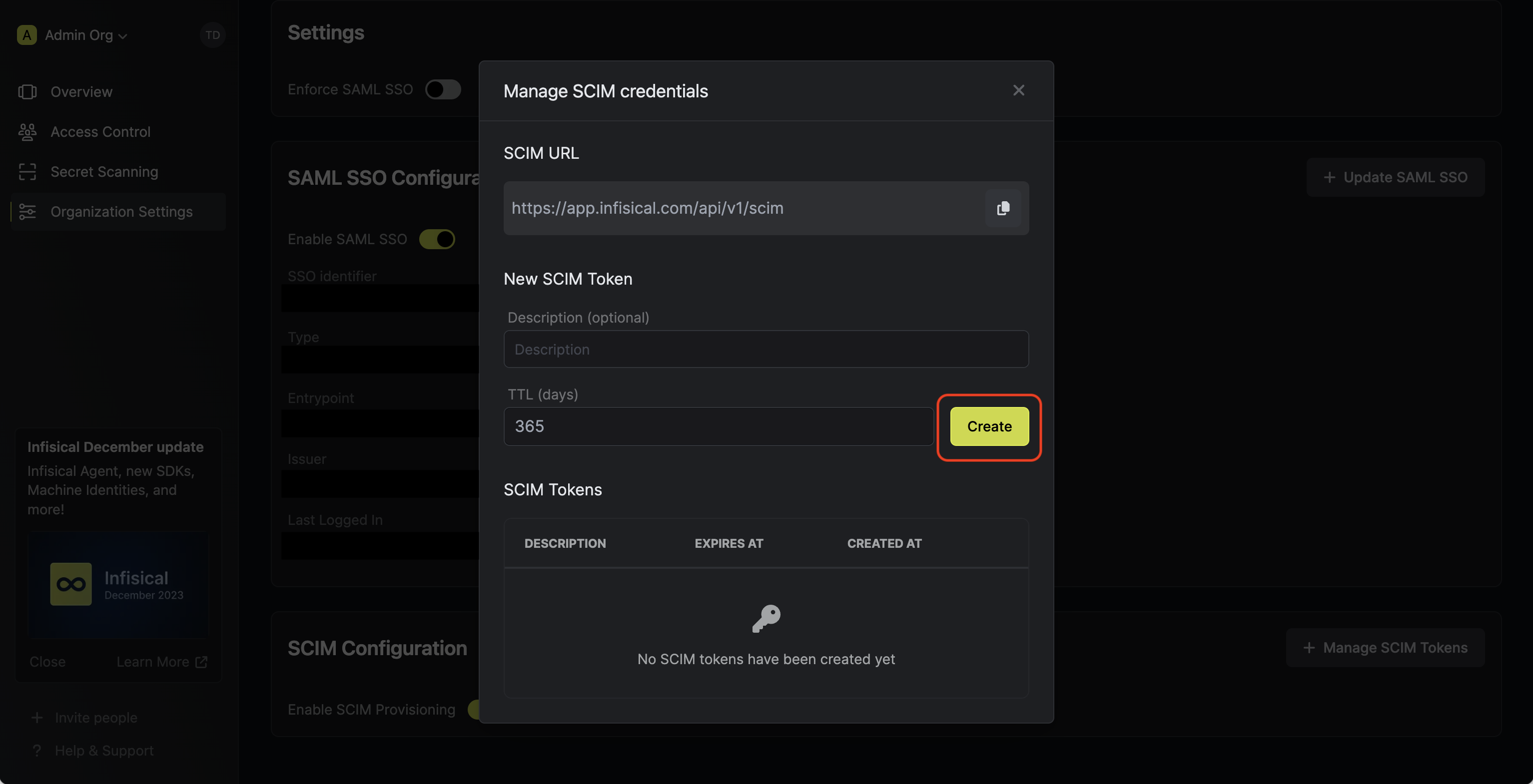
Next, copy the **SCIM URL** and **New SCIM Token** to use when configuring SCIM in Okta.
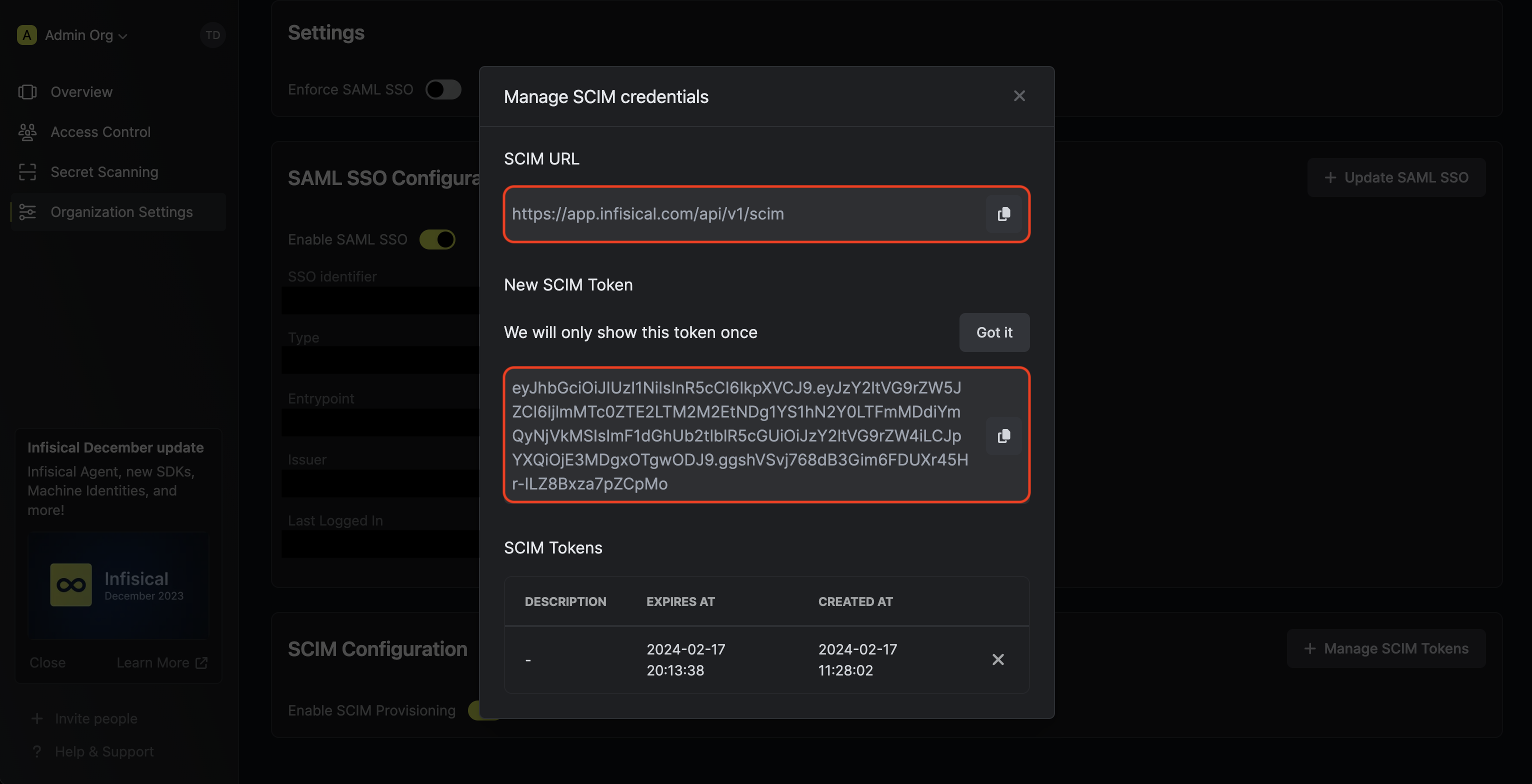
In Okta, head to your Application > General > App Settings. Next, select **Edit** and check the box
labled **Enable SCIM provisioning**.
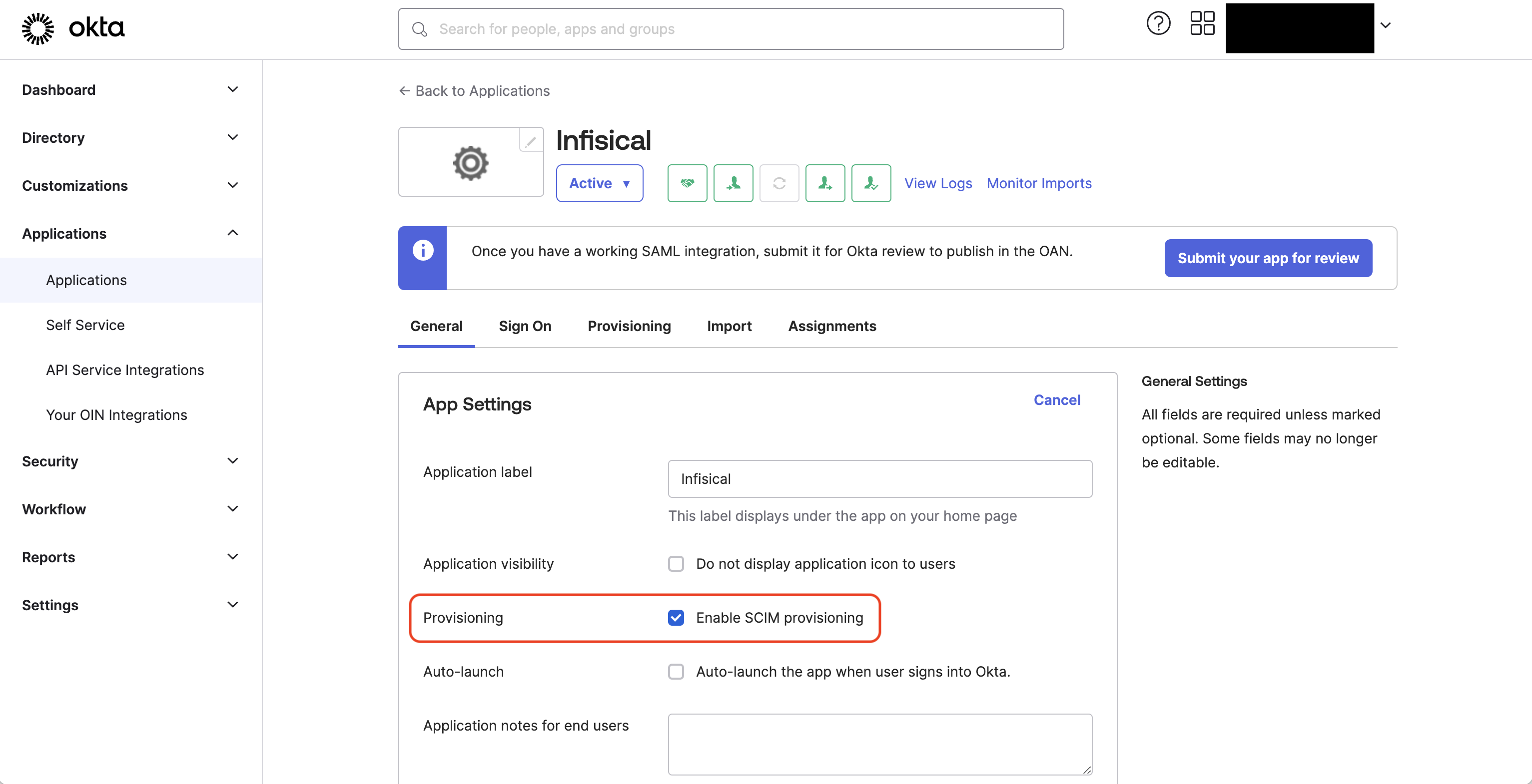
Next, head to Provisioning > Integration and set the following SCIM connection fields:
* SCIM connector base URL: Input the **SCIM URL** from Step 1.
* Unique identifier field for users: Input `email`.
* Supported provisioning actions: Select **Push New Users**, **Push Profile Updates**, and **Push Groups**.
* Authentication Mode: `HTTP Header`.
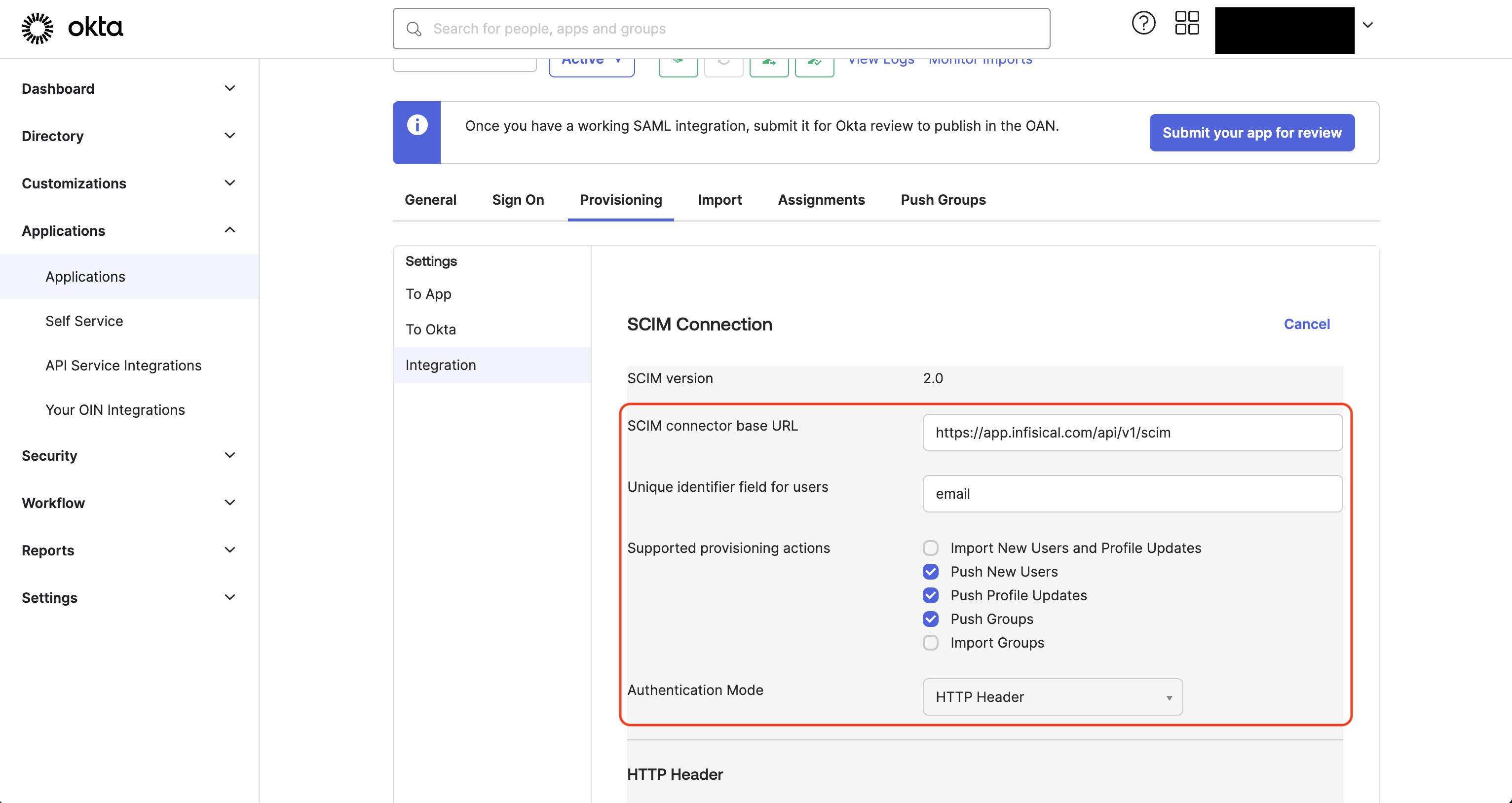
Under HTTP Header > Authorization: Bearer, input the **New SCIM Token** from Step 1.
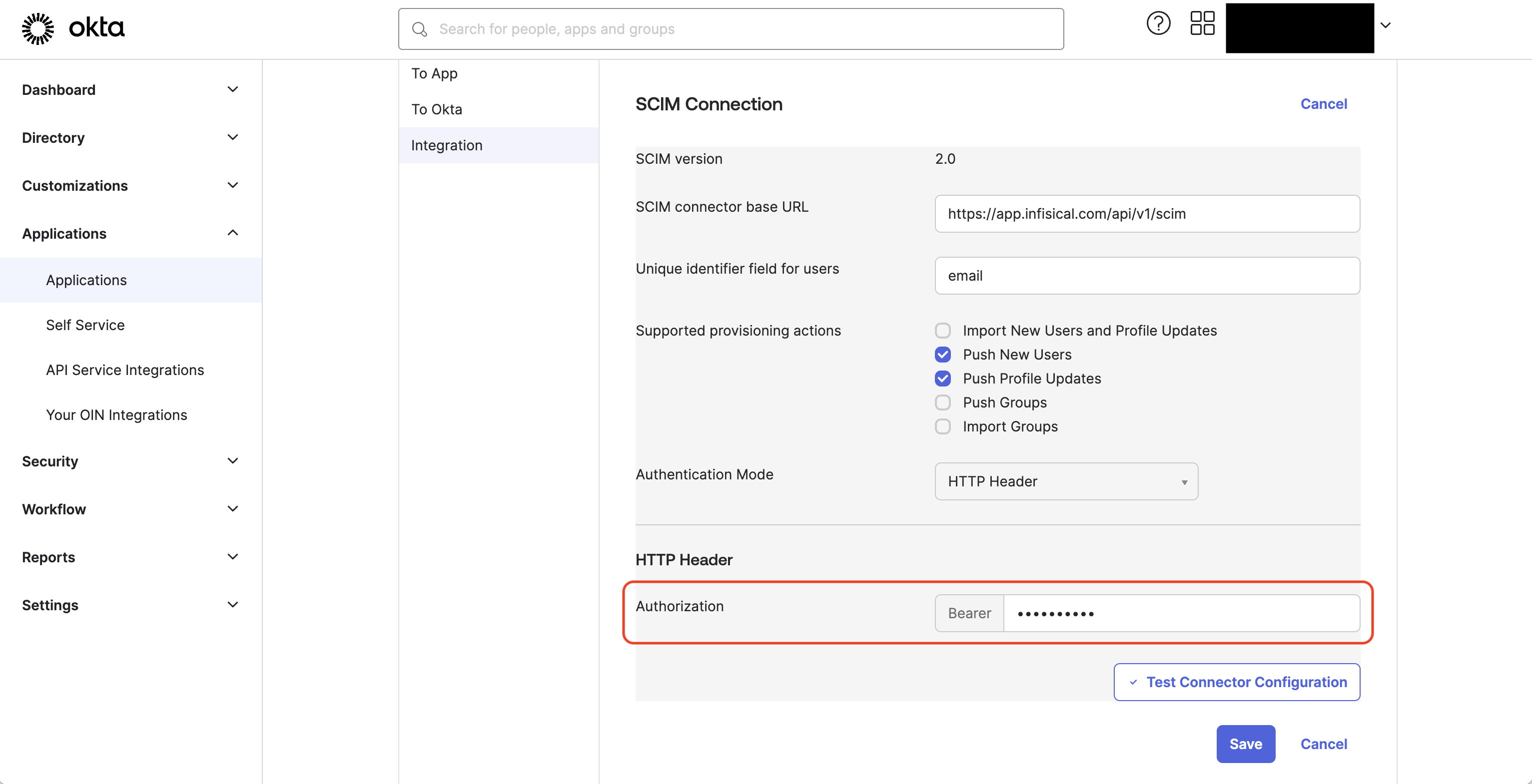
Next, press **Test Connector Configuration** to check that SCIM is configured properly.
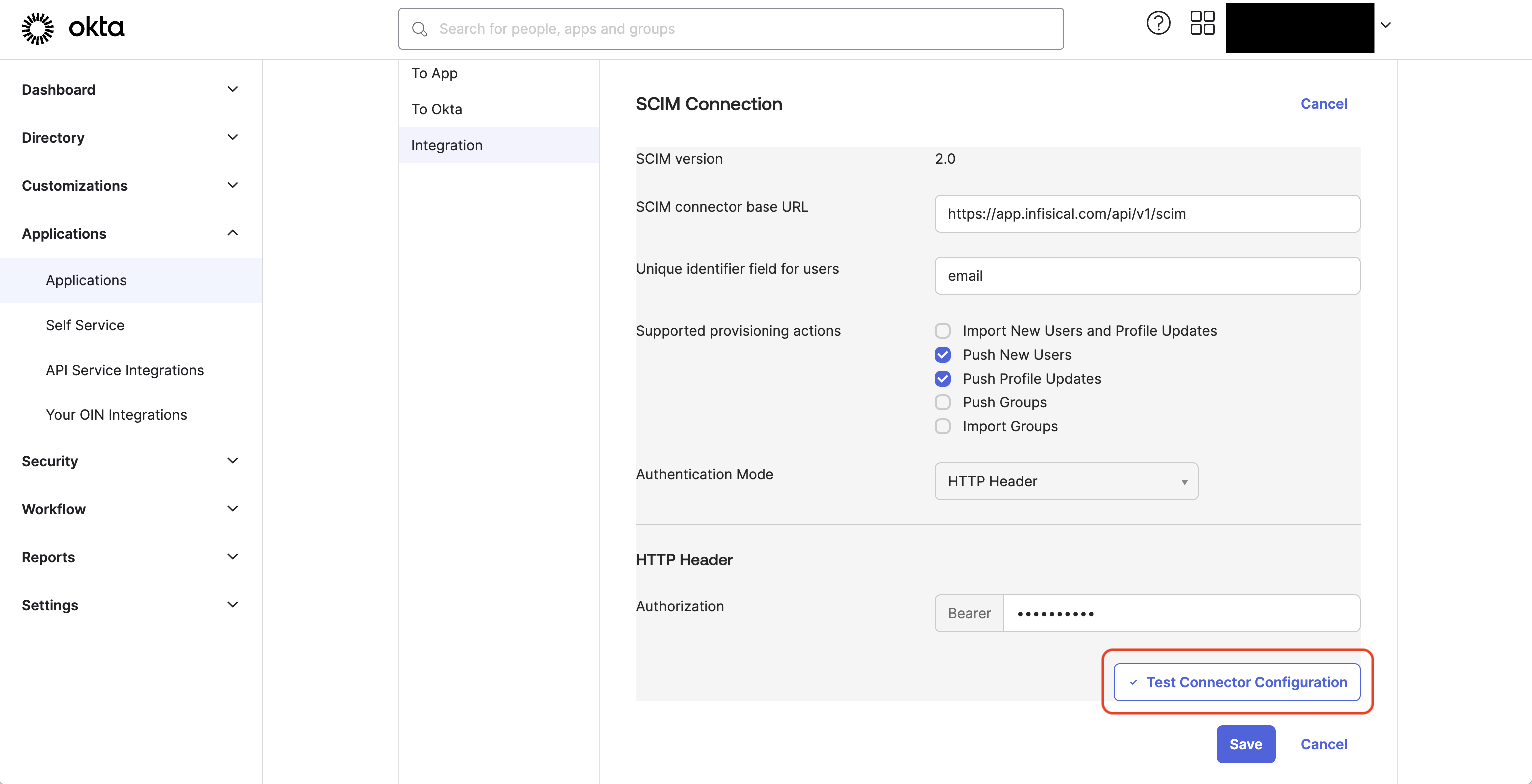
Next, head to Provisioning > To App and check the boxes labeled **Enable** for **Create Users**, **Update User Attributes**, and **Deactivate Users**.
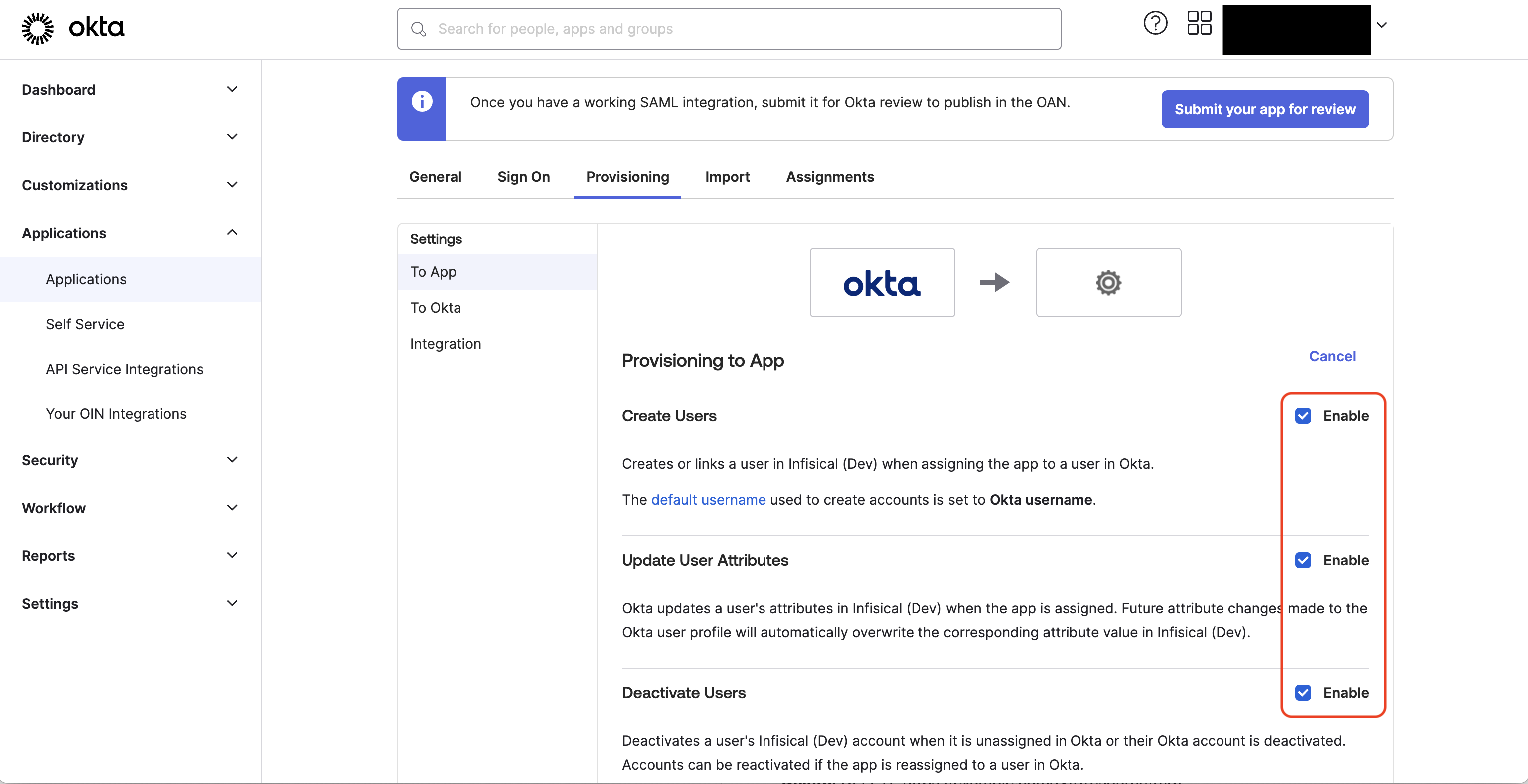
Now Okta can provision/deprovision users and user groups to/from your organization in Infisical.
**FAQ**
Infisical's SCIM implmentation accounts for retaining the end-to-end encrypted architecture of Infisical because we decouple the **authentication** and **decryption** steps in the platform.
For this reason, SCIM-provisioned users are initialized but must finish setting up their account when logging in the first time by creating a master encryption/decryption key. With this implementation, IdPs and SCIM providers cannot and will not have access to the decryption key needed to decrypt your secrets.
# SCIM Overview
Learn how to provision users for Infisical via SCIM.
SCIM provisioning is a paid feature.
If you're using Infisical Cloud, then it is available under the **Enterprise Tier**. If you're self-hosting Infisical,
then you should contact [sales@infisical.com](mailto:sales@infisical.com) to purchase an enterprise license to use it.
You can configure your organization in Infisical to have users and user groups be provisioned/deprovisioned using [SCIM](https://scim.cloud/#Implementations2) via providers like Okta, Azure, JumpCloud, etc.
* Provisioning: The SCIM provider pushes user information to Infisical. If the user exists in Infisical, Infisical sends an email invitation to add them to the relevant organization in Infisical; if not, Infisical initializes a new user and sends them an email invitation to finish setting up their account in the organization.
* Deprovisioning: The SCIM provider instructs Infisical to remove user(s) from an organization in Infisical.
SCIM providers:
* [Okta SCIM](/documentation/platform/scim/okta)
* [Azure SCIM](/documentation/platform/scim/azure)
* [JumpCloud SCIM](/documentation/platform/scim/jumpcloud)
**FAQ**
Infisical's SCIM implementation accounts for retaining the end-to-end encrypted architecture of Infisical because we decouple the **authentication** and **decryption** steps in the platform.
For this reason, SCIM-provisioned users are initialized but must finish setting up their account when logging in the first time by creating a master encryption/decryption key. With this implementation, IdPs and SCIM providers cannot and will not have access to the decryption key needed to decrypt your secrets.
# Secret Referencing and Importing
Learn the fundamentals of secret referencing and importing in Infisical.
## Secret Referencing
Infisical's secret referencing functionality makes it possible to reference the value of a "base" secret when defining the value of another secret.
This means that updating the value of a base secret propagates directly to other secrets whose values depend on the base secret.
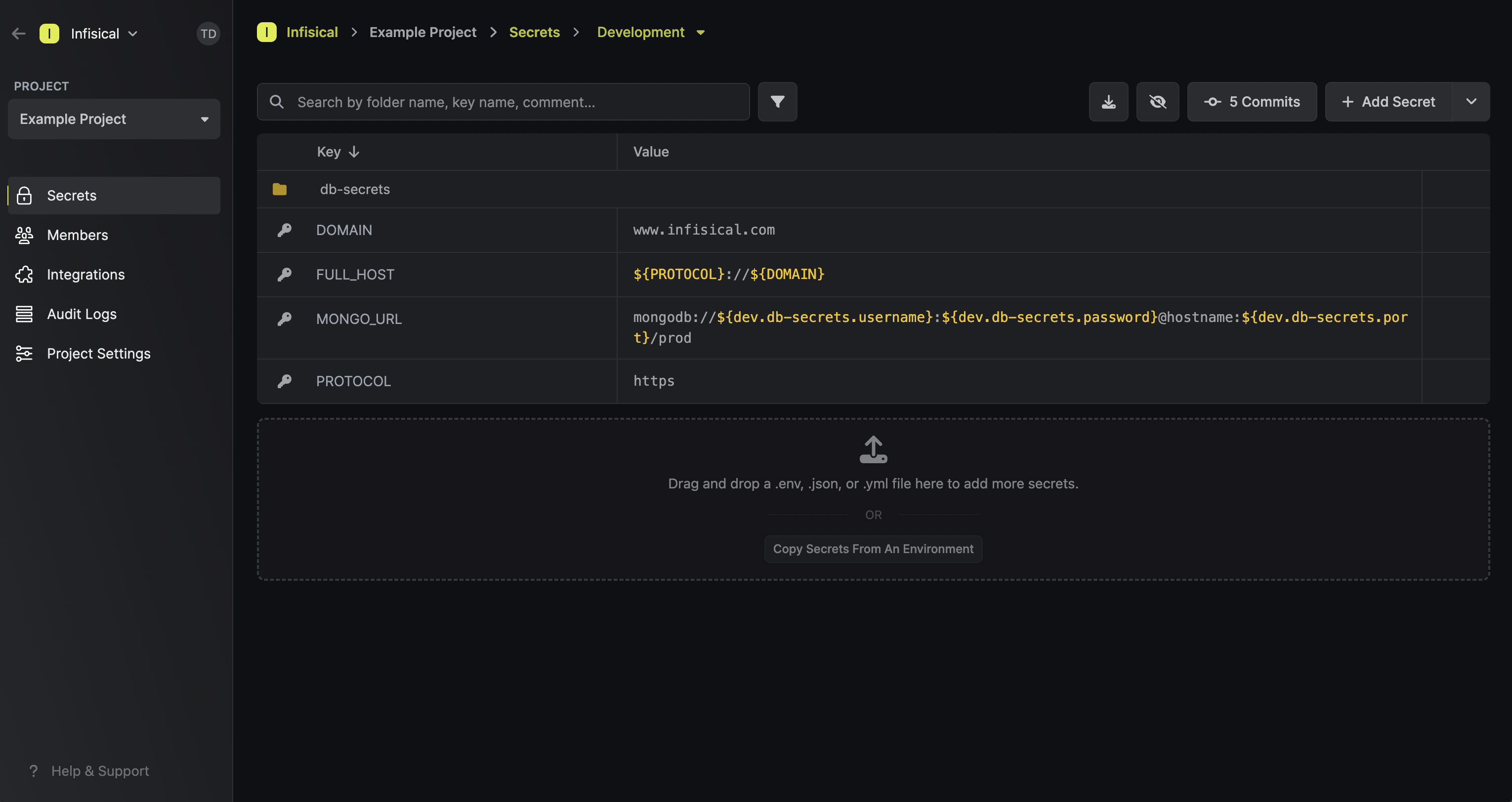
Since secret referencing works by reconstructing values back on the client side, the client, be it a user, service token, or a machine identity, fetching back secrets
must be permissioned access to all base and dependent secrets.
For example, to access some secret `A` whose values depend on secrets `B` and `C` from different scopes, a client must have `read` access to the scopes of secrets `A`, `B`, and `C`.
### Syntax
When defining a secret reference, interpolation syntax is used to define references to secrets in other environments and [folders](./folder).
Suppose you have some secret `MY_SECRET` at the root of some environment and want to reference part of its value from another base secret `BASE_SECRET` located elsewhere.
Then consider the following scenarios:
* If `BASE_SECRET` is in the same environment and folder as `MY_SECRET`, then you'd reference it using `${BASE_SECRET}`.
* If `BASE_SECRET` is at the root of another environment with the slug `dev`, then you'd reference it using `${dev.MY_SECRET}`.
Here are a few more helpful examples for how to reference secrets in different contexts:
| Reference syntax | Environment | Folder | Secret Key |
| ----------------------- | ----------- | ----------------------------- | ---------- |
| `${KEY1}` | same env | same folder | KEY1 |
| `${dev.KEY2}` | `dev` | `/` (root of dev environment) | KEY2 |
| `${prod.frontend.KEY2}` | `prod` | `/frontend` | KEY2 |
## Secret Imports
Infisical's Secret Imports functionality makes it possible to import the secrets from another environment or folder into the current folder context.
This can be useful if you have common secrets that need to be available across multiple environments/folders.
To add a secret import, press the downward chevron to the right of the **Add Secret** button; then press on the **Add Import** button.
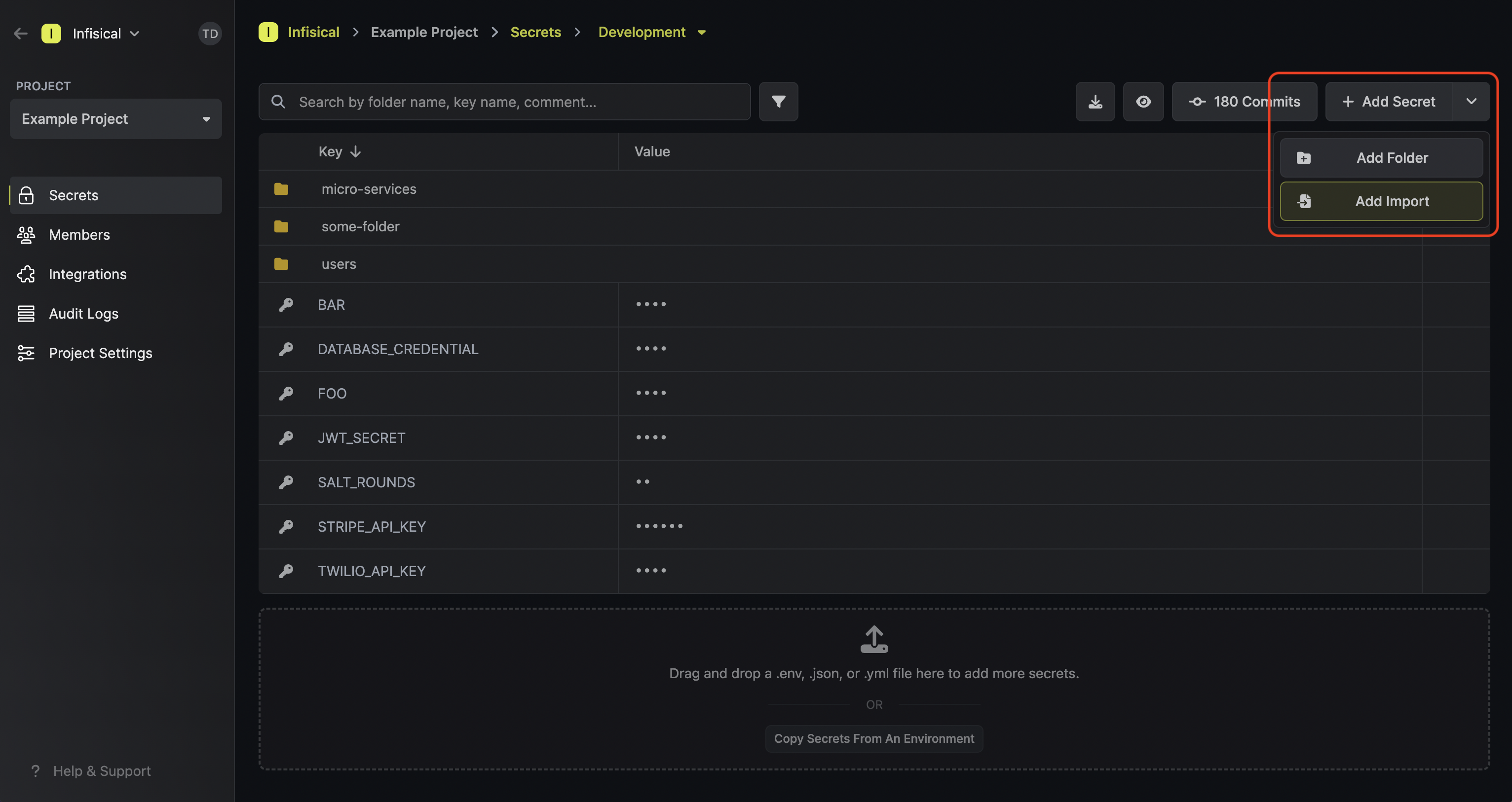
Once added, a secret import will show up with a green import icon on the secrets dashboard.
In the example below, you can see that the items in the path `/some-folder` are being imported into
the current folder context.
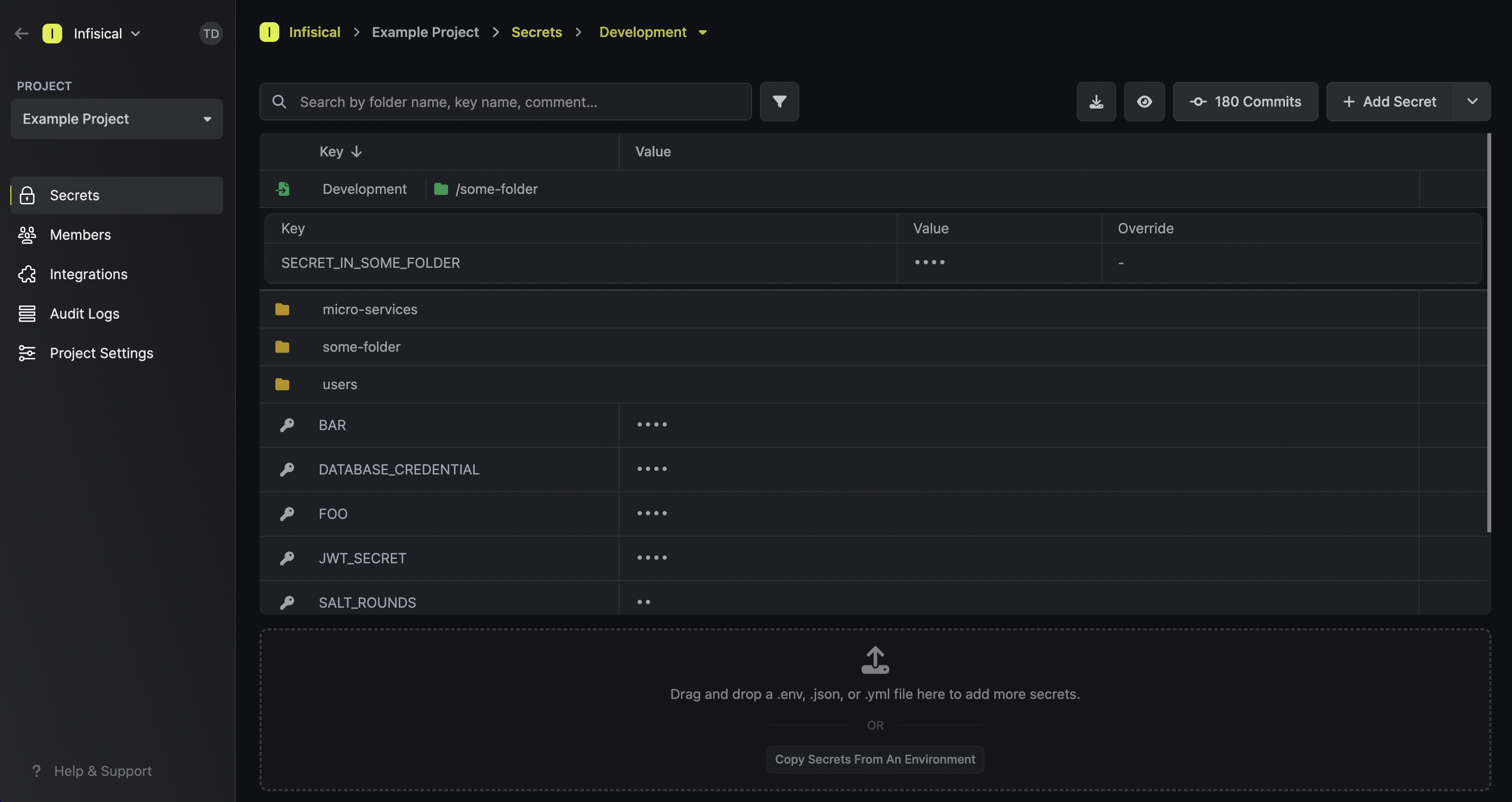
To delete a secret import, hover over it and press the **X** button that appears on the right side.
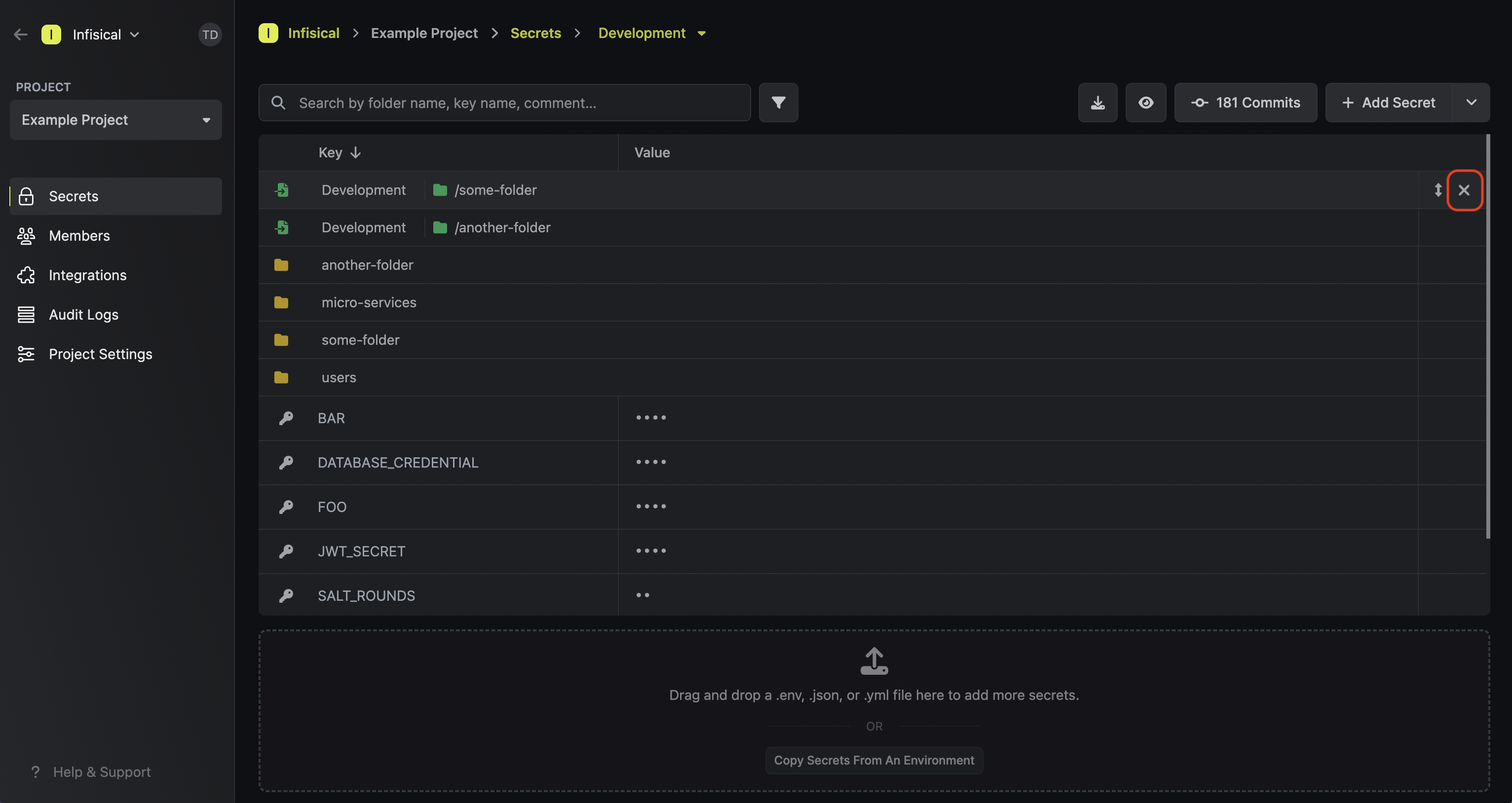
Lastly, note that the order of secret imports matters. If two secret imports contain secrets with the same name, then the secret value from the bottom-most secret import is taken — "the last one wins."
To reorder a secret import, hover over it and drag the arrows handle to the position you want.
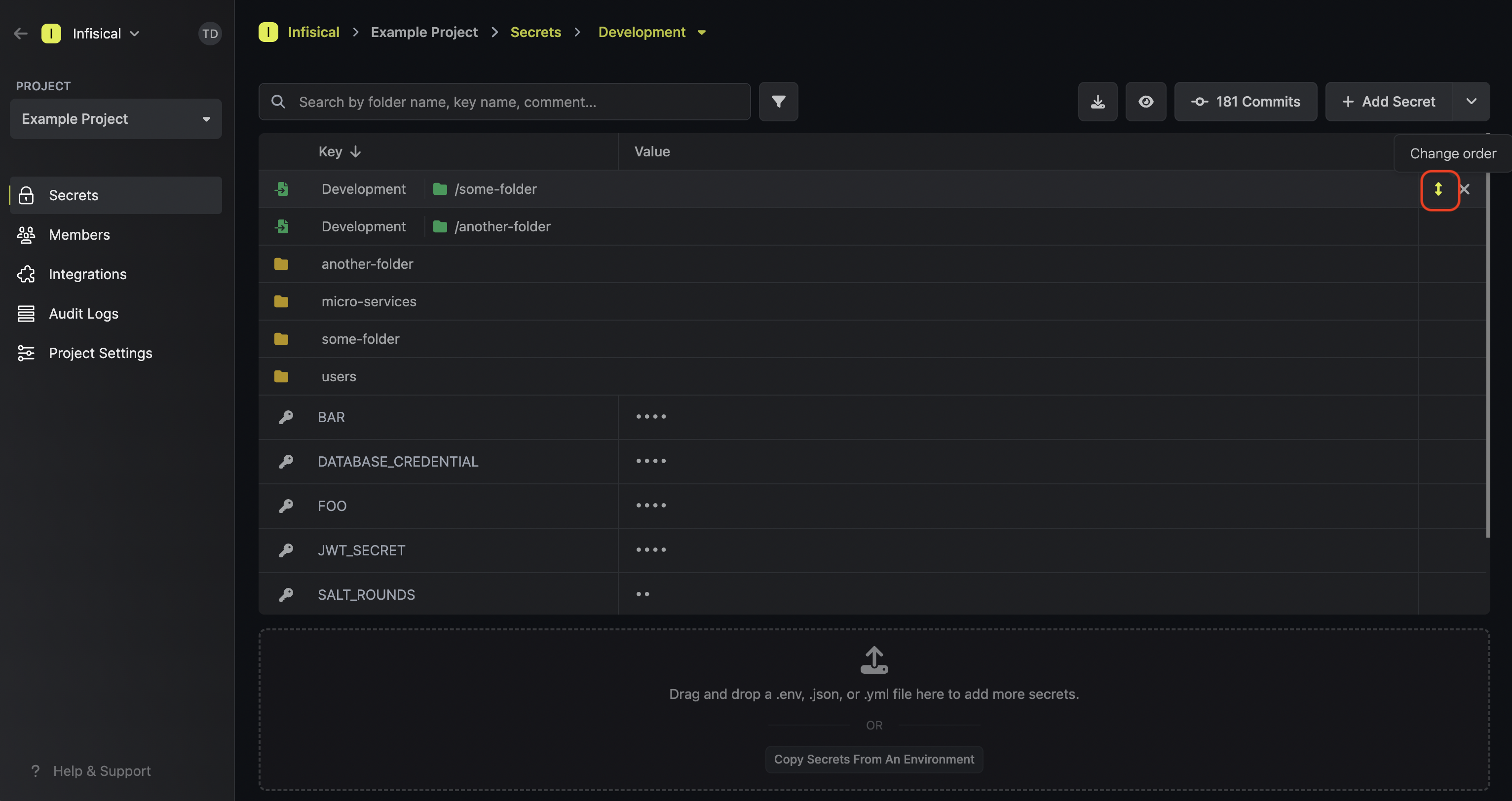
# AWS IAM User
Learn how to automatically rotate Access Key Id and Secret Key of AWS IAM Users.
Infisical's AWS IAM User secret rotation capability lets you update the **Access key** and **Secret access key** credentials of a target IAM user from within Infisical
at a specified interval or on-demand.
## Workflow
The typical workflow for using the AWS IAM User rotation strategy consists of four steps:
1. Creating the target IAM user whose credentials you wish to rotate.
2. Creating the managing IAM user used by Infisical to rotate the credentials of the target IAM user.
3. Configuring the rotation strategy in Infisical with the credentials of the managing IAM user.
4. Pressing the **Rotate** button in the Infisical dashboard to trigger the rotation of the target IAM user's credentials. The strategy can also be configured to rotate the credentials automatically at a specified interval.
In the following steps, we explore the end-to-end workflow for setting up this strategy in Infisical.
To begin, create an IAM user whose credentials you wish to rotate. If you already have an IAM user,
then you can skip this step.
Next, create another IAM user to be used by Infisical to rotate the credentials of the IAM user in the previous step.
2.1. In your AWS console, head to IAM > Access management > Users and press **Create user**.
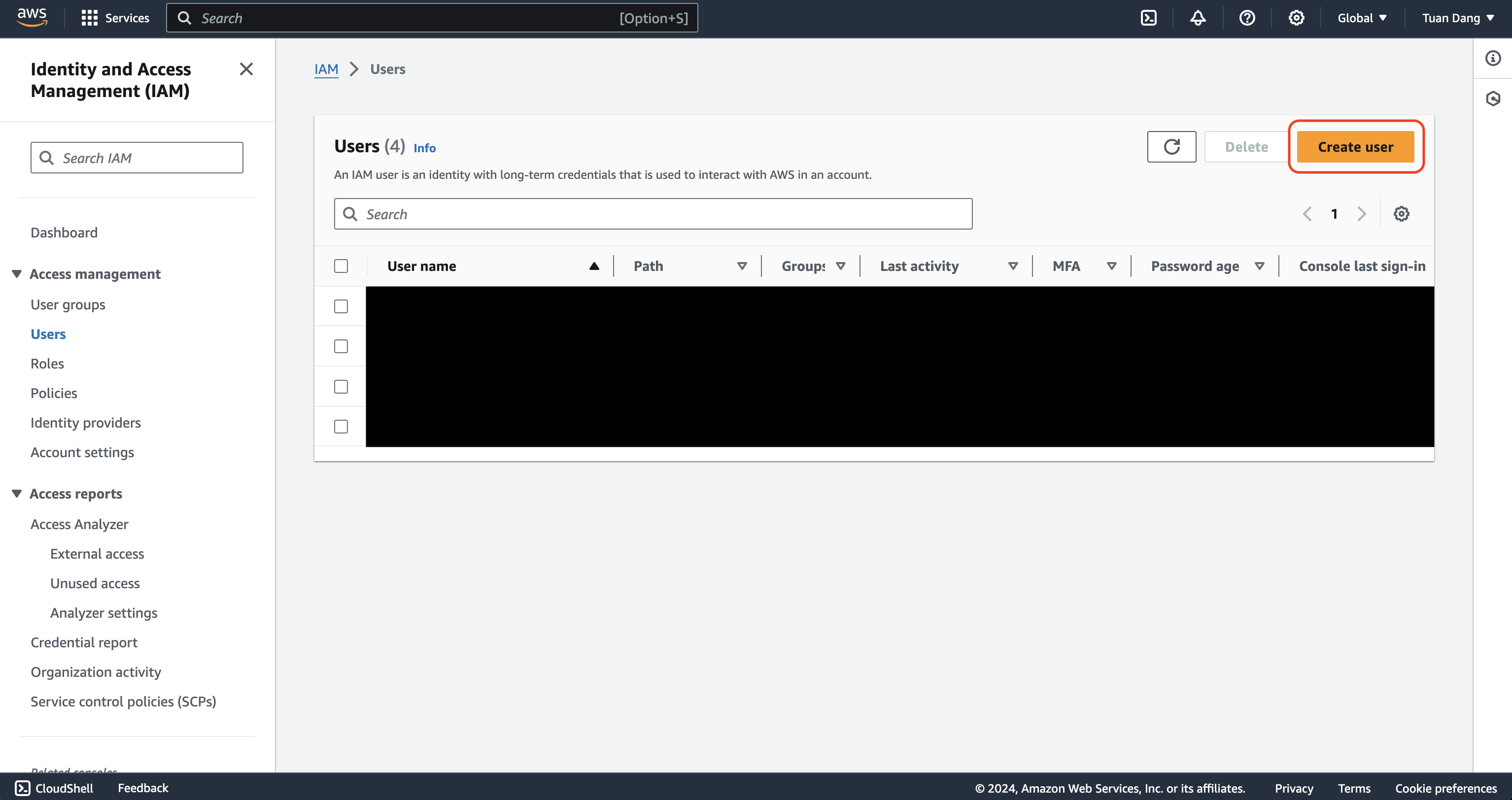
2.2. Next, give the user a username like **infisical-rotation-manager** and press **Next**.
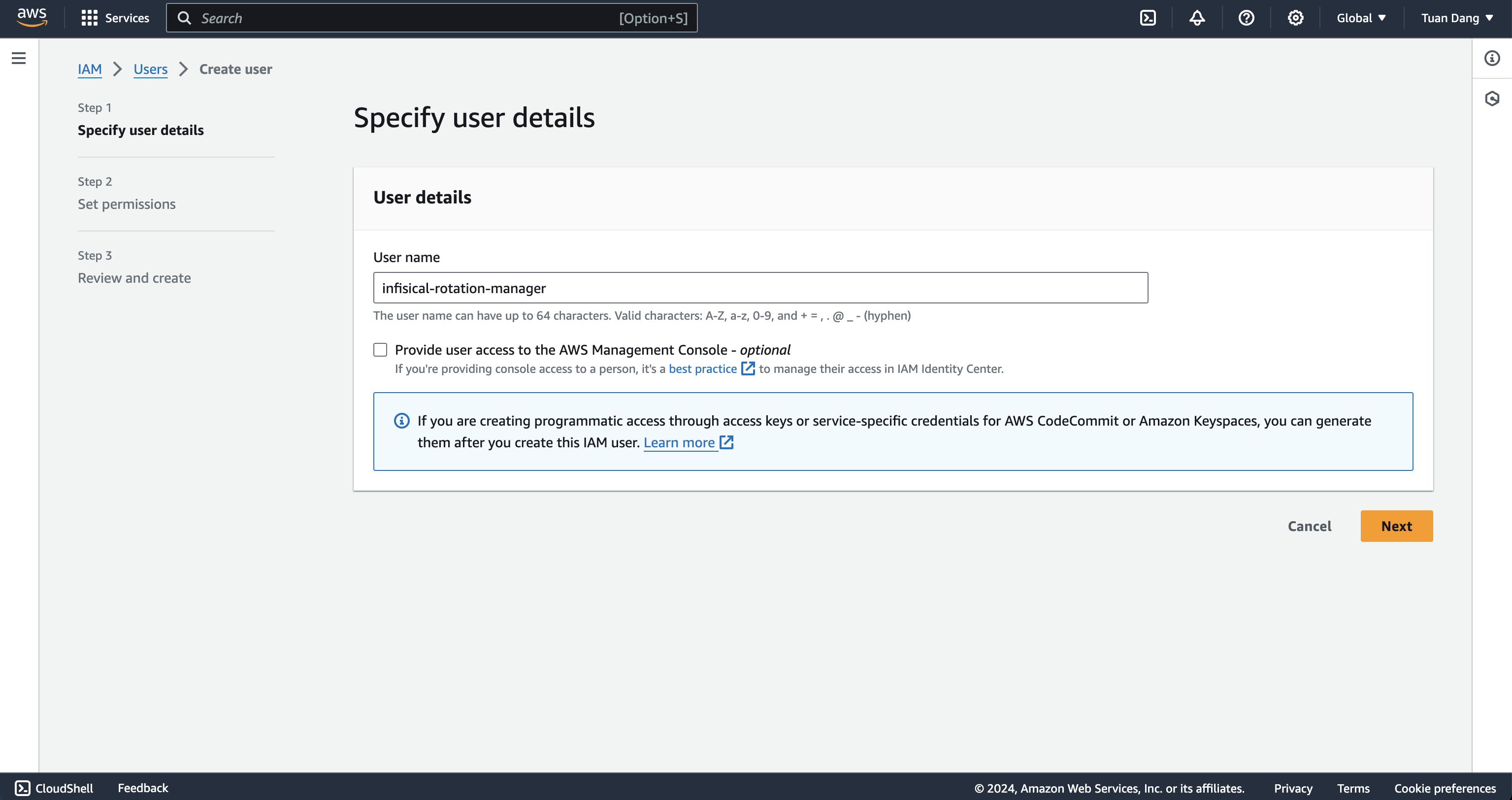
2.3. Next, in the **Set permissions** step, select **Attach policies directly** and then press **Create policy**.
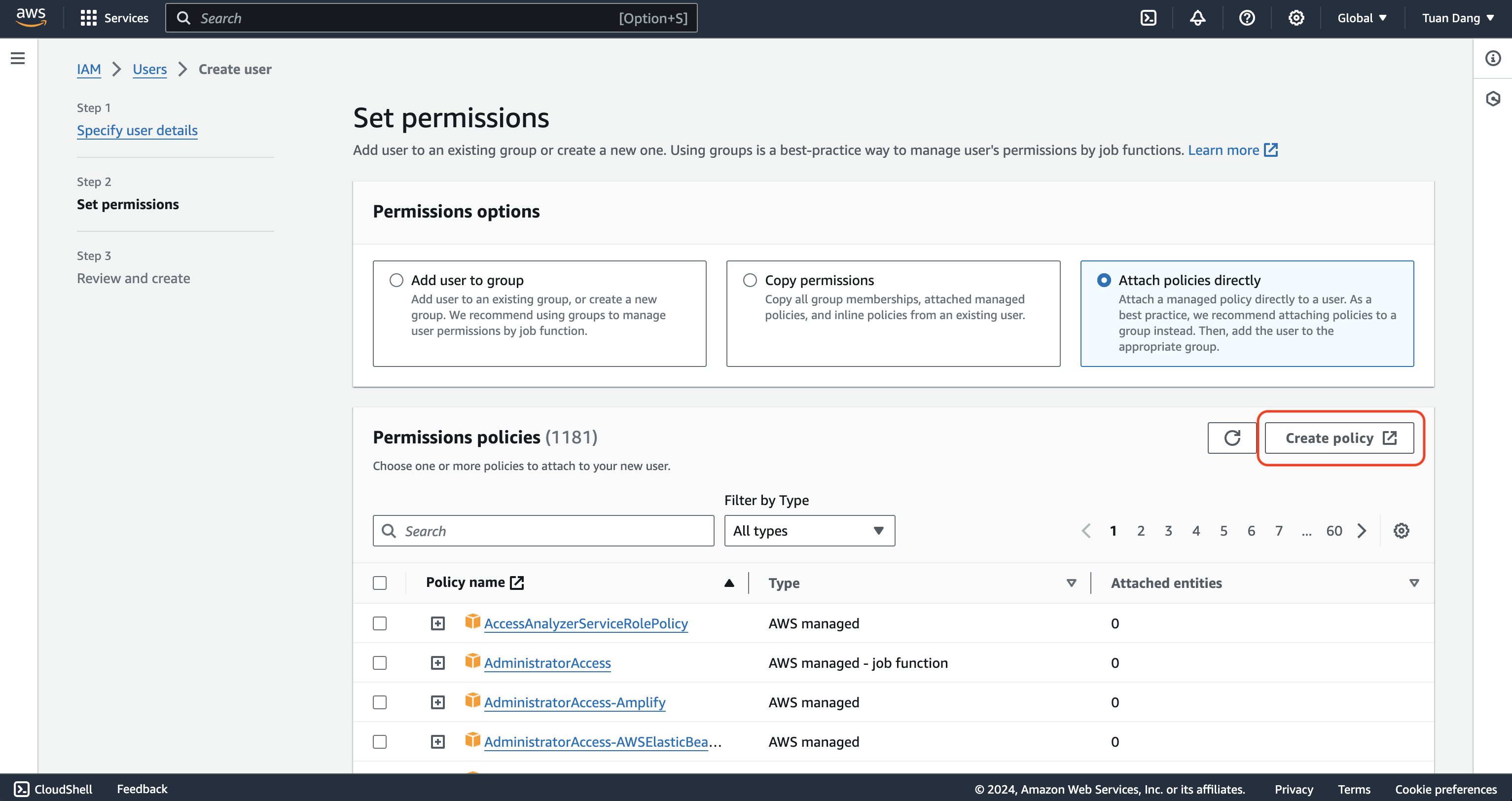
2.4. Next, in the **Policy editor**, paste the following JSON and press **Next**:
```json
{
"Version": "2012-10-17",
"Statement": [
{
"Sid": "VisualEditor0",
"Effect": "Allow",
"Action": [
"iam:DeleteAccessKey",
"iam:GetAccessKeyLastUsed",
"iam:CreateAccessKey"
],
"Resource": "*"
}
]
}
```
The IAM policy above uses the wildcard option in Resource: "\*".
You may want to restrict the policy to a specific path, and make any adjustments as necessary, to control access for the managing user in production.
Read more about this [here](https://aws.amazon.com/blogs/security/optimize-aws-administration-with-iam-paths/).
In the **Review and create** step, give the policy a name like **infisical-rotation-manager**, press **Create policy** to finish creating the policy.
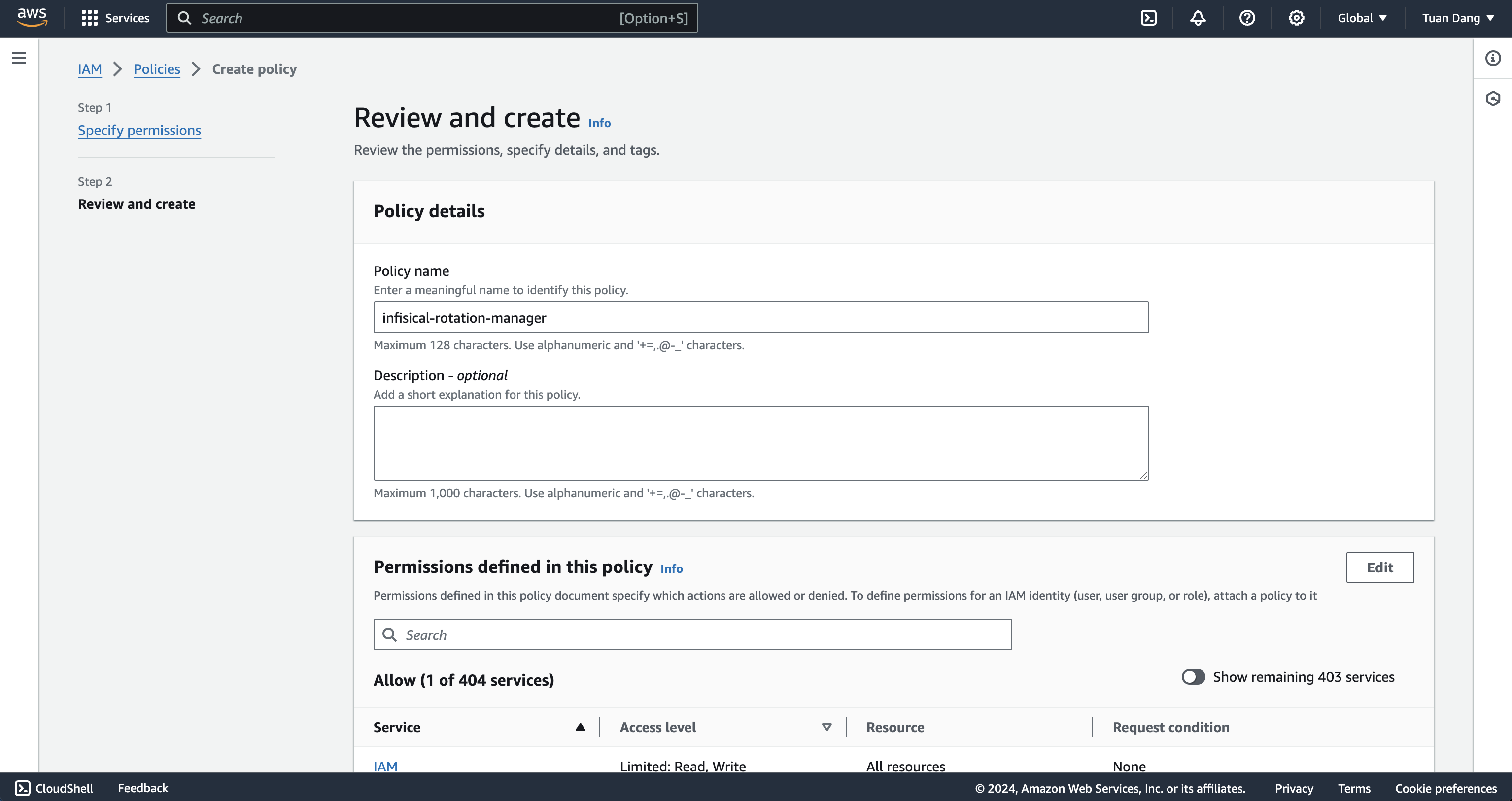
2.5. Back in the **Set permissions** step from step 2.3, refresh the policy list and search for the policy you just created from step 2.4.
Select the policy and press **Next**.
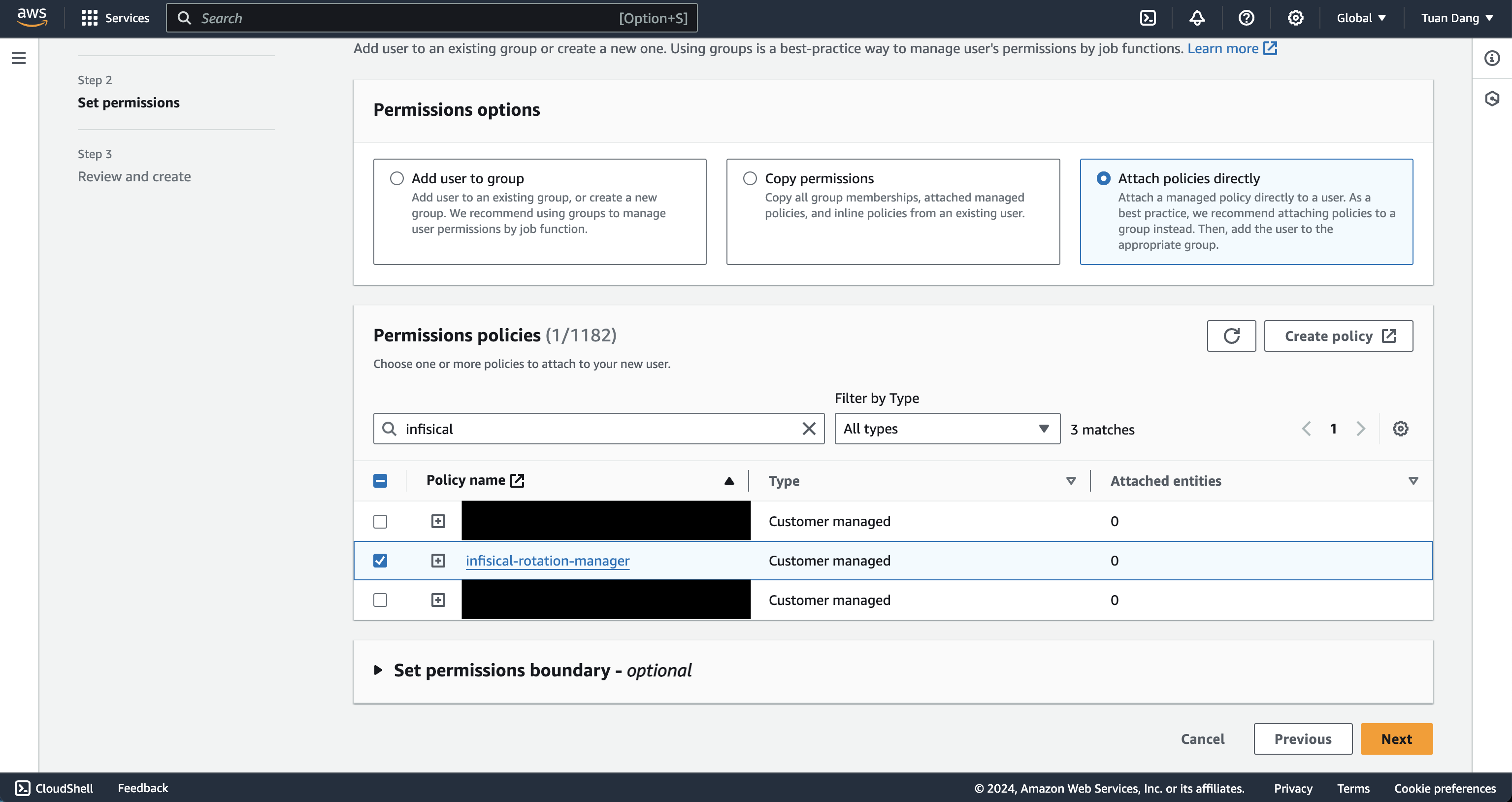
In the **Review and create** step, press **Create user** to finish creating the IAM user.
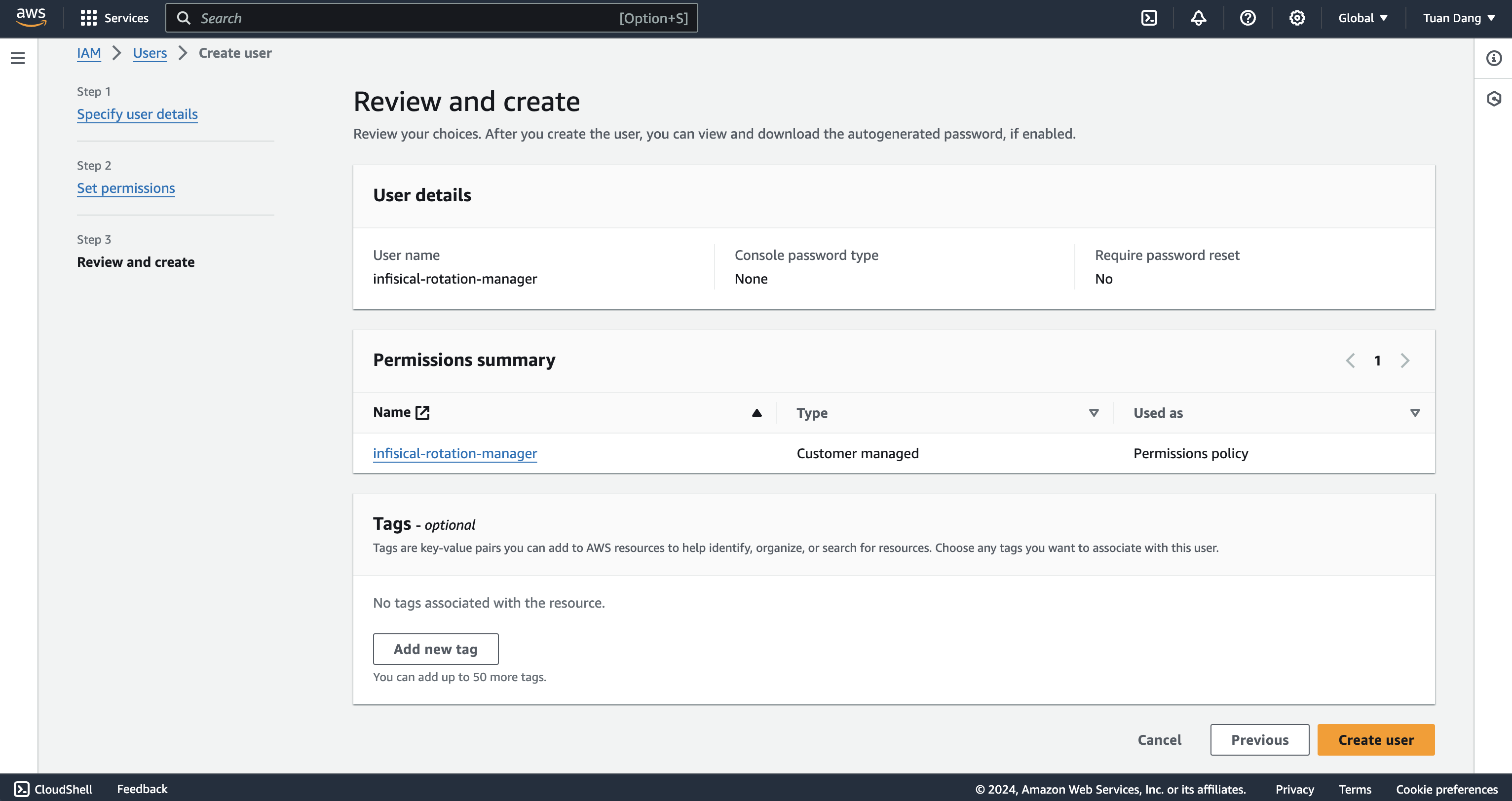
2.5. Having created the user, head to its Security credentials > Access keys and press **Create access key**.
Follow the subsequent steps to create the **access key** and **secret access key** credential pair for the user.
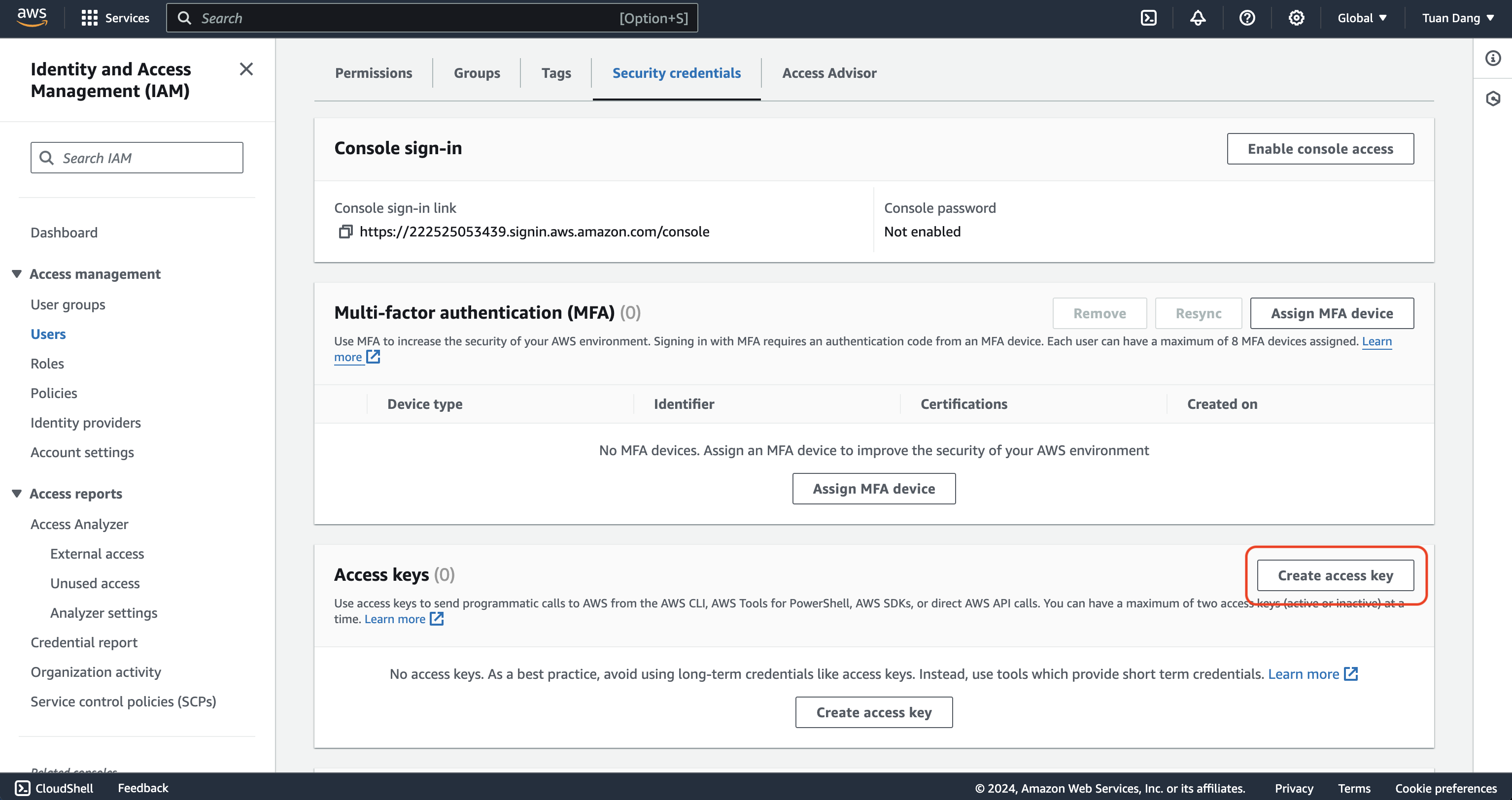
At the end of the flow, copy the **Access key** and **Secret access key** to use when configuring the AWS IAM User rotation strategy back in Infisical next.
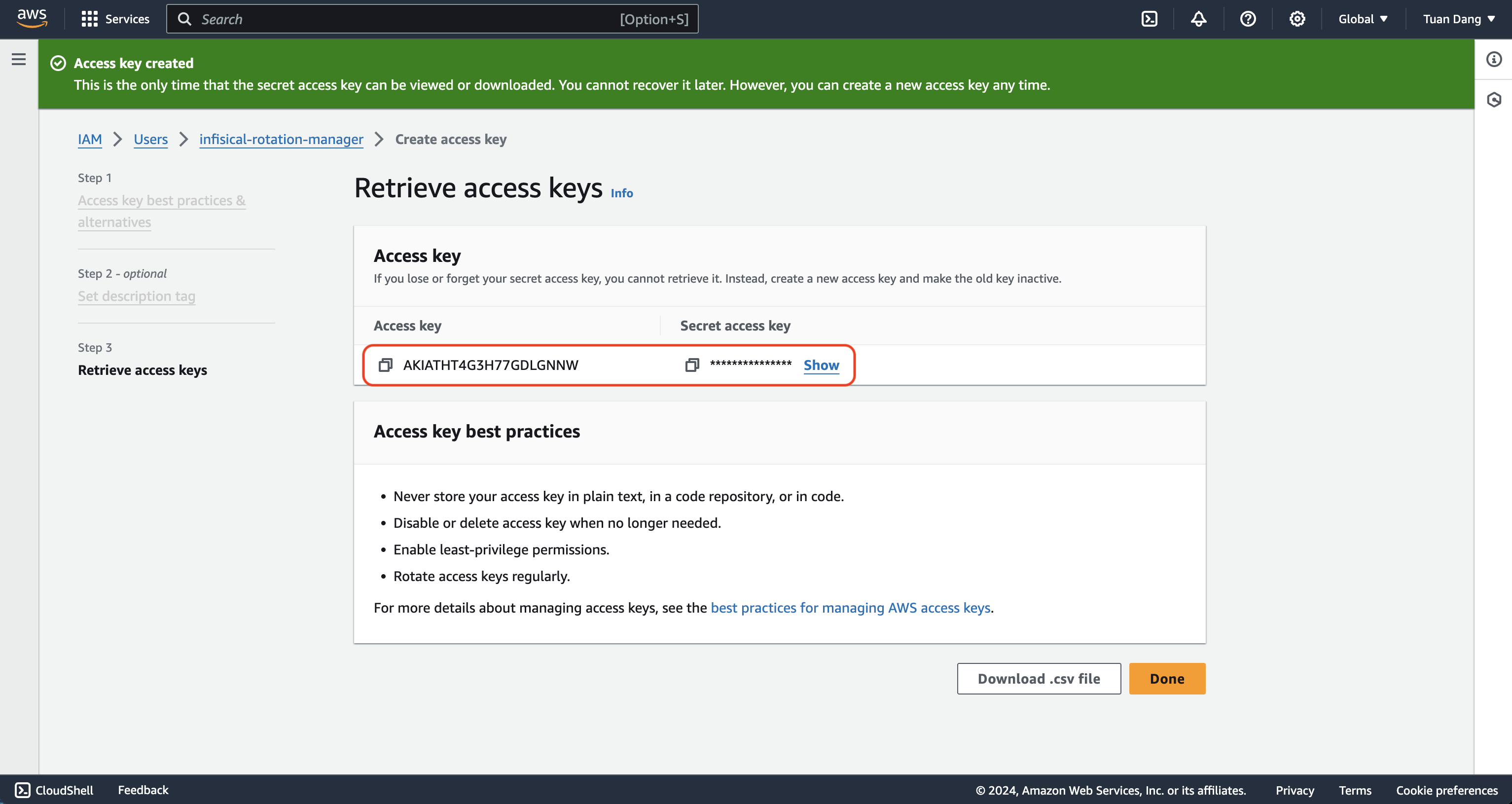
3.1. Back in Infisical, head to the Project > Secrets > Environment and path where you want the rotated AWS IAM credentials to appear and create two placeholder secrets.
In this example, we'll create two secrets called `AWS_ACCESS_KEY` and `AWS_SECRET_ACCESS_KEY`.
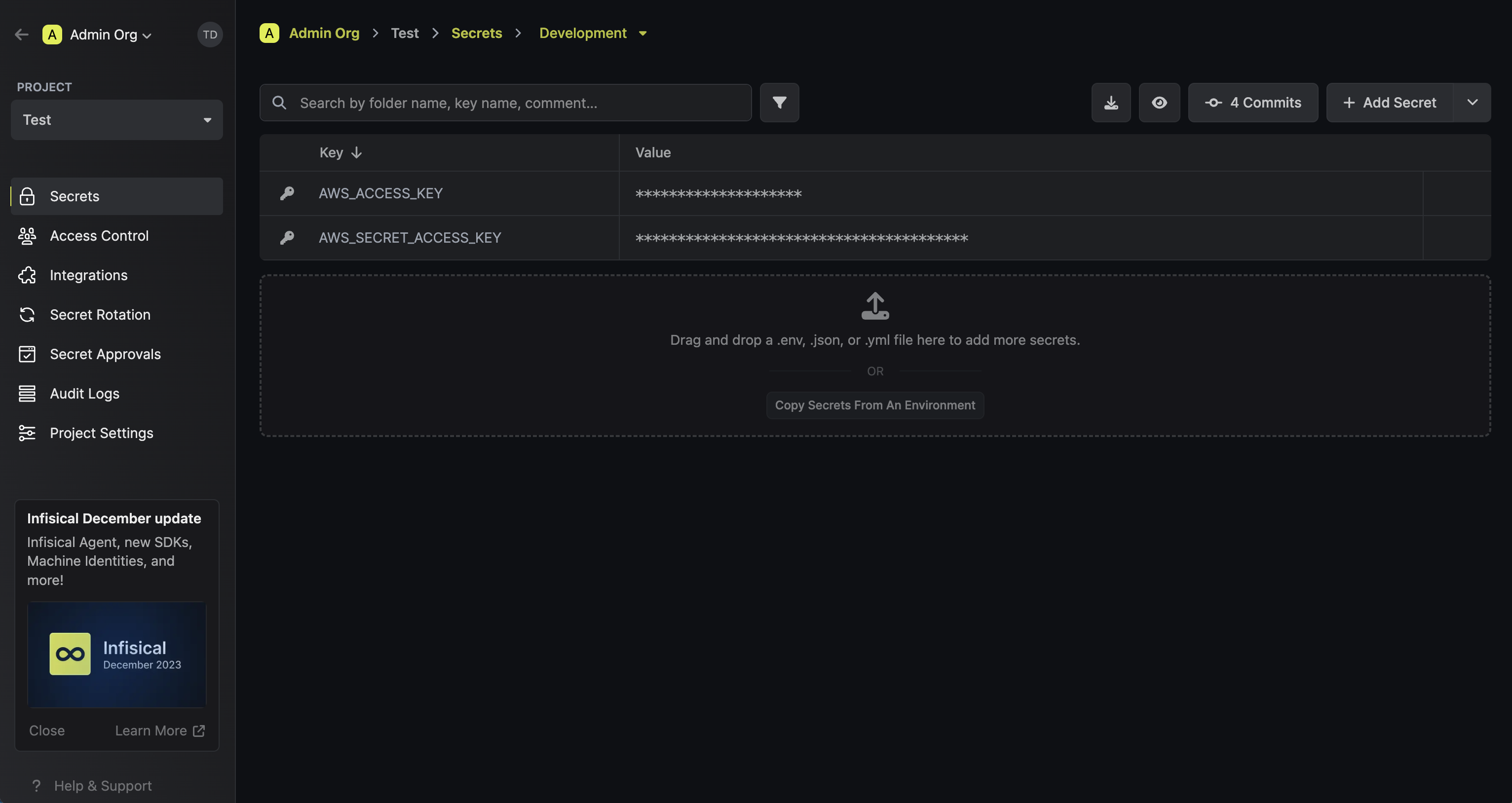
3.2. Next, in the **Secret Rotation** tab, press on the **AWS IAM** tile to configure the AWS IAM User rotation strategy.
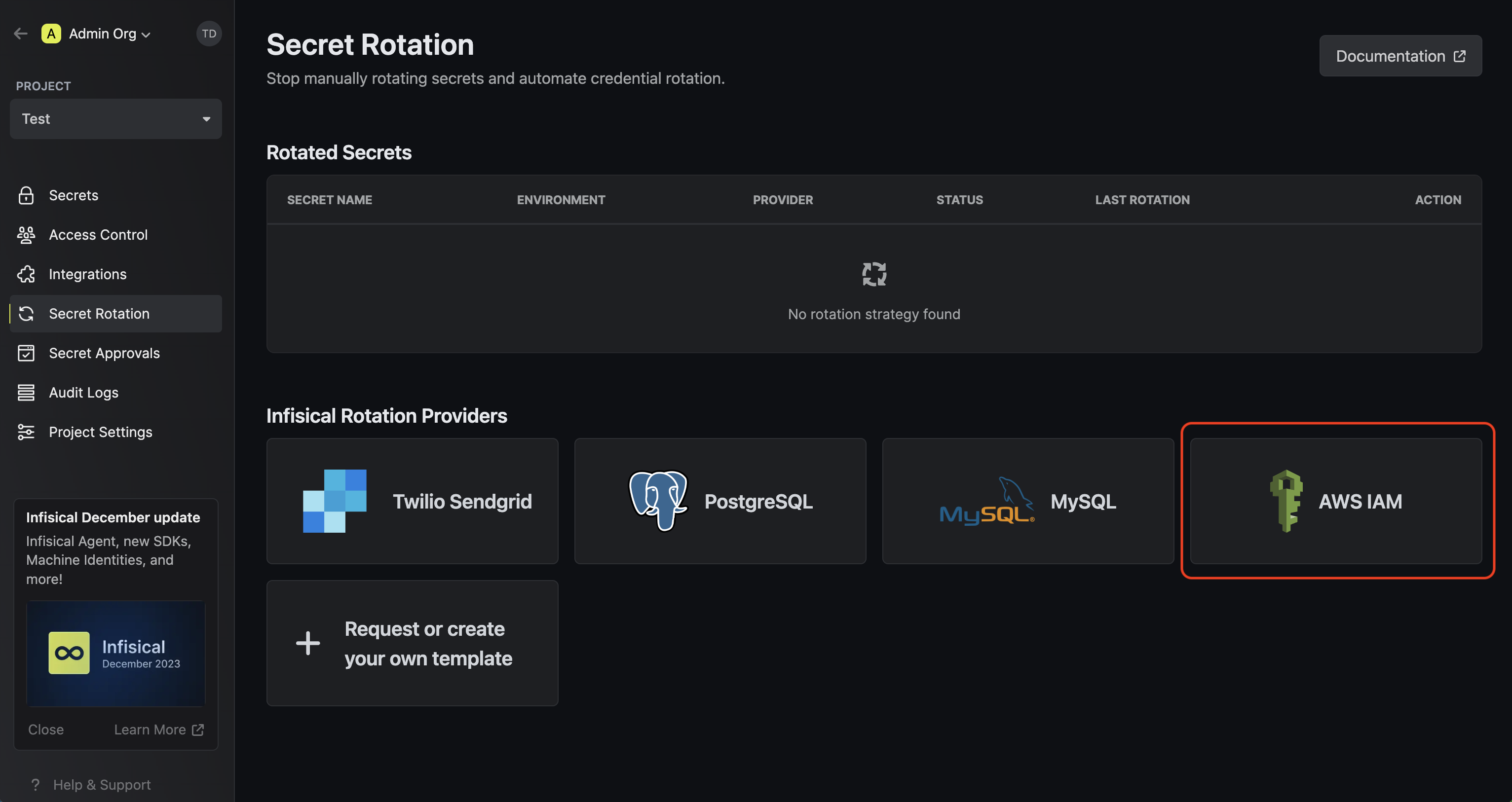
3.3. Input the configuration details for the AWS IAM User rotation strategy obtained from steps 1 and 2:
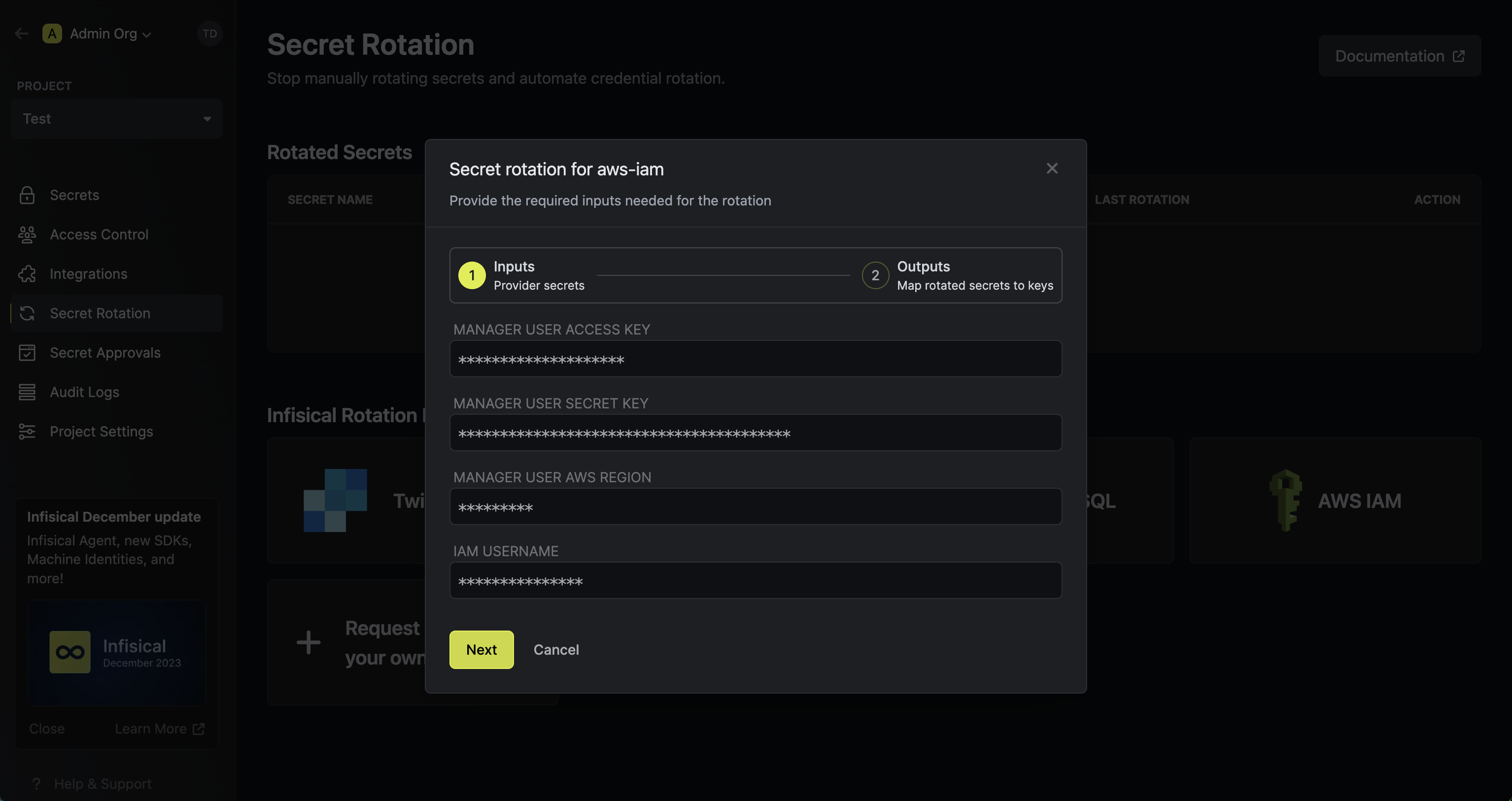
Here's some guidance on each field:
* Manager User Access Key: The managing IAM user's access key from step 2.5.
* Manager User Secret Key: The managing IAM user's secret access key from step 2.5.
* Manager User AWS Region: The [AWS region](https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/Concepts.RegionsAndAvailabilityZones.html) for Infisical to make requests to such as `us-east-1`.
* IAM Username: The IAM username of the user from step 1.
Next, specify the output secret mappings configuration for the rotated AWS IAM credentials; this is the secrets whose values will be replaced with new credentials after each rotation.
Here, you can also specify a rotation interval for the credentials to be automatically rotated periodically.
In this example, we want to map the output of the rotated AWS IAM credentials to the secrets that we created in step 3.1 (i.e. `AWS_ACCESS_KEY` and `AWS_SECRET_ACCESS_KEY`).
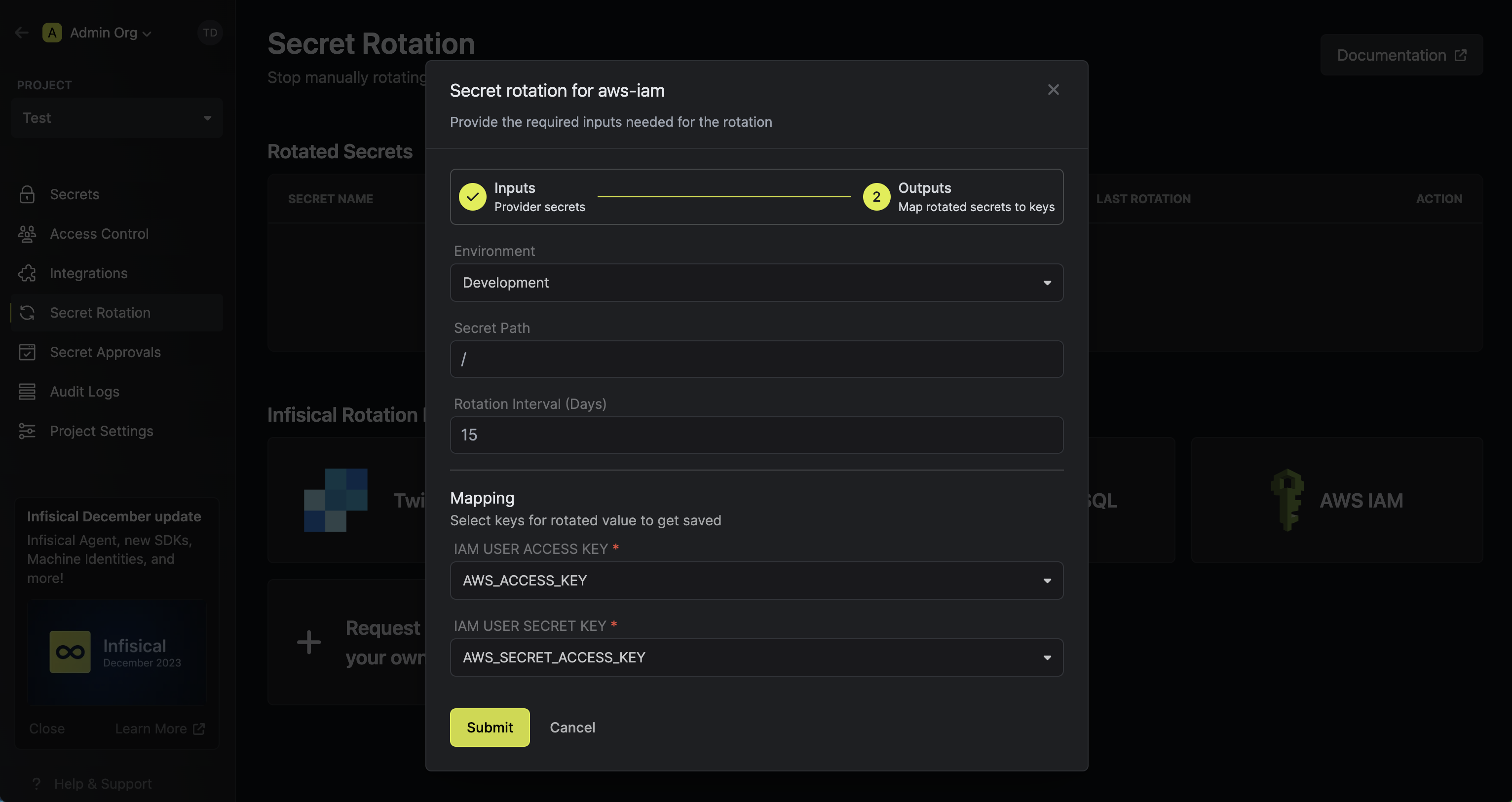
Finally, press **Submit** to create the secret rotation strategy.
You should now see the AWS IAM User rotation strategy listed in the **Secret Rotation** tab.
To manually trigger a rotation, you can press the **Rotate** button on the strategy.
Once triggered, the secrets in step 3.1 should be updated with new rotated credential values.
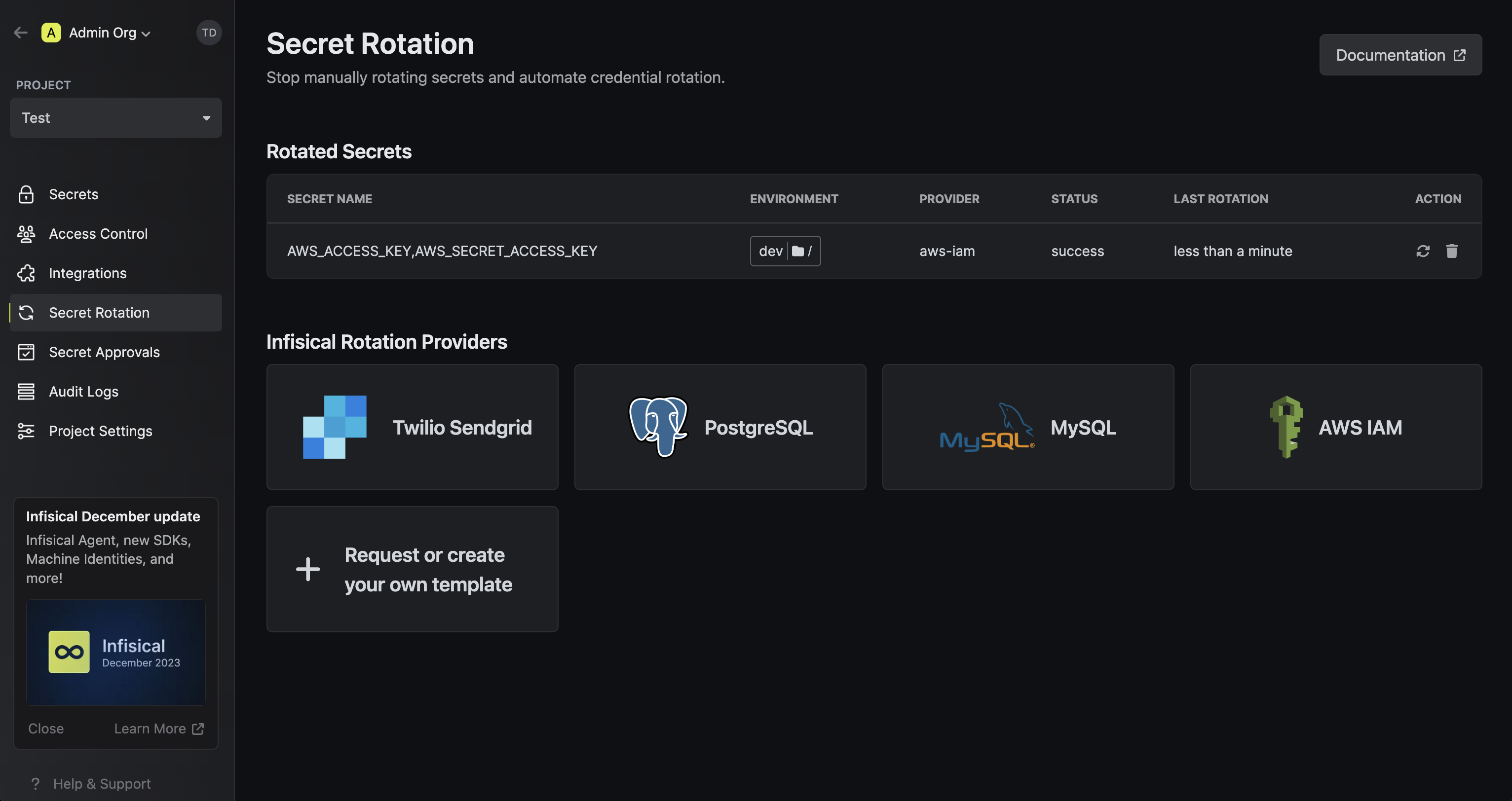
**FAQ**
There are a few reasons for why this might happen:
* The strategy configuration is invalid (e.g. the managing IAM user's credentials are incorrect, the target IAM username is incorrect, etc.).
* The managing IAM user is insufficently permissioned to rotate the credentials of the target IAM user. For instance, you may have setup [paths](https://aws.amazon.com/blogs/security/optimize-aws-administration-with-iam-paths/) for the managing IAM user and the policy does not have the necessary permissions to rotate the credentials.
* The target IAM user already has 2 access keys configured in AWS; you should delete one of the access keys to allow for rotation.
# Microsoft SQL Server
Learn how to automatically rotate Microsoft SQL Server user passwords.
The Infisical SQL Server secret rotation allows you to automatically rotate your database users' passwords at a predefined interval.
## Prerequisites
1. Create two SQL Server logins and database users with the required permissions. We'll refer to them as `user-a` and `user-b`.
2. Create another SQL Server login with permissions to alter logins for `user-a` and `user-b`. We'll refer to this as the `admin` login.
Here's how to set up the prerequisites:
```sql
-- Create the logins (at server level)
CREATE LOGIN [user-a] WITH PASSWORD = 'ComplexPassword1';
CREATE LOGIN [user-b] WITH PASSWORD = 'ComplexPassword2';
-- Create database users for the logins (in your specific database)
USE [YourDatabase];
CREATE USER [user-a] FOR LOGIN [user-a];
CREATE USER [user-b] FOR LOGIN [user-b];
-- Grant necessary permissions to the users
GRANT SELECT, INSERT, UPDATE, DELETE ON SCHEMA::dbo TO [user-a];
GRANT SELECT, INSERT, UPDATE, DELETE ON SCHEMA::dbo TO [user-b];
-- Create admin login with permission to alter other logins
CREATE LOGIN [admin] WITH PASSWORD = 'AdminComplexPassword';
CREATE USER [admin] FOR LOGIN [admin];
-- Grant permission to alter any login
GRANT ALTER ANY LOGIN TO [admin];
```
To learn more about SQL Server's permission system, please visit this [documentation](https://learn.microsoft.com/en-us/sql/relational-databases/security/authentication-access/getting-started-with-database-engine-permissions).
## How it works
1. Infisical connects to your database using the provided `admin` login credentials.
2. A random value is generated and the password for `user-a` is updated with the new value.
3. The new password is then tested by logging into the database.
4. If test is successful, it's saved to the output secret mappings so that rest of the system gets the newly rotated value(s).
5. The process is then repeated for `user-b` on the next rotation.
6. The cycle repeats until secret rotation is deleted/stopped.
## Rotation Configuration
Head over to Secret Rotation configuration page of your project by clicking on `Secret Rotation` in the left side bar
SQL Server admin username
SQL Server admin password
SQL Server host url (e.g., your-server.database.windows.net)
Database port number (default: 1433)
Database name (default: master)
The first login name to rotate - `user-a`
The second login name to rotate - `user-b`
Optional database certificate to connect with database
When a secret rotation is successful, the updated values needs to be saved to an existing key(s) in your project.
The environment where the rotated credentials should be mapped to.
The secret path where the rotated credentials should be mapped to.
What interval should the credentials be rotated in days.
Select an existing secret key where the rotated database username value should be saved to.
Select an existing select key where the rotated database password value should be saved to.
## FAQ
When a system has multiple nodes by horizontal scaling, redeployment doesn't happen instantly.
This means that when the secrets are rotated, and the redeployment is triggered, the existing system will still be using the old credentials until the change rolls out.
To avoid causing failure for them, the old credentials are not removed. Instead, in the next rotation, the previous user's credentials are updated.
The admin account is used by Infisical to update the credentials for `user-a` and `user-b`.
You don't need to grant all permissions for your admin account but rather just the permission to alter logins (ALTER ANY LOGIN).
When using Azure SQL Database, you'll need to:
1. Use the full server name as your host (e.g., your-server.database.windows.net)
2. Ensure your admin account is either the Azure SQL Server admin or an Azure AD account with appropriate permissions
3. Configure your Azure SQL Server firewall rules to allow connections from Infisical's IP addresses
# MySQL/MariaDB
Learn how to automatically rotate MySQL/MariaDB user passwords.
The Infisical MySQL secret rotation allows you to automatically rotate your MySQL database user's password at a predefined interval.
## Prerequisite
1. Create two users with the required permission in your MySQL instance. We'll refer to them as `user-a` and `user-b`.
2. Create another MySQL user with just the permission to update the passwords of `user-a` and `user-b`. We'll refer to this user as the `admin` user.
To learn more about MySQL permission system, please visit this [documentation](https://dev.mysql.com/doc/refman/8.0/en/privileges-provided.html).
## How it works
1. Infisical connects to your database using the provided `admin` user account.
2. A random value is generated and the password for `user-a` is updated with the new value.
3. The new password is then tested by logging into the database
4. If test is success, it's saved to the output secret mappings so that rest of the system gets the newly rotated value(s).
5. The process is then repeated for `user-b` on the next rotation.
6. The cycle repeats until secret rotation is deleted/stopped.
## Rotation Configuration
Head over to Secret Rotation configuration page of your project by clicking on `Secret Rotation` in the left side bar
Rotator admin username
Rotator admin password
Database host url
Database port number
The first username of two to rotate - `user-a`
The second username of two to rotate - `user-b`
Optional database certificate to connect with database
When a secret rotation is successful, the updated values needs to be saved to an existing key(s) in your project.
The environment where the rotated credentials should be mapped to.
The secret path where the rotated credentials should be mapped to.
What interval should the credentials be rotated in days.
Select an existing secret key where the rotated database username value should be saved to.
Select an existing select key where the rotated database password value should be saved to.
## FAQ
When a system has multiple nodes by horizontal scaling, redeployment doesn't happen instantly.
This means that when the secrets are rotated, and the redeployment is triggered, the existing system will still be using the old credentials until the change rolls out.
To avoid causing failure for them, the old credentials are not removed. Instead, in the next rotation, the previous user's credentials are updated.
The admin account is used by Infisical to update the credentials for `user-a` and `user-b`.
You don't need to grant all permission for your admin account but rather just the permissions to update both of the user's passwords.
# Secret Rotation
Learn how to set up automated secret rotation in Infisical.
## Introduction
Secret rotation is a process that involves updating secret credentials periodically to minimize the risk of their compromise.
Rotating secrets helps prevent unauthorized access to systems and sensitive data by ensuring that old credentials are replaced with new ones regularly.
Rotated secrets may include, but are not limited to:
1. API keys for external services;
2. Database credentials for various platforms.
## Rotation Process
The practice of rotating secrets is a systematic and interval-based operation, carried out in four fundamental phases.
### 1. Creation
The system initiates the rotation process by either making an API call to an external service or generating a new secret value internally.
Upon successful creation, the system will temporarily have three versions of the secret:
* **Current active secret**: The one currently in use.
* **Future active secret (pending)**: The newly created secret, awaiting validation.
* **Previous active secret**: The old secret, soon to be retired.
### 2. Testing
The newly generated secret is subjected to a verification process to ensure its validity and functionality.
This involves conducting checks or tests that simulate actual operations the secret would perform.
Only the current active and the future active (pending) secrets are considered operational at this stage, while the previous active secret remains in standby mode.
### 3. Deletion
Post-verification, the system deactivates and deletes the previous active secret, leaving only the current and future active (pending) secrets in the system.
### 4. Activation
Finally, the system promotes the future active (pending) secret to be the new current active secret. It then triggers necessary side effects, such as invoking webhooks and generating events, to notify other services of the change.
## Infisical Secret Rotation Strategies
1. [SendGrid Integration](./sendgrid)
2. [PostgreSQL/CockroachDB Implementation](./postgres)
3. [MySQL/MariaDB Configuration](./mysql)
4. [AWS IAM User](./aws-iam)
# PostgreSQL/CockroachDB
Learn how to automatically rotate PostgreSQL/CockroachDB user passwords.
The Infisical Postgres secret rotation allows you to automatically rotate your Postgres database user's password at a predefined interval.
## Prerequisite
1. Create two users with the required permission in your PostgreSQL instance. We'll refer to them as `user-a` and `user-b`.
2. Create another PostgreSQL user with just the permission to update the passwords of `user-a` and `user-b`. We'll refer to this user as the `admin` user.
To learn more about Postgres permission system, please visit this [documentation](https://www.postgresql.org/docs/9.1/sql-grant.html).
## How it works
1. Infisical connects to your database using the provided `admin` user account.
2. A random value is generated and the password for `user-a` is updated with the new value.
3. The new password is then tested by logging into the database
4. If test is success, it's saved to the output secret mappings so that rest of the system gets the newly rotated value(s).
5. The process is then repeated for `user-b` on the next rotation.
6. The cycle repeats until secret rotation is deleted/stopped.
## Rotation Configuration
Head over to Secret Rotation configuration page of your project by clicking on `Secret Rotation` in the left side bar
Rotator admin username
Rotator admin password
Database host url
Database port number
The first username of two to rotate - `user-a`
The second username of two to rotate - `user-b`
Optional database certificate to connect with database
When a secret rotation is successful, the updated values needs to be saved to an existing key(s) in your project.
The environment where the rotated credentials should be mapped to.
The secret path where the rotated credentials should be mapped to.
What interval should the credentials be rotated in days.
Select an existing secret key where the rotated database username value should be saved to.
Select an existing select key where the rotated database password value should be saved to.
## FAQ
When a system has multiple nodes by horizontal scaling, redeployment doesn't happen instantly.
This means that when the secrets are rotated, and the redeployment is triggered, the existing system will still be using the old credentials until the change rolls out.
To avoid causing failure for them, the old credentials are not removed. Instead, in the next rotation, the previous user's credentials are updated.
The admin account is used by Infisical to update the credentials for `user-a` and `user-b`.
You don't need to grant all permission for your admin account but rather just the permissions to update both of the user's passwords.
# Twilio SendGrid
Find out how to rotate Twilio SendGrid API keys.
Eliminate the use of long lived secrets by rotating Twilio SendGrid API keys with Infisical.
## Prerequisite
You will need a valid SendGrid admin key with the necessary scope to create additional API keys.
Follow the [SendGrid Docs to create an admin api key](https://docs.sendgrid.com/ui/account-and-settings/api-keys).
## How it works
Using the provided admin API key, Infisical will attempt to create child API keys with the specified permissions.
New keys will ge generated every time a rotation occurs. Behind the scenes, Infisical uses the [SendGrid API](https://docs.sendgrid.com/api-reference/api-keys/create-api-keys) to generate new API keys.
## Rotation Configuration
Head over to Secret Rotation configuration page of your project by clicking on `Secret Rotation` in the left side bar
SendGrid admin API key with permission to create child scoped API keys.
The permissions that the newly generated API keys will have. To view possible permissions, visit [this documentation](https://docs.sendgrid.com/api-reference/api-key-permissions/api-key-permissions).
Permissions must be entered as a list of strings.
Example: `["user.profile.read", "user.profile.update"]`
When a secret rotation is successful, the updated values needs to be saved to an existing key(s) in your project.
The environment where the rotated credentials should be mapped to.
The secret path where the rotated credentials should be mapped to.
What interval should the credentials be rotated in days.
Select an existing select key where the newly rotated API key will get saved to.
Now your output mapped secret value will be replaced periodically by SendGrid.
# Secret Sharing
Learn how to share time & view-count bound secrets securely with anyone on the internet.
Developers frequently need to share secrets with team members, contractors, or other third parties, which can be risky due to potential leaks or misuse.
Infisical offers a secure solution for sharing secrets over the internet in a time and view count bound manner. It is possible to share secrets without signing up via [share.infisical.com](https://share.infisical.com) or via Infisical Dashboard (which has more advanced funcitonality).
With its zero-knowledge architecture, secrets shared via Infisical remain unreadable even to Infisical itself.
## Share a Secret
1. Navigate to the **Organization** page.
2. Click on the **Secret Sharing** tab from the sidebar.
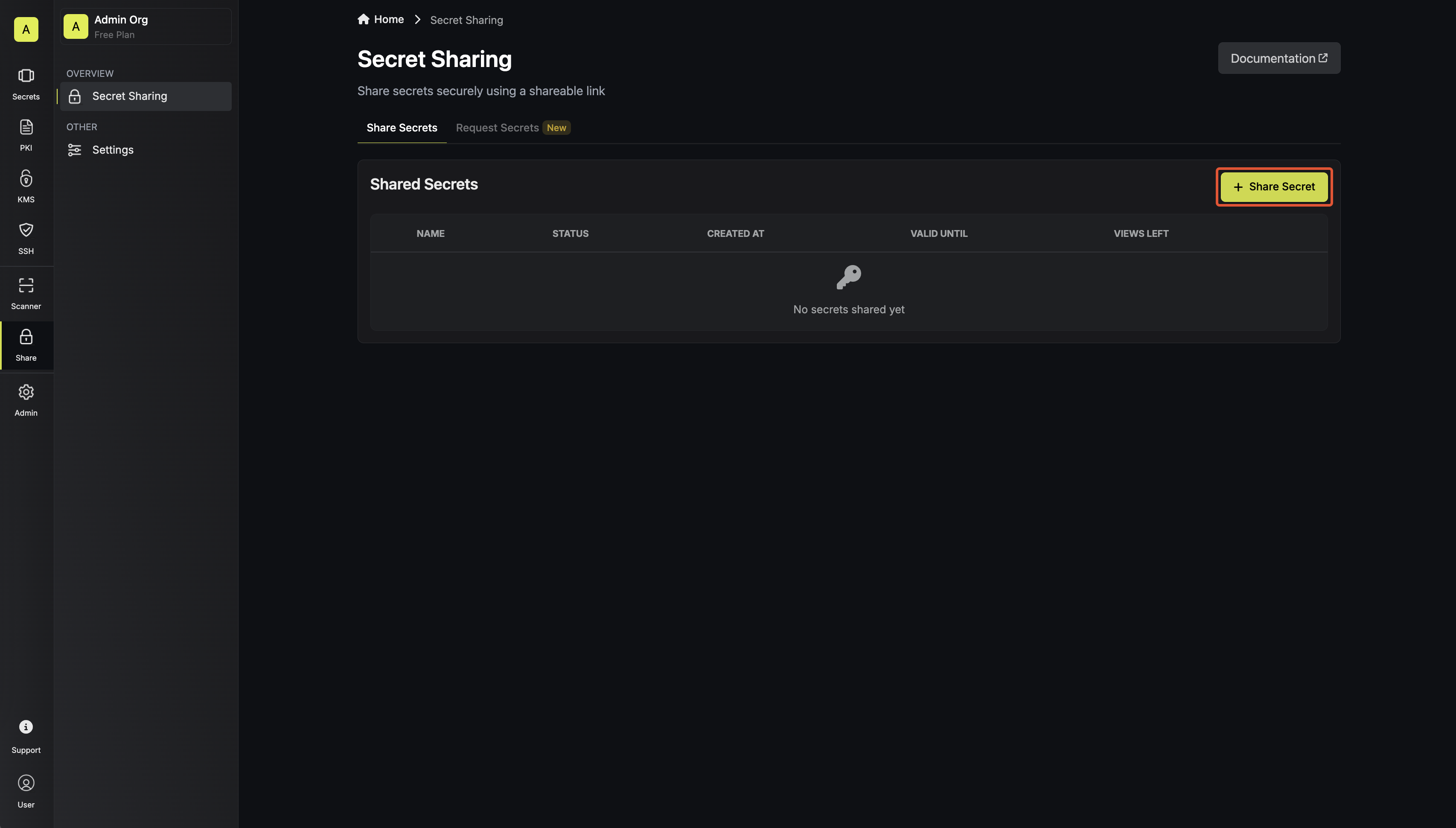
Infisical does not have access to the shared secrets. This is a part of our
zero knowledge architecture.
3. Click on the **Share Secret** button. Set the secret, its expiration time and specify if the secret can be viewed only once. It expires as soon as any of the conditions are met.
Also, specify if the secret can be accessed by anyone or only people within your organization.
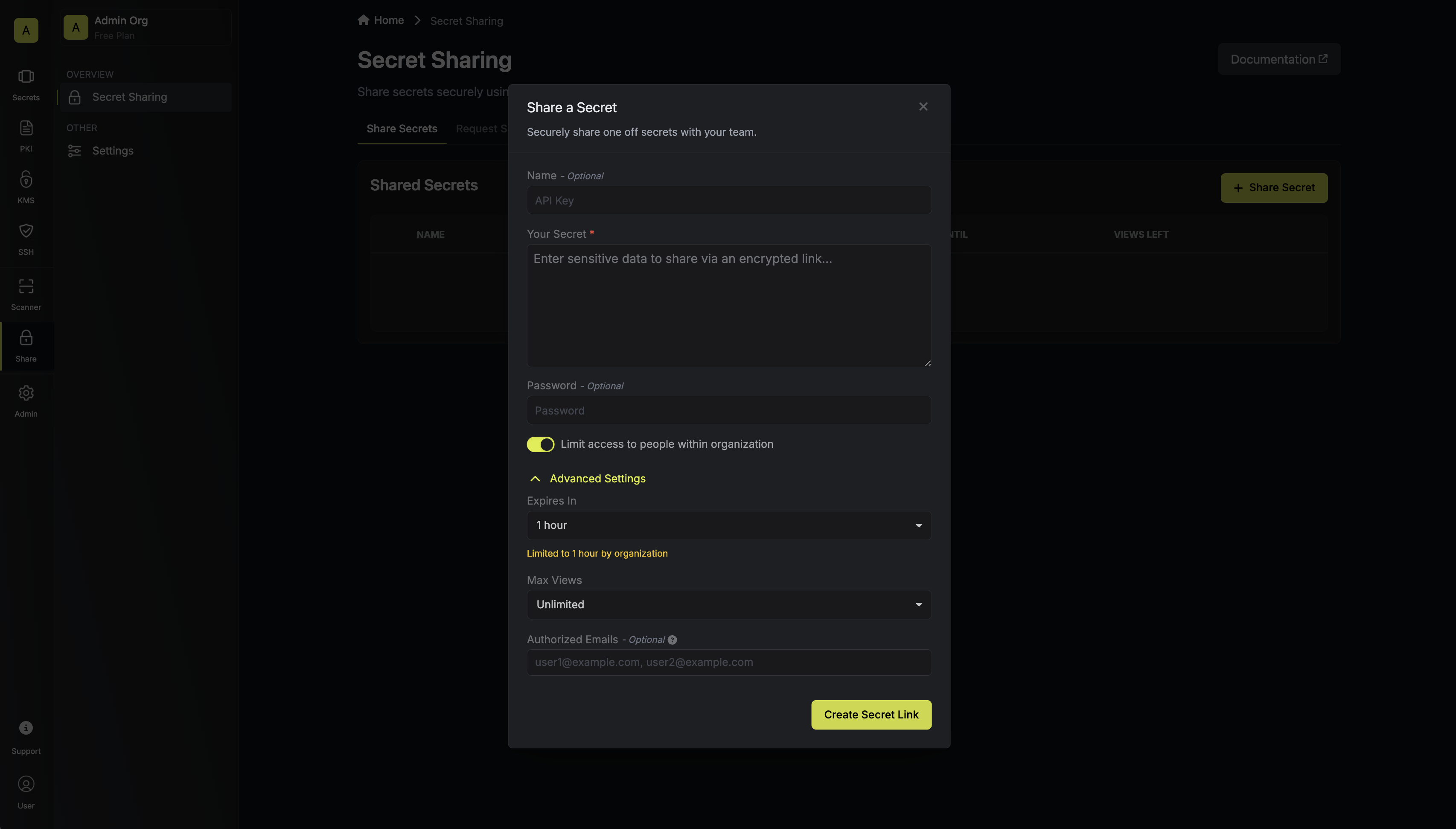
Secret once set cannot be changed. This is to ensure that the secret is not
tampered with.
5. Copy the link and share it with the intended recipient. Anyone with the link can access the secret before its expiration condition. Hence, it is recommended to share the link only with the intended recipient.
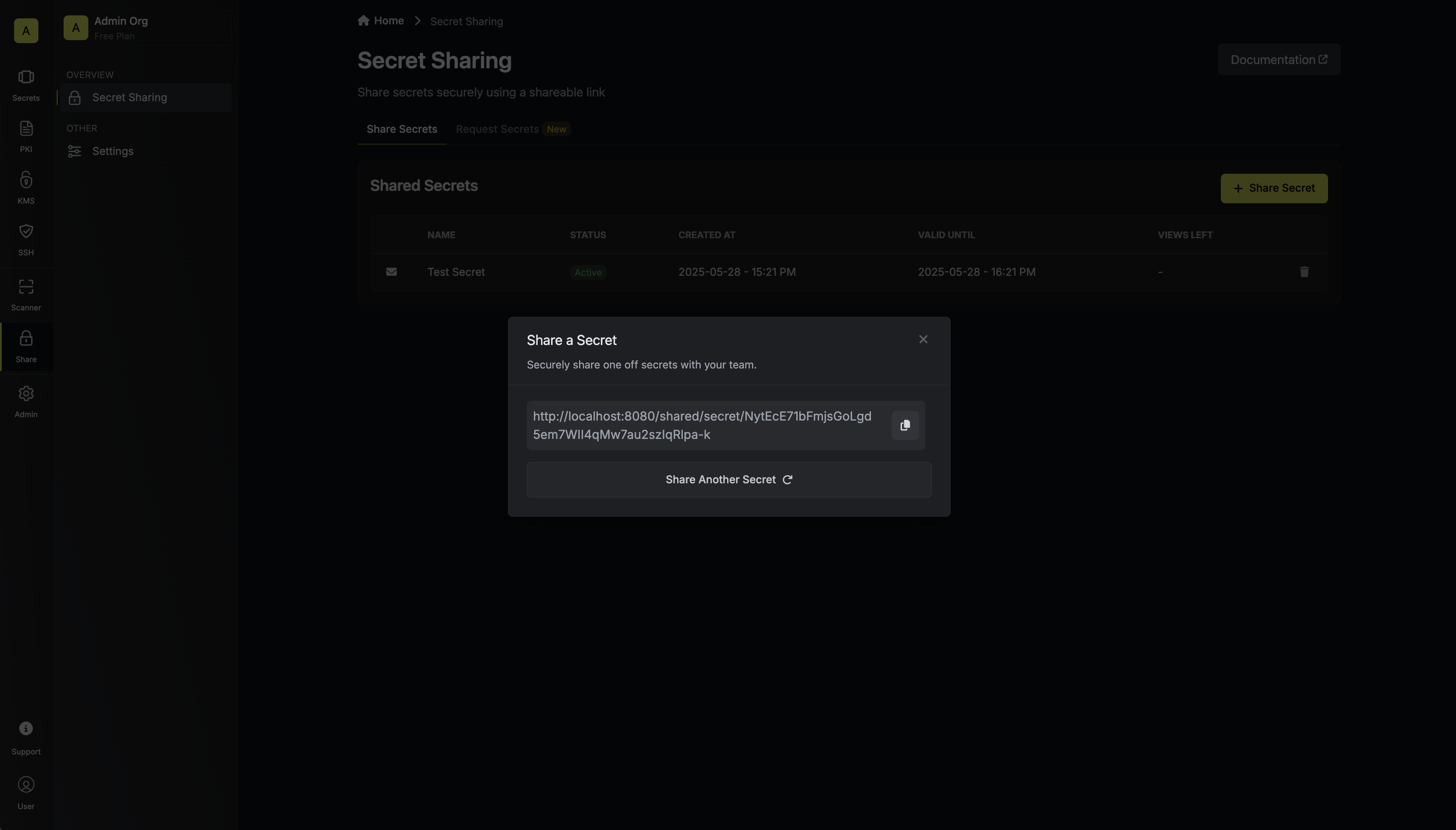
## Access a Shared Secret
Just click on the link you received to access the secret. The secret will be displayed on the screen & for how long it is valid.
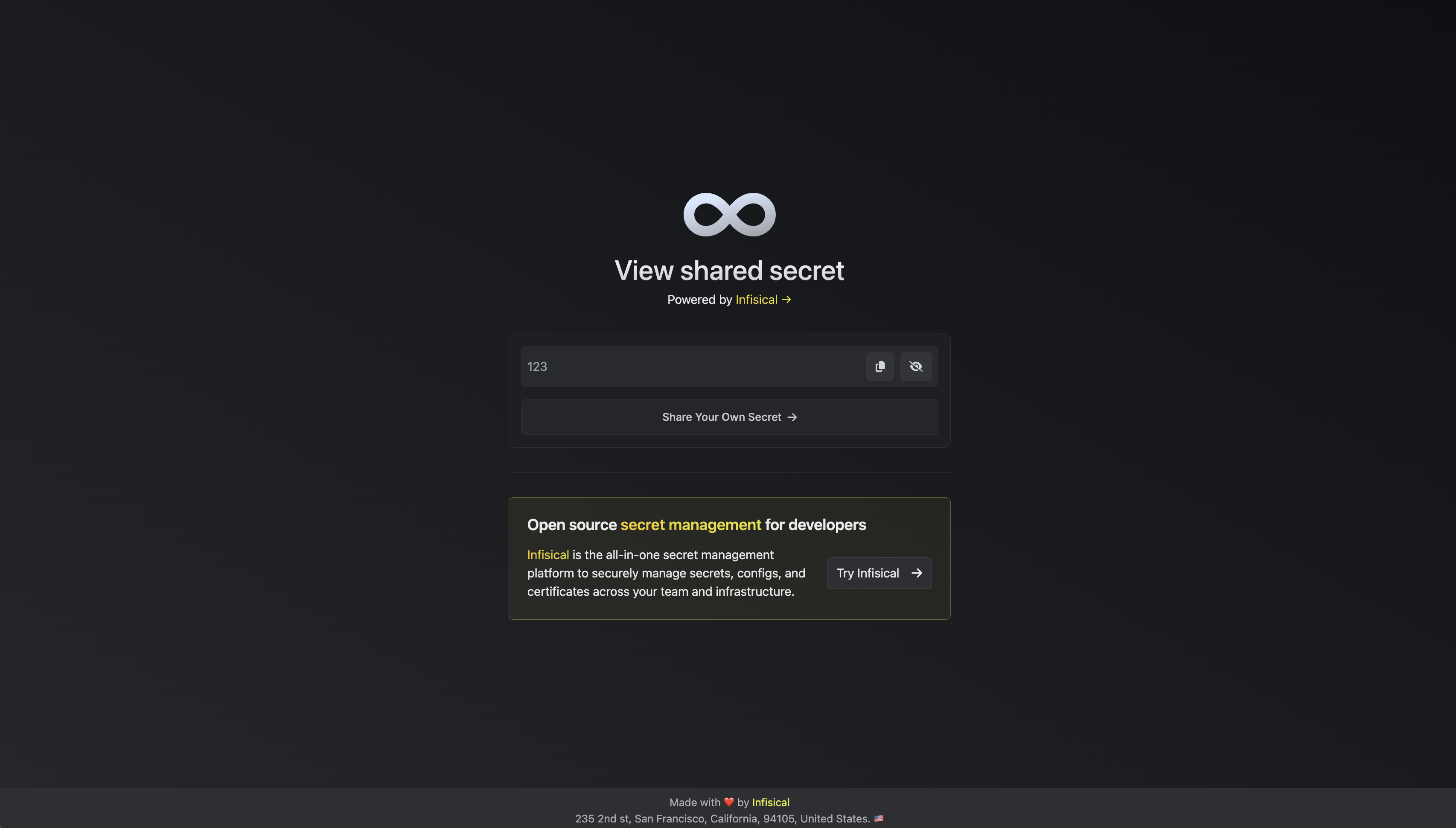
## Delete a Shared Secret
In the **Secret Sharing** tab, click on the **Delete** button next to the secret you want to delete. This will delete the secret immediately & the link will no longer be accessible.
# Secret Versioning
Learn how secret versioning works in Infisical.
Every time a secret change is performed, a new version of the same secret is created.
Such versions can be accessed visually by opening up the [secret sidebar](/documentation/platform/project#drawer) (as seen below) or [retrieved via API](/api-reference/endpoints/secrets/read)
by specifying the `version` query parameter.
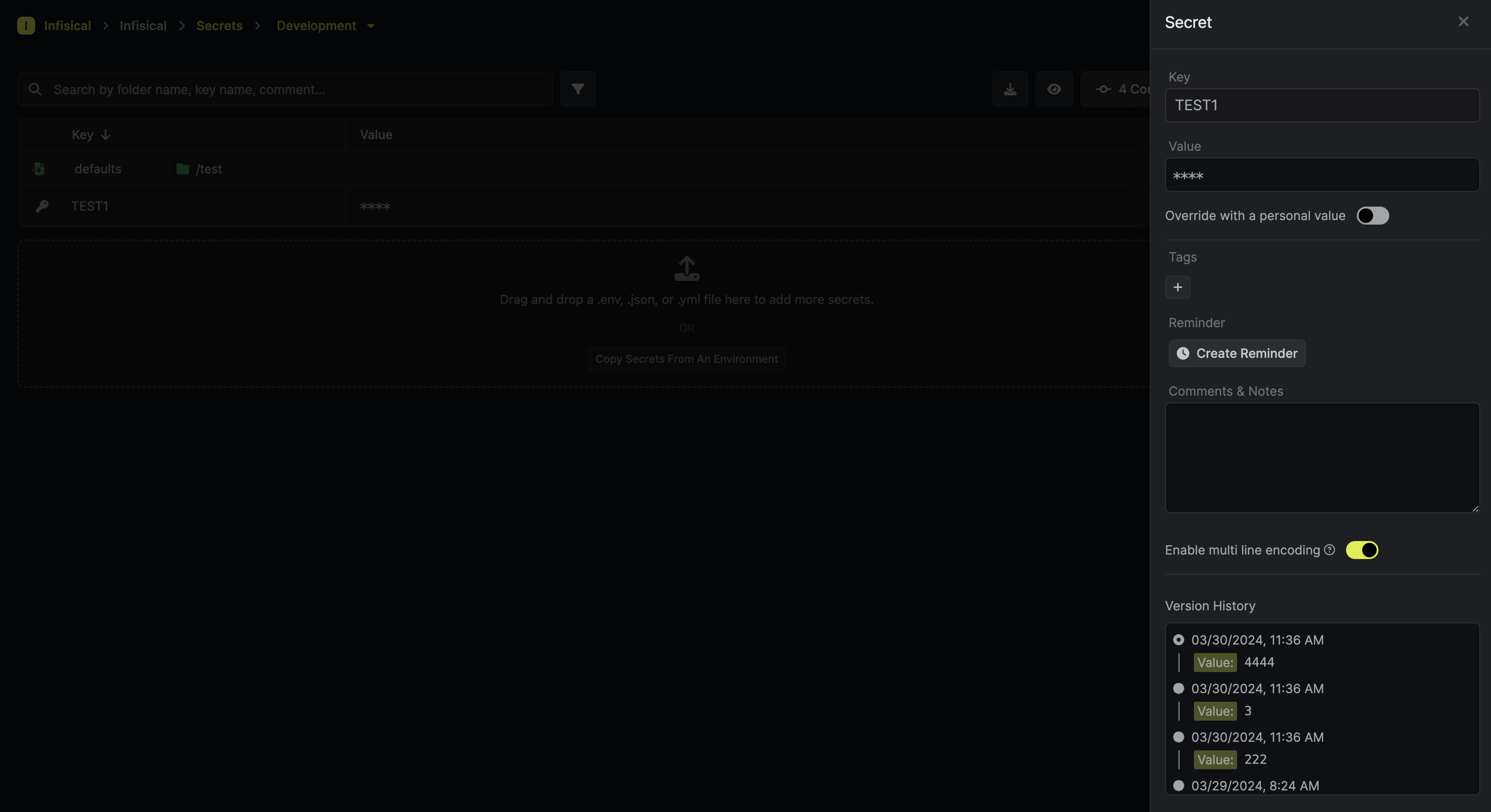
The secret versioning functionality is heavily connected to [Point-in-time Recovery](/documentation/platform/pit-recovery) of secrets in Infisical.
You can copy and paste a secret version value to the "Value" input field "roll
back" to that secret version. This creates a new secret version at the top of
the stack. We're releasing the ability to automatically roll back to
a secret version soon.
# Auth0 OIDC
Learn how to configure Auth0 OIDC for Infisical SSO.
Auth0 OIDC SSO is a paid feature. If you're using Infisical Cloud, then it is
available under the **Pro Tier**. If you're self-hosting Infisical, then you
should contact [sales@infisical.com](mailto:sales@infisical.com) to purchase an enterprise license to use
it.
1.1. From the Application's Page, navigate to the settings tab of the Auth0 application you want to integrate with Infisical.
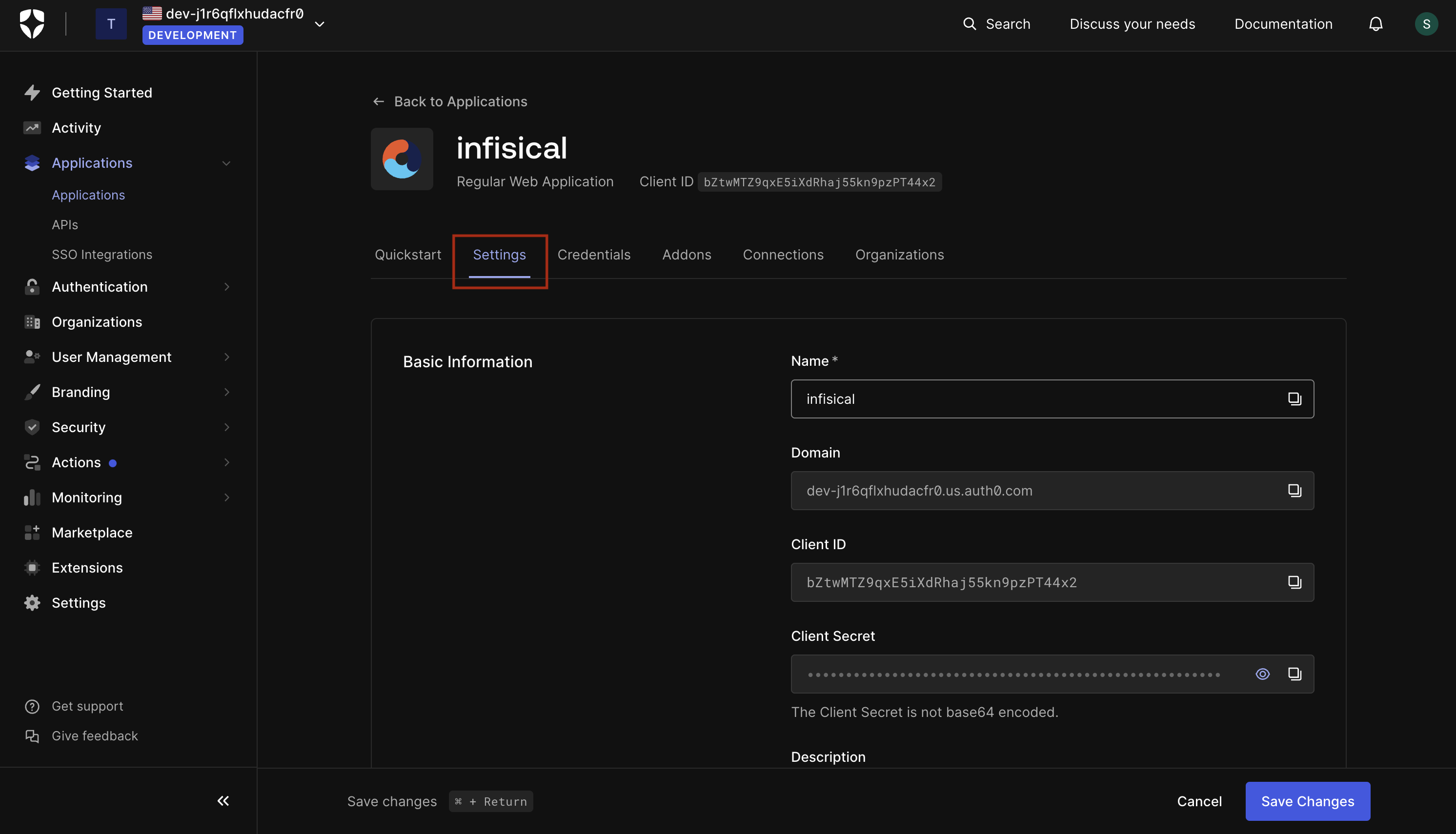
1.2. In the Application URIs section, set the **Application Login URI** and **Allowed Web Origins** fields to `https://app.infisical.com` and the **Allowed Callback URL** field to `https://app.infisical.com/api/v1/sso/oidc/callback`.
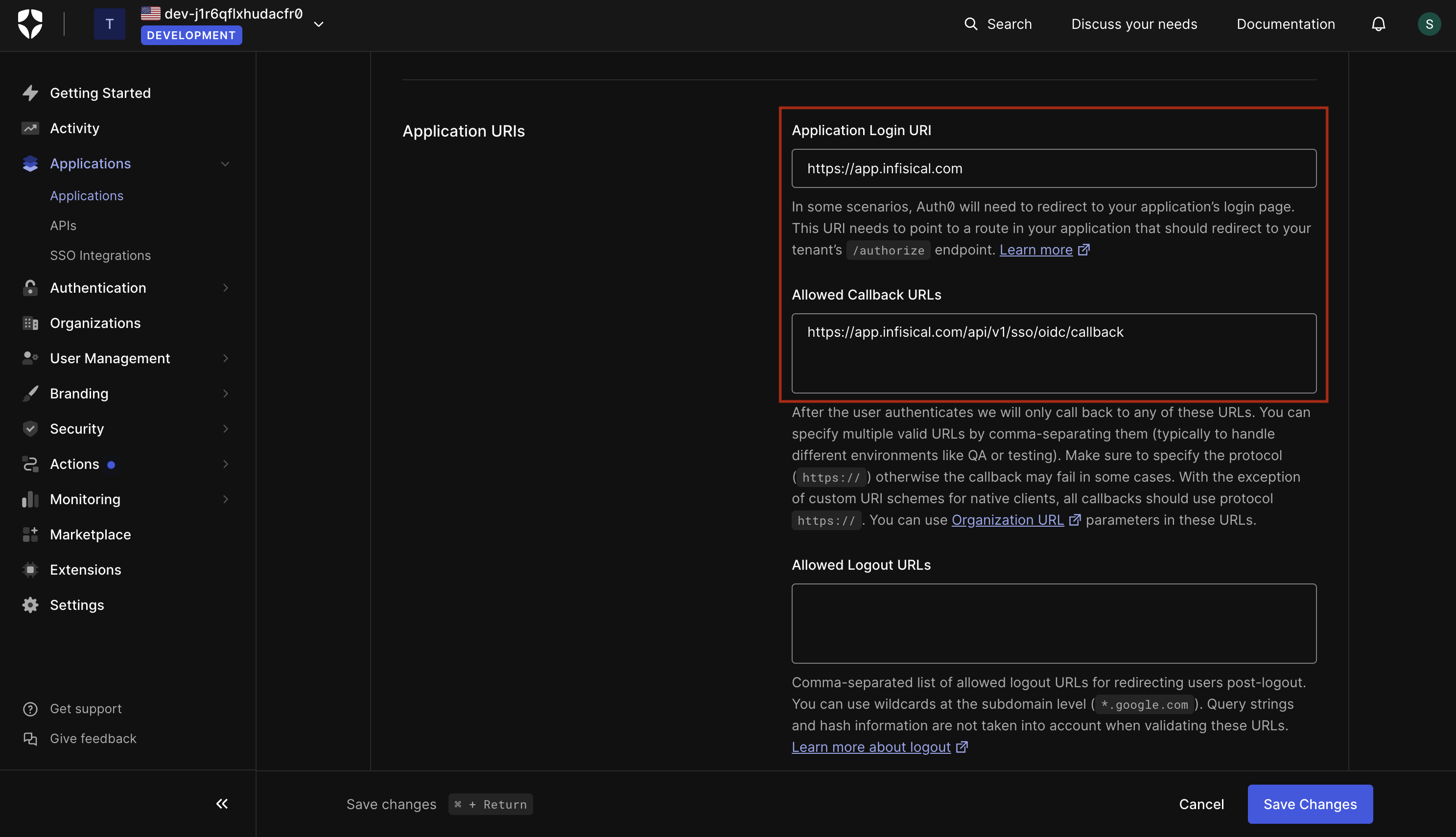
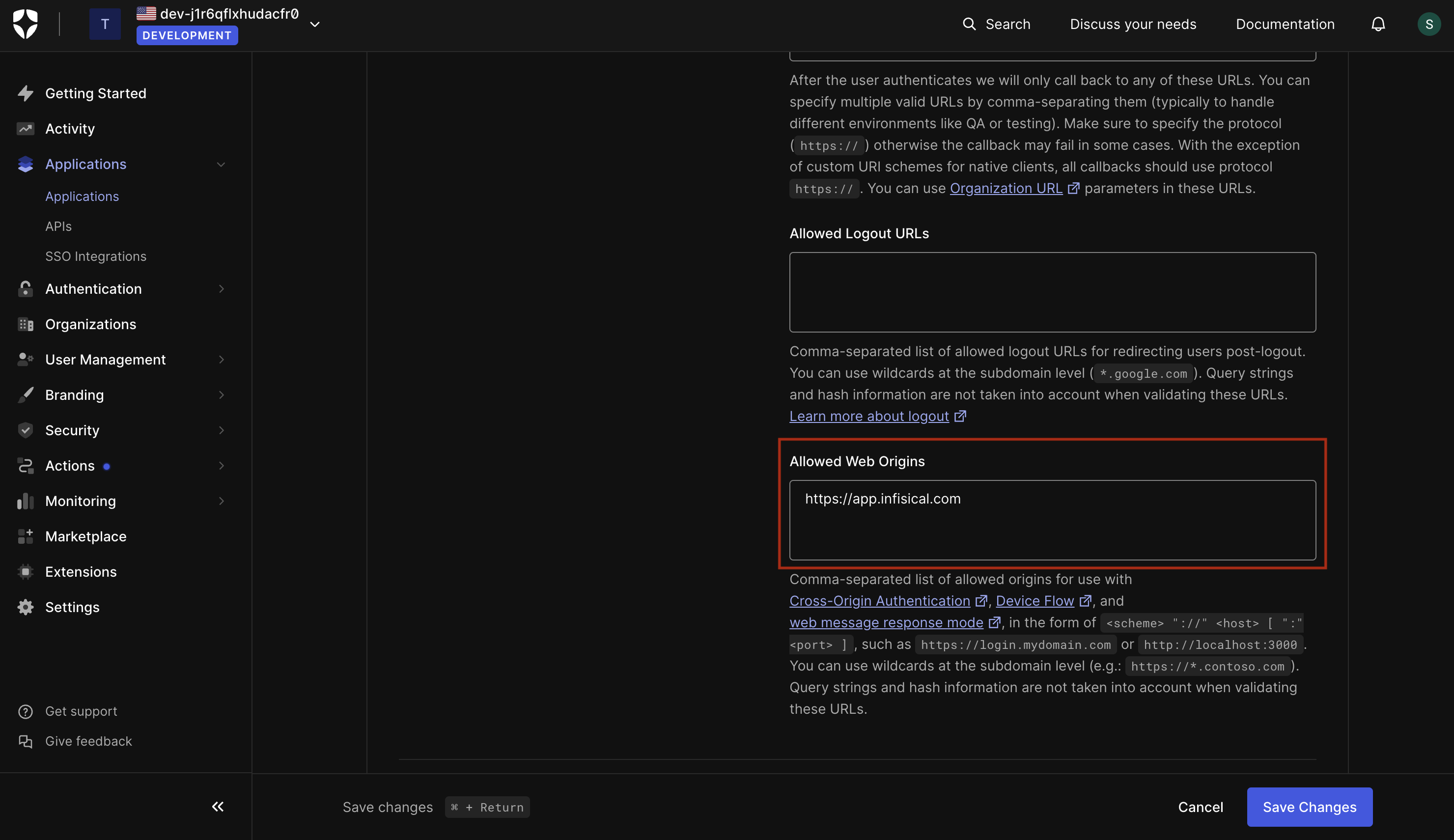
If you’re self-hosting Infisical, then you will want to replace [https://app.infisical.com](https://app.infisical.com) with your own domain.
Once done, click **Save Changes**.
1.3. Proceed to the Connections Tab and enable desired connections.
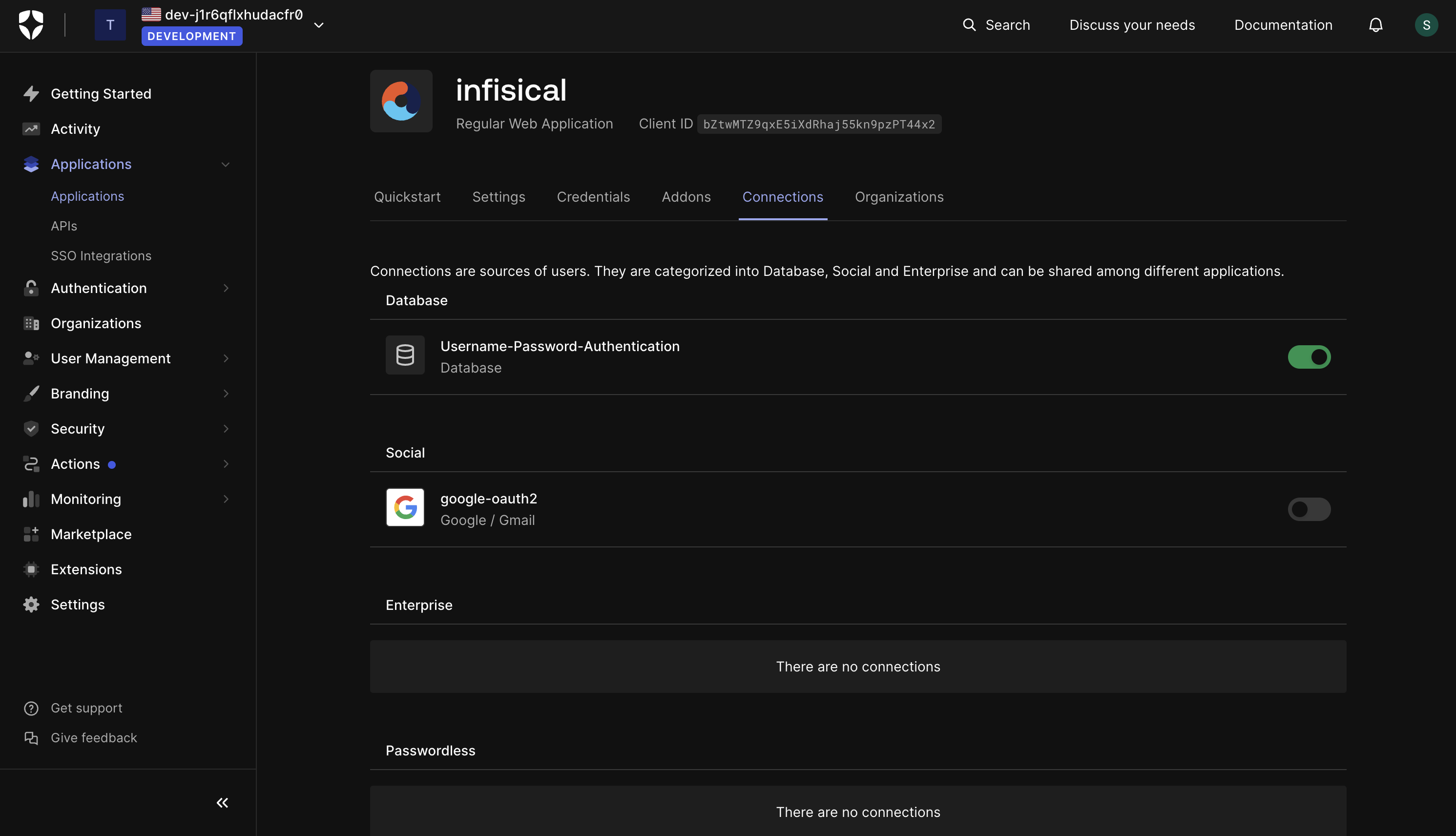
2.1. From the application settings page, retrieve the **Client ID** and **Client Secret**
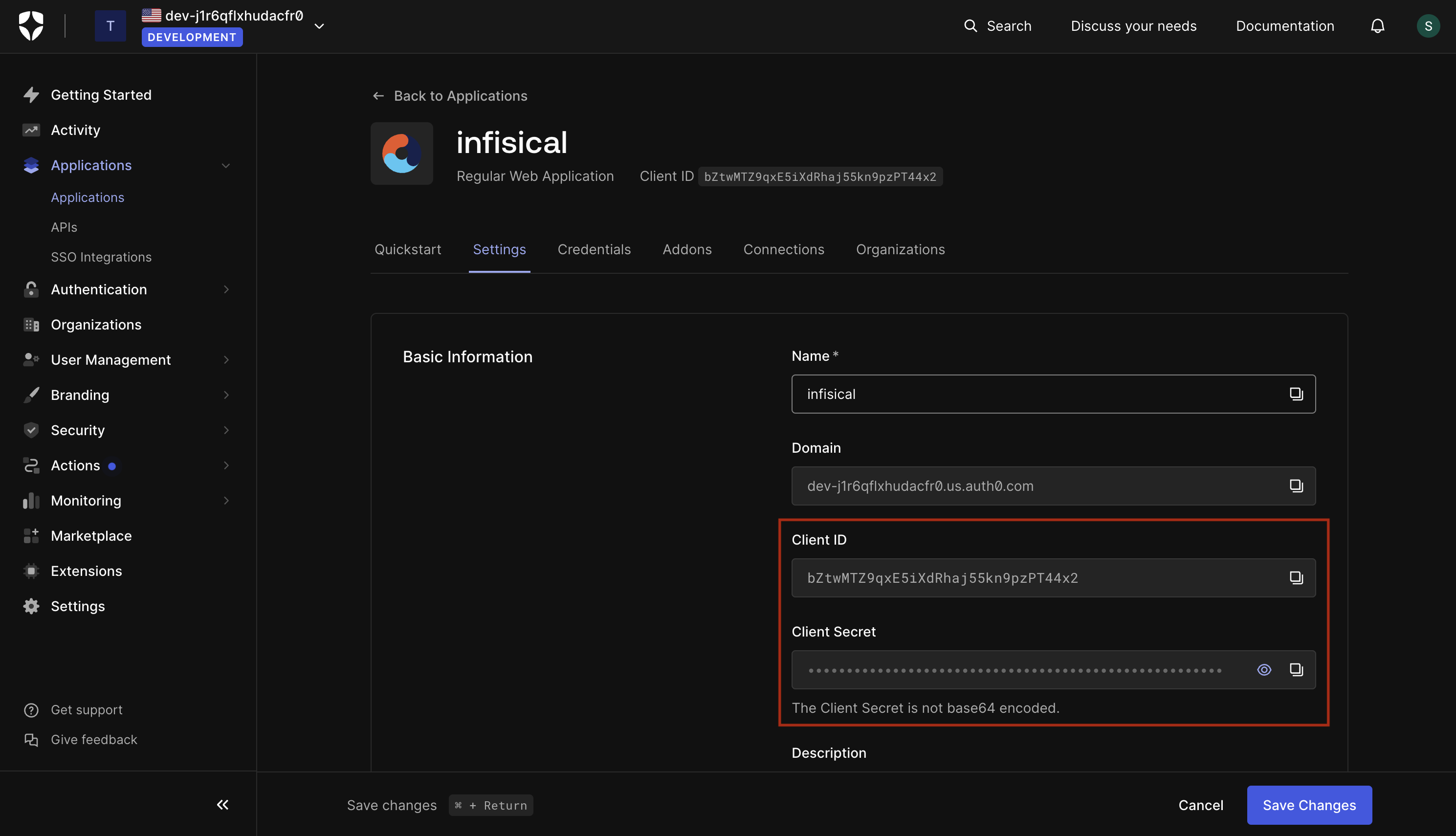
2.2. In the advanced settings (bottom-most section), retrieve the **OpenID Configuration URL** from the Endpoints tab.
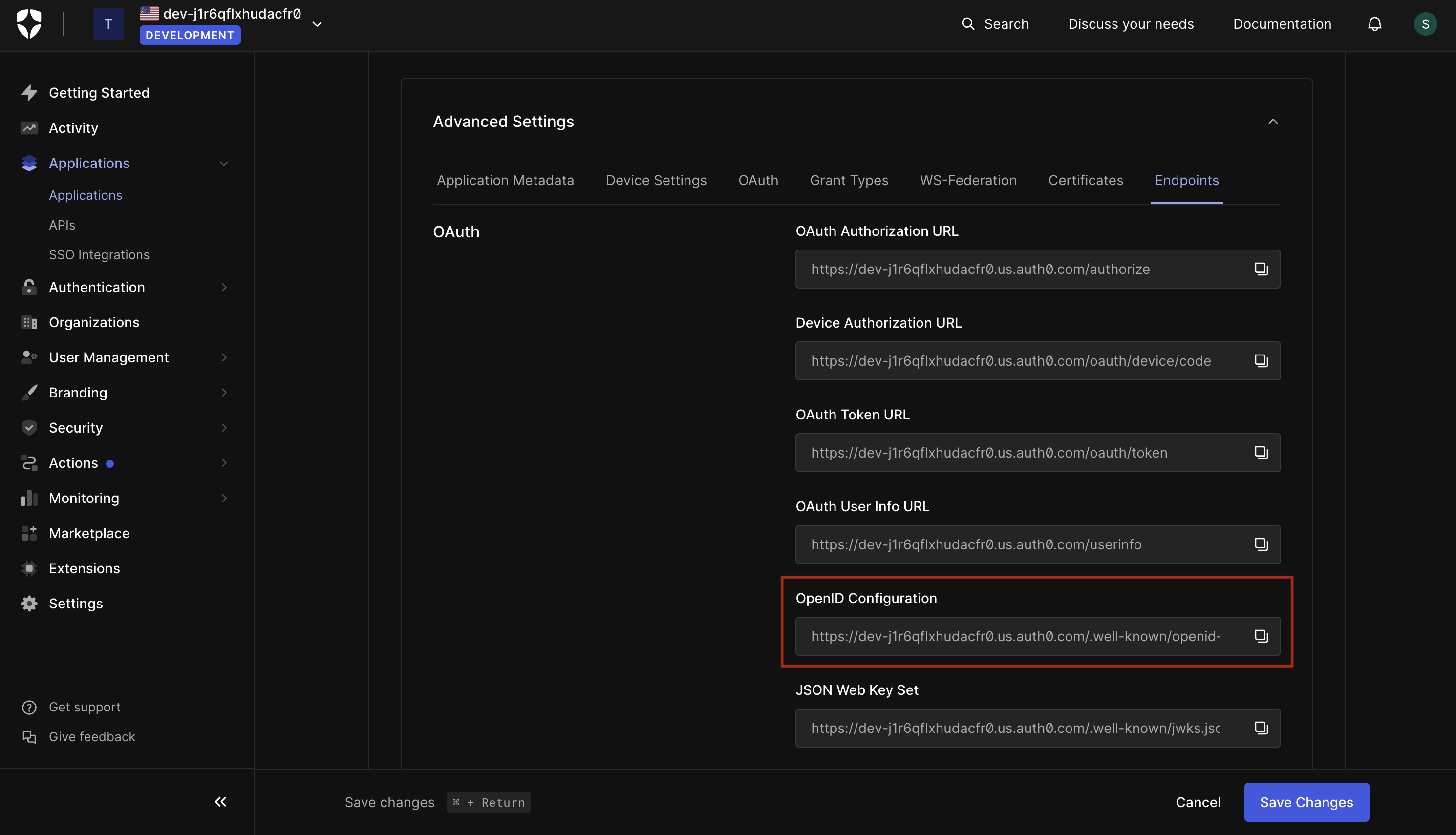
Keep these values handy as we will need them in the next steps.
3.1. Back in Infisical, in the Organization settings > Security > OIDC, click **Connect**.

3.2. For configuration type, select **Discovery URL**. Then, set **Discovery Document URL**, **Client ID**, and **Client Secret** from step 2.1 and 2.2.
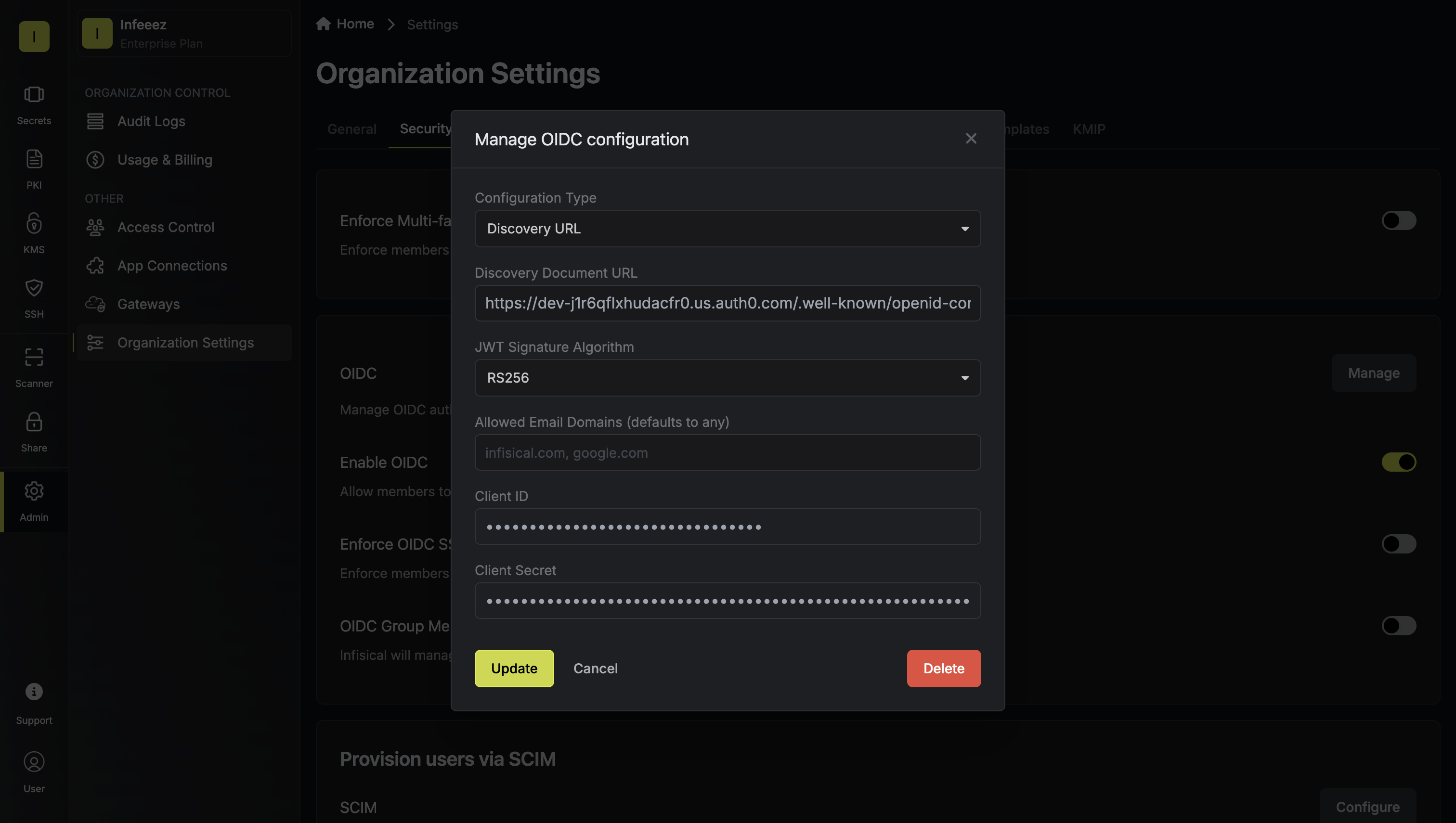
Once you've done that, press **Update** to complete the required configuration.
Enabling OIDC allows members in your organization to log into Infisical via Auth0.
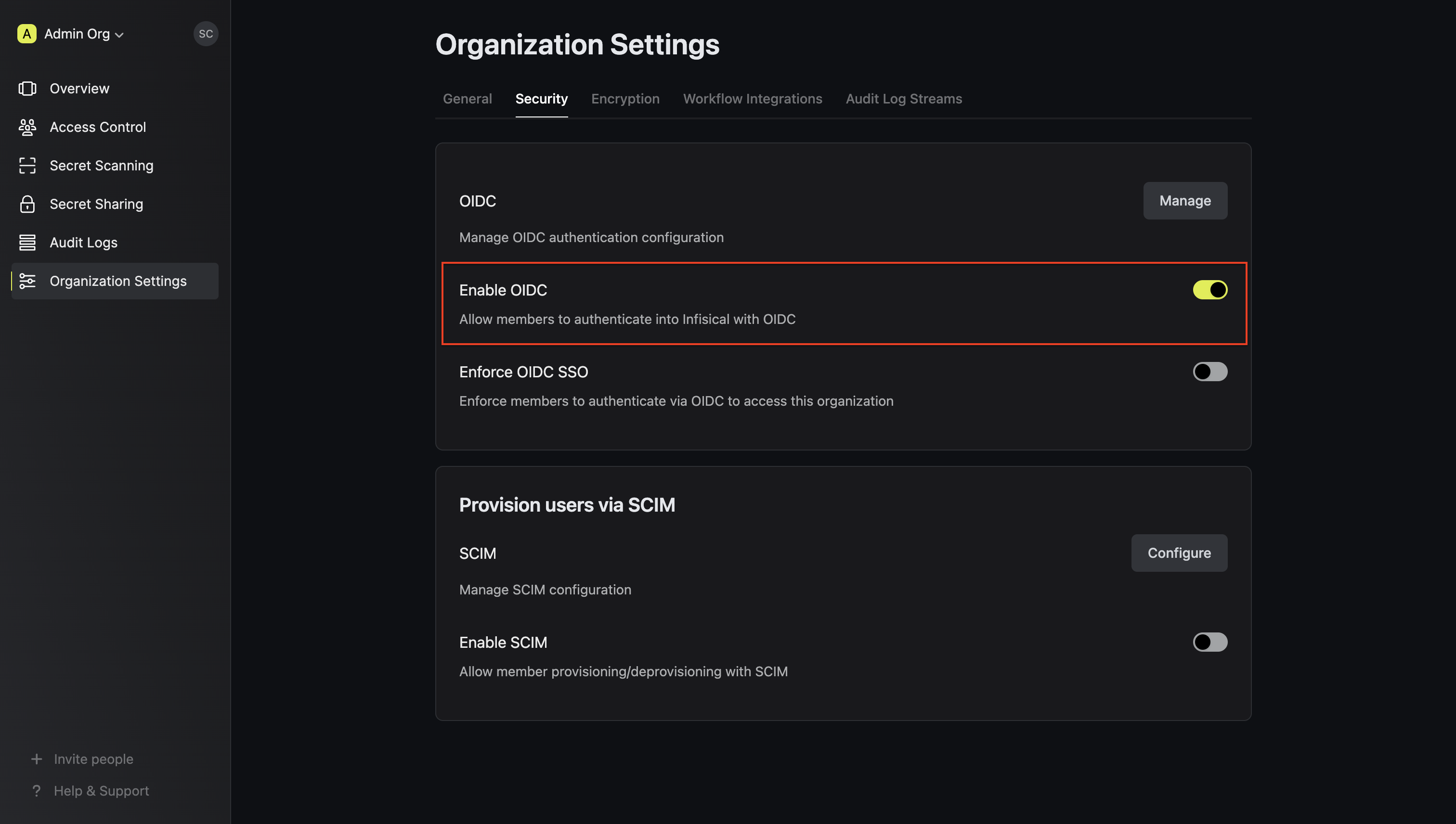
Enforcing OIDC SSO ensures that members in your organization can only access Infisical
by logging into the organization via Auth0.
To enforce OIDC SSO, you're required to test out the OpenID connection by successfully authenticating at least one Auth0 user with Infisical.
Once you've completed this requirement, you can toggle the **Enforce OIDC SSO** button to enforce OIDC SSO.
We recommend ensuring that your account is provisioned using the application in Auth0
prior to enforcing OIDC SSO to prevent any unintended issues.
If you are only using one organization on your Infisical instance, you can configure a default organization in the [Server Admin Console](../admin-panel/server-admin#default-organization) to expedite OIDC login.
If you're configuring OIDC SSO on a self-hosted instance of Infisical, make
sure to set the `AUTH_SECRET` and `SITE_URL` environment variable for it to
work:
* `AUTH_SECRET`: A secret key used for signing and verifying JWT. This
can be a random 32-byte base64 string generated with `openssl rand -base64
32`.
* `SITE_URL`: The absolute URL of your self-hosted instance of Infisical including the protocol (e.g. [https://app.infisical.com](https://app.infisical.com))
# Entra ID / Azure AD SAML
Learn how to configure Microsoft Entra ID for Infisical SSO.
Azure SAML SSO is a paid feature.
If you're using Infisical Cloud, then it is available under the **Pro Tier**. If you're self-hosting Infisical,
then you should contact [sales@infisical.com](mailto:sales@infisical.com) to purchase an enterprise license to use it.
In Infisical, head to your Organization Settings > Authentication > SAML SSO Configuration and select **Set up SAML SSO**.
Next, copy the **Reply URL (Assertion Consumer Service URL)** and **Identifier (Entity ID)** to use when configuring the Azure SAML application.
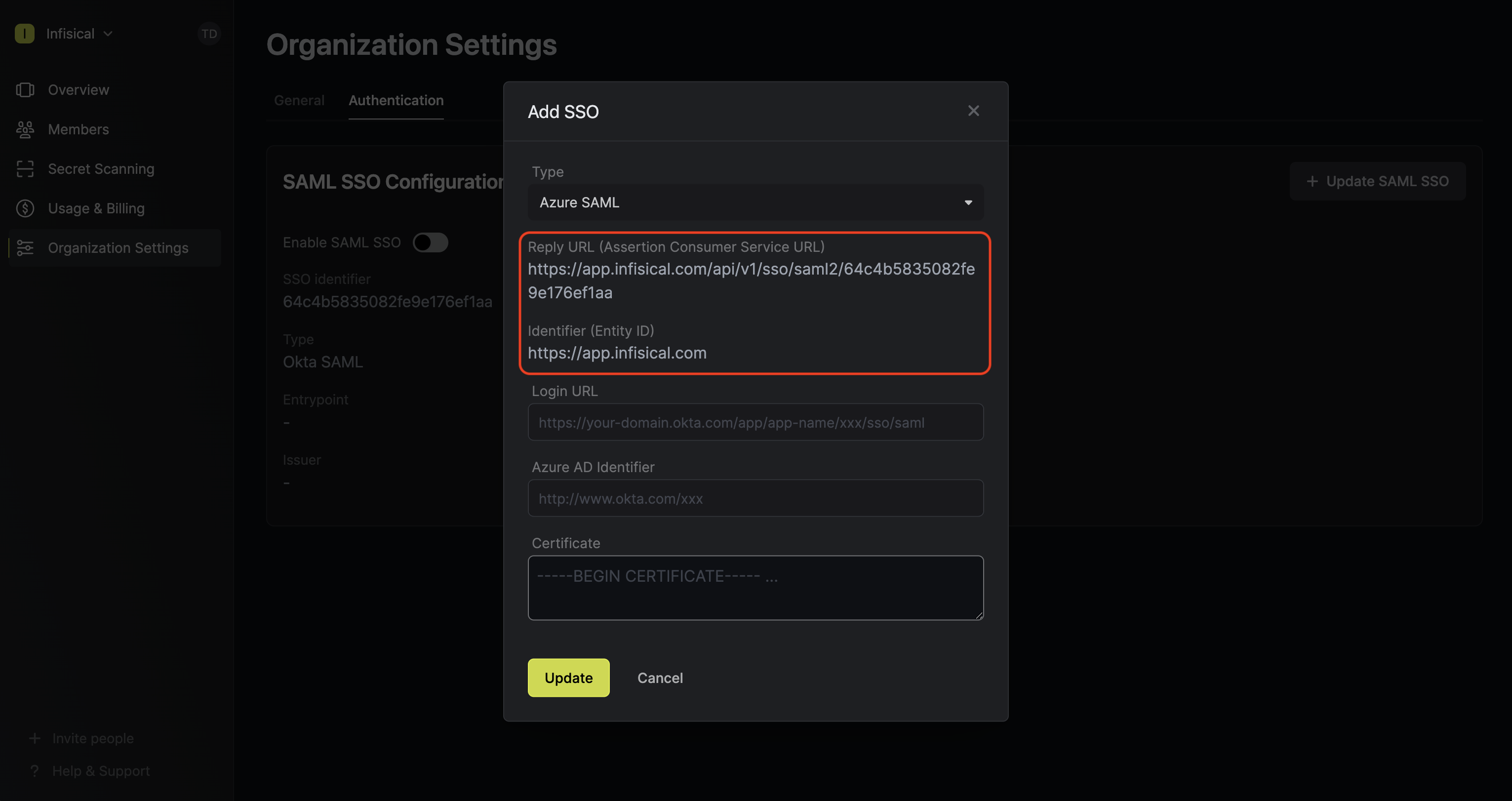
In the Azure Portal, navigate to the Azure Active Directory and select **Enterprise applications**. On this screen, select **+ New application**.
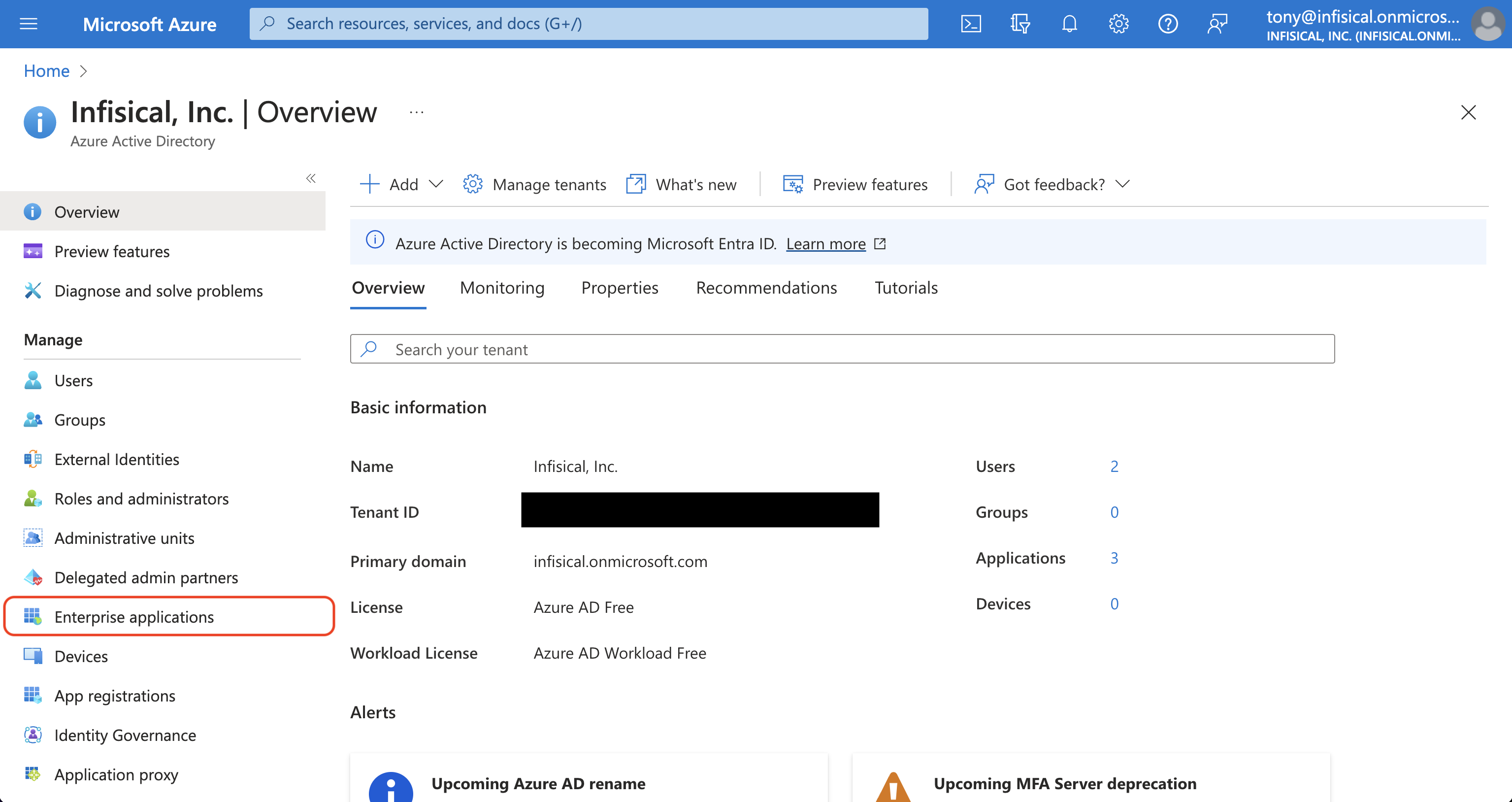
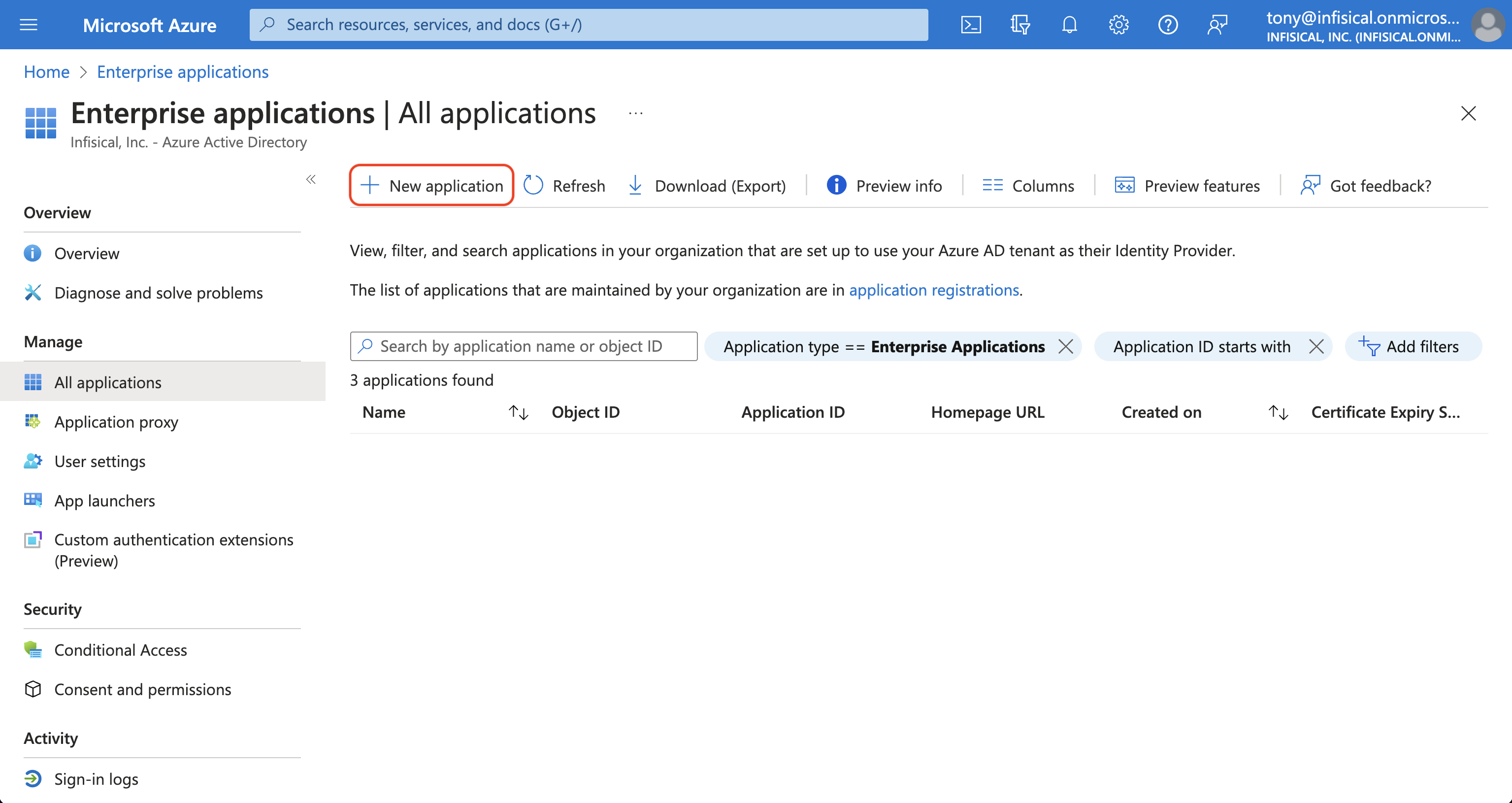
On the next screen, press the **+ Create your own application** button.
Give the application a unique name like Infisical; choose the "Integrate any other application you don't find in the gallery (Non-gallery)"
option and hit the **Create** button.
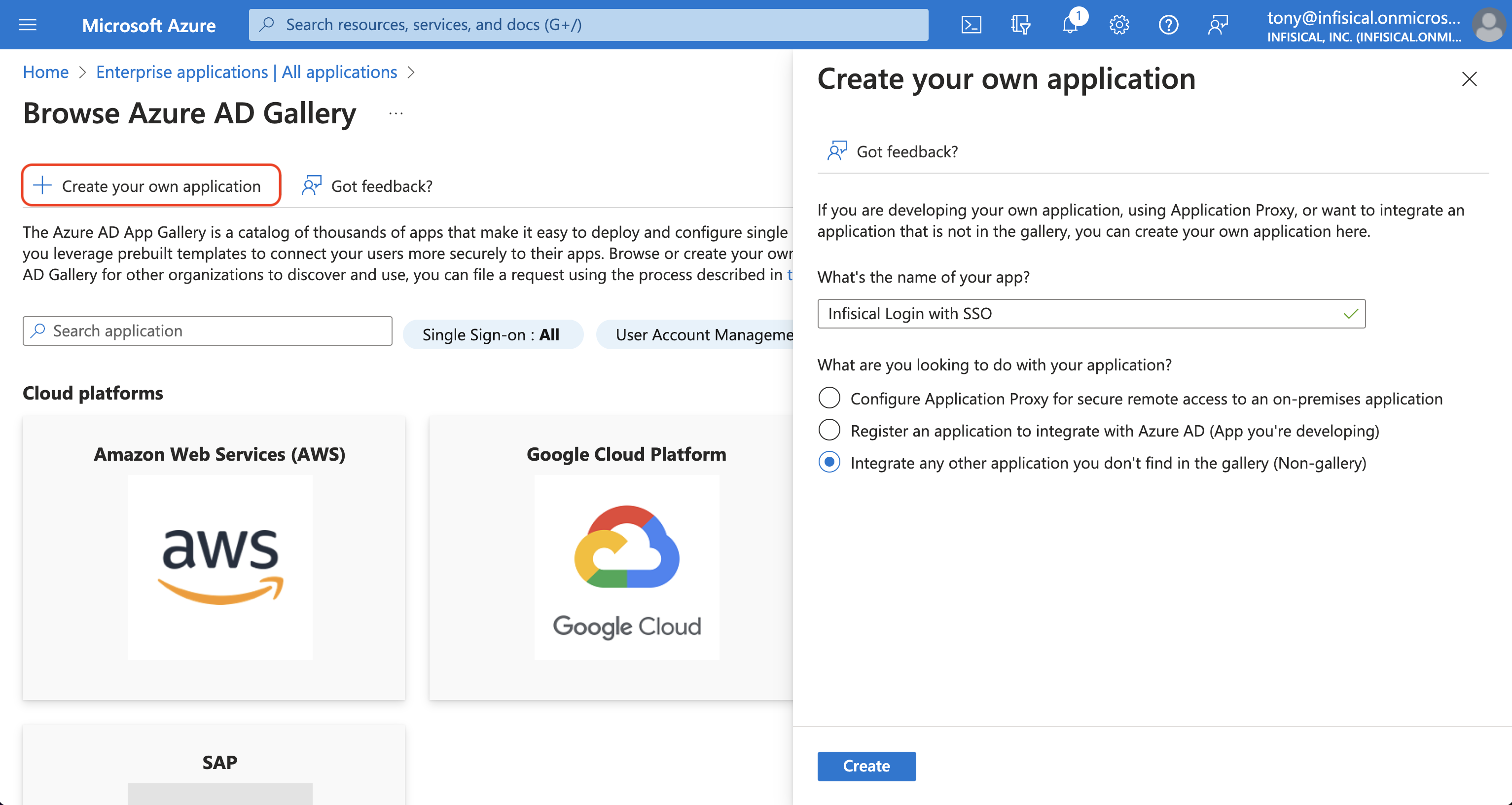
On the application overview screen, select **Single sign-on** from the left sidebar. From there, select the **SAML** single sign-on method.
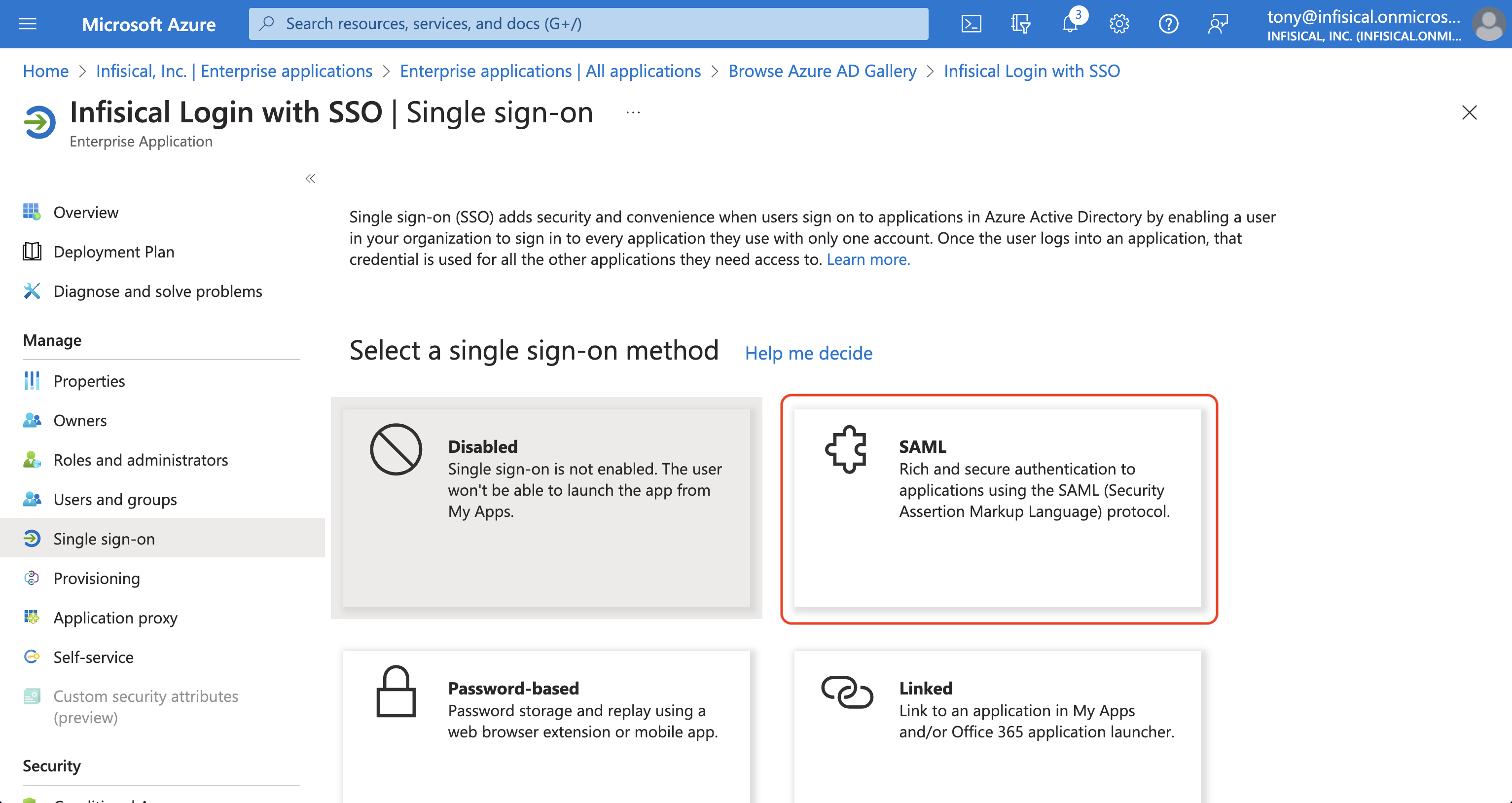
Next, select **Edit** in the **Basic SAML Configuration** section and add/set the **Identifier (Entity ID)** to **Entity ID** and add/set the **Reply URL (Assertion Consumer Service URL)** to **ACS URL** from step 1.
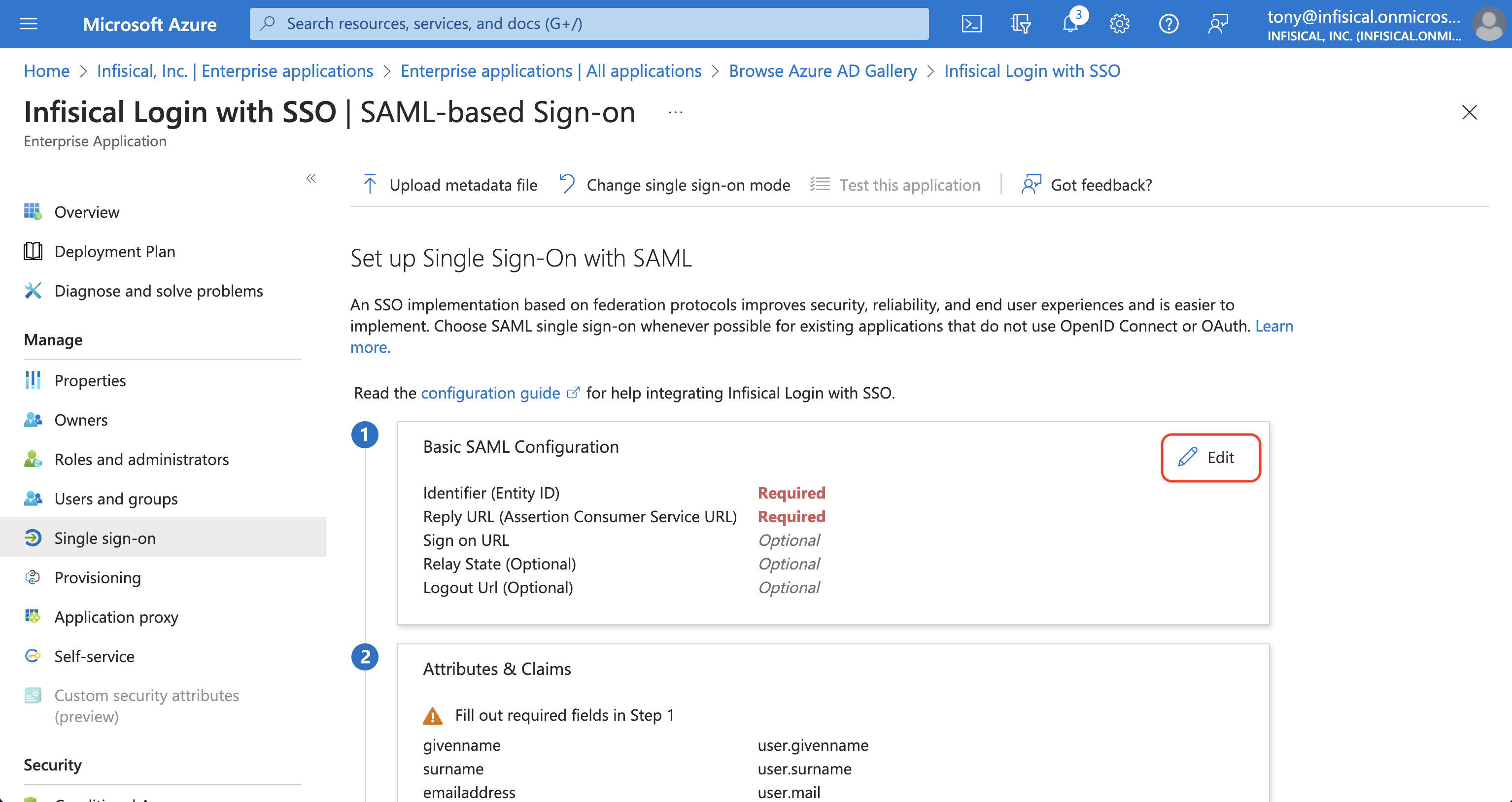
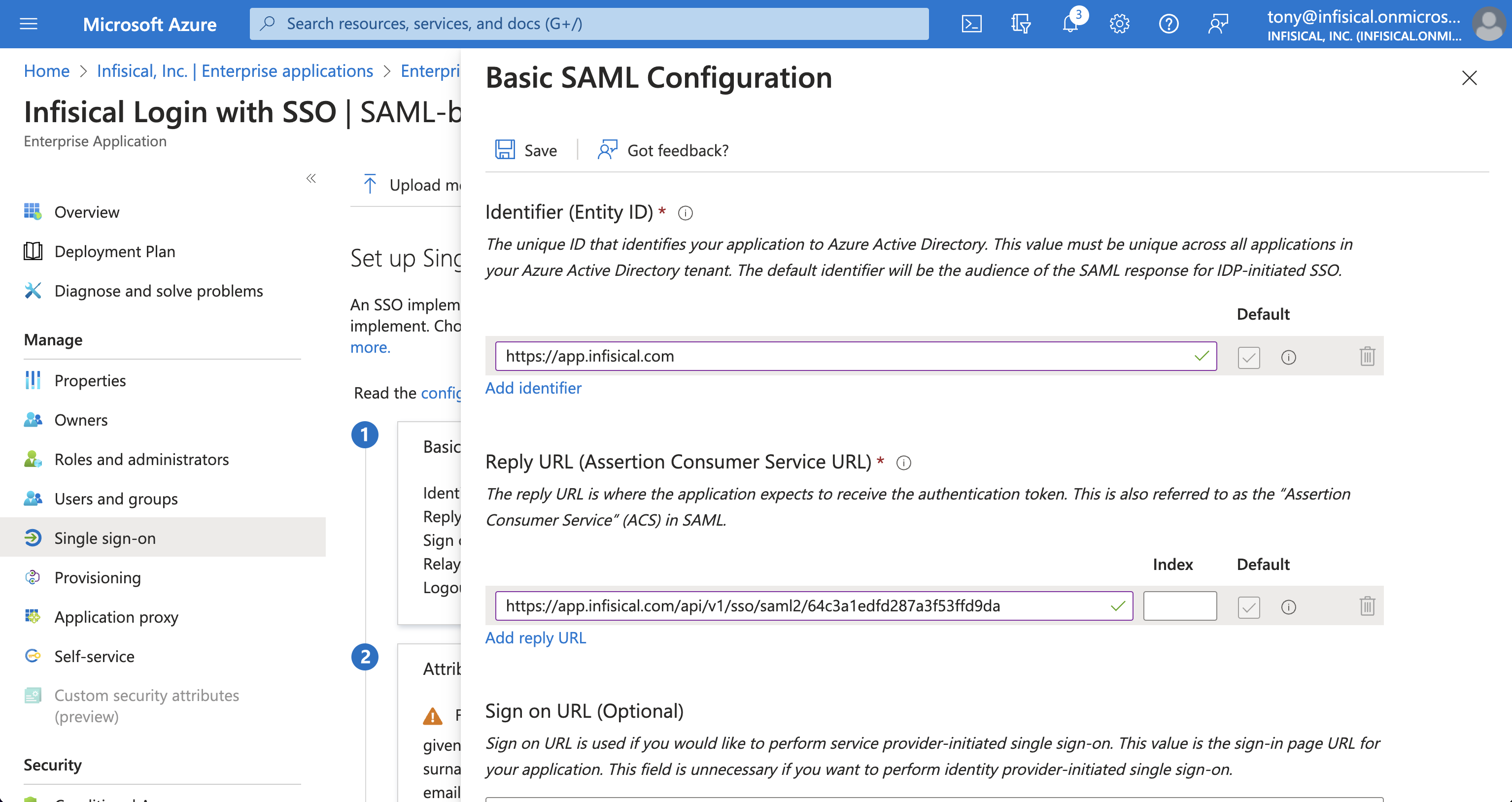
If you're self-hosting Infisical, then you will want to replace
`https://app.infisical.com` with your own domain.
Back in the **Set up Single Sign-On with SAML** screen, select **Edit** in the **Attributes & Claims** section and configure the following map:
* `email -> user.userprinciplename`
* `firstName -> user.givenname`
* `lastName -> user.surname`
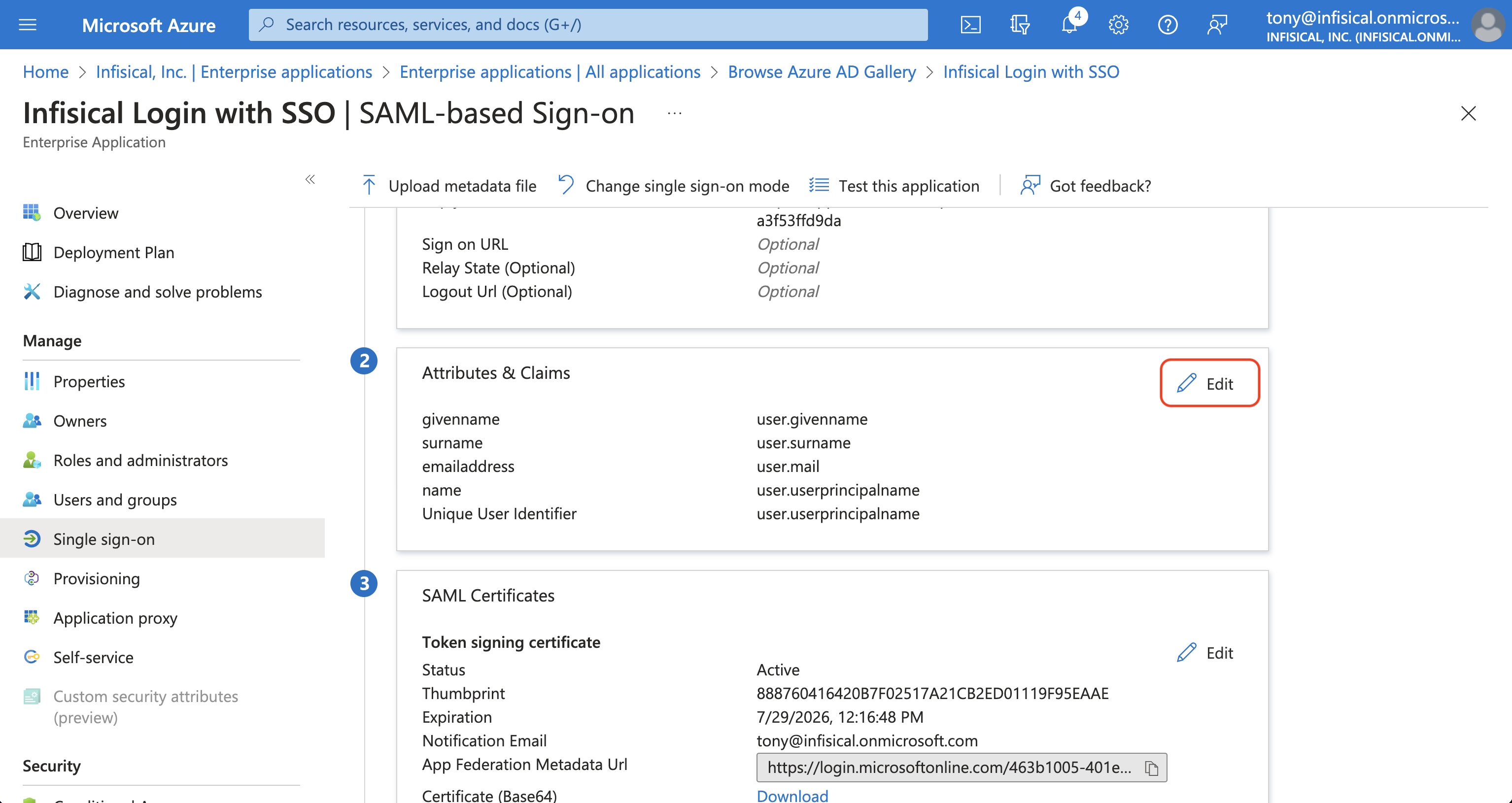
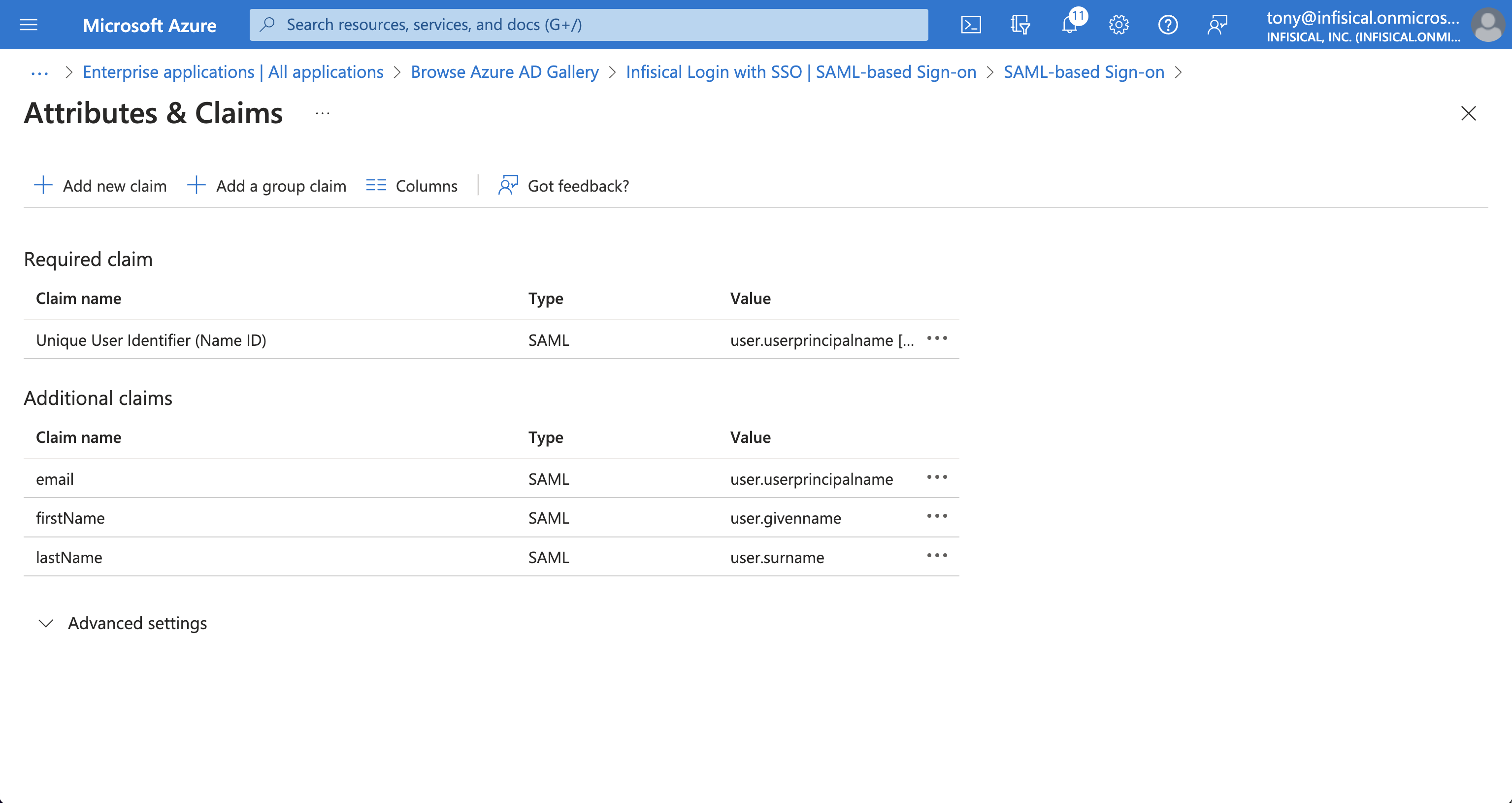
Back in the **Set up Single Sign-On with SAML** screen, select **Edit** in the **SAML Certificates** section and set the **Signing Option** field to **Sign SAML response and assertion**.
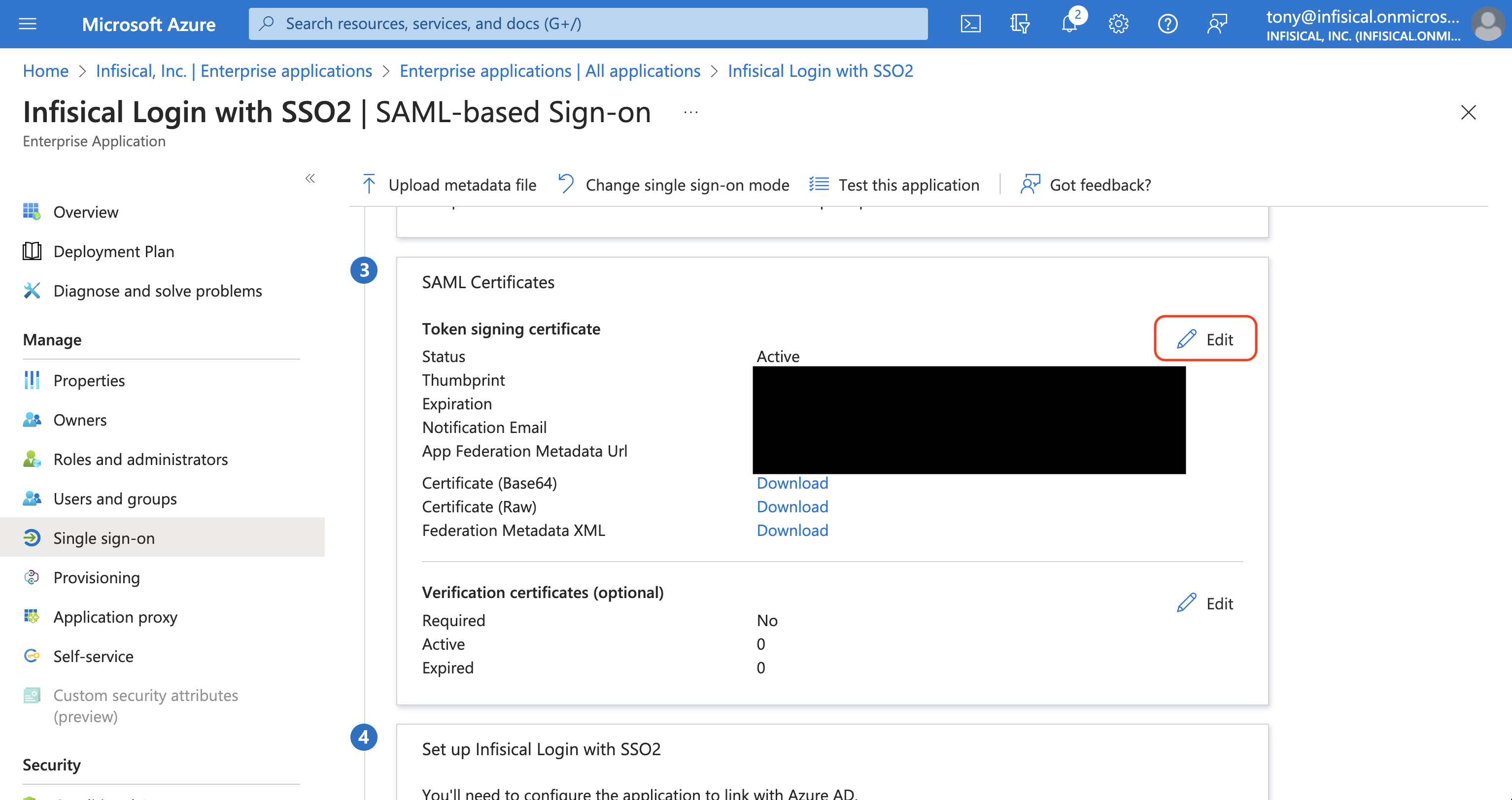
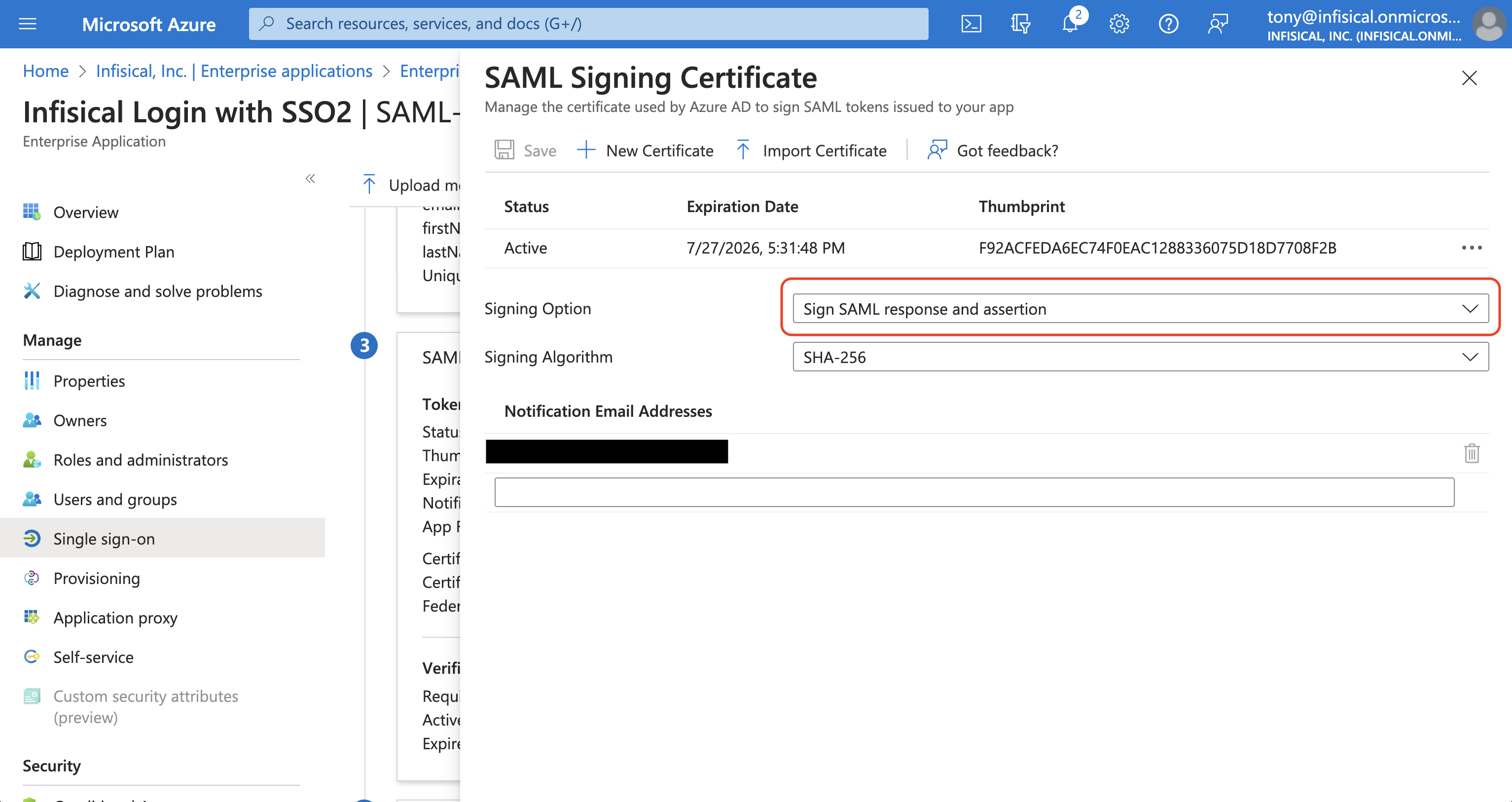
In the **Set up Single Sign-On with SAML** screen, copy the **Login URL** and **SAML Certificate** to use when finishing configuring Azure SAML in Infisical.
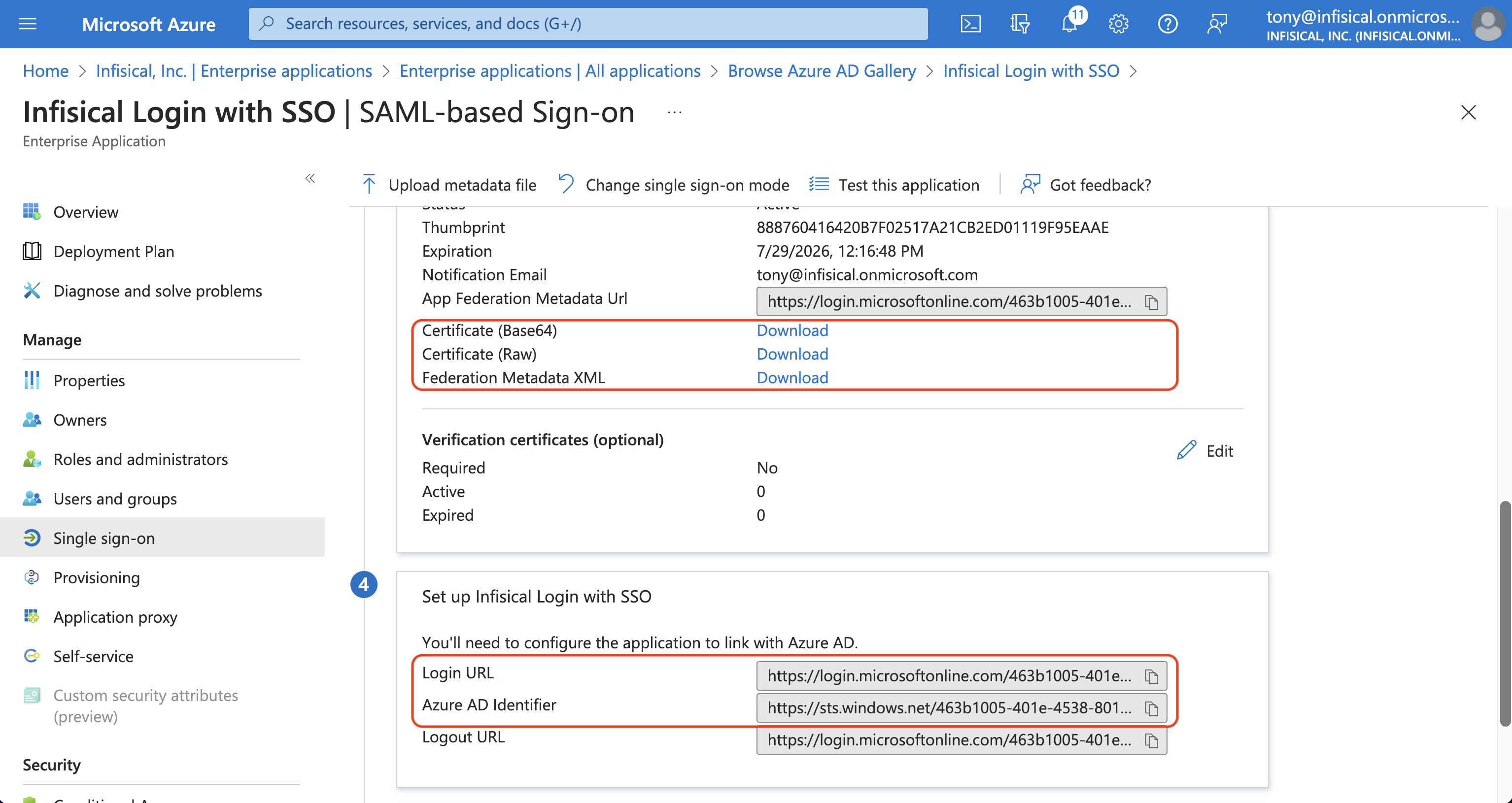
In the **Properties** screen, copy the **Application ID** to use when finishing configuring Azure SAML in Infisical.
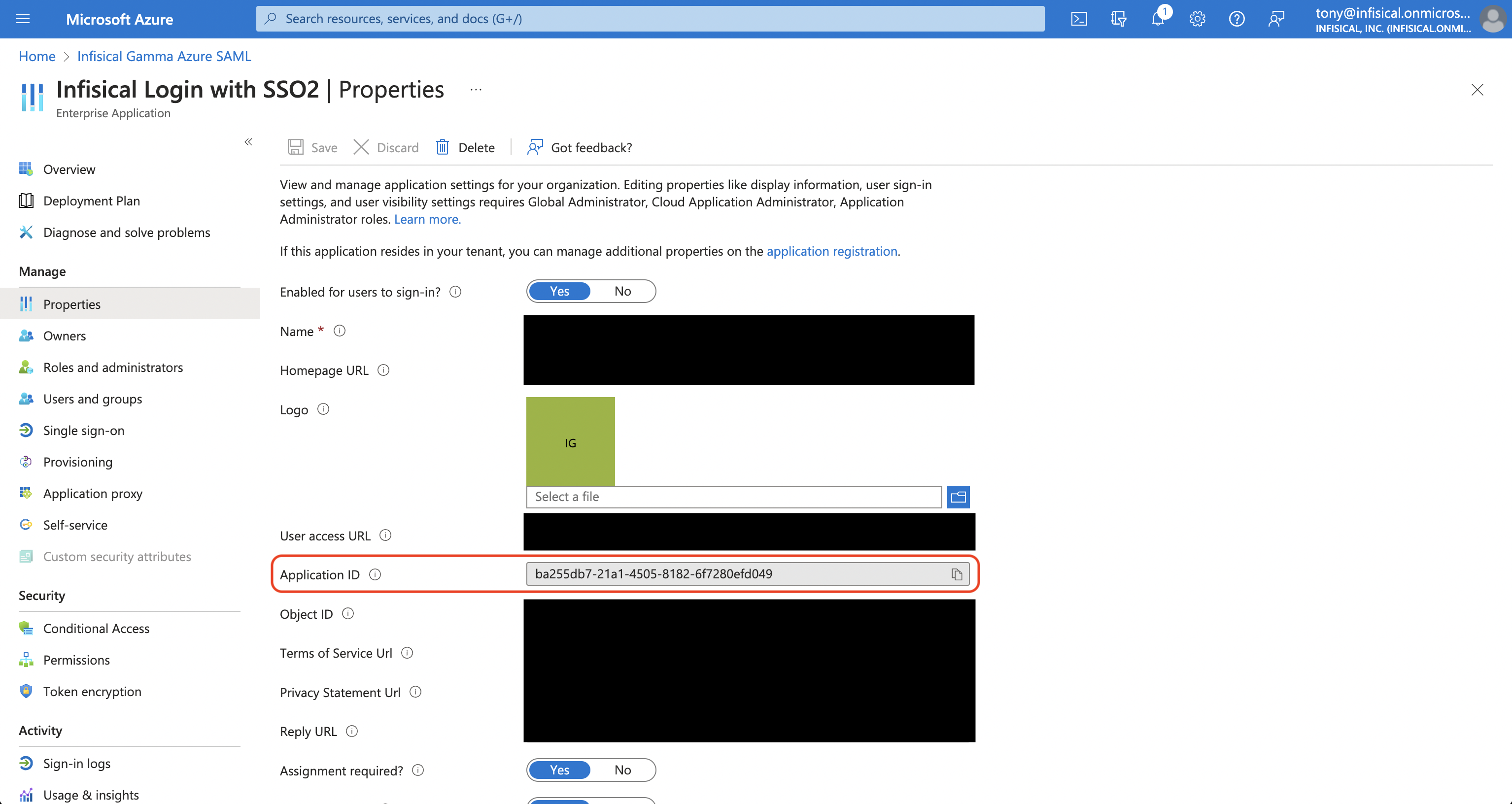
Back in Infisical, set **Login URL**, **Azure Application ID**, and **SAML Certificate** from step 3. Once you've done that, press **Update** to complete the required configuration.
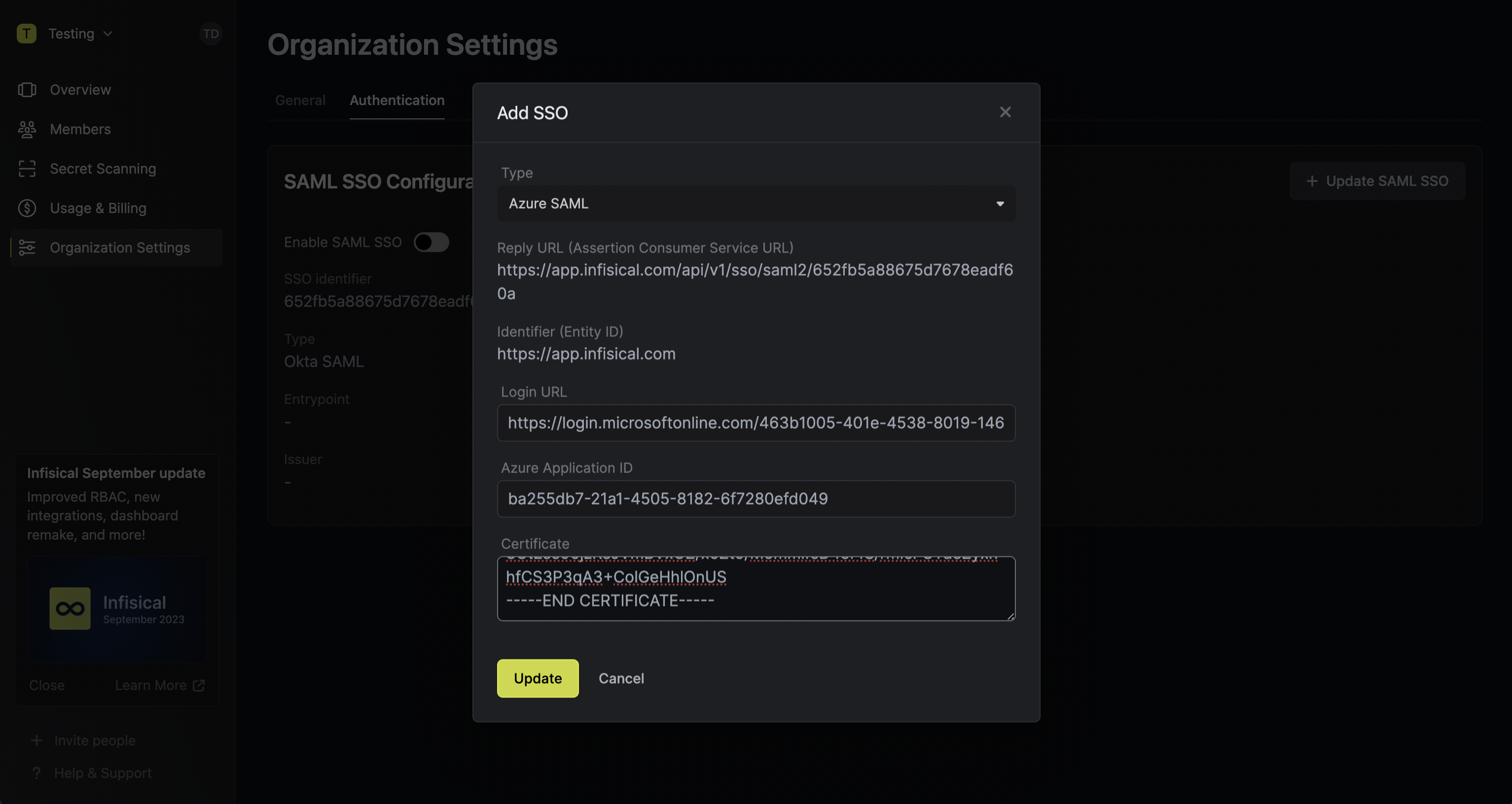
When pasting the certificate into Infisical, you'll want to retain `-----BEGIN
CERTIFICATE-----` and `-----END CERTIFICATE-----` at the first and last line
of the text area respectively.
Having trouble?, try copying the X509 certificate information from the Federation Metadata XML file in Azure.
Back in Azure, navigate to the **Users and groups** tab and select **+ Add user/group** to assign access to the login with SSO application on a user or group-level.
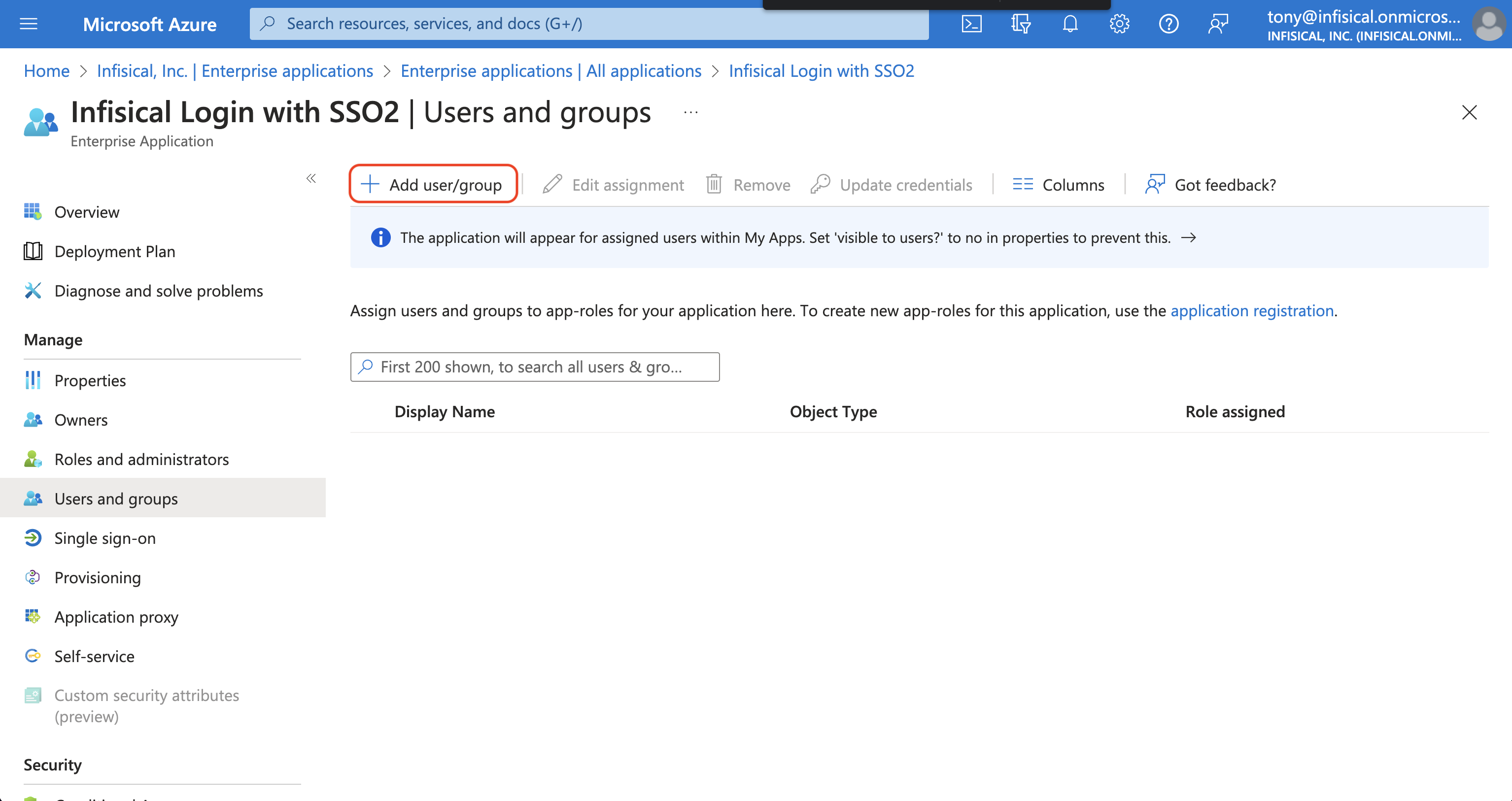
Enabling SAML SSO allows members in your organization to log into Infisical via Azure.
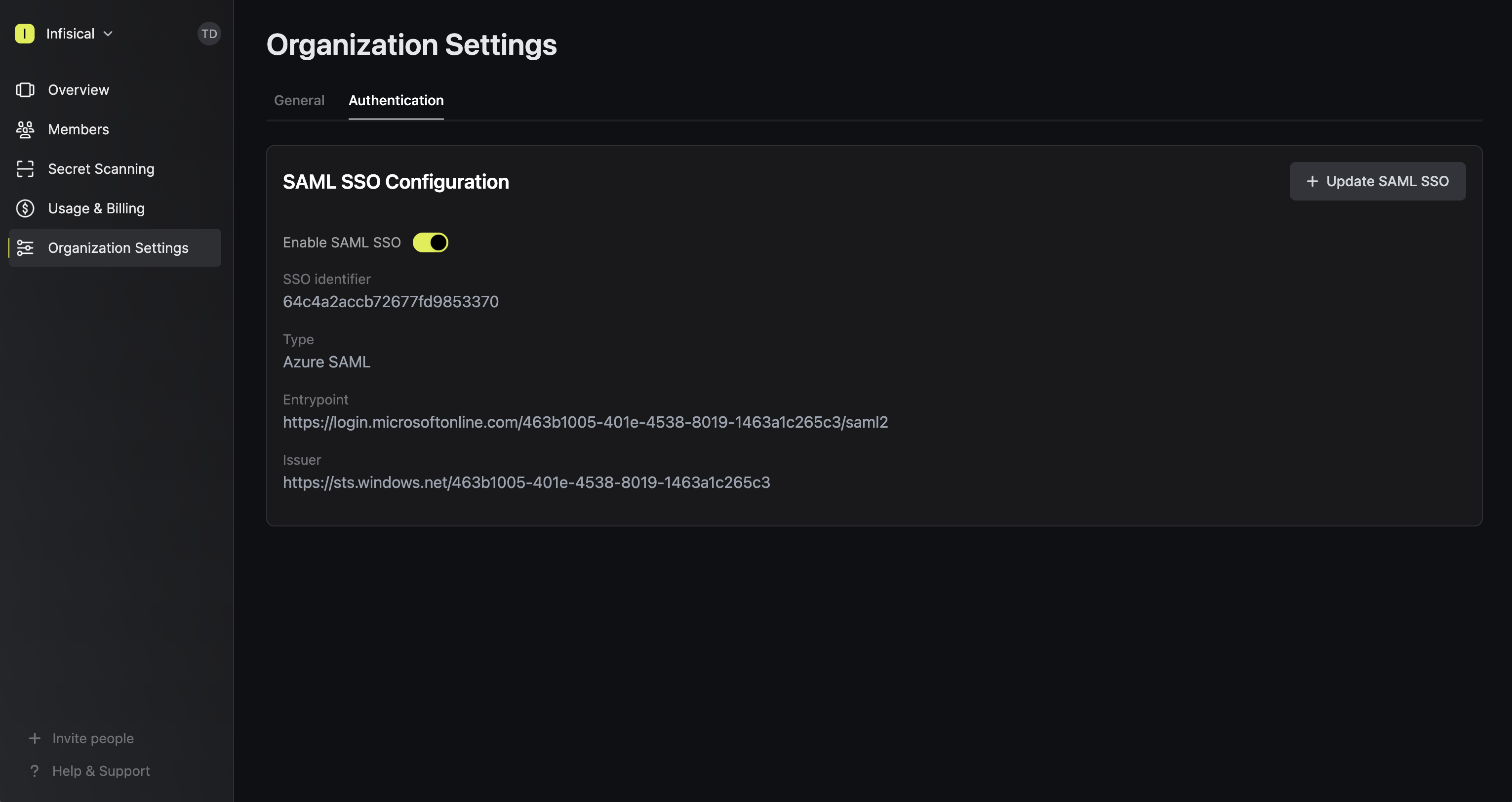
Enforcing SAML SSO ensures that members in your organization can only access Infisical
by logging into the organization via Azure.
To enforce SAML SSO, you're required to test out the SAML connection by successfully authenticating at least one Azure user with Infisical;
Once you've completed this requirement, you can toggle the **Enforce SAML SSO** button to enforce SAML SSO.
We recommend ensuring that your account is provisioned the application in Azure
prior to enforcing SAML SSO to prevent any unintended issues.
If you are only using one organization on your Infisical instance, you can configure a default organization in the [Server Admin Console](../admin-panel/server-admin#default-organization) to expedite SAML login.
If you're configuring SAML SSO on a self-hosted instance of Infisical, make
sure to set the `AUTH_SECRET` and `SITE_URL` environment variable for it to
work:
* `AUTH_SECRET`: A secret key used for signing and verifying JWT. This
can be a random 32-byte base64 string generated with `openssl rand -base64
32`.
* `SITE_URL`: The absolute URL of your self-hosted instance of Infisical including the protocol (e.g. [https://app.infisical.com](https://app.infisical.com))
If you'd like to require Multi-factor Authentication for your team members to access Infisical check out our [Entra ID / Azure AD MFA](../mfa#entra-id-azure-ad-mfa) guide.
# General OIDC
Learn how to configure OIDC for Infisical SSO with any OIDC-compliant identity provider
OIDC SSO is a paid feature. If you're using Infisical Cloud, then it is
available under the **Pro Tier**. If you're self-hosting Infisical, then you
should contact [sales@infisical.com](mailto:sales@infisical.com) to purchase an enterprise license to use
it.
You can configure your organization in Infisical to have members authenticate with the platform through identity providers via [OpenID Connect](https://openid.net/specs/openid-connect-core-1_0.html).
Prerequisites:
* The identity provider (Okta, Google, Azure AD, etc.) should support OIDC.
* Users in the IdP should have a configured `email` and `given_name`.
1.1. Register your application with the IdP to obtain a **Client ID** and **Client Secret**. These credentials are used by Infisical to authenticate with your IdP.
1.2. Configure **Redirect URL** to be `https://app.infisical.com/api/v1/sso/oidc/callback`. If you're self-hosting Infisical, replace the domain with your own.
1.3. Configure the scopes needed by Infisical (email, profile, openid) and ensure that they are mapped to the ID token claims.
1.4. Access the IdP’s OIDC discovery document (usually located at `https:///.well-known/openid-configuration`). This document contains important endpoints such as authorization, token, userinfo, and keys.
2.1. Back in Infisical, in the Organization settings > Security > OIDC, click Connect.

2.2. You can configure OIDC either through the Discovery URL (Recommended) or by inputting custom endpoints.
To configure OIDC via Discovery URL, set the **Configuration Type** field to **Discovery URL** and fill out the **Discovery Document URL** field.
Note that the Discovery Document URL typically takes the form: `https:///.well-known/openid-configuration`.
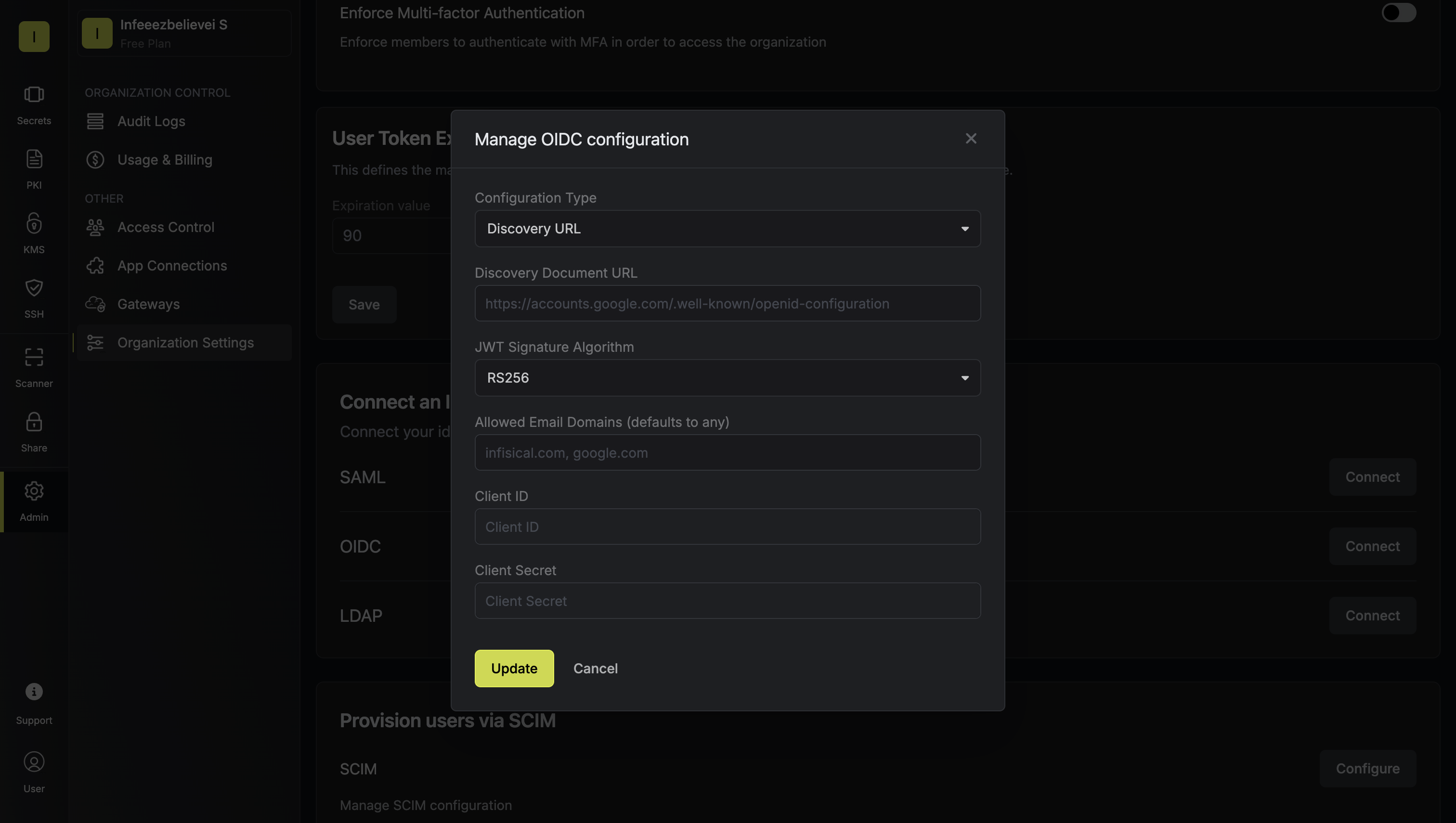
To configure OIDC via the custom endpoints, set the **Configuration Type** field to **Custom** and input the required endpoint fields.
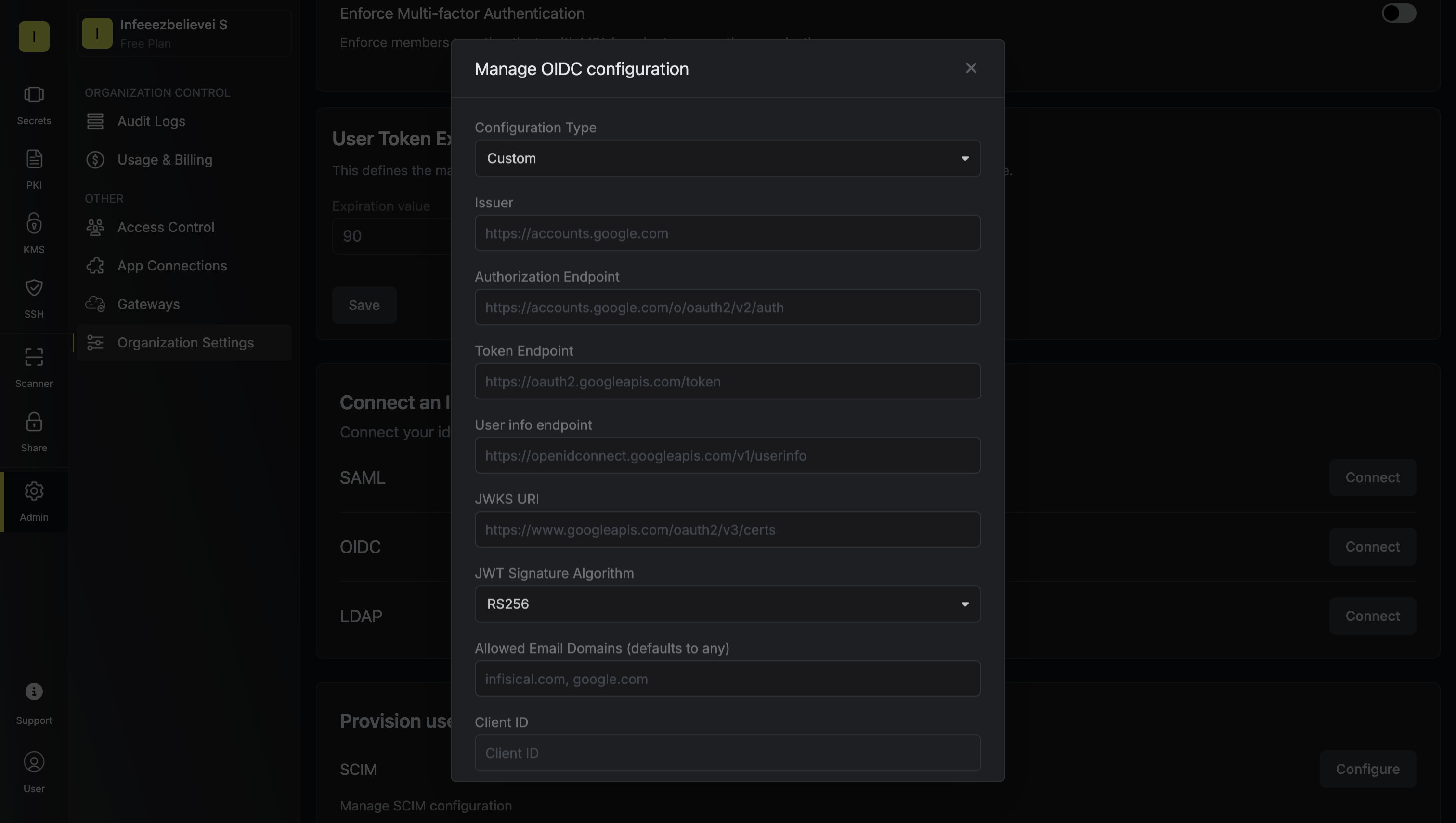
2.3. Optionally, you can define a whitelist of allowed email domains.
Finally, fill out the **Client ID** and **Client Secret** fields and press **Update** to complete the required configuration.
Enabling OIDC SSO allows members in your organization to log into Infisical via the configured Identity Provider
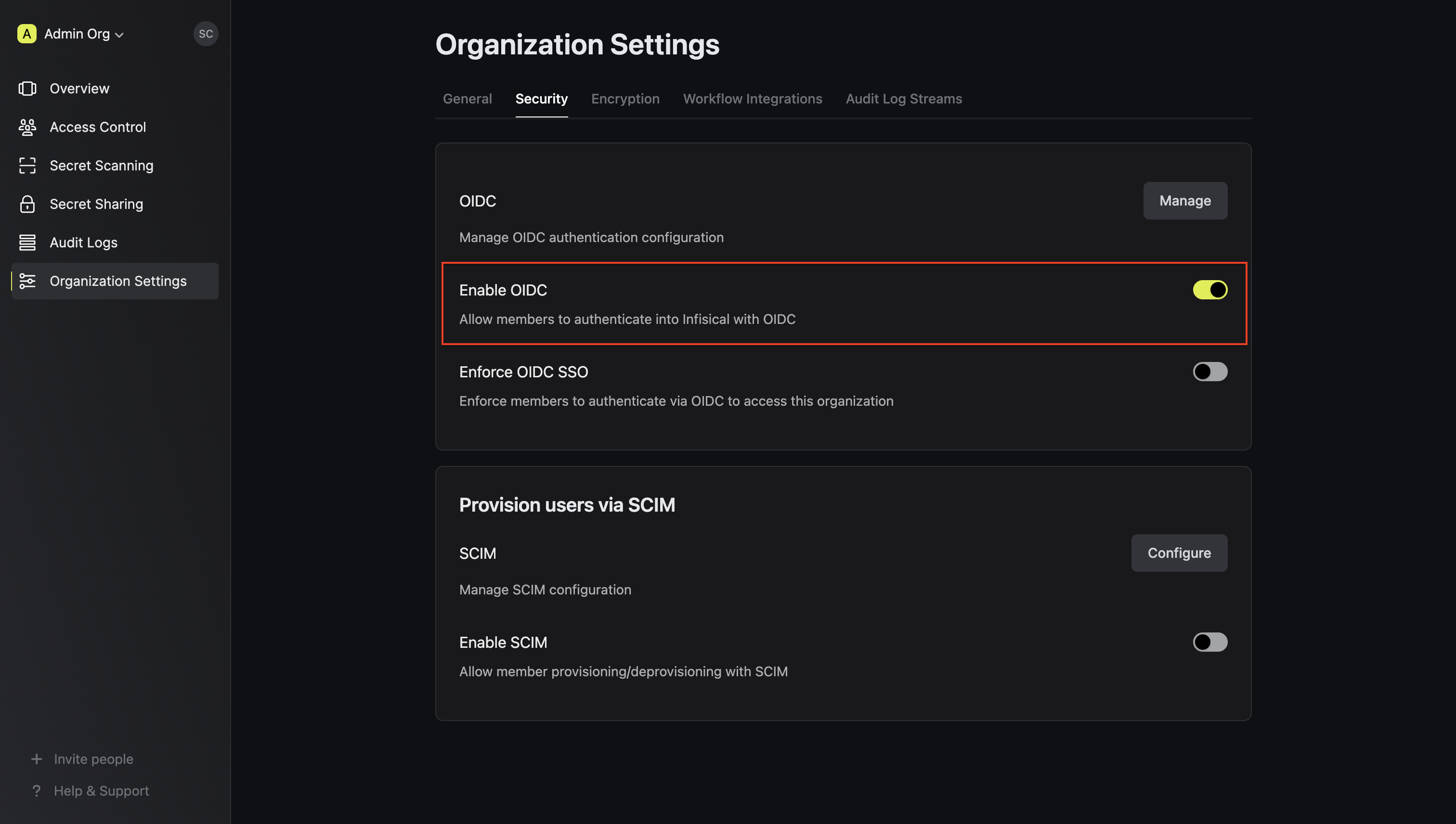
Enforcing OIDC SSO ensures that members in your organization can only access Infisical
by logging into the organization via the Identity provider.
To enforce OIDC SSO, you're required to test out the OpenID connection by successfully authenticating at least one IdP user with Infisical.
Once you've completed this requirement, you can toggle the **Enforce OIDC SSO** button to enforce OIDC SSO.
We recommend ensuring that your account is provisioned using the identity provider prior to enforcing OIDC SSO to prevent any unintended issues.
If you are only using one organization on your Infisical instance, you can configure a default organization in the [Server Admin Console](../admin-panel/server-admin#default-organization) to expedite OIDC login.
If you're configuring OIDC SSO on a self-hosted instance of Infisical, make
sure to set the `AUTH_SECRET` and `SITE_URL` environment variable for it to
work:
* `AUTH_SECRET`: A secret key used for signing and verifying JWT. This
can be a random 32-byte base64 string generated with `openssl rand -base64
32`.
* `SITE_URL`: The absolute URL of your self-hosted instance of Infisical including the protocol (e.g. [https://app.infisical.com](https://app.infisical.com))
# GitHub SSO
Learn how to configure GitHub SSO for Infisical.
Using GitHub SSO on a self-hosted instance of Infisical requires configuring an OAuth2 application in GitHub and registering your instance with it.
Navigate to your user Settings > Developer settings > OAuth Apps to create a new GitHub OAuth application.
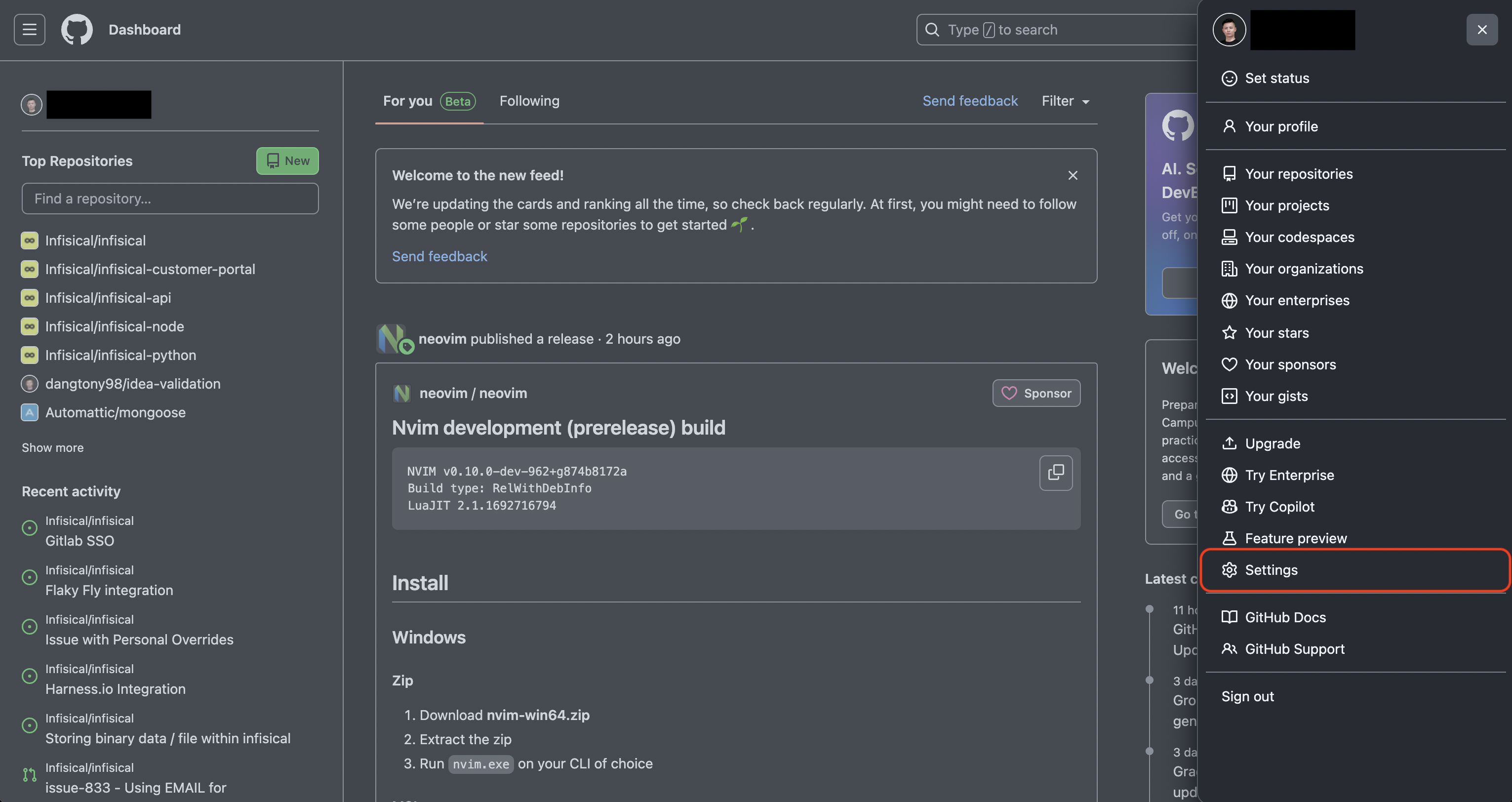
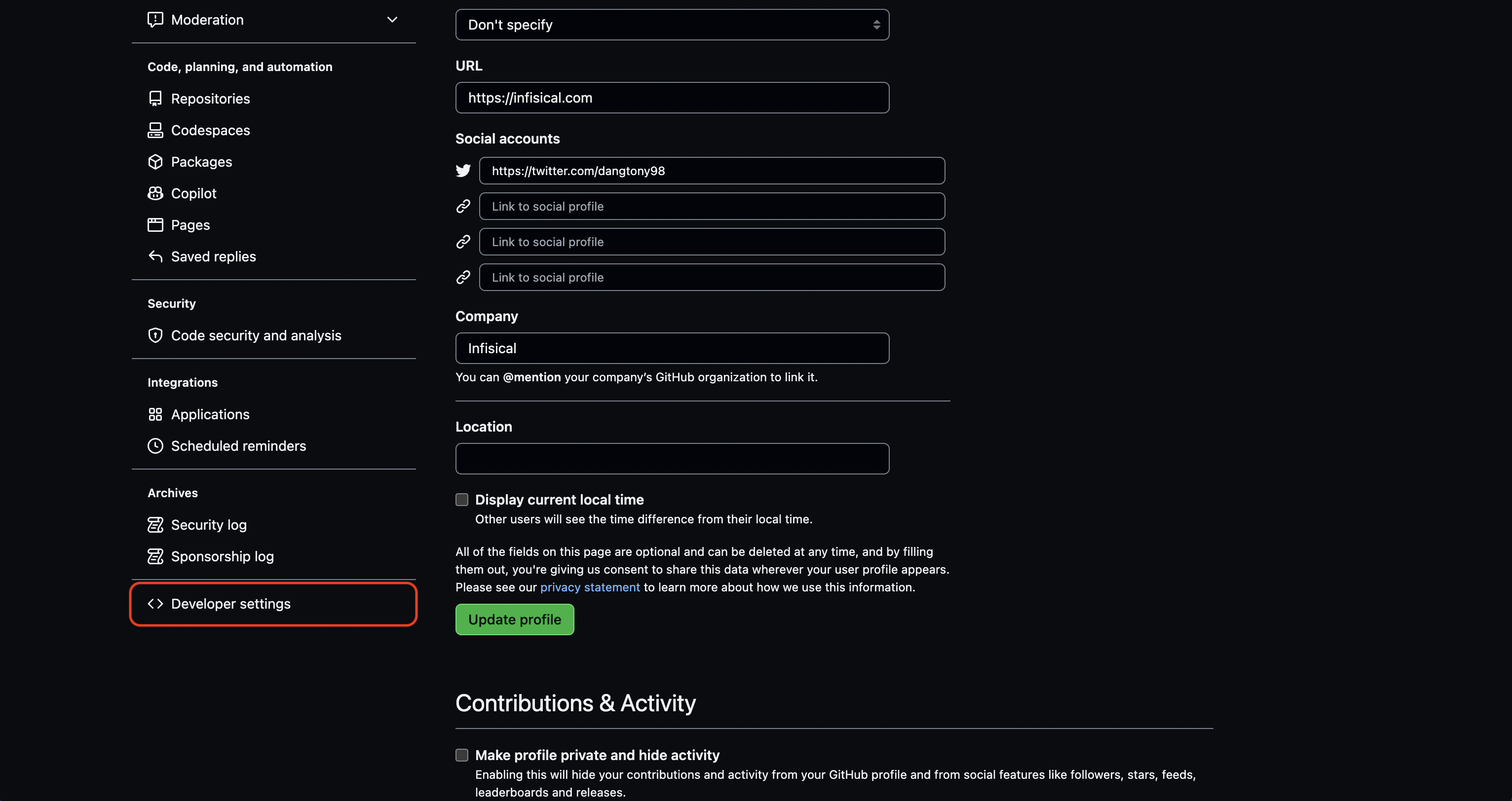
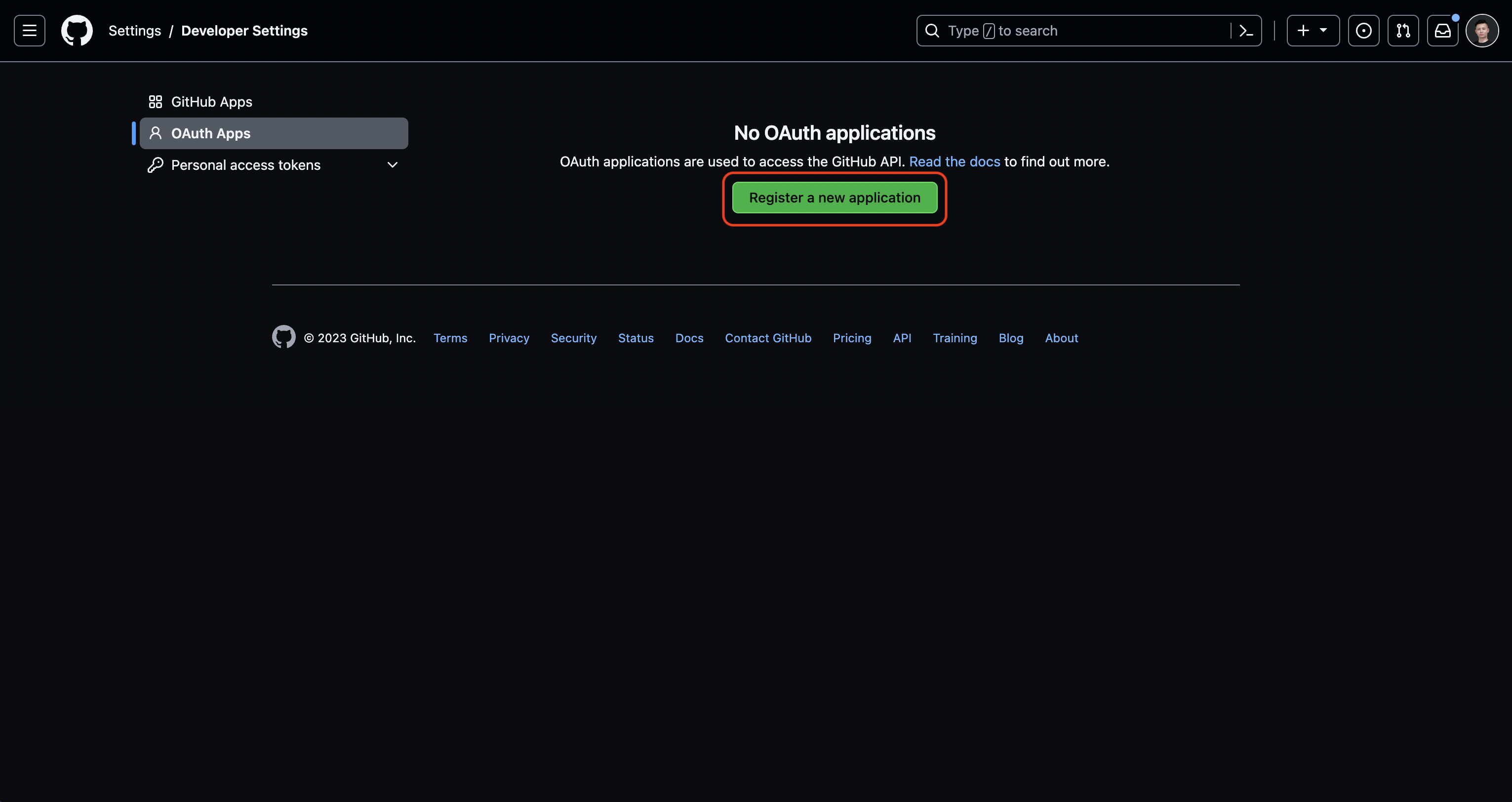
Create the OAuth application. As part of the form, set the **Homepage URL** to your self-hosted domain `https://your-domain.com`
and the **Authorization callback URL** to `https://your-domain.com/api/v1/sso/github`.
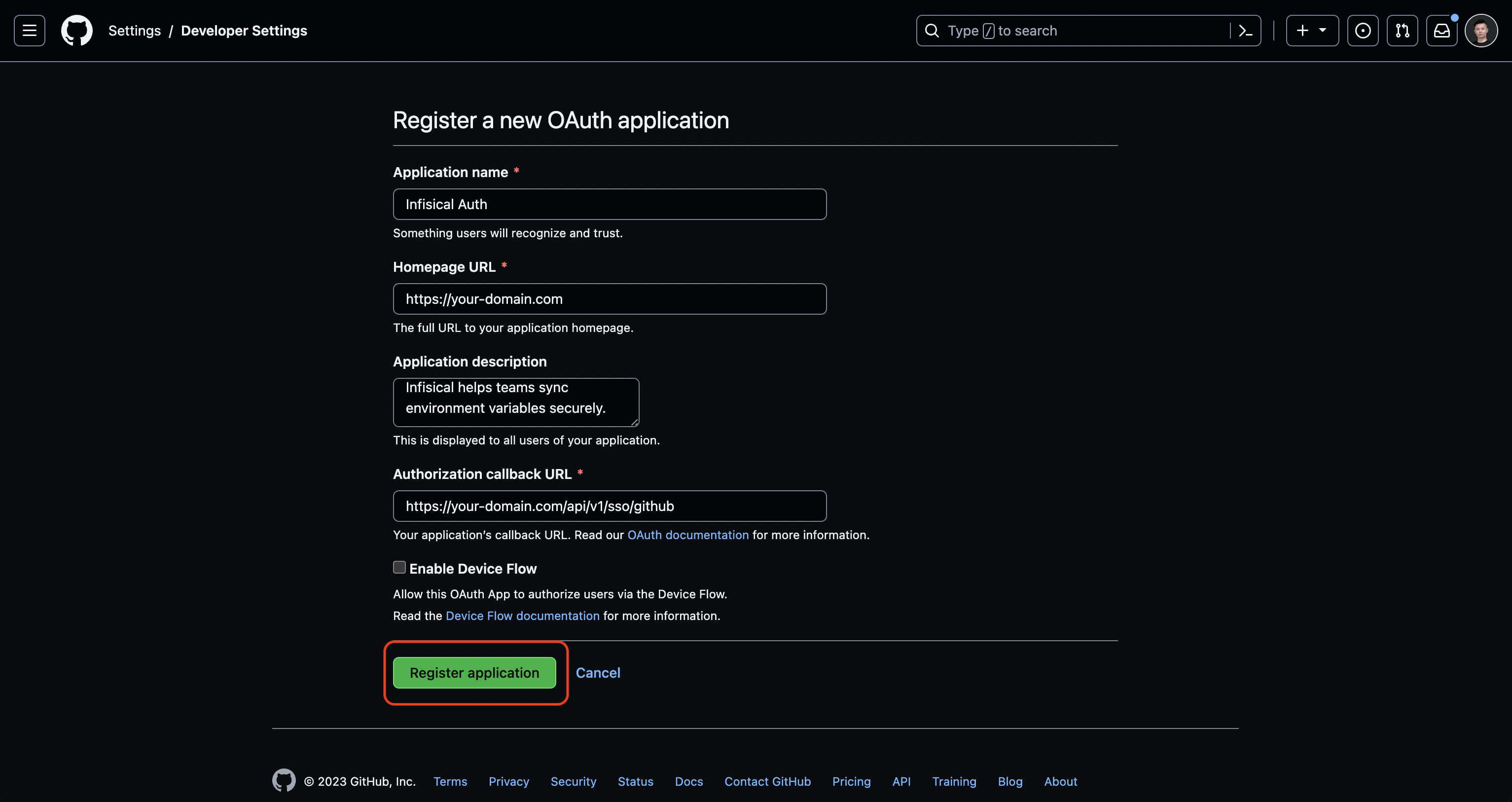
If you have a GitHub organization, you can create an OAuth application under it
in your organization Settings > Developer settings > OAuth Apps > New Org OAuth App.
Obtain the **Client ID** and generate a new **Client Secret** for your GitHub OAuth application.
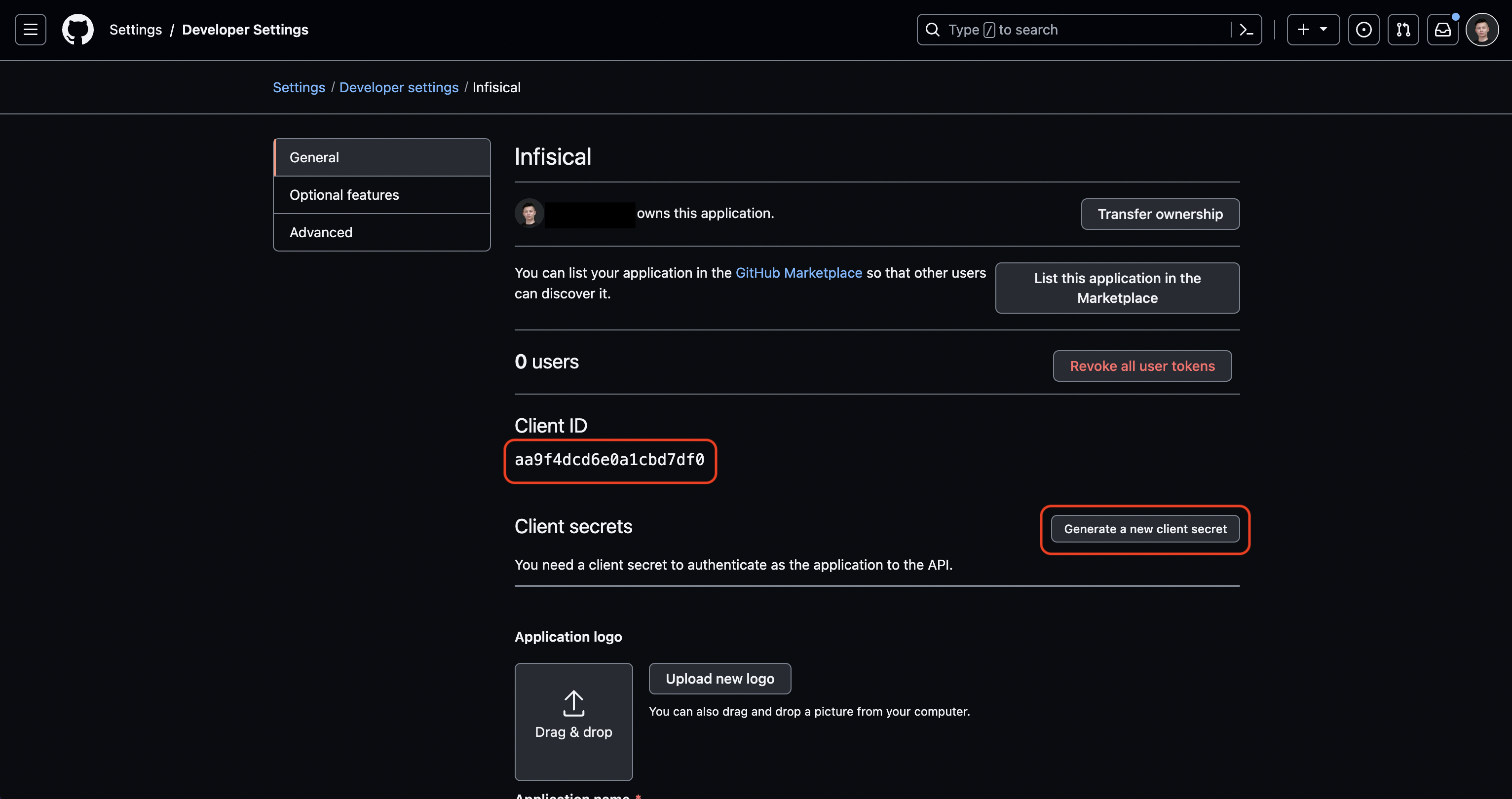
Back in your Infisical instance, make sure to set the following environment variables:
* `CLIENT_ID_GITHUB_LOGIN`: The **Client ID** of your GitHub OAuth application.
* `CLIENT_SECRET_GITHUB_LOGIN`: The **Client Secret** of your GitHub OAuth application.
* `AUTH_SECRET`: A secret key used for signing and verifying JWT. This can be a random 32-byte base64 string generated with `openssl rand -base64 32`.
* `SITE_URL`: The URL of your self-hosted instance of Infisical - should be an absolute URL including the protocol (e.g. [https://app.infisical.com](https://app.infisical.com))
Once added, restart your Infisical instance and log in with GitHub.
## FAQ
It is likely that you have misconfigured your self-hosted instance of Infisical. You should:
* Check that you have set the `CLIENT_ID_GITHUB_LOGIN`, `CLIENT_SECRET_GITHUB_LOGIN`,
`AUTH_SECRET`, and `SITE_URL` environment variables.
* Check that the **Authorization callback URL** specified in GitHub matches the `SITE_URL` environment variable.
For example, if the former is `https://app.infisical.com/api/v1/sso/github` then the latter should be `https://app.infisical.com`.
# GitLab SSO
Learn how to configure GitLab SSO for Infisical.
Using GitLab SSO on a self-hosted instance of Infisical requires configuring an OAuth application in GitLab and registering your instance with it.
Navigate to your user Settings > Applications to create a new GitLab application.
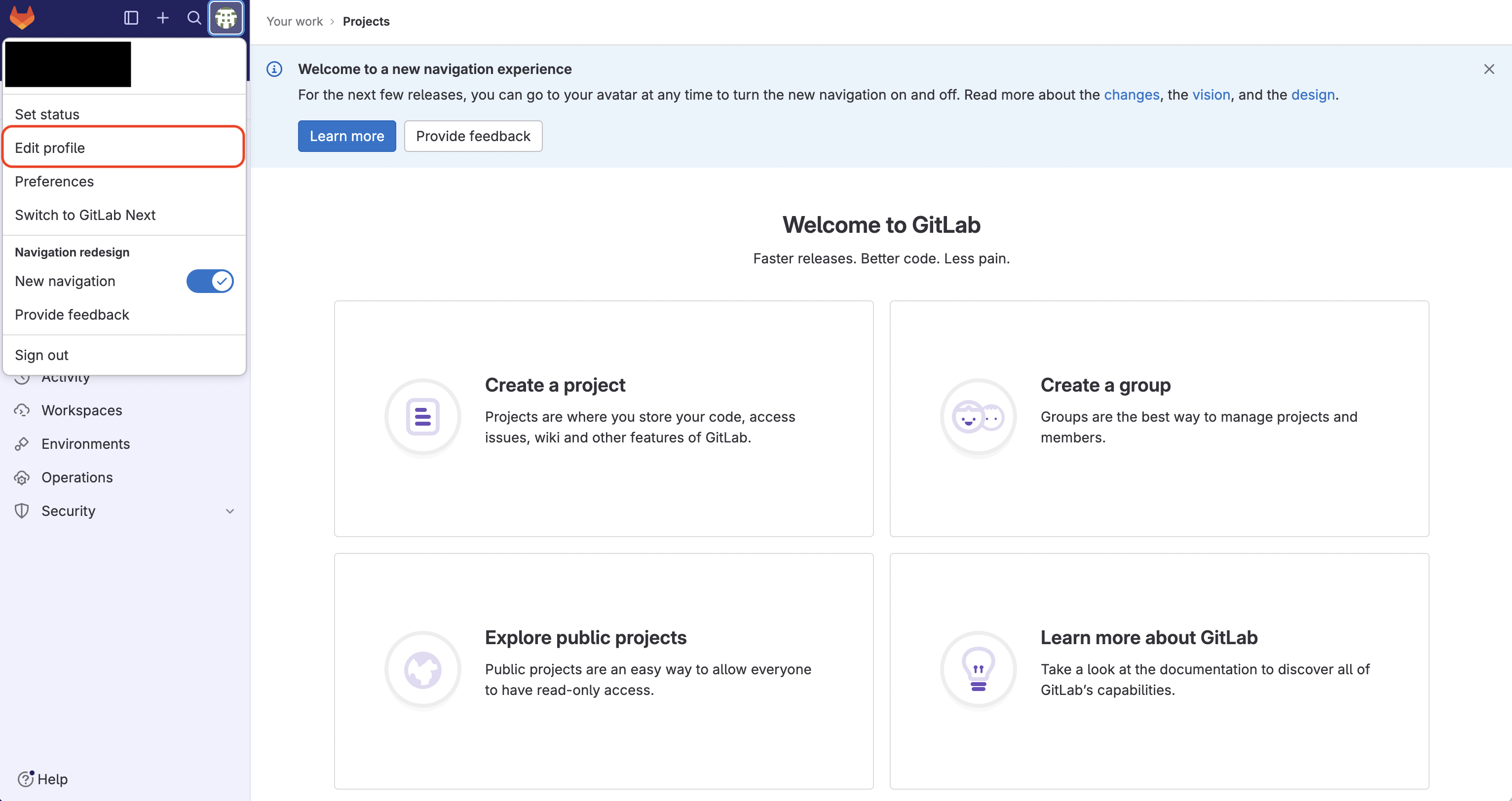
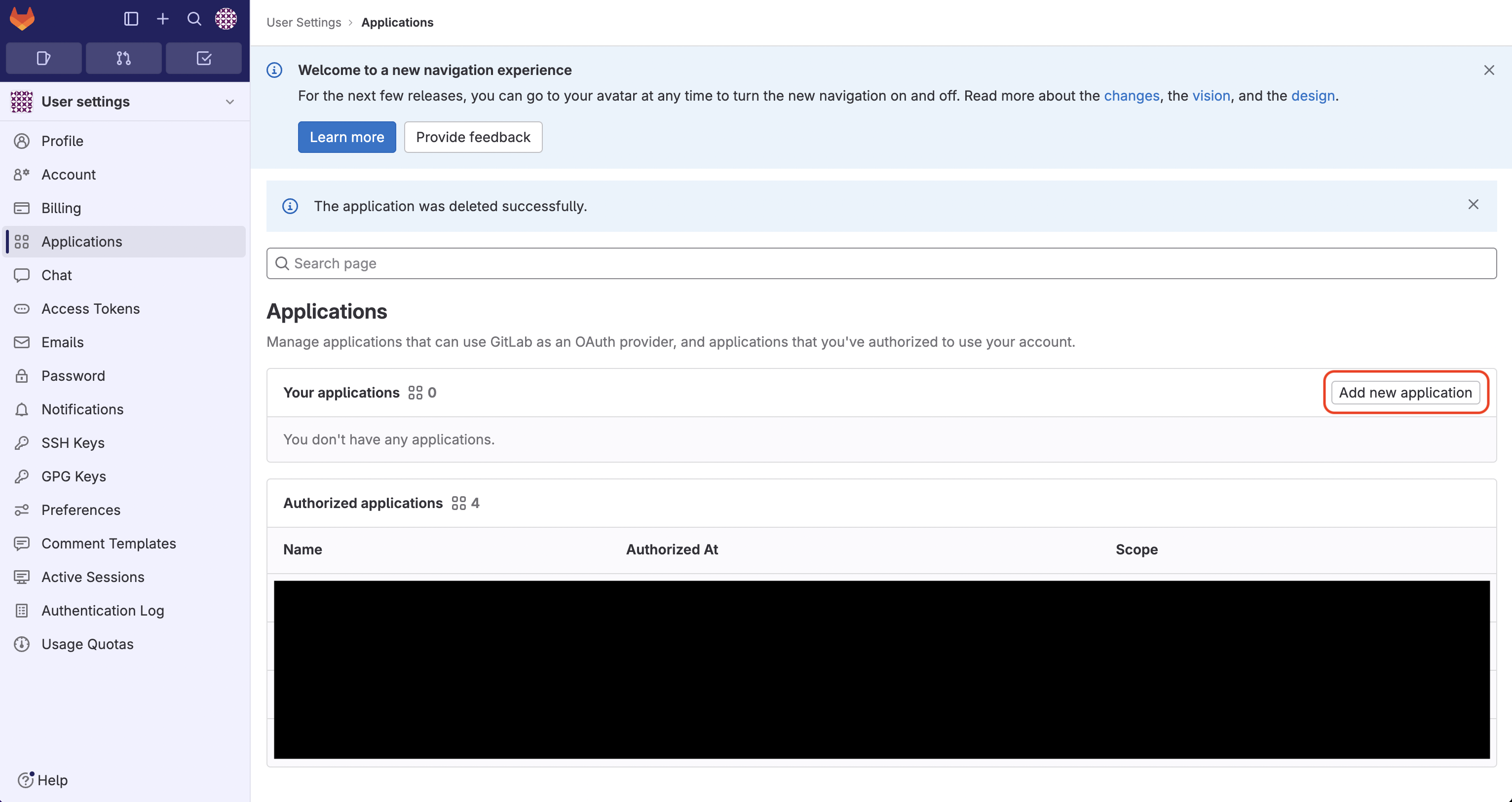
Create the application. As part of the form, set the **Redirect URI** to `https://your-domain.com/api/v1/sso/gitlab`.
Note that only `read_user` is required as part of the **Scopes** configuration.
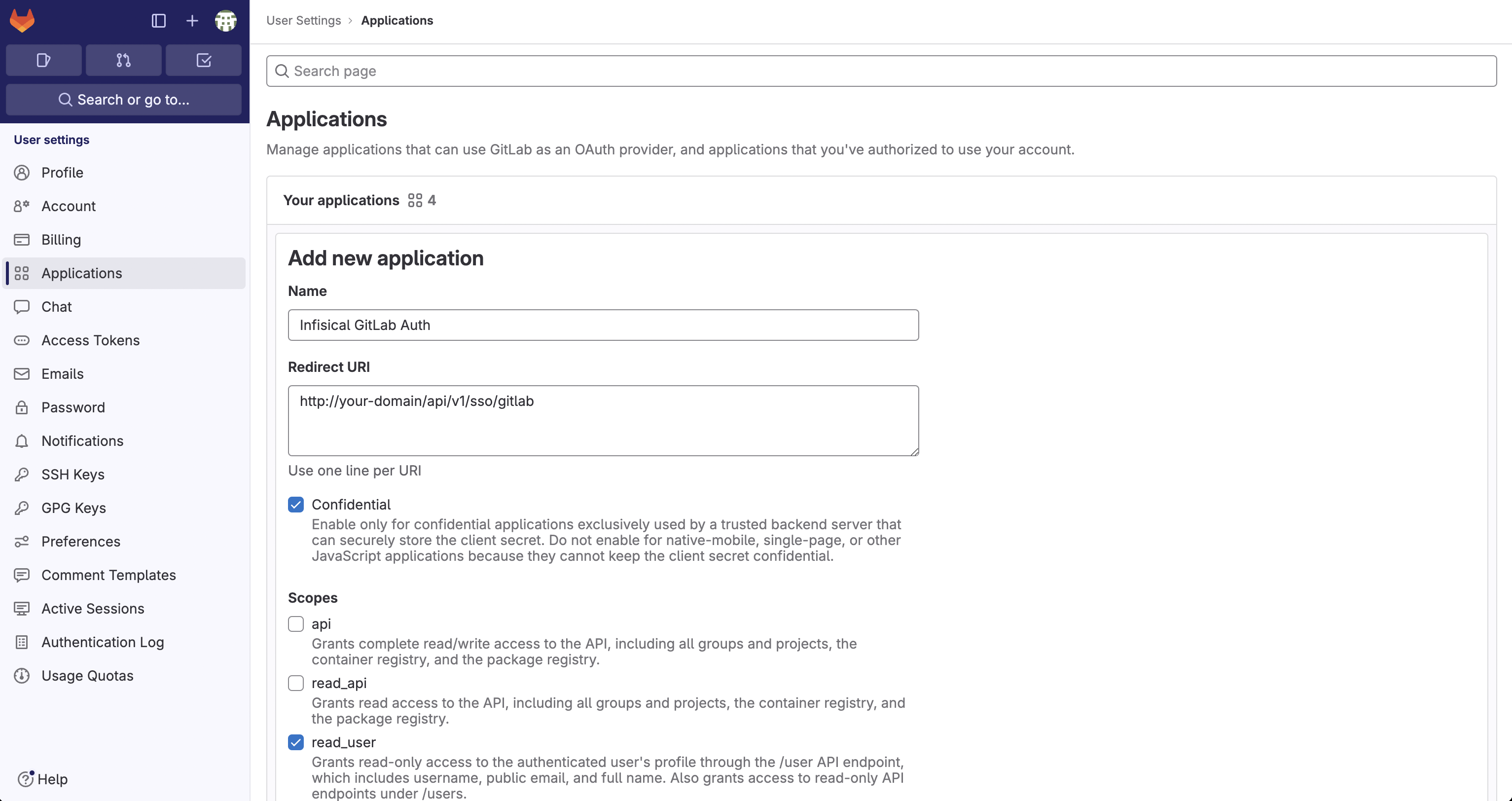
If you have a GitLab group, you can create an OAuth application under it
in your group Settings > Applications.
Obtain the **Application ID** and **Secret** for your GitLab application.
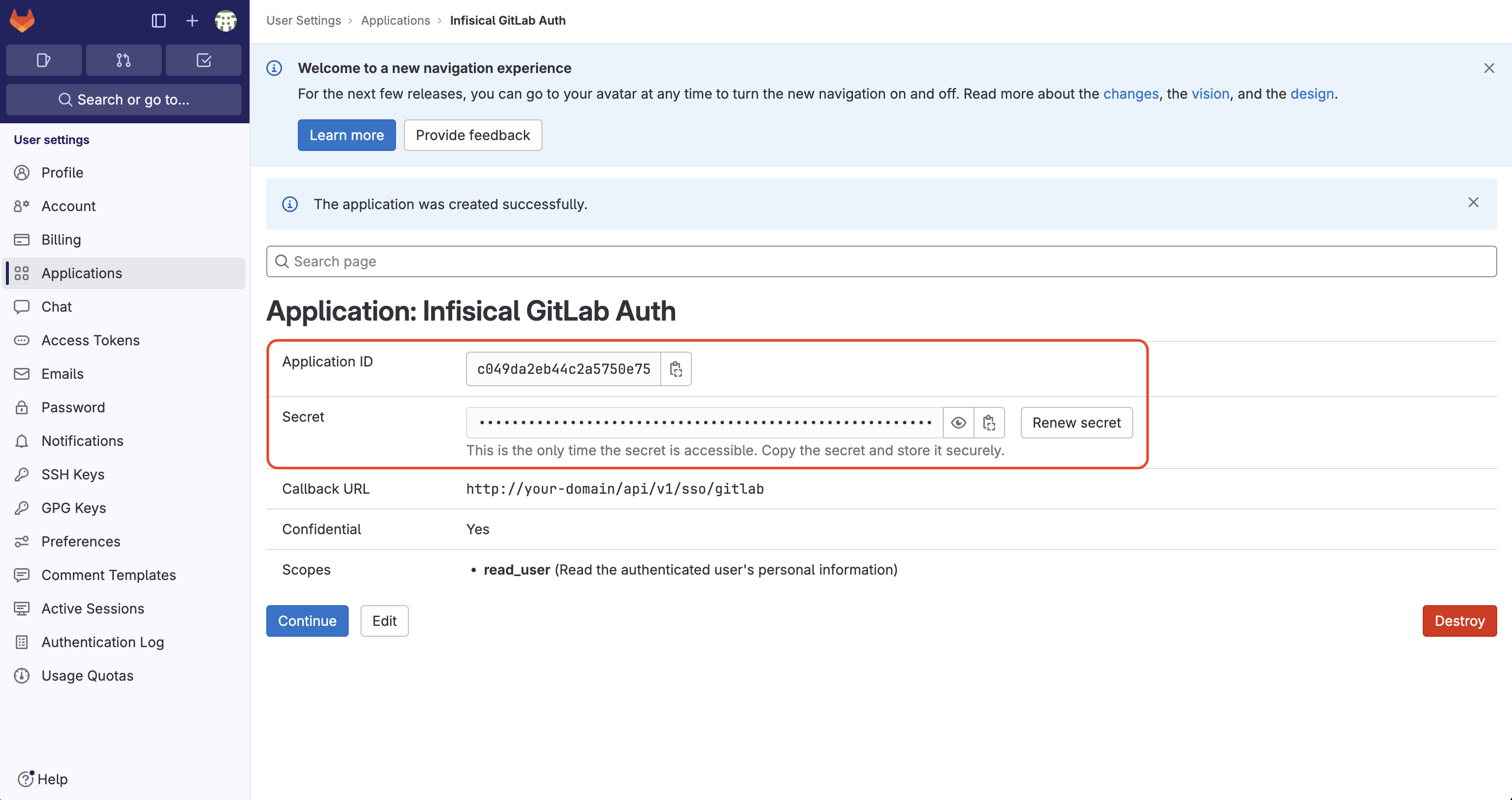
Back in your Infisical instance, make sure to set the following environment variables:
* `CLIENT_ID_GITLAB_LOGIN`: The **Client ID** of your GitLab application.
* `CLIENT_SECRET_GITLAB_LOGIN`: The **Secret** of your GitLab application.
* (optional) `URL_GITLAB_LOGIN`: The URL of your self-hosted instance of GitLab where the OAuth application is registered. If no URL is passed in, this will default to `https://gitlab.com`.
* `AUTH_SECRET`: A secret key used for signing and verifying JWT. This can be a random 32-byte base64 string generated with `openssl rand -base64 32`.
* `SITE_URL`: The URL of your self-hosted instance of Infisical - should be an absolute URL including the protocol (e.g. [https://app.infisical.com](https://app.infisical.com))
Once added, restart your Infisical instance and log in with GitLab.
## FAQ
It is likely that you have misconfigured your self-hosted instance of Infisical. You should:
* Check that you have set the `CLIENT_ID_GITLAB_LOGIN`, `CLIENT_SECRET_GITLAB_LOGIN`,
`AUTH_SECRET`, and `SITE_URL` environment variables.
* Check that the **Redirect URI** specified in GitLab matches the `SITE_URL` environment variable.
For example, if the former is `https://app.infisical.com/api/v1/sso/gitlab` then the latter should be `https://app.infisical.com`.
# Google SSO
Learn how to configure Google SSO for Infisical.
Using Google SSO on a self-hosted instance of Infisical requires configuring an OAuth2 application in GCP and registering your instance with it.
Navigate to your project API & Services > Credentials to create a new OAuth2 application.
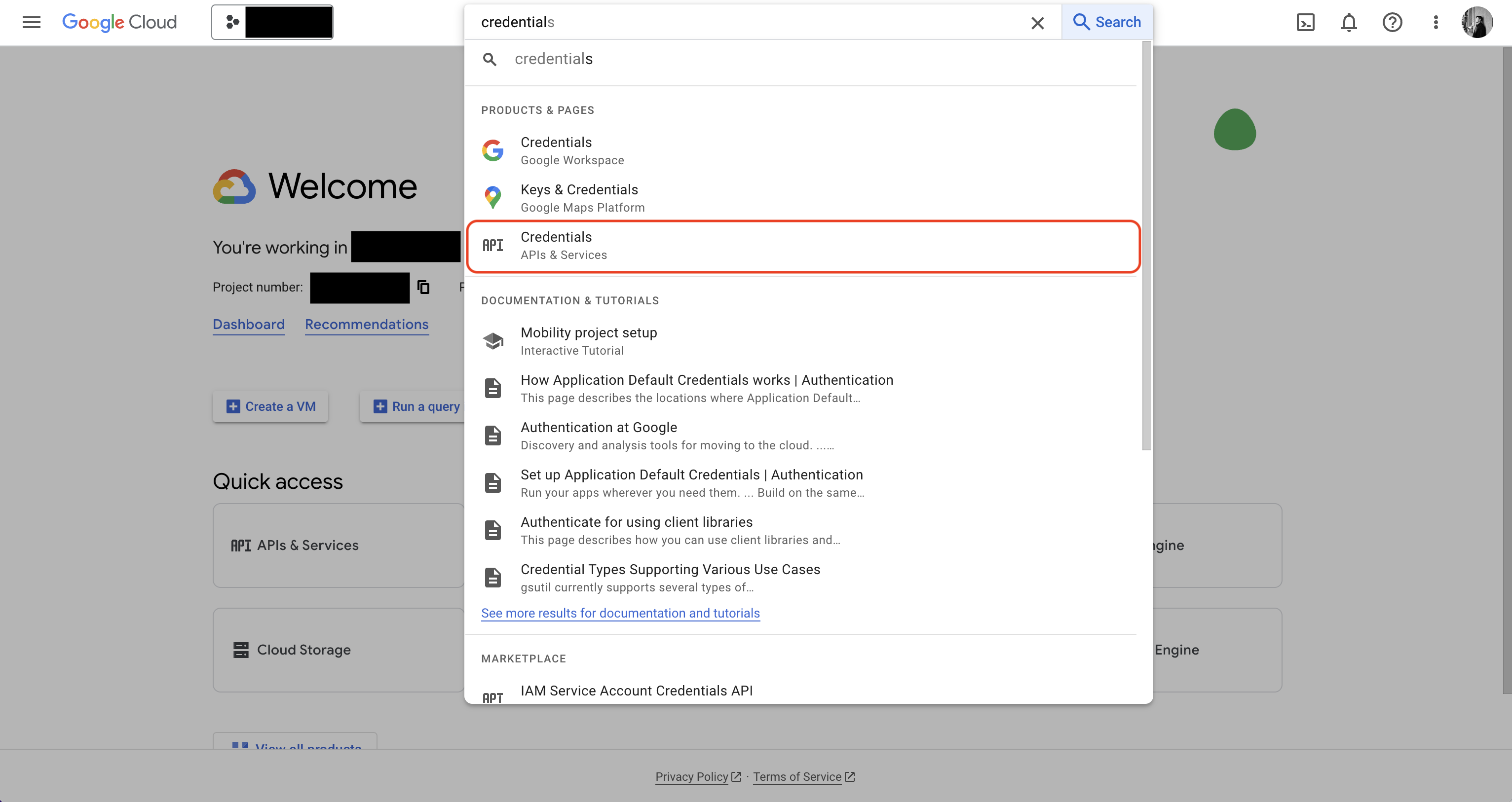
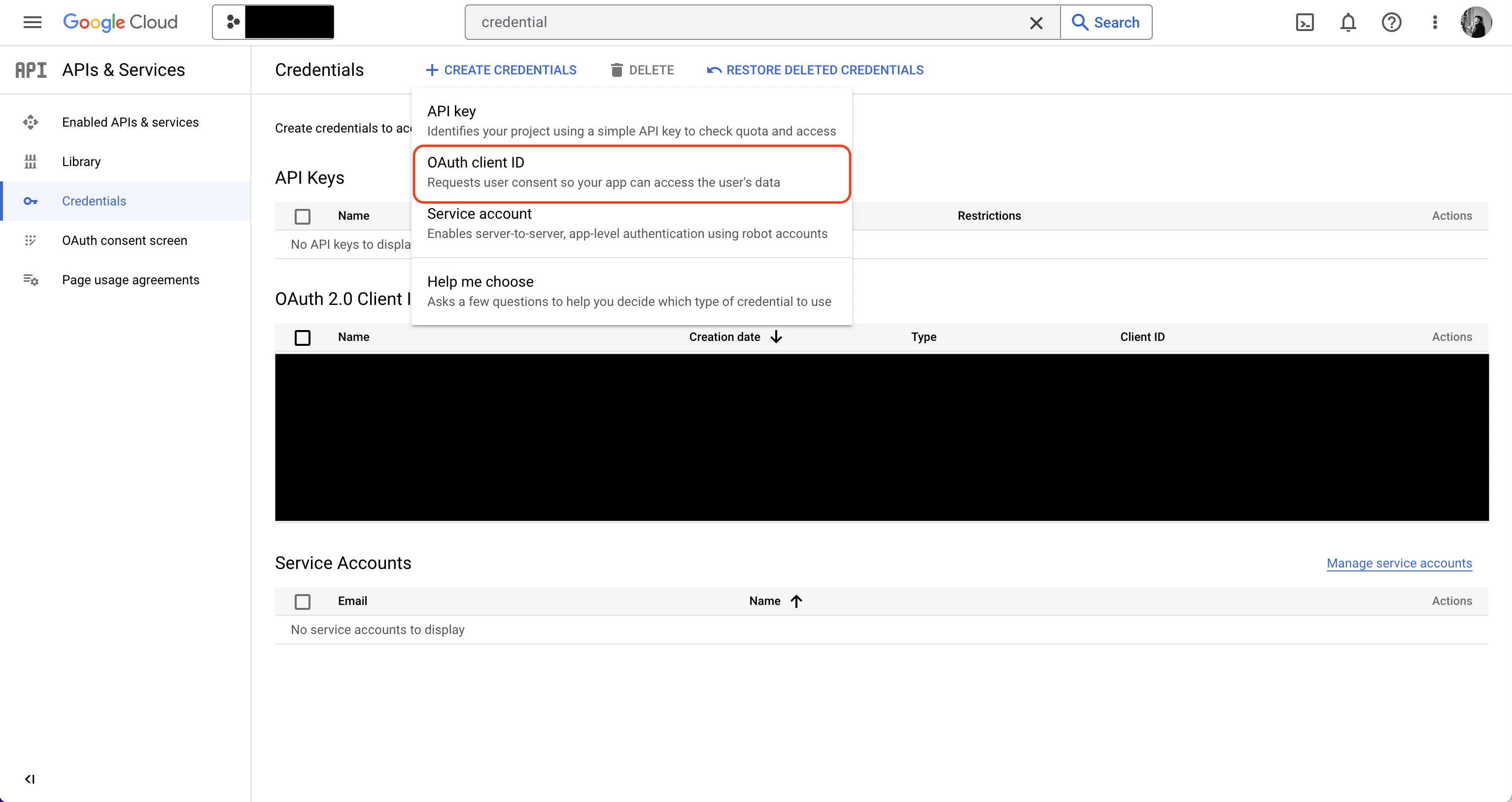
Create the application. As part of the form, add to **Authorized redirect URIs**: `https://your-domain.com/api/v1/sso/google`.
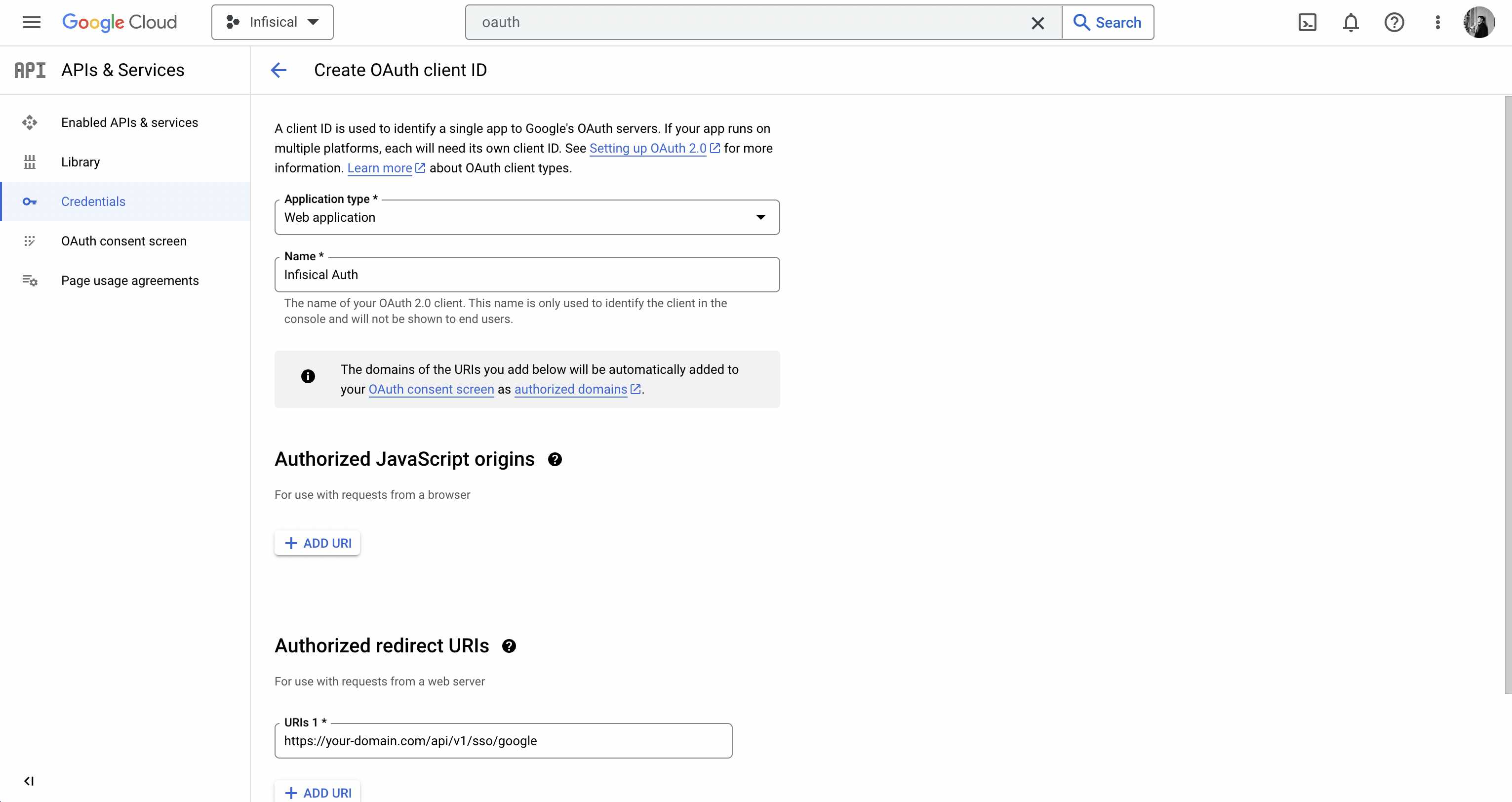
Obtain the **Client ID** and **Client Secret** for your GCP OAuth2 application.
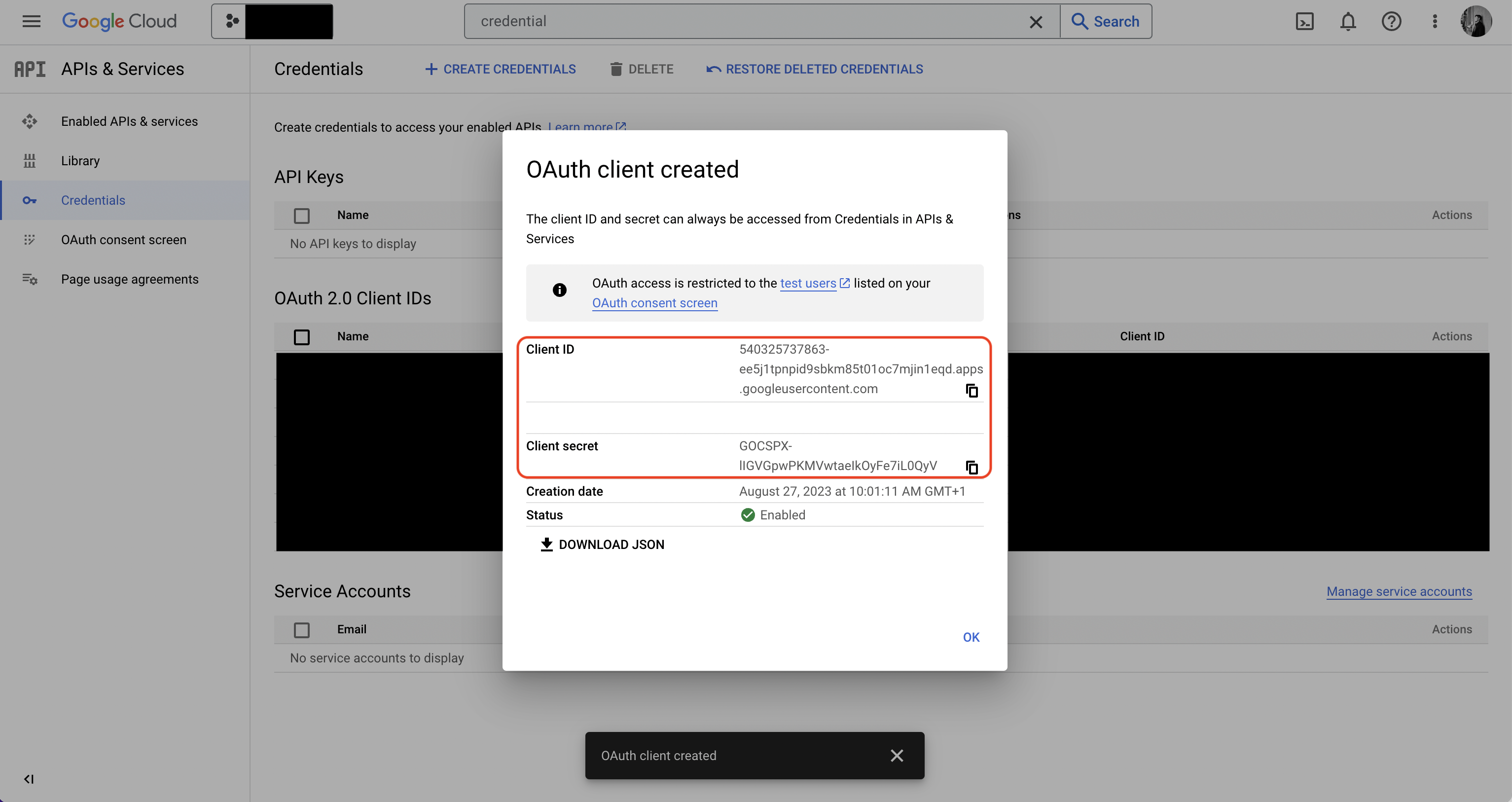
Back in your Infisical instance, make sure to set the following environment variables:
* `CLIENT_ID_GOOGLE_LOGIN`: The **Client ID** of your GCP OAuth2 application.
* `CLIENT_SECRET_GOOGLE_LOGIN`: The **Client Secret** of your GCP OAuth2 application.
* `AUTH_SECRET`: A secret key used for signing and verifying JWT. This can be a random 32-byte base64 string generated with `openssl rand -base64 32`.
* `SITE_URL`: The URL of your self-hosted instance of Infisical - should be an absolute URL including the protocol (e.g. [https://app.infisical.com](https://app.infisical.com))
Once added, restart your Infisical instance and log in with Google
## FAQ
It is likely that you have misconfigured your self-hosted instance of Infisical. You should:
* Check that you have set the `CLIENT_ID_GOOGLE_LOGIN`, `CLIENT_SECRET_GOOGLE_LOGIN`,
`AUTH_SECRET`, and `SITE_URL` environment variables.
* Check that the **Authorized redirect URI** specified in GCP matches the `SITE_URL` environment variable.
For example, if the former is `https://app.infisical.com/api/v1/sso/google` then the latter should be `https://app.infisical.com`.
# Google SAML
Learn how to configure Google SAML for Infisical SSO.
Google SAML SSO feature is a paid feature. If you're using Infisical Cloud,
then it is available under the **Pro Tier**. If you're self-hosting Infisical,
then you should contact [sales@infisical.com](mailto:sales@infisical.com) to purchase an enterprise license
to use it.
In Infisical, head to your Organization Settings > Authentication > SAML SSO Configuration and select **Set up SAML SSO**.
Next, note the **ACS URL** and **SP Entity ID** to use when configuring the Google SAML application.
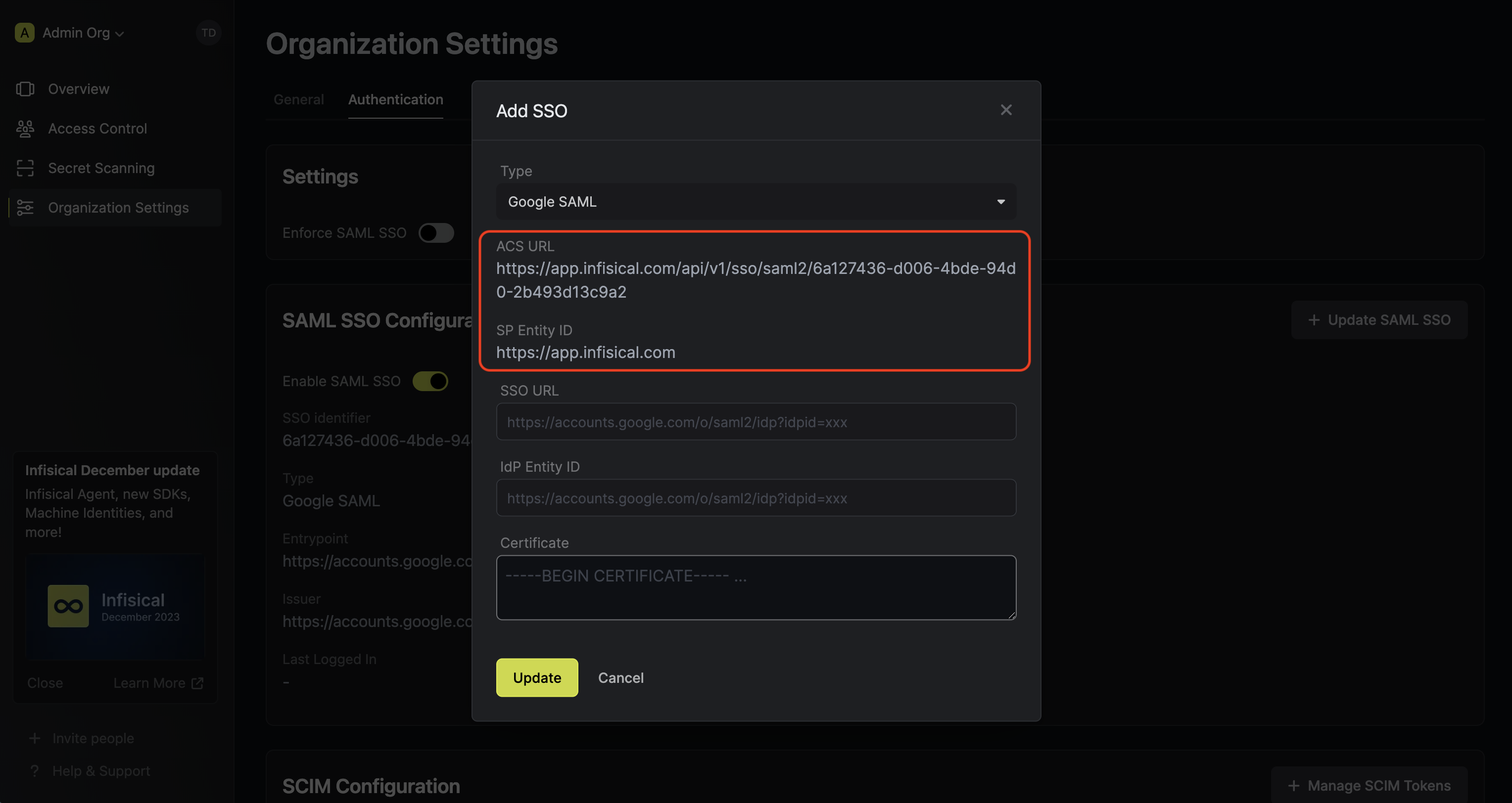
2.1. In your [Google Admin console](https://support.google.com/a/answer/182076), head to Menu > Apps > Web and mobile apps and
create a **custom SAML app**.
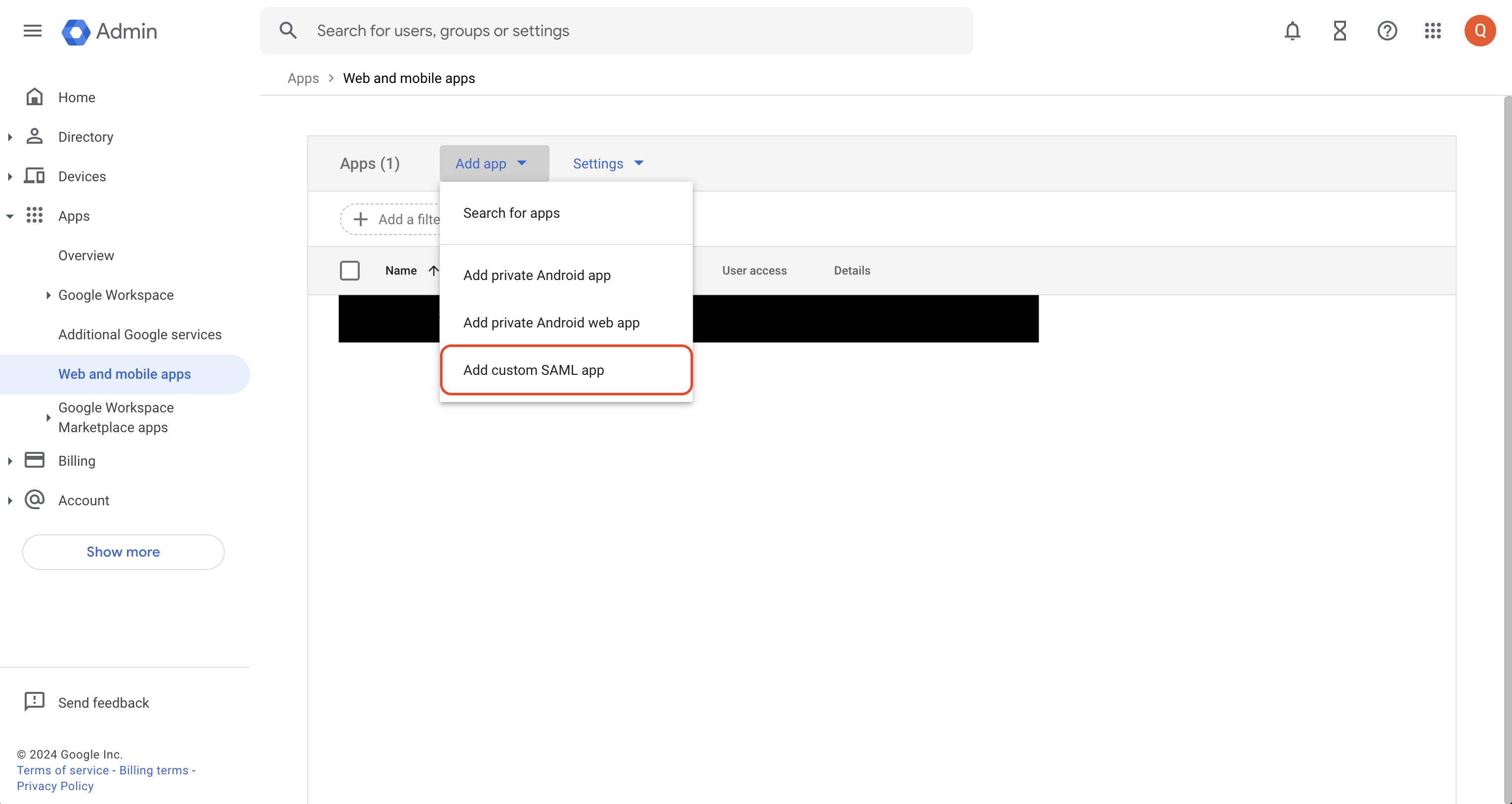
2.2. In the **App details** tab, give the application a unique name like Infisical.
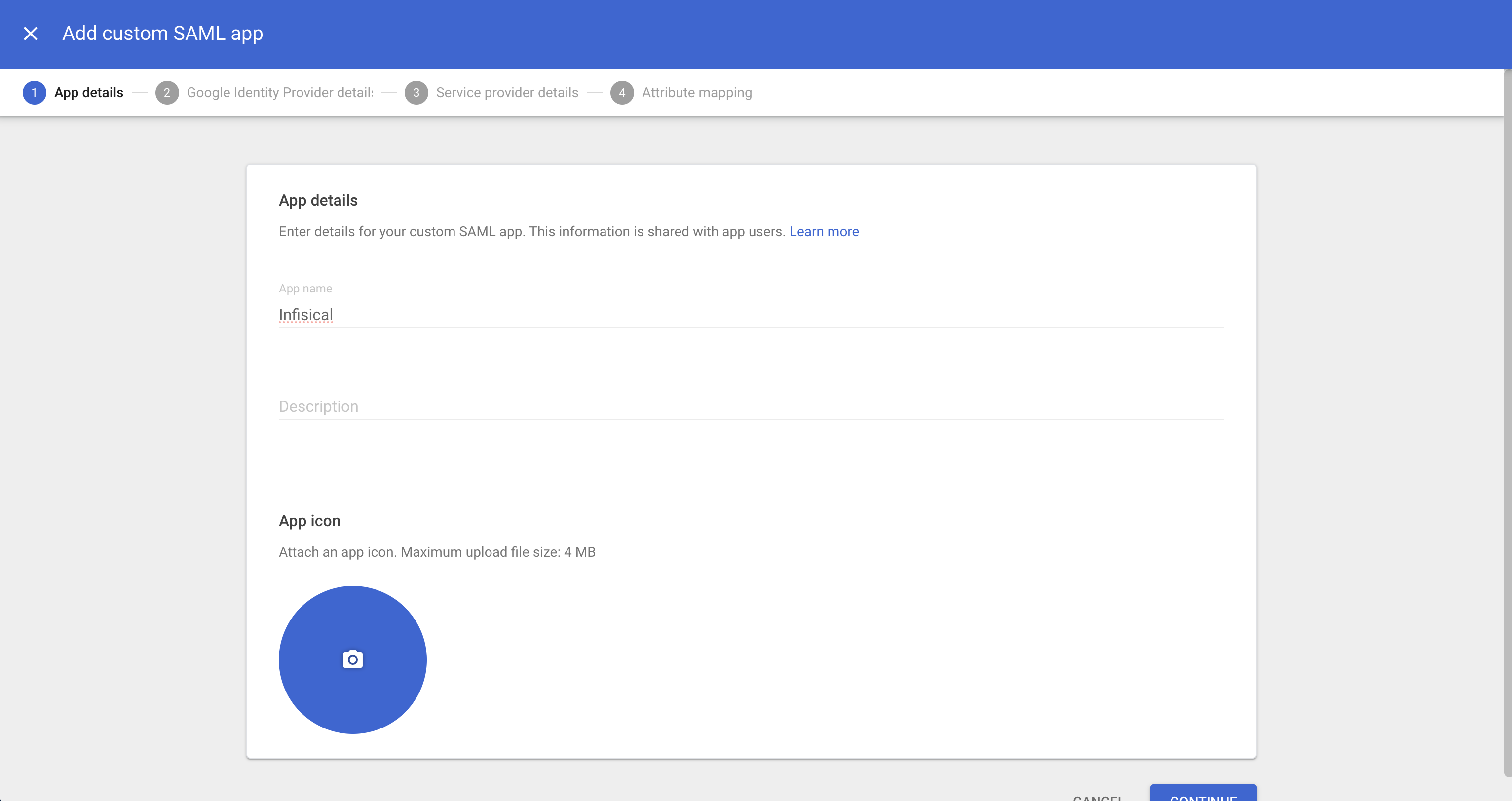
2.3. In the **Google Identity Provider details** tab, copy the **SSO URL**, **Entity ID** and **Certificate**.
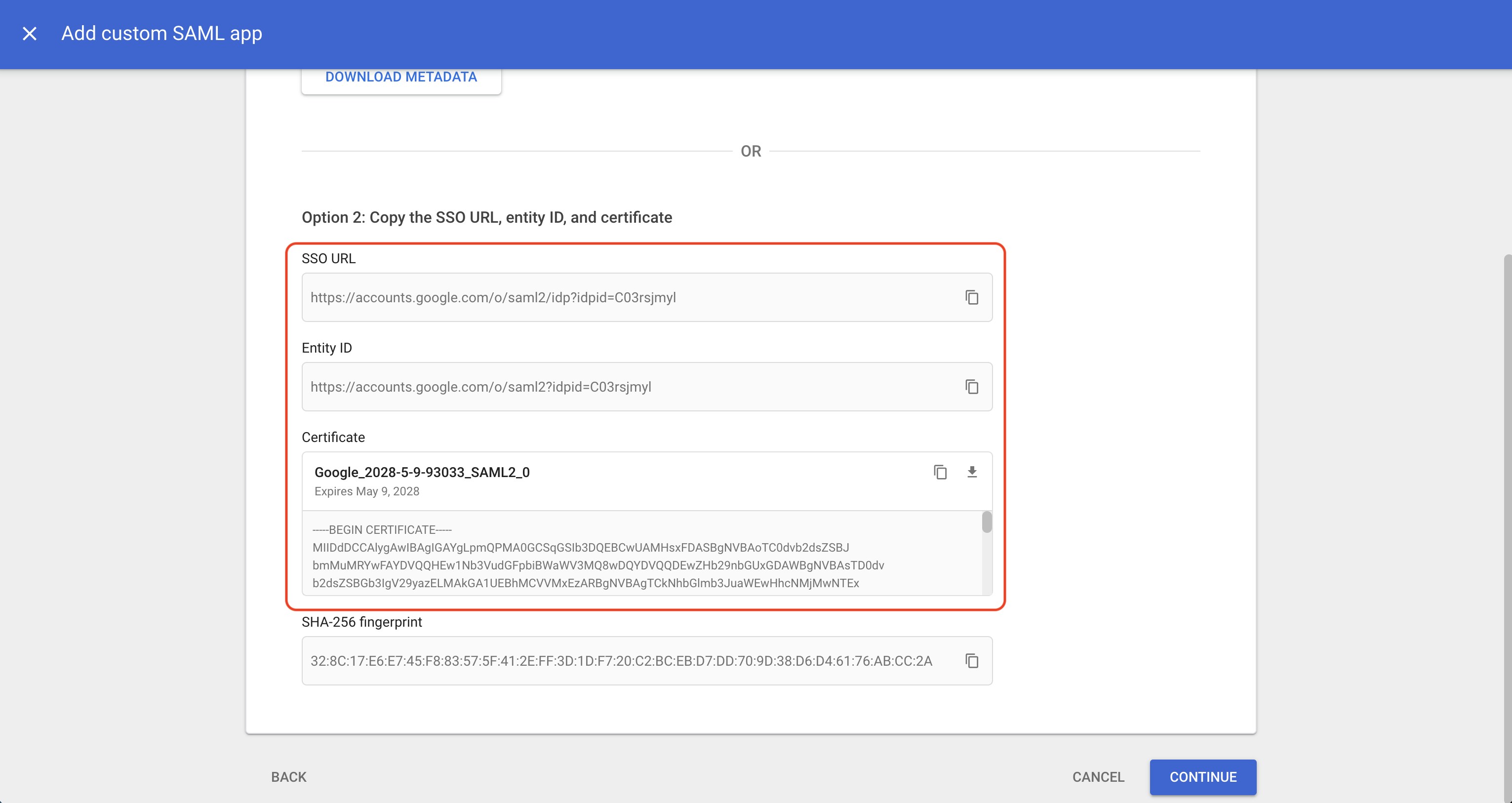
2.4. Back in Infisical, set **SSO URL** and **Certificate** to the corresponding items from step 2.3.
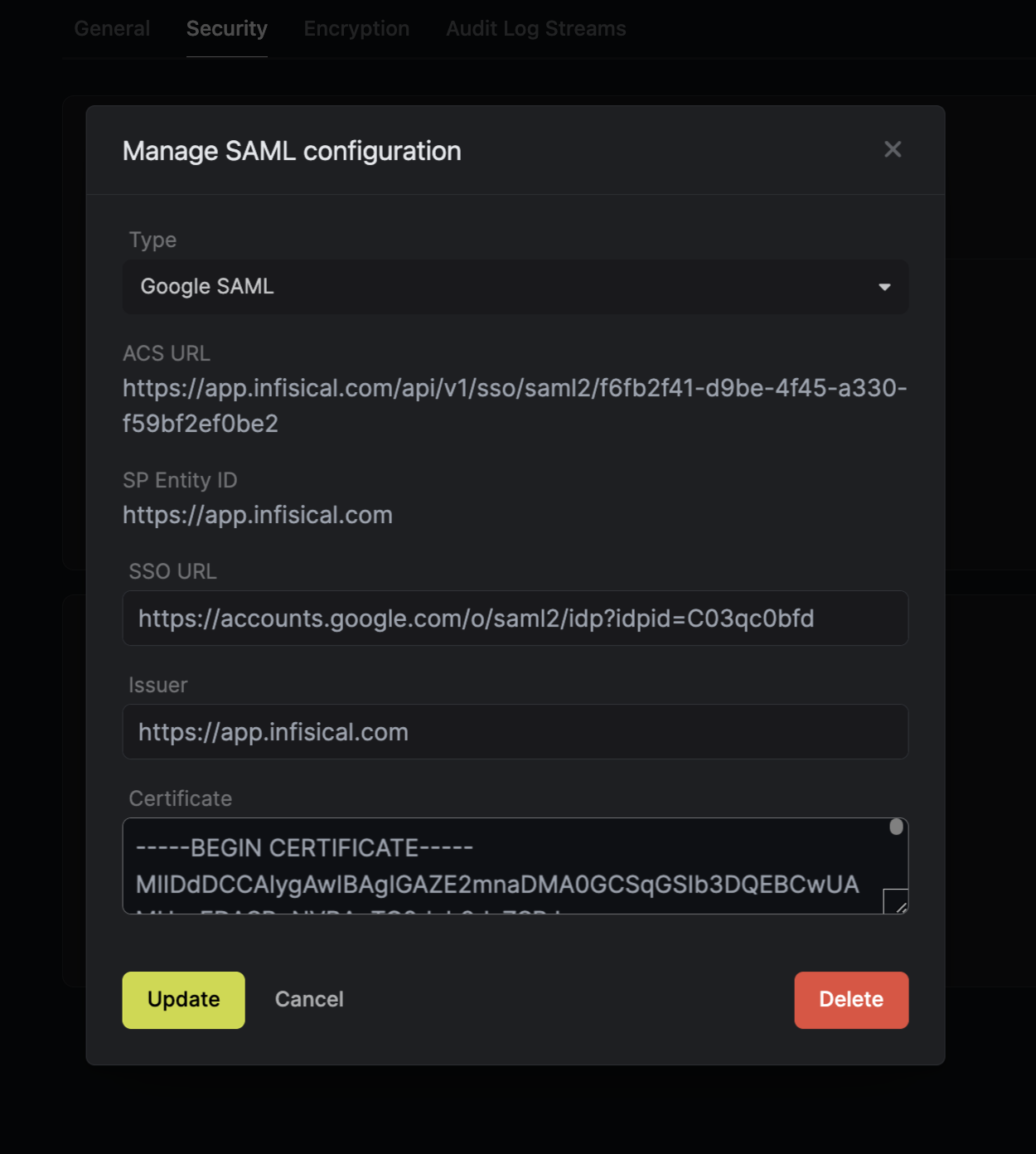
2.5. Back in the Google Admin console, in the **Service provider details** tab, set the **ACS URL** and **Entity ID** to the corresponding items from step 1.
Also, check the **Signed response** checkbox.
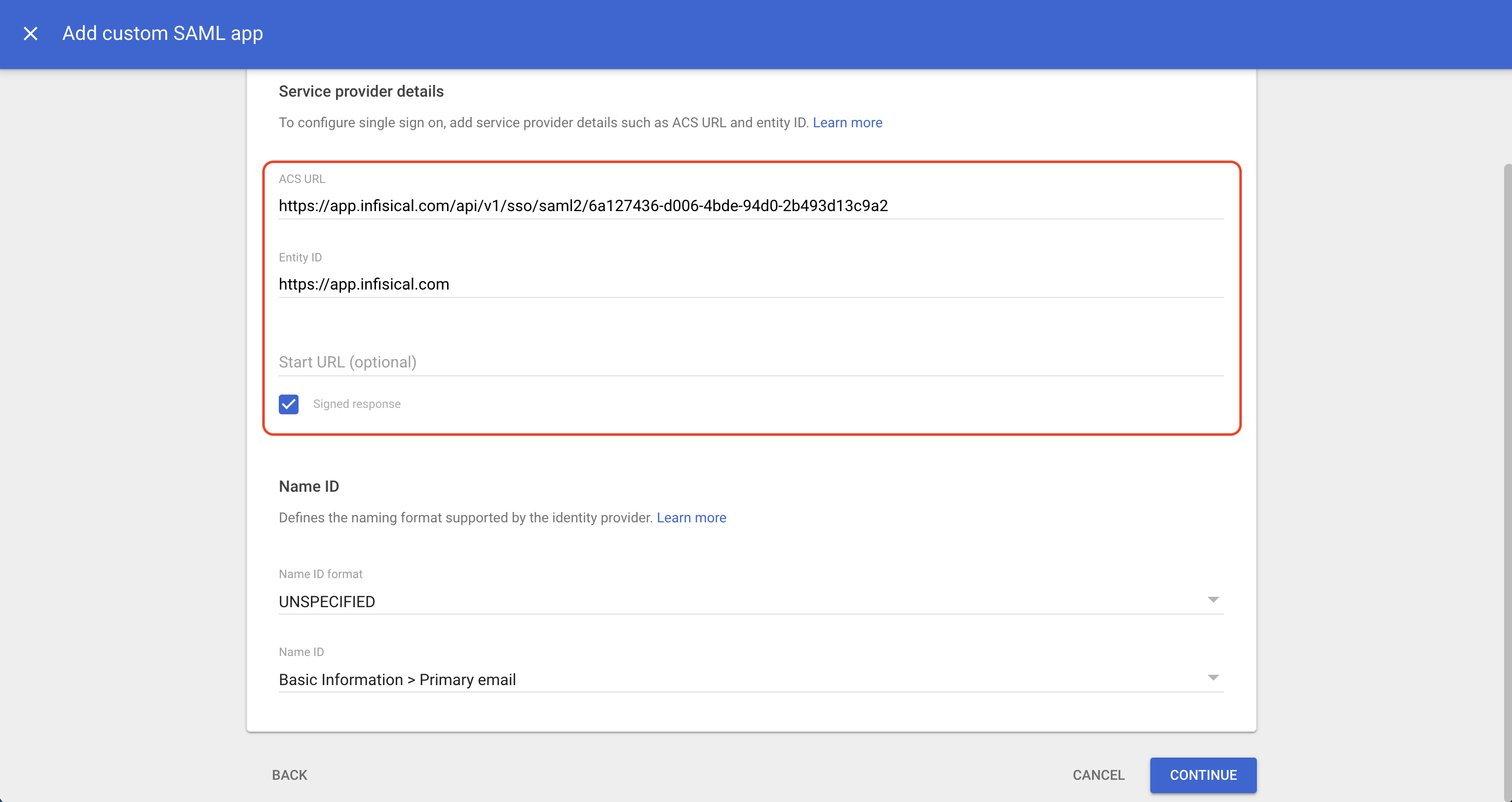
2.6. In the **Attribute mapping** tab, configure the following map:
* **First name** -> **firstName**
* **Last name** -> **lastName**
* **Primary email** -> **email**
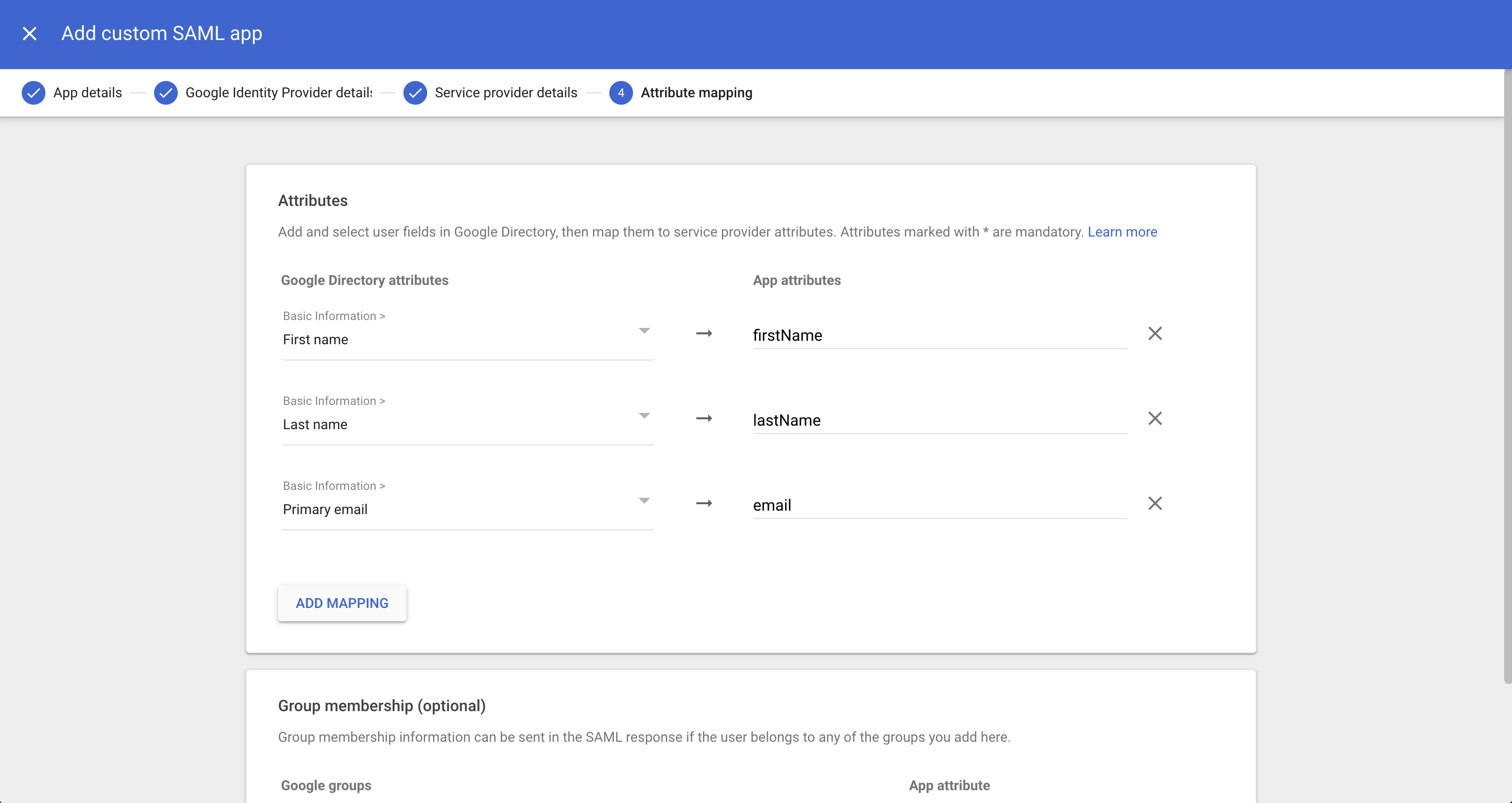
Click **Finish**.
Back in your [Google Admin console](https://support.google.com/a/answer/182076), head to Menu > Apps > Web and mobile apps > your SAML app
and press on **User access**.
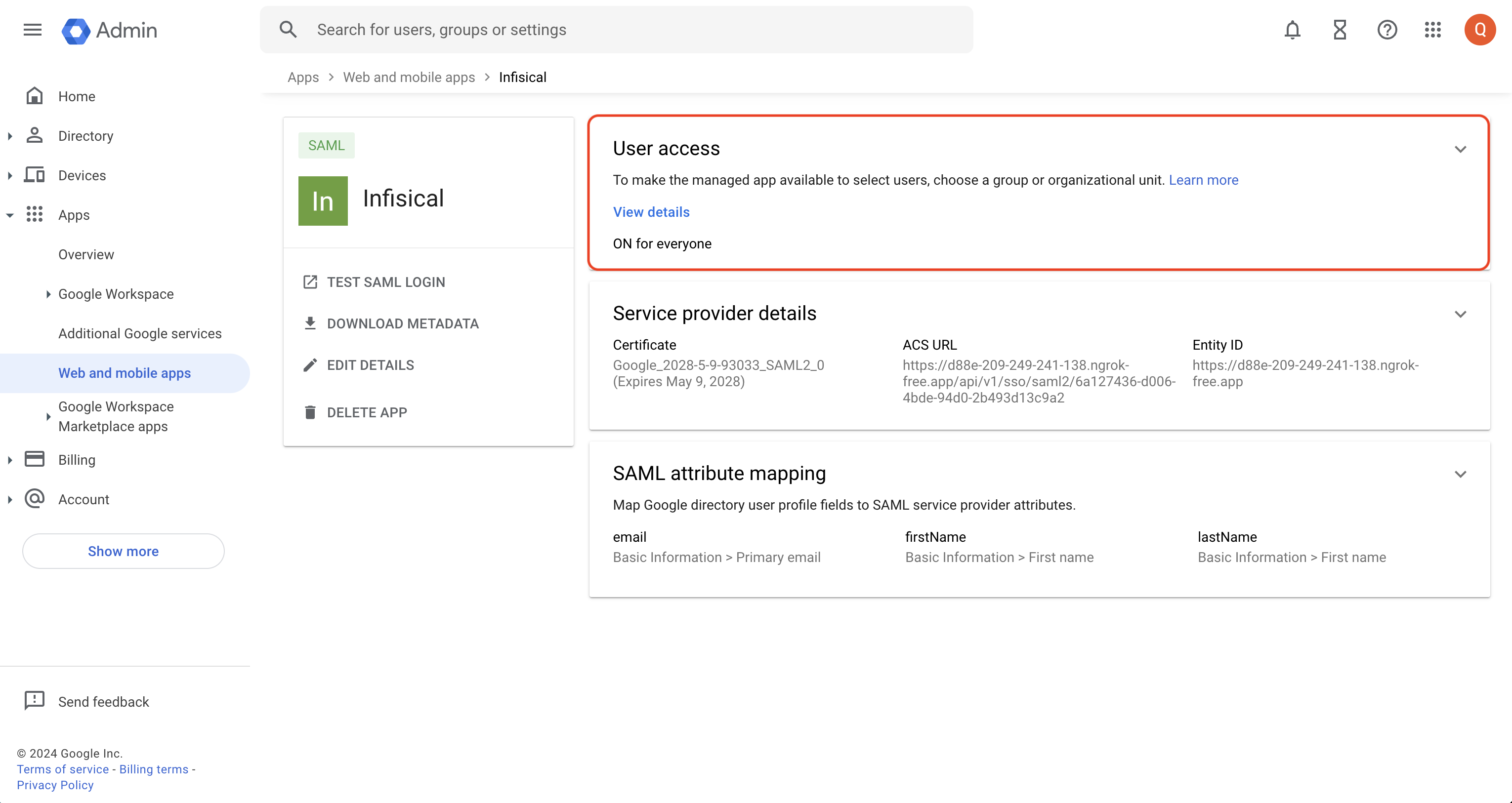
To assign everyone in your organization to the application, click **On for everyone** or **Off for everyone** and then click **Save**.
You can also assign an organizational unit or set of users to an application; you can learn more about that [here](https://support.google.com/a/answer/6087519?hl=en#add_custom_saml\&turn_on\&verify_sso&\&zippy=%2Cstep-add-the-custom-saml-app%2Cstep-turn-on-your-saml-app%2Cstep-verify-that-sso-is-working-with-your-custom-app).
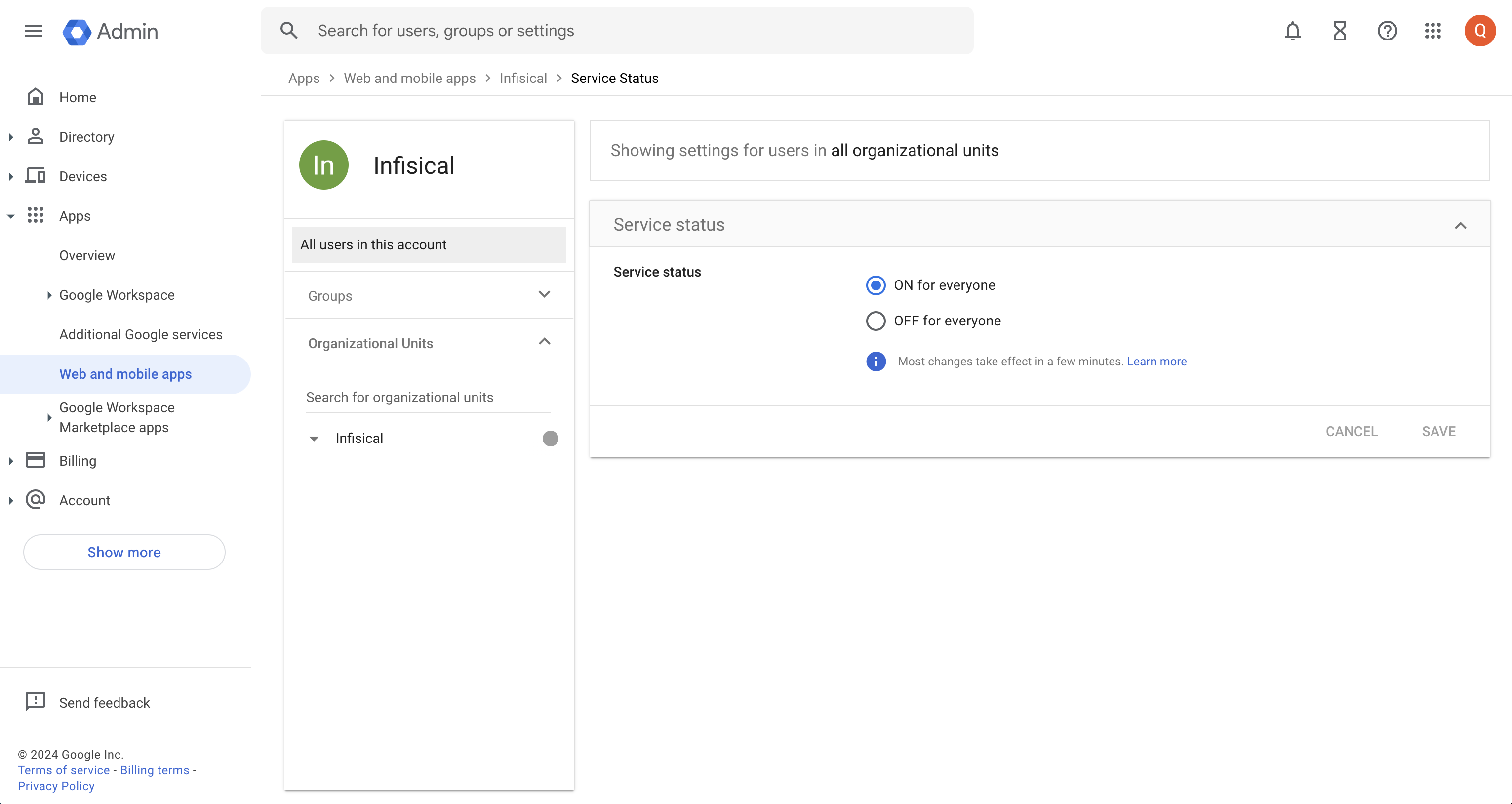
Enabling SAML SSO allows members in your organization to log into Infisical via Google Workspace.
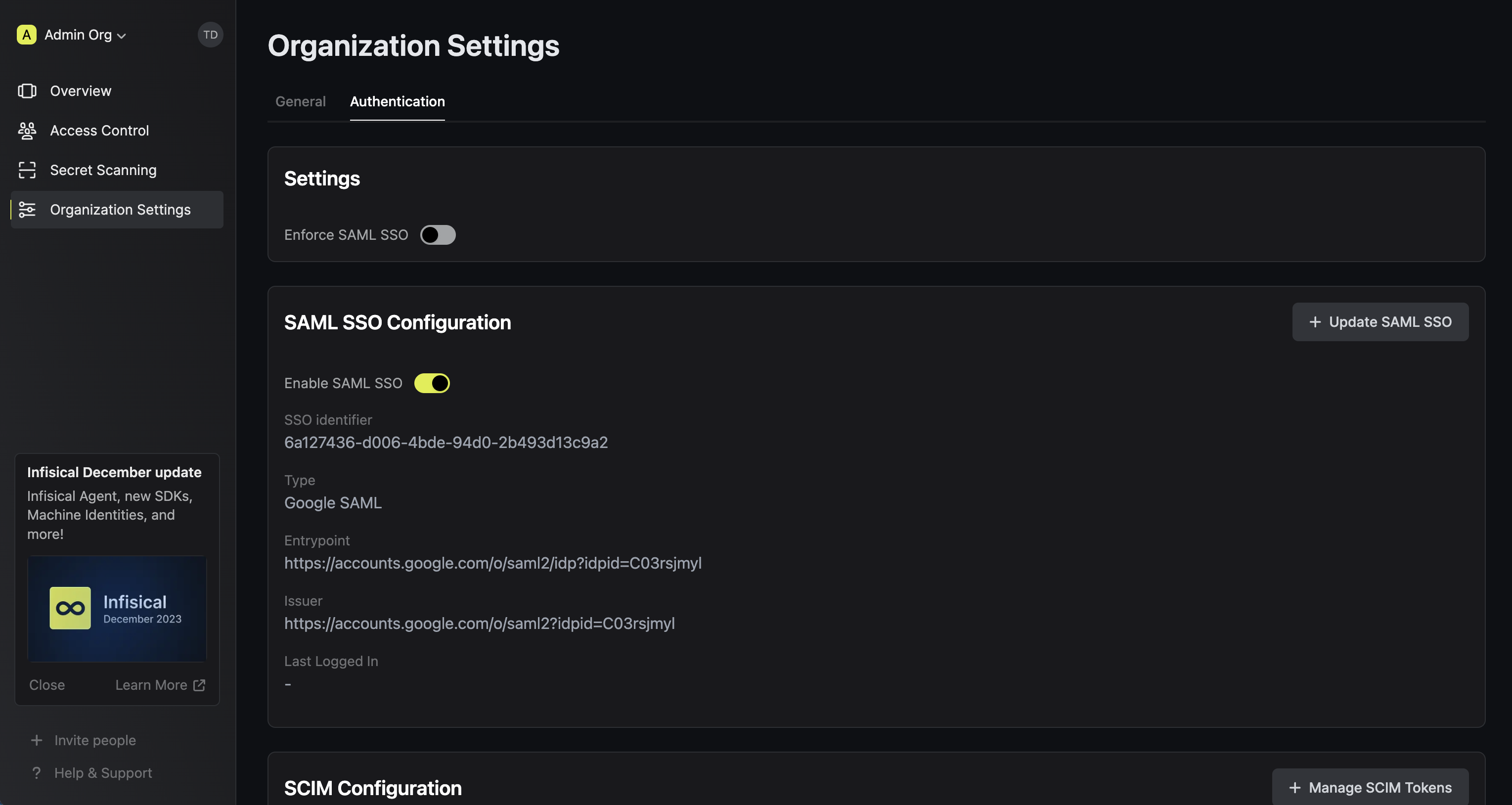
Enforcing SAML SSO ensures that members in your organization can only access Infisical
by logging into the organization via Google.
To enforce SAML SSO, you're required to test out the SAML connection by successfully authenticating at least one Google user with Infisical;
Once you've completed this requirement, you can toggle the **Enforce SAML SSO** button to enforce SAML SSO.
We recommend ensuring that your account is provisioned the application in Google
prior to enforcing SAML SSO to prevent any unintended issues.
If you are only using one organization on your Infisical instance, you can configure a default organization in the [Server Admin Console](../admin-panel/server-admin#default-organization) to expedite SAML login.
If you're configuring SAML SSO on a self-hosted instance of Infisical, make
sure to set the `AUTH_SECRET` and `SITE_URL` environment variable for it to
work:
* `AUTH_SECRET`: A secret key used for signing and verifying JWT. This
can be a random 32-byte base64 string generated with `openssl rand -base64
32`.
* `SITE_URL`: The absolute URL of your self-hosted instance of Infisical including the protocol (e.g. [https://app.infisical.com](https://app.infisical.com))
References:
* Google's guide to [set up your own custom SAML app](https://support.google.com/a/answer/6087519?hl=en#add_custom_saml\&turn_on\&verify_sso&\&zippy=%2Cstep-add-the-custom-saml-app%2Cstep-turn-on-your-saml-app%2Cstep-verify-that-sso-is-working-with-your-custom-app).
# JumpCloud SAML
Learn how to configure JumpCloud SAML for Infisical SSO.
JumpCloud SAML SSO is a paid feature.
If you're using Infisical Cloud, then it is available under the **Pro Tier**. If you're self-hosting Infisical,
then you should contact [sales@infisical.com](mailto:sales@infisical.com) to purchase an enterprise license to use it.
In Infisical, head to your Organization Settings > Authentication > SAML SSO Configuration and select **Set up SAML SSO**.
Next, copy the **ACS URL** and **SP Entity ID** to use when configuring the JumpCloud SAML application.
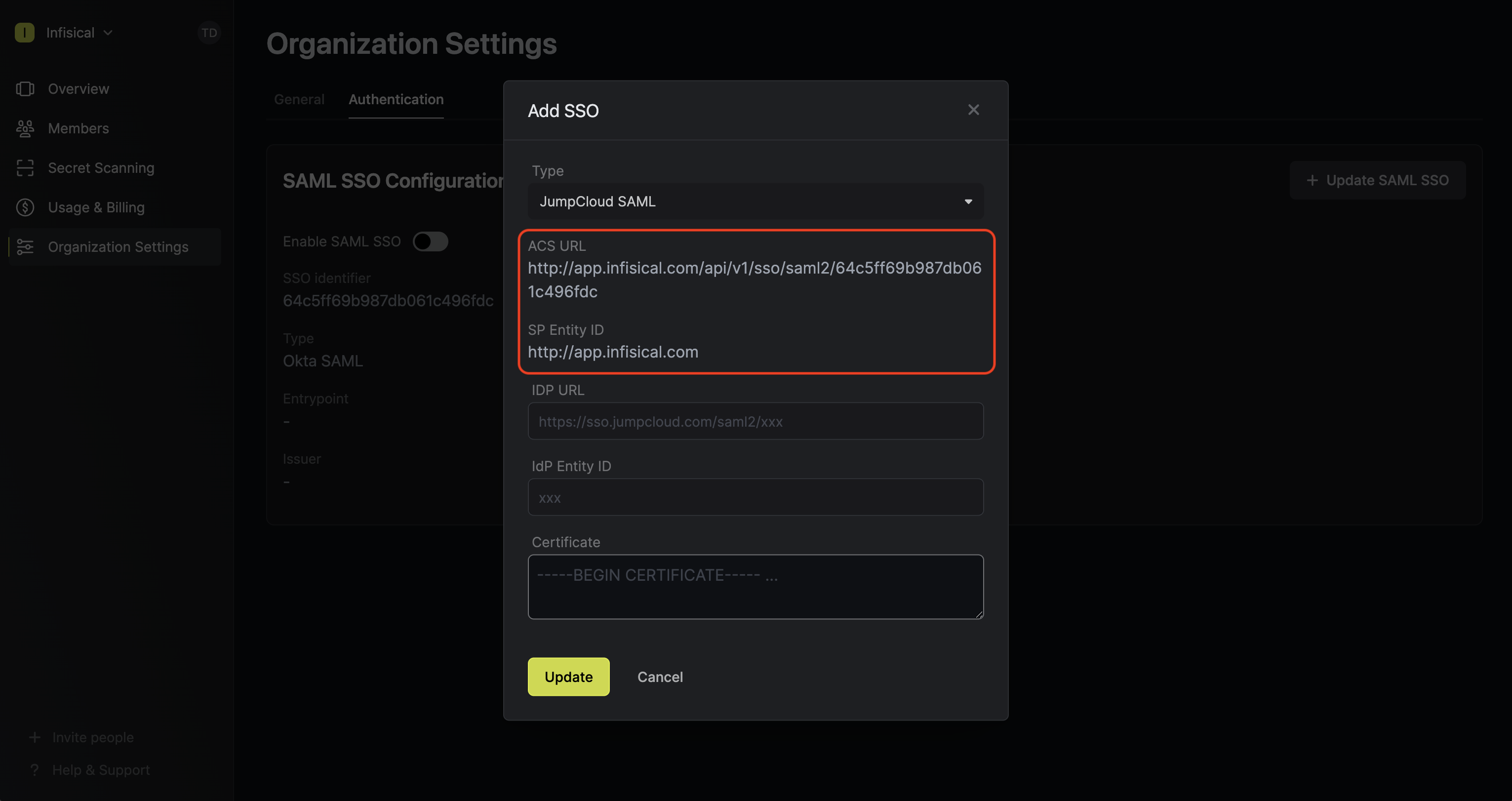
2.1. In the JumpCloud Admin Portal, navigate to User Authentication > SSO and create an application. If this is your first application, select **Get Started**; if not, select **+Add New Application**
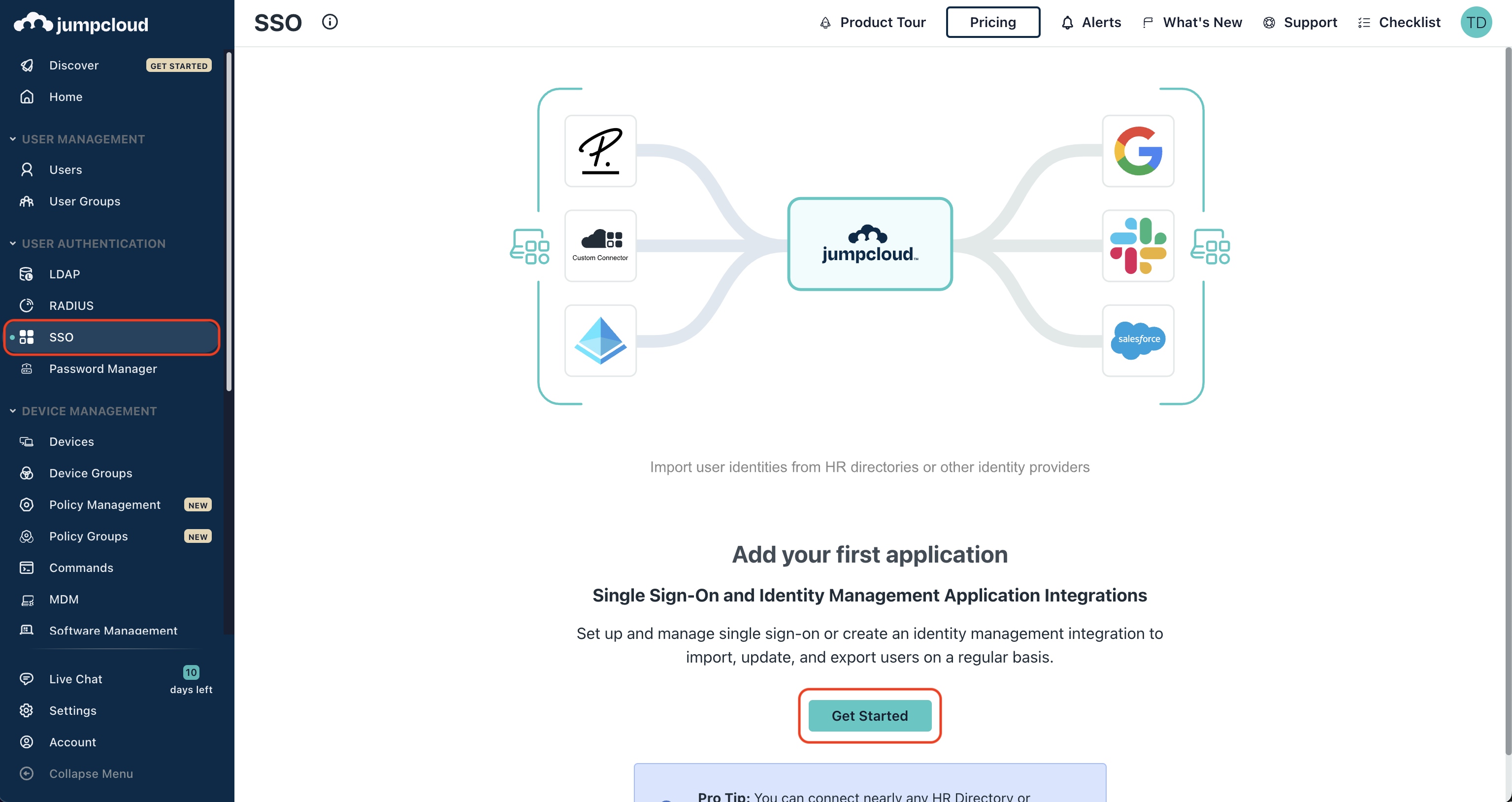
2.2. Next, select **Custom SAML App** to open up the **New SSO** dialog.
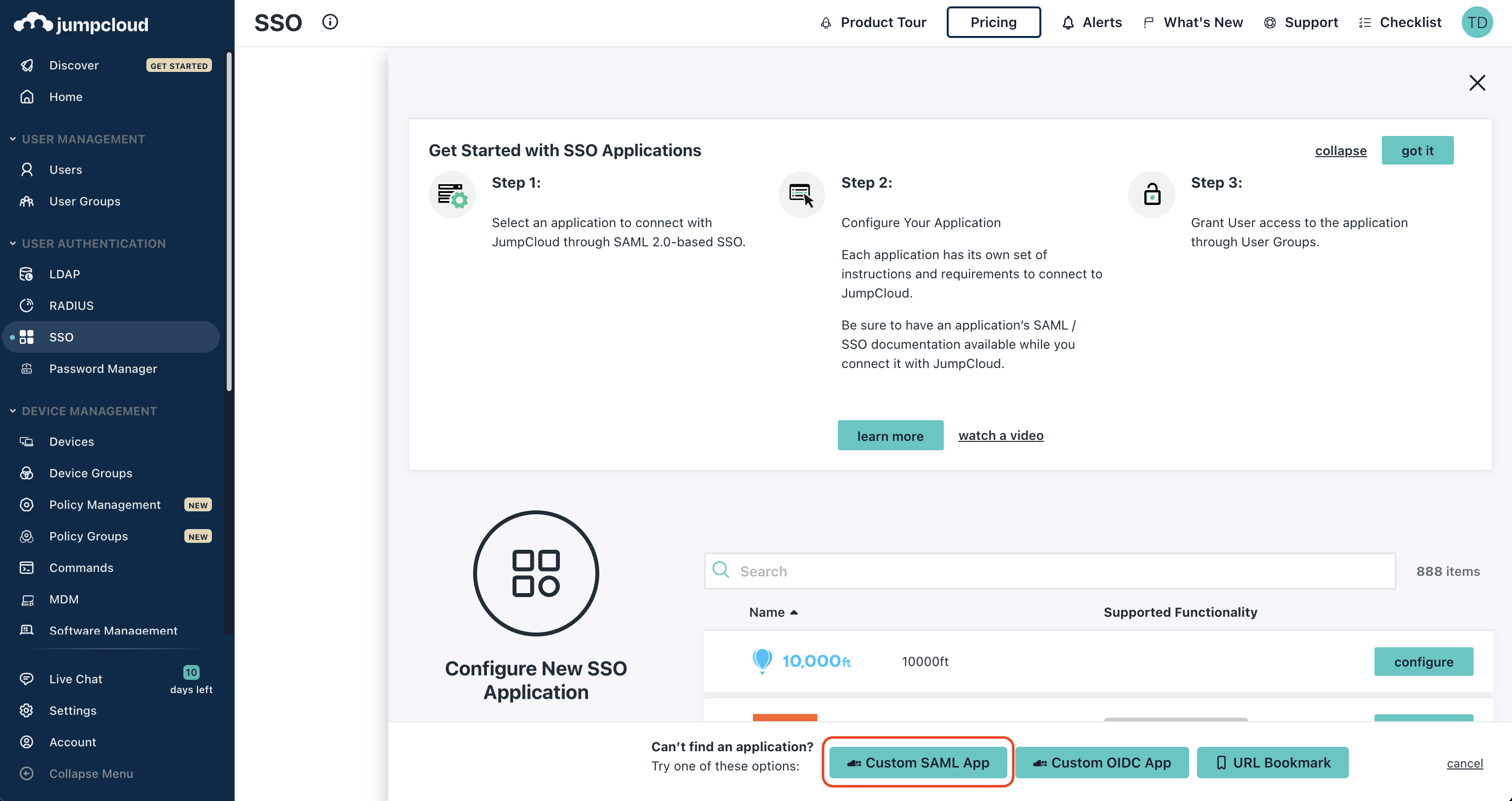
2.3. In the **General Info** tab, give the application a unique name like Infisical.
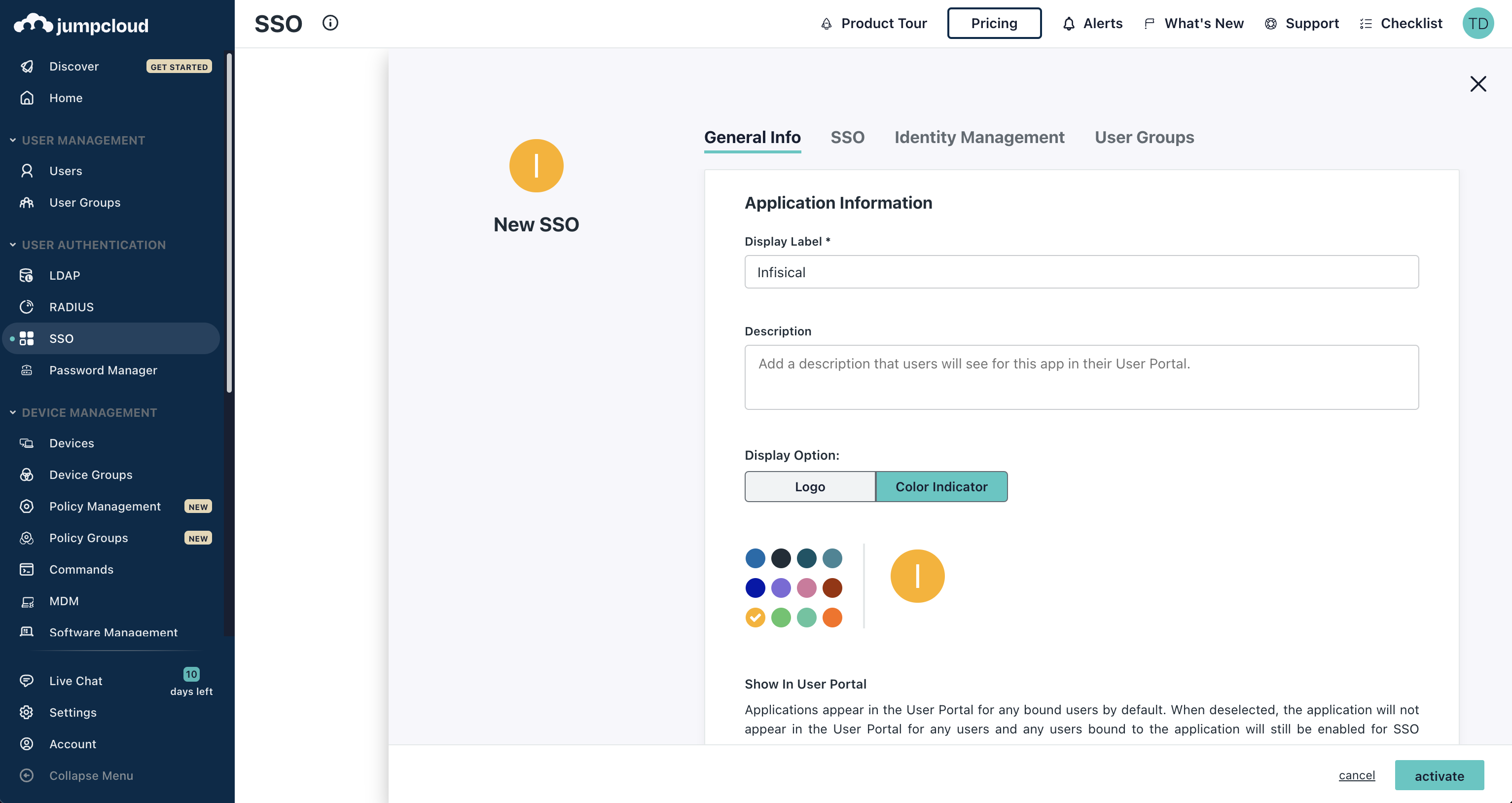
2.4. In the **SSO** tab, set the **SP Entity ID** and **ACS URL** from step 1; set the **IdP Entity ID** to the same value as the **SP Entity ID**.
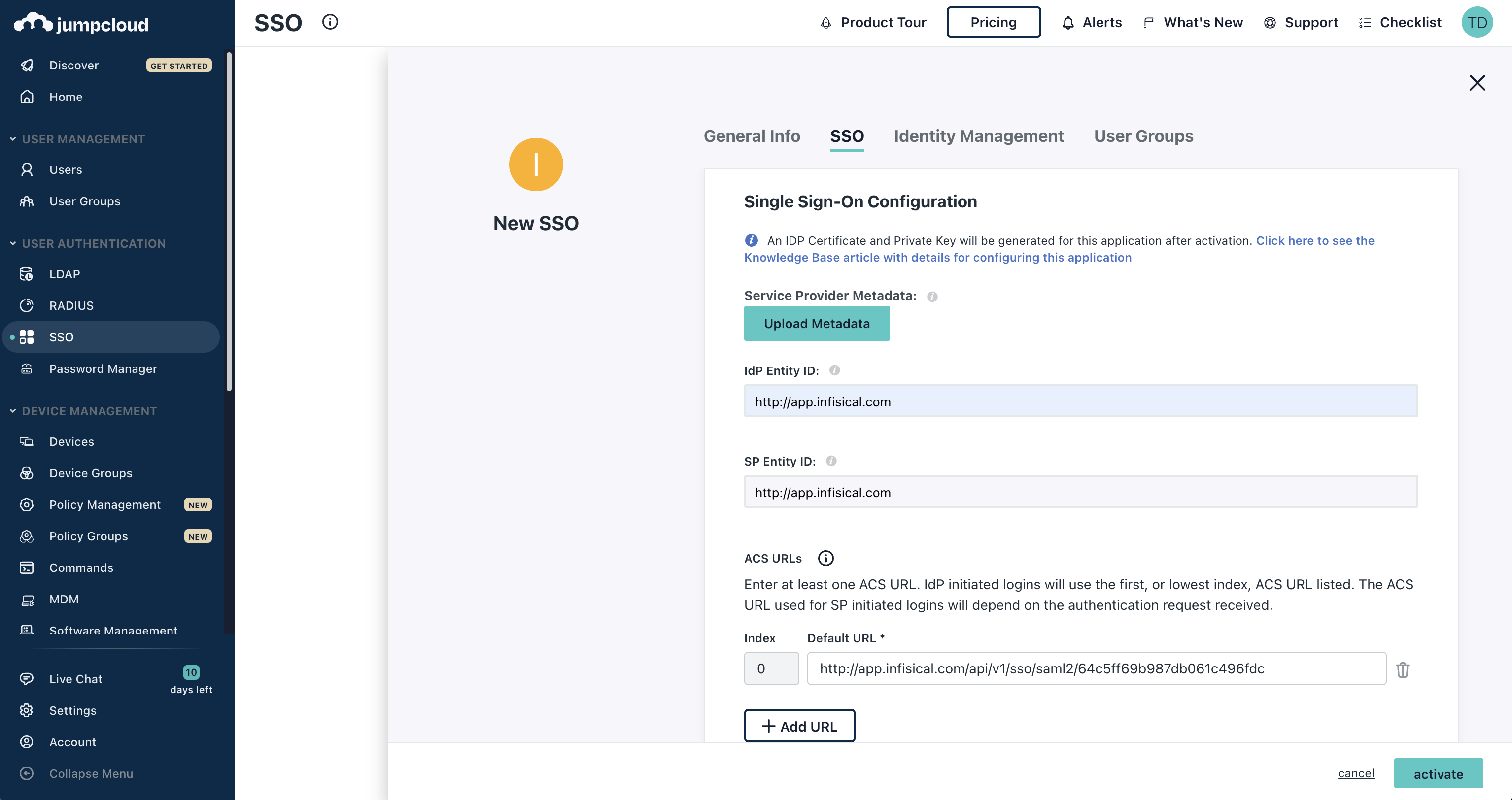
2.5. On the same tab, check the **Sign Assertion** checkbox and fill the **IDP URL** to something unique.
Copy the **IDP URL** to use when finishing configuring the JumpCloud SAML in Infisical.
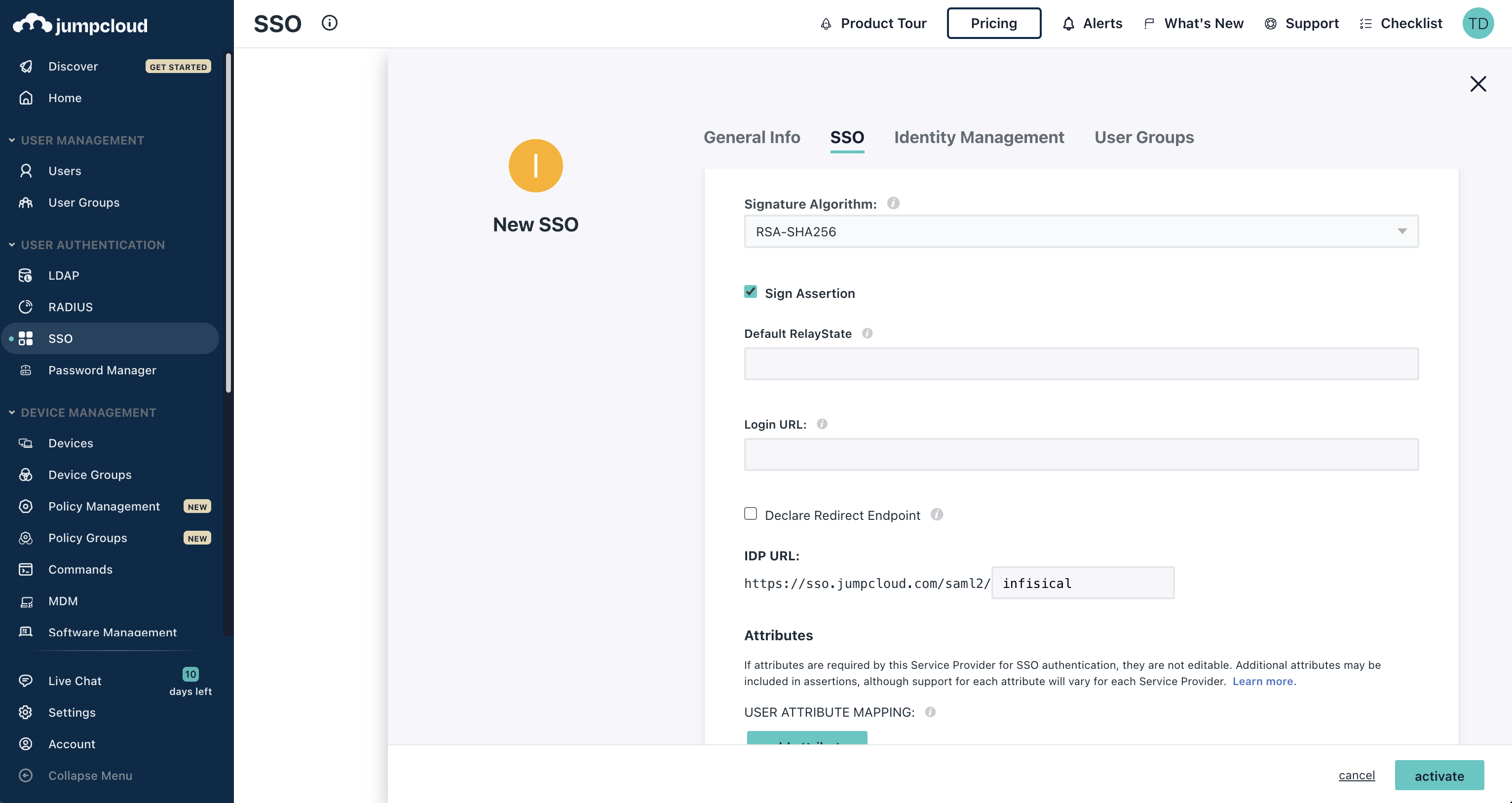
2.6. On the same tab, in the **Attributes** section, configure the following map:
* `email -> email`
* `firstName -> firstname`
* `lastName -> lastname`
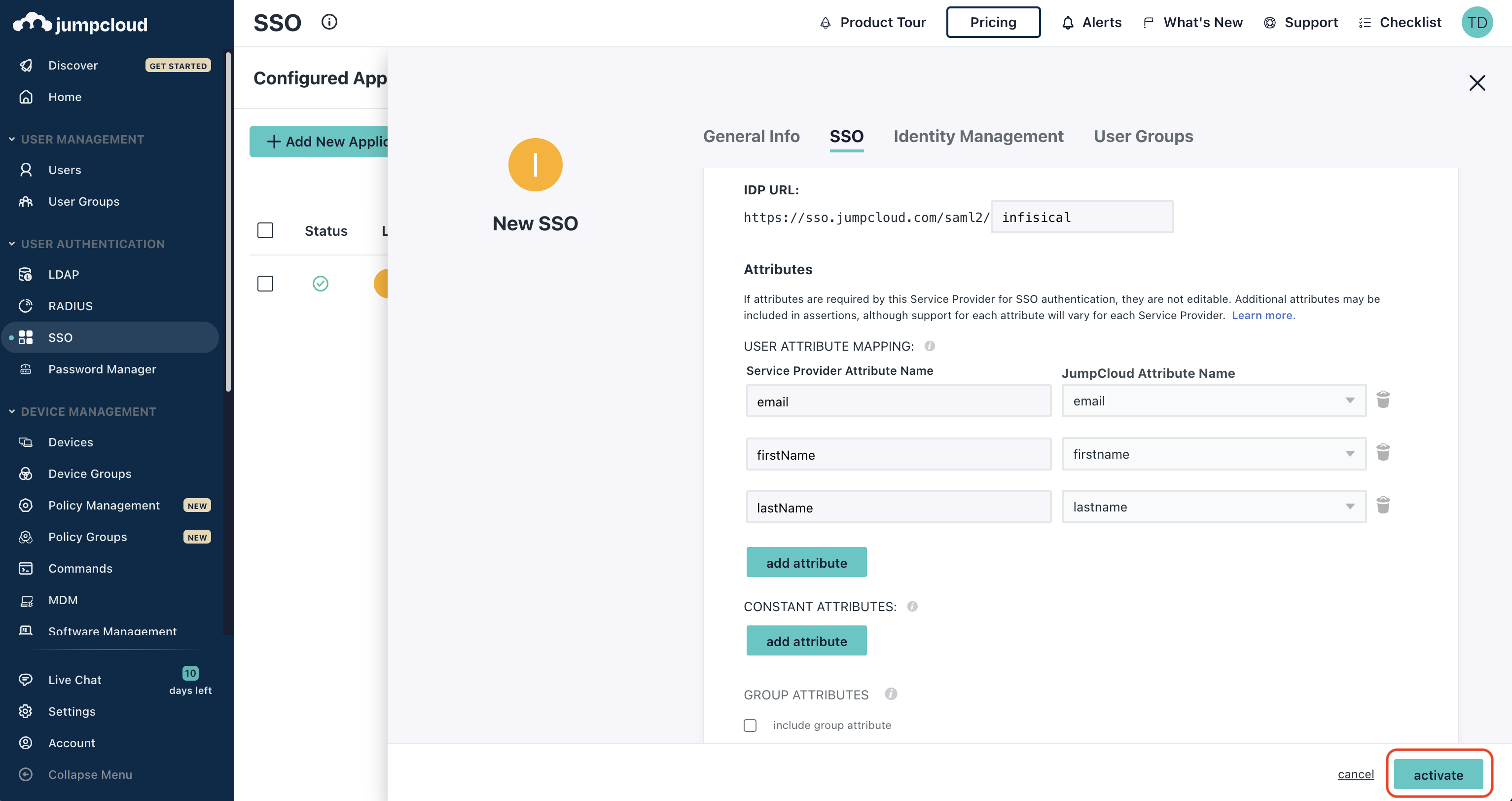
Finally press activate to create the SAML application.
2.7. Next, select the newly created SAML application and select **Download certificate** under the **IDP Certificate Valid** dropdown
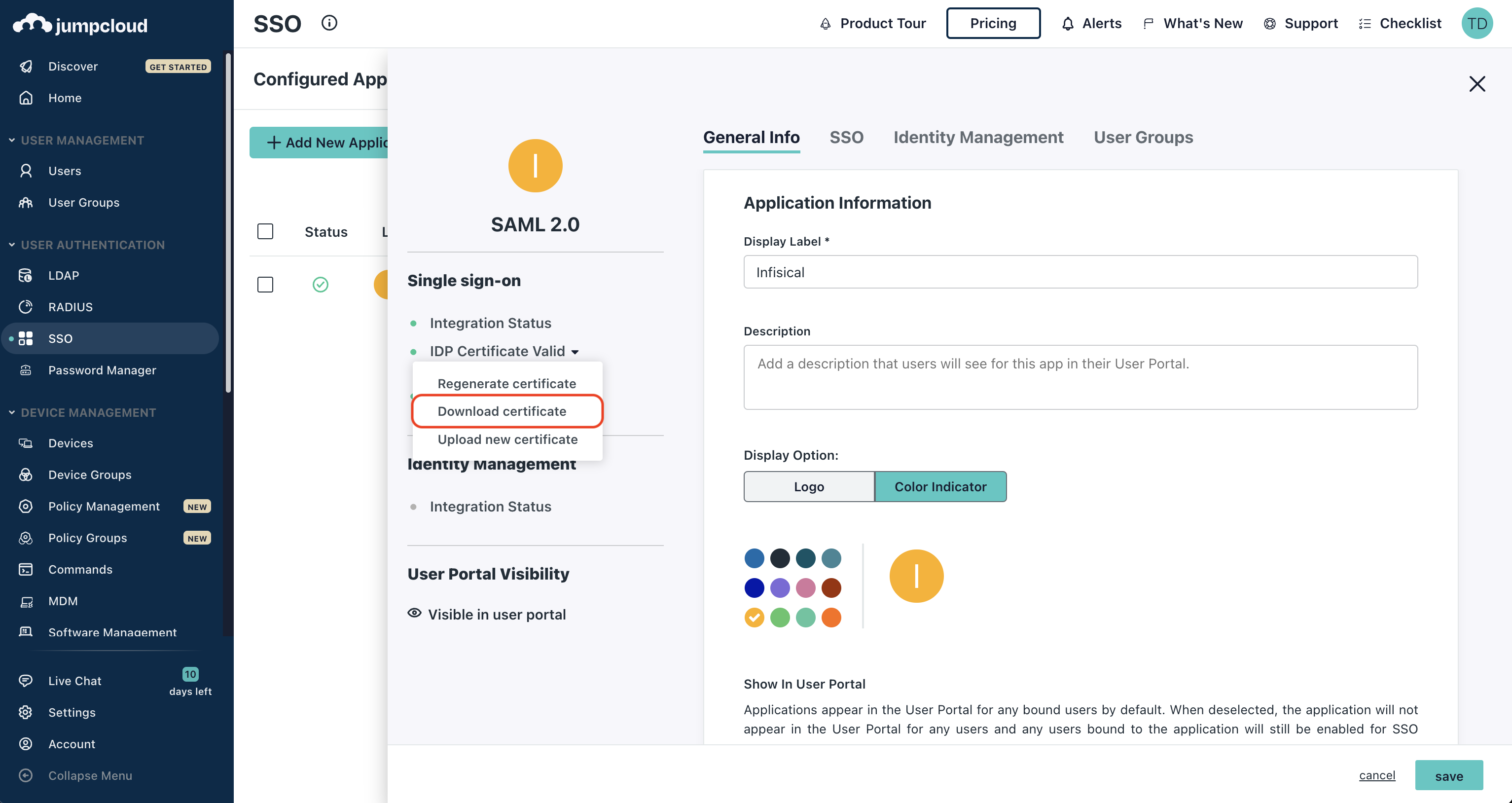
Back in Infisical, set the **IDP URL** from step 2.5 and the **IdP Entity ID** from step 2.4. Also, paste the certificate from the previous step.
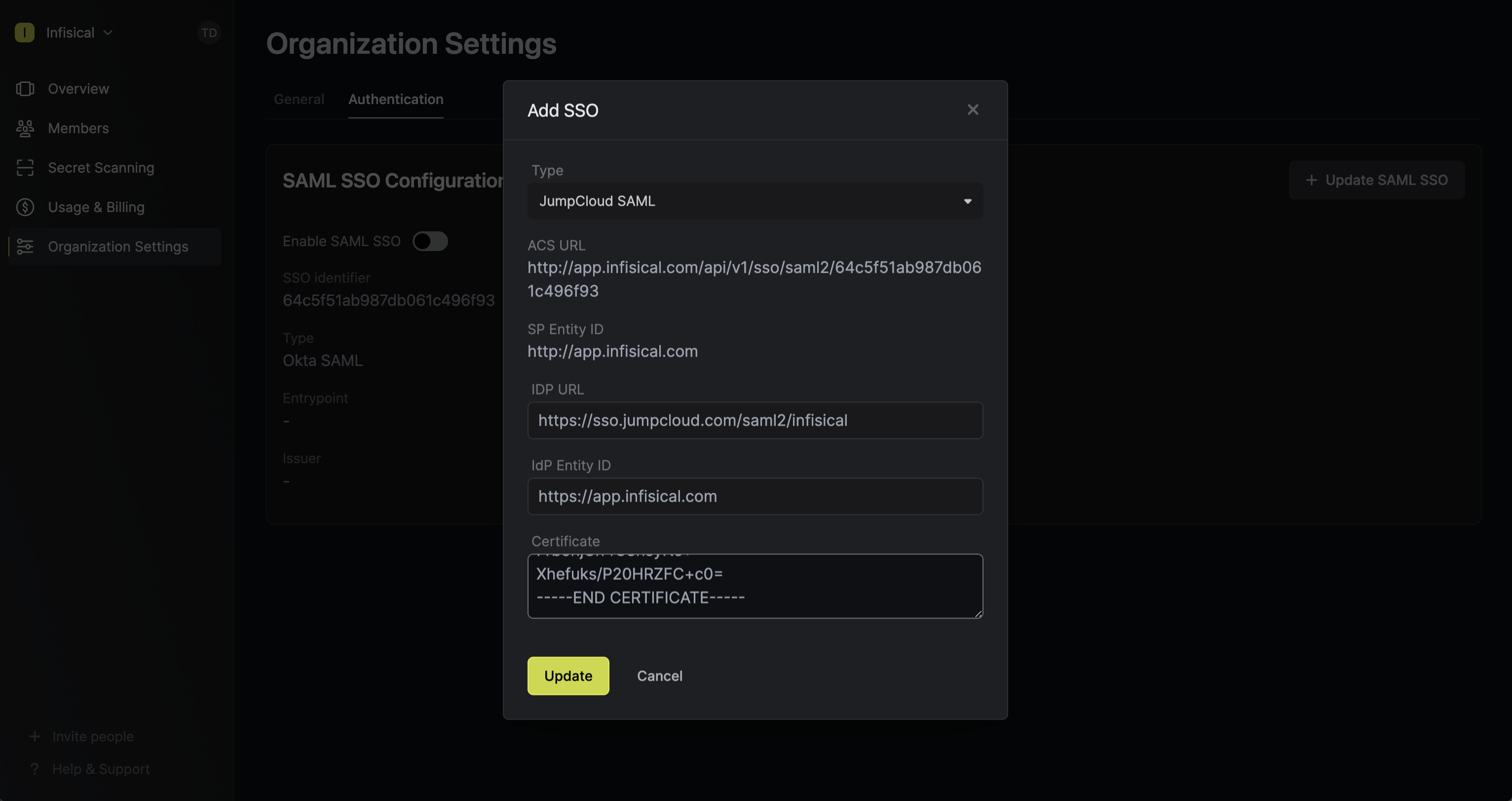
When pasting the certificate into Infisical, you'll want to retain `-----BEGIN
CERTIFICATE-----` and `-----END CERTIFICATE-----` at the first and last line
of the text area respectively.
Back in JumpCloud, navigate to the **User Groups** tab and assign users to the newly created application.
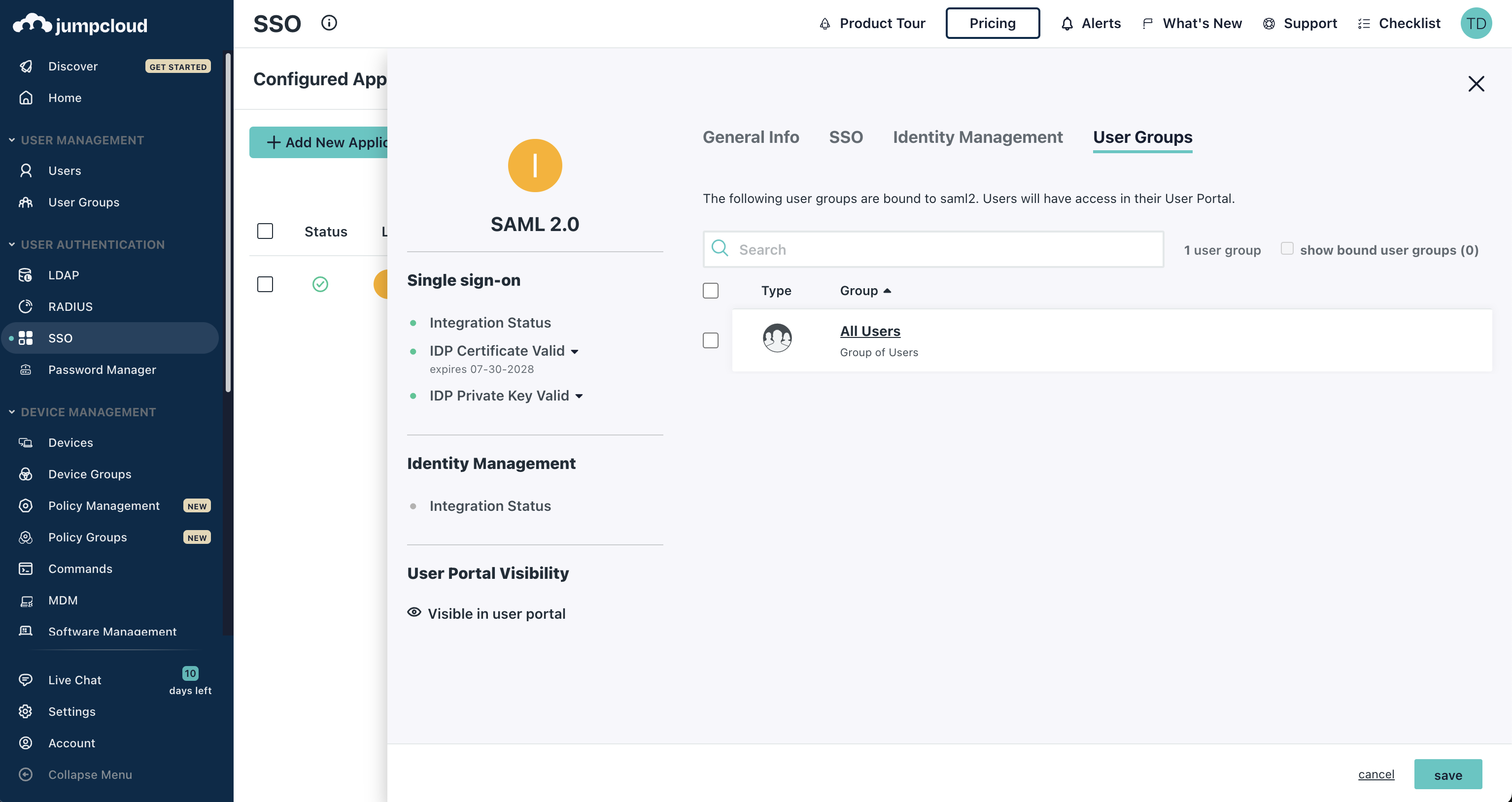
Enabling SAML SSO allows members in your organization to log into Infisical via JumpCloud.
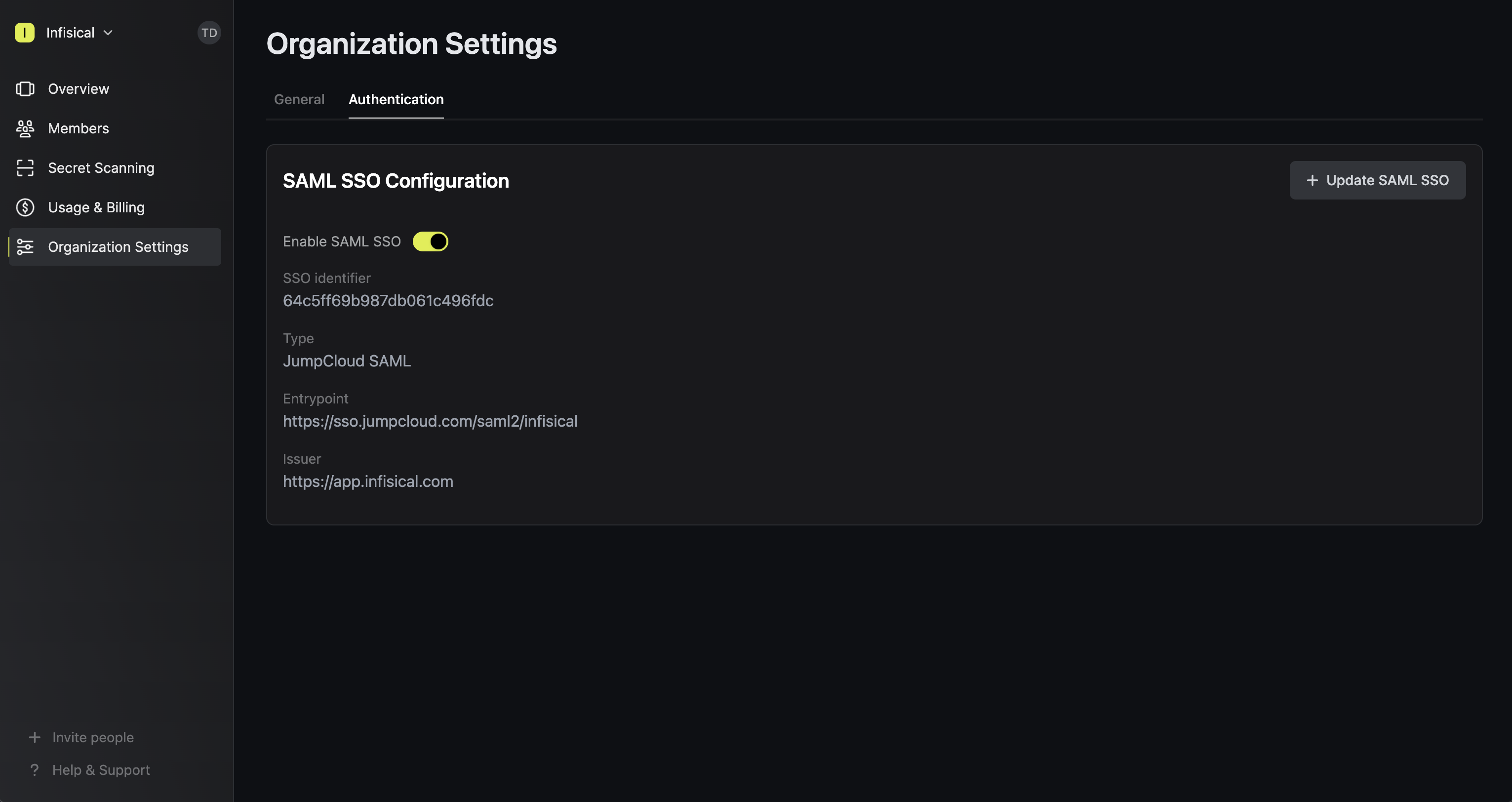
Enforcing SAML SSO ensures that members in your organization can only access Infisical
by logging into the organization via JumpCloud.
To enforce SAML SSO, you're required to test out the SAML connection by successfully authenticating at least one JumpCloud user with Infisical;
Once you've completed this requirement, you can toggle the **Enforce SAML SSO** button to enforce SAML SSO.
We recommend ensuring that your account is provisioned the application in JumpCloud
prior to enforcing SAML SSO to prevent any unintended issues.
If you are only using one organization on your Infisical instance, you can configure a default organization in the [Server Admin Console](../admin-panel/server-admin#default-organization) to expedite SAML login.
If you're configuring SAML SSO on a self-hosted instance of Infisical, make
sure to set the `AUTH_SECRET` and `SITE_URL` environment variable for it to
work:
* `AUTH_SECRET`: A secret key used for signing and verifying JWT. This
can be a random 32-byte base64 string generated with `openssl rand -base64
32`.
* `SITE_URL`: The absolute URL of your self-hosted instance of Infisical including the protocol (e.g. [https://app.infisical.com](https://app.infisical.com))
# Keycloak OIDC
Learn how to configure Keycloak OIDC for Infisical SSO.
Keycloak OIDC SSO is a paid feature. If you're using Infisical Cloud, then it
is available under the **Pro Tier**. If you're self-hosting Infisical, then
you should contact [sales@infisical.com](mailto:sales@infisical.com) to purchase an enterprise license to
use it.
1.1. In your realm, navigate to the **Clients** tab and click **Create client** to create a new client application.
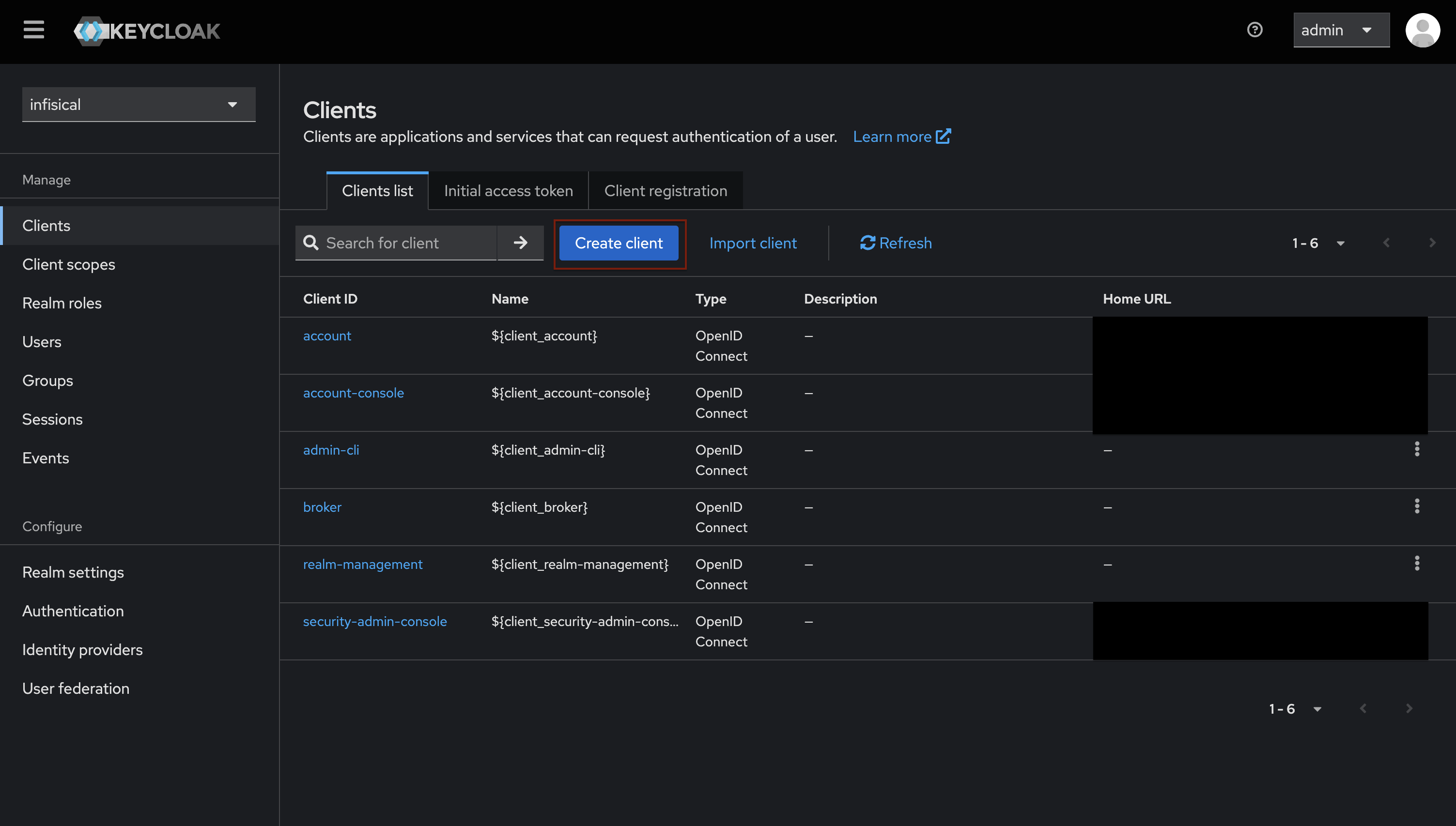
You don’t typically need to make a realm dedicated to Infisical. We recommend adding Infisical as a client to your primary realm.
1.2. In the General Settings step, set **Client type** to **OpenID Connect**, the **Client ID** field to an appropriate identifier, and the **Name** field to a friendly name like **Infisical**.
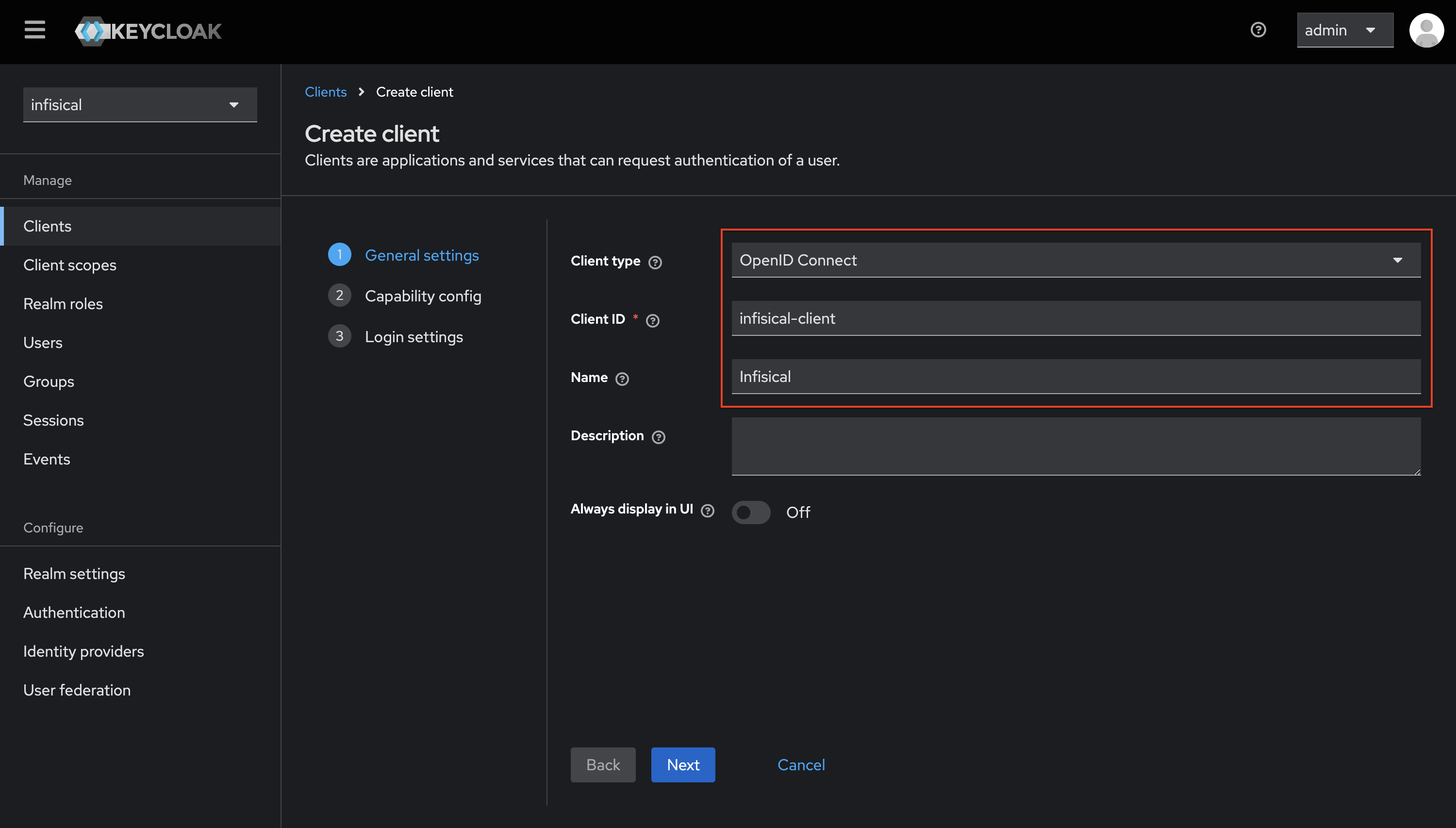
1.3. Next, in the Capability Config step, ensure that **Client Authentication** is set to On and that **Standard flow** is enabled in the Authentication flow section.
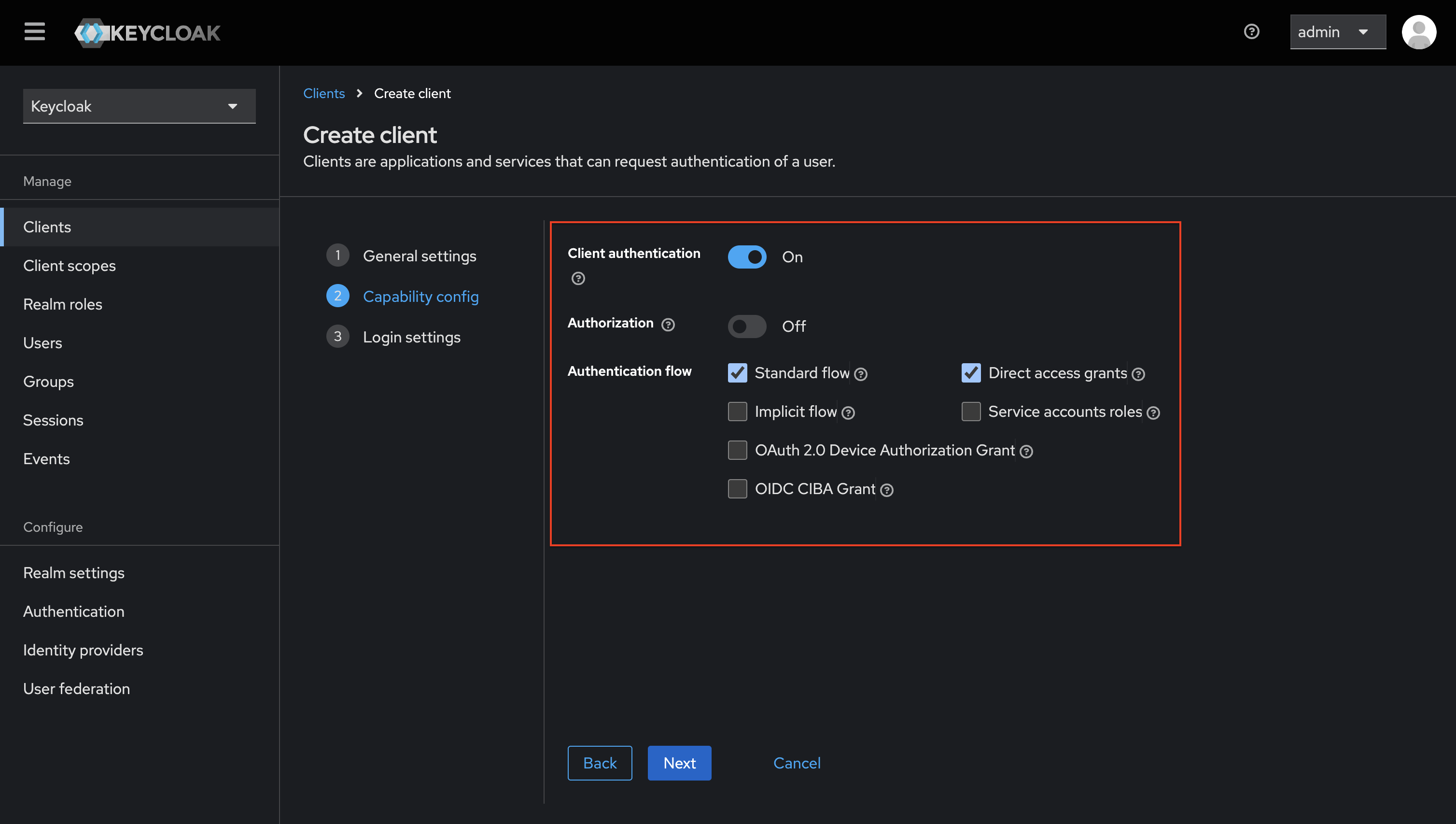
1.4. In the Login Settings step, set the following values:
* Root URL: `https://app.infisical.com`.
* Home URL: `https://app.infisical.com`.
* Valid Redirect URIs: `https://app.infisical.com/api/v1/sso/oidc/callback`.
* Web origins: `https://app.infisical.com`.
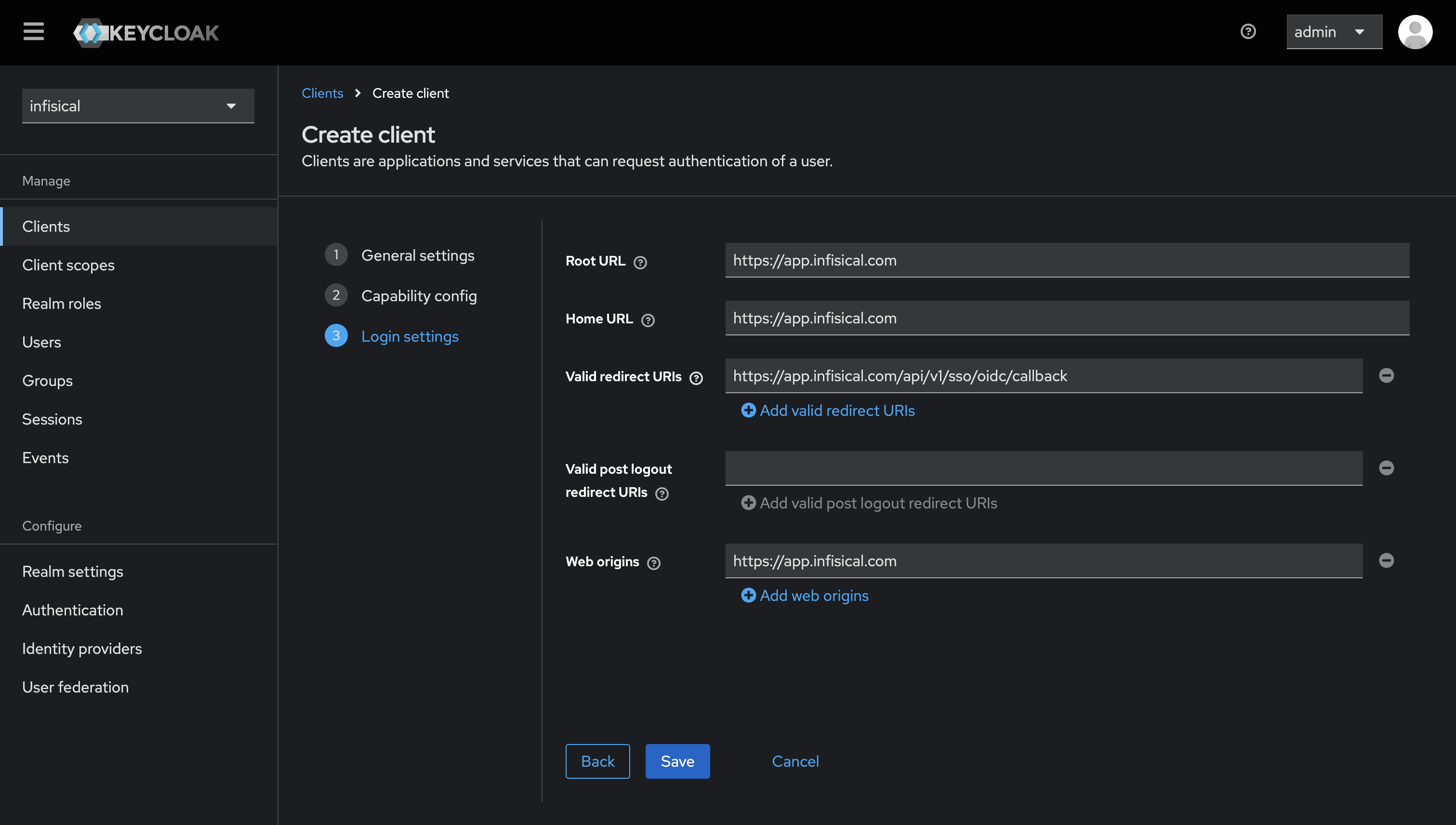
If you’re self-hosting Infisical, then you will want to replace [https://app.infisical.com](https://app.infisical.com) (base URL) with your own domain.
1.5. Next, navigate to the **Client scopes** tab and select the client's dedicated scope.
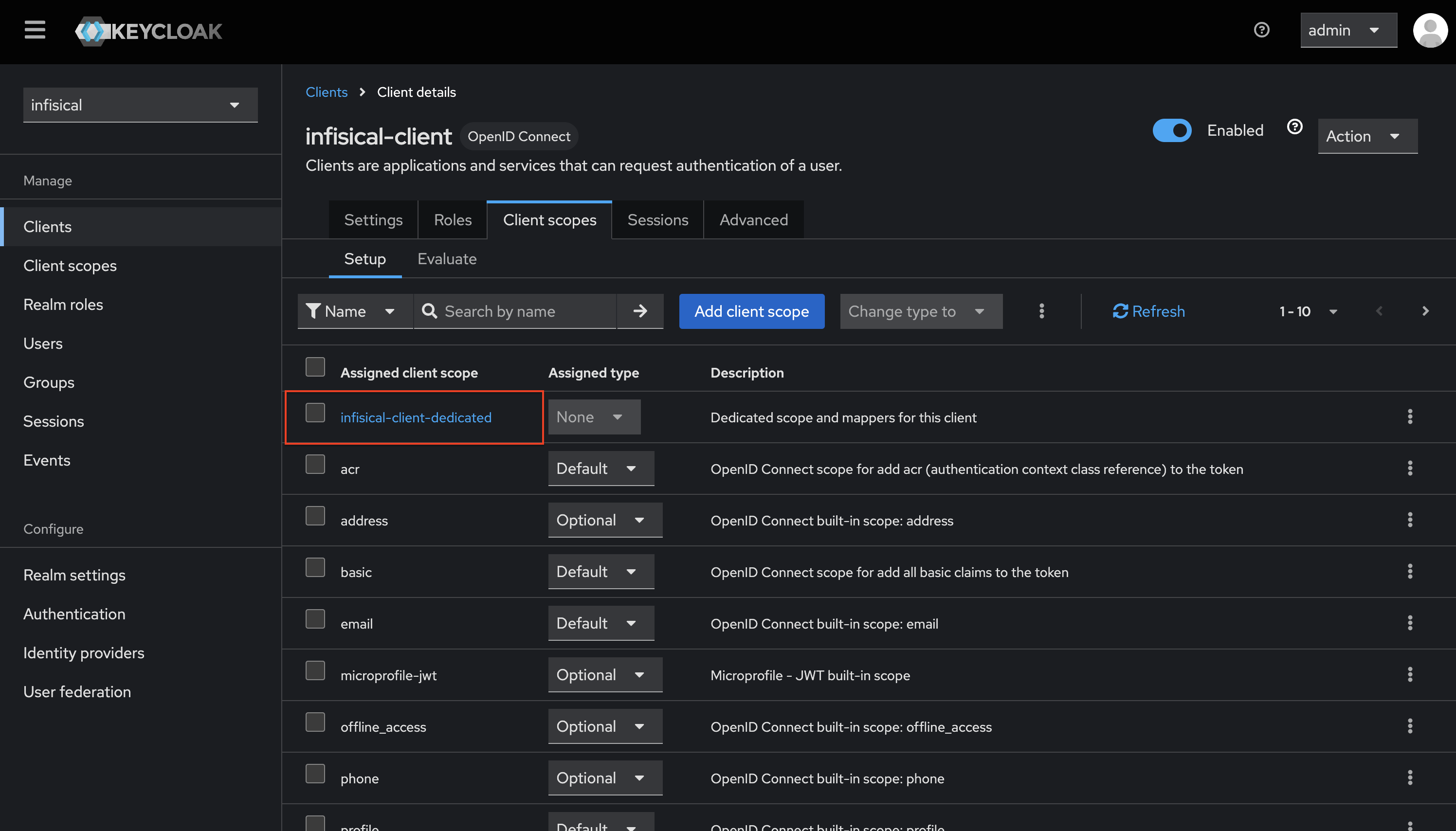
1.6. Next, click **Add predefined mapper**.
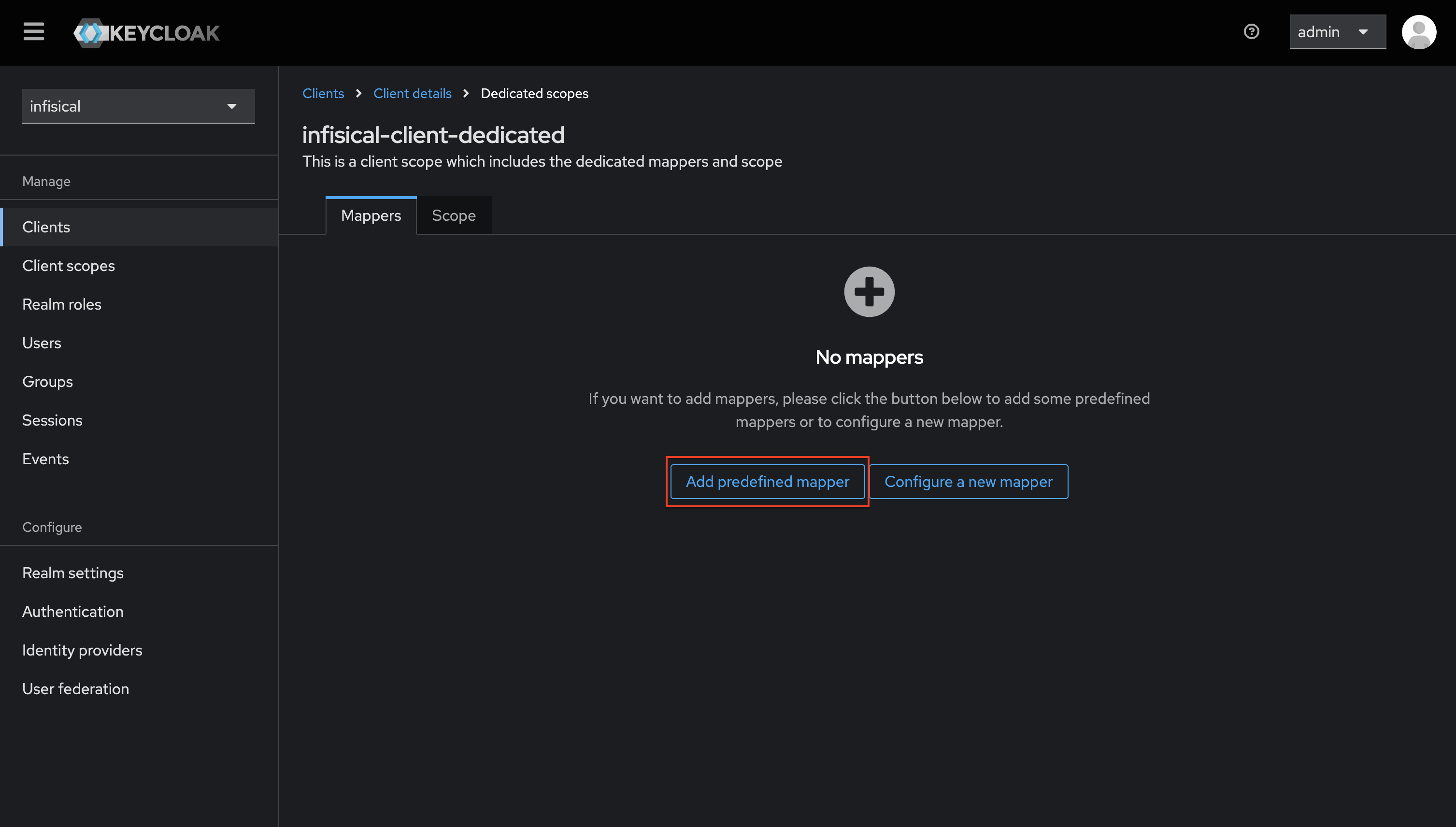
1.7. Select the **email**, **given name**, **family name** attributes and click **Add**.
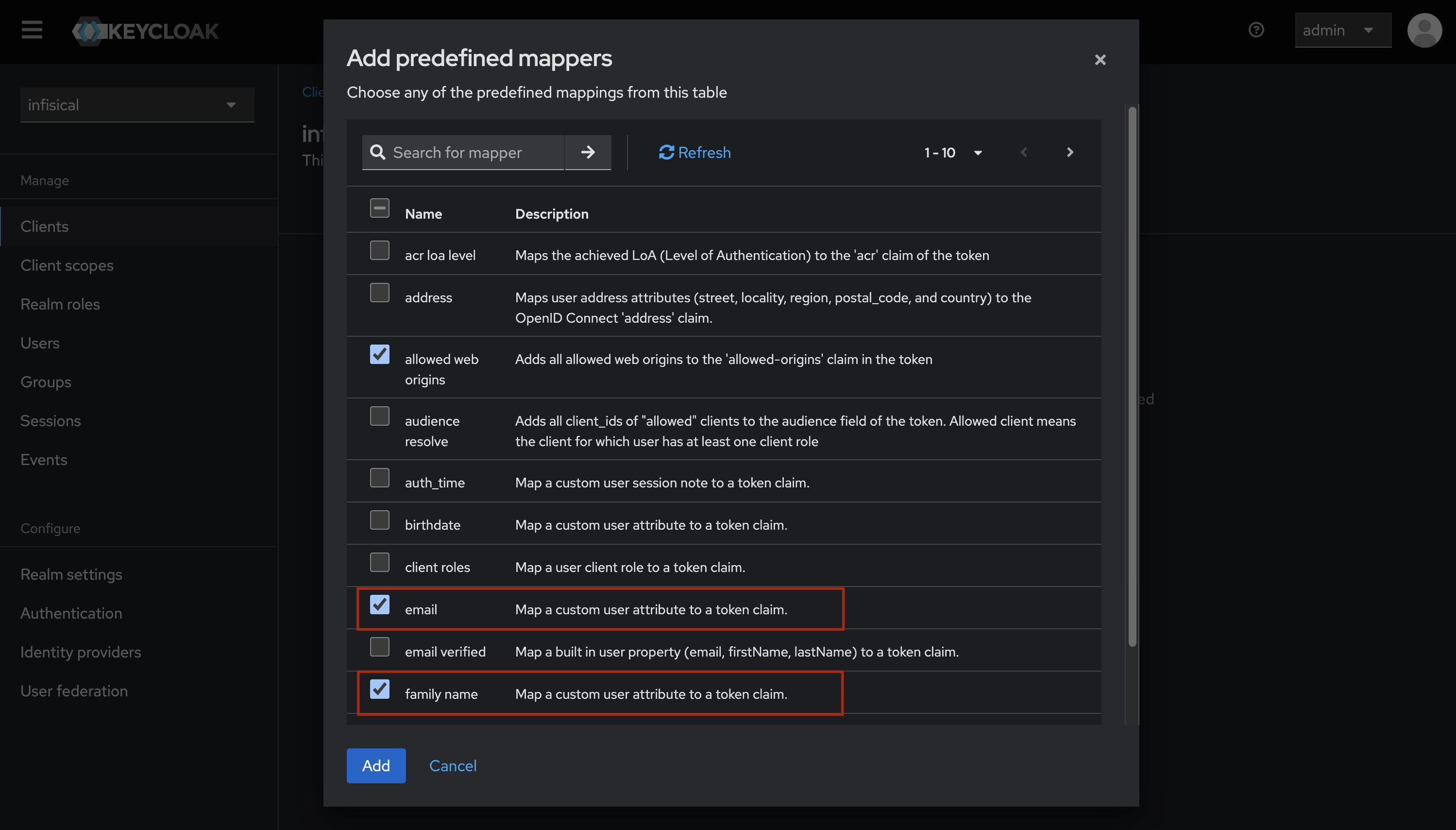
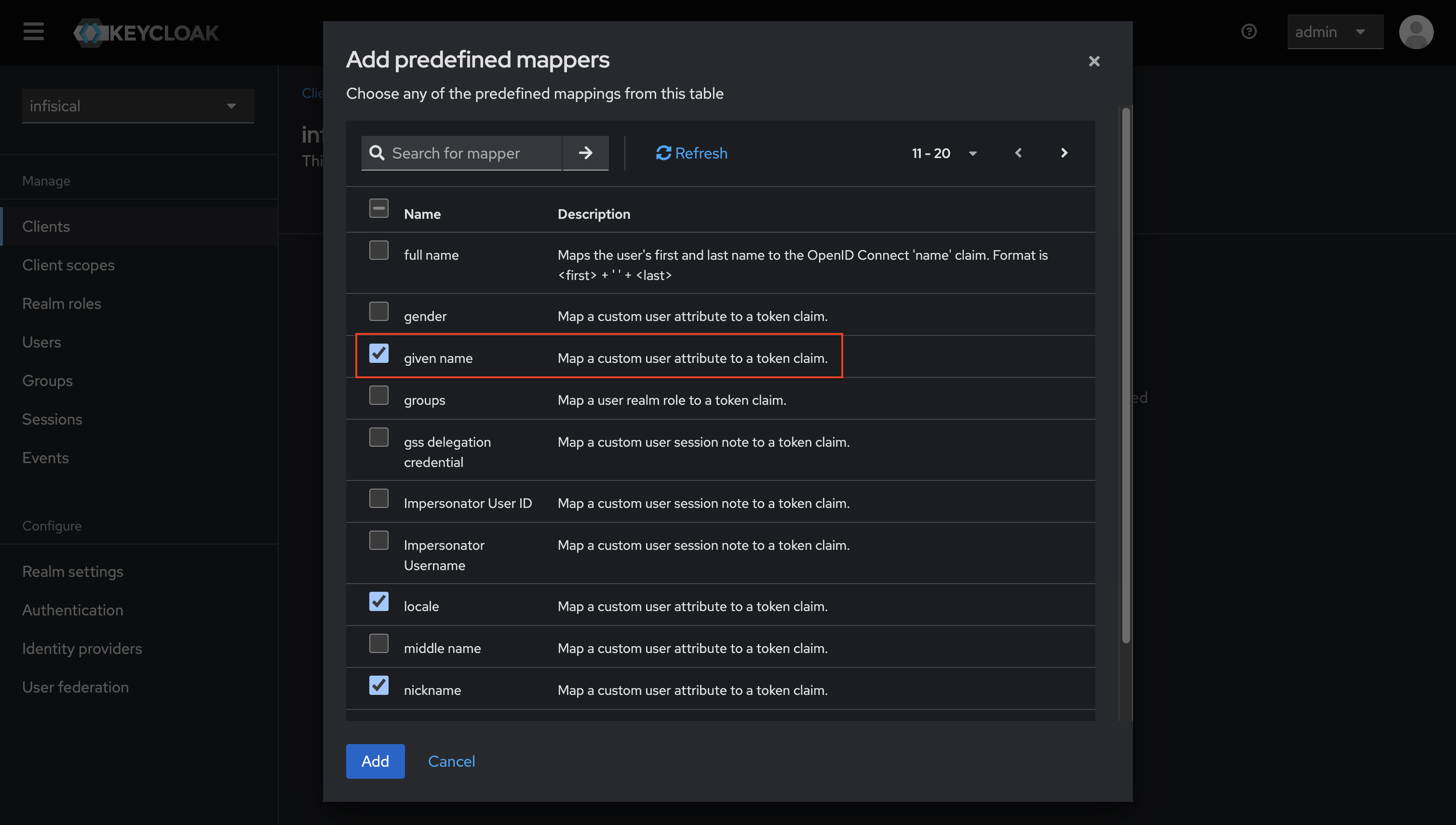
Once you've completed the above steps, the list of mappers should look like the following:
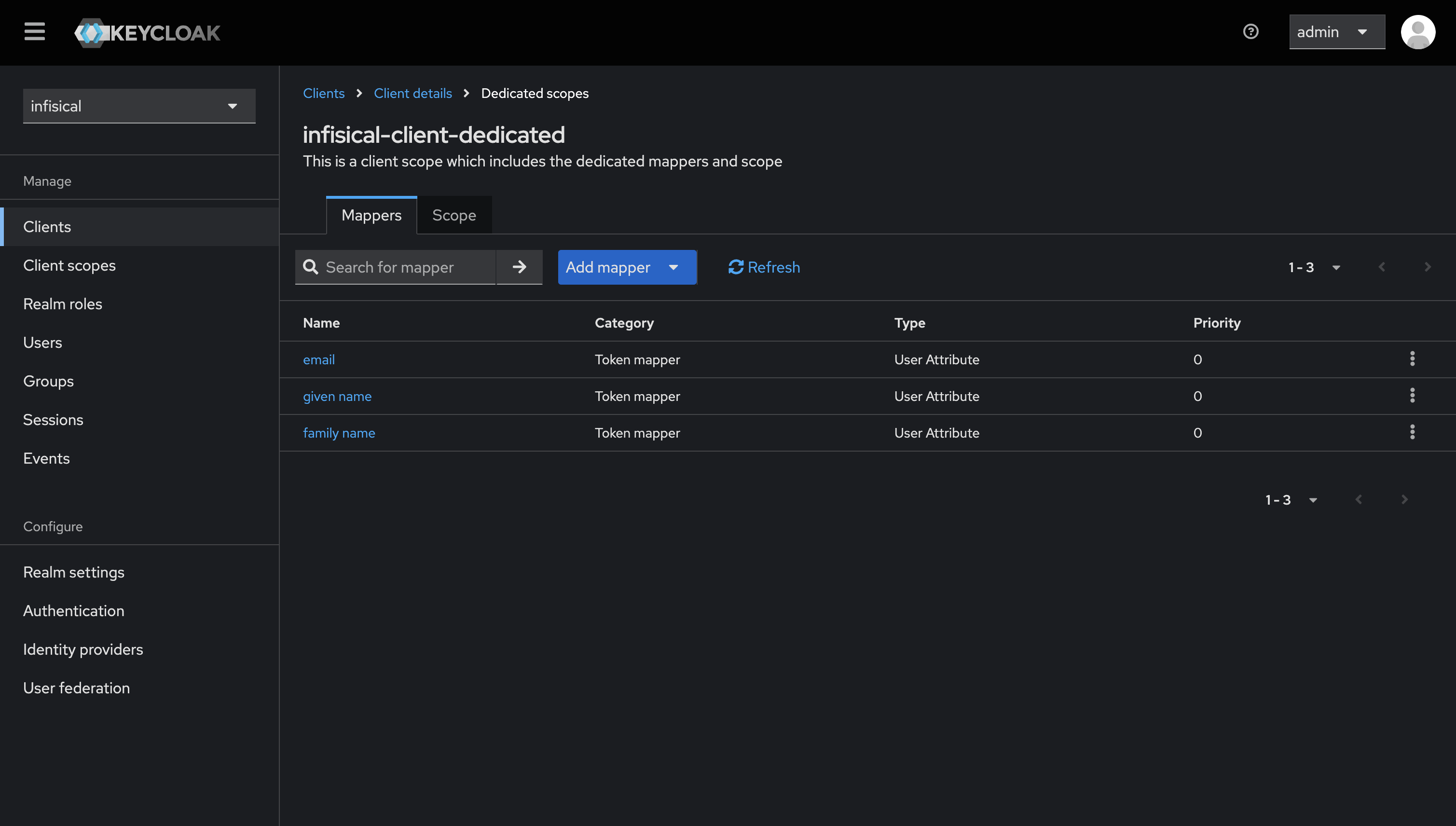
2.1. Back in Keycloak, navigate to Configure > Realm settings > General tab > Endpoints > OpenID Endpoint Configuration and copy the opened URL. This is what is to referred to as the Discovery Document URL and it takes the form: `https://keycloak-mysite.com/realms/myrealm/.well-known/openid-configuration`.
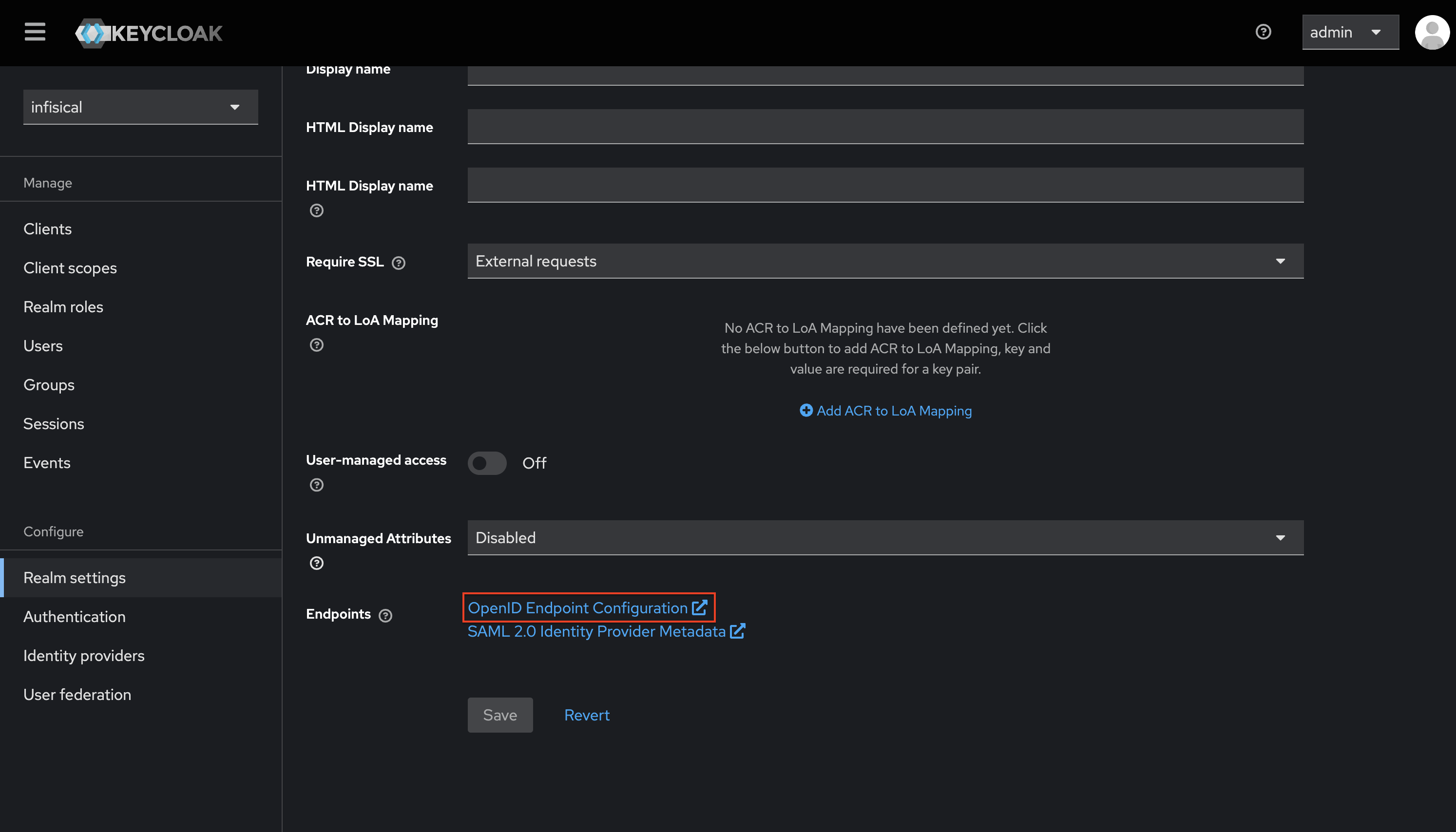
2.2. From the Clients page, navigate to the Credential tab and copy the **Client Secret** to be used in the next steps.
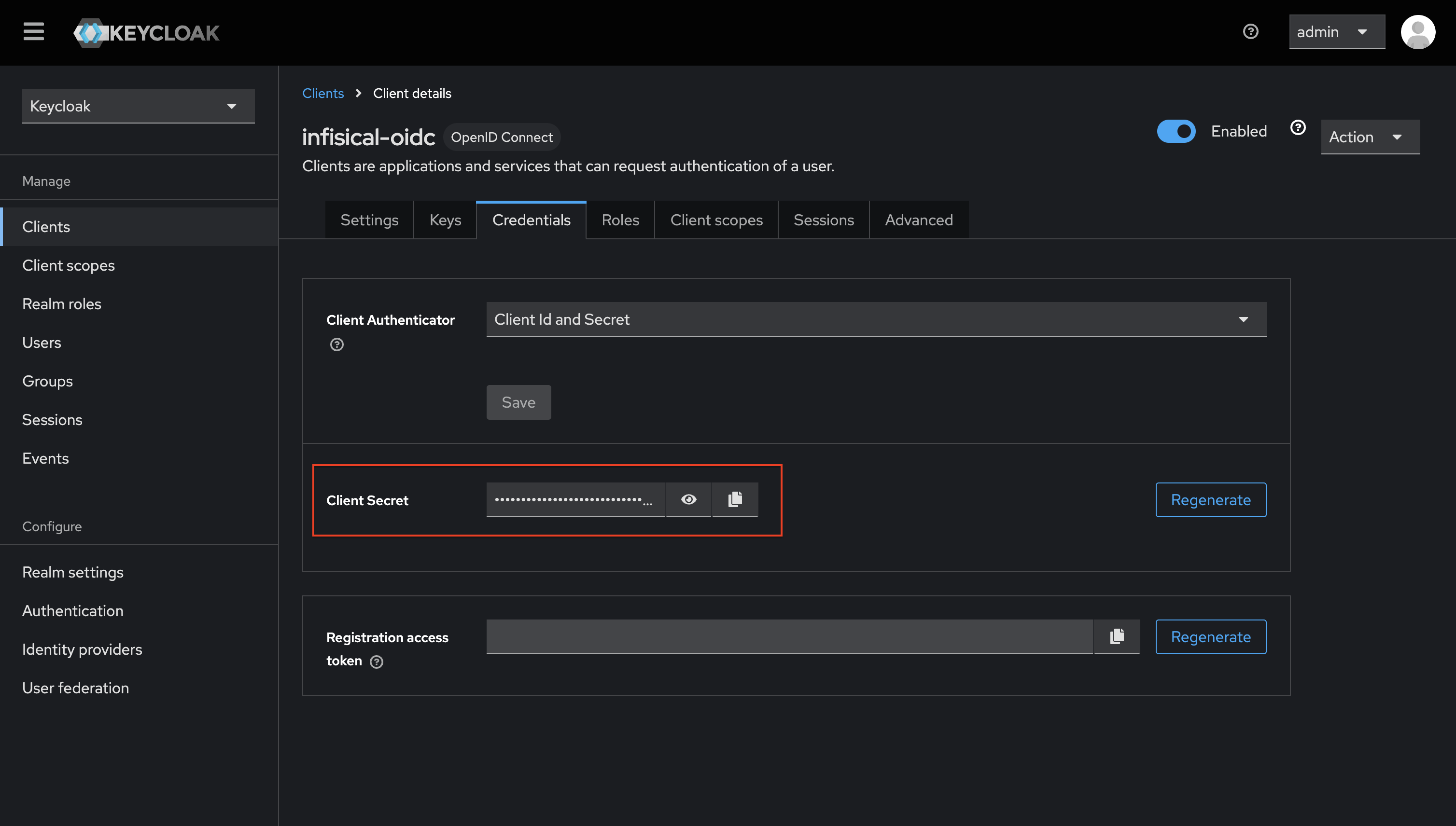
3.1. Back in Infisical, in the Organization settings > Security > OIDC, click Connect.

3.2. For configuration type, select Discovery URL. Then, set the appropriate values for **Discovery Document URL**, **Client ID**, and **Client Secret**.
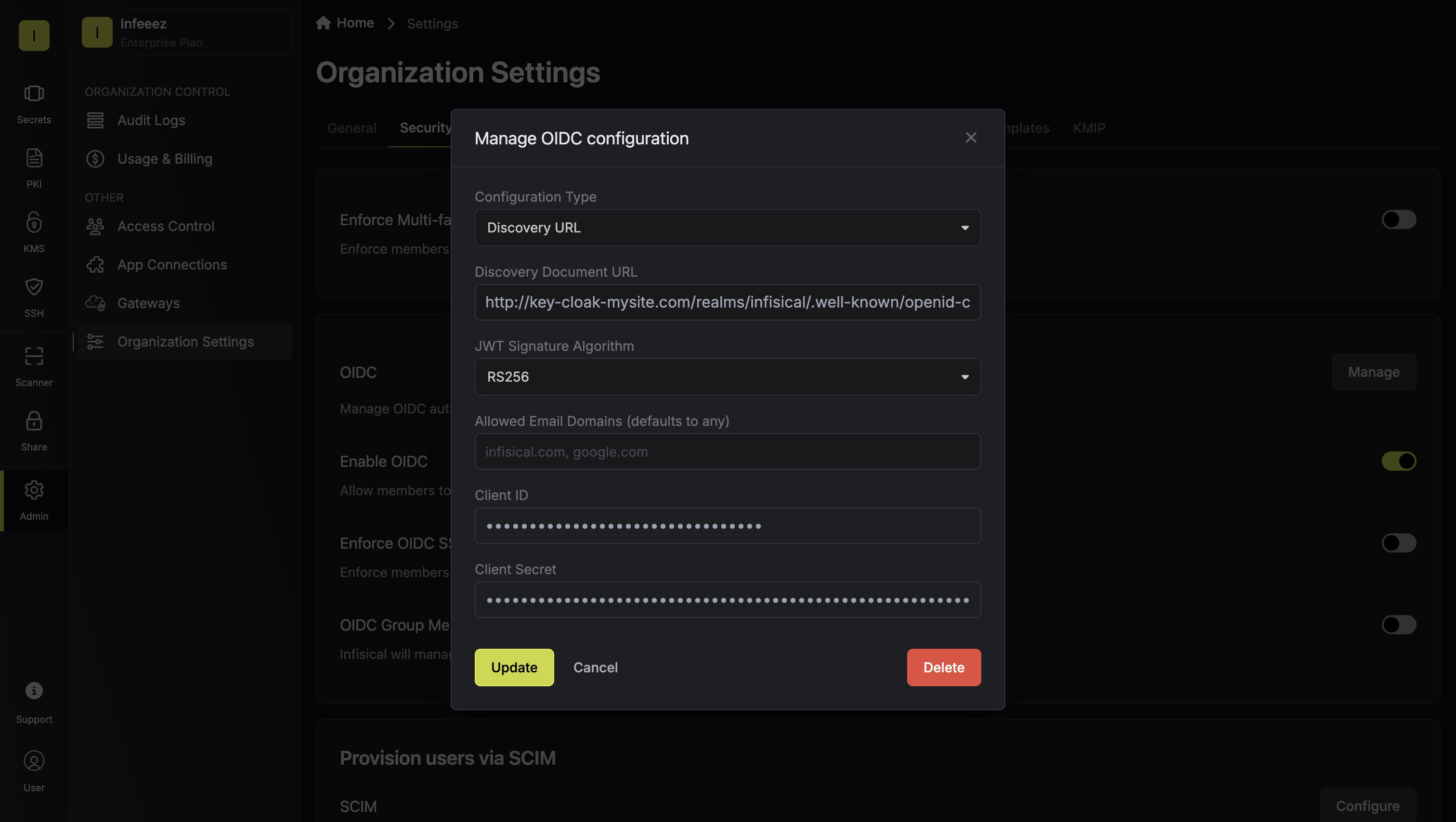
Once you've done that, press **Update** to complete the required configuration.
Enabling OIDC SSO allows members in your organization to log into Infisical via Keycloak.
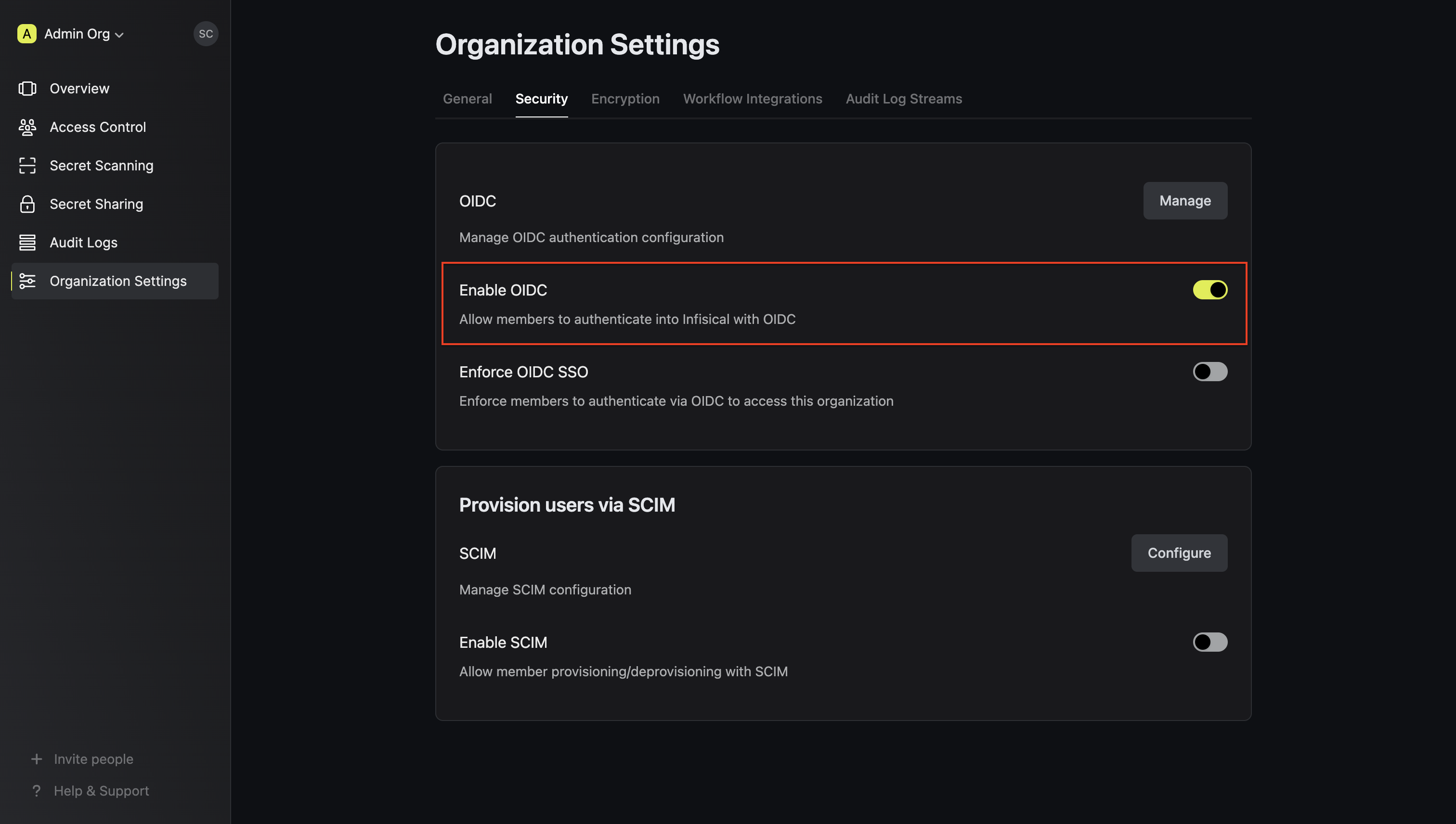
Enforcing OIDC SSO ensures that members in your organization can only access Infisical
by logging into the organization via Keycloak.
To enforce OIDC SSO, you're required to test out the OpenID connection by successfully authenticating at least one Keycloak user with Infisical.
Once you've completed this requirement, you can toggle the **Enforce OIDC SSO** button to enforce OIDC SSO.
We recommend ensuring that your account is provisioned using the application in Keycloak
prior to enforcing OIDC SSO to prevent any unintended issues.
If you are only using one organization on your Infisical instance, you can configure a default organization in the [Server Admin Console](../admin-panel/server-admin#default-organization) to expedite OIDC login.
If you're configuring OIDC SSO on a self-hosted instance of Infisical, make
sure to set the `AUTH_SECRET` and `SITE_URL` environment variable for it to
work:
* `AUTH_SECRET`: A secret key used for signing and verifying JWT. This
can be a random 32-byte base64 string generated with `openssl rand -base64
32`.
* `SITE_URL`: The absolute URL of your self-hosted instance of Infisical including the protocol (e.g. [https://app.infisical.com](https://app.infisical.com))
# Keycloak SAML
Learn how to configure Keycloak SAML for Infisical SSO.
Keycloak SAML SSO is a paid feature.
If you're using Infisical Cloud, then it is available under the **Pro Tier**. If you're self-hosting Infisical,
then you should contact [sales@infisical.com](mailto:sales@infisical.com) to purchase an enterprise license to use it.
In Infisical, head to your Organization Settings > Authentication > SAML SSO Configuration and select **Manage**.
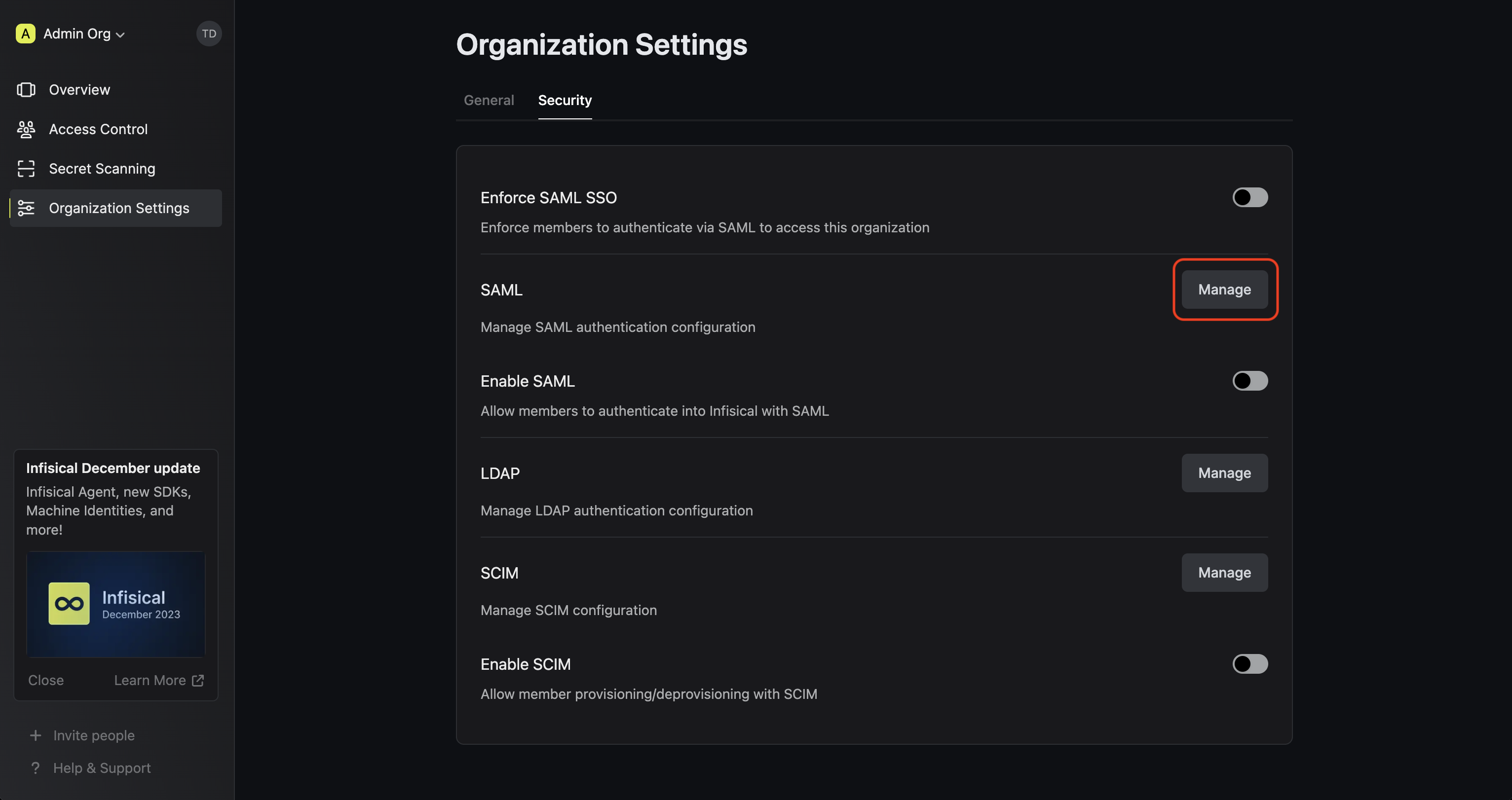
Next, copy the **Valid redirect URI** and **SP Entity ID** to use when configuring the Keycloak SAML application.
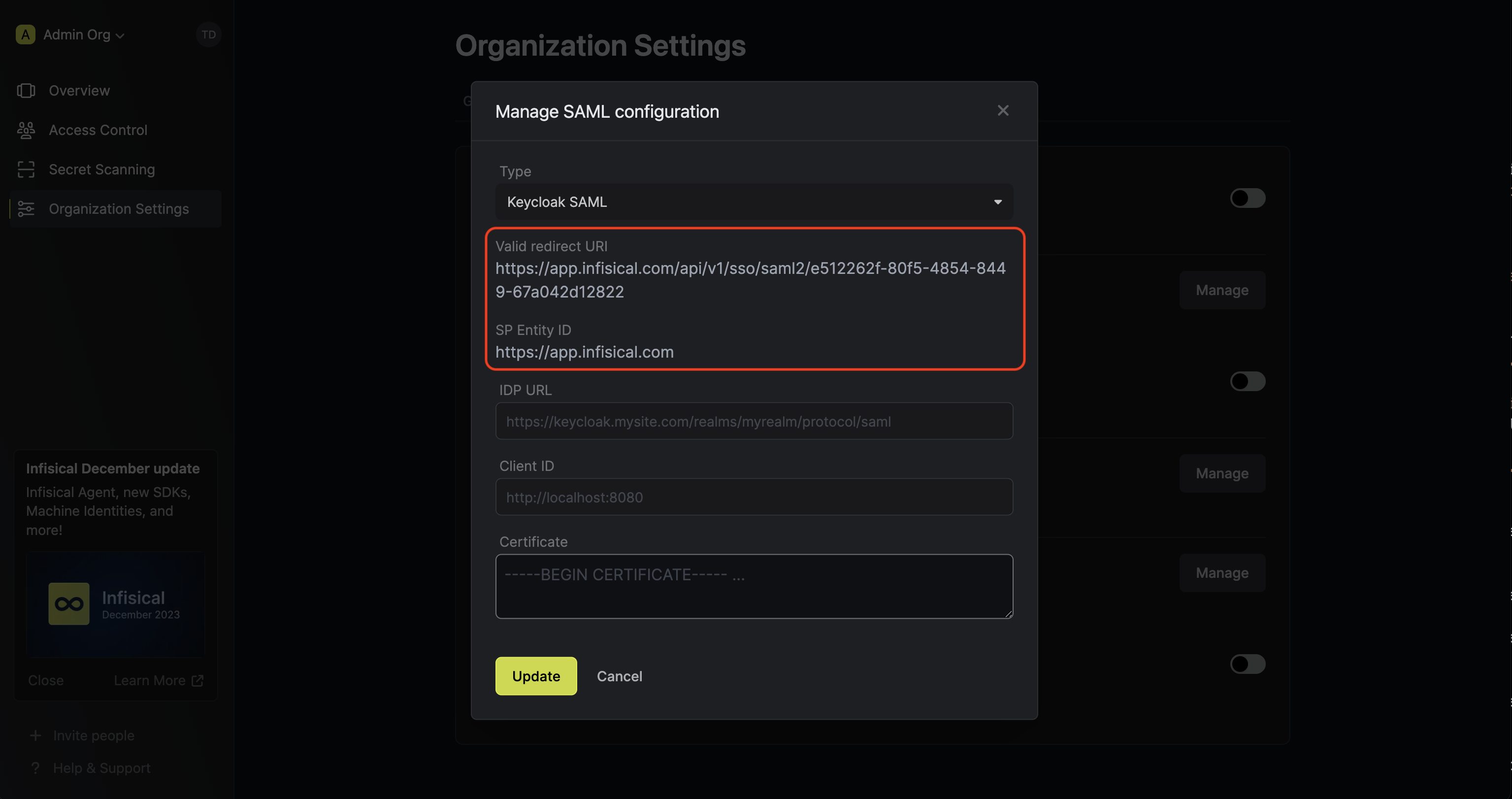
2.1. In your realm, navigate to the **Clients** tab and click **Create client** to create a new client application.
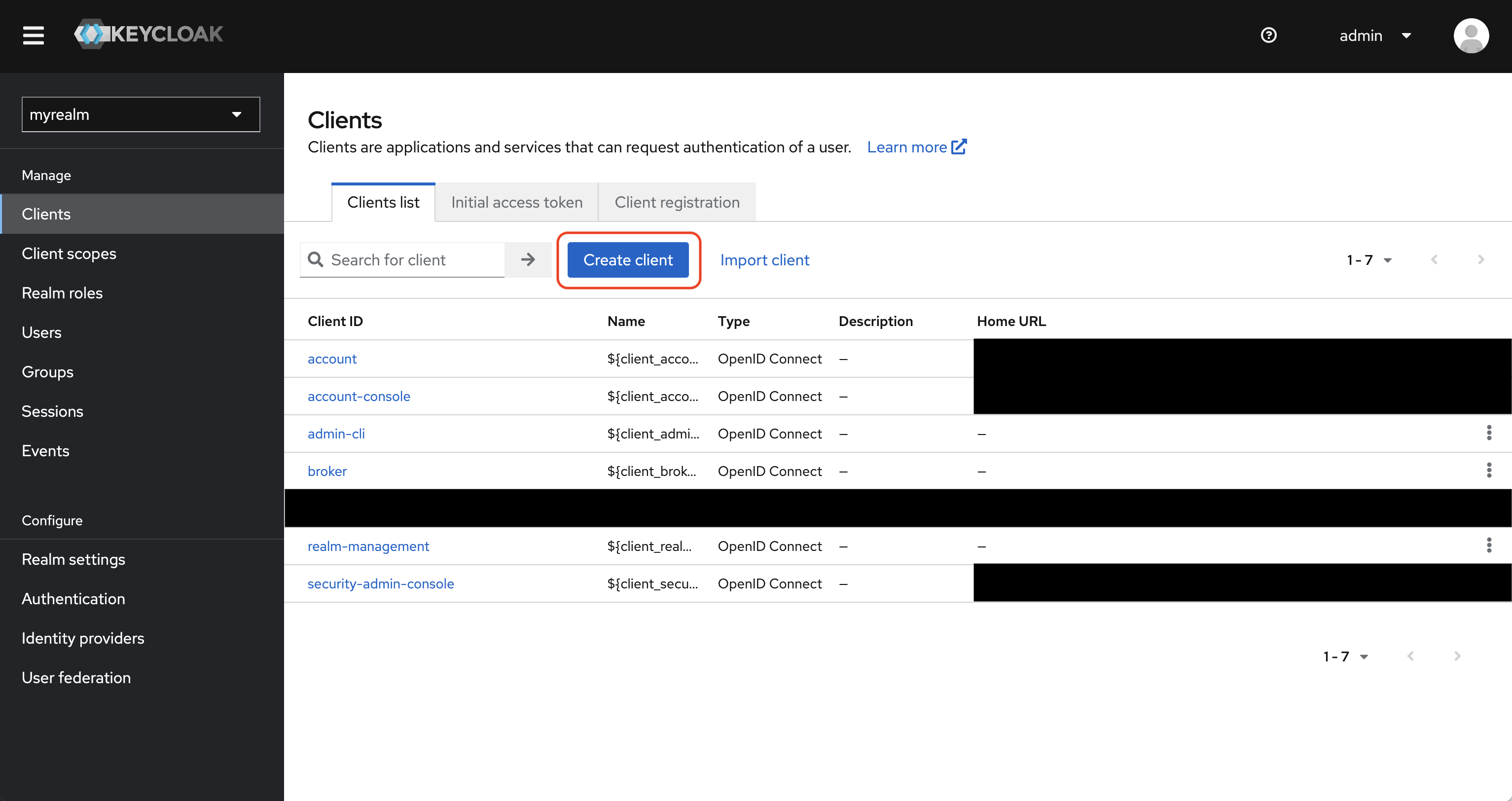
You don’t typically need to make a realm dedicated to Infisical. We recommend adding Infisical as a client to your primary realm.
In the General Settings step, set **Client type** to **SAML**, the **Client ID** field to `https://app.infisical.com`, and the **Name** field to a friendly name like **Infisical**.
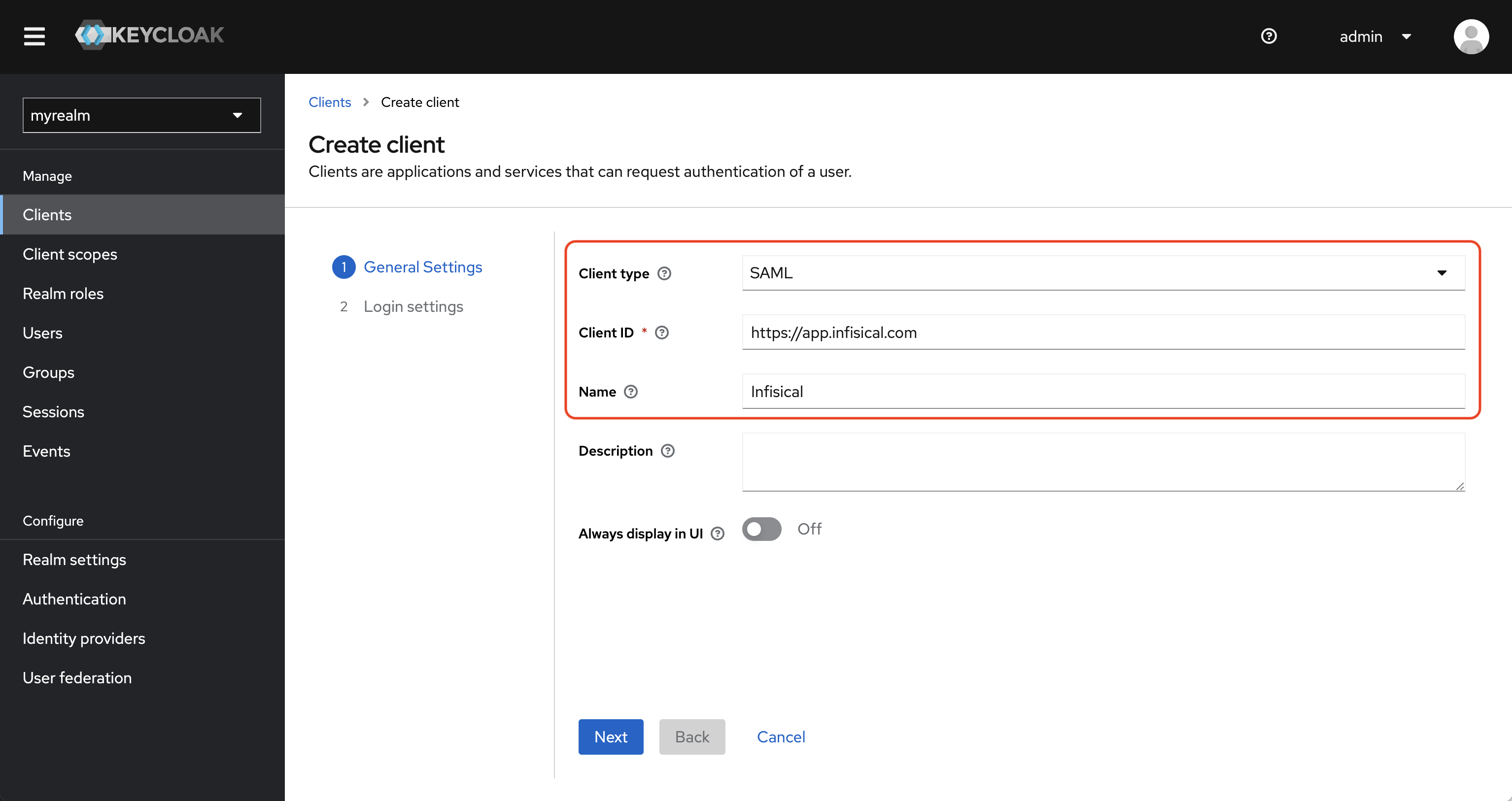
If you’re self-hosting Infisical, then you will want to replace [https://app.infisical.com](https://app.infisical.com) with your own domain.
Next, in the Login Settings step, set both the **Home URL** field and **Valid redirect URIs** field to the **Valid redirect URI** from step 1 and press **Save**.
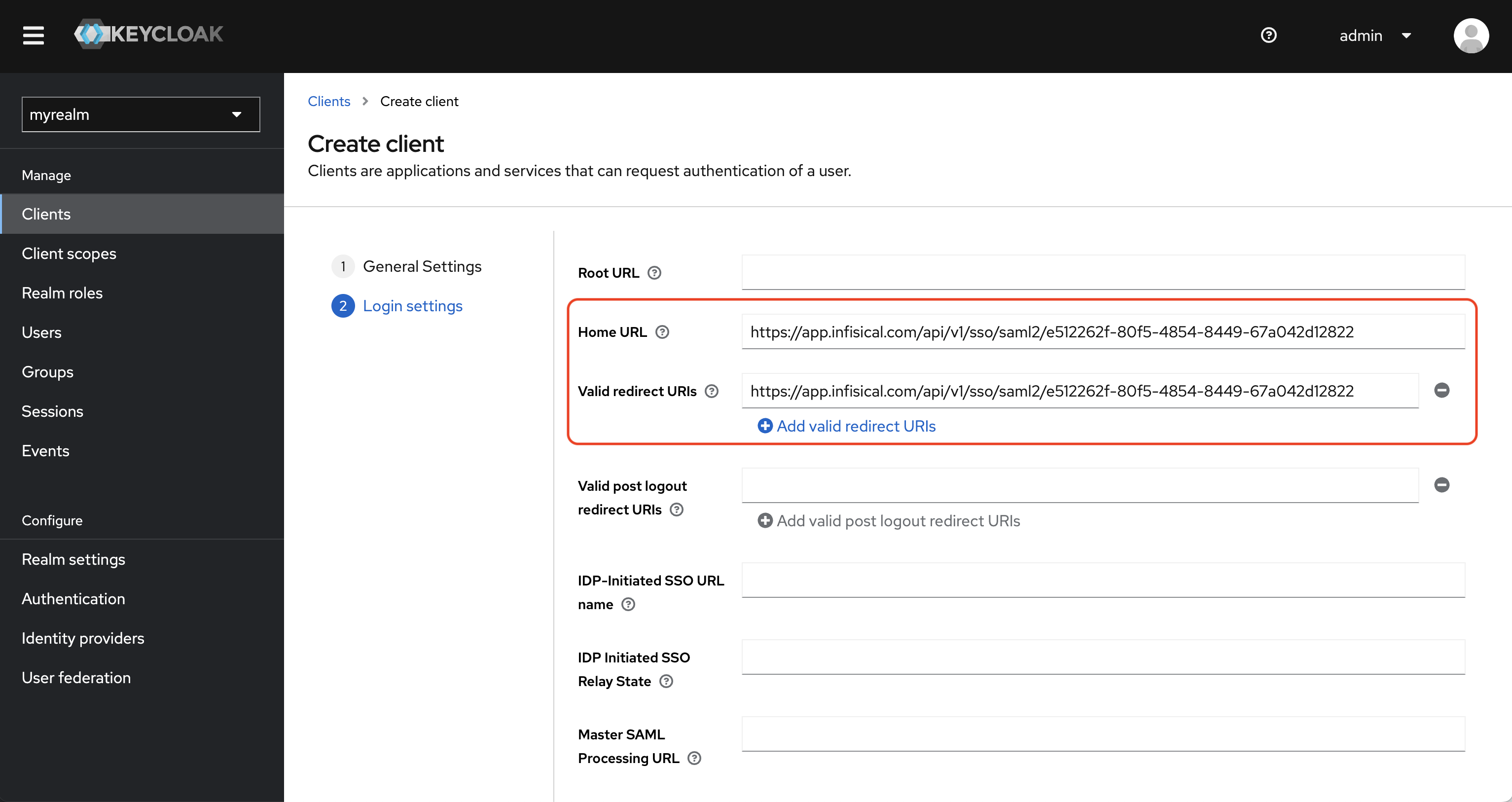
2.2. Once you've created the client, under its **Settings** tab, make sure to set the following values:
* Under **SAML Capabilities**:
* Name ID format: email (or username).
* Force name ID format: On.
* Force POST binding: On.
* Include AuthnStatement: On.
* Under **Signature and Encryption**:
* Sign documents: On.
* Sign assertions: On.
* Signature algorithm: RSA\_SHA256.
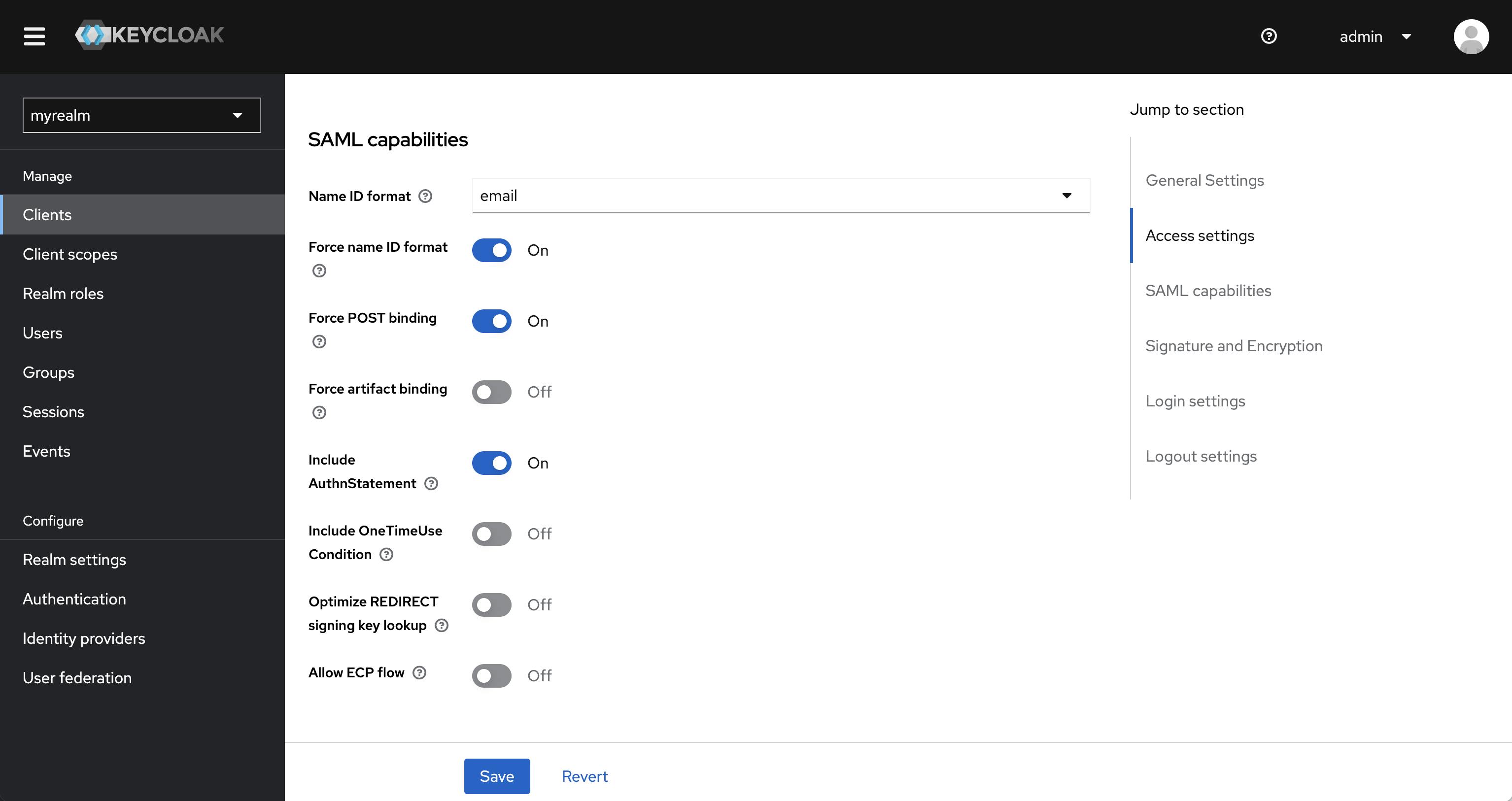
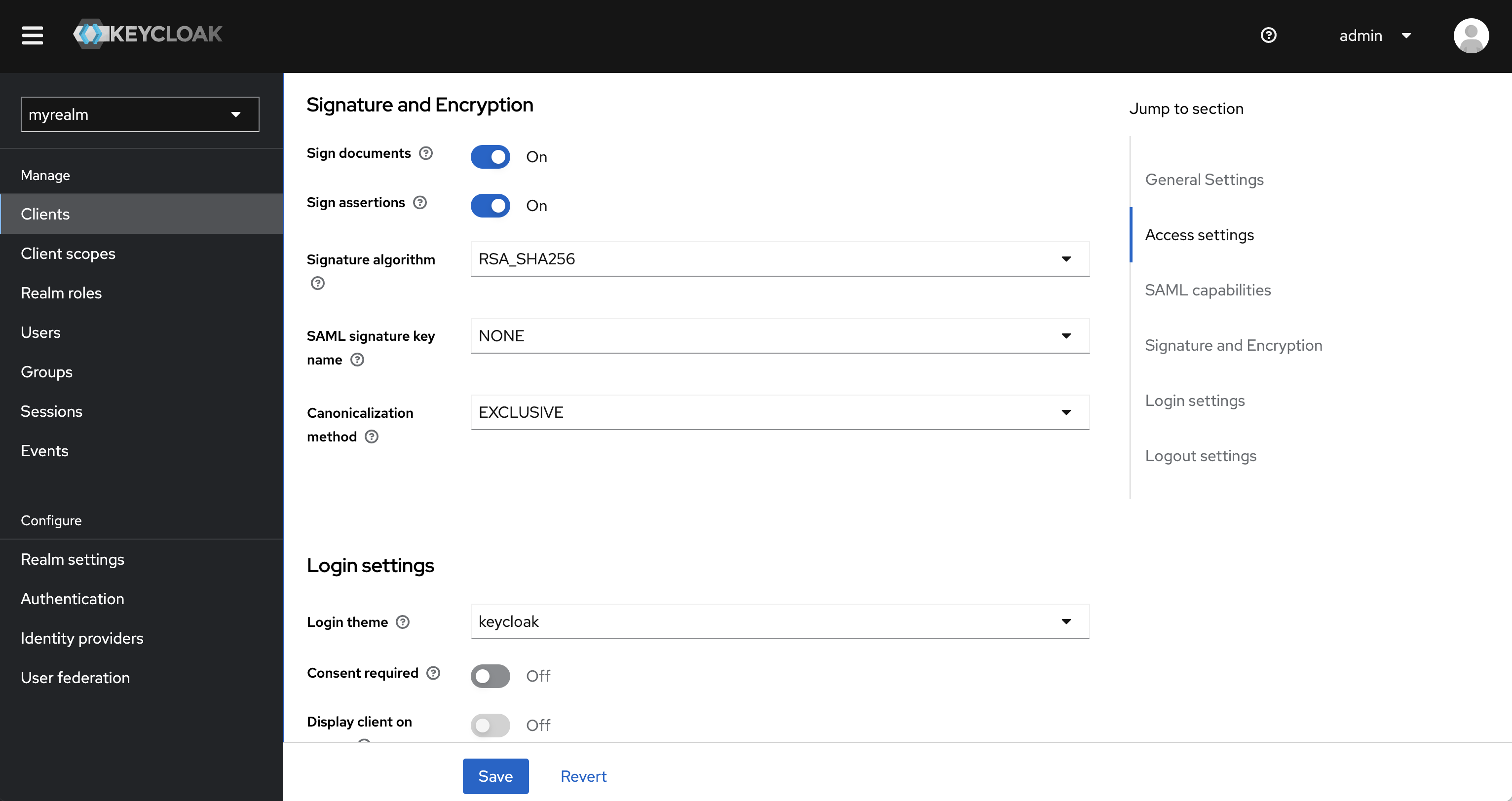
2.3. Next, navigate to the **Client scopes** tab select the client's dedicated scope.
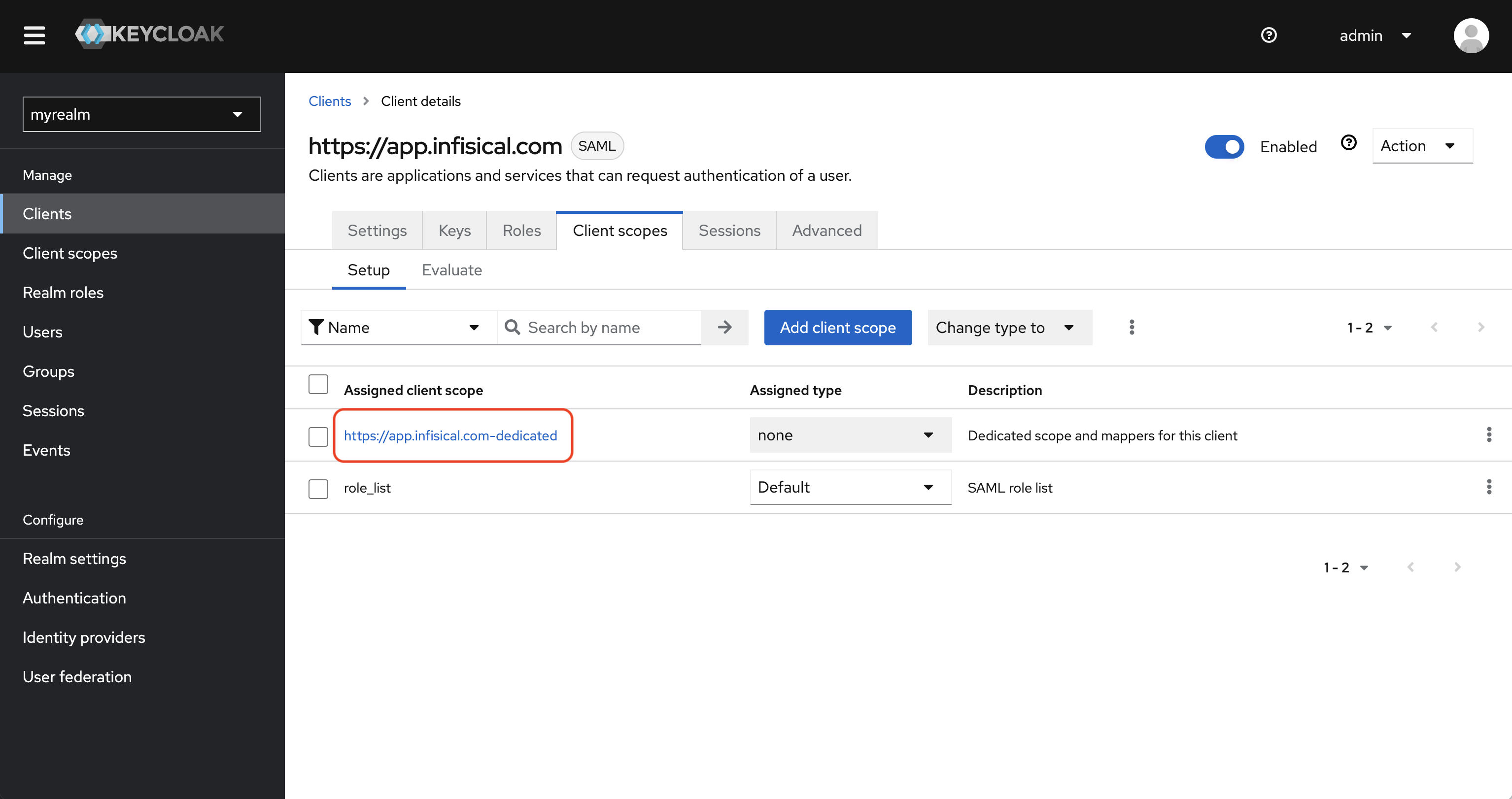
Next click **Add predefined mapper**.
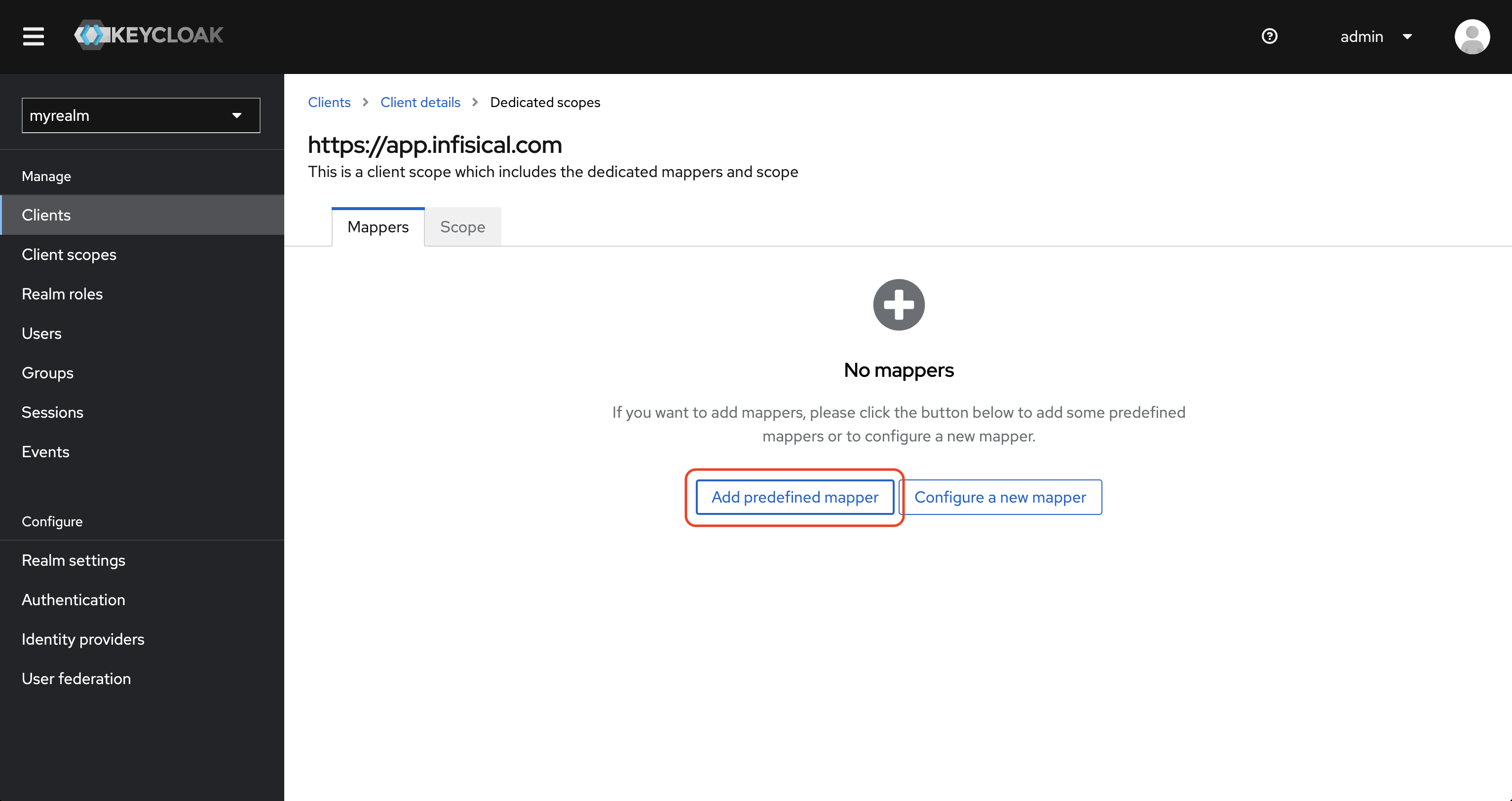
Select the **X500 email**, **X500 givenName**, and **X500 surname** attributes and click **Add**.
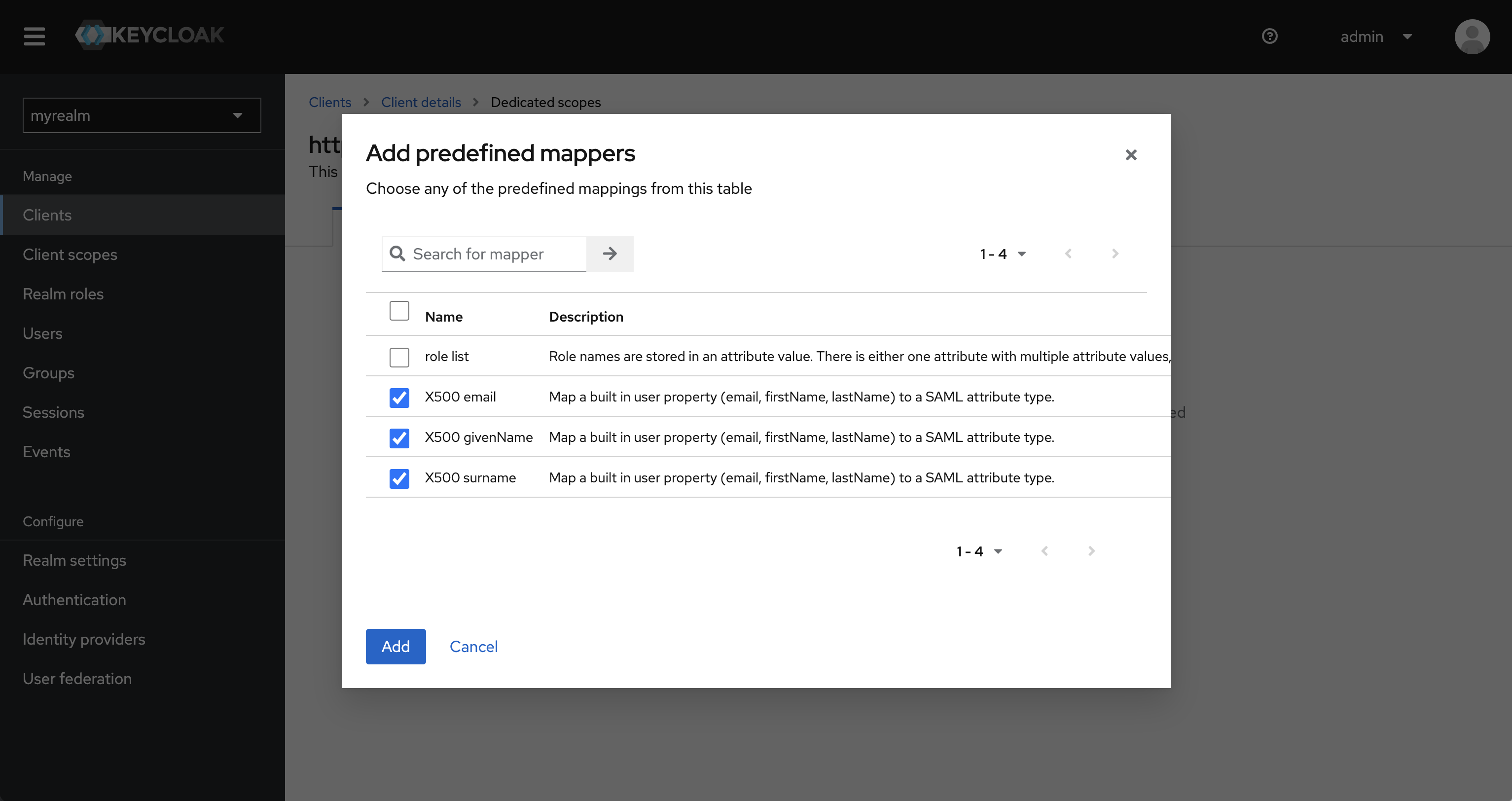
Now click on the **X500 email** mapper and set the **SAML Attribute Name** field to **email**.
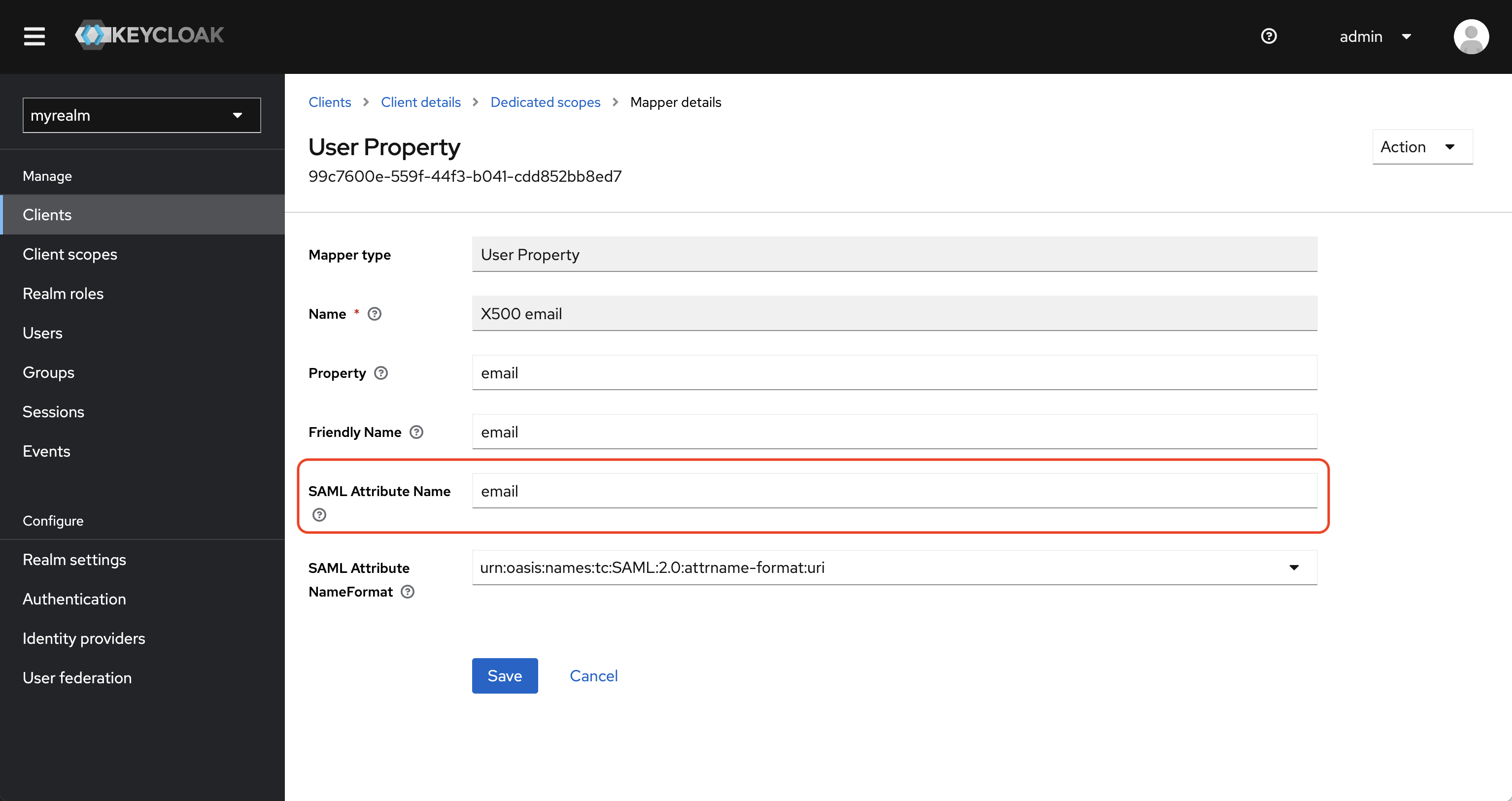
Repeat the same for **X500 givenName** and **X500 surname** mappers, setting the **SAML Attribute Name** field to **firstName** and **lastName** respectively.
Next, back in the client scope's **Mappers**, click **Add mapper** and select **by configuration**.
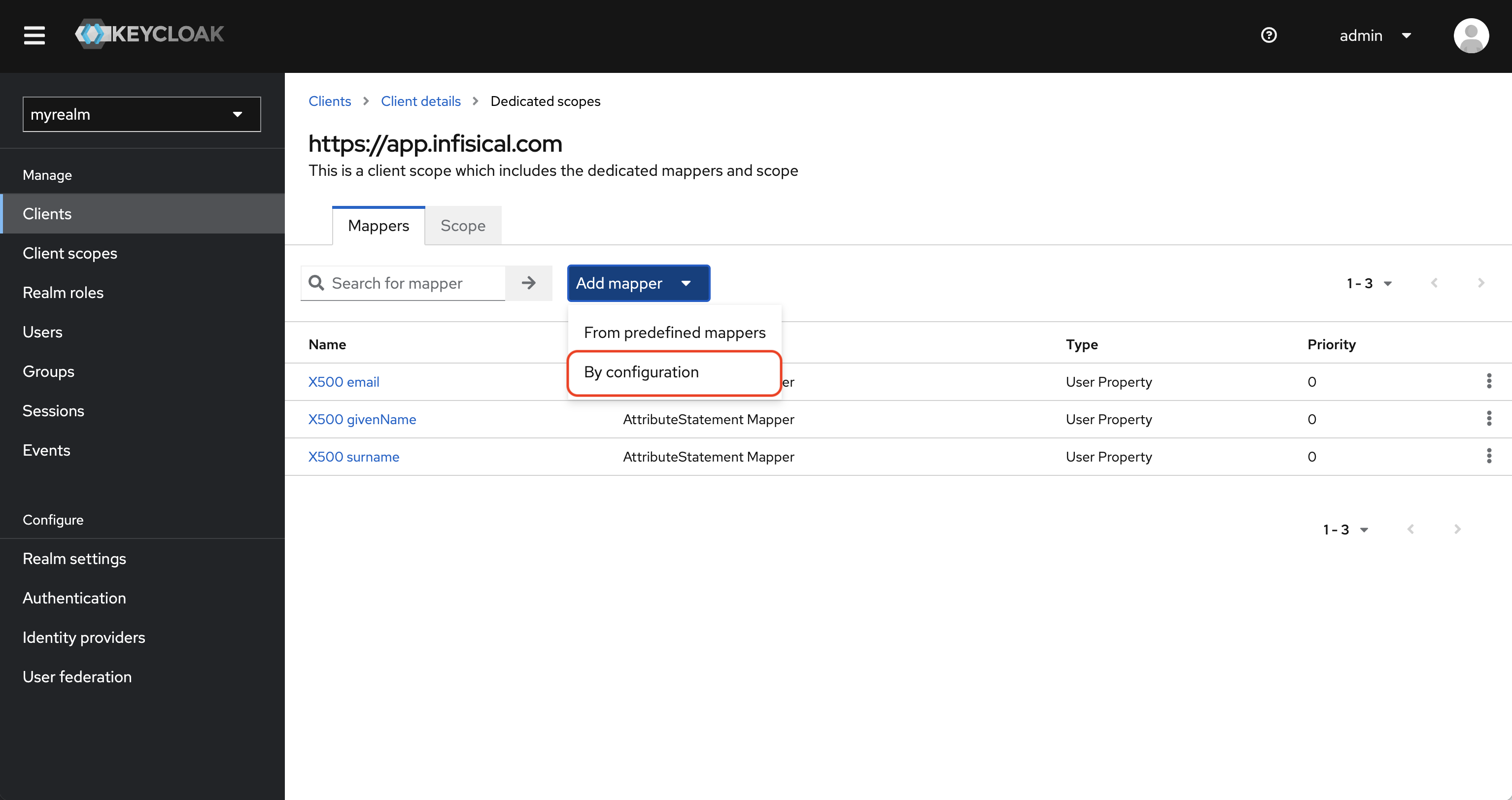
Select **User Property**.
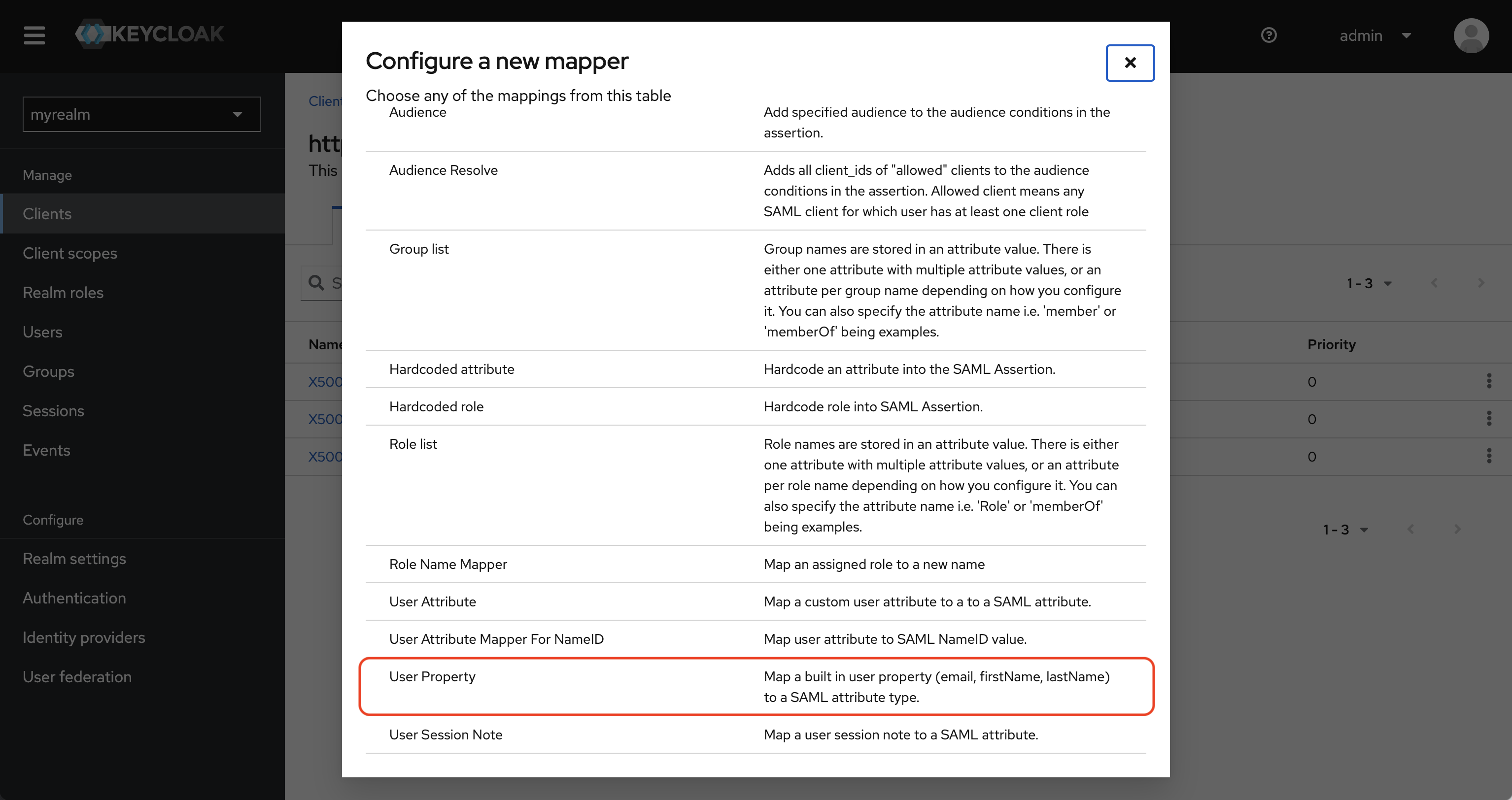
Set the the **Name** field to **Username**, the **Property** field to **username**, and the **SAML Attribtue Name** to **username**.
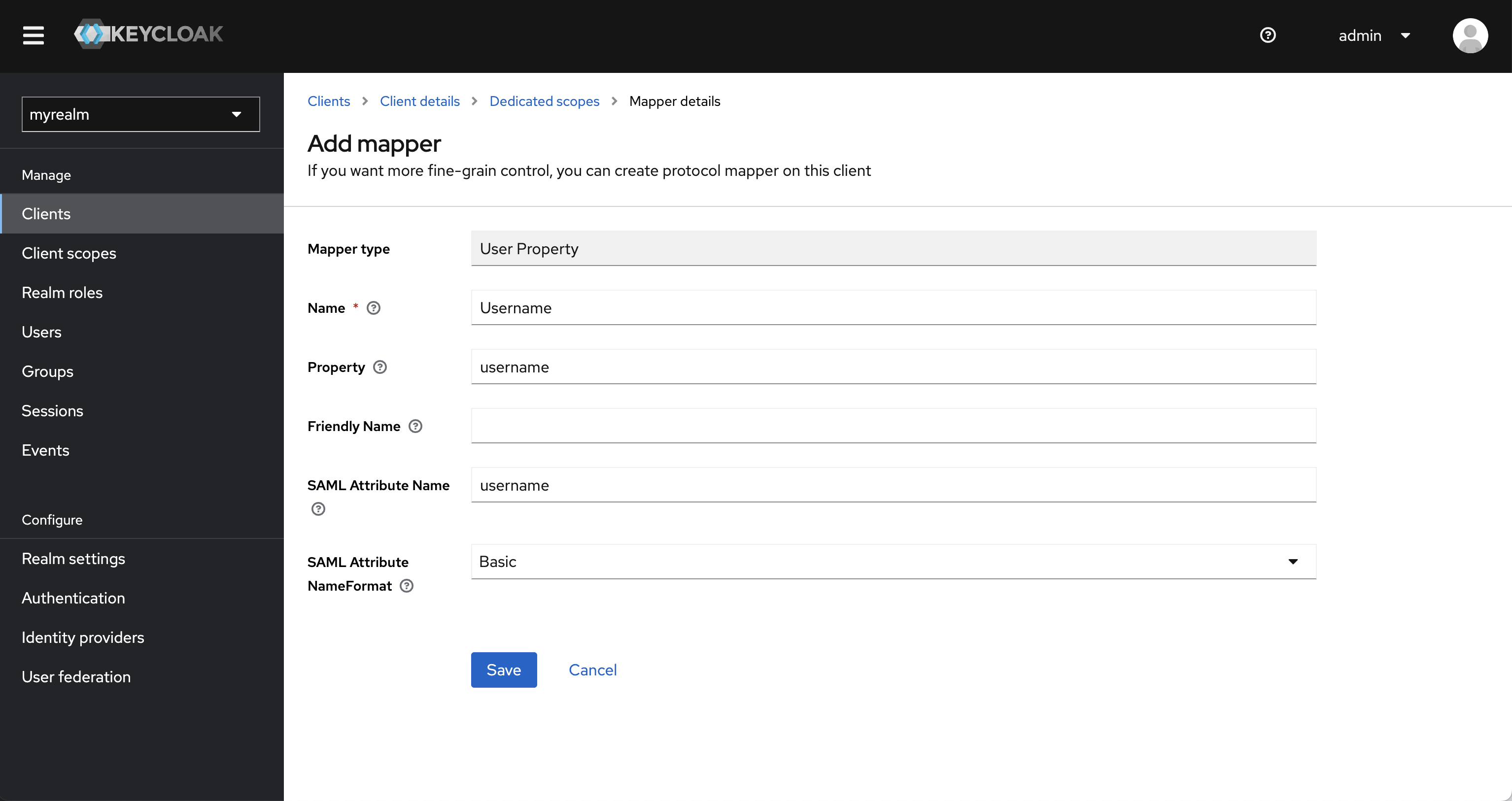
Repeat the same for the `id` attribute, setting the **Name** field to **ID**, the **Property** field to **id**, and the **SAML Attribute Name** to **id**.
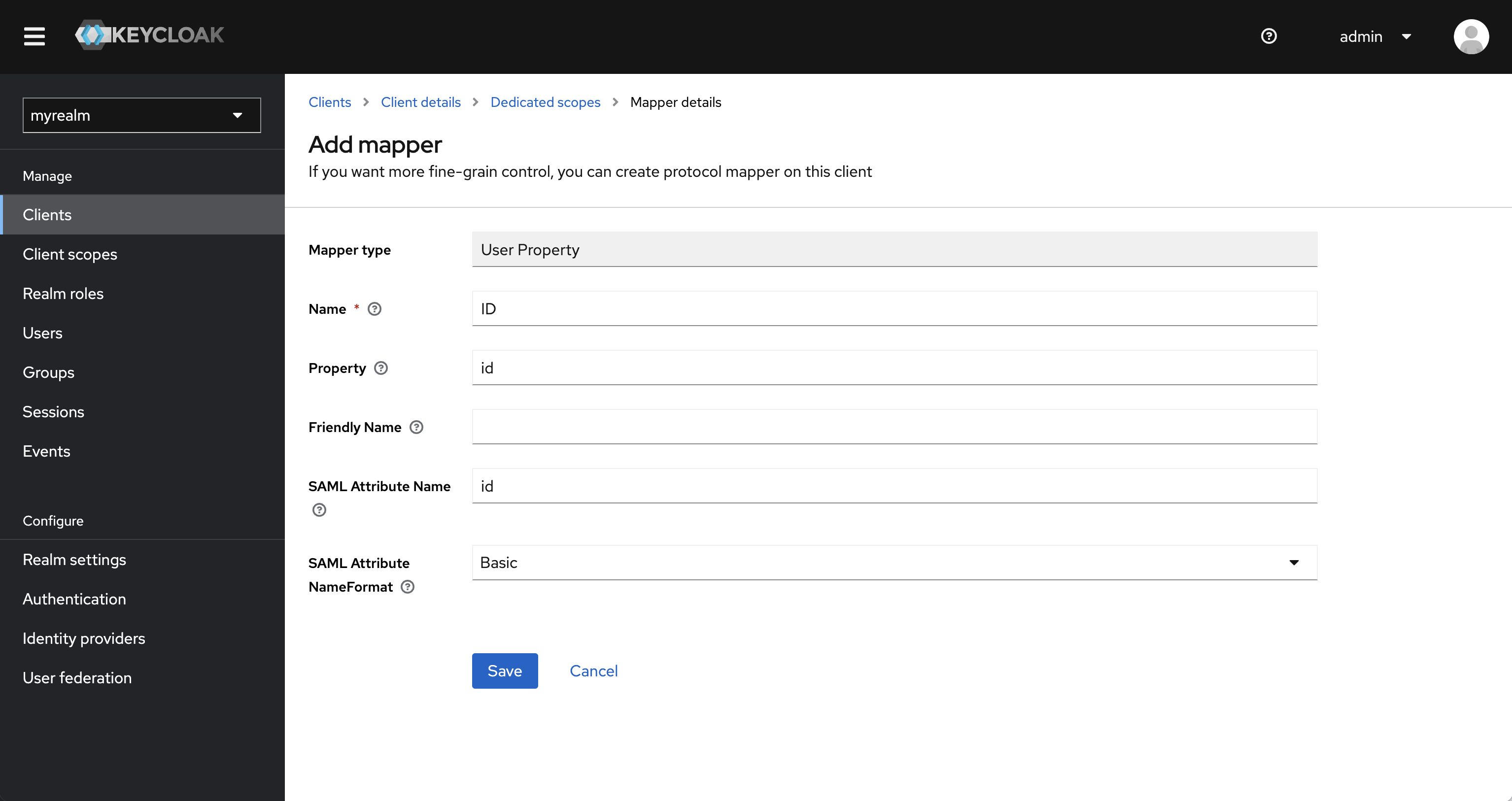
Once you've completed the above steps, the list of mappers should look like this:
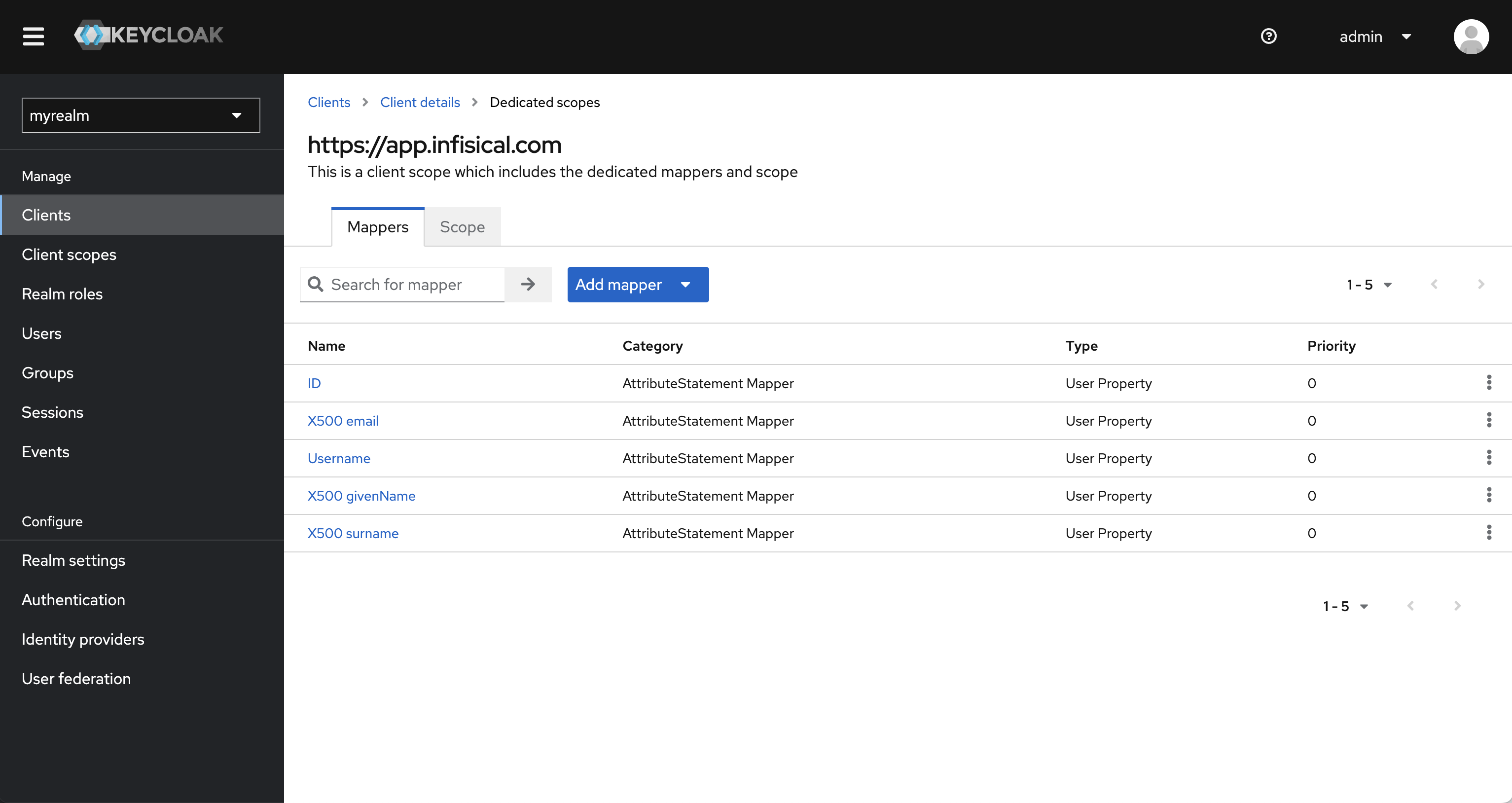
Back in Keycloak, navigate to Configure > Realm settings > General tab > Endpoints > SAML 2.0 Identity Provider Metadata and copy the IDP URL. This should appear in various places and take the form: `https://keycloak-mysite.com/realms/myrealm/protocol/saml`.
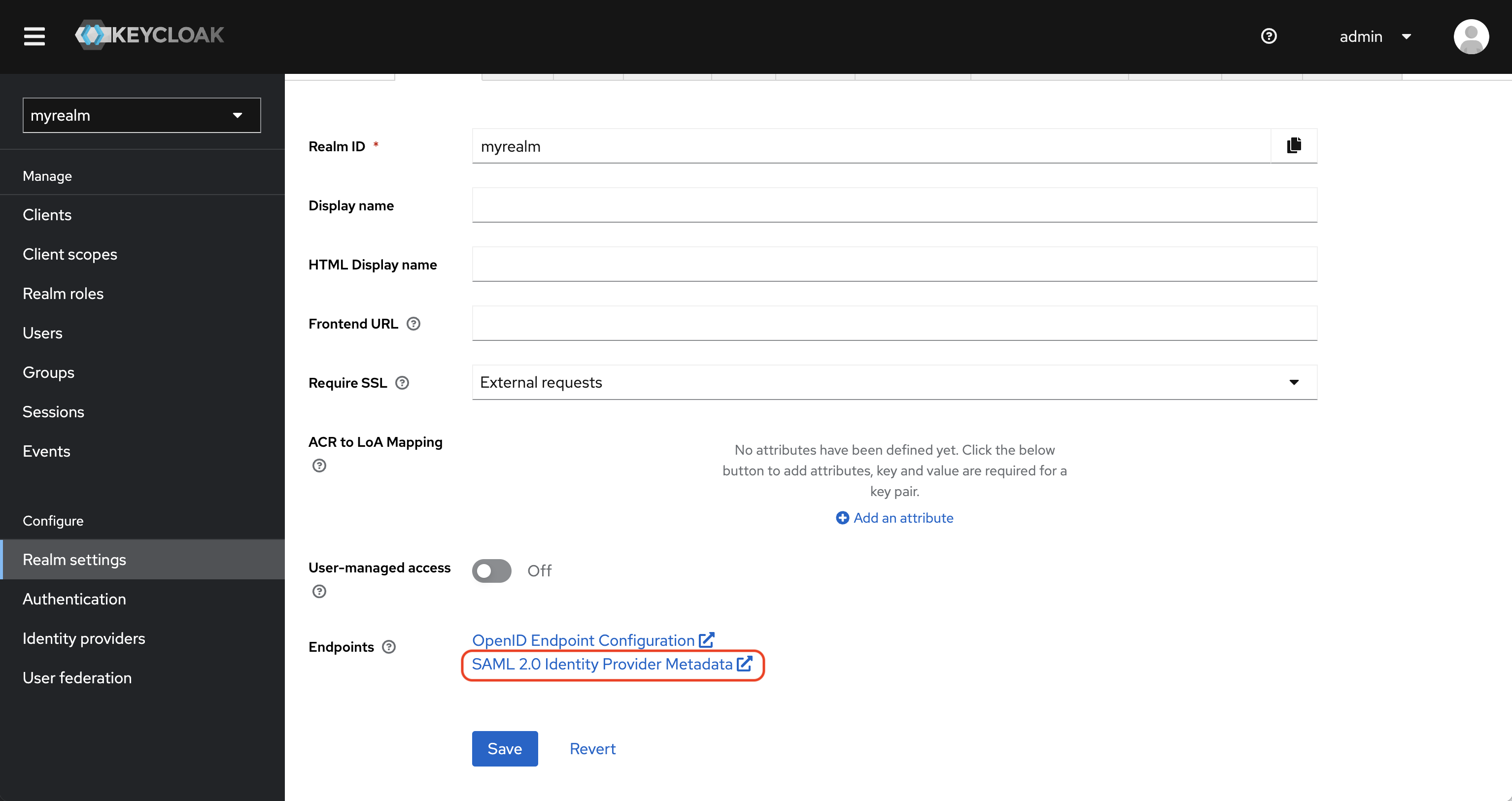
Also, in the **Keys** tab, locate the RS256 key and copy the certificate to use when finishing configuring Keycloak SAML in Infisical.
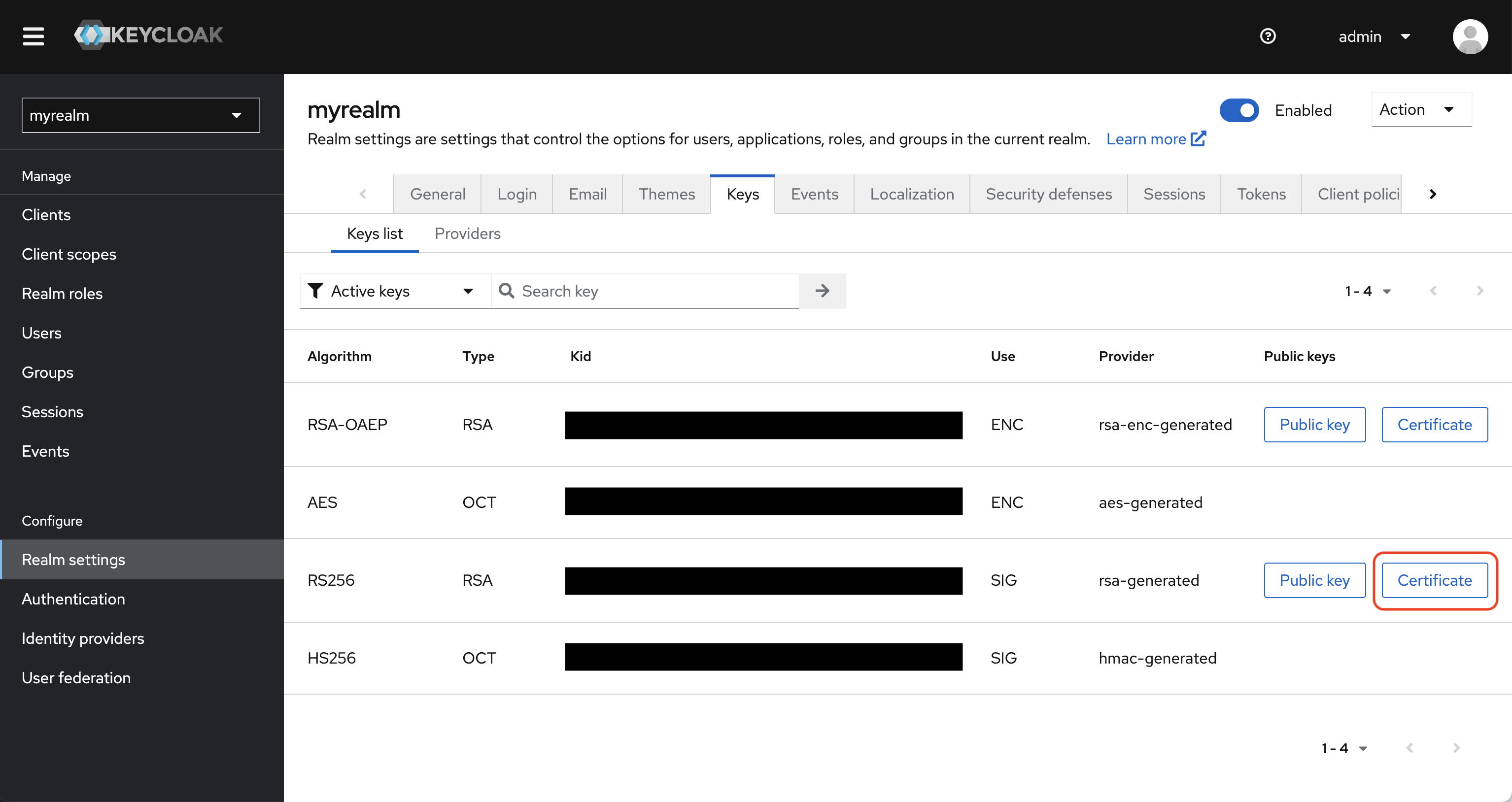
Back in Infisical, set **IDP URL** and **Certificate** to the items from step 3. Also, set the **Client ID** to the `https://app.infisical.com`.
Once you've done that, press **Update** to complete the required configuration.
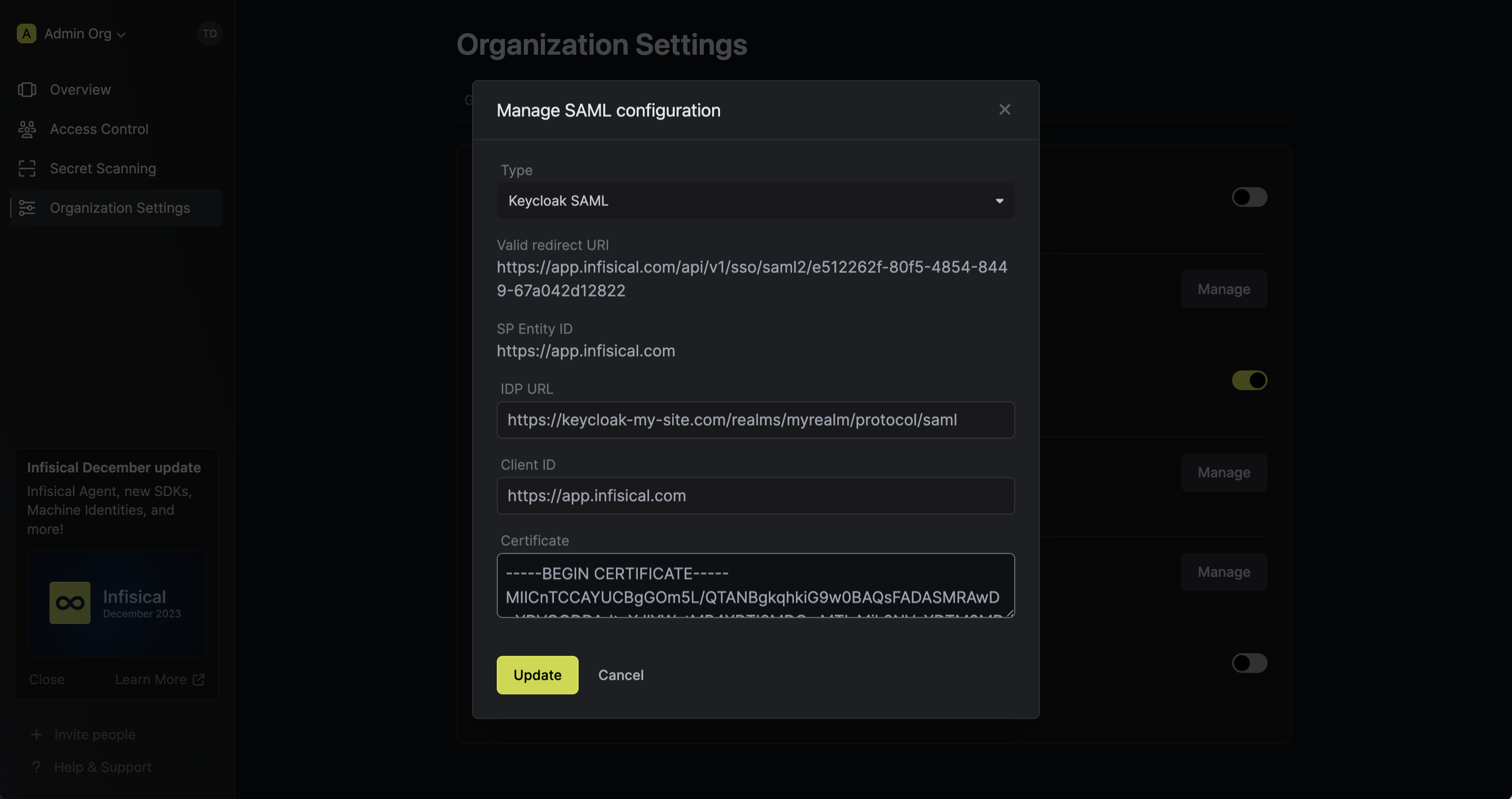
Enabling SAML SSO allows members in your organization to log into Infisical via Keycloak.
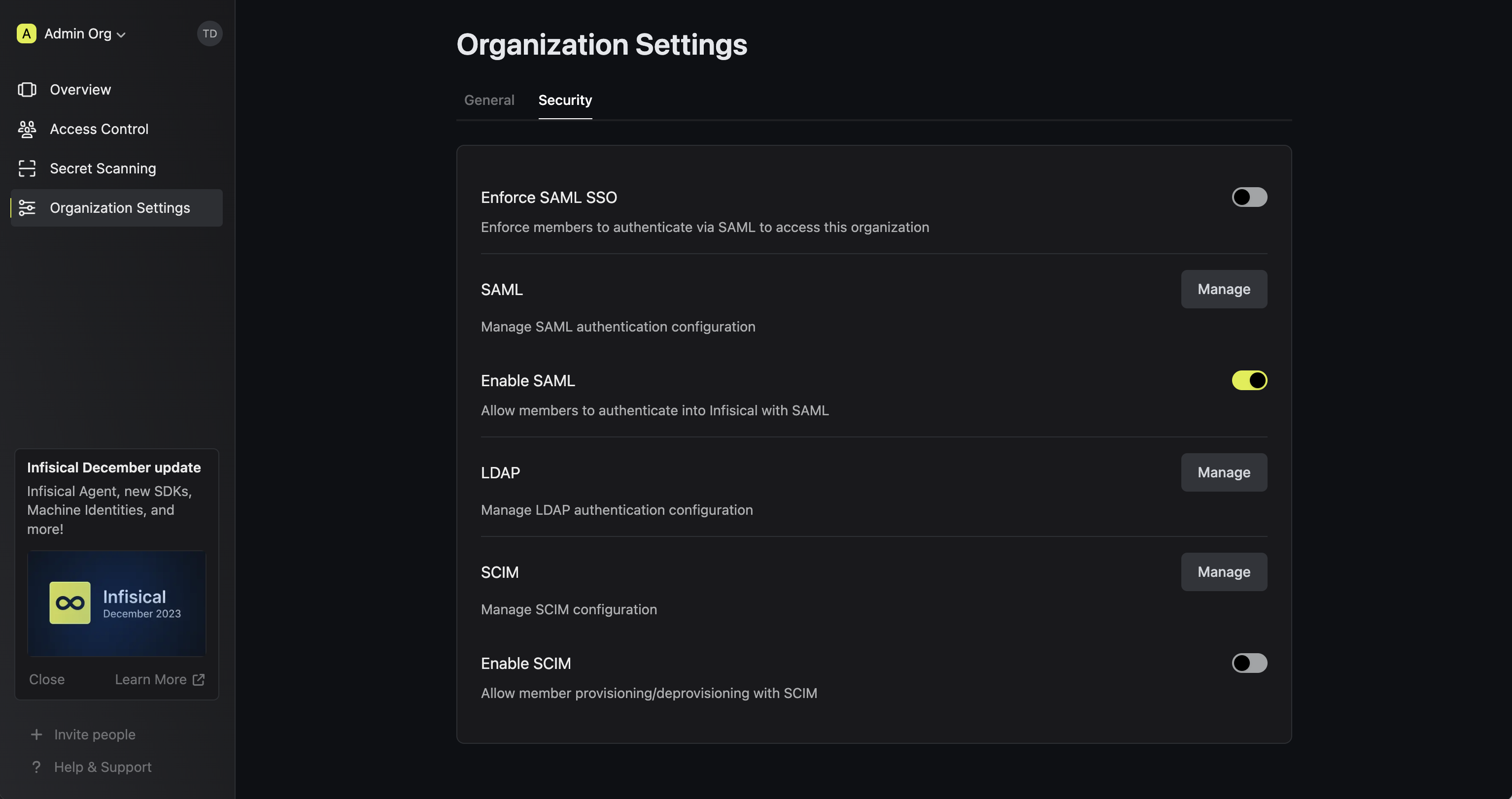
Enforcing SAML SSO ensures that members in your organization can only access Infisical
by logging into the organization via Keycloak.
To enforce SAML SSO, you're required to test out the SAML connection by successfully authenticating at least one Keycloak user with Infisical;
Once you've completed this requirement, you can toggle the **Enforce SAML SSO** button to enforce SAML SSO.
We recommend ensuring that your account is provisioned the application in Keycloak
prior to enforcing SAML SSO to prevent any unintended issues.
If you are only using one organization on your Infisical instance, you can configure a default organization in the [Server Admin Console](../admin-panel/server-admin#default-organization) to expedite SAML login.
If you're configuring SAML SSO on a self-hosted instance of Infisical, make
sure to set the `AUTH_SECRET` and `SITE_URL` environment variable for it to
work:
* `AUTH_SECRET`: A secret key used for signing and verifying JWT. This
can be a random 32-byte base64 string generated with `openssl rand -base64
32`.
* `SITE_URL`: The absolute URL of your self-hosted instance of Infisical including the protocol (e.g. [https://app.infisical.com](https://app.infisical.com))
# Okta SAML
Learn how to configure Okta SAML 2.0 for Infisical SSO.
Okta SAML SSO is a paid feature. If you're using Infisical Cloud, then it is
available under the **Pro Tier**. If you're self-hosting Infisical, then you
should contact [sales@infisical.com](mailto:sales@infisical.com) to purchase an enterprise license to use
it.
In Infisical, head to your Organization Settings > Authentication > SAML SSO Configuration and select **Set up SAML SSO**.
Next, copy the **Single sign-on URL** and **Audience URI (SP Entity ID)** to use when configuring the Okta SAML 2.0 application.
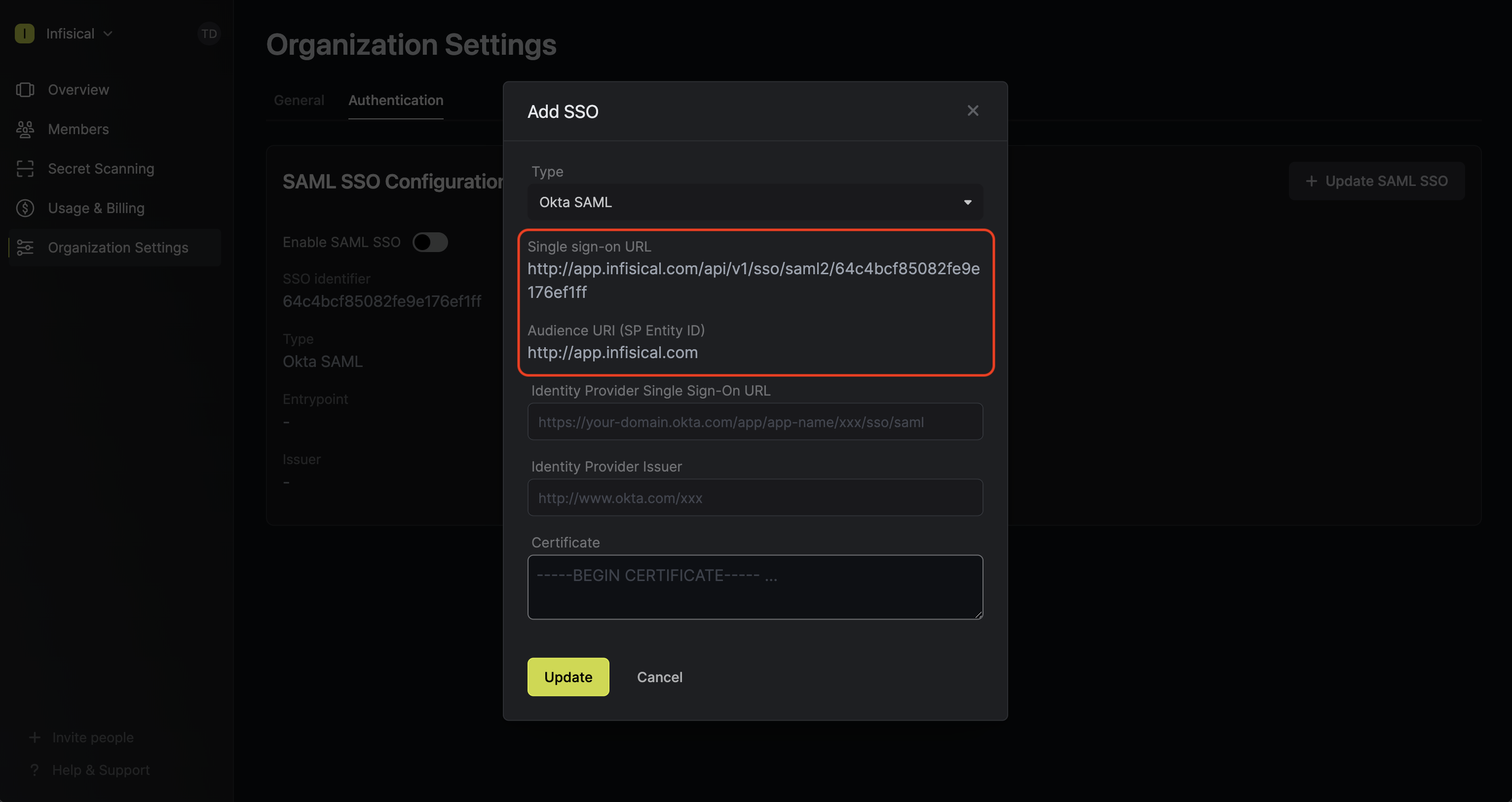
In the Okta Admin Portal, select Applications > Applications from the navigation. On the Applications screen, select the **Create App Integration**
button.
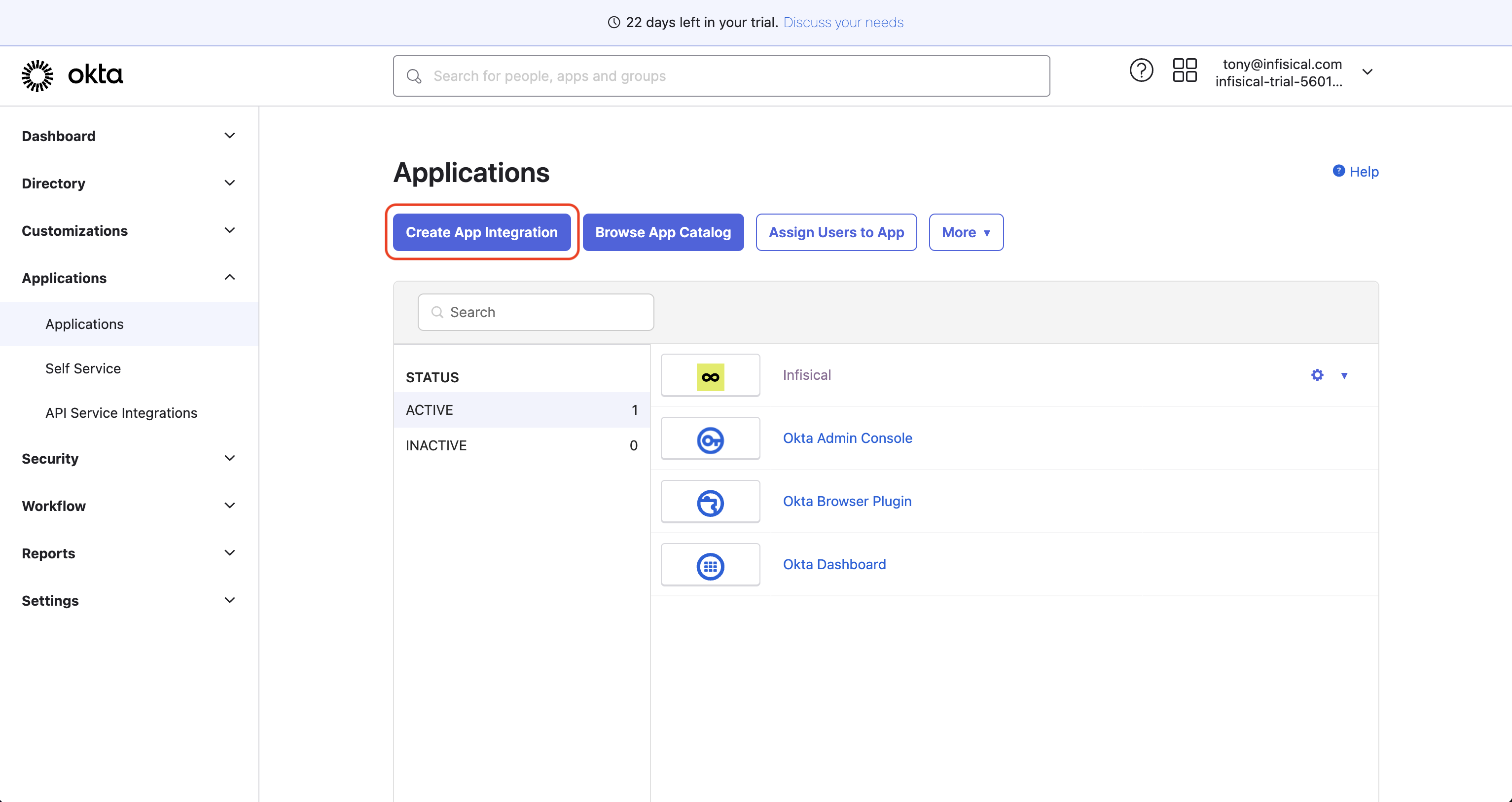
In the Create a New Application Integration dialog, select the **SAML 2.0** radio button:
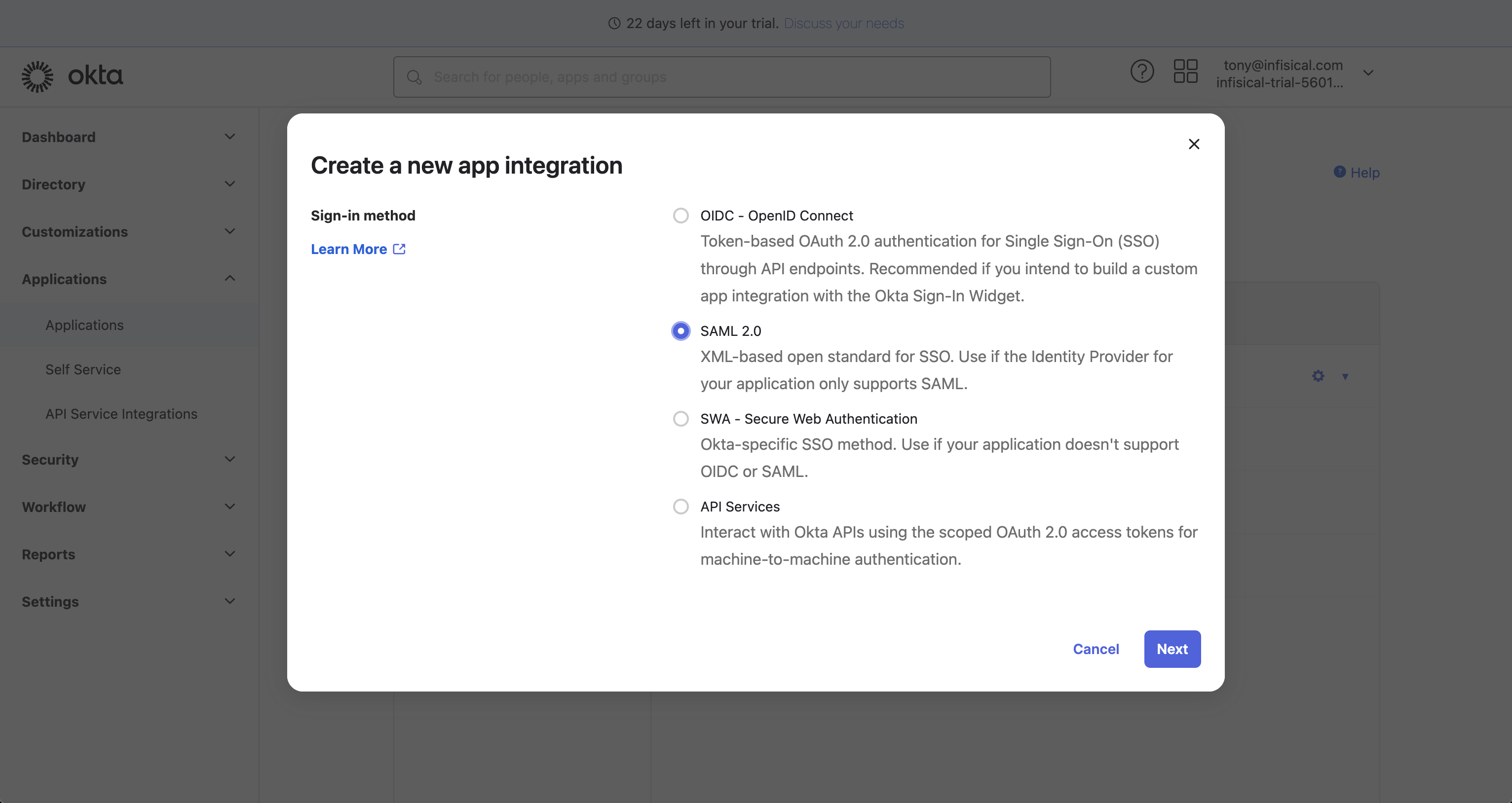
On the General Settings screen, give the application a unique name like Infisical and select **Next**.
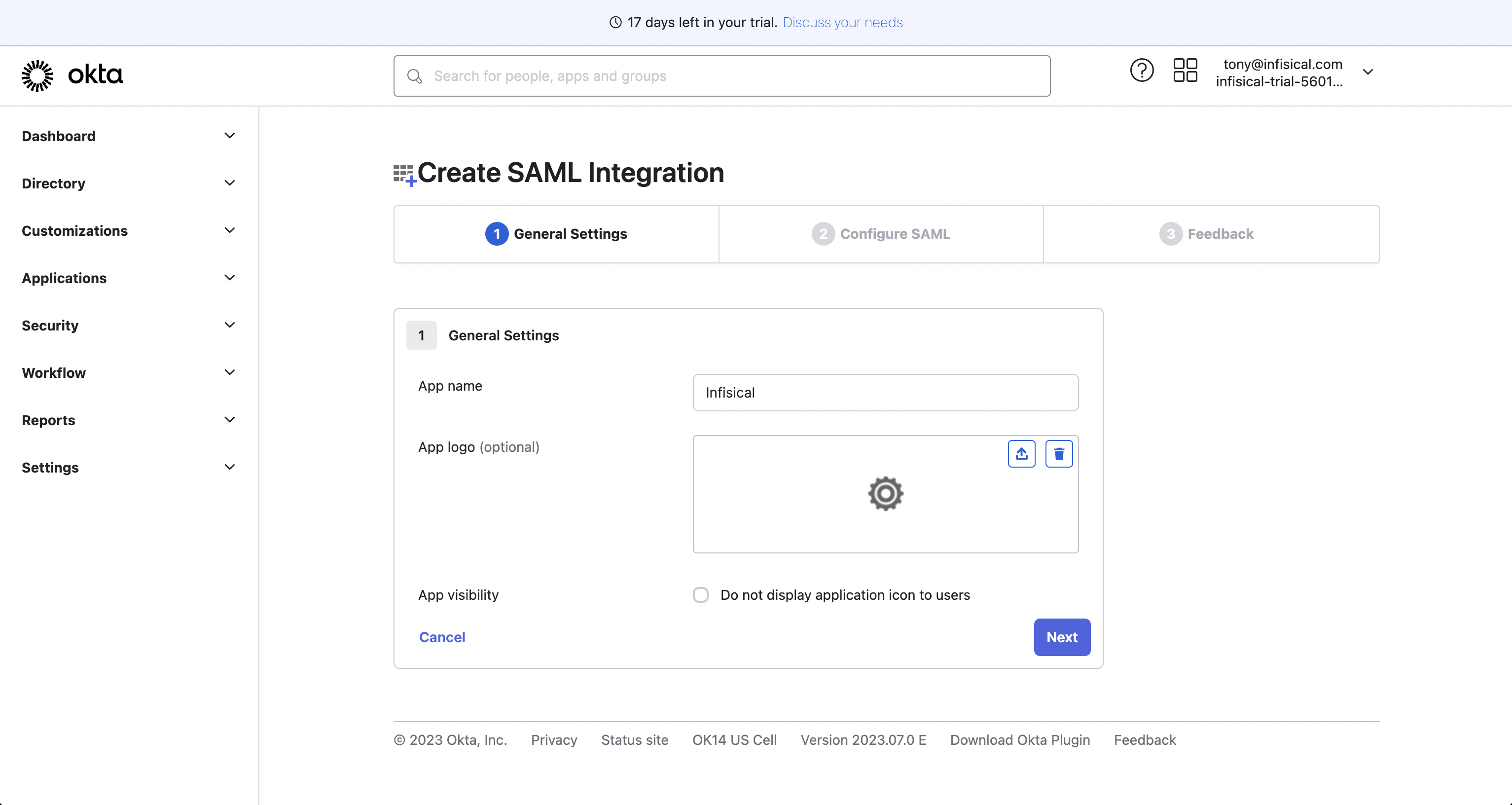
On the Configure SAML screen, set the **Single sign-on URL** and **Audience URI (SP Entity ID)** from step 1.
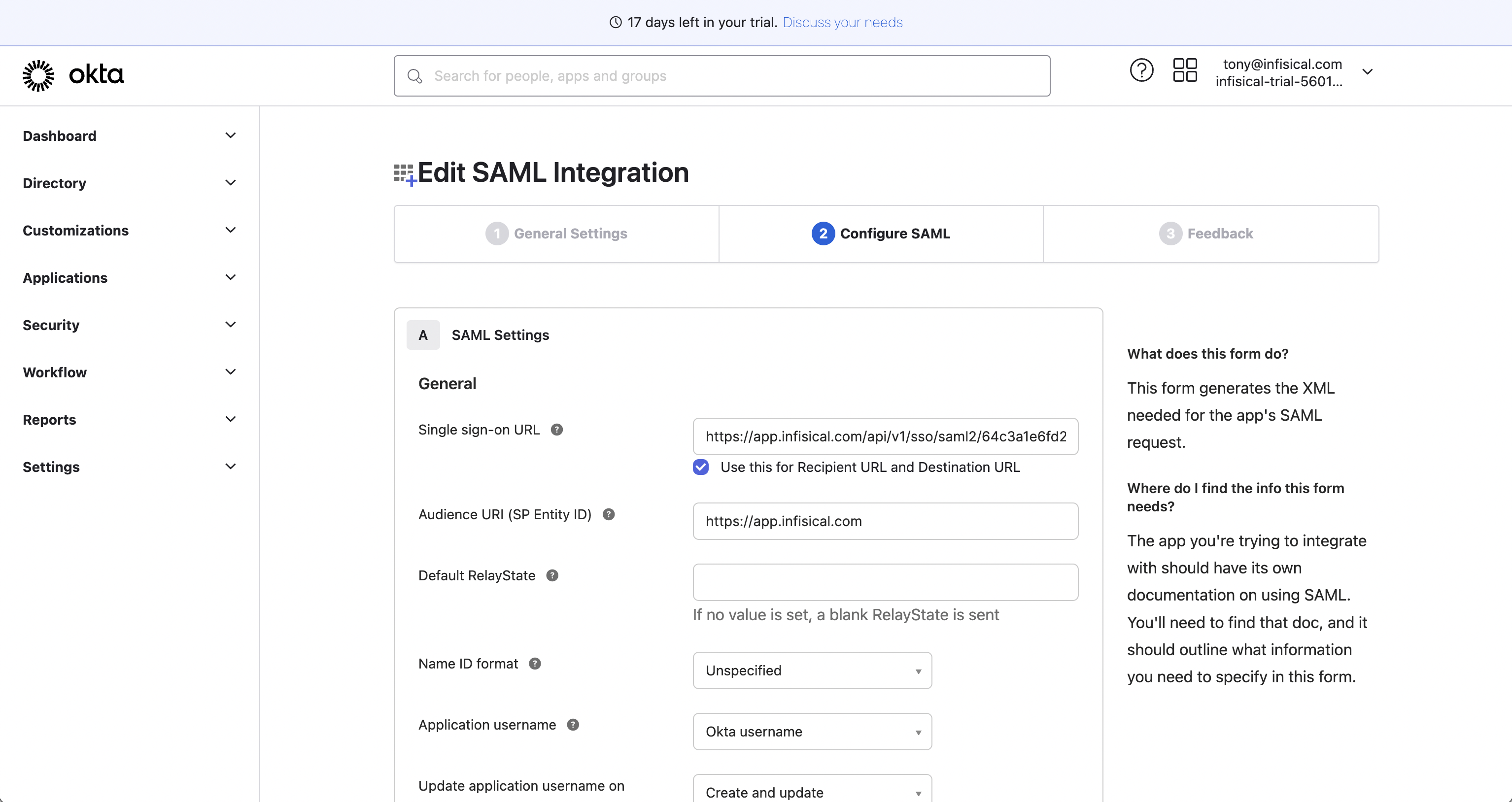
If you're self-hosting Infisical, then you will want to replace
`https://app.infisical.com` with your own domain.
Also on the Configure SAML screen, configure the **Attribute Statements** to map:
* `id -> user.id`,
* `email -> user.email`,
* `firstName -> user.firstName`
* `lastName -> user.lastName`
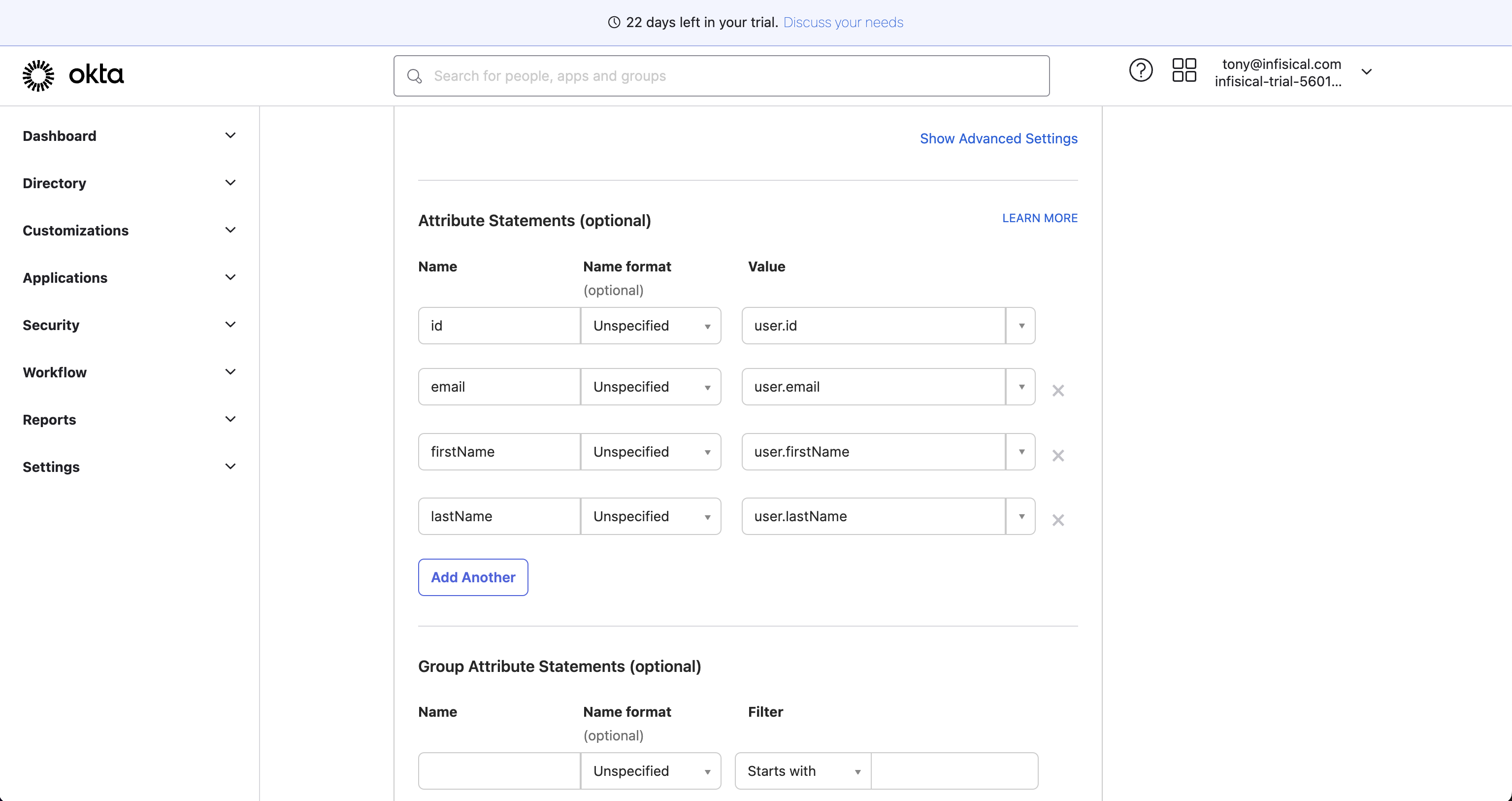
Once configured, select **Next** to proceed to the Feedback screen and select **Finish**.
Once your application is created, select the **Sign On** tab for the app and select the **View Setup Instructions** button located on the right side of the screen:
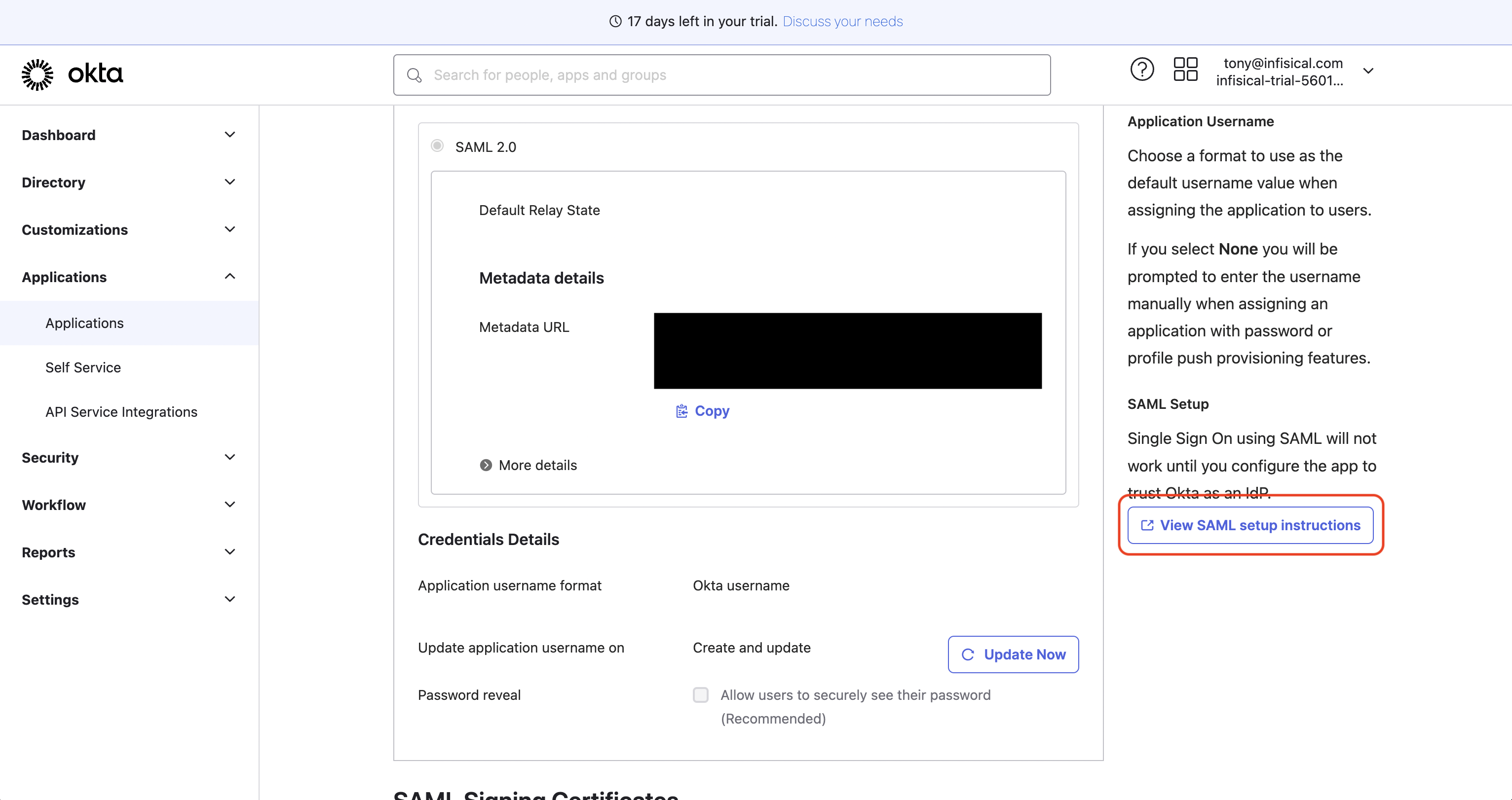
Copy the **Identity Provider Single Sign-On URL**, the **Identity Provider Issuer**, and the **X.509 Certificate** to use when finishing configuring Okta SAML in Infisical.
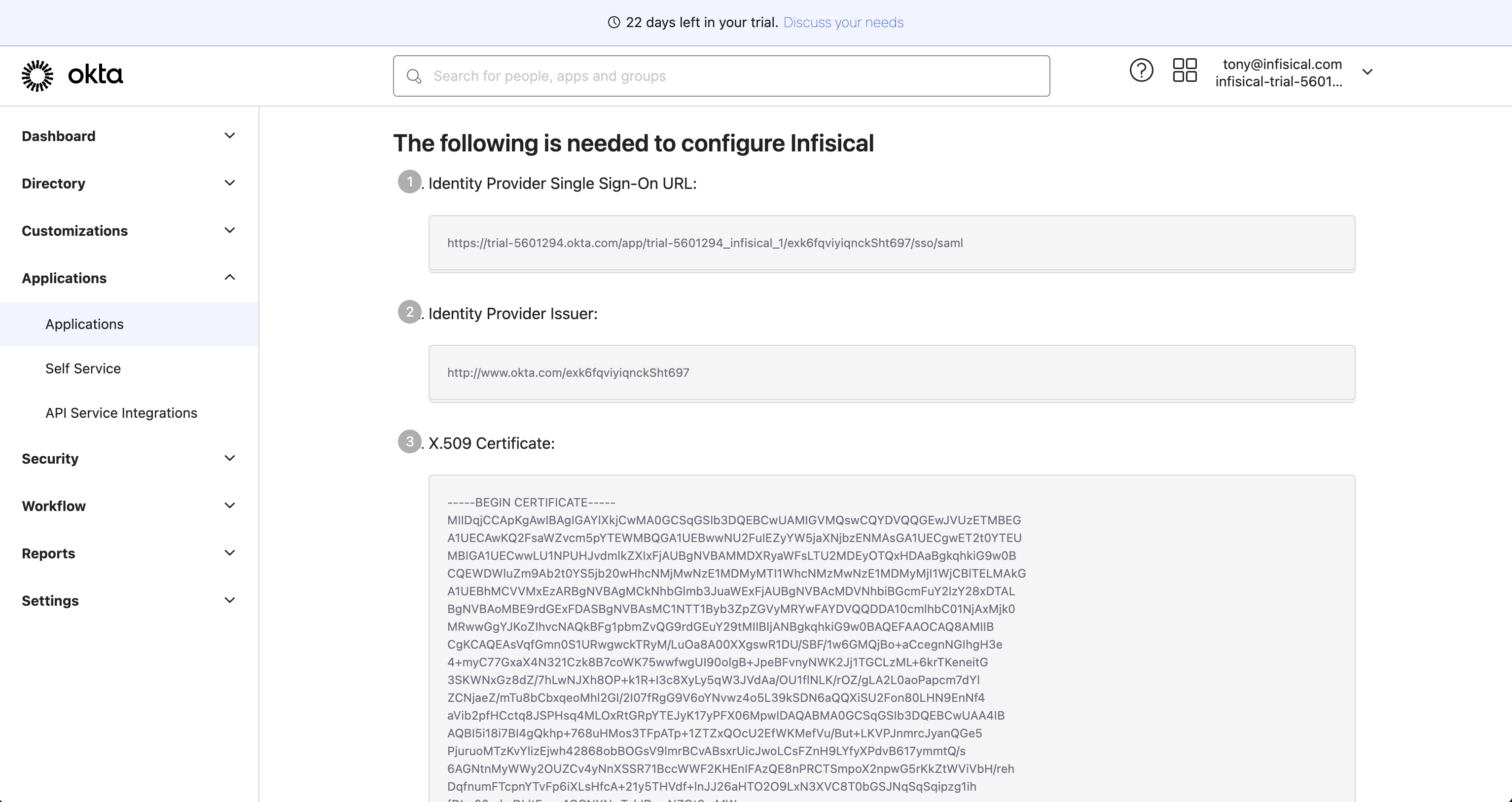
Back in Infisical, set **Identity Provider Single Sign-On URL**, **Identity Provider Issuer**,
and **Certificate** to **X.509 Certificate** from step 3. Once you've done that, press **Update** to complete the required configuration.
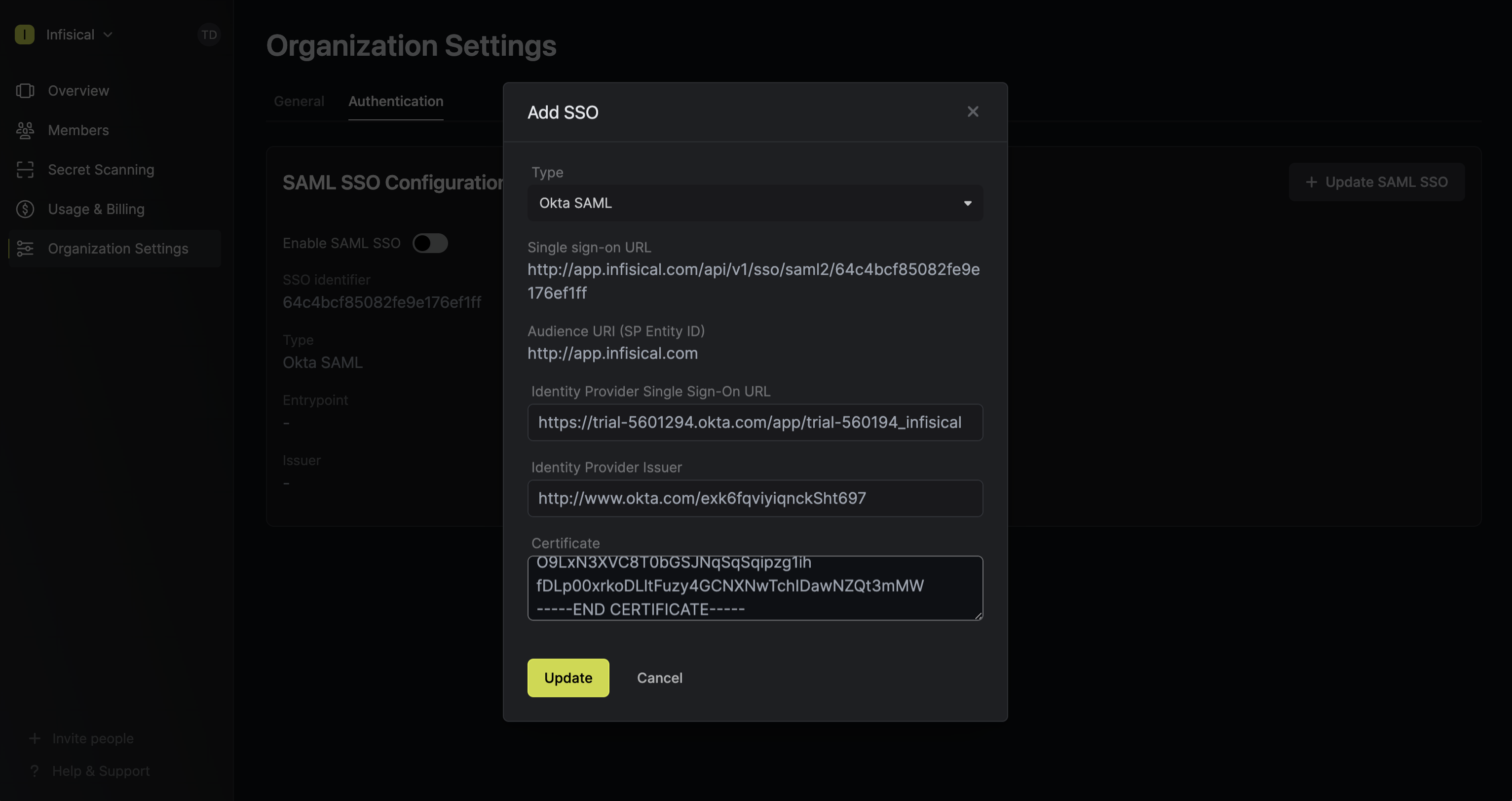
Back in Okta, navigate to the **Assignments** tab and select **Assign**. You can assign access to the application on a user-by-user basis using the Assign to People option, or in-bulk using the Assign to Groups option.
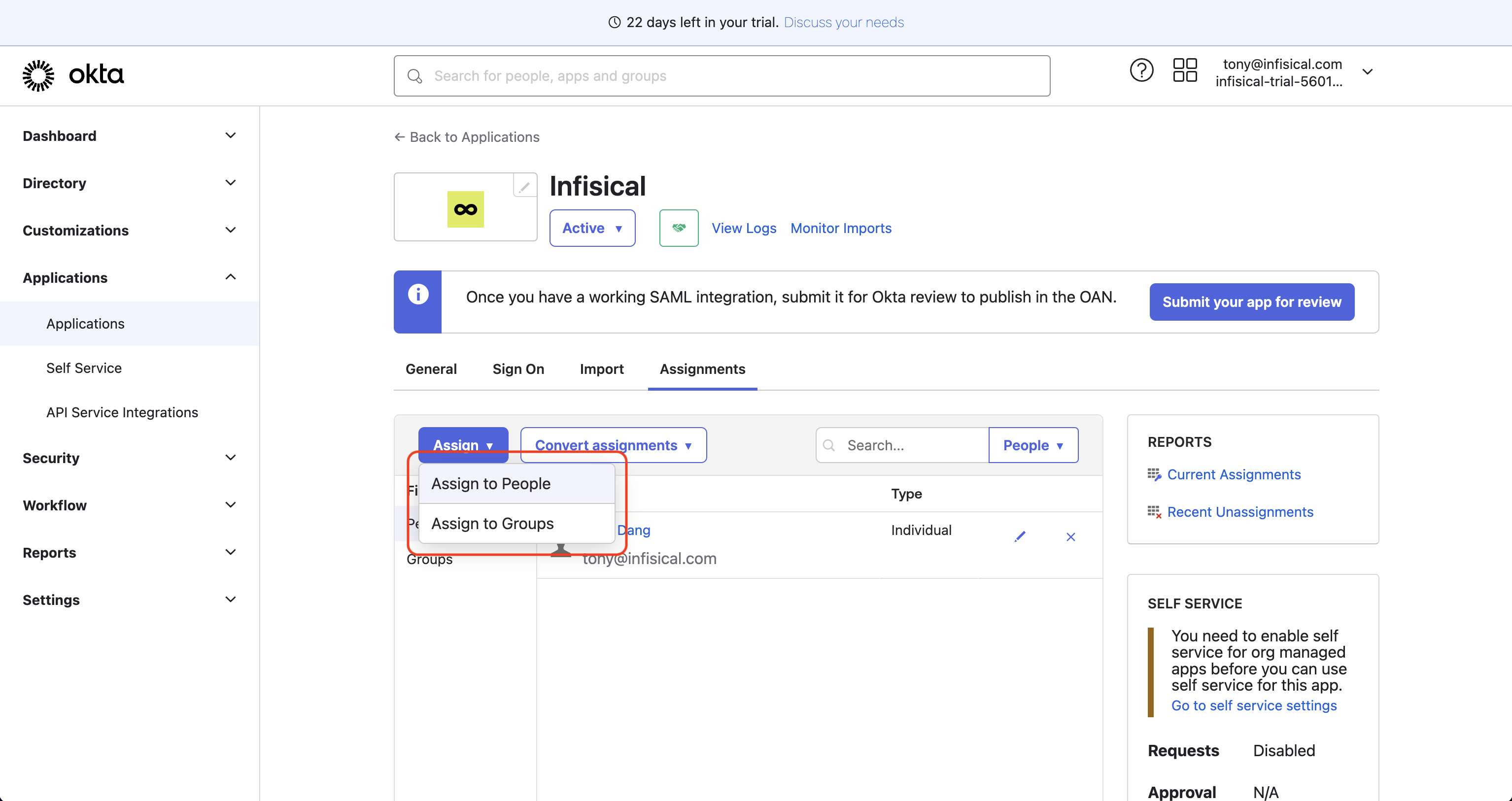
At this point, you have configured everything you need within the context of the Okta Admin Portal.
Enabling SAML SSO allows members in your organization to log into Infisical via Okta.
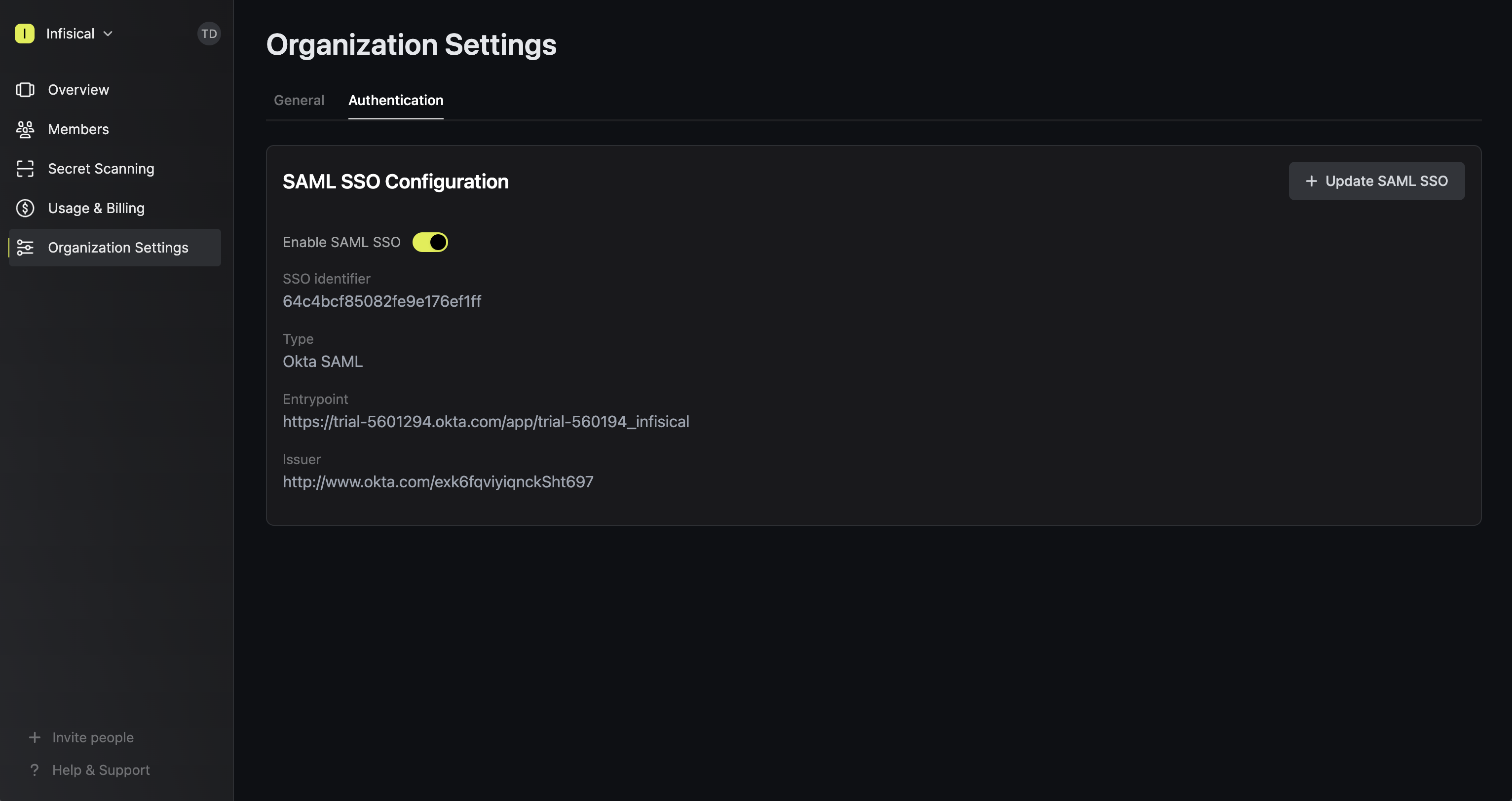
Enforcing SAML SSO ensures that members in your organization can only access Infisical
by logging into the organization via Okta.
To enforce SAML SSO, you're required to test out the SAML connection by successfully authenticating at least one Okta user with Infisical;
Once you've completed this requirement, you can toggle the **Enforce SAML SSO** button to enforce SAML SSO.
We recommend ensuring that your account is provisioned the application in Okta
prior to enforcing SAML SSO to prevent any unintended issues.
If you are only using one organization on your Infisical instance, you can configure a default organization in the [Server Admin Console](../admin-panel/server-admin#default-organization) to expedite SAML login.
If you're configuring SAML SSO on a self-hosted instance of Infisical, make
sure to set the `AUTH_SECRET` and `SITE_URL` environment variable for it to
work:
* `AUTH_SECRET`: A secret key used for signing and verifying JWT. This
can be a random 32-byte base64 string generated with `openssl rand -base64
32`.
* `SITE_URL`: The absolute URL of your self-hosted instance of Infisical including the protocol (e.g. [https://app.infisical.com](https://app.infisical.com))
# SSO Overview
Learn how to log in to Infisical via SSO protocols.
Infisical offers Google SSO and GitHub SSO for free across both Infisical
Cloud and Infisical Self-hosted. Infisical also offers SAML SSO authentication
and OpenID Connect (OIDC) but as paid features that can be unlocked on
Infisical Cloud's **Pro** tier or via enterprise license on self-hosted
instances of Infisical. On this front, we support industry-leading providers
including Okta, Azure AD, and JumpCloud; with any questions, please reach out
to [team@infisical.com](mailto:team@infisical.com).
You can configure your organization in Infisical to have members authenticate with the platform via protocols like [SAML 2.0](https://en.wikipedia.org/wiki/SAML_2.0) or [OpenID Connect](https://openid.net/specs/openid-connect-core-1_0.html).
## Identity providers
Infisical supports these and many other identity providers:
* [Google SSO](/documentation/platform/sso/google)
* [GitHub SSO](/documentation/platform/sso/github)
* [GitLab SSO](/documentation/platform/sso/gitlab)
* [Okta SAML](/documentation/platform/sso/okta)
* [Azure SAML](/documentation/platform/sso/azure)
* [JumpCloud SAML](/documentation/platform/sso/jumpcloud)
* [Keycloak SAML](/documentation/platform/sso/keycloak-saml)
* [Google SAML](/documentation/platform/sso/google-saml)
* [Keycloak OIDC](/documentation/platform/sso/keycloak-oidc)
* [Auth0 OIDC](/documentation/platform/sso/auth0-oidc)
* [General OIDC](/documentation/platform/sso/general-oidc)
If your required identity provider is not shown in the list above, please reach out to [team@infisical.com](mailto:team@infisical.com) for assistance.
## FAQ
By default, Infisical Cloud is configured to not trust emails from external
identity providers to prevent any malicious account takeover attempts via
email spoofing. Accordingly, Infisical creates a new user for anyone provisioned
through an external identity provider and requires an additional email
verification step upon their first login.
If you're running a self-hosted instance of Infisical and would like it to trust emails from external identity providers,
you can configure this behavior in the Server Admin Console.
# Service Token
Infisical service tokens allow users to programmatically interact with Infisical.
Service tokens are authentication credentials that services can use to access designated endpoints in the Infisical API to manage project resources like secrets.
Each service token can be provisioned scoped access to select environment(s) and path(s) within them.
## Service Tokens
You can manage service tokens in Access Control > Service Tokens (tab).
### Service Token (Current)
Service Token (ST) is the current widely-used authentication method for managing secrets.
Here's a few pointers to get you acquainted with it:
* When you create a ST, you get a token prefixed with `st`. The part after the last `.` delimiter is a symmetric key; everything
before it is an access token. When authenticating with the Infisical API, it is important to send in only the access token portion
of the token.
* ST supports expiration; it gets deleted automatically upon expiration.
* ST supports provisioning `read` and/or `write` permissions broadly applied to all accessible environment(s) and path(s).
* ST is not editable.
## Creating a service token
To create a service token, head to Access Control > Service Tokens as shown below and press **Create token**.
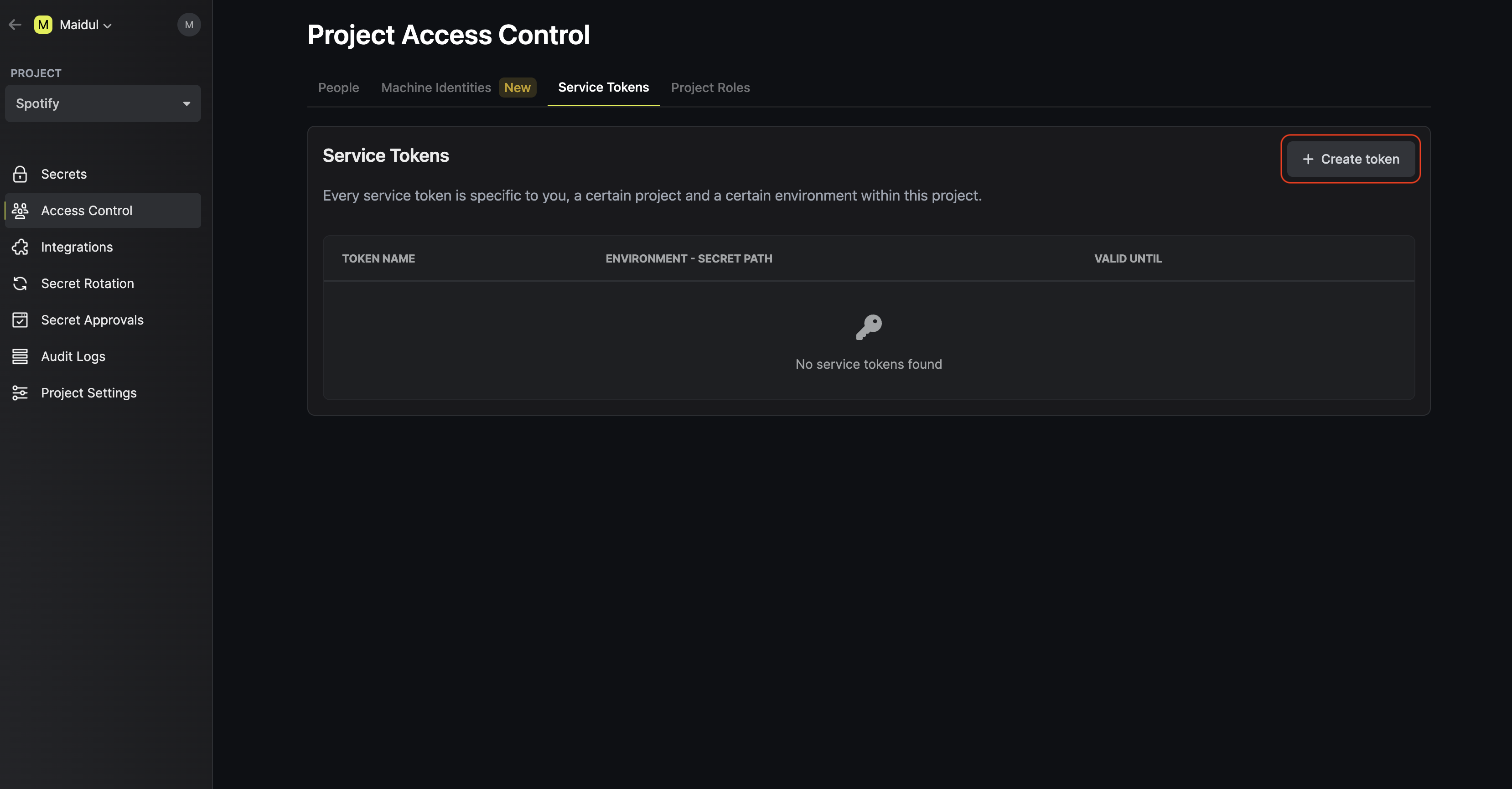
Now input any token configuration details such as which environment(s) and path(s) you'd like to provision
the token access to. Here's some guidance for each field:
* Name: A friendly name for the token.
* Scopes: The environment(s) and path(s) the token should have access to.
* Permissions: You can indicate whether or not the token should have `read/write` access to the paths.
Also, note that Infisical supports [glob patterns](https://www.malikbrowne.com/blog/a-beginners-guide-glob-patterns/) when defining access scopes to path(s).
* Expiration: The time when this token should be rendered inactive.
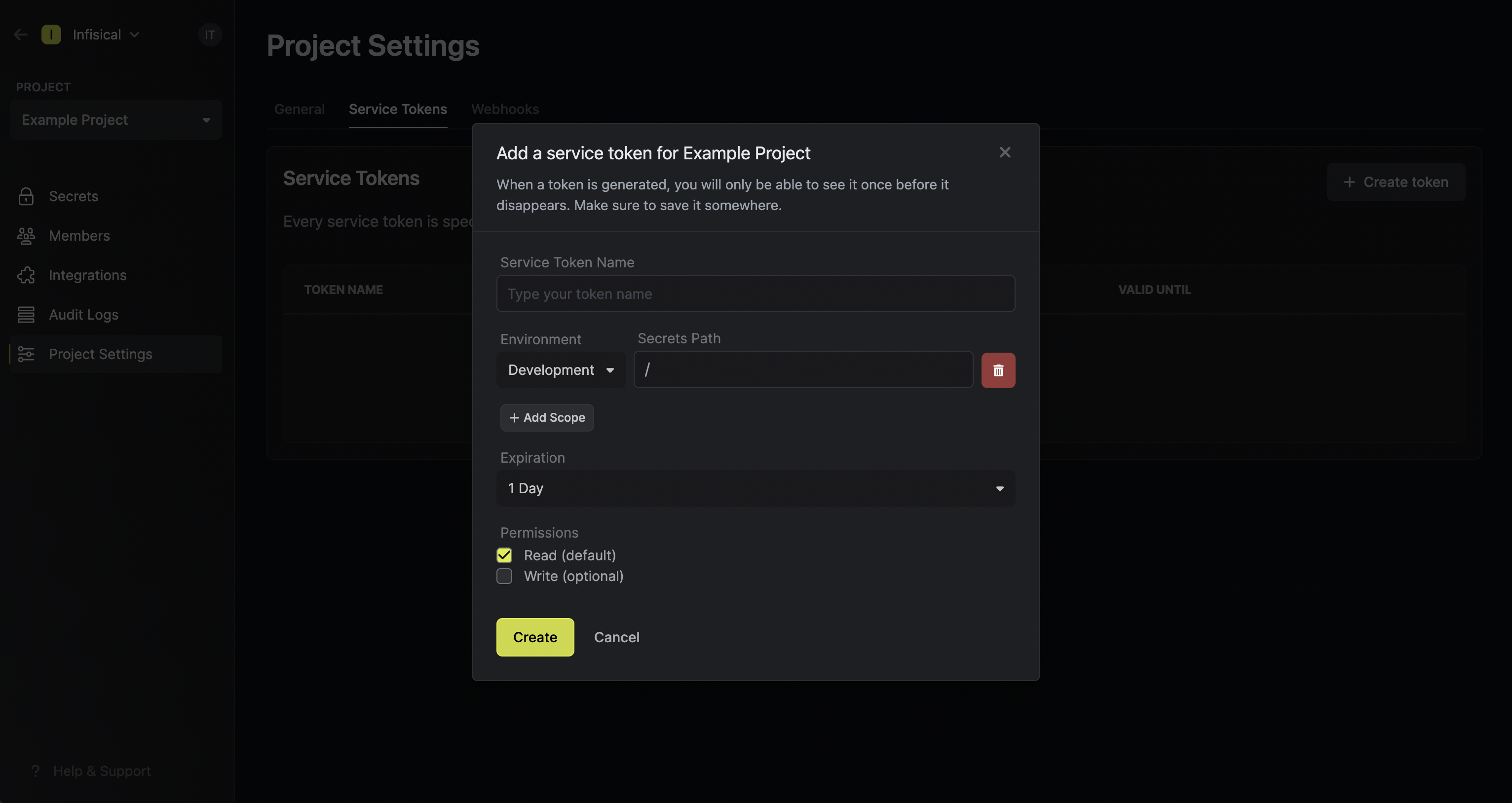
In the above screenshot, you can see that we are creating a token token with `read` access to all subfolders at any depth
of the `/common` path within the development environment of the project; the token expires in 6 months and can be used from any IP address.
For a deeper understanding of service tokens, it is recommended to read [this
guide](https://infisical.com/docs/internals/service-tokens).
**FAQ**
There are a few reasons for why this might happen:
* The service token has expired.
* The service token is insufficiently permissioned to interact with the secrets in the given environment and path.
* You are attempting to access a `/raw` secrets endpoint that requires your project to disable E2EE.
* (If using ST V3) The service token has not been activated yet.
* (If using ST V3) The service token is being used from an untrusted IP.
1. `/**`: This pattern matches all folders at any depth in the directory structure. For example, it would match folders like `/folder1/`, `/folder1/subfolder/`, and so on.
2. `/*`: This pattern matches all immediate subfolders in the current directory. It does not match any folders at a deeper level. For example, it would match folders like `/folder1/`, `/folder2/`, but not `/folder1/subfolder/`.
3. `/*/*`: This pattern matches all subfolders at a depth of two levels in the current directory. It does not match any folders at a shallower or deeper level. For example, it would match folders like `/folder1/subfolder/`, `/folder2/subfolder/`, but not `/folder1/` or `/folder1/subfolder/subsubfolder/`.
4. `/folder1/*`: This pattern matches all immediate subfolders within the `/folder1/` directory. It does not match any folders outside of `/folder1/`, nor does it match any subfolders within those immediate subfolders. For example, it would match folders like `/folder1/subfolder1/`, `/folder1/subfolder2/`, but not `/folder2/subfolder/`.
# Webhooks
Learn the fundamentals of Infisical webhooks.
Webhooks can be used to trigger changes to your integrations when secrets are modified, providing smooth integration with other third-party applications.
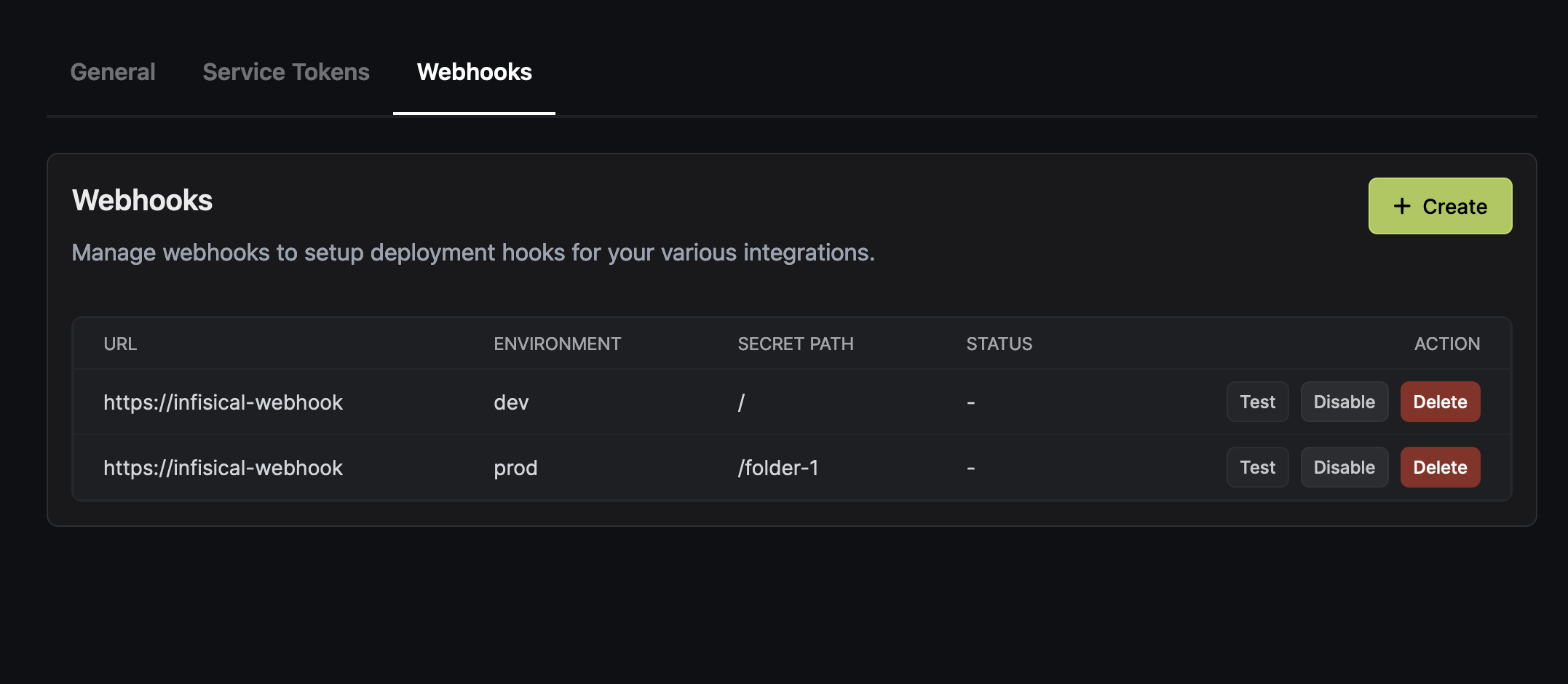
To create a webhook for a particular project, go to `Project Settings > Webhooks`.
Infisical supports two webhook types - General and Slack. If you need to integrate with Slack, use the Slack type with an [Incoming Webhook](https://api.slack.com/messaging/webhooks). When creating a webhook, you can specify an environment and folder path to trigger only specific integrations.
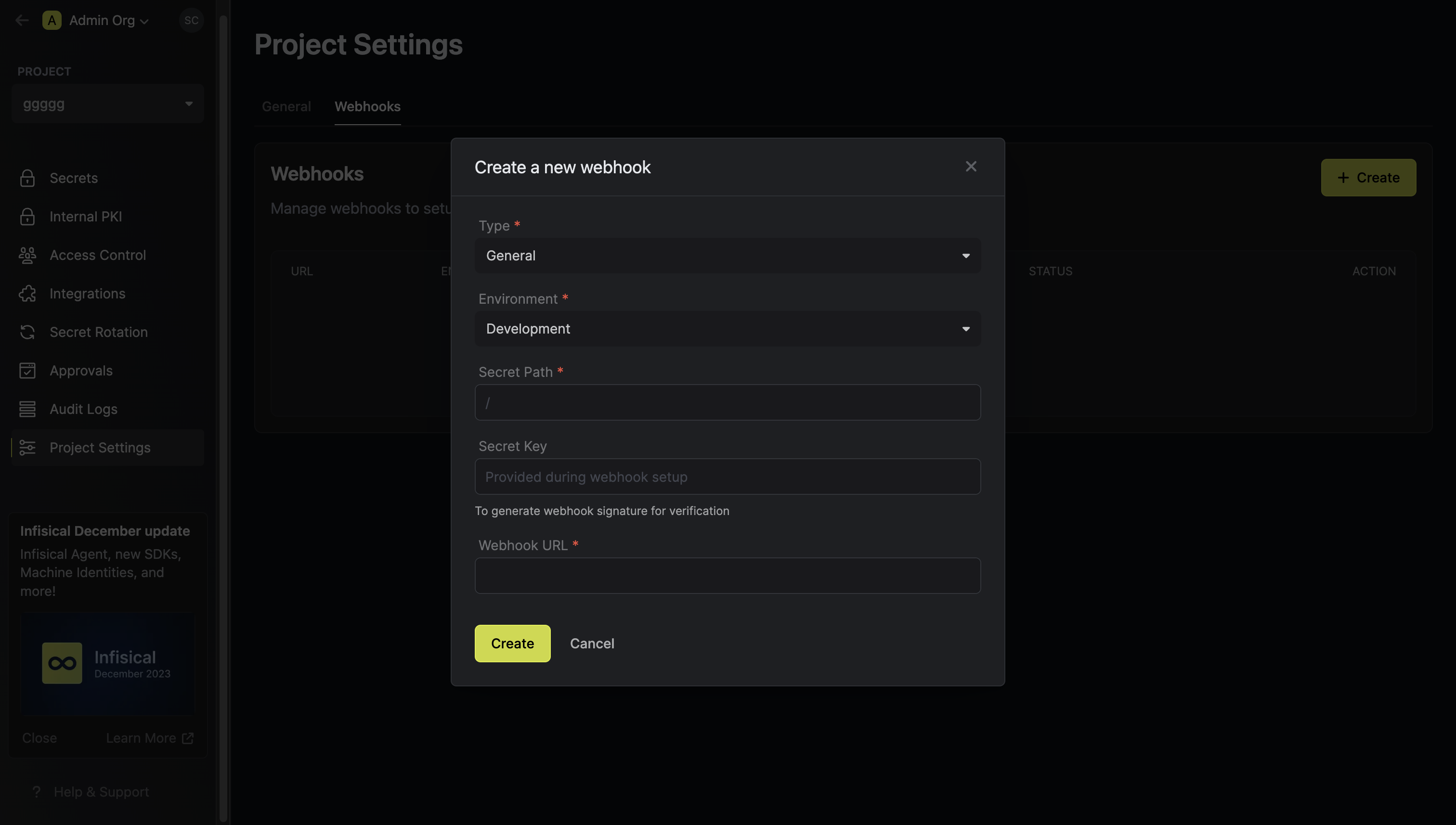
## Secret Key Verification
A secret key is a way for users to verify that a webhook request was sent by Infisical and is intended for the correct integration.
When you provide a secret key, Infisical will sign the payload of the webhook request using the key and attach a header called `x-infisical-signature` to the request with a payload.
The header will be in the format `t=;`. You can then generate the signature yourself by generating a SHA256 hash of the payload with the secret key that you know.
If the signature in the header matches the signature that you generated, then you can be sure that the request was sent by Infisical and is intended for your integration. The timestamp in the header ensures that the request is not replayed.
### Webhook Payload Format
```json
{
"event": "secret.modified",
"project": {
"workspaceId": "the workspace id",
"environment": "project environment",
"secretPath": "project folder path"
},
"timestamp": ""
}
```
# Slack integration
Learn how to setup Slack integration
This guide will provide step by step instructions on how to configure Slack integration for your Infisical projects.
## Setting up Slack integration in your projects
### Create Slack workflow integration
In order to use Slack integration in your projects, you will first have to
configure a Slack workflow integration in your organization.
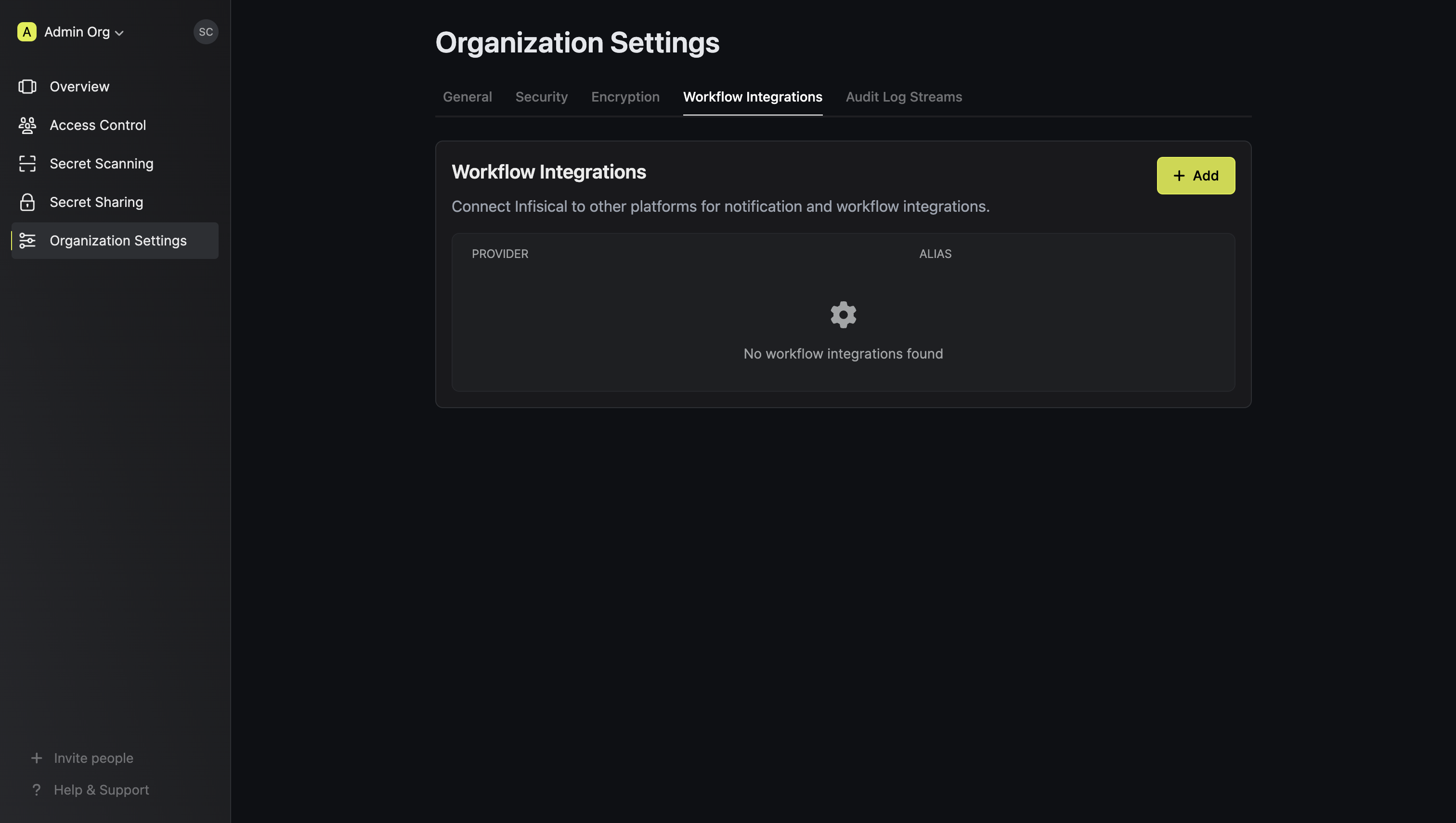
Press "Add" and select "Slack" as the platform.
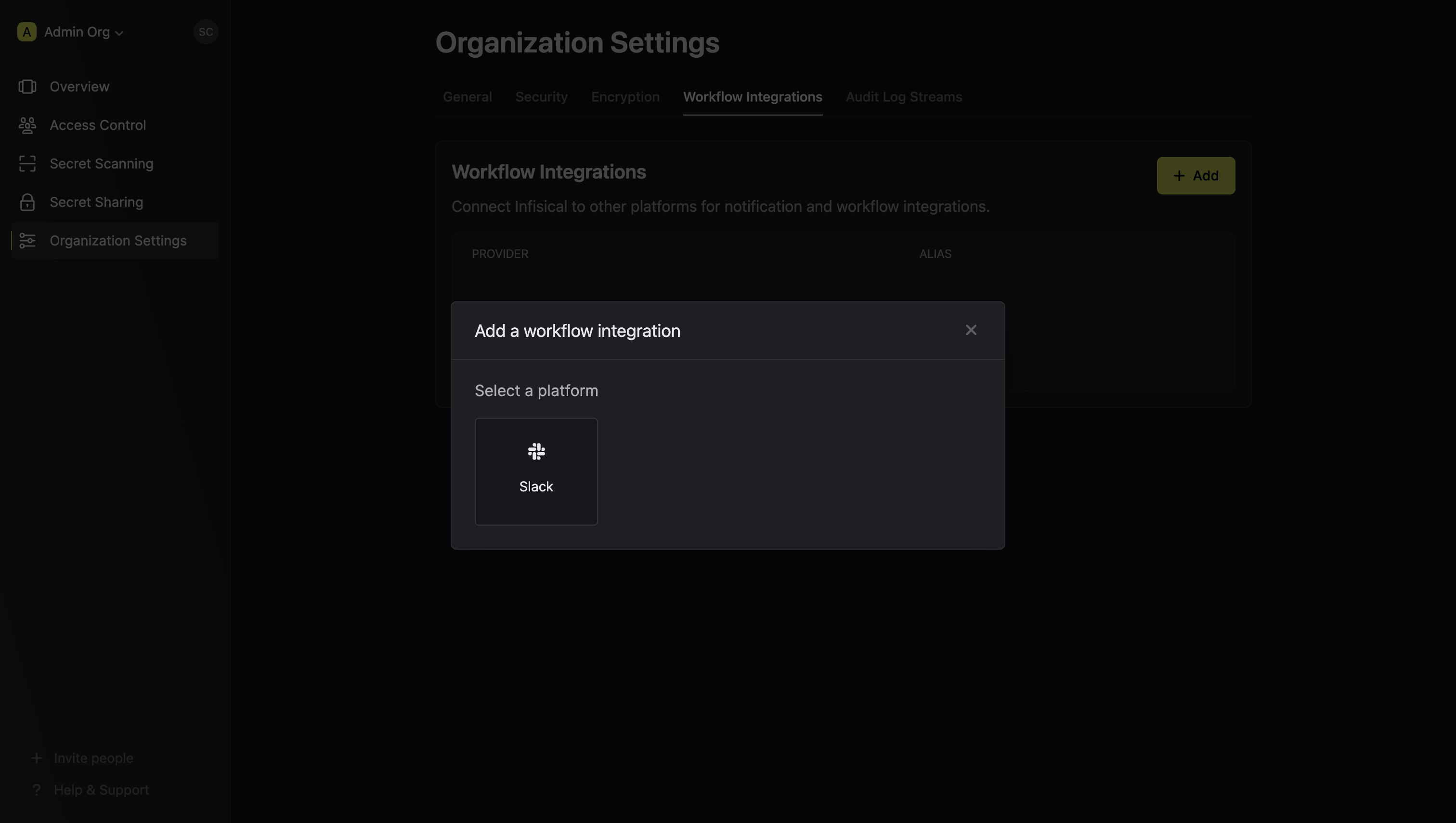
Give your Slack integration a descriptive alias. You will use this to select the Slack integration for your project.
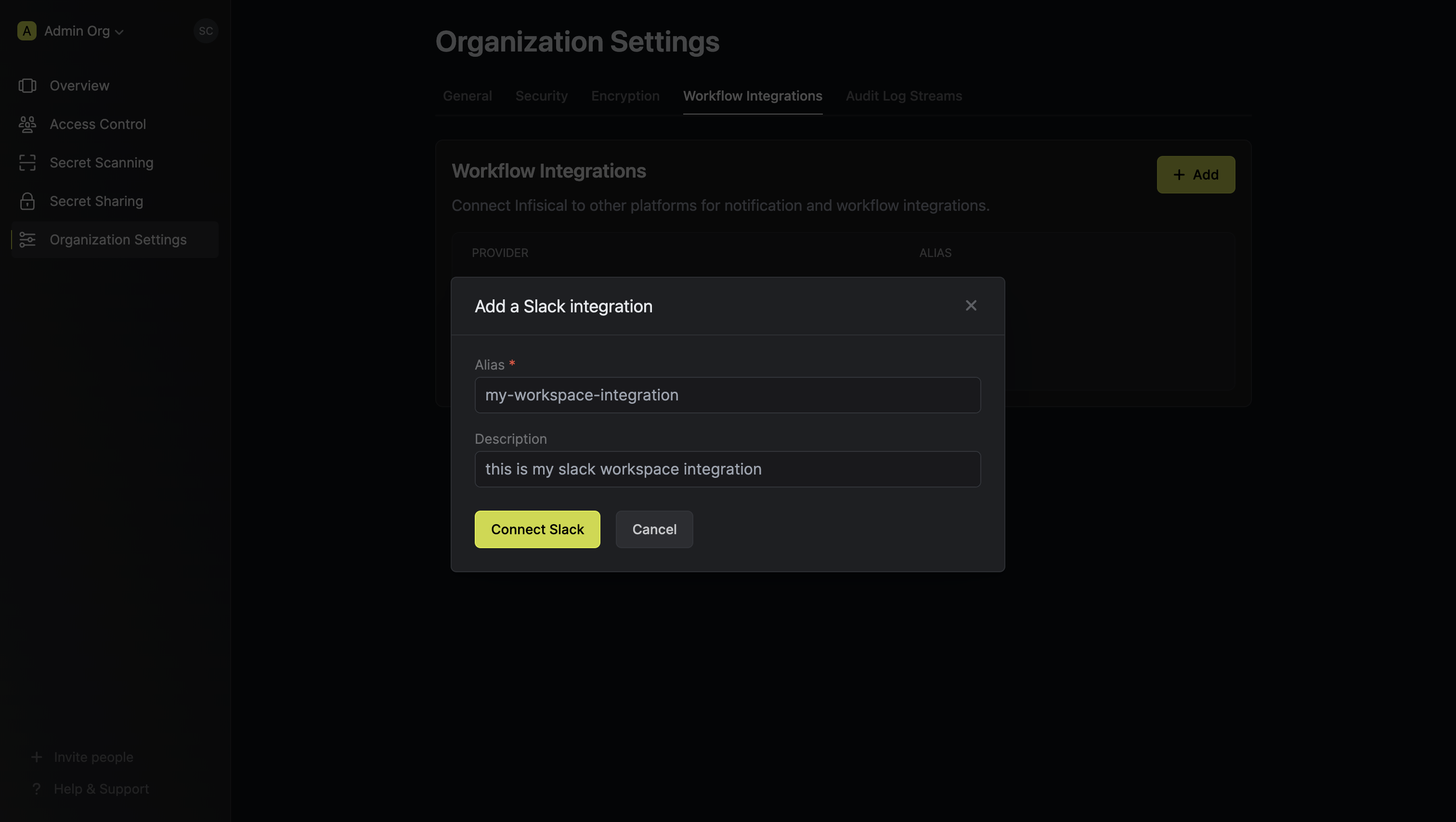
Press **Connect Slack**. This opens up the Slack app installation flow. Select the Slack workspace you want to install the custom Slack app to and press **Allow**.
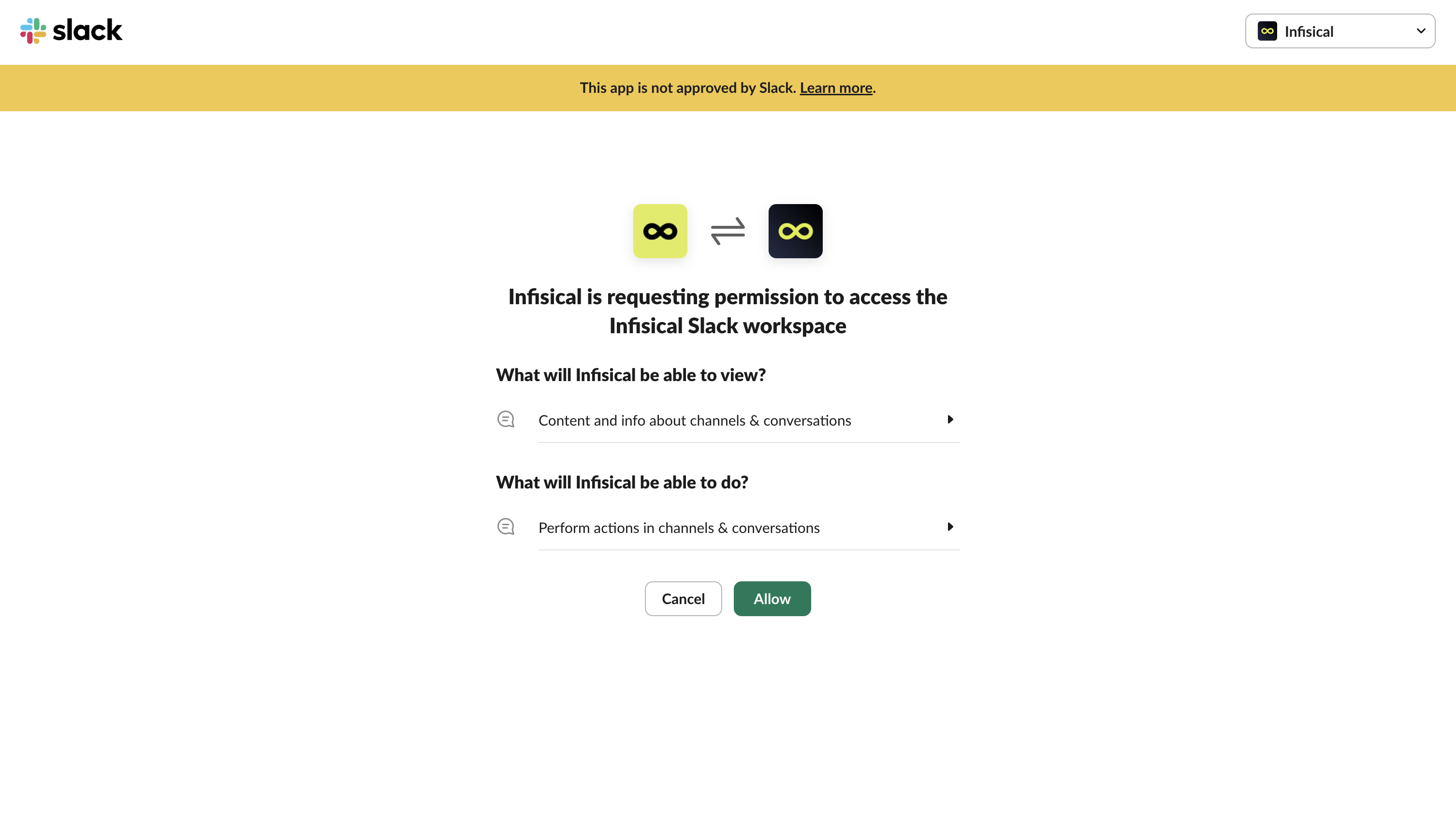
This completes the workflow integration creation flow. The projects in your organization can now use this Slack integration to send real-time updates to your Slack workspace.
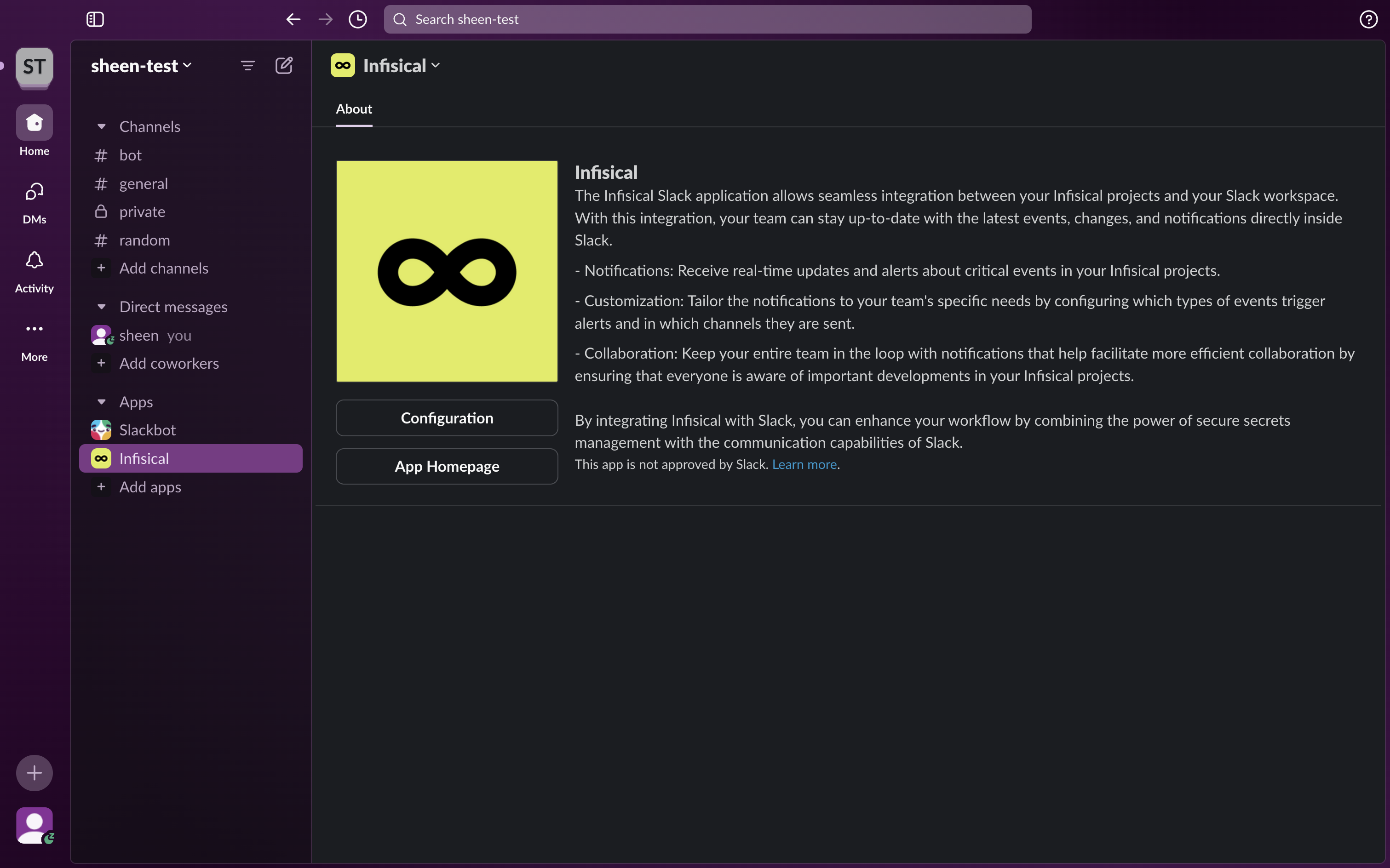
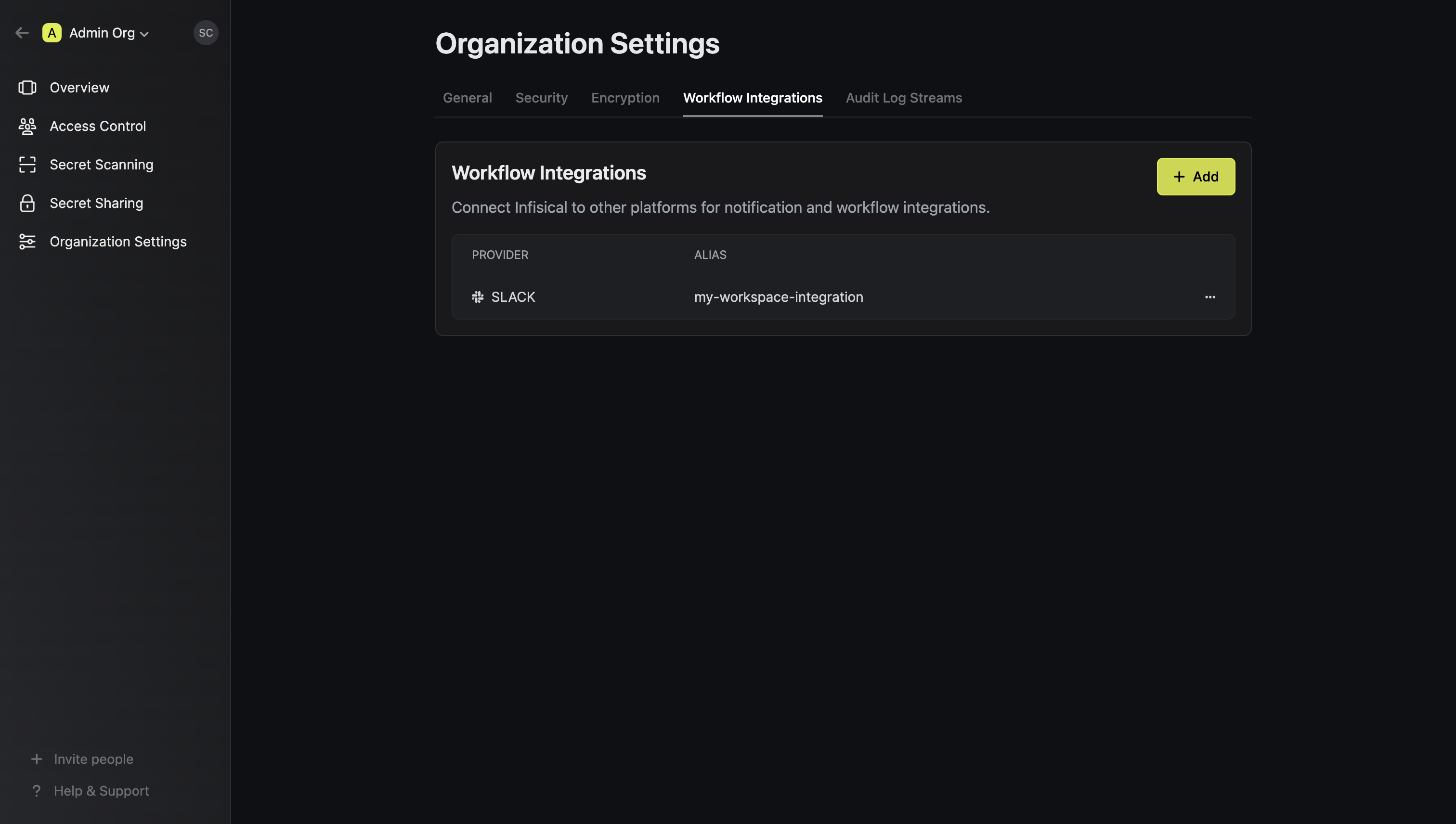
### Configure project to use Slack workflow integration
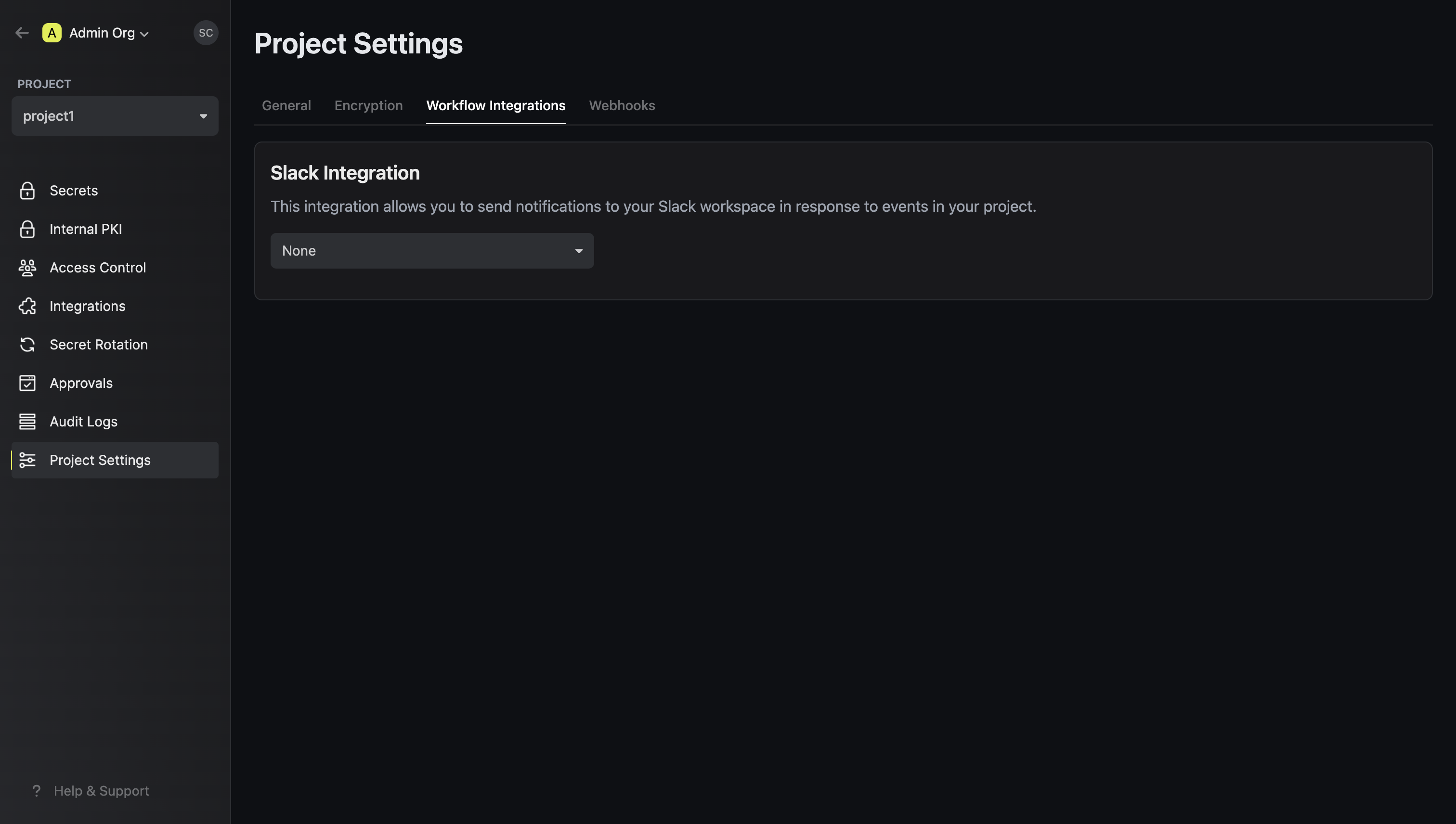
Your project will send notifications to the connected Slack workspace of the
selected Slack integration when the configured events are triggered.
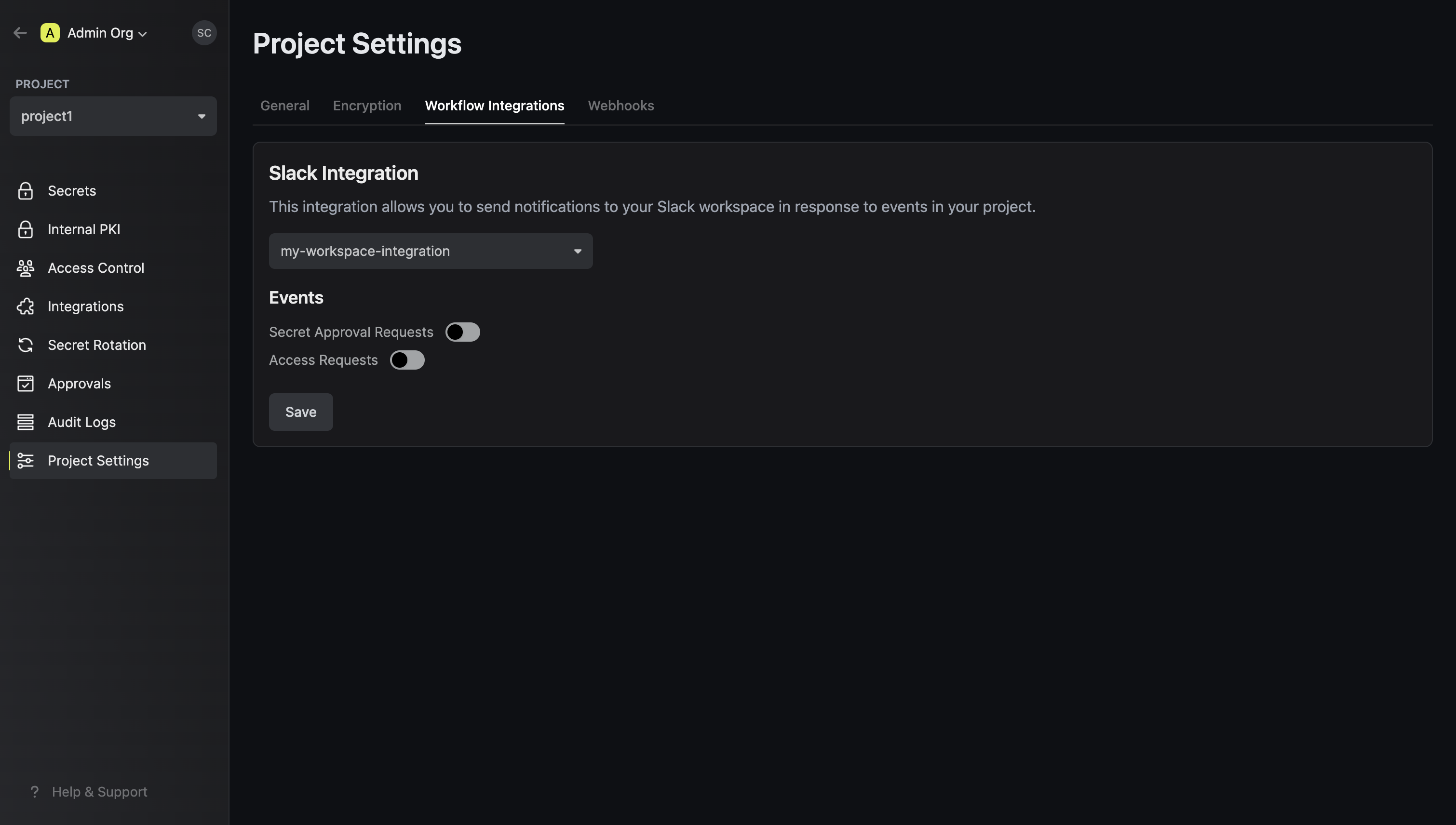
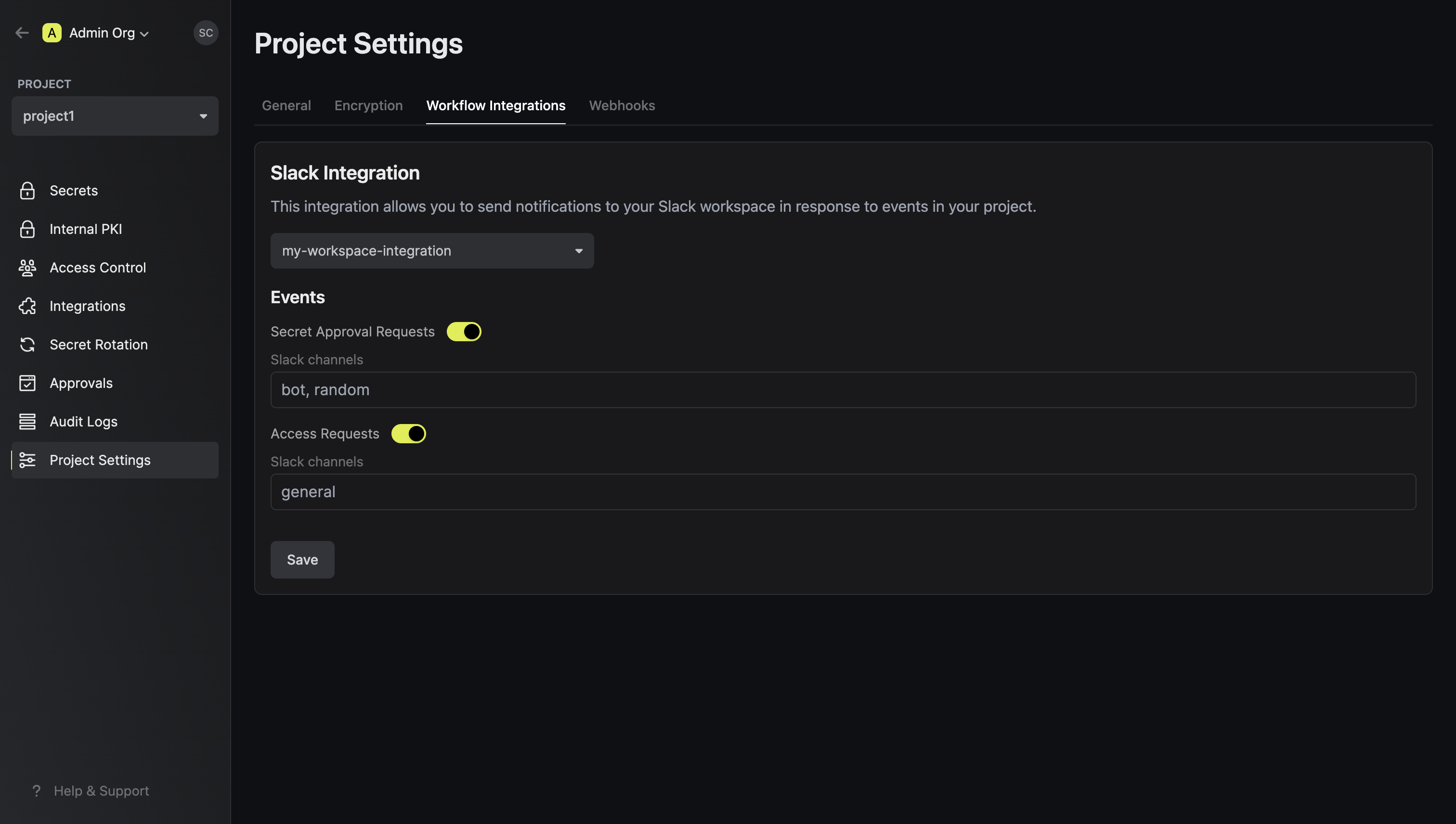
To enable notifications in private Slack channels, you need to invite the Infisical Slack bot to join those channels.
You now have a working native integration with Slack!
### Configure admin settings
Note that this step only has to be done once for the entire instance.
Before anything else, you need to setup the Slack app to be used by
your Infisical instance. Because you're self-hosting, you will need to
create this Slack application as demonstrated in the preceding step.
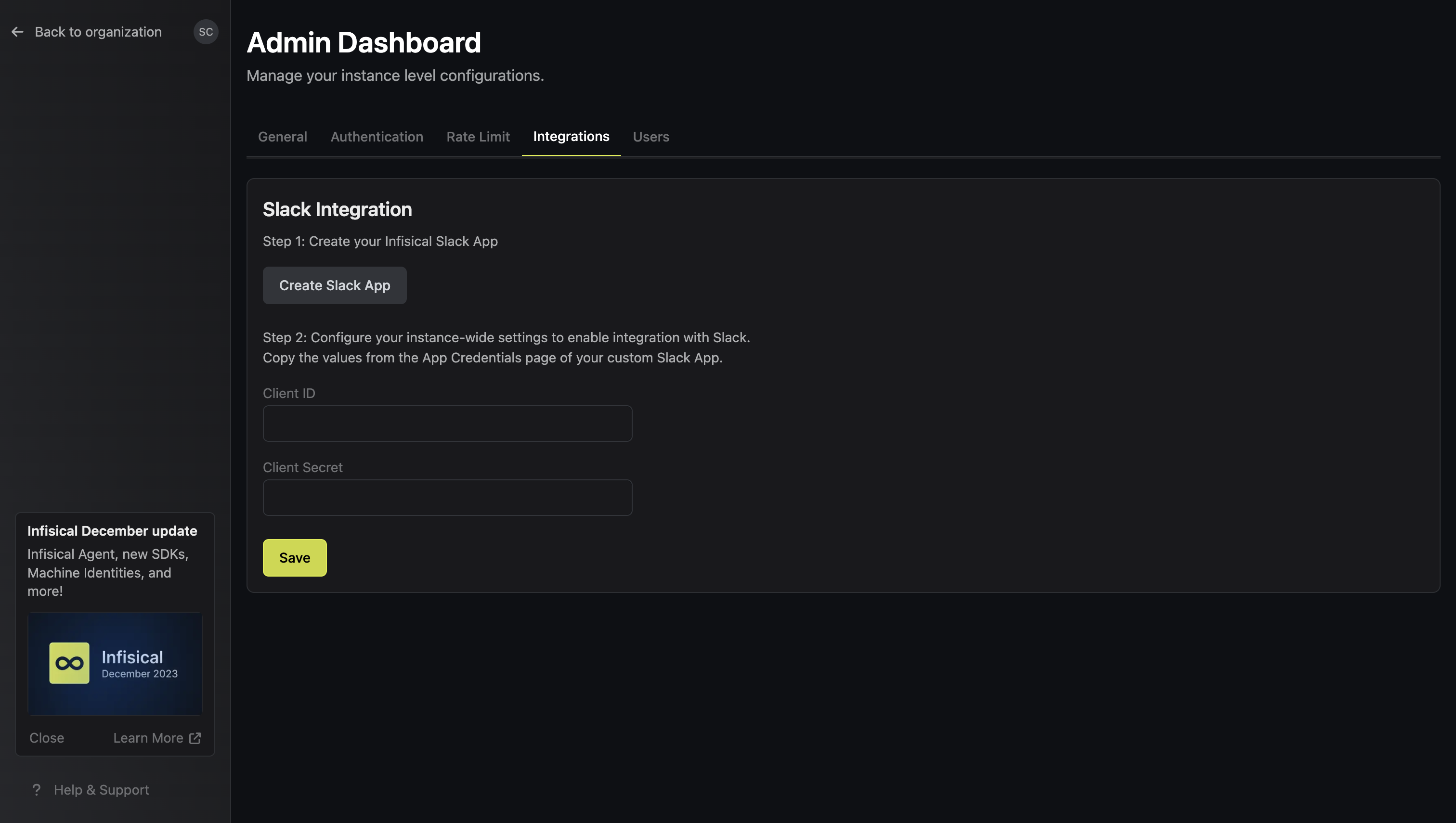
Click the "Create Slack app" button. This will open up a new window with the
custom app creation flow on Slack.
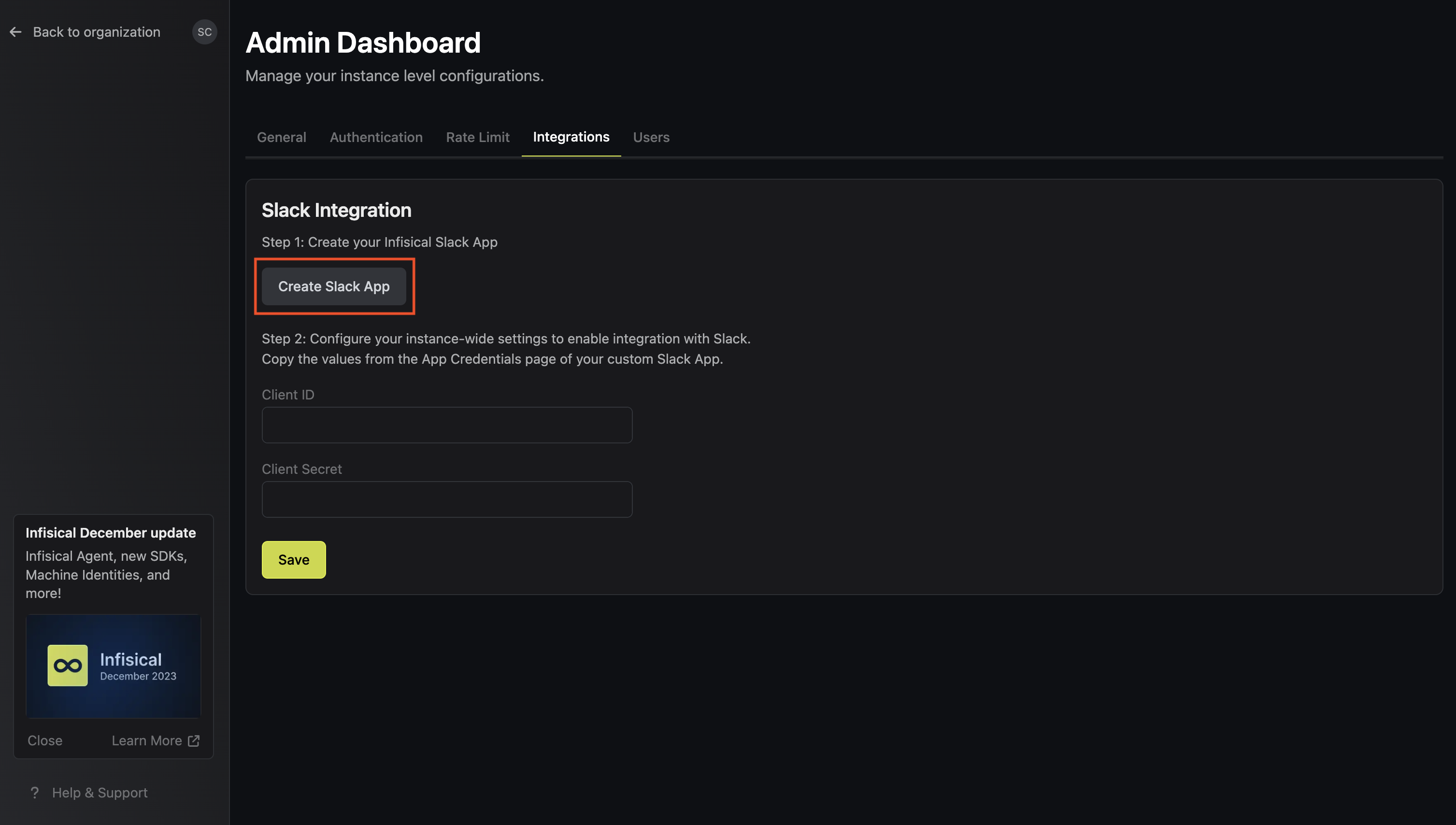
Select the Slack workspace you want to integrate with Infisical.
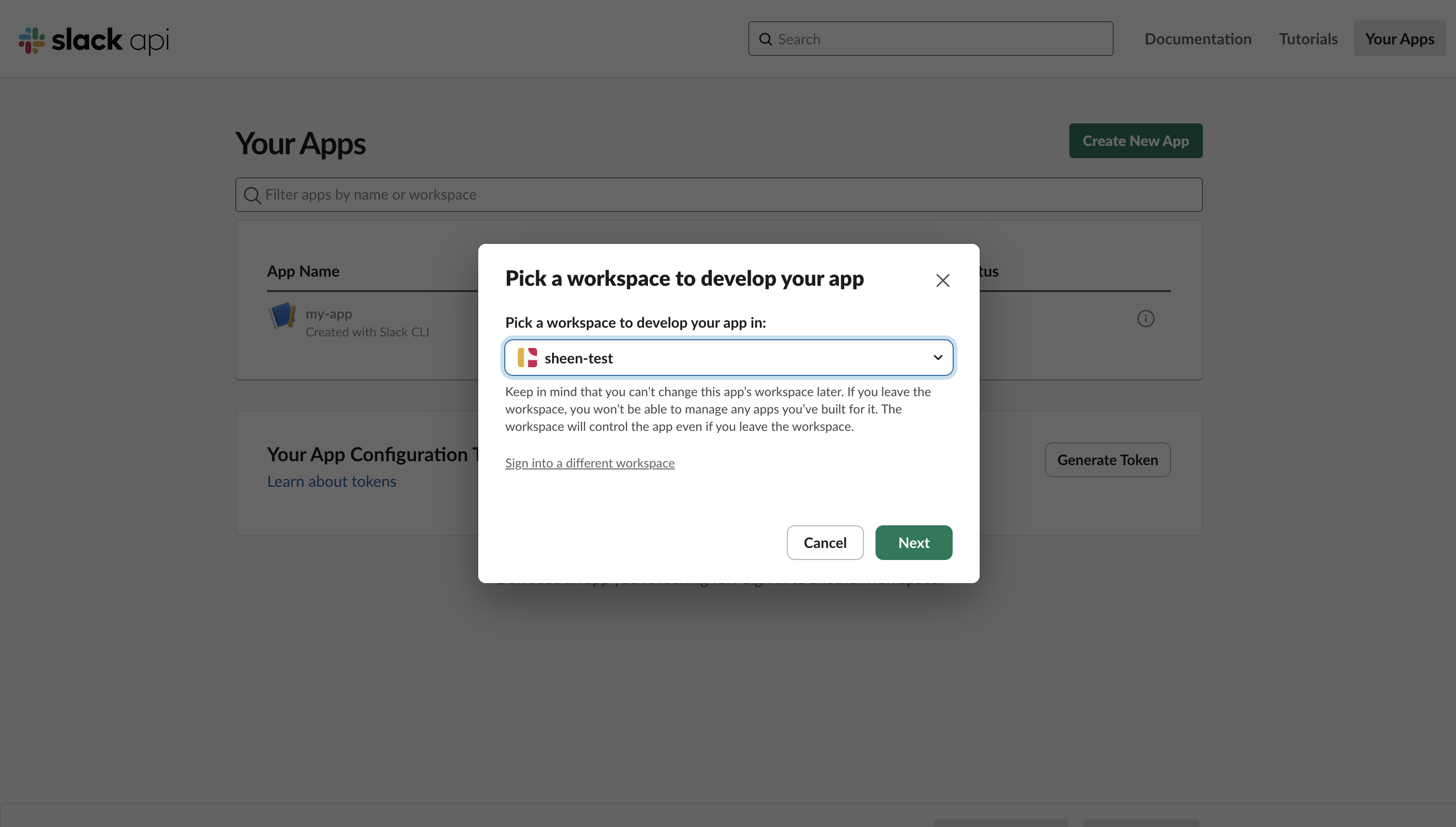
The configuration values of your custom Slack app will be pre-filled for you. You can view or edit the app manifest by clicking **Edit Configurations**.
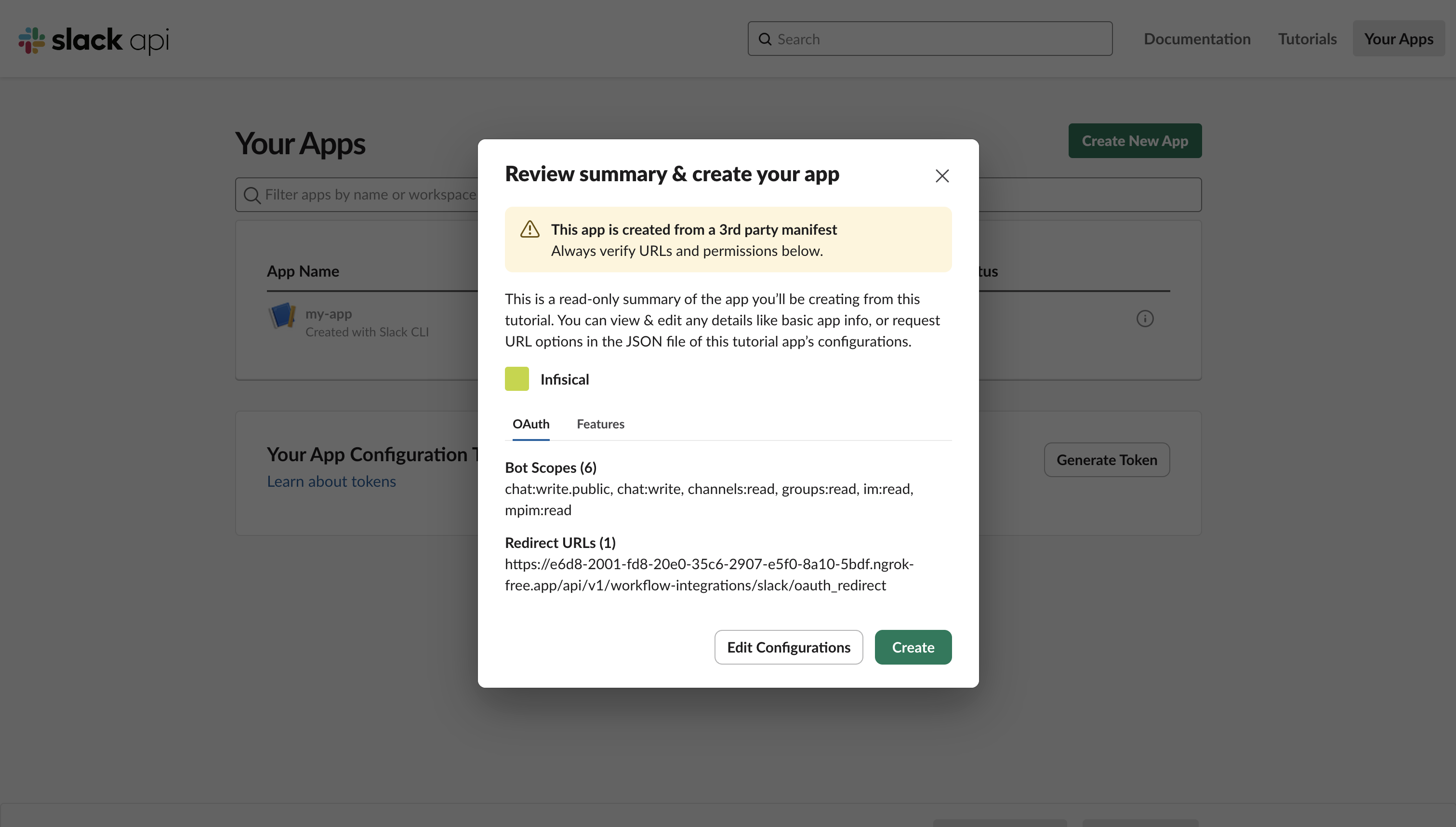
Once everything's confirmed, press Create.
Copy the Client ID and Client Secret values from your newly created custom Slack app and add them to Infisical.
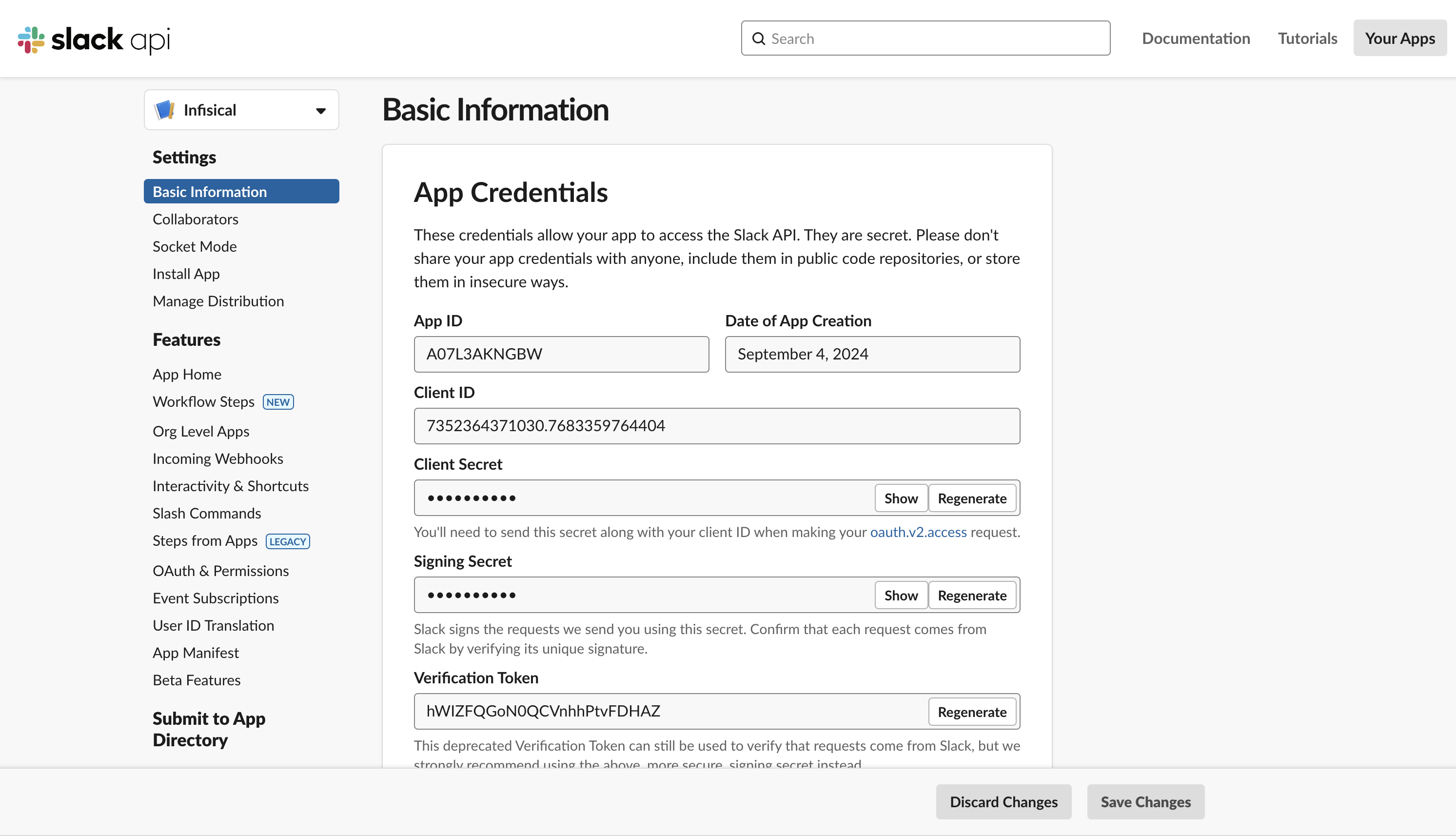
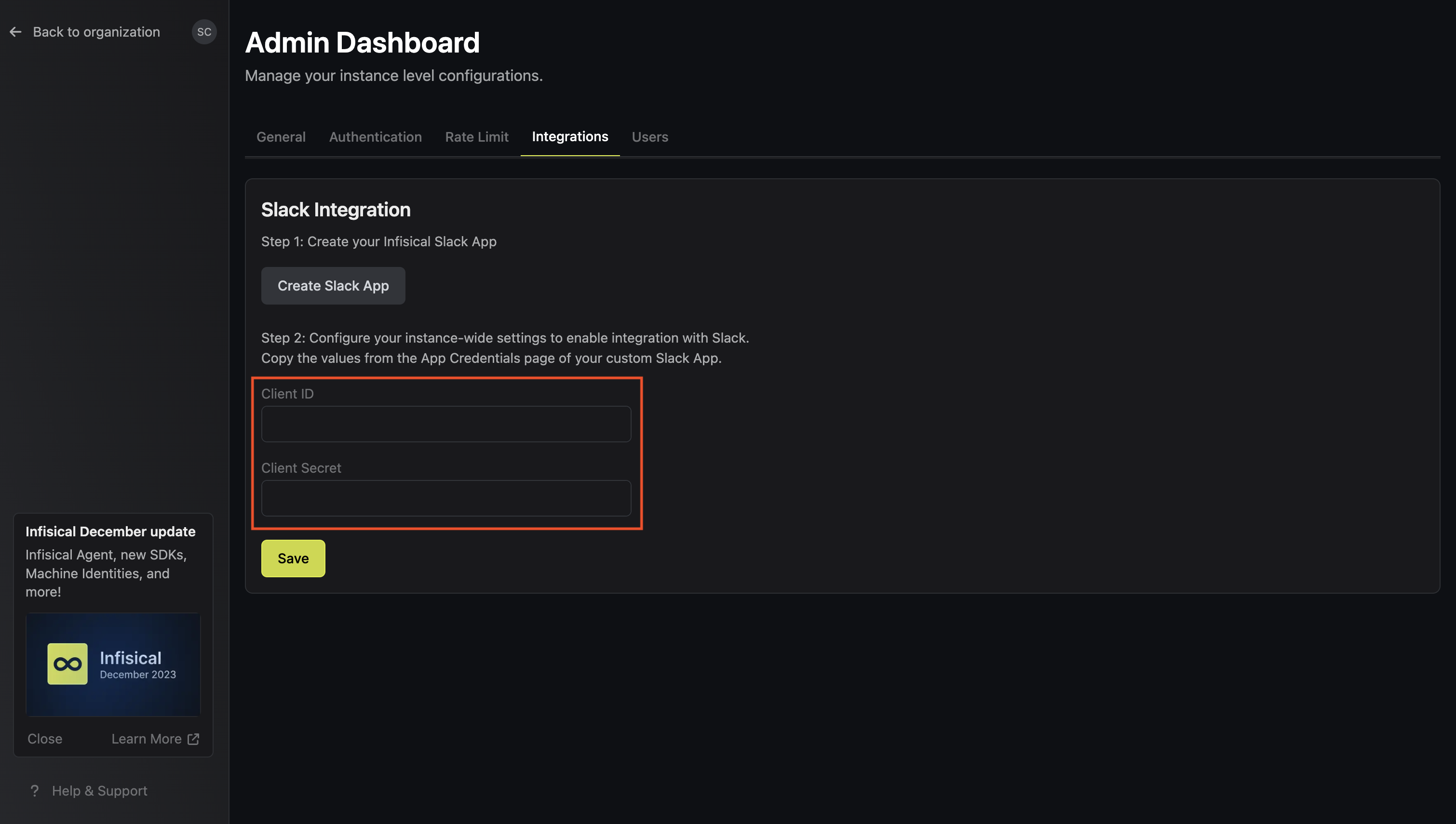
Complete the admin setup by pressing Save.
### Create Slack workflow integration
In order to use Slack integration in your projects, you will first have to
configure a Slack workflow integration in your organization.
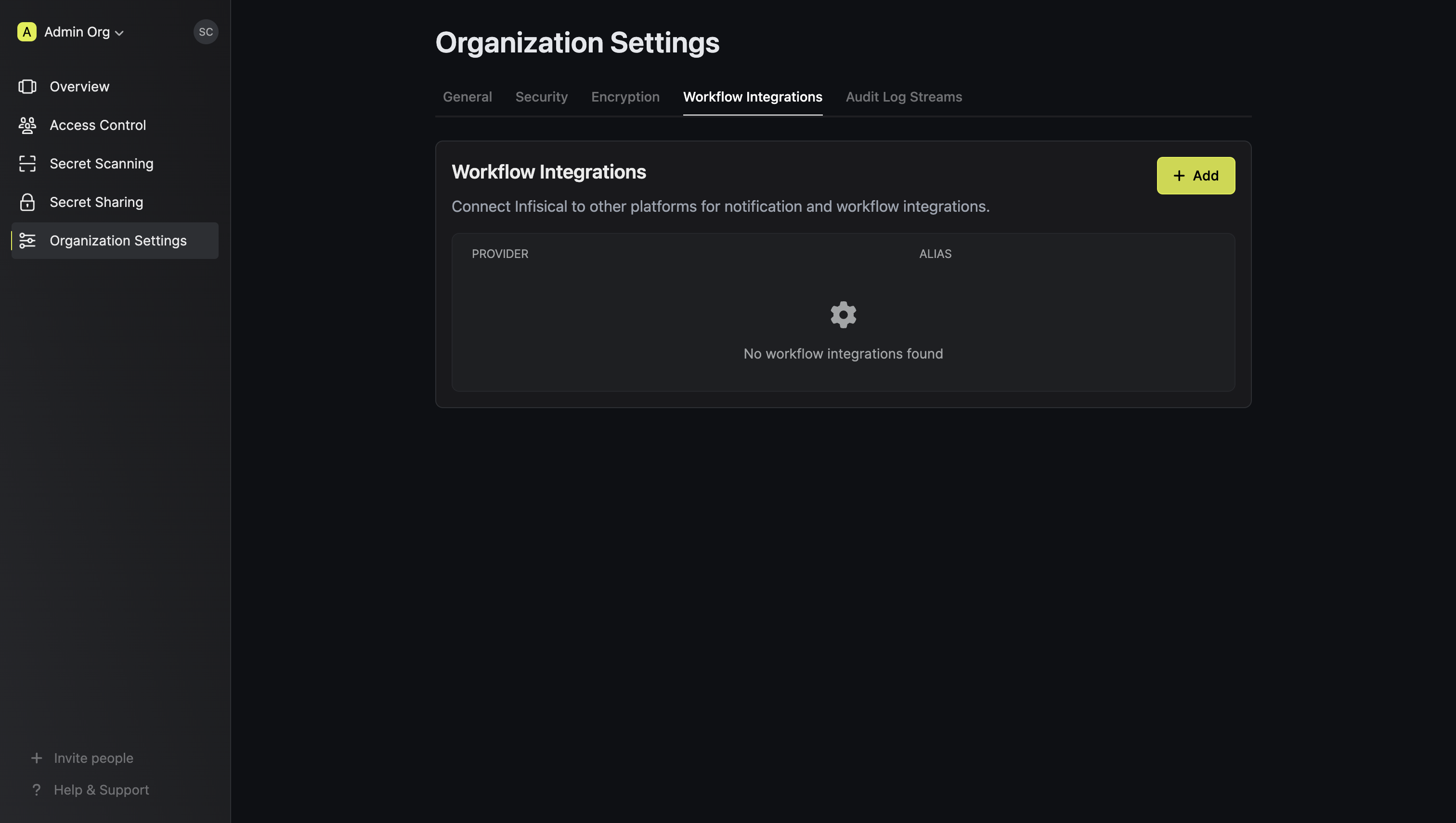
Press "Add" and select "Slack" as the platform.
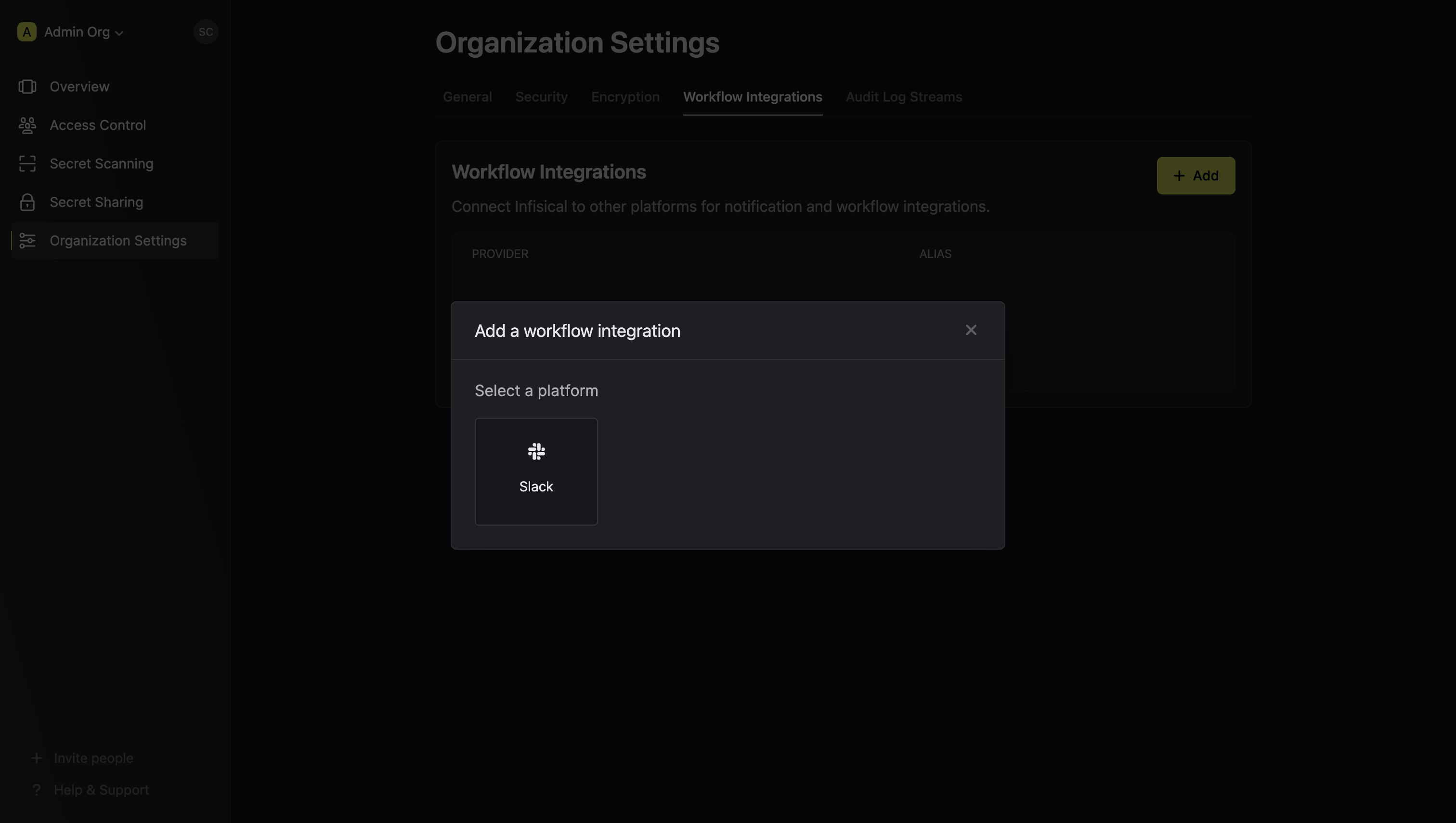
Give your Slack integration a descriptive alias. You will use this to select the Slack integration for your project.
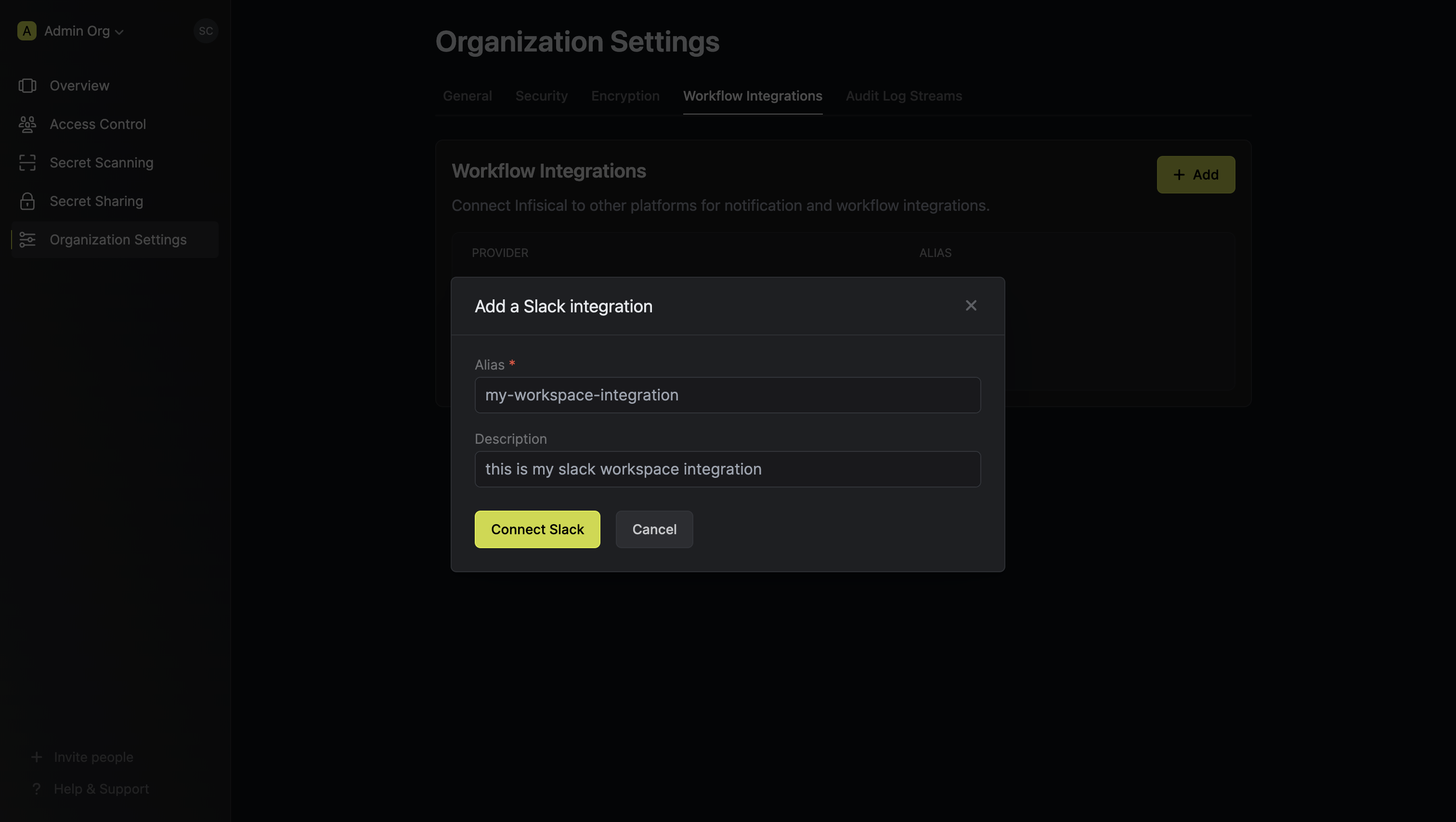
Press **Connect Slack**. This opens up the Slack app installation flow. Select the Slack workspace you want to install the custom Slack app to and press **Allow**.
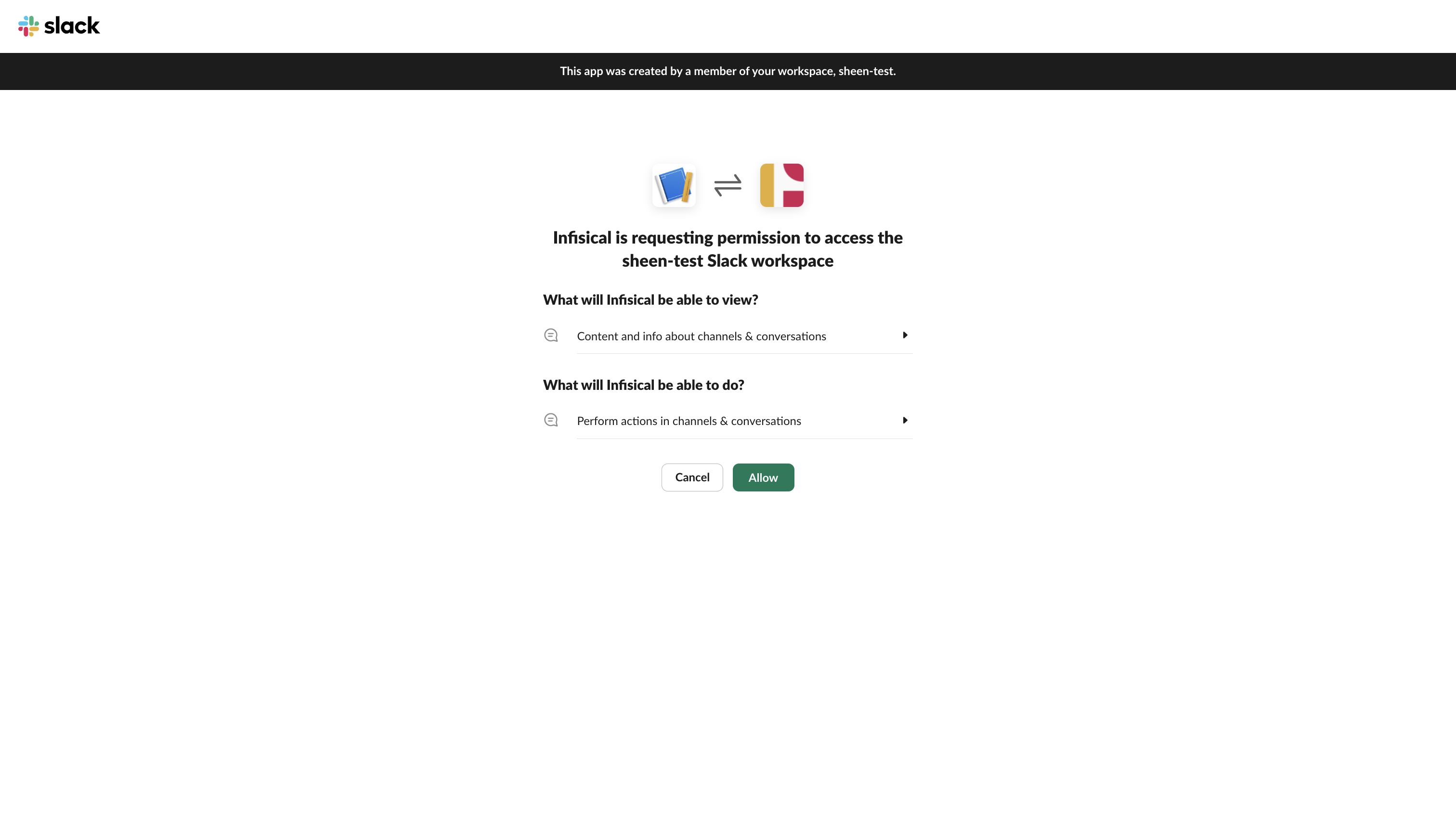
Your Slack bot will then be added to your selected Slack workspace. This completes the workflow integration creation flow. Your projects in the organization can now use this Slack integration to send real-time updates to your Slack workspace.
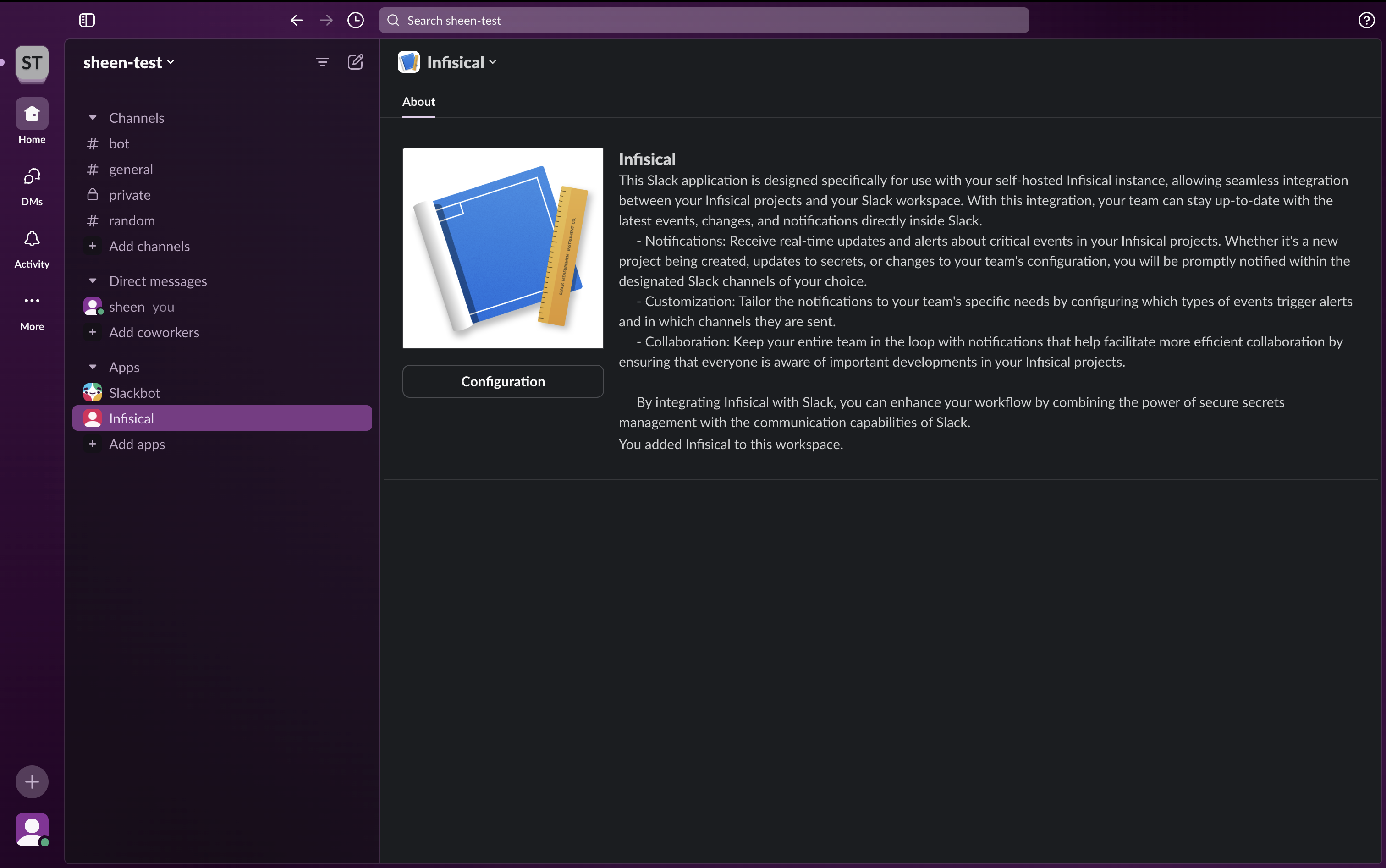
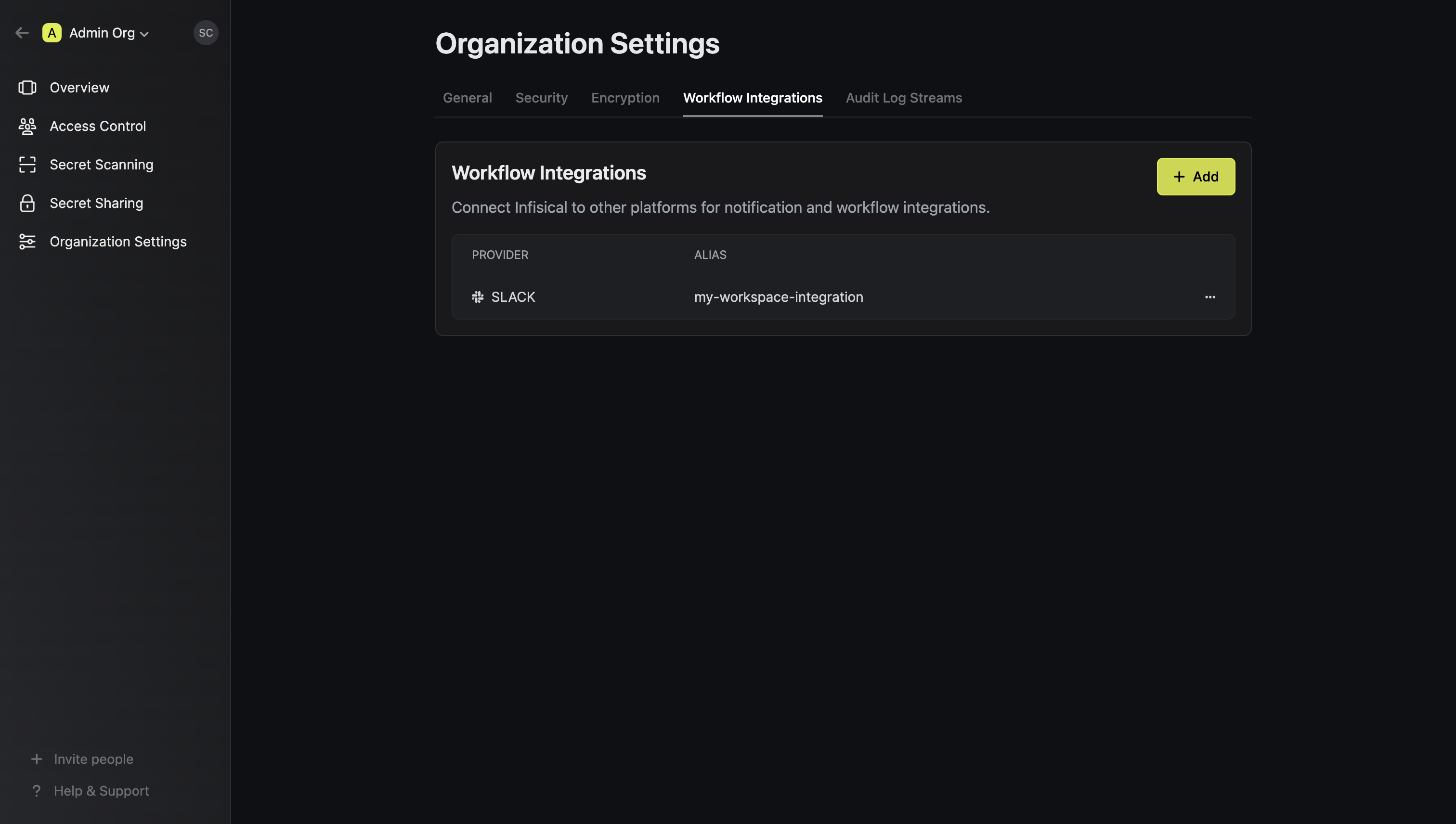
### Configure project to use Slack workflow integration
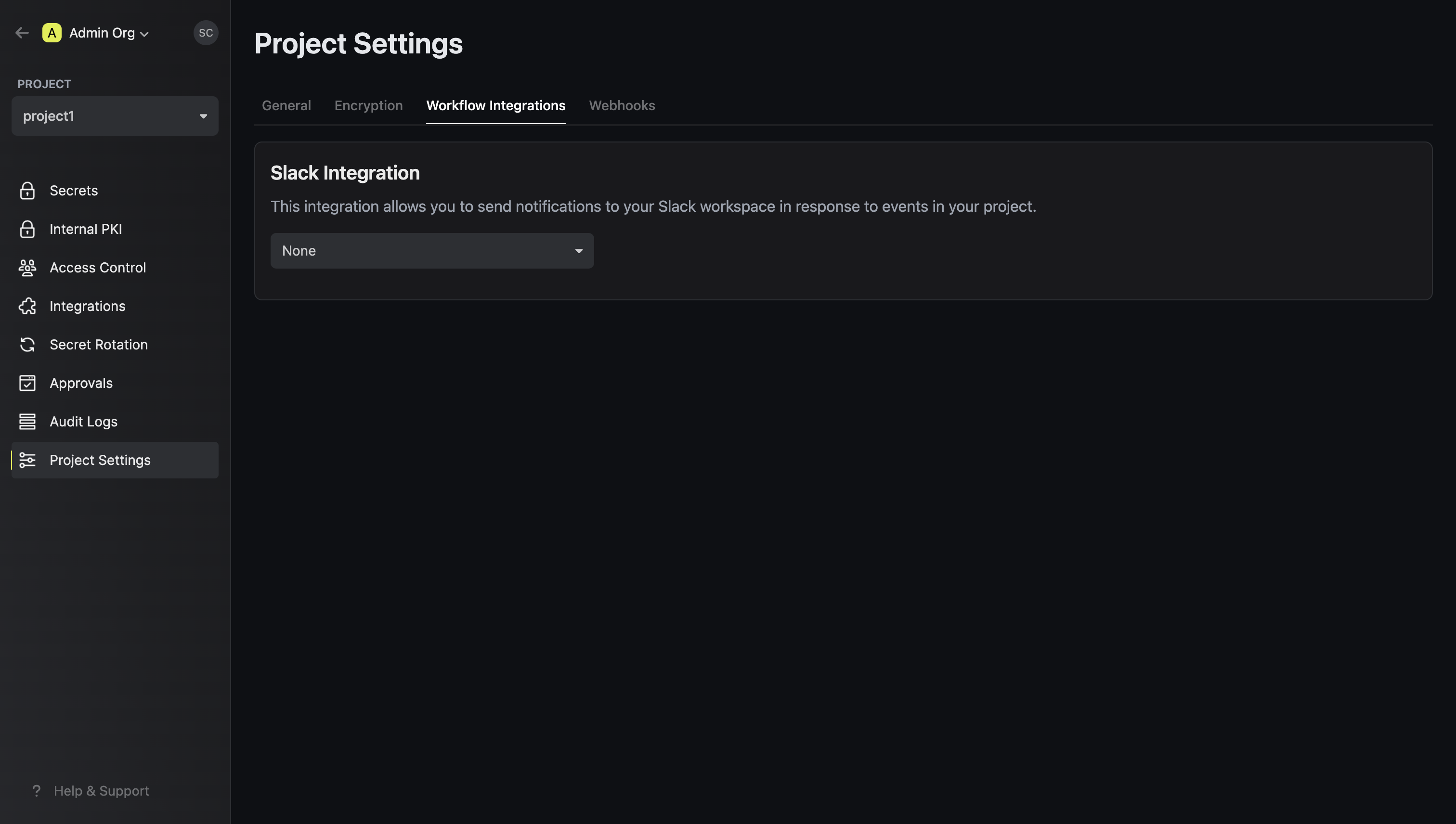
Your project will send notifications to the connected Slack workspace of the
selected Slack integration when the configured events are triggered.
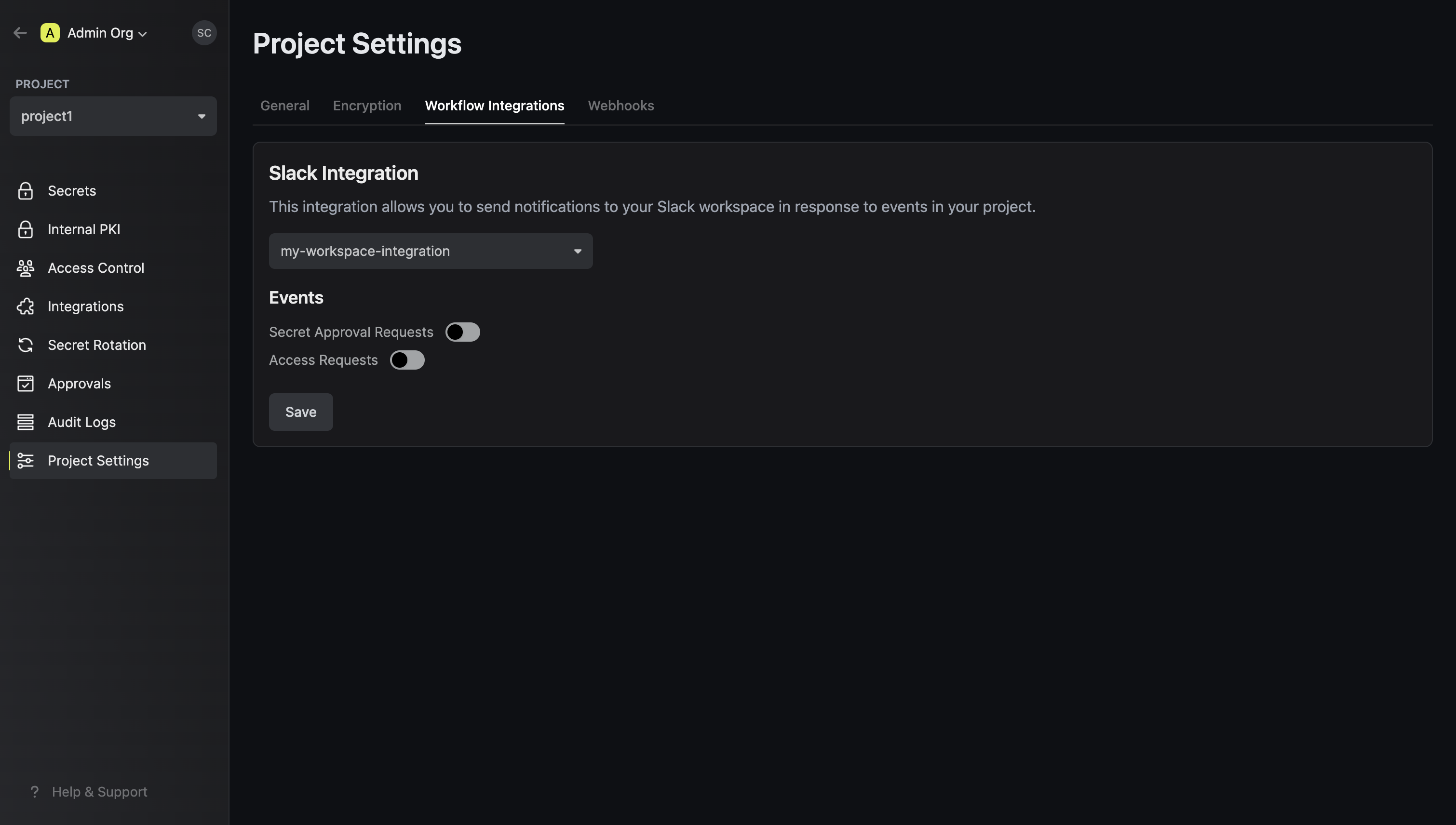
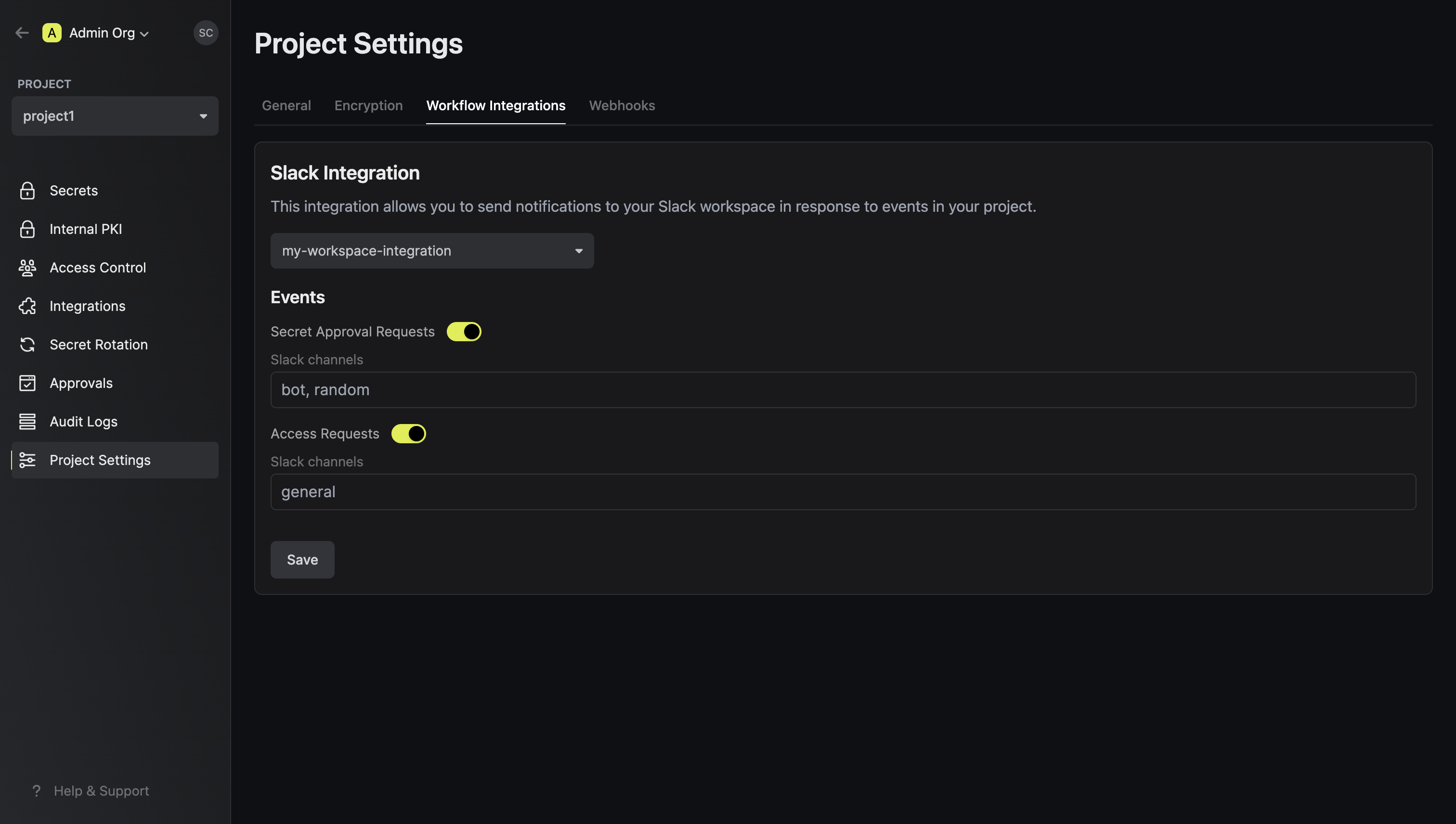
To enable notifications in private Slack channels, you need to invite your Slack bot to join those channels.
You now have a working native integration with Slack!
## Using the Slack integration in your private channels
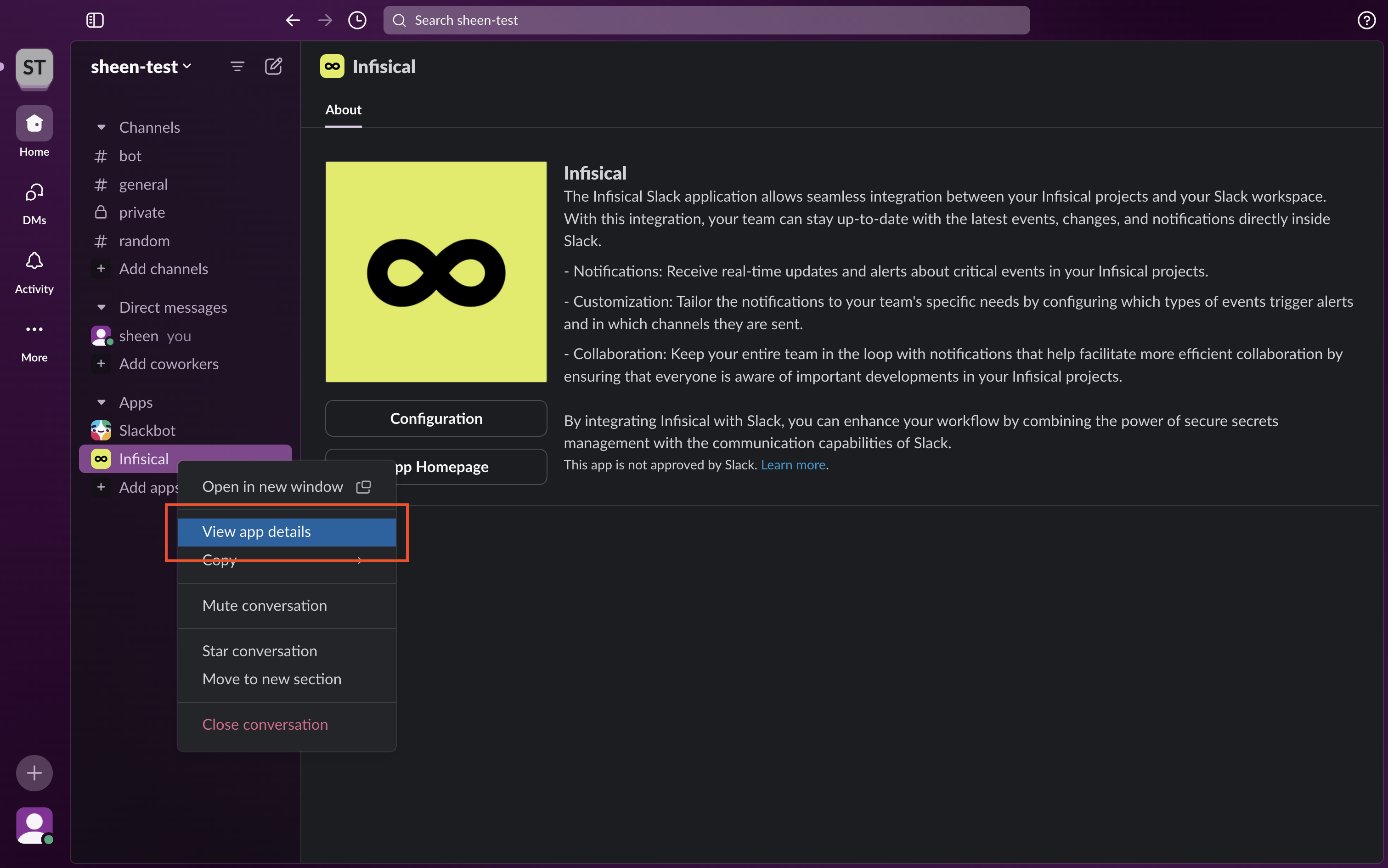
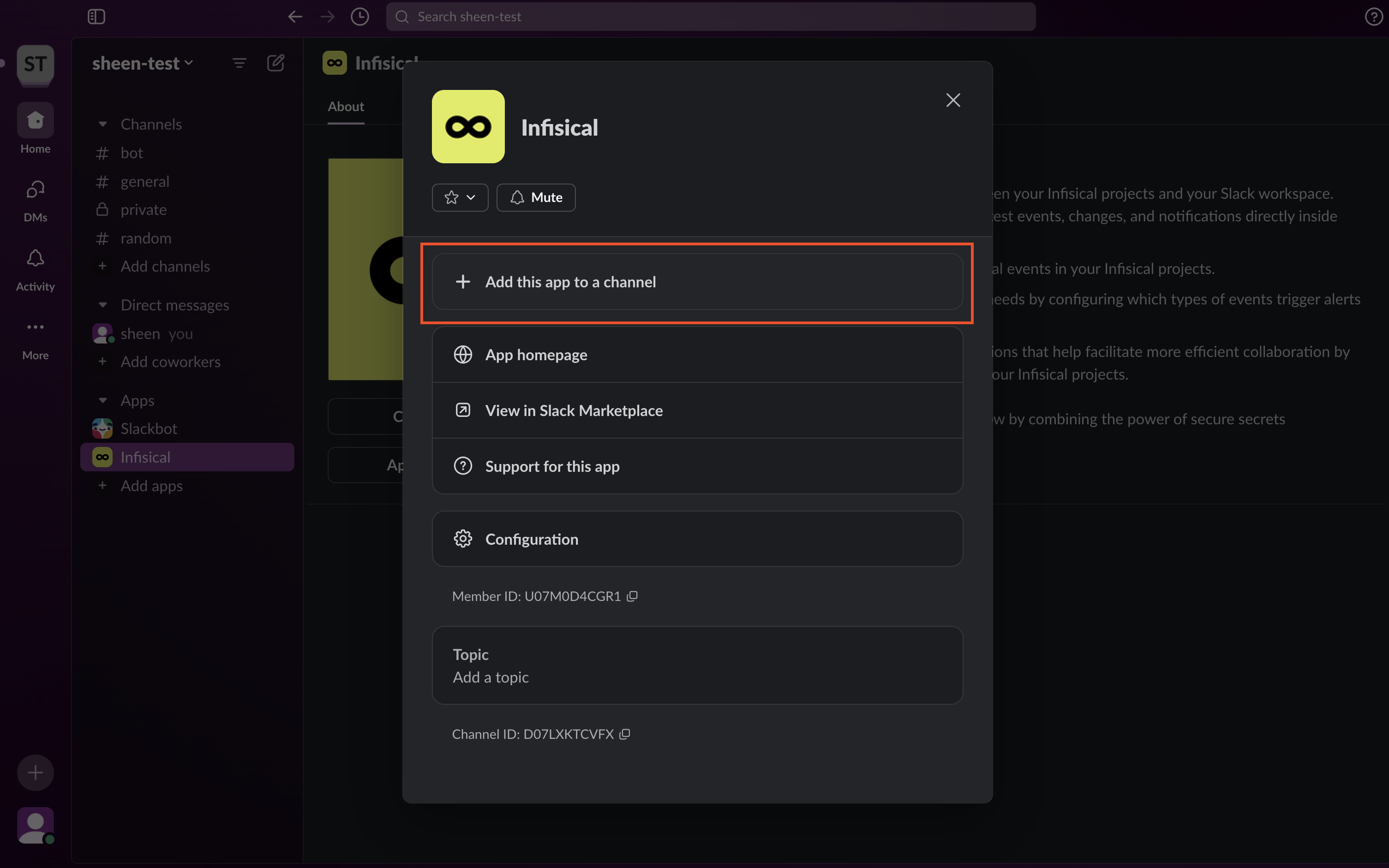
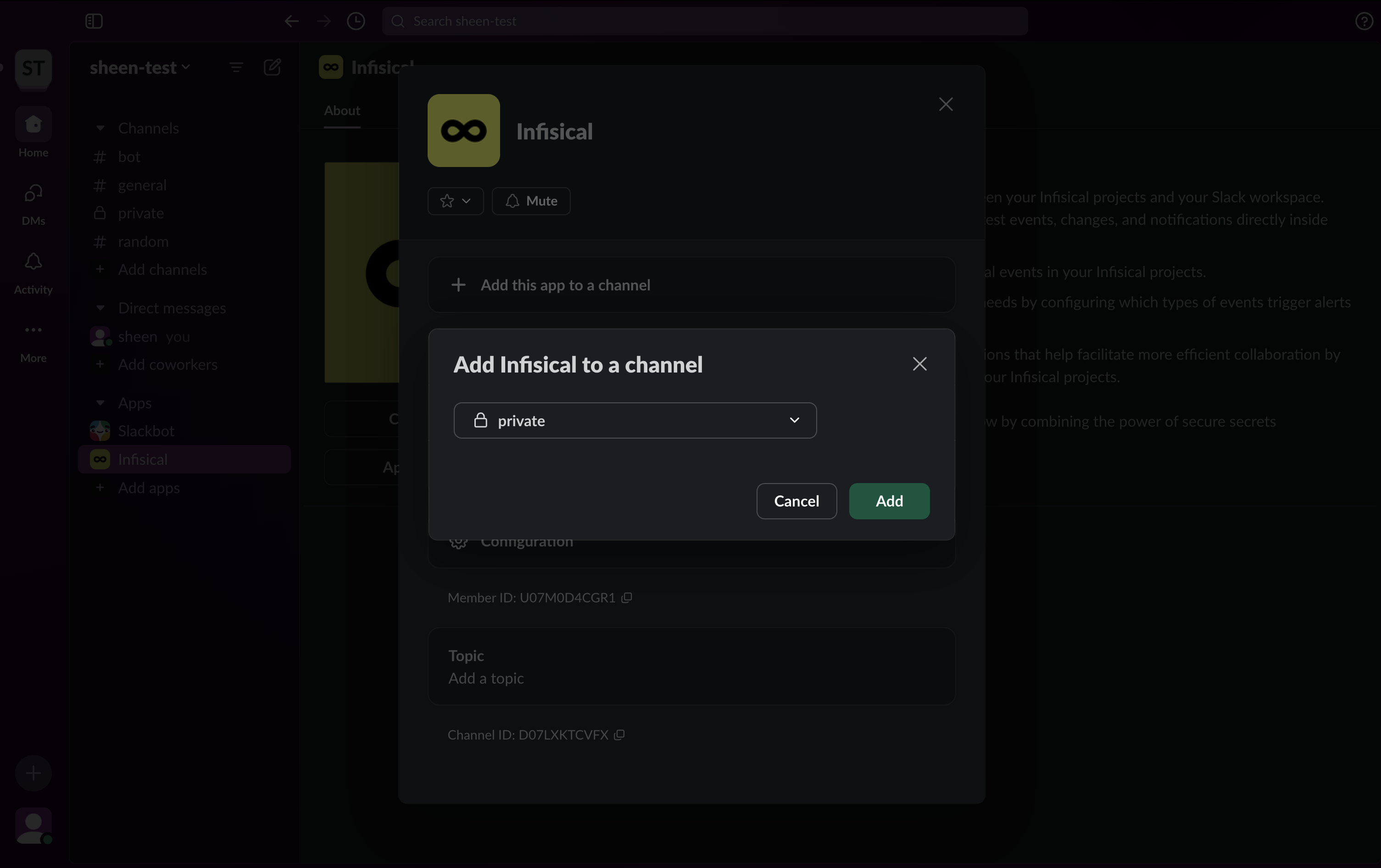
You can now view the private channels in the Slack channel selection fields!
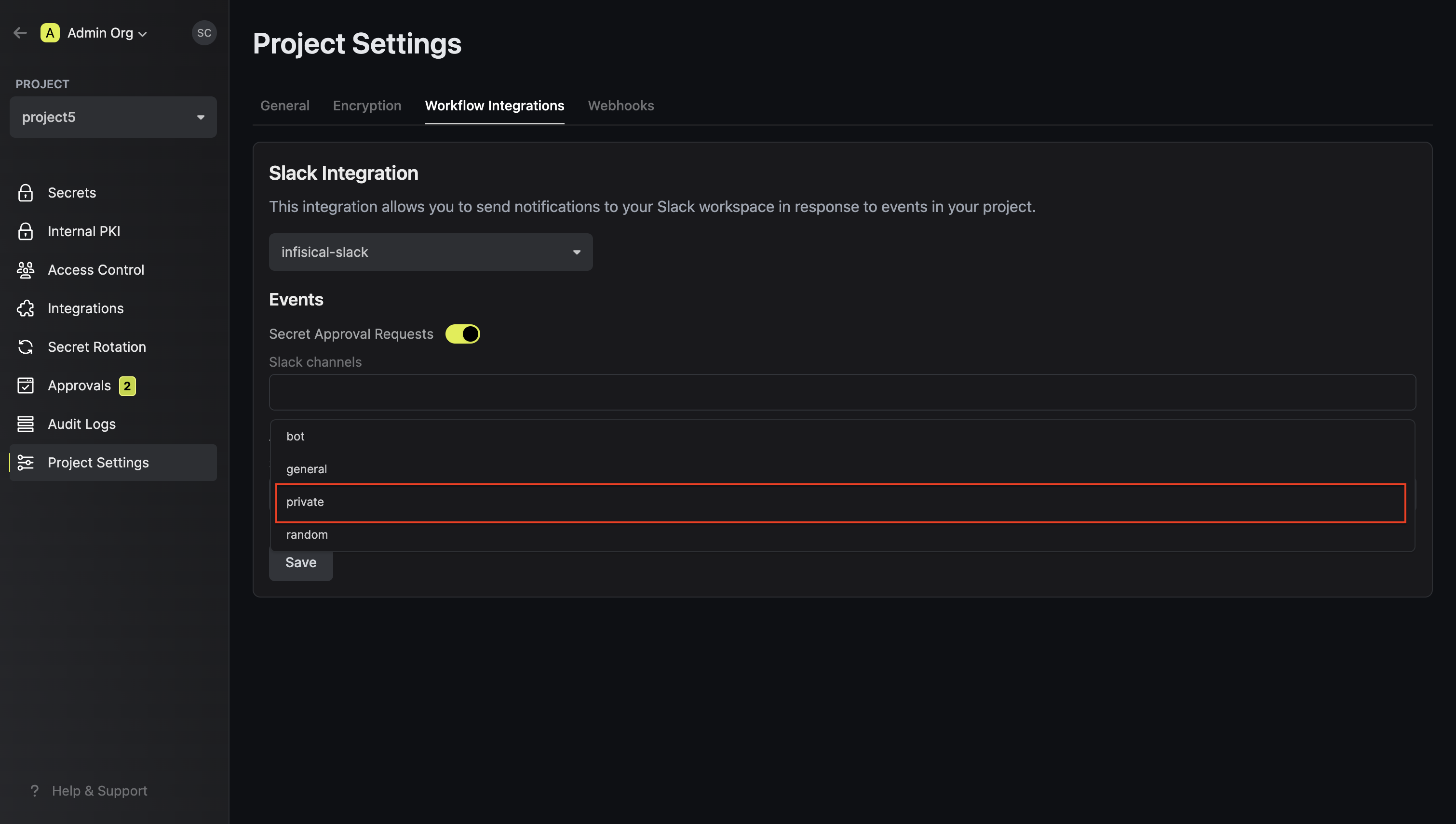
# Gradle
How to use Infisical to inject environment variables with Gradle
# Using Infisical with Gradle
By integrating [Infisical CLI](../../cli/overview) with Gradle, you can configure your builds and scripts to different environments, CI/CD pipelines, and more without explicitly setting variables in the command line.
This documentation provides an overview of how to use Infisical with [Gradle](https://gradle.org/).
## Basic Usage
To run a Gradle task with Infisical, you can use the `run` command. The basic structure is:
```
infisical run -- [Your command here]
```
For example, to run the `generateFile` task in Gradle:
```groovy build.gradle
task generateFile {
doLast {
String content = System.getenv('ENV_NAME_FROM_INFISICAL') ?: 'Default Content'
file('output.txt').text = content
println "Generated output.txt with content: $content"
}
}
```
```
infisical run -- gradle generateFile
```
With this command, Infisical will automatically inject the environment variables associated with the current Infisical project into the Gradle process.
Your Gradle script can then access these variables using `System.getenv('VARIABLE_NAME')`.
## More Examples
### 1. Building a Project with a Specific Profile
Assuming you have different build profiles (e.g., 'development', 'production'), you can use Infisical to switch between them:
```
infisical run -- gradle build
```
Inside your `build.gradle`, you might have:
```groovy build.gradle
if (System.getenv('PROFILE') == 'production') {
// production-specific configurations
}
```
### 2. Running Tests with Different Database Configurations
If you want to run tests against different database configurations:
```
infisical run -- gradle test
```
Your test configuration in `build.gradle` can then adjust the database URL accordingly:
```groovy build.gradle
test {
systemProperty 'db.url', System.getenv('DB_URL')
}
```
### 3. Generating Artifacts with Versioning
For automated CI/CD pipelines, you might want to inject a build number or version:
```
infisical run -- gradle assemble
```
And in `build.gradle`:
```groovy build.gradle
version = System.getenv('BUILD_NUMBER') ?: '1.0.0-SNAPSHOT'
```
## Advantages of Using Infisical with Gradle
1. **Flexibility**: Easily adapt your Gradle builds to different environments without modifying the build scripts or setting environment variables manually.
2. **Reproducibility**: Ensure consistent builds by leveraging the environment variables from the related Infisical project.
3. **Security**: Protect sensitive information by using Infisical's secrets management without exposing them in scripts or logs.
# Bitbucket
How to sync secrets from Infisical to Bitbucket
Infisical lets you sync secrets to Bitbucket at the repository-level and deployment environment-level.
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
Navigate to your project's integrations tab in Infisical.
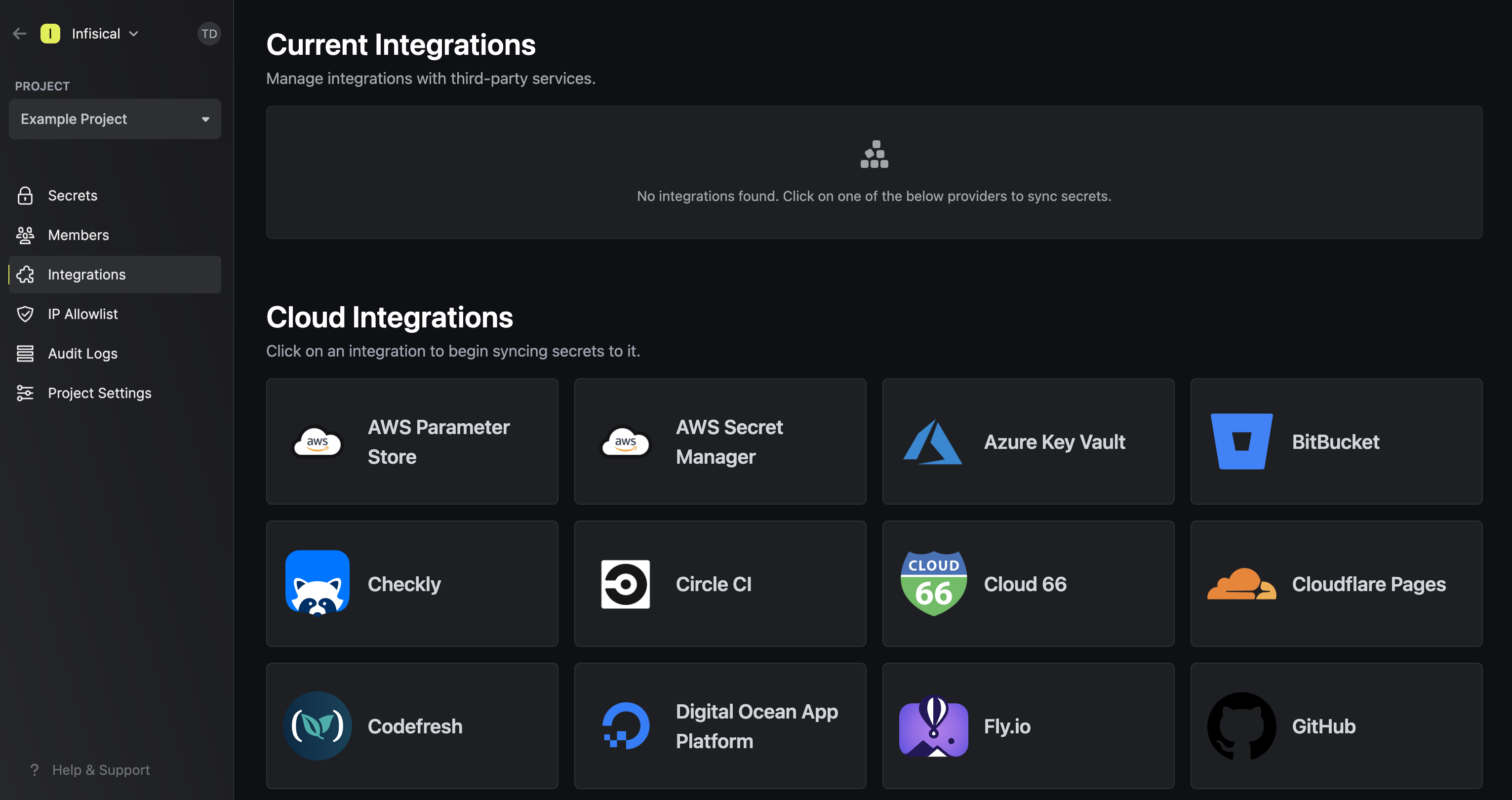
Press on the Bitbucket tile and grant Infisical access to your Bitbucket account.
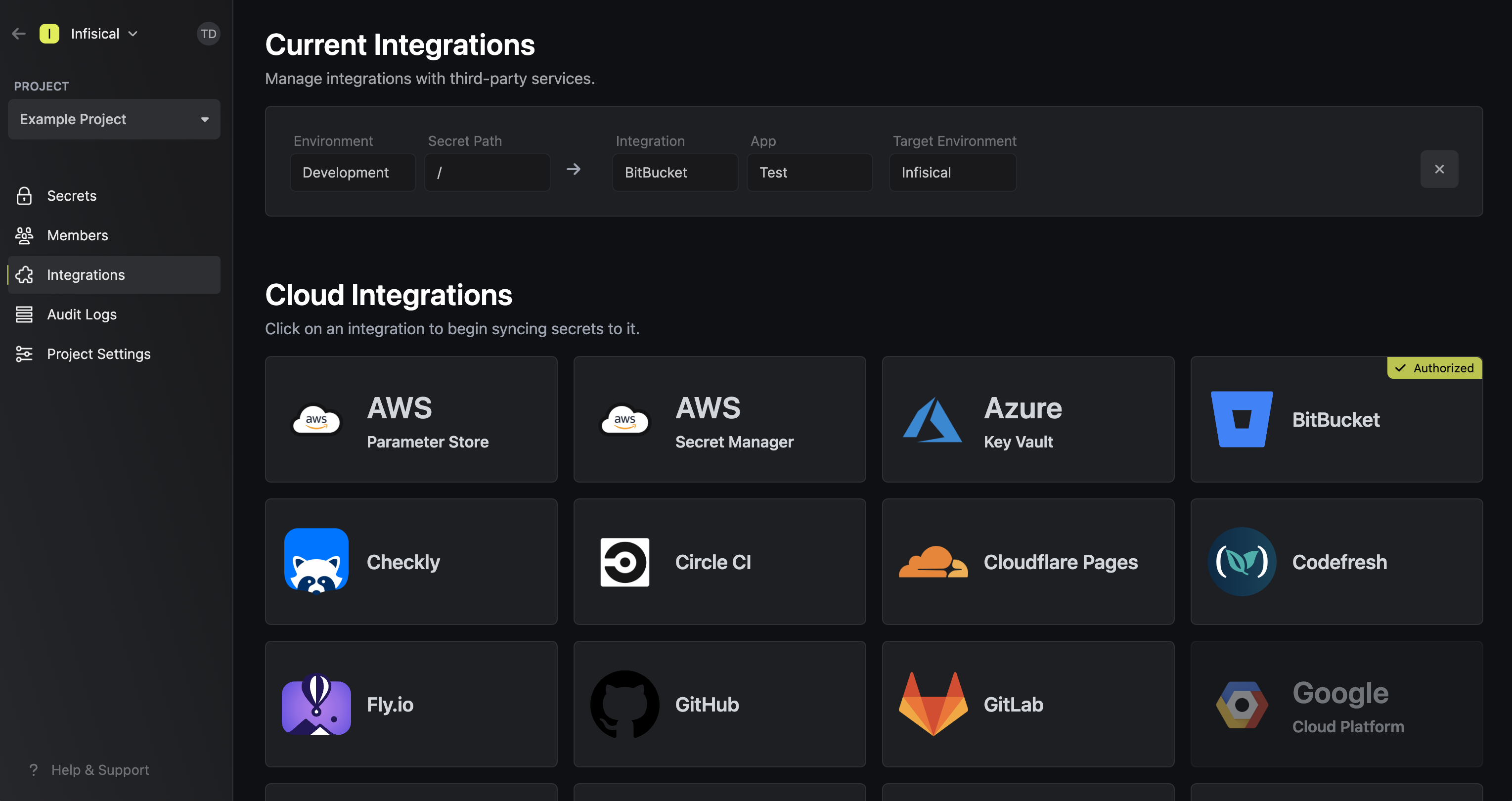
Select which workspace, repository, and optionally, deployment environment, you'd like to sync your secrets
to.
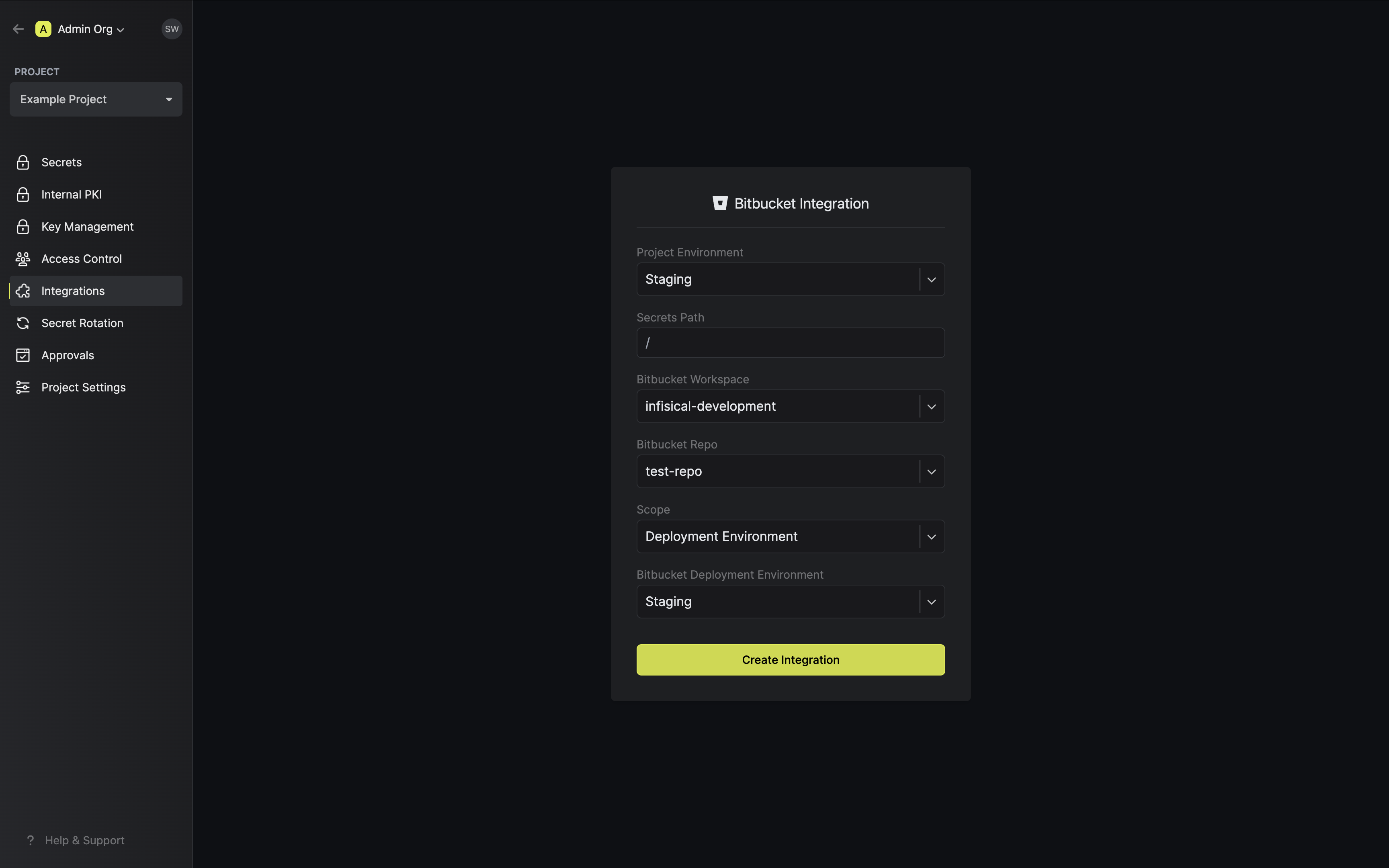
Once created, your integration will begin syncing secrets to the configured repository or deployment
environment.
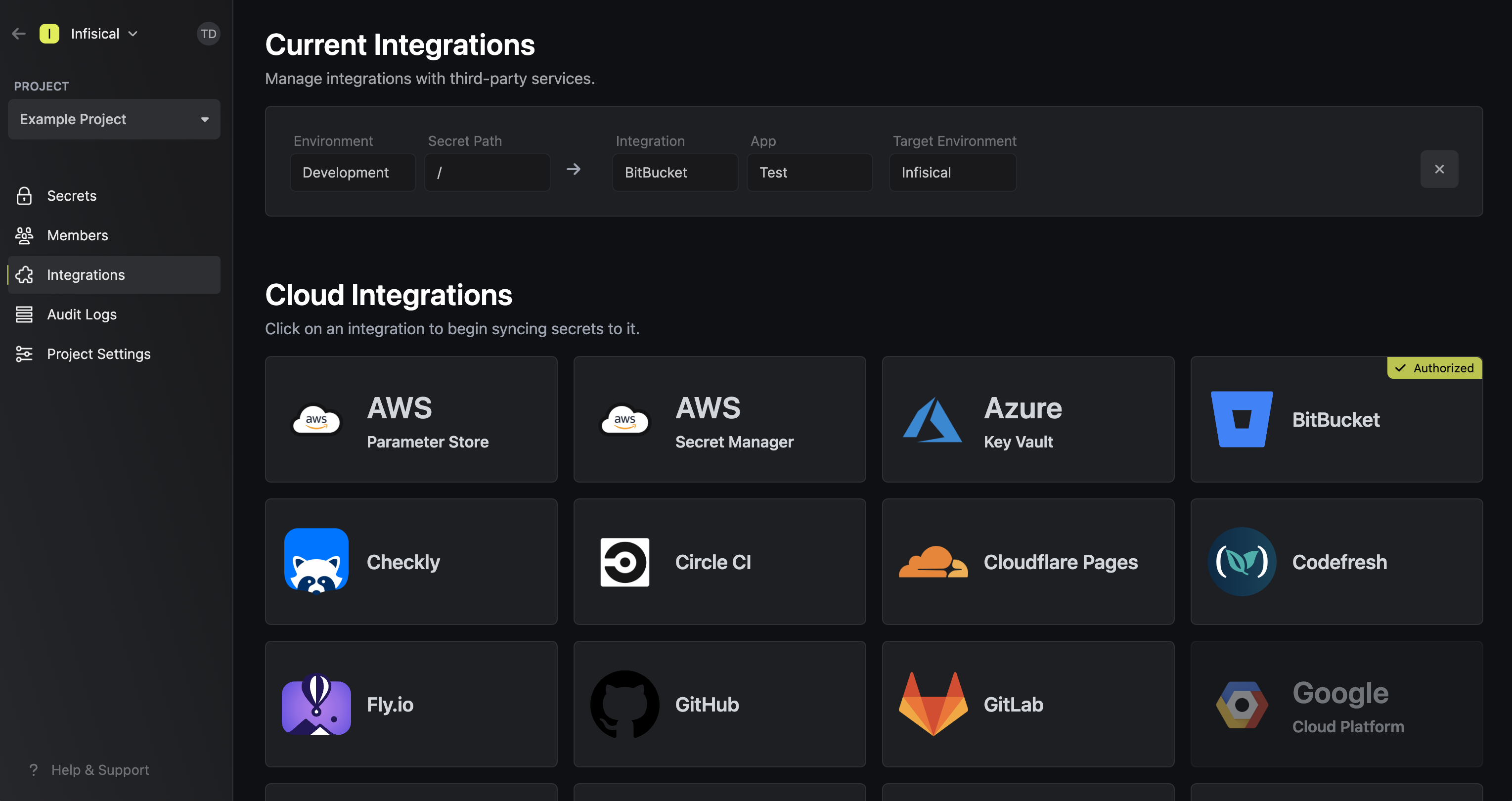
Configure a [Machine Identity](https://infisical.com/docs/documentation/platform/identities/universal-auth) for your project and give it permissions to read secrets from your desired Infisical projects and environments.
Create Bitbucket variables (can be either workspace, repository, or deployment-level) to store Machine Identity Client ID and Client Secret.
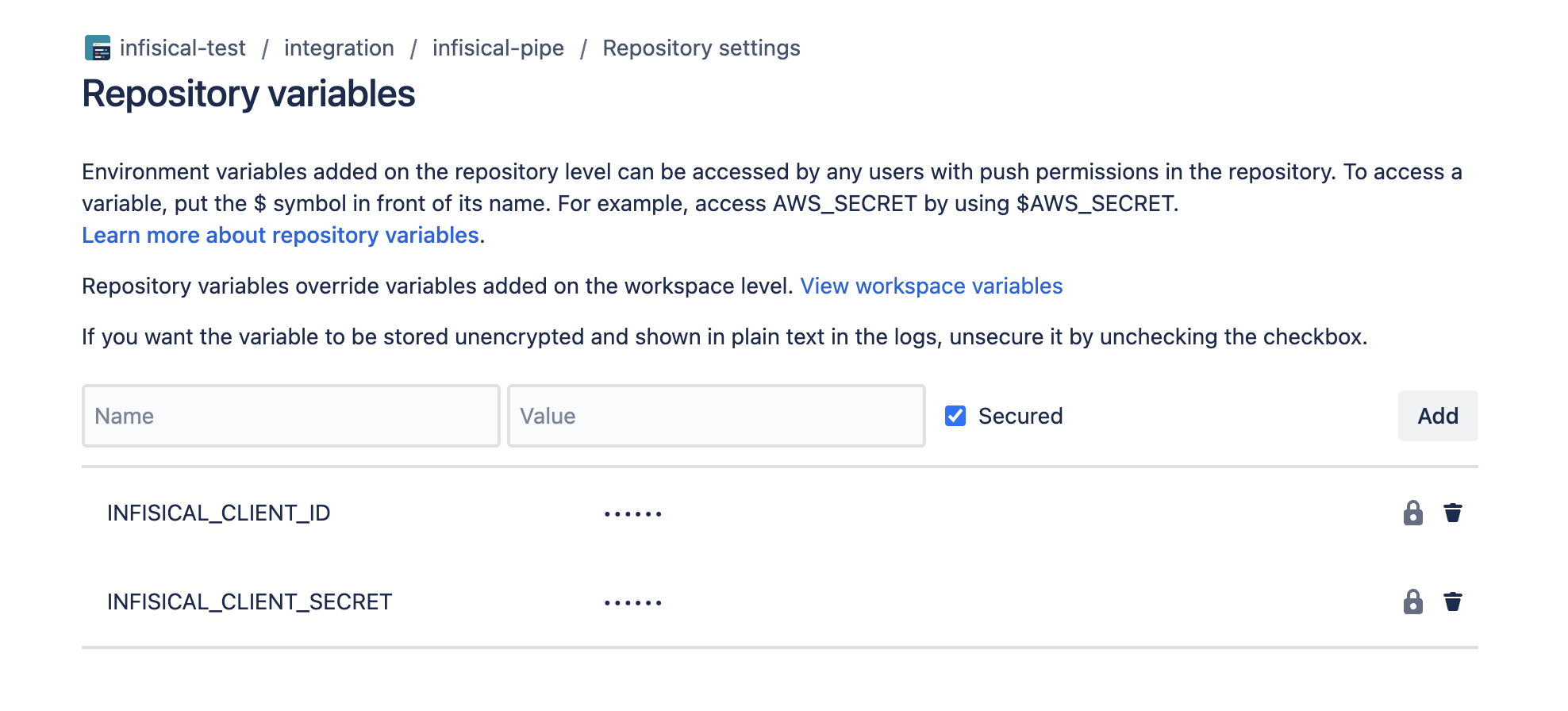
Edit your Bitbucket pipeline YAML file to include the use of the Infisical CLI to fetch and inject secrets into any script or command within the pipeline.
#### Example
```yaml
image: atlassian/default-image:3
pipelines:
default:
- step:
name: Build application with secrets from Infisical
script:
- apt update && apt install -y curl
- curl -1sLf 'https://dl.cloudsmith.io/public/infisical/infisical-cli/setup.deb.sh' | bash
- apt-get update && apt-get install -y infisical
- export INFISICAL_TOKEN=$(infisical login --method=universal-auth --client-id=$INFISICAL_CLIENT_ID --client-secret=$INFISICAL_CLIENT_SECRET --silent --plain)
- infisical run --projectId=1d0443c1-cd43-4b3a-91a3-9d5f81254a89 --env=dev -- npm run build
```
Set the values of `projectId` and `env` flags in the `infisical run` command to your intended source path. For more options, refer to the CLI command reference [here](https://infisical.com/docs/cli/commands/run).
# CircleCI
How to sync secrets from Infisical to CircleCI
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
Obtain an API token in User Settings > Personal API Tokens
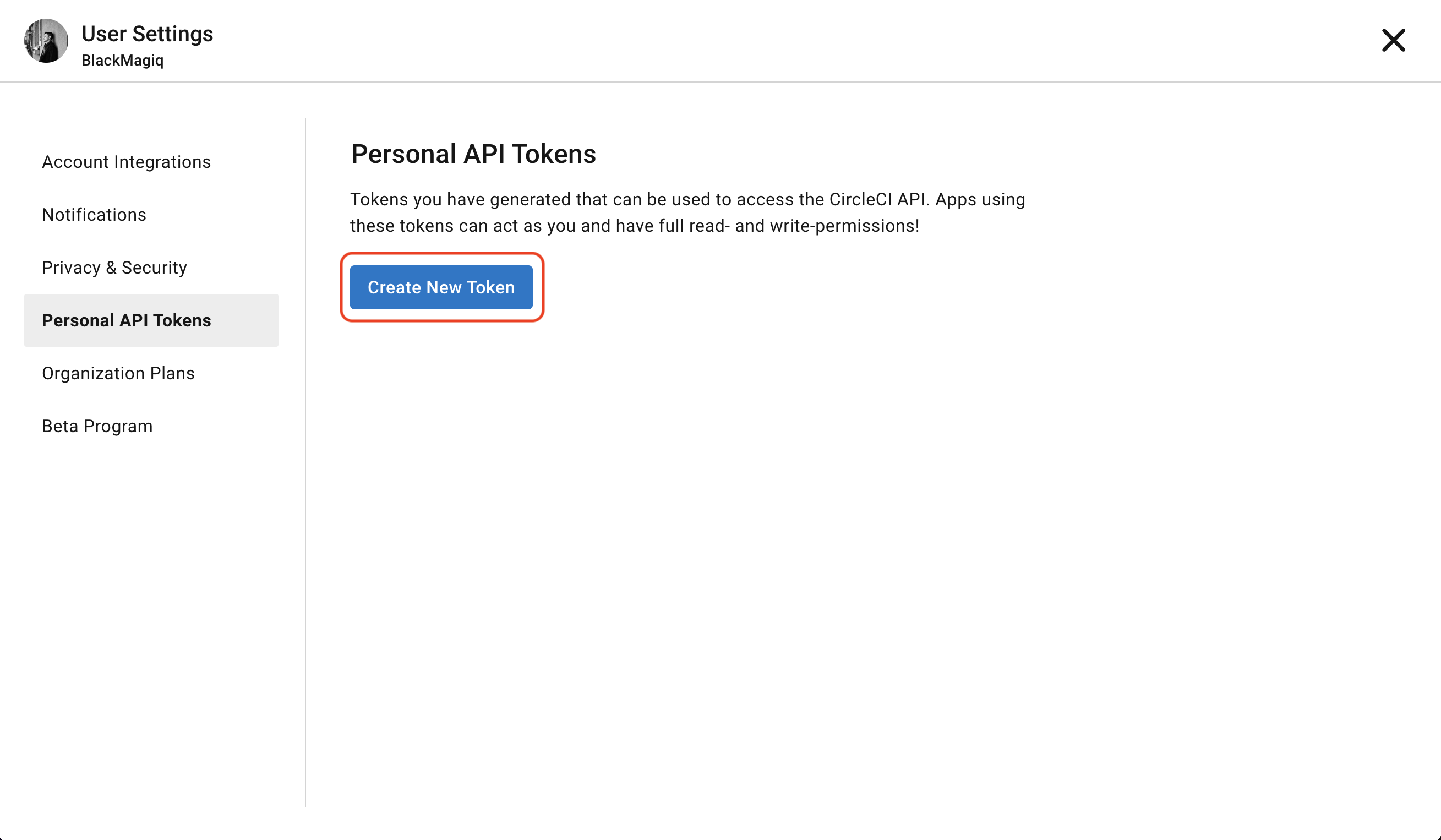
Navigate to your project's integrations tab in Infisical.
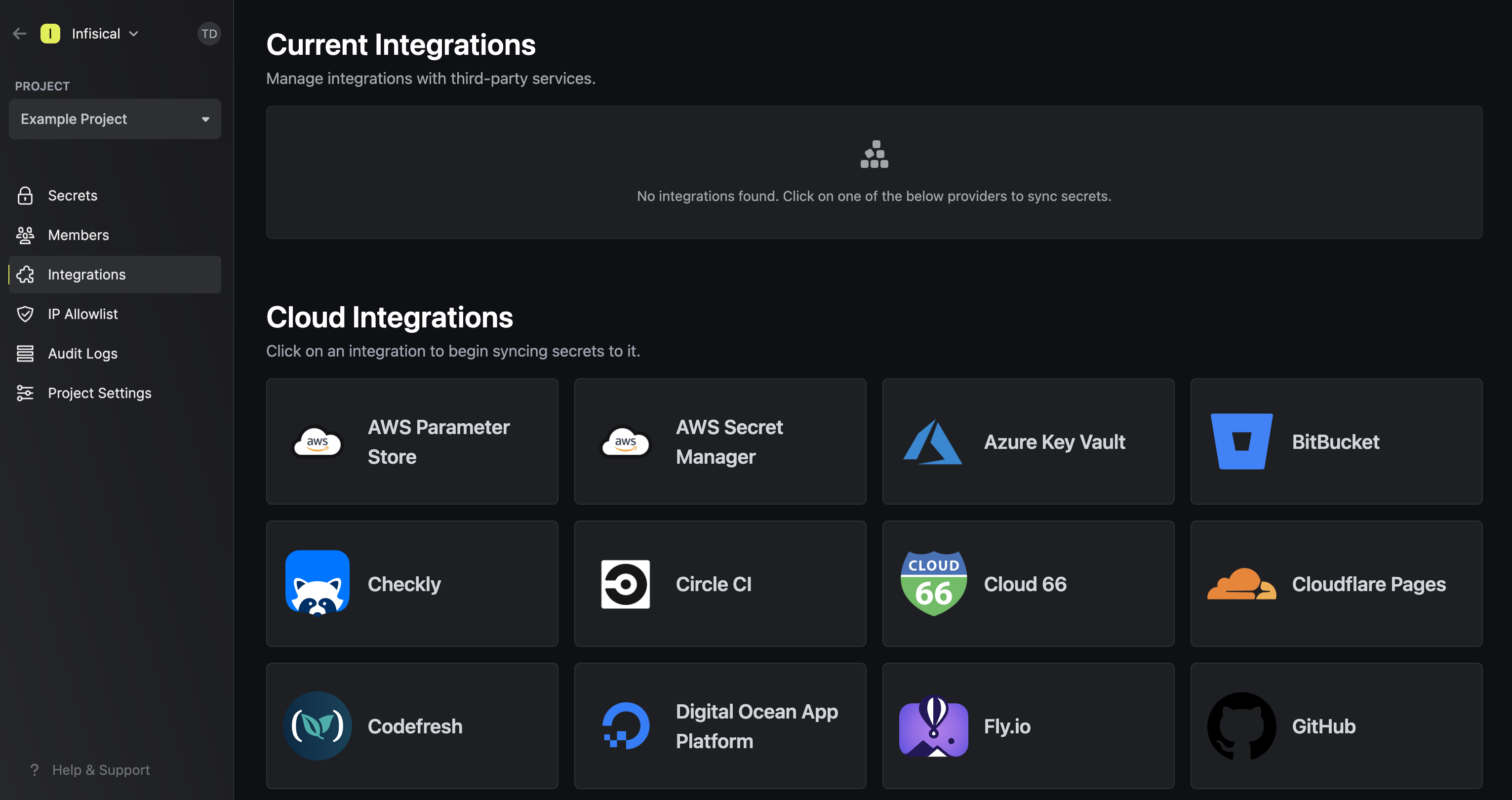
Press on the CircleCI tile and input your CircleCI API token to grant Infisical access to your CircleCI account.
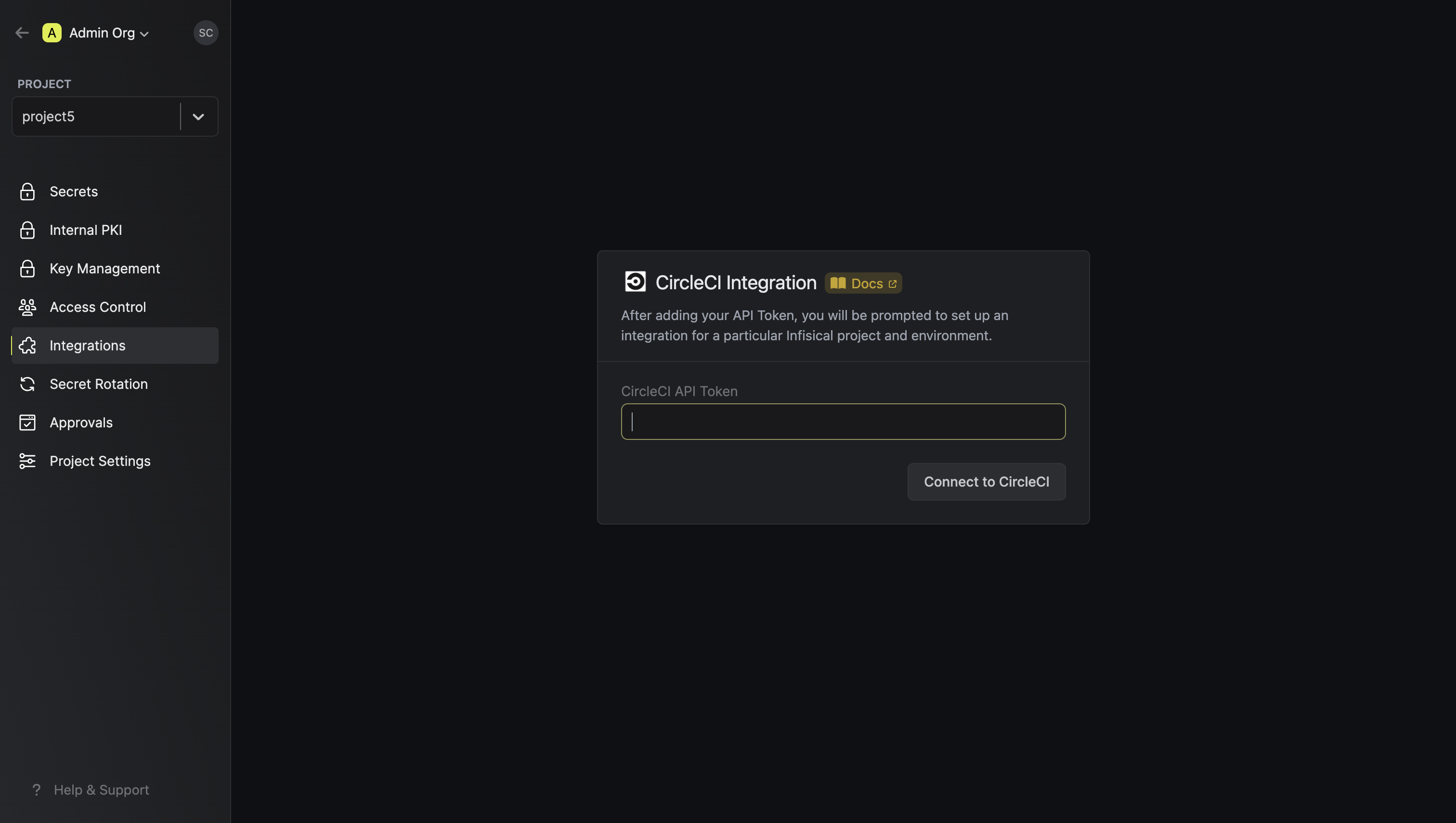
Select which Infisical environment secrets you want to sync to which CircleCI project and press create integration to start syncing secrets to CircleCI.
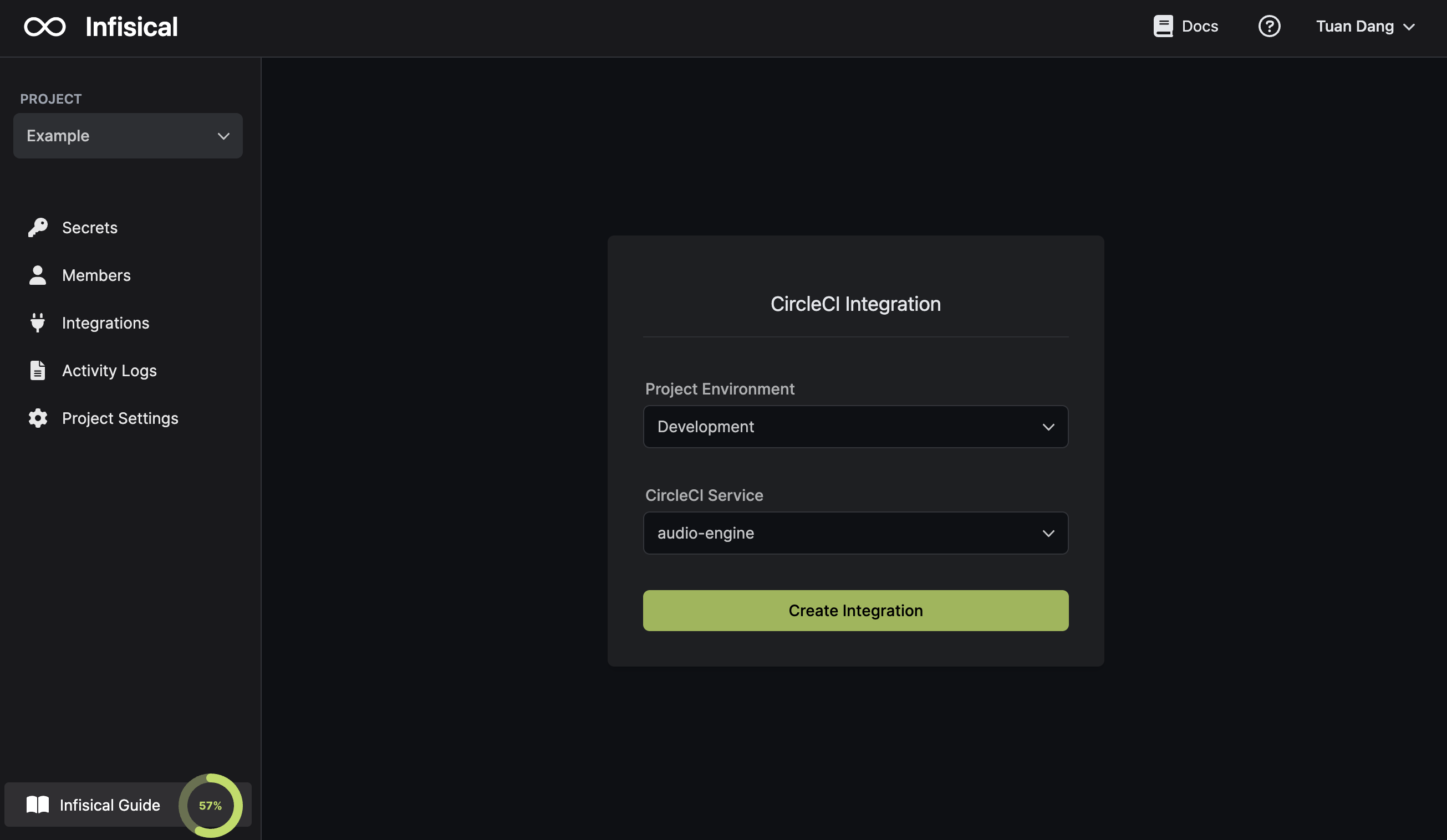
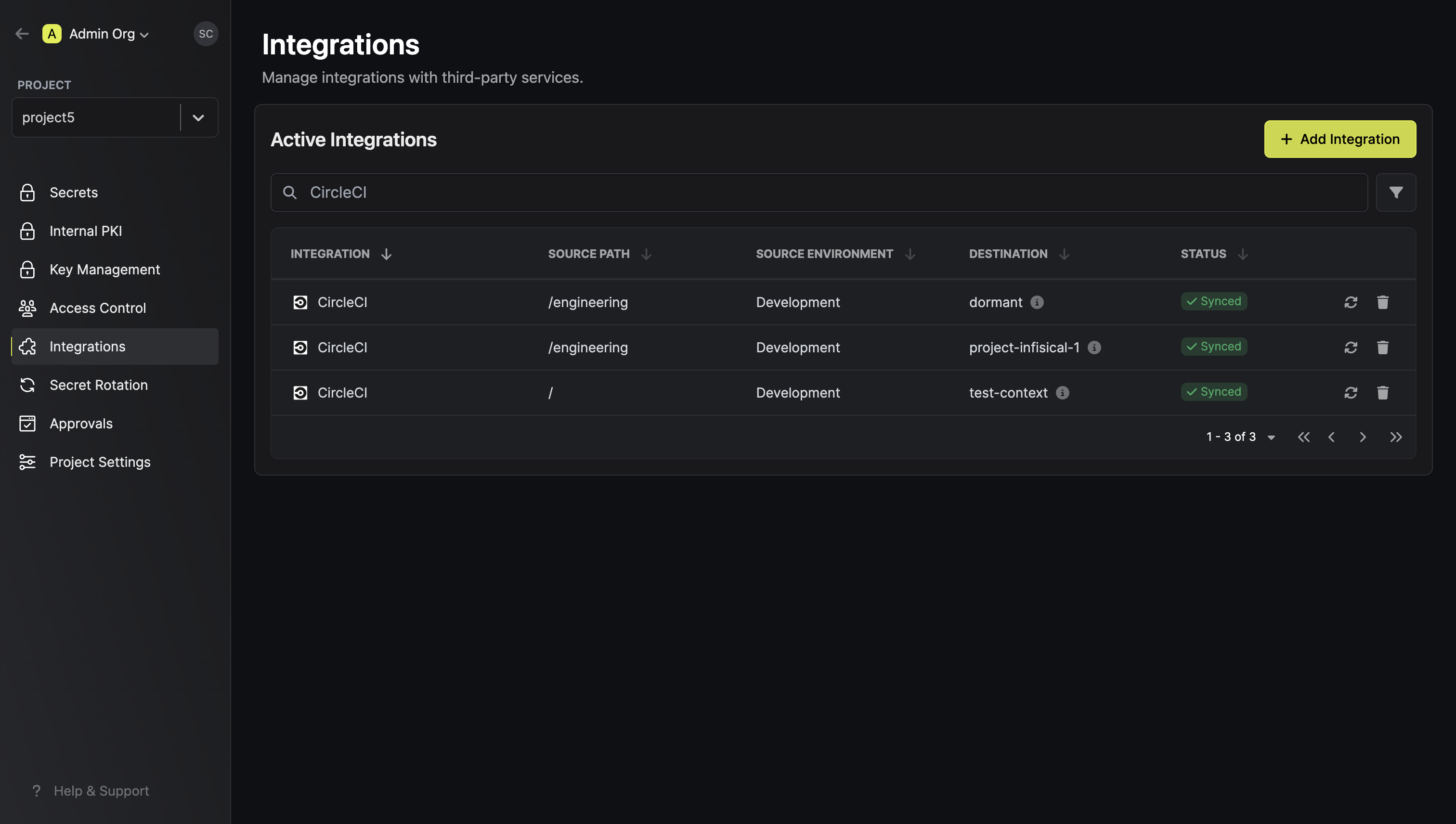
# Codefresh
How to sync secrets from Infisical to Codefresh
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
Obtain an API key in User Settings > API Keys
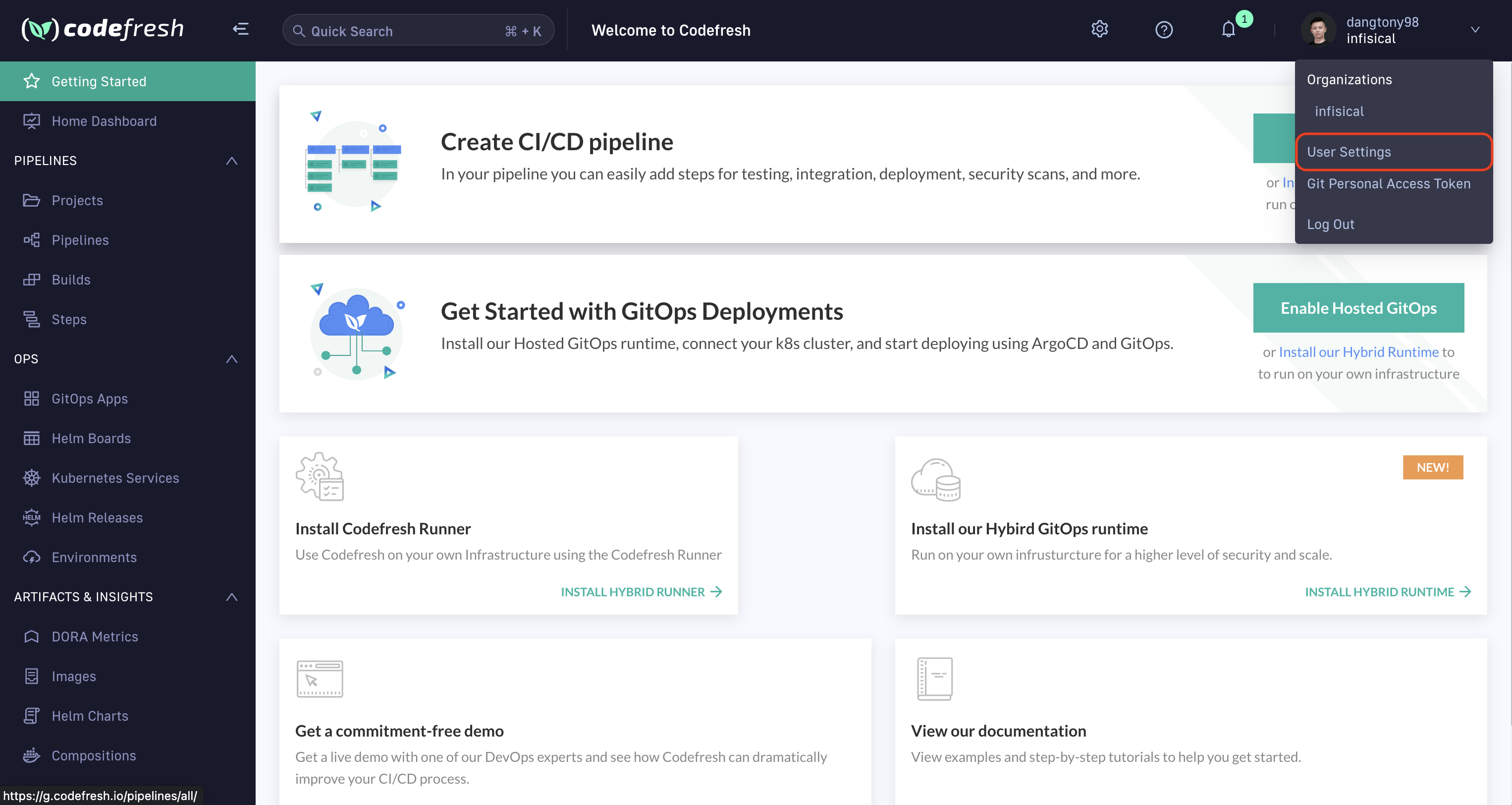
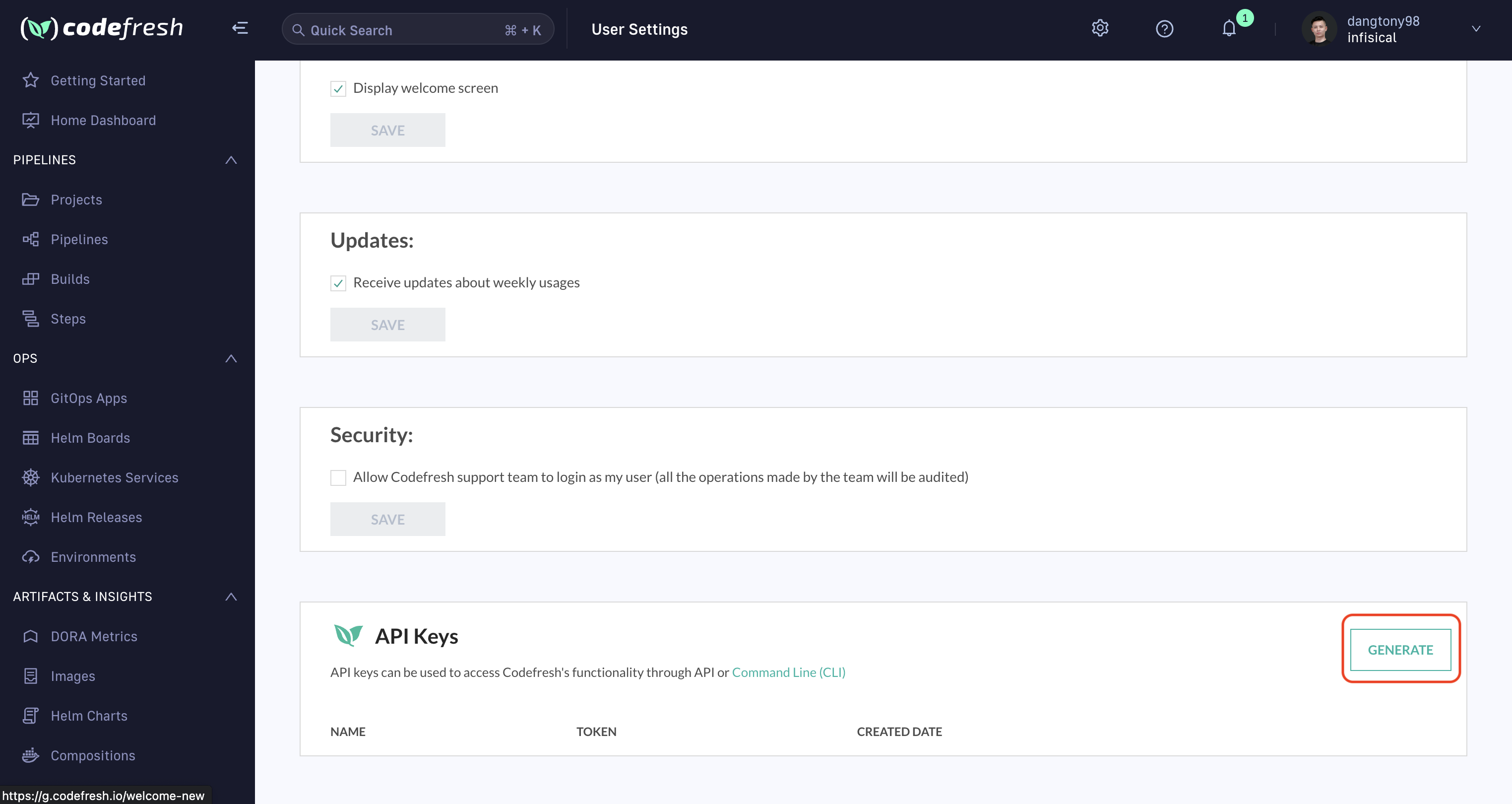
Navigate to your project's integrations tab in Infisical.
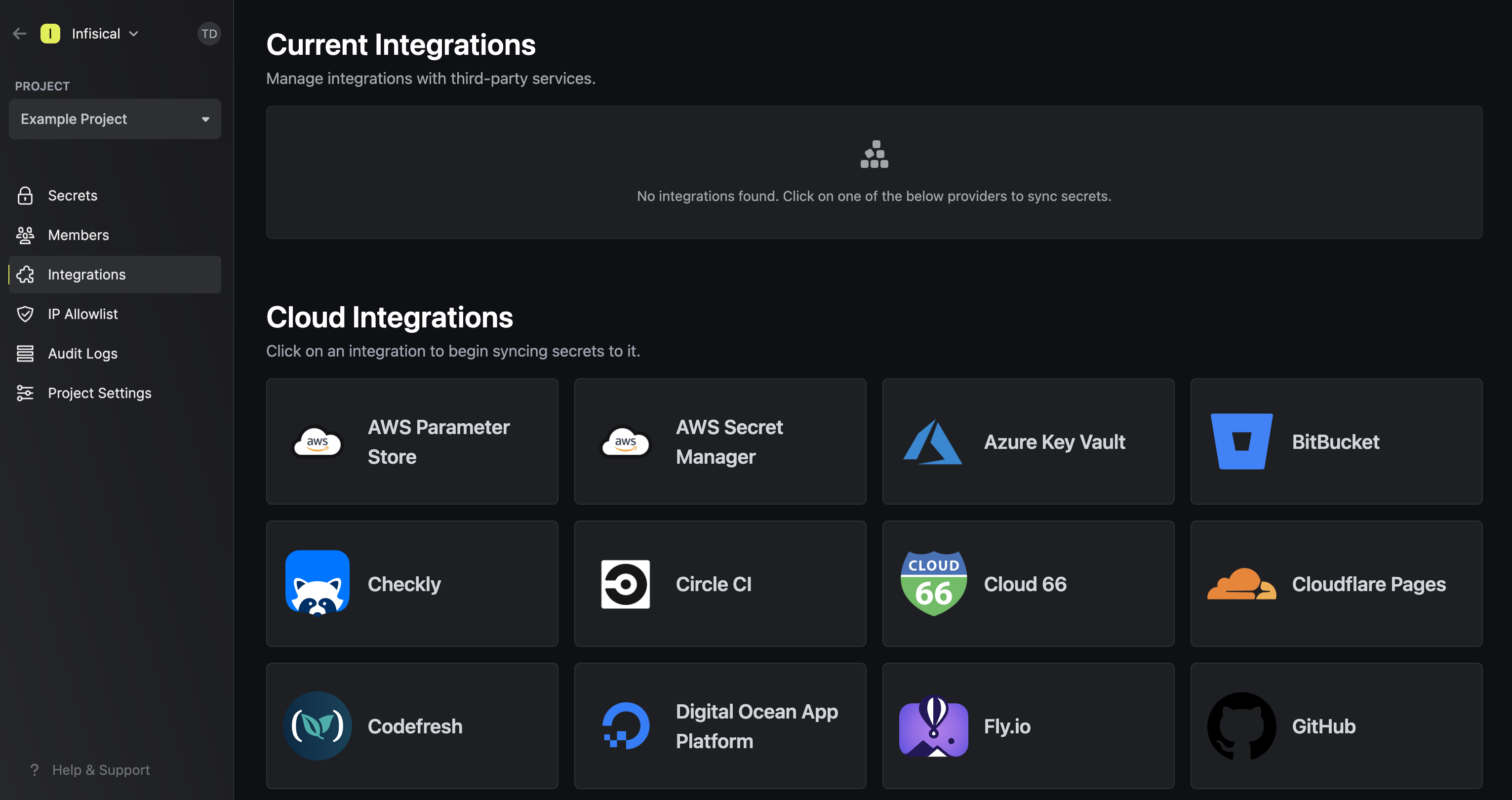
Press on the Codefresh tile and input your Codefresh API key to grant Infisical access to your Codefresh account.
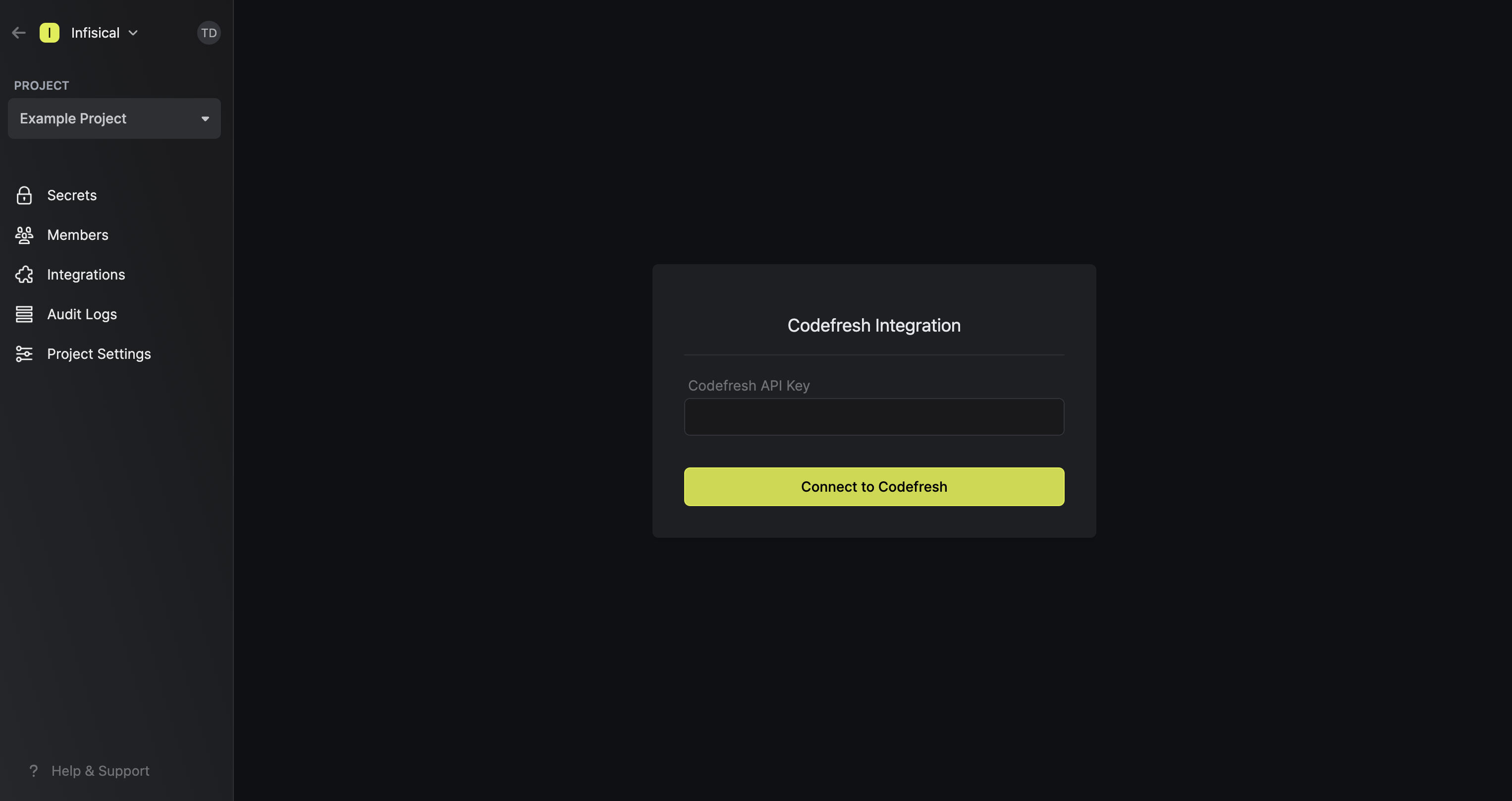
Select which Infisical environment secrets you want to sync to which Codefresh service and press create integration to start syncing secrets to Codefresh.
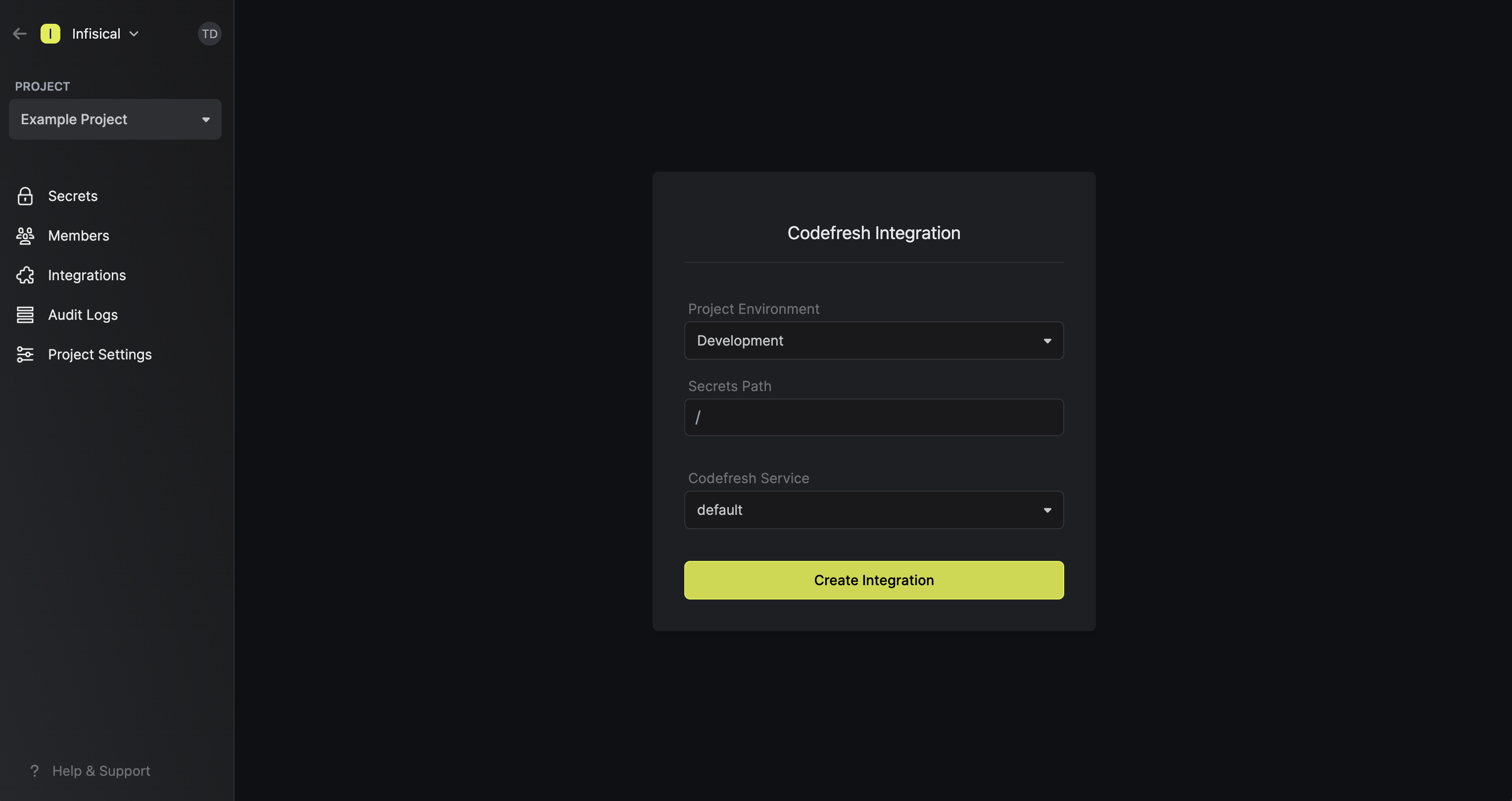
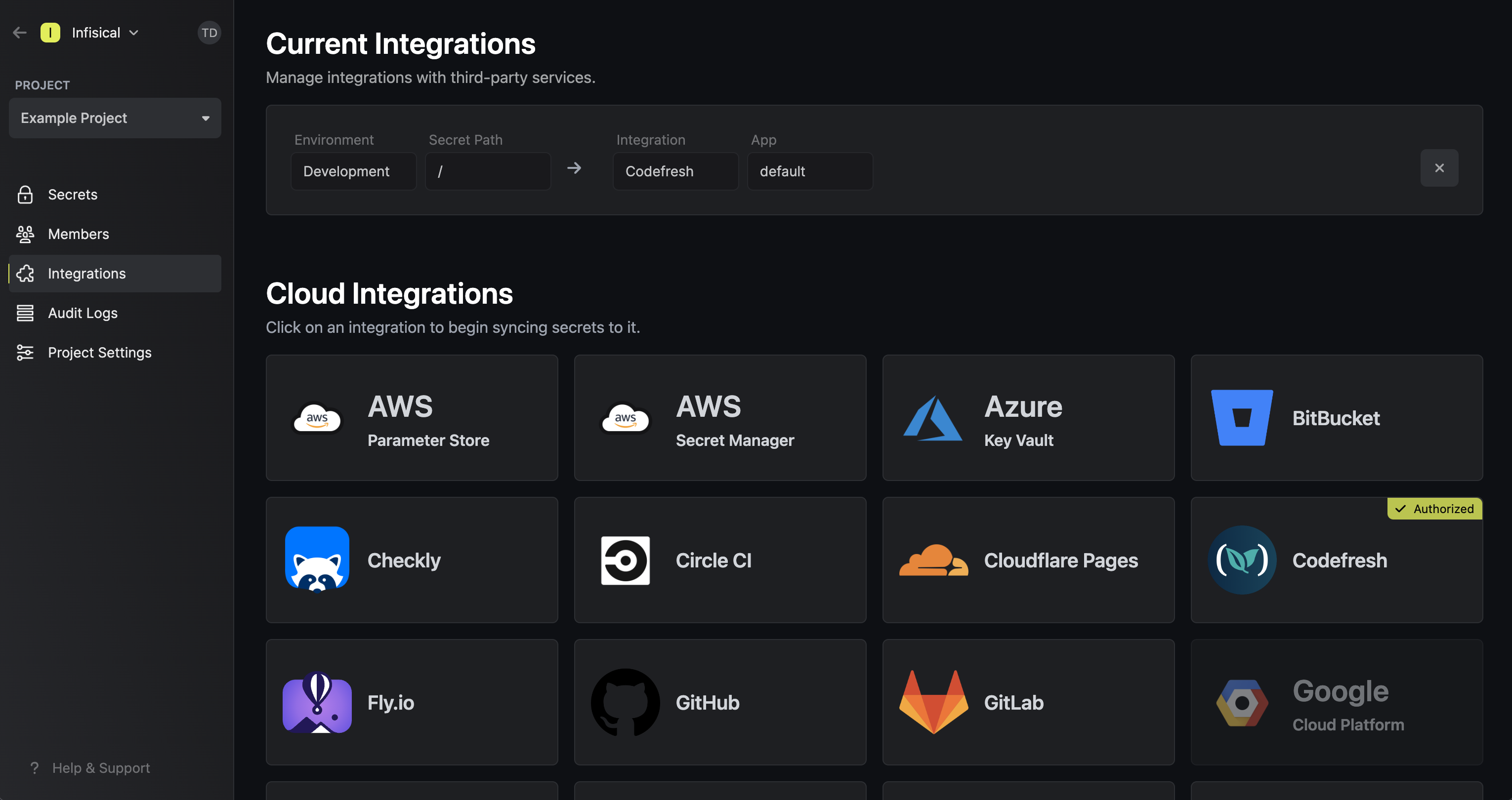
# GitHub Actions
How to sync secrets from Infisical to GitHub Actions
Alternatively, you can use Infisical's official GitHub Action
[here](https://github.com/Infisical/secrets-action).
Infisical lets you sync secrets to GitHub at the organization-level, repository-level, and repository environment-level.
## Connecting with GitHub App (Recommended)
Navigate to your project's integrations tab in Infisical and press on the GitHub tile.
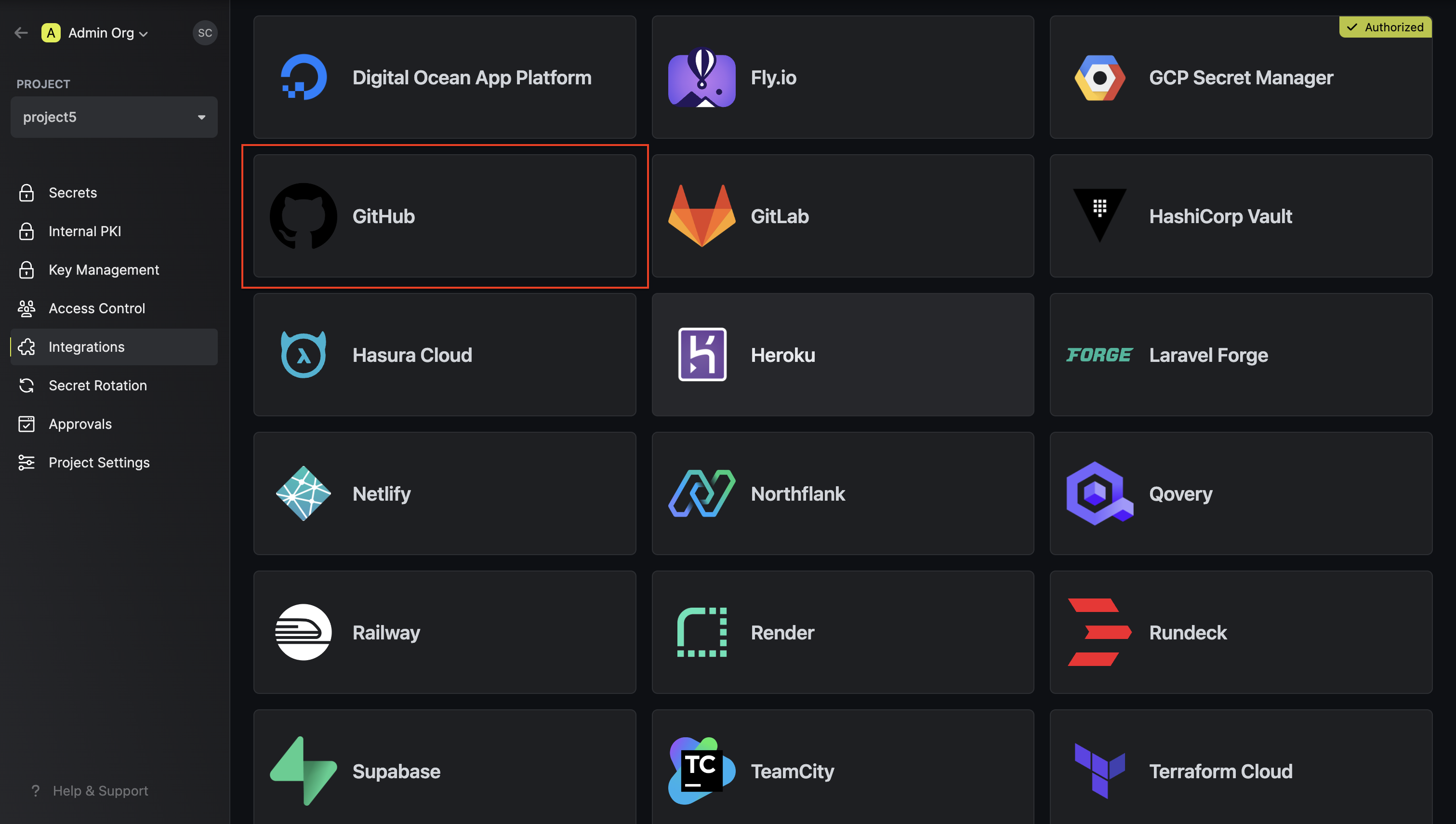
Select GitHub App as the authentication method and click **Connect to GitHub**.
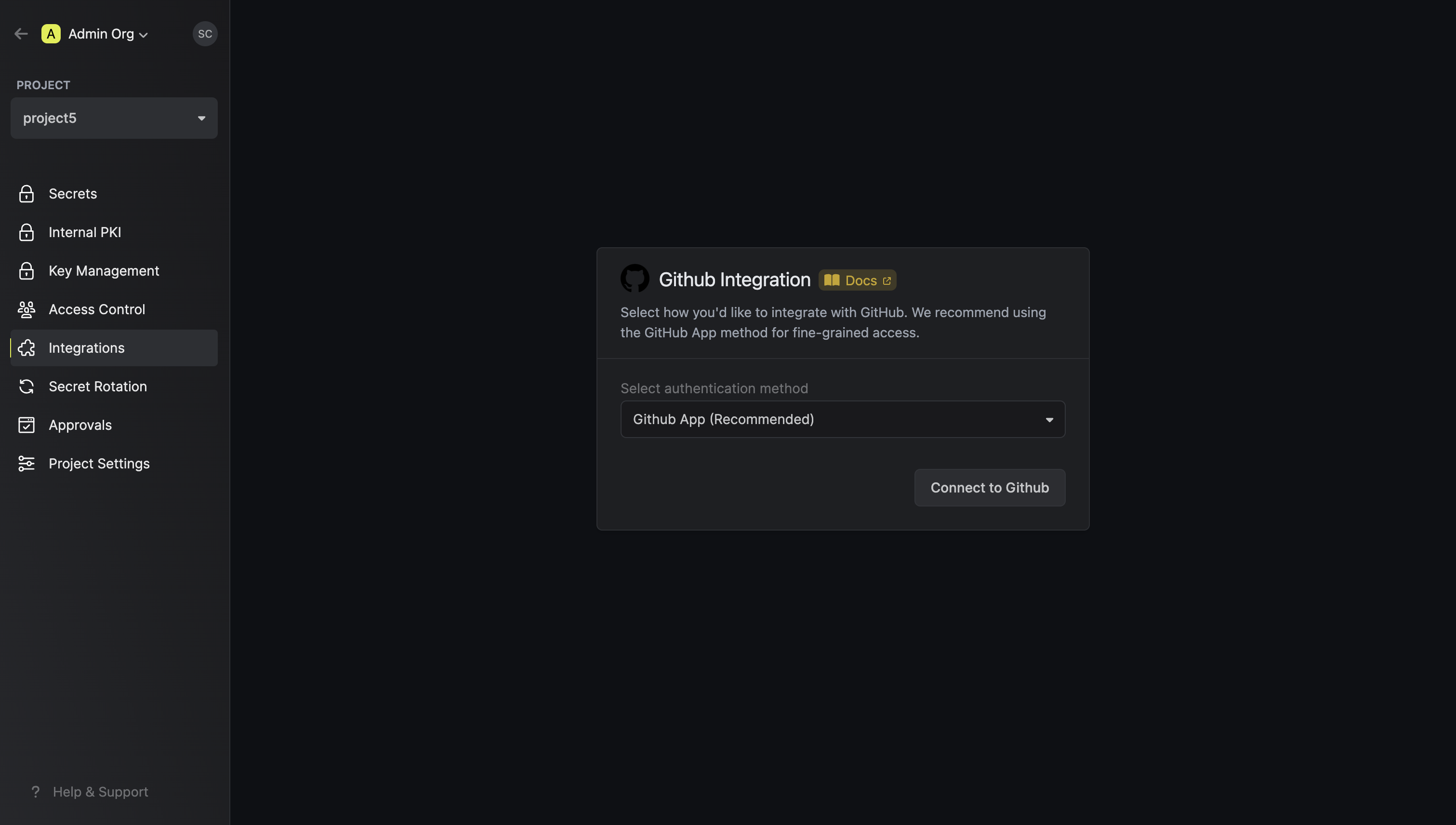
You will then be redirected to the GitHub app installation page.
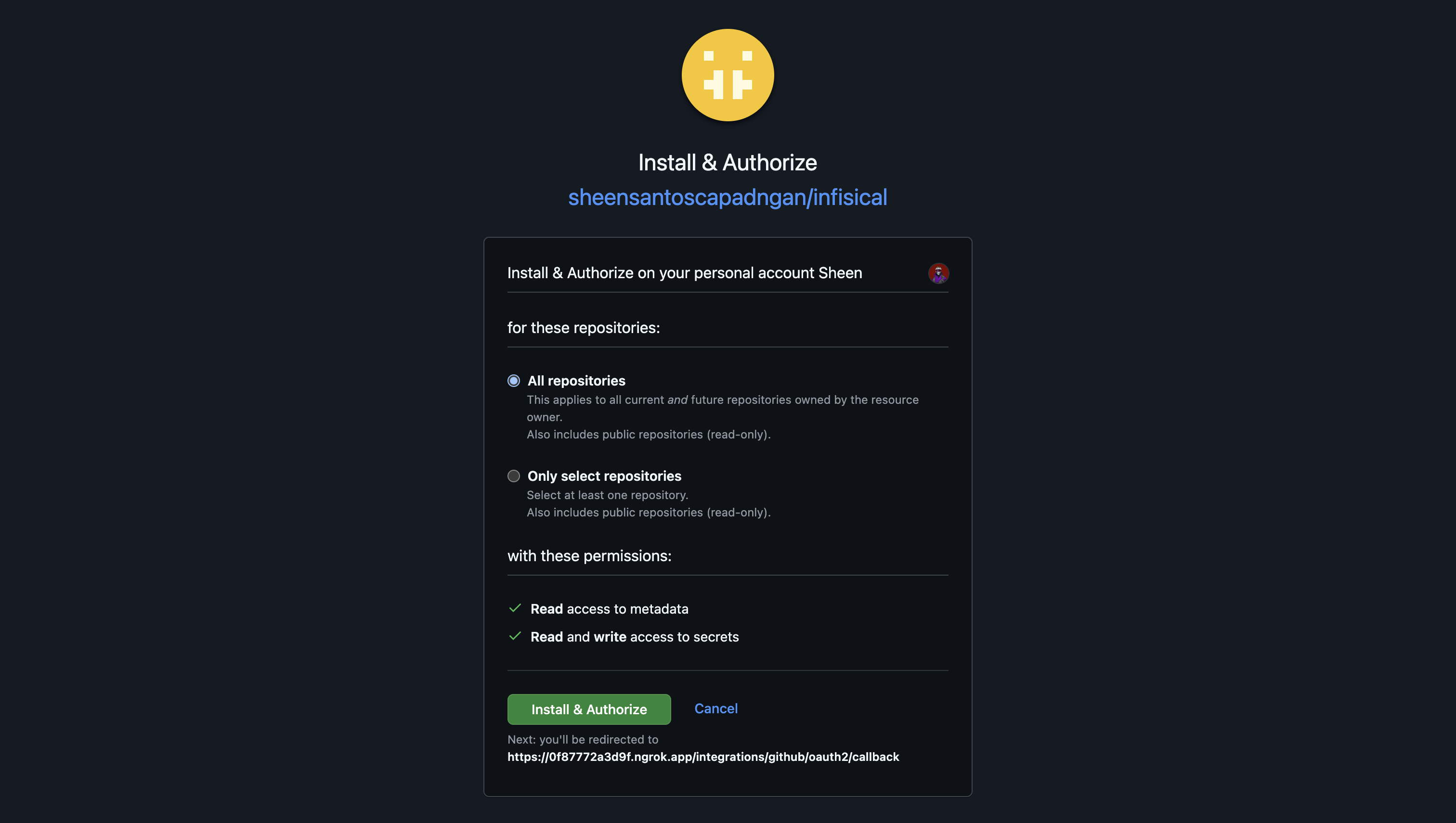
Install and authorize the GitHub application. This will redirect you back to the Infisical integration page.
Select which Infisical environment secrets you want to sync to which GitHub organization, repository, or repository environment.
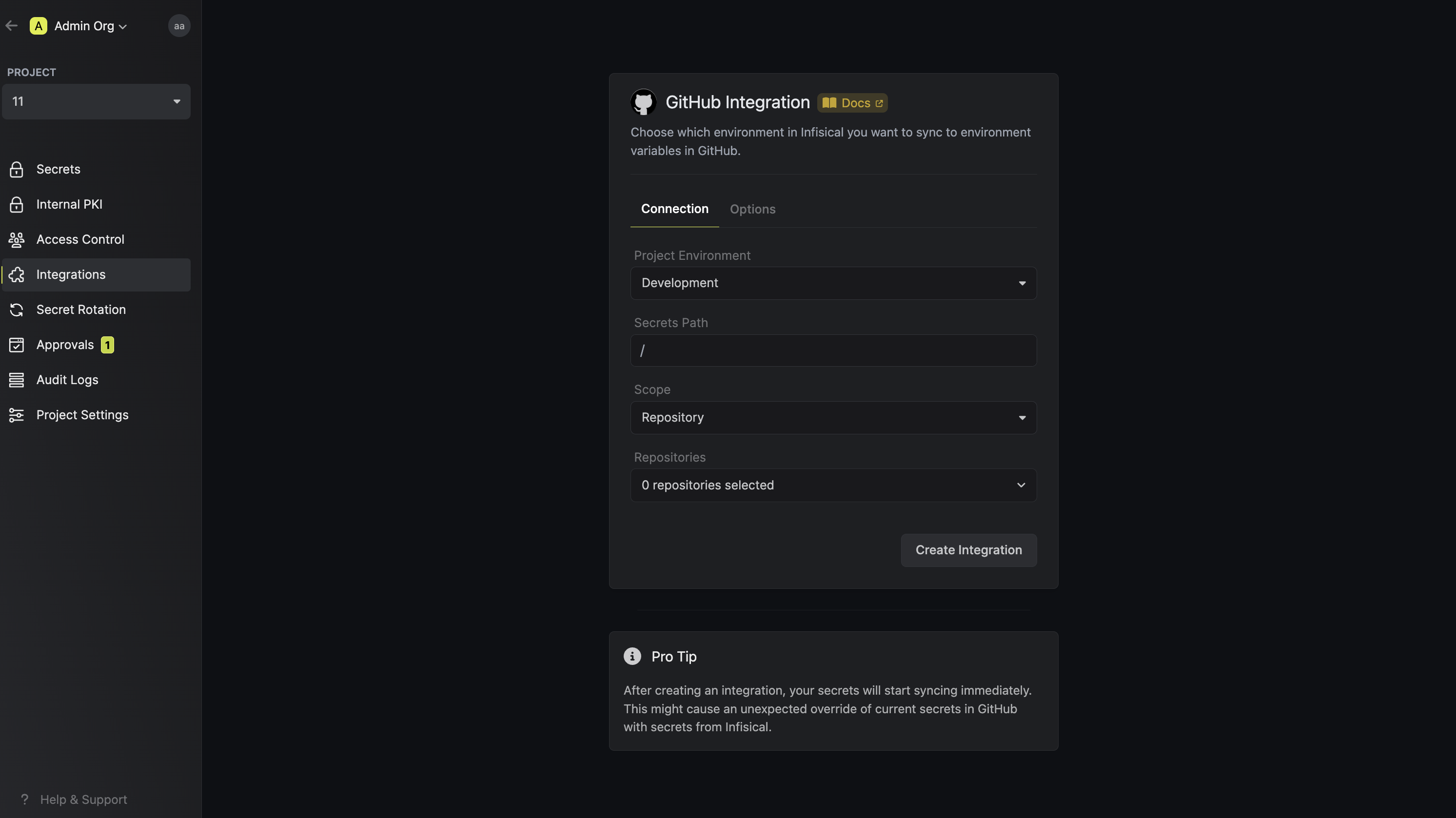
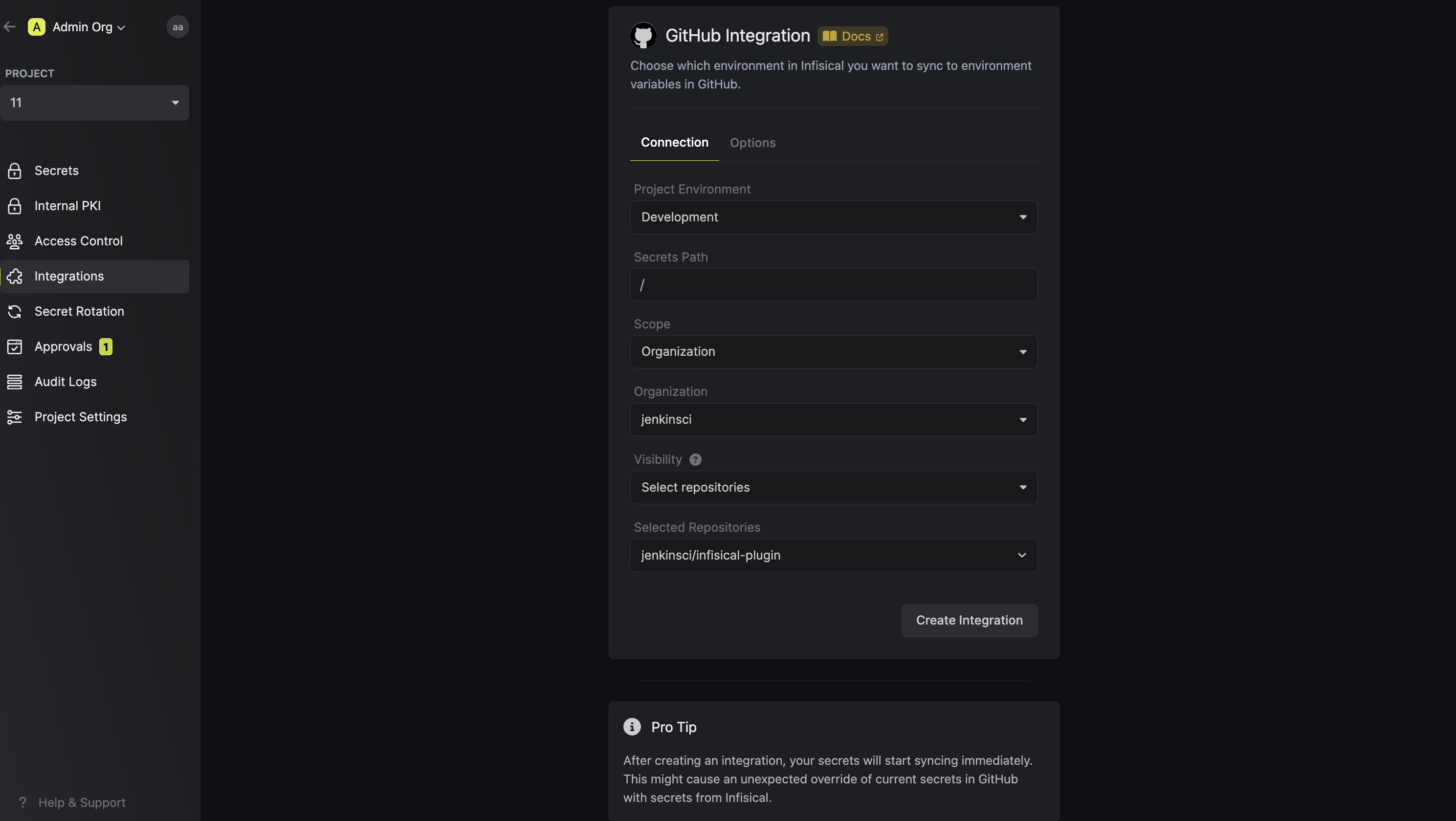
When using the organization scope, your secrets will be saved in the top-level of your GitHub Organization.
You can choose the visibility, which defines which repositories can access the secrets. The options are:
* **All public repositories**: All public repositories in the organization can access the secrets.
* **All private repositories**: All private repositories in the organization can access the secrets.
* **Selected repositories**: Only the selected repositories can access the secrets. This gives a more fine-grained control over which repositories can access the secrets. You can select *both* private and public repositories with this option.
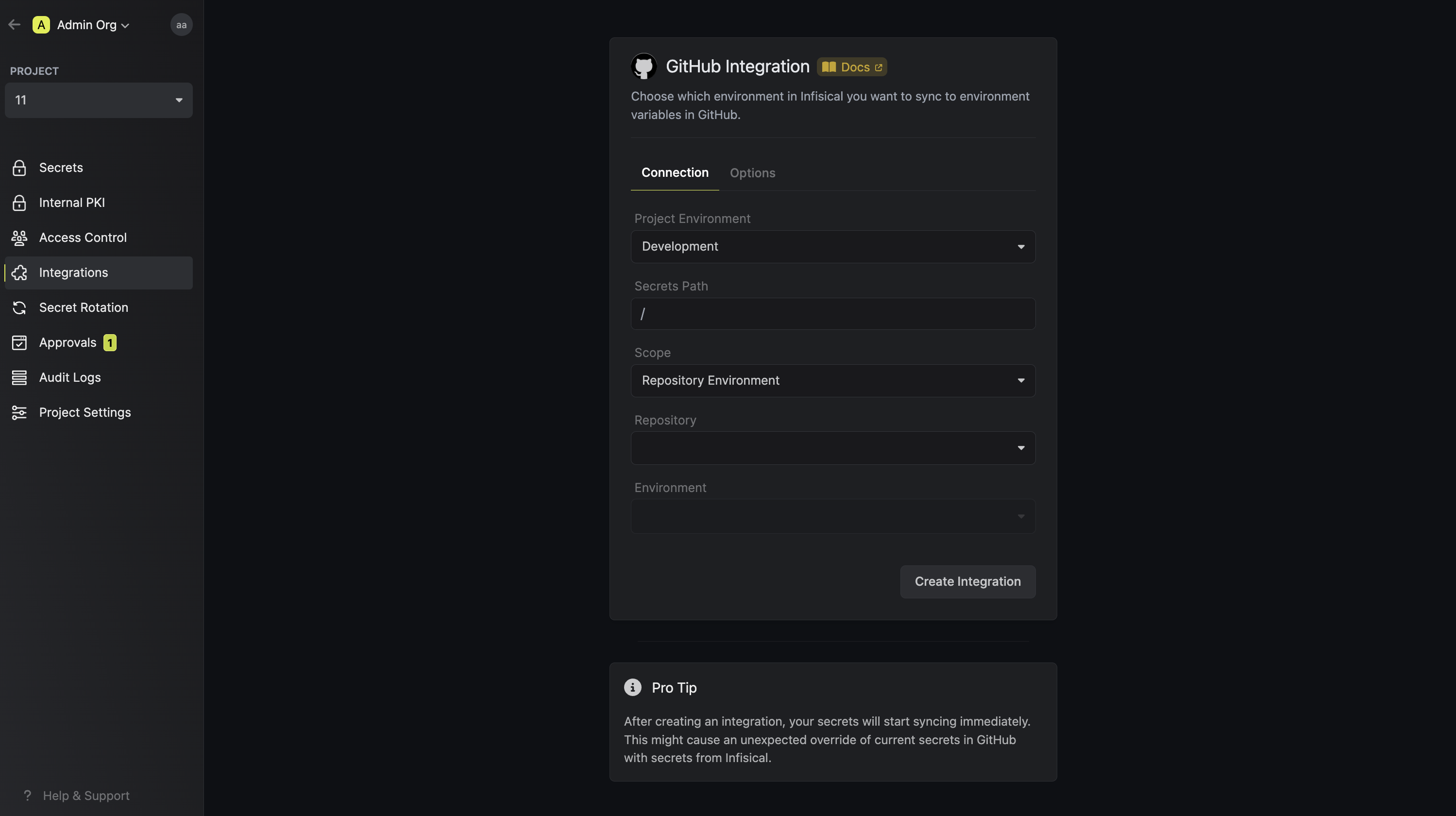
Finally, press create integration to start syncing secrets to GitHub.
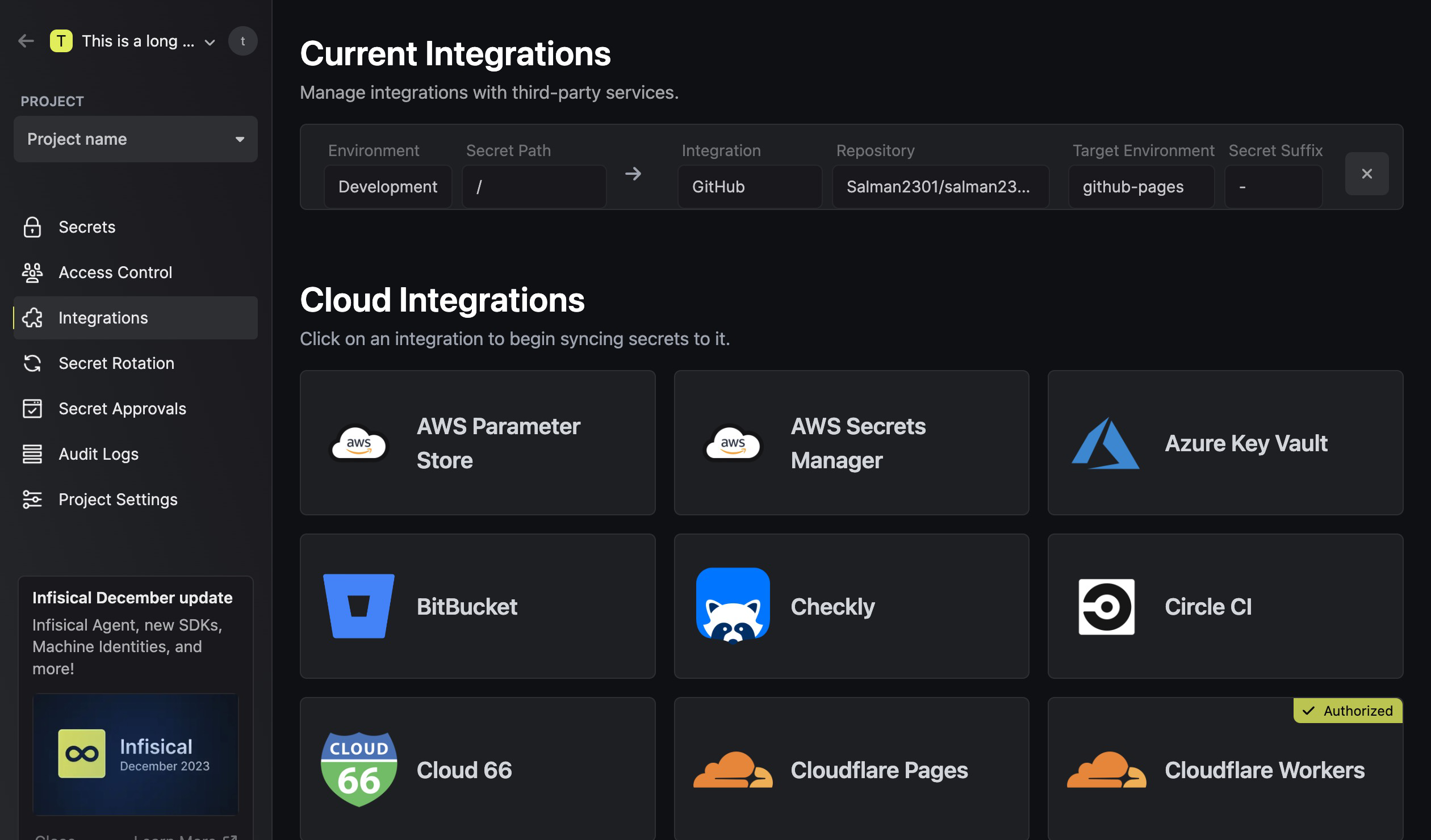
Using the GitHub integration with app authentication on a self-hosted instance of Infisical requires configuring an application on GitHub
and registering your instance with it.
Navigate to the GitHub app settings [here](https://github.com/settings/apps). Click **New GitHub App**.
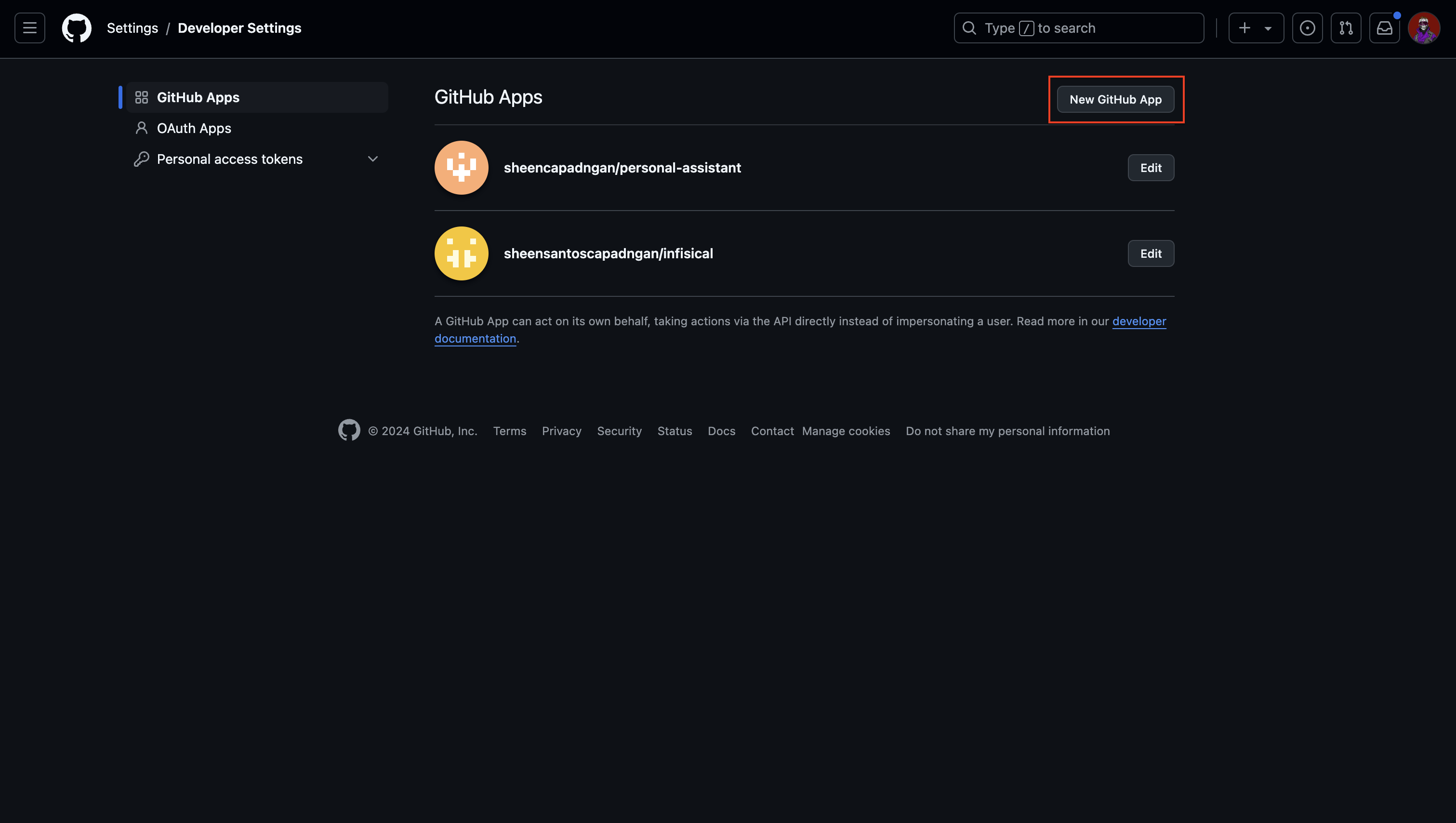
Give the application a name, a homepage URL (your self-hosted domain i.e. `https://your-domain.com`), and a callback URL (i.e. `https://your-domain.com/integrations/github/oauth2/callback`).
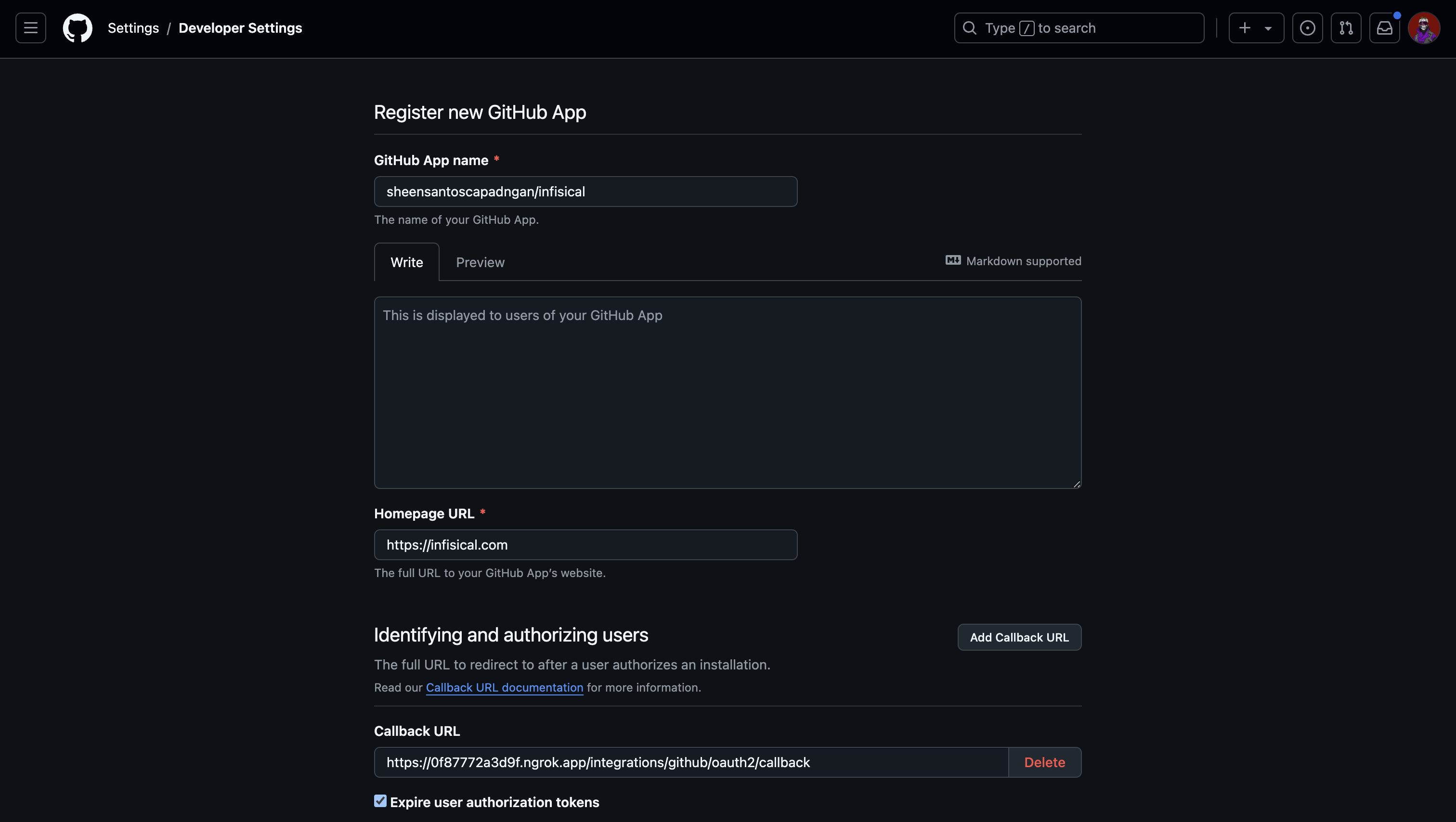
Enable request user authorization during app installation.
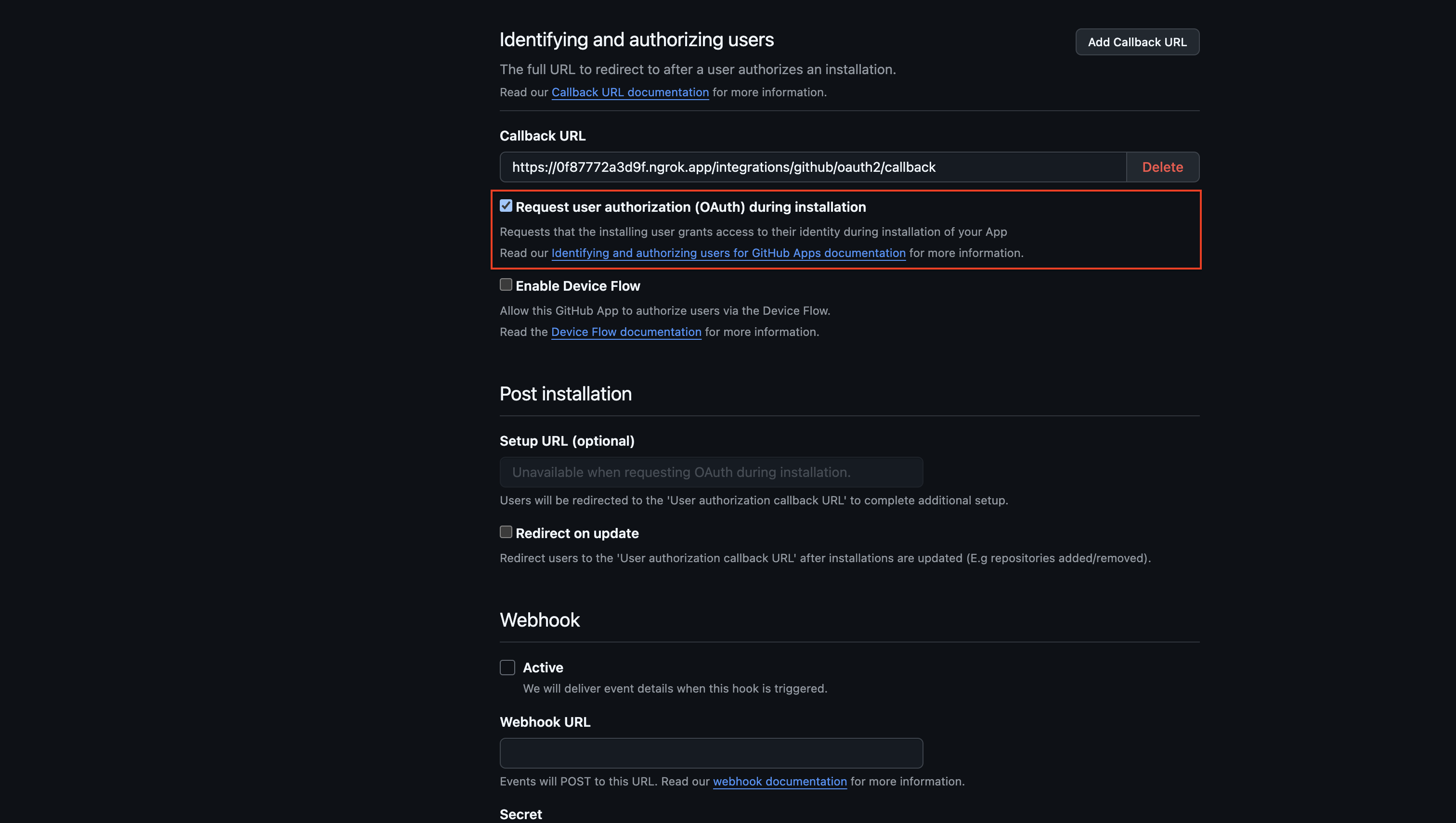
Disable webhook by unchecking the Active checkbox.
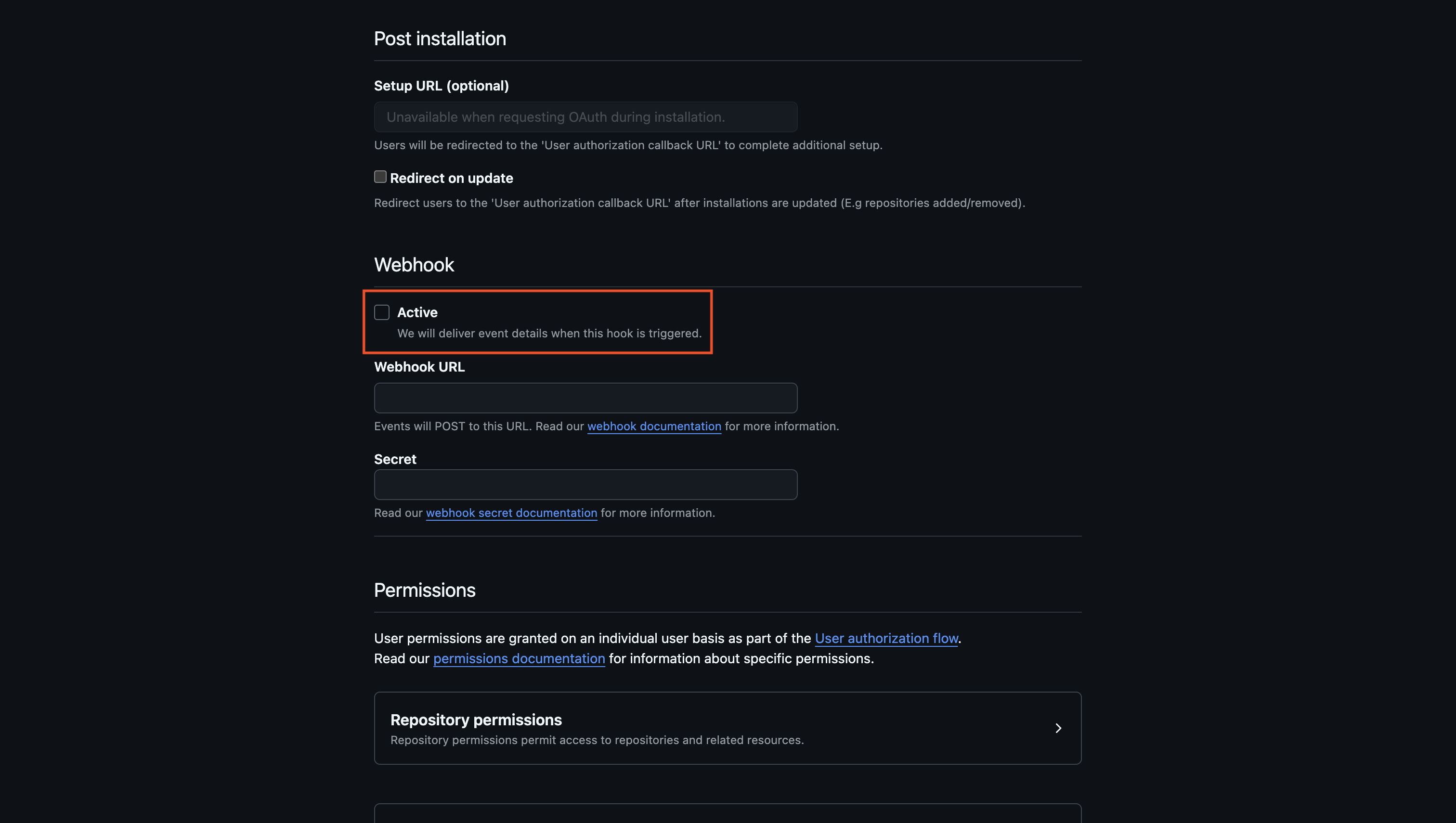
Set the repository permissions as follows: Metadata: Read-only, Secrets: Read and write, Environments: Read and write, Actions: Read.
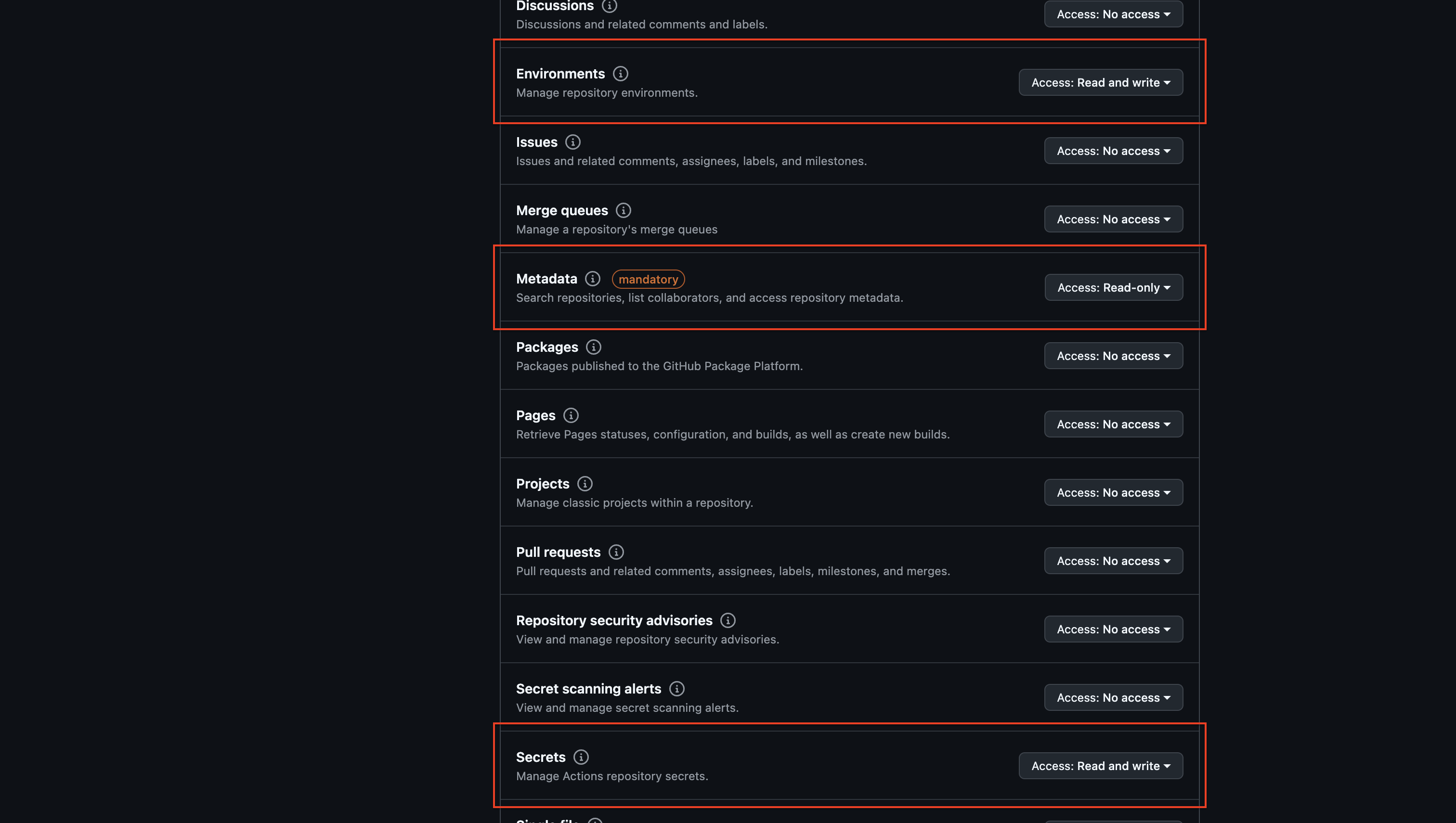
Similarly, set the organization permissions as follows: Secrets: Read and write.
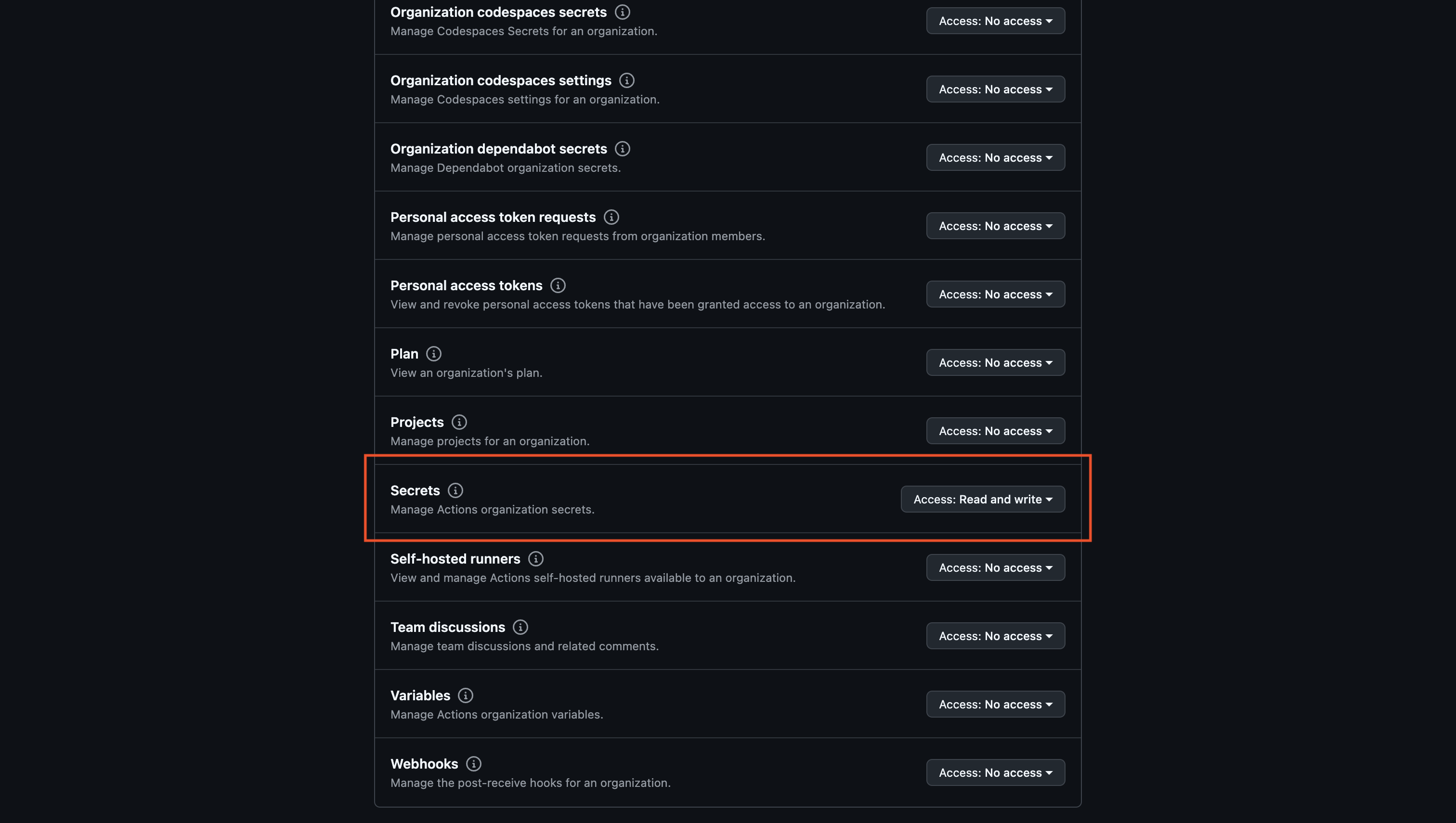
Create the Github application.
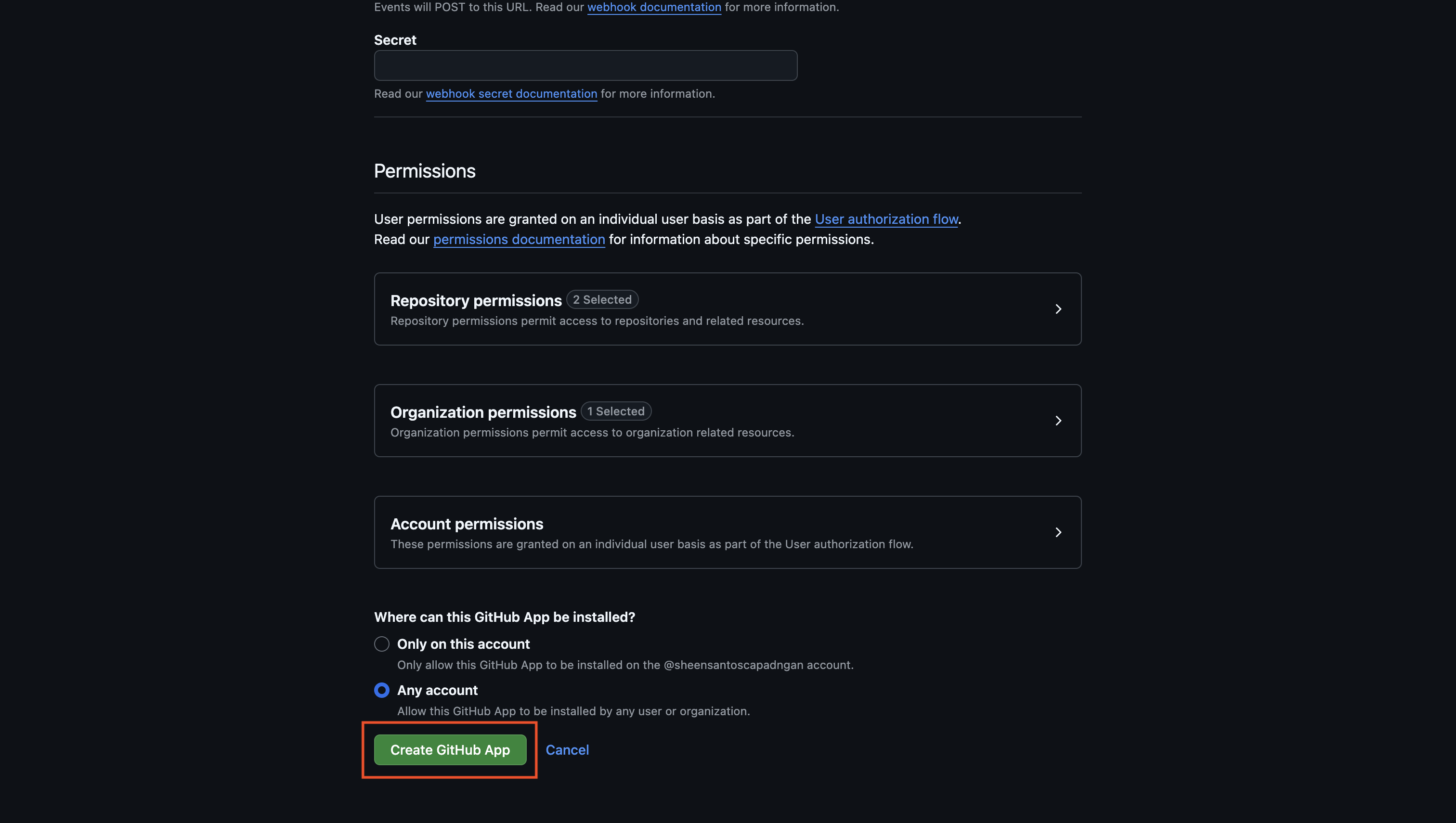
If you have a GitHub organization, you can create an application under it
in your organization Settings > Developer settings > GitHub Apps > New GitHub App.
Generate a new **Client Secret** for your GitHub application.
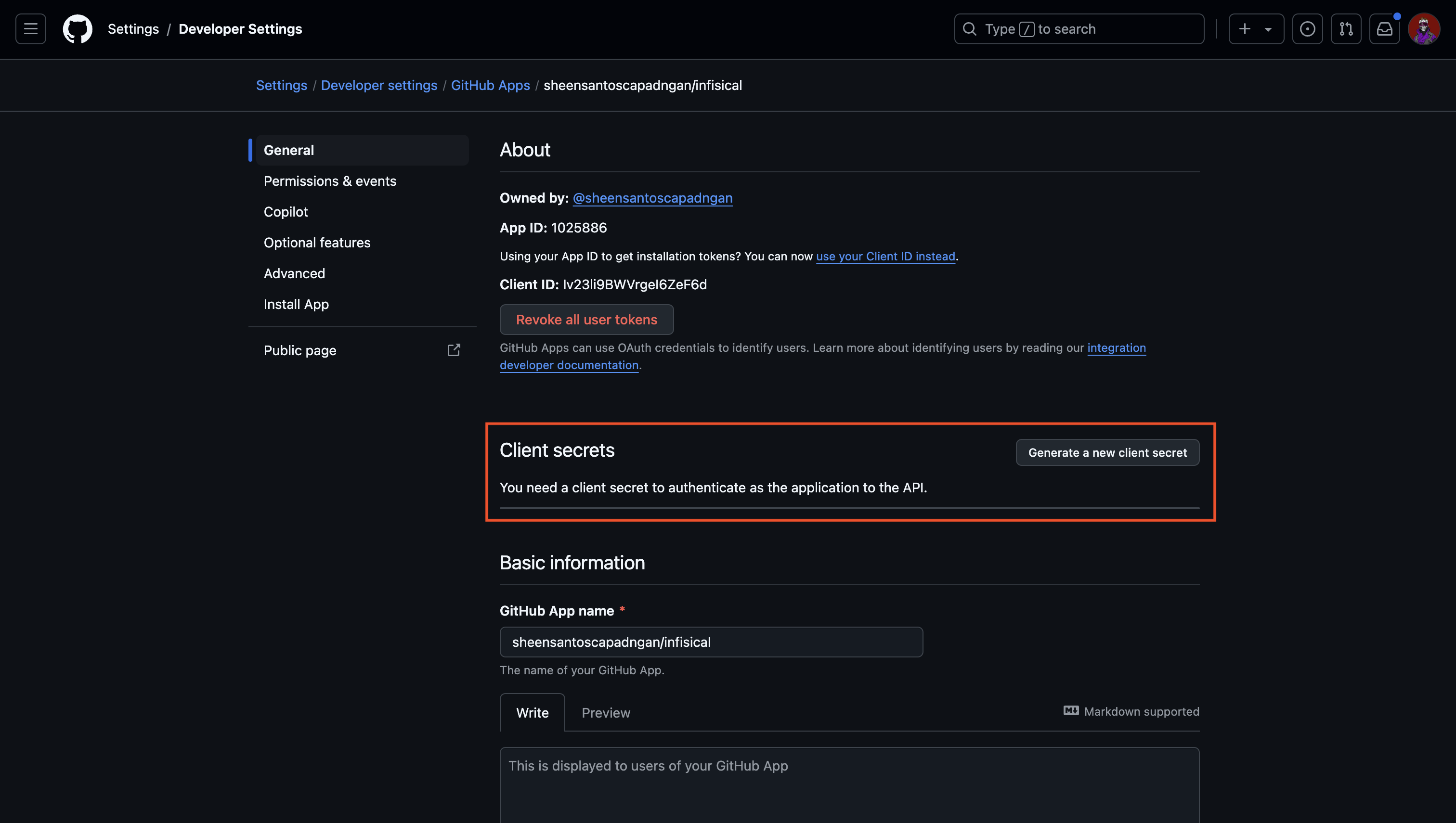
Generate a new **Private Key** for your Github application.
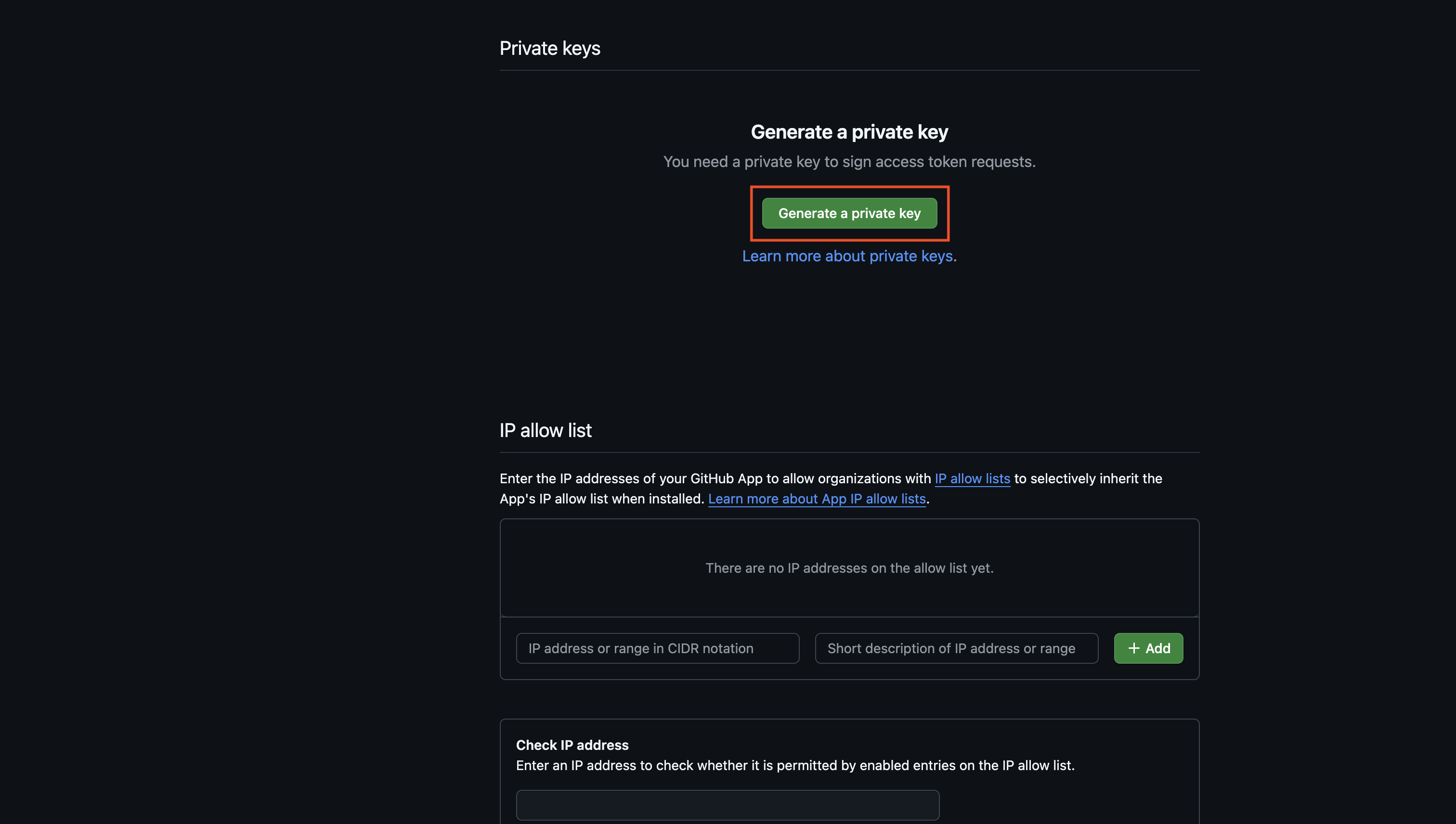
Obtain the necessary Github application credentials. This would be the application slug, client ID, app ID, client secret, and private key.
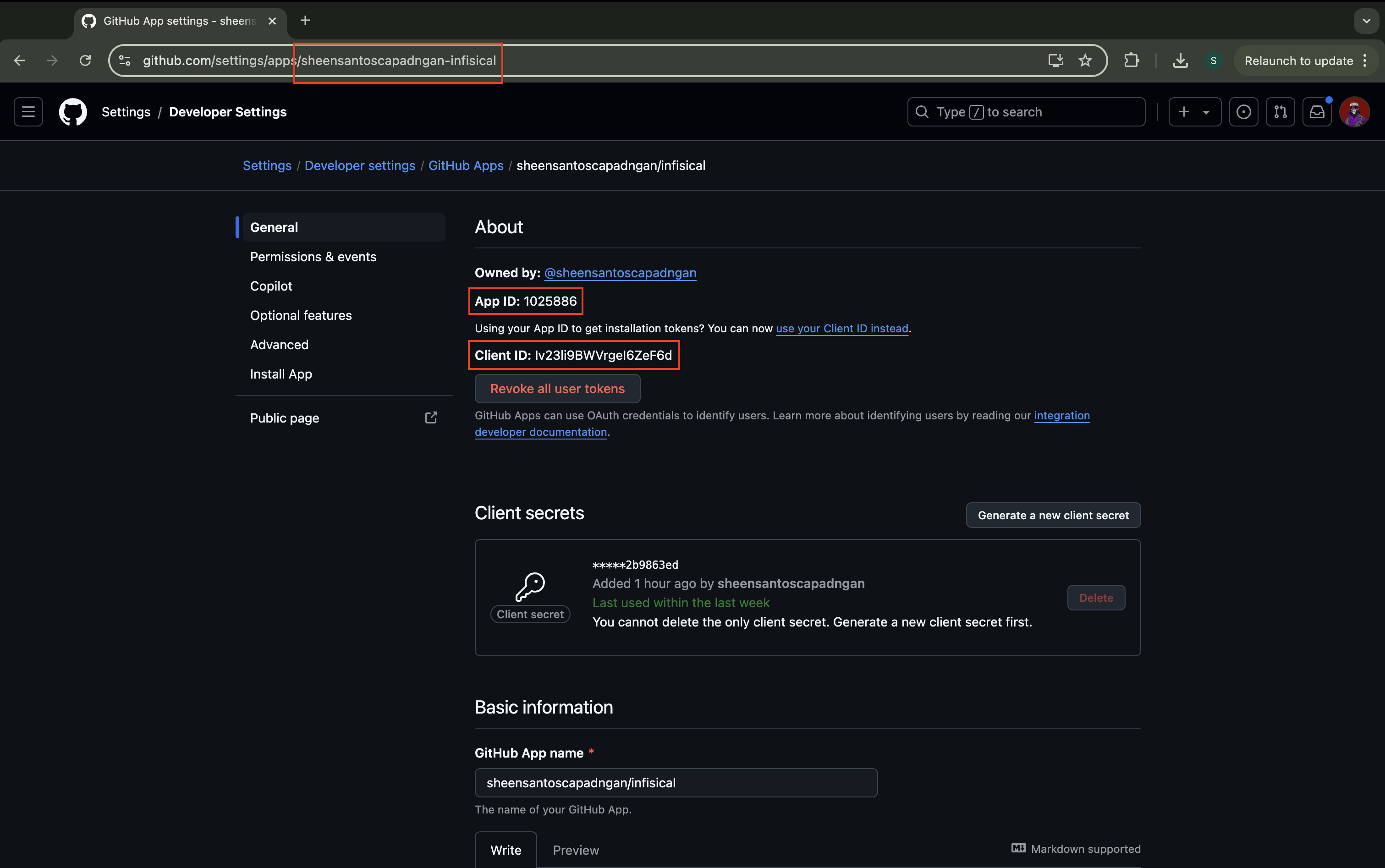
Back in your Infisical instance, add the five new environment variables for the credentials of your GitHub application:
* `CLIENT_ID_GITHUB_APP`: The **Client ID** of your GitHub application.
* `CLIENT_SECRET_GITHUB_APP`: The **Client Secret** of your GitHub application.
* `CLIENT_SLUG_GITHUB_APP`: The **Slug** of your GitHub application. This is the one found in the URL.
* `CLIENT_APP_ID_GITHUB_APP`: The **App ID** of your GitHub application.
* `CLIENT_PRIVATE_KEY_GITHUB_APP`: The **Private Key** of your GitHub application.
Once added, restart your Infisical instance and use the GitHub integration via app authentication.
## Connecting with GitHub OAuth
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
* Ensure that you have admin privileges to the repository you want to sync secrets to.
Navigate to your project's integrations tab in Infisical and press on the GitHub tile.
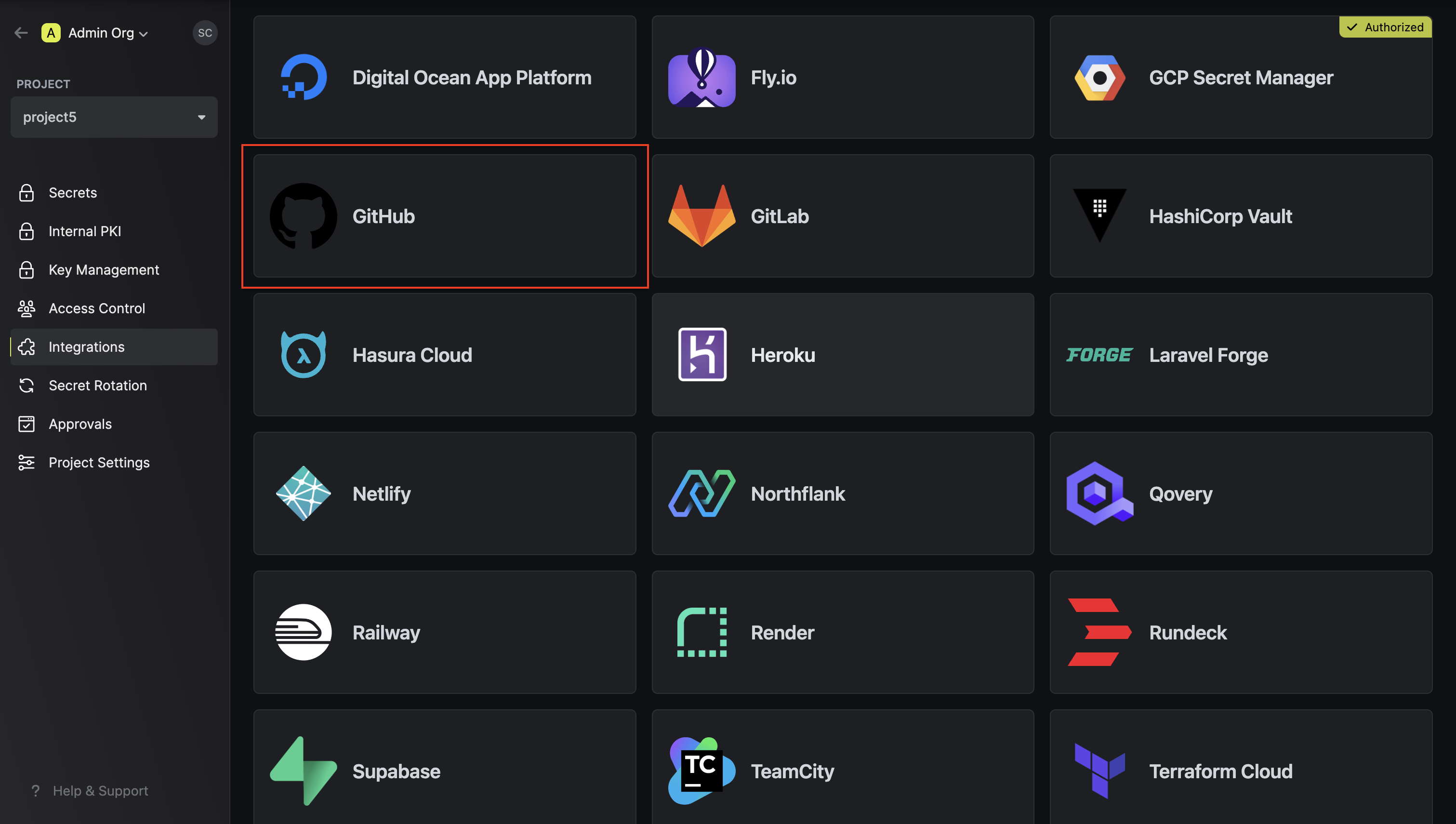
Select OAuth as the authentication method and click **Connect to GitHub**.
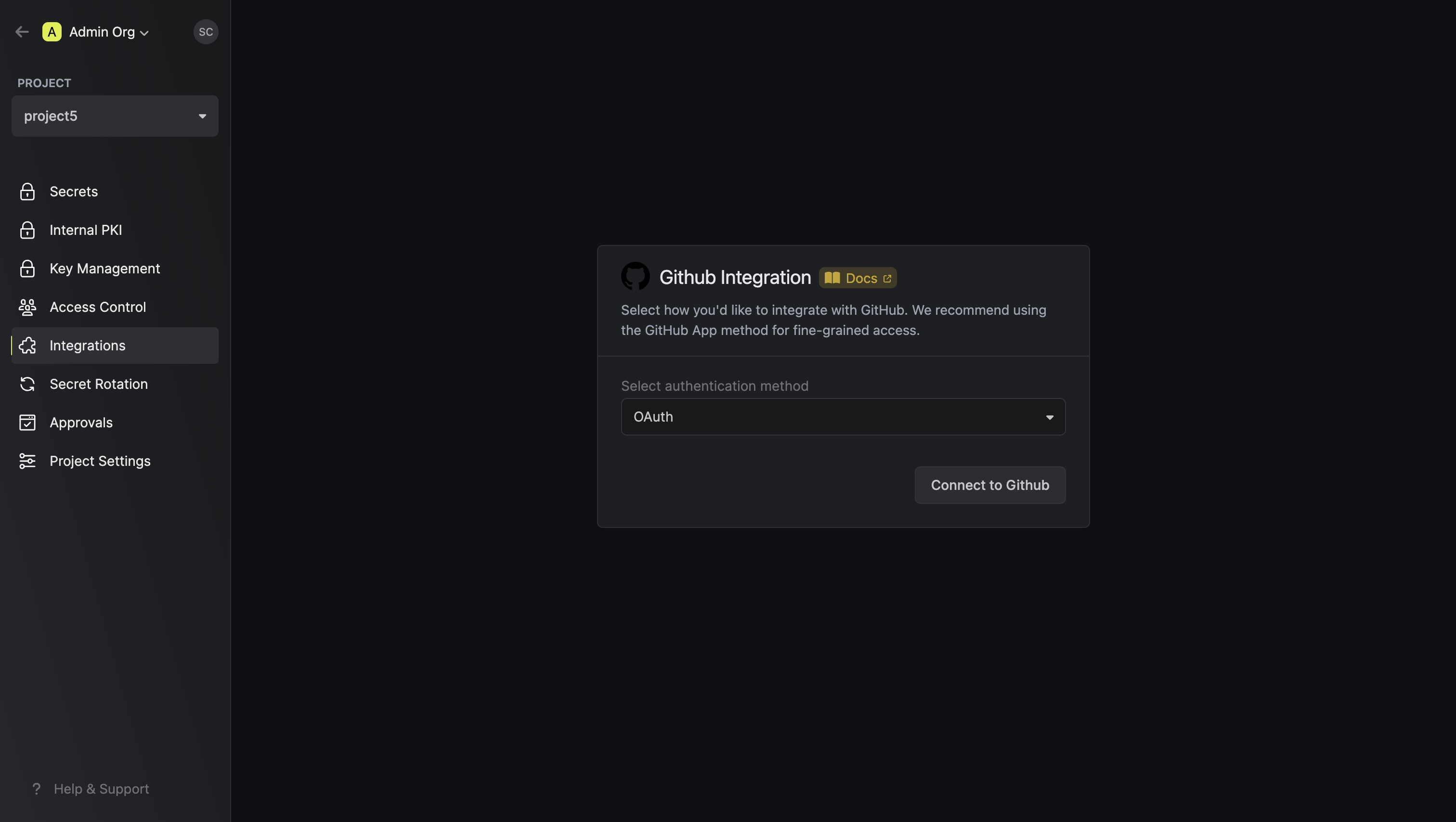
Grant Infisical access to your GitHub account (organization and repo privileges).
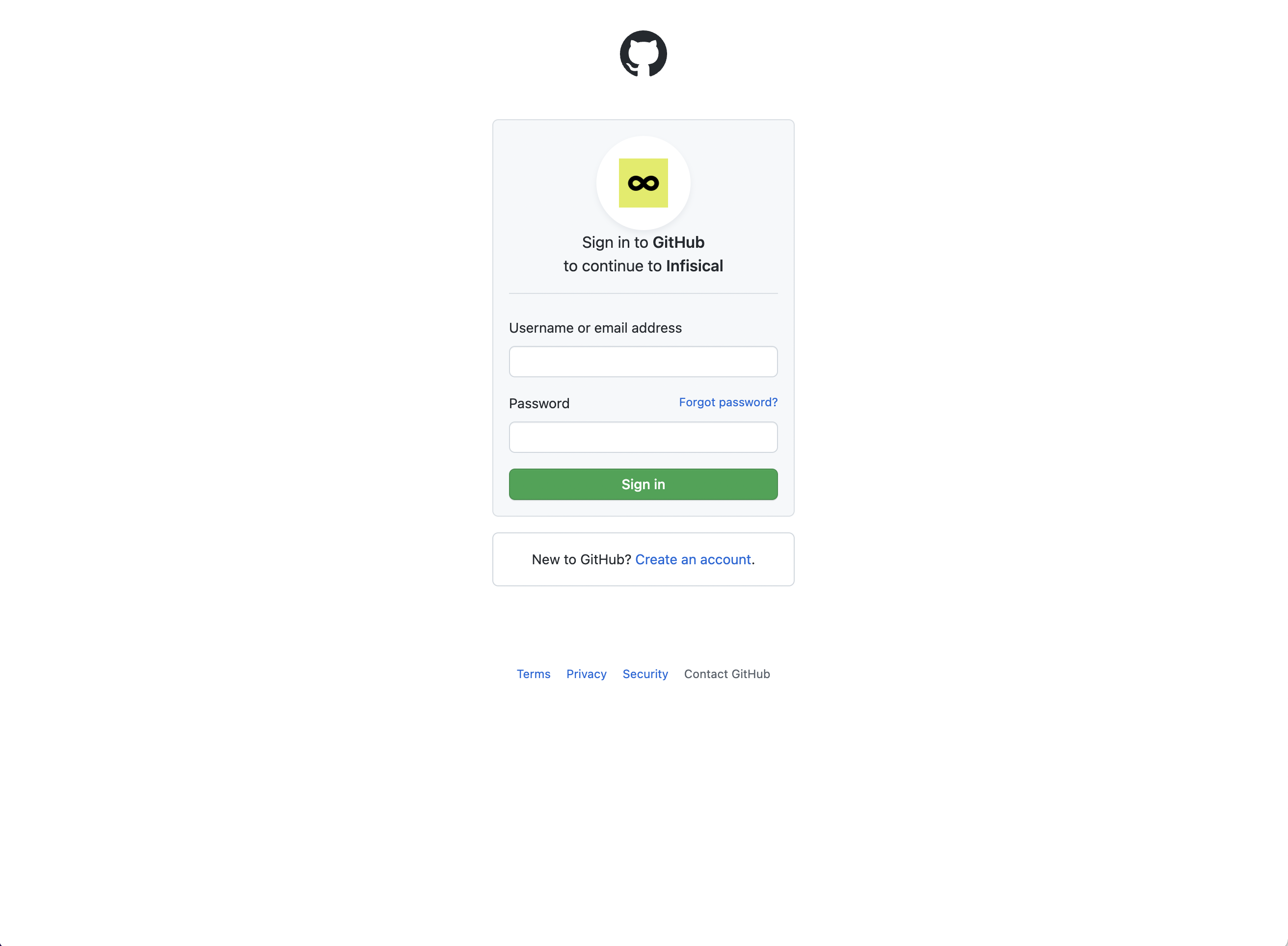
Select which Infisical environment secrets you want to sync to which GitHub organization, repository, or repository environment.
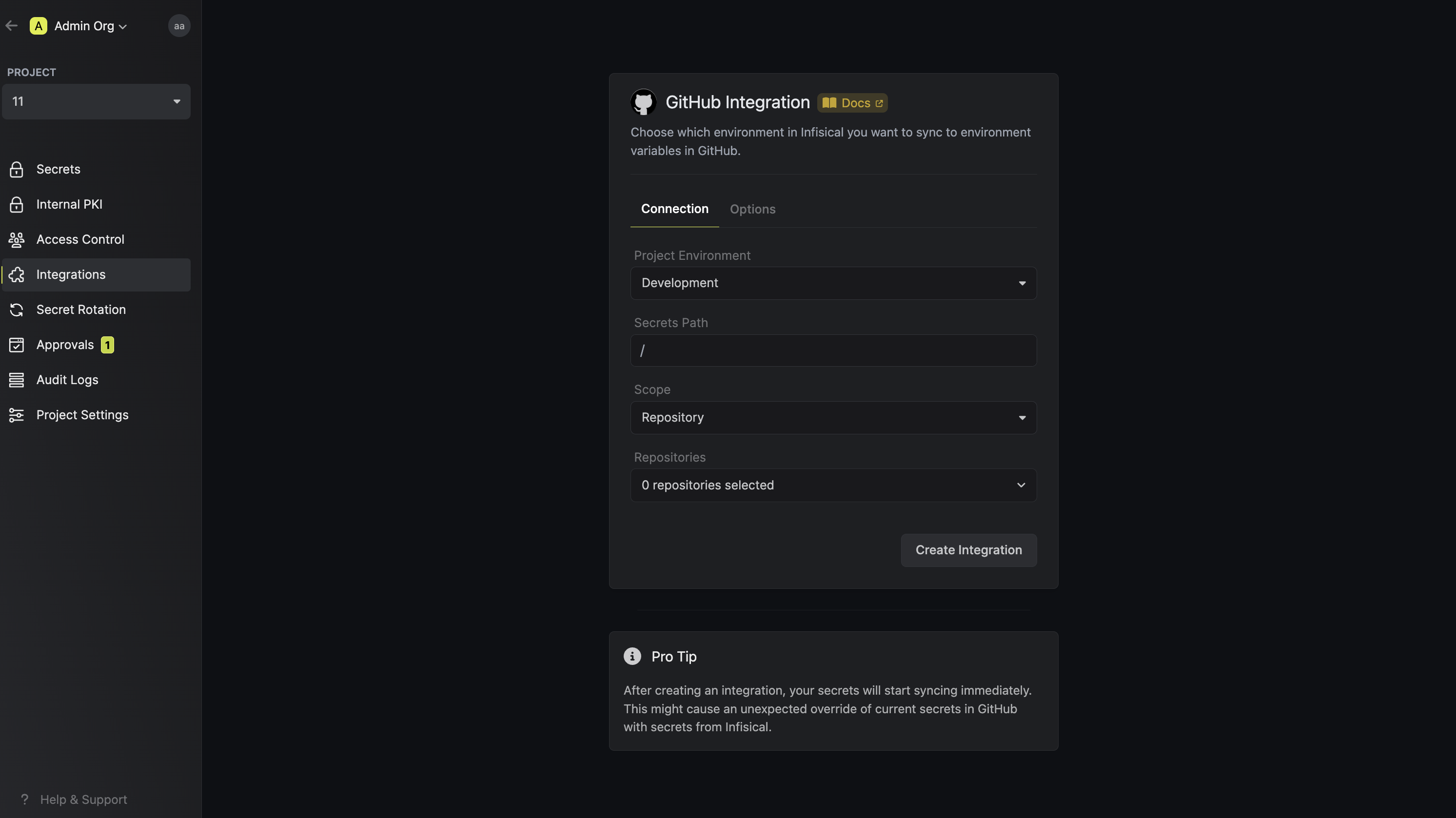
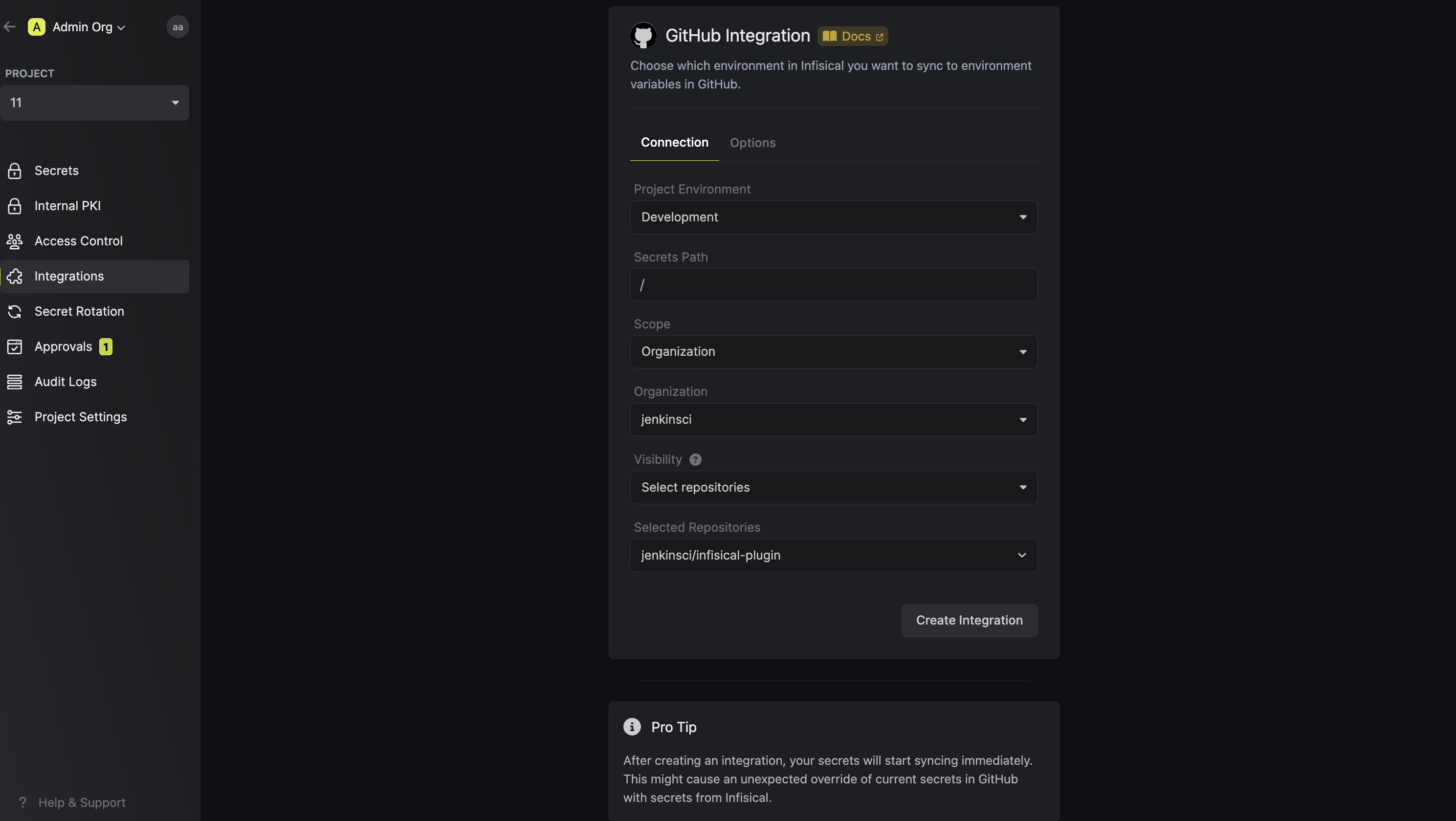
When using the organization scope, your secrets will be saved in the top-level of your GitHub Organization.
You can choose the visibility, which defines which repositories can access the secrets. The options are:
* **All public repositories**: All public repositories in the organization can access the secrets.
* **All private repositories**: All private repositories in the organization can access the secrets.
* **Selected repositories**: Only the selected repositories can access the secrets. This gives a more fine-grained control over which repositories can access the secrets. You can select *both* private and public repositories with this option.
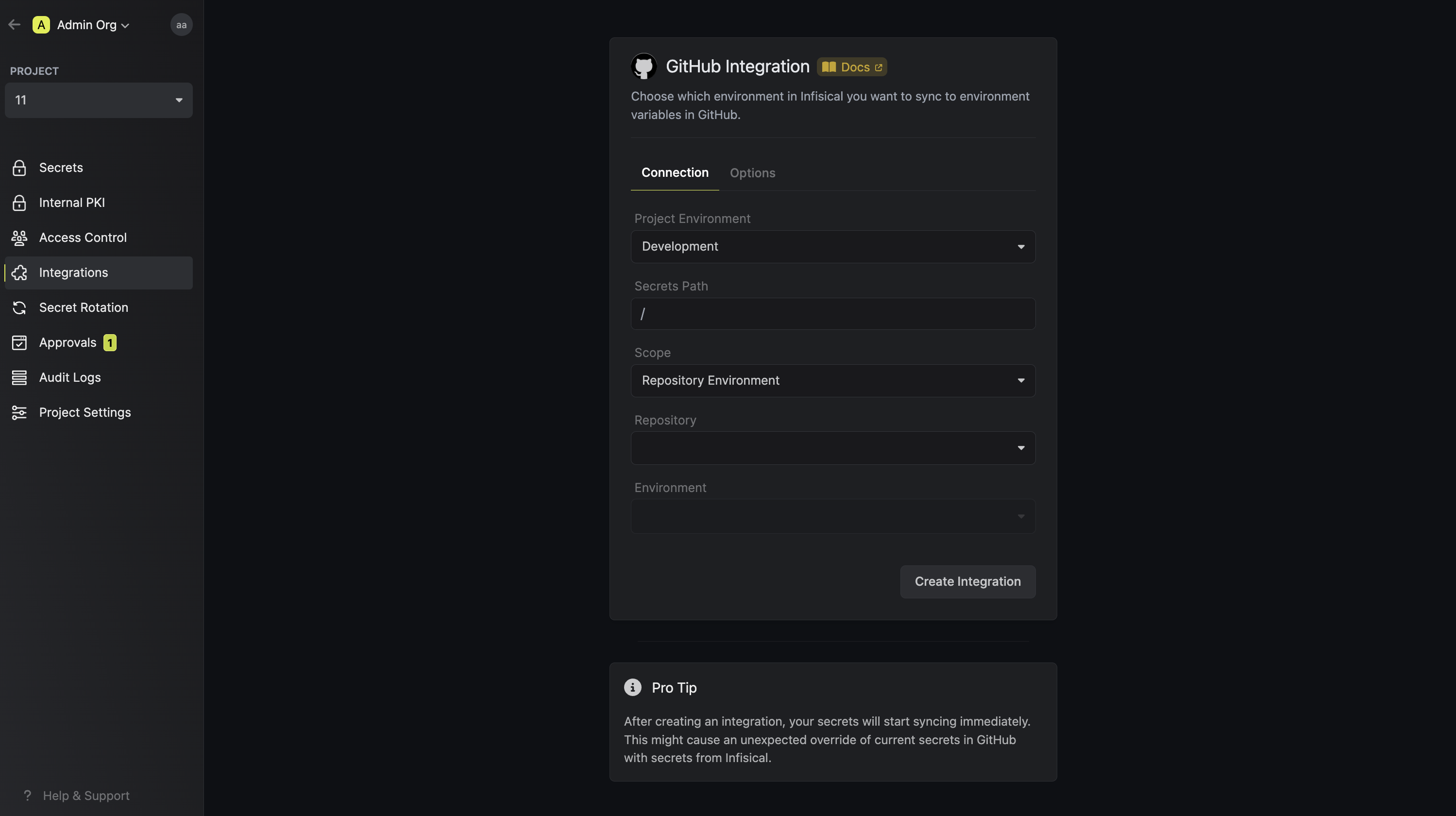
Finally, press create integration to start syncing secrets to GitHub.
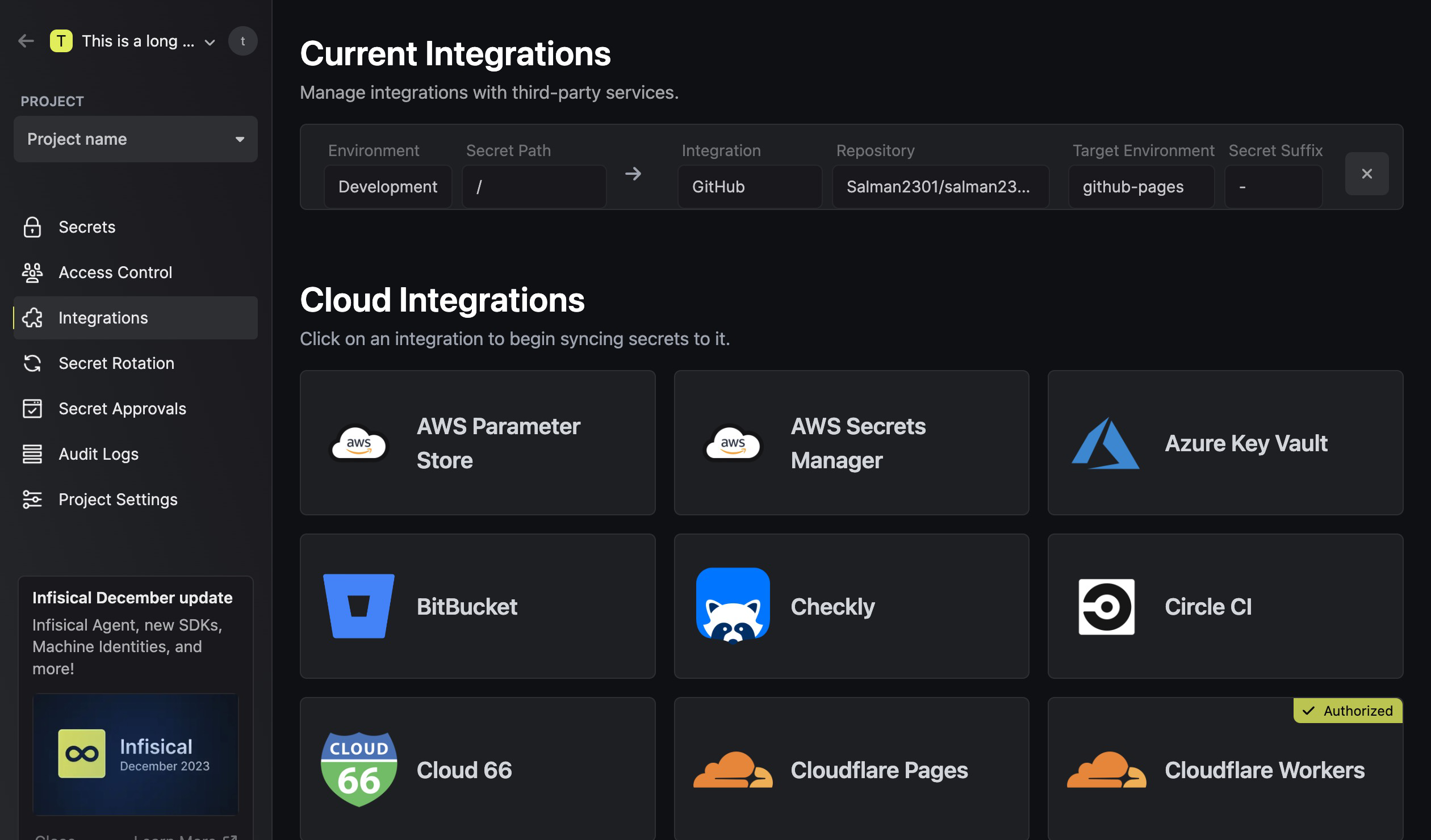
Using the GitHub integration on a self-hosted instance of Infisical requires configuring an OAuth application in GitHub
and registering your instance with it.
Navigate to your user Settings > Developer settings > OAuth Apps to create a new GitHub OAuth application.
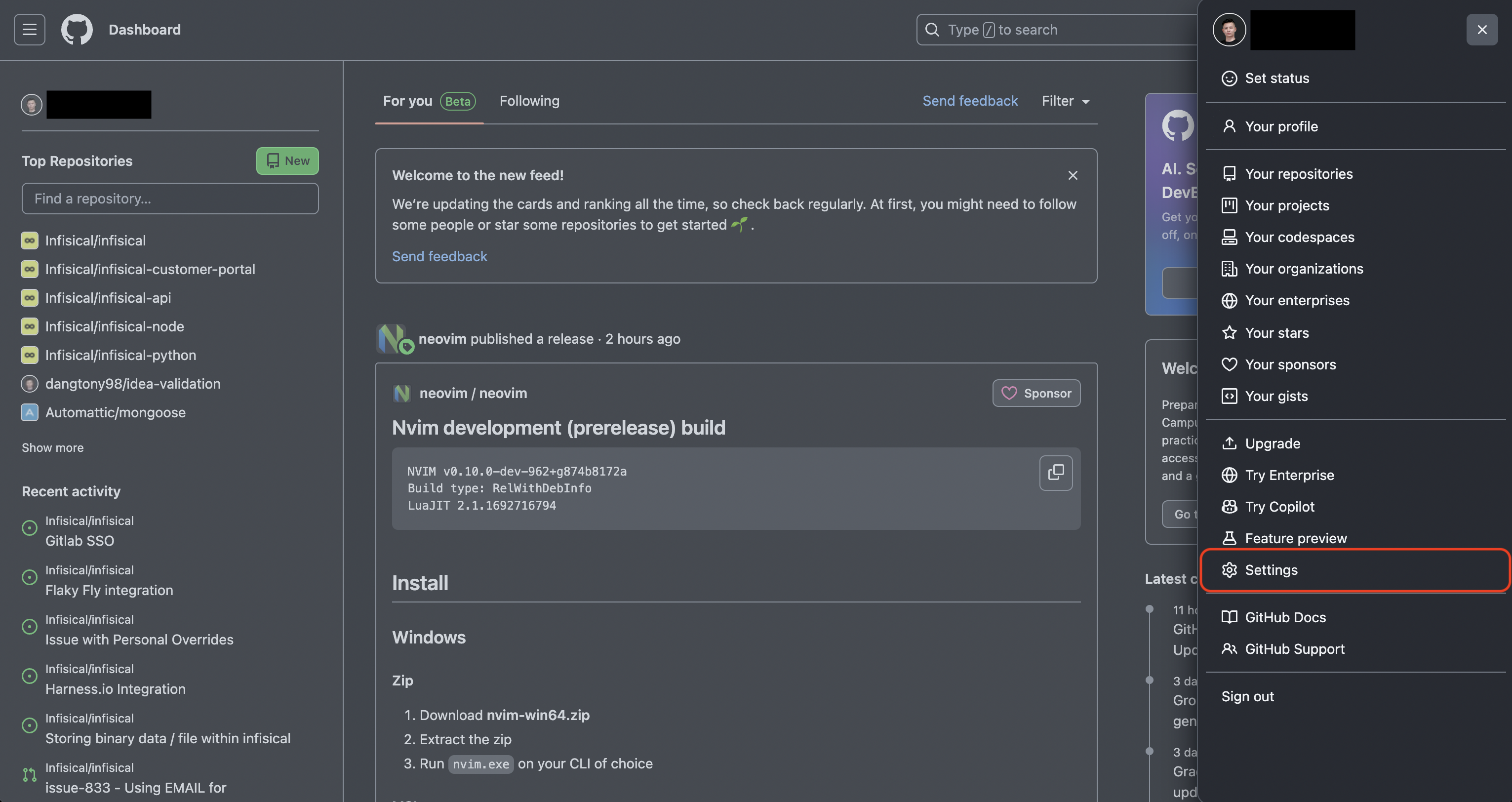
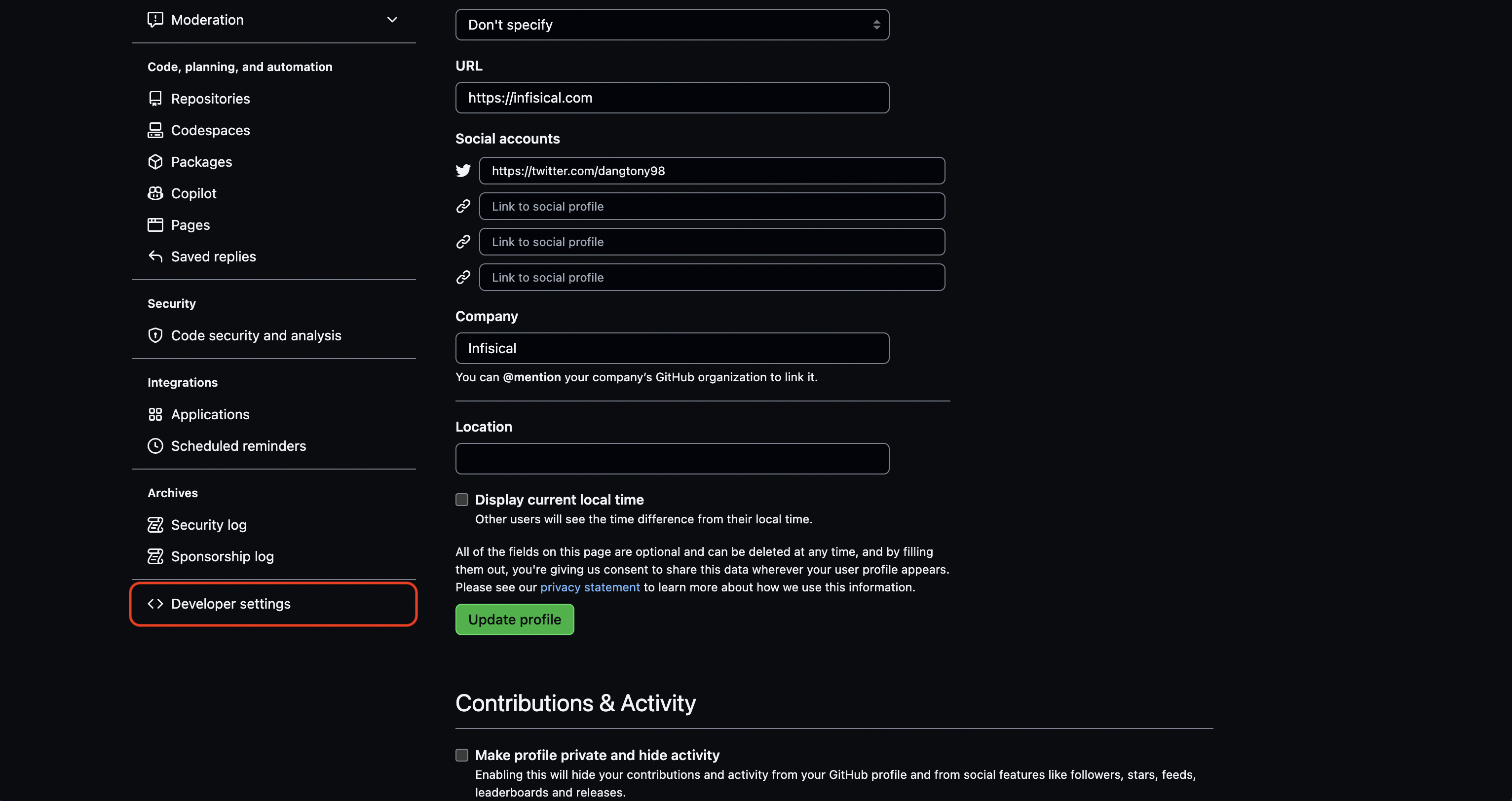
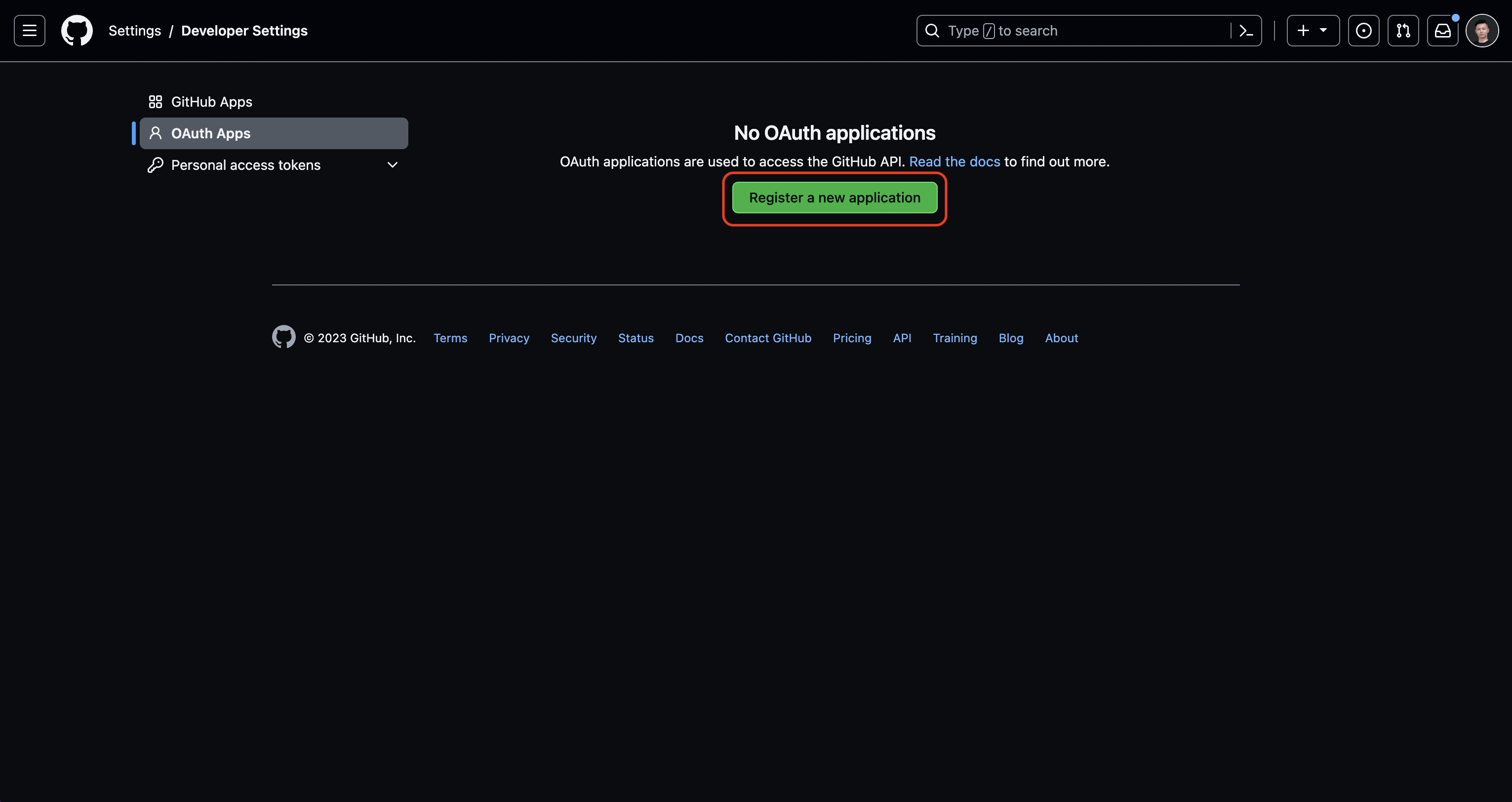
Create the OAuth application. As part of the form, set the **Homepage URL** to your self-hosted domain `https://your-domain.com`
and the **Authorization callback URL** to `https://your-domain.com/integrations/github/oauth2/callback`.
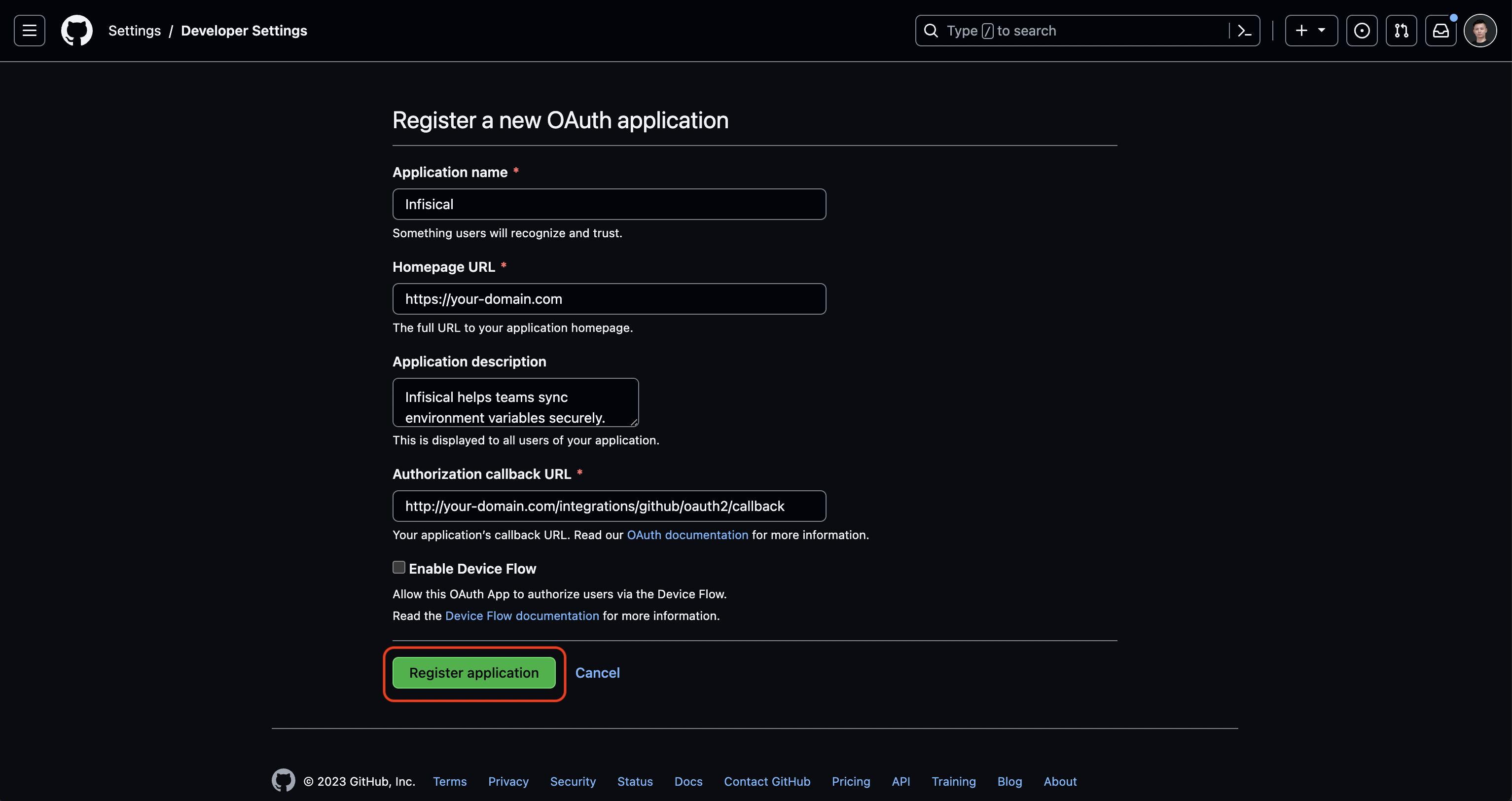
If you have a GitHub organization, you can create an OAuth application under it
in your organization Settings > Developer settings > OAuth Apps > New Org OAuth App.
Obtain the **Client ID** and generate a new **Client Secret** for your GitHub OAuth application.
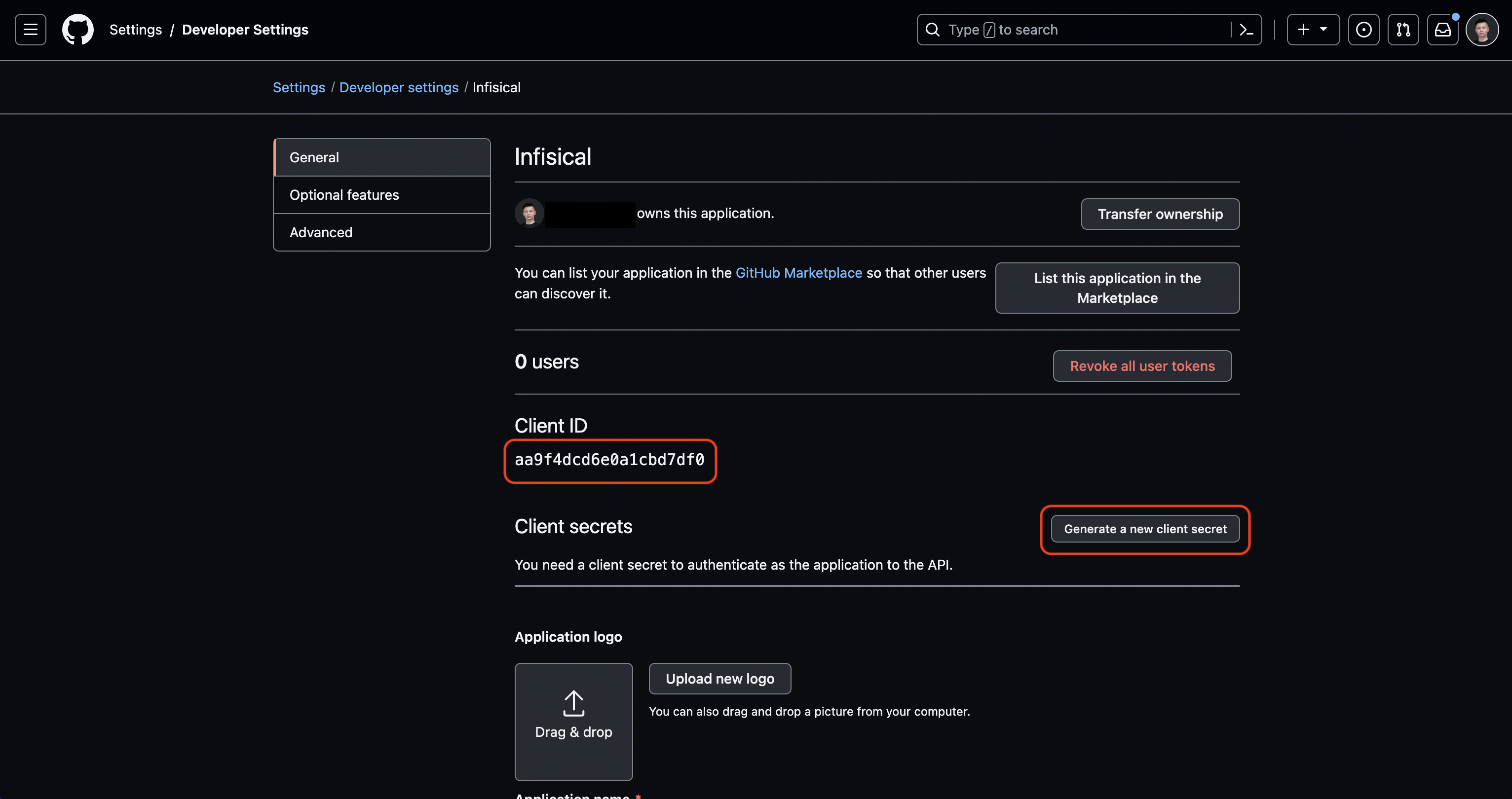
Back in your Infisical instance, add two new environment variables for the credentials of your GitHub OAuth application:
* `CLIENT_ID_GITHUB`: The **Client ID** of your GitHub OAuth application.
* `CLIENT_SECRET_GITHUB`: The **Client Secret** of your GitHub OAuth application.
Once added, restart your Infisical instance and use the GitHub integration.
# GitLab
How to sync secrets from Infisical to GitLab
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
Navigate to your project's integrations tab in Infisical.
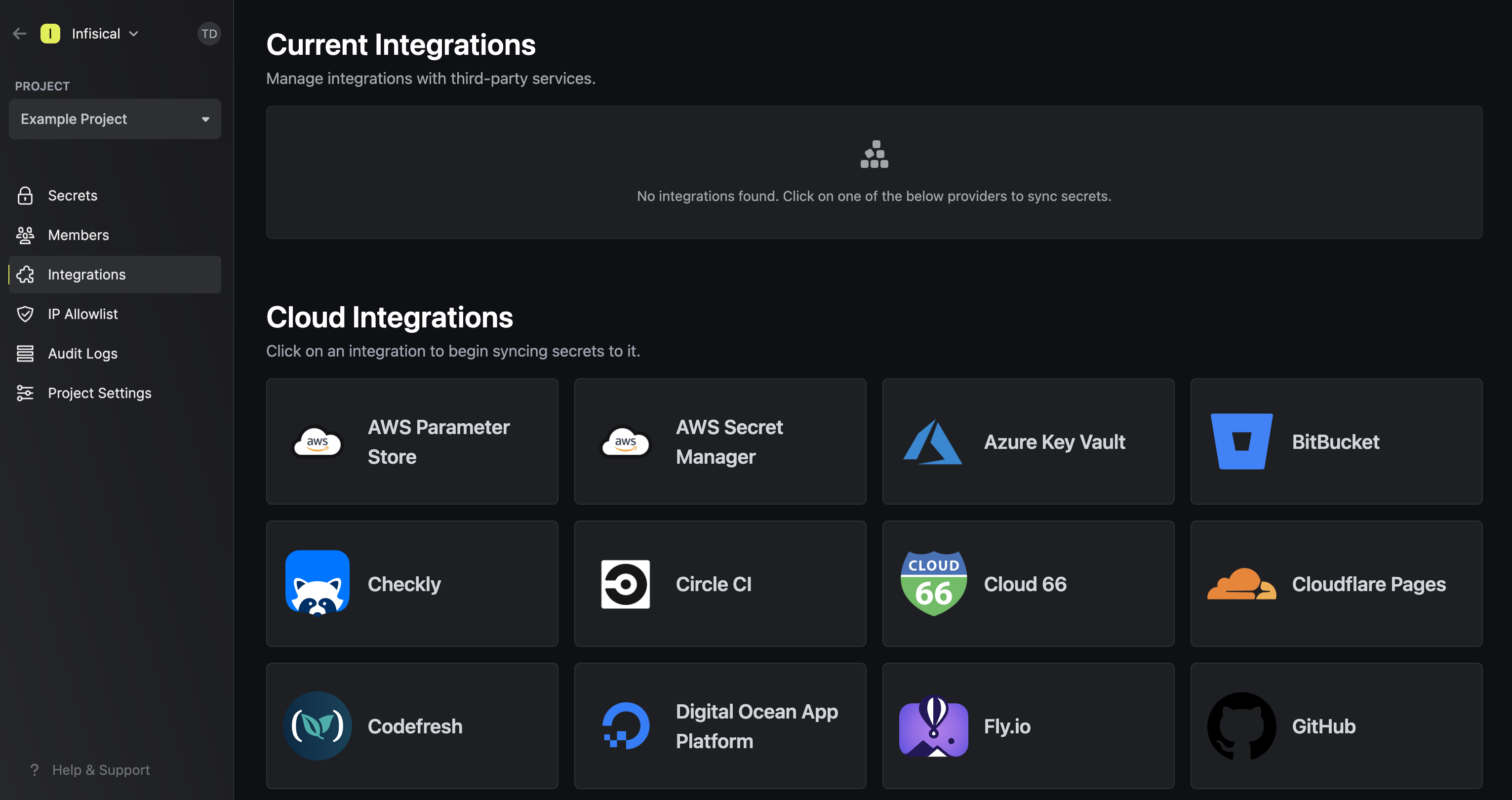
Press on the GitLab tile and grant Infisical access to your GitLab account.
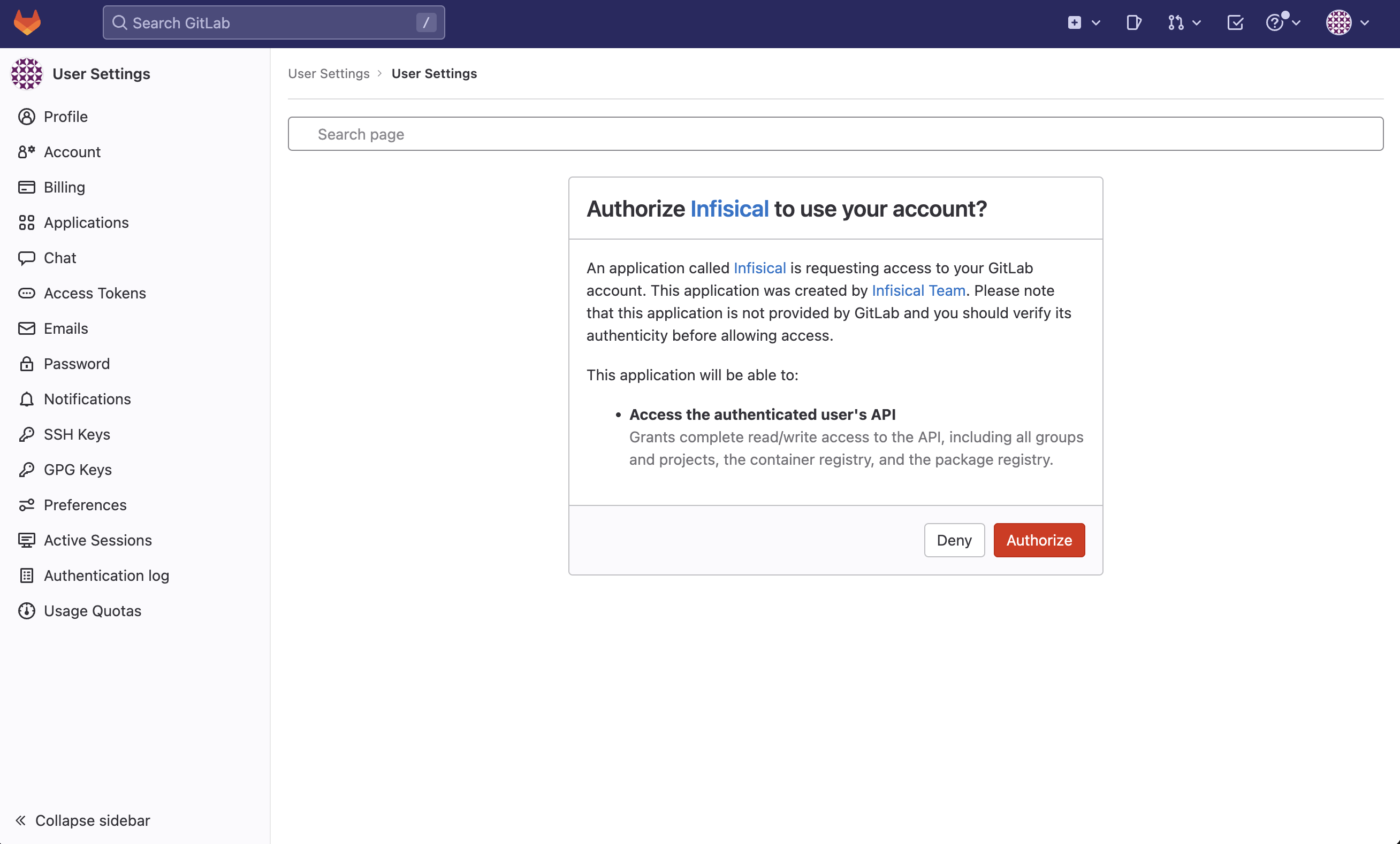
Select which Infisical environment secrets you want to sync to which GitLab repository and press create integration to start syncing secrets to GitLab.
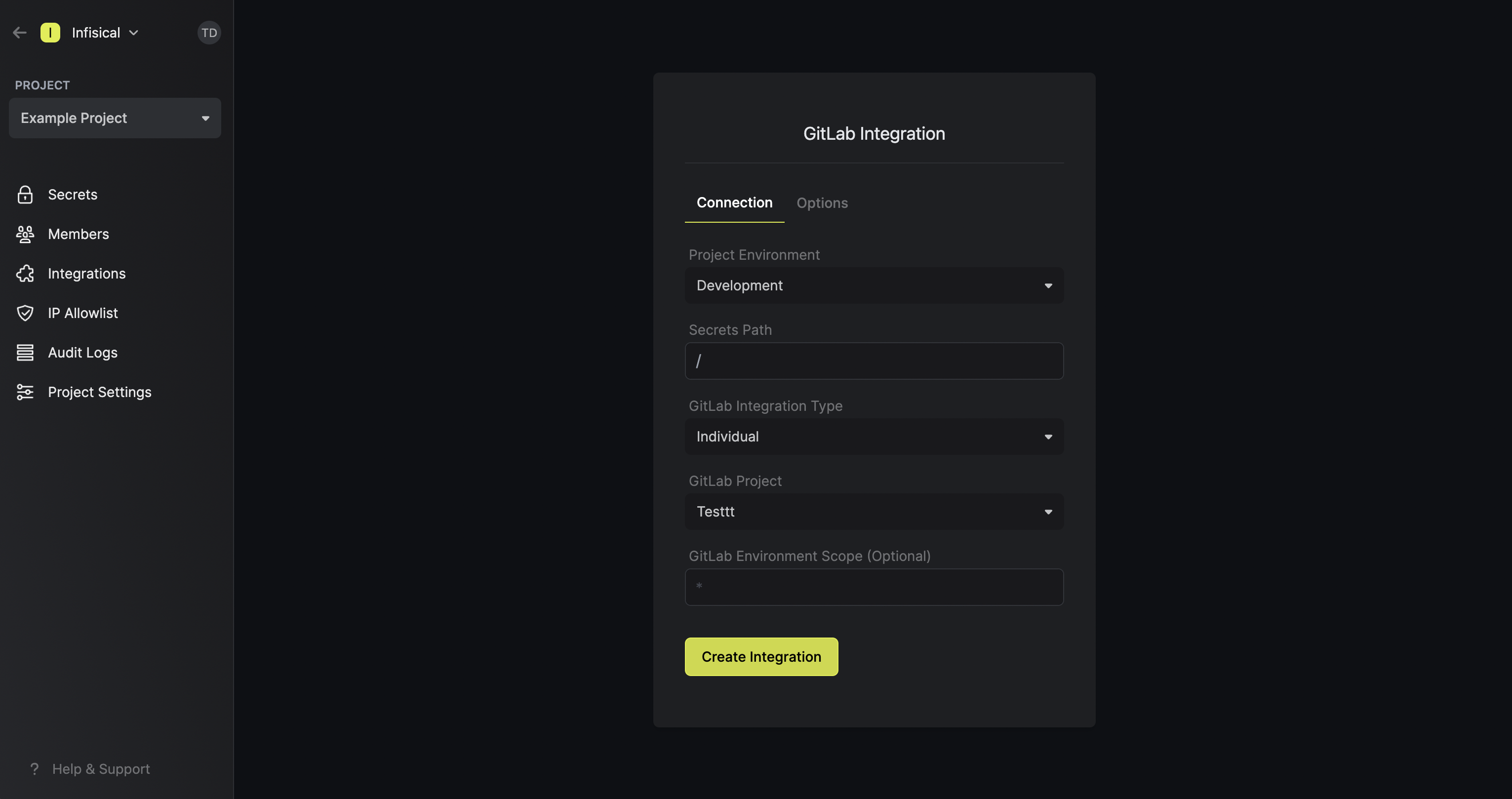
Note that the GitLab integration supports a few options in the **Options** tab:
* Secret Prefix: If inputted, the prefix is appended to the front of every secret name prior to being synced.
* Secret Suffix: If inputted, the suffix to appended to the back of every name of every secret prior to being synced.
Setting a secret prefix or suffix ensures that existing secrets in GitLab are not overwritten during the sync. As part of this process, Infisical abstains from mutating any secrets in GitLab without the specified prefix or suffix.
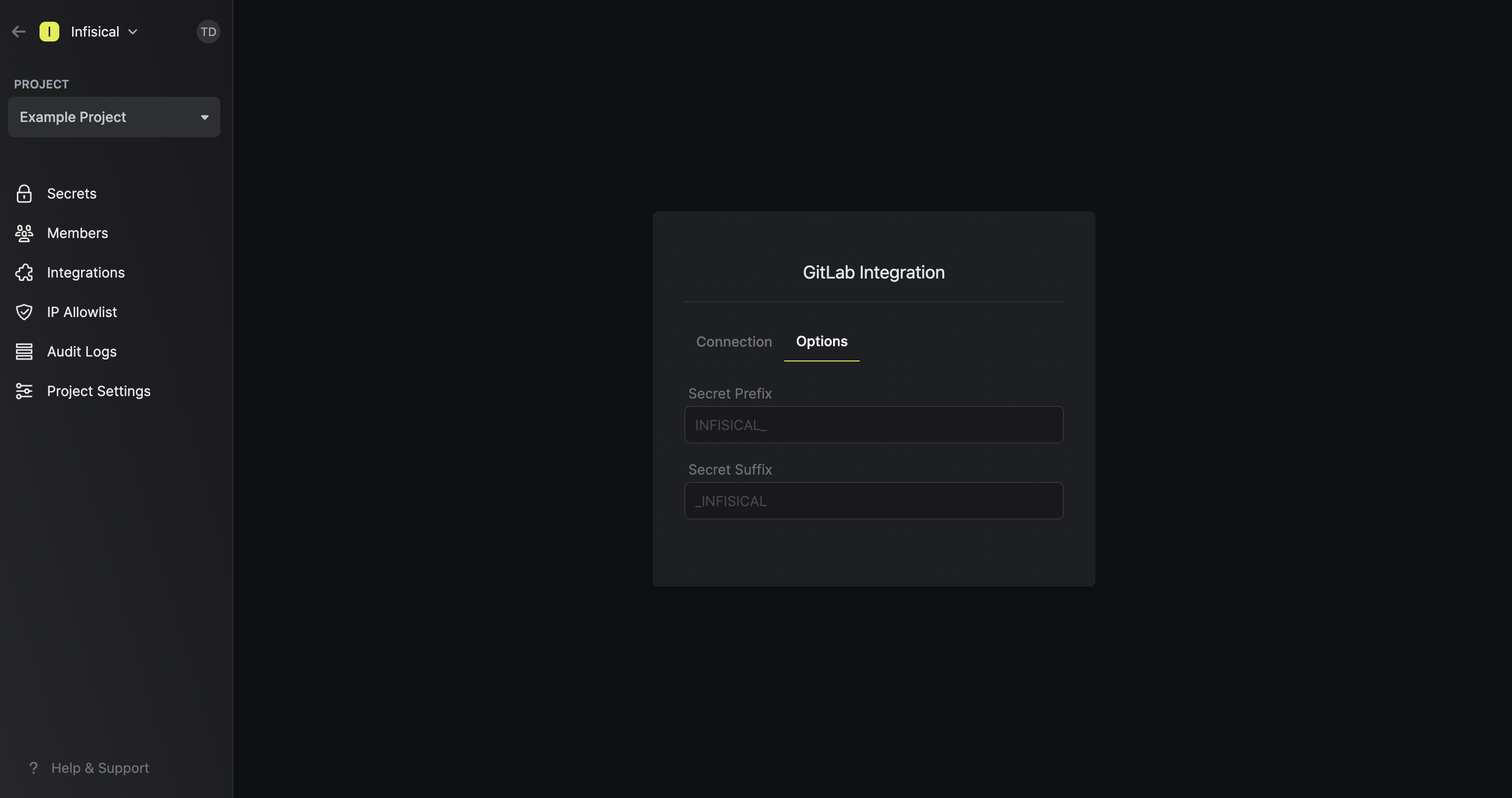
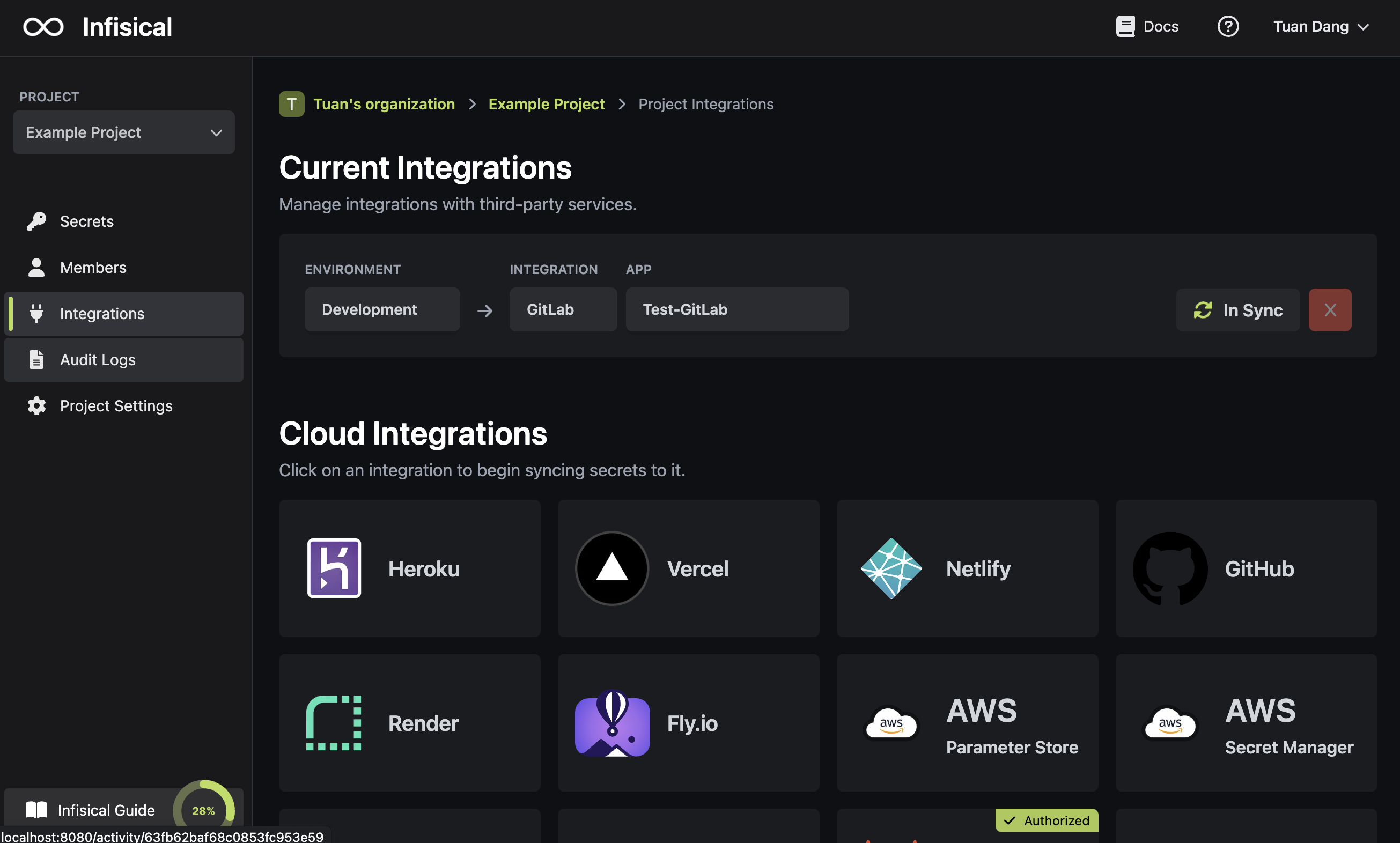
Generate an [Infisical Token](/documentation/platform/token) for the specific project and environment in Infisical.
Next, create a new variable called `INFISICAL_TOKEN` with the value set to the token from the previous step in Settings > CI/CD > Variables of your GitLab repository.
Edit your `.gitlab-ci.yml` to include the Infisical CLI installation. This will allow you to use the CLI for fetching and injecting secrets into any script or command within your Gitlab CI/CD process.
#### Example
```yaml
image: ubuntu
stages:
- build
- test
- deploy
build-job:
stage: build
script:
- apt update && apt install -y curl
- curl -1sLf 'https://dl.cloudsmith.io/public/infisical/infisical-cli/setup.deb.sh' | bash
- apt-get update && apt-get install -y infisical
- infisical run -- npm run build
```
Using the GitLab integration on a self-hosted instance of Infisical requires configuring an application in GitLab
and registering your instance with it.
If you're self-hosting Gitlab with custom certificates, you will have to configure your Infisical instance to trust these certificates. To learn how, please follow [this guide](../../self-hosting/guides/custom-certificates).
Navigate to your user Settings > Applications to create a new GitLab application.
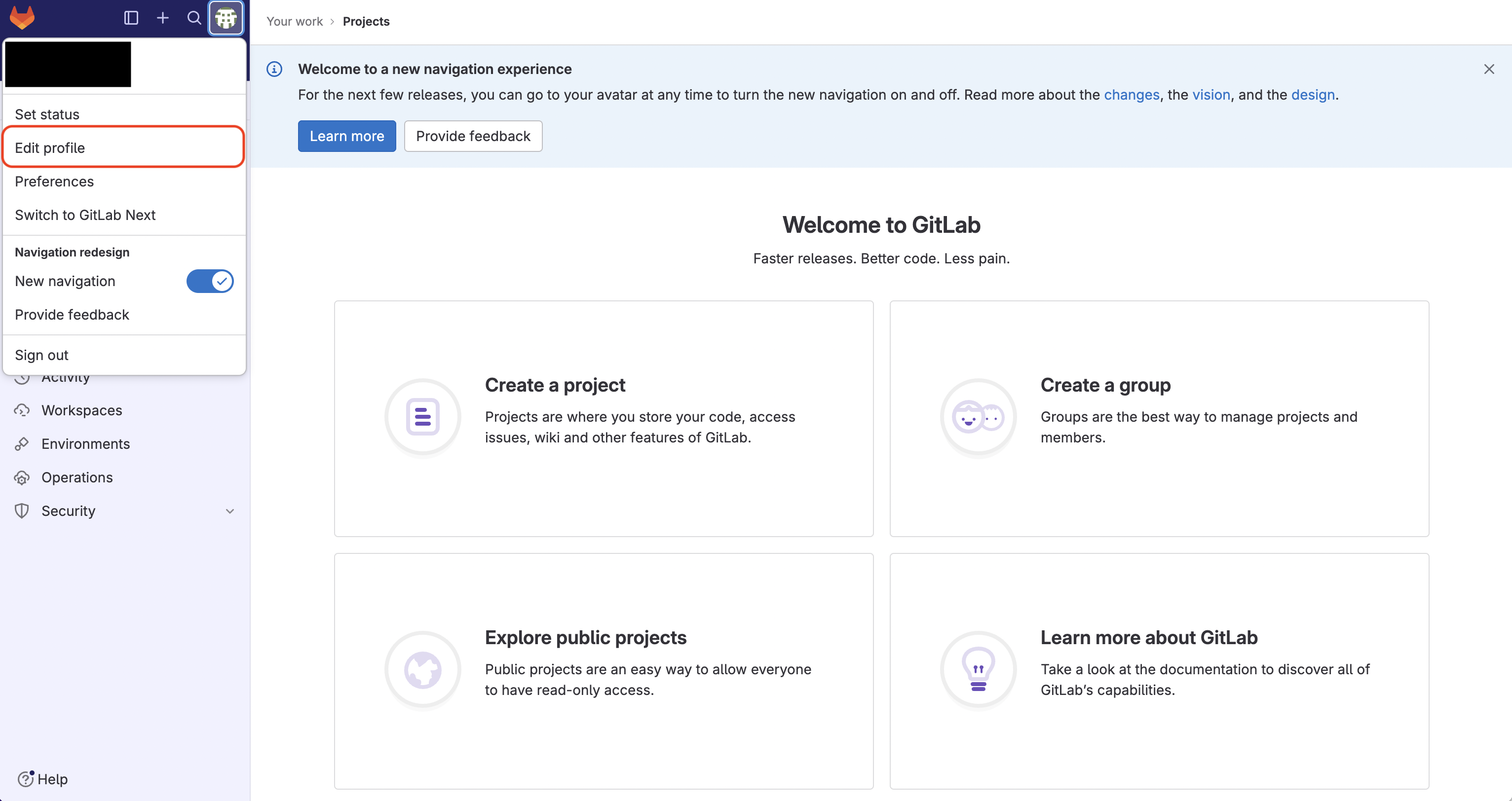
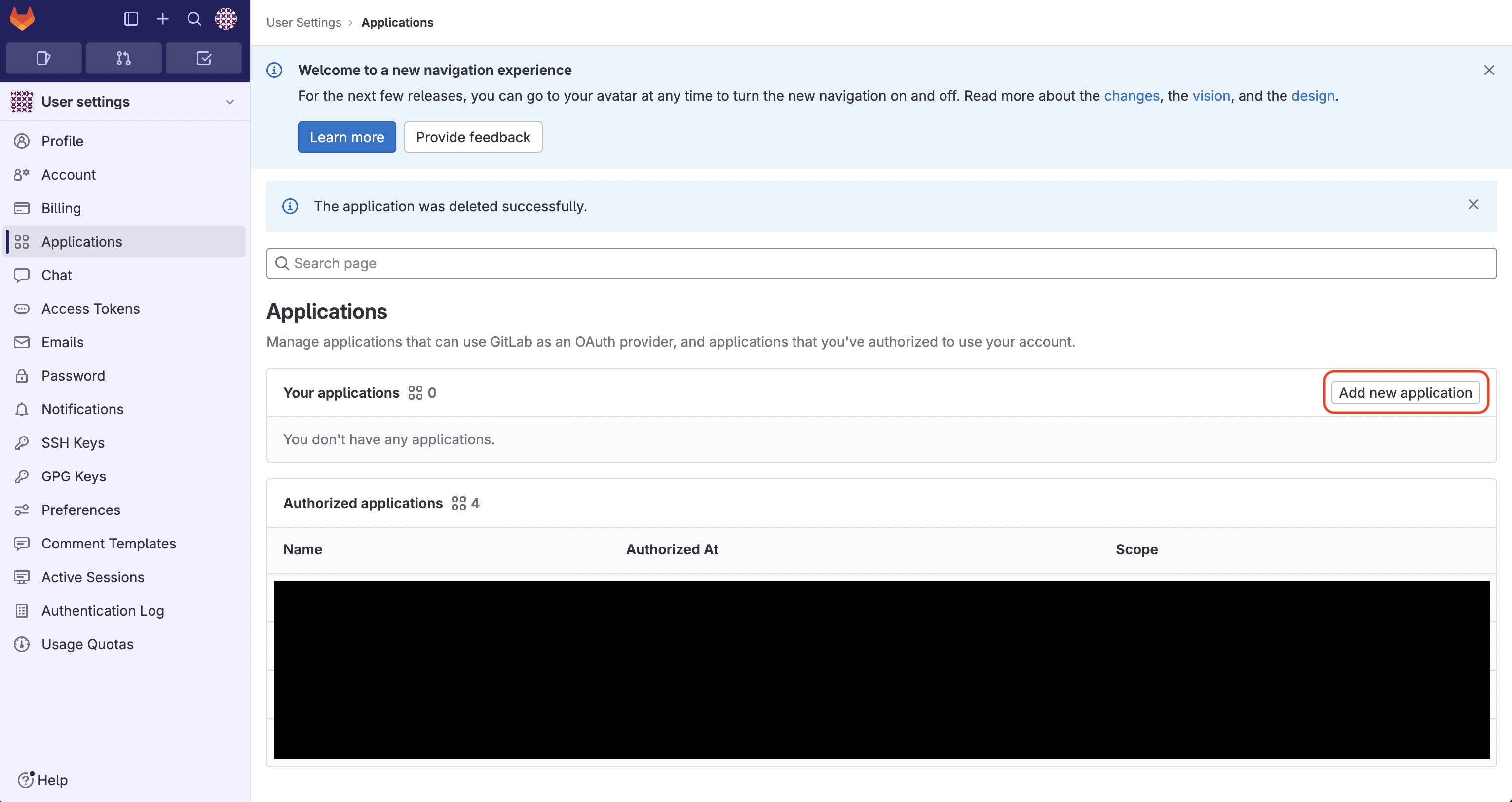
Create the application. As part of the form, set the **Redirect URI** to `https://your-domain.com/integrations/gitlab/oauth2/callback`.
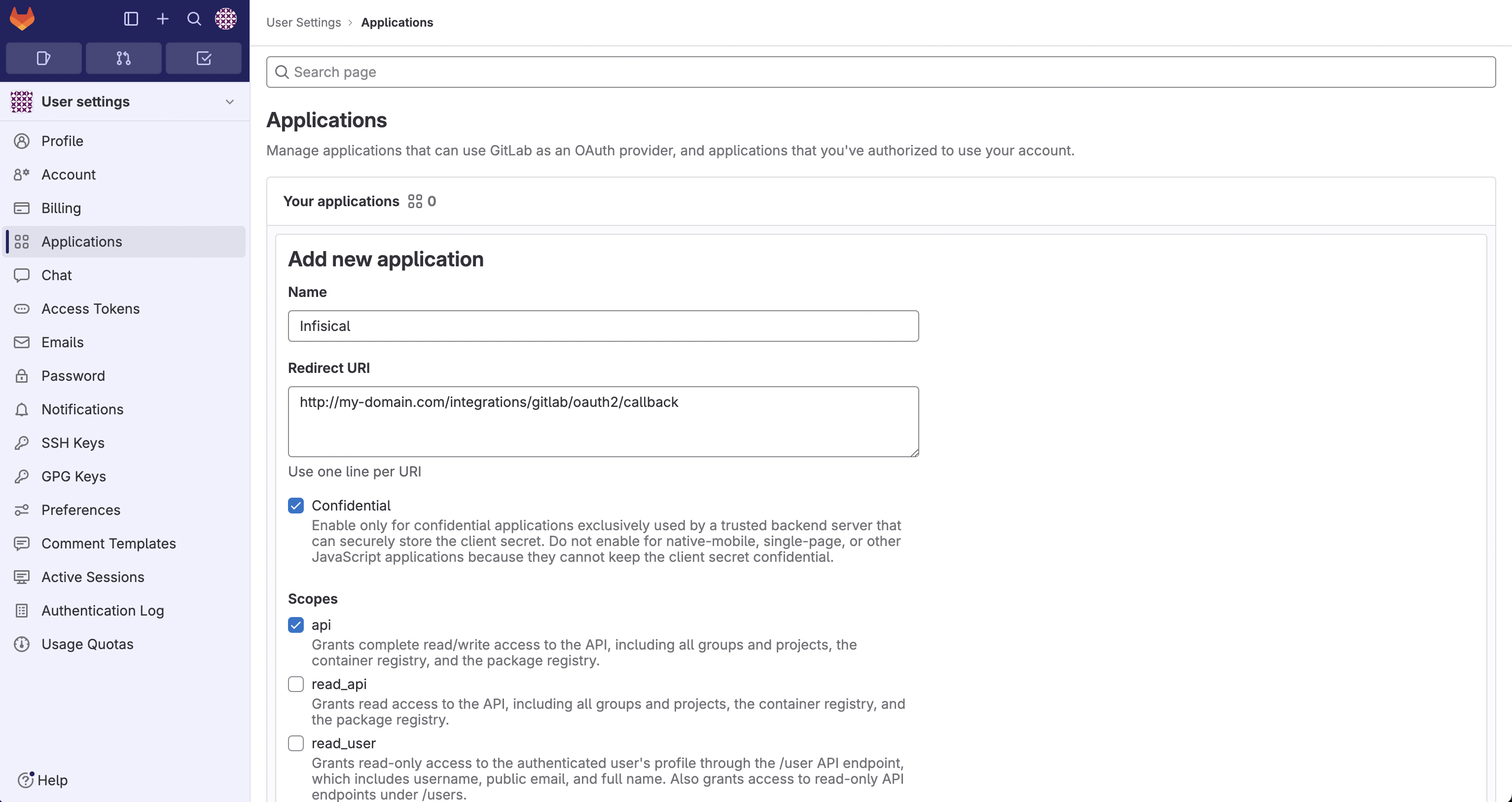
If you have a GitLab group, you can create an OAuth application under it
in your group Settings > Applications.
Obtain the **Application ID** and **Secret** for your GitLab application.
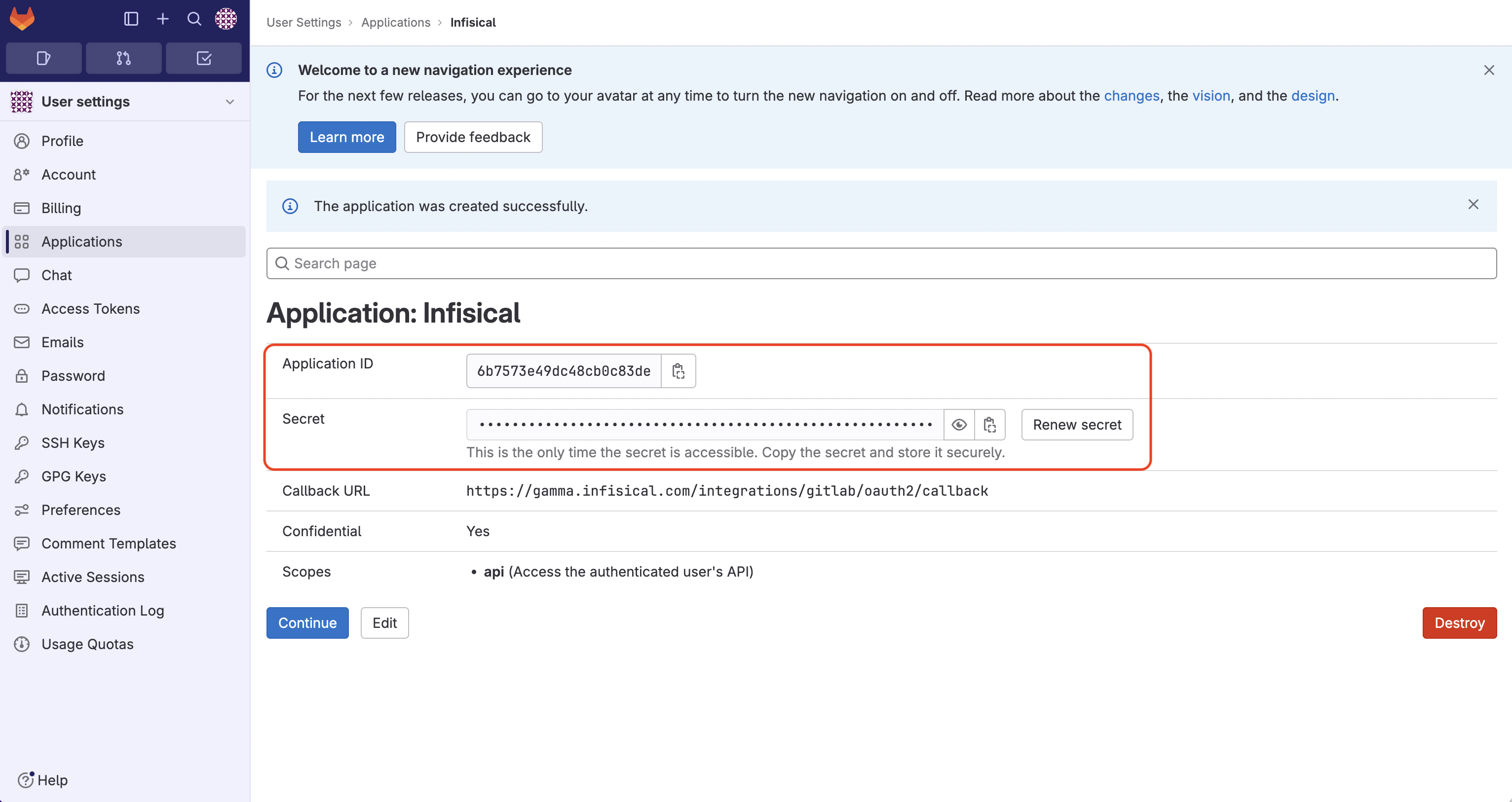
Back in your Infisical instance, add two new environment variables for the credentials of your GitLab application:
* `CLIENT_ID_GITLAB`: The **Client ID** of your GitLab application.
* `CLIENT_SECRET_GITLAB`: The **Secret** of your GitLab application.
Once added, restart your Infisical instance and use the GitLab integration.
# Jenkins Plugin
How to effectively and securely manage secrets in Jenkins using Infisical
**Objective**: Fetch secrets from Infisical to Jenkins pipelines
In this guide, we'll outline the steps to deliver secrets from Infisical to Jenkins via the Infisical CLI.
At a high level, the Infisical CLI will be executed within your build environment and use a machine identity to authenticate with Infisical.
This token must be added as a Jenkins Credential and then passed to the Infisical CLI as an environment variable, enabling it to access and retrieve secrets within your workflows.
Prerequisites:
* Set up and add secrets to [Infisical](https://app.infisical.com).
* Create a [machine identity](/documentation/platform/identities/machine-identities) (Recommended), or a service token in Infisical.
* You have a working Jenkins installation with the [credentials plugin](https://plugins.jenkins.io/credentials/) installed.
* You have the [Infisical CLI](/cli/overview) installed on your Jenkins executor nodes or container images.
## Jenkins Infisical Plugin
This plugin adds a build wrapper to set environment variables from [Infisical](https://infisical.com). Secrets are generally masked in the build log, so you can't accidentally print them.
## Installation
To install the plugin, navigate to `Manage Jenkins -> Plugins -> Available plugins` and search for `Infisical`. Install the plugin and restart Jenkins.
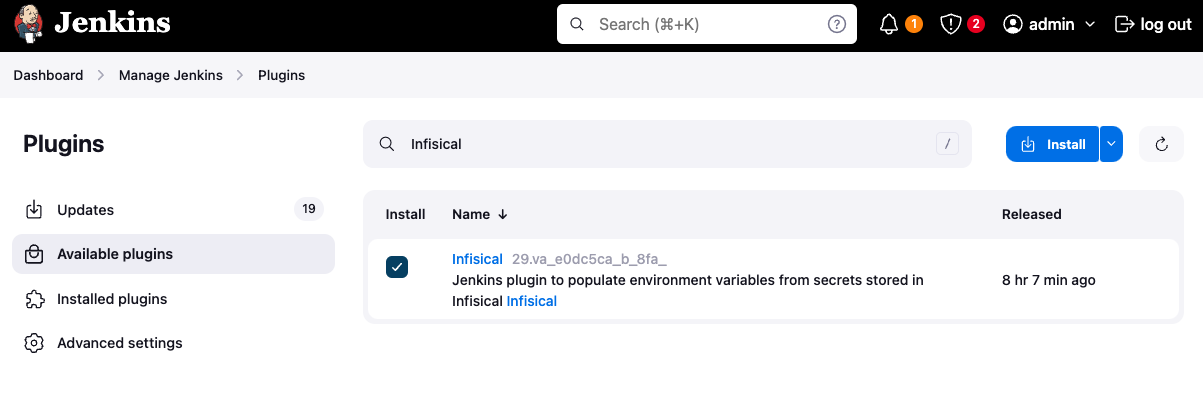
## Infisical Authentication
Authenticating with Infisical is done through the use of [Machine Identities](https://infisical.com/docs/documentation/platform/identities/machine-identities).
Currently the Jenkins plugin only supports [Universal Auth](https://infisical.com/docs/documentation/platform/identities/universal-auth) for authentication. More methods will be added soon.
### How does Universal Auth work?
To use Universal Auth, you'll need to create a new Credential *(Infisical Universal Auth Credential)*. The credential should contain your Universal Auth client ID, and your Universal Auth client secret.
Please [read more here](https://infisical.com/docs/documentation/platform/identities/universal-auth) on how to setup a Machine Identity to use universal auth.
### Creating a Universal Auth credential
Creating a universal auth credential inside Jenkins is very straight forward.
Simply navigate to
`Dashboard -> Manage Jenkins -> Credentials -> System -> Global credentials (unrestricted)`.
Press the `Add Credentials` button and select `Infisical Universal Auth Credential` in the `Kind` field.
The `ID` and `Description` field doesn't matter much in this case, as they won't be read anywhere. The description field will be displayed as the credential name during the plugin configuration.
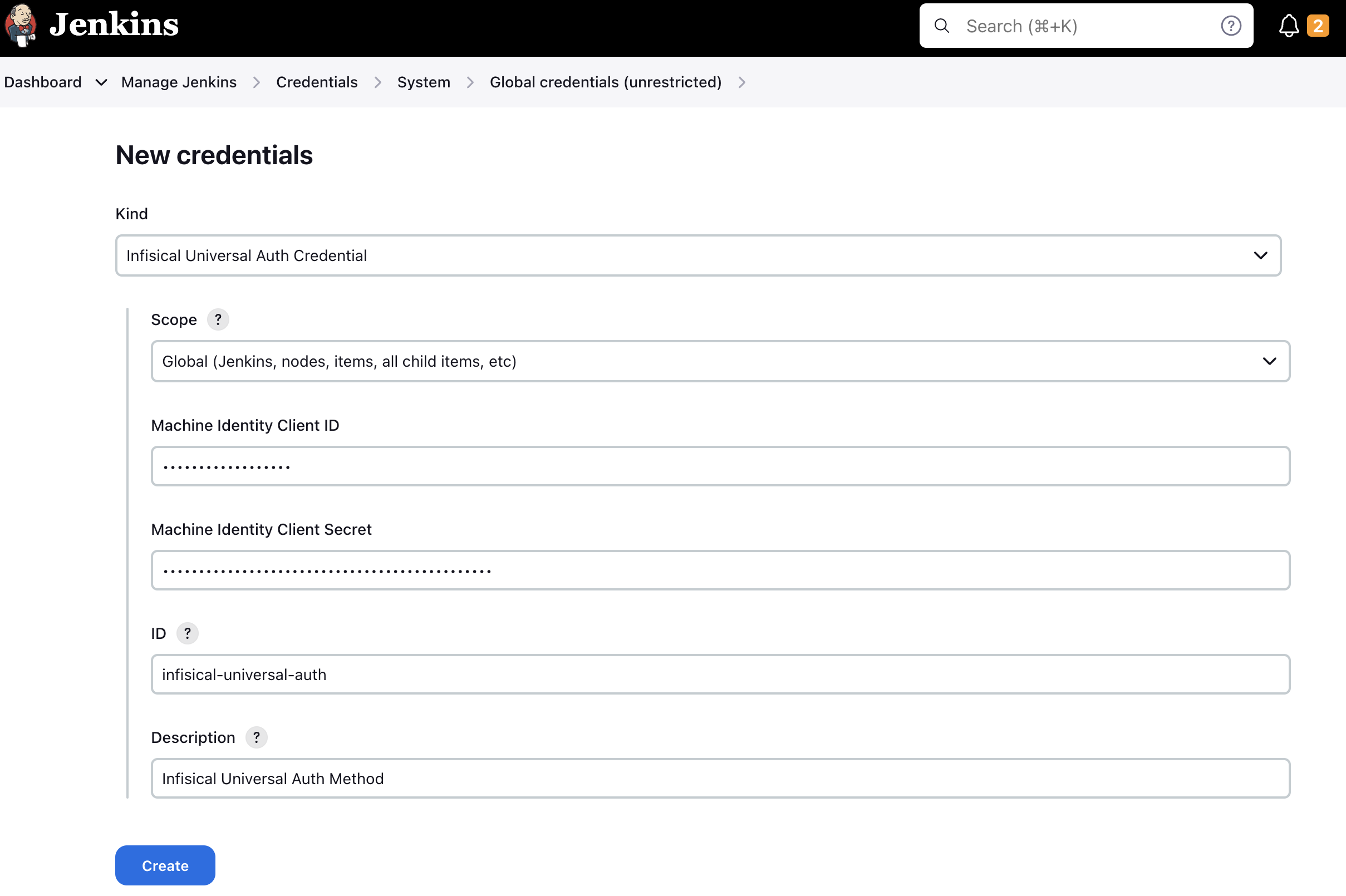
## Plugin Usage
### Configuration
Configuration takes place on a job-level basis.
Inside your job, you simply tick the `Infisical Plugin` checkbox under "Build Environment". After enabling the plugin, you'll see a new section appear where you'll have to configure the plugin.
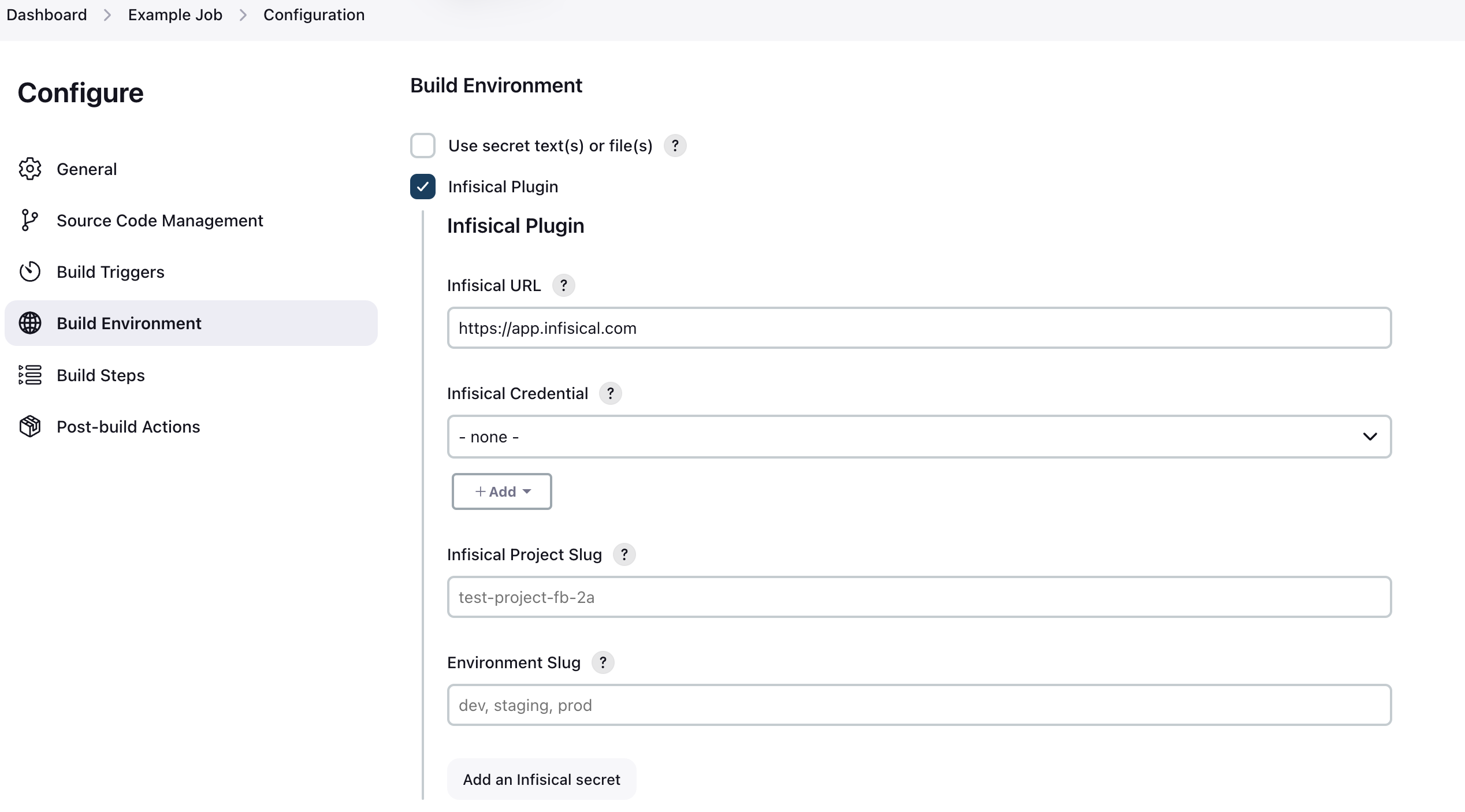
You'll be prompted with 4 options to fill:
* Infisical URL
* This defaults to [https://app.infisical.com](https://app.infisical.com). This field is only relevant if you're running a managed or self-hosted instance. If you are using Infisical Cloud, leave this as-is, otherwise enter the URL of your Infisical instance.
* Infisical Credential
* This is where you select your Infisical credential to use for authentication. In the step above [Creating a Universal Auth credential](#creating-a-universal-auth-credential), you can read on how to configure the credential. Simply select the credential you have created for this field.
* Infisical Project Slug
* This is the slug of the project you wish to fetch secrets from. You can find this in your project settings on Infisical by clicking "Copy project slug".
* Environment Slug
* This is the slug of the environment to fetch secrets from. In most cases it's either `dev`, `staging`, or `prod`. You can however create custom environments in Infisical. If you are using custom environments, you need to enter the slug of the custom environment you wish to fetch secrets from.
That's it! Now you're ready to select which secrets you want to fetch into Jenkins.
By clicking the `Add an Infisical secret` in the Jenkins UI like seen in the screenshot below.
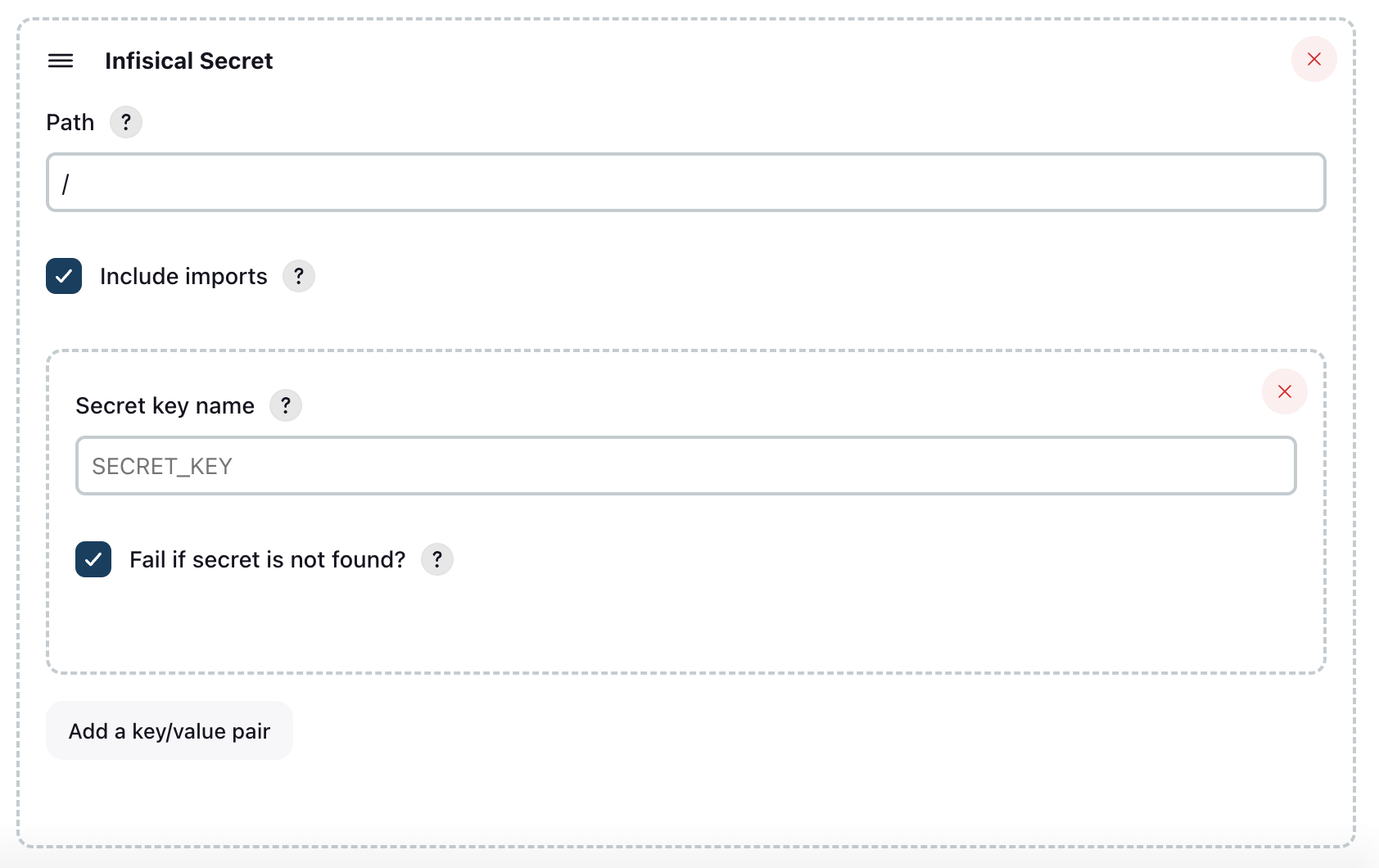
You need to select which secrets that should be pulled into Jenkins.
You start by specifying a [folder path from Infisical](https://infisical.com/docs/documentation/platform/folder#comparing-folders). The root path is simply `/`. You also need to select wether or not you want to [include imports](https://infisical.com/docs/documentation/platform/secret-reference#secret-imports). Now you can add secrets the secret keys that you want to pull from Infisical into Jenkins. If you want to add multiple secrets, press the "Add key/value pair".
If you wish to pull secrets from multiple paths, you can press the "Add an Infisical secret" button at the bottom, and configure a new set of secrets to pull.
## Pipeline usage
### Generating pipeline block
Using the Infisical Plugin in a Jenkins pipeline is very straight forward. To generate a block to use the Infisical Plugin in a Pipeline, simply to go `{JENKINS_URL}/jenkins/job/{JOB_ID}/pipeline-syntax/`.
You can find a direct link on the Pipeline configuration page in the very bottom of the page, see image below.
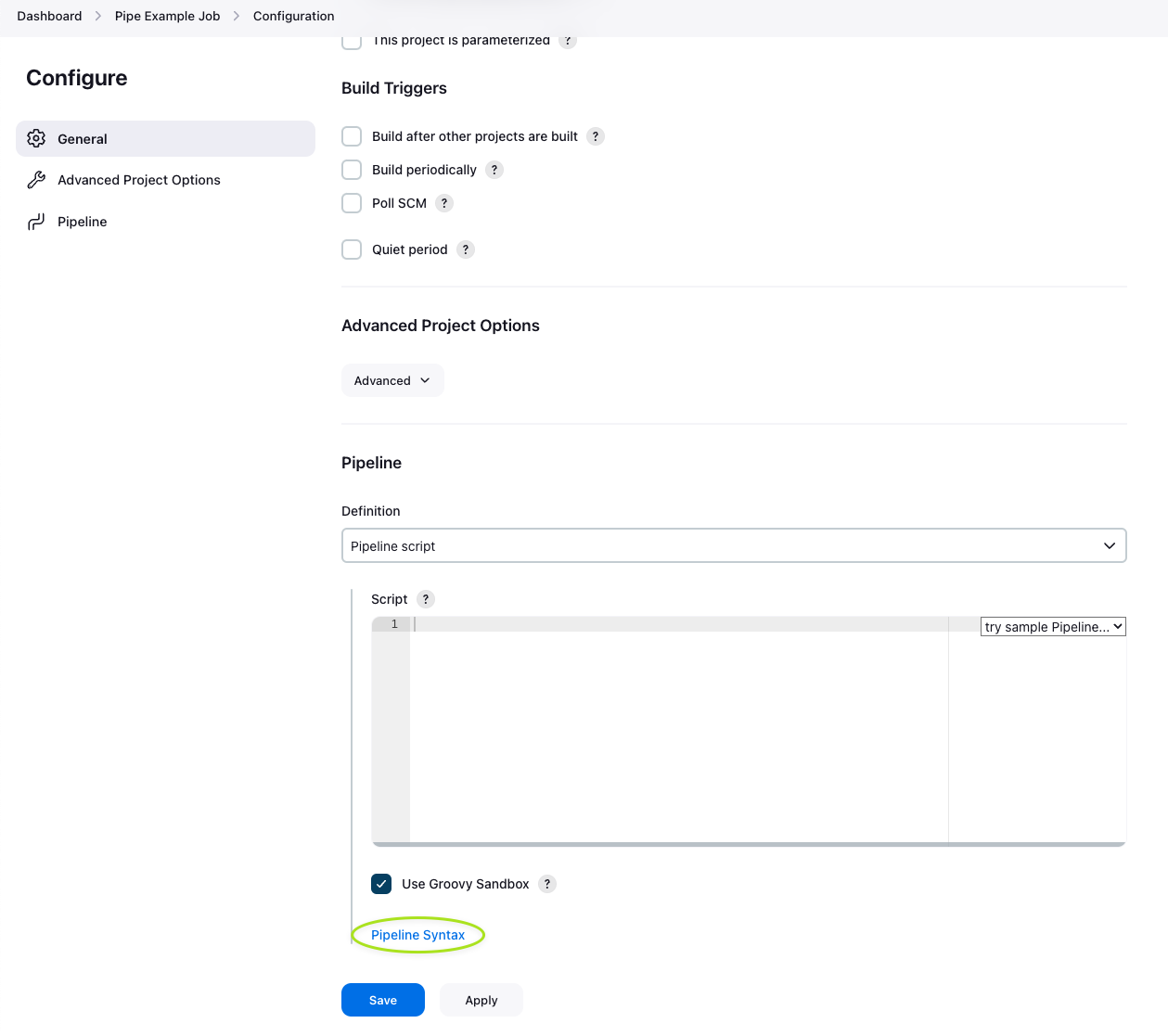
On the Snippet Generator page, simply configure the Infisical Plugin like it's documented in the [Configuration documentation](#configuration) step.
Once you have filled out the configuration, press `Generate Pipeline Script`, and it will generate a block you can use in your pipeline.
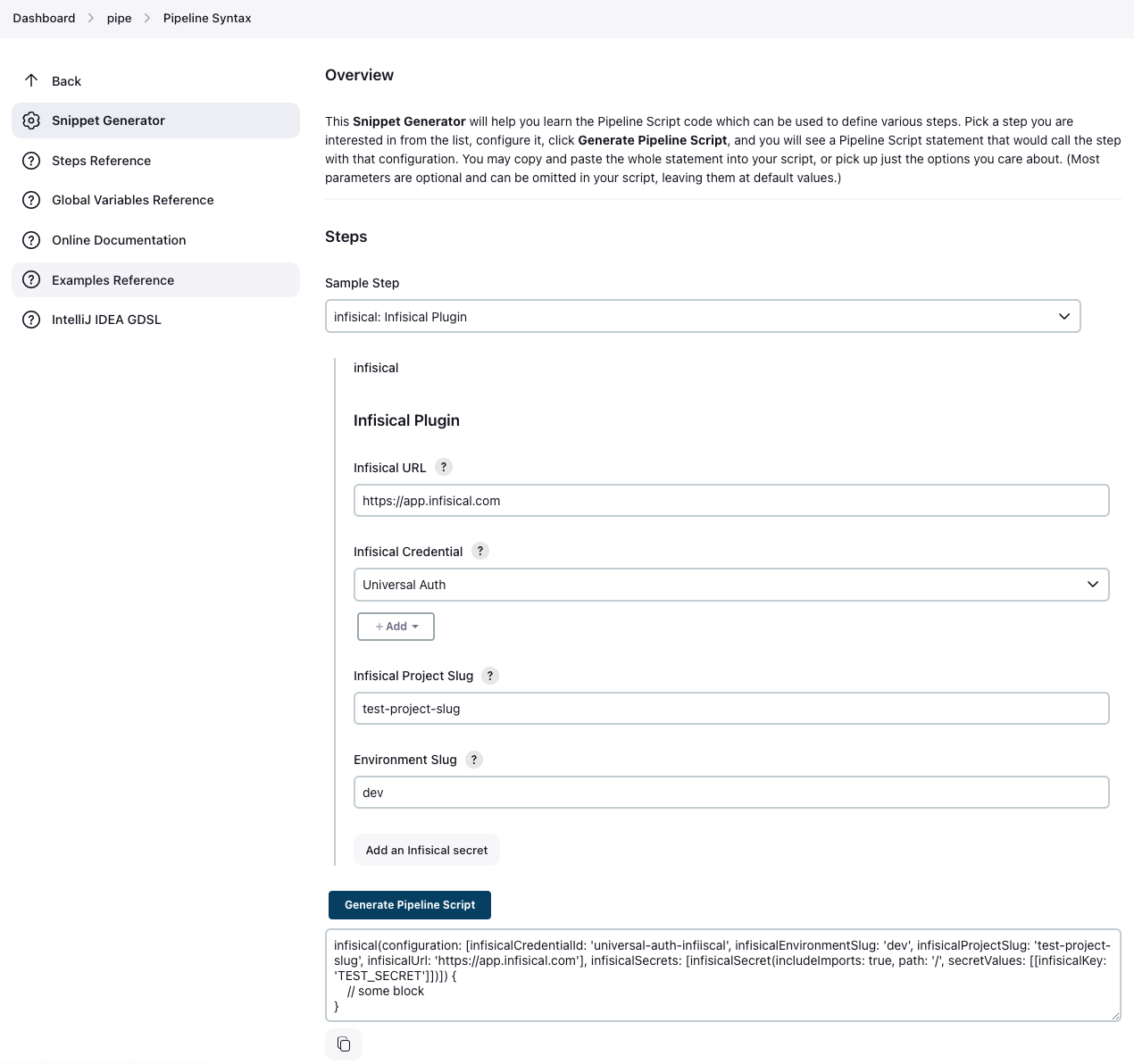
### Using Infisical in a Pipeline
Using the generated block in a pipeline is very straight forward. There's a few approaches on how to implement the block in a Pipeline script.
Here's an example of using the generated block in a pipeline script. Make sure to replace the placeholder values with your own values.
The script is formatted for clarity. All these fields will be pre-filled for you if you use the `Snippet Generator` like described in the [step above](#generating-pipeline-block).
```groovy
node {
withInfisical(
configuration: [
infisicalCredentialId: 'YOUR_CREDENTIAL_ID',
infisicalEnvironmentSlug: 'PROJECT_ENV_SLUG',
infisicalProjectSlug: 'PROJECT_SLUG',
infisicalUrl: 'https://app.infisical.com' // Change this to your Infisical instance URL if you aren't using Infisical Cloud.
],
infisicalSecrets: [
infisicalSecret(
includeImports: true,
path: '/',
secretValues: [
[infisicalKey: 'DATABASE_URL'],
[infisicalKey: "API_URL"],
[infisicalKey: 'THIS_KEY_MIGHT_NOT_EXIST', isRequired: false],
]
)
]
) {
// Code runs here
sh "printenv"
}
}
```
## Add Infisical Service Token to Jenkins
After setting up your project in Infisical and installing the Infisical CLI to the environment where your Jenkins builds will run, you will need to add the Infisical Service Token to Jenkins.
To generate a Infisical service token, follow the guide [here](/documentation/platform/token).
Once you have generated the token, navigate to **Manage Jenkins > Manage Credentials** in your Jenkins instance.
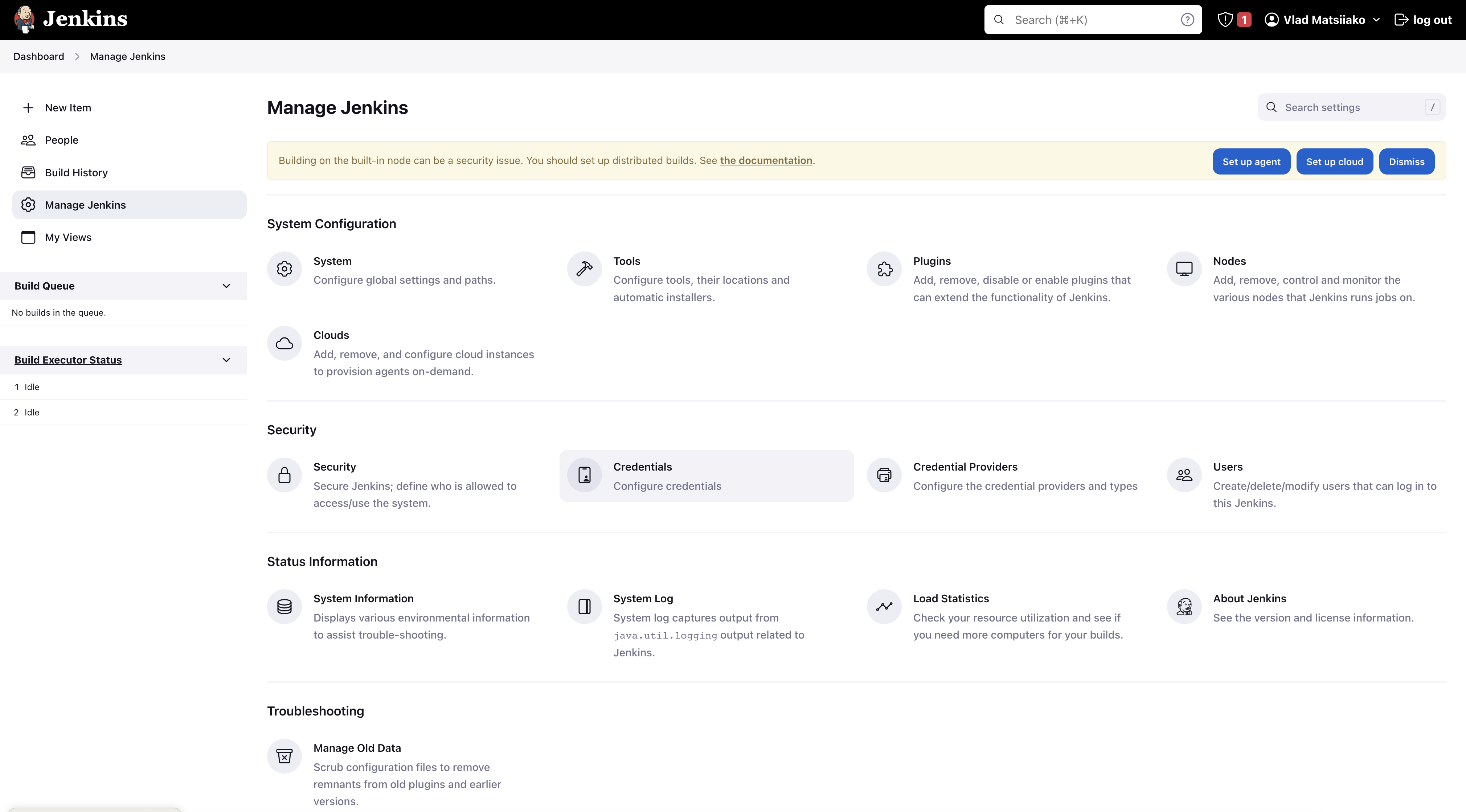
Click on the credential store you want to store the Infisical Service Token in. In this case, we're using the default Jenkins global store.
Each of your projects will have a different `INFISICAL_TOKEN`.
As a result, it may make sense to spread these out into separate credential domains depending on your use case.
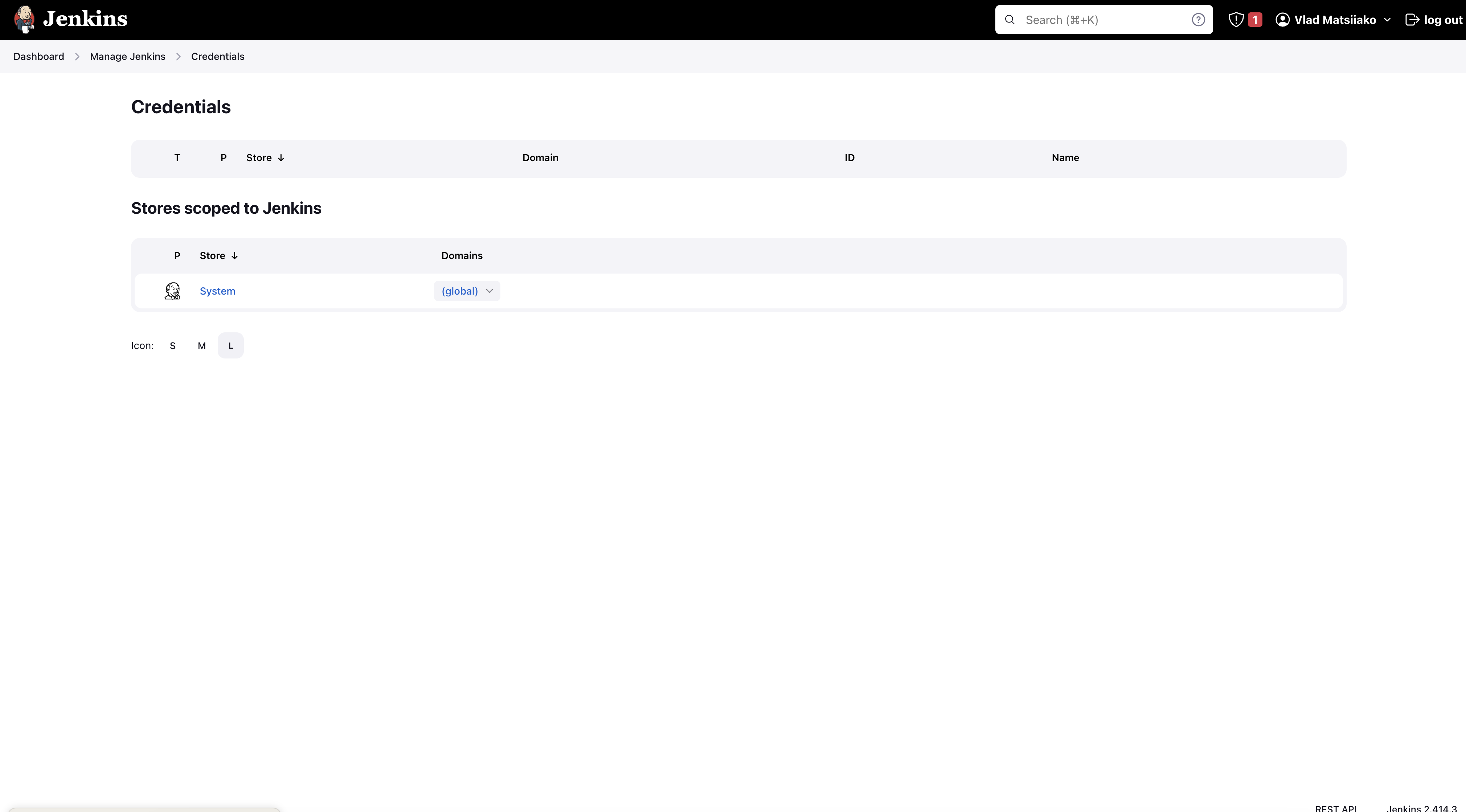
Now, click Add Credentials.

Choose **Secret text** for the **Kind** option from the dropdown list and enter the Infisical Service Token in the **Secret** field.
Although the **ID** can be any value, we'll set it to `infisical-service-token` for the sake of this guide.
The description is optional and can be any text you prefer.
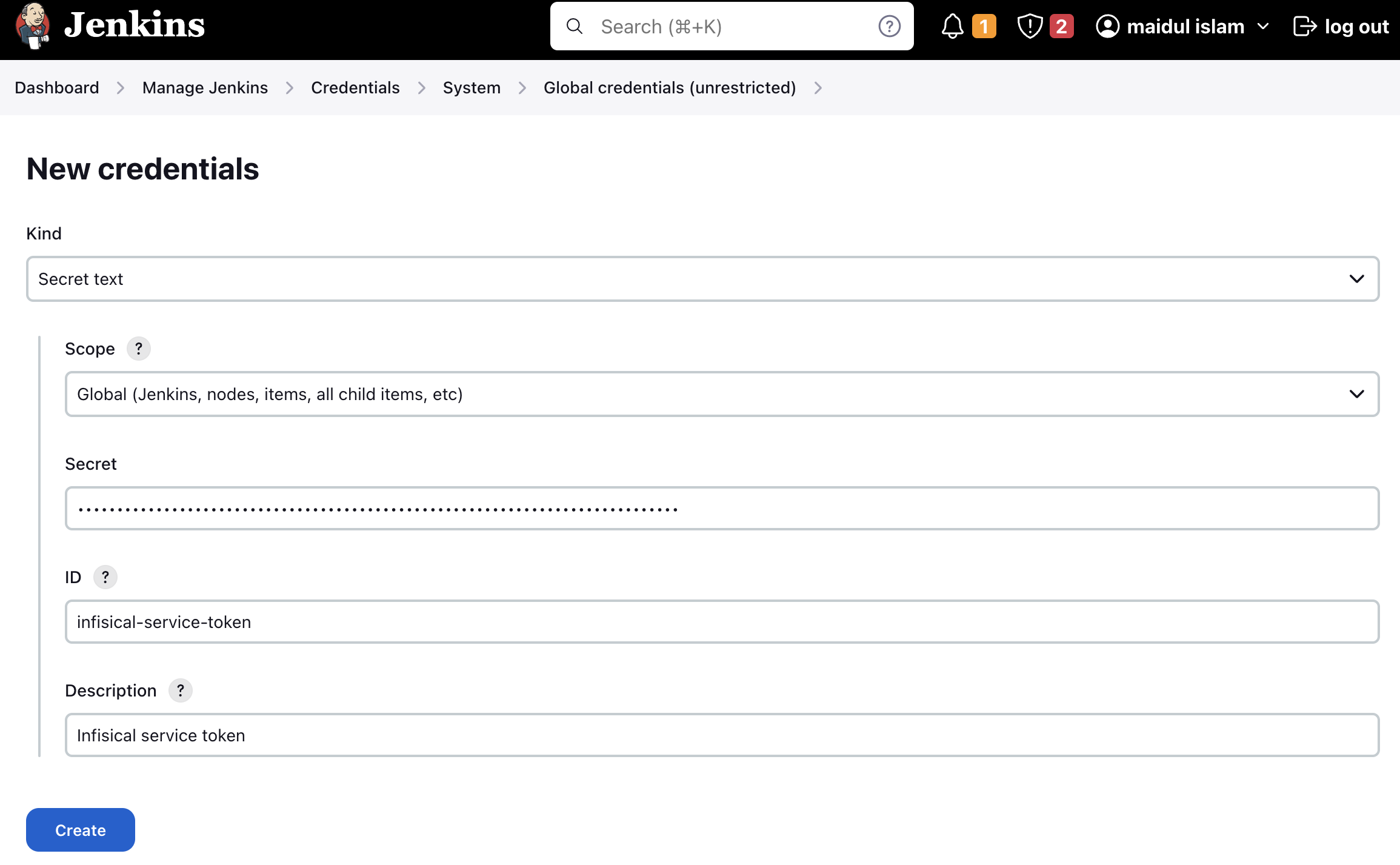
When you're done, you should see a credential similar to the one below:
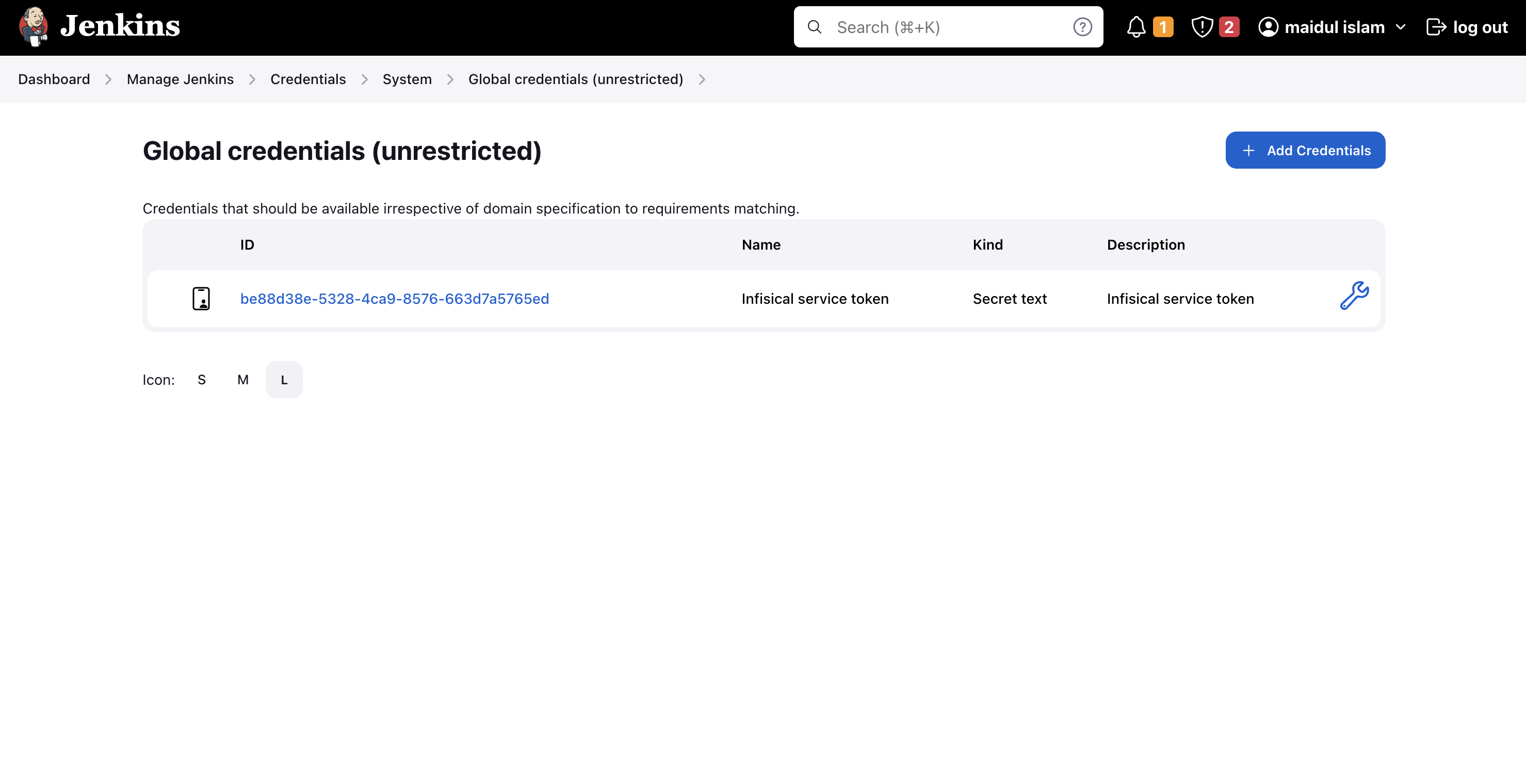
## Use Infisical in a Freestyle Project
To fetch secrets with Infisical in a Freestyle Project job, you'll need to expose the credential you created above as an environment variable to the Infisical CLI.
To do so, first click **New Item** from the dashboard navigation sidebar:
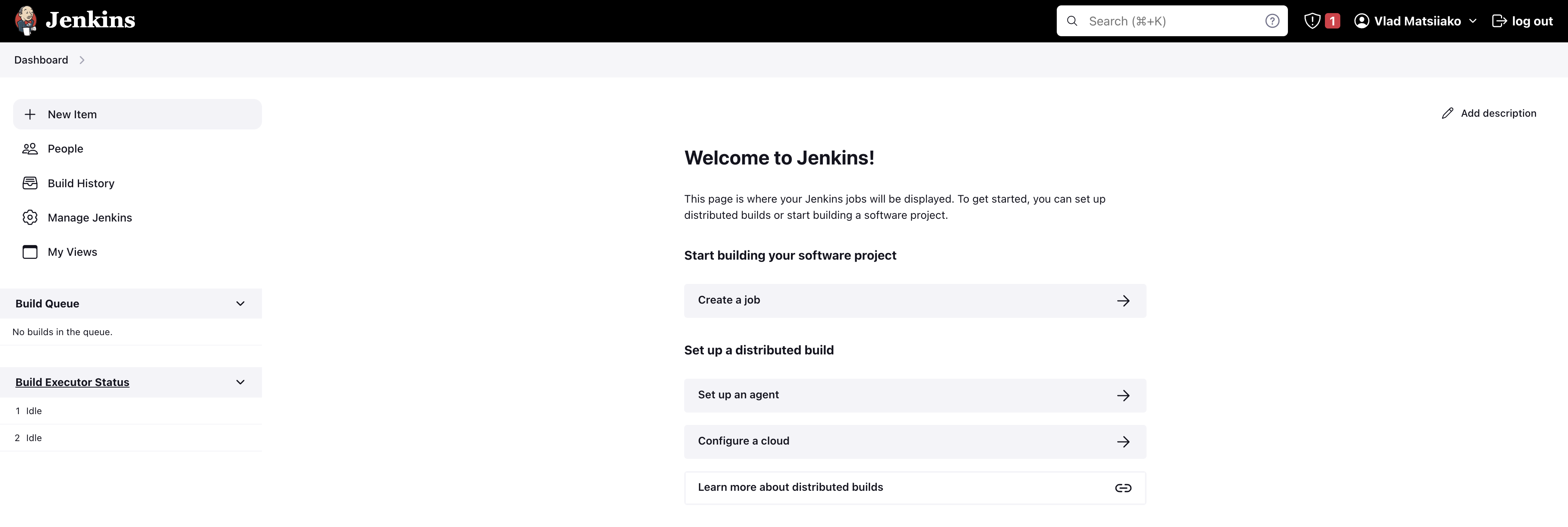
Enter the name of the job, choose the **Freestyle Project** option, and click **OK**.
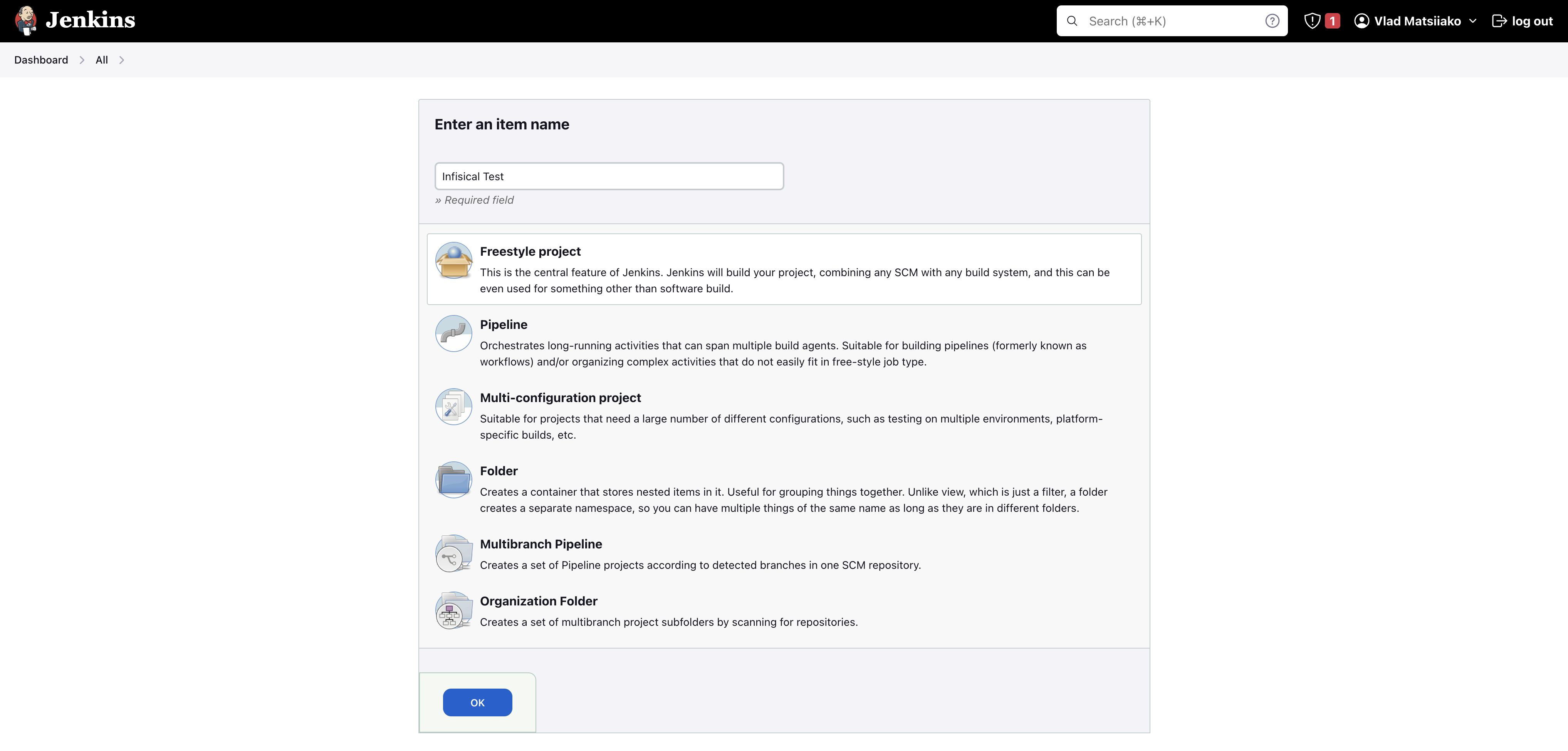
Scroll down to the **Build Environment** section and enable the **Use secret text(s) or file(s)** option. Then click **Add** under the **Bindings** section and choose **Secret text** from the dropdown menu.
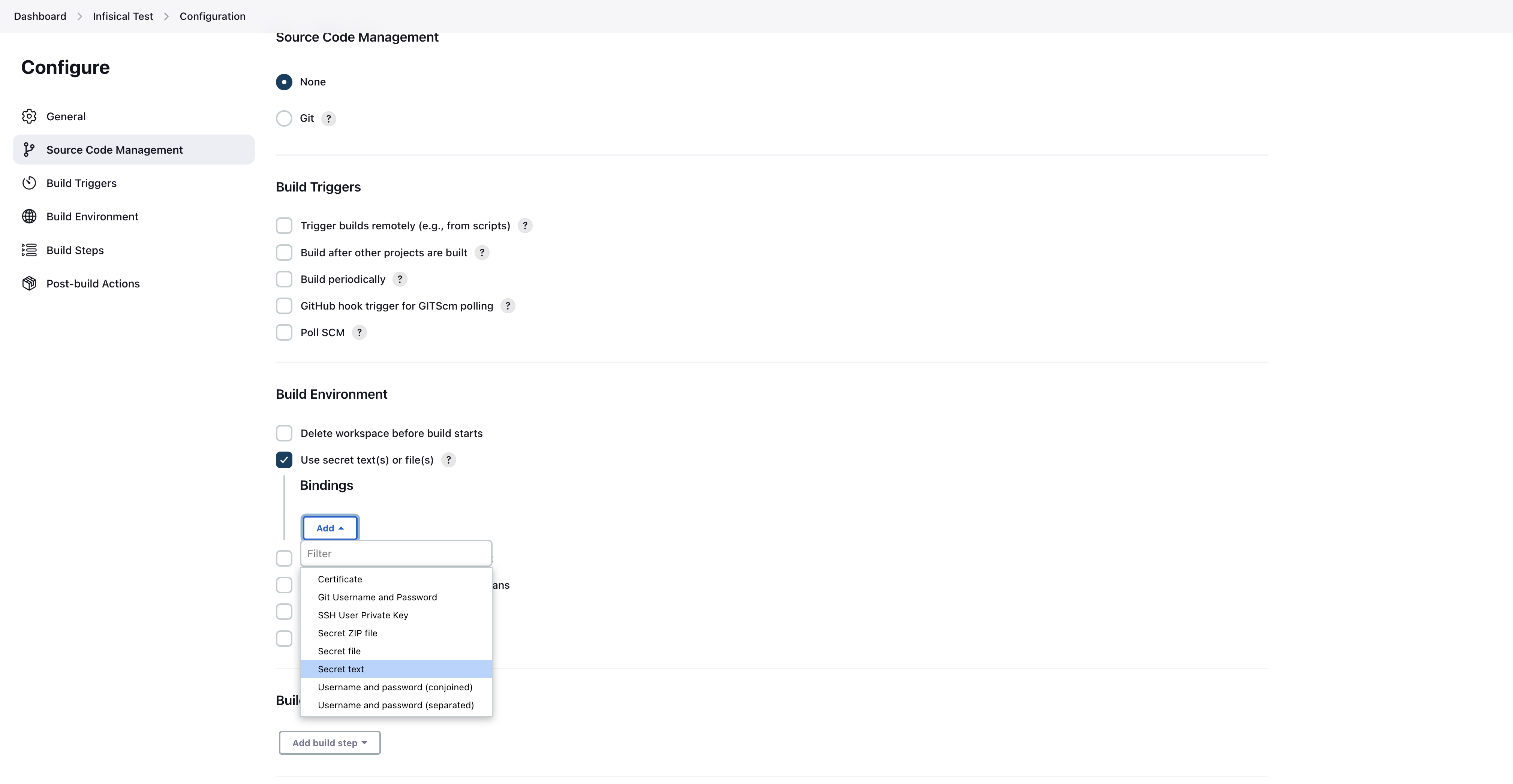
Enter `INFISICAL_TOKEN` in the **Variable** field then click the **Specific credentials** option from the Credentials section and select the credential you created earlier.
In this case, we saved it as `Infisical service token` so we'll choose that from the dropdown menu.
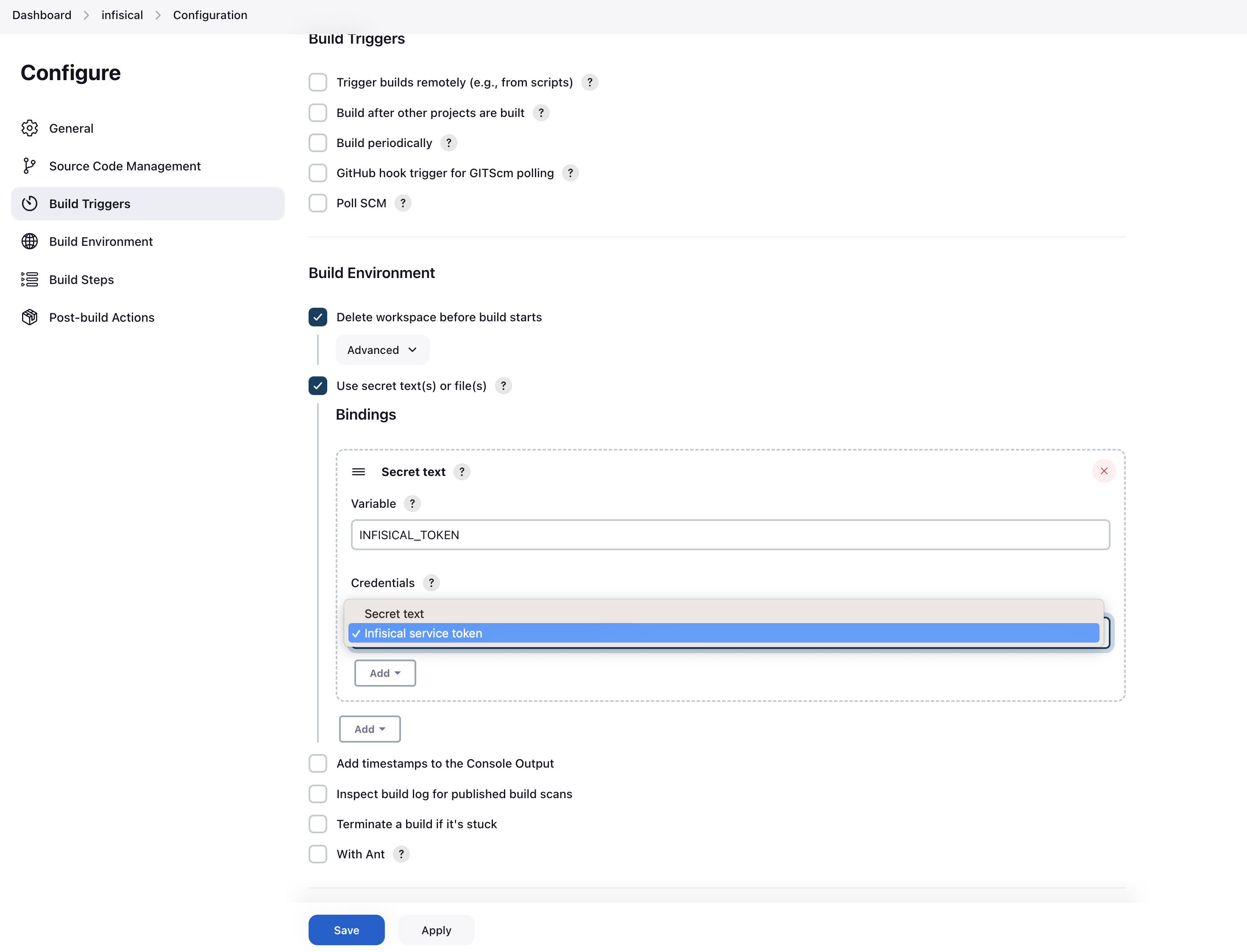
Scroll down to the **Build** section and choose **Execute shell** from the **Add build step** menu.
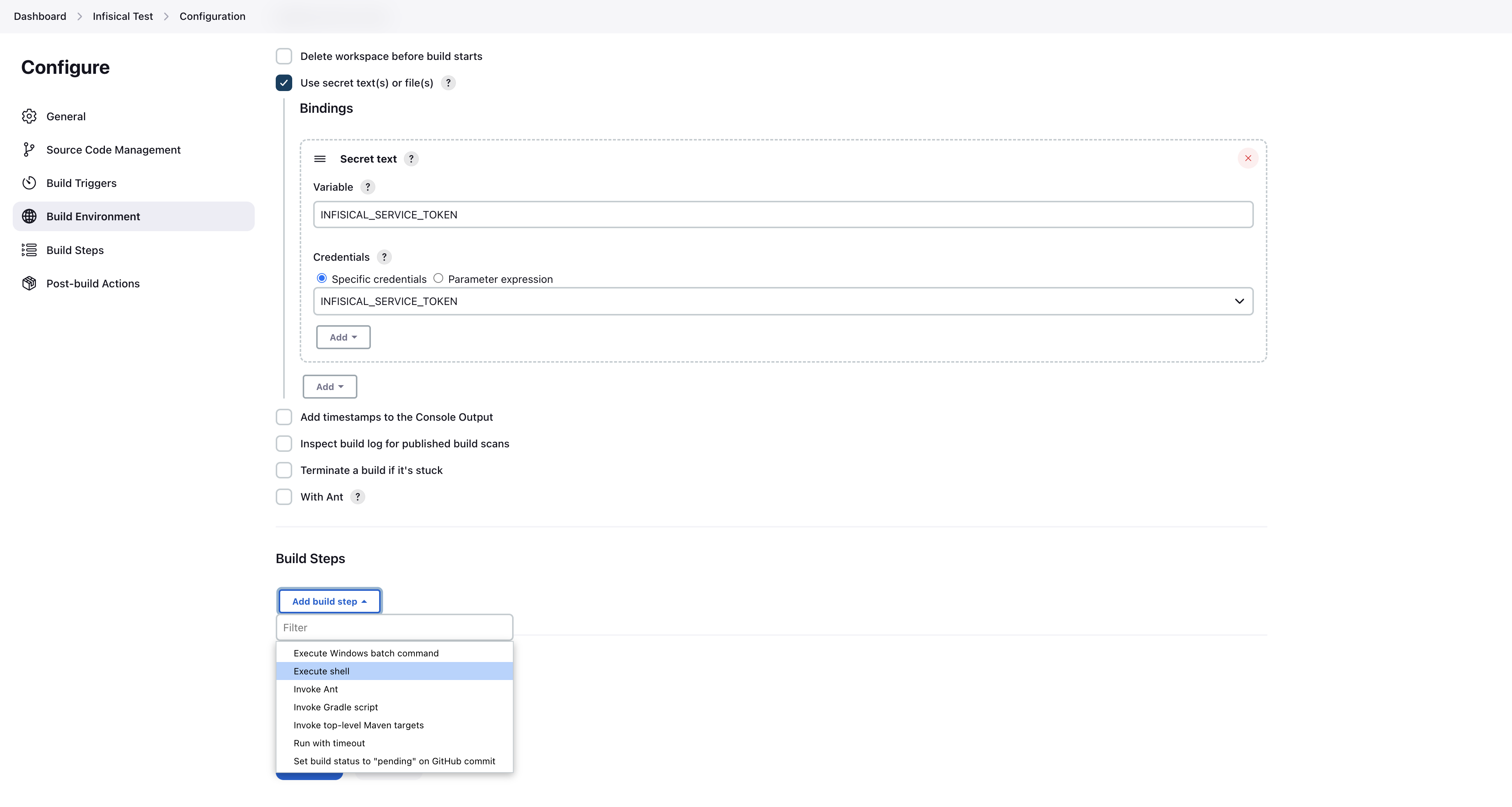
In the command field, you can now use the Infisical CLI to fetch secrets.
The example command below will print the secrets using the service token passed as a credential. When done, click **Save**.
```
infisical secrets --env=dev --path=/
```
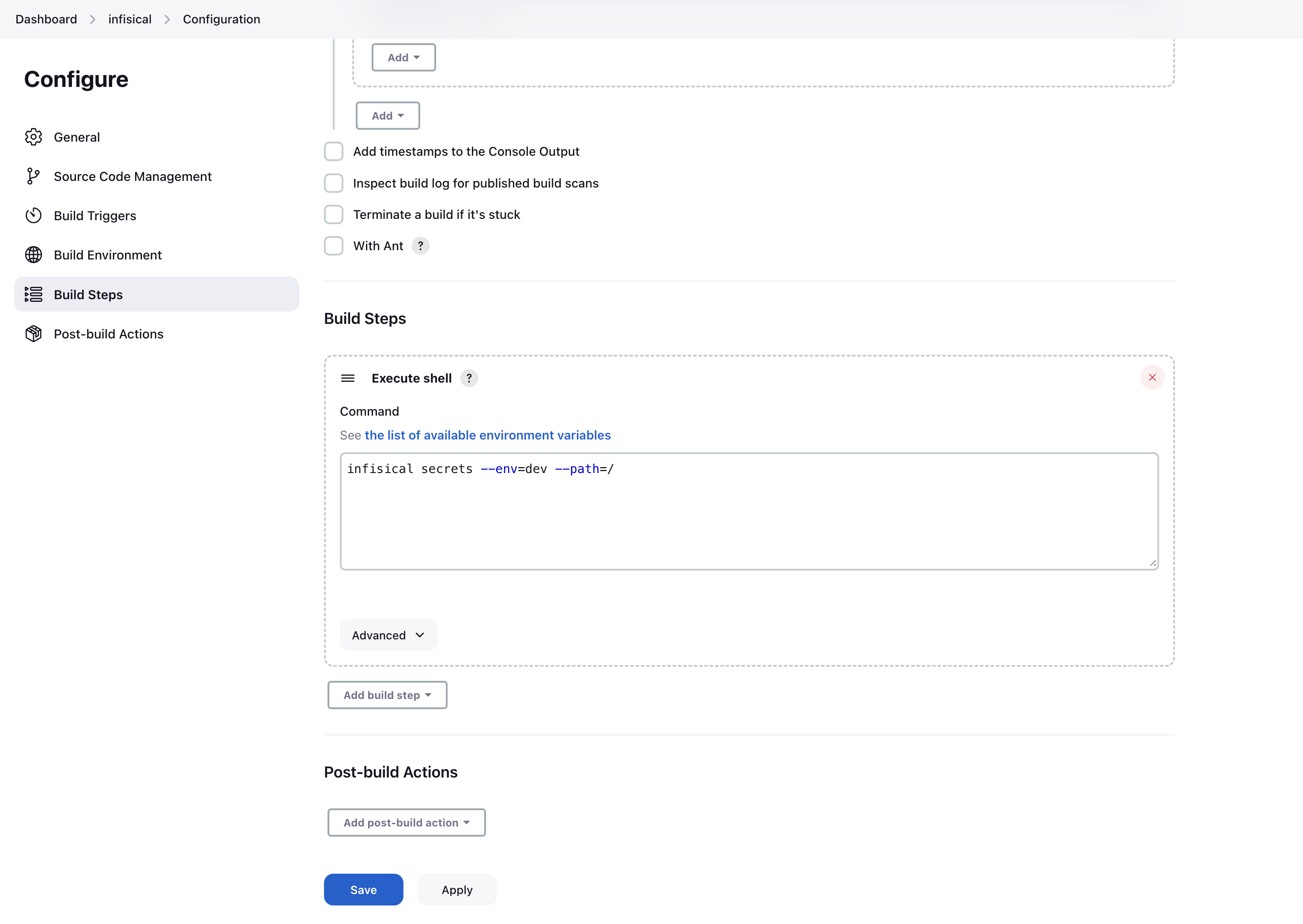
Finally, click **Build Now** from the navigation sidebar to run your new job.
Running into issues? Join Infisical's [community Slack](https://infisical.com/slack) for quick support.
## Use Infisical in a Jenkins Pipeline
To fetch secrets using Infisical in a Pipeline job, you'll need to expose the Jenkins credential you created above as an environment variable.
To do so, click **New Item** from the dashboard navigation sidebar:
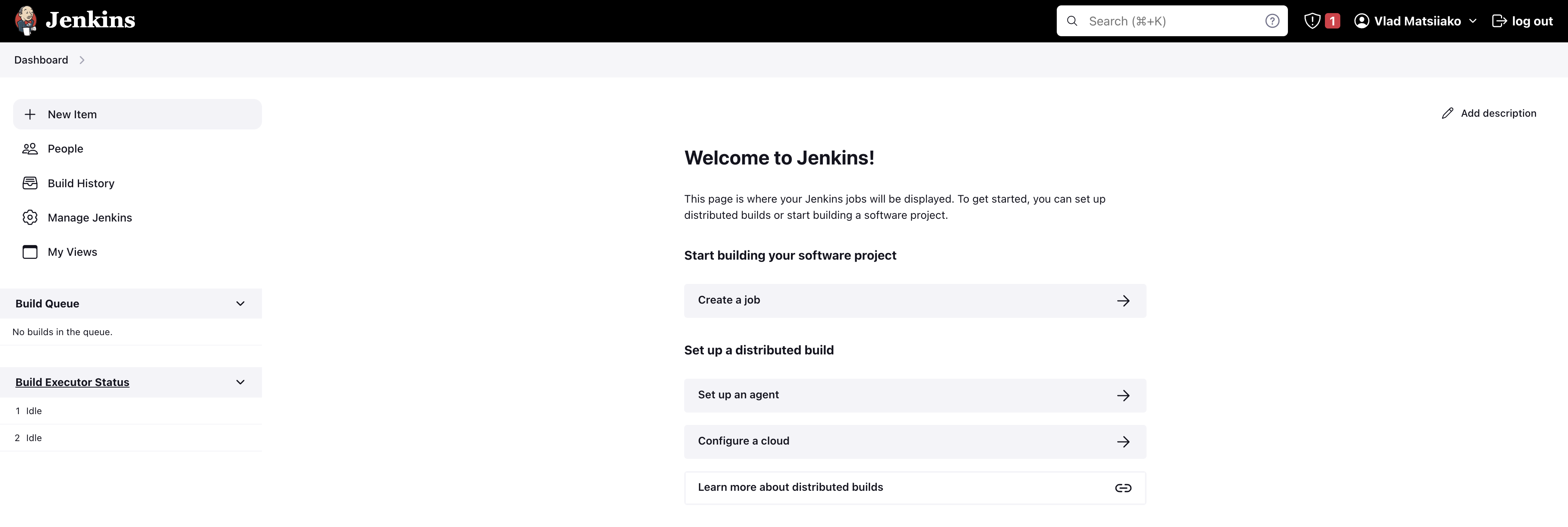
Enter the name of the job, choose the **Pipeline** option, and click OK.
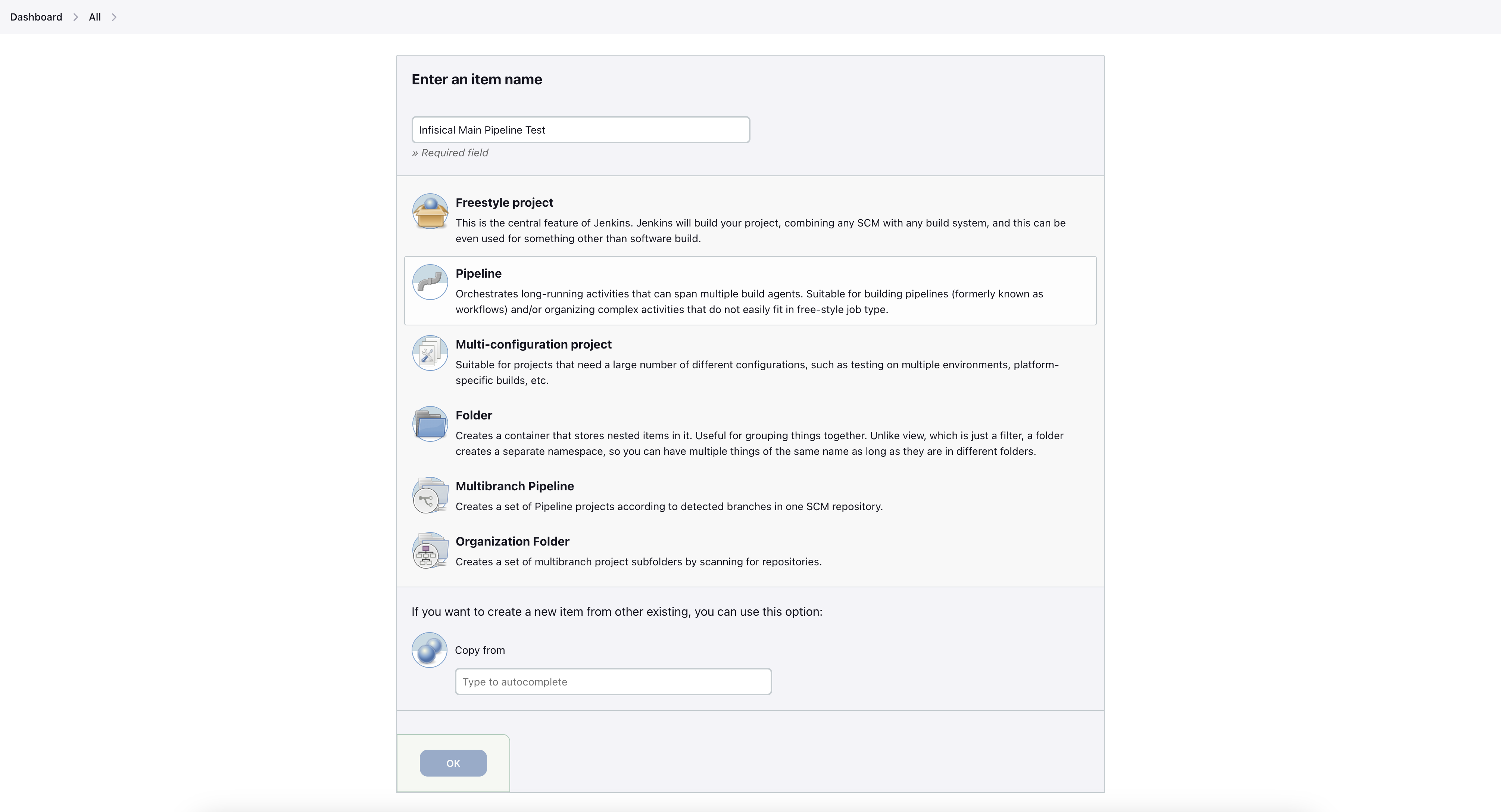
Scroll down to the **Pipeline** section, paste the following into the **Script** field, and click **Save**.
```
pipeline {
agent any
environment {
INFISICAL_TOKEN = credentials('infisical-service-token')
}
stages {
stage('Run Infisical') {
steps {
sh("infisical secrets --env=dev --path=/")
// doesn't work
// sh("docker run --rm test-container infisical secrets")
// works
// sh("docker run -e INFISICAL_TOKEN=${INFISICAL_TOKEN} --rm test-container infisical secrets --env=dev --path=/")
// doesn't work
// sh("docker-compose up -d")
// works
// sh("INFISICAL_TOKEN=${INFISICAL_TOKEN} docker-compose up -d")
}
}
}
}
```
The example provided above serves as an initial guide. It shows how Jenkins adds the `INFISICAL_TOKEN` environment variable, which is configured in the pipeline, into the shell for executing commands.
There may be instances where this doesn't work as expected in the context of running Docker commands.
However, the list of working examples should provide some insight into how this can be handled properly.
# Rundeck
How to sync secrets from Infisical to Rundeck
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
Obtain a User API Token in the Profile settings of Rundeck
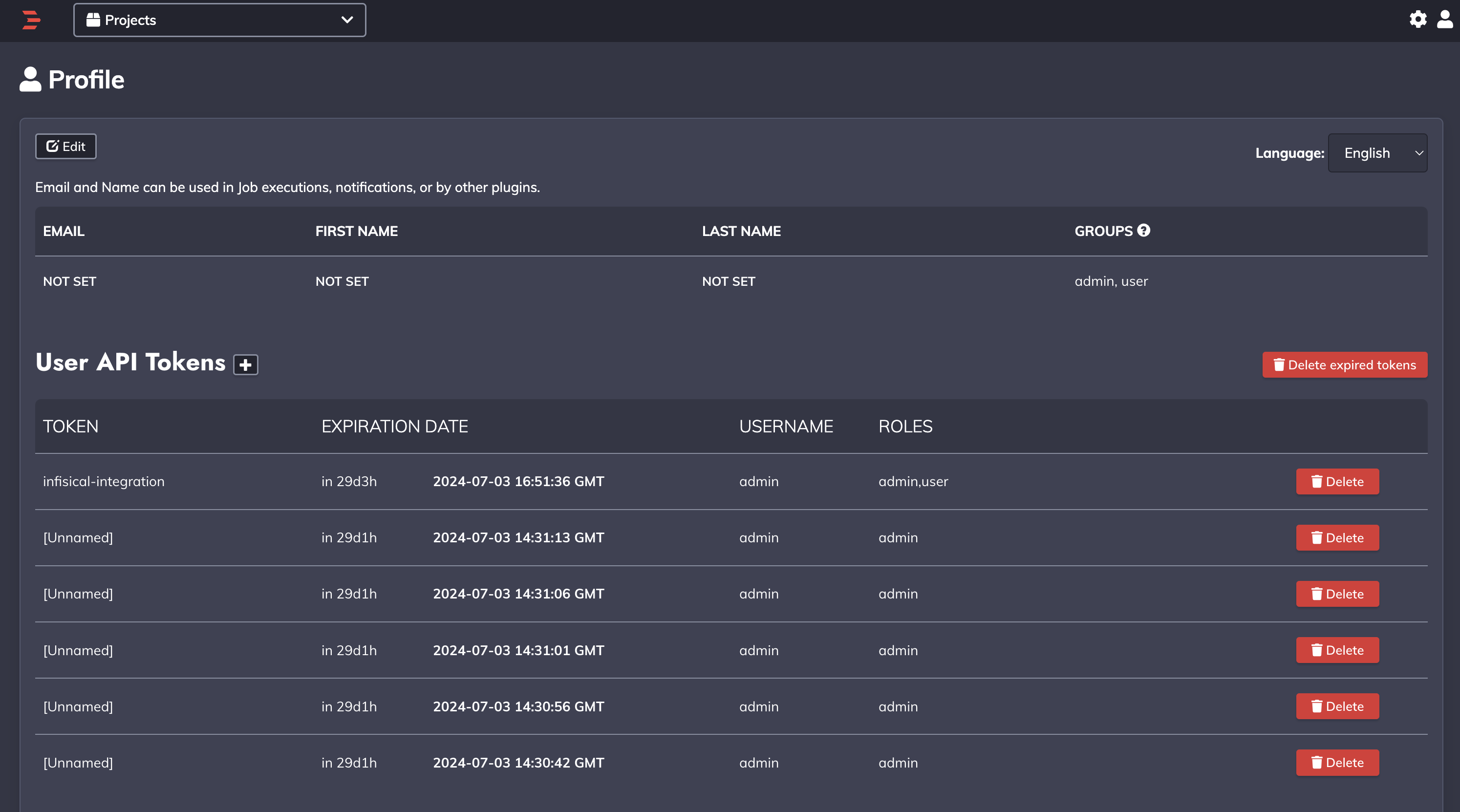
Navigate to your project's integrations tab in Infisical.
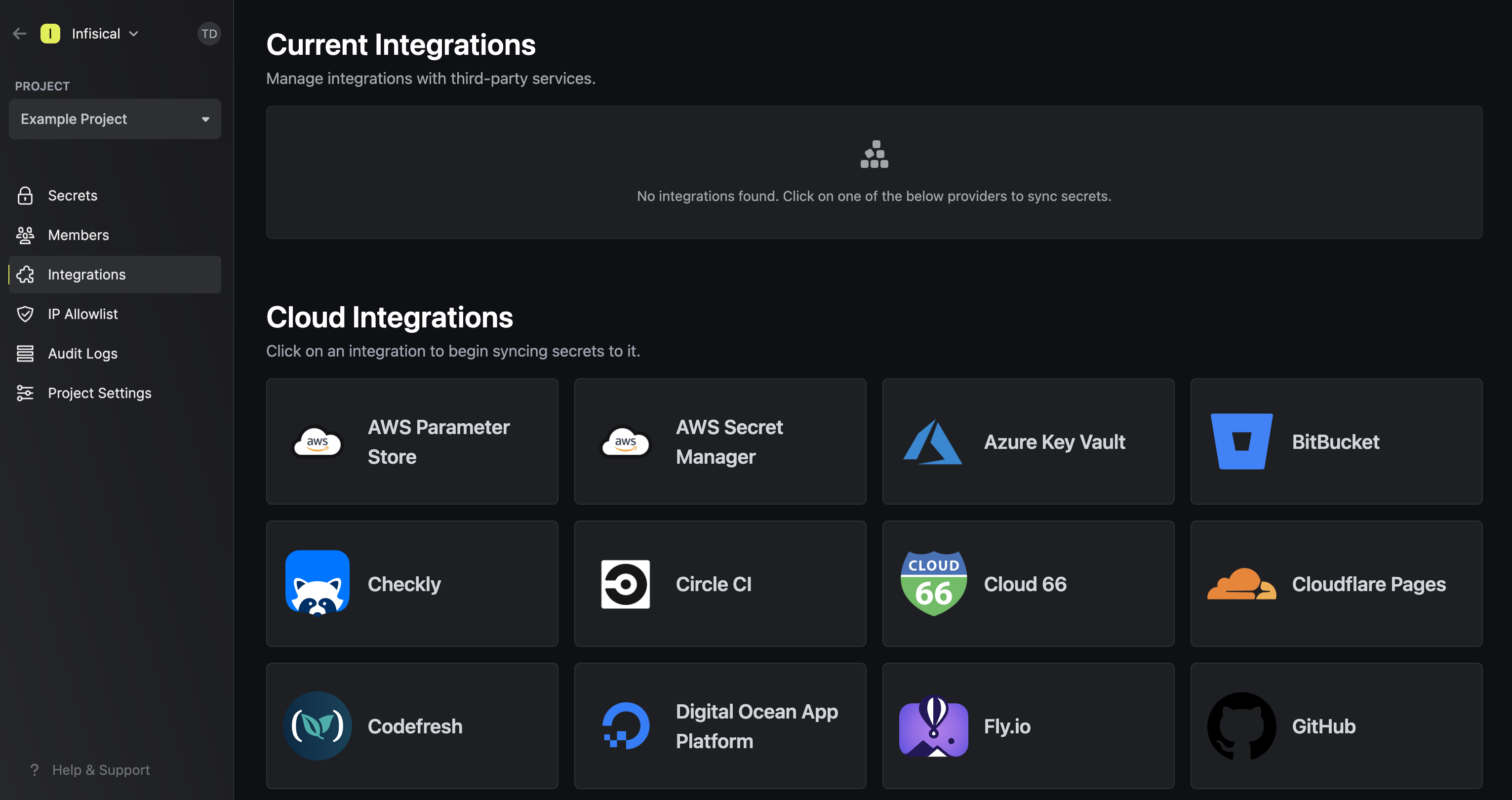
Press on the Rundeck tile and input your Rundeck instance Base URL and User API token to grant Infisical access to manage Rundeck keys
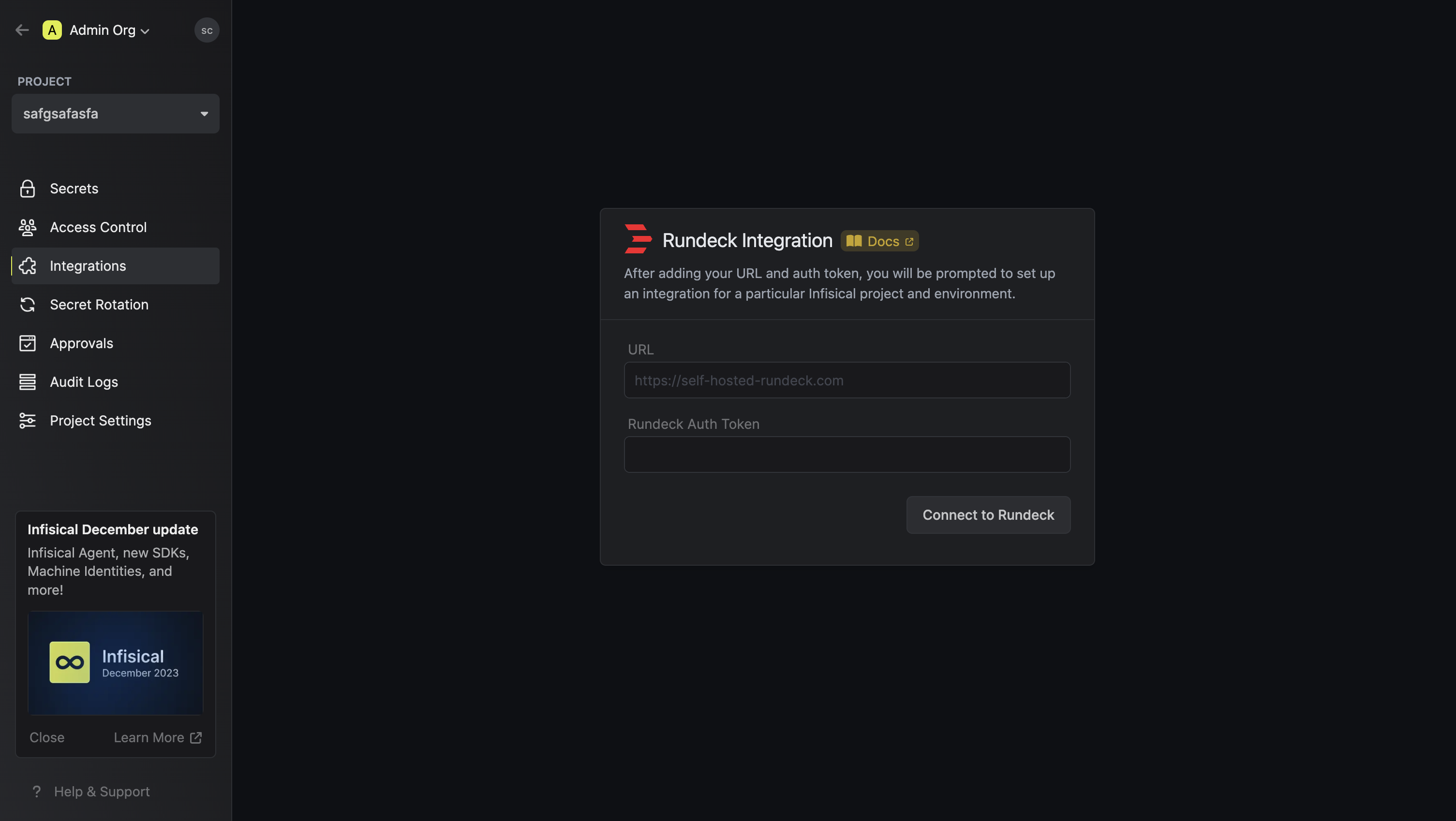
Select which Infisical environment secrets you want to sync to a Rundeck Key Storage Path and press create integration to start syncing secrets to Rundeck.
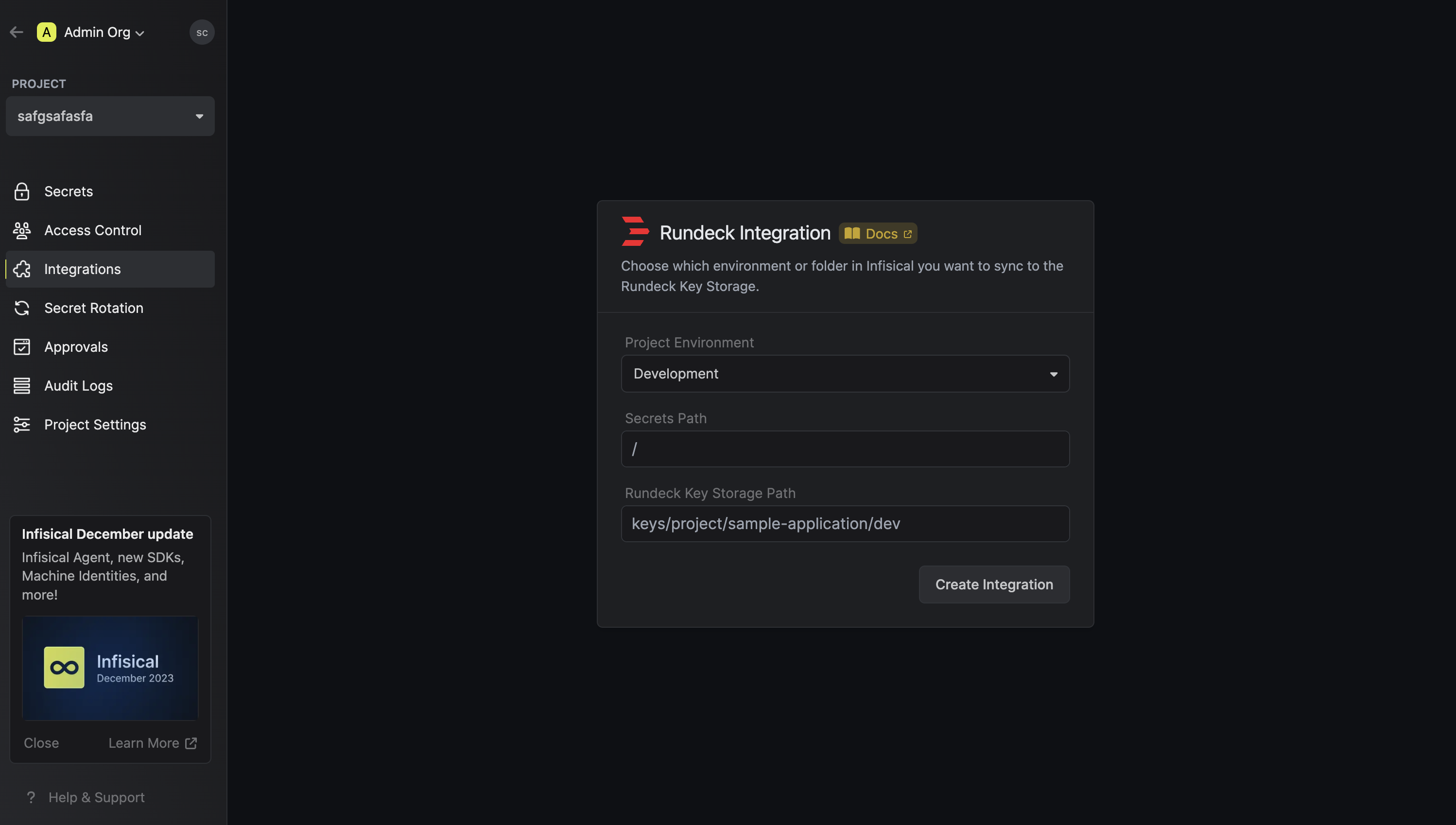
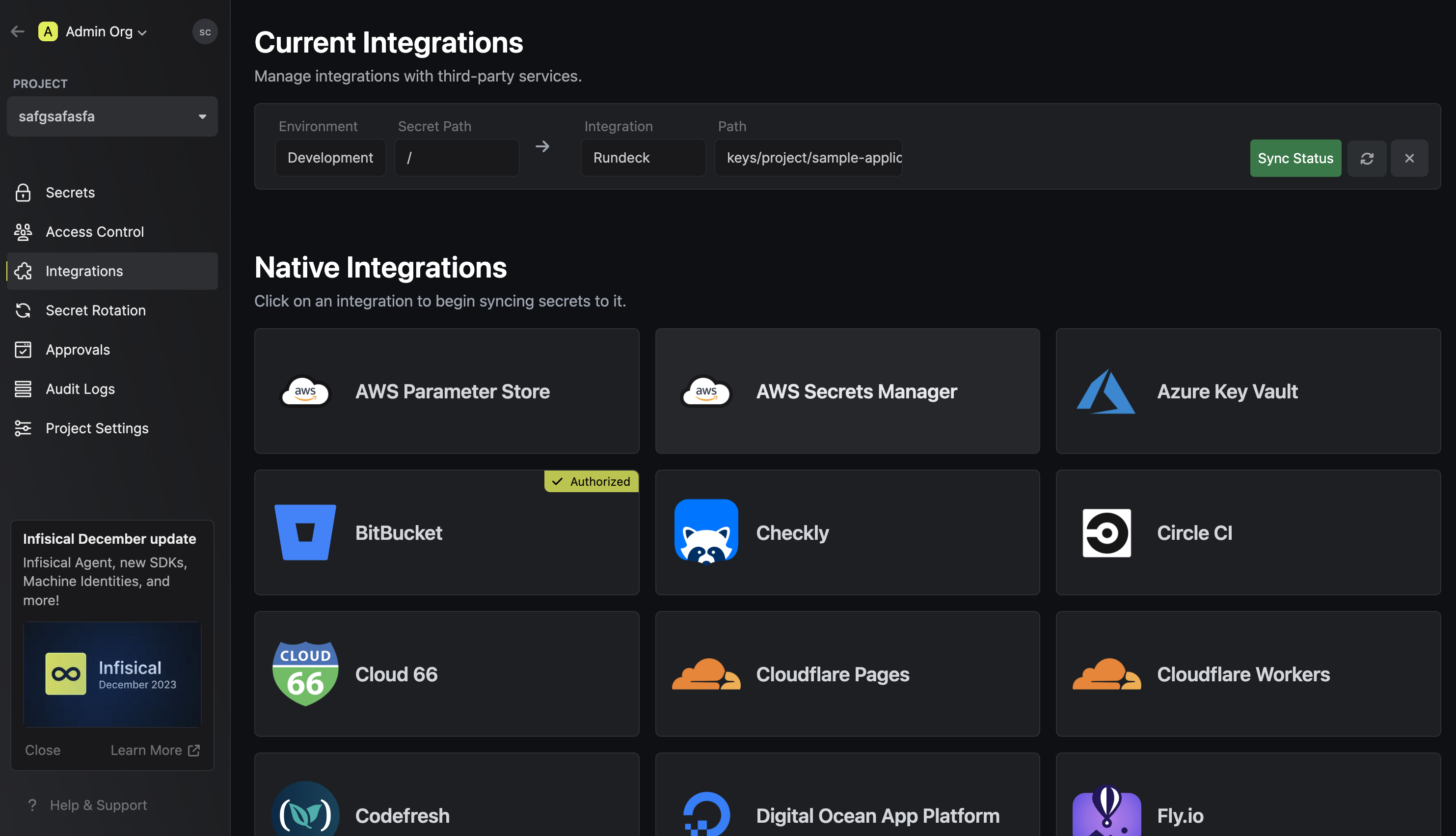
# Travis CI
How to sync secrets from Infisical to Travis CI
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
Obtain your API token in User Settings > API authentication > Token
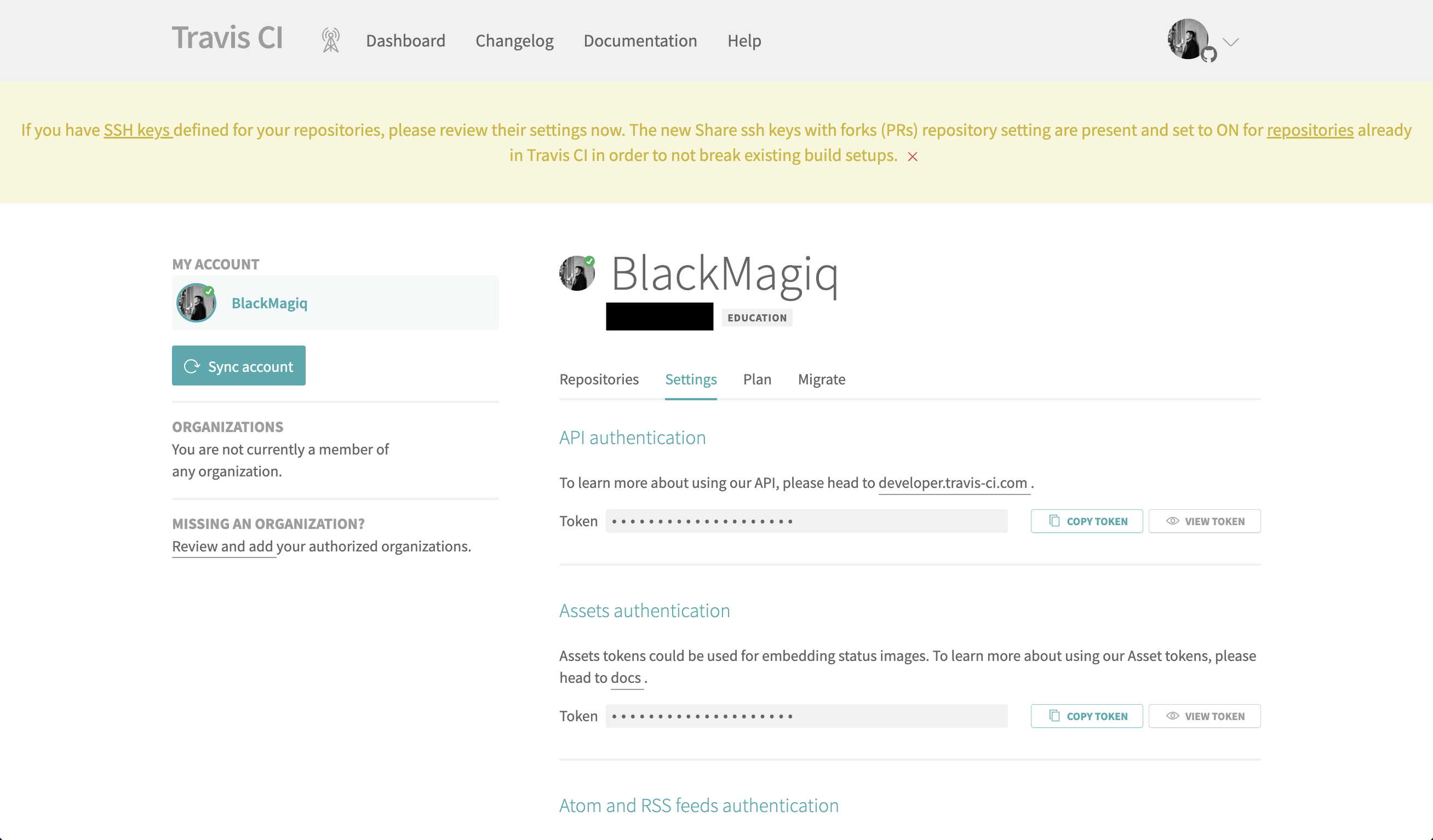
Navigate to your project's integrations tab in Infisical.
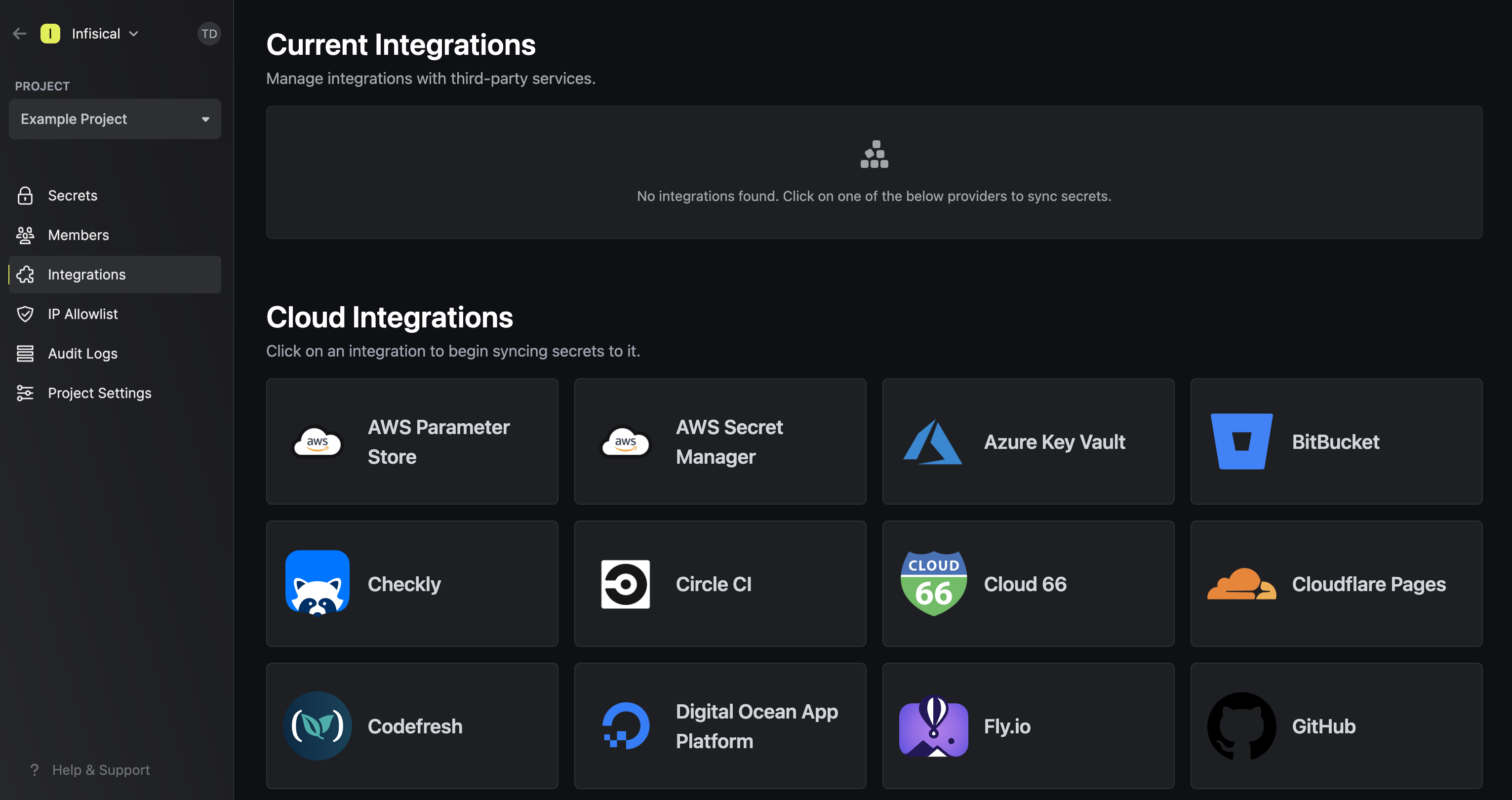
Press on the Travis CI tile and input your Travis CI API token to grant Infisical access to your Travis CI account.
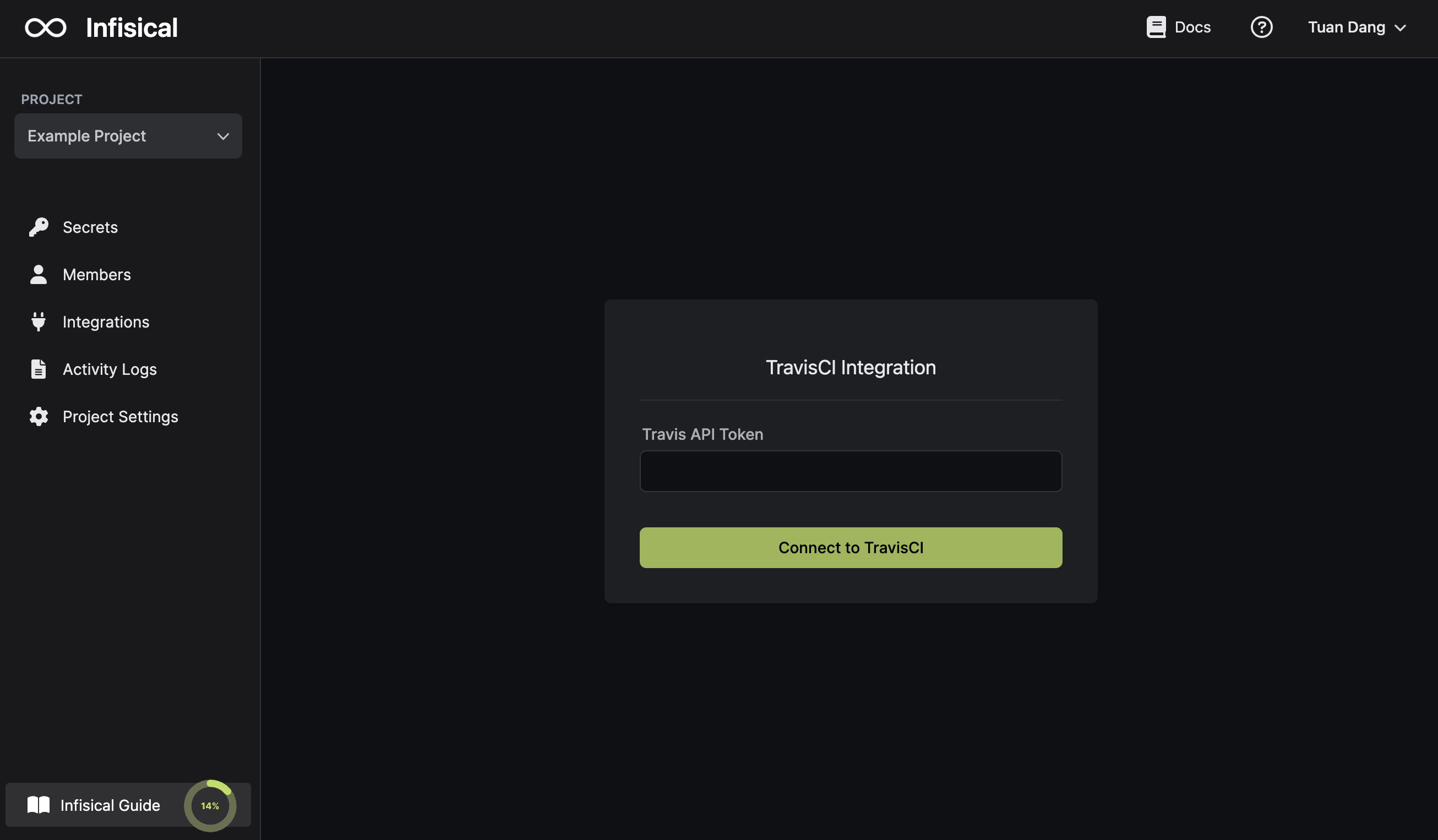
Select which Infisical environment secrets you want to sync to which Travis CI repository and press create integration to start syncing secrets to Travis CI.
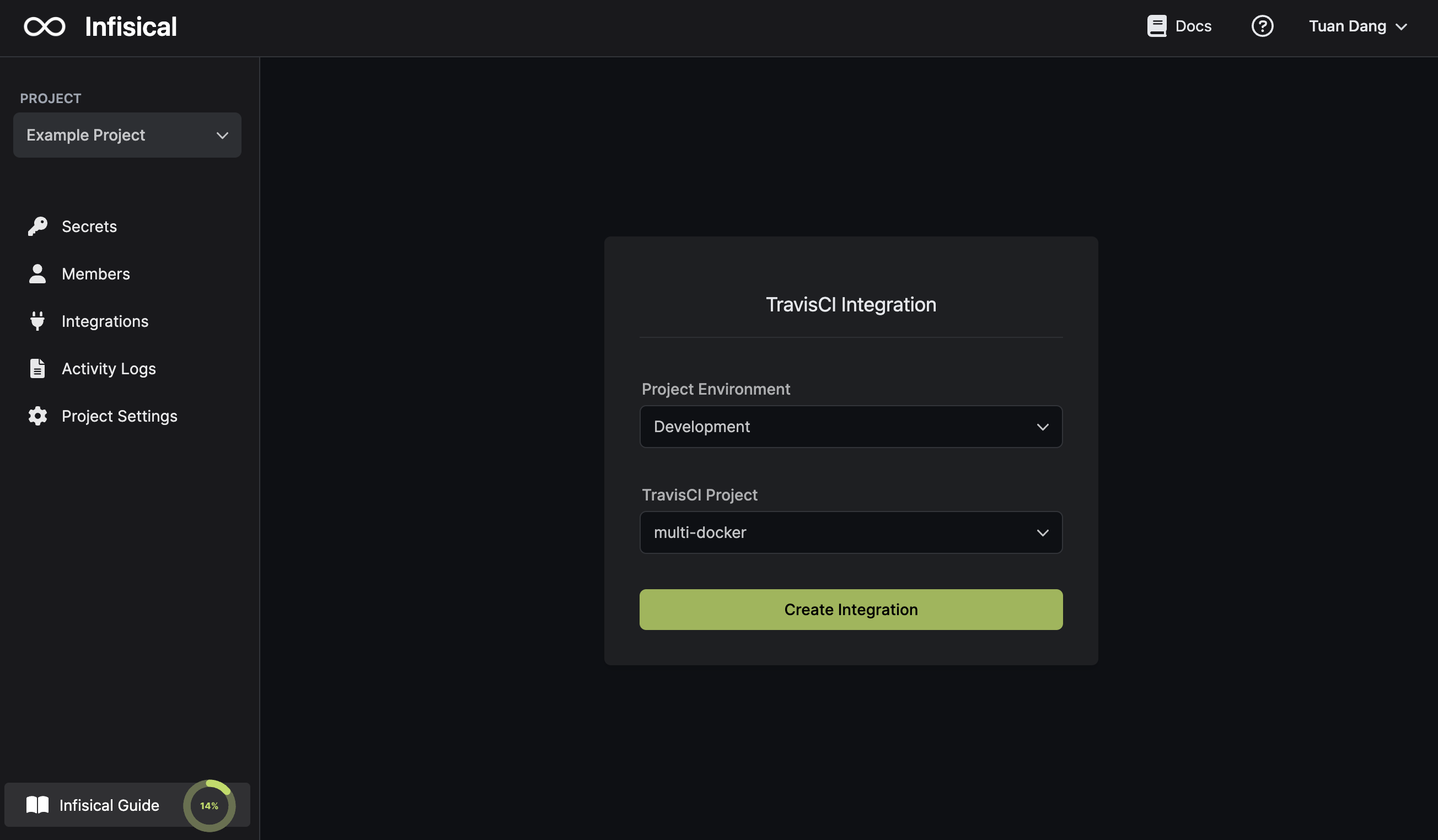
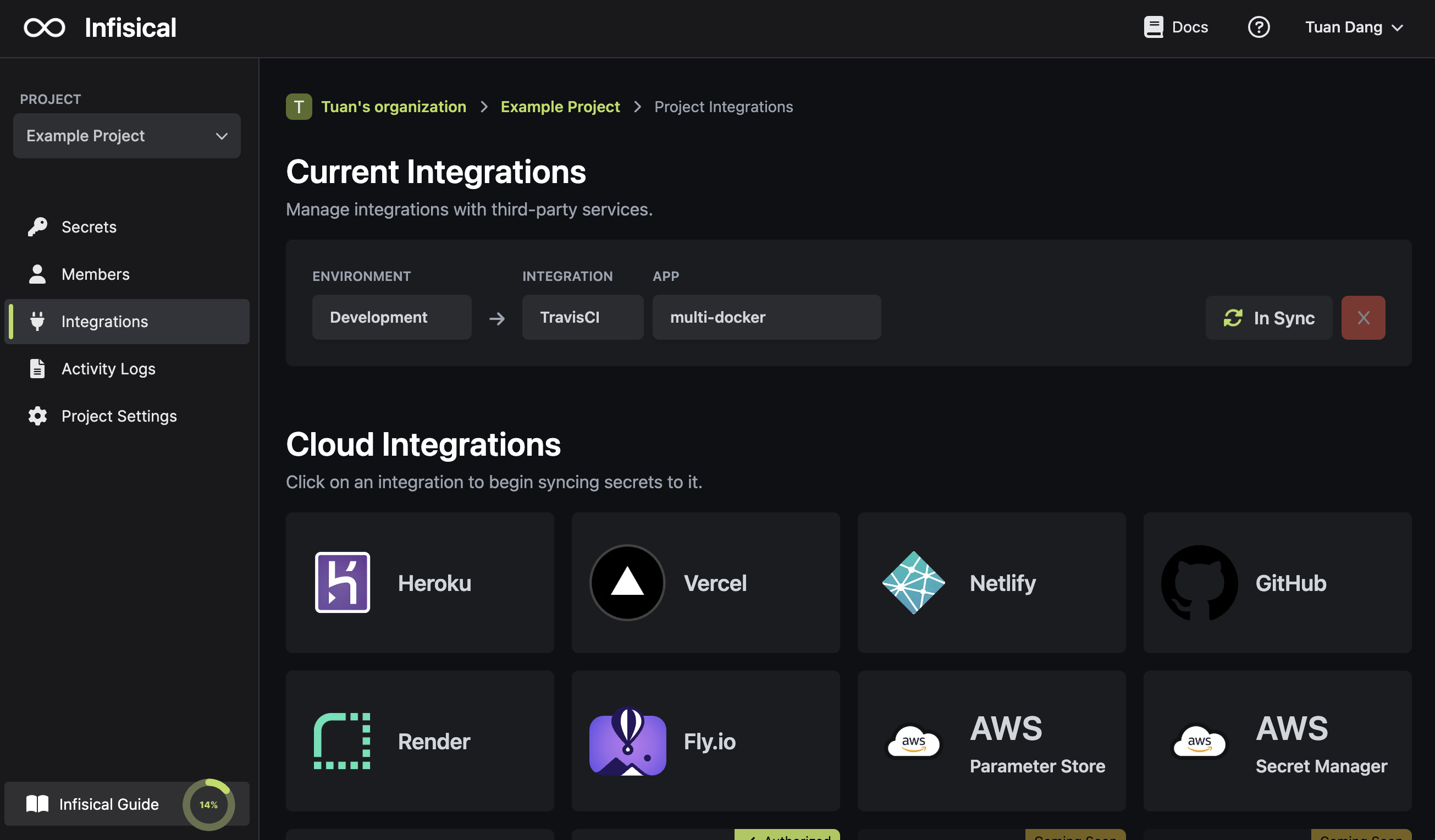
# AWS Amplify
Learn how to sync secrets from Infisical to AWS Amplify.
Prerequisites:
* Infisical Cloud account
* Add the secrets you wish to sync to Amplify to [Infisical Cloud](https://app.infisical.com)
There are many approaches to sync secrets stored within Infisical to AWS Amplify. This guide describes two such approaches below.
## Access Infisical secrets at Amplify build time
This approach enables you to fetch secrets from Infisical during Amplify build time.
Create a machine identtiy and connect it to your Infisical project. You can read more about how to use machine identities [here](/documentation/platform/identities/machine-identities). The machine identity will allow you to authenticate and fetch secrets from Infisical.
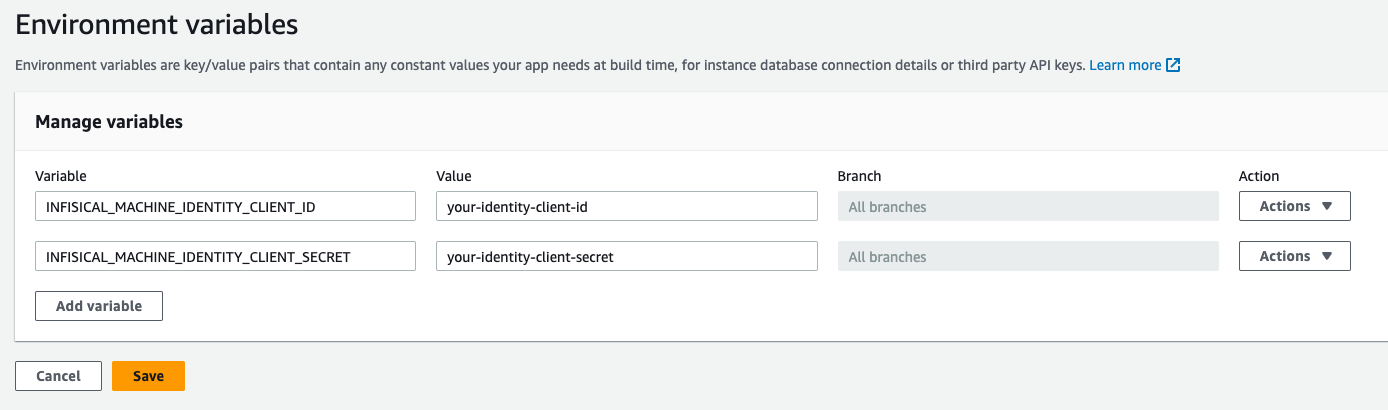
1. In the Amplify console, choose App Settings, and then select Environment variables.
2. In the Environment variables section, select Manage variables.
3. Under the first Variable enter `INFISICAL_MACHINE_IDENTITY_CLIENT_ID`, and for the value, enter the client ID of the machine identity you created in the previous step.
4. Under the second Variable enter `INFISICAL_MACHINE_IDENTITY_CLIENT_SECRET`, and for the value, enter the client secret of the machine identity you created in the previous step.
5. Click save.
In the prebuild phase, add the command in AWS Amplify to install the Infisical CLI.
```yaml
build:
phases:
preBuild:
commands:
- sudo curl -1sLf 'https://dl.cloudsmith.io/public/infisical/infisical-cli/setup.rpm.sh' | sudo -E bash
- sudo yum -y install infisical
```
You can now pull secrets from Infisical using the CLI and save them as a `.env` file. To do this, modify the build commands.
```yaml
build:
phases:
build:
commands:
- INFISICAL_TOKEN=$(infisical login --method=universal-auth --client-id=${INFISICAL_MACHINE_IDENTITY_CLIENT_ID} --client-secret=${INFISICAL_MACHINE_IDENTITY_CLIENT_SECRET} --silent --plain)
- infisical export --format=dotenv > .env
-
```
Go to your project settings in the Infisical dashboard to generate a [service token](/documentation/platform/token). This service token will allow you to authenticate and fetch secrets from Infisical. Once you have created a service token with the required permissions, you’ll need to provide the token to the CLI installed in your Docker container.
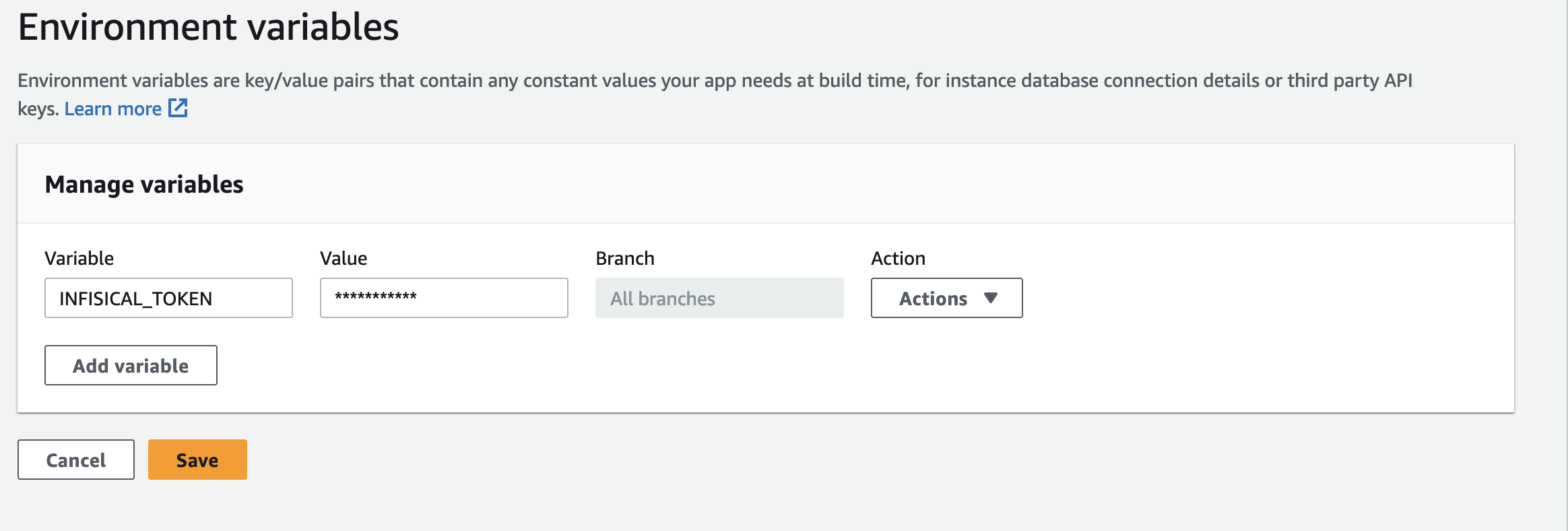
1. In the Amplify console, choose App Settings, and then select Environment variables.
2. In the Environment variables section, select Manage variables.
3. Under Variable, enter the key **INFISICAL\_TOKEN**. For the value, enter the generated service token from the previous step.
4. Click save.
In the prebuild phase, add the command in AWS Amplify to install the Infisical CLI.
```yaml
build:
phases:
preBuild:
commands:
- sudo curl -1sLf 'https://dl.cloudsmith.io/public/infisical/infisical-cli/setup.rpm.sh' | sudo -E bash
- sudo yum -y install infisical
```
You can now pull secrets from Infisical using the CLI and save them as a `.env` file. To do this, modify the build commands.
```yaml
build:
phases:
build:
commands:
- INFISICAL_TOKEN=${INFISICAL_TOKEN}
- infisical export --format=dotenv > .env
-
```
## Sync Secrets Using AWS SSM Parameter Store
Another approach to use secrets from Infisical in AWS Amplify is to utilize AWS Parameter Store.
At high level, you begin by using Infisical's AWS SSM Parameter Store integration to sync secrets from Infisical to AWS SSM Parameter Store. You then instruct AWS Amplify to consume those secrets from AWS SSM Parameter Store as [environment secrets](https://docs.aws.amazon.com/amplify/latest/userguide/environment-variables.html#environment-secrets).
Follow the [Infisical AWS SSM Parameter Store Integration Guide](./aws-parameter-store) to set up the integration. Pause once you reach the step where it asks you to select the path you would like to sync.
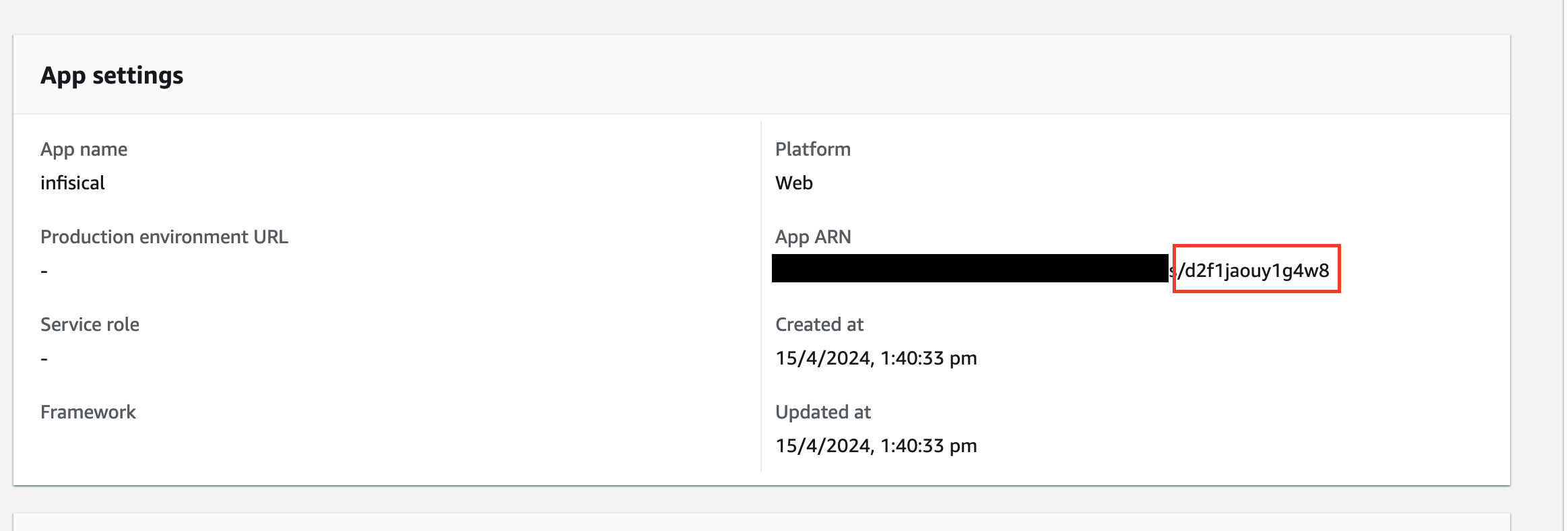
1. Open your AWS Amplify App console.
2. Go to **Actions >> View App Settings**
3. The App ID will be the last part of the App ARN field after the slash.
You need to set the path in the format `/amplify/[amplify_app_id]/[your-amplify-environment-name]` as the path option in AWS SSM Parameter Infisical Integration.
Accessing an environment secret during a build is similar to accessing
environment variables, except that environment secrets are stored in
`process.env.secrets` as a JSON string.
# AWS Parameter Store
Learn how to sync secrets from Infisical to AWS Parameter Store.
Infisical will assume the provided role in your AWS account securely, without the need to share any credentials.
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
To connect your Infisical instance with AWS, you need to set up an AWS IAM User account that can assume the AWS IAM Role for the integration.
If your instance is deployed on AWS, the aws-sdk will automatically retrieve the credentials. Ensure that you assign the provided permission policy to your deployed instance, such as ECS or EC2.
The following steps are for instances not deployed on AWS
Navigate to [Create IAM User](https://console.aws.amazon.com/iamv2/home#/users/create) in your AWS Console.
Attach the following inline permission policy to the IAM User to allow it to assume any IAM Roles:
```json
{
"Version": "2012-10-17",
"Statement": [
{
"Sid": "AllowAssumeAnyRole",
"Effect": "Allow",
"Action": "sts:AssumeRole",
"Resource": "arn:aws:iam::*:role/*"
}
]
}
```
Obtain the AWS access key ID and secret access key for your IAM User by navigating to IAM > Users > \[Your User] > Security credentials > Access keys.
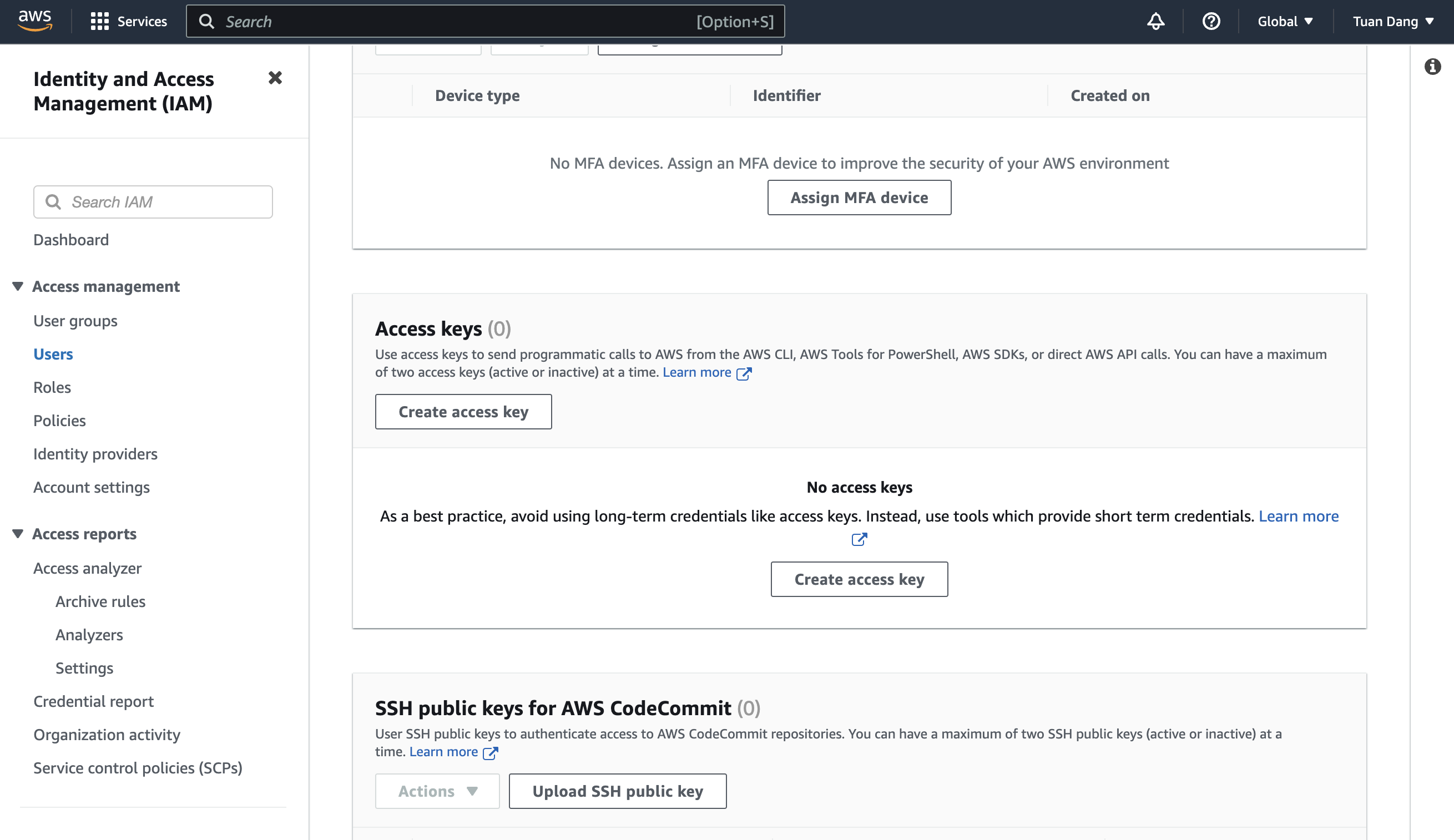
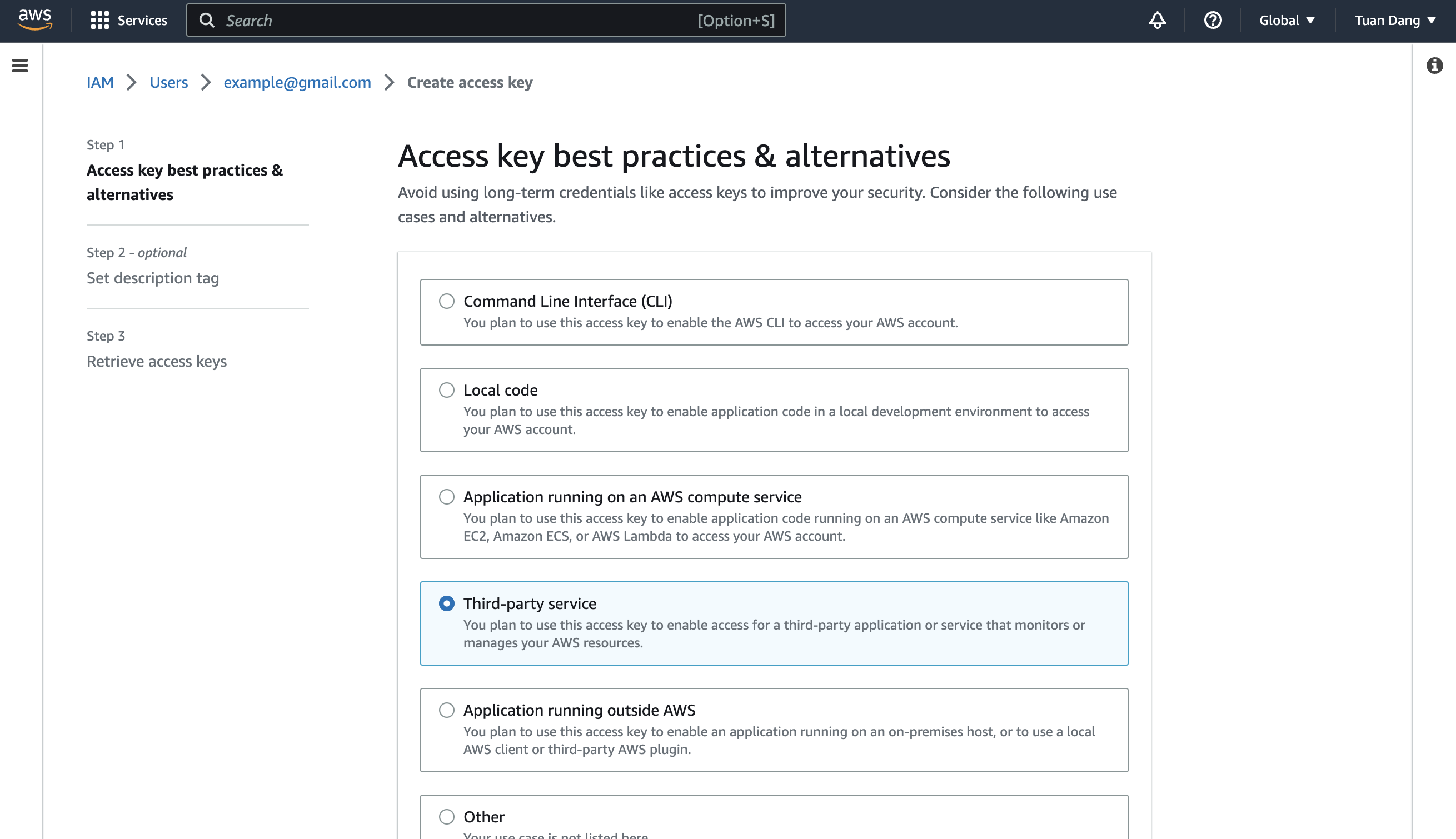
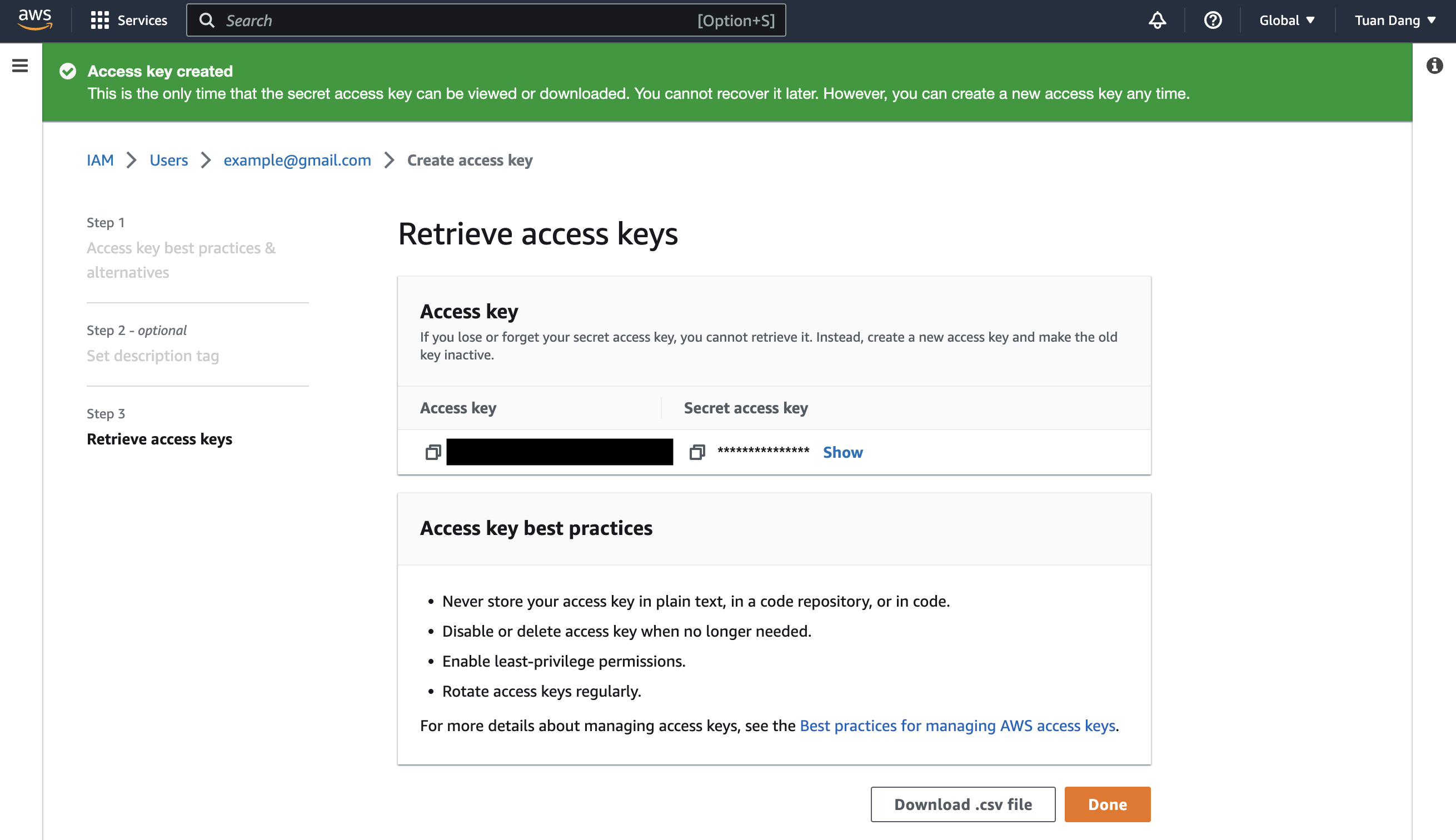
1. Set the access key as **CLIENT\_ID\_AWS\_INTEGRATION**.
2. Set the secret key as **CLIENT\_SECRET\_AWS\_INTEGRATION**.
1. Navigate to the [Create IAM Role](https://console.aws.amazon.com/iamv2/home#/roles/create?step=selectEntities) page in your AWS Console.
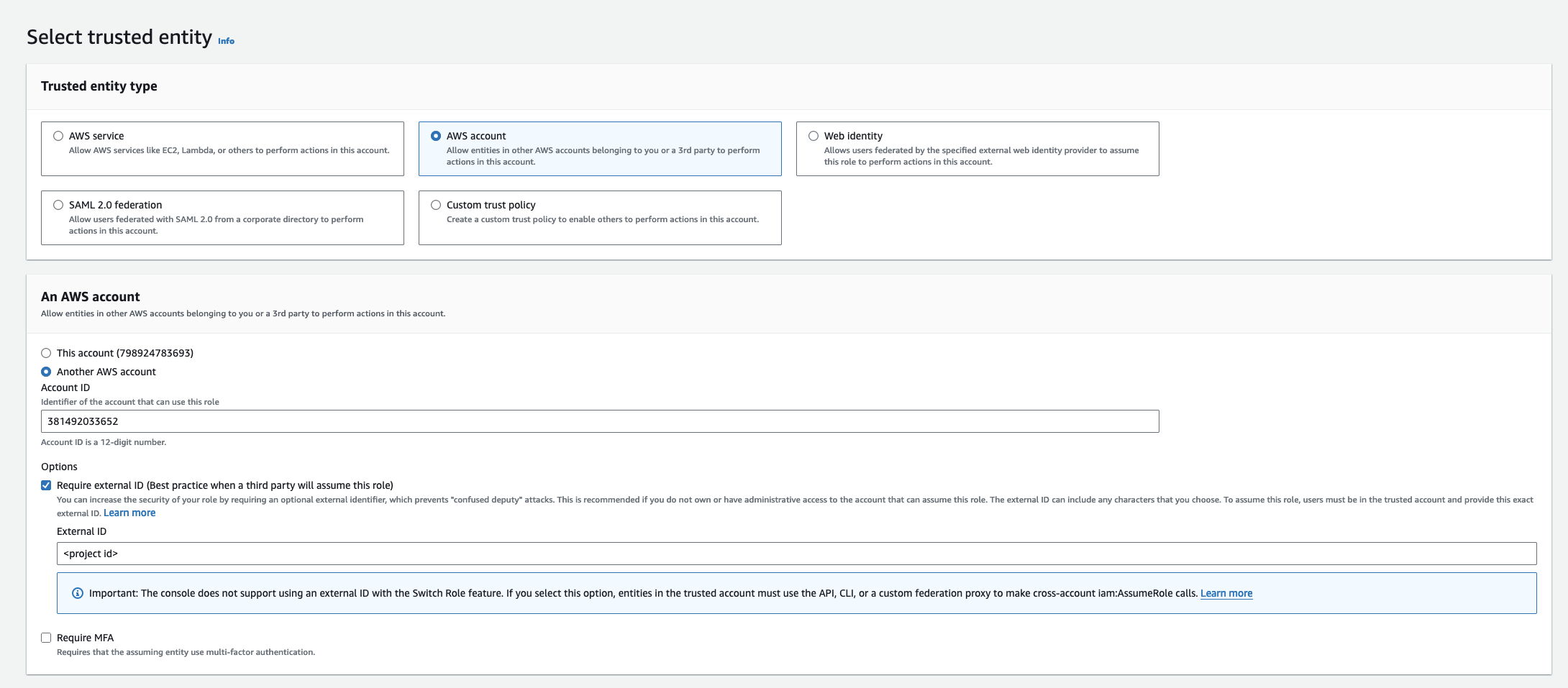
2. Select **AWS Account** as the **Trusted Entity Type**.
3. Choose **Another AWS Account** and enter **381492033652** (Infisical AWS Account ID). This restricts the role to be assumed only by Infisical. If self-hosting, provide your AWS account number instead.
4. Optionally, enable **Require external ID** and enter your **project ID** to further enhance security.
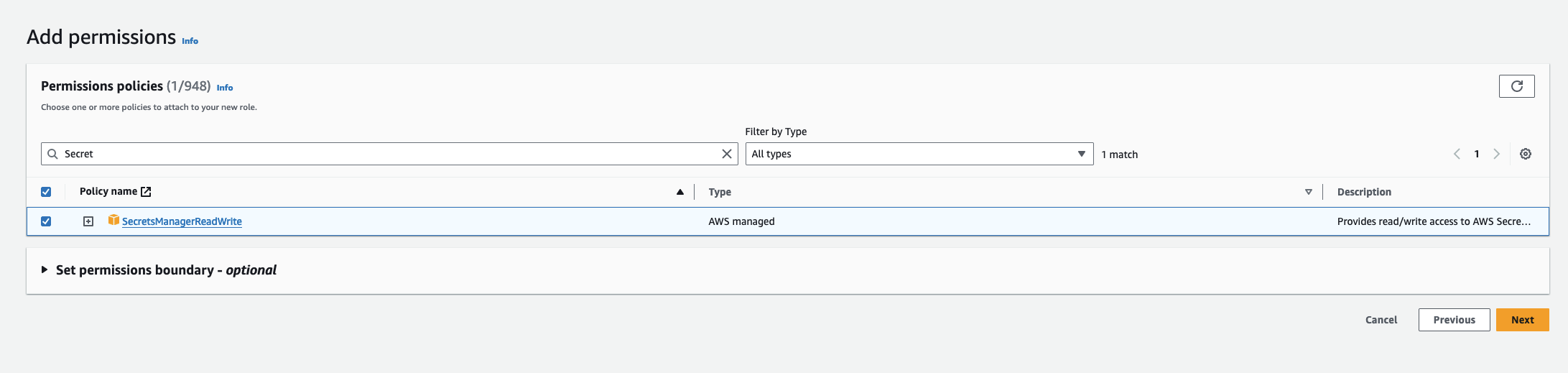
Use the following custom policy to grant the minimum permissions required by Infisical to sync secrets to AWS Parameter Store:
```json
{
"Version": "2012-10-17",
"Statement": [
{
"Sid": "AllowSSMAccess",
"Effect": "Allow",
"Action": [
"ssm:PutParameter",
"ssm:DeleteParameter",
"ssm:GetParameters",
"ssm:GetParametersByPath",
"ssm:DescribeParameters",
"ssm:DeleteParameters",
"ssm:AddTagsToResource", // if you need to add tags to secrets
"kms:ListKeys", // if you need to specify the KMS key
"kms:ListAliases", // if you need to specify the KMS key
"kms:Encrypt", // if you need to specify the KMS key
"kms:Decrypt" // if you need to specify the KMS key
],
"Resource": "*"
}
]
}
```
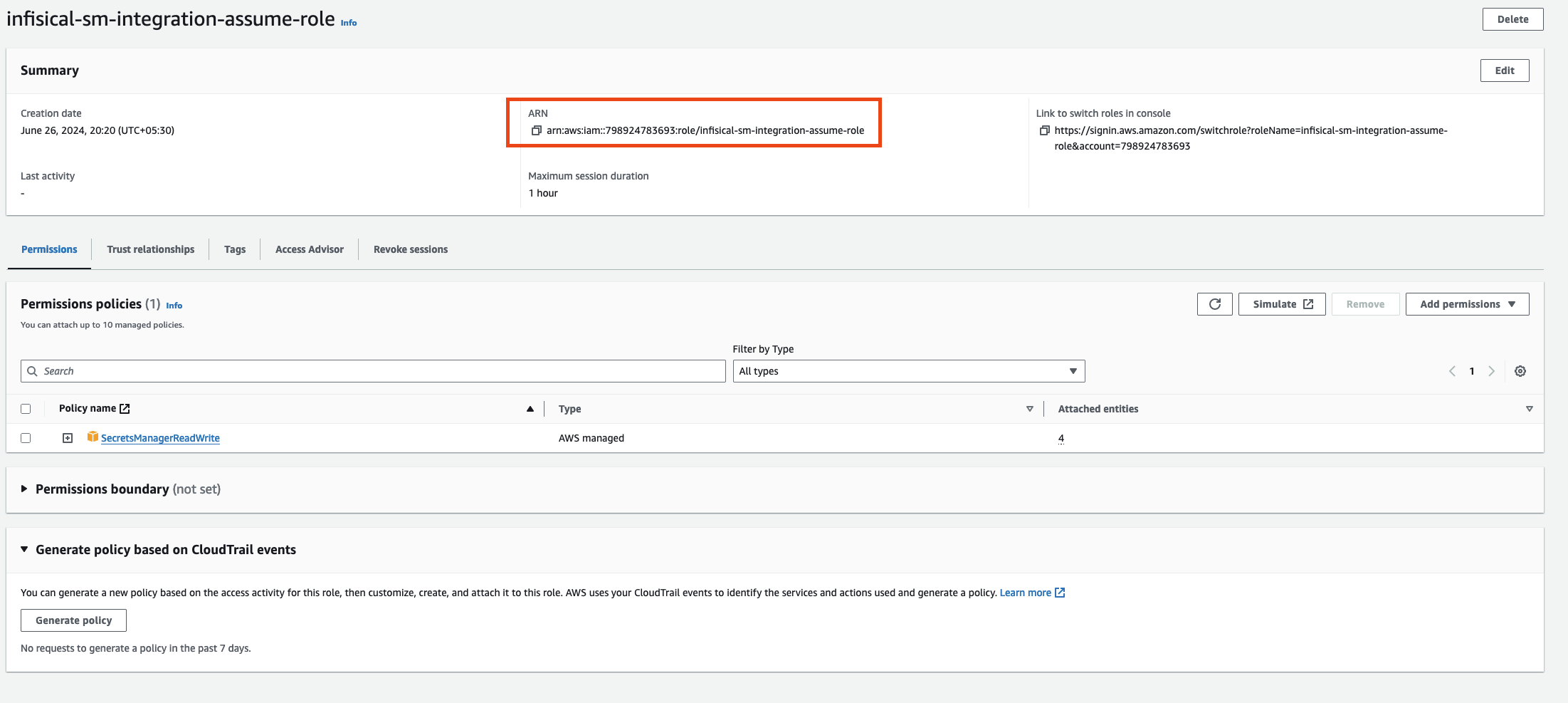
1. Navigate to your project's integrations tab in Infisical.
2. Click on the **AWS Parameter Store** tile.
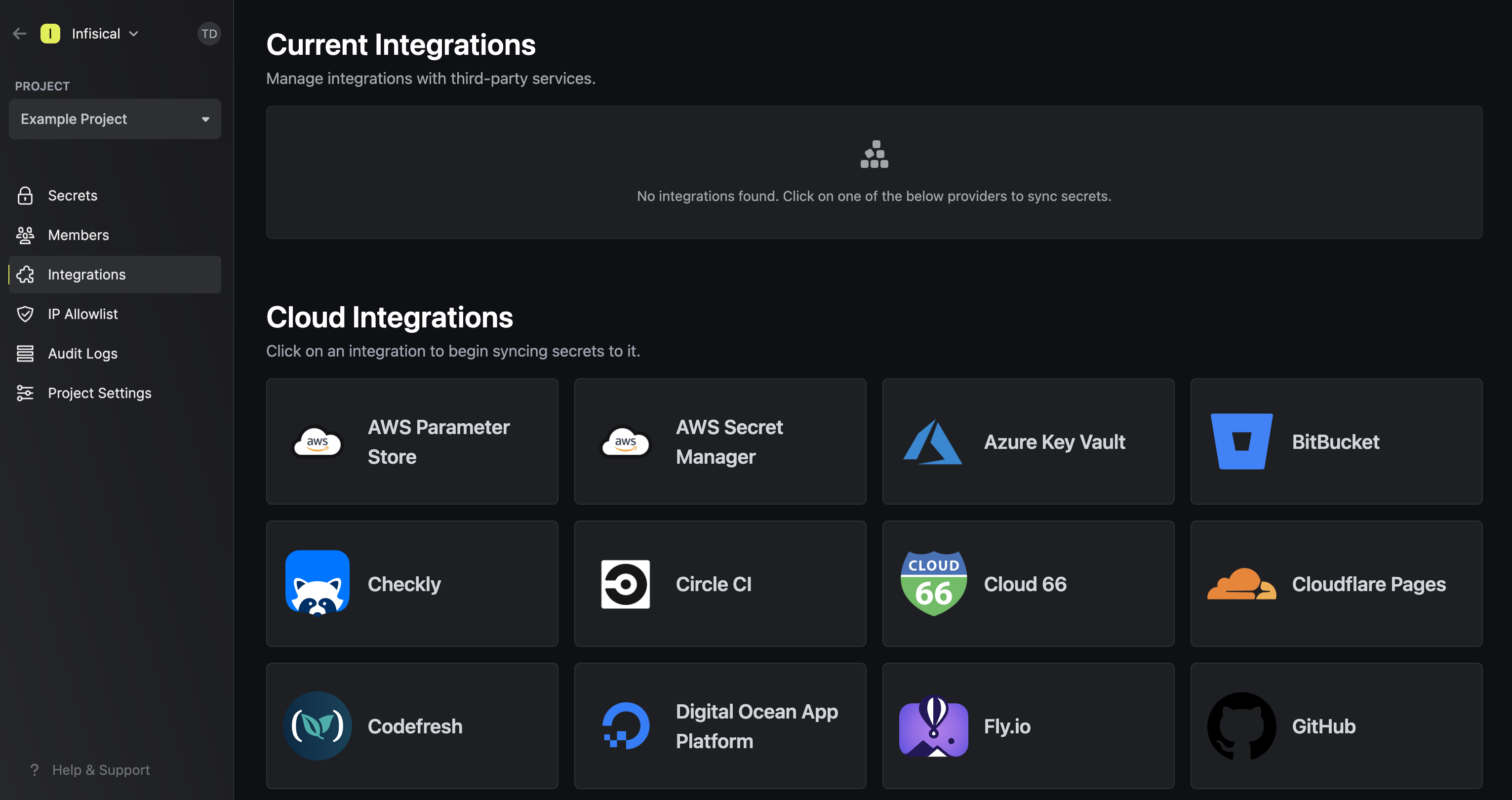
3. Select the **AWS Assume Role** option.
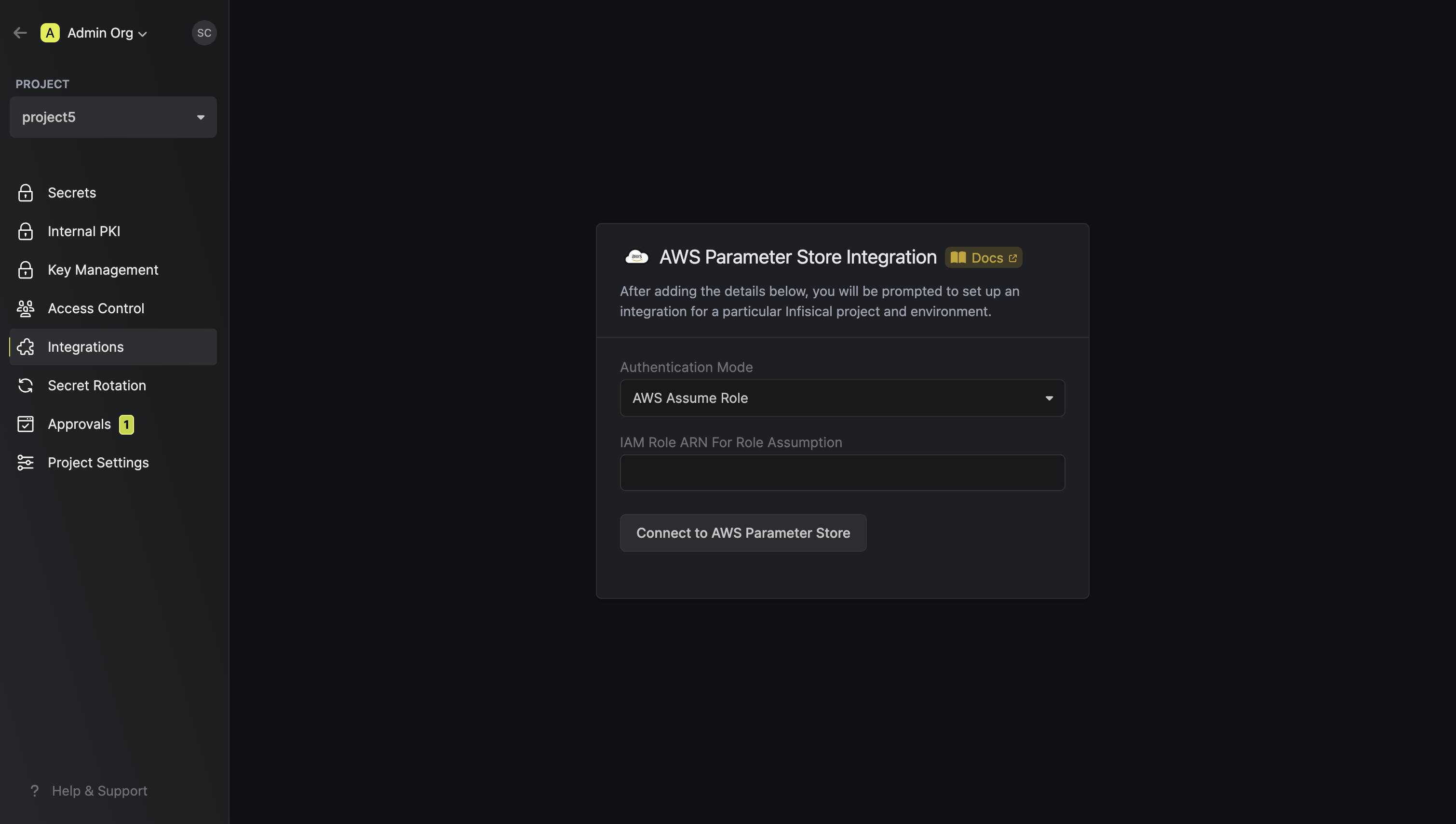
4. Provide the **AWS IAM Role ARN** obtained from the previous step and press connect.
Select which Infisical environment secrets you want to sync to which AWS Parameter Store region and indicate the path for your secrets. Then, press create integration to start syncing secrets to AWS Parameter Store.
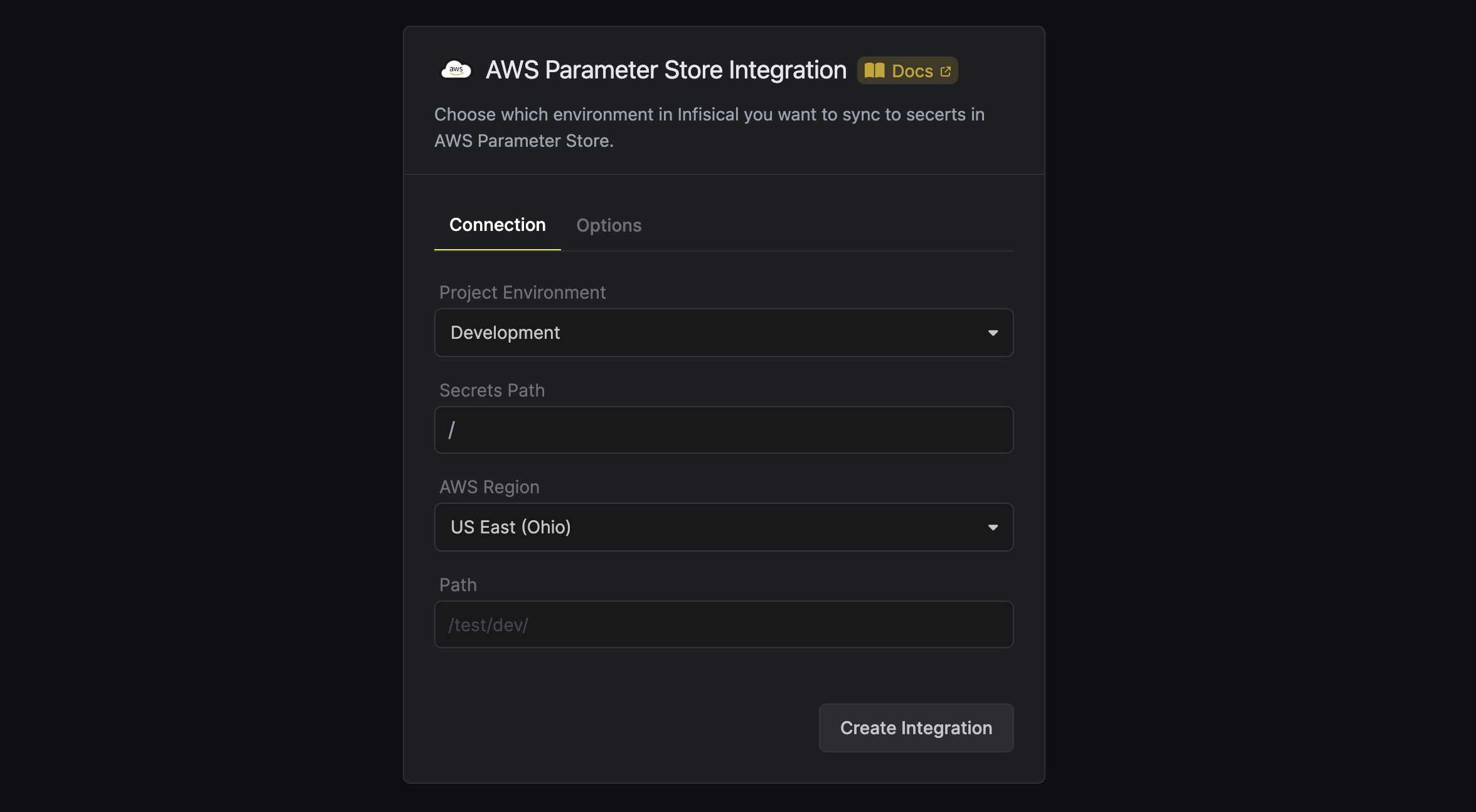
Infisical requires you to add a path for your secrets to be stored in AWS
Parameter Store and recommends setting the path structure to
`/[project_name]/[environment]/` according to best practices. This enables a
secret like `TEST` to be stored as `/[project_name]/[environment]/TEST` in AWS
Parameter Store.
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
Navigate to your IAM user permissions and add a permission policy to grant access to AWS Parameter Store.
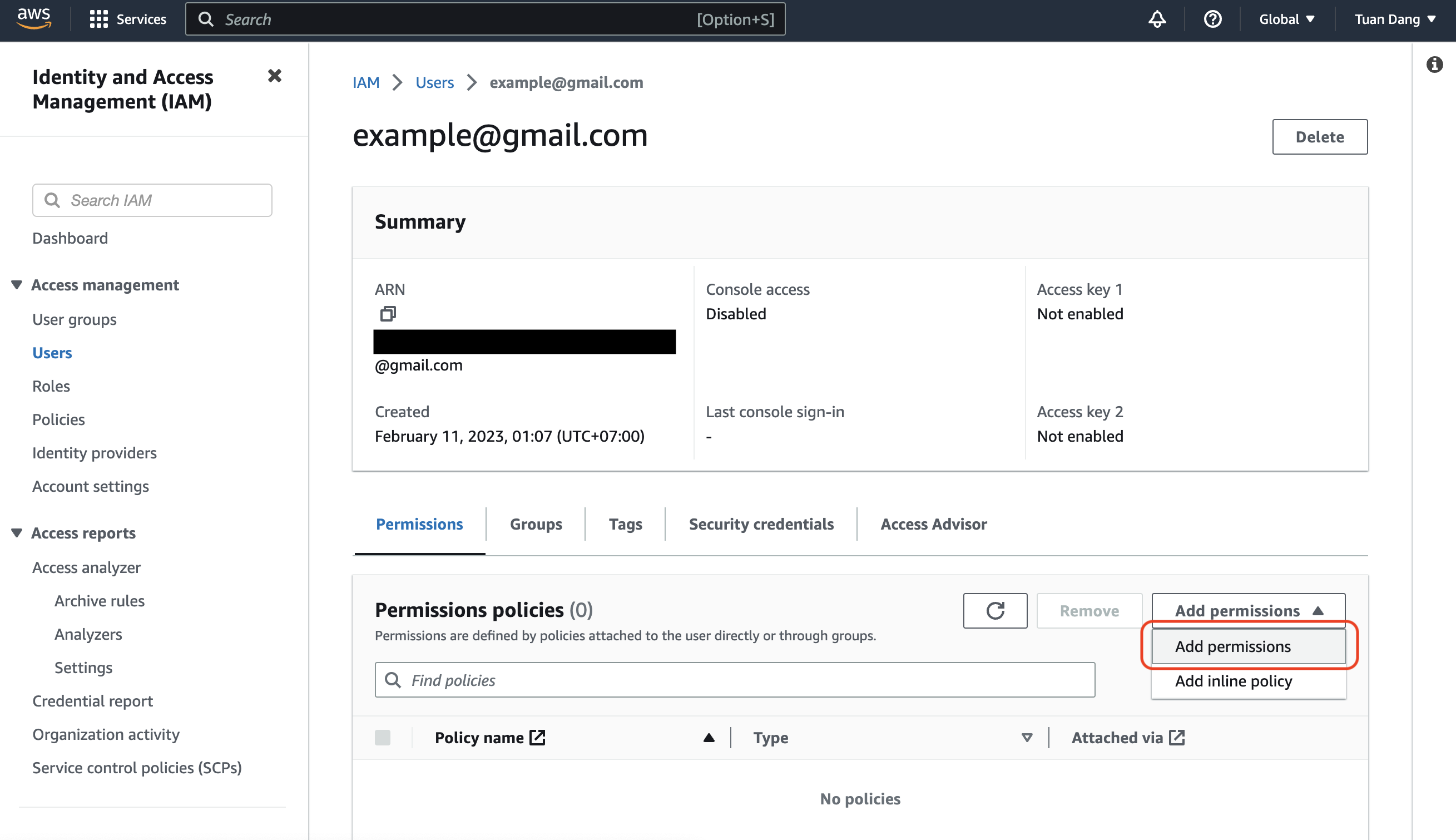
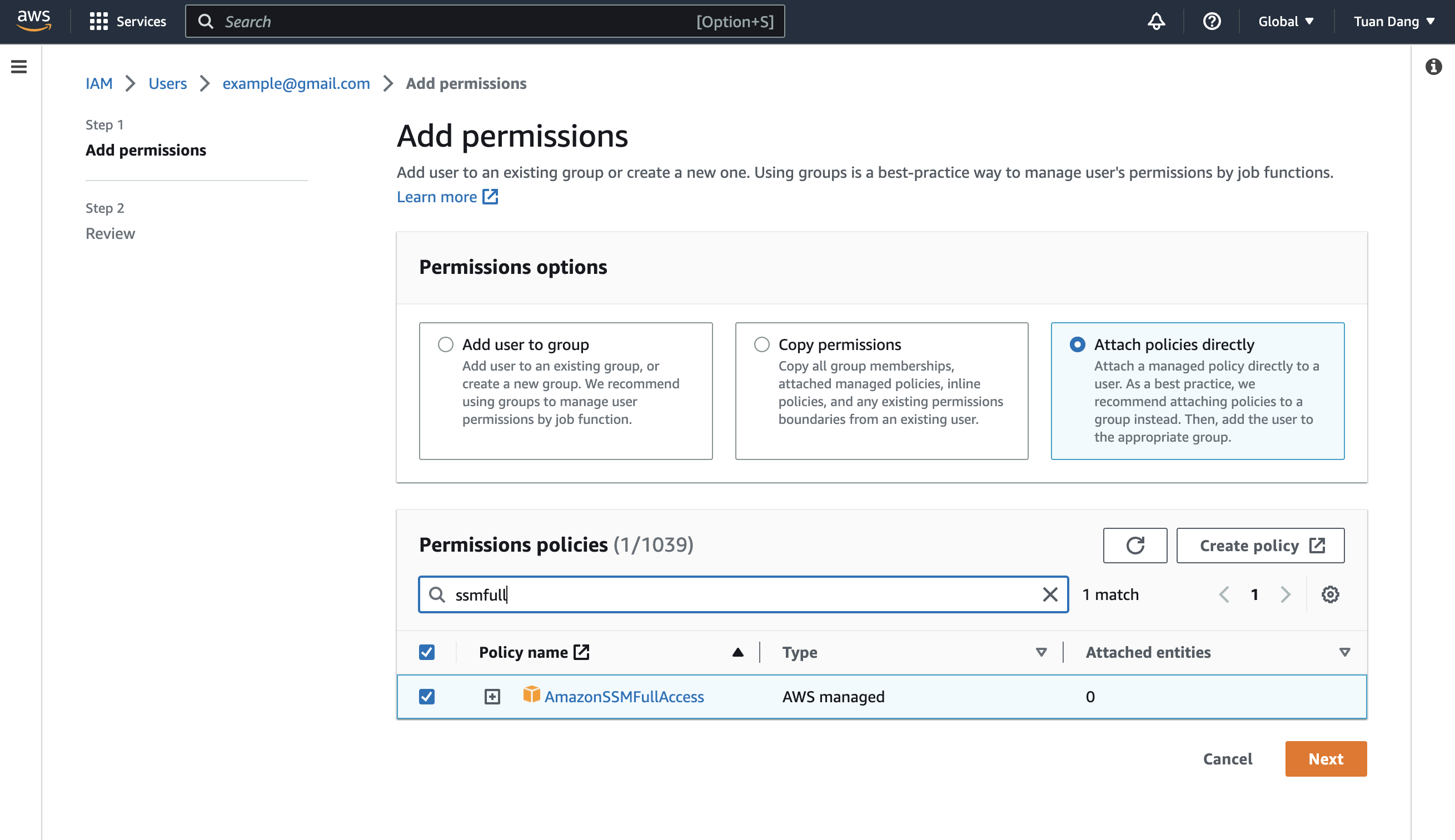
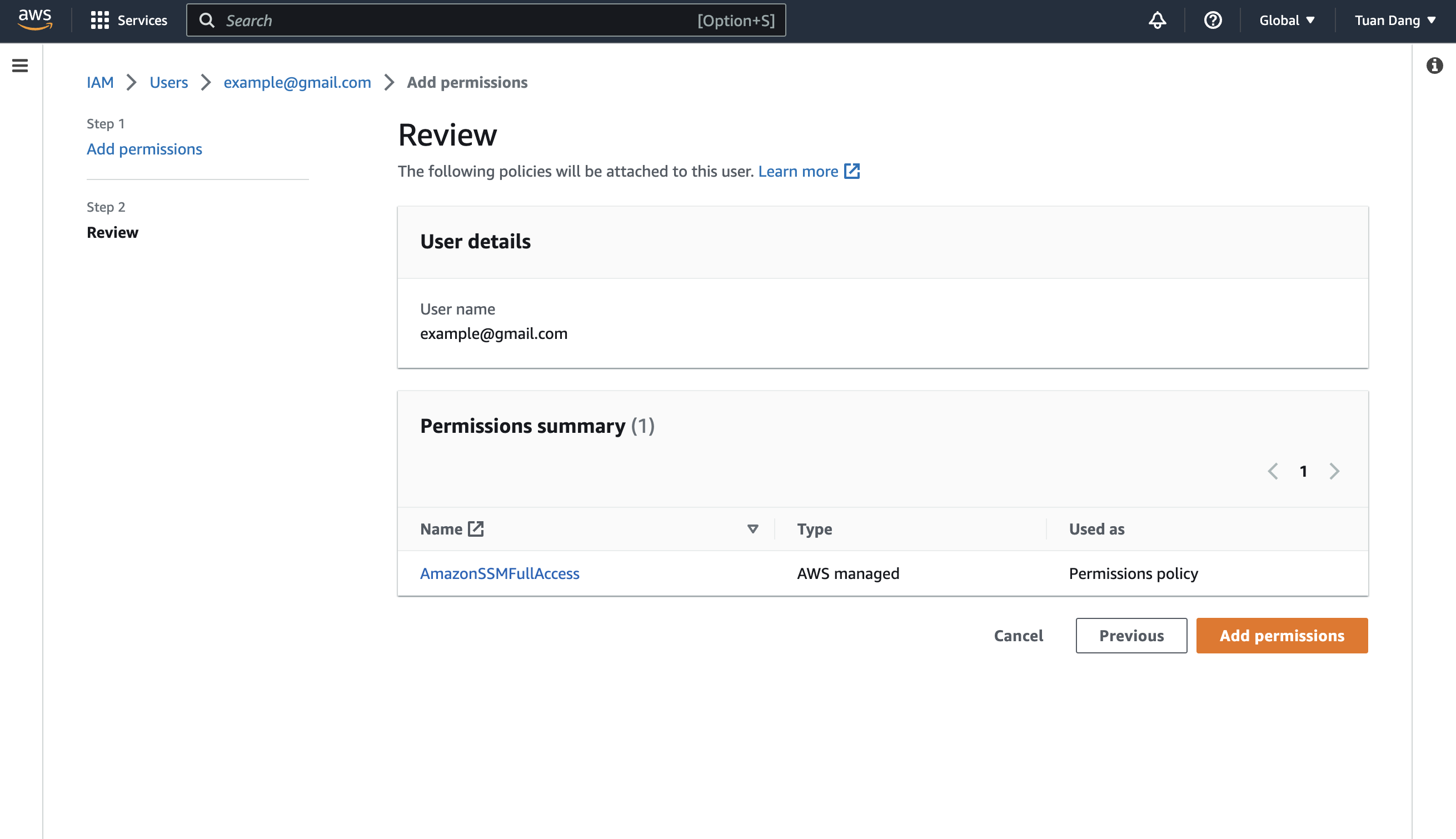
For enhanced security, here's a custom policy containing the minimum permissions required by Infisical to sync secrets to AWS Parameter Store for the IAM user that you can use:
```json
{
"Version": "2012-10-17",
"Statement": [
{
"Sid": "AllowSSMAccess",
"Effect": "Allow",
"Action": [
"ssm:PutParameter",
"ssm:DeleteParameter",
"ssm:GetParameters",
"ssm:GetParametersByPath",
"ssm:DescribeParameters",
"ssm:DeleteParameters",
"ssm:AddTagsToResource", // if you need to add tags to secrets
"kms:ListKeys", // if you need to specify the KMS key
"kms:ListAliases", // if you need to specify the KMS key
"kms:Encrypt", // if you need to specify the KMS key
"kms:Decrypt" // if you need to specify the KMS key
],
"Resource": "*"
}
]
}
```
Obtain a AWS access key ID and secret access key for your IAM user in IAM > Users > User > Security credentials > Access keys
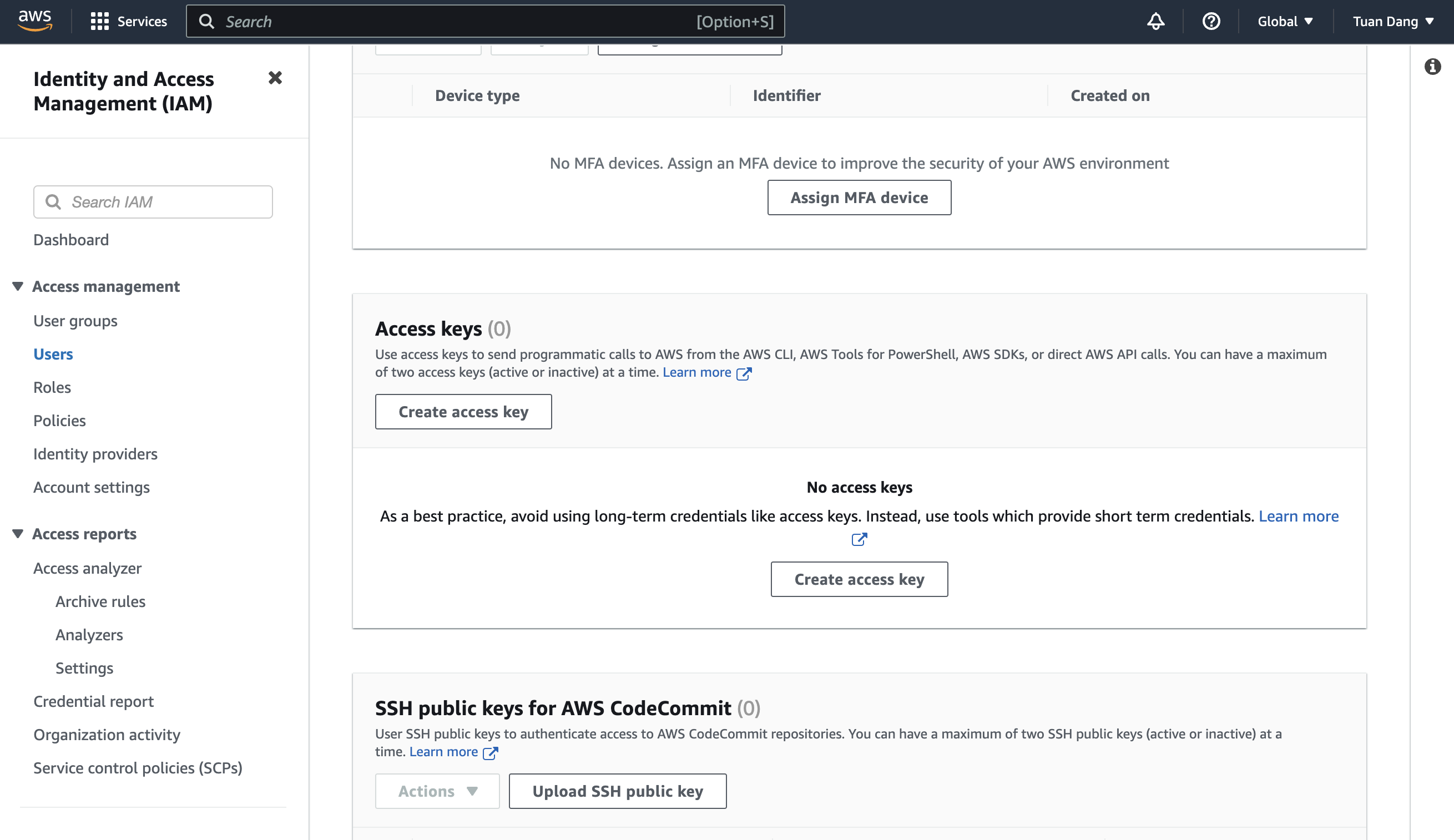
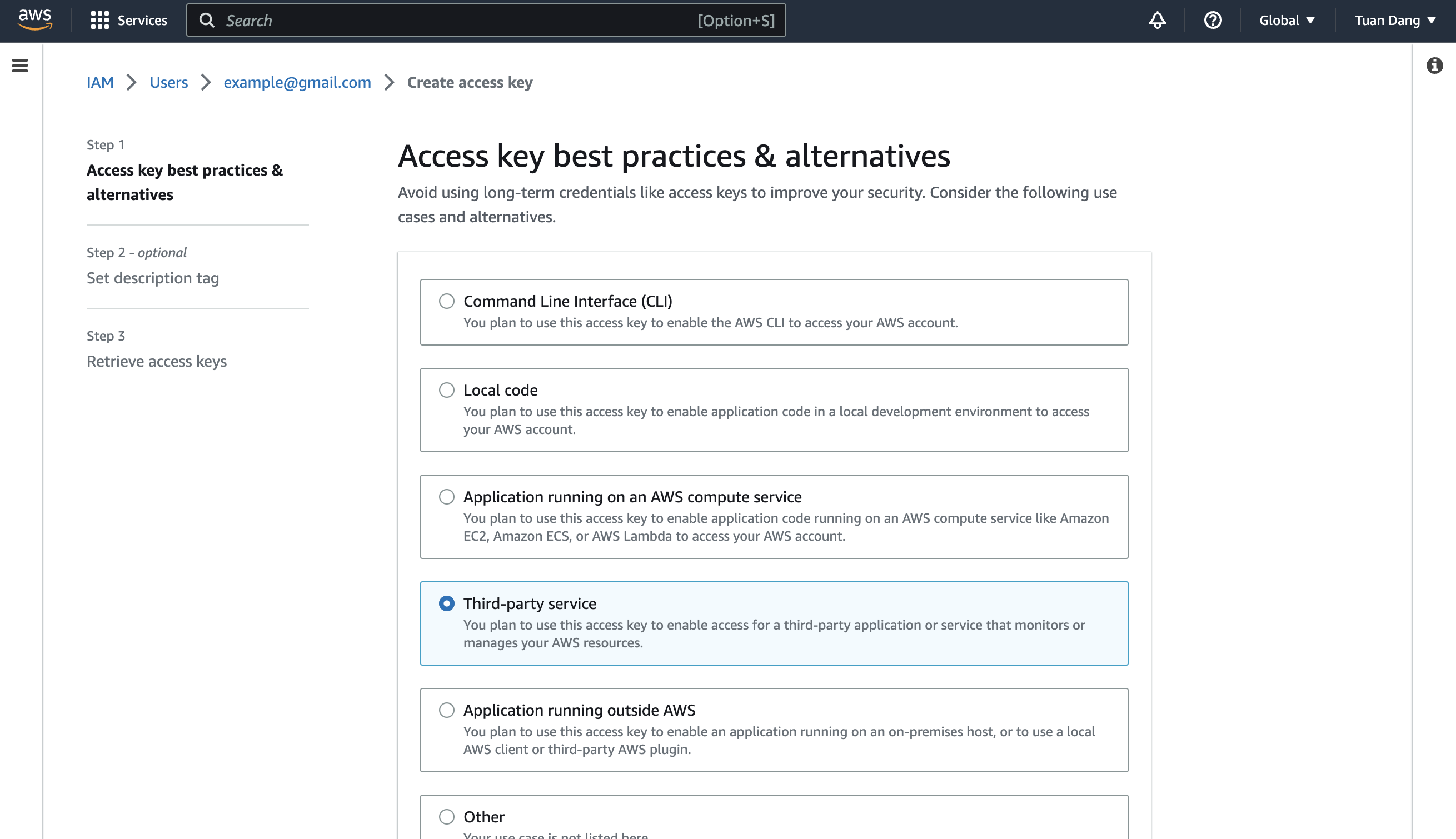
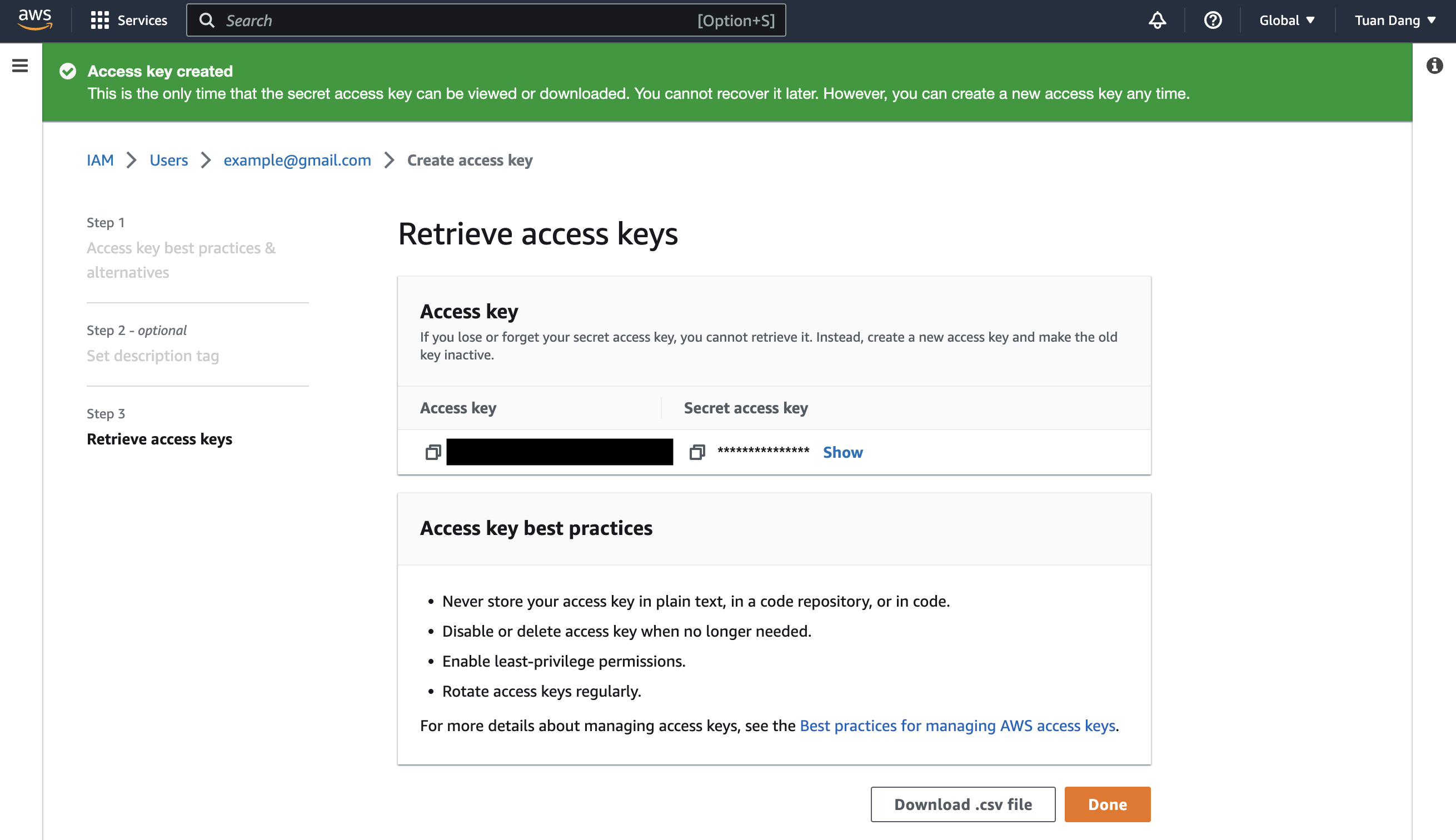
Navigate to your project's integrations tab in Infisical.
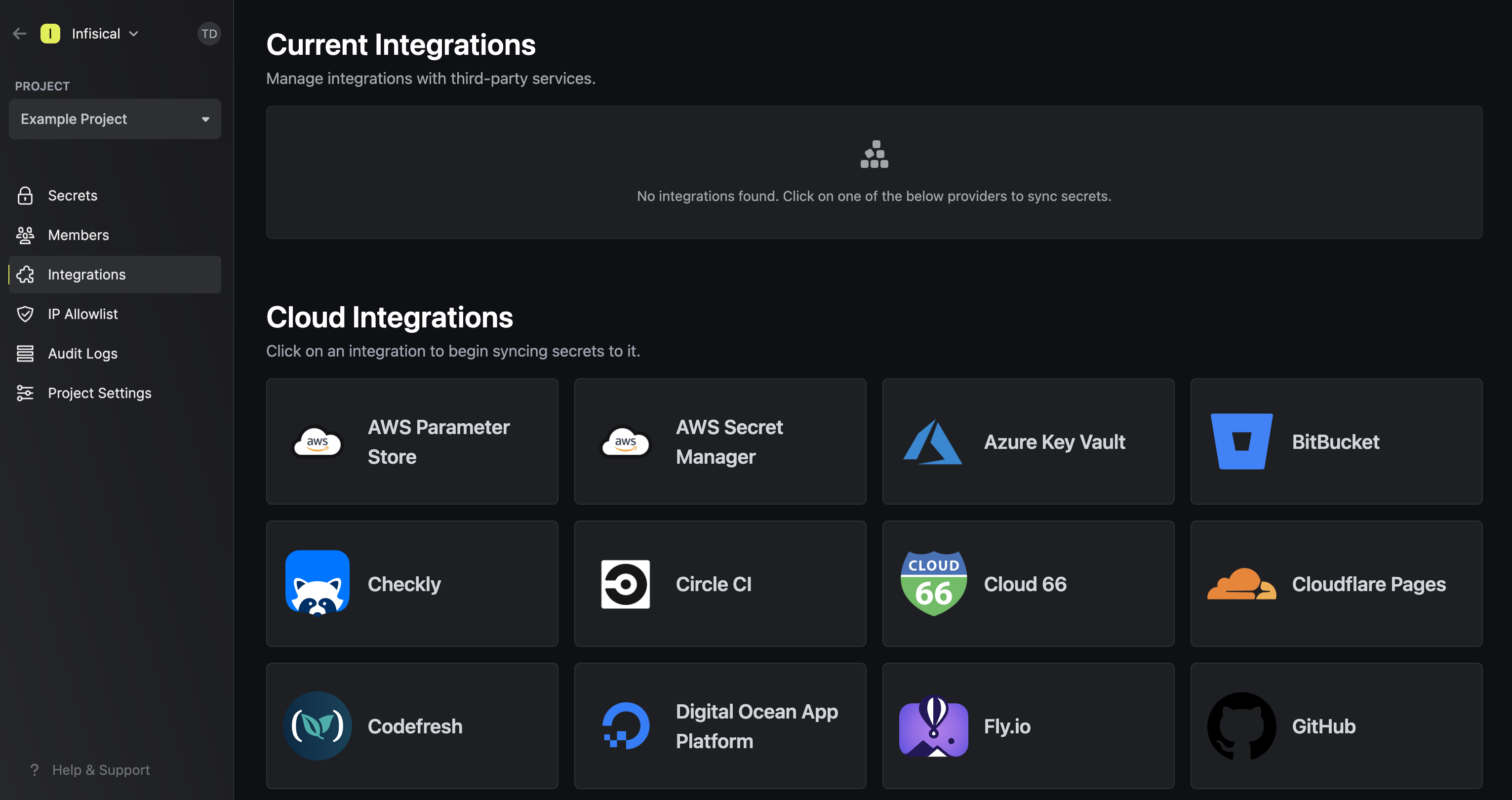
Press on the AWS Parameter Store tile and select Access Key as the authentication mode. Input your AWS access key ID and secret access key from the previous step.
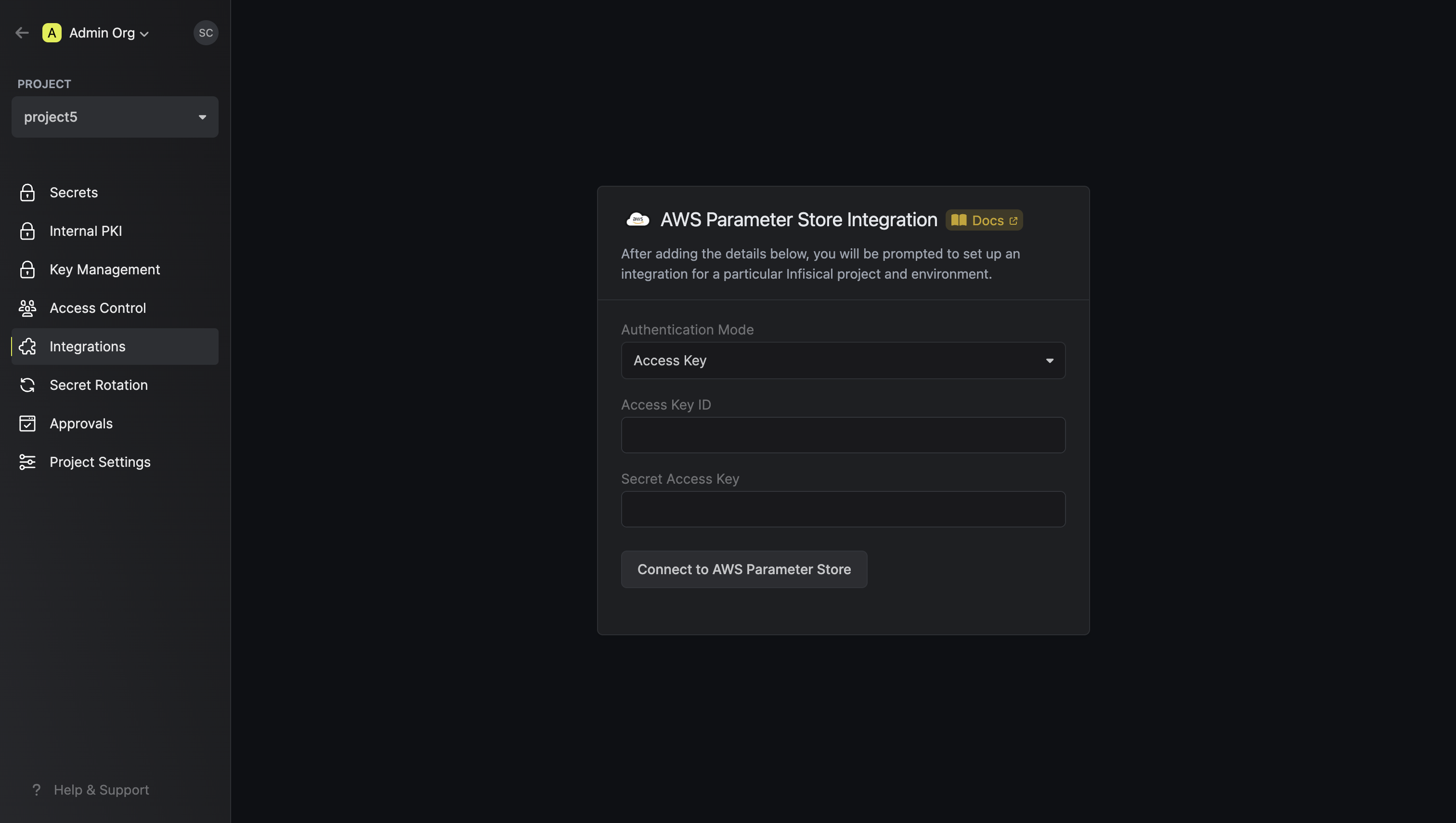
Select which Infisical environment secrets you want to sync to which AWS Parameter Store region and indicate the path for your secrets. Then, press create integration to start syncing secrets to AWS Parameter Store.
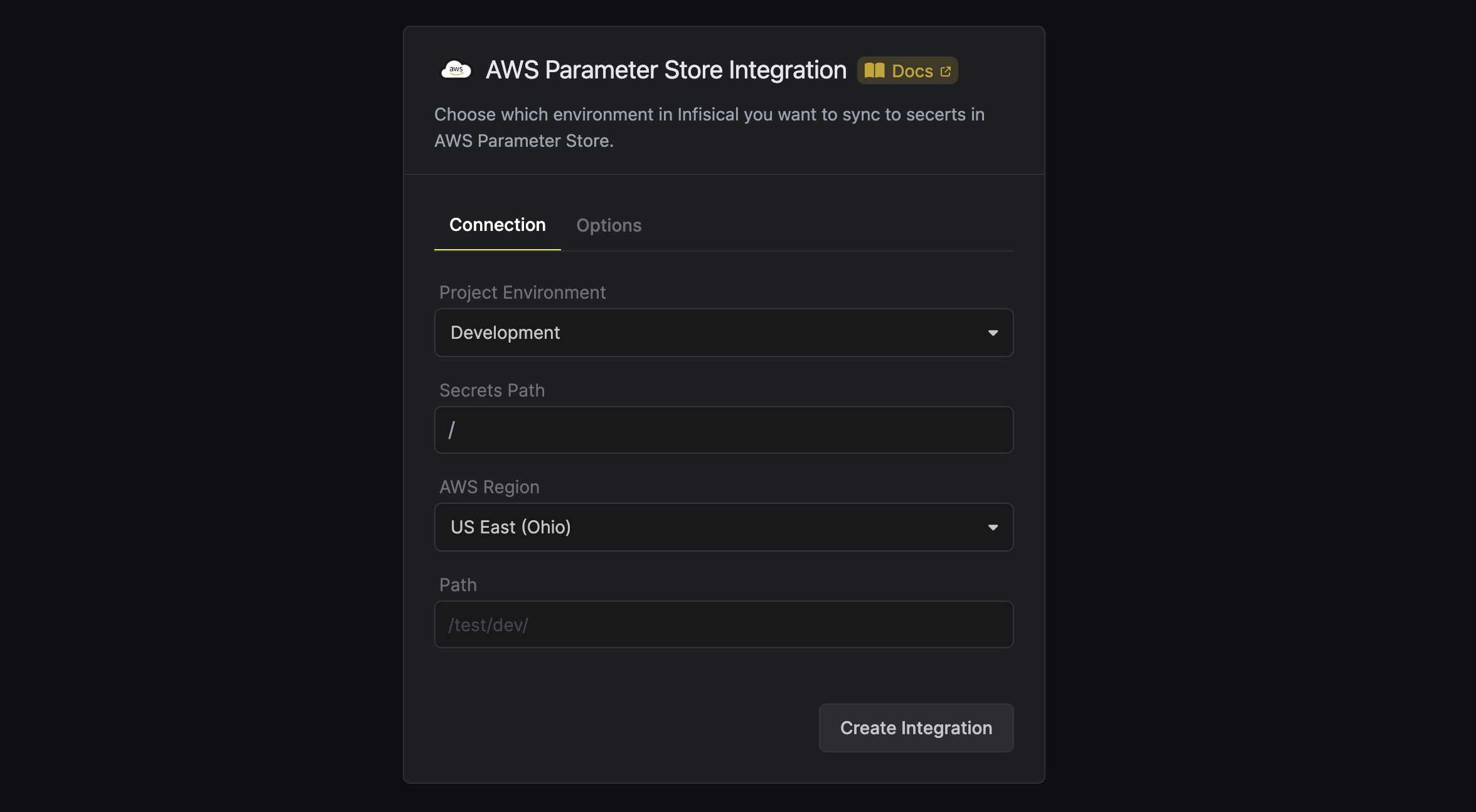
Infisical requires you to add a path for your secrets to be stored in AWS
Parameter Store and recommends setting the path structure to
`/[project_name]/[environment]/` according to best practices. This enables a
secret like `TEST` to be stored as `/[project_name]/[environment]/TEST` in AWS
Parameter Store.
# AWS Secrets Manager
Learn how to sync secrets from Infisical to AWS Secrets Manager.
Infisical will assume the provided role in your AWS account securely, without the need to share any credentials.
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
To connect your Infisical instance with AWS, you need to set up an AWS IAM User account that can assume the AWS IAM Role for the integration.
If your instance is deployed on AWS, the aws-sdk will automatically retrieve the credentials. Ensure that you assign the provided permission policy to your deployed instance, such as ECS or EC2.
The following steps are for instances not deployed on AWS
Navigate to [Create IAM User](https://console.aws.amazon.com/iamv2/home#/users/create) in your AWS Console.
Attach the following inline permission policy to the IAM User to allow it to assume any IAM Roles:
```json
{
"Version": "2012-10-17",
"Statement": [
{
"Sid": "AllowAssumeAnyRole",
"Effect": "Allow",
"Action": "sts:AssumeRole",
"Resource": "arn:aws:iam::*:role/*"
}
]
}
```
Obtain the AWS access key ID and secret access key for your IAM User by navigating to IAM > Users > \[Your User] > Security credentials > Access keys.
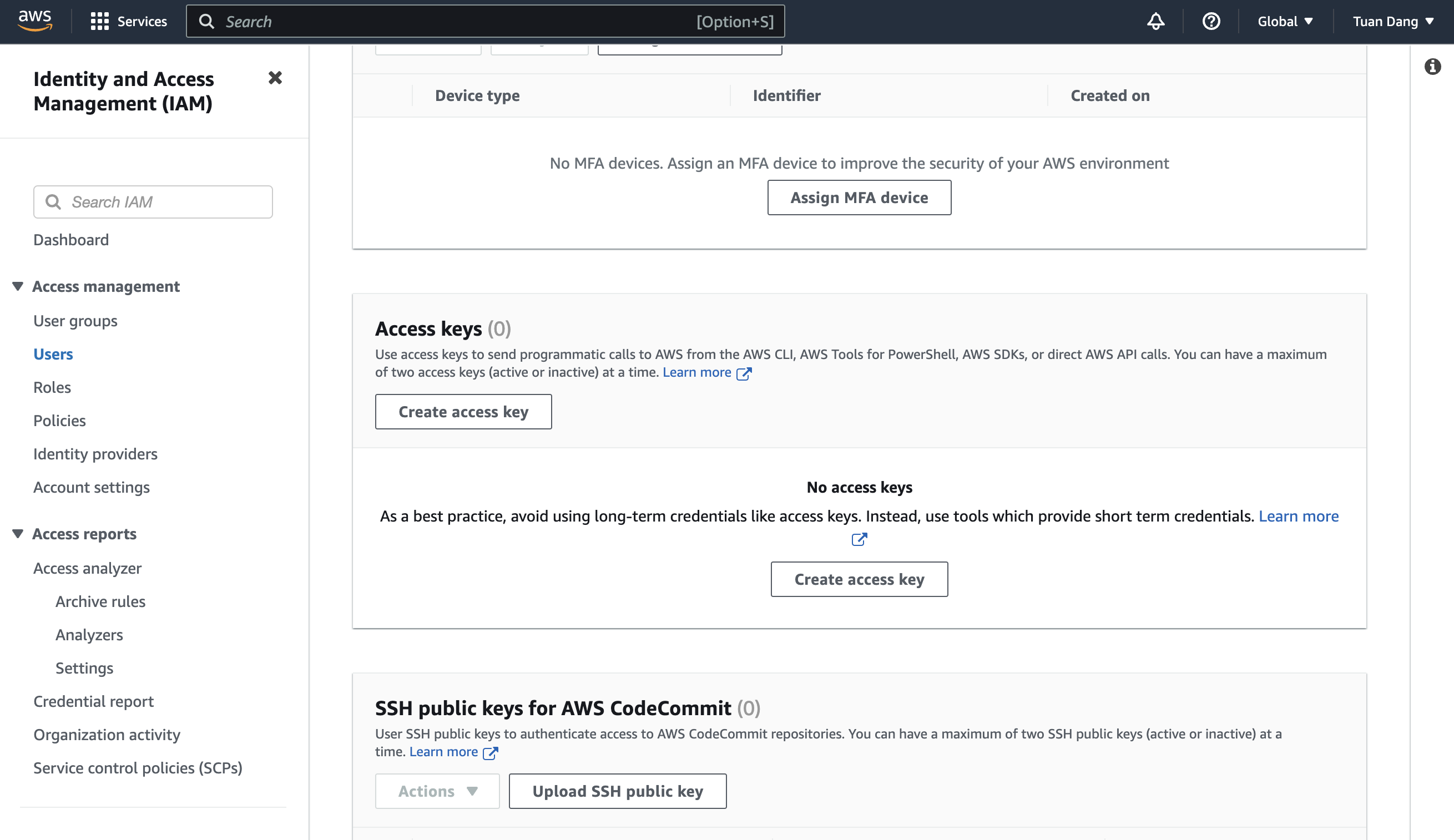
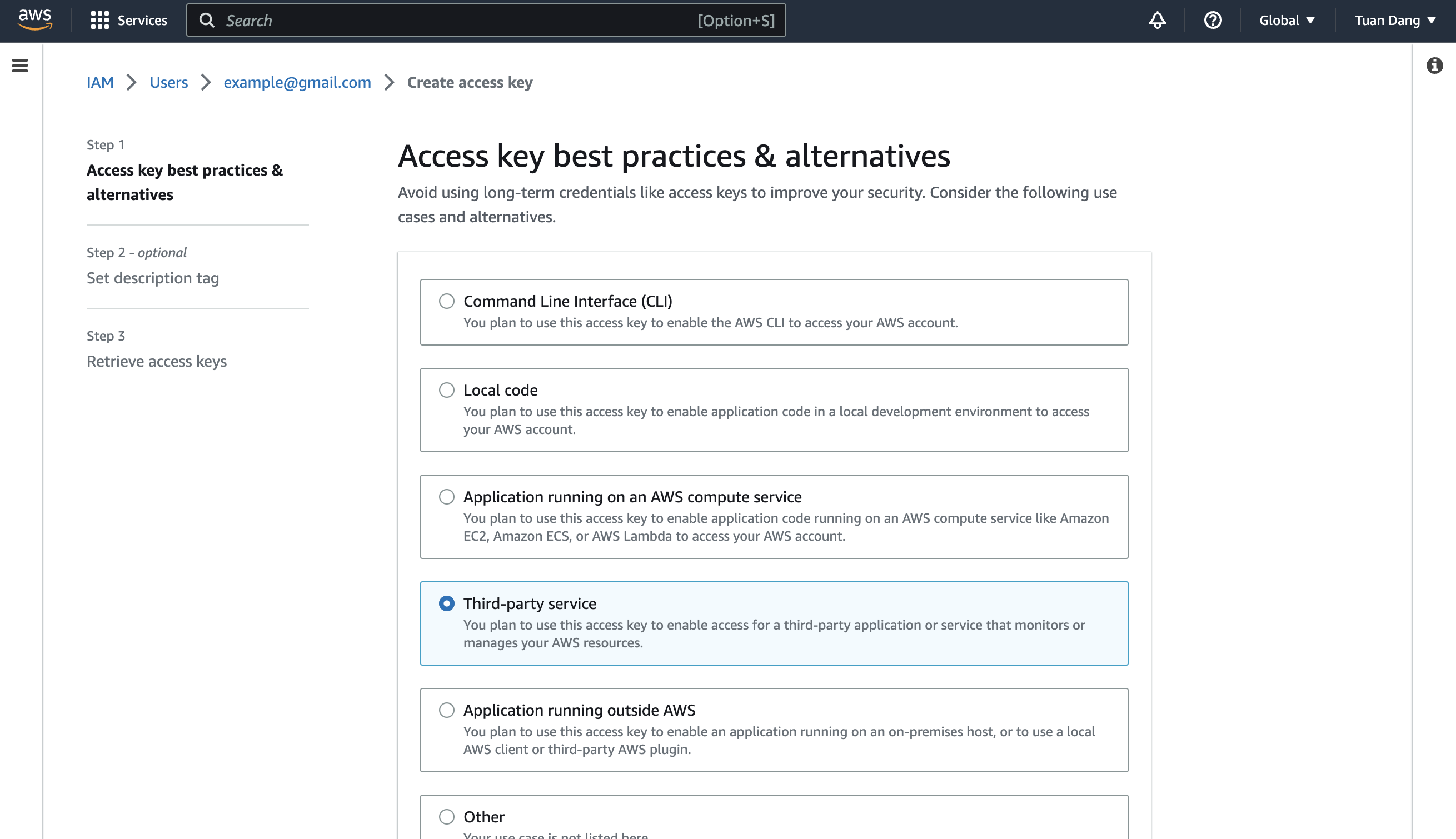
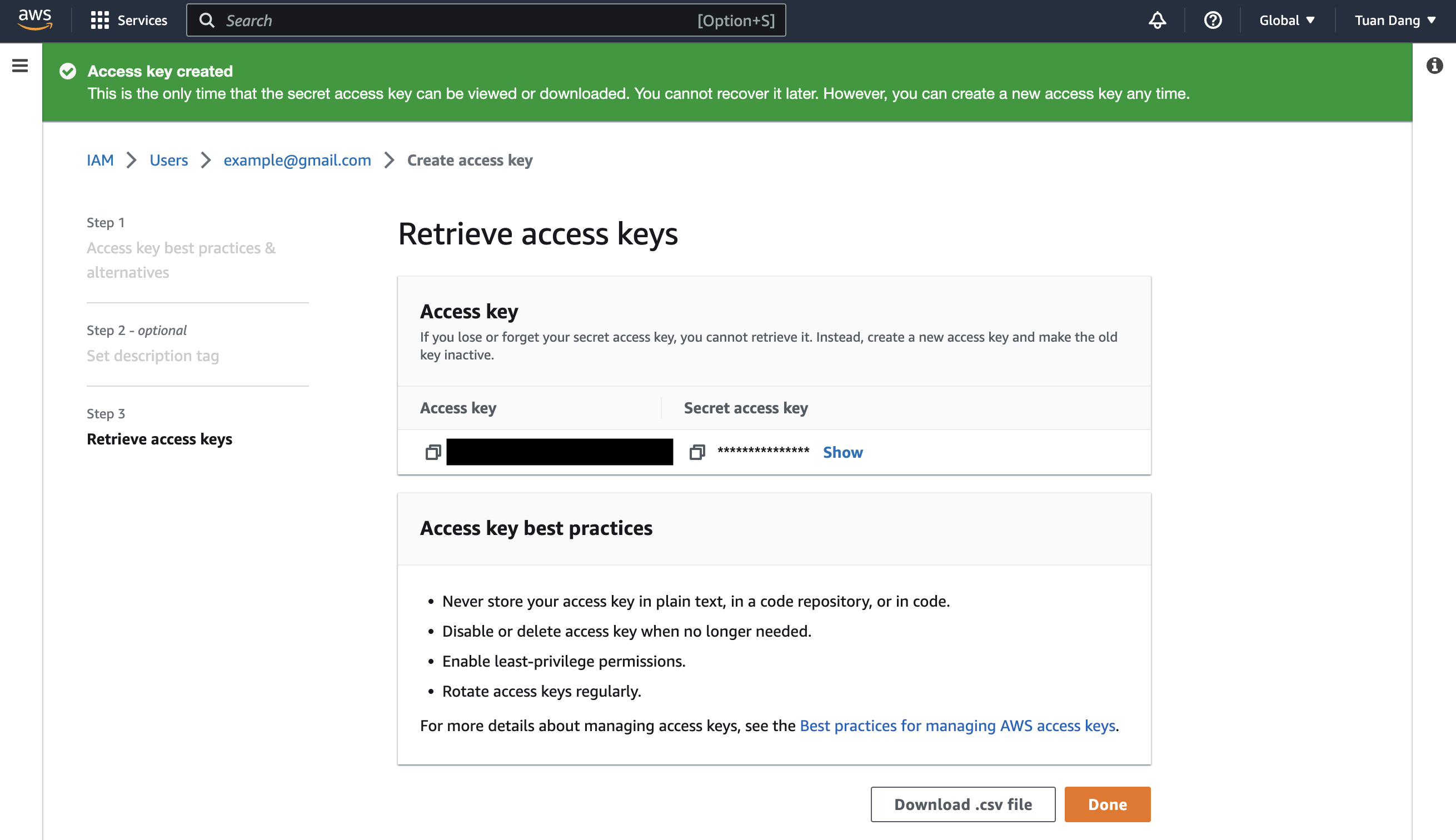
1. Set the access key as **CLIENT\_ID\_AWS\_INTEGRATION**.
2. Set the secret key as **CLIENT\_SECRET\_AWS\_INTEGRATION**.
1. Navigate to the [Create IAM Role](https://console.aws.amazon.com/iamv2/home#/roles/create?step=selectEntities) page in your AWS Console.
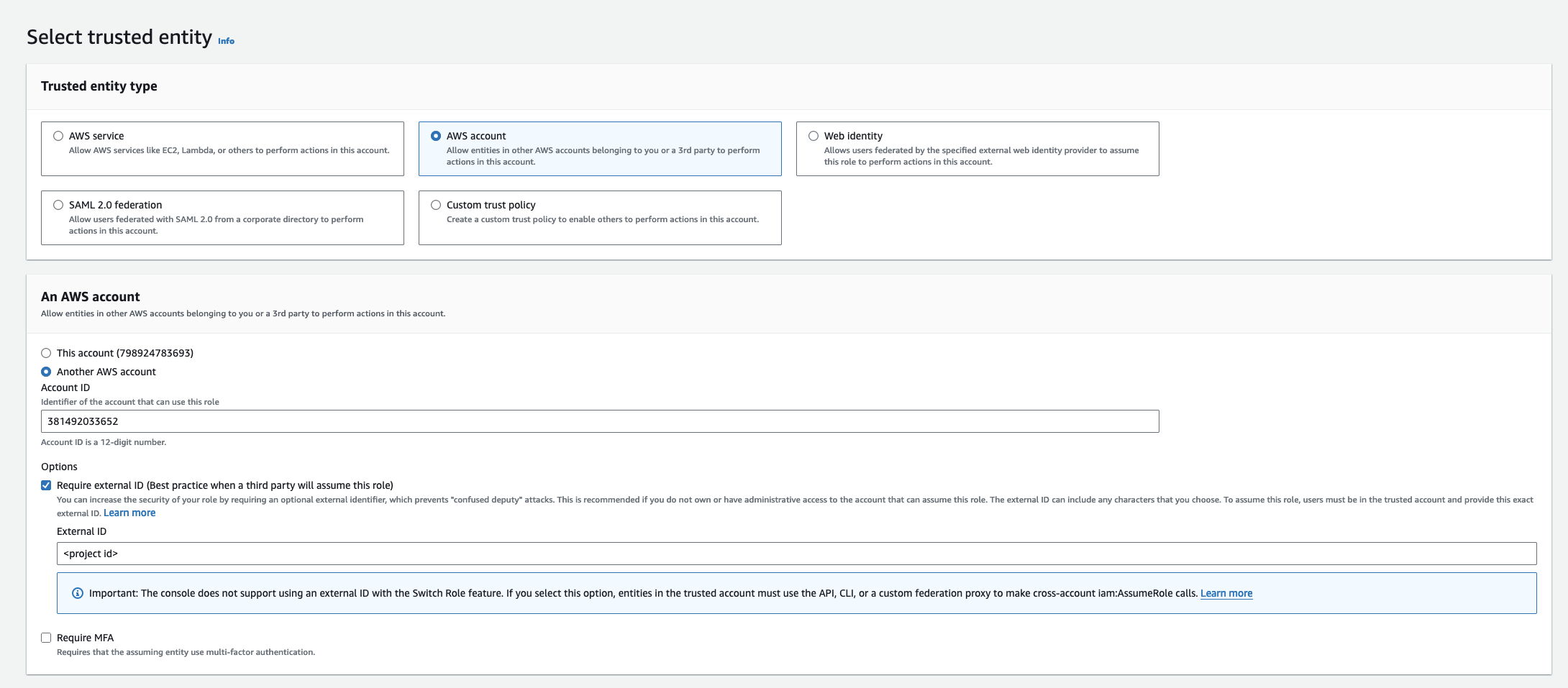
2. Select **AWS Account** as the **Trusted Entity Type**.
3. Choose **Another AWS Account** and enter **381492033652** (Infisical AWS Account ID). This restricts the role to be assumed only by Infisical. If self-hosting, provide your AWS account number instead.
4. Optionally, enable **Require external ID** and enter your **project ID** to further enhance security.
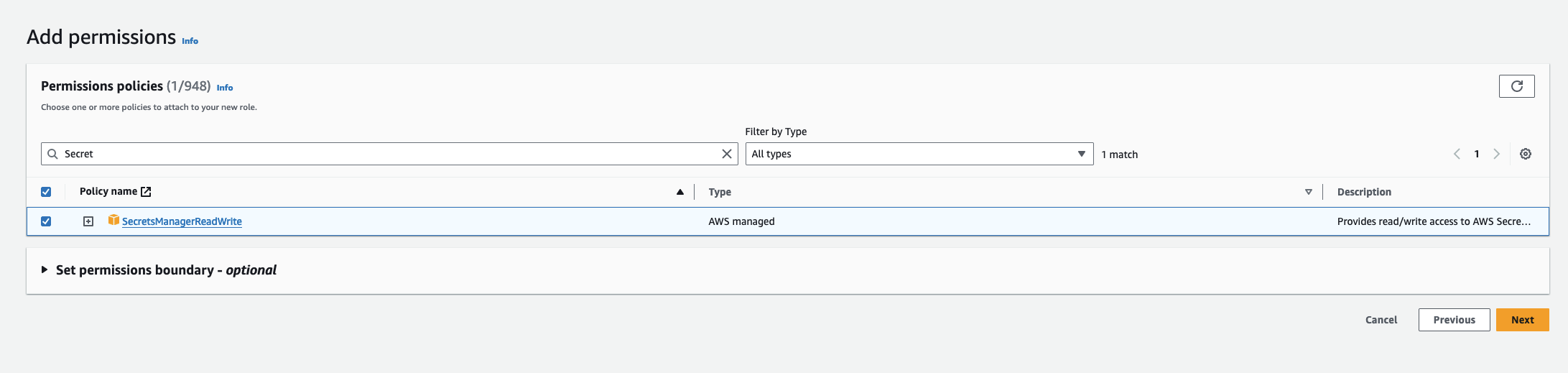
Use the following custom policy to grant the minimum permissions required by Infisical to sync secrets to AWS Secrets Manager:
```json
{
"Version": "2012-10-17",
"Statement": [
{
"Sid": "AllowSecretsManagerAccess",
"Effect": "Allow",
"Action": [
"secretsmanager:GetSecretValue",
"secretsmanager:CreateSecret",
"secretsmanager:UpdateSecret",
"secretsmanager:DescribeSecret",
"secretsmanager:TagResource",
"secretsmanager:UntagResource",
"kms:ListKeys",
"kms:ListAliases",
"kms:Encrypt",
"kms:Decrypt"
],
"Resource": "*"
}
]
}
```
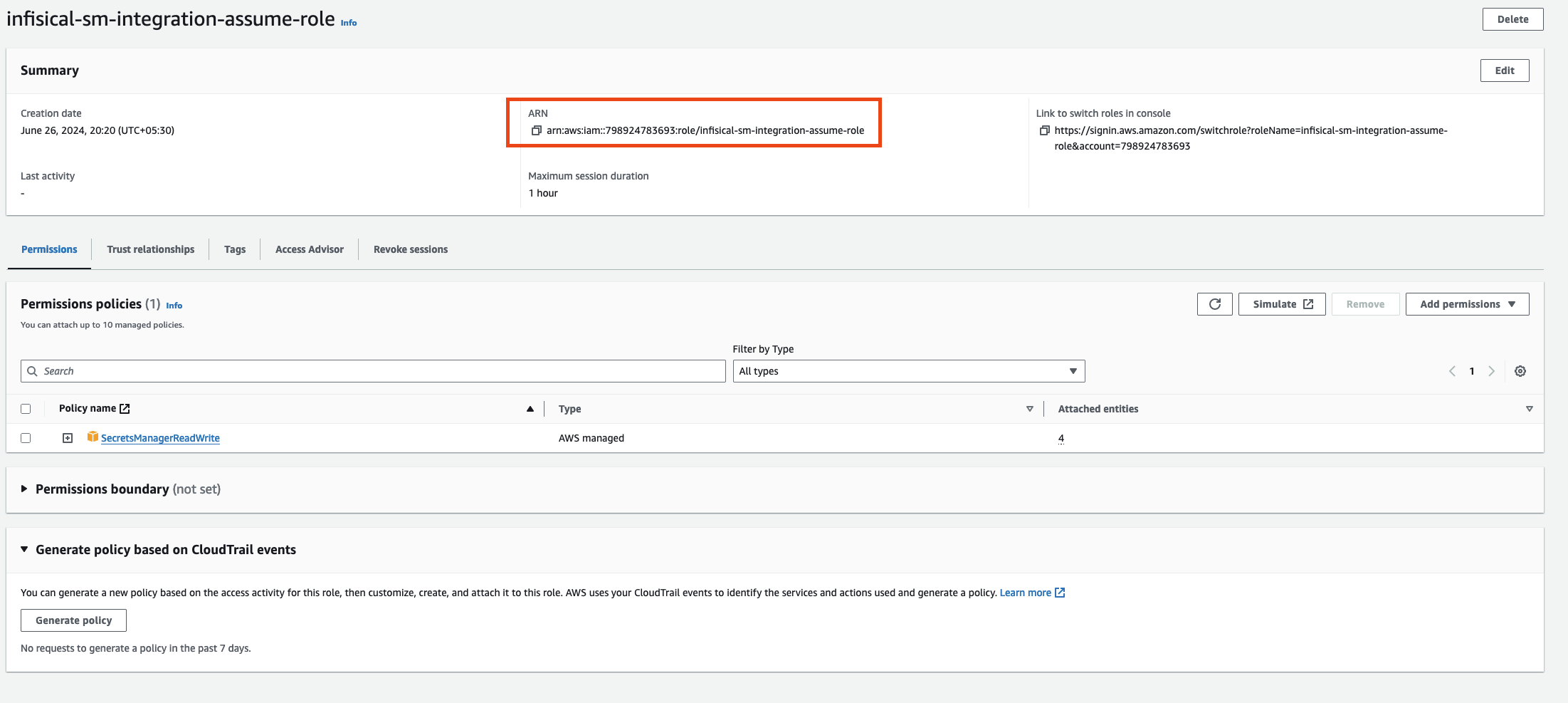
1. Navigate to your project's integrations tab in Infisical.
2. Click on the **AWS Secrets Manager** tile.
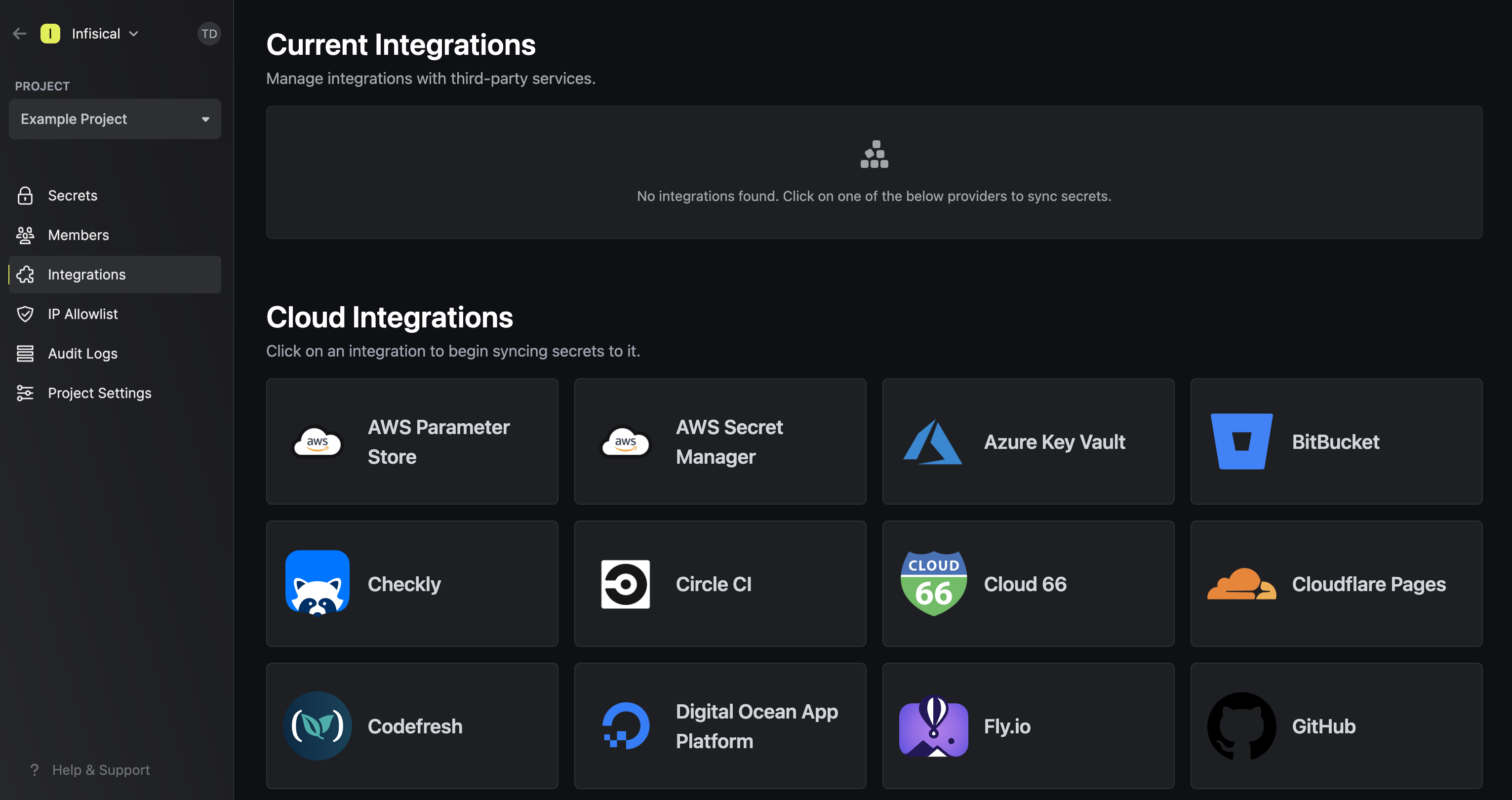
3. Select the **AWS Assume Role** option.
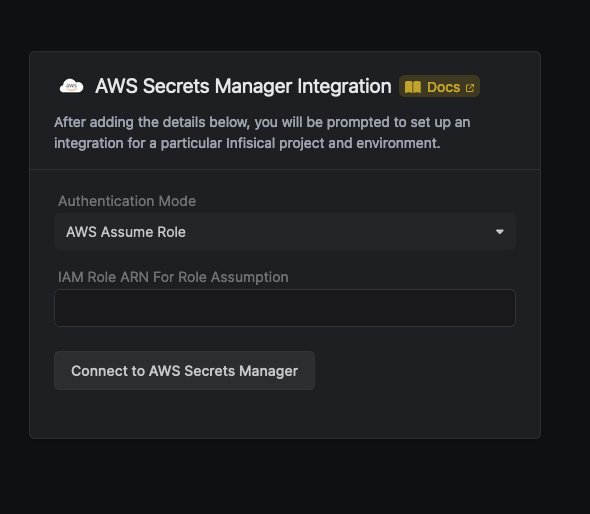
4. Provide the **AWS IAM Role ARN** obtained from the previous step.
Select how you want to integration to work by specifying a number of parameters:
The environment in Infisical from which you want to sync secrets to AWS Secrets Manager.
The path within the preselected environment form which you want to sync secrets to AWS Secrets Manager.
The region that you want to integrate with in AWS Secrets Manager.
How you want the integration to map the secrets. The selected value could be either one to one or one to many.
The secret name/path in AWS into which you want to sync the secrets from Infisical.
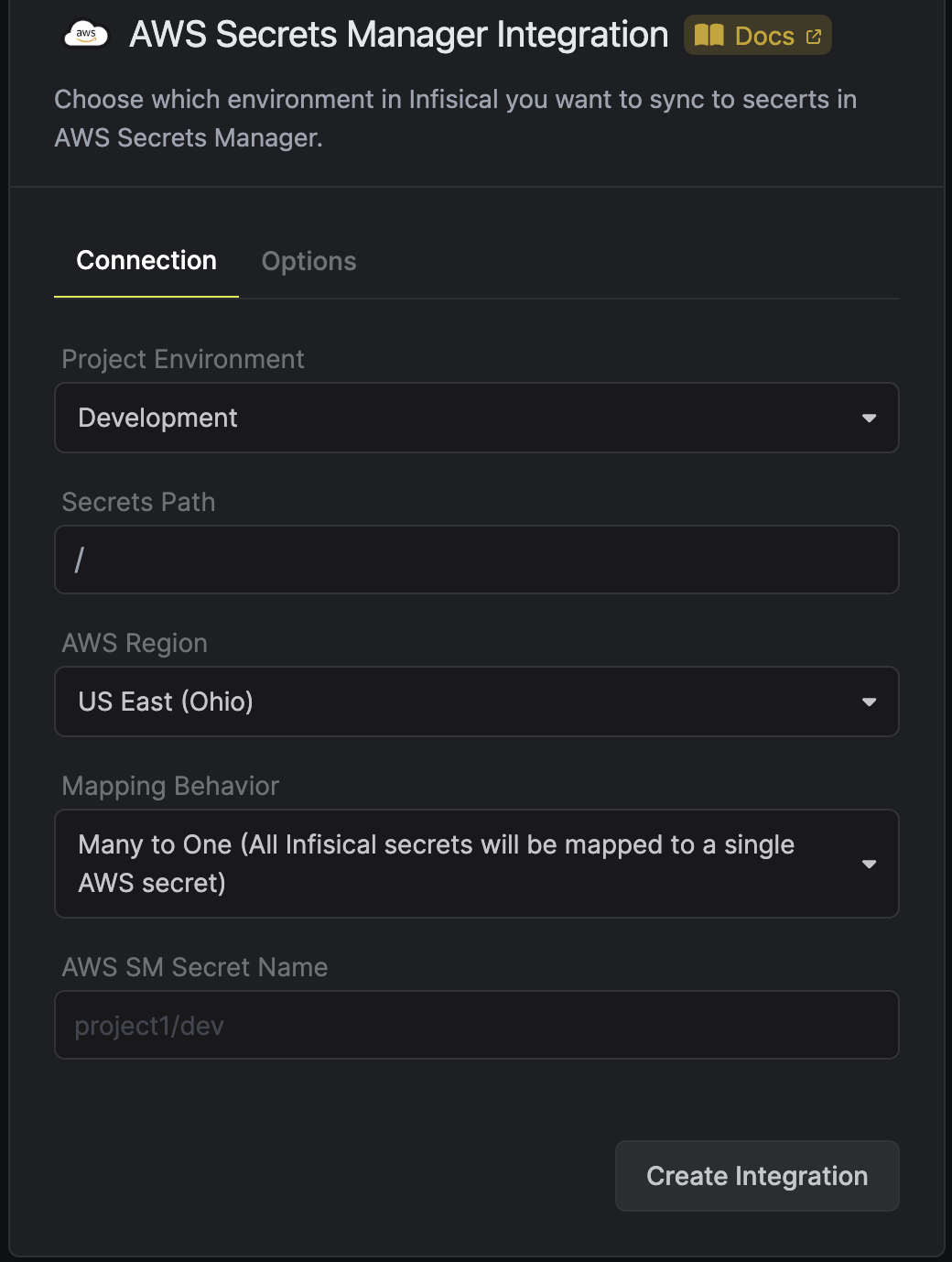
Optionally, you can add tags or specify the encryption key of all the secrets created via this integration:
The Key/Value of a tag that will be added to secrets in AWS. Please note that it is possible to add multiple tags via API.
The alias/ID of the AWS KMS key used for encryption. Please note that key should be enabled in order to work and the IAM user should have access to it.
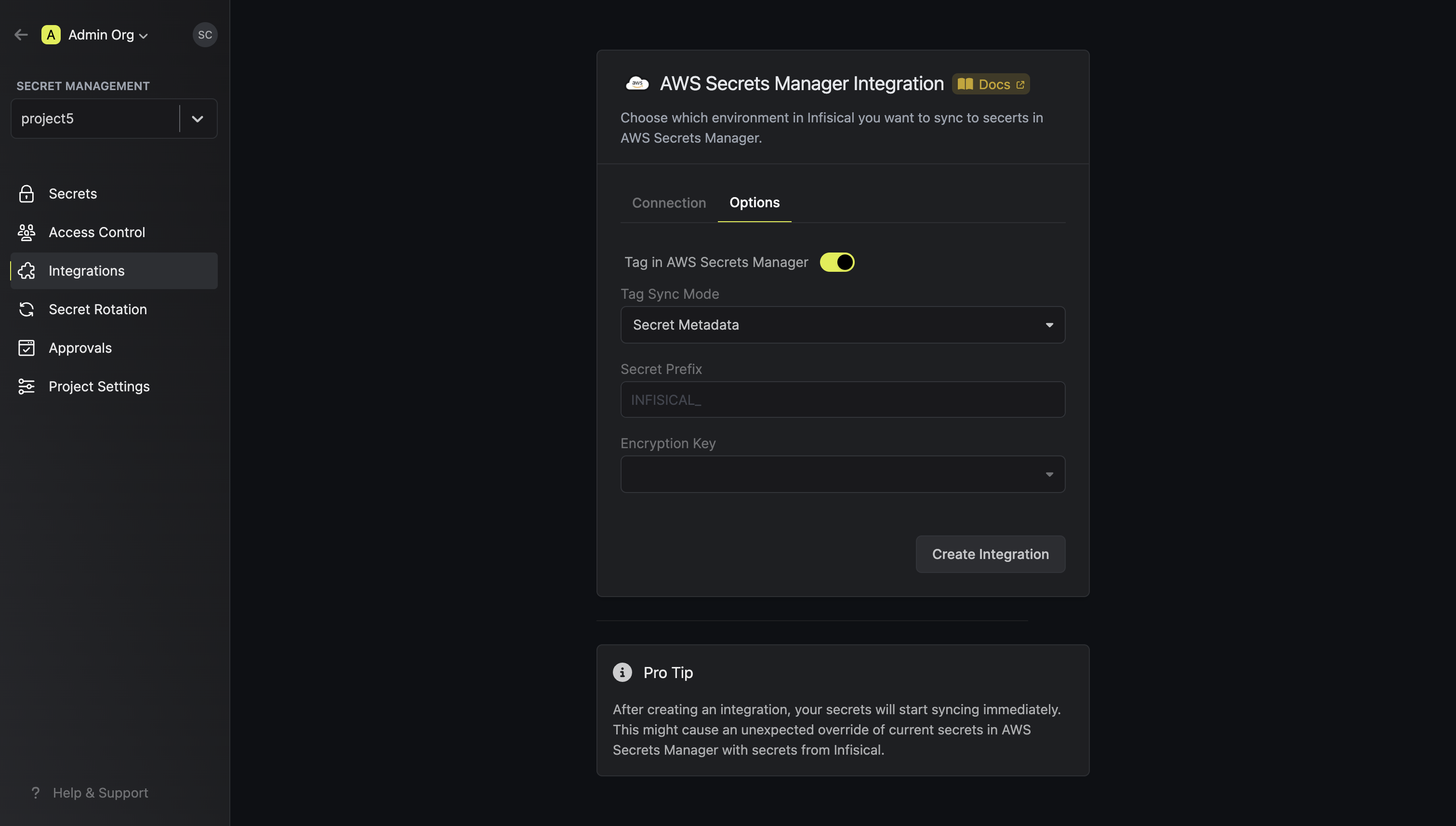
Then, press `Create Integration` to start syncing secrets to AWS Secrets Manager.
Infisical currently syncs environment variables to AWS Secrets Manager as
key-value pairs under one secret. We're actively exploring ways to help users
group environment variable key-pairs under multiple secrets for greater
control.
Please note that upon deleting secrets in Infisical, AWS Secrets Manager immediately makes the secrets inaccessible but only schedules them for deletion after at least 7 days.
Infisical will access your account using the provided AWS access key and secret key.
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
* Set up AWS and have/create an IAM user
Navigate to your IAM user permissions and add a permission policy to grant access to AWS Secrets Manager.
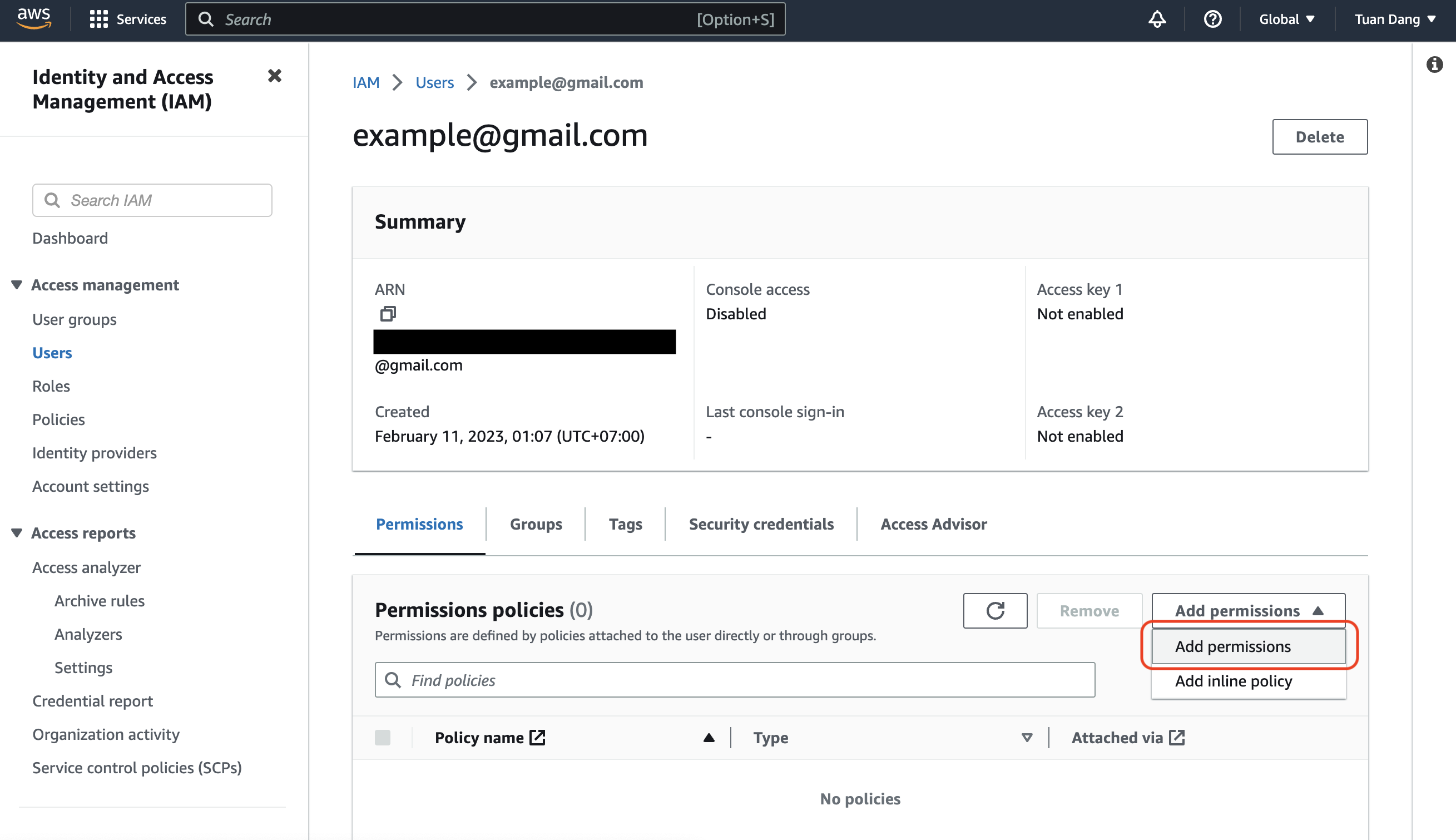
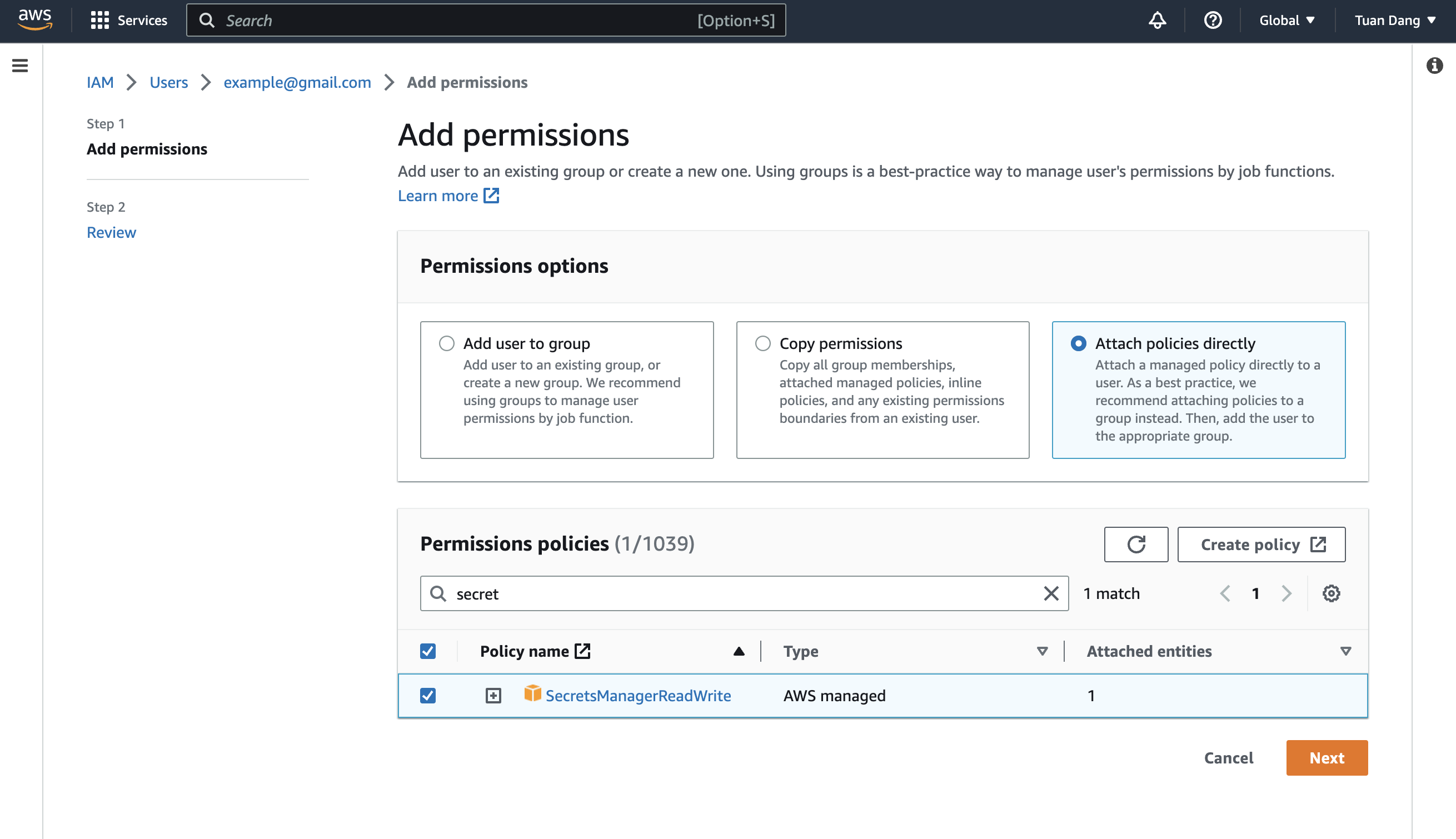
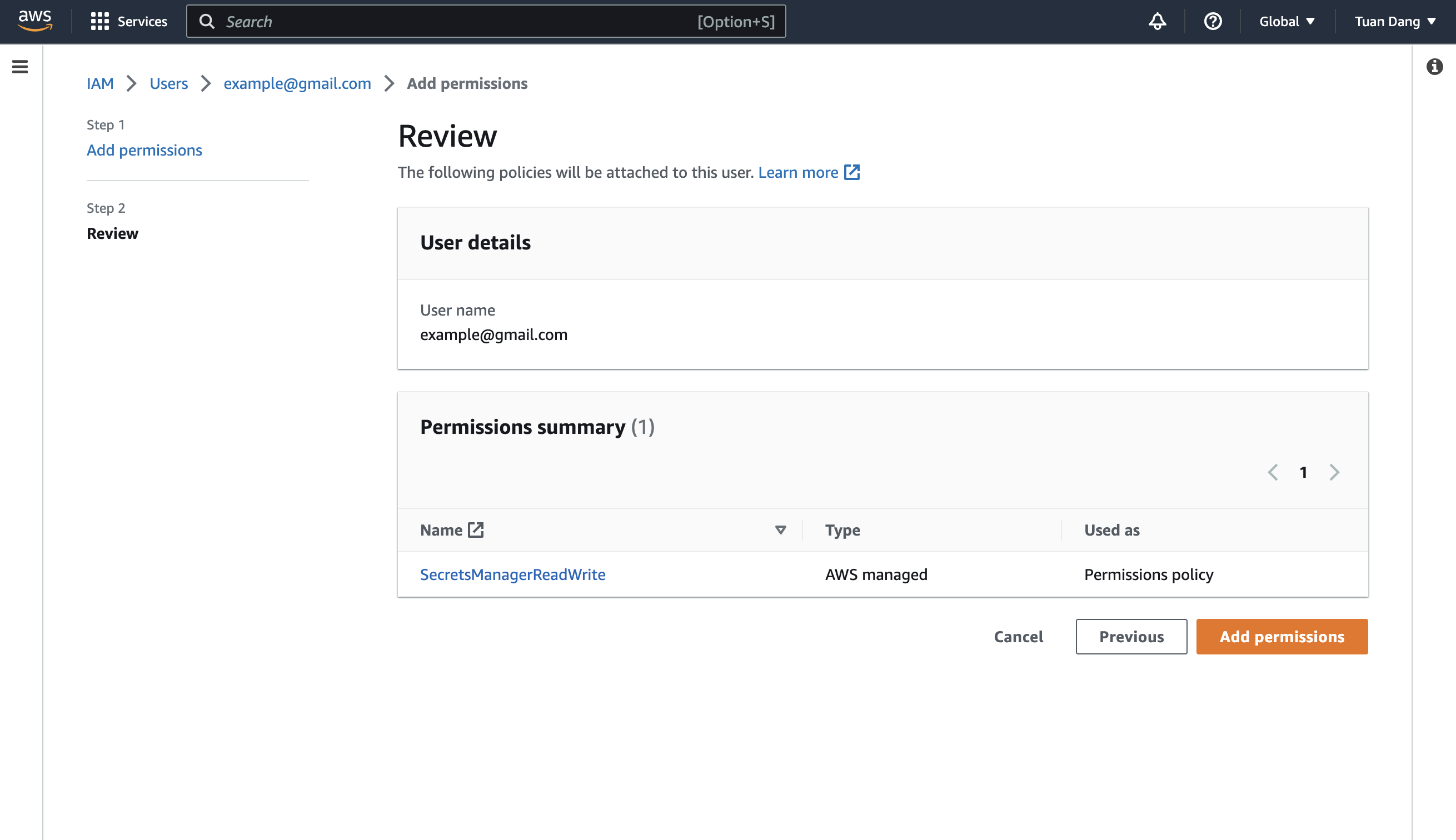
For better security, here's a custom policy containing the minimum permissions required by Infisical to sync secrets to AWS Secrets Manager for the IAM user that you can use:
```json
{
"Version": "2012-10-17",
"Statement": [
{
"Sid": "AllowSecretsManagerAccess",
"Effect": "Allow",
"Action": [
"secretsmanager:GetSecretValue",
"secretsmanager:CreateSecret",
"secretsmanager:UpdateSecret",
"secretsmanager:DescribeSecret", // if you need to add tags to secrets
"secretsmanager:TagResource", // if you need to add tags to secrets
"secretsmanager:UntagResource", // if you need to add tags to secrets
"kms:ListKeys", // if you need to specify the KMS key
"kms:ListAliases", // if you need to specify the KMS key
"kms:Encrypt", // if you need to specify the KMS key
"kms:Decrypt" // if you need to specify the KMS key
],
"Resource": "*"
}
]
}
```
Obtain a AWS access key ID and secret access key for your IAM user in IAM > Users > User > Security credentials > Access keys
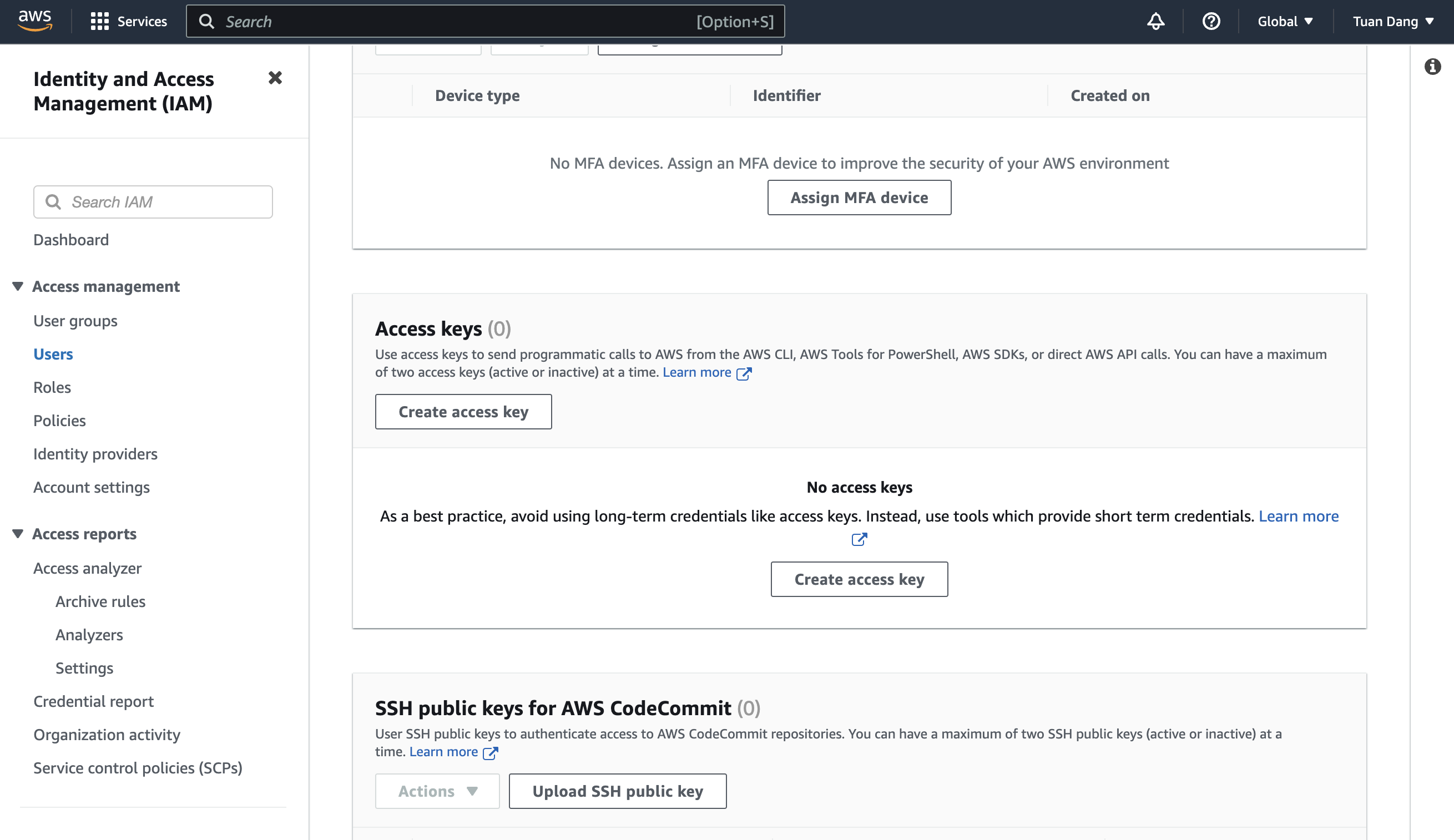
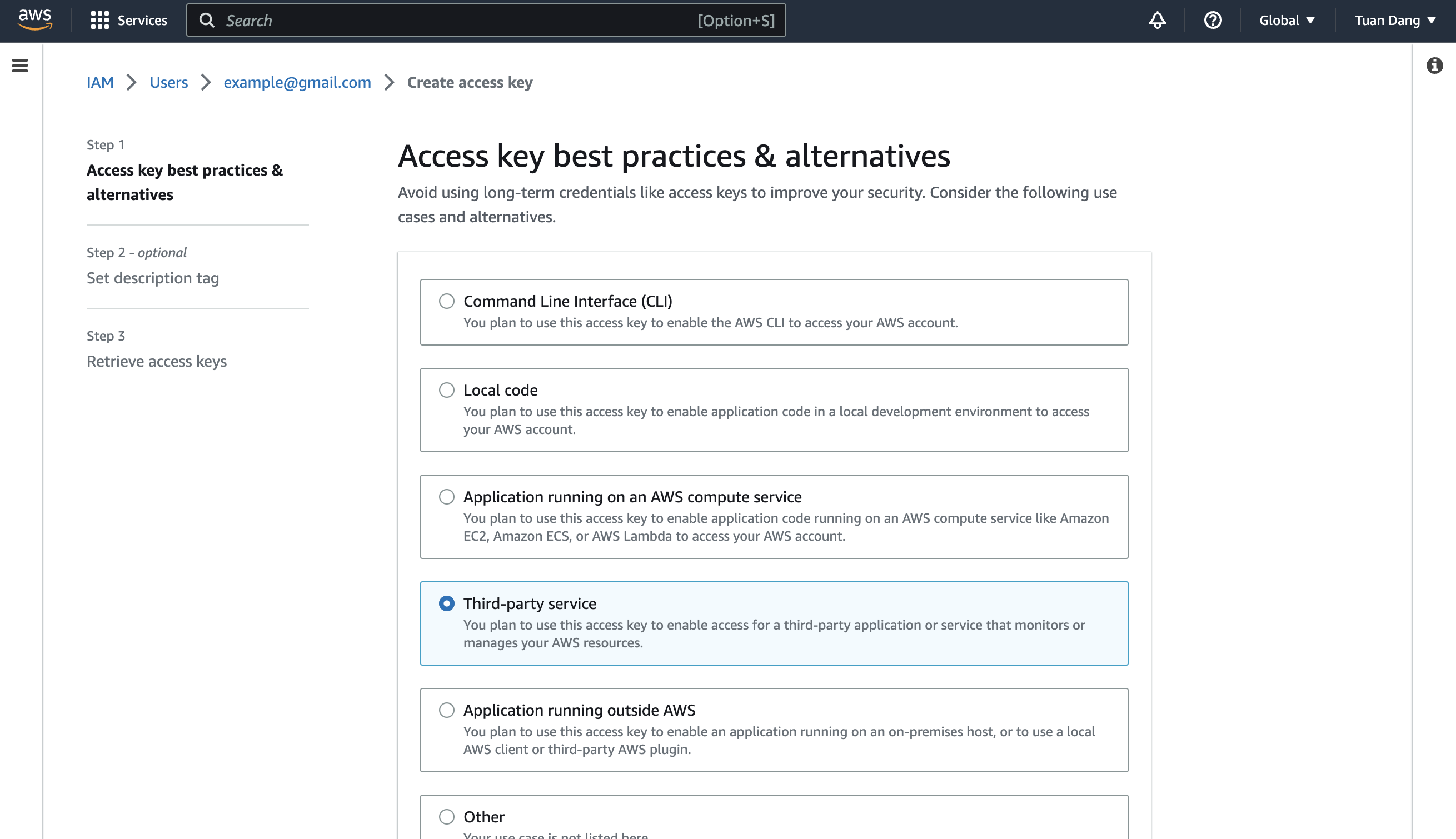
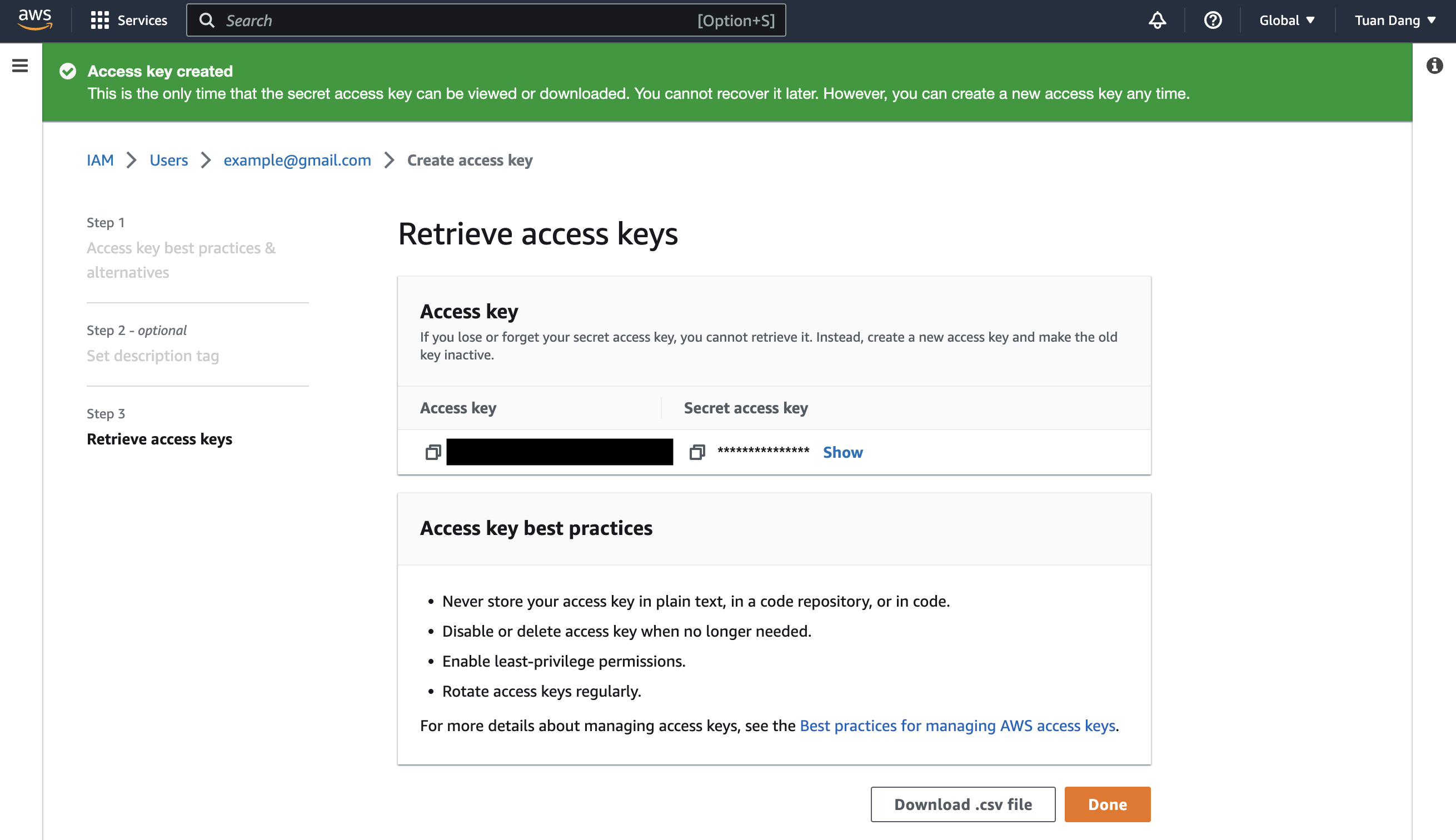
1. Navigate to your project's integrations tab in Infisical.
2. Click on the **AWS Secrets Manager** tile.
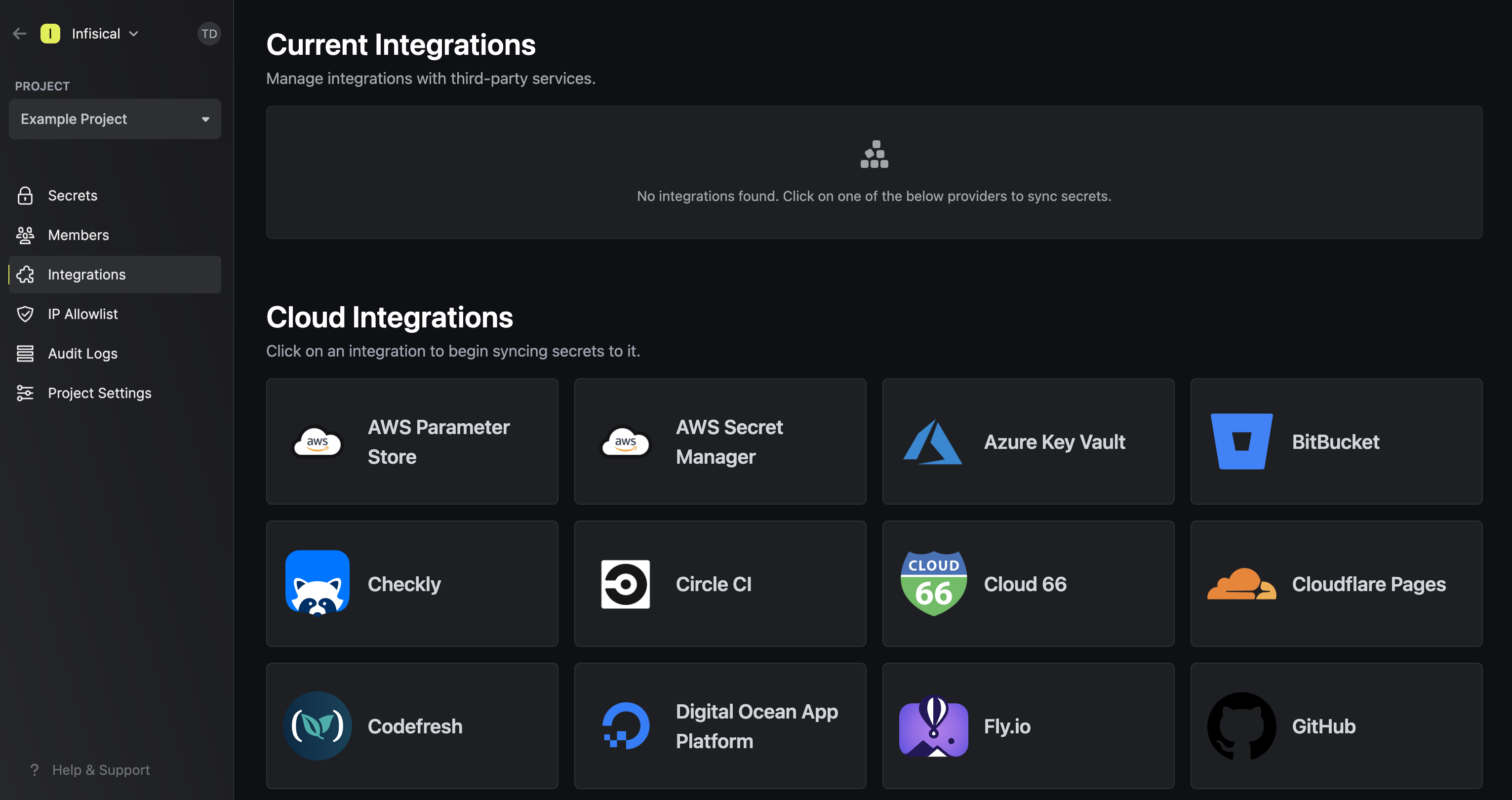
3. Select the **Access Key** option for Authentication Mode.
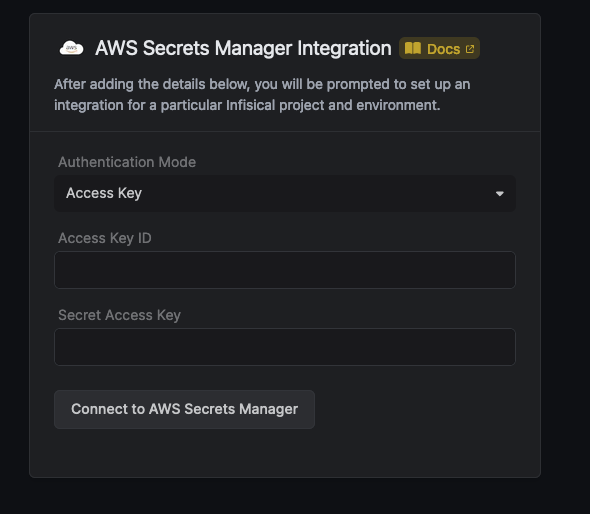
4. Provide the **access key** and **secret key** for the AWS Iam User.
Select how you want to integration to work by specifying a number of parameters:
The environment in Infisical from which you want to sync secrets to AWS Secrets Manager.
The path within the preselected environment form which you want to sync secrets to AWS Secrets Manager.
The region that you want to integrate with in AWS Secrets Manager.
How you want the integration to map the secrets. The selected value could be either one to one or one to many.
The secret name/path in AWS into which you want to sync the secrets from Infisical.
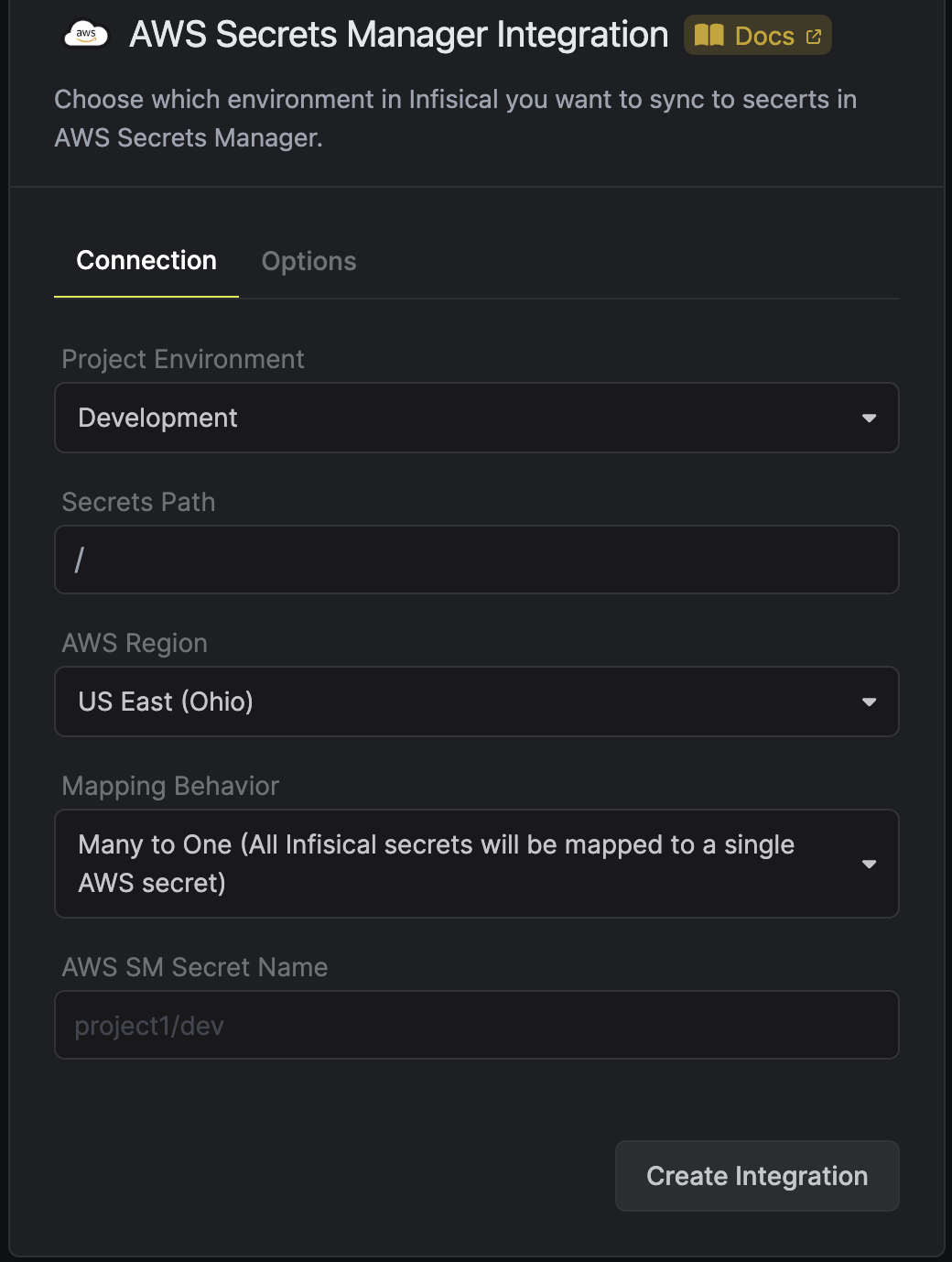
Optionally, you can add tags or specify the encryption key of all the secrets created via this integration:
The Key/Value of a tag that will be added to secrets in AWS. Please note that it is possible to add multiple tags via API.
The alias/ID of the AWS KMS key used for encryption. Please note that key should be enabled in order to work and the IAM user should have access to it.
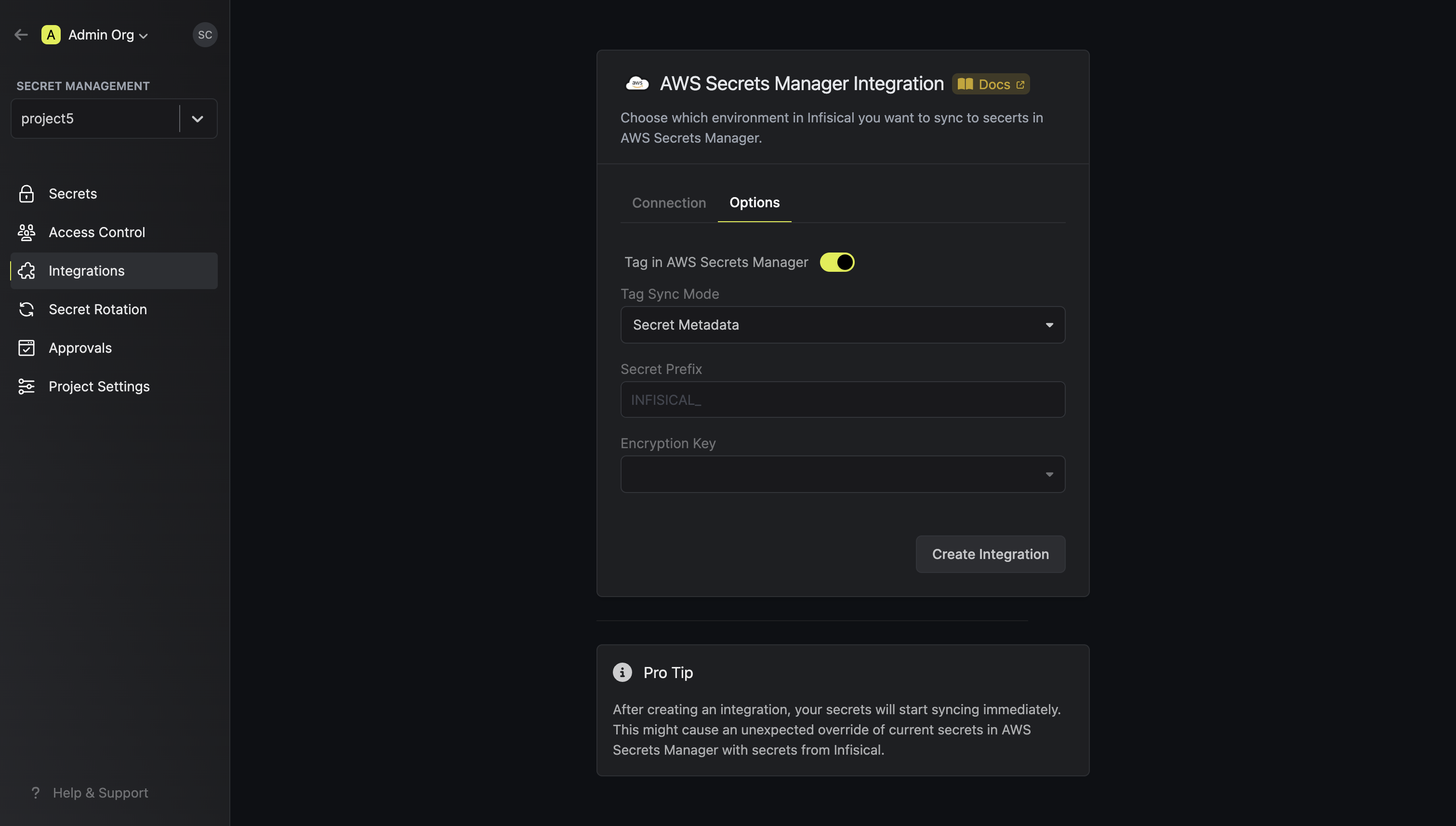
Then, press `Create Integration` to start syncing secrets to AWS Secrets Manager.
Infisical currently syncs environment variables to AWS Secrets Manager as
key-value pairs under one secret. We're actively exploring ways to help users
group environment variable key-pairs under multiple secrets for greater
control.
Please note that upon deleting secrets in Infisical, AWS Secrets Manager immediately makes the secrets inaccessible but only schedules them for deletion after at least 7 days.
# Azure App Configuration
How to sync secrets from Infisical to Azure App Configuration
**Prerequisites:**
* Set up and add envars to [Infisical Cloud](https://app.infisical.com).
* Set up Azure and have an existing App Configuration instance.
* User setting up the integration on Infisical must have the `App Configuration Data Owner` role for the intended Azure App Configuration instance.
* Azure App Configuration instance must be reachable by Infisical.
Navigate to your project's integrations tab
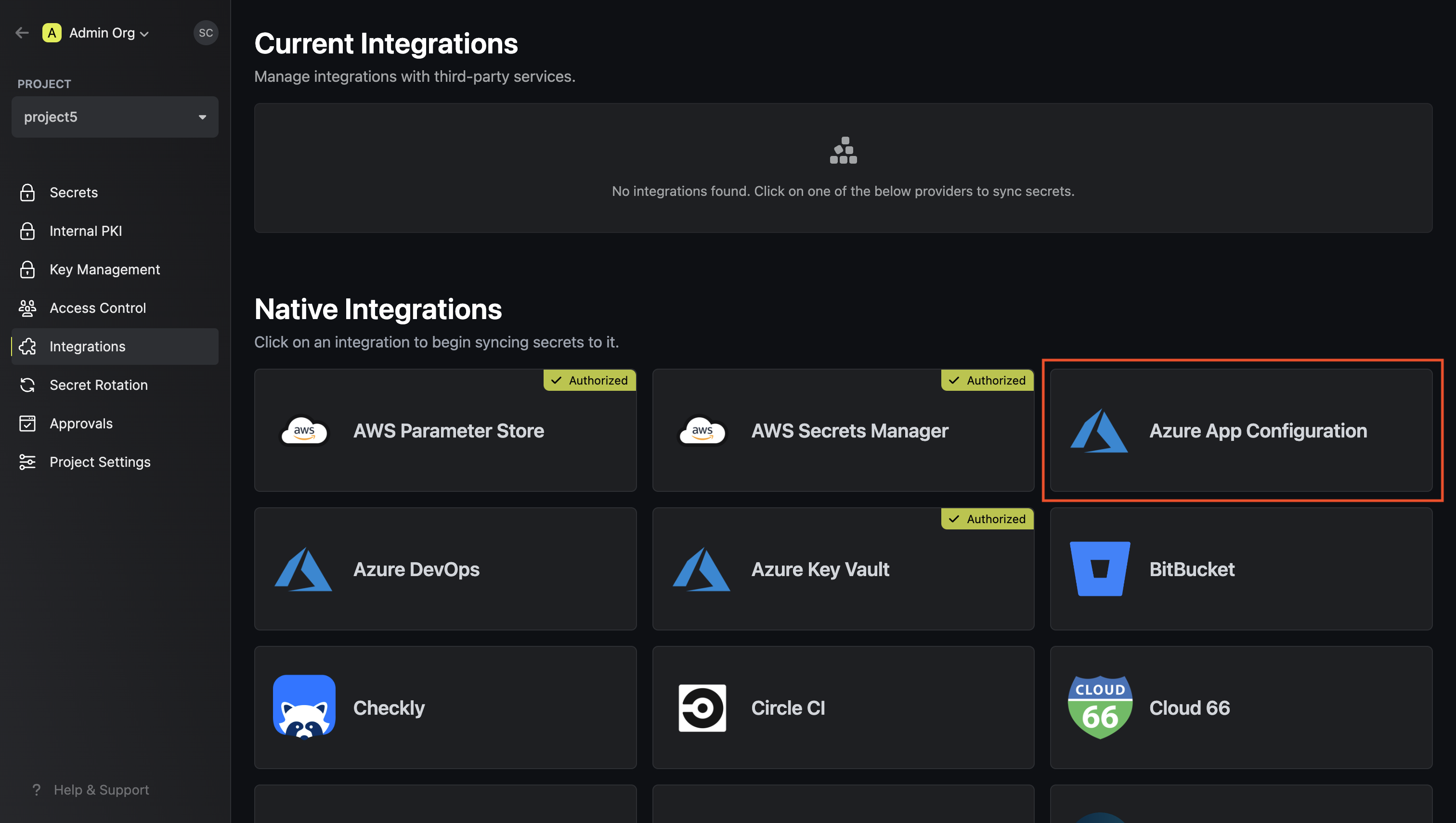
Press on the Azure App Configuration tile and grant Infisical access to App Configuration.
Obtain the Azure App Configuration endpoint from the overview tab.
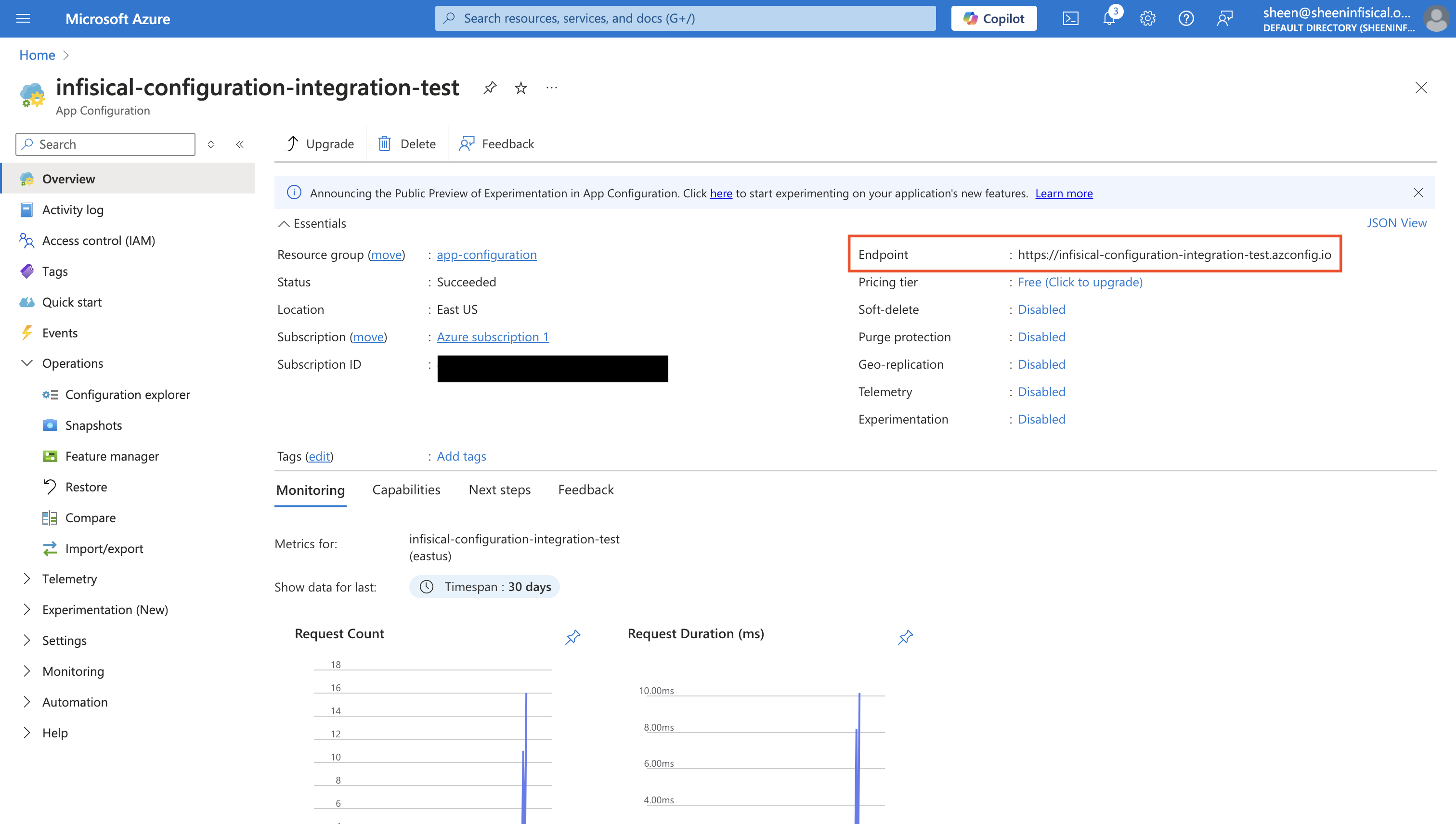
Select which Infisical environment secrets you want to sync to your Azure App Configuration. Then, input your App Configuration instance endpoint. Optionally, you can define a prefix for your secrets which will be appended to the keys upon syncing.
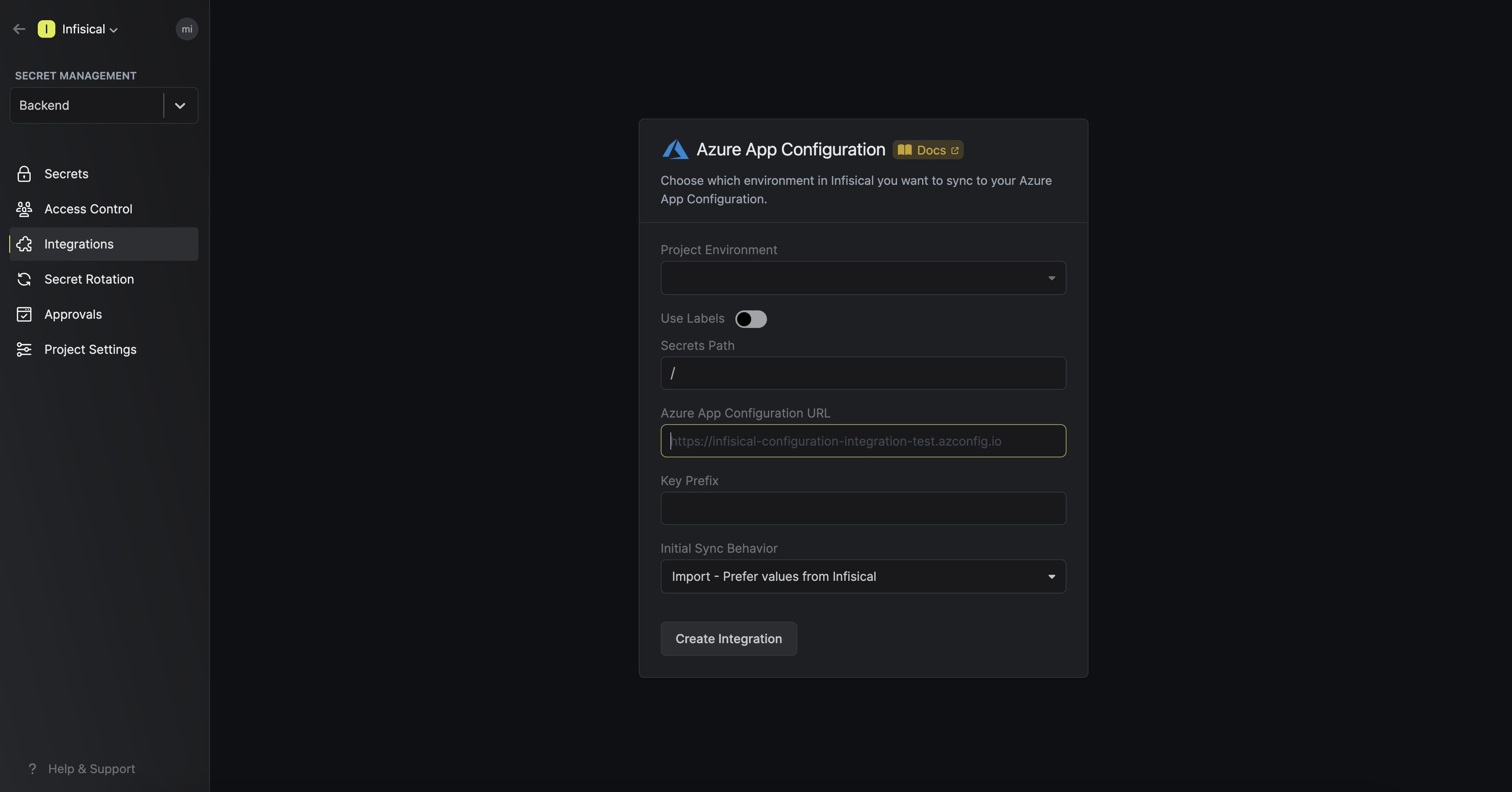
Press create integration to start syncing secrets to Azure App Configuration.
Using the Azure App Configuration integration on a self-hosted instance of Infisical requires configuring an application in Azure
and registering your instance with it.
**Prerequisites:**
* Set up Azure and have an existing App Configuration instance.
Navigate to Azure Active Directory > App registrations to create a new application.
Azure Active Directory is now Microsoft Entra ID.
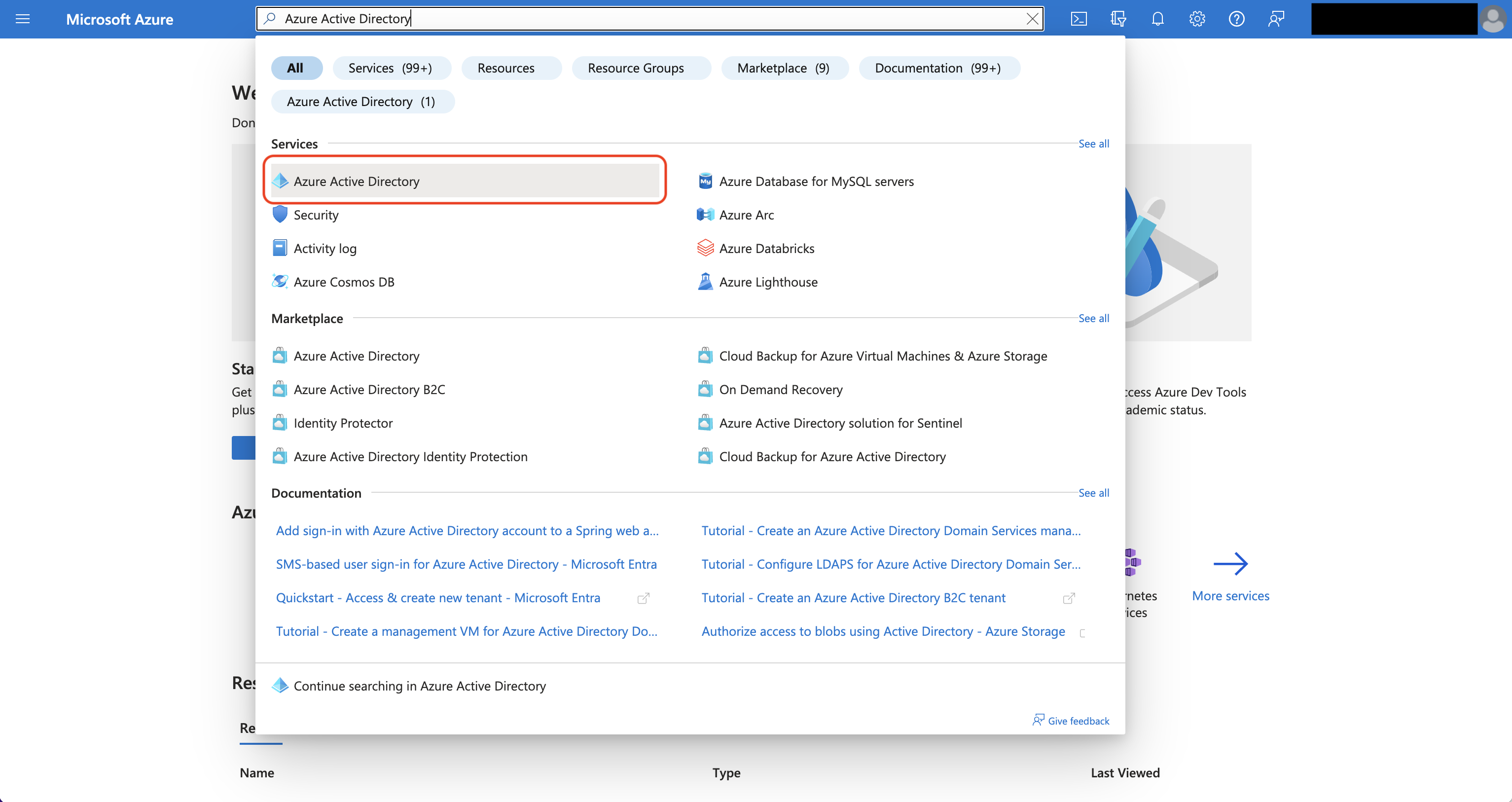
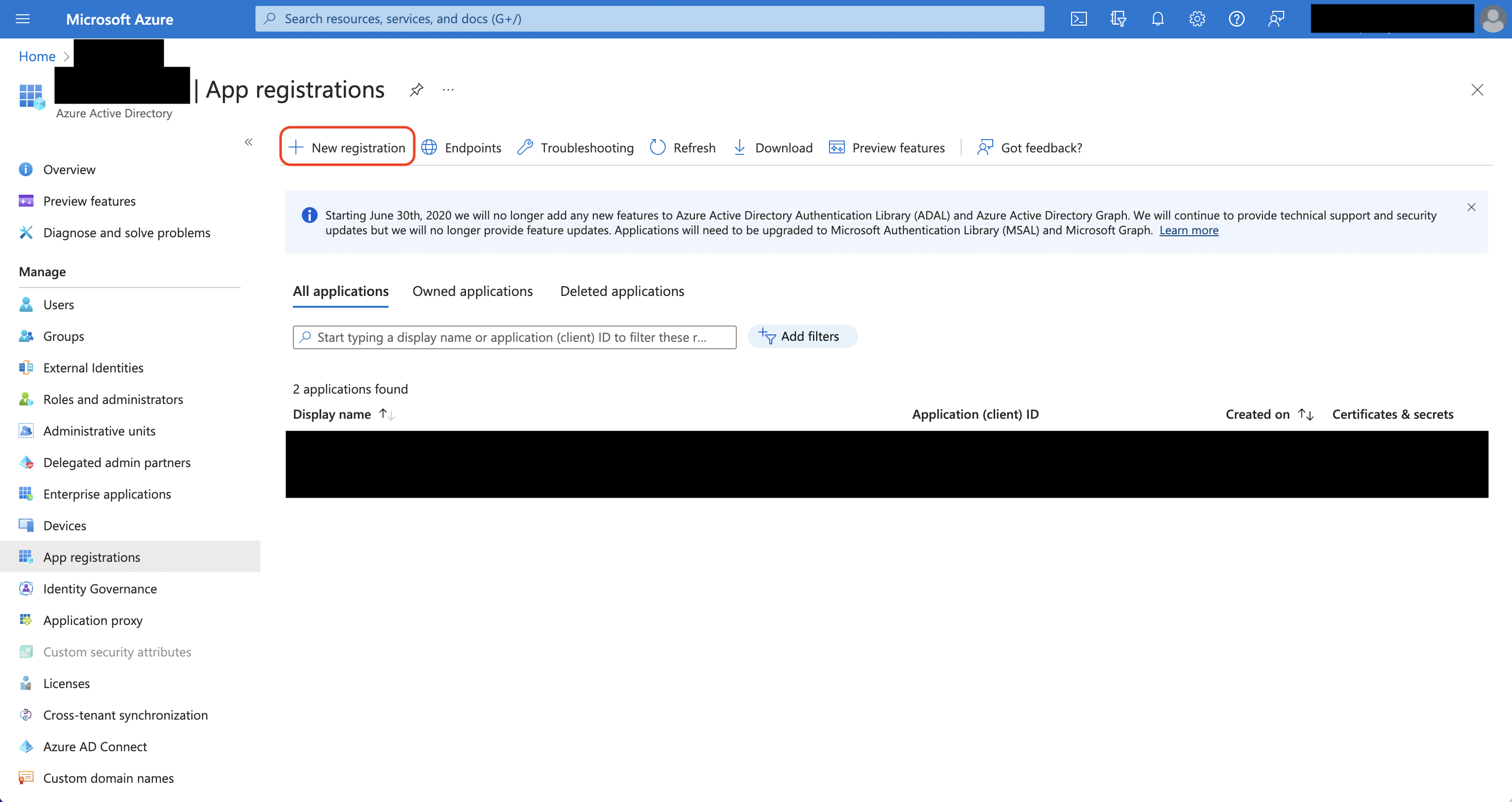
Create the application. As part of the form, set the **Redirect URI** to `https://your-domain.com/integrations/azure-app-configuration/oauth2/callback`.
The domain you defined in the Redirect URI should be equivalent to the `SITE_URL` configured in your Infisical instance.
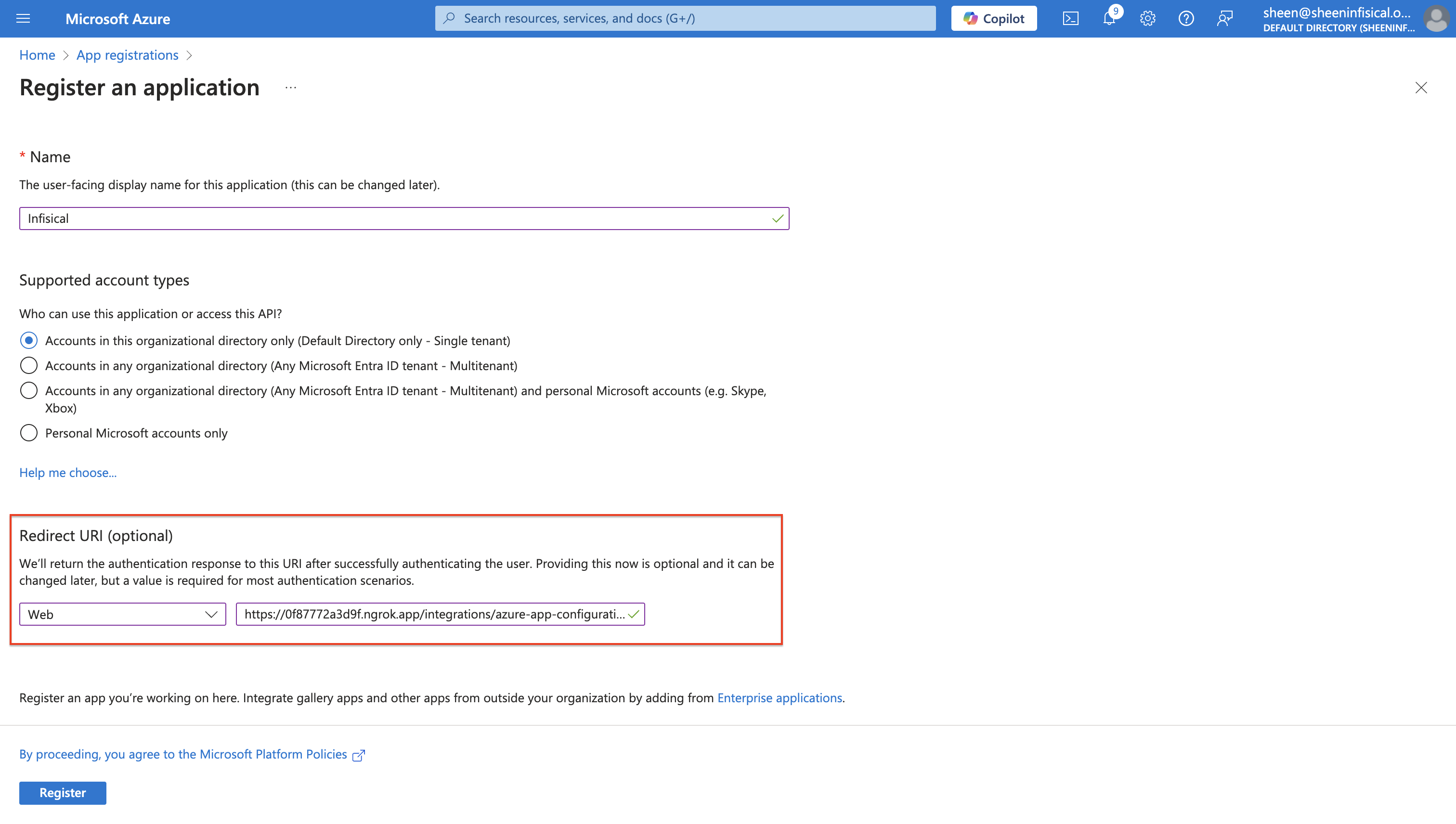
After registration, set the API permissions of the app to include the following Azure App Configuration permissions: KeyValue.Delete, KeyValue.Read, and KeyValue.Write.
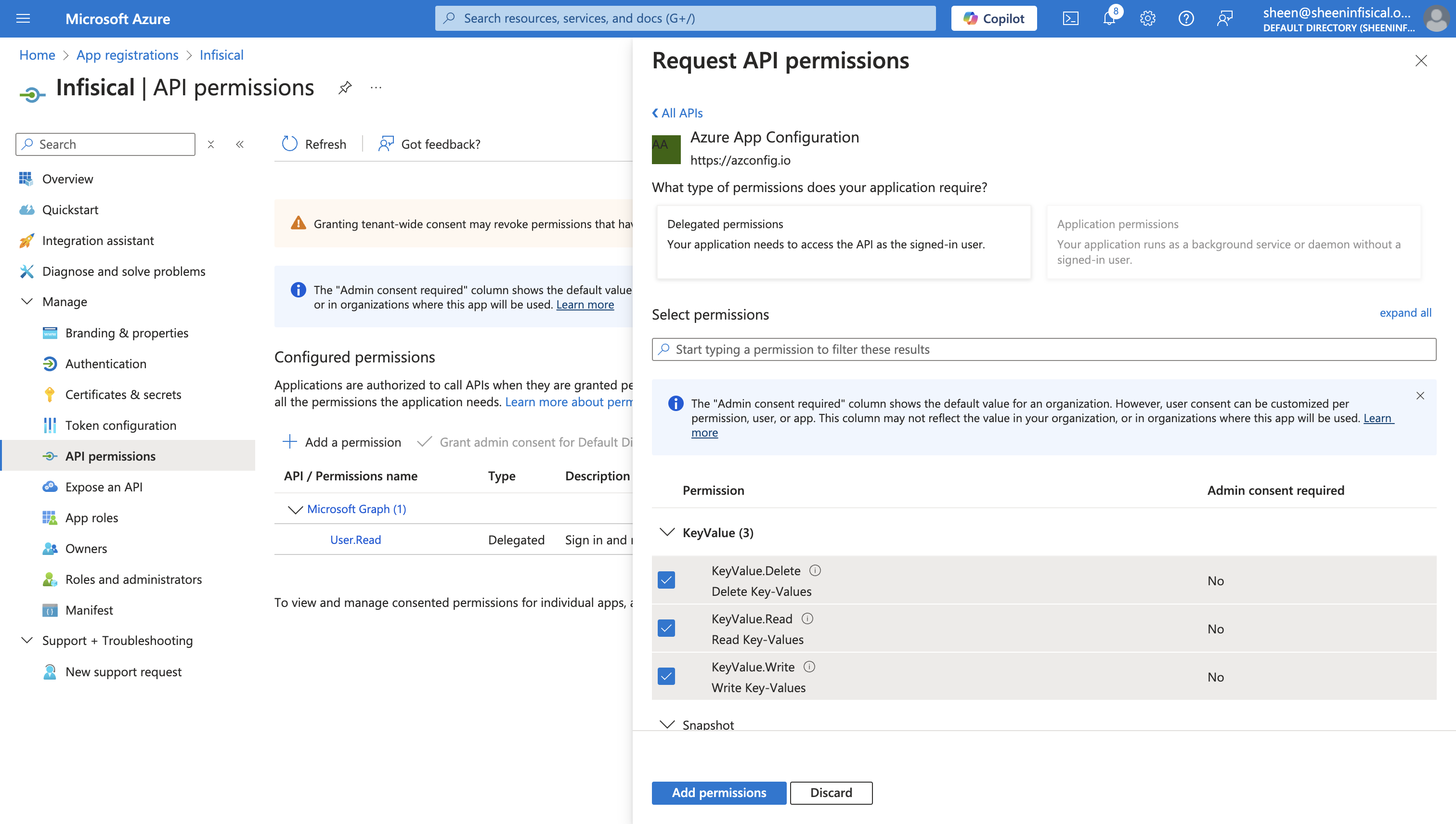
Obtain the **Application (Client) ID** in Overview and generate a **Client Secret** in Certificate & secrets for your Azure application.
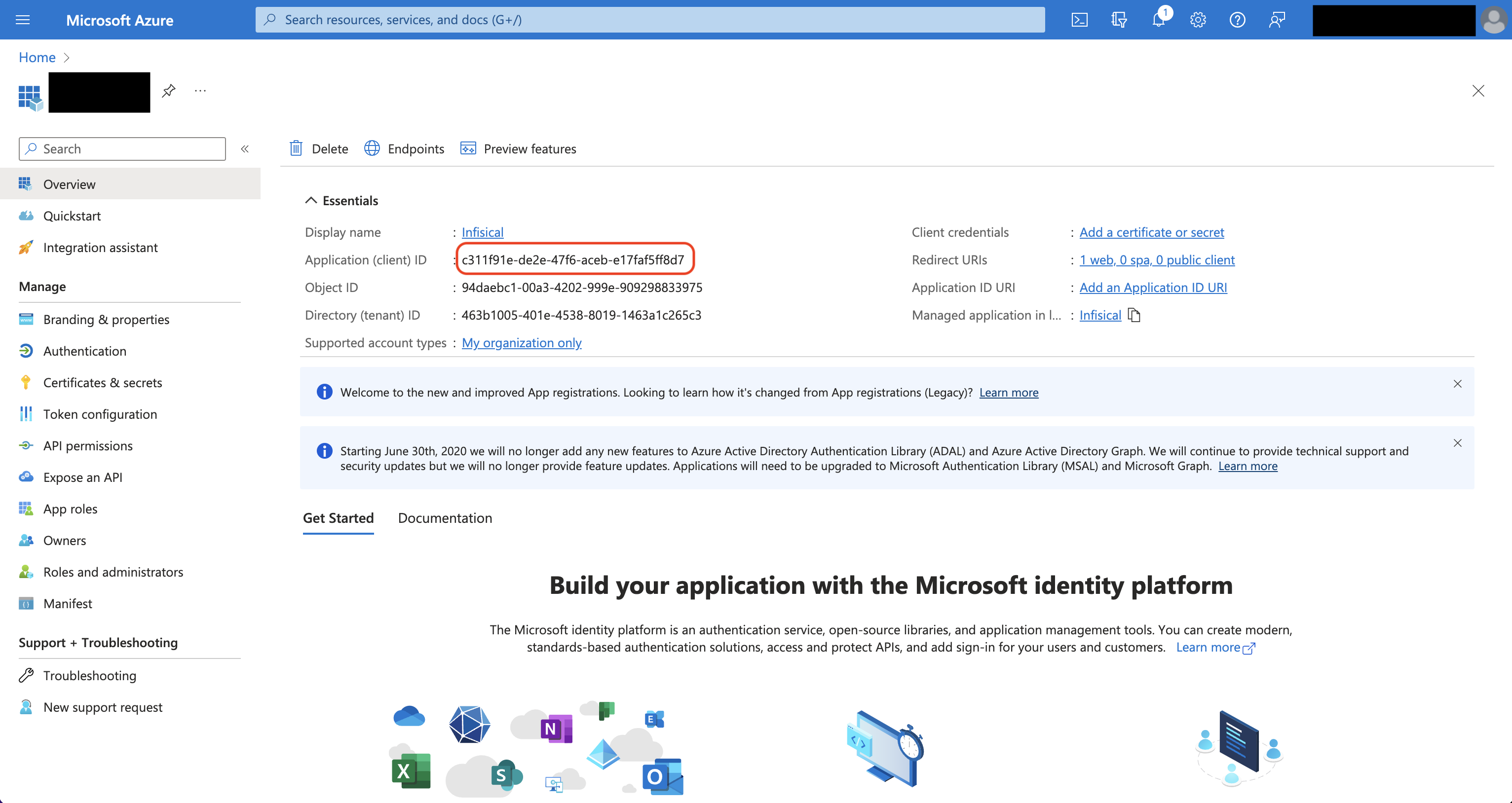
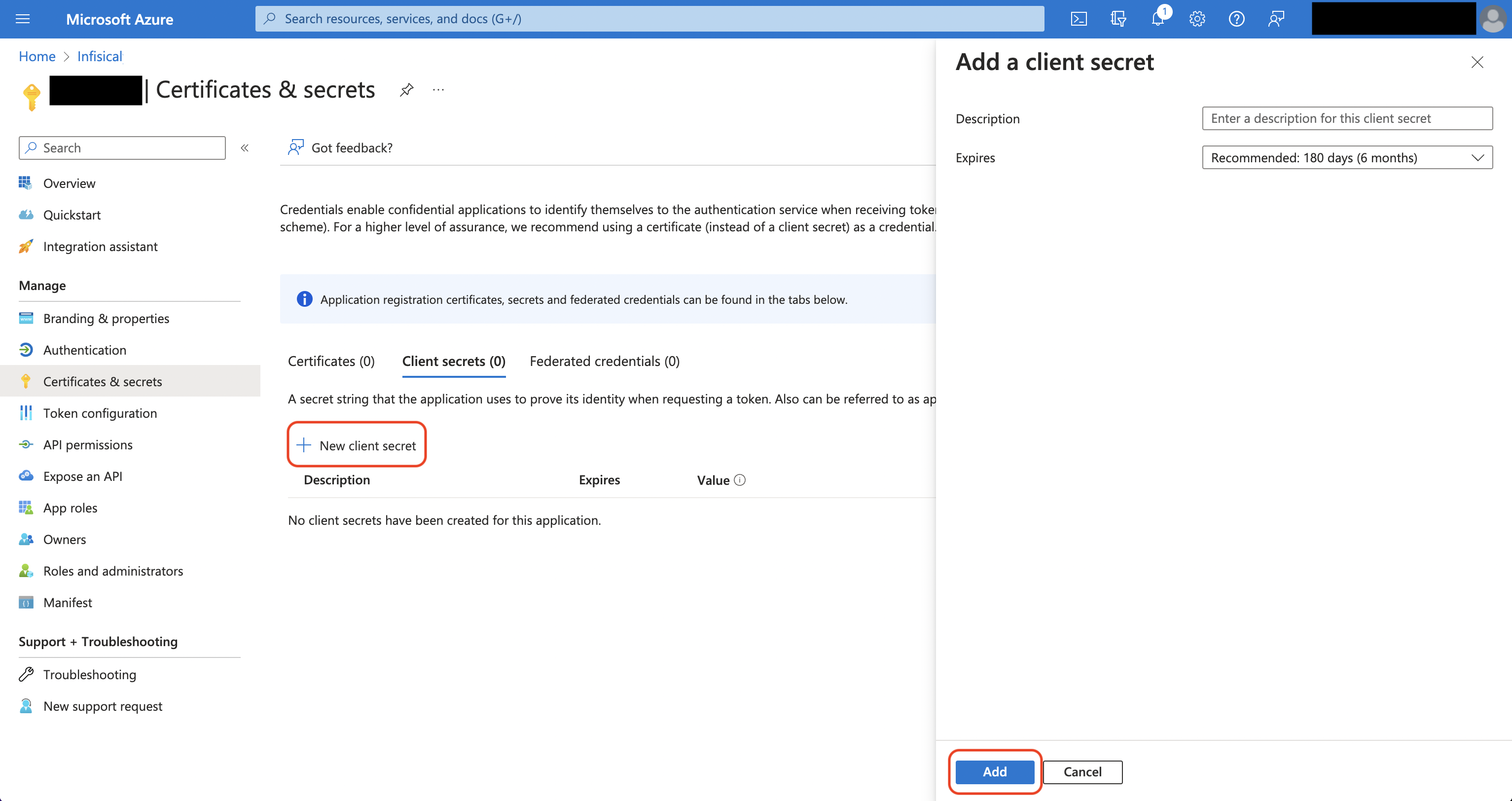
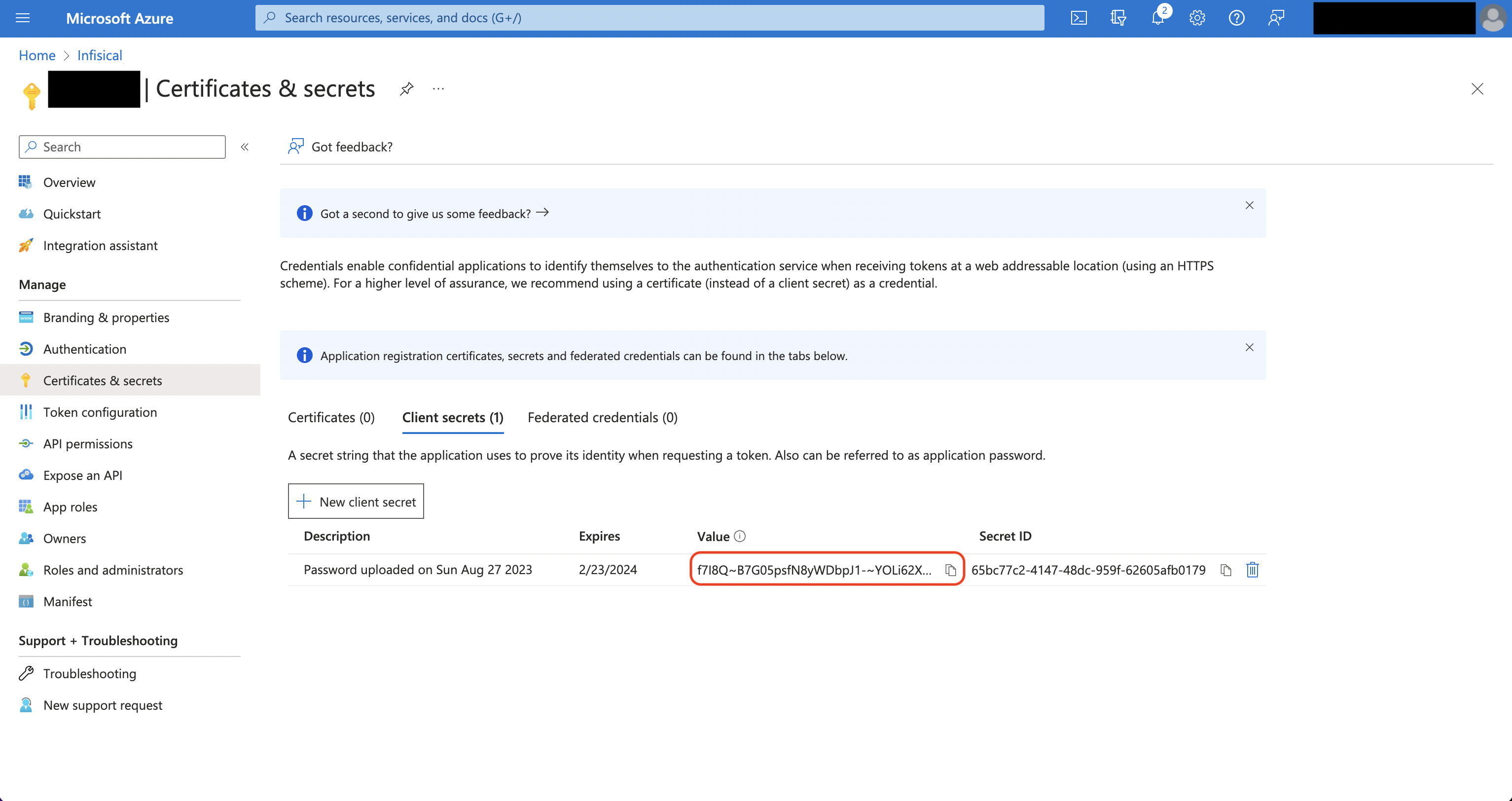
Back in your Infisical instance, add two new environment variables for the credentials of your Azure application.
* `CLIENT_ID_AZURE`: The **Application (Client) ID** of your Azure application.
* `CLIENT_SECRET_AZURE`: The **Client Secret** of your Azure application.
Once added, restart your Infisical instance and use the Azure App Configuration integration.
# Azure DevOps
How to sync secrets from Infisical to Azure DevOps
### Usage
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com).
* Create a new [Azure DevOps](https://dev.azure.com) project if you don't have one already.
#### Create a new Azure DevOps personal access token (PAT)
You'll need to create a new personal access token (PAT) in order to authenticate Infisical with Azure DevOps.
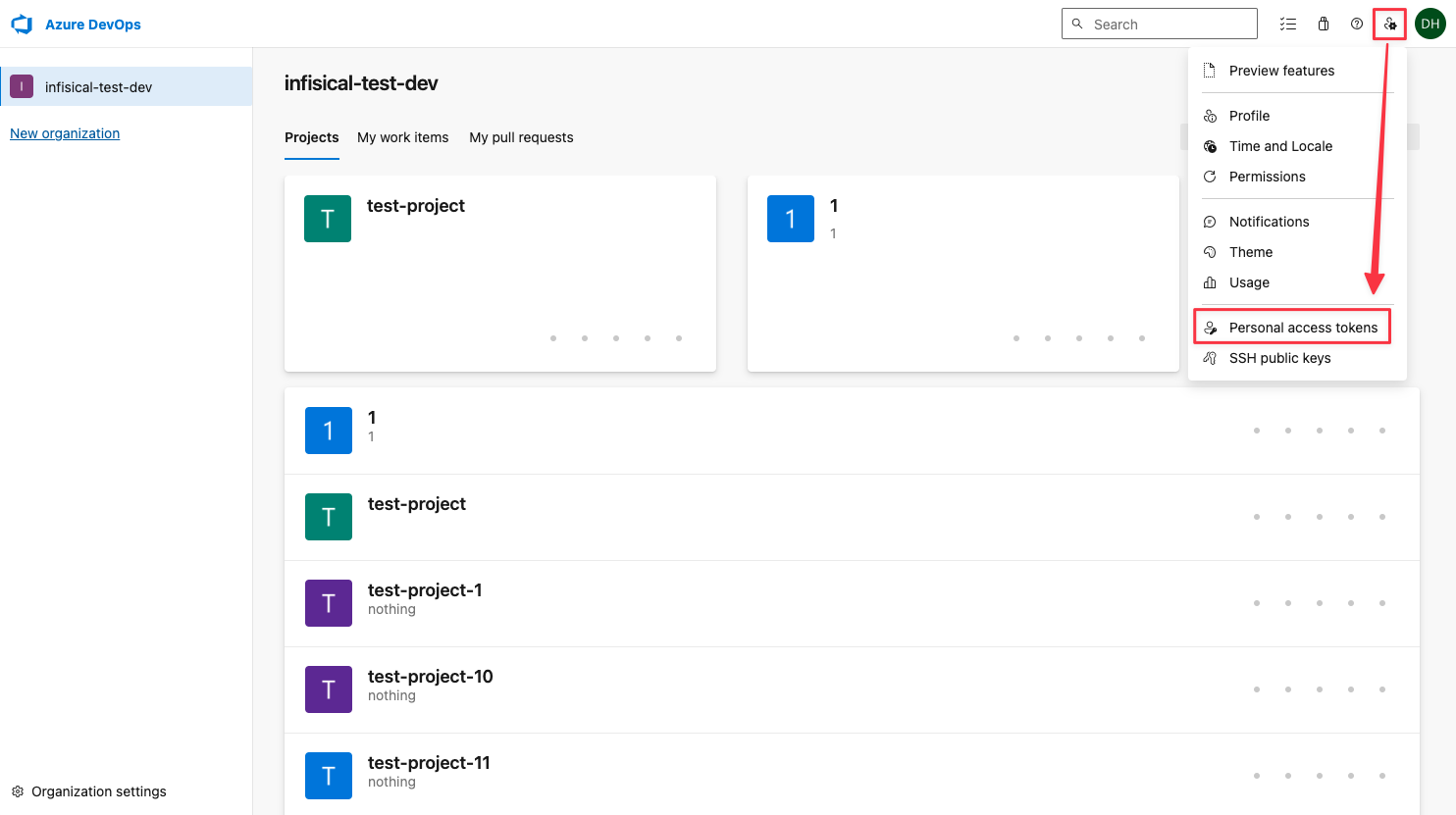
Make sure the newly created token has Read/Write access to the Release scope.
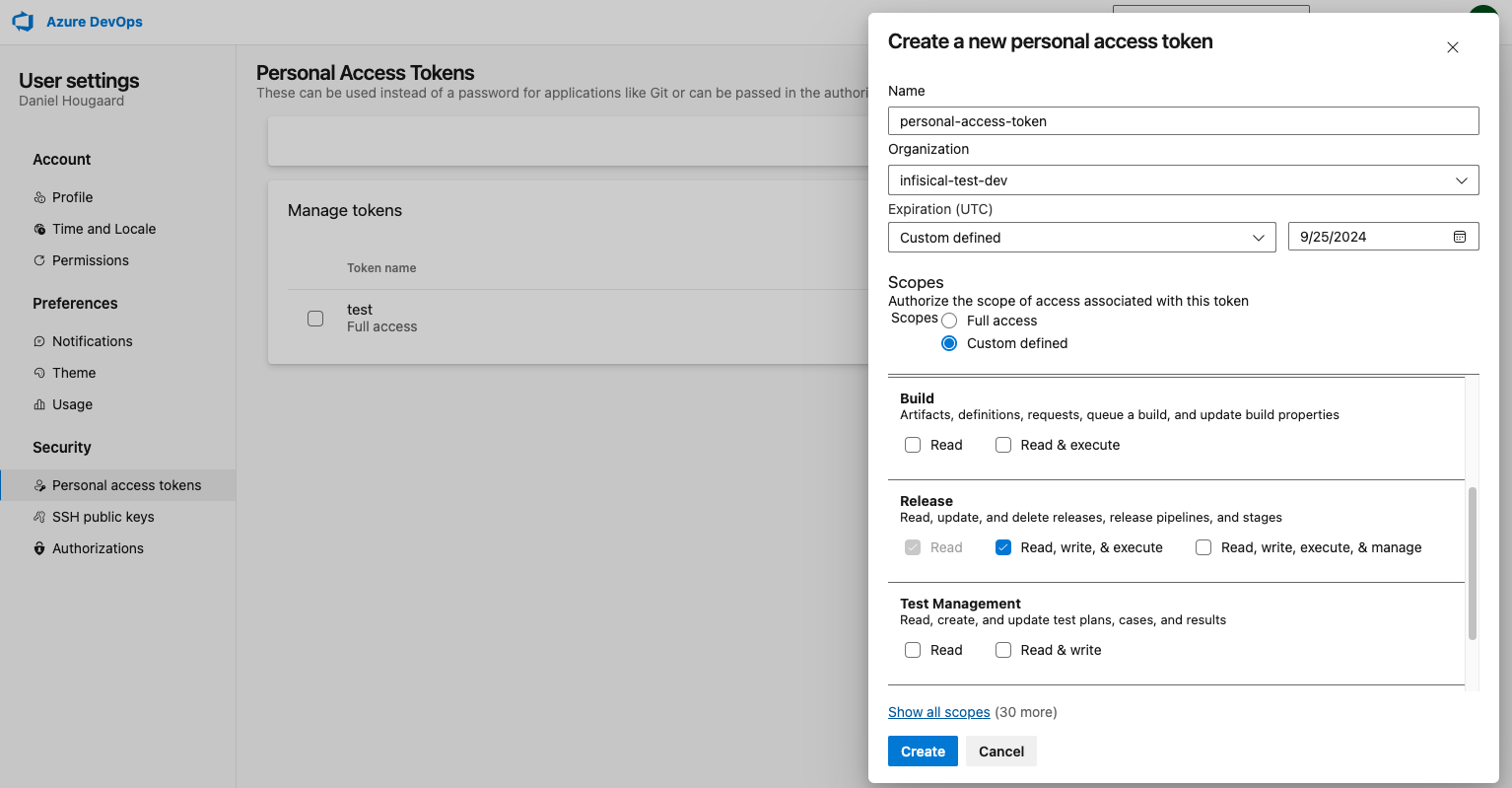
Please make sure that the token has access to the following scopes: Variable Groups *(read/write)*, Release *(read/write)*, Project and Team *(read)*, Service Connections *(read & query)*
Copy the newly created token as this will be used to authenticate Infisical with Azure DevOps.
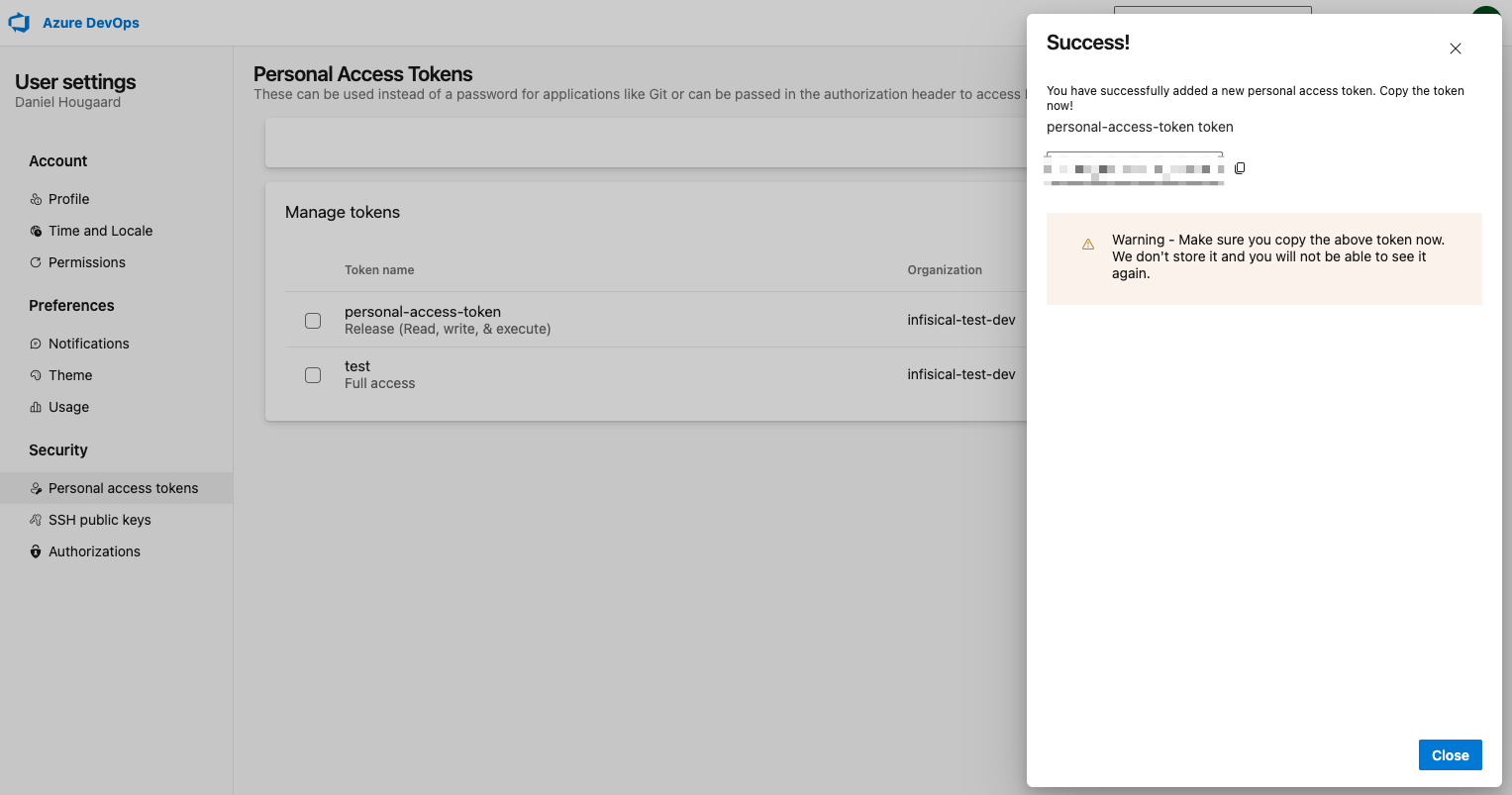
#### Setup the Infisical Azure DevOps integration
Navigate to your project's integrations tab and select the 'Azure DevOps' integration.
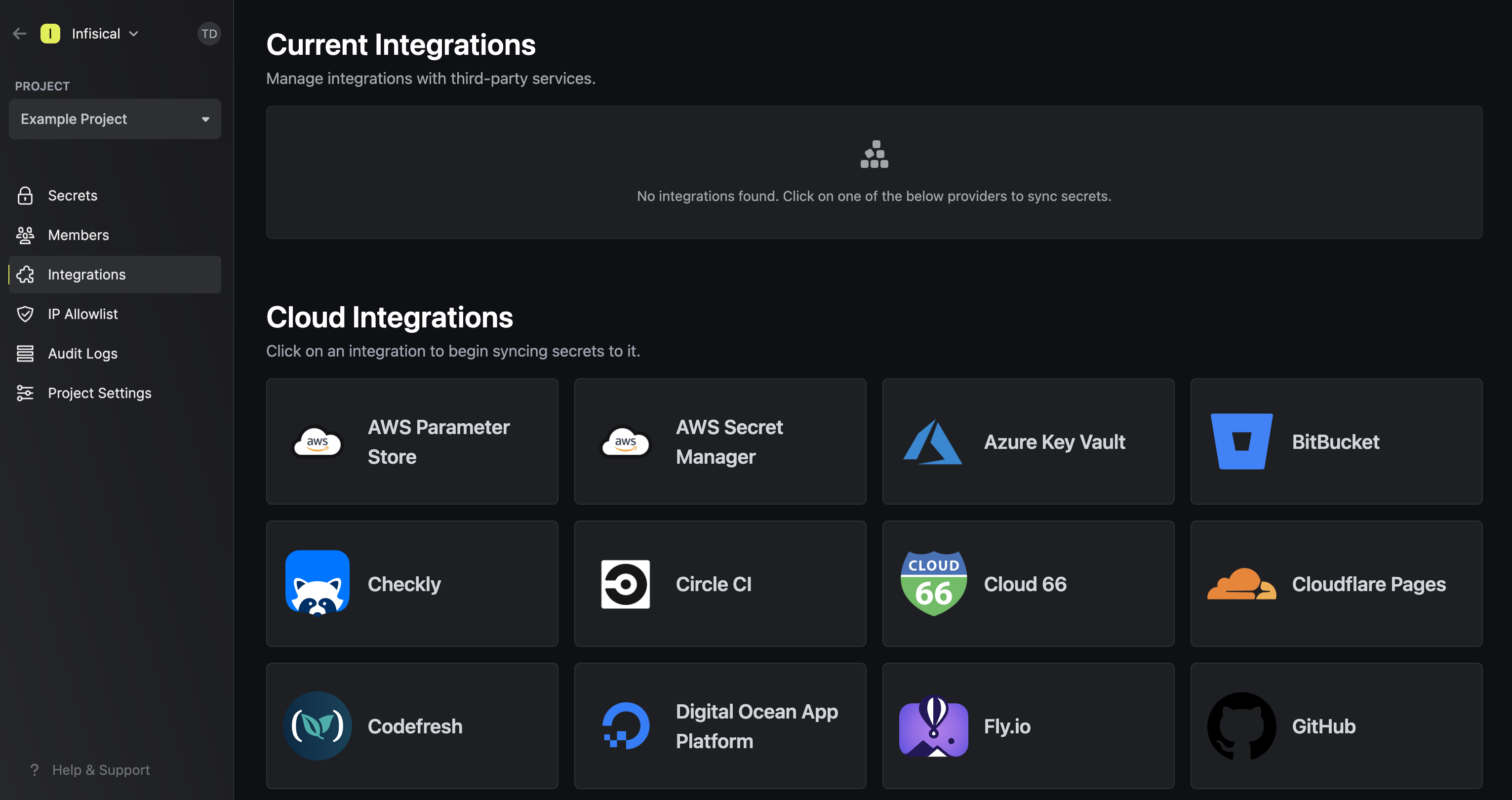
Enter your credentials that you obtained from the previous step.
1. Azure DevOps API token is the personal access token (PAT) you created in the previous step.
2. Azure DevOps organization name is the name of your Azure DevOps organization.
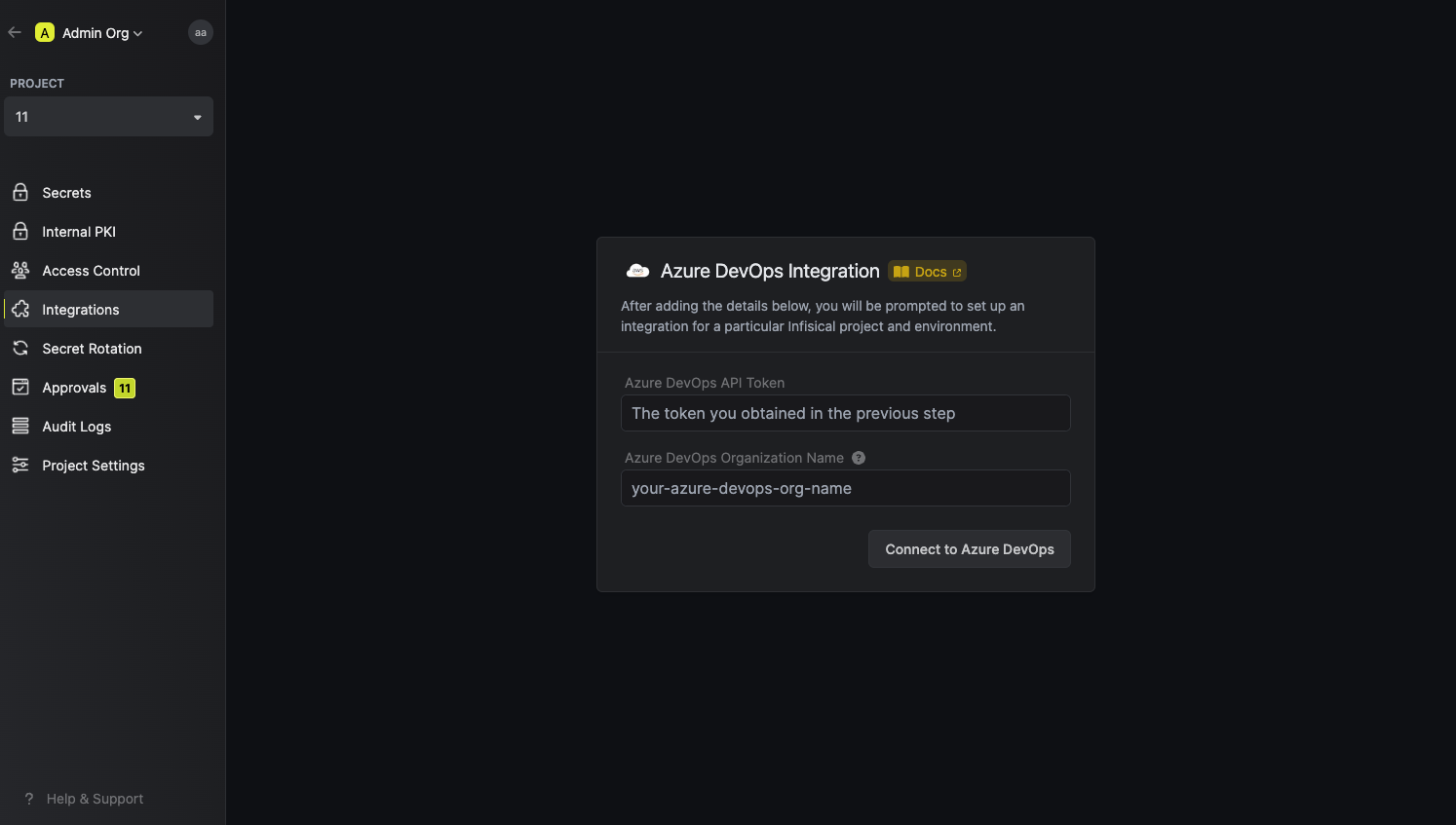
Select Infisical project and secret path you want to sync into Azure DevOps.
Finally, press create integration to start syncing secrets to Azure DevOps.
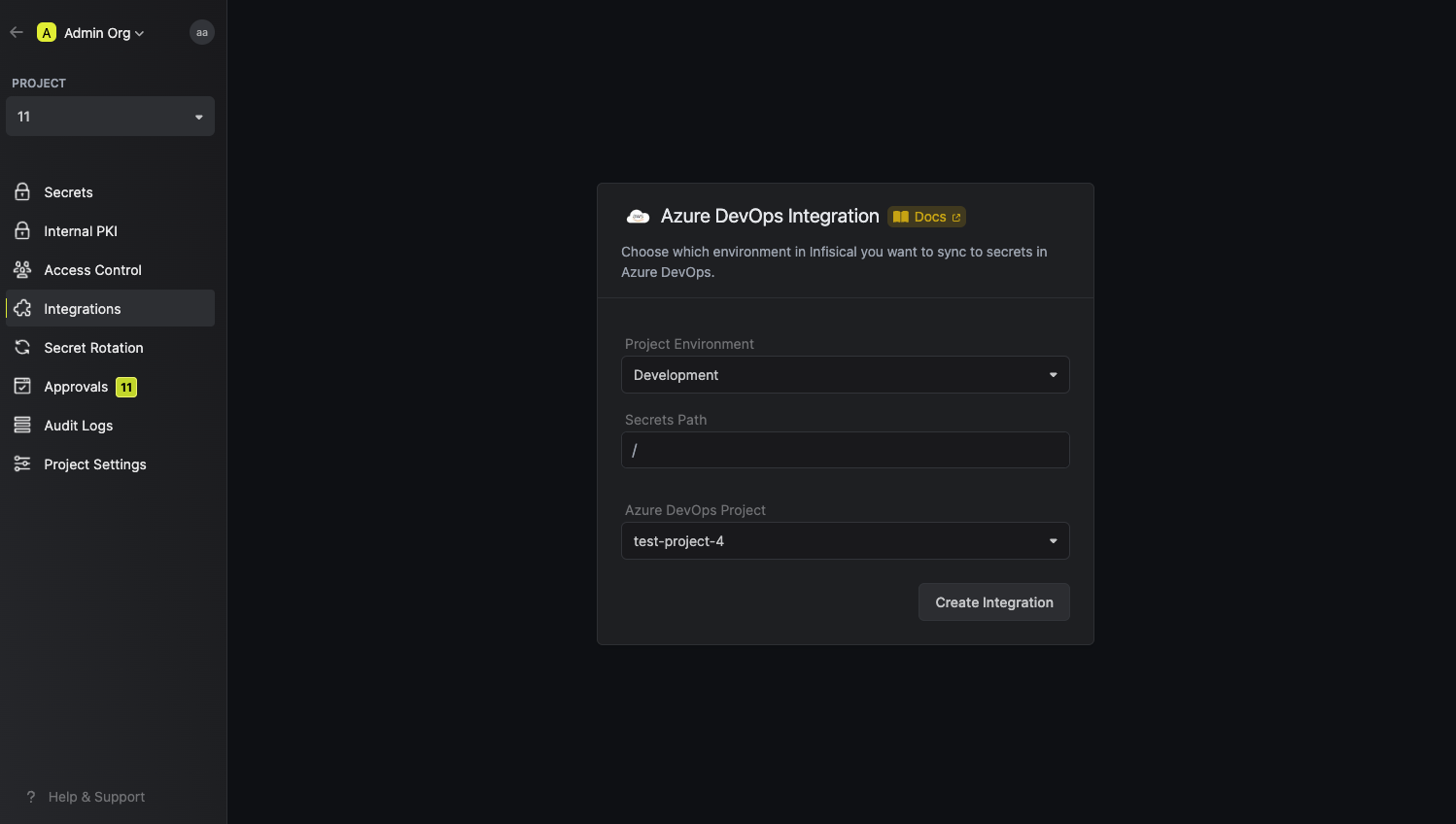
Now you have successfully integrated Infisical with Azure DevOps. Your existing and future secret changes will automatically sync to Azure DevOps.
You can view your secrets by navigating to your Azure DevOps project and selecting the 'Library' tab under 'Pipelines' in the 'Library' section.
# Azure Key Vault
How to sync secrets from Infisical to Azure Key Vault
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
* Set up Azure and have an existing key vault
Navigate to your project's integrations tab
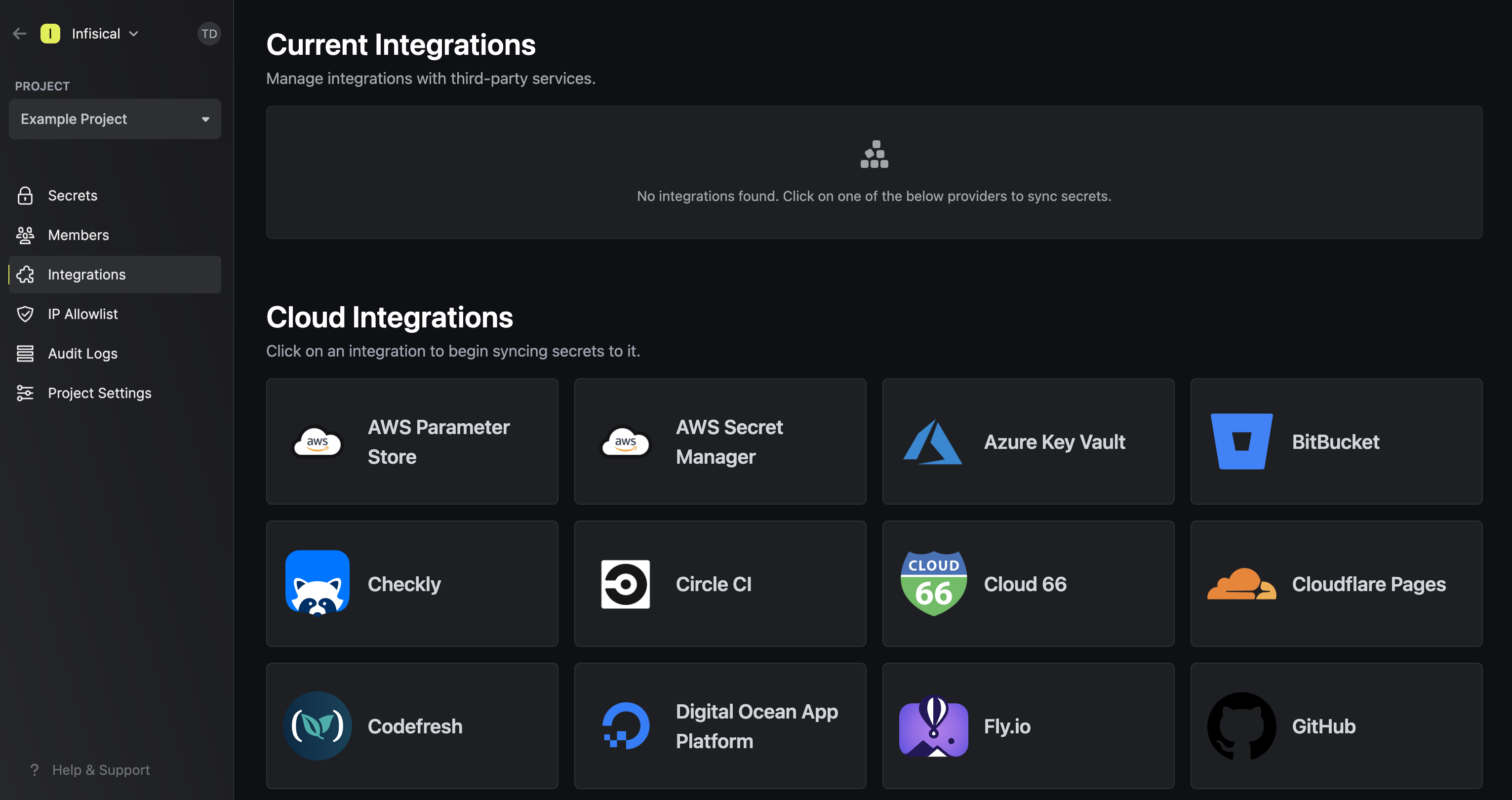
Press on the Azure Key Vault tile and grant Infisical access to Azure Key Vault.
Obtain the Vault URI of your key vault in the Overview tab.
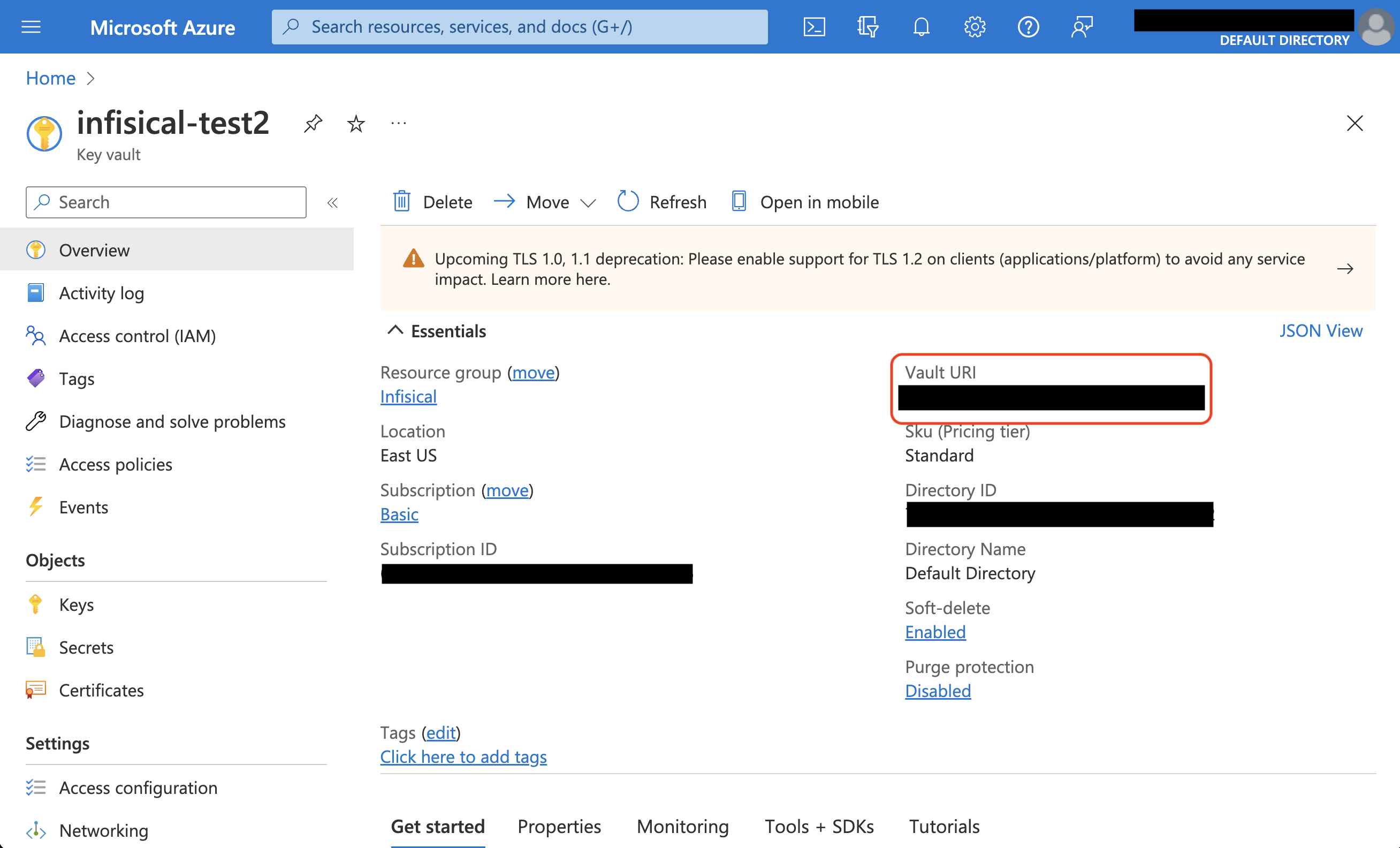
Select which Infisical environment secrets you want to sync to your key vault. Then, input your Vault URI from the previous step. Finally, press create integration to start syncing secrets to Azure Key Vault.
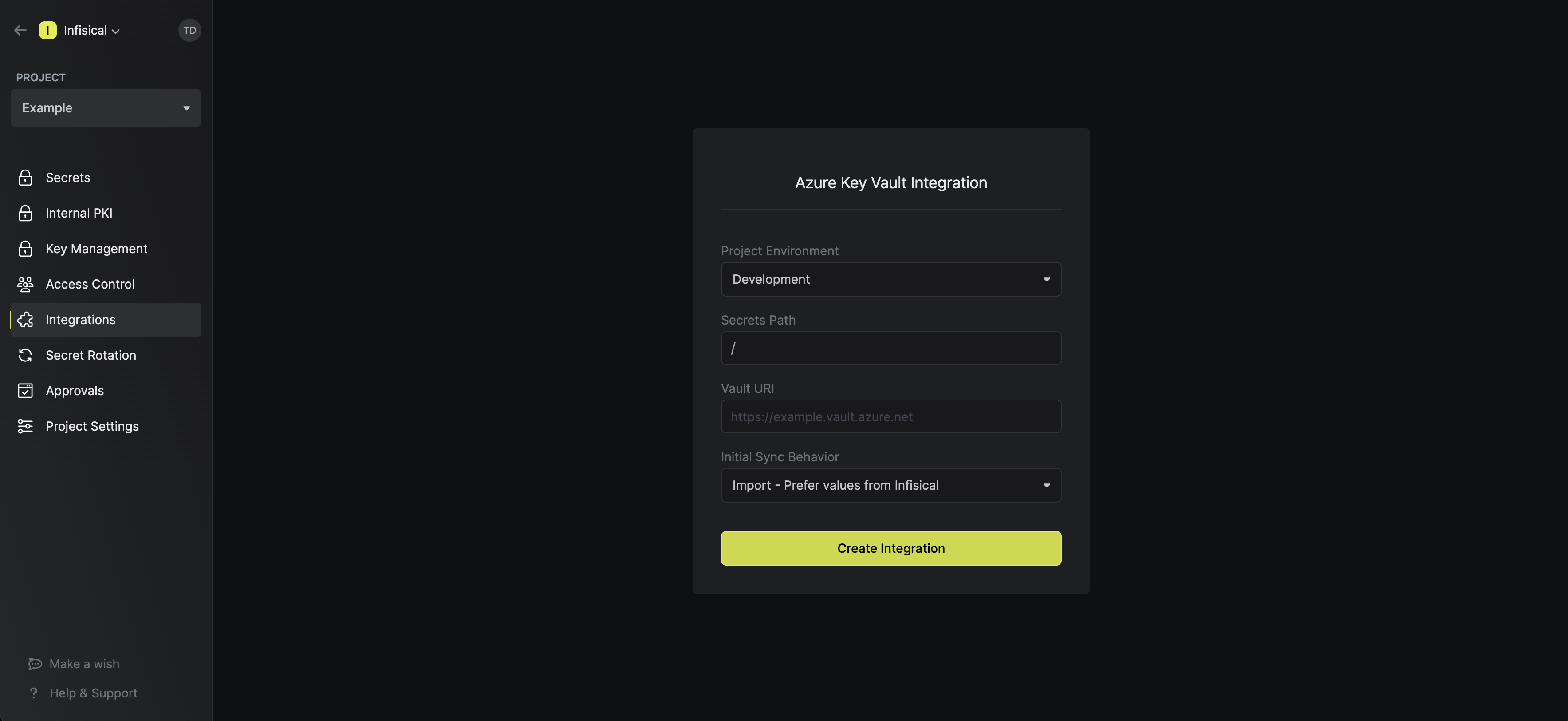
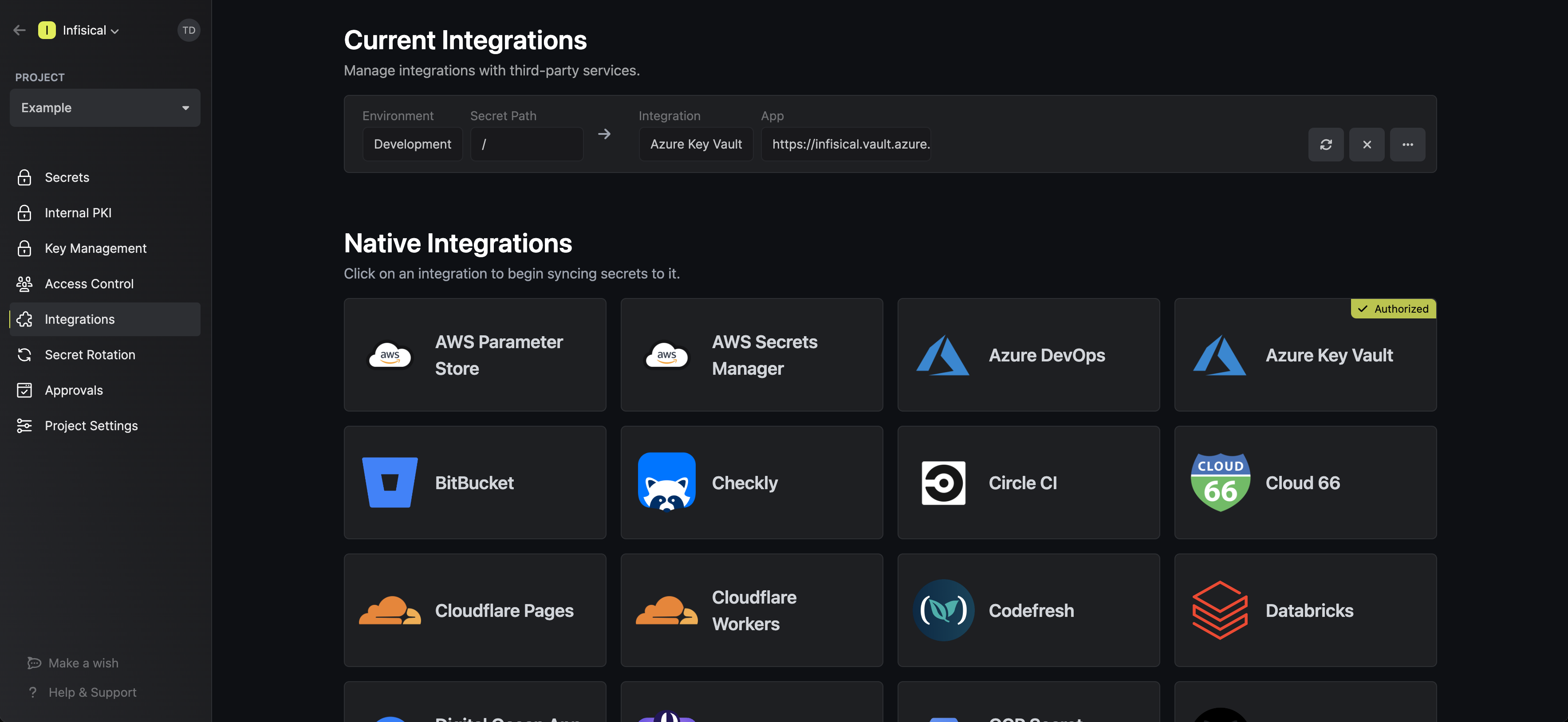
The Azure Key Vault integration requires the following secrets permissions to be set on the user / service principal
for Infisical to sync secrets to Azure Key Vault: `secrets/list`, `secrets/get`, `secrets/set`, `secrets/recover`.
Any role with these permissions would work such as the **Key Vault Secrets Officer** role.
Using the Azure KV integration on a self-hosted instance of Infisical requires configuring an application in Azure
and registering your instance with it.
Navigate to Azure Active Directory > App registrations to create a new application.
Azure Active Directory is now Microsoft Entra ID.
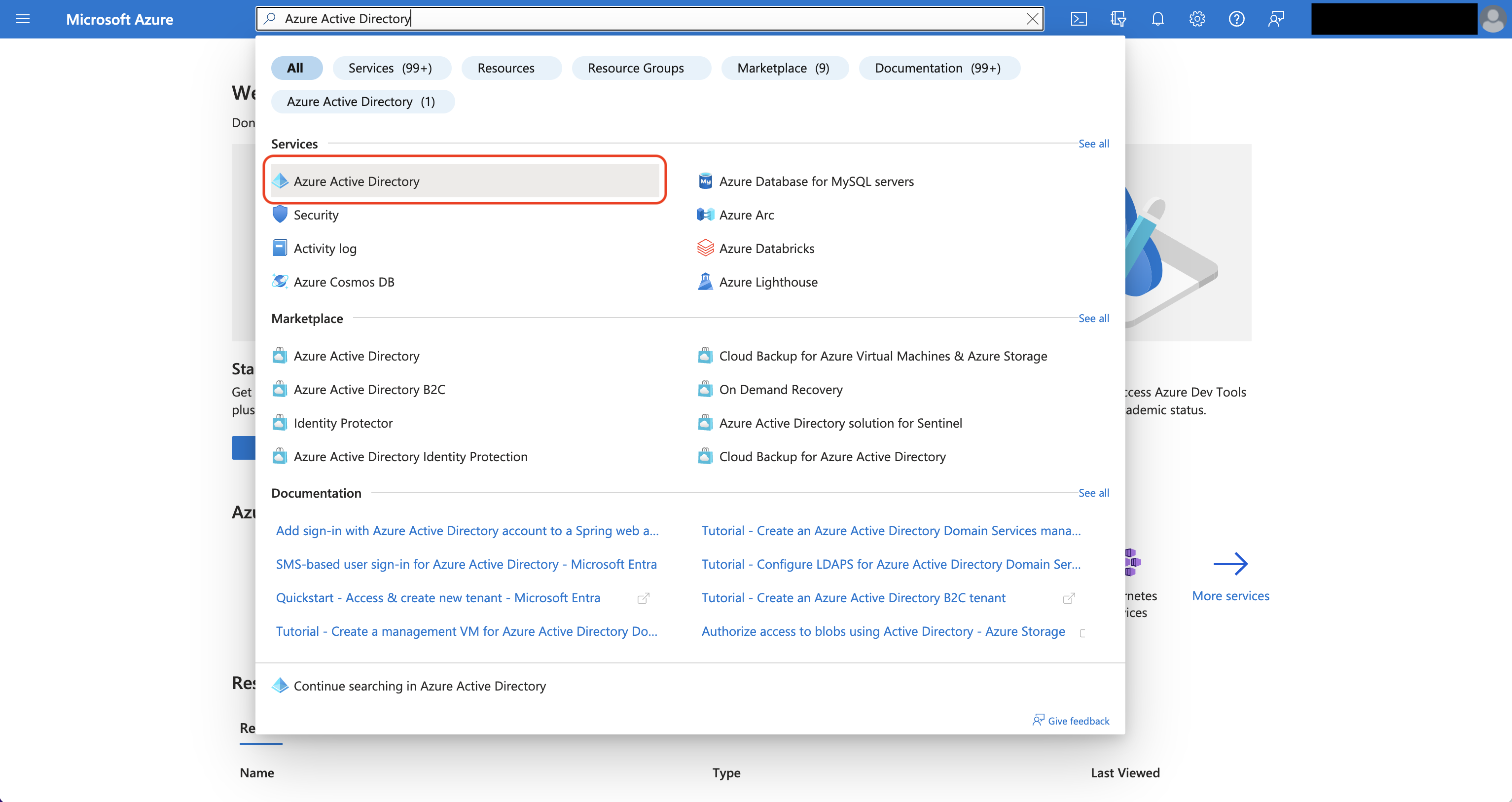
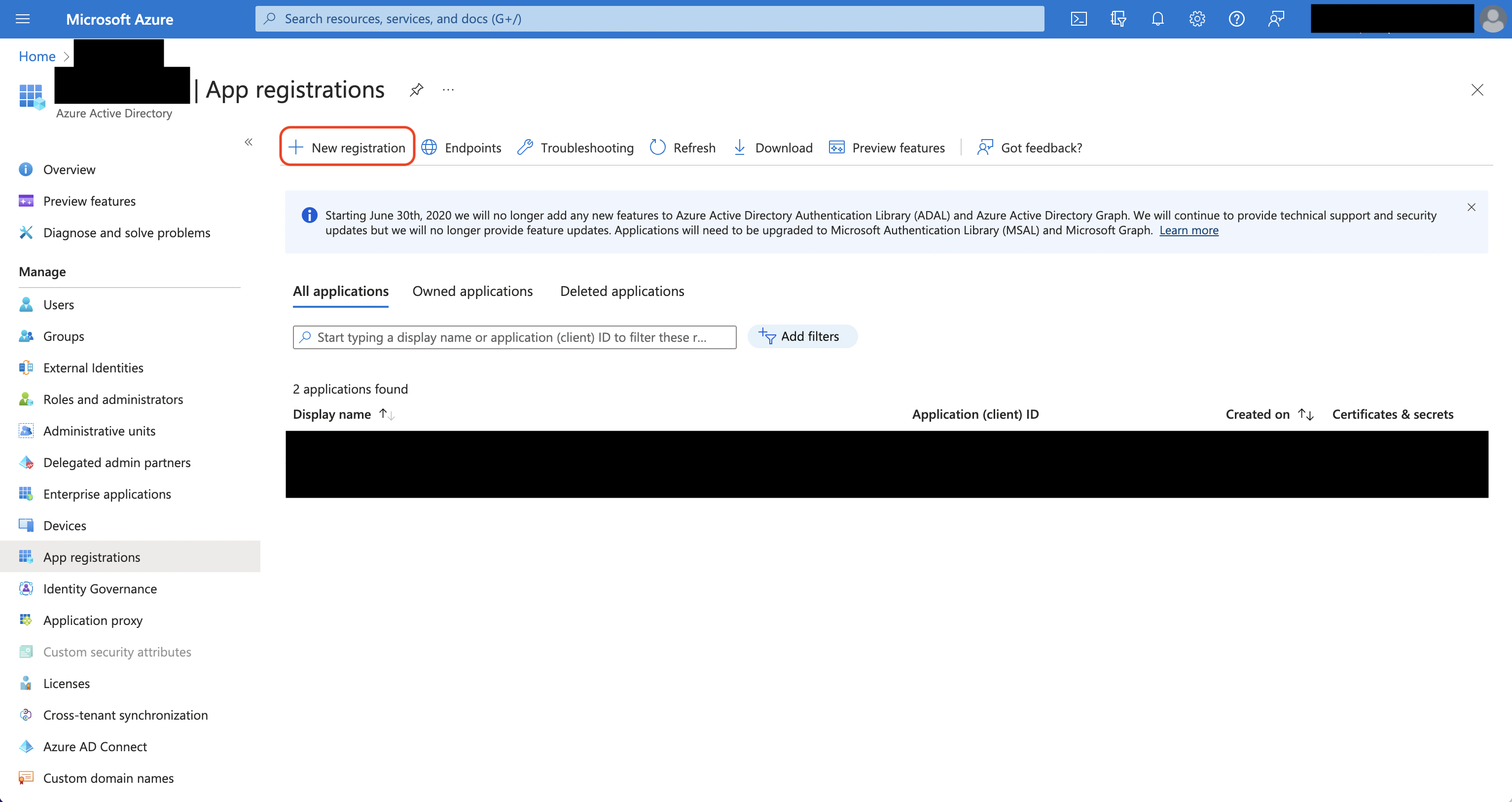
Create the application. As part of the form, set the **Redirect URI** to `https://your-domain.com/integrations/azure-key-vault/oauth2/callback`.
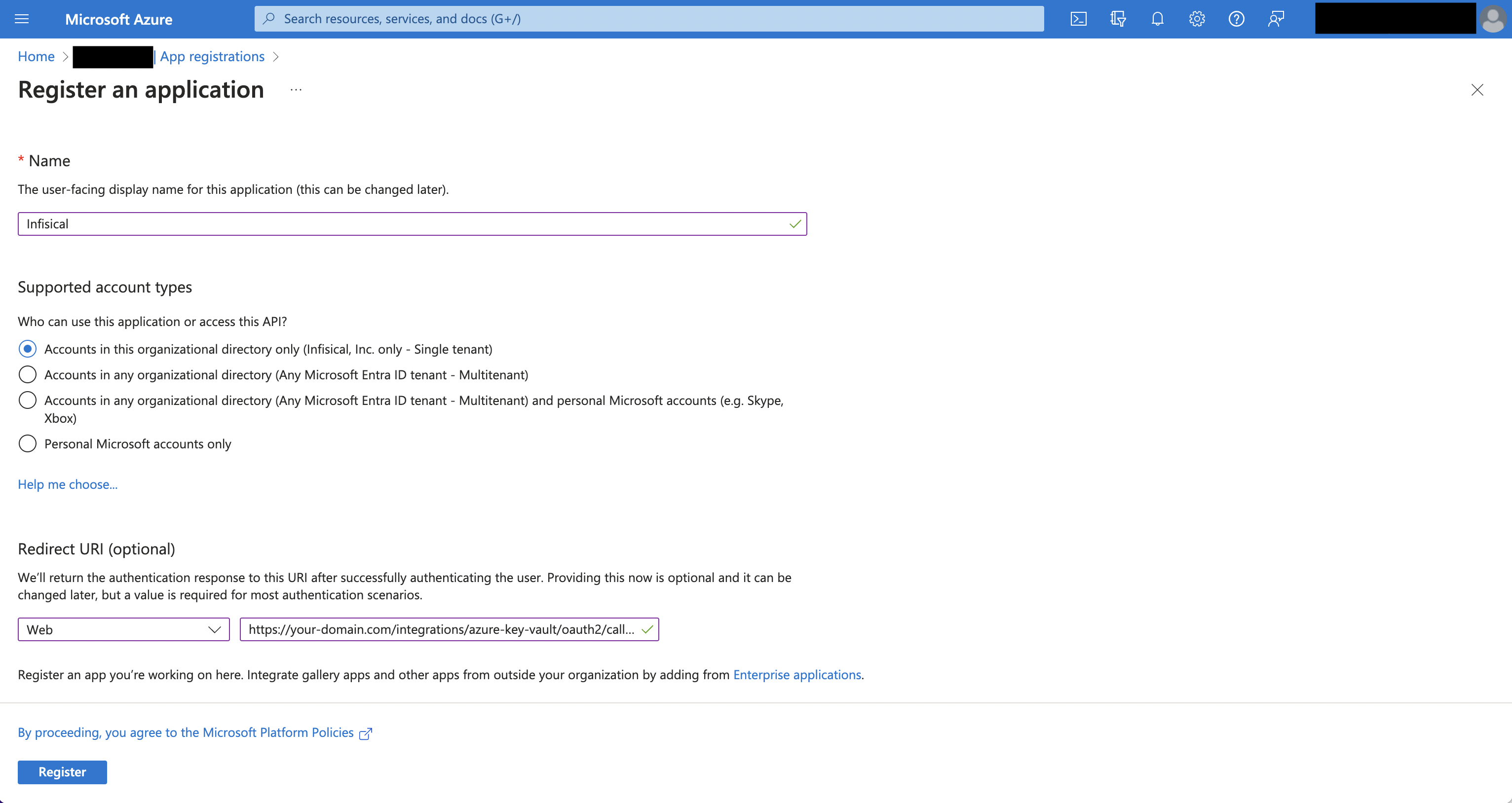
Obtain the **Application (Client) ID** in Overview and generate a **Client Secret** in Certificate & secrets for your Azure application.
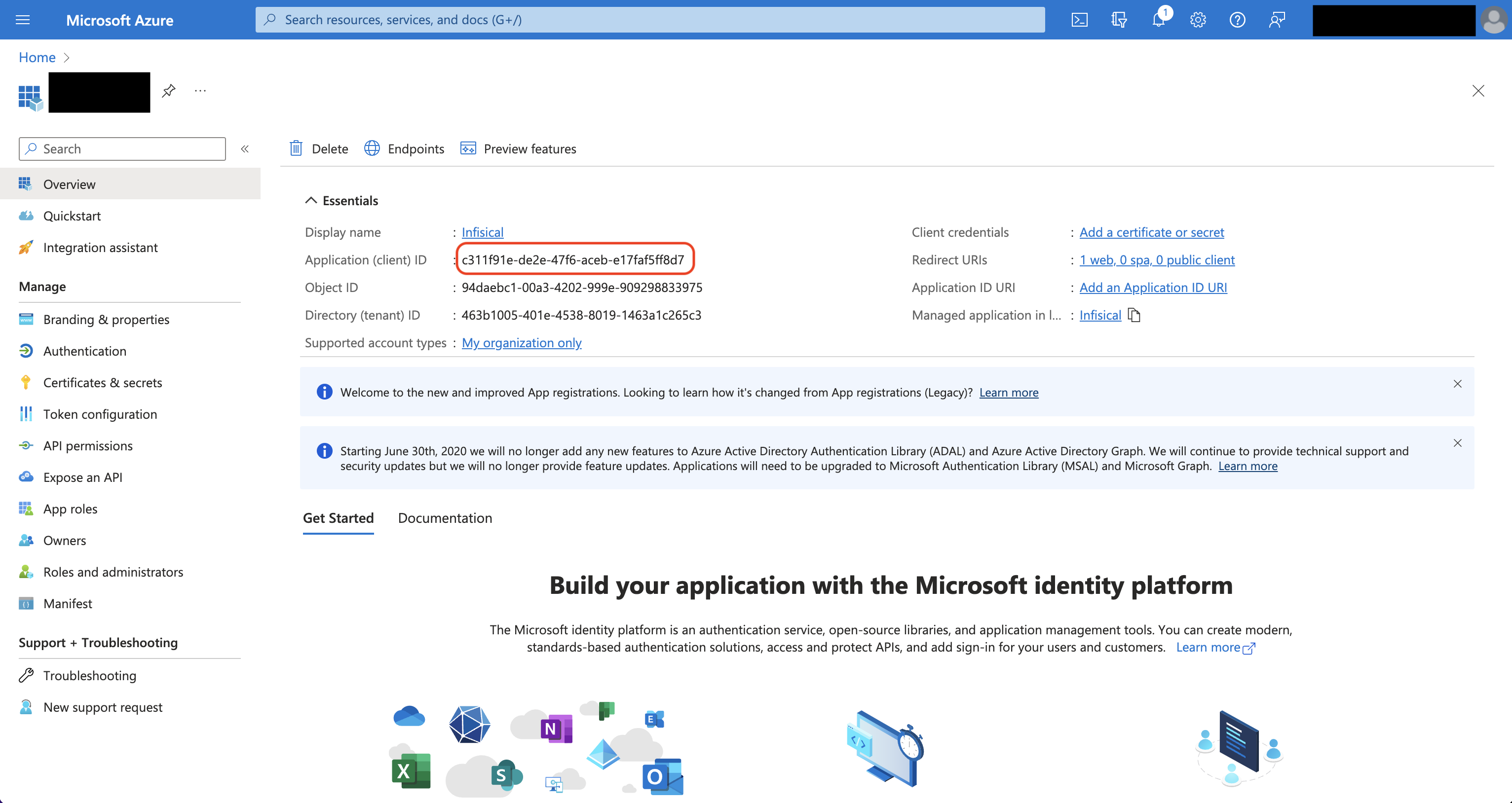
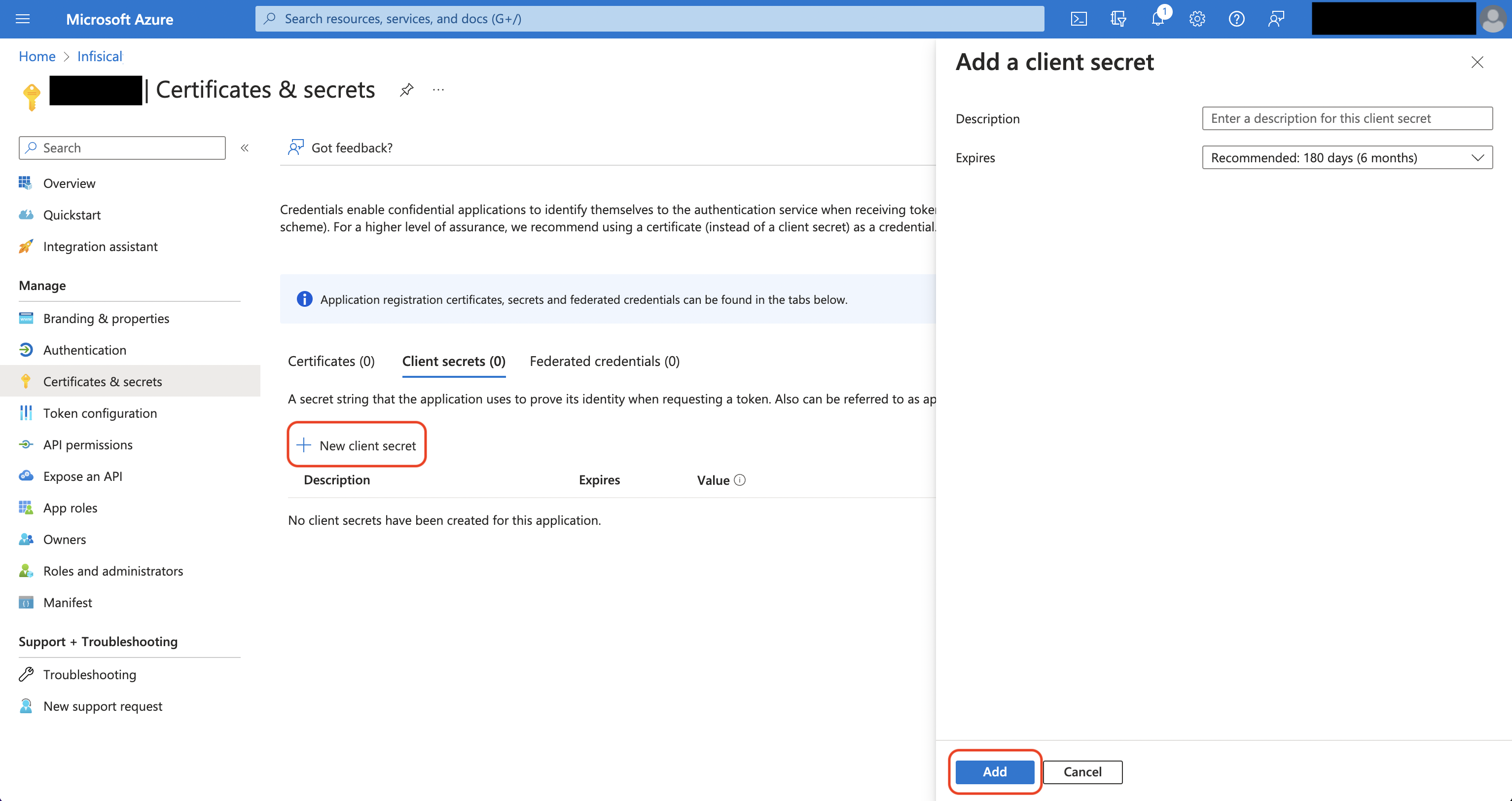
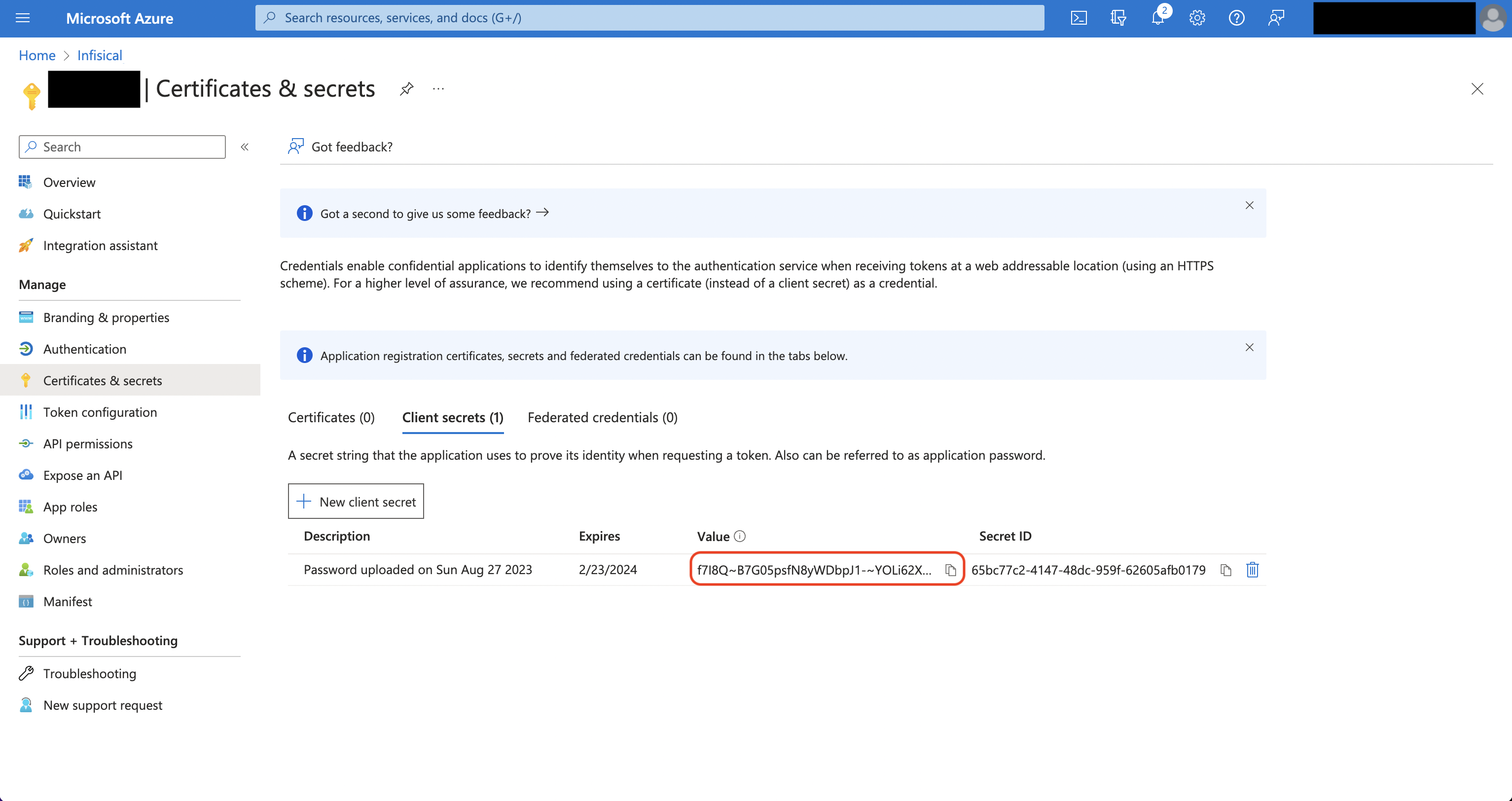
Back in your Infisical instance, add two new environment variables for the credentials of your Azure application.
* `CLIENT_ID_AZURE`: The **Application (Client) ID** of your Azure application.
* `CLIENT_SECRET_AZURE`: The **Client Secret** of your Azure application.
Once added, restart your Infisical instance and use the Azure KV integration.
# Checkly
How to sync secrets from Infisical to Checkly
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
Obtain a Checkly API Key in User Settings > API Keys.
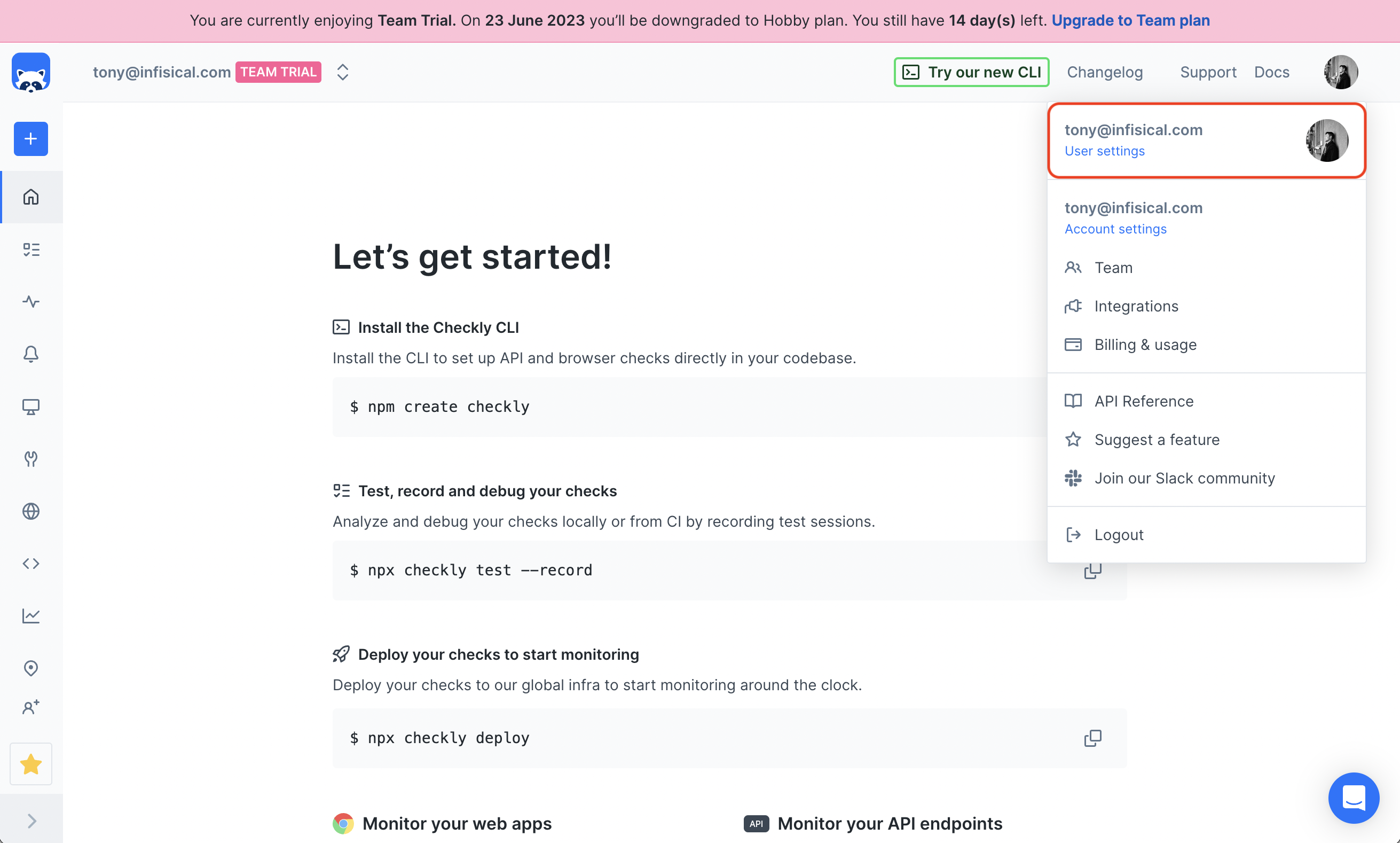
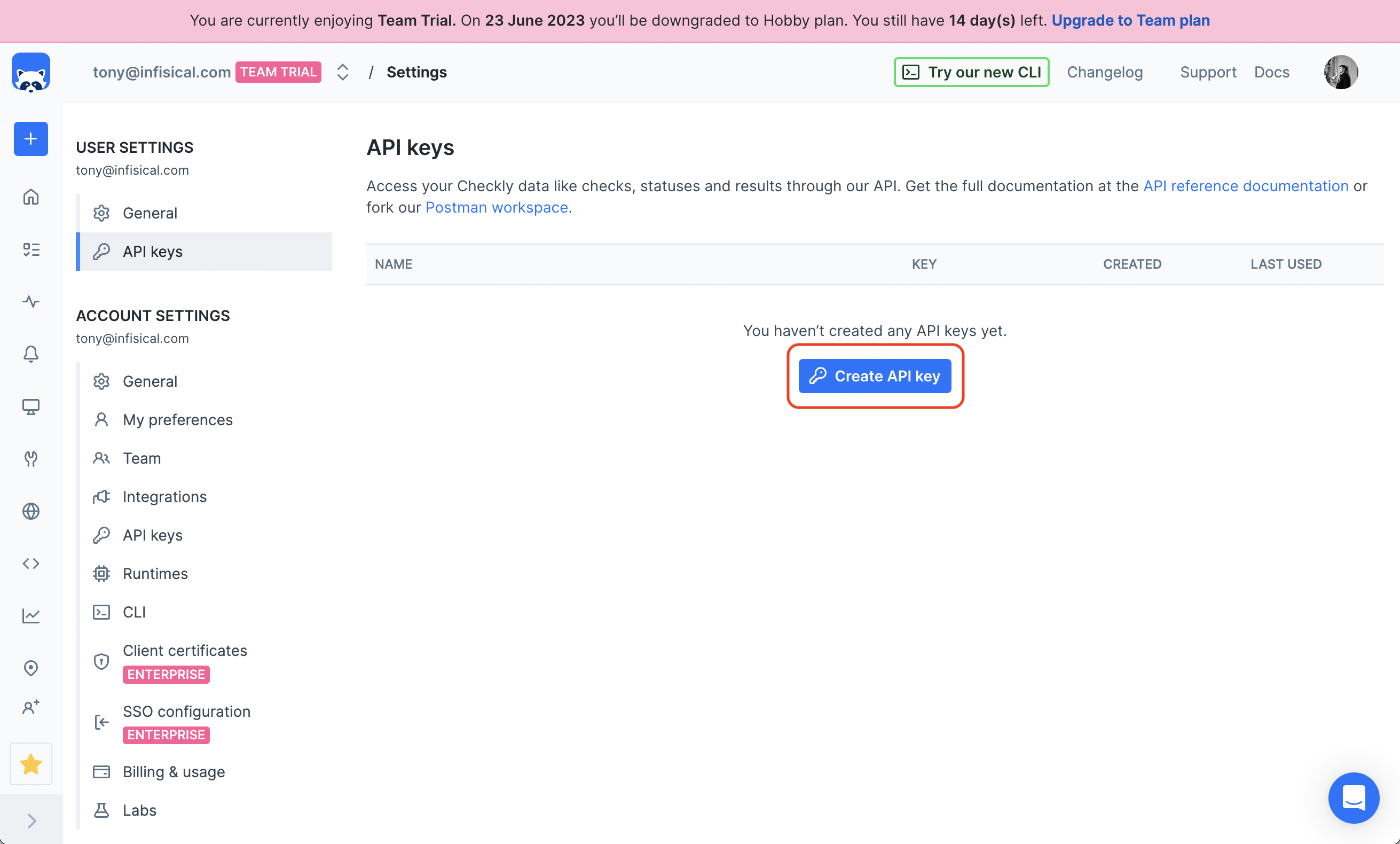
Navigate to your project's integrations tab in Infisical.
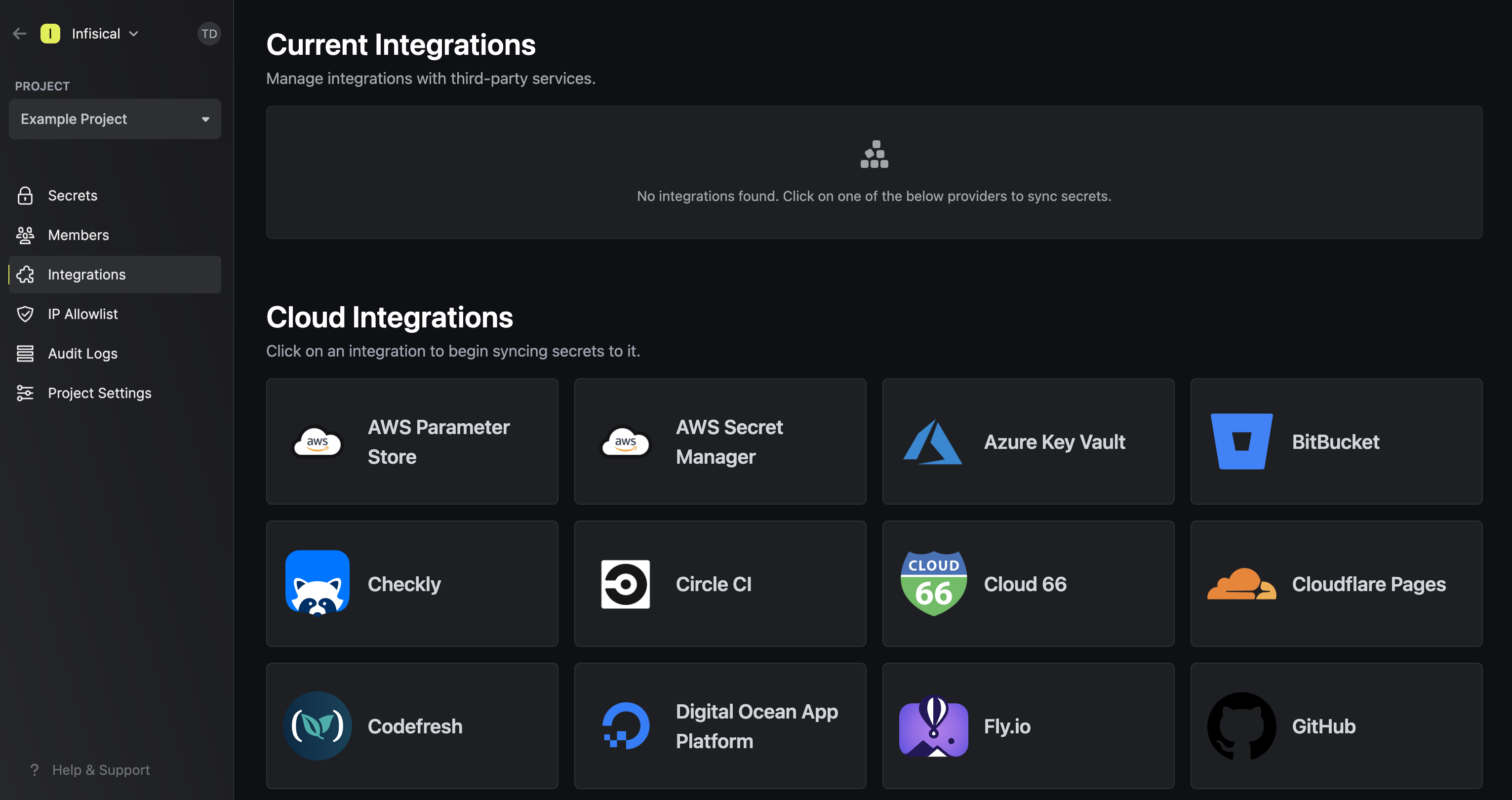
Press on the Checkly tile and input your Checkly API Key to grant Infisical access to your Checkly account.
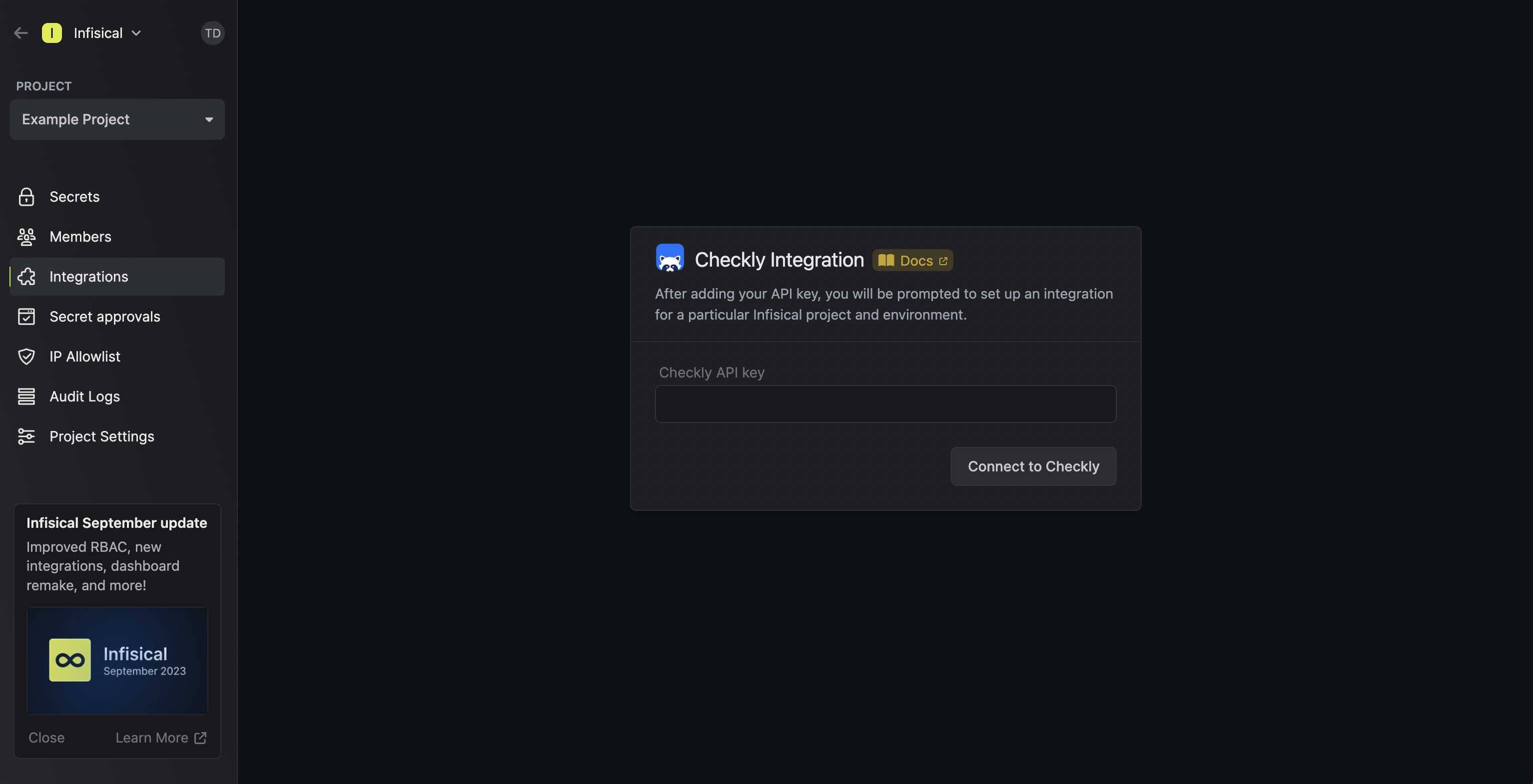
Select which Infisical environment secrets you want to sync to Checkly and press create integration to start syncing secrets.
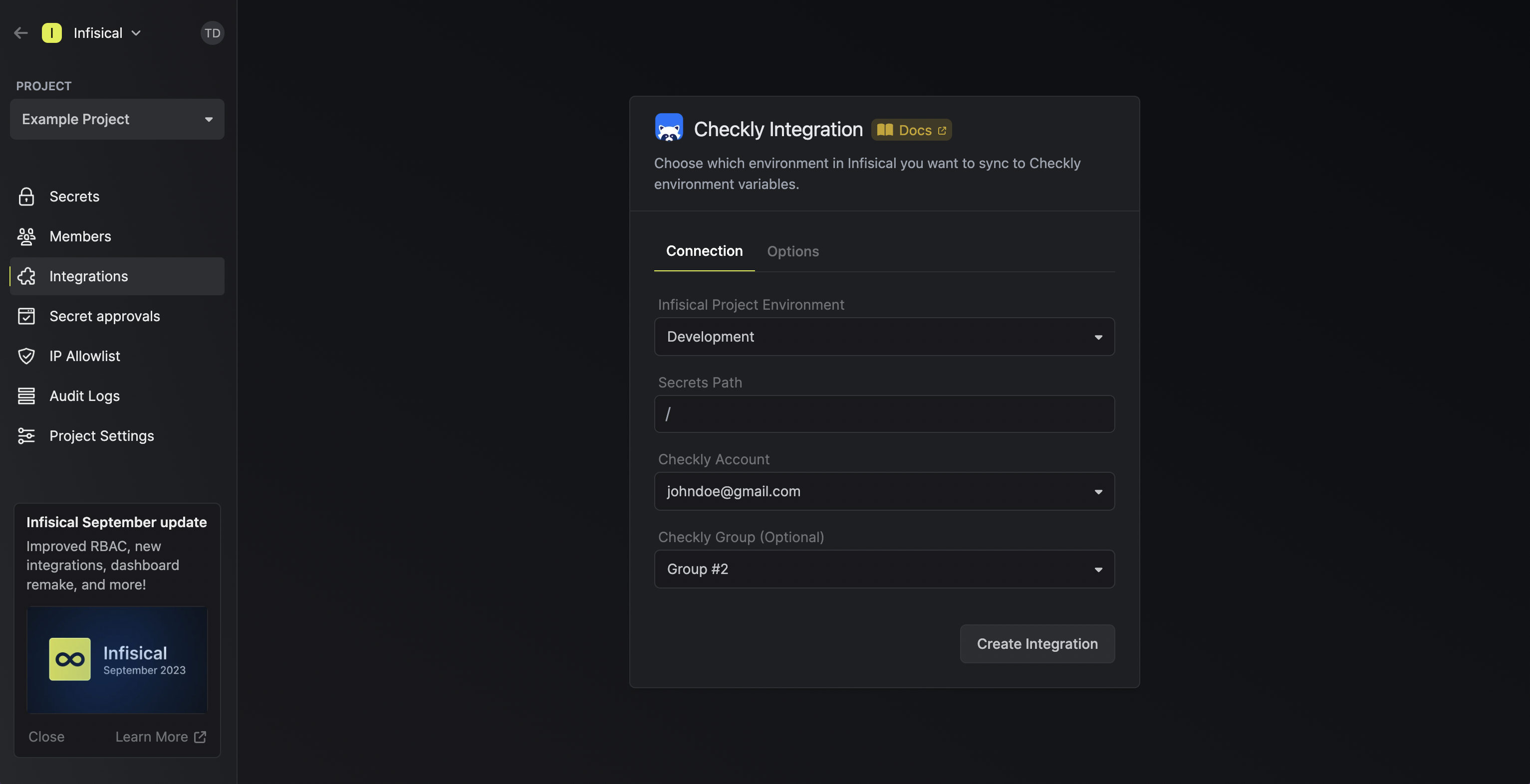
Infisical integrates with Checkly's environment variables at the **global** and **group** levels.
To sync secrets to a specific group, you can select a group from the Checkly Group dropdown; otherwise, leaving it empty will sync secrets globally.
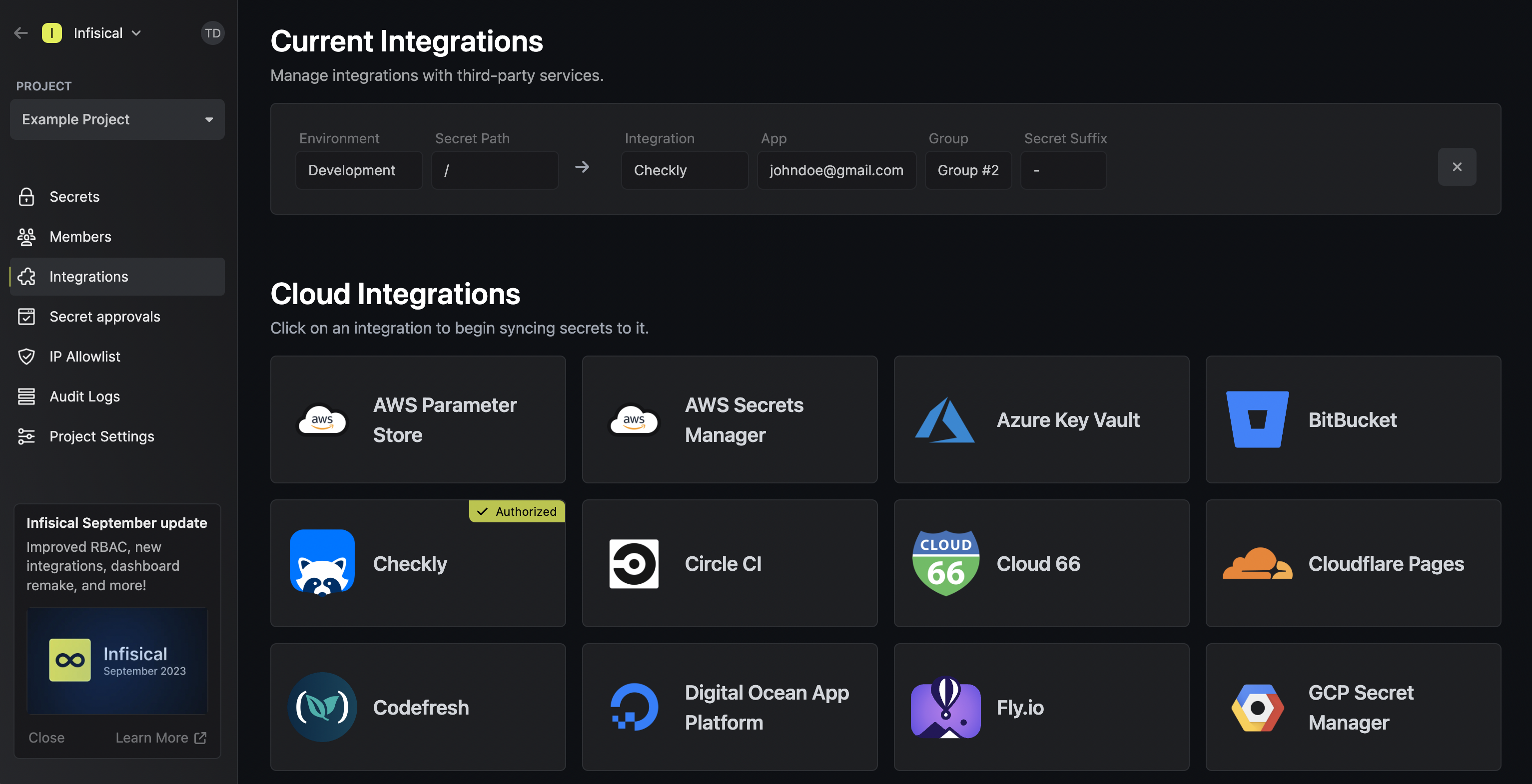
In the new version of the Checkly integration, you are able to specify suffixes that depend on the secrets' environment and path.
If you choose to do so, you should utilize such suffixes for ALL Checkly integrations – otherwise the integration system
might run into issues with deleting secrets from the wrong environments.
# Cloud 66
How to sync secrets from Infisical to Cloud 66
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
## Navigate to your project's integrations tab
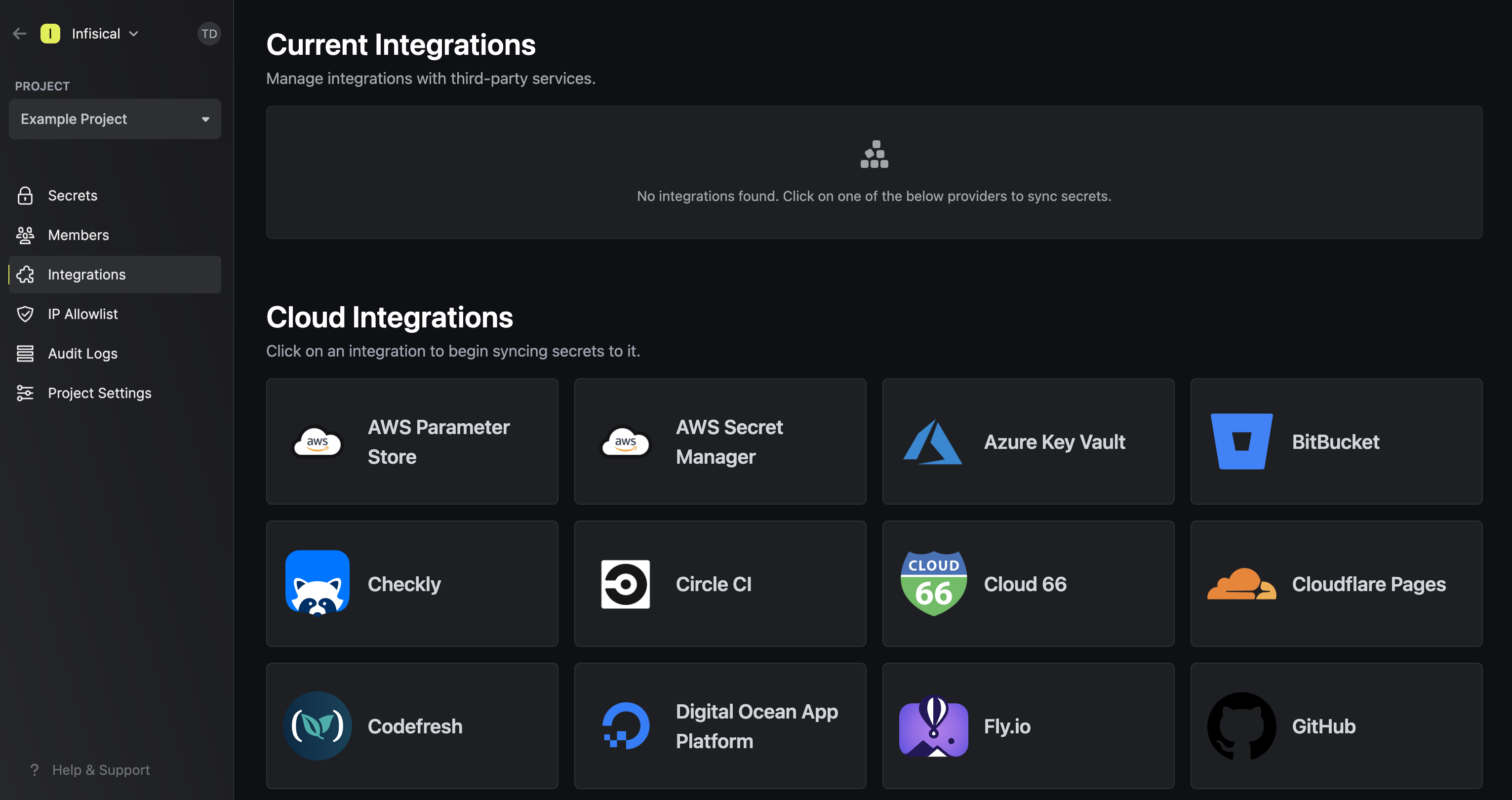
## Enter your Cloud 66 Access Token
In Cloud 66 Dashboard, click on the top right icon > Account Settings > Access Token
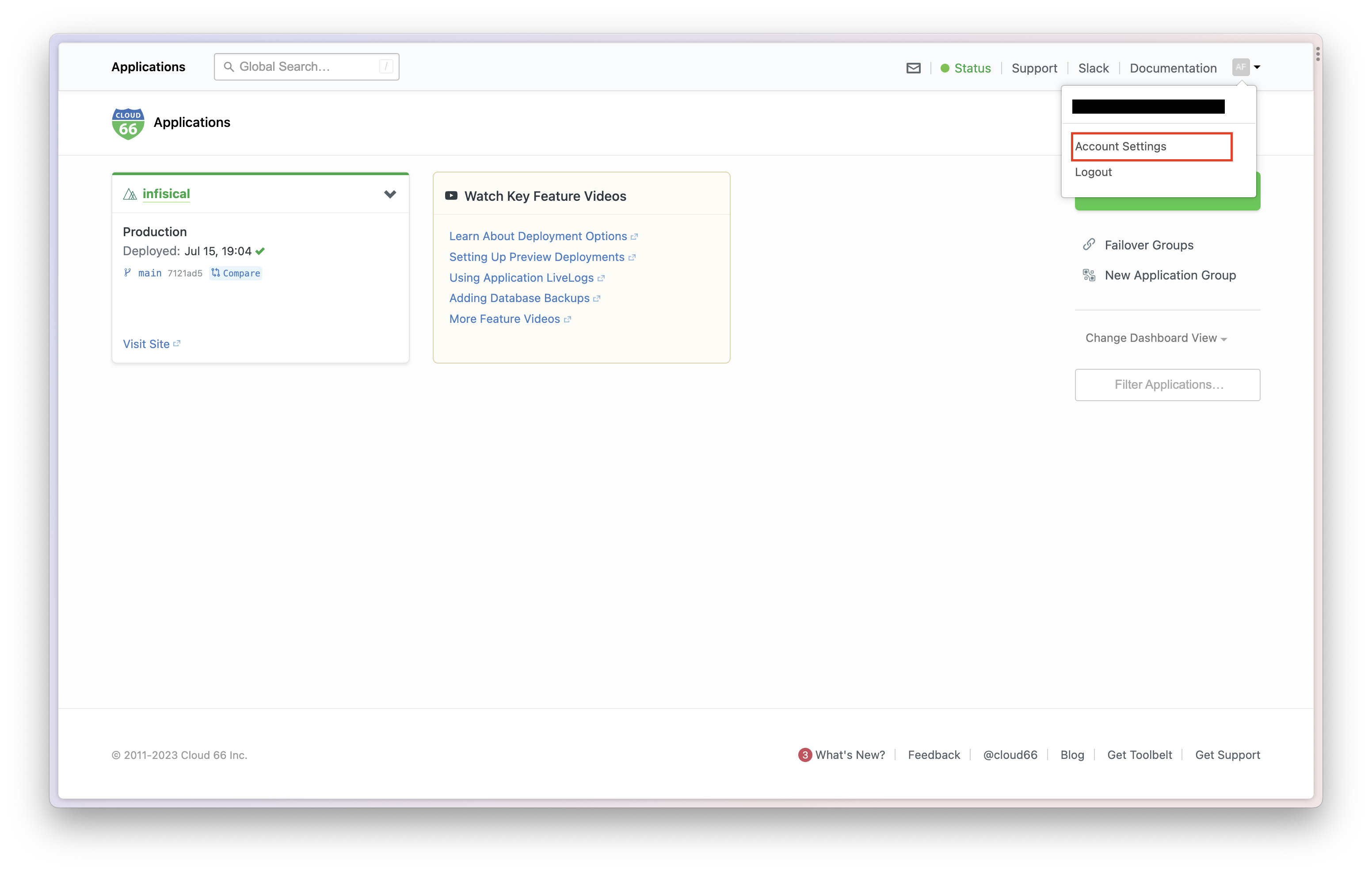
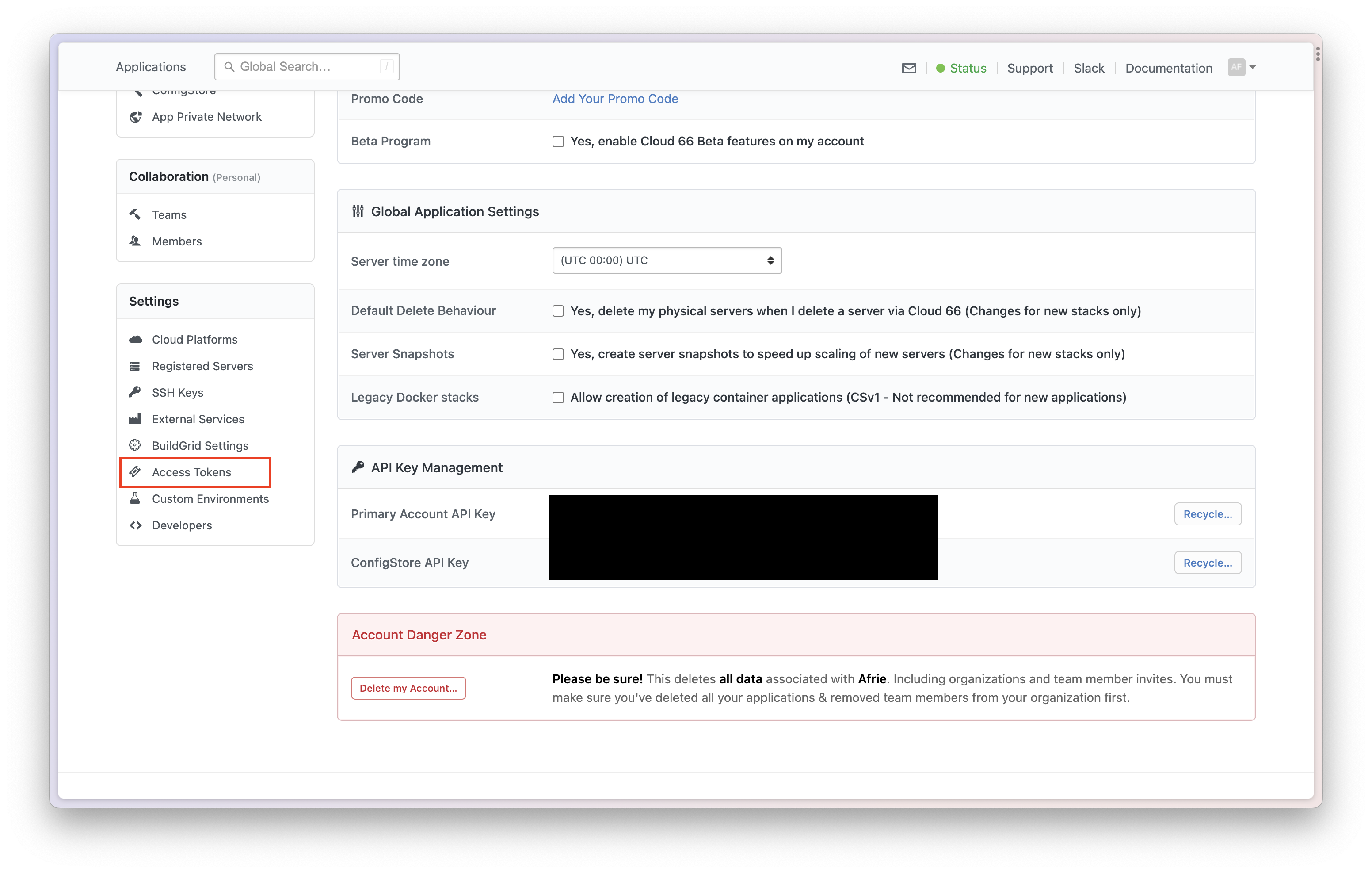
Create new Personal Access Token.
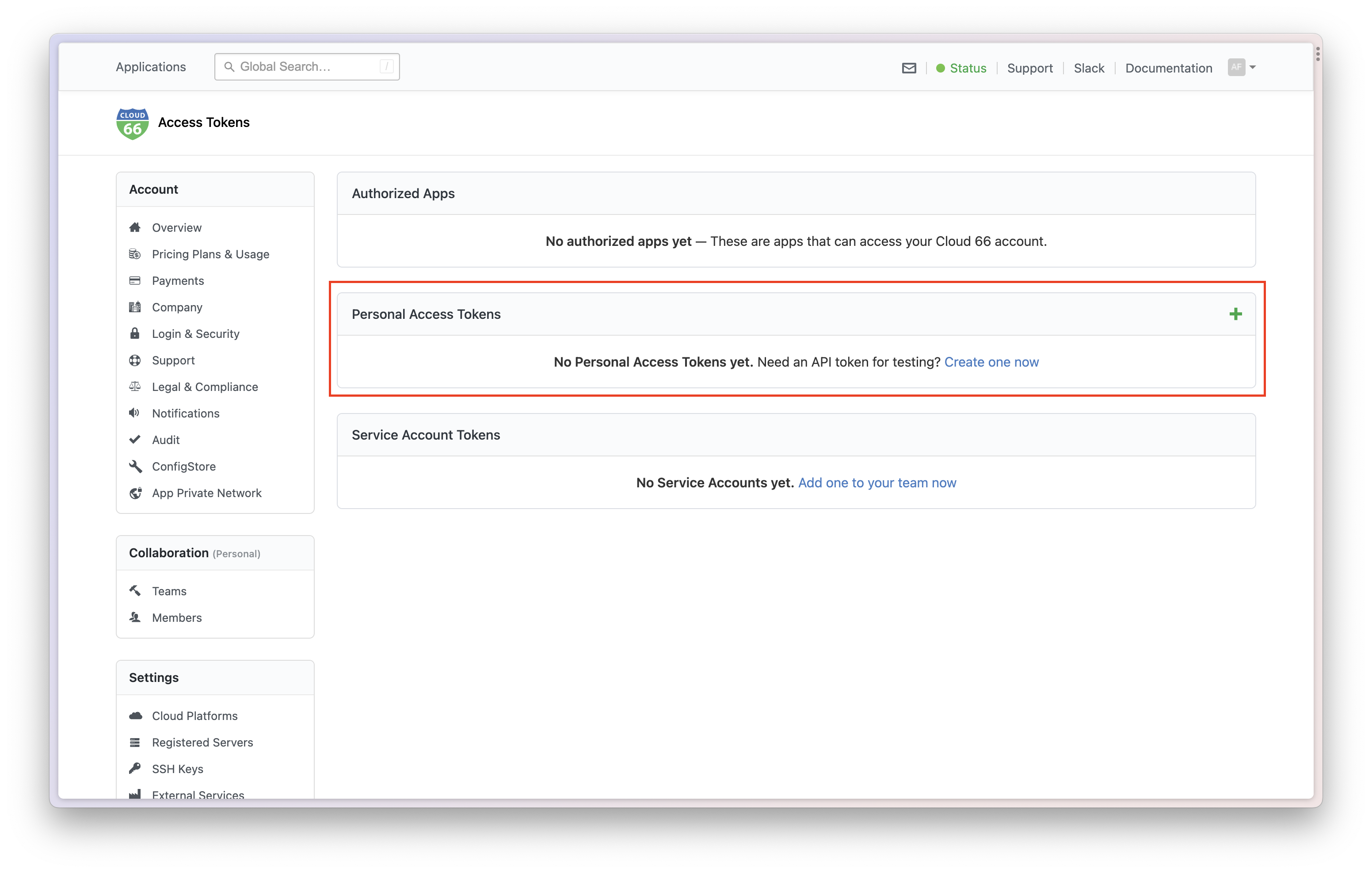
Name it **infisical** and check **Public** and **Admin**. Then click "Create Token"
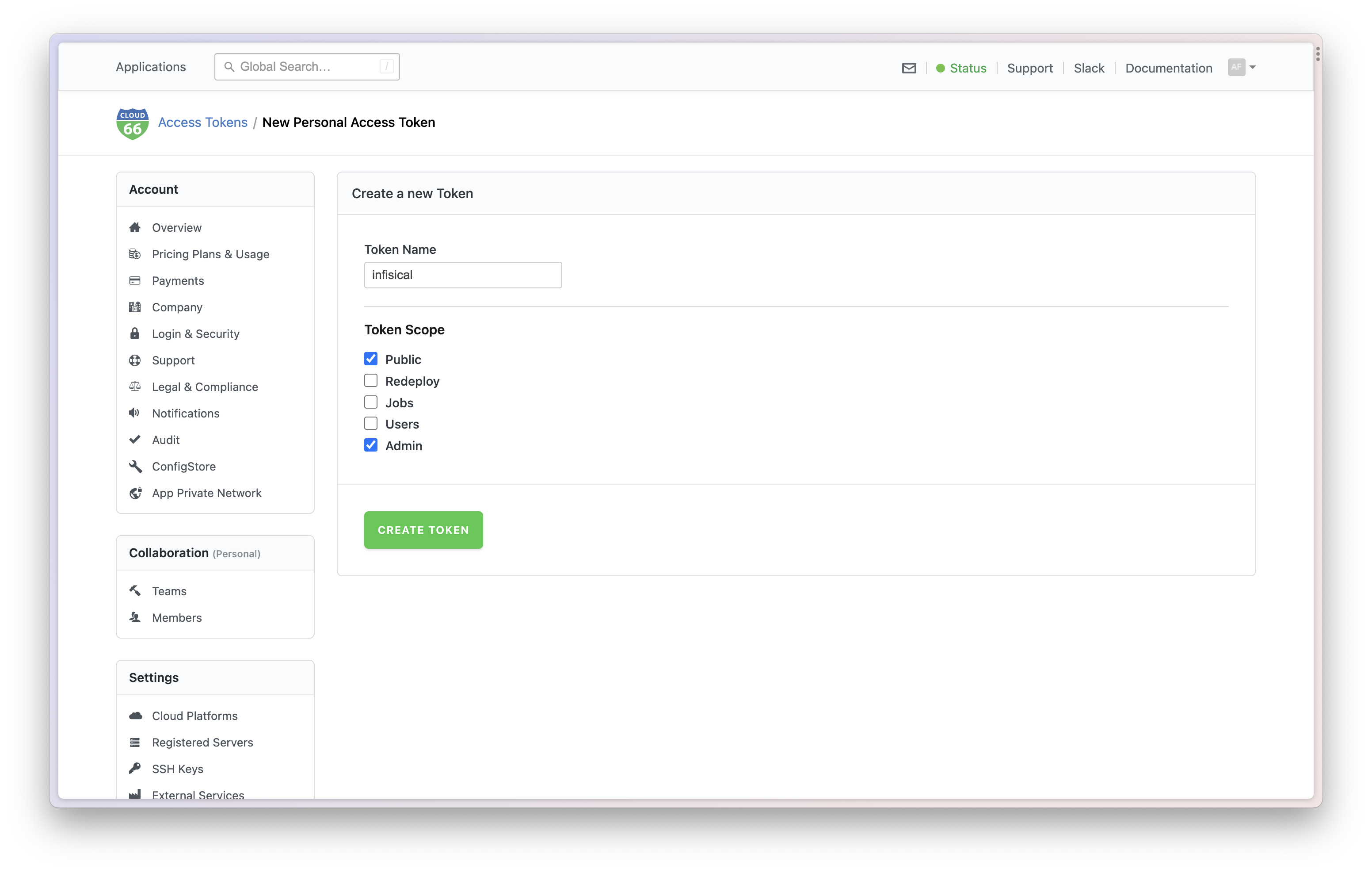
Copy and save your token.
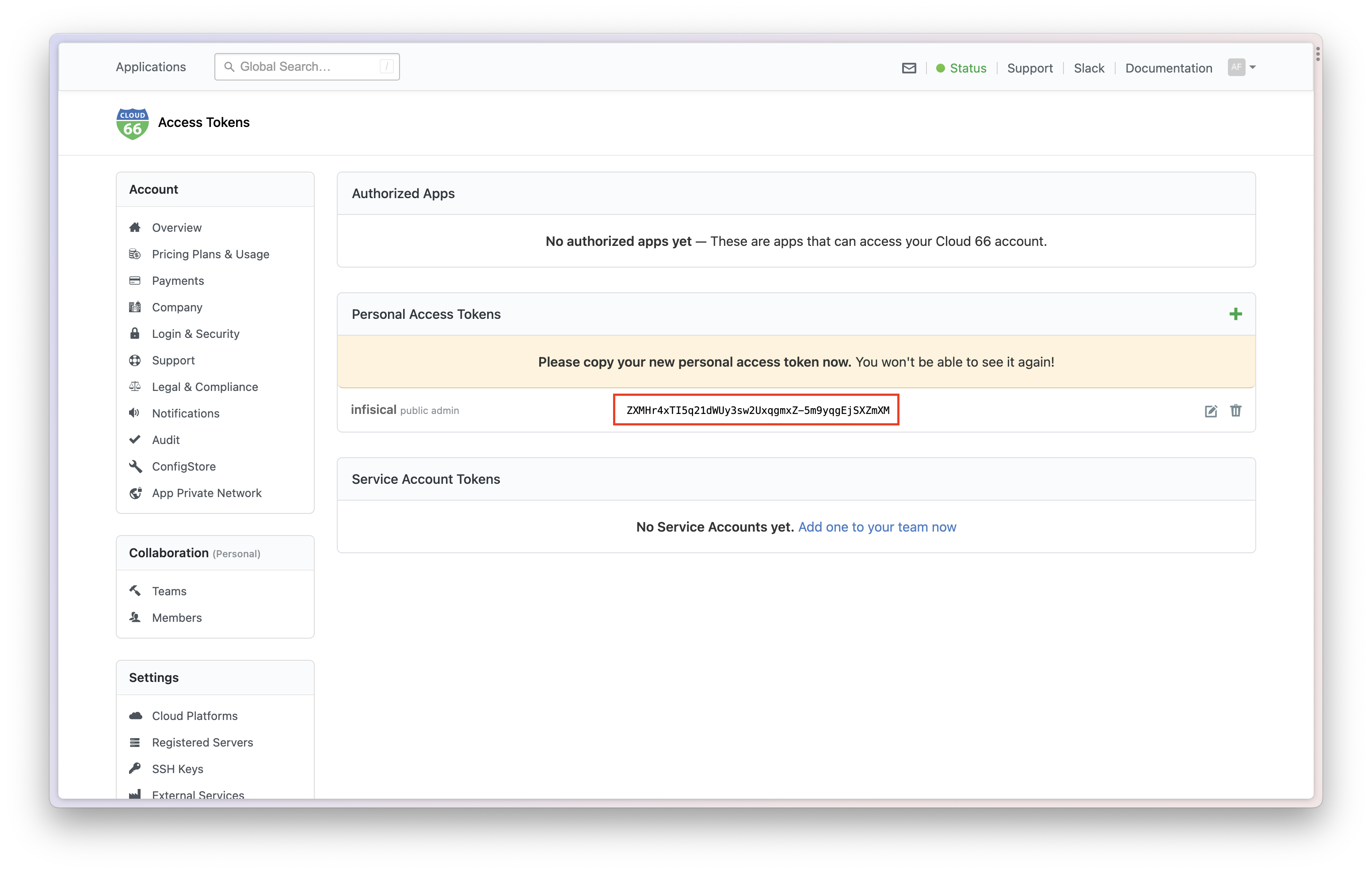
### Go to Infisical Integration Page
Click on the Cloud 66 tile and enter your API token to grant Infisical access to your Cloud 66 account.
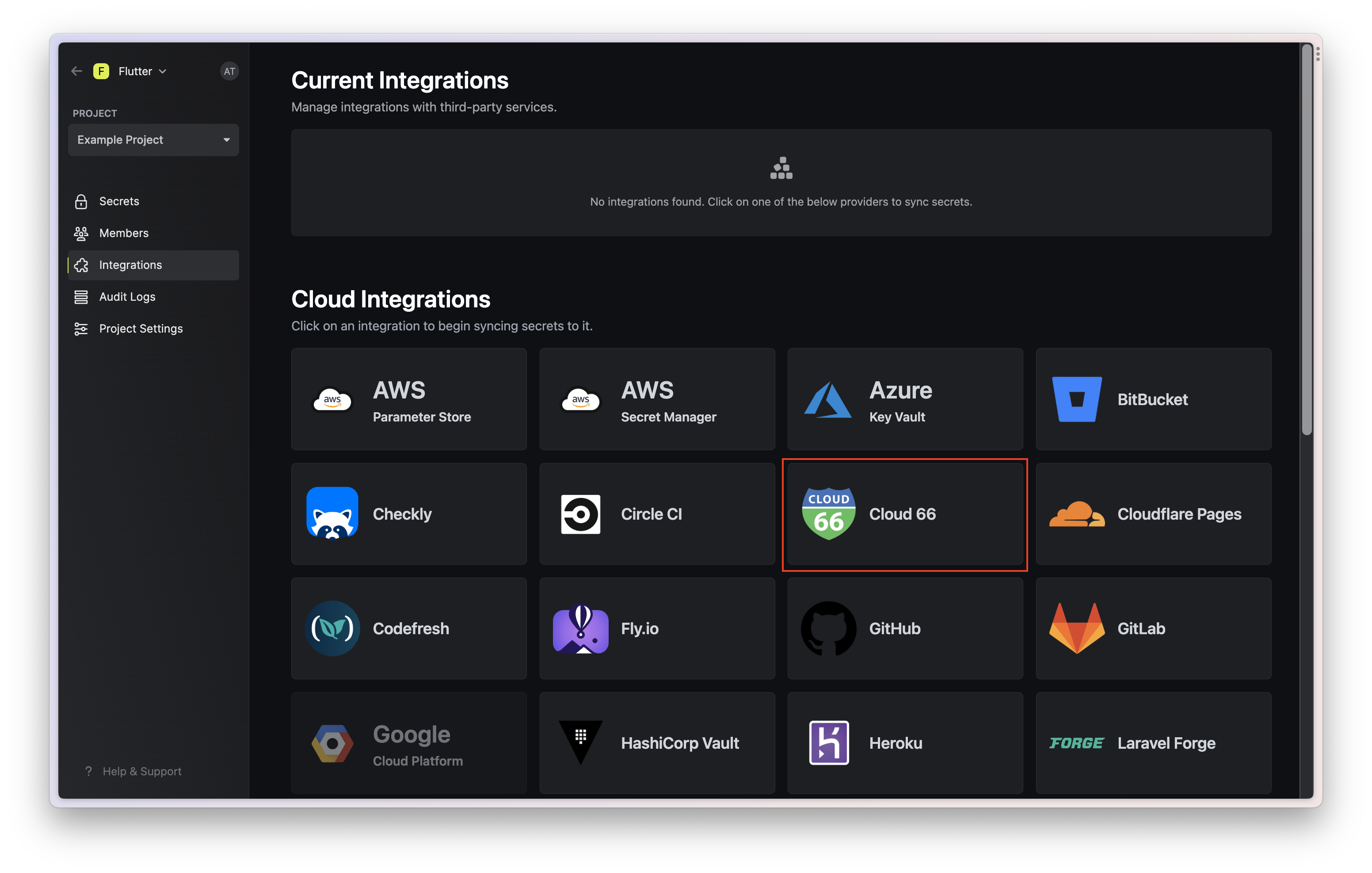
Enter your Cloud 66 Personal Access Token here. Then click "Connect to Cloud 66".
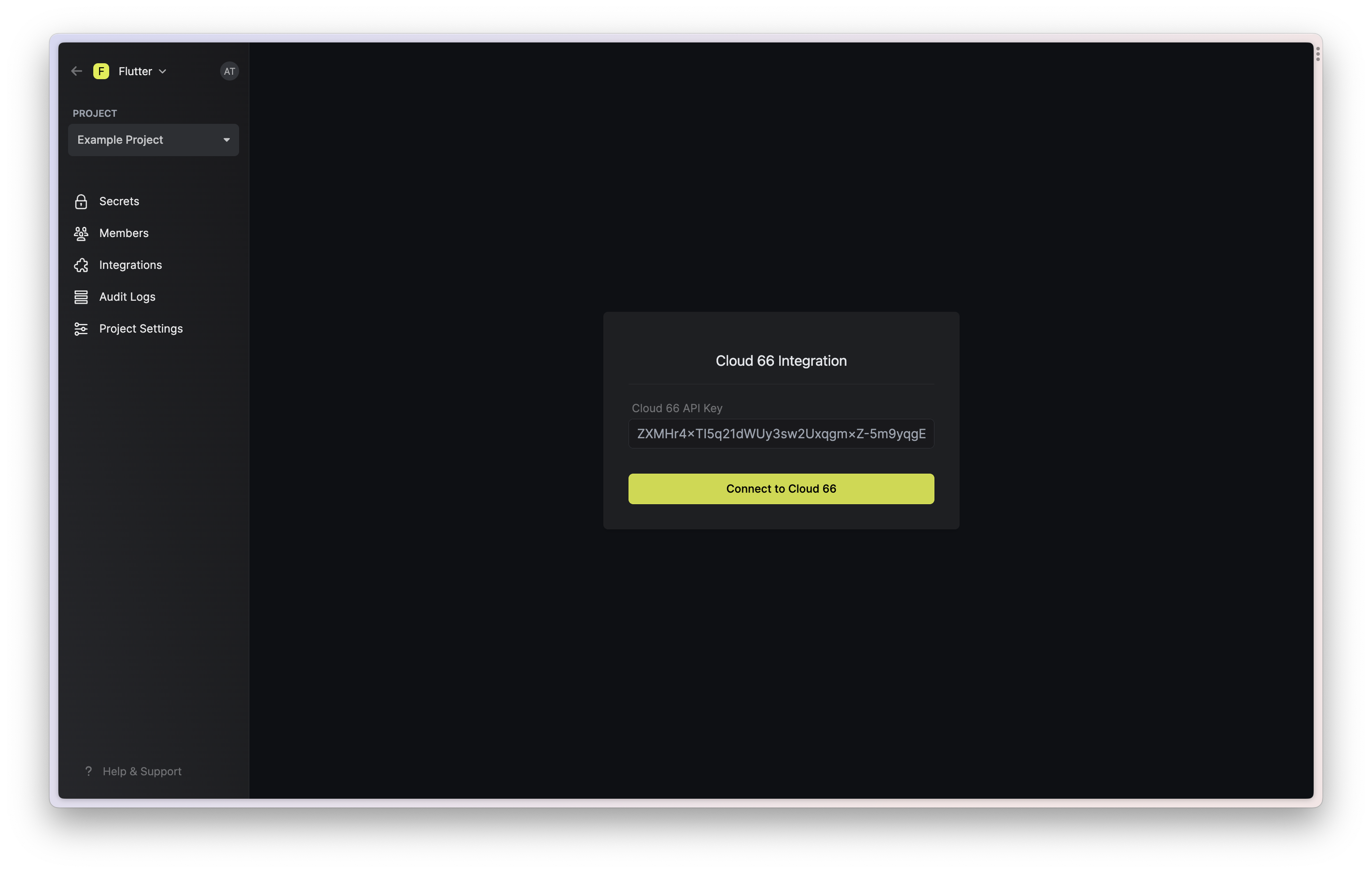
## Start integration
Select which Infisical environment secrets you want to sync to which Cloud 66 stacks and press create integration to start syncing secrets to Cloud 66.
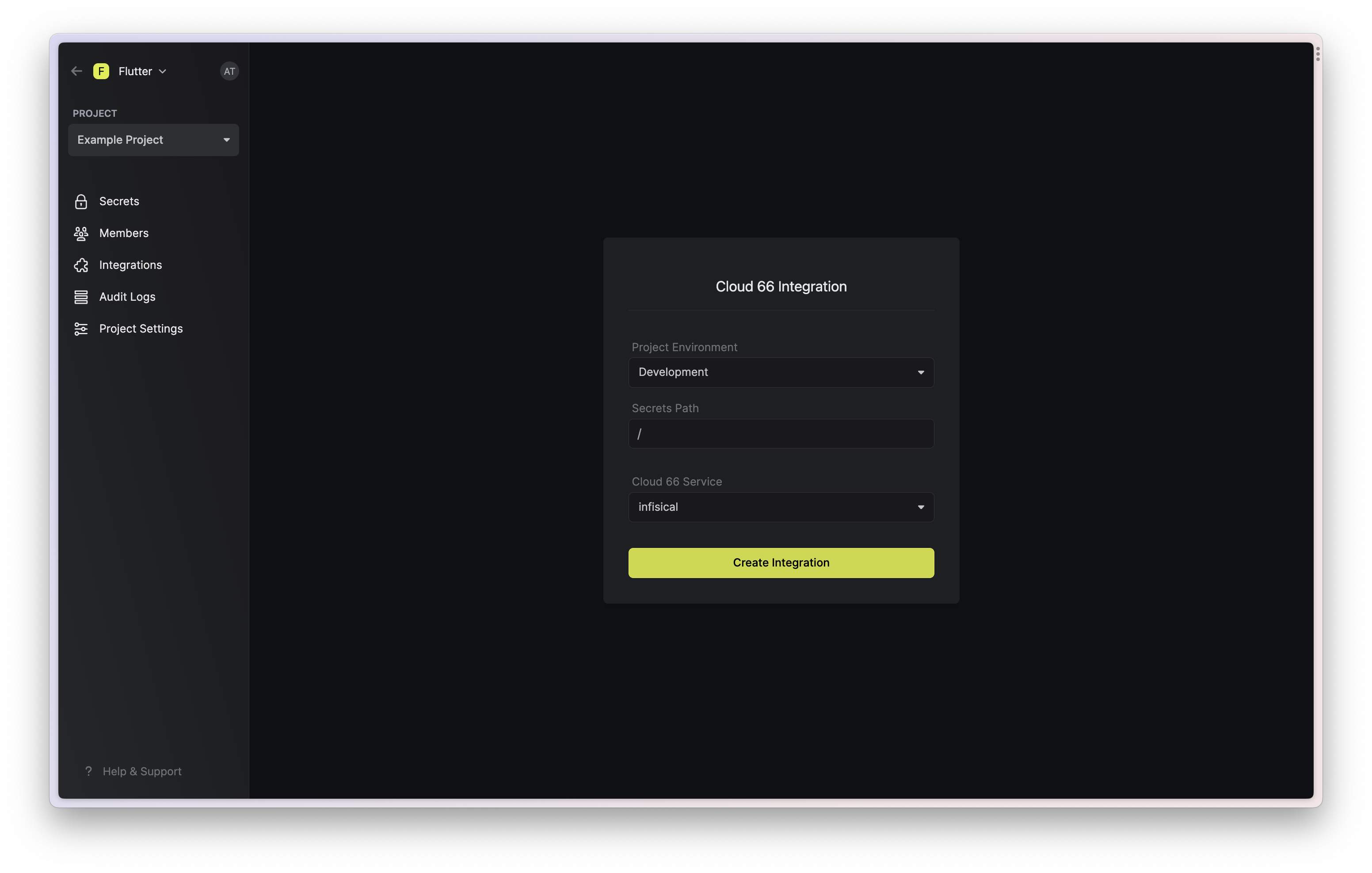
Any existing environment variables in Cloud 66 will be deleted when you start syncing. Make sure to add all the secrets into the Infisical dashboard first before doing any integrations.
Done!
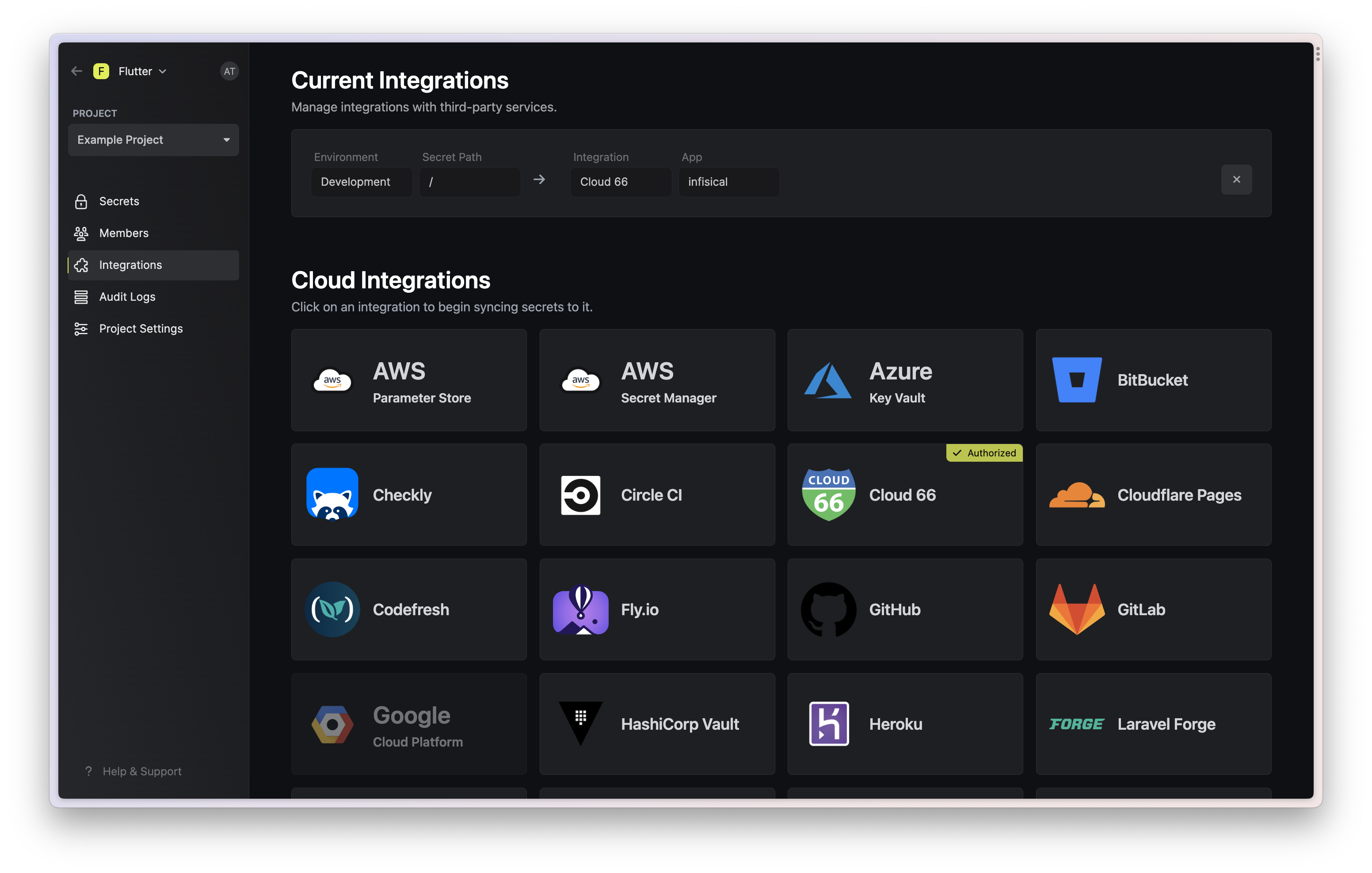
# Cloudflare Pages
How to sync secrets from Infisical to Cloudflare Pages
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
Obtain a Cloudflare [API token](https://dash.cloudflare.com/profile/api-tokens) and [Account ID](https://developers.cloudflare.com/fundamentals/get-started/basic-tasks/find-account-and-zone-ids/):
Create a new [API token](https://dash.cloudflare.com/profile/api-tokens) in My Profile > API Tokens
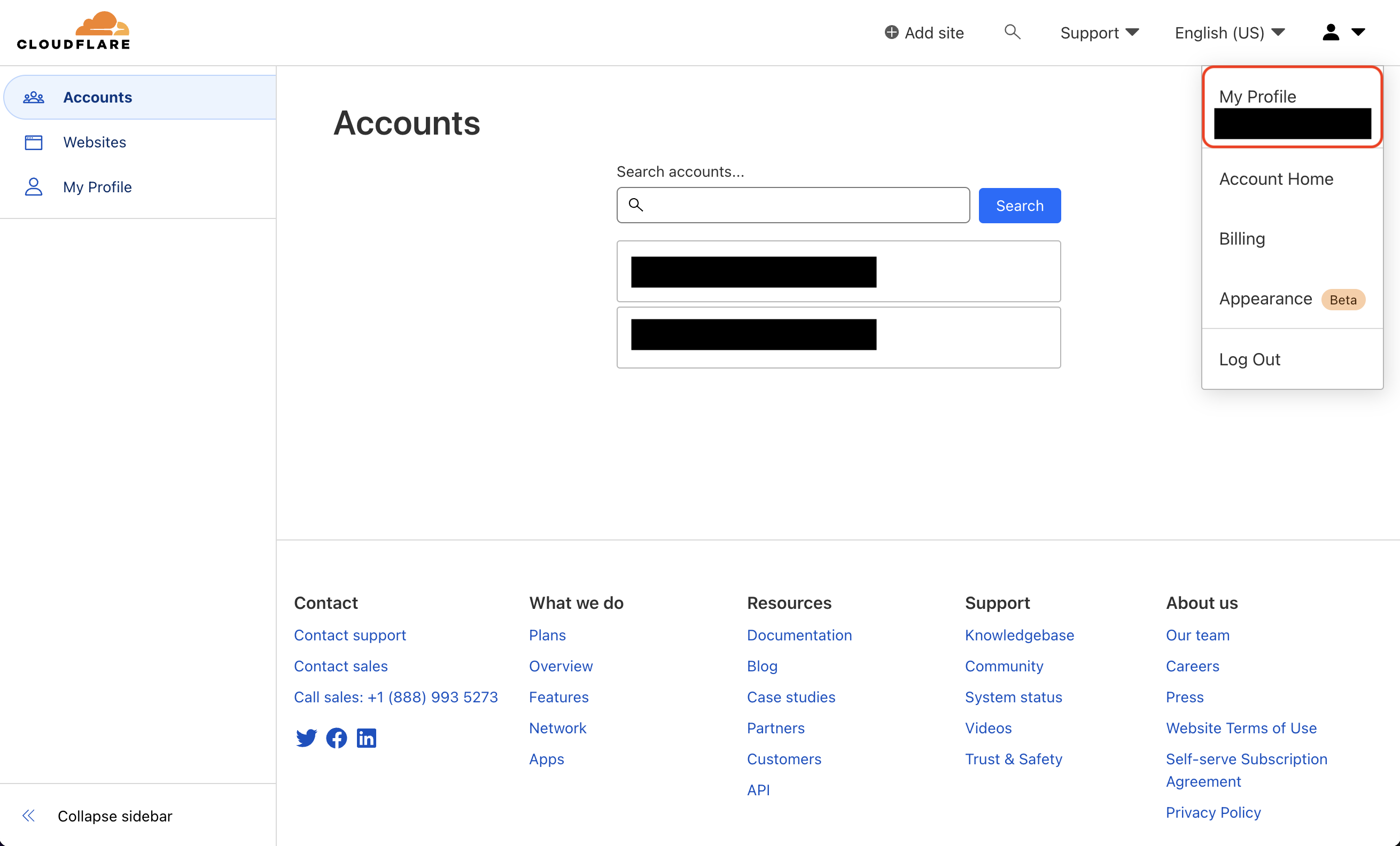
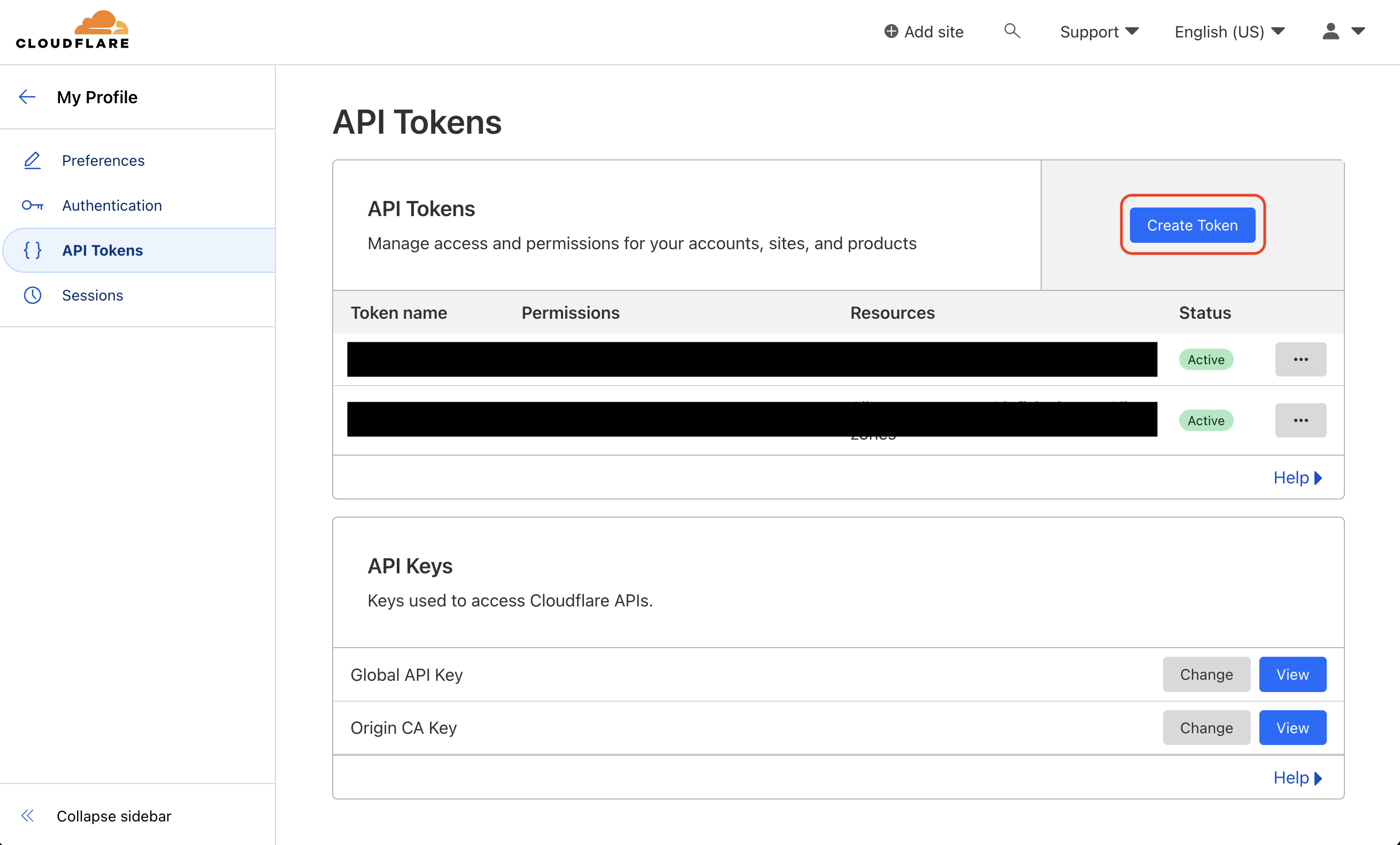
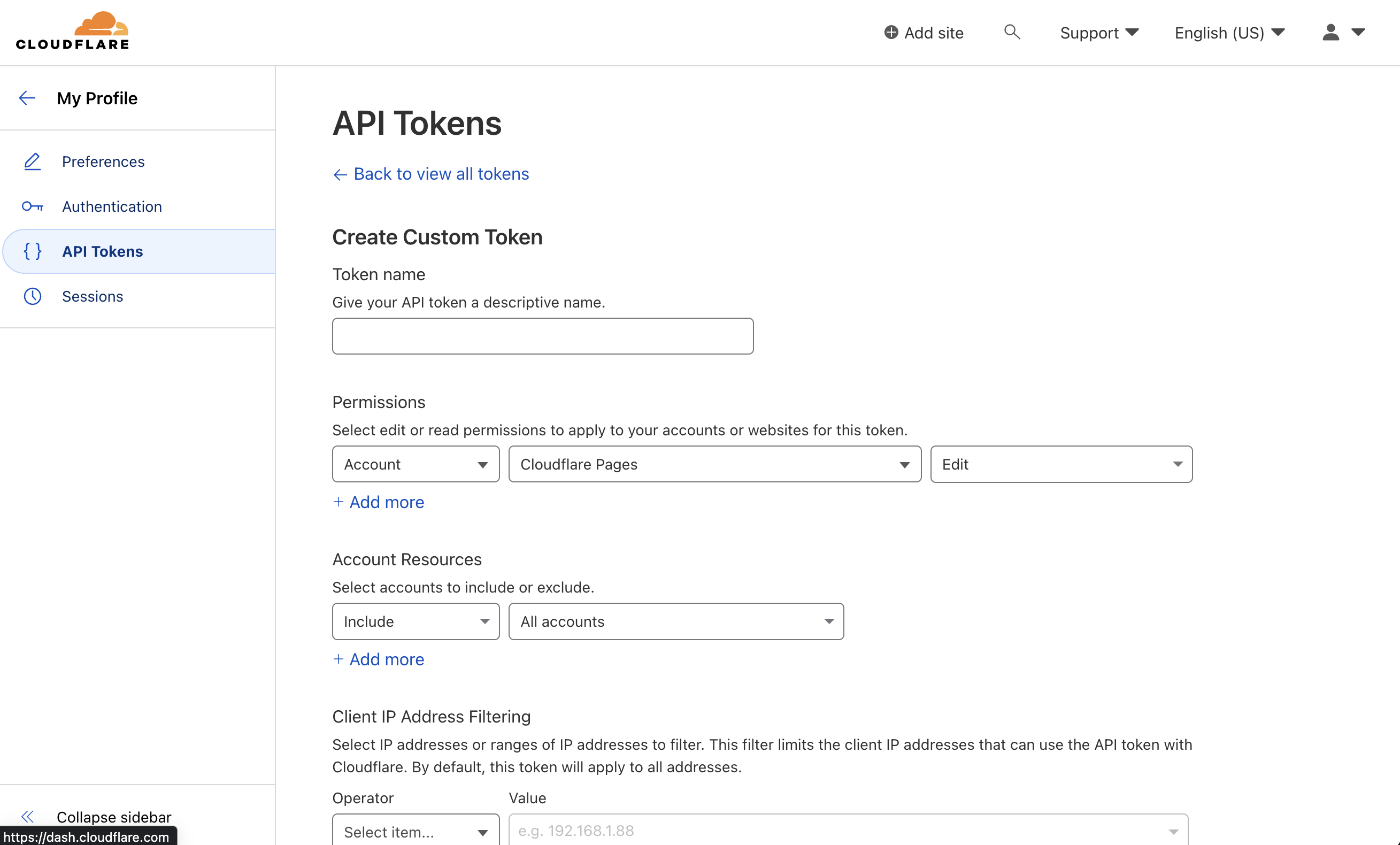
Copy your [Account ID](https://developers.cloudflare.com/fundamentals/get-started/basic-tasks/find-account-and-zone-ids/) from Account > Workers & Pages > Overview
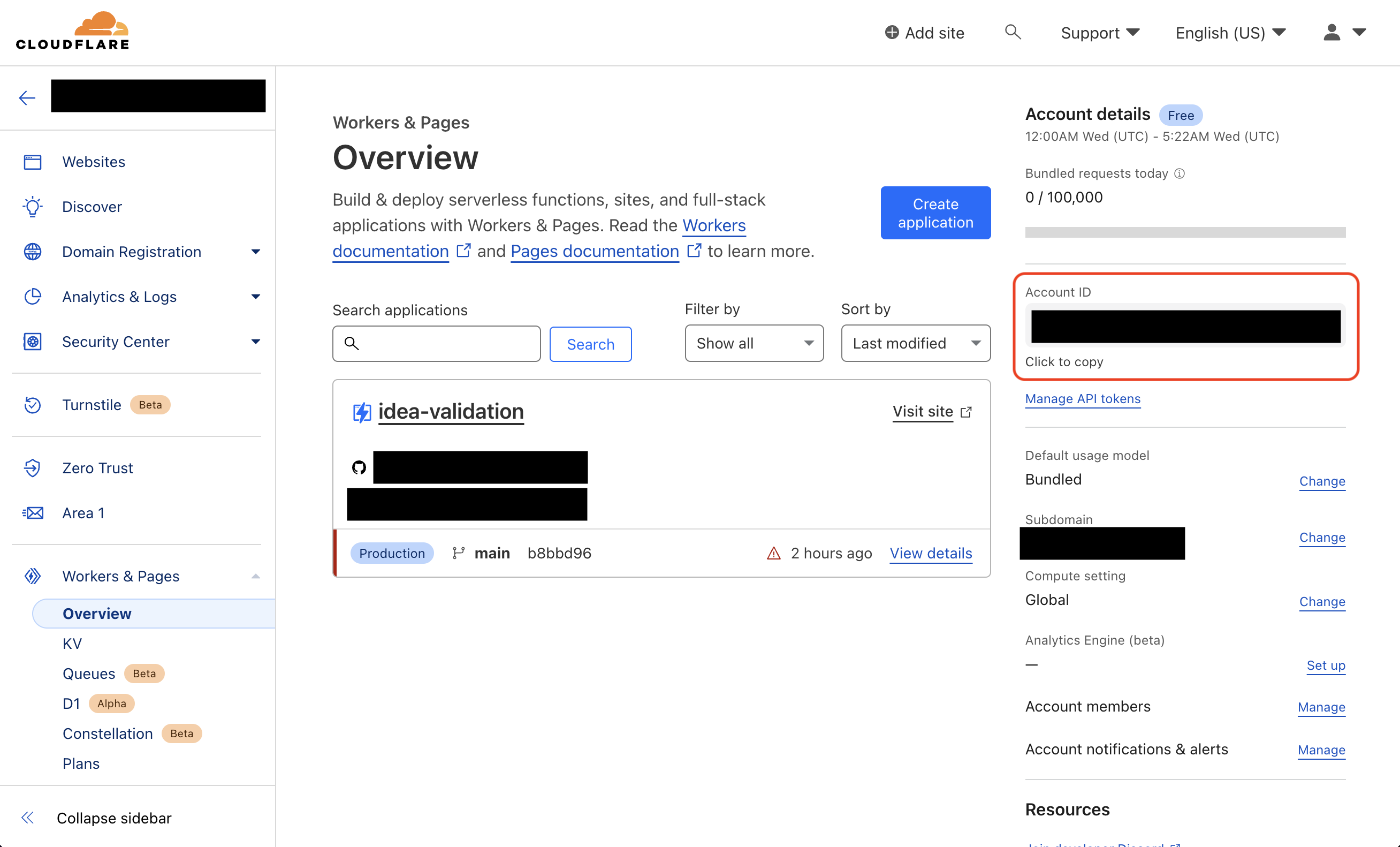
Navigate to your project's integrations tab in Infisical.
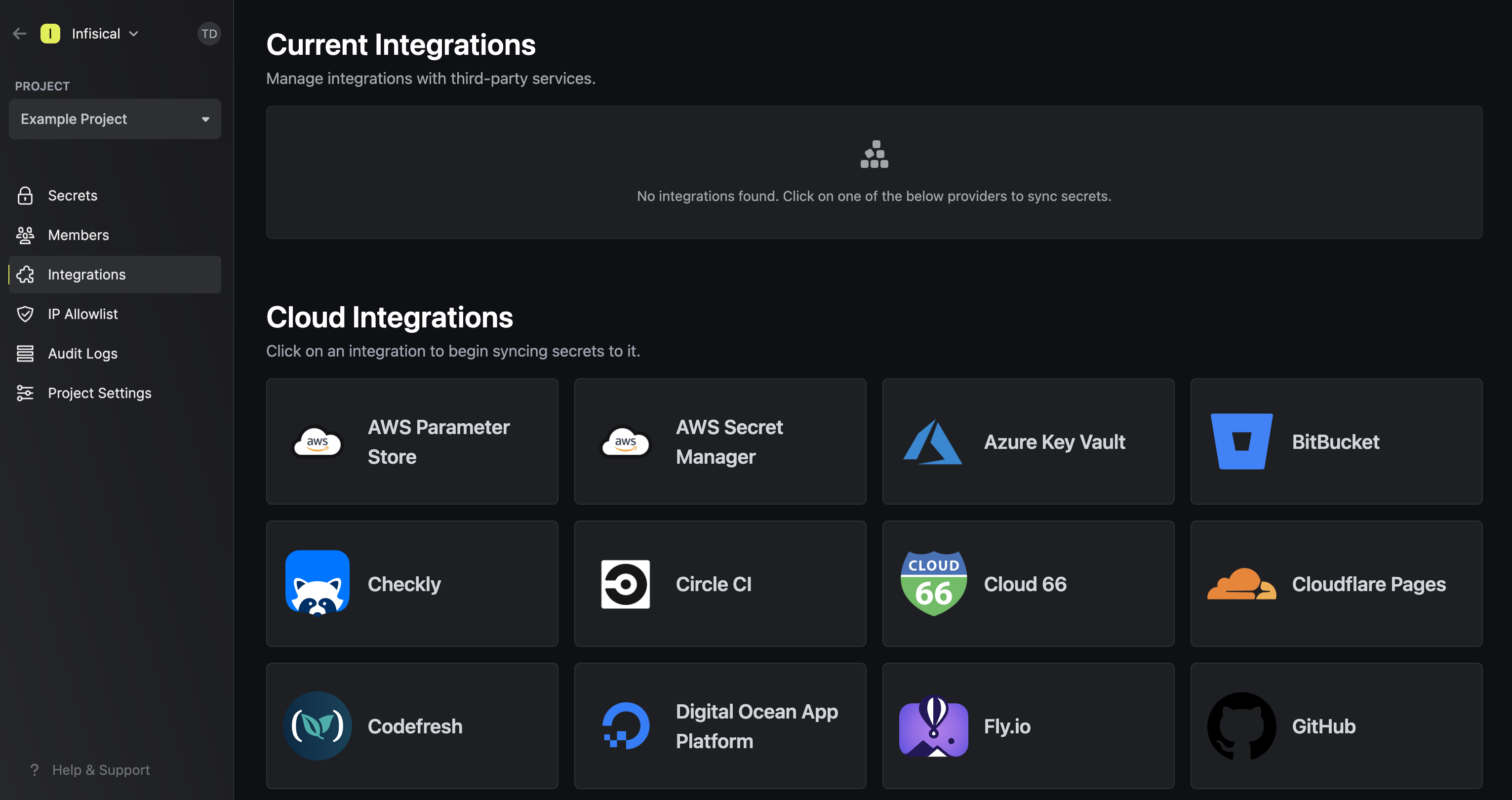
Press on the Cloudflare Pages tile and input your Cloudflare API token and account ID to grant Infisical access to your Cloudflare Pages.
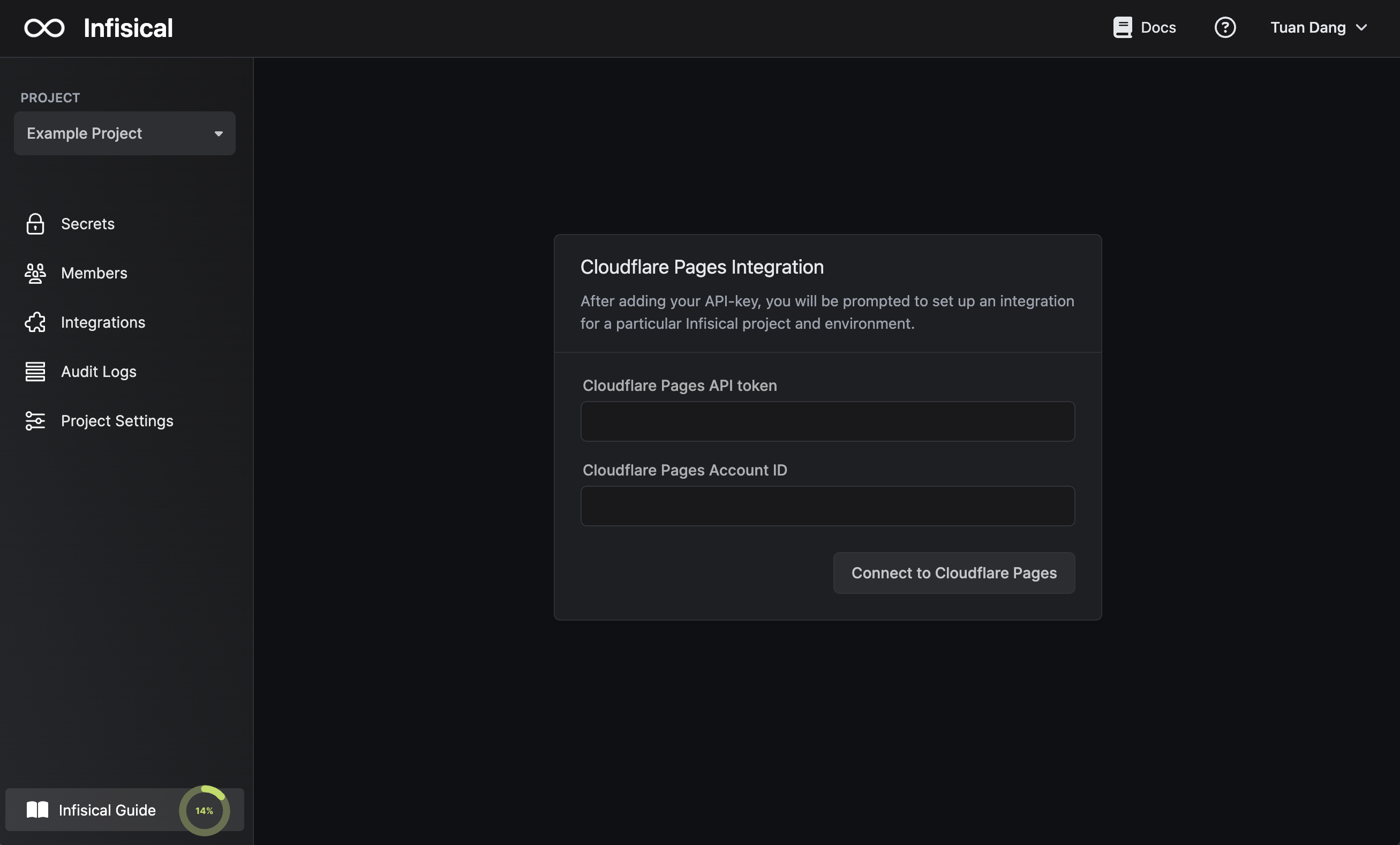
Select which Infisical environment secrets you want to sync to Cloudflare and press create integration to start syncing secrets.
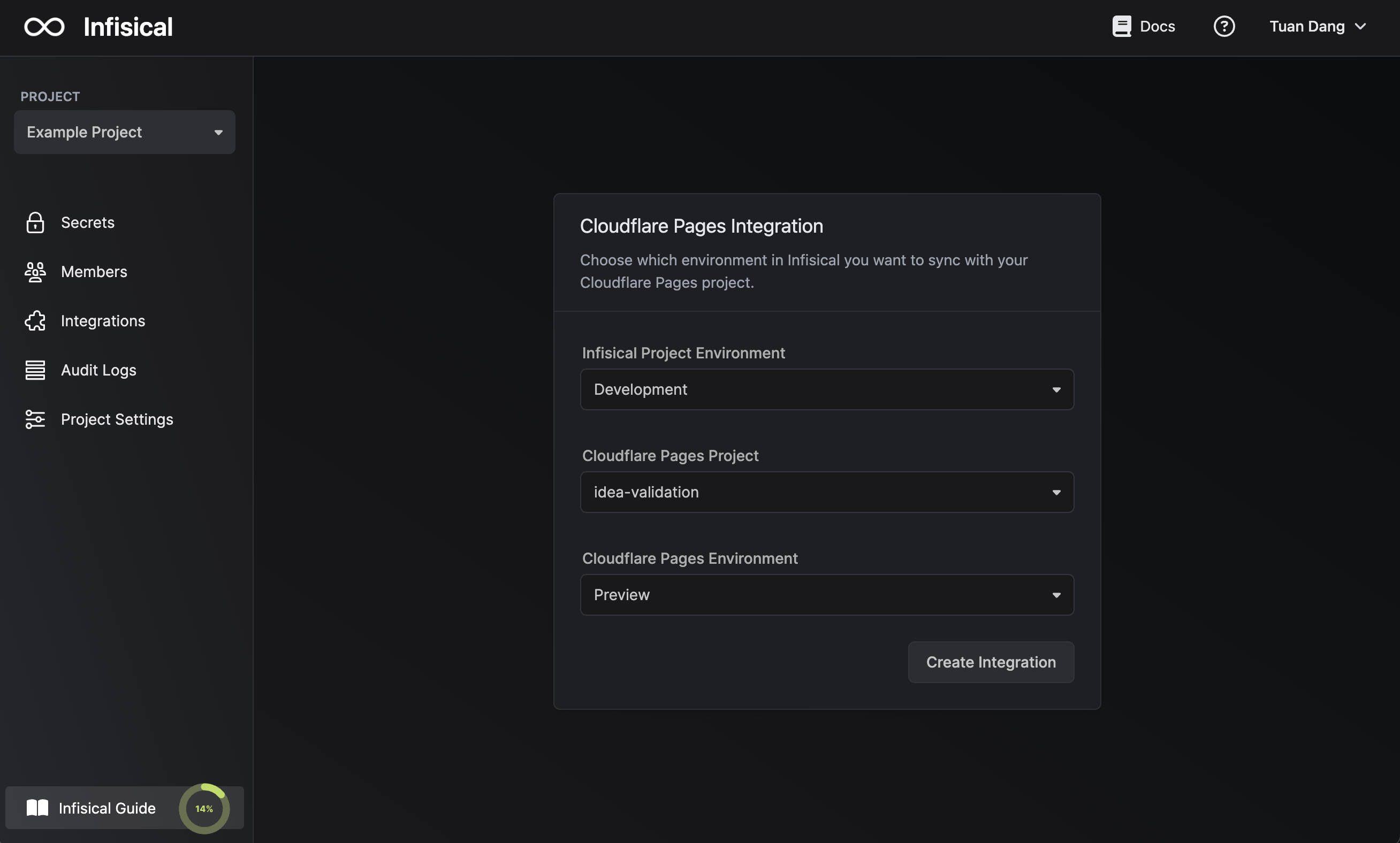
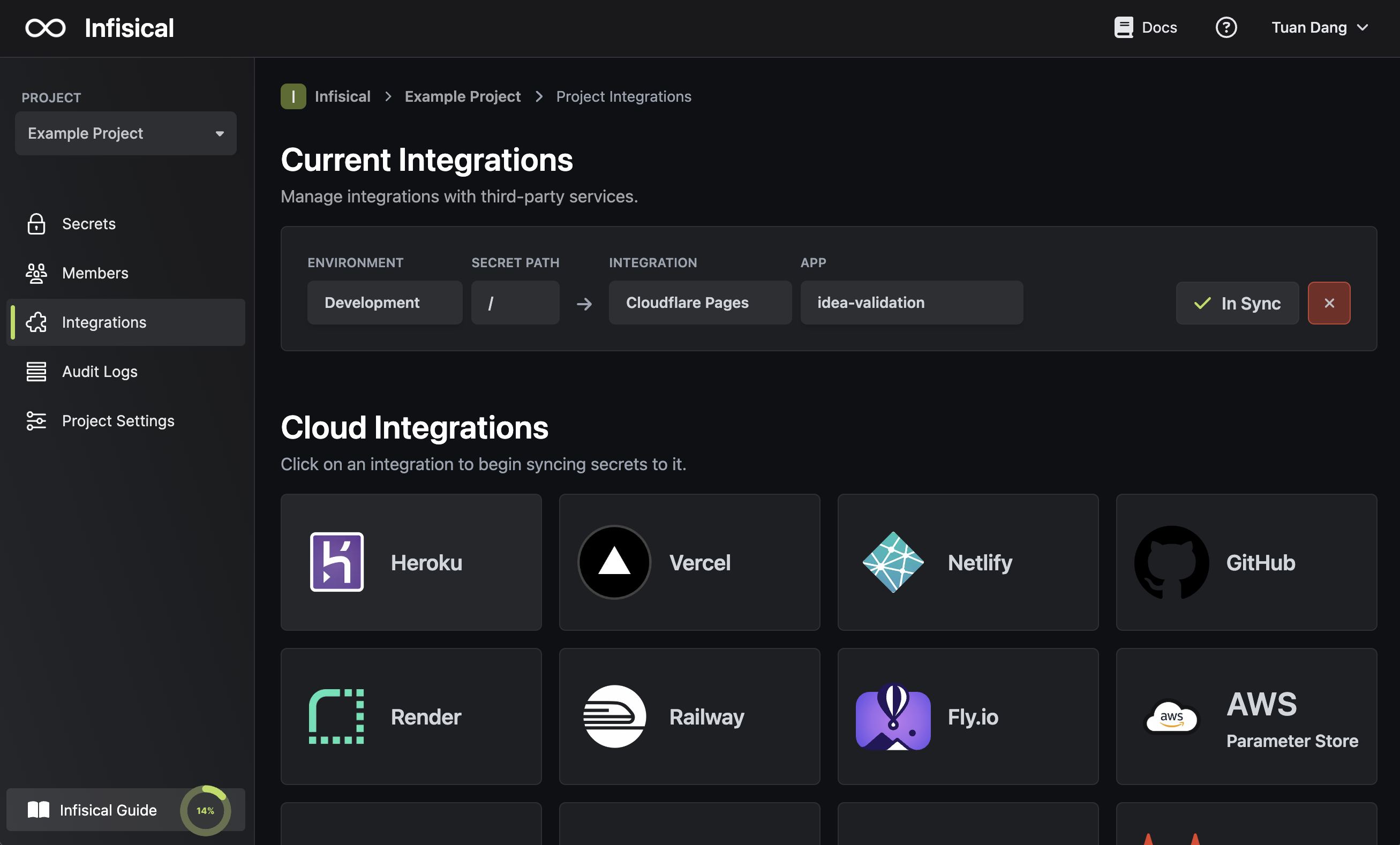
# Cloudflare Workers
How to sync secrets from Infisical to Cloudflare Workers
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
Obtain a Cloudflare [API token](https://dash.cloudflare.com/profile/api-tokens) and [Account ID](https://developers.cloudflare.com/fundamentals/get-started/basic-tasks/find-account-and-zone-ids/):
Create a new [API token](https://dash.cloudflare.com/profile/api-tokens) in My Profile > API Tokens
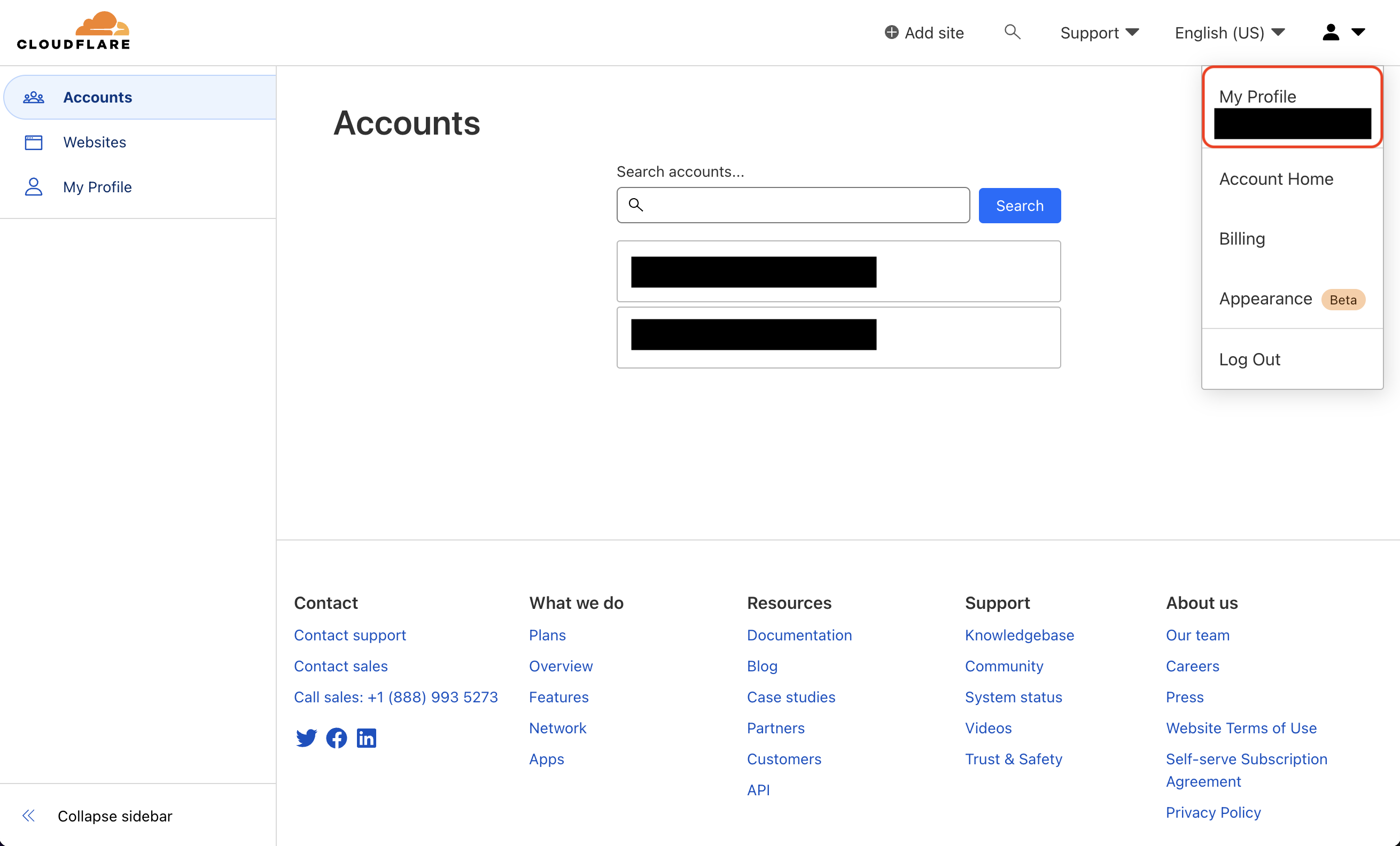
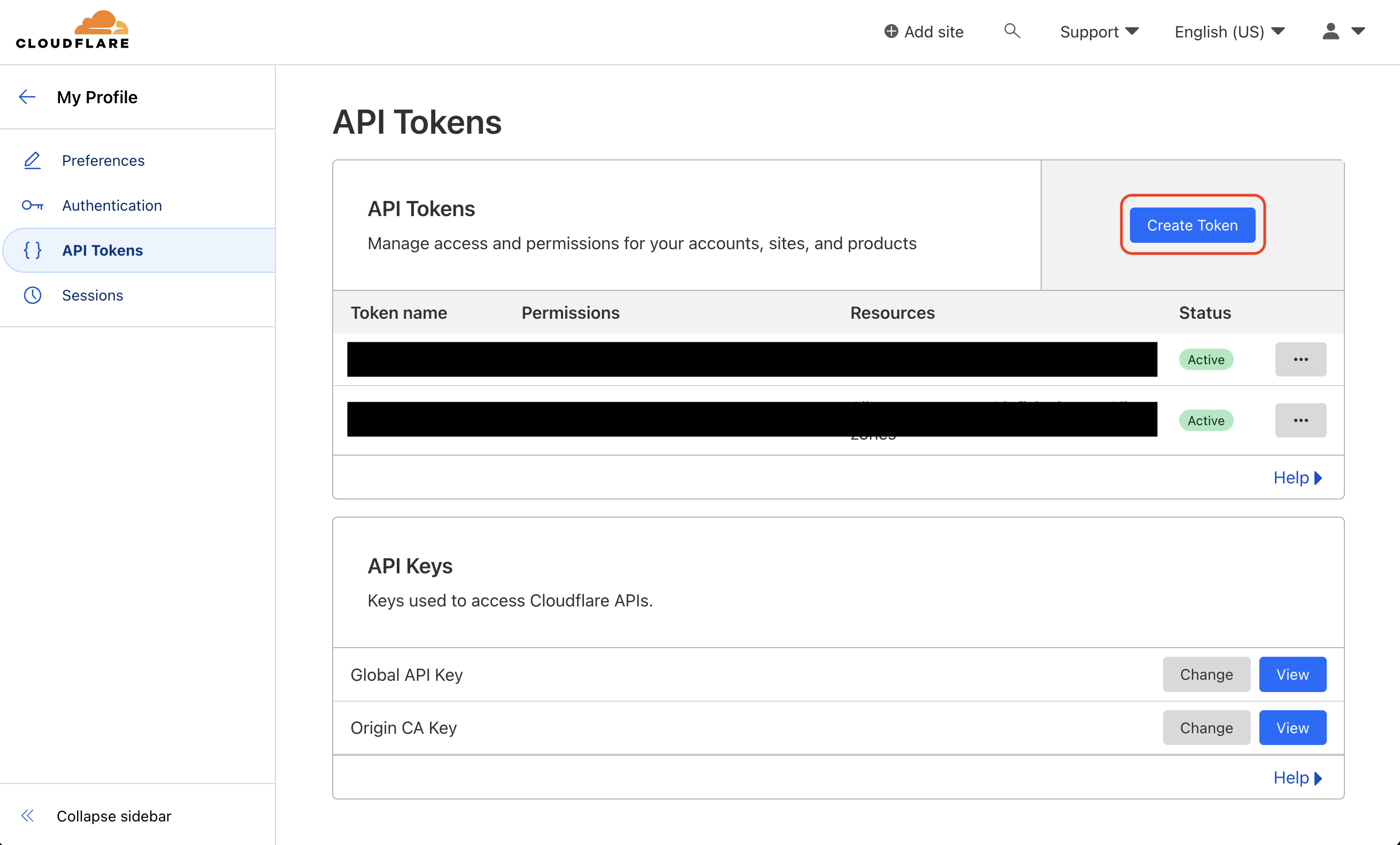
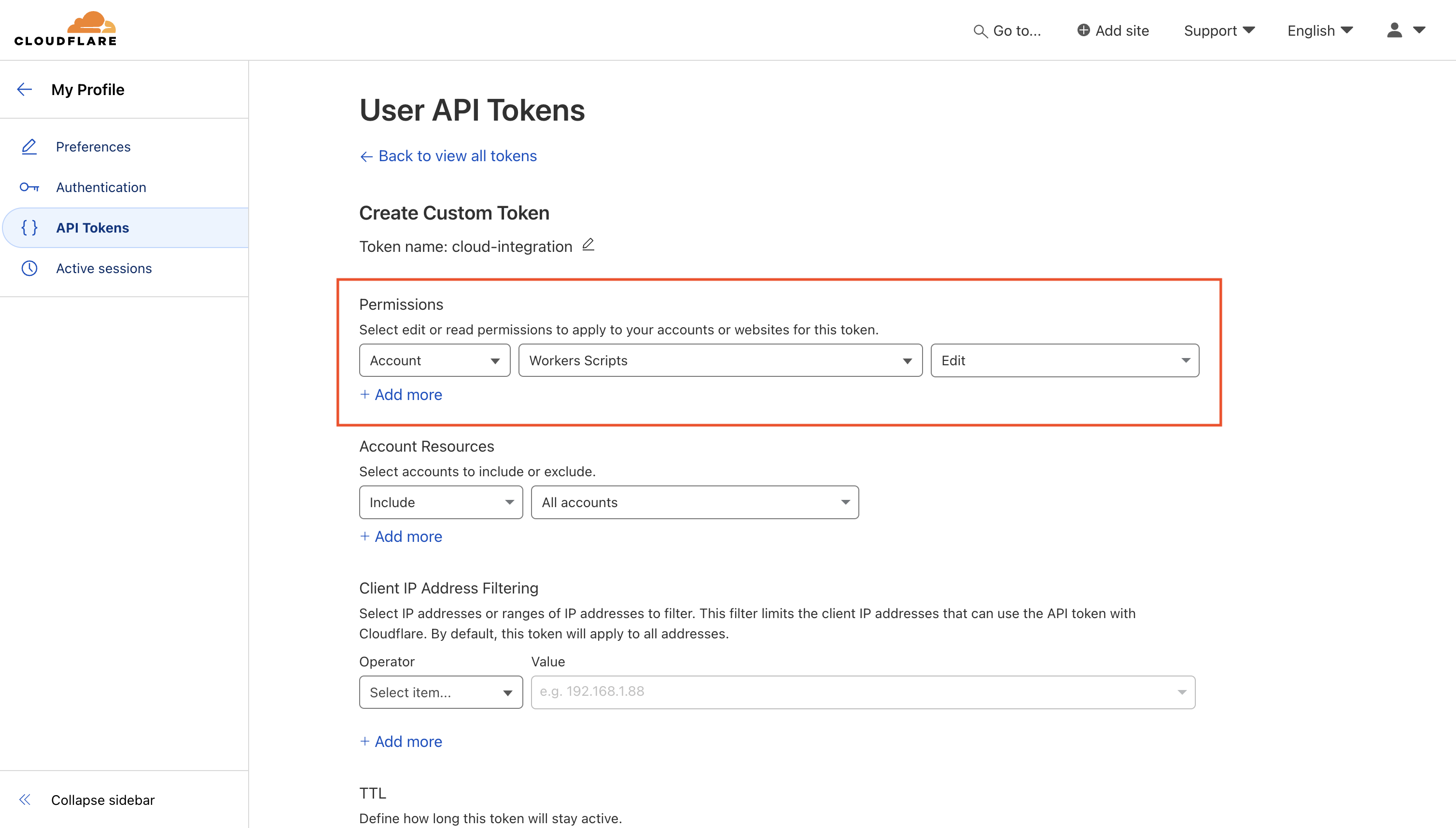
Copy your [Account ID](https://developers.cloudflare.com/fundamentals/get-started/basic-tasks/find-account-and-zone-ids/) from Account > Workers & Pages > Overview
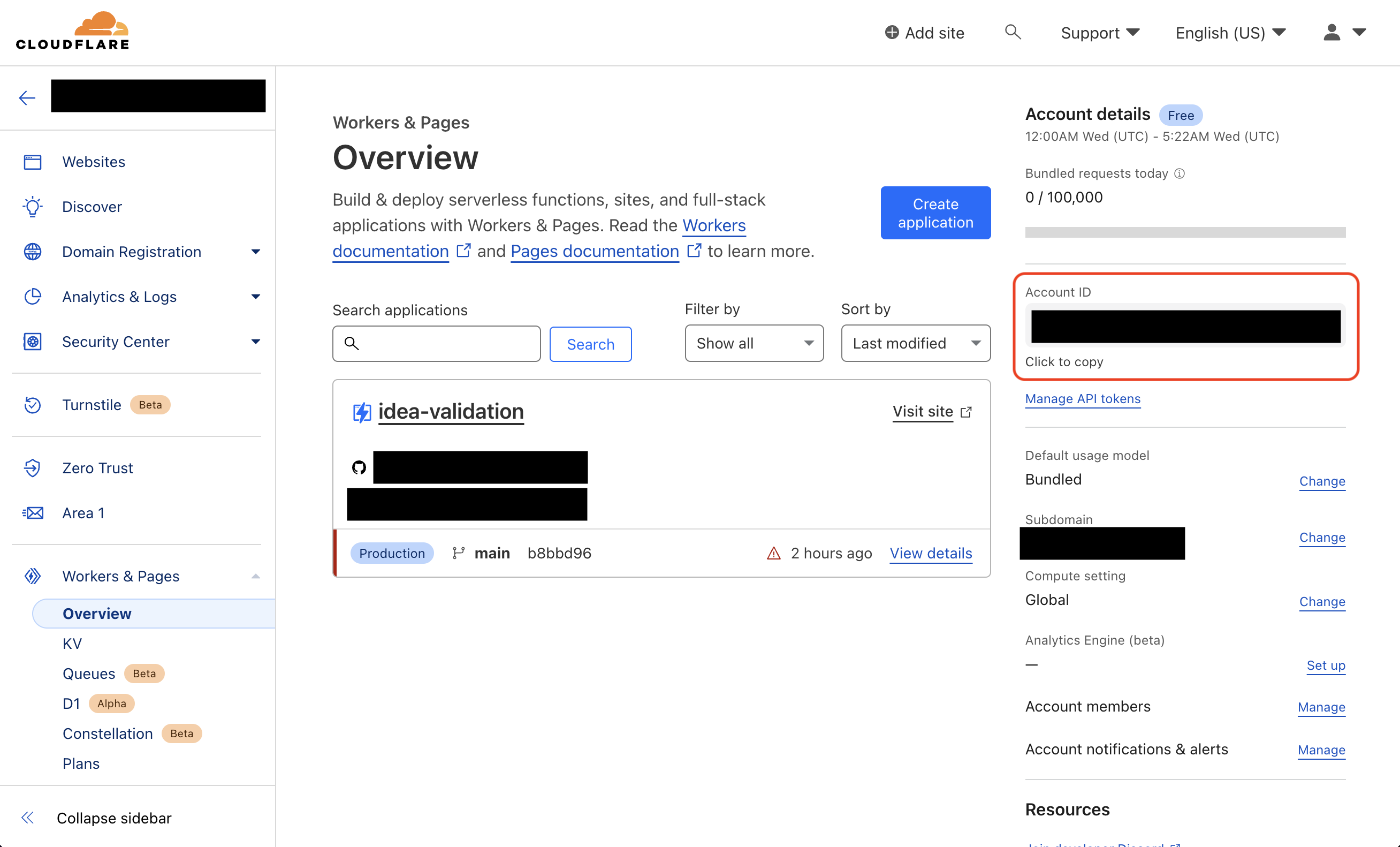
Navigate to your project's integrations tab in Infisical.
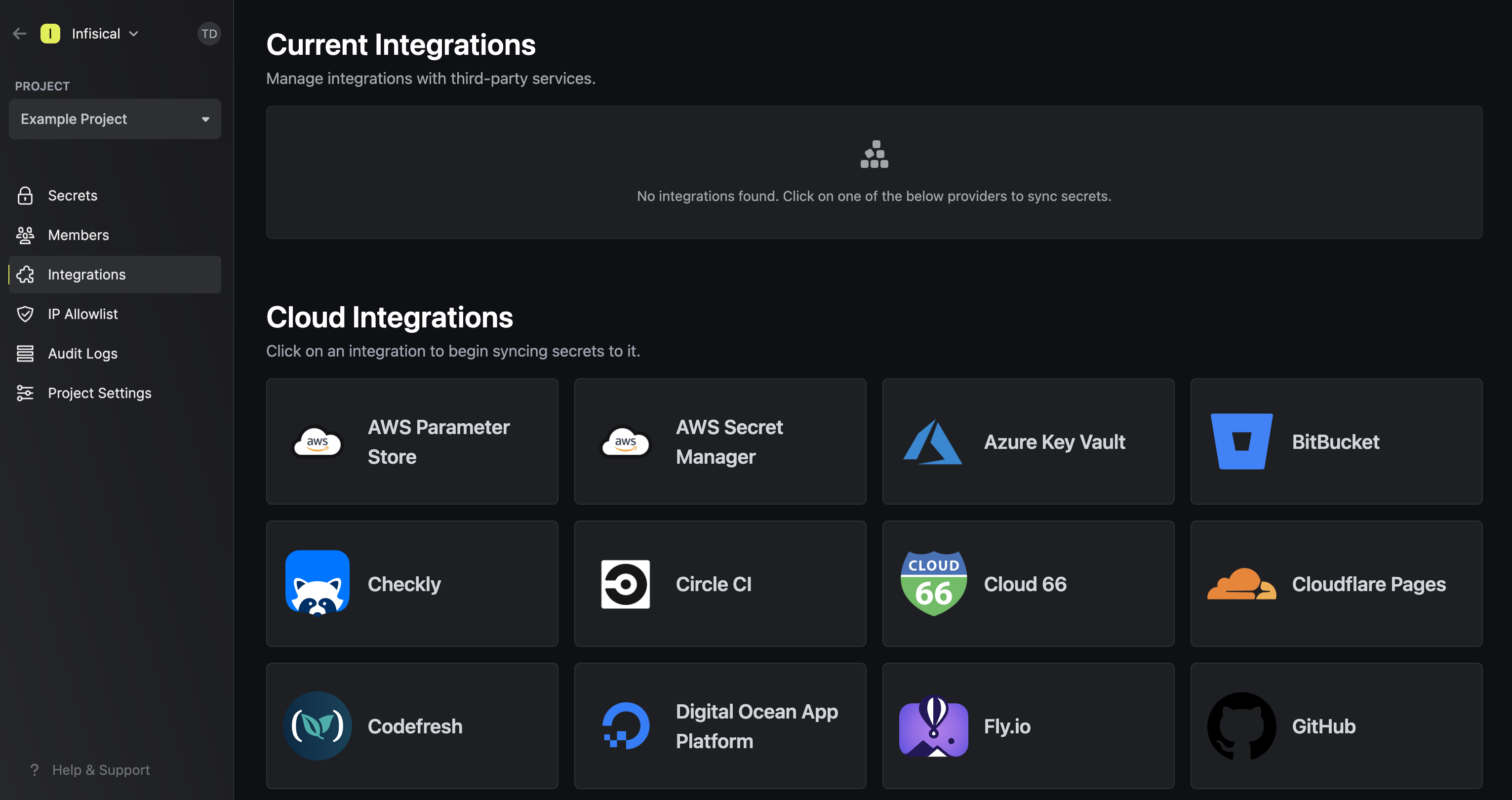
Press on the Cloudflare Workers tile and input your Cloudflare API token and account ID to grant Infisical access to your Cloudflare Workers.
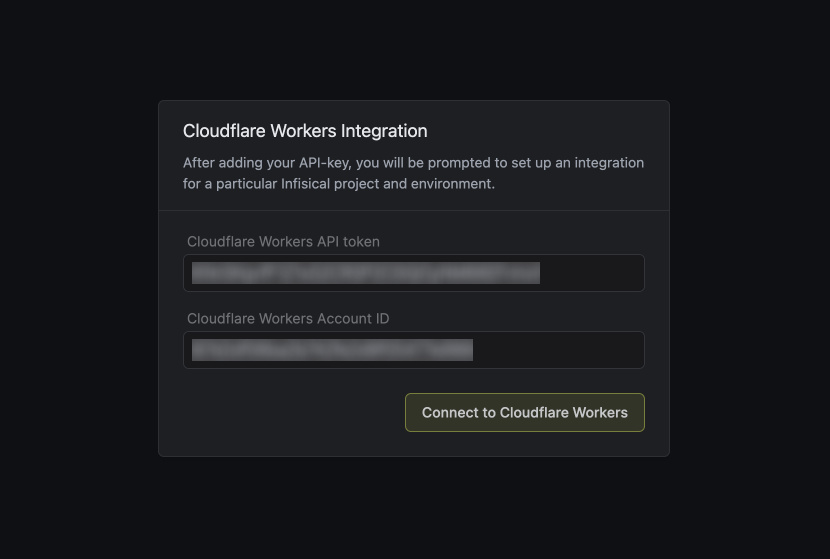
Select which Infisical environment secrets you want to sync to Cloudflare Workers and press create integration to start syncing secrets.
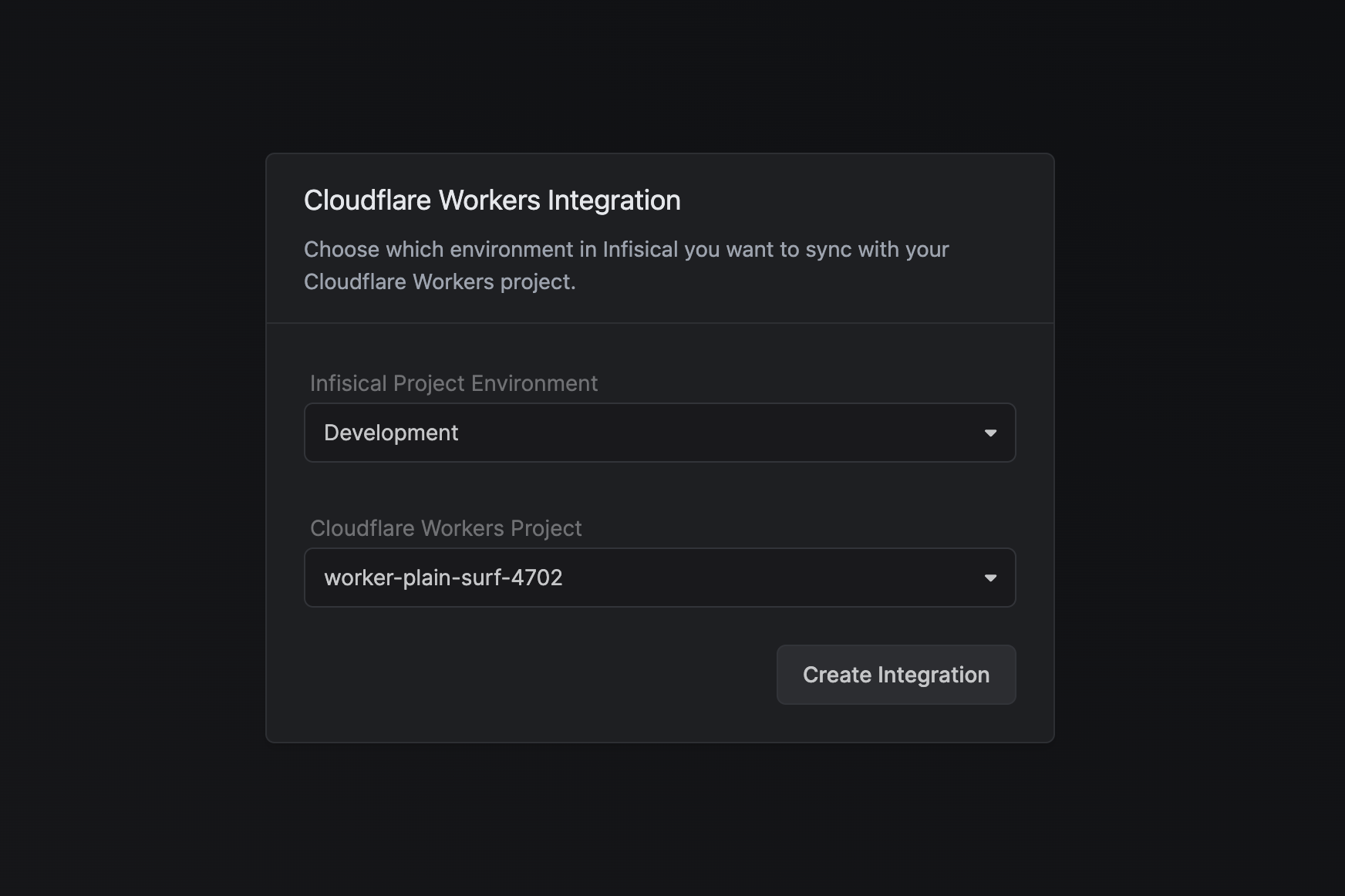
# Databricks
Learn how to sync secrets from Infisical to Databricks.
Prerequisites:
* Set up and add secrets to [Infisical Cloud](https://app.infisical.com)
Obtain a Personal Access Token in **User Settings** > **Developer** > **Access Tokens**.
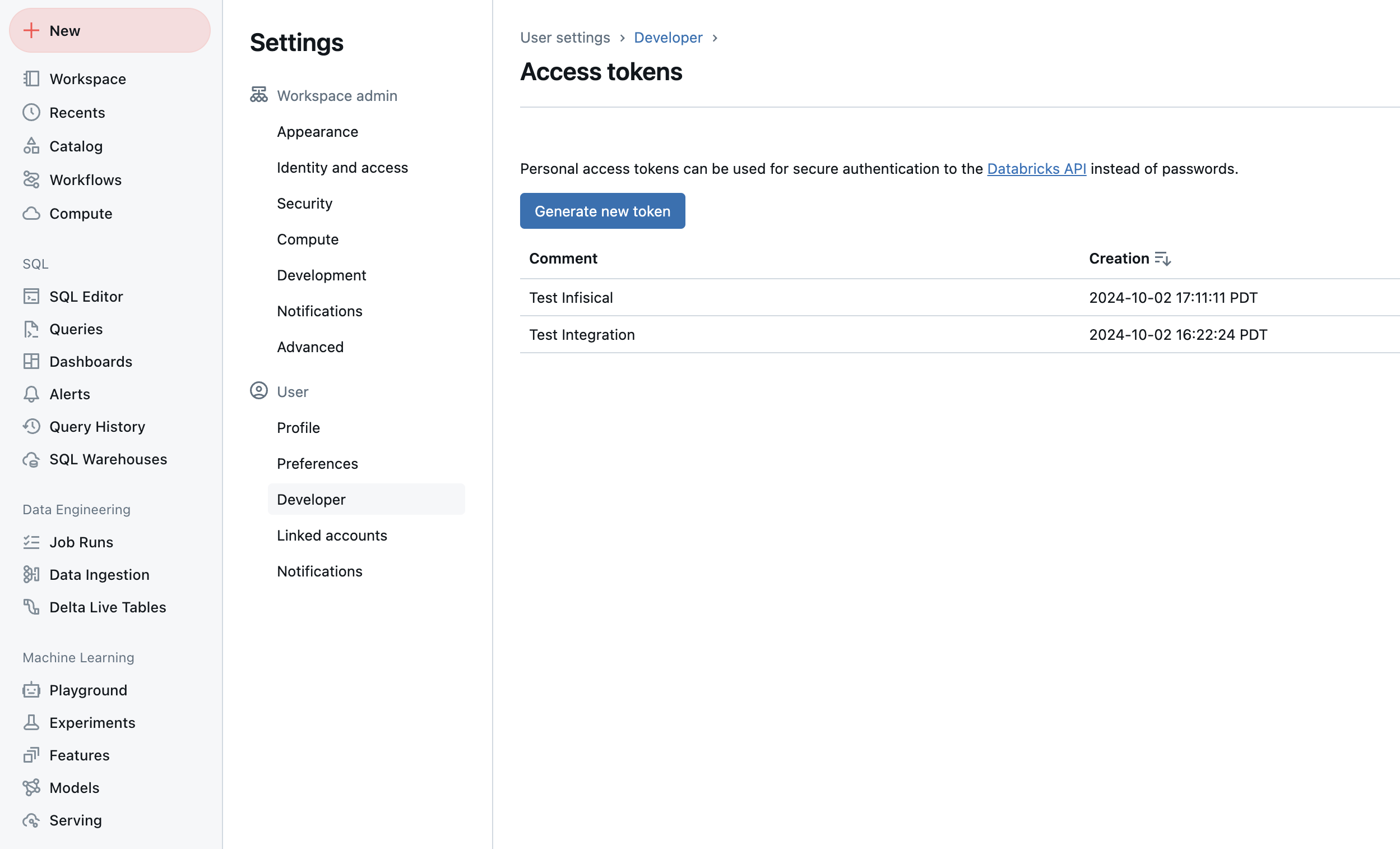
Navigate to your project's integrations tab in Infisical.
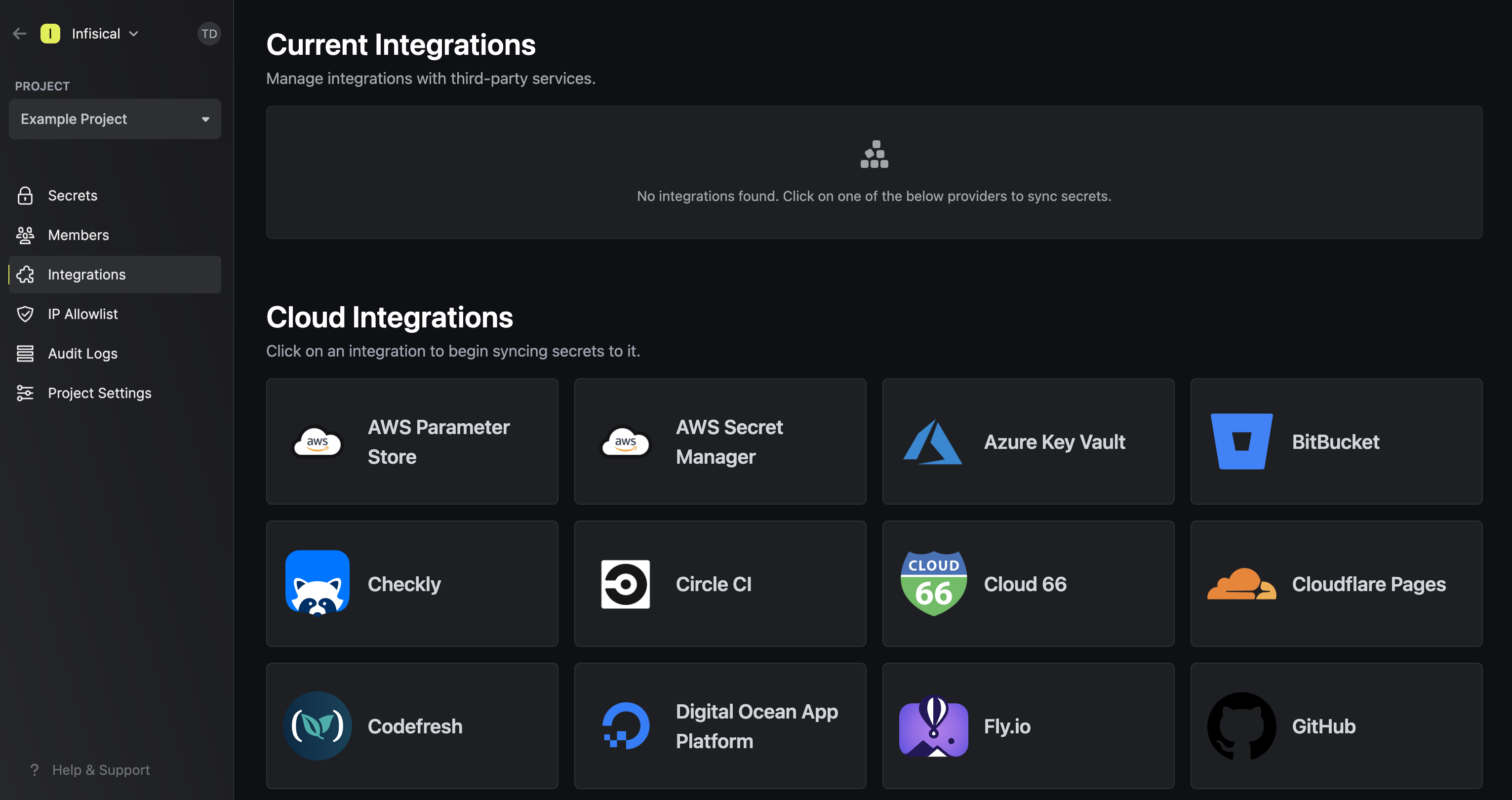
Press on the Databricks tile and enter your Databricks instance URL in the following format: `https://xxx.cloud.databricks.com`. Then, input your Databricks Access Token to grant Infisical the necessary permissions in your Databricks account.
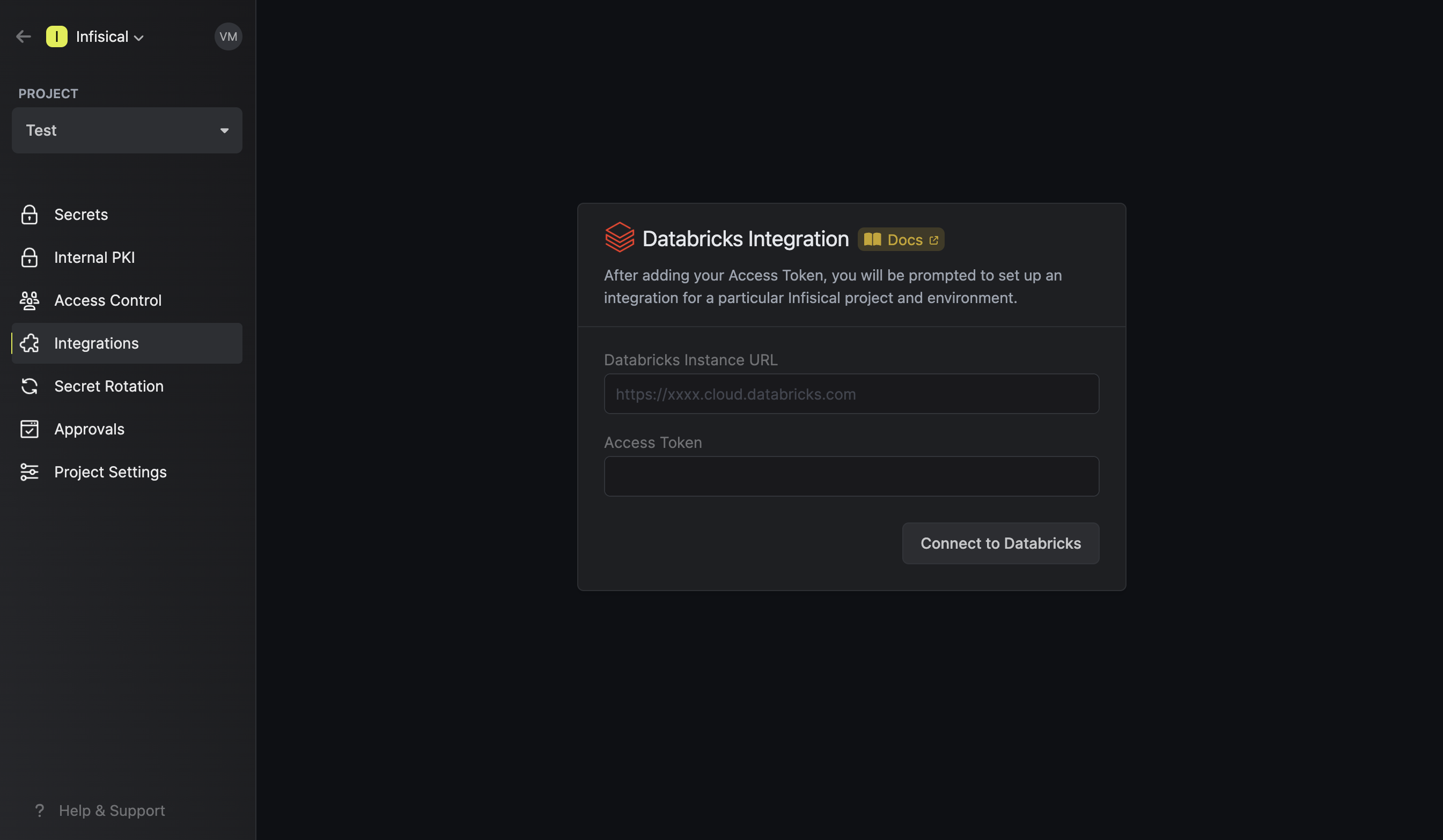
Select which Infisical environment and secret path you want to sync to which Databricks scope. Then, press create integration to start syncing secrets to Databricks.
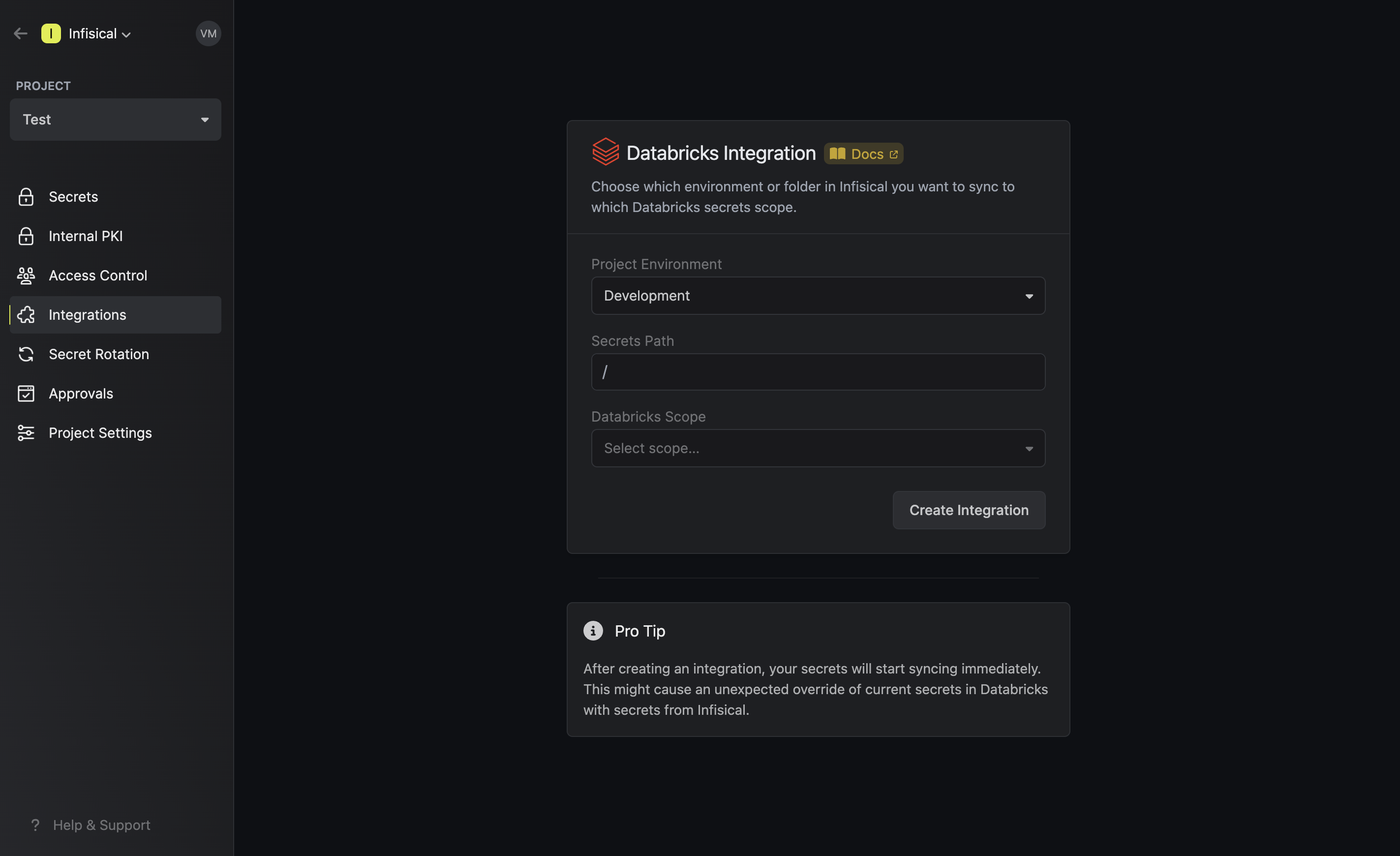
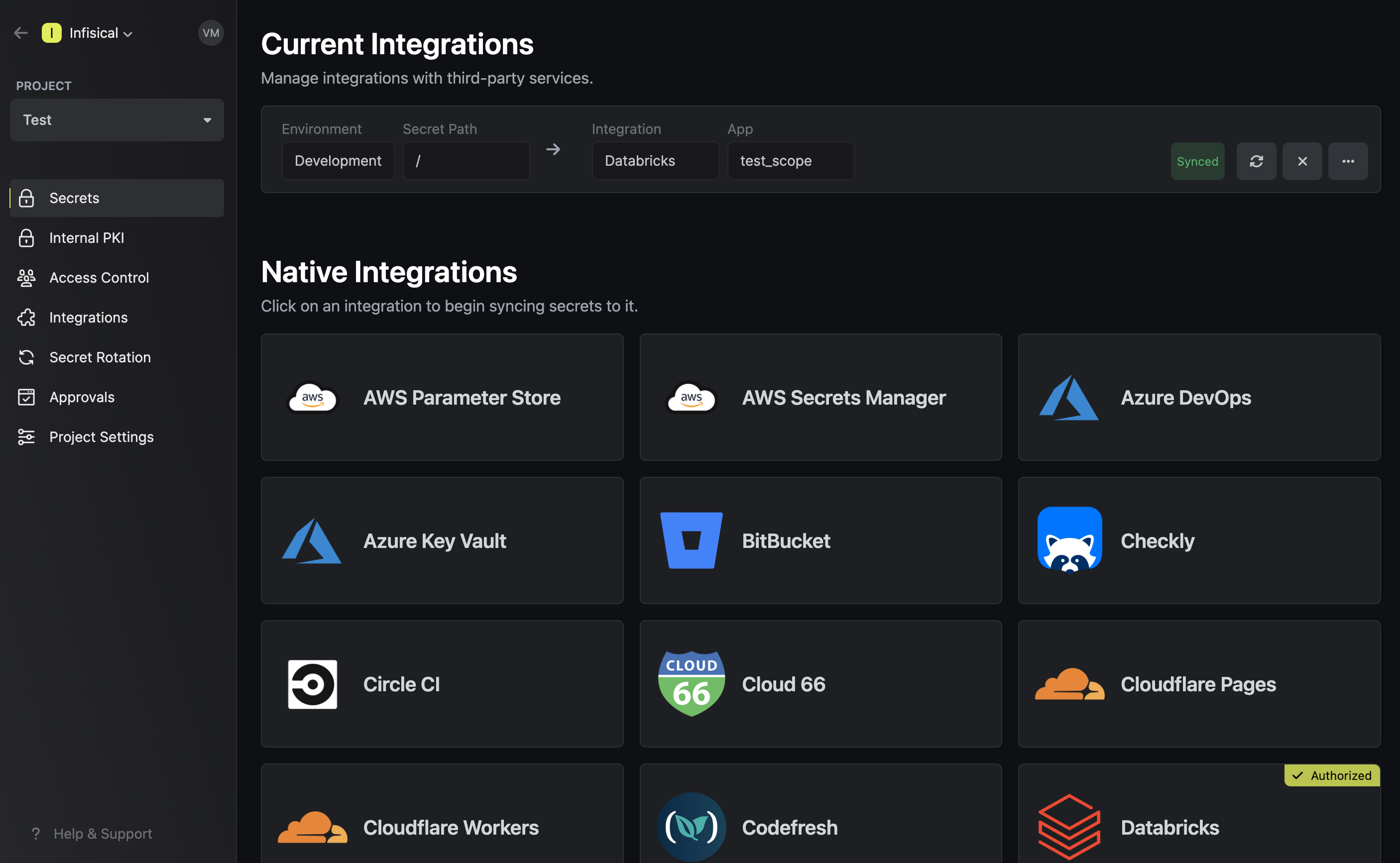
# Digital Ocean App Platform
How to sync secrets from Infisical to Digital Ocean App Platform
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
## Get your Digital Ocean Personal Access Tokens
On Digital Ocean dashboard, navigate to **API > Tokens** and click on "Generate New Token"
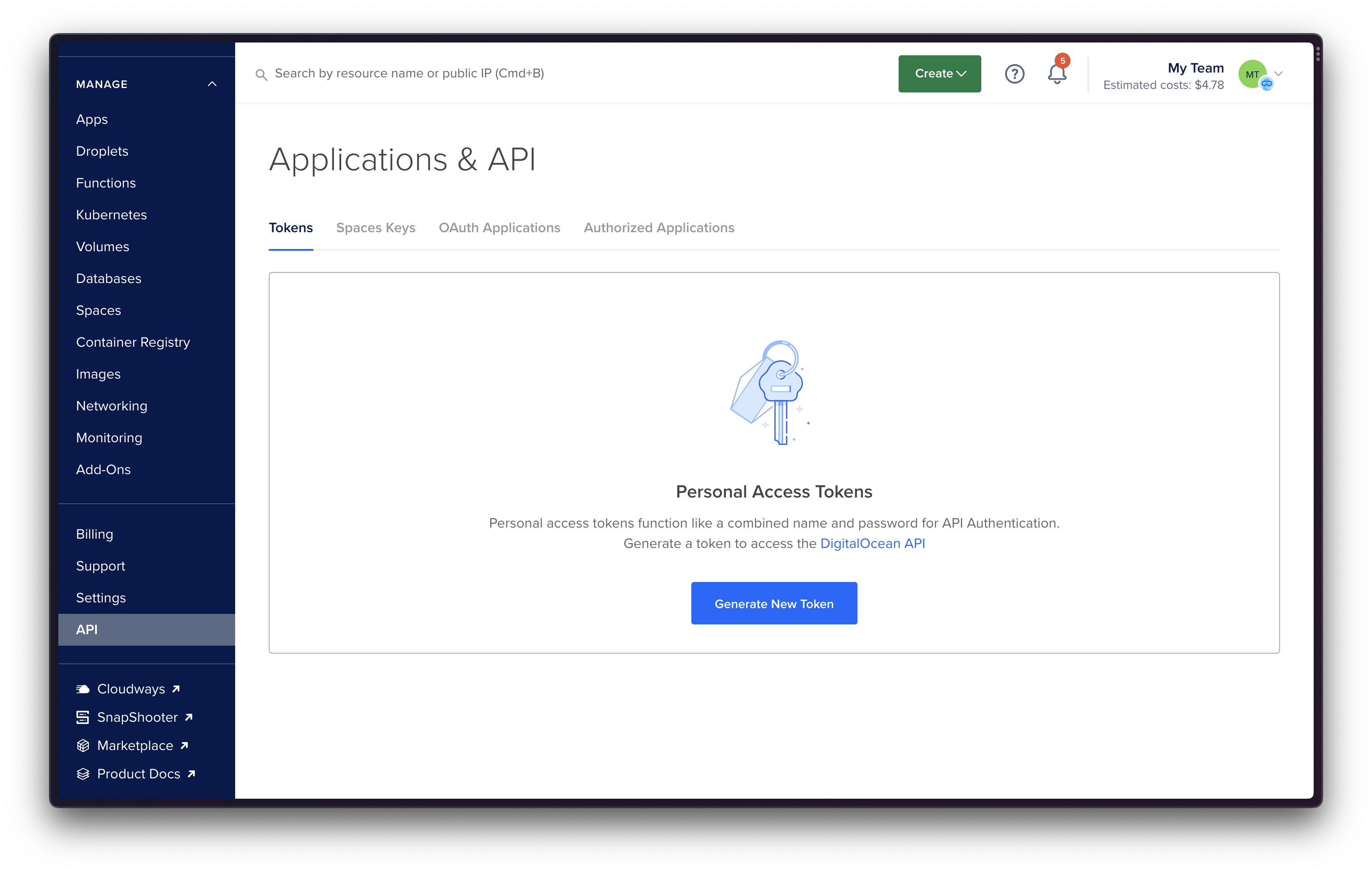
Name it **infisical**, choose **No expiry**, and make sure to check **Write (optional)**. Then click on "Generate Token" and copy your API token.
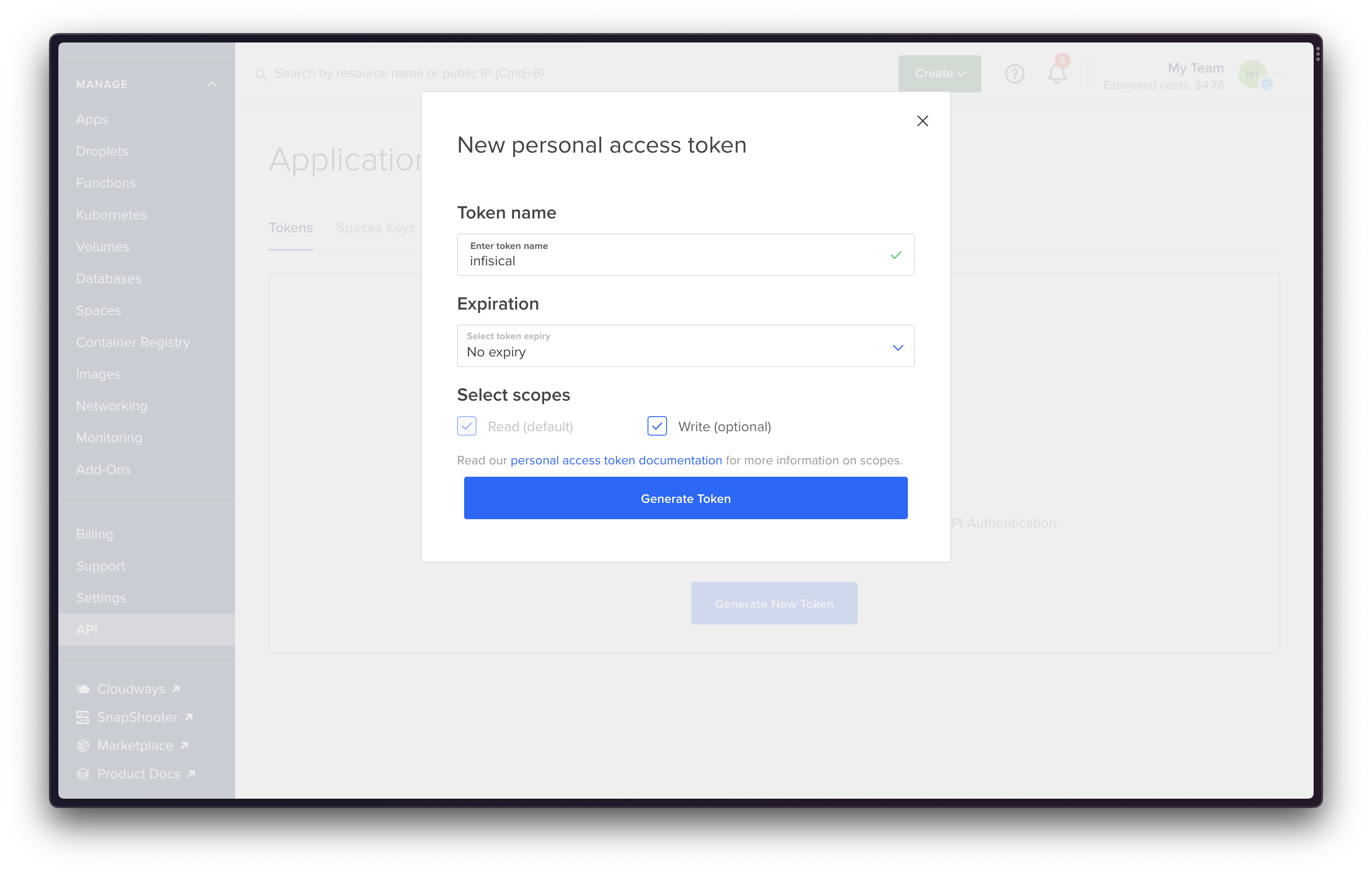
## Navigate to your project's integrations tab
Click on the **Digital Ocean App Platform** tile and enter your API token to grant Infisical access to your Digital Ocean account.
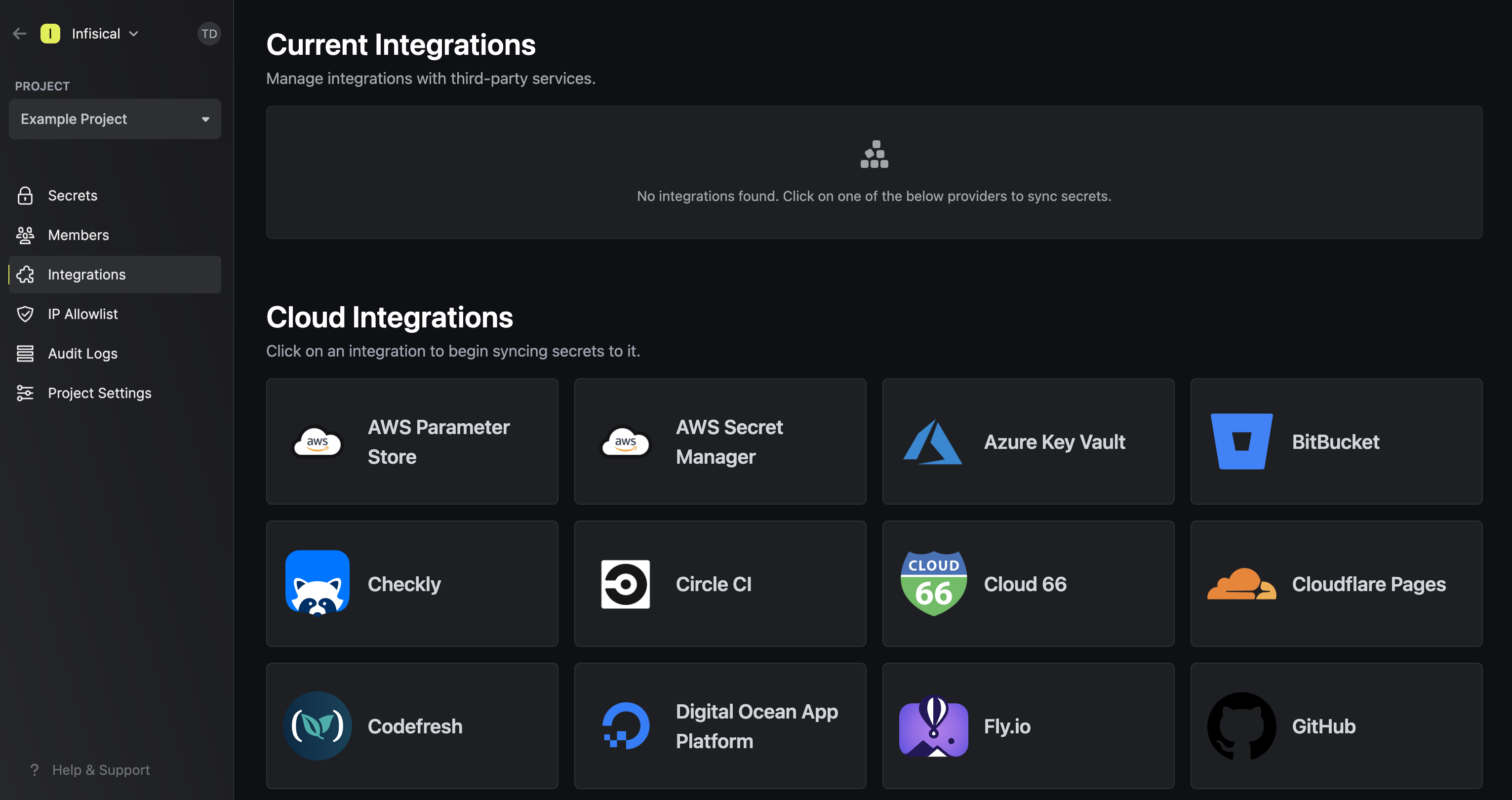
Then enter your Digital Ocean Personal Access Token here. Then click "Connect to Digital Ocean App Platform".
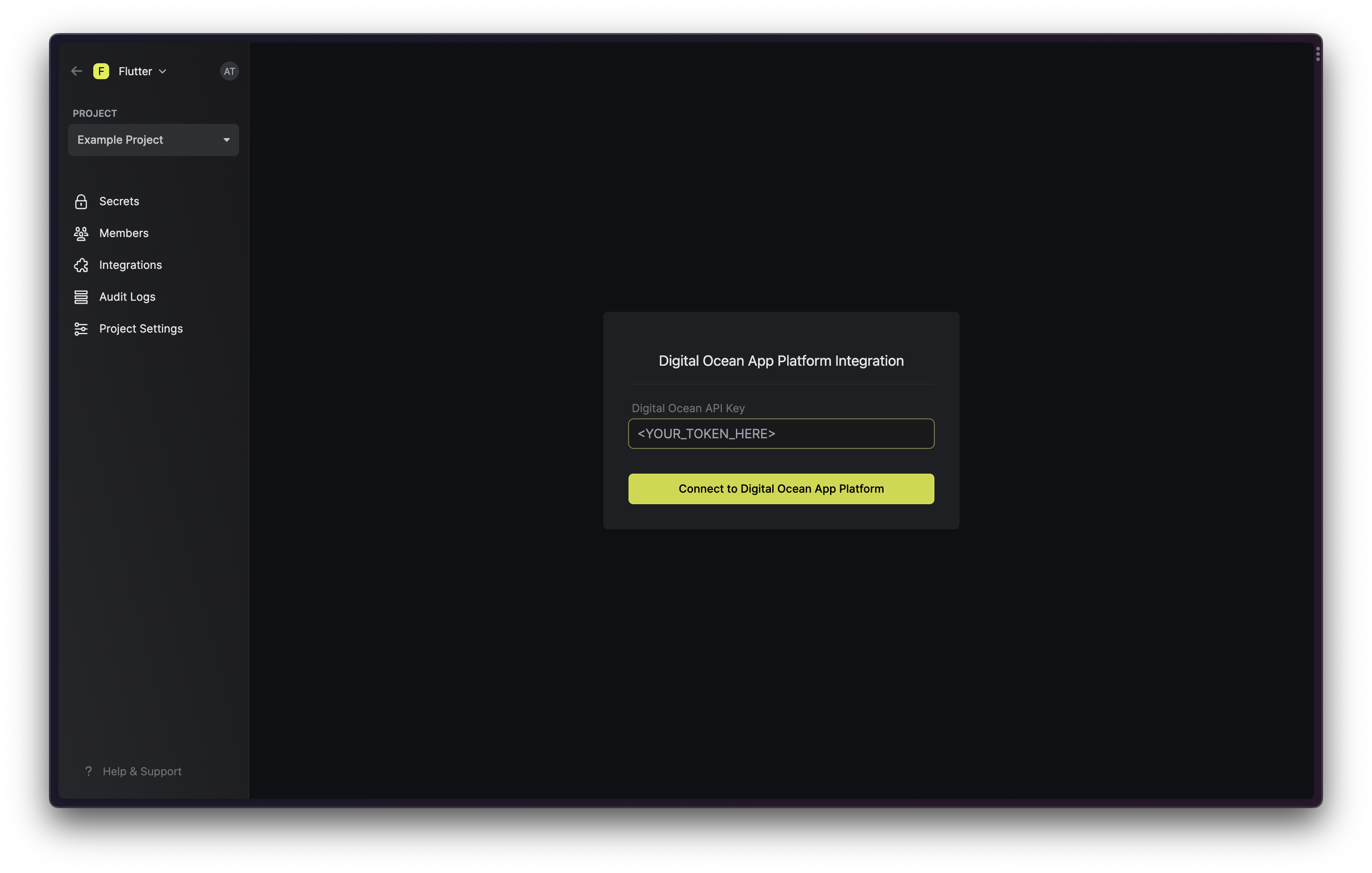
## Start integration
Select which Infisical environment secrets you want to sync to which Digital Ocean App and click "Create Integration".
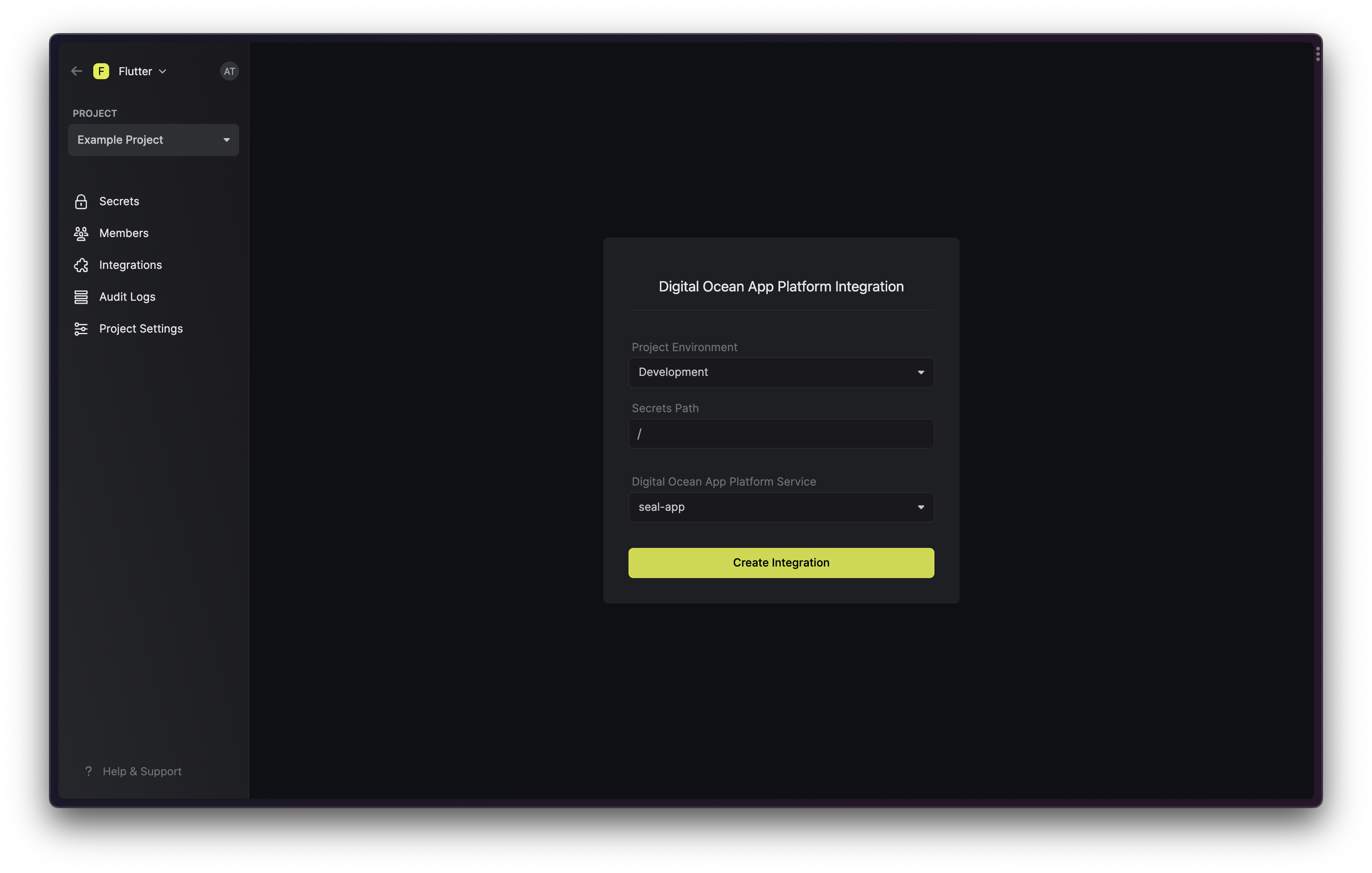
Done!
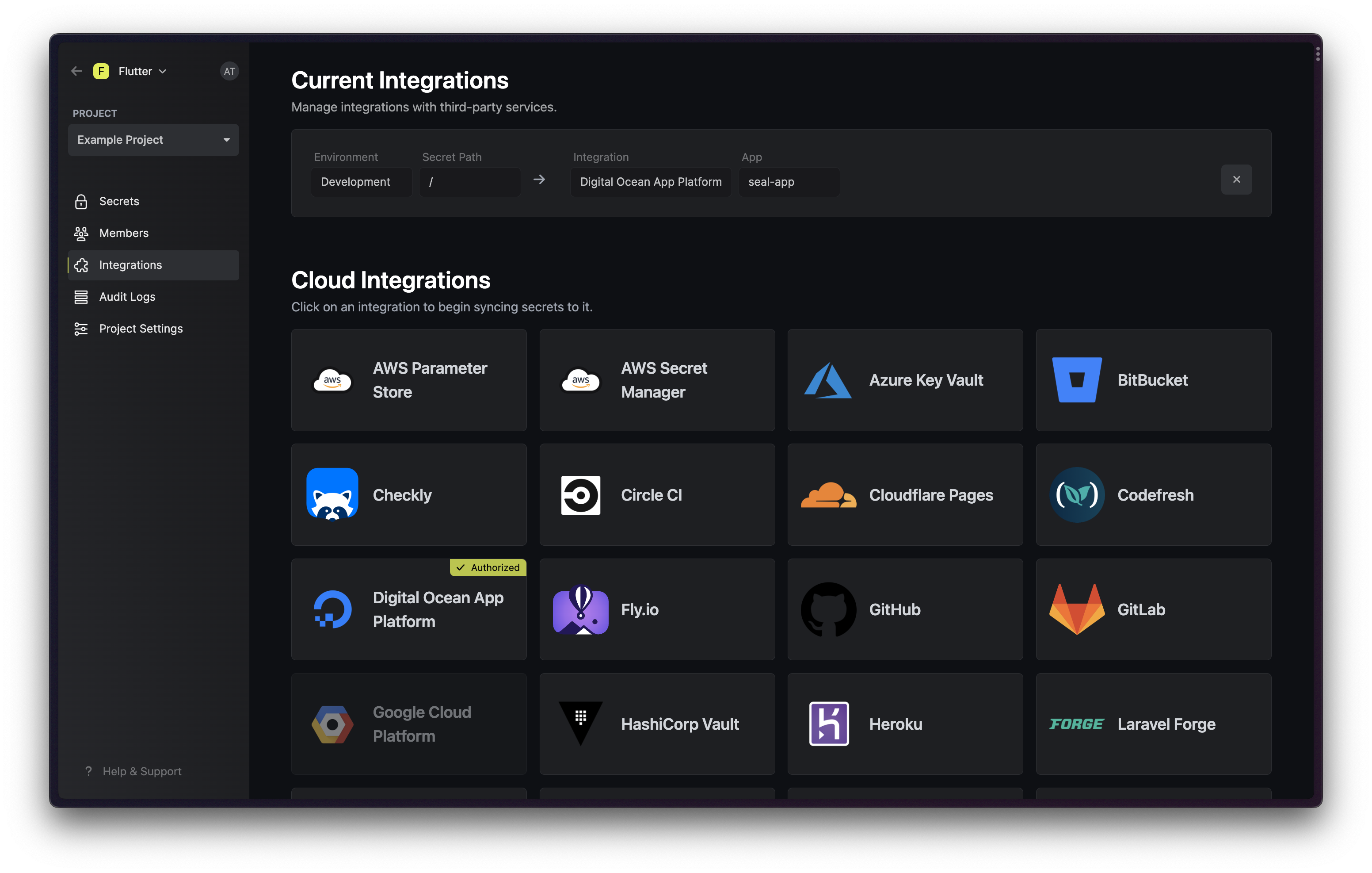
# Fly.io
How to sync secrets from Infisical to Fly.io
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
Obtain a Fly.io access token in Access Tokens
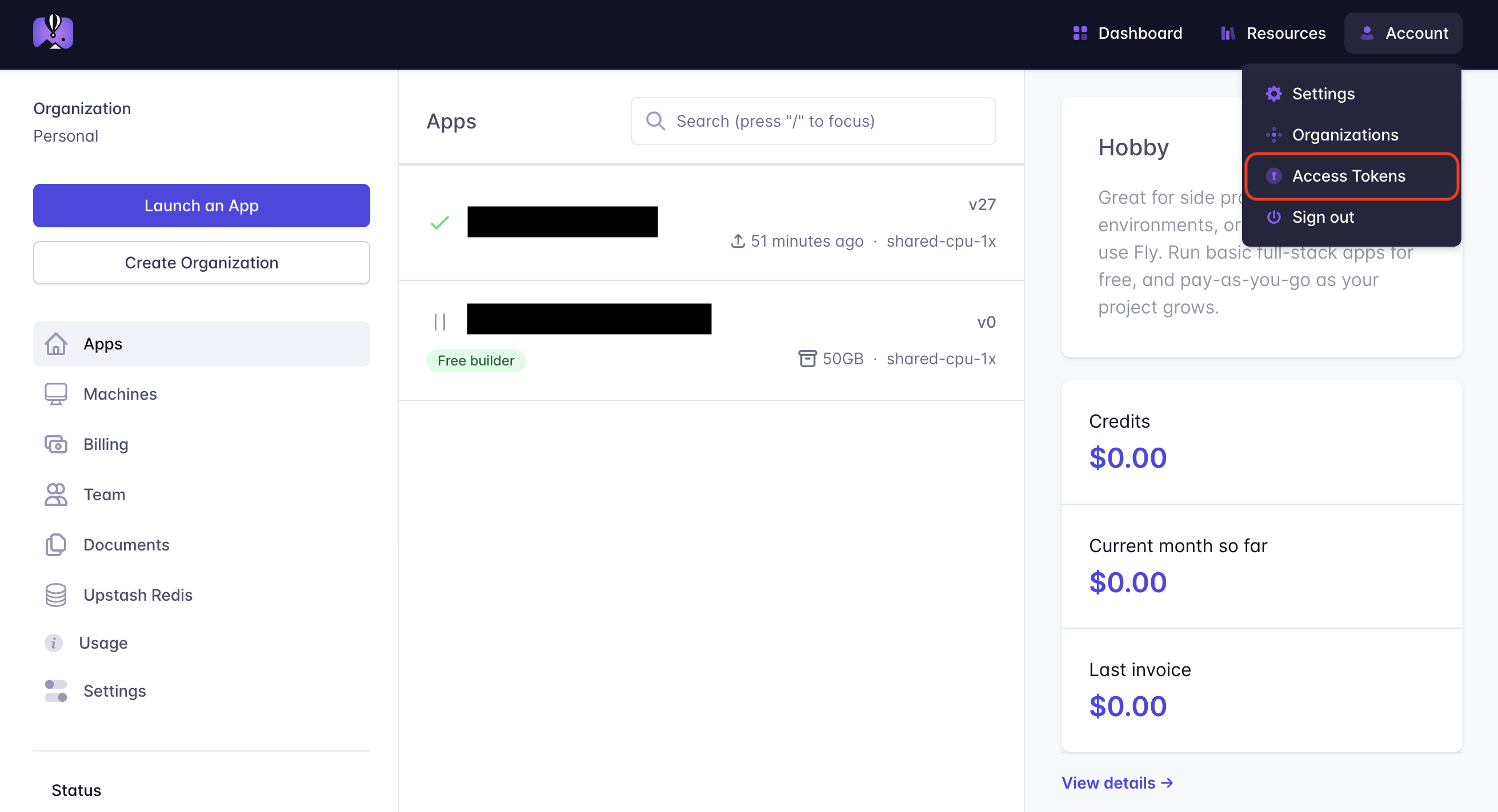
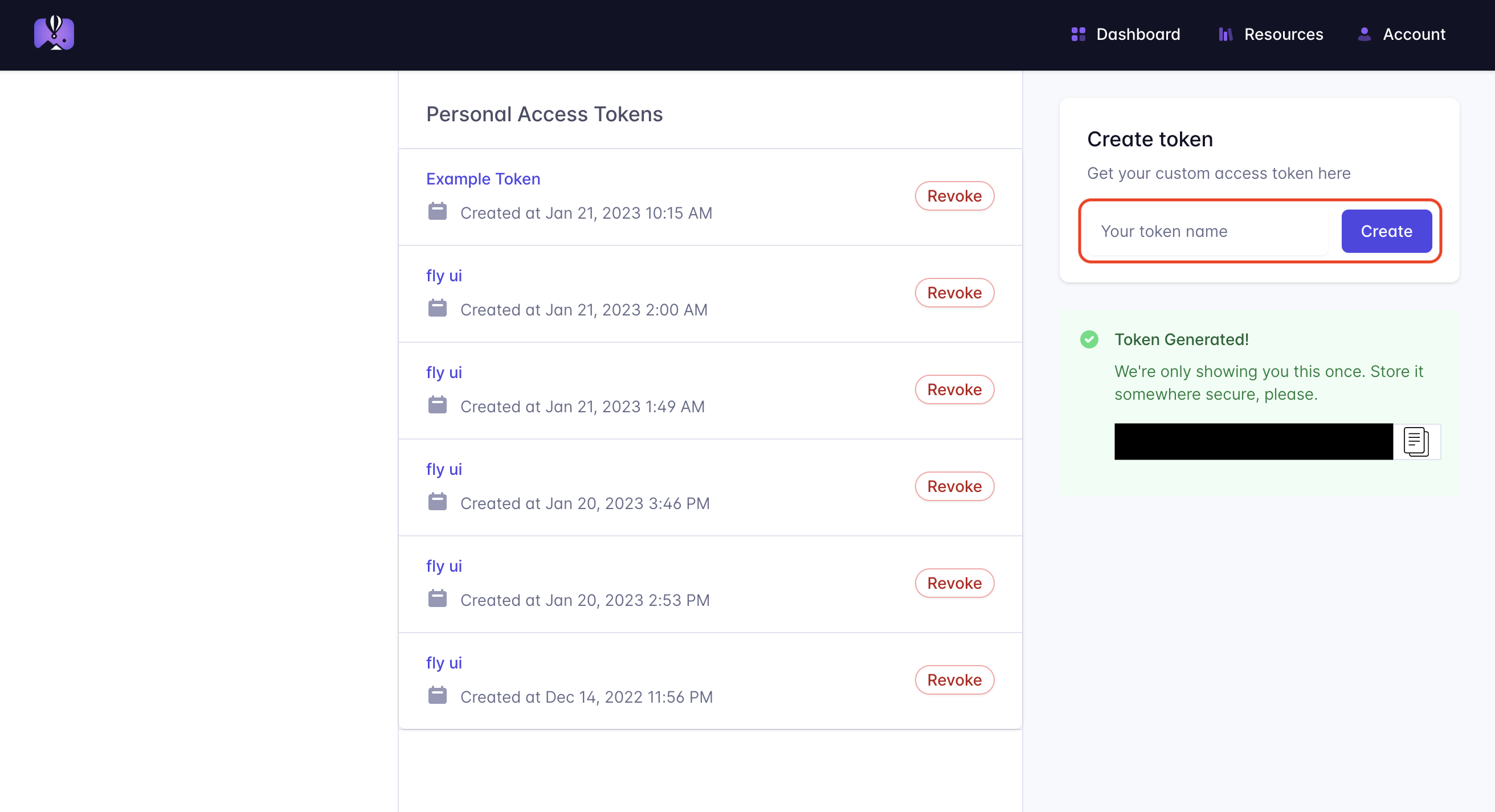
Navigate to your project's integrations tab in Infisical.
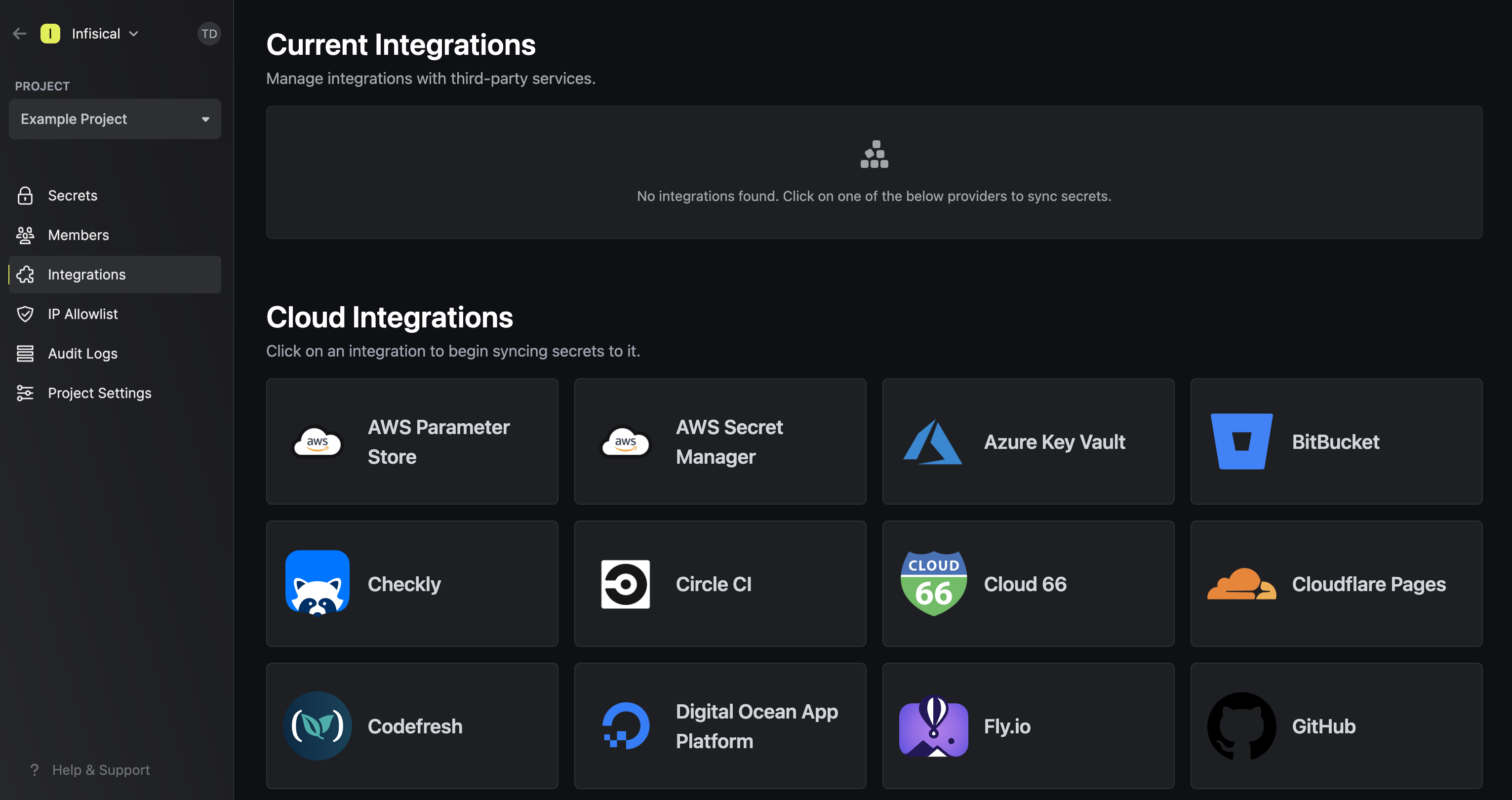
Press on the Fly.io tile and input your Fly.io access token to grant Infisical access to your Fly.io account.
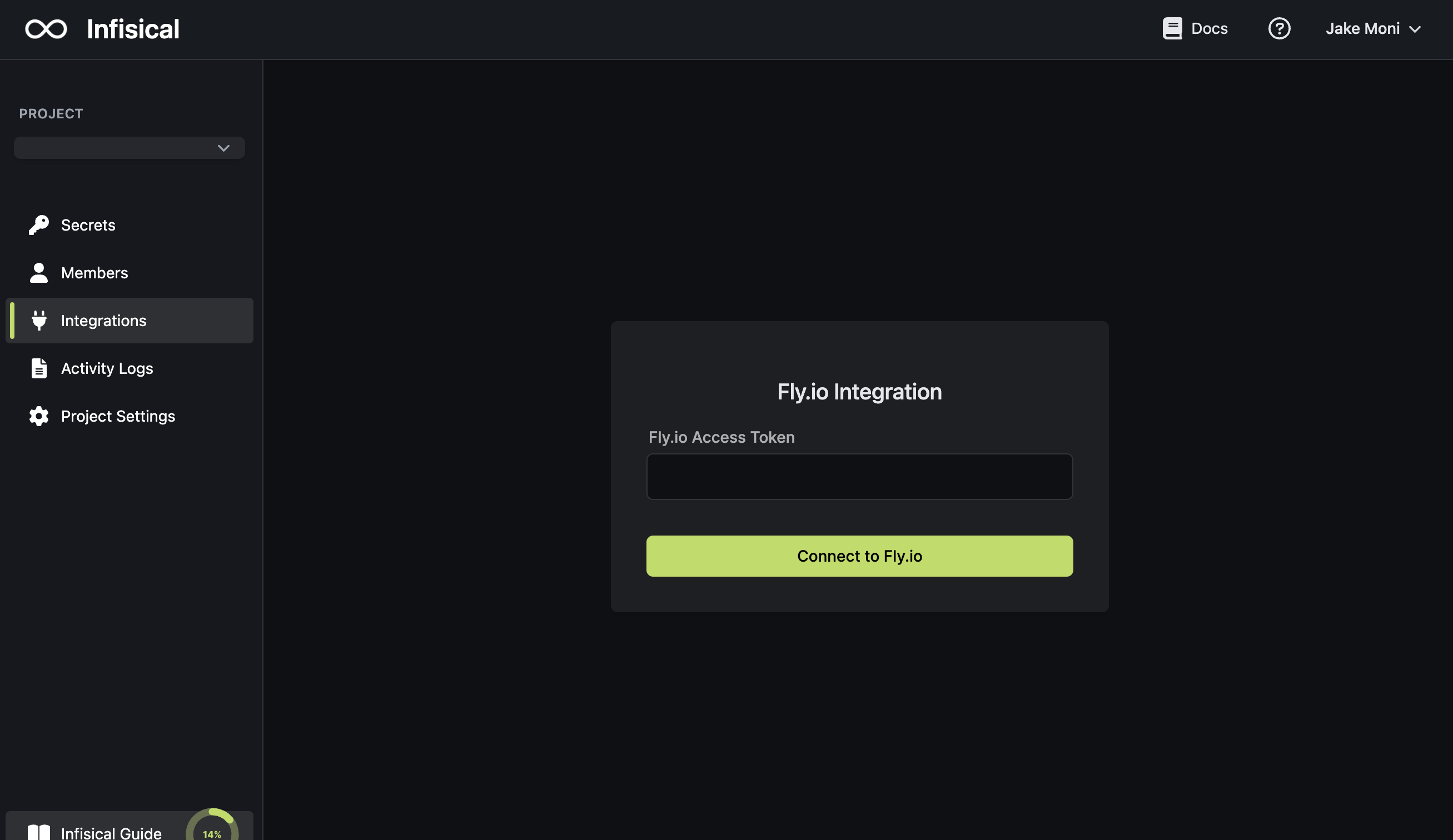
Select which Infisical environment secrets you want to sync to which Fly.io app and press create integration to start syncing secrets to Fly.io.
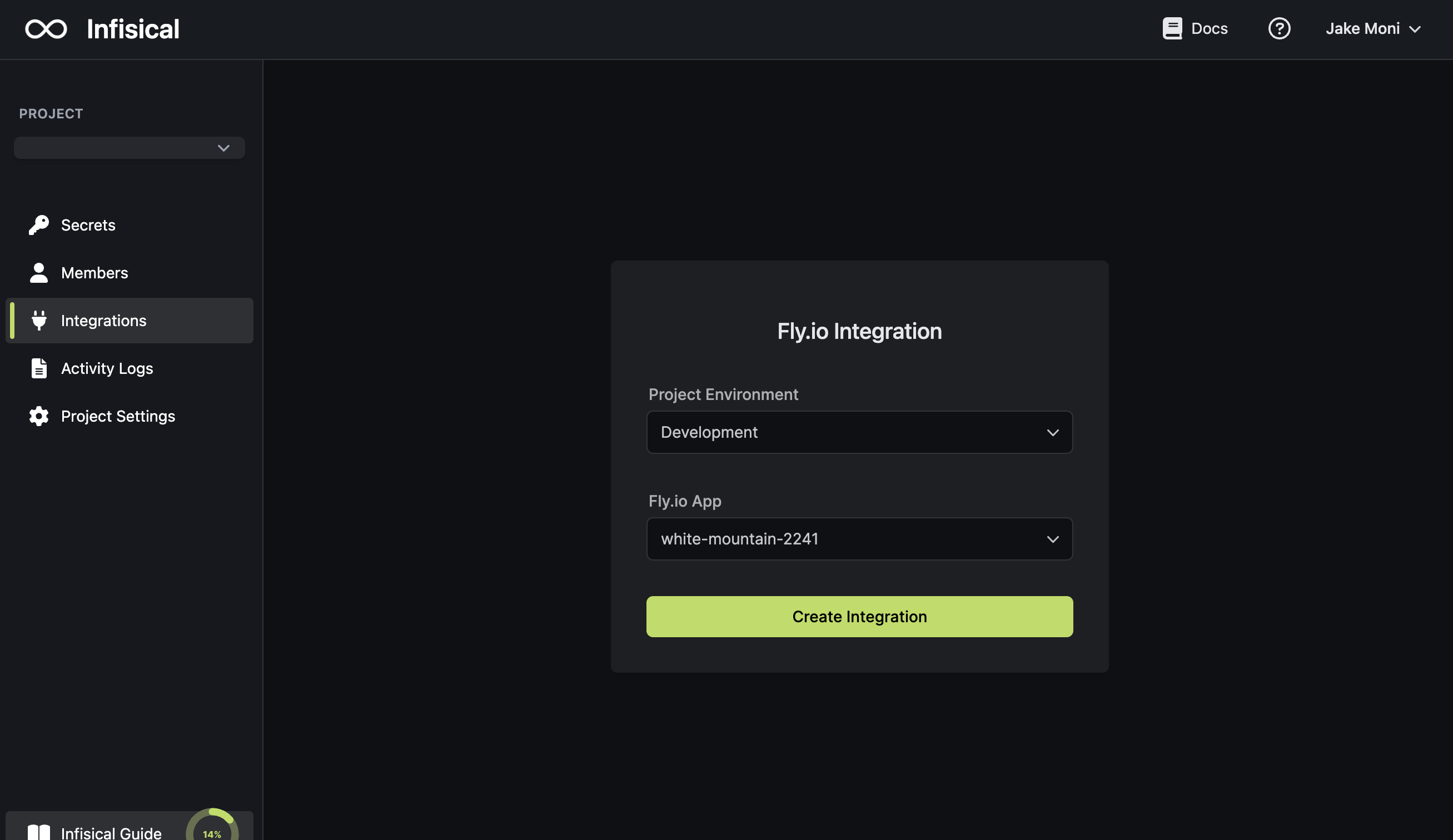
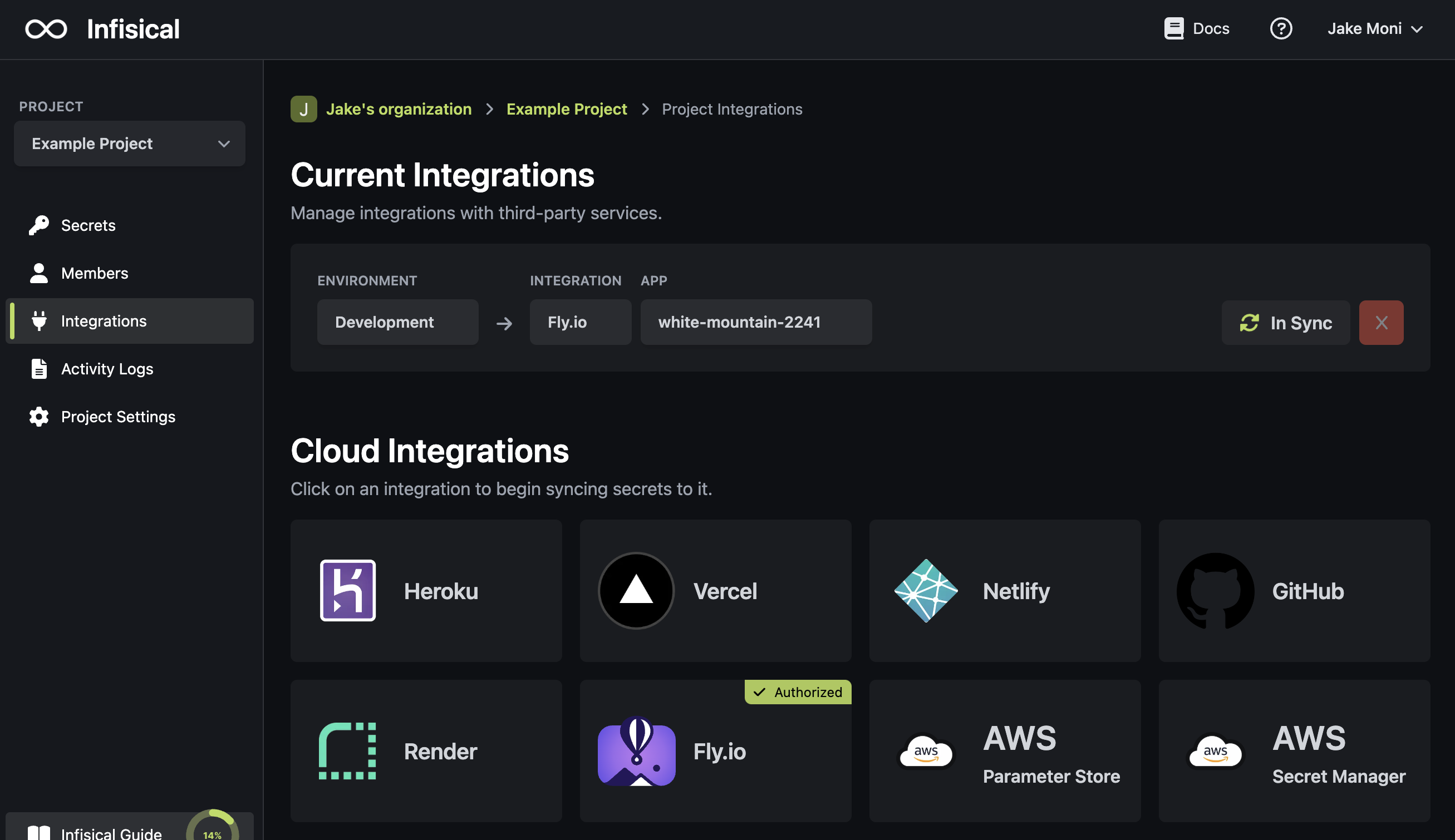
# GCP Secret Manager
How to sync secrets from Infisical to GCP Secret Manager
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
Navigate to your project's integrations tab in Infisical.
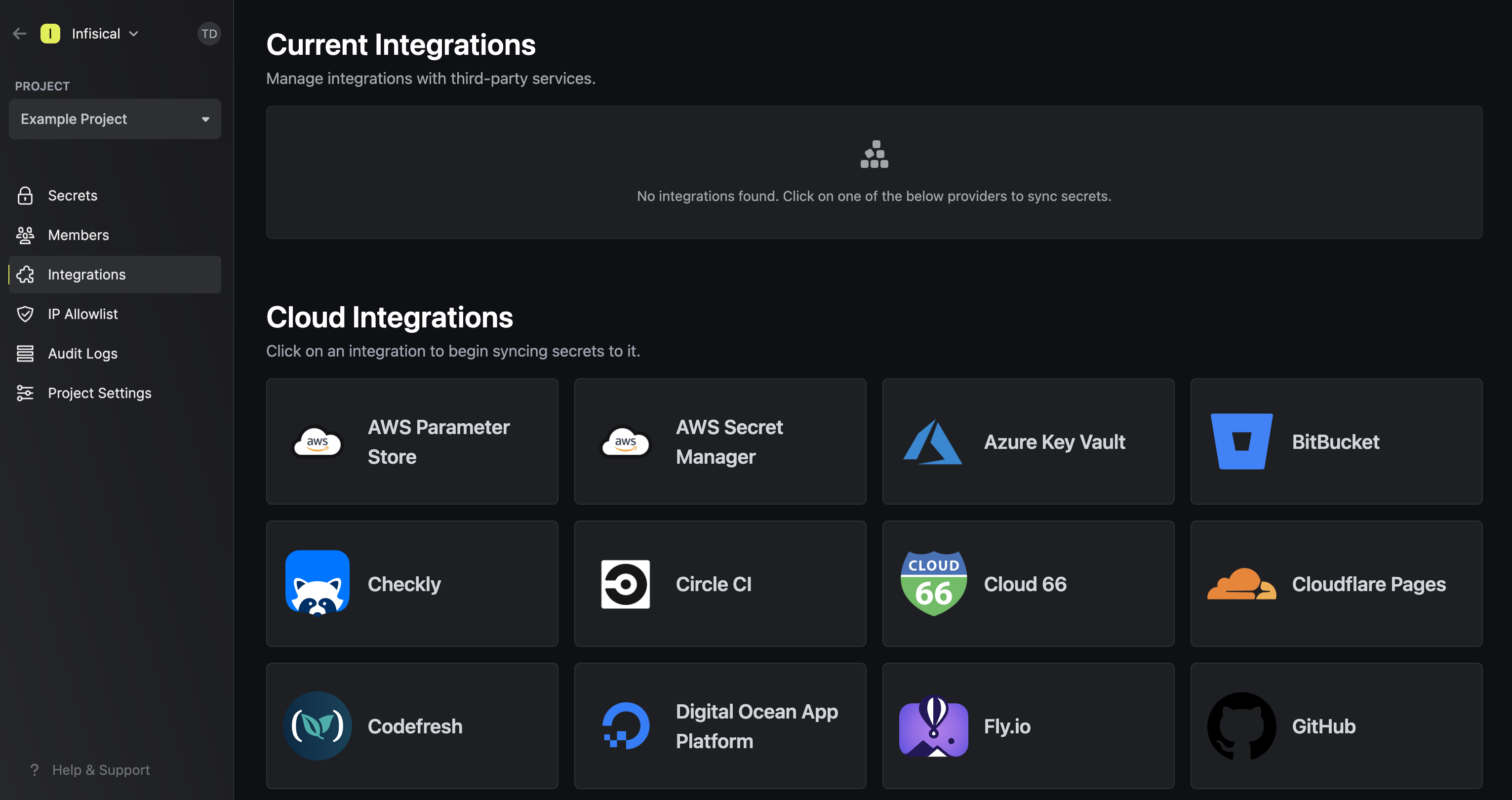
Press on the GCP Secret Manager tile and select **Continue with OAuth**
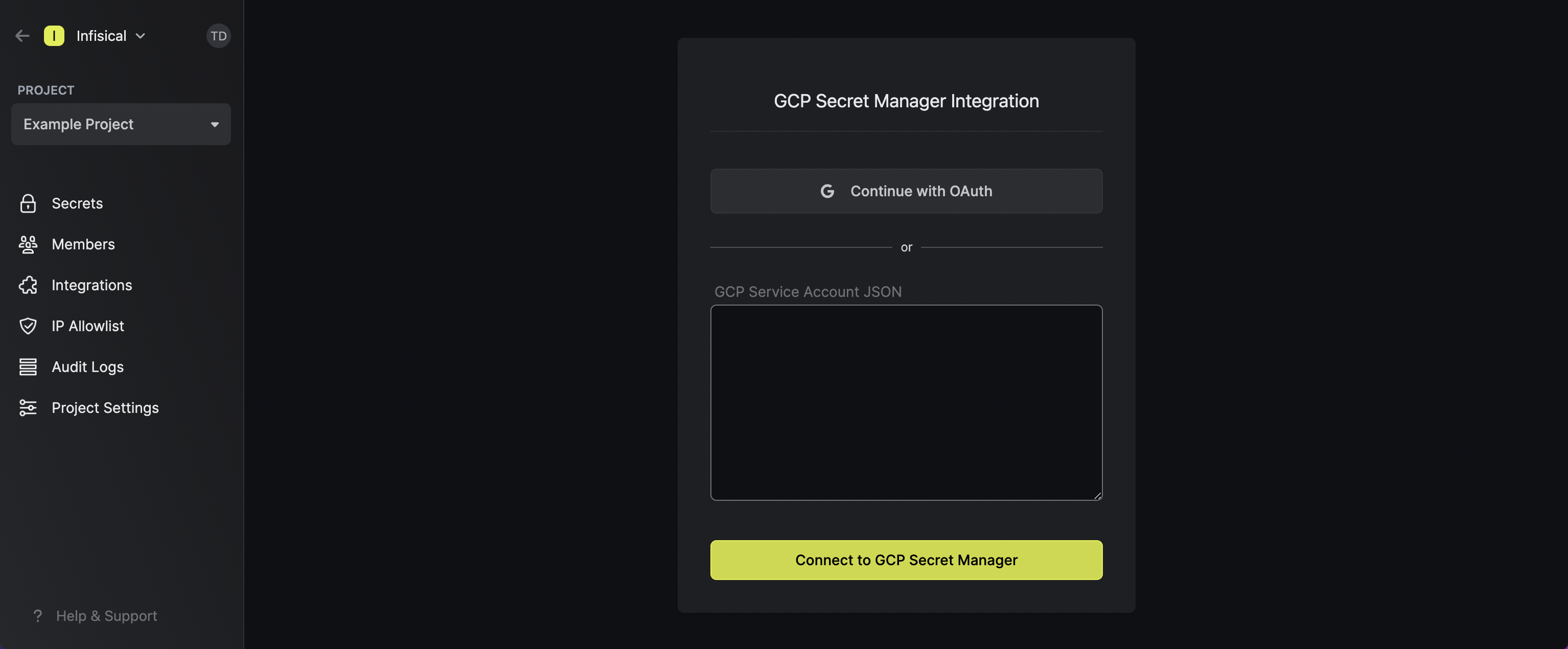
Grant Infisical access to GCP.
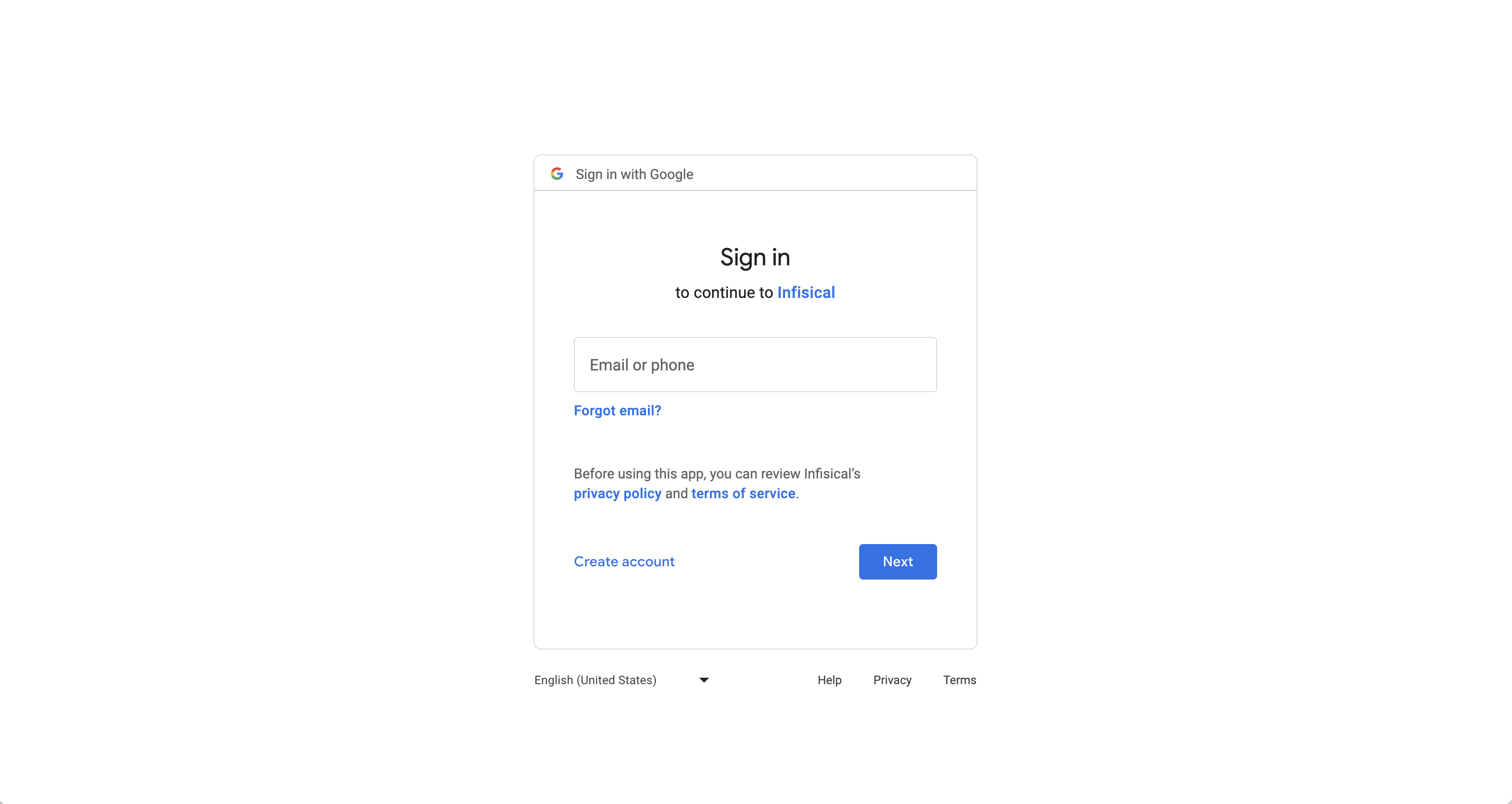
In the **Connection** tab, select which Infisical environment secrets you want to sync to which GCP secret manager project. Lastly, press create integration to start syncing secrets to GCP secret manager.
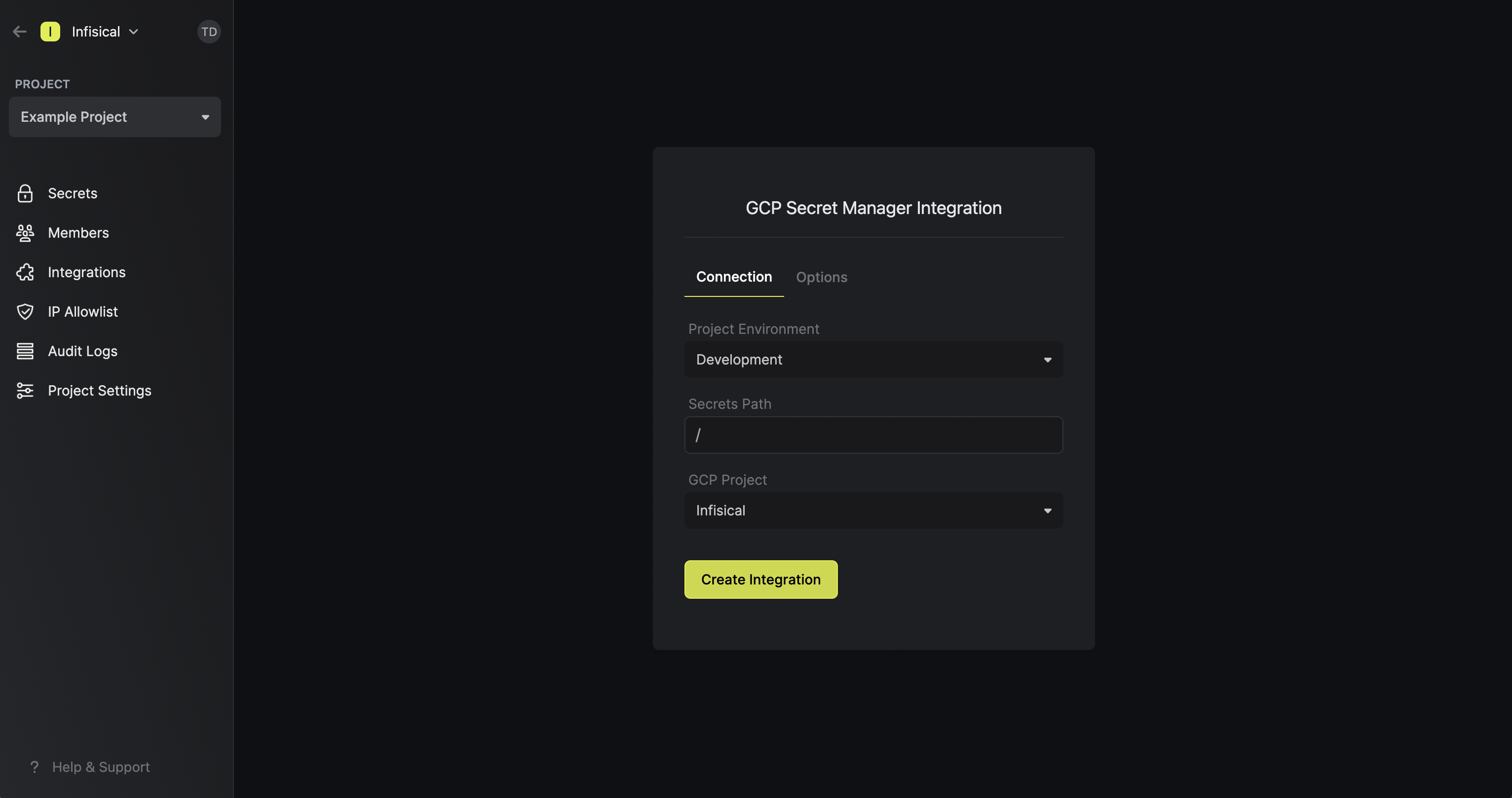
Note that the GCP Secret Manager integration supports a few options in the **Options** tab:
* Secret Prefix: If inputted, the prefix is appended to the front of every secret name prior to being synced.
* Secret Suffix: If inputted, the suffix to appended to the back of every name of every secret prior to being synced.
* Label in GCP Secret Manager: If selected, every secret will be labeled in GCP Secret Manager (e.g. as `managed-by:infisical`); labels can be customized.
Setting a secret prefix, suffix, or enabling the labeling option ensures that existing secrets in GCP Secret Manager are not overwritten during the sync. As part of this process, Infisical abstains from mutating any secrets in GCP Secret Manager without the specified prefix, suffix, or attached label.
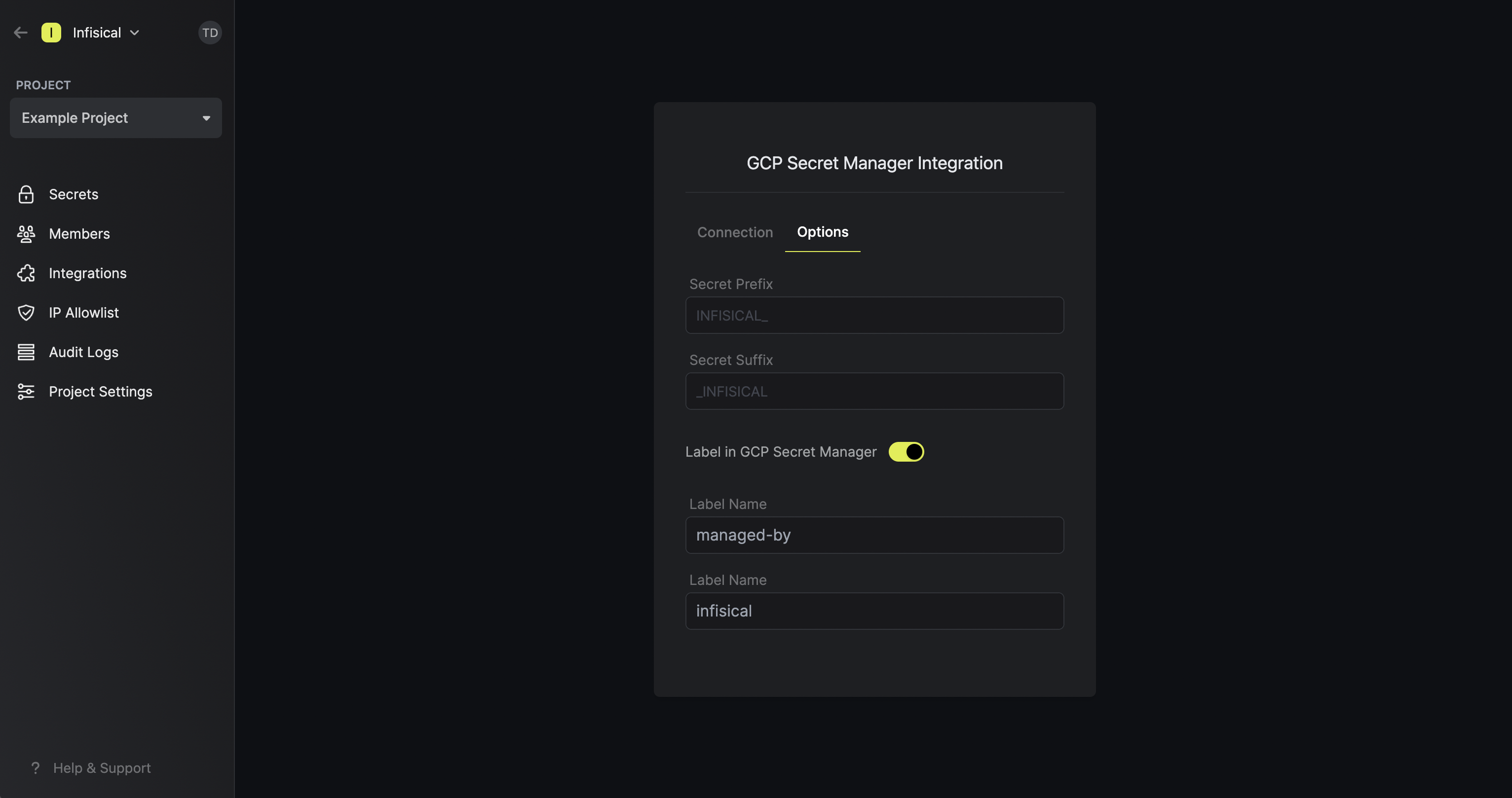
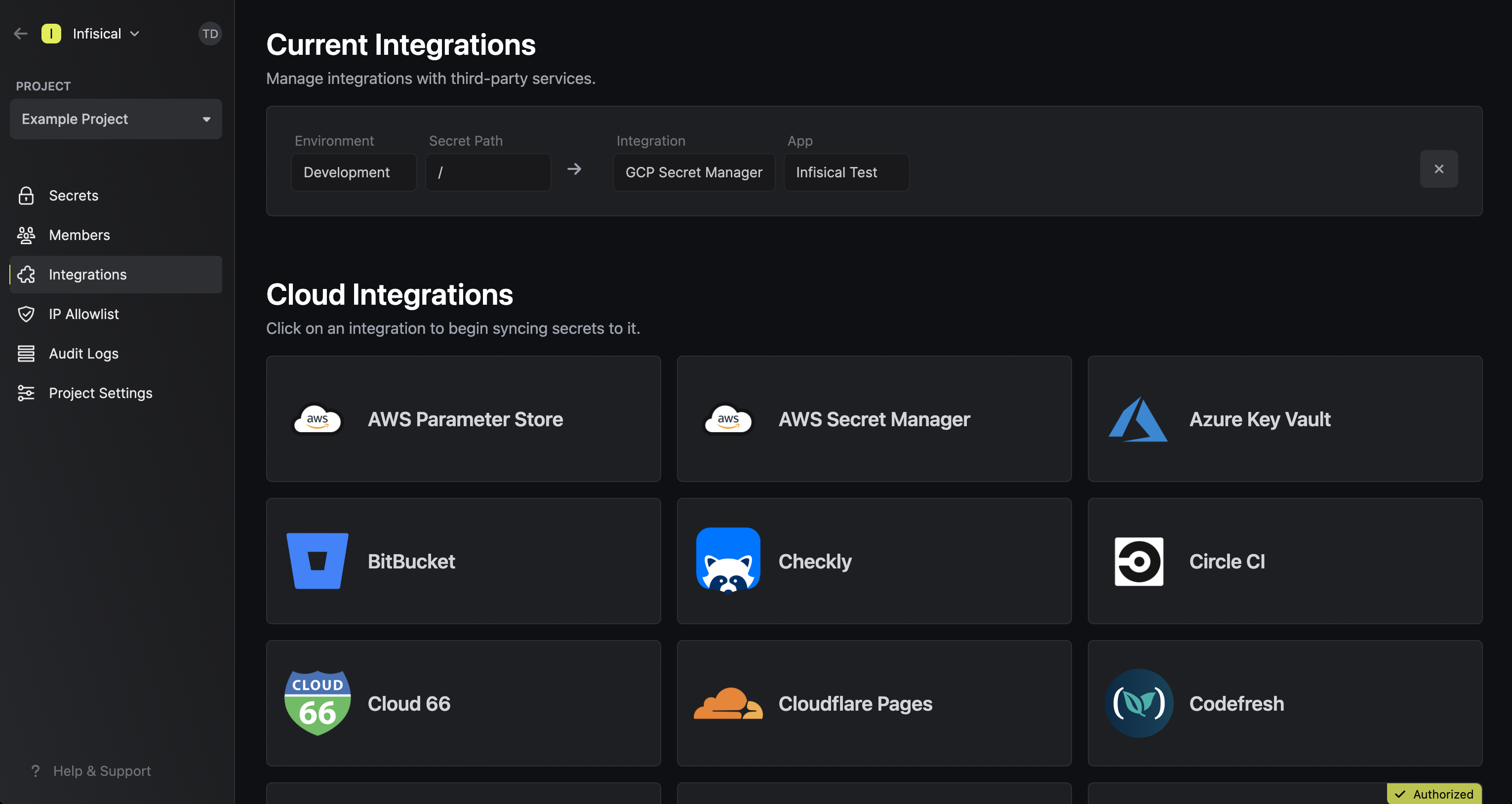
Using Infisical to sync secrets to GCP Secret Manager requires that you enable
the Service Usage API and Cloud Resource Manager API in the Google Cloud project you want to sync secrets to. More on that [here](https://cloud.google.com/service-usage/docs/set-up-development-environment).
Additionally, ensure that your GCP account has sufficient permission to manage secret and service resources (you can assign Secret Manager Admin and Service Usage Admin roles for testing purposes)
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
* Have a GCP project and have/create a [service account](https://cloud.google.com/iam/docs/service-account-overview) in it
Navigate to **IAM & Admin** page in GCP and add the **Secret Manager Admin** and **Service Usage Admin** roles to the service account.
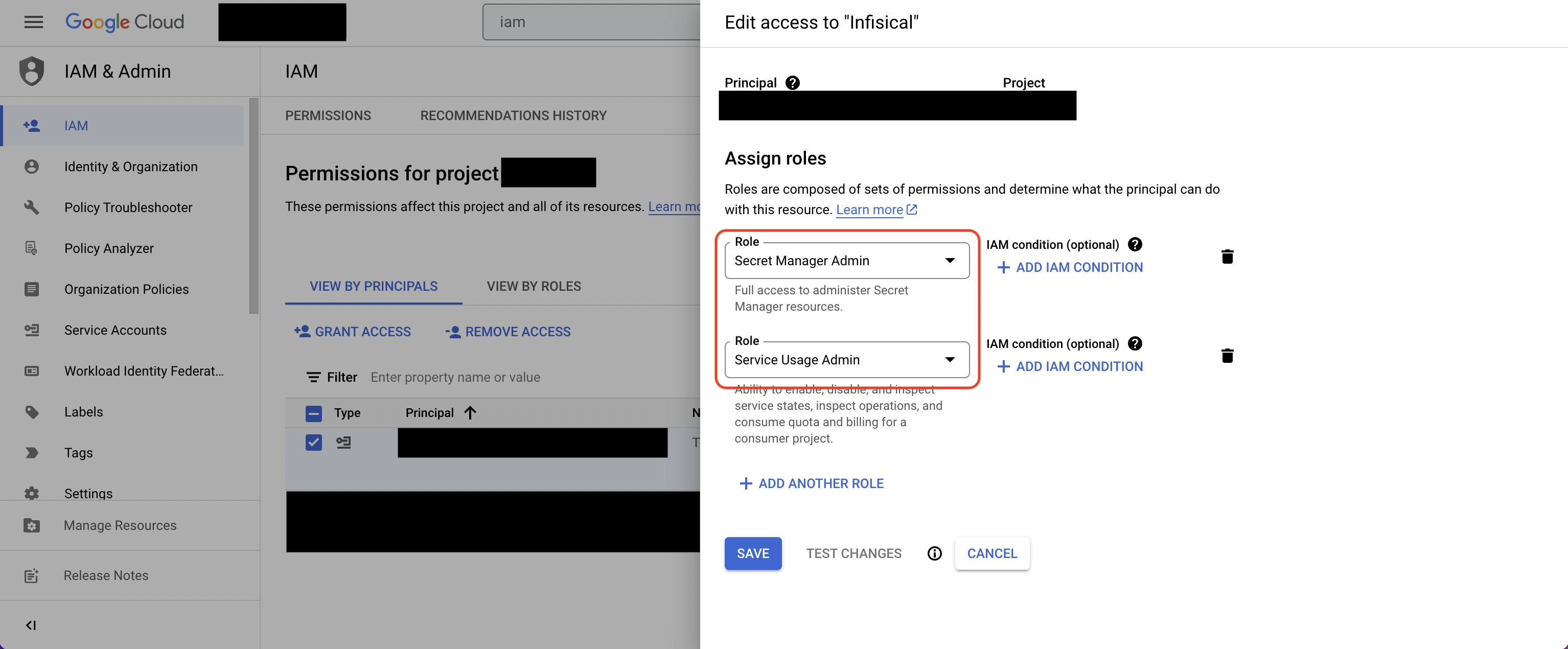
For enhanced security, you may want to assign more granular permissions to the service account. At minimum,
the service account should be able to read/write secrets from/to GCP Secret Manager (e.g. **Secret Manager Admin** role)
and list which GCP services are enabled/disabled (e.g. **Service Usage Admin** role).
Navigate to your project's integrations tab in Infisical.
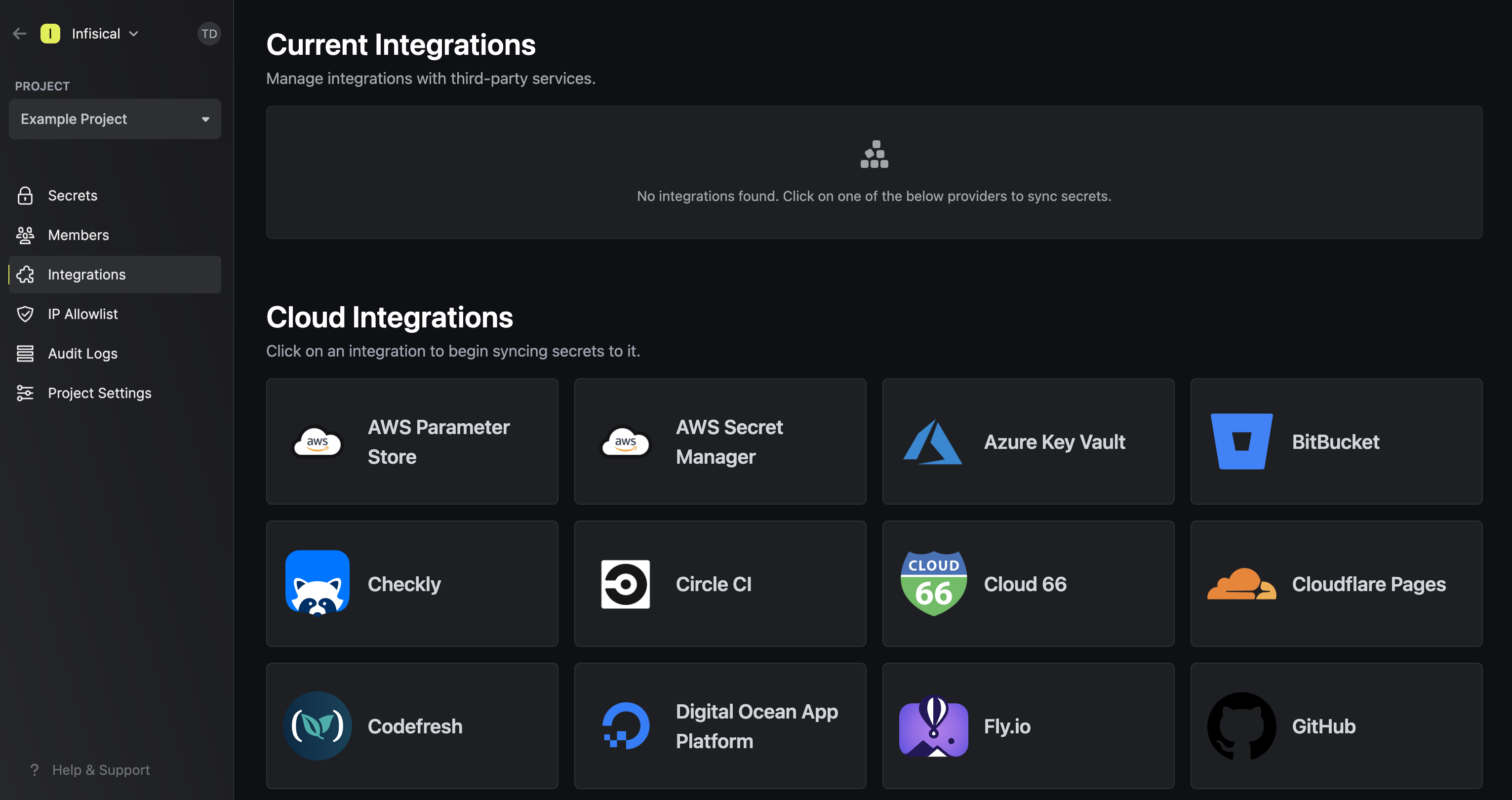
Press on the GCP Secret Manager tile and paste in your **GCP Service Account JSON** (you can create and download the JSON for your
service account in IAM & Admin > Service Accounts > Service Account > Keys).
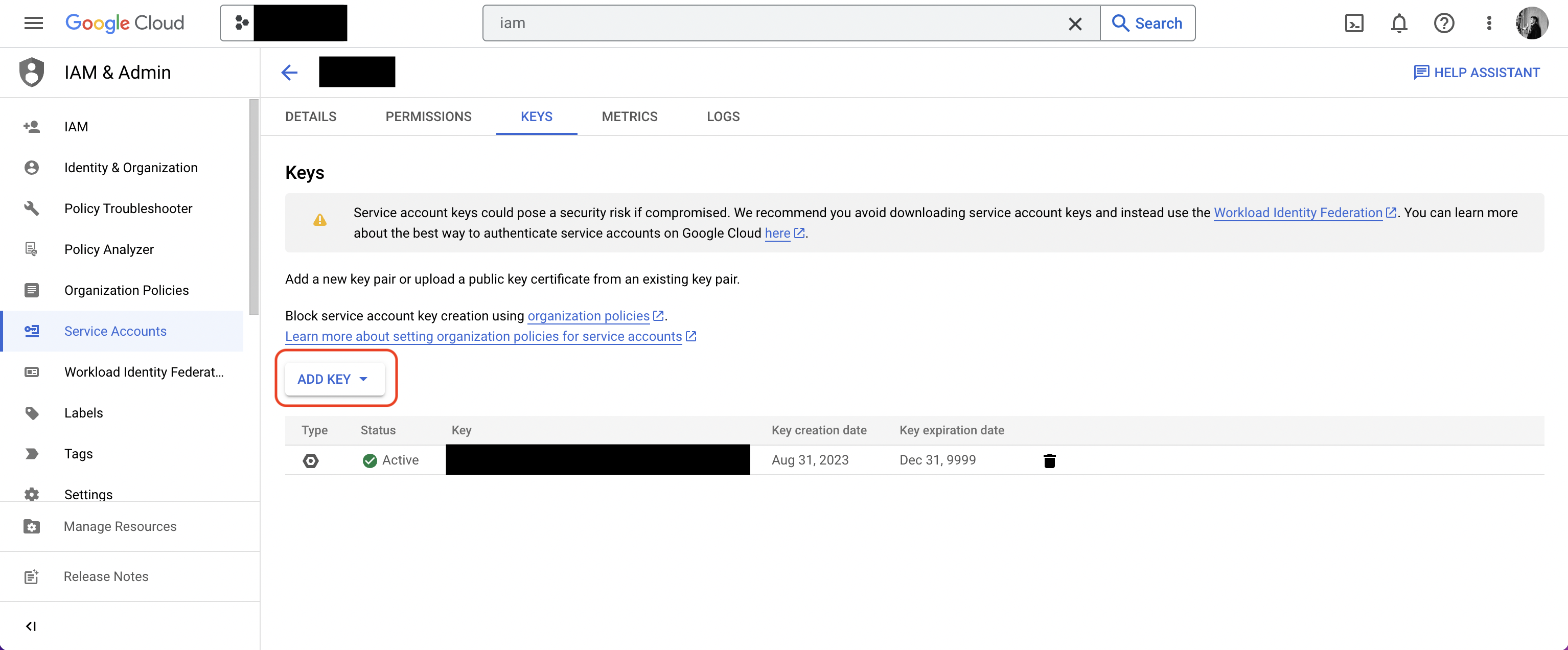
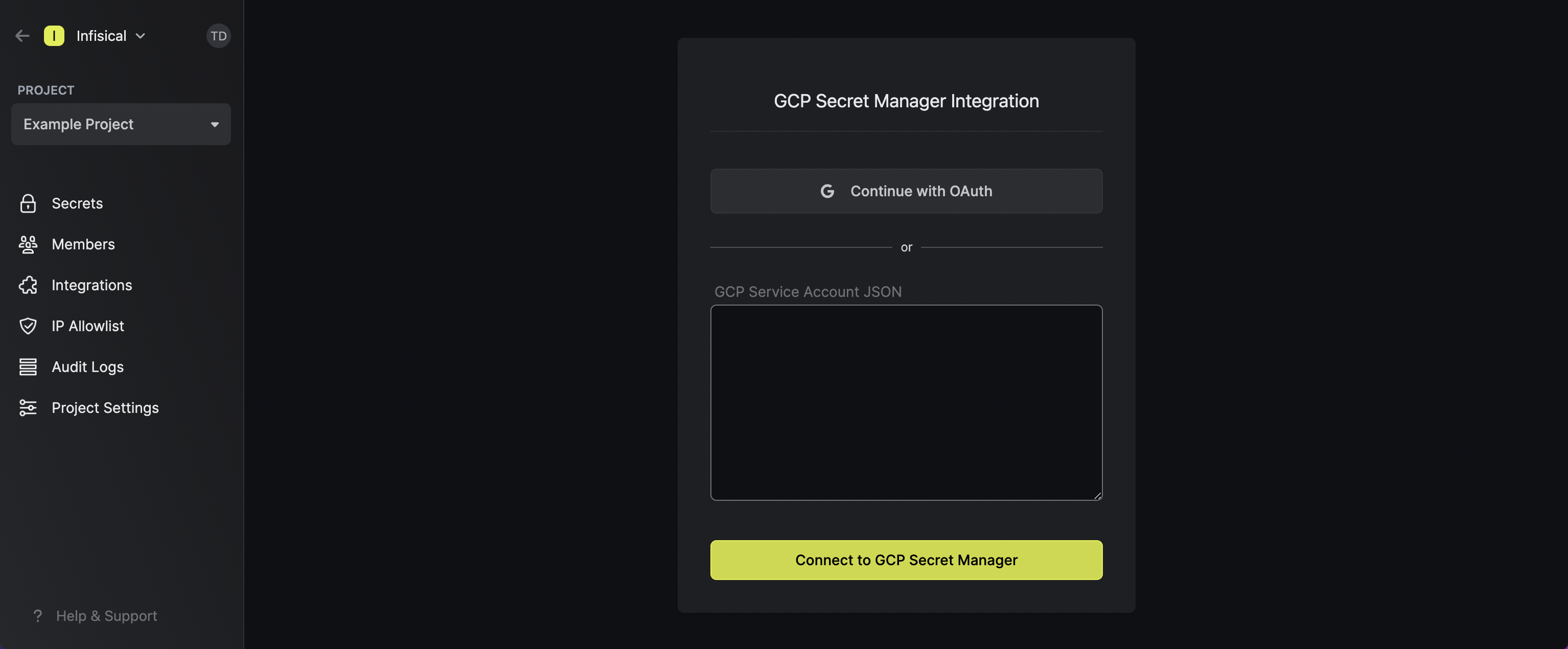
In the **Connection** tab, select which Infisical environment secrets you want to sync to the GCP secret manager project. Lastly, press create integration to start syncing secrets to GCP secret manager.
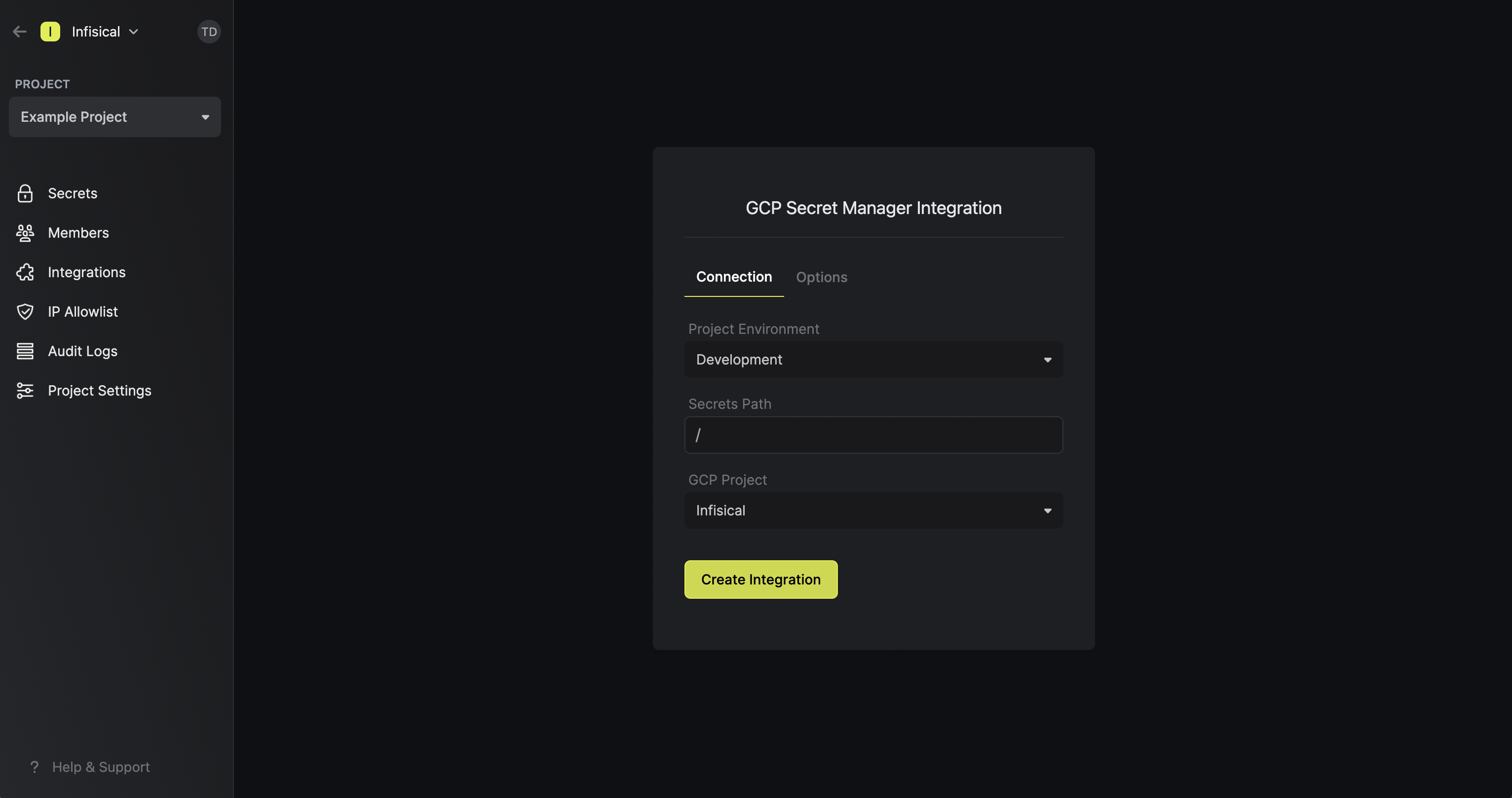
Note that the GCP Secret Manager integration supports a few options in the **Options** tab:
* Secret Prefix: If inputted, the prefix is appended to the front of every secret name prior to being synced.
* Secret Suffix: If inputted, the suffix to appended to the back of every name of every secret prior to being synced.
* Label in GCP Secret Manager: If selected, every secret will be labeled in GCP Secret Manager (e.g. as `managed-by:infisical`); labels can be customized.
Setting a secret prefix, suffix, or enabling the labeling option ensures that existing secrets in GCP Secret Manager are not overwritten during the sync. As part of this process, Infisical abstains from mutating any secrets in GCP Secret Manager without the specified prefix, suffix, or attached label.
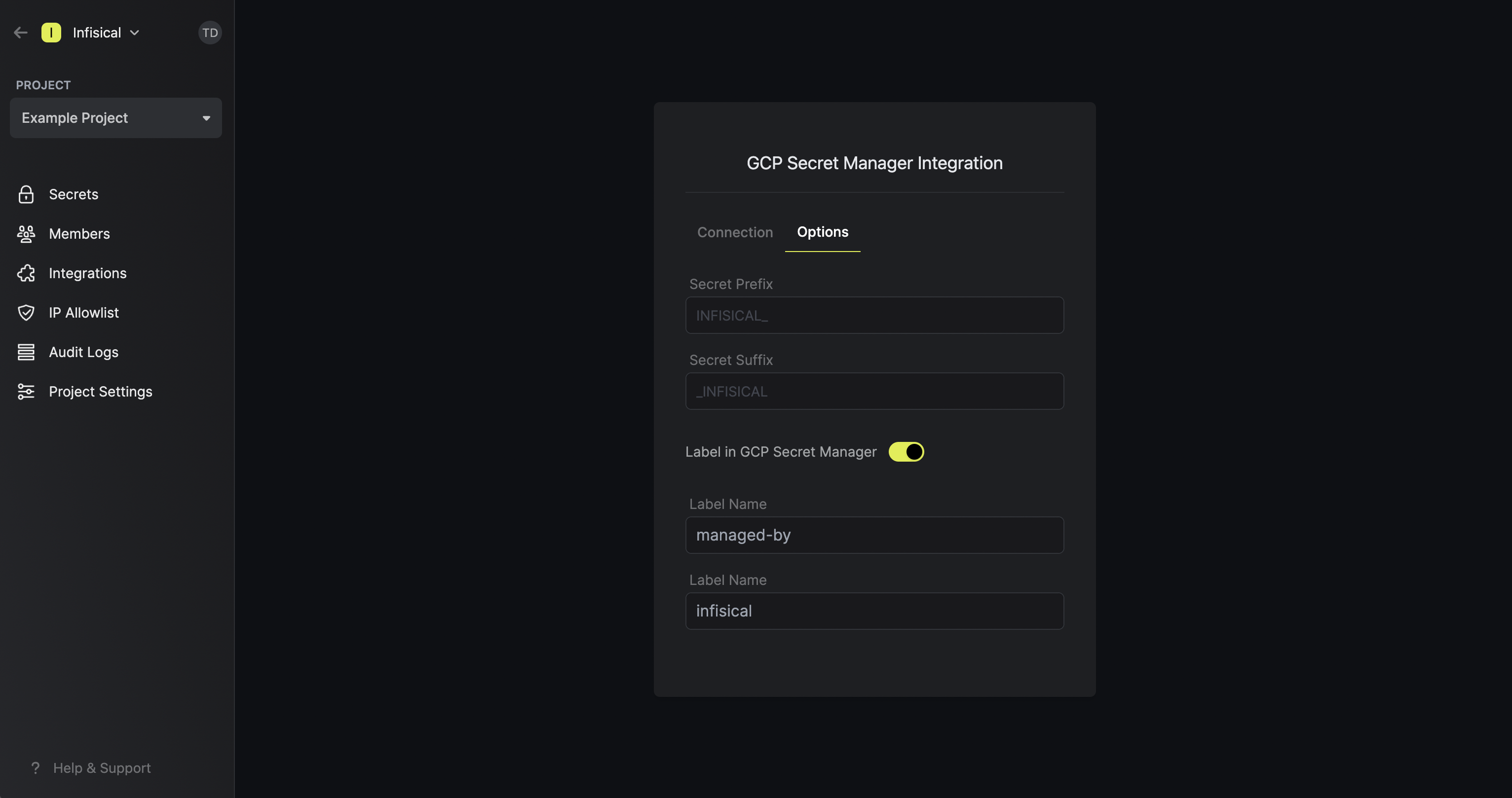
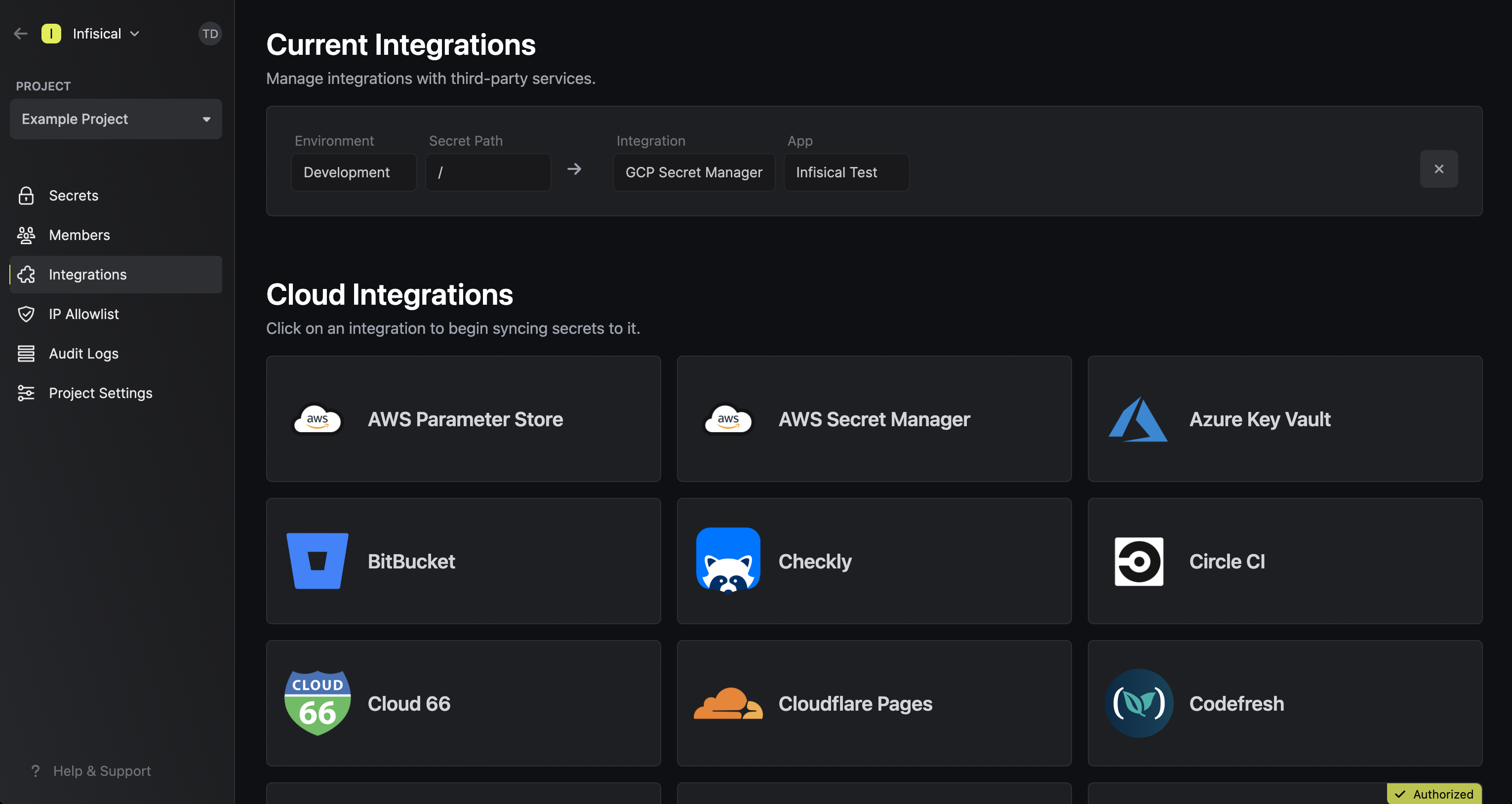
Using Infisical to sync secrets to GCP Secret Manager requires that you enable
the Service Usage API and Cloud Resource Manager API in the Google Cloud project you want to sync secrets to. More on that [here](https://cloud.google.com/service-usage/docs/set-up-development-environment).
Using the GCP Secret Manager integration (via the OAuth2 method) on a self-hosted instance of Infisical requires configuring an OAuth2 application in GCP
and registering your instance with it.
Navigate to your project API & Services > Credentials to create a new OAuth2 application.
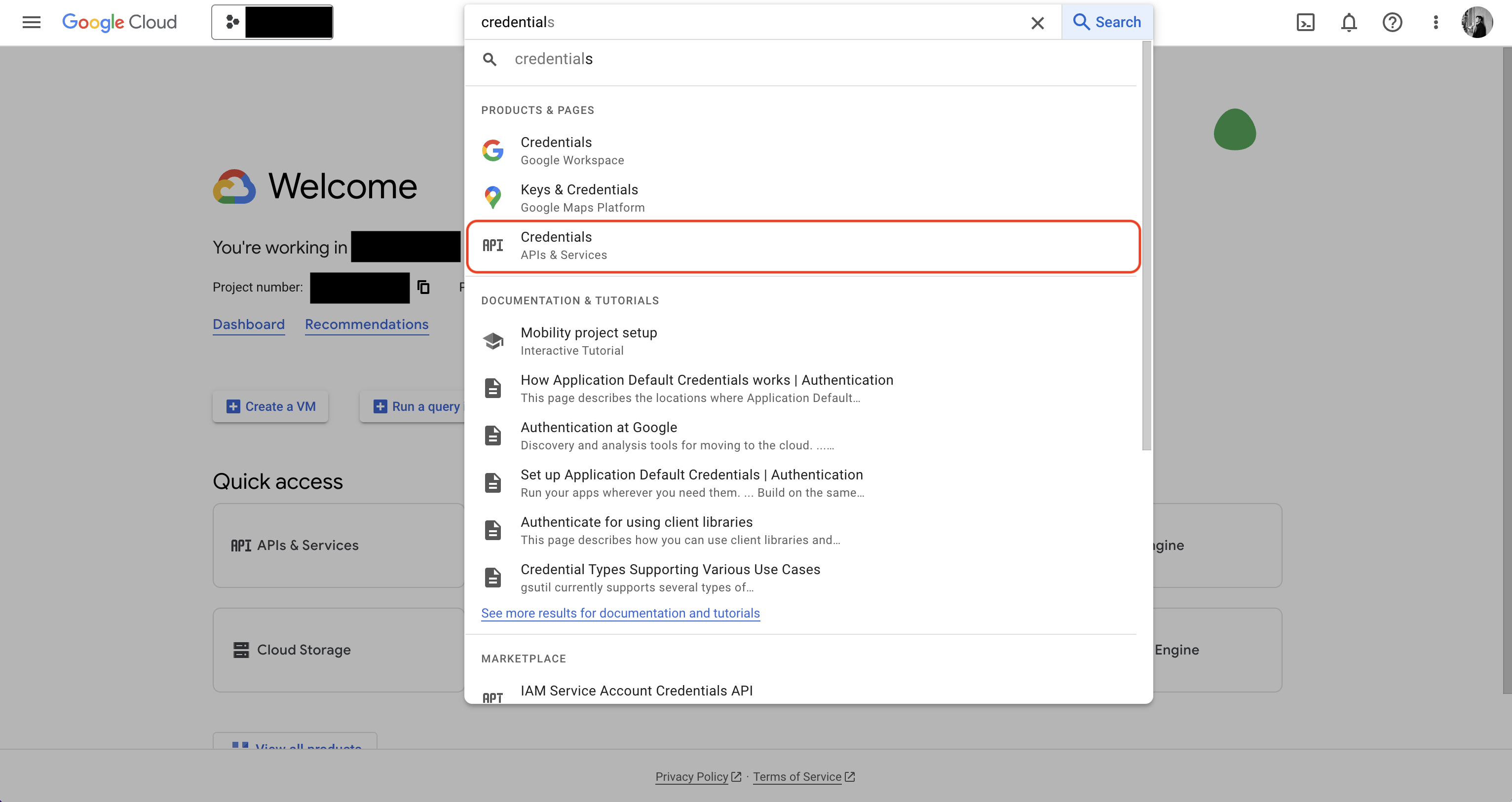
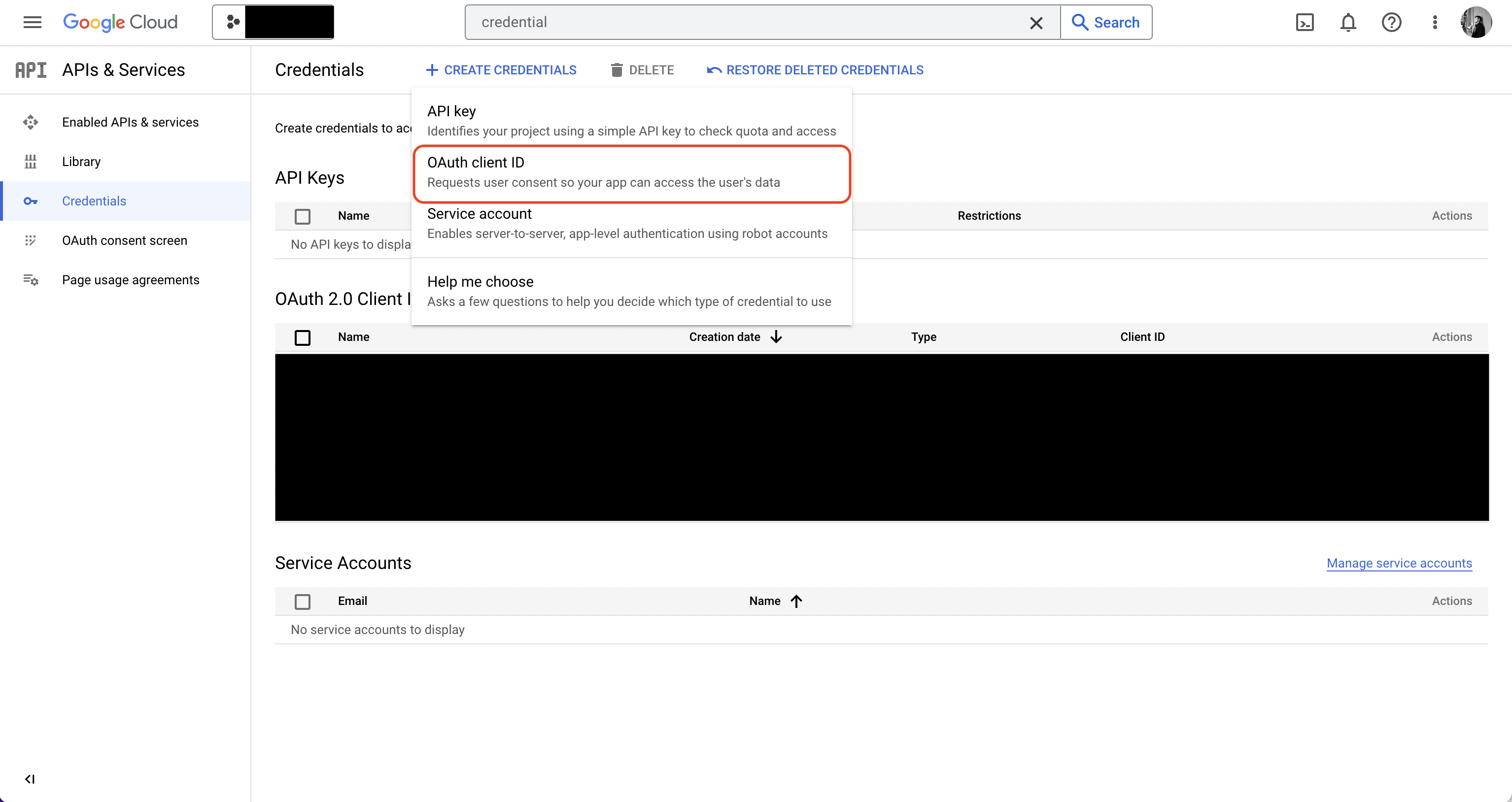
Create the application. As part of the form, add to **Authorized redirect URIs**: `https://your-domain.com/integrations/gcp-secret-manager/oauth2/callback`.
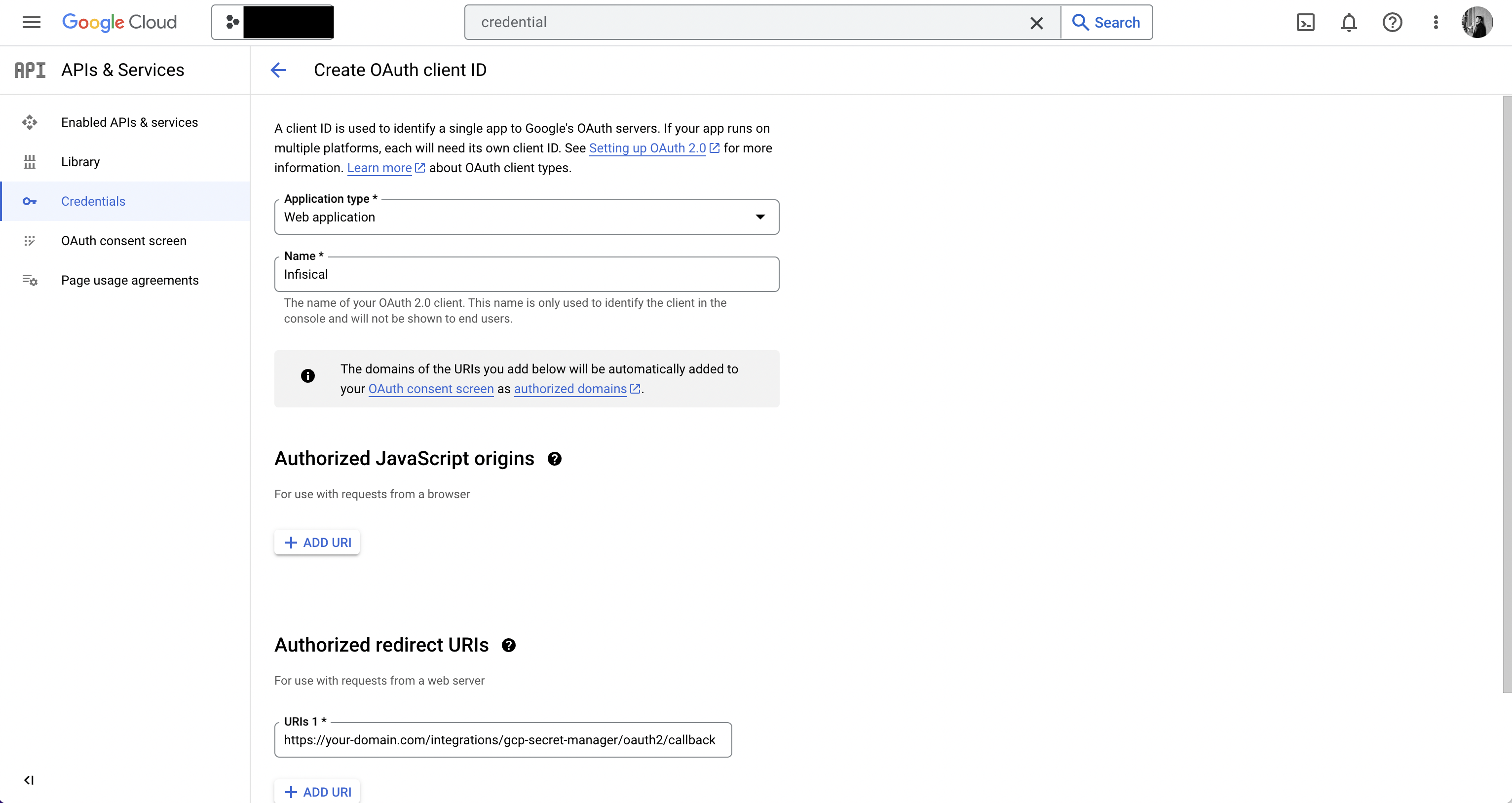
Obtain the **Client ID** and **Client Secret** for your GCP OAuth2 application.
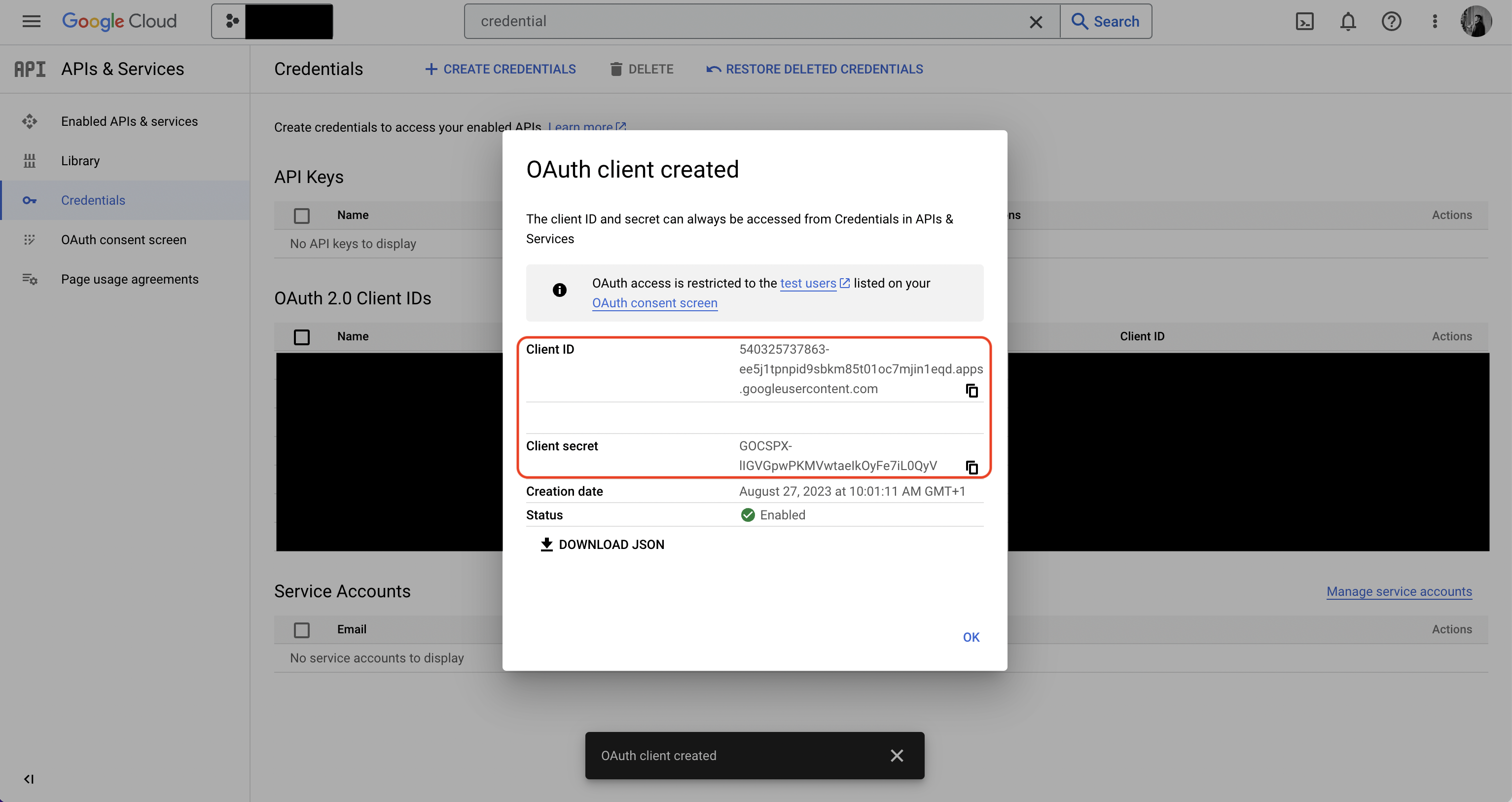
Back in your Infisical instance, add two new environment variables for the credentials of your GCP OAuth2 application:
* `CLIENT_ID_GCP_SECRET_MANAGER`: The **Client ID** of your GCP OAuth2 application.
* `CLIENT_SECRET_GCP_SECRET_MANAGER`: The **Client Secret** of your GCP OAuth2 application.
Once added, restart your Infisical instance and use the GCP Secret Manager integration.
# HashiCorp Vault
How to sync secrets from Infisical to HashiCorp Vault
Infisical connects to Vault via the AppRole auth method.
Currently, each Infisical project can only point and sync secrets to one Vault cluster / namespace
but with unlimited integrations to different paths within it.
This tutorial makes use of Vault's UI but, in principle, instructions can executed via
Vault CLI or API call.
Lastly, you should note that we provide a simple use-case and, in practice, you should adapt and extend it to your own Vault use-case and follow best practices, for instance when defining fine-grained ACL policies.
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
* Have experience with [HashiCorp Vault](https://www.vaultproject.io/).
## Navigate to your project's integrations tab
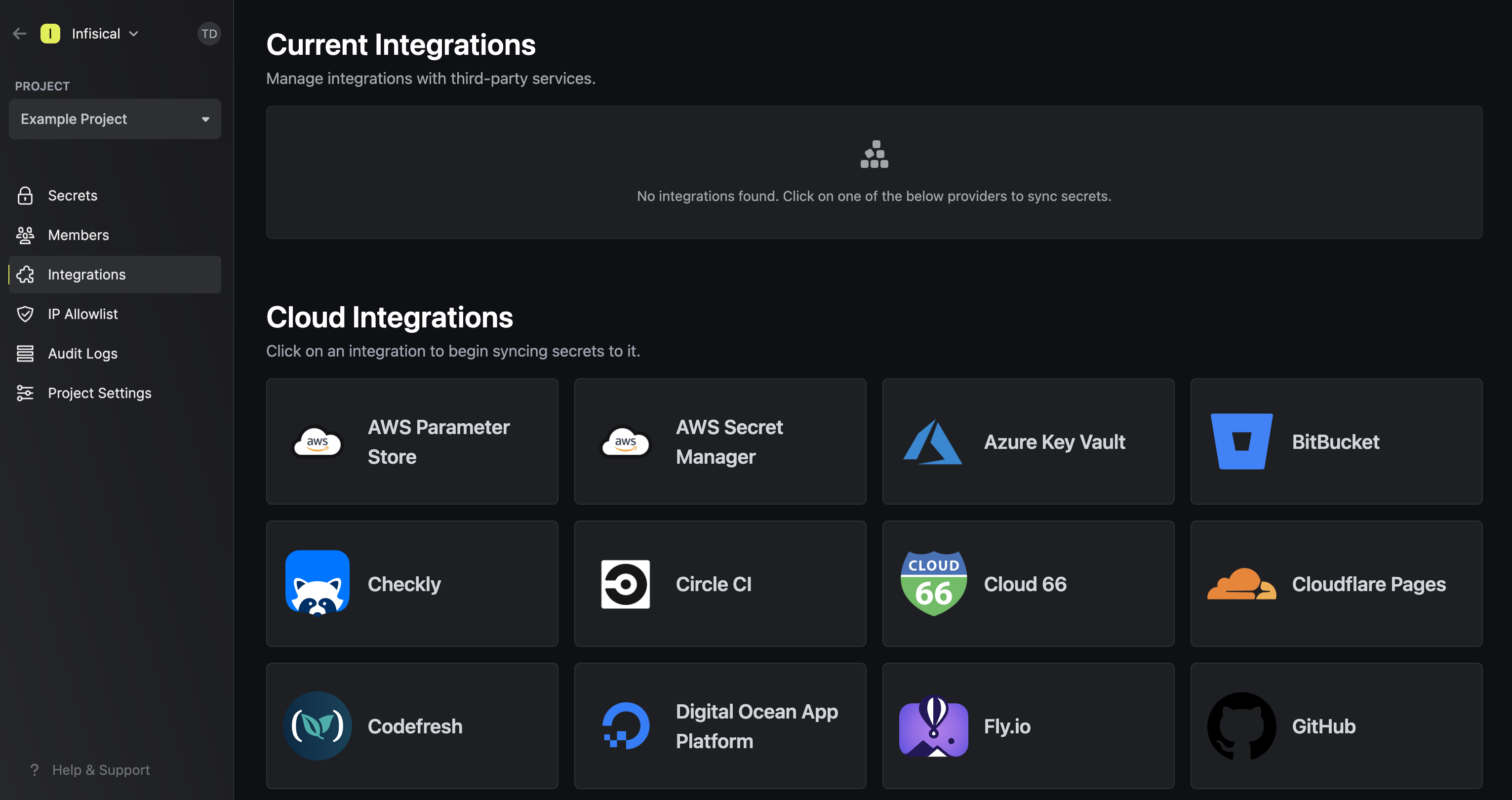
## Prepare Vault
This section mirrors the latter parts of the [Vault quickstart](https://developer.hashicorp.com/vault/tutorials/cloud/getting-started-intro) provided by HashiCorp and uses sample names/values for demonstration.
To begin, navigate to the cluster / namespace that you want to sync secrets to in Vault; we'll use the default `admin` namespace (in practice, we recommend creating a namespace and not using the default `admin` namespace).
### Enable KV Secrets Engine
In Secrets, enable a KV Secrets Engine at a path for Infisical to sync secrets to; we'll use the path `kv`.



### Enable the AppRole auth method
In Access > Auth Methods, enable the AppRole auth method.



### Create an ACL Policy
Now in Policies, create a new ACL policy scoped to the path(s) you wish Infisical to be able to sync secrets to.
We'll call the policy `test` and have it grant access to the `dev` path in the KV Secrets Engine where we will be syncing secrets to from Infisical.
```console
path "kv/data/dev" {
capabilities = [ "create", "read", "update" ]
}
path "sys/namespaces/*" {
capabilities = [ "create", "read", "update", "delete", "list" ]
}
```
`kv` comes from the path of the KV Secrets Engine that we enabled and `dev` is the chosen path within it
that we want to sync secrets to.



### Create a role with the policy attached
We now create a `infisical` role with the generated token's time-to-live (TTL) set to 1 hour and can be renewed for up to 4 hours from the time of its creation.
1. Click the Vault CLI shell icon (`>_`) to open a command shell in the browser.

2. Copy the command below.
```console
vault write auth/approle/role/infisical token_policies="test" token_ttl=1h token_max_ttl=4h
```
3. Paste the command into the command shell in the browser and press the enter button.
### Generate a RoleID and SecretID
Finally, we need to generate a **RoleID** and **SecretID** (like a username and password) that Infisical can use
to authenticate with Vault.
1. Click the Vault CLI shell icon (>\_) again to open a command shell.
2. Read the RoleID.
```console
vault read auth/approle/role/infisical/role-id
```
Example output:
```console
Key Value
role_id b6ccdcca-183b-ce9c-6b98-b556b9a0edb9
```
3. Generate a new SecretID of the `infisical` role.
```console
vault write -force auth/approle/role/infisical/secret-id
```
Example output:
```console
Key Value
secret_id 735a47cc-7a98-77cc-0128-12b1e96a4157
secret_id_accessor 3ab305d1-1eab-df4b-4079-ef7135635c49
...snip...
```
Great. We're now ready to connect Infisical to Vault!
## Enter your Vault instance and authentication details
Back in Infisical, press on the HashiCorp Vault tile and input your Vault instance and `infisical` role RoleID and SecretID.

For additional details on each field:
* Vault Cluster URL: The address of your cluster, either HCP or self-hosted.
If using HCP, you can copy your Cluster URL in the Cluster Overview:

* Vault Namespace: The Vault namespace you wish to connect to.
* Vault RoleID: The RoleID previously created for the `infisical` role.
* Vault SecretID: The SecretID previously created for the `infisical` role.
## Start integration
Select which Infisical environment secrets you want to sync to Vault.
For additional details on each field:
* Vault KV Secrets Engine Path: the path at which you enabled the intended KV Secrets Engine; in this demonstration, we used `kv`.
* Vault Secret(s) Path: the path in the KV Secrets Engine that you wish to sync secrets to.
Press create integration to start syncing secrets to Vault.


# Hasura Cloud
How to sync secrets from Infisical to Hasura Cloud
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
Obtain a Hasura Cloud Access Token in My Account > Access Tokens
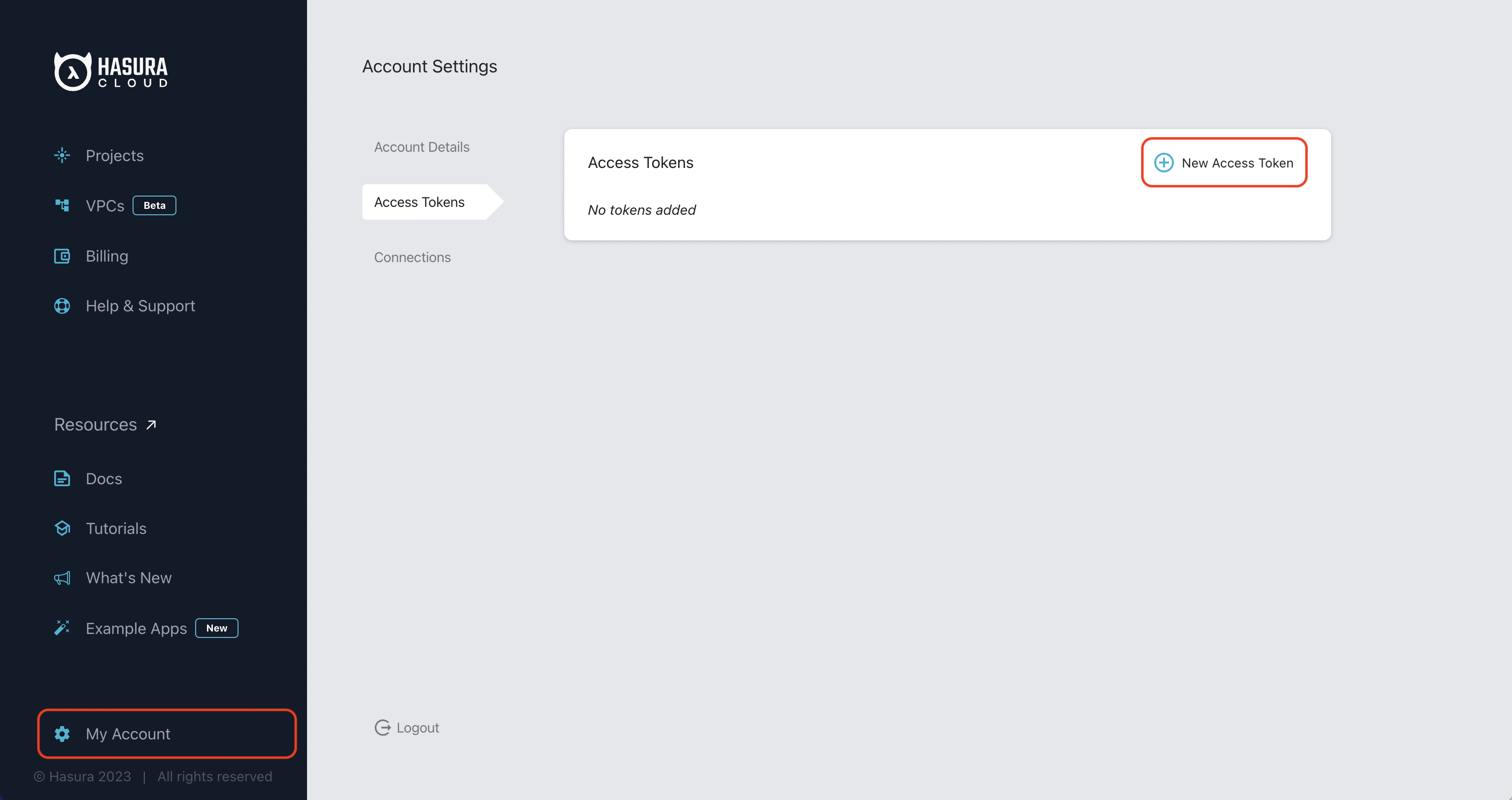
Navigate to your project's integrations tab in Infisical.
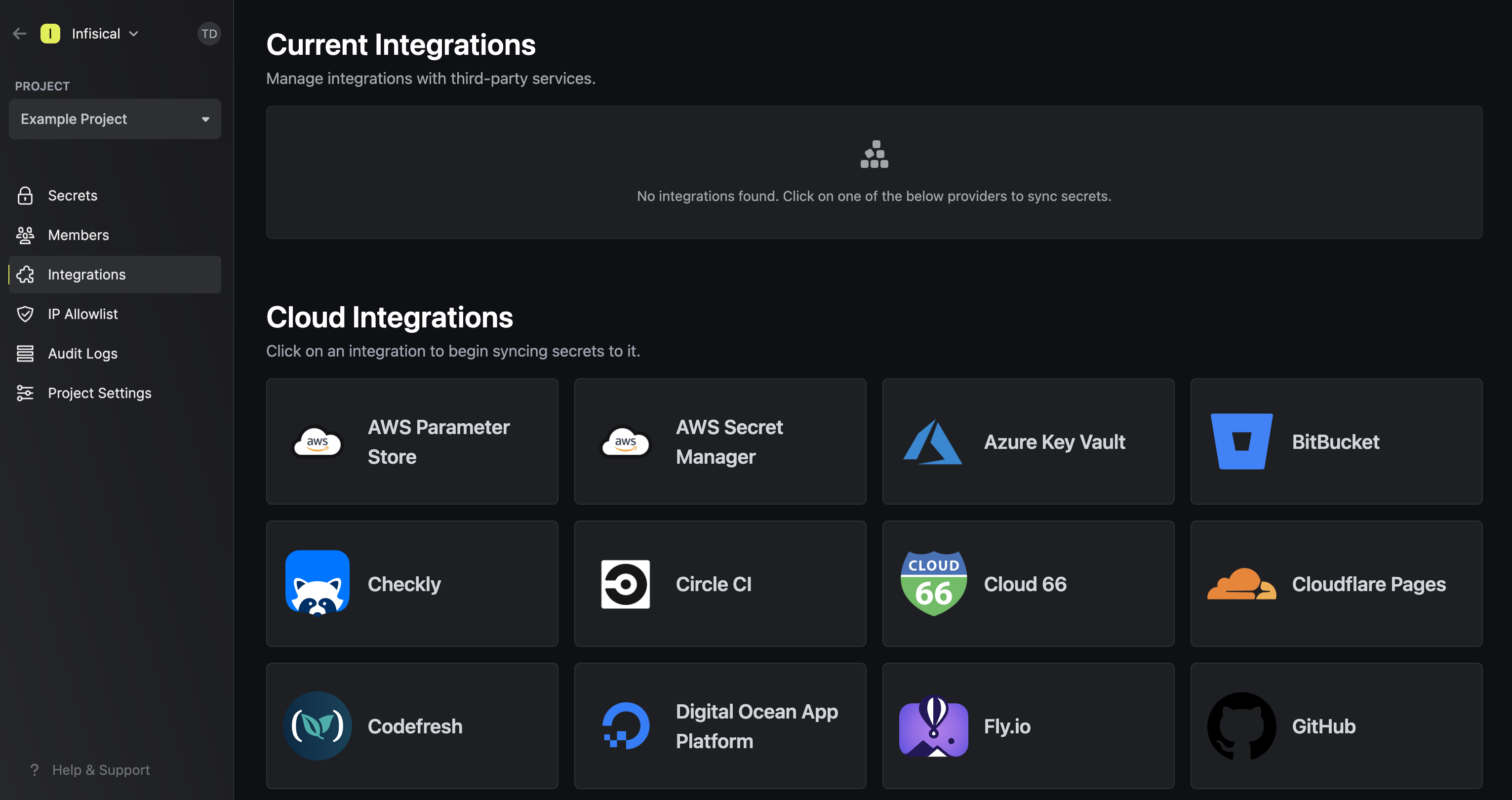
Press on the Hasura Cloud tile and input your Hasura Cloud access token to grant Infisical access to your Hasura Cloud account.
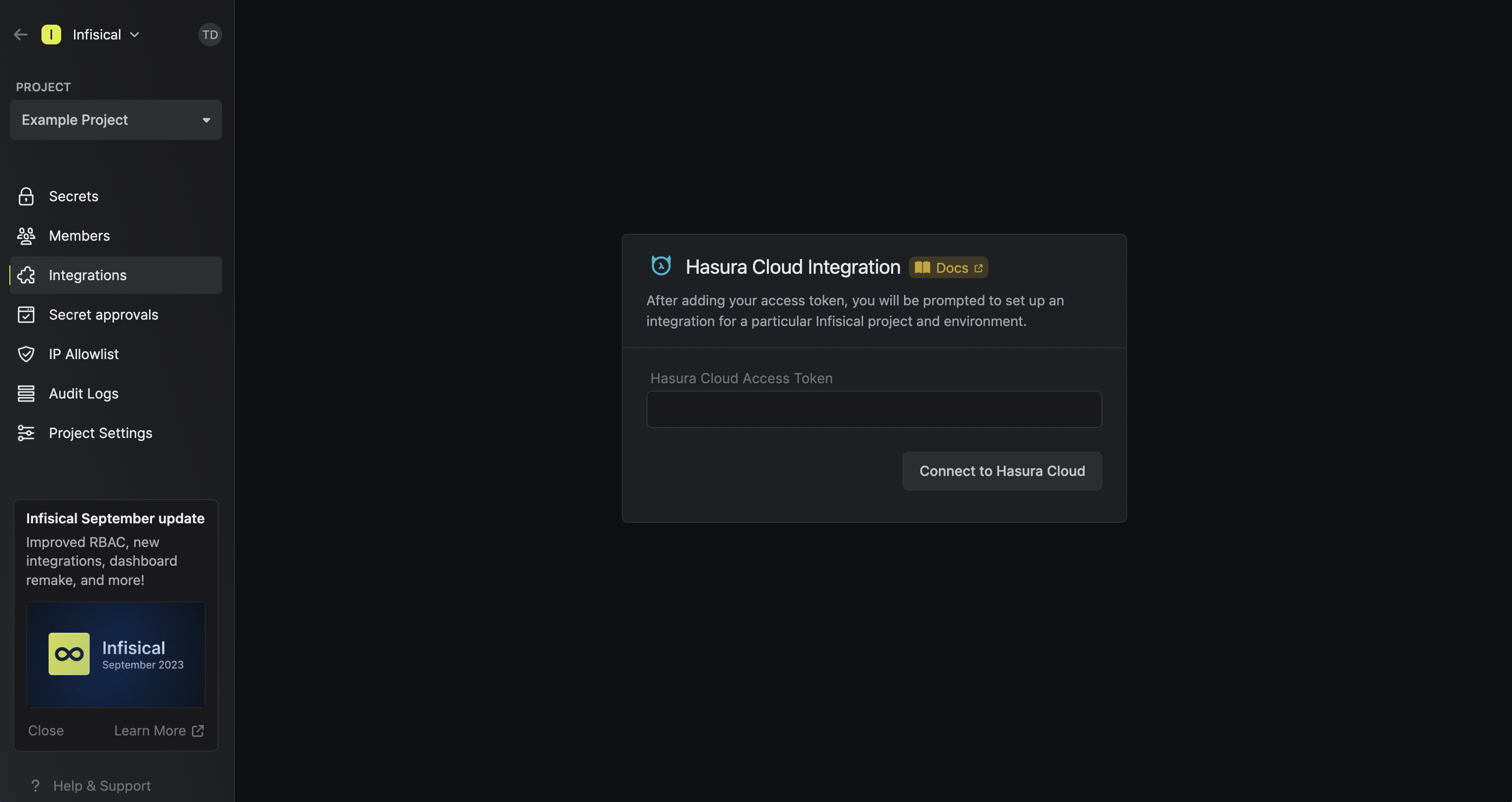
Select which Infisical environment secrets you want to sync to which Hasura Cloud project and press create integration to start syncing secrets to Hasura Cloud.
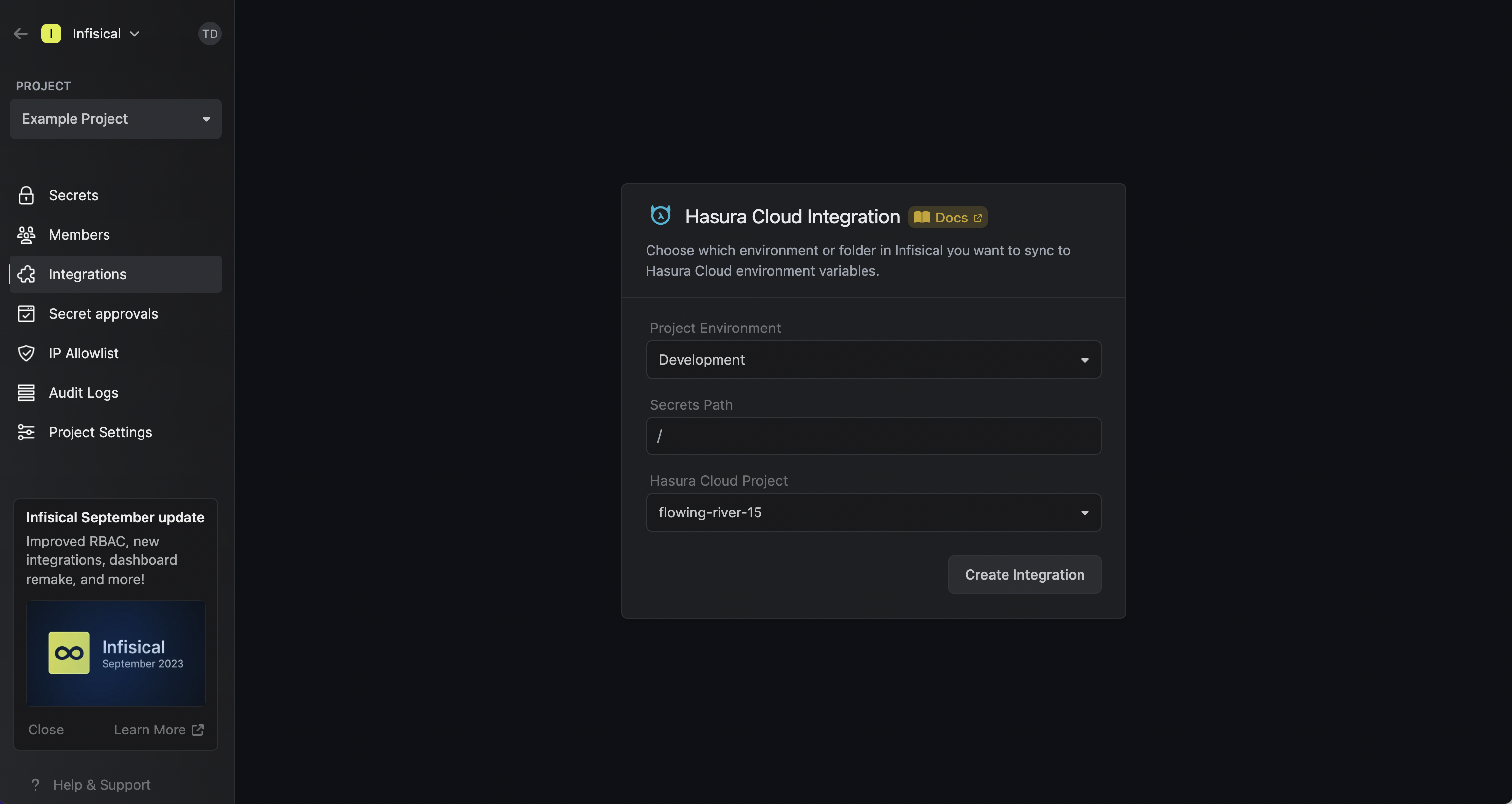
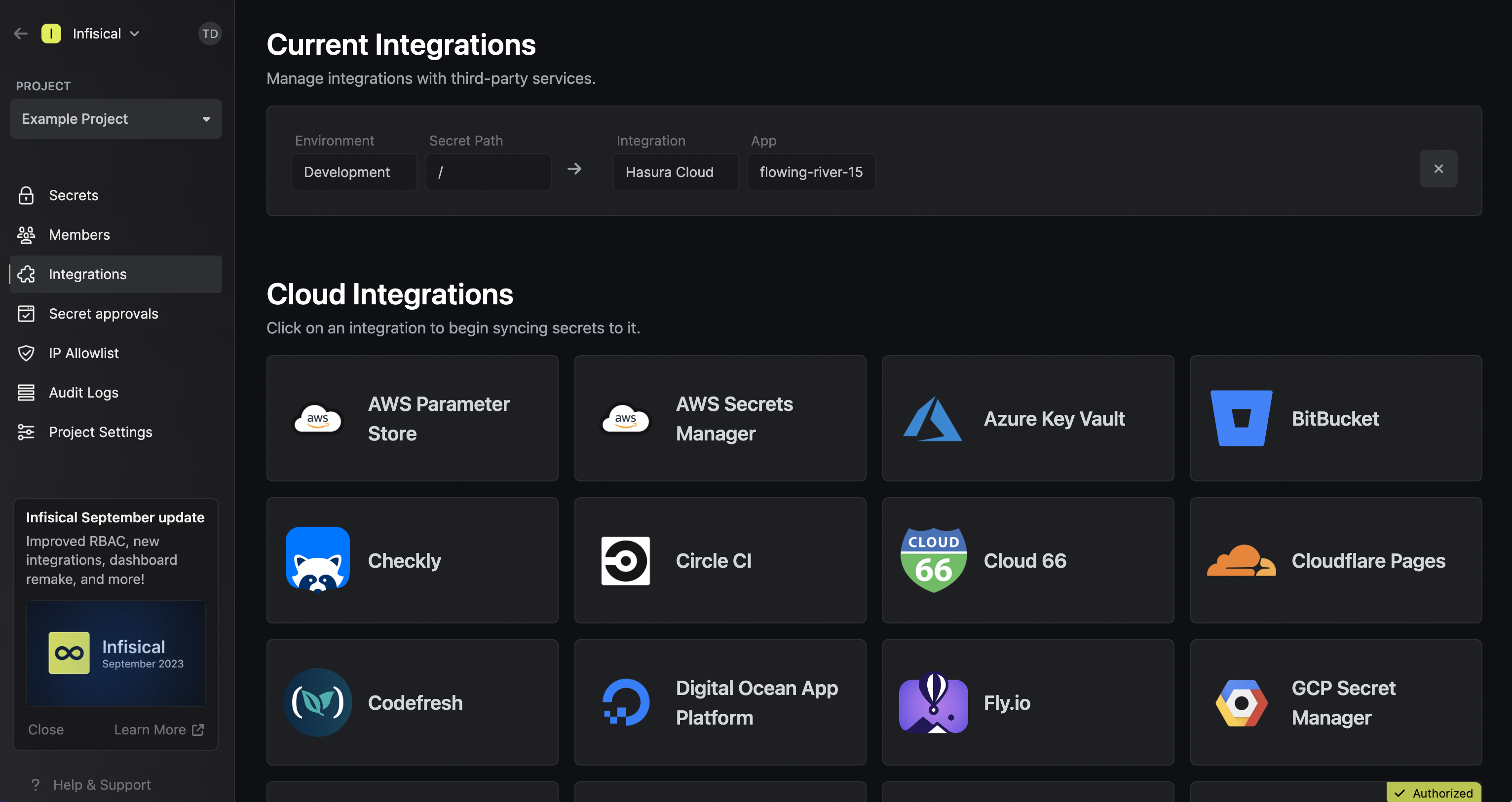
# Heroku
How to sync secrets from Infisical to Heroku
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
Navigate to your project's integrations tab in Infisical.
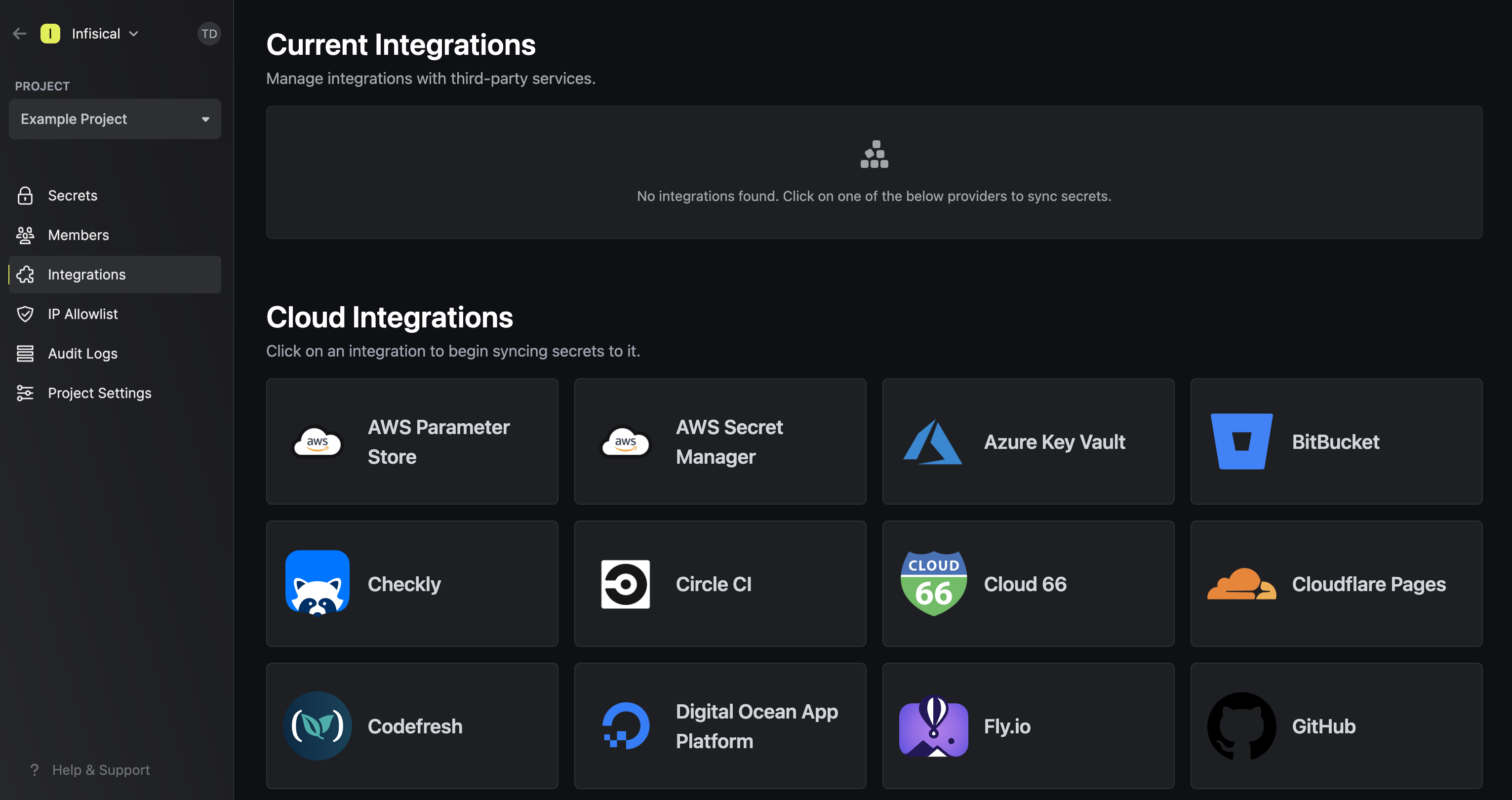
Press on the Heroku tile and grant Infisical access to your Heroku account.
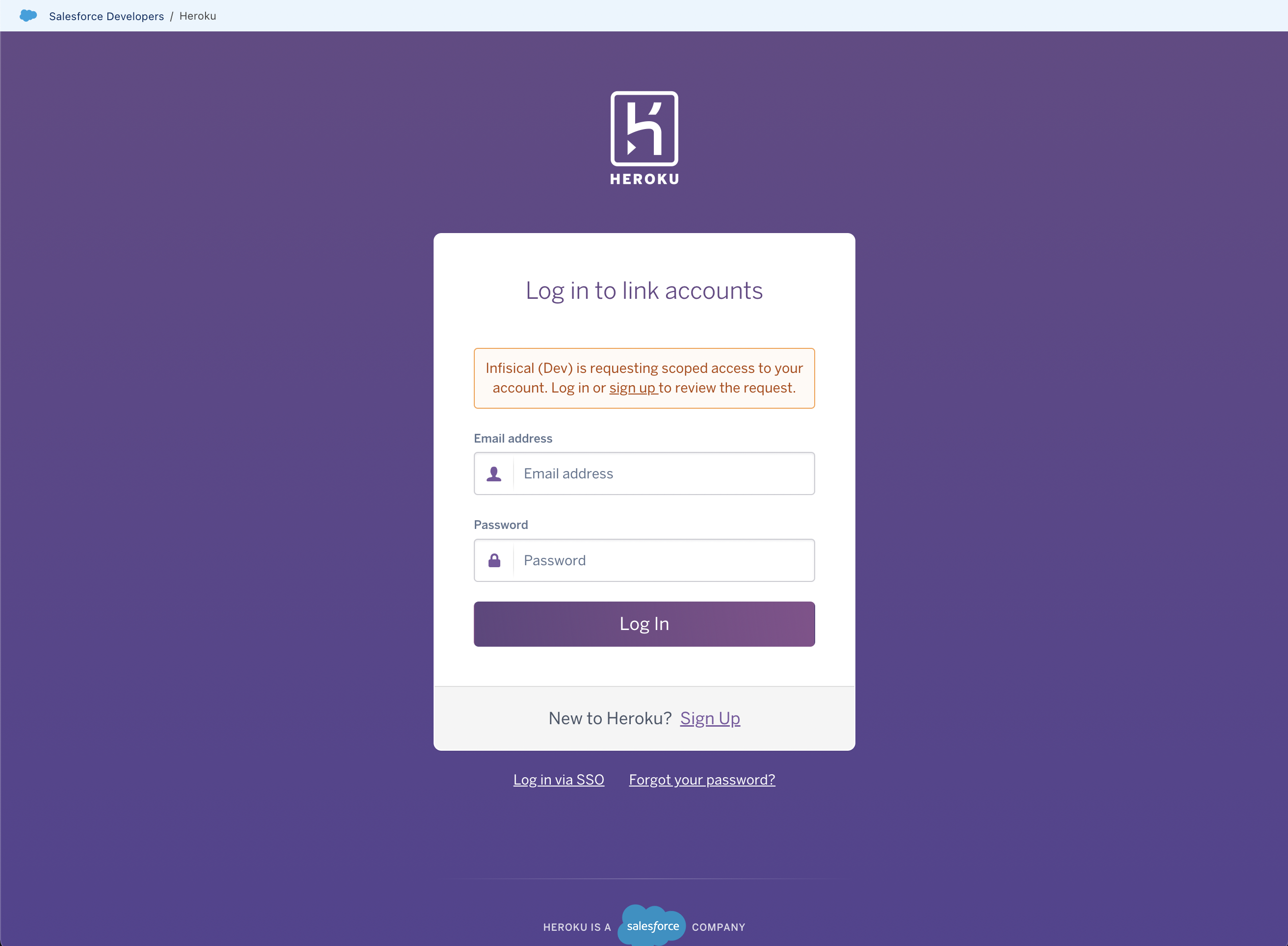
Select which Infisical environment secrets you want to sync to which Heroku app and press create integration to start syncing secrets to Heroku.
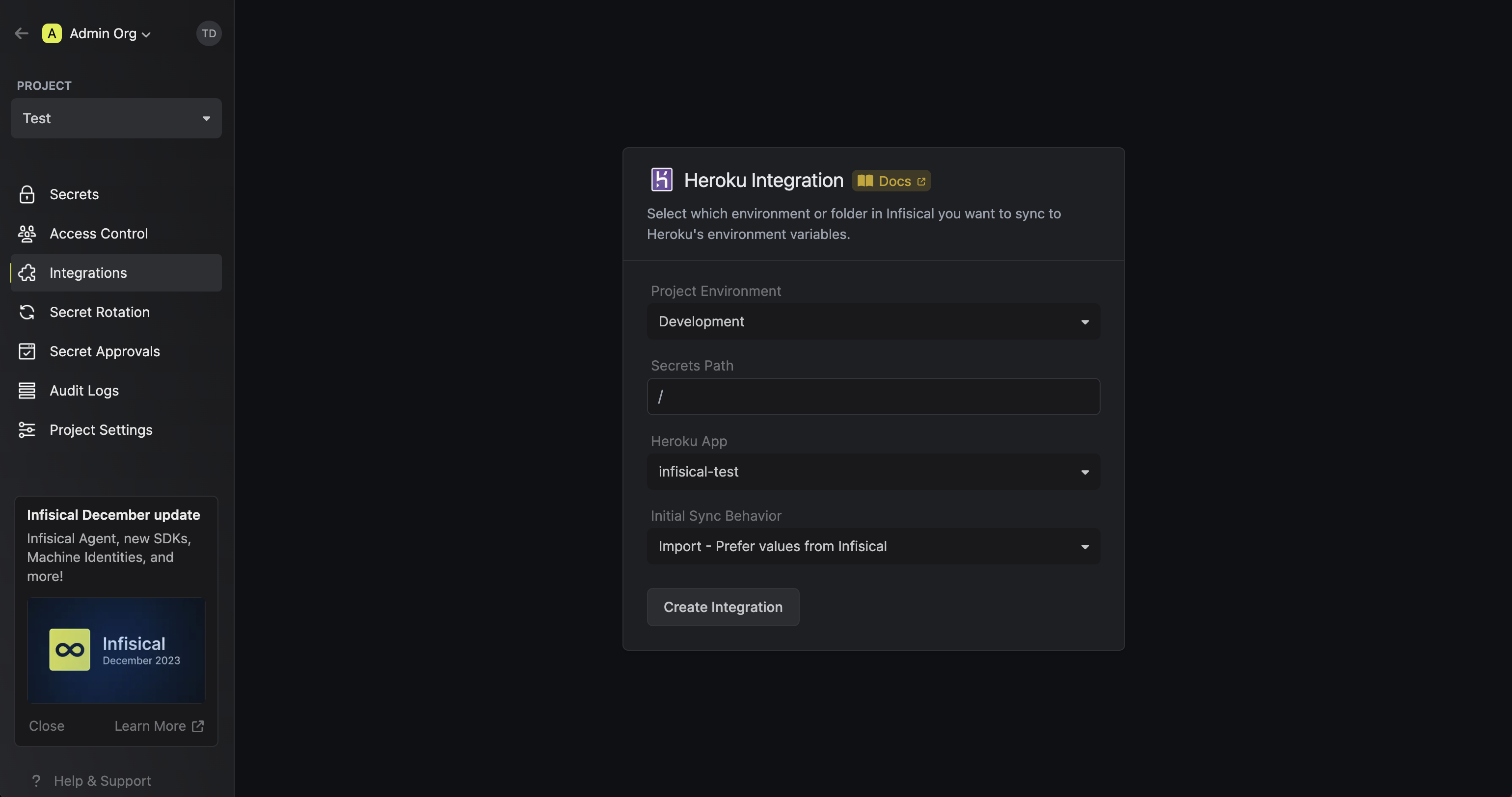
Here's some guidance on each field:
* Project Environment: The environment in the current Infisical project from which you want to sync secrets from.
* Secrets Path: The path in the current Infisical project from which you want to sync secrets from such as `/` (for secrets that do not reside in a folder) or `/foo/bar` (for secrets nested in a folder, in this case a folder called `bar` in another folder called `foo`).
* Heroku App: The application in Heroku that you want to sync secrets to.
* Initial Sync Behavior (default is **Import - Prefer values from Infisical**): The behavior of the first sync operation triggered after creating the integration.
* **No Import - Overwrite all values in Heroku**: Sync secrets and overwrite any existing secrets in Heroku.
* **Import - Prefer values from Infisical**: Import secrets from Heroku to Infisical; if a secret with the same name already exists in Infisical, do nothing. Afterwards, sync secrets to Heroku.
* **Import - Prefer values from Heroku**: Import secrets from Heroku to Infisical; if a secret with the same name already exists in Infisical, replace its value with the one from Heroku. Afterwards, sync secrets to Heroku.
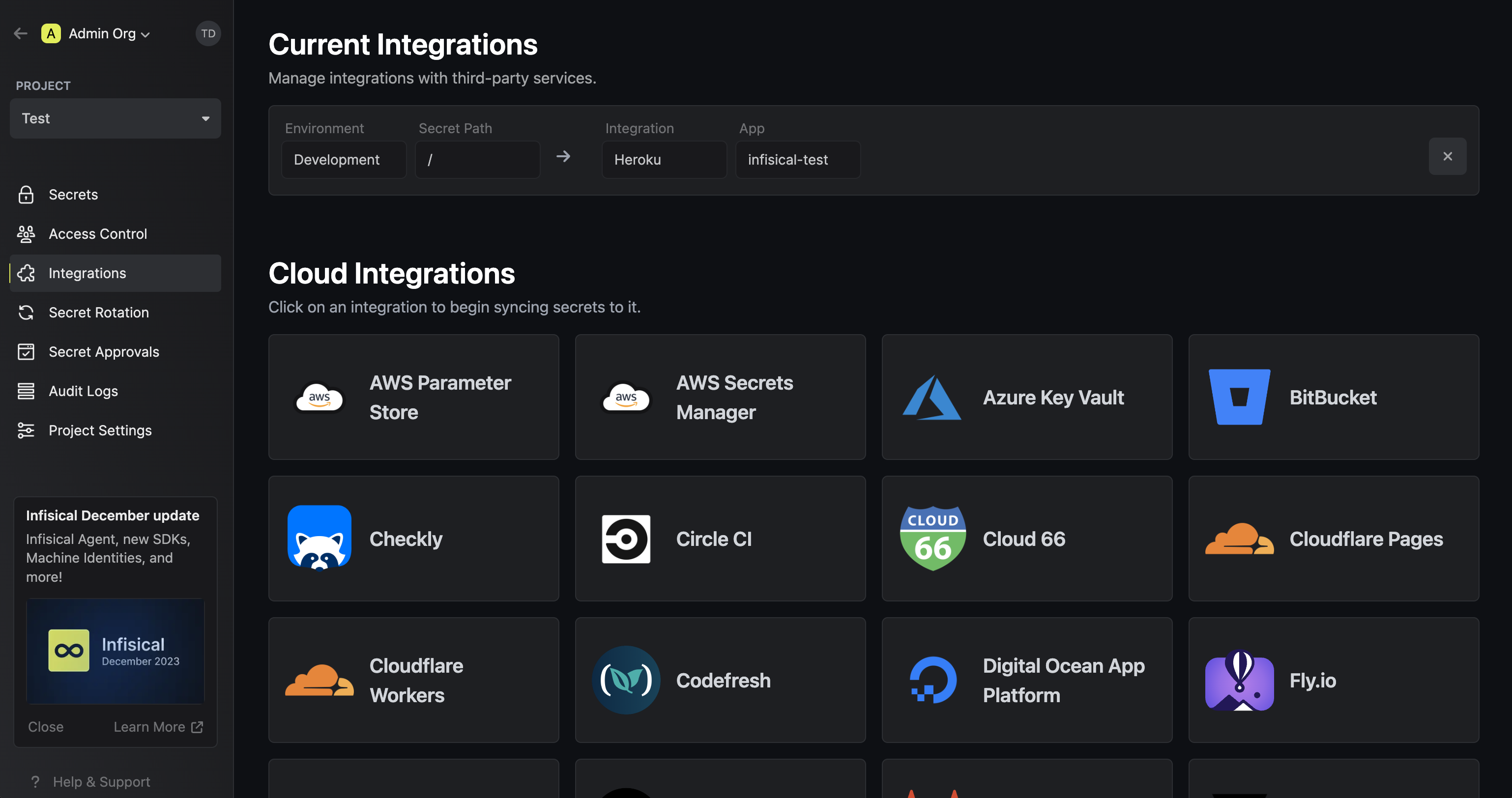
Using the Heroku integration on a self-hosted instance of Infisical requires configuring an API client in Heroku
and registering your instance with it.
Navigate to your user Account settings > Applications to create a new API client.
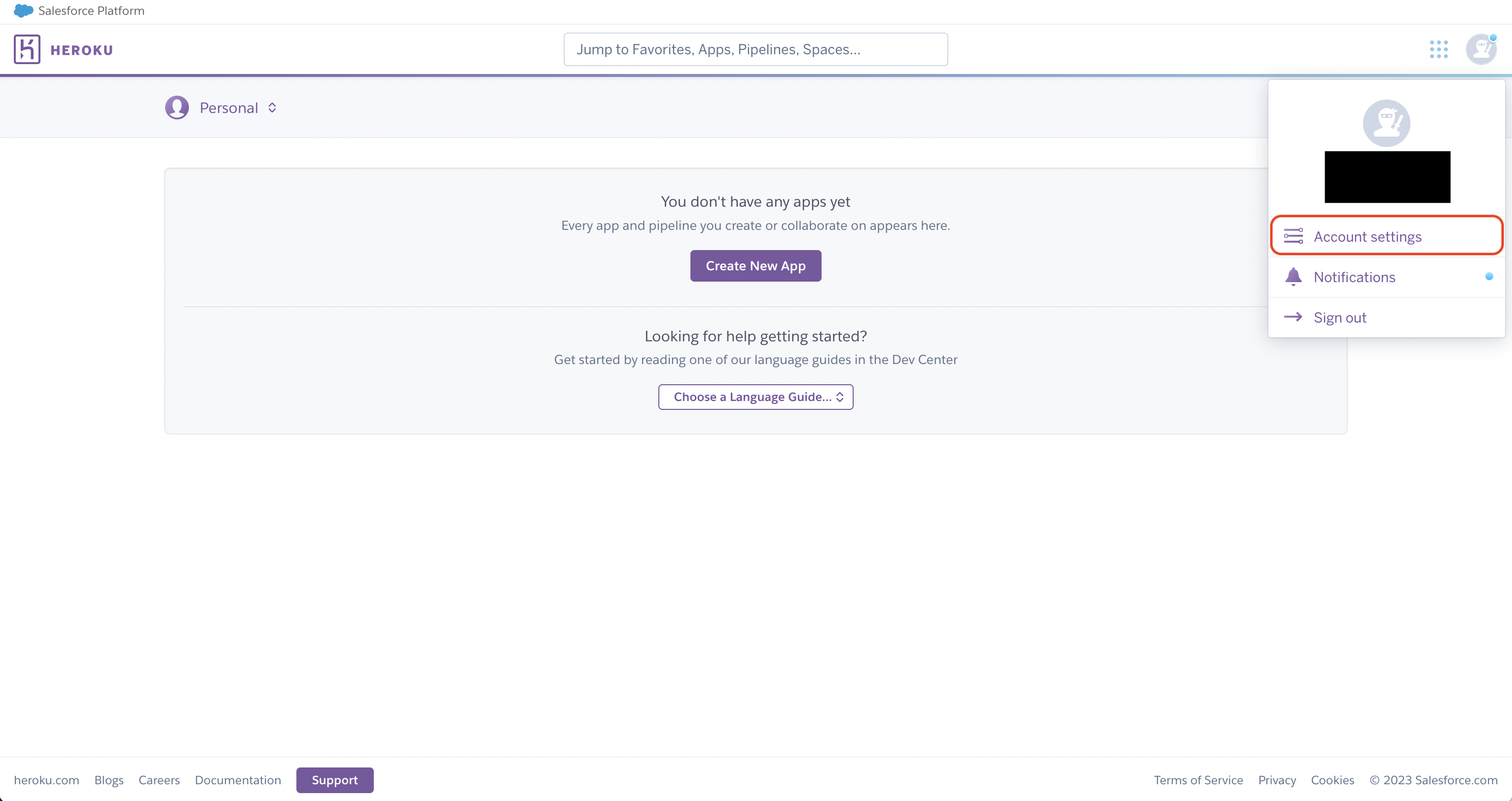
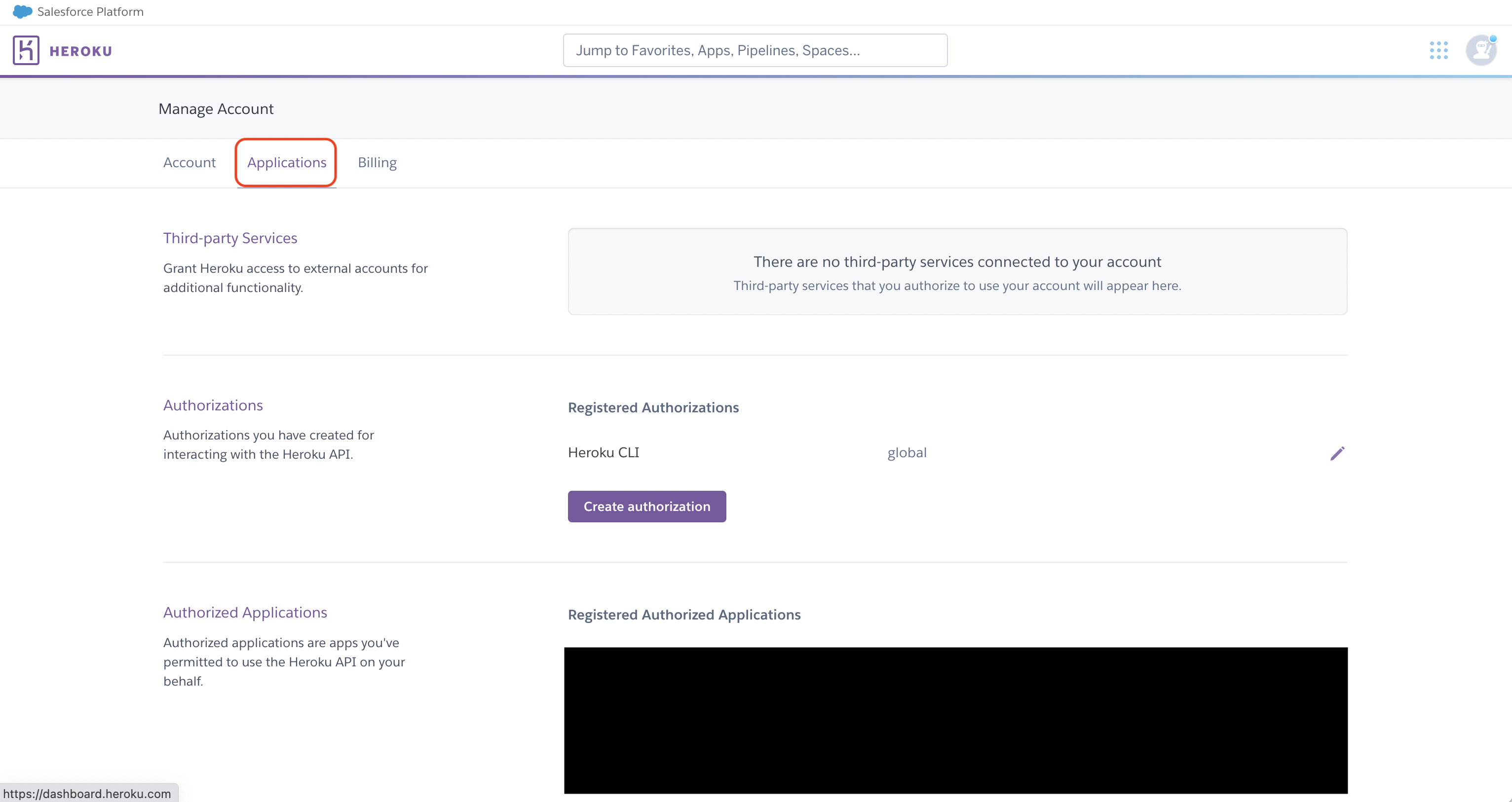
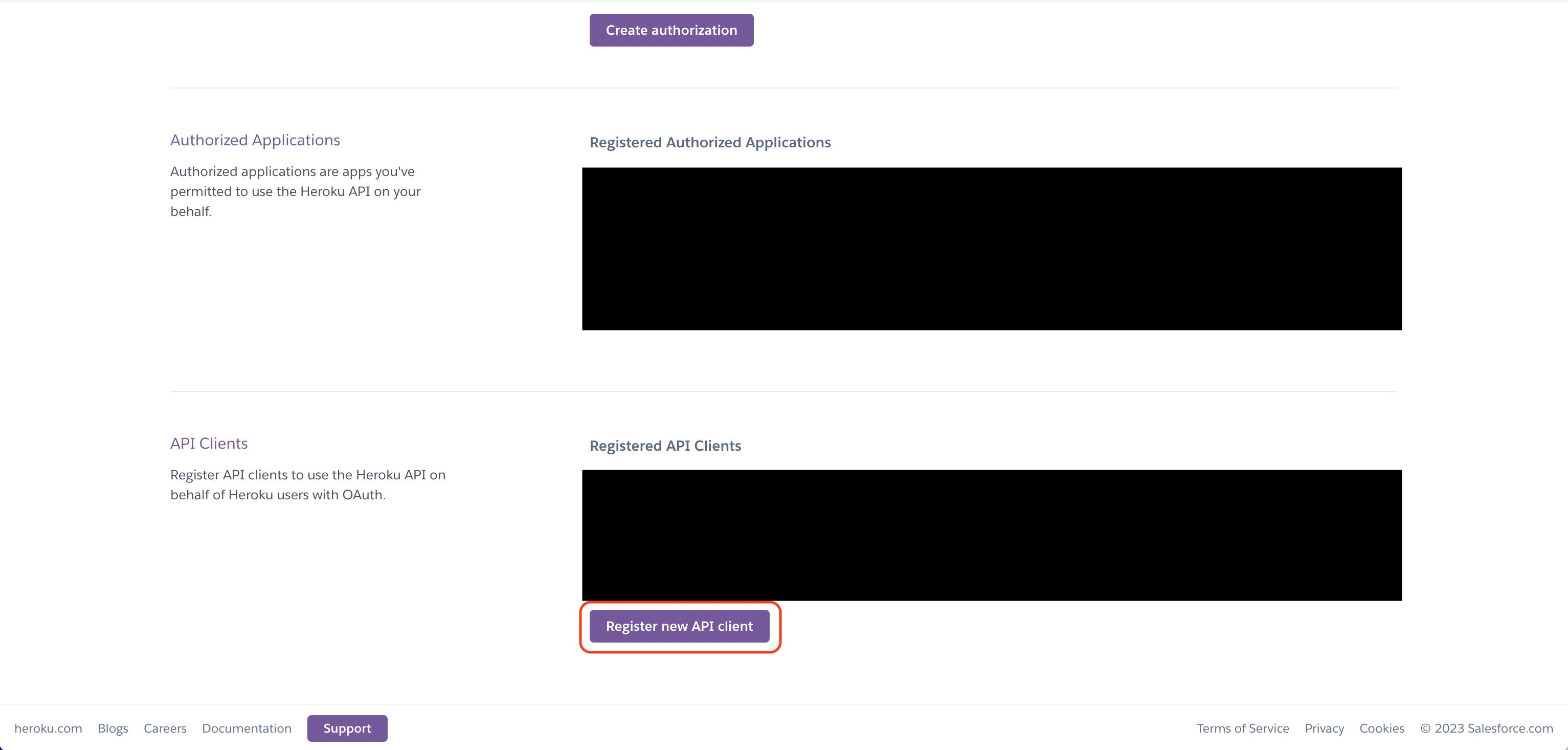
Create the API client. As part of the form, set the **OAuth callback URL** to `https://your-domain.com/integrations/heroku/oauth2/callback`.
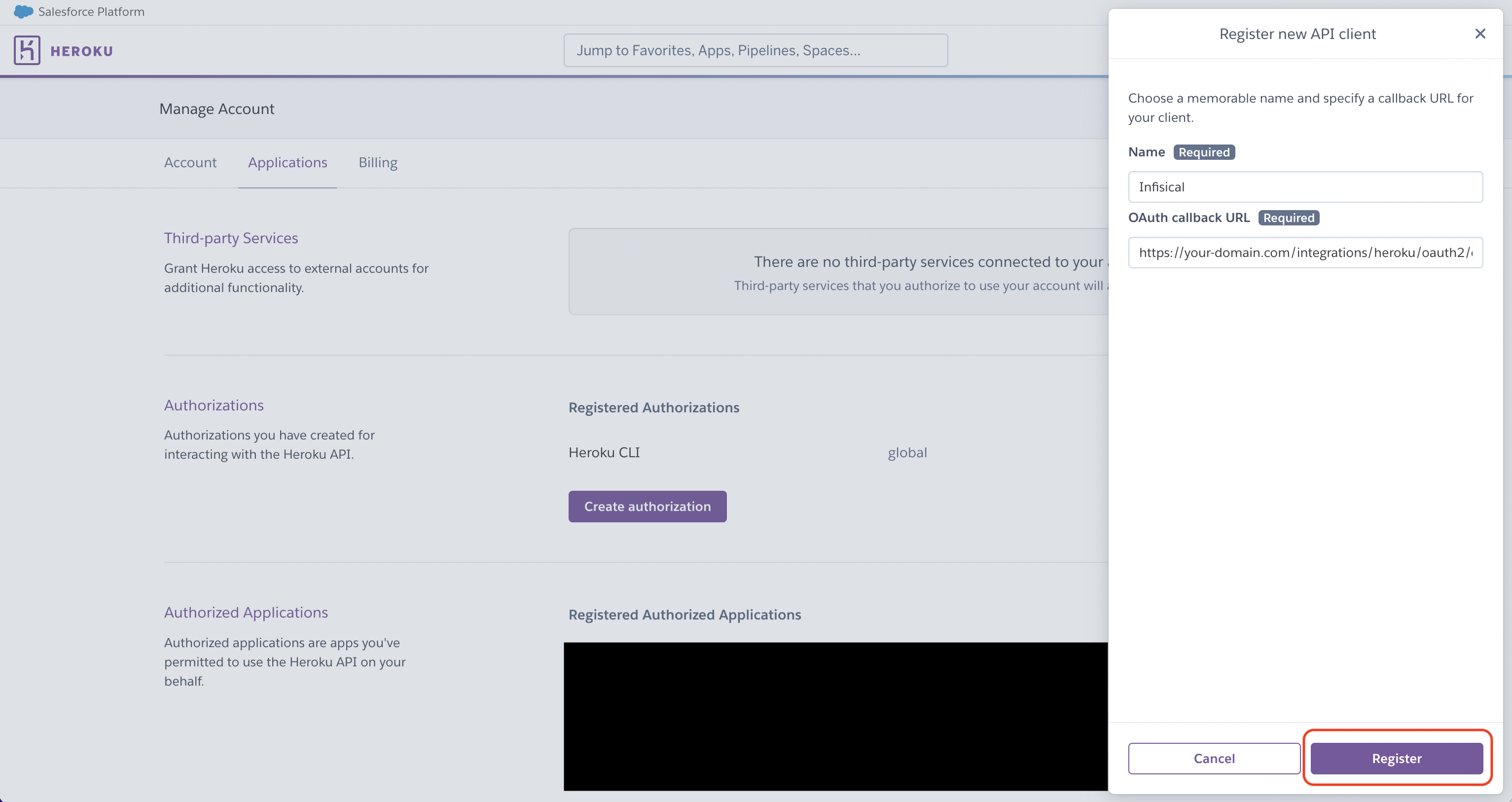
Obtain the **Client ID** and **Client Secret** for your Heroku API client.
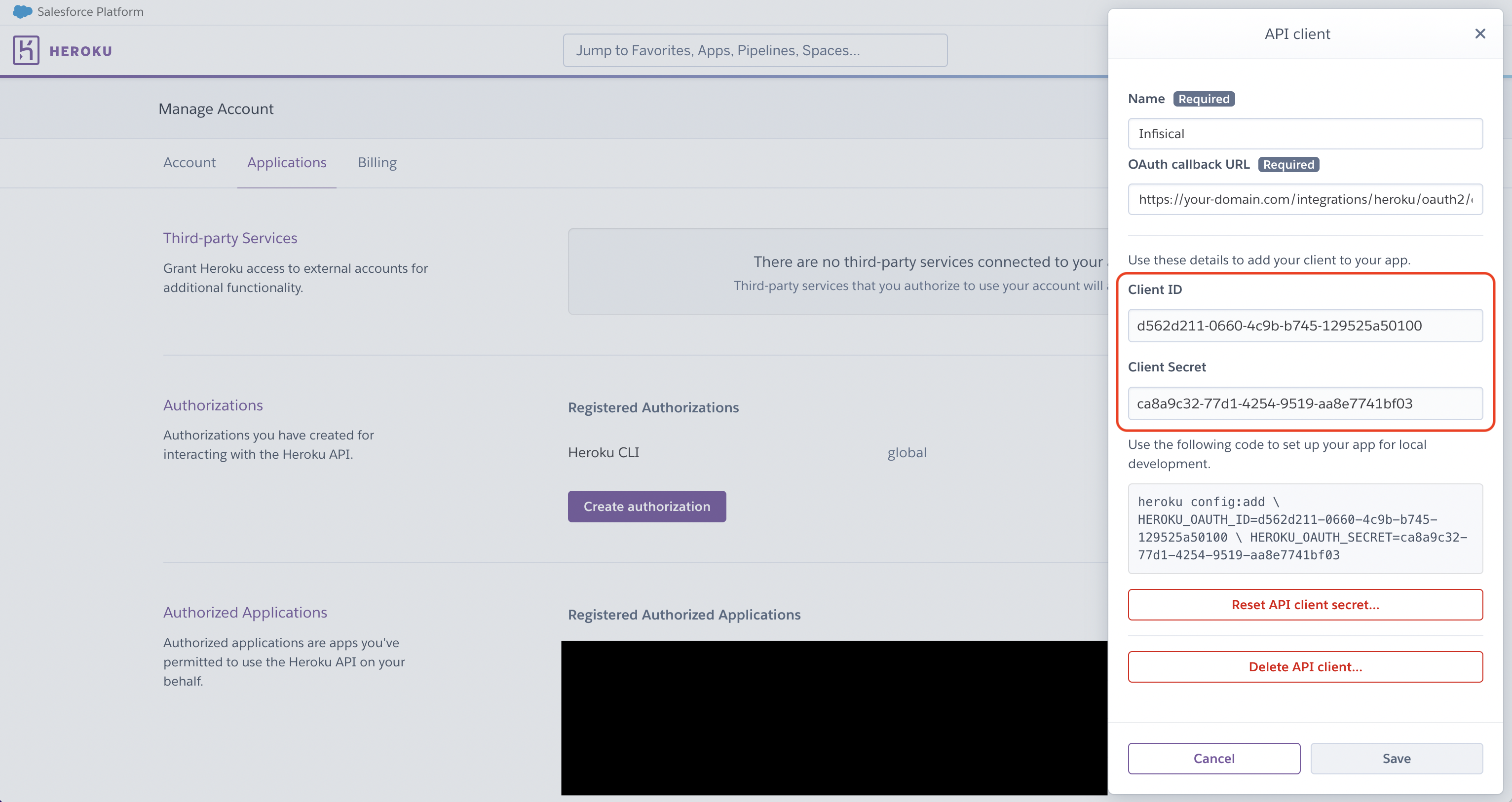
Back in your Infisical instance, add two new environment variables for the credentials of your Heroku API client.
* `CLIENT_ID_HEROKU`: The **Client ID** of your Heroku API client.
* `CLIENT_SECRET_HEROKU`: The **Client Secret** of your Heroku API client.
Once added, restart your Infisical instance and use the Heroku integration.
# Laravel Forge
How to sync secrets from Infisical to Laravel Forge
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
Obtain a Laravel Forge access token in API Tokens
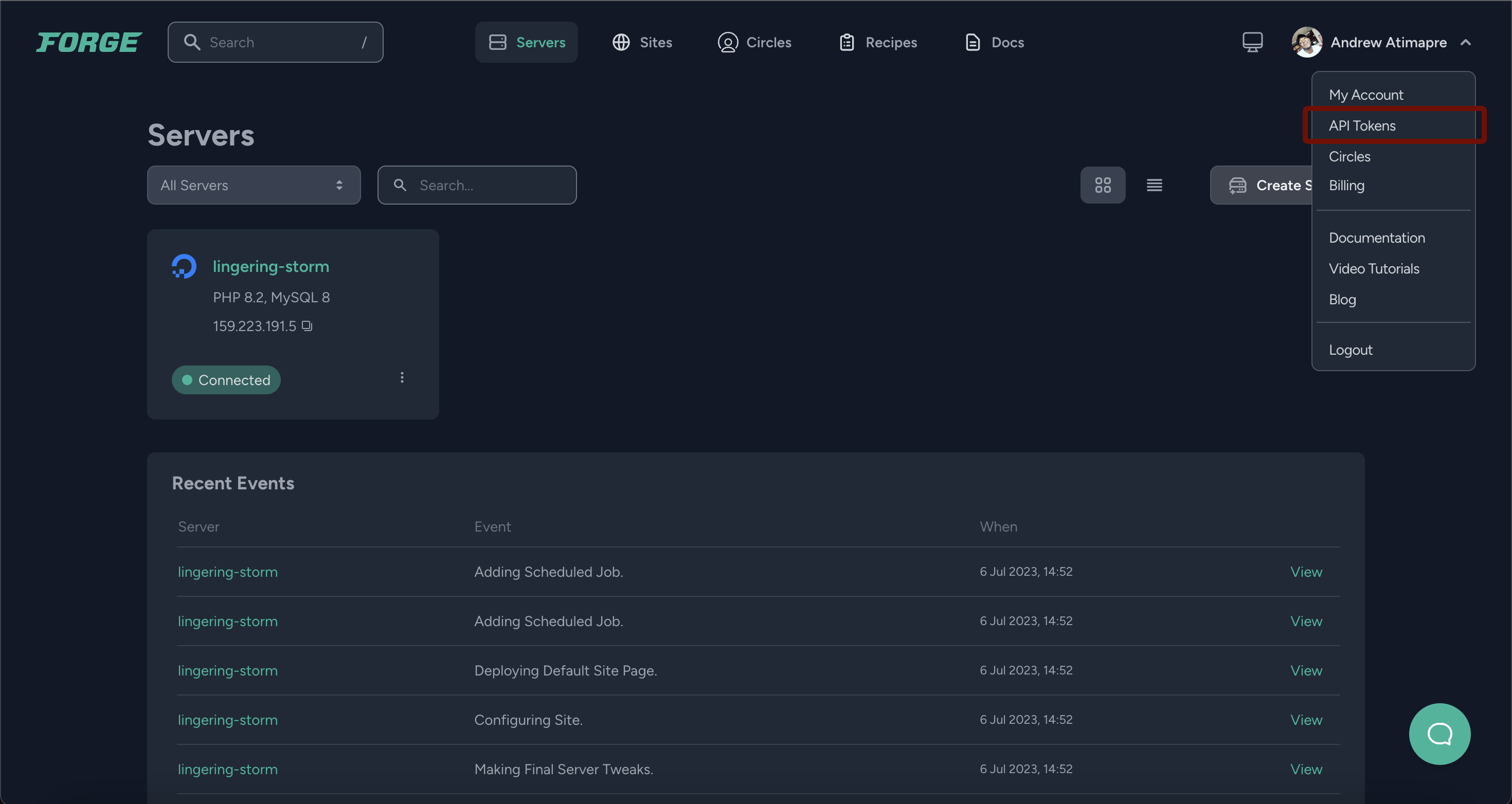
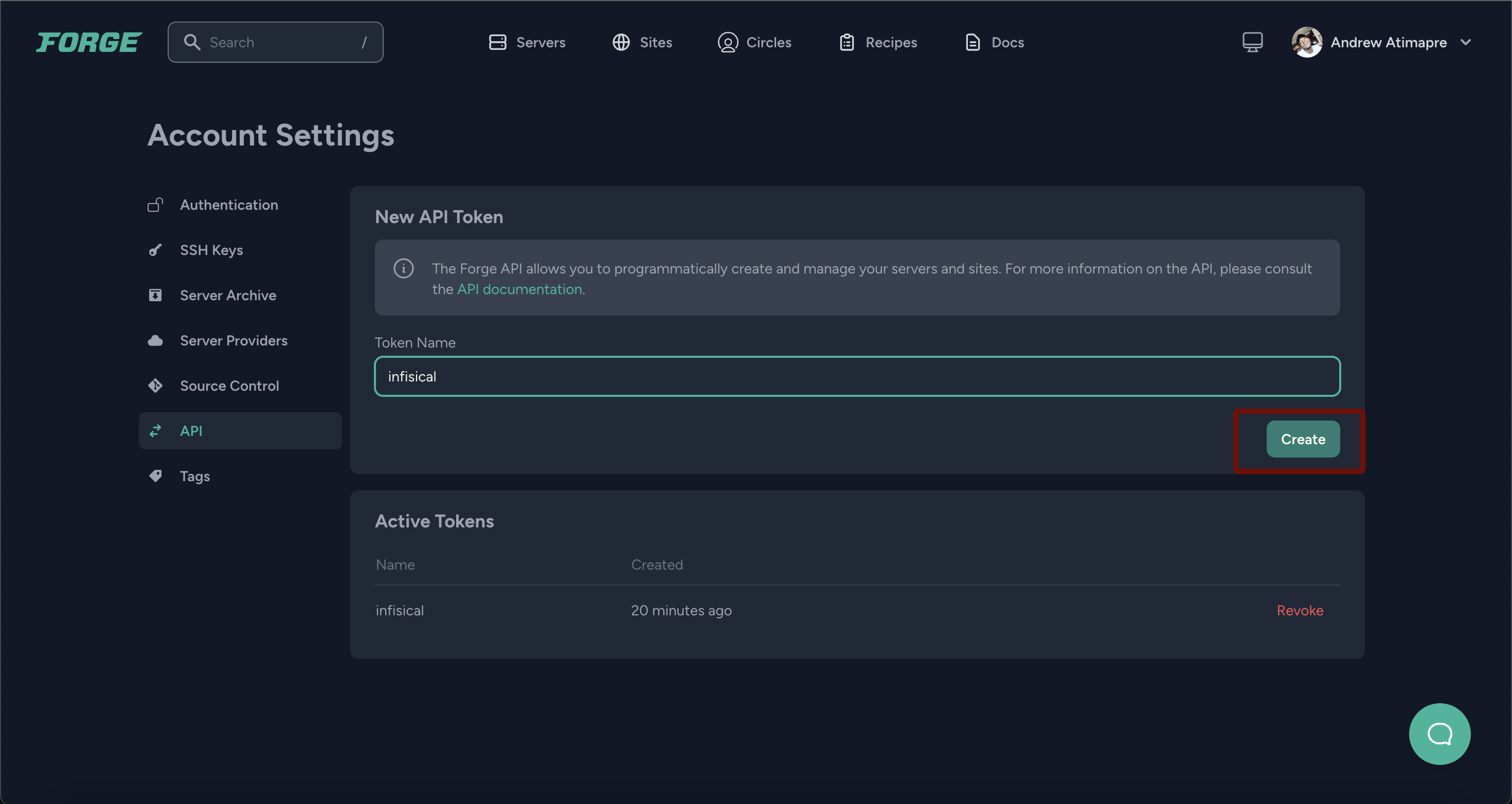
Obtain your Laravel Forge Server ID in Servers > Server ID
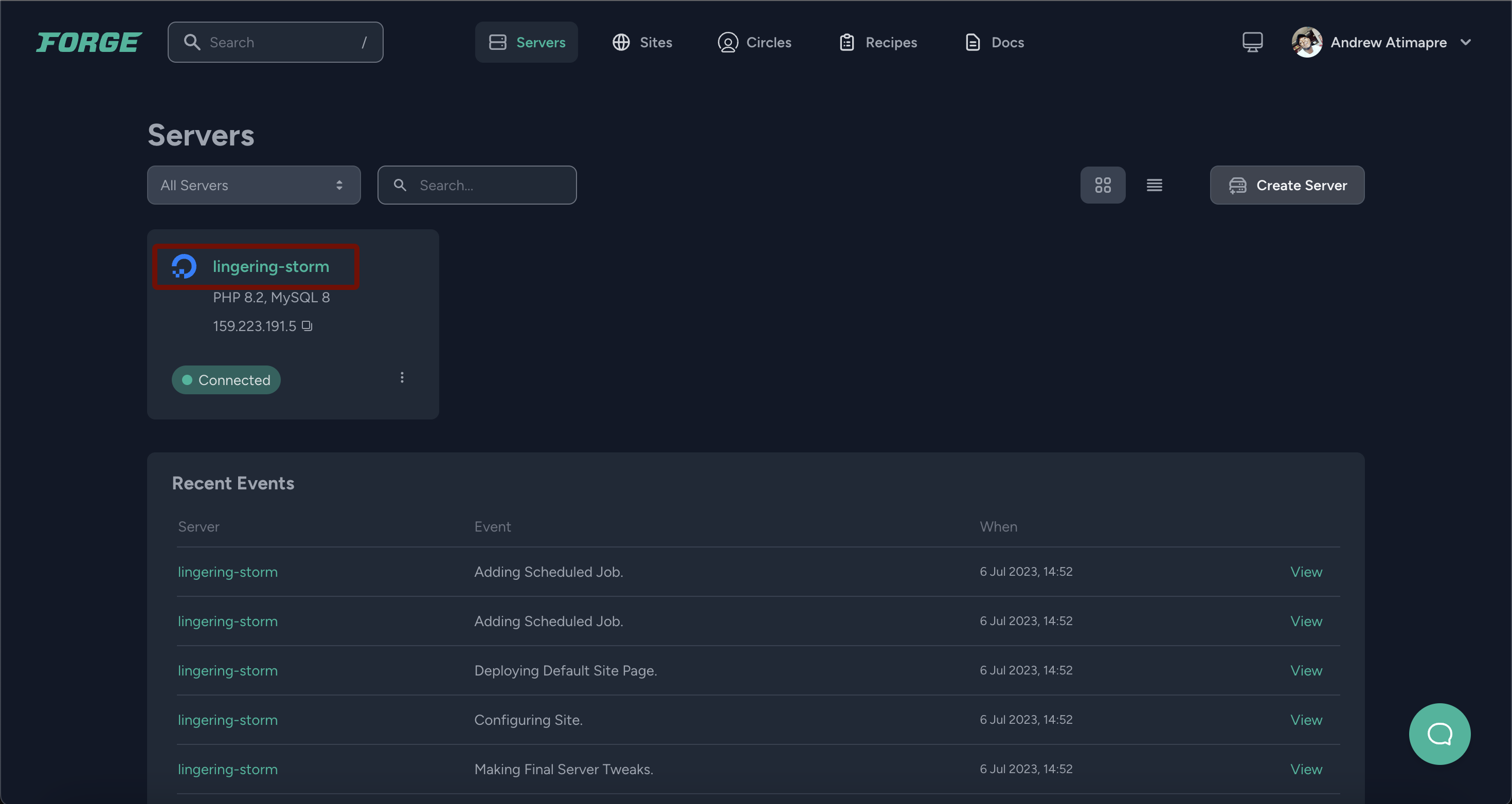
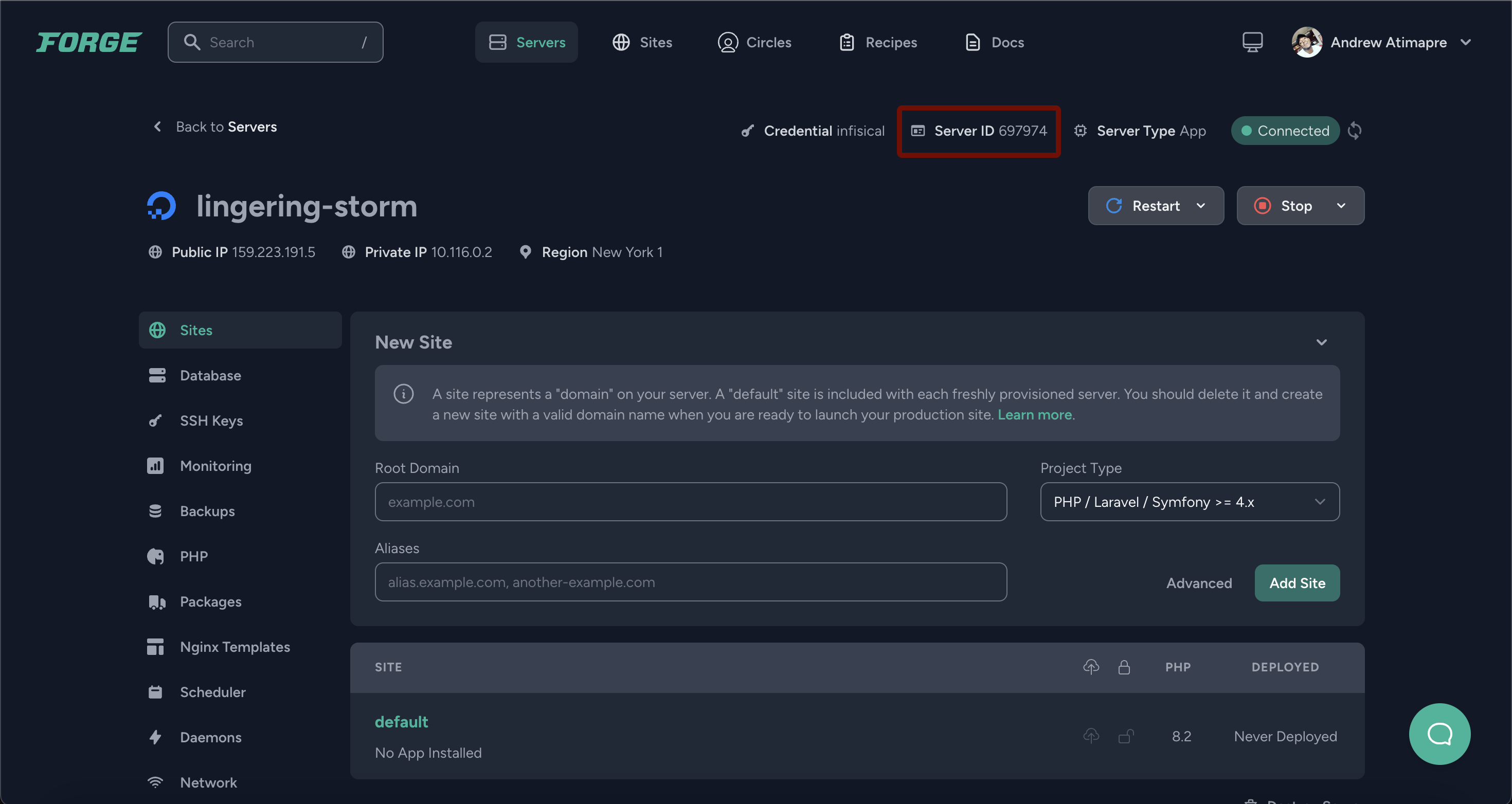
Navigate to your project's integrations tab in Infisical.
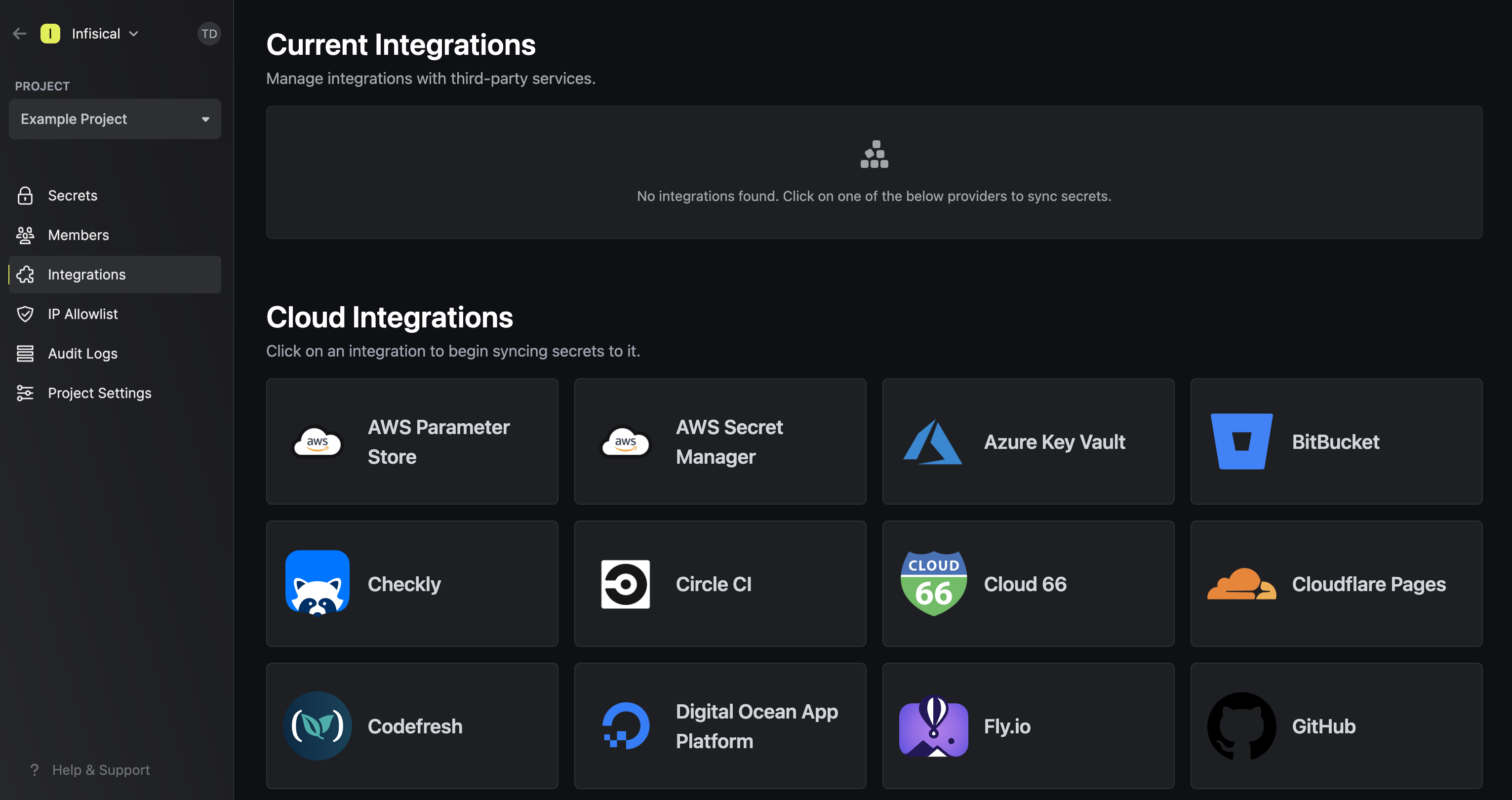
Press on the Laravel Forge tile and input your Laravel Forge access token and server ID to grant Infisical access to your Laravel Forge account.
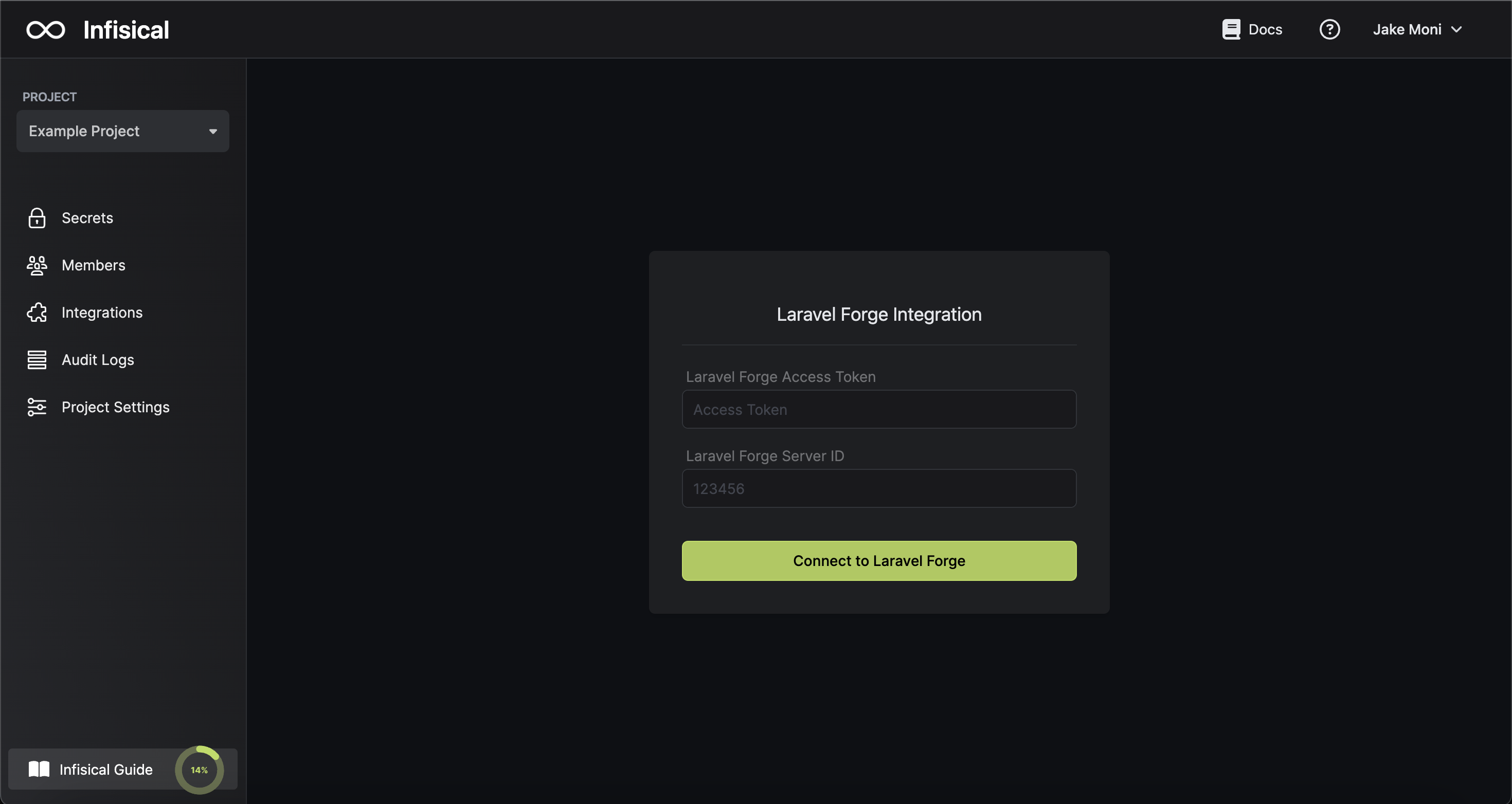
Select which Infisical environment secrets you want to sync to which Laravel Forge site and press create integration to start syncing secrets to Laravel Forge.
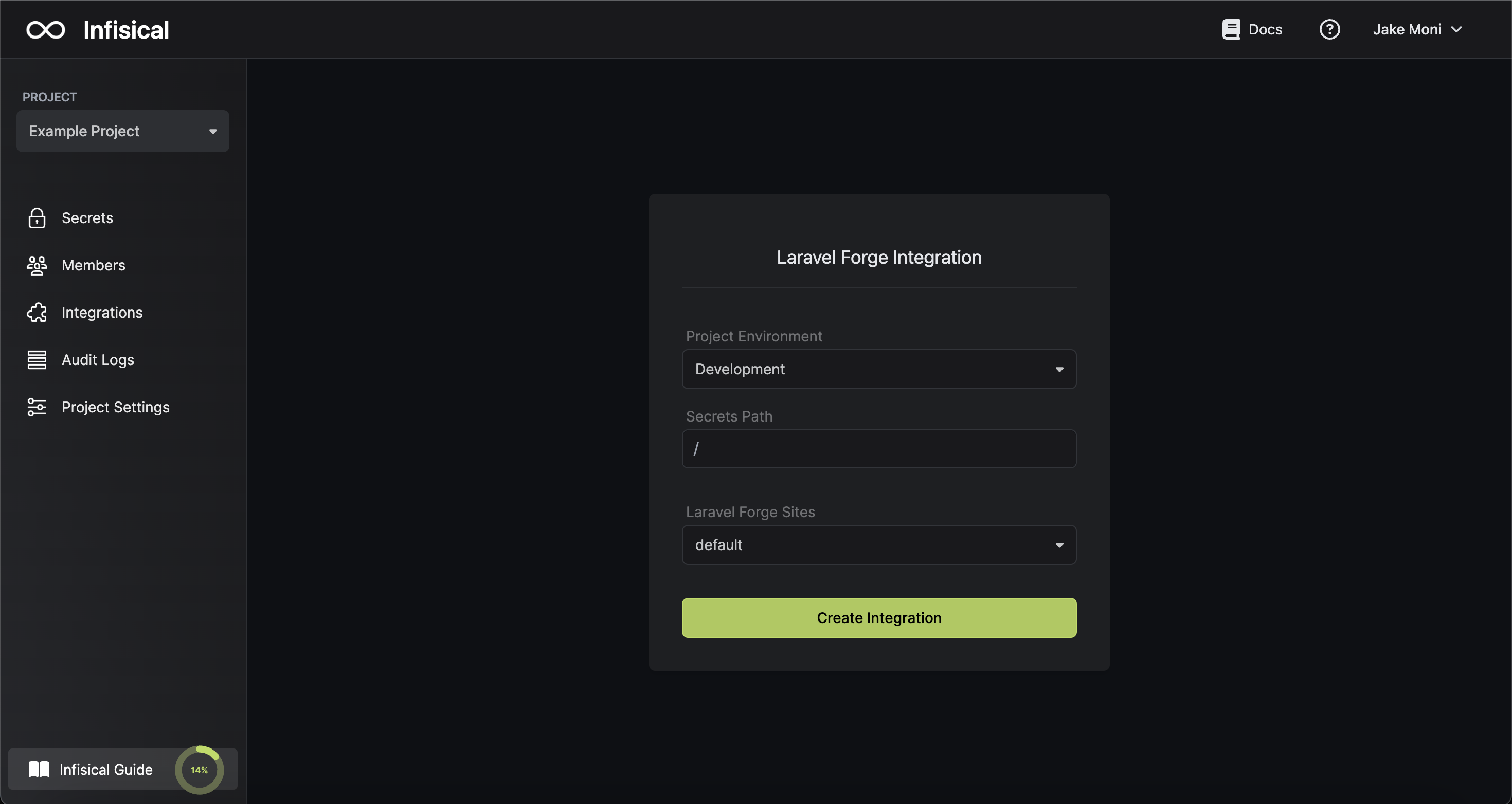
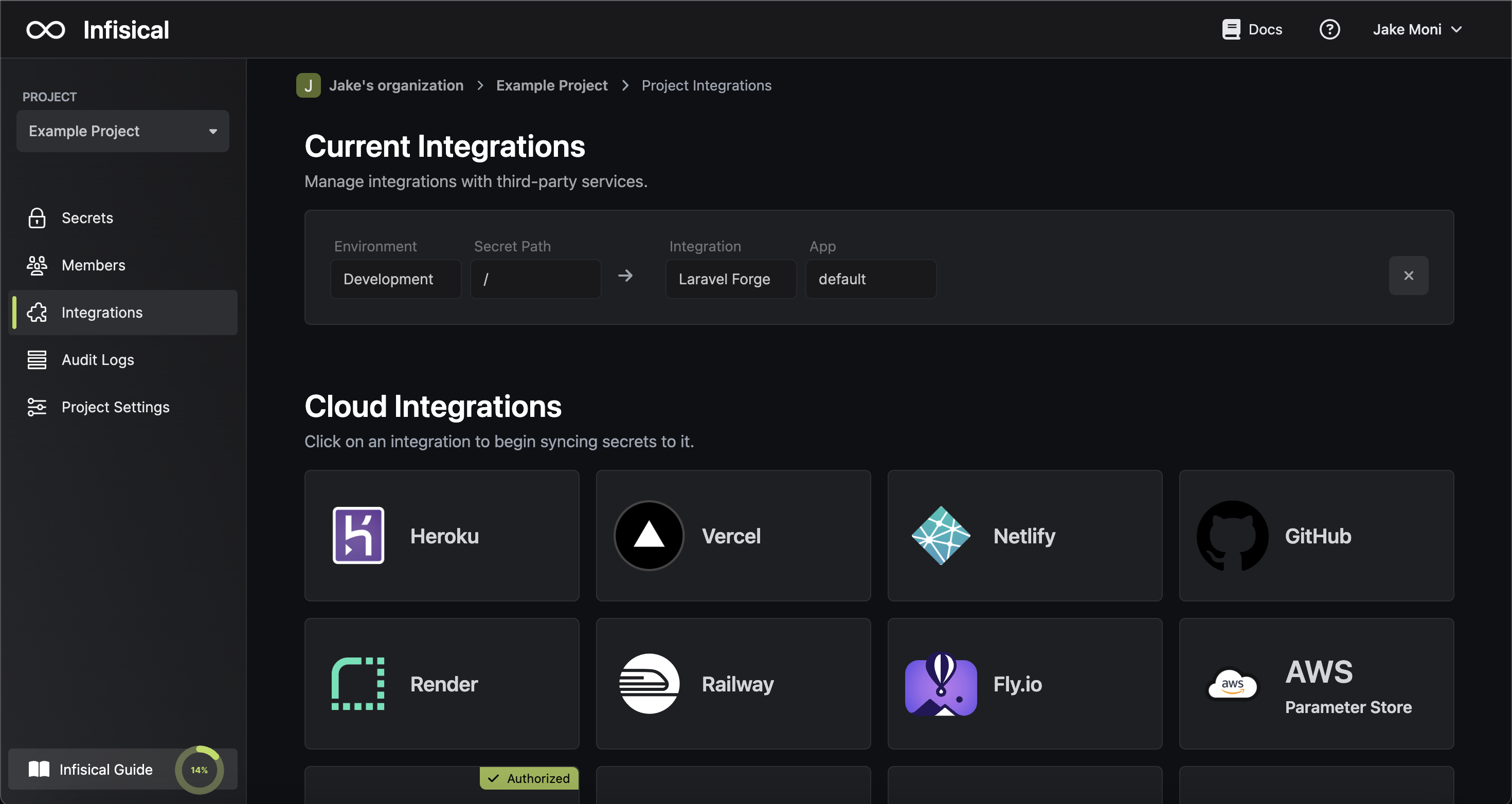
# Netlify
How to sync secrets from Infisical to Netlify
Infisical integrates with Netlify's new environment variable experience. If
your site uses Netlify's old environment variable experience, you'll have to
upgrade it to the new one to use this integration.
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
Navigate to your project's integrations tab in Infisical.
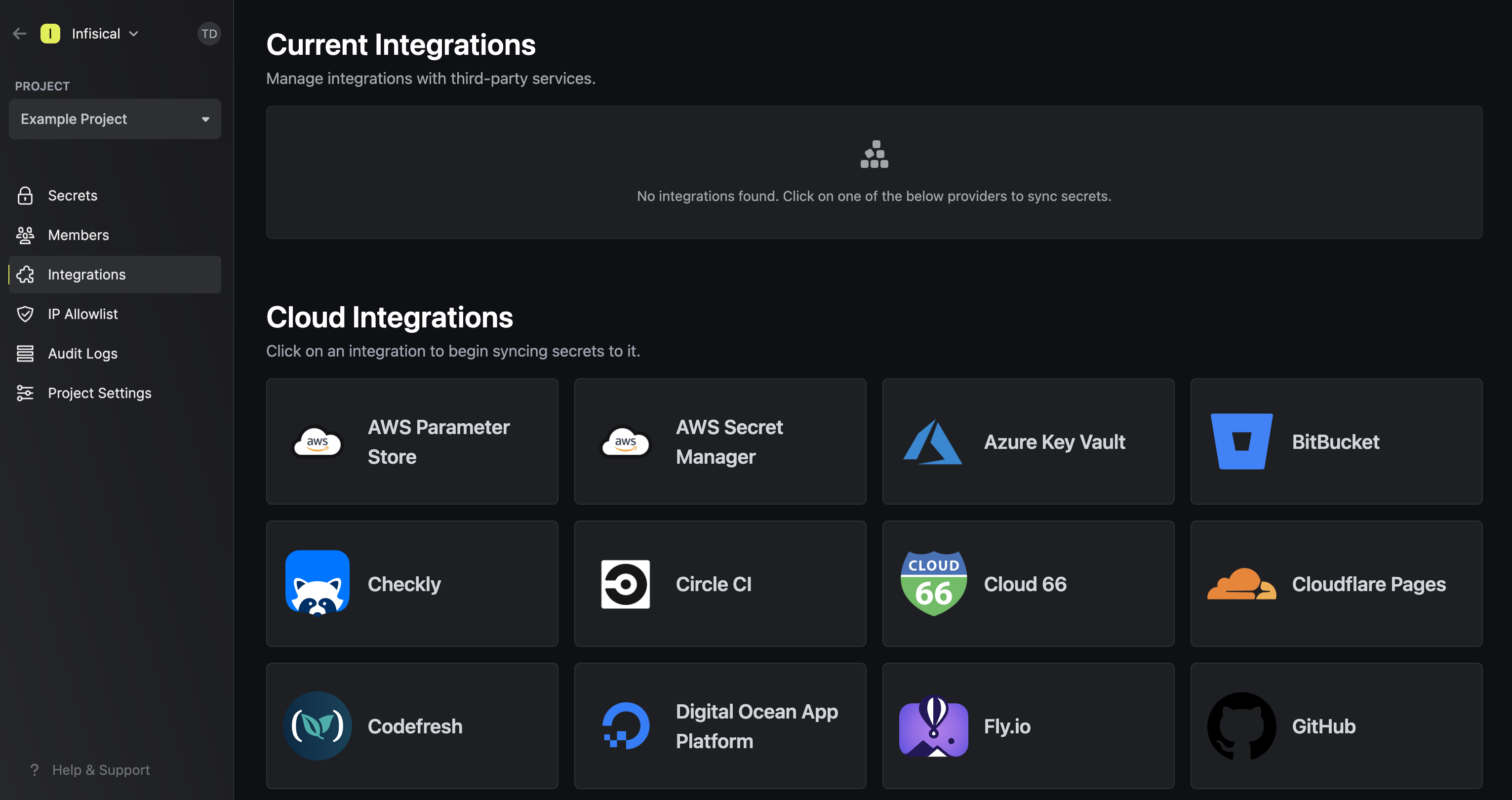
Press on the Netlify tile and grant Infisical access to your Netlify account.
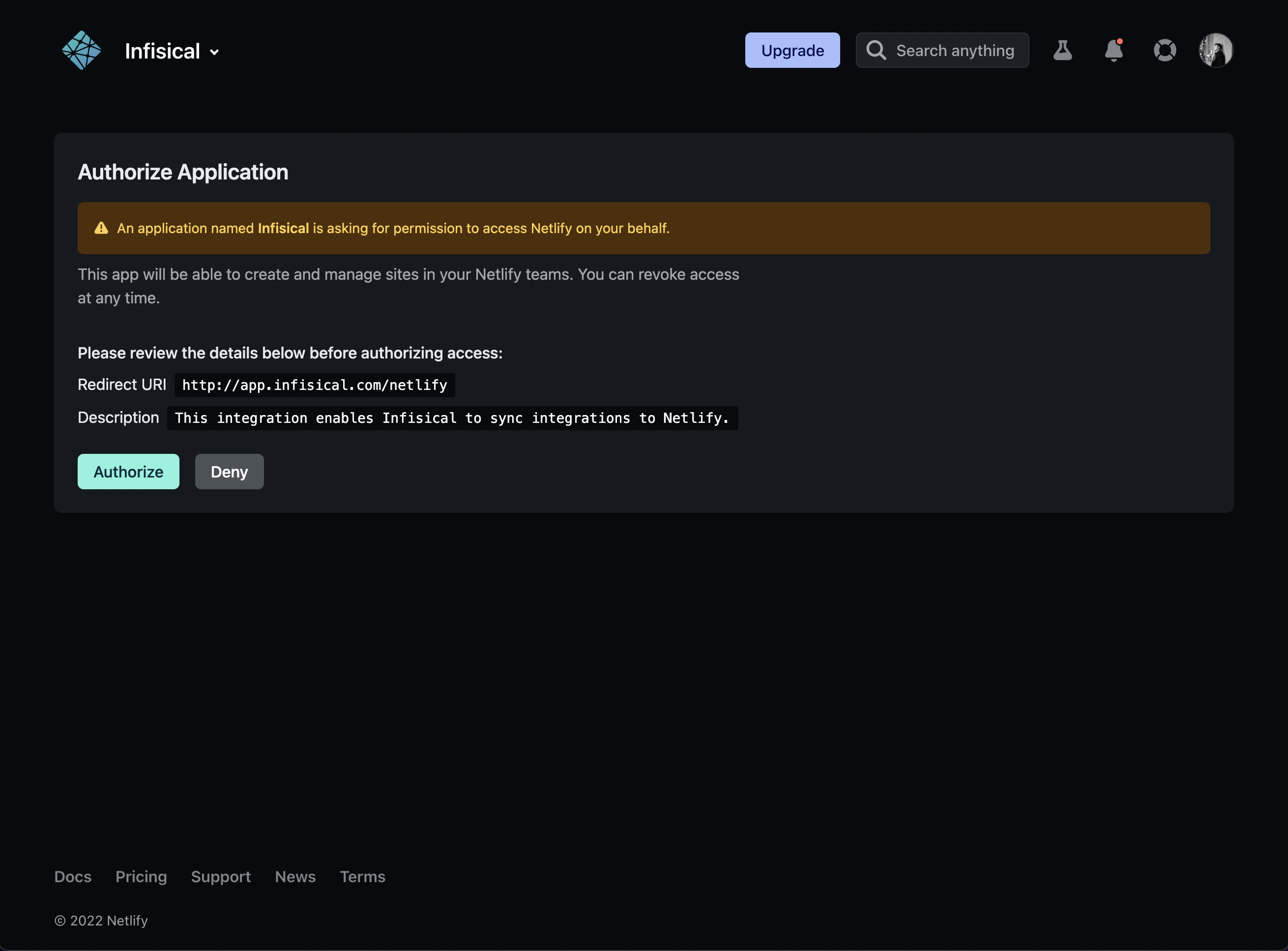
Select which Infisical environment secrets you want to sync to which Netlify app and context. Lastly, press create integration to start syncing secrets to Netlify.
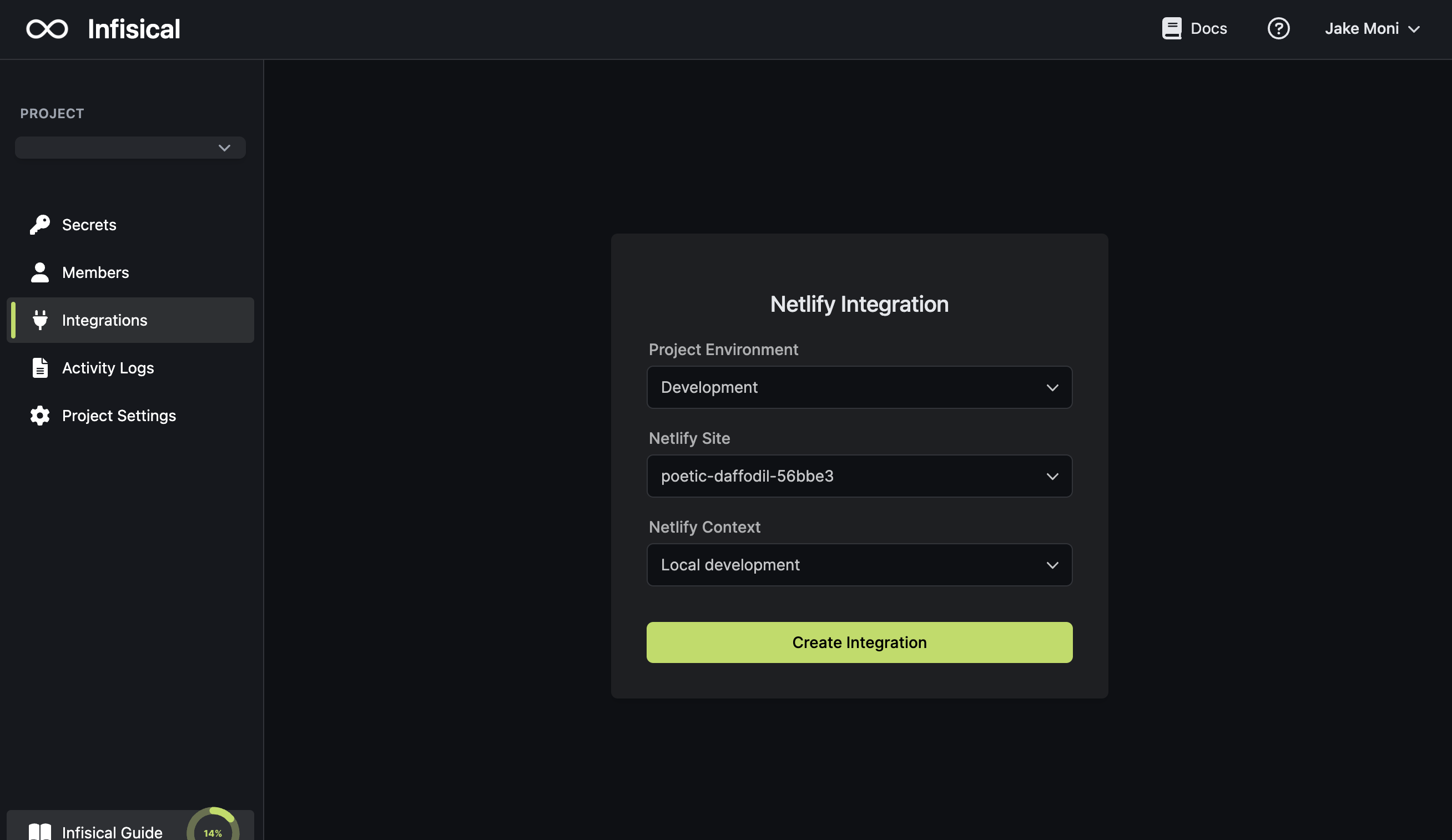
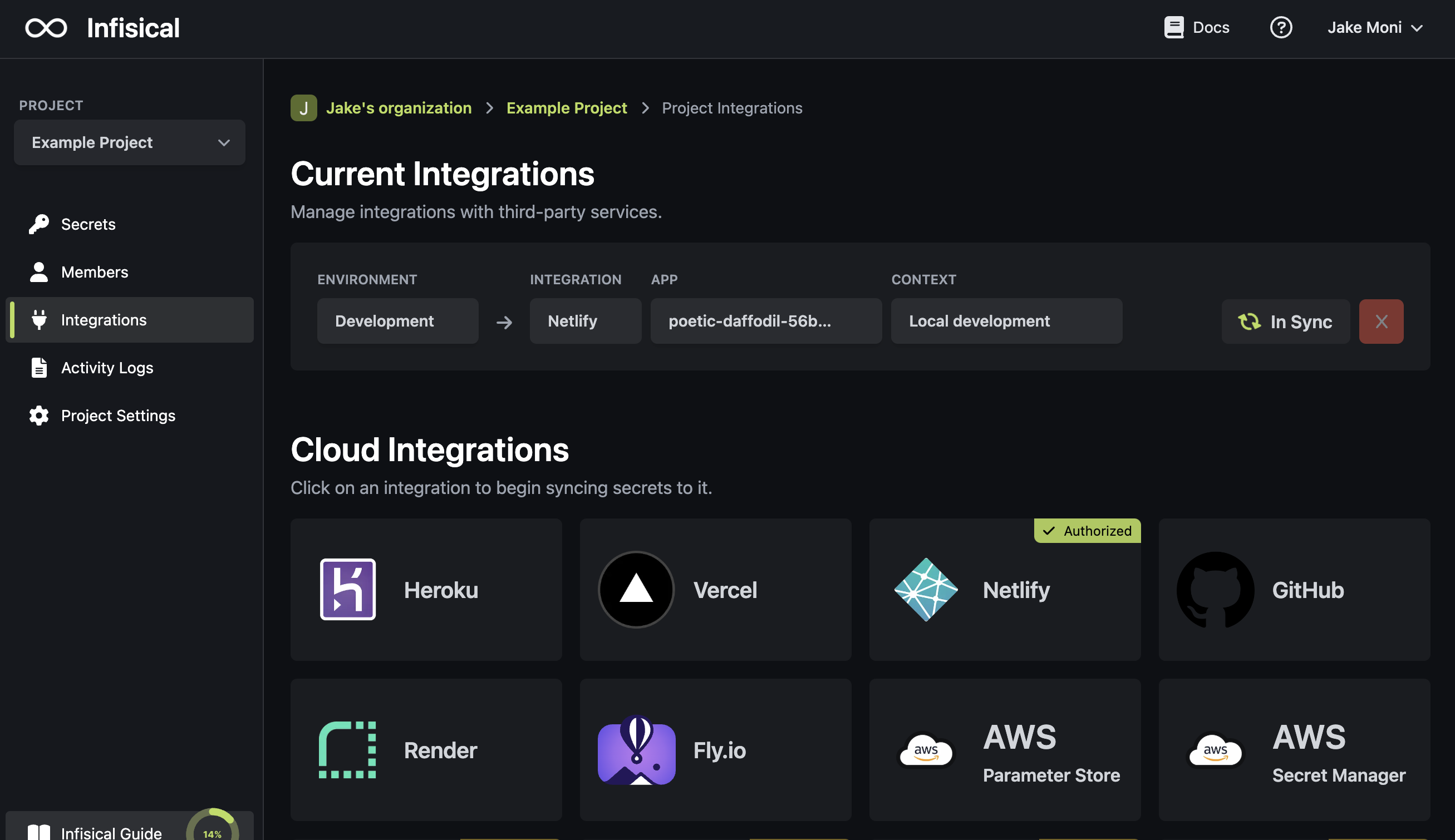
Using the Netlify integration on a self-hosted instance of Infisical requires configuring an OAuth application in Netlify
and registering your instance with it.
Navigate to your User settings > Applications > OAuth to create a new OAuth application.
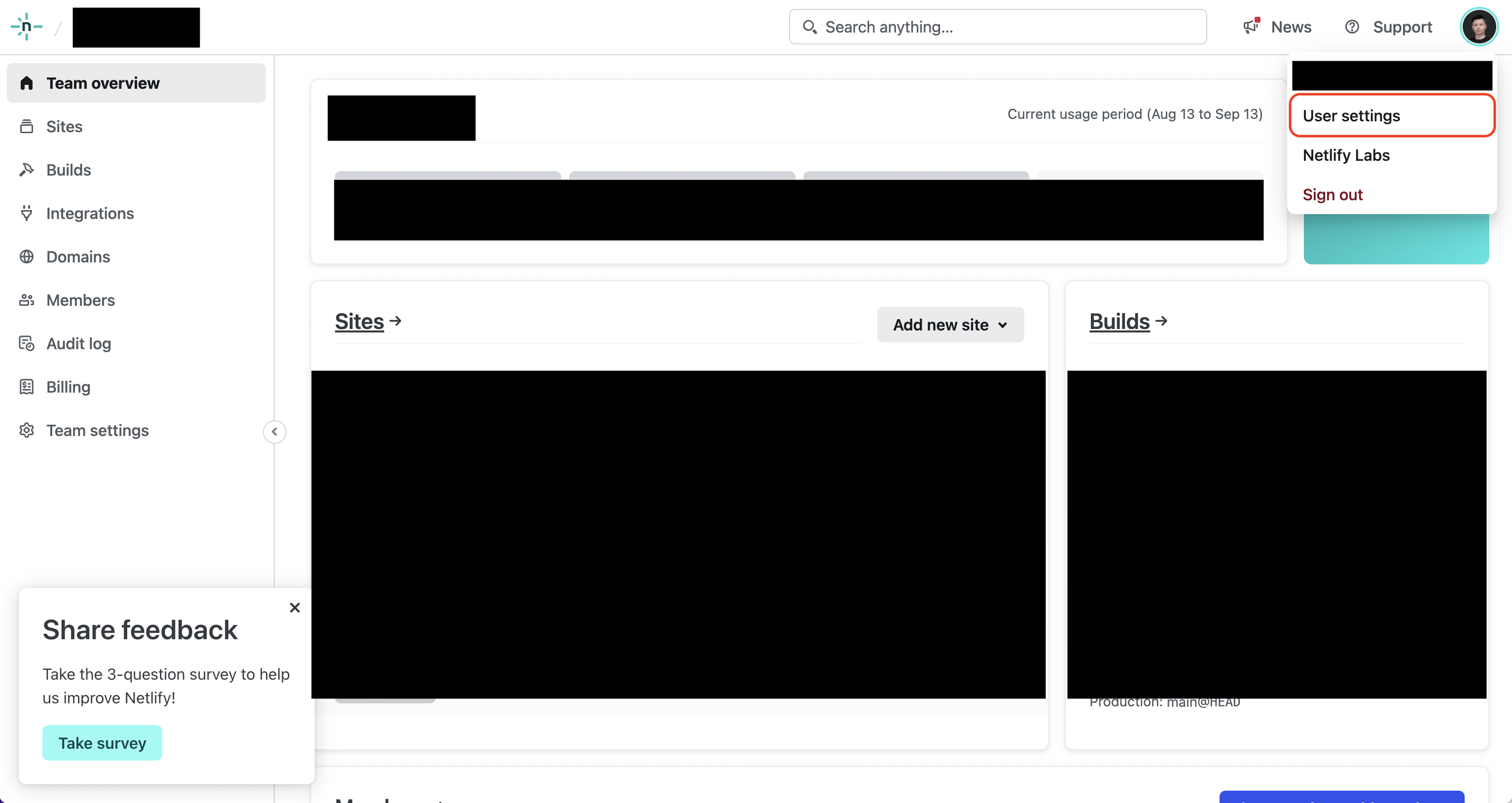
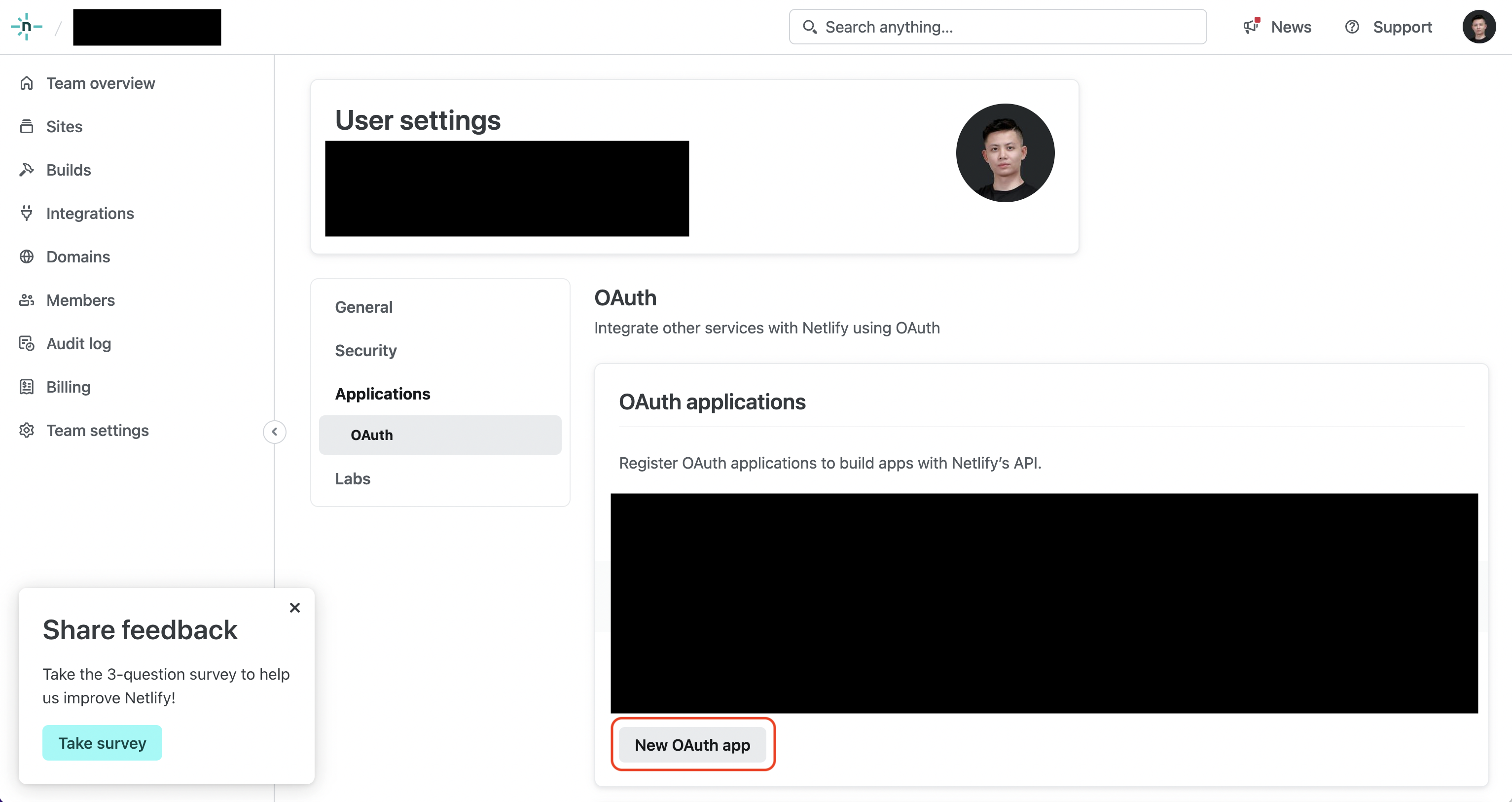
Create the OAuth application. As part of the form, set the **Redirect URI** to `https://your-domain.com/integrations/netlify/oauth2/callback`.
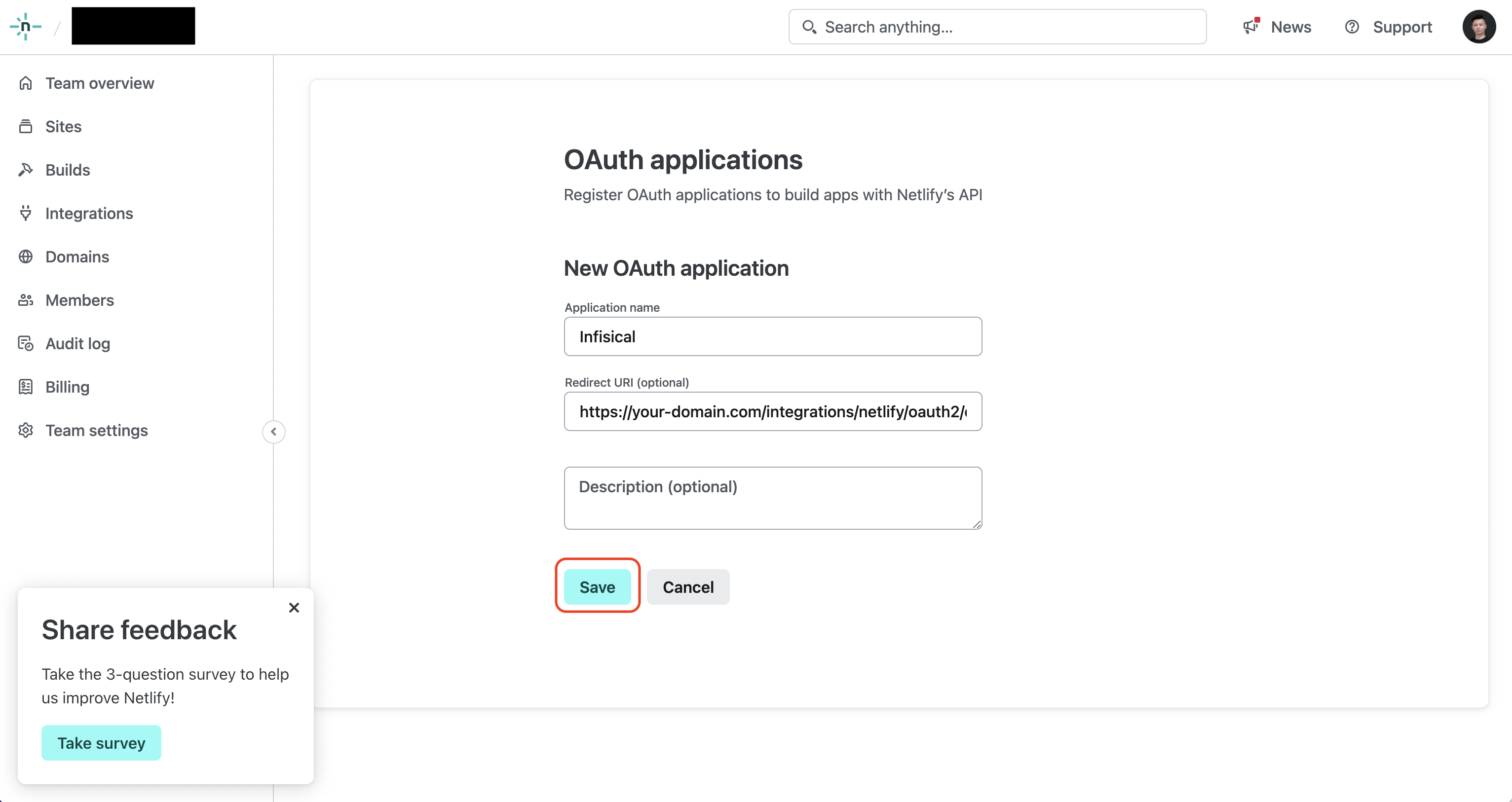
Obtain the **Client ID** and **Secret** for your Netlify OAuth application.
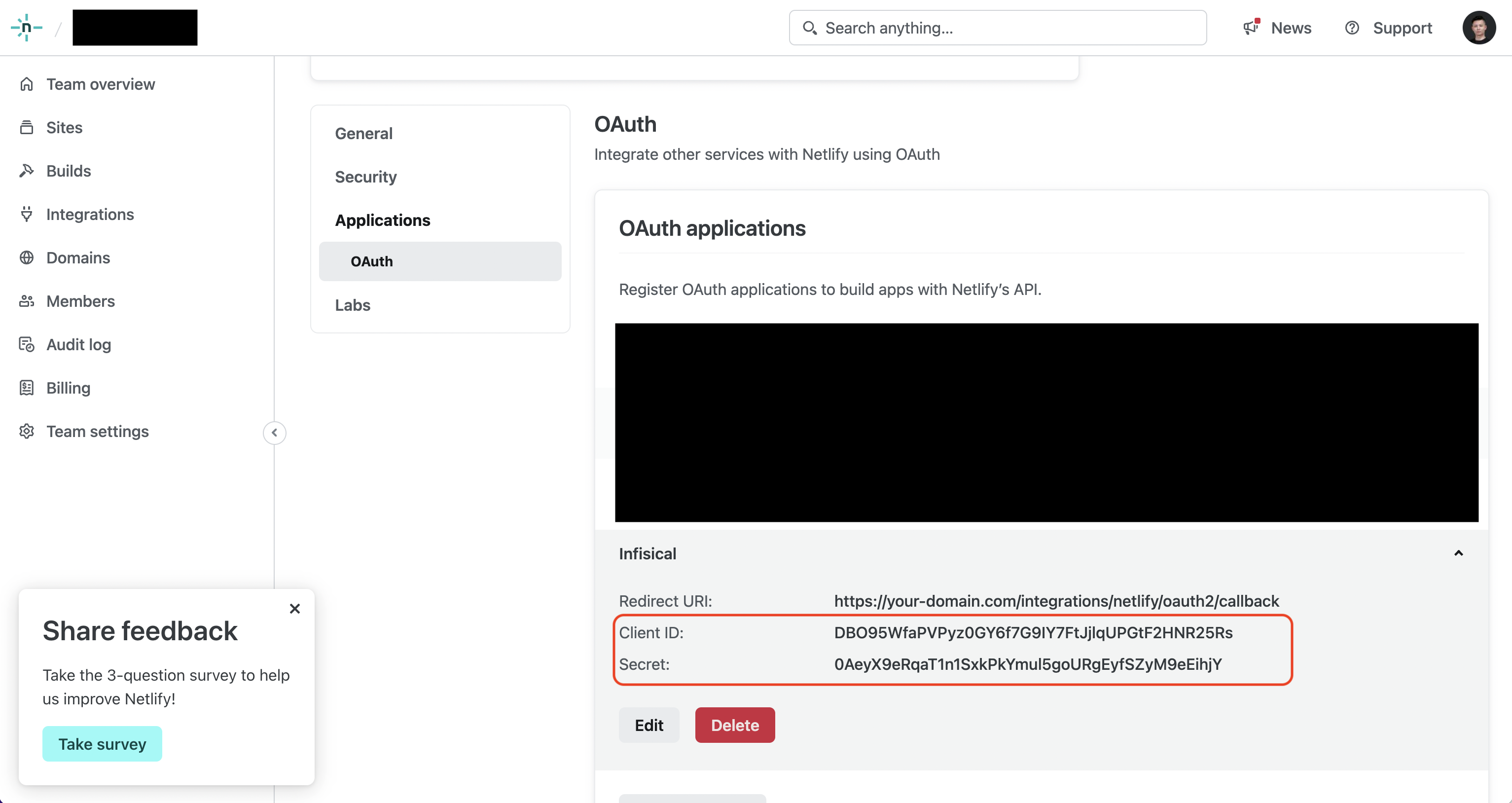
Back in your Infisical instance, add two new environment variables for the credentials of your Netlify OAuth application.
* `CLIENT_ID_NETLIFY`: The **Client ID** of your Netlify OAuth application.
* `CLIENT_SECRET_NETLIFY`: The **Secret** of your Netlify OAuth application.
Once added, restart your Infisical instance and use the Netlify integration.
# Northflank
How to sync secrets from Infisical to Northflank
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
* Have a [Northflank](https://northflank.com) project with a secret group ready
Obtain a Northflank API token in Account settings > API > Tokens
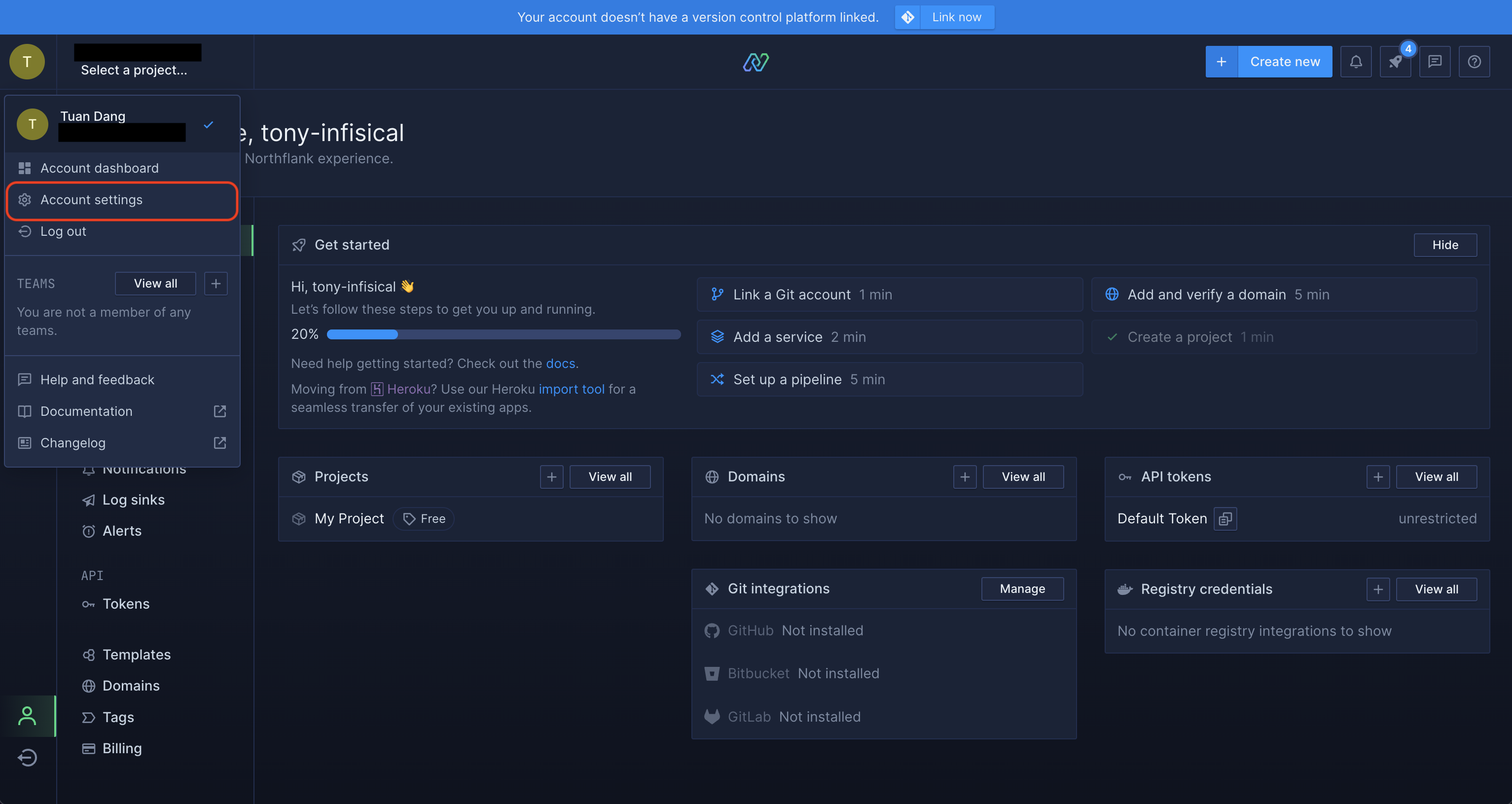
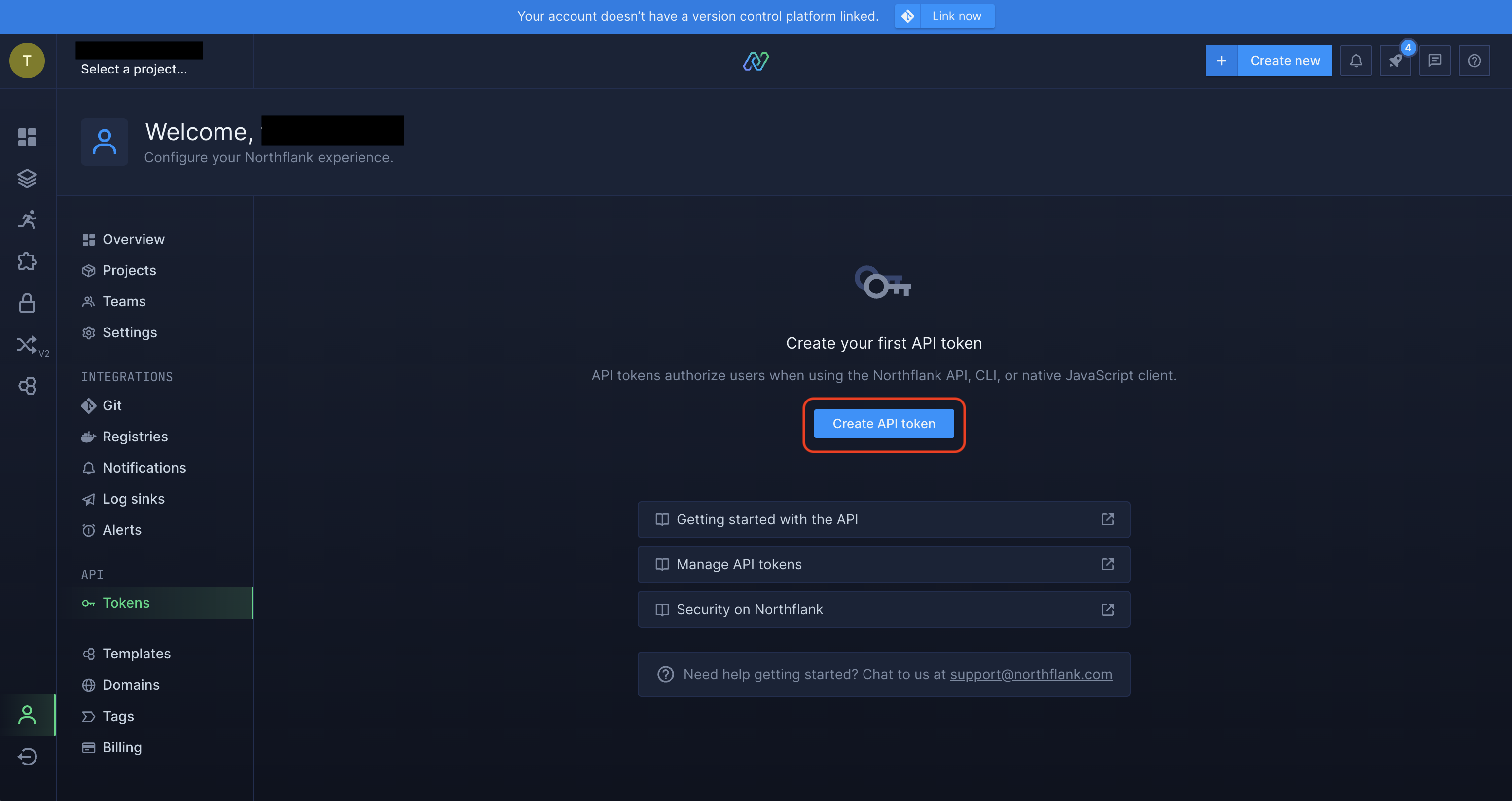
Navigate to your project's integrations tab in Infisical.
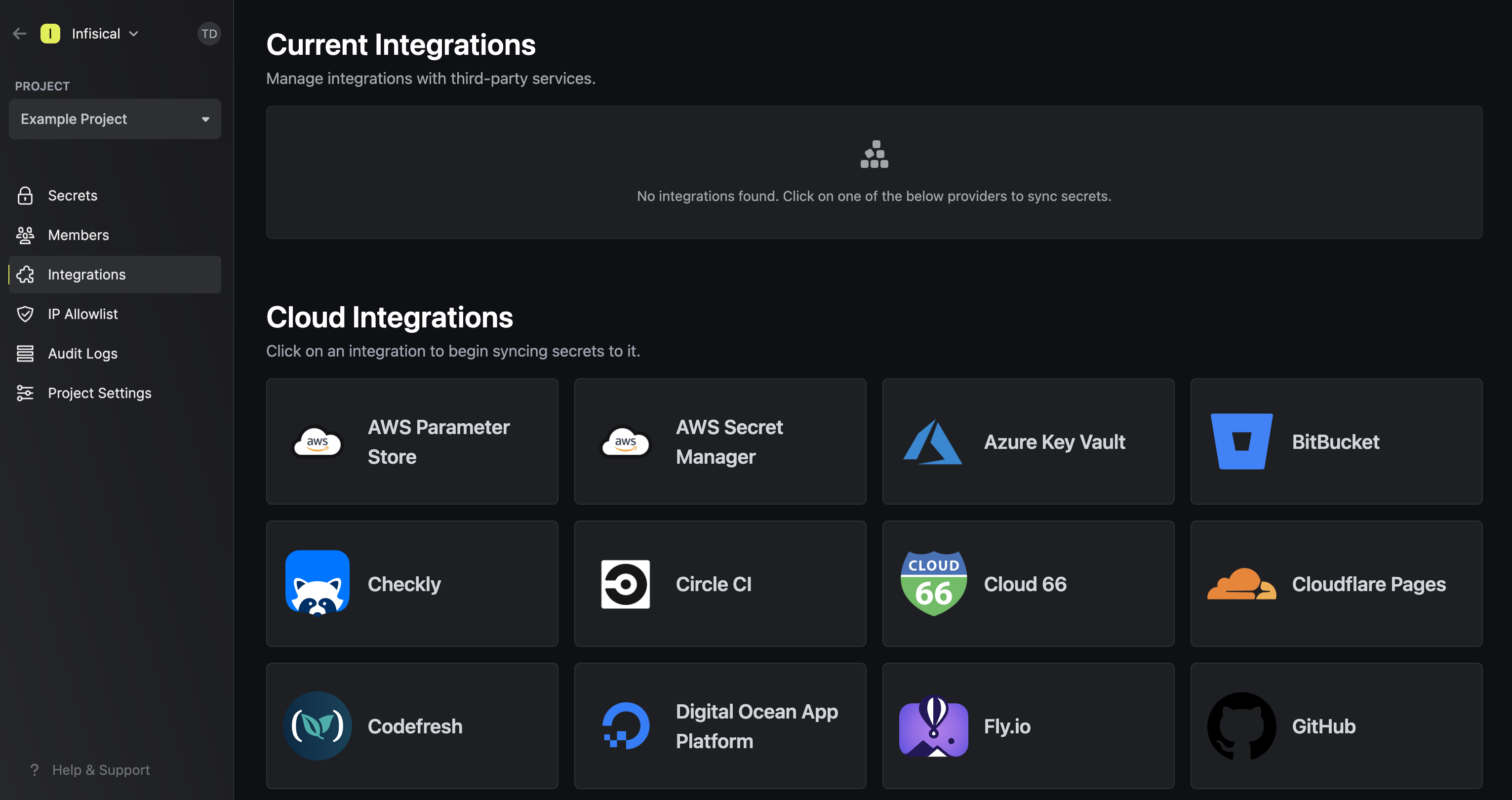
Press on the Northflank tile and input your Northflank API token to grant Infisical access to your Northflank account.
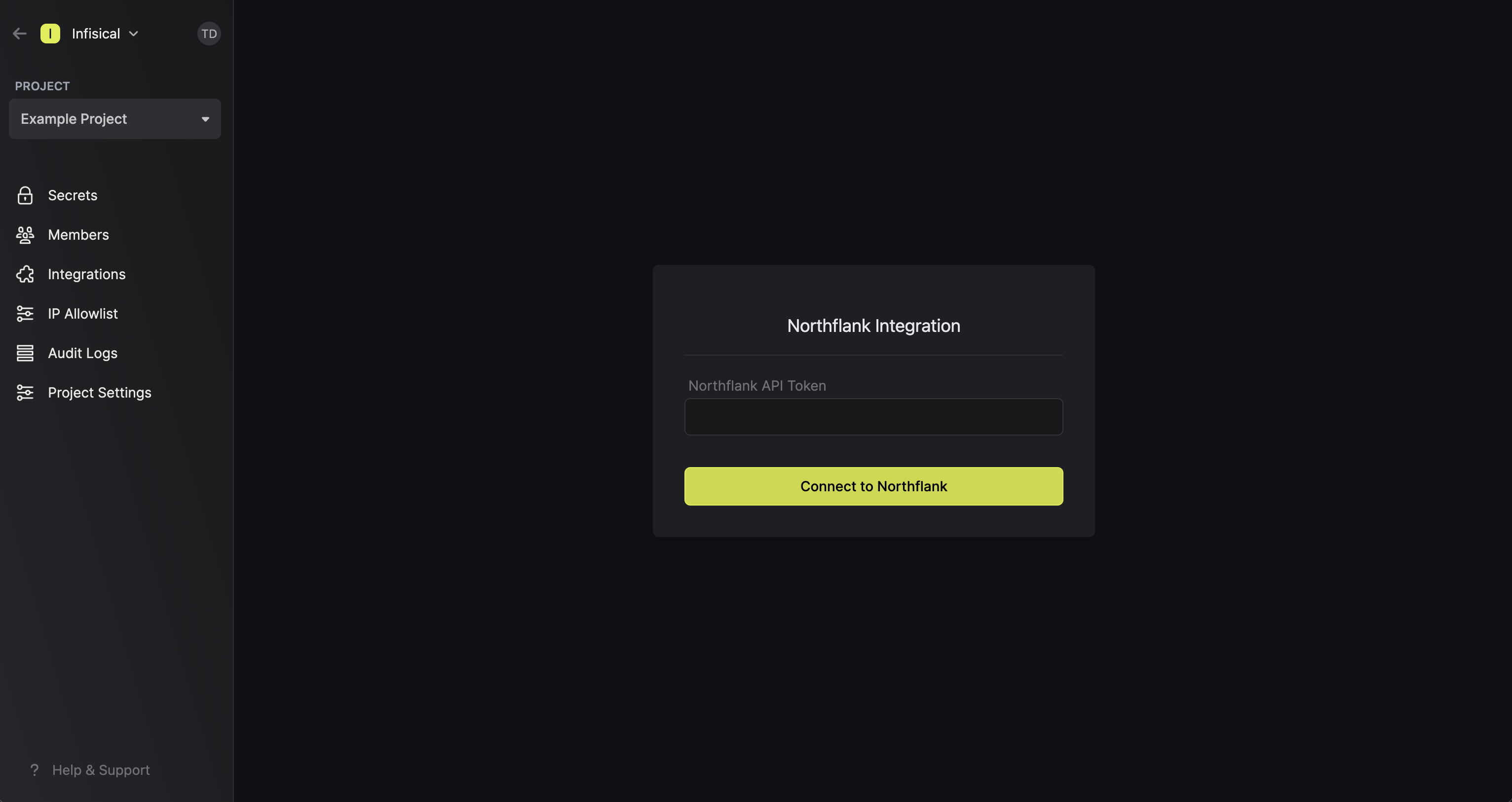
Select which Infisical environment secrets you want to sync to which Northflank project and secret group. Finally, press create integration to start syncing secrets to Northflank.
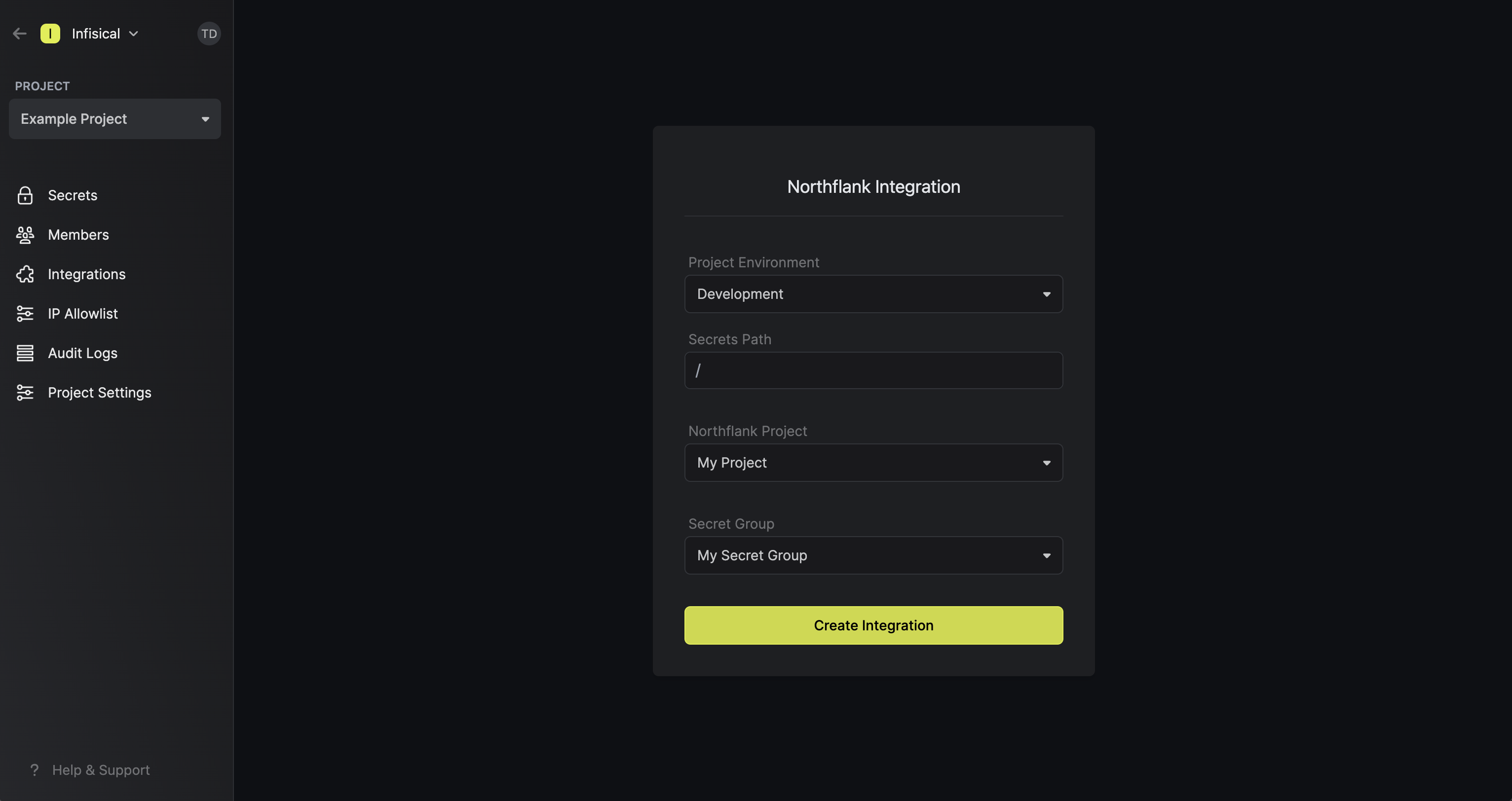
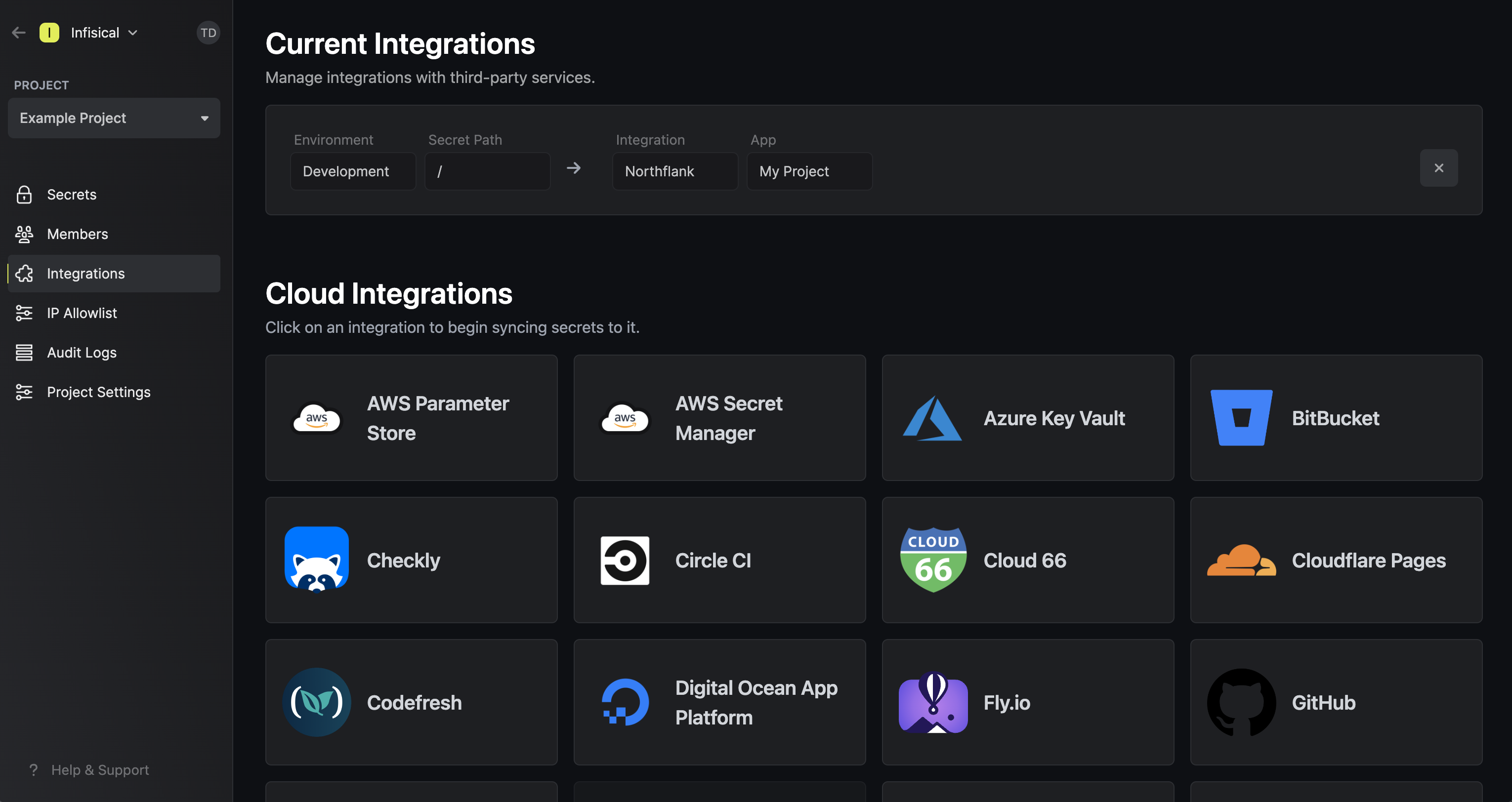
# Qovery
How to sync secrets from Infisical to Qovery
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
Obtain a Qovery API Token in Settings > API Token.
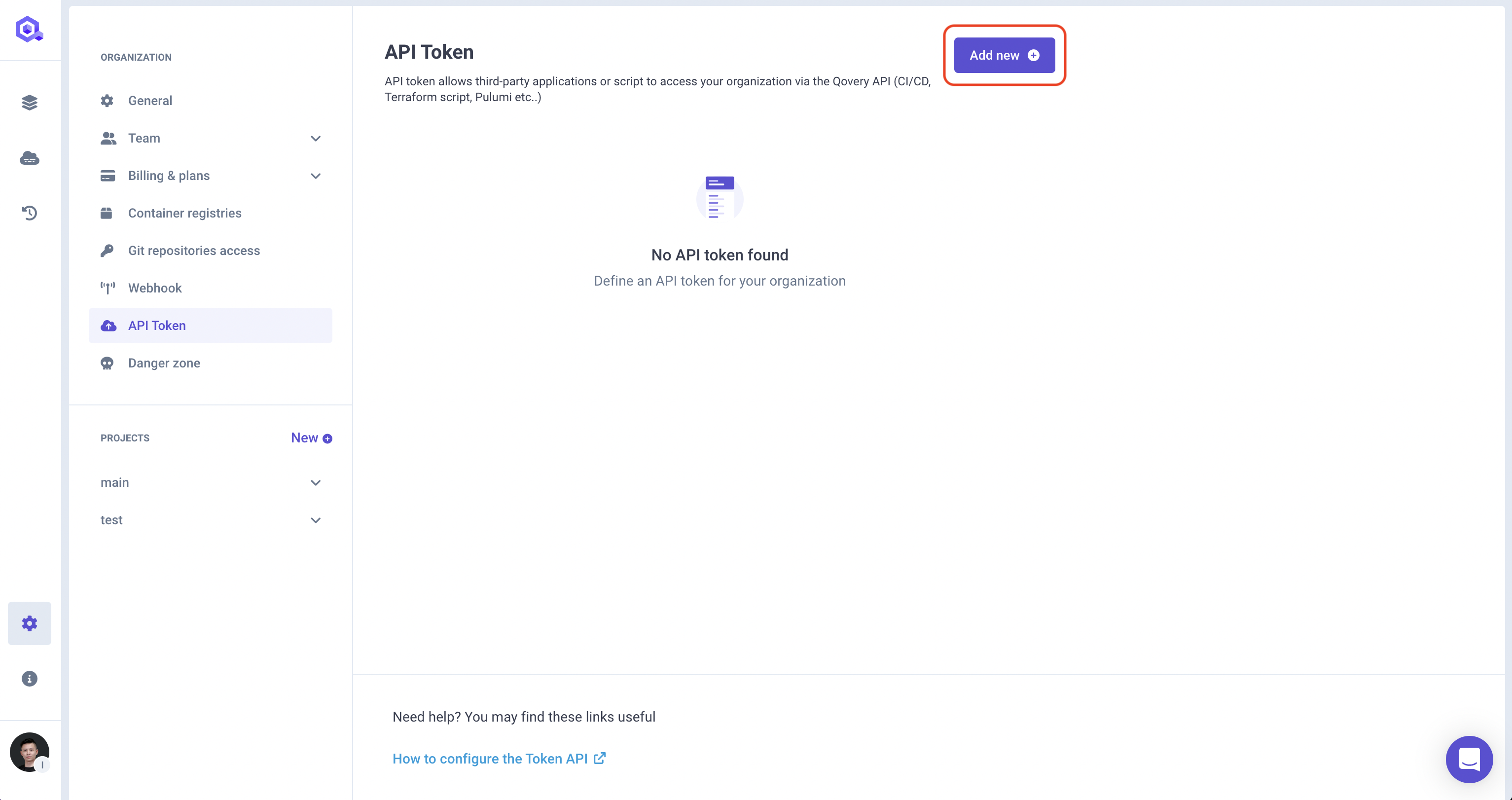
Navigate to your project's integrations tab in Infisical.
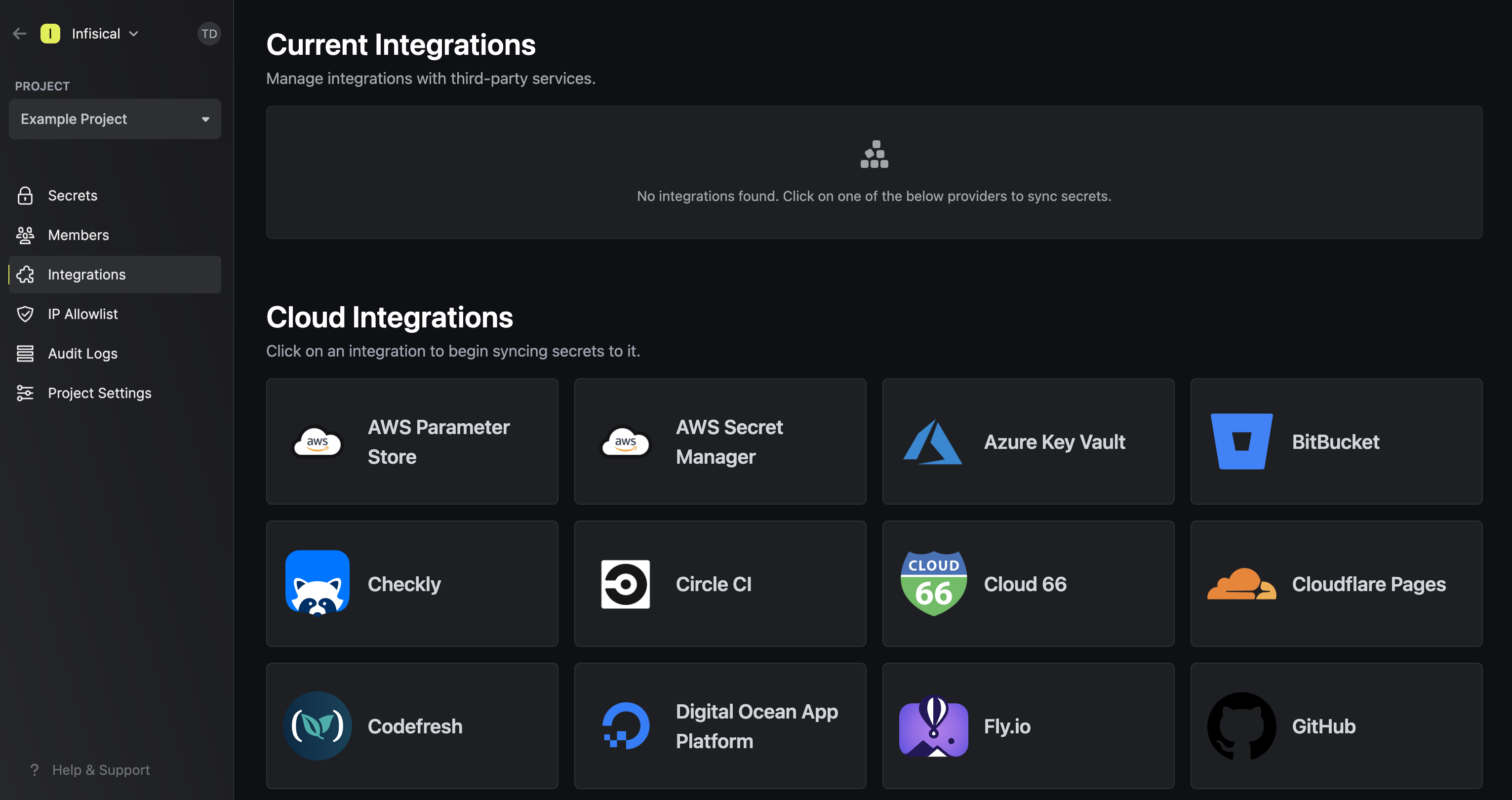
Press on the Qovery tile and input your Qovery API Token to grant Infisical access to your Qovery account.
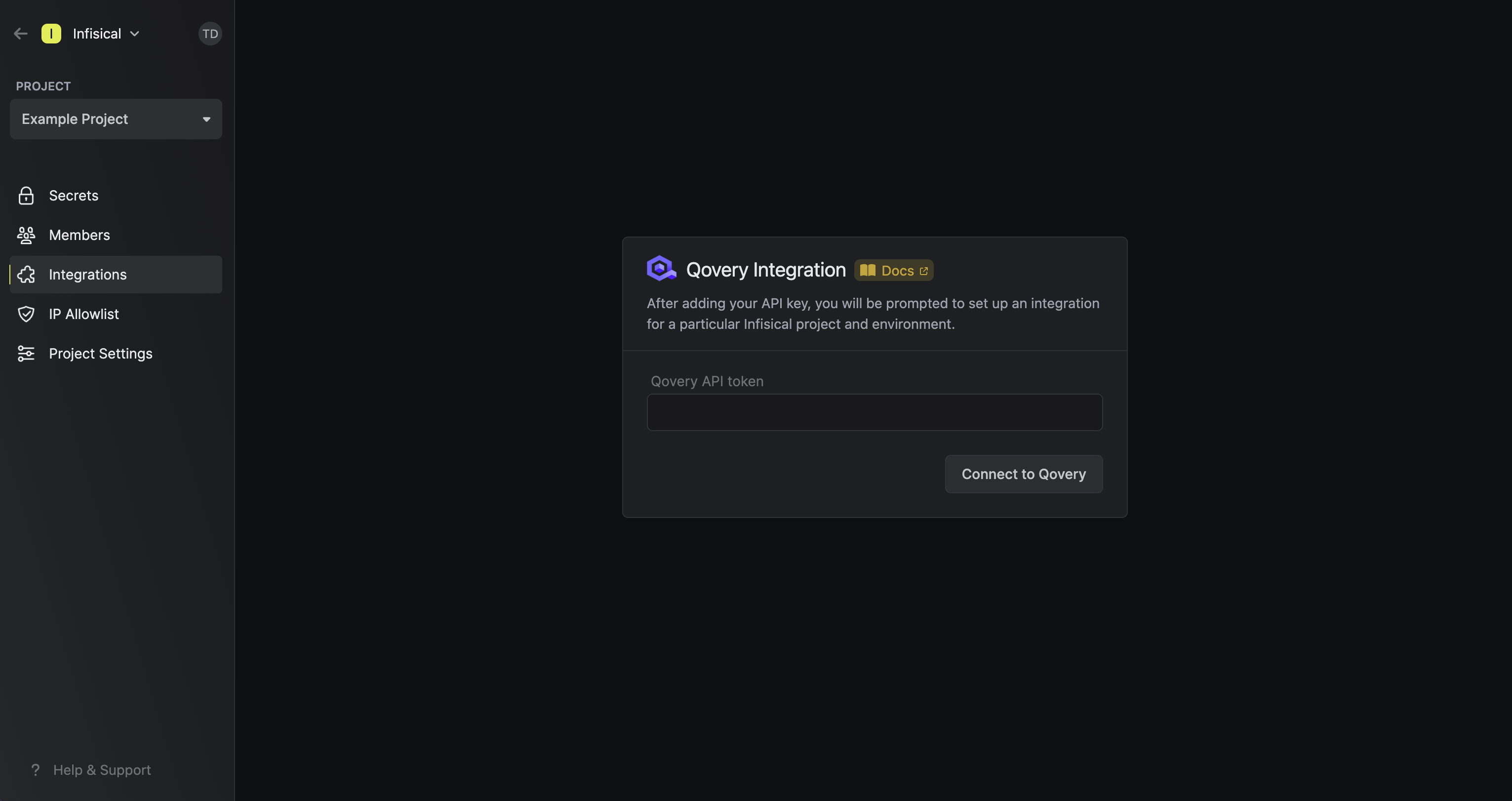
Select which Infisical environment secrets you want to sync to Qovery and press create integration to start syncing secrets.
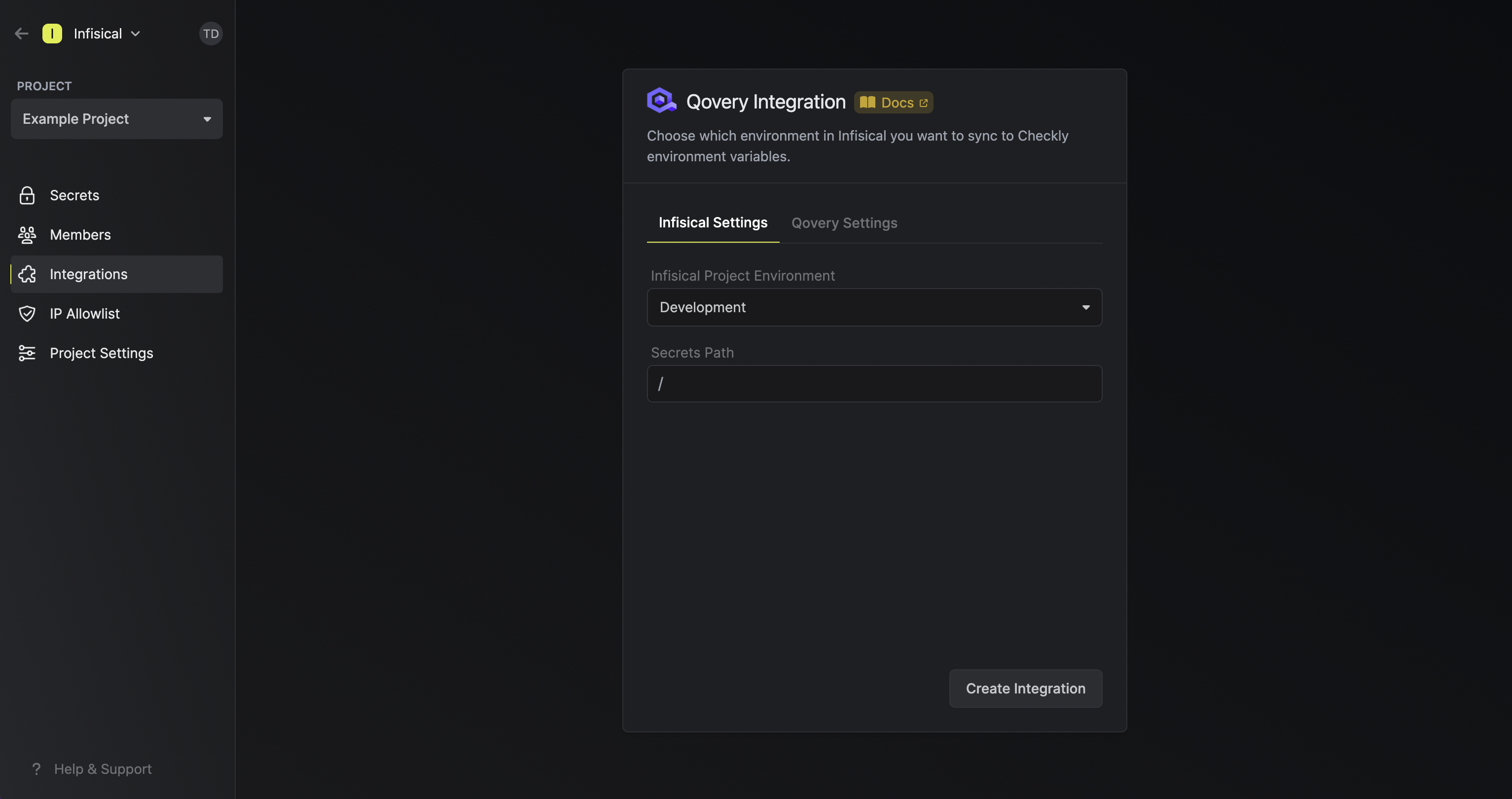
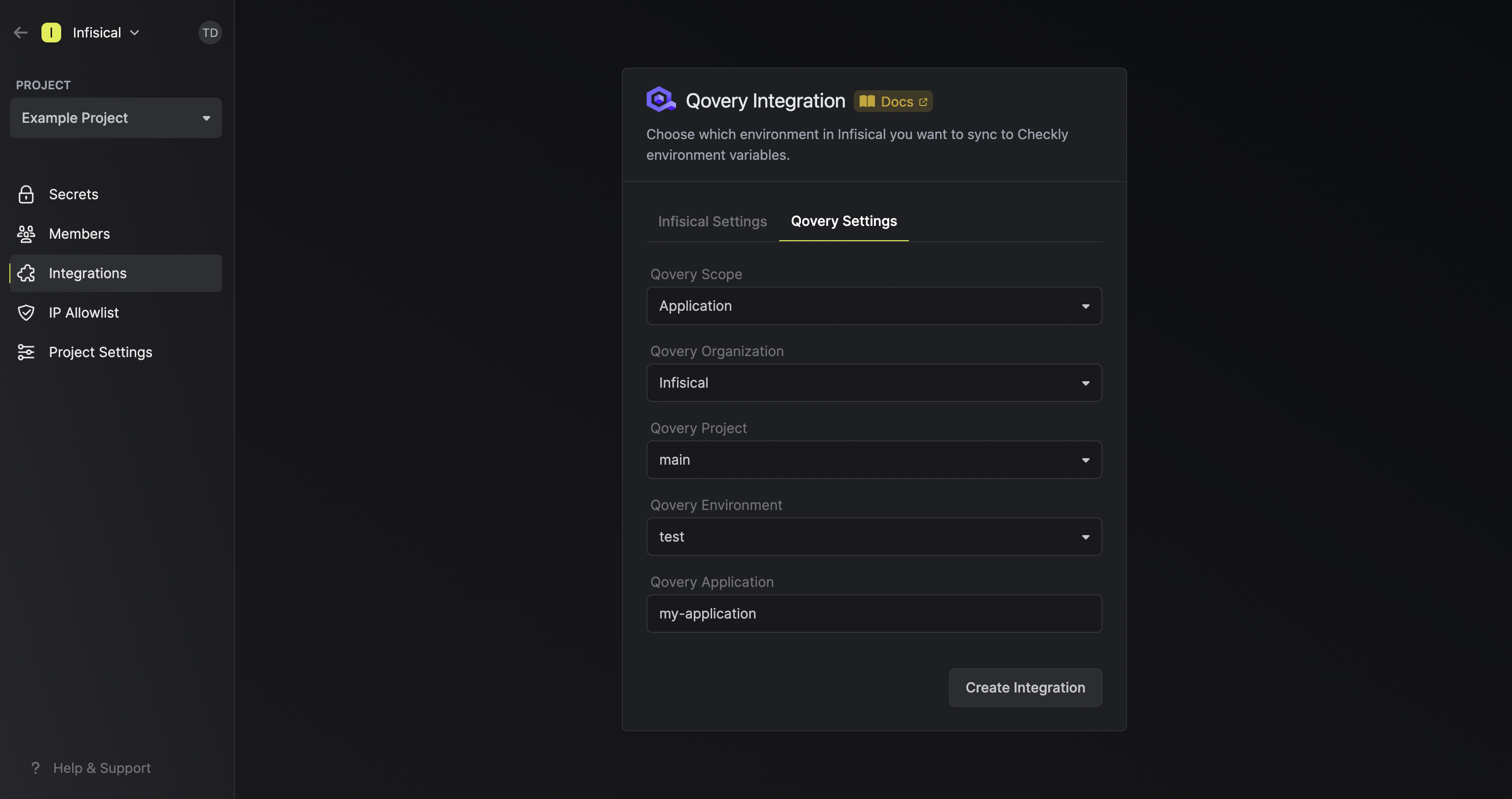
Infisical supports syncing secrets to various Qovery scopes including applications, jobs, or containers.
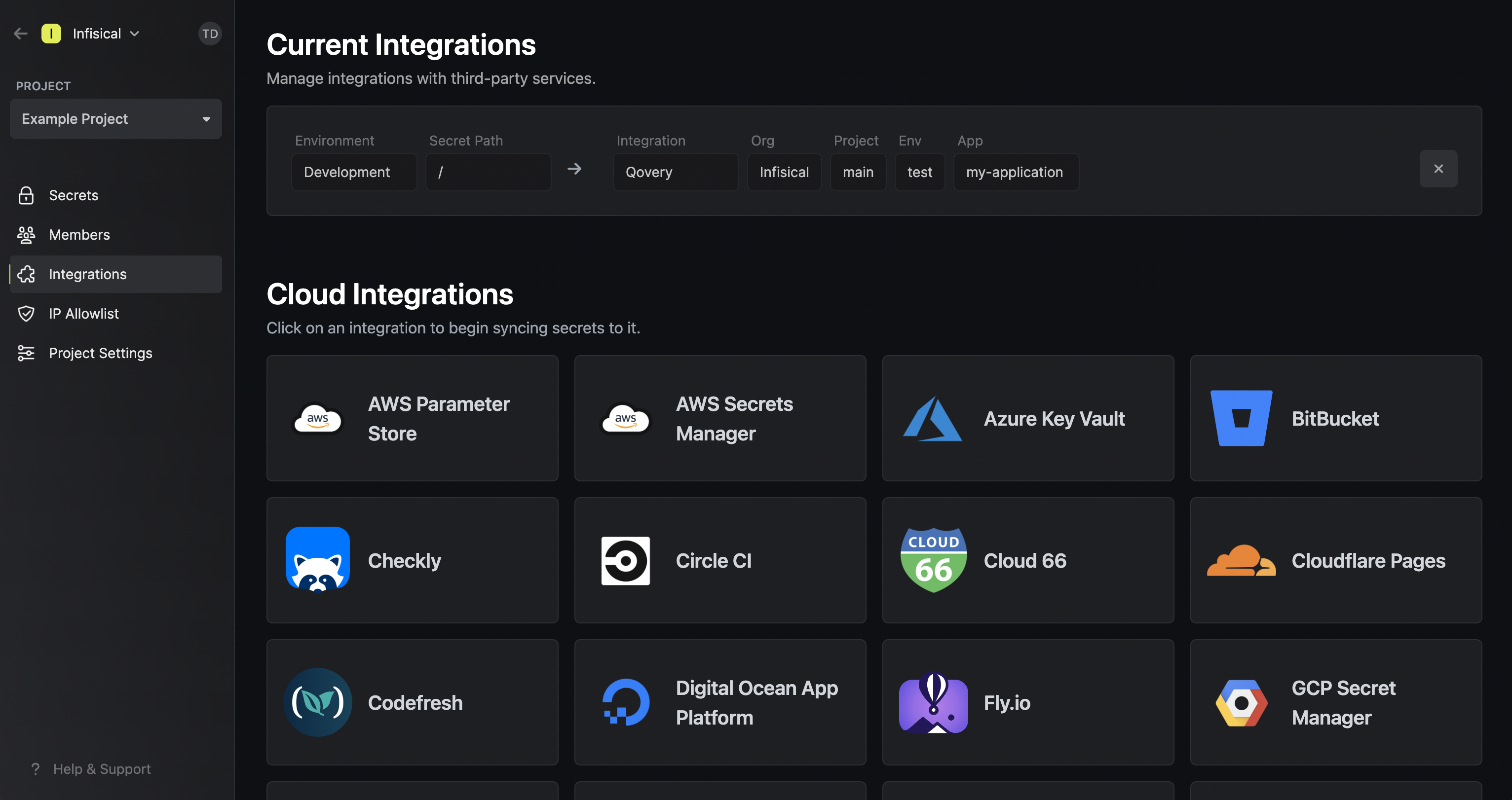
# Railway
How to sync secrets from Infisical to Railway
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
Obtain a Railway API Token in your Railway [Account Settings > Tokens](https://railway.app/account/tokens).
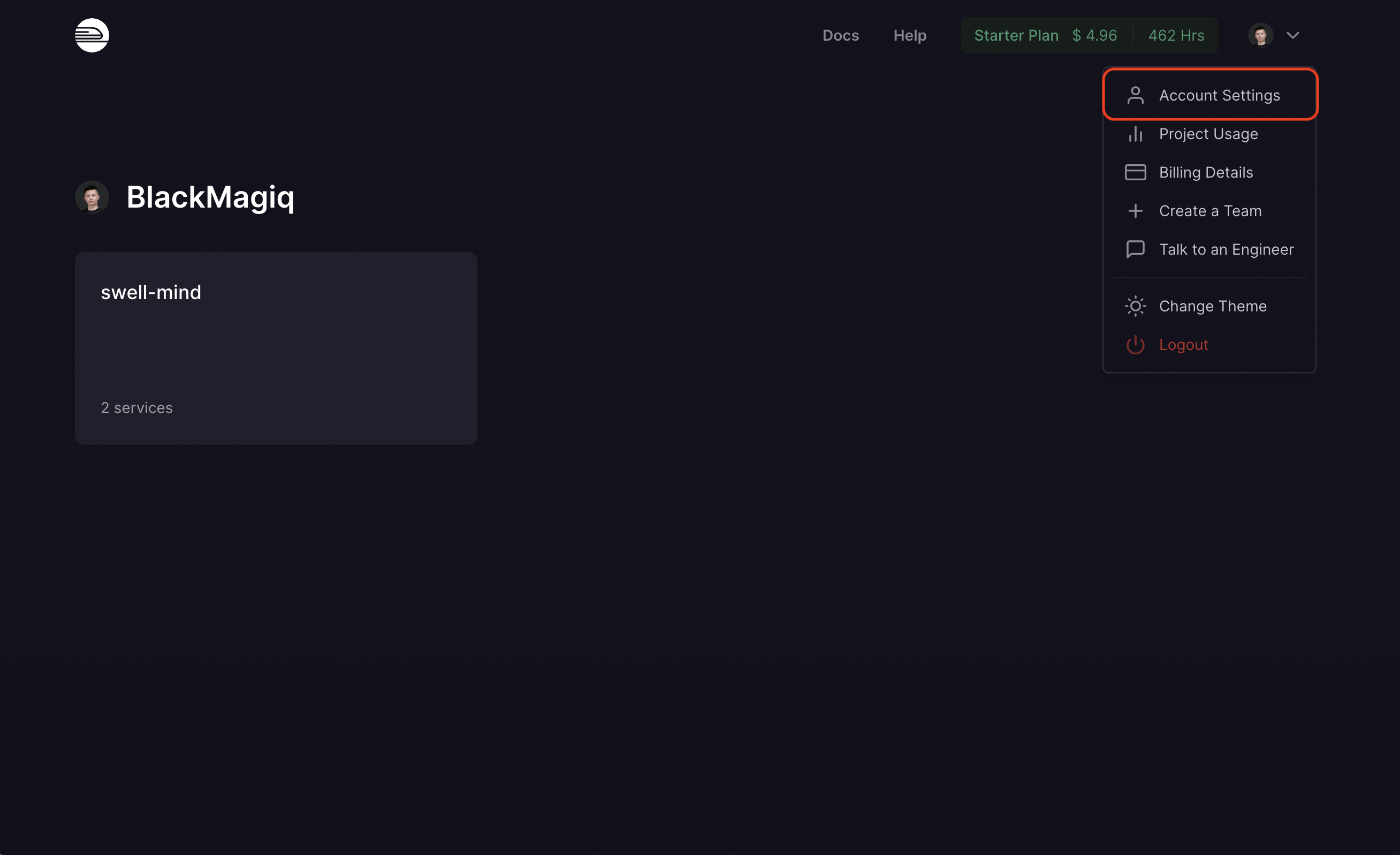
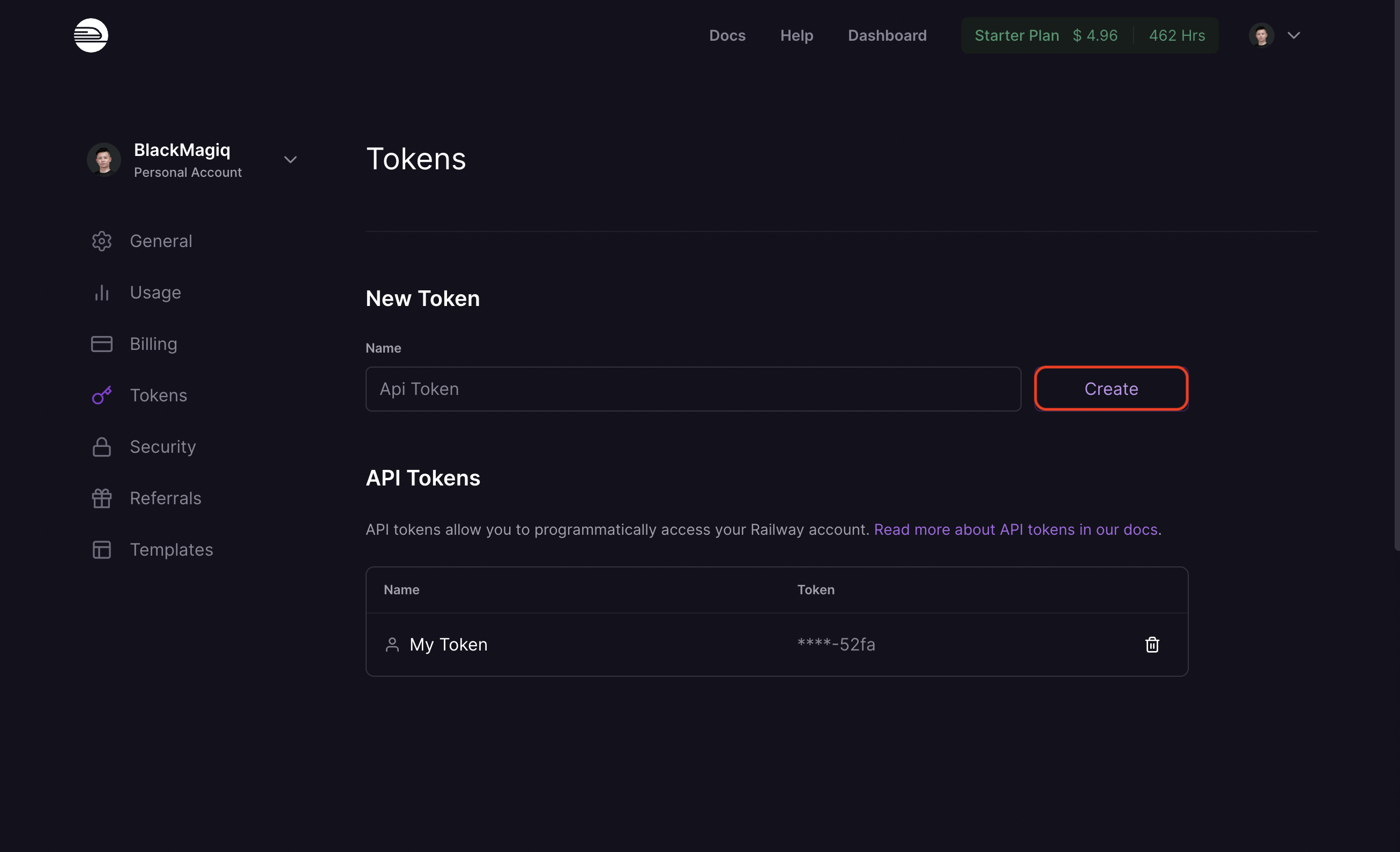
If this is your first time creating a Railway API token, then you'll be prompted to join
Railway's Private Boarding Beta program on the Railway Account Settings > Tokens page.
Note that Railway project tokens will not work for this integration since they don't work with
Railway's Public API.
Navigate to your project's integrations tab in Infisical.
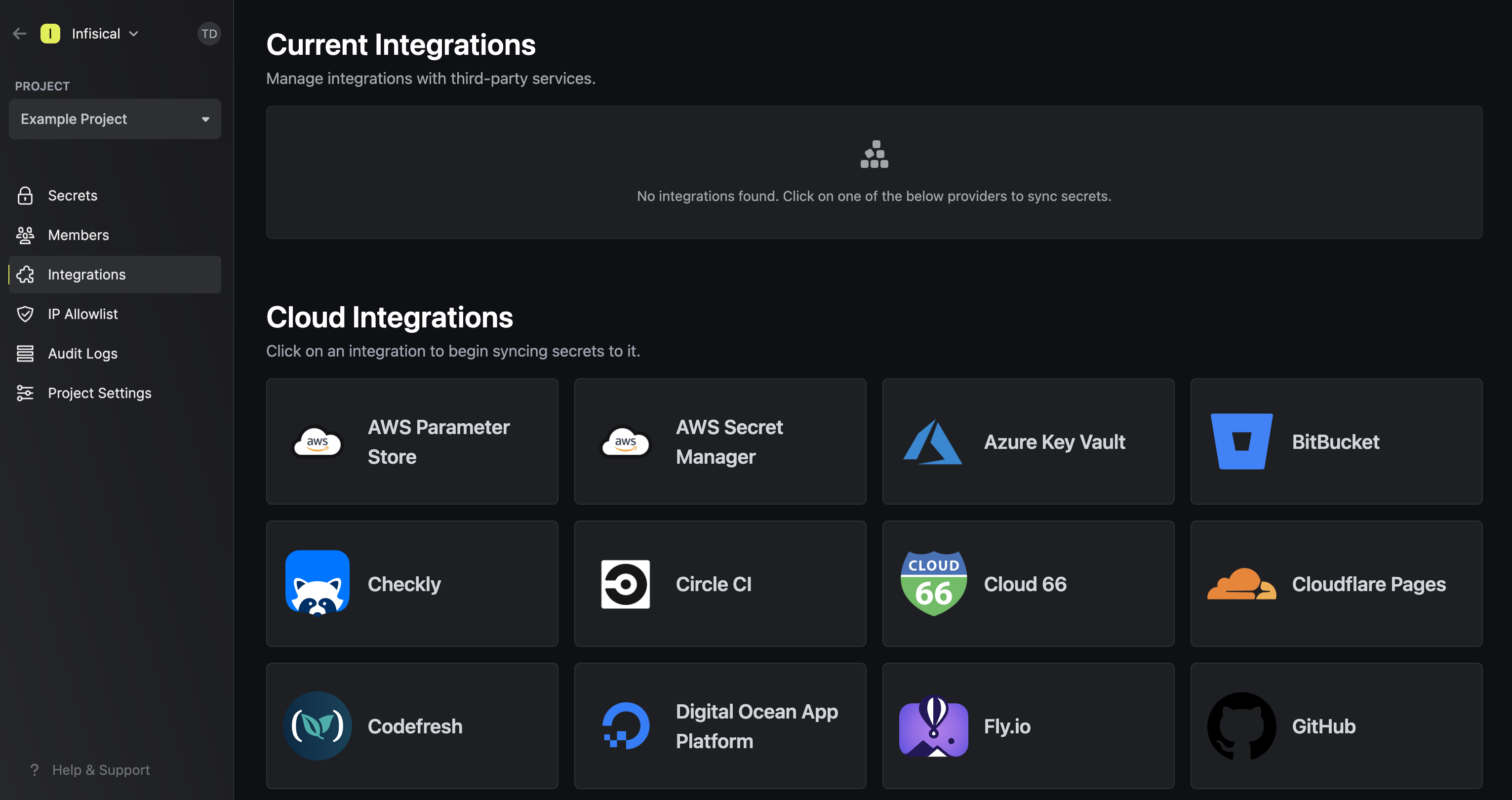
Press on the Railway tile and input your Railway API Key to grant Infisical access to your Railway account.
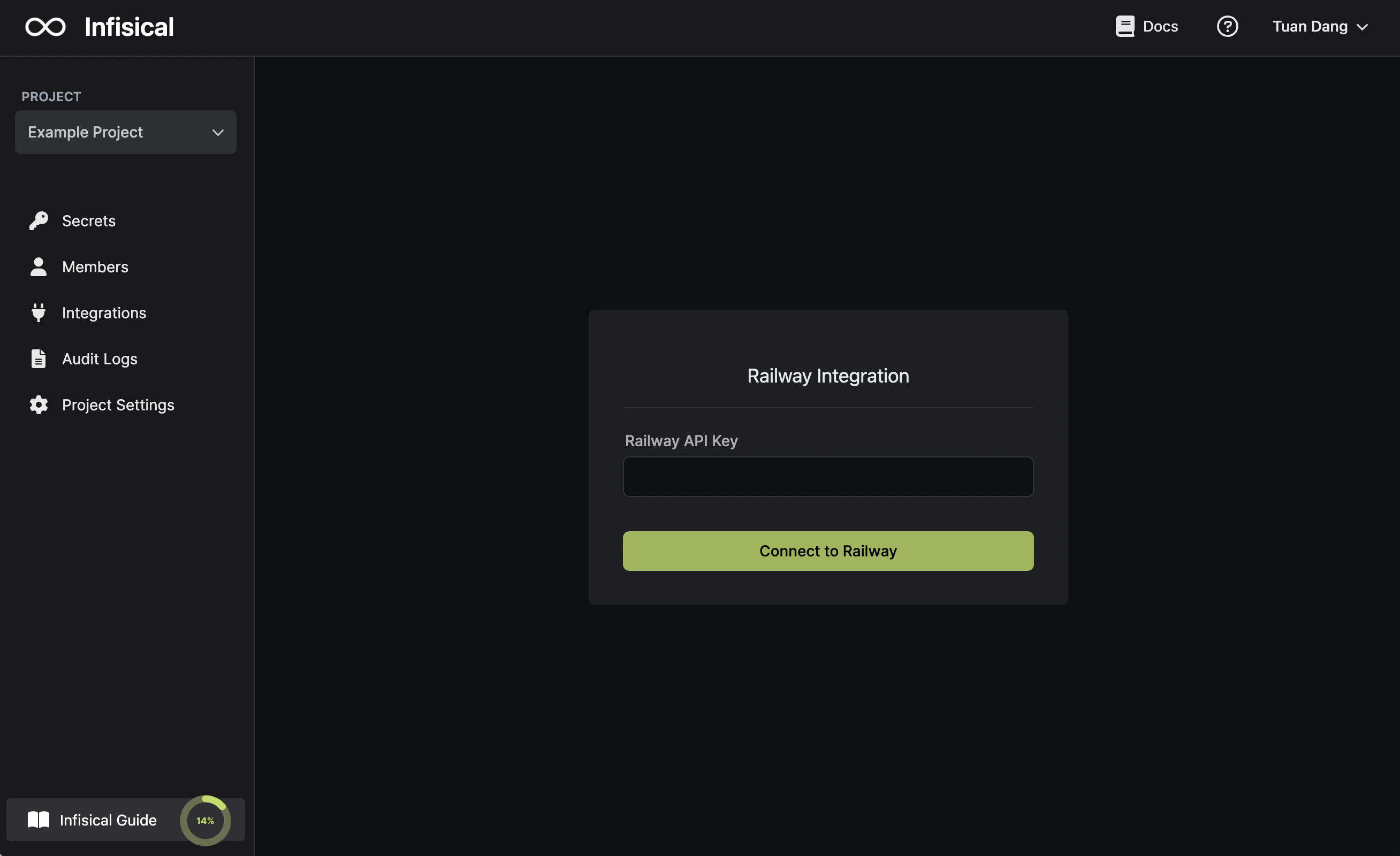
Select which Infisical environment secrets you want to sync to which Railway project and environment (and optionally service). Lastly, press create integration to start syncing secrets to Railway.
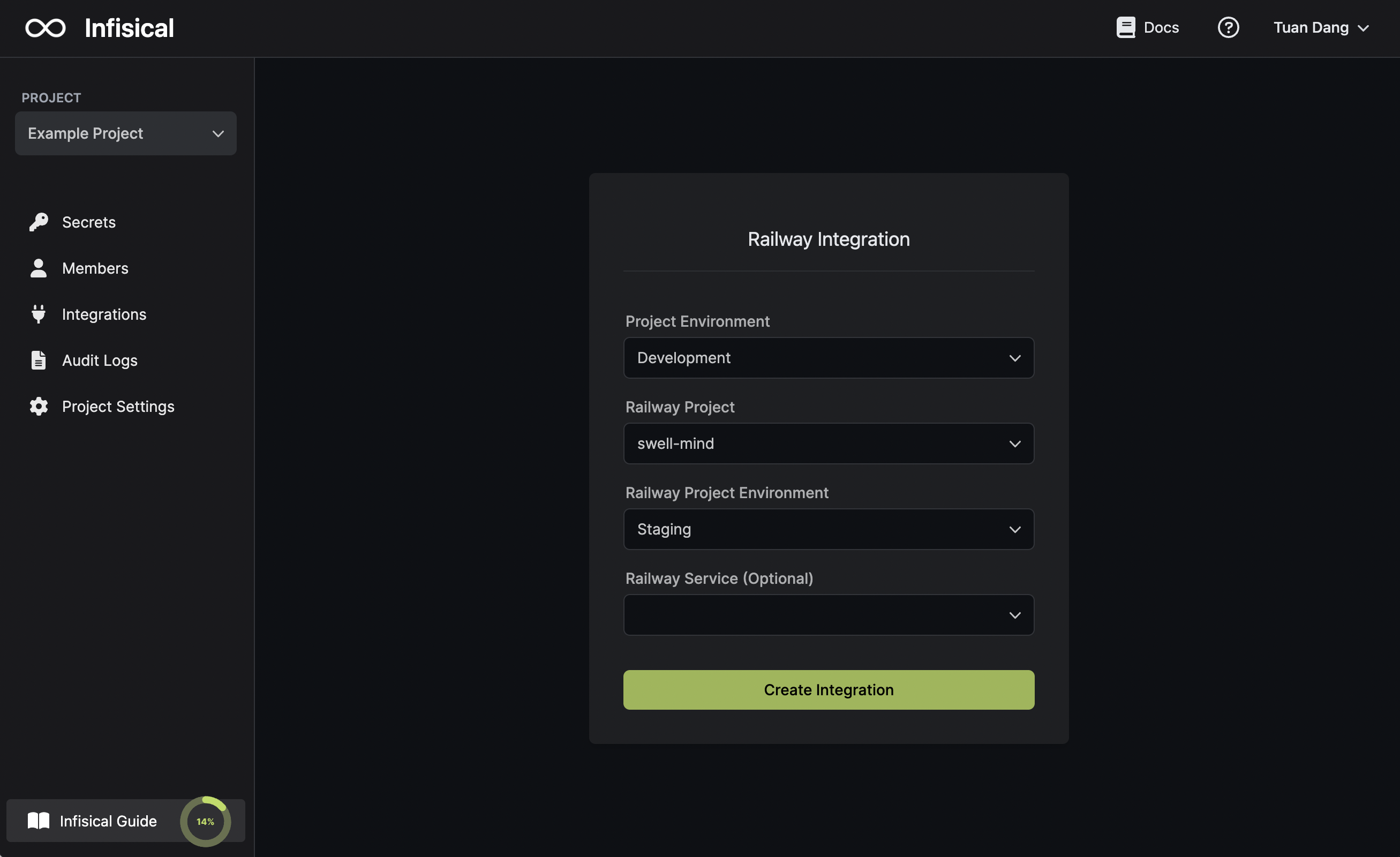
Infisical integrates with both Railway's [shared variables](https://blog.railway.app/p/shared-variables-release) at the project environment level as well as service variables at the service level.
To sync secrets to a specific service in a project, you can select a service from the Railway Service dropdown; otherwise, leaving it empty will sync secrets to the shared variables of that project.
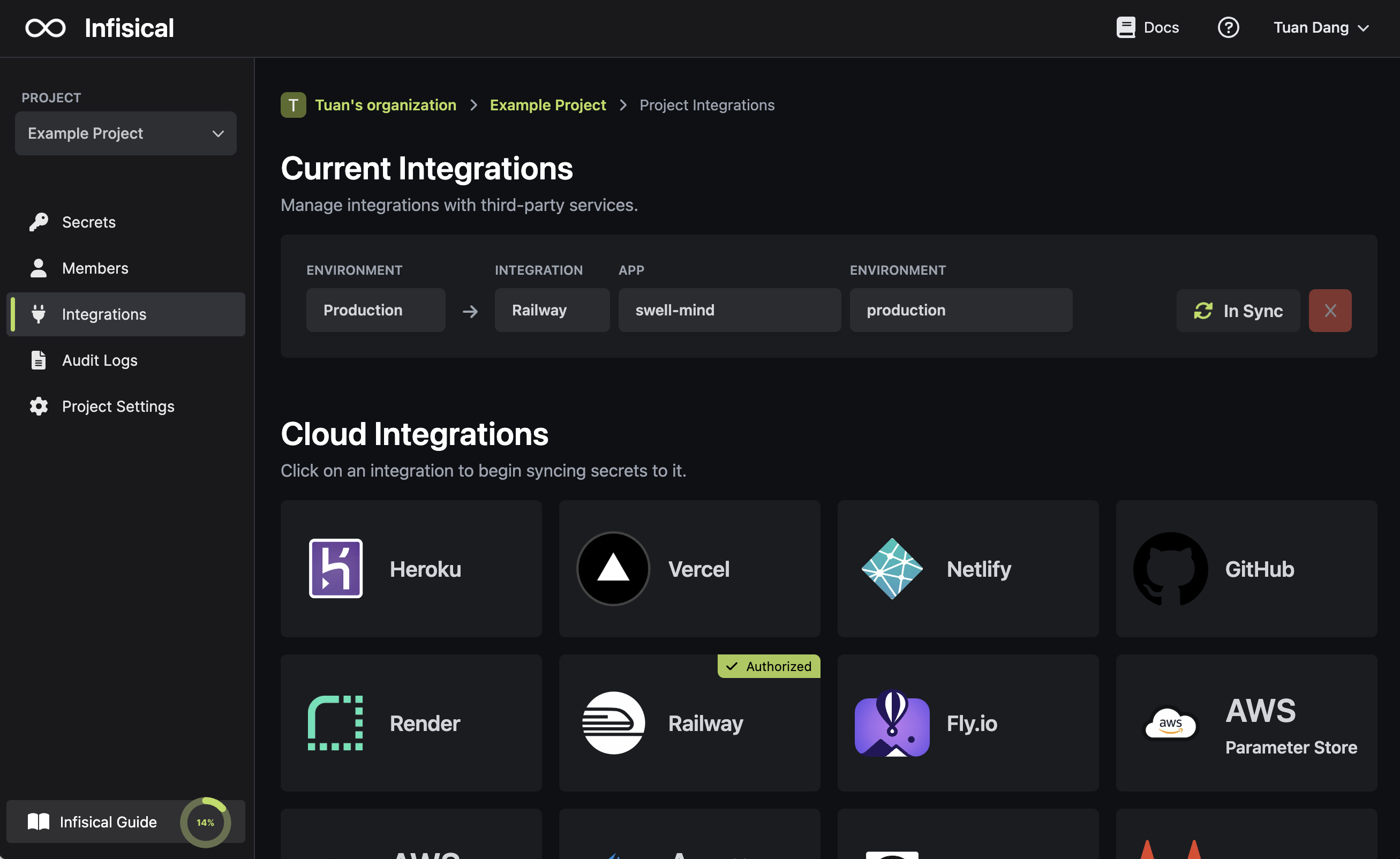
# Render
How to sync secrets from Infisical to Render
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
Obtain a Render API Key in your Render Account Settings > API Keys.
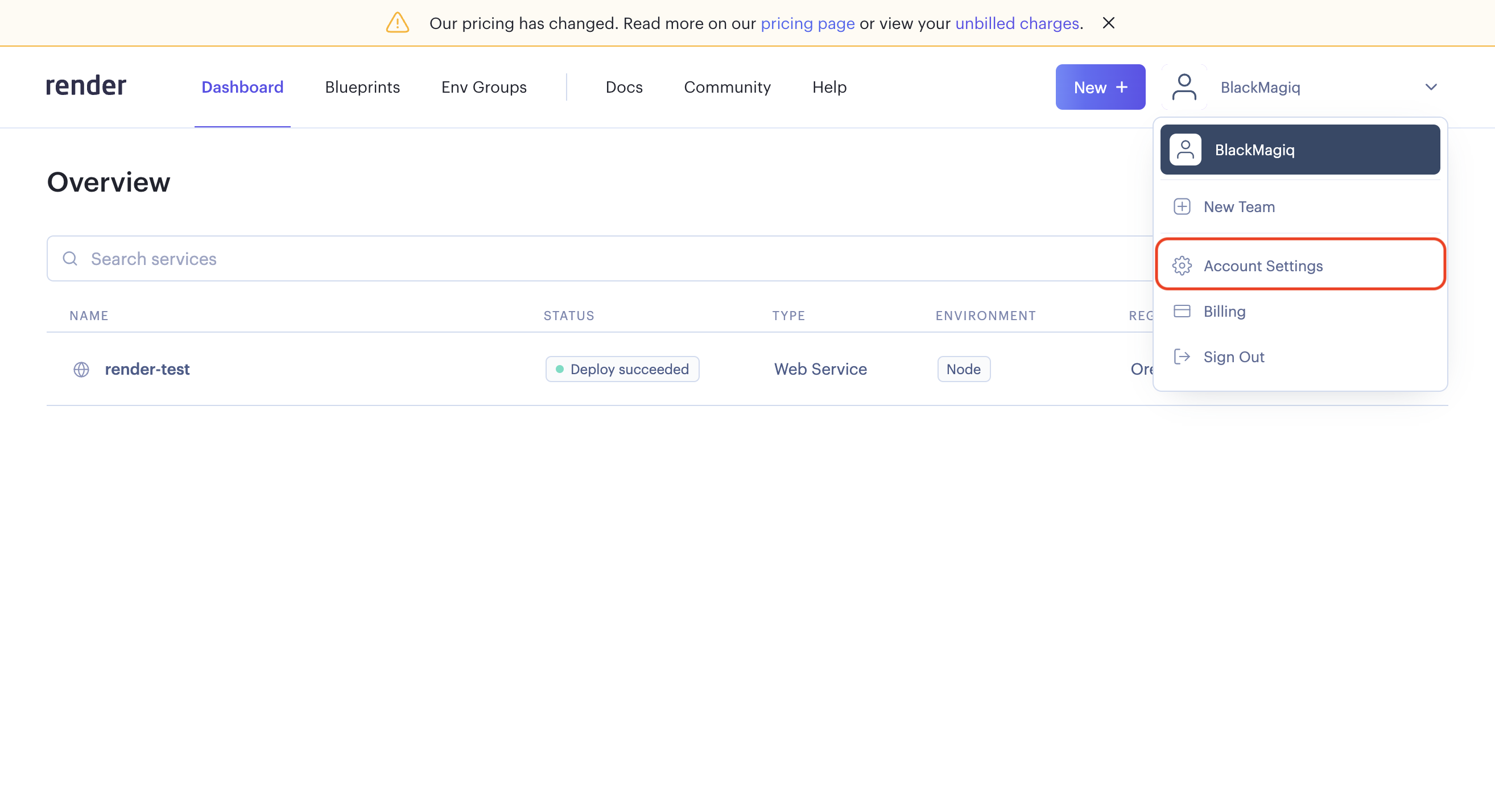
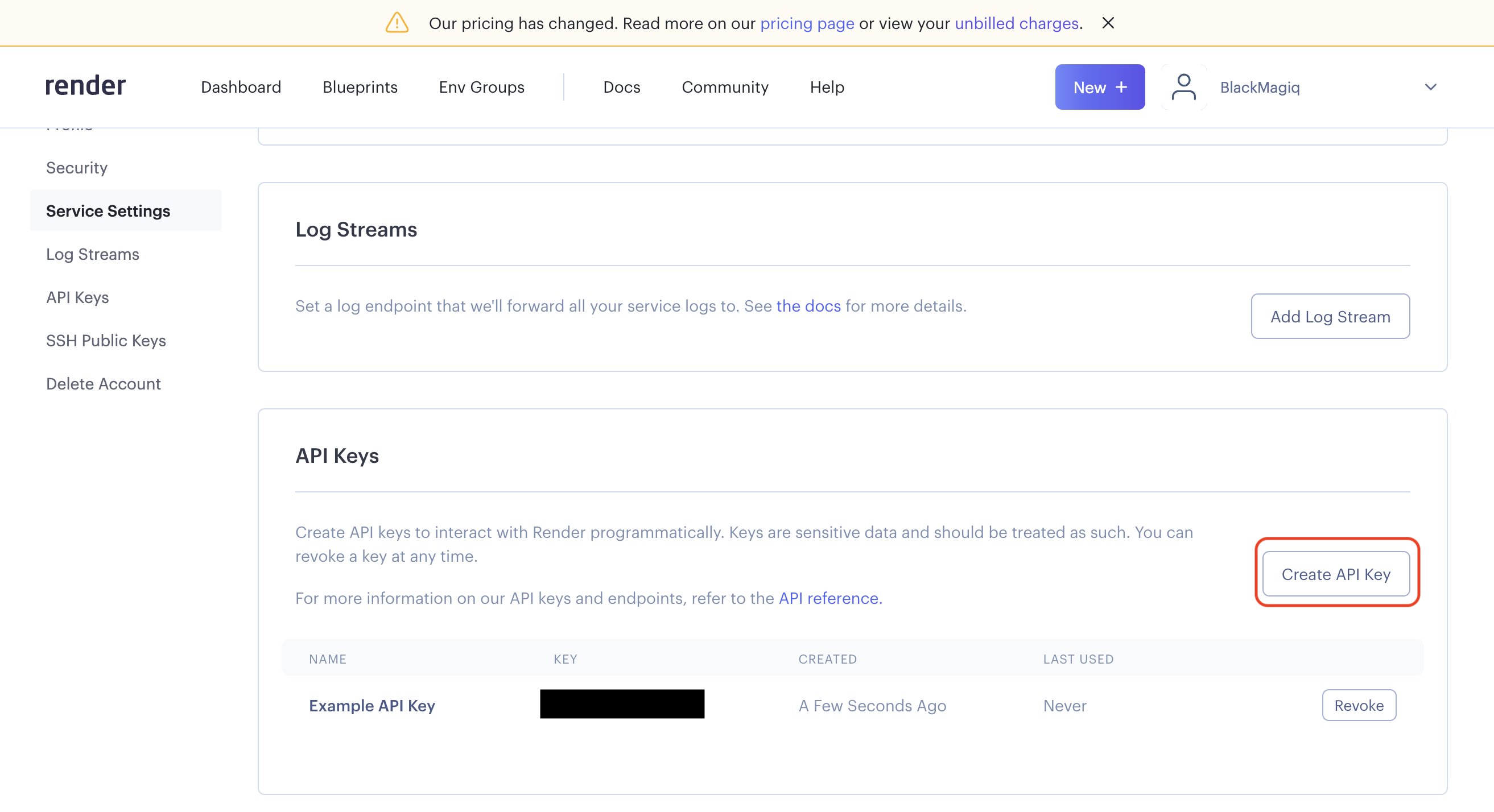
Navigate to your project's integrations tab in Infisical.
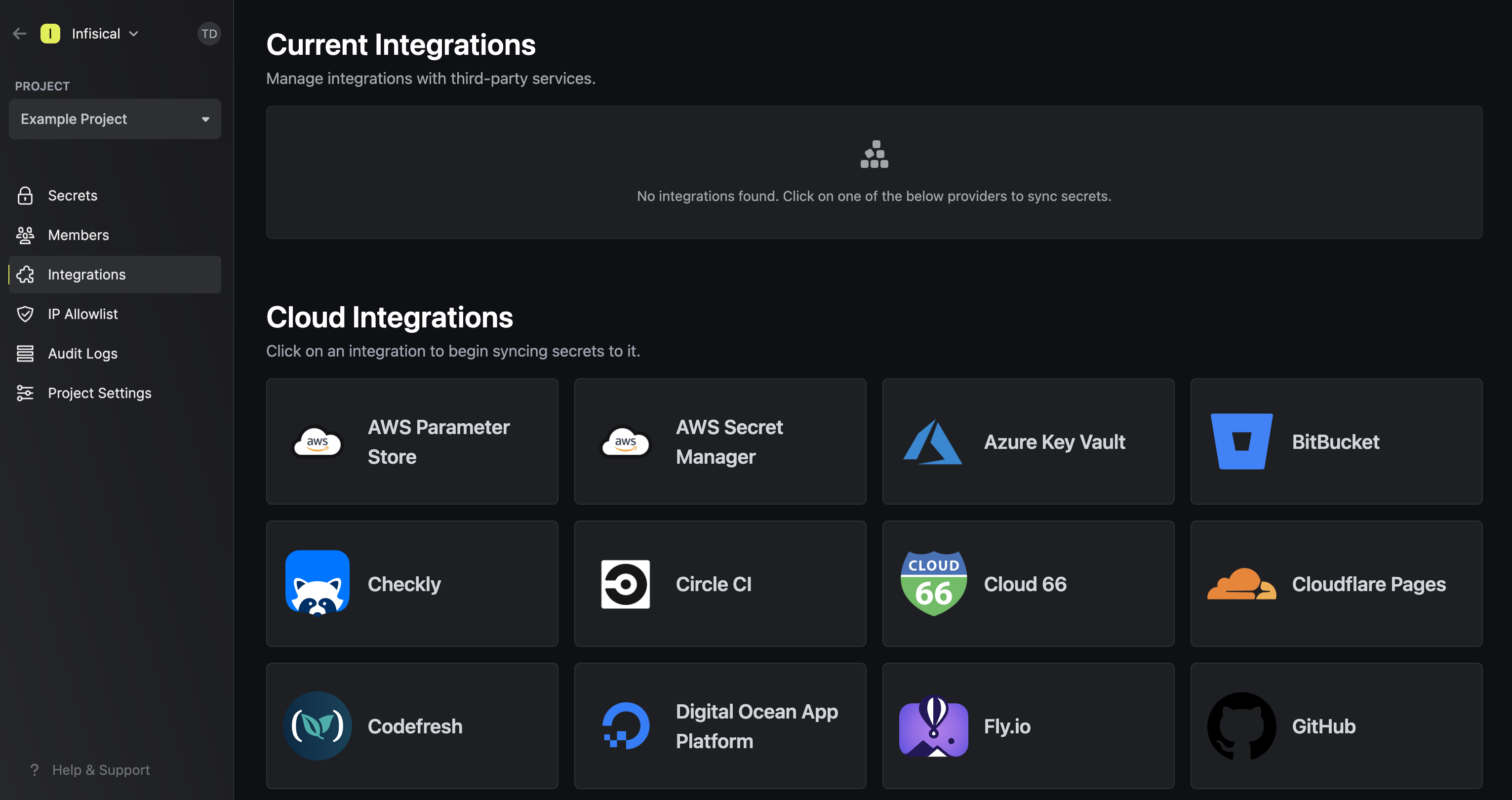
Press on the Render tile and input your Render API Key to grant Infisical access to your Render account.
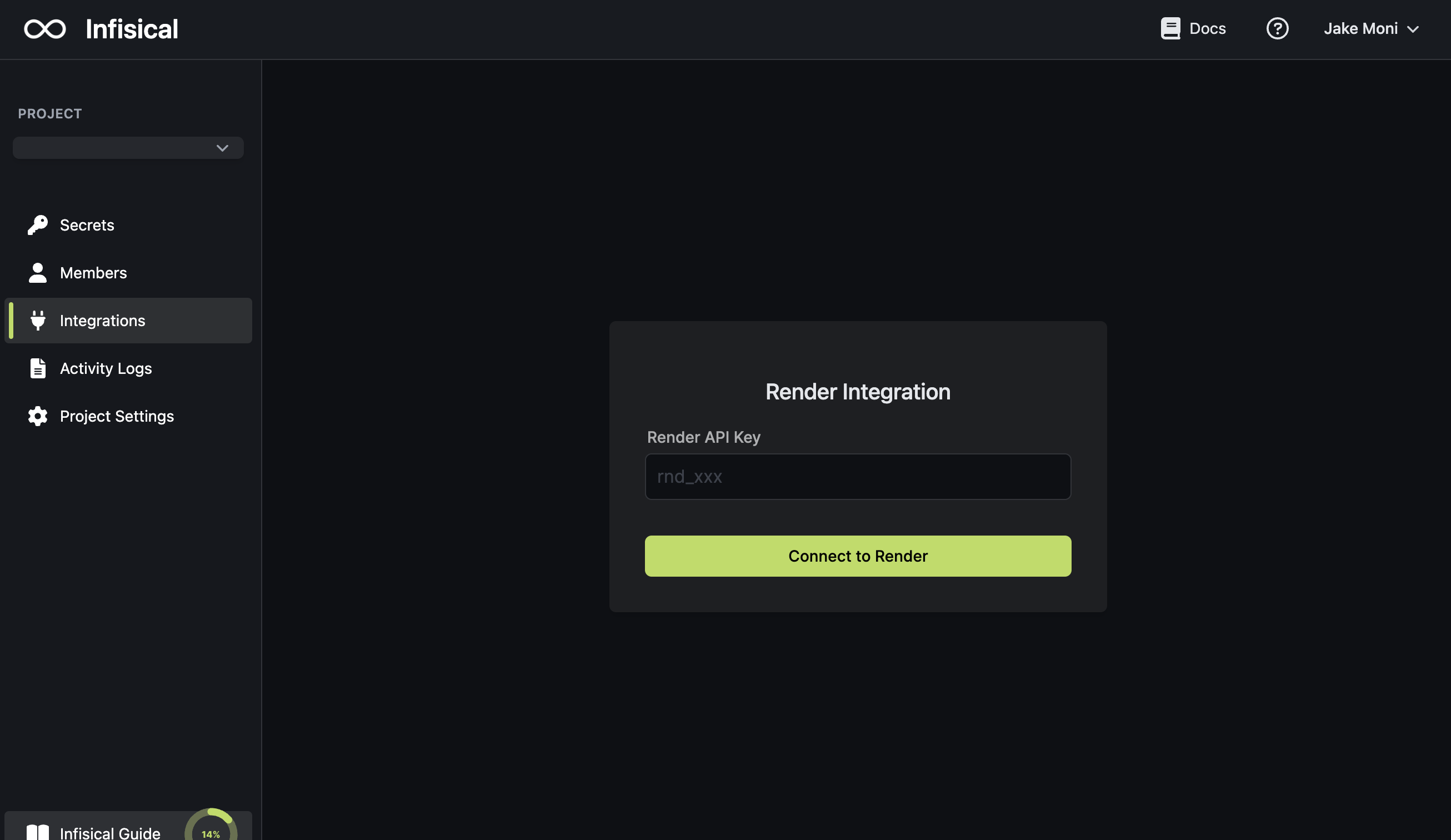
Select which Infisical environment secrets you want to sync to which Render service and press create integration to start syncing secrets to Render.
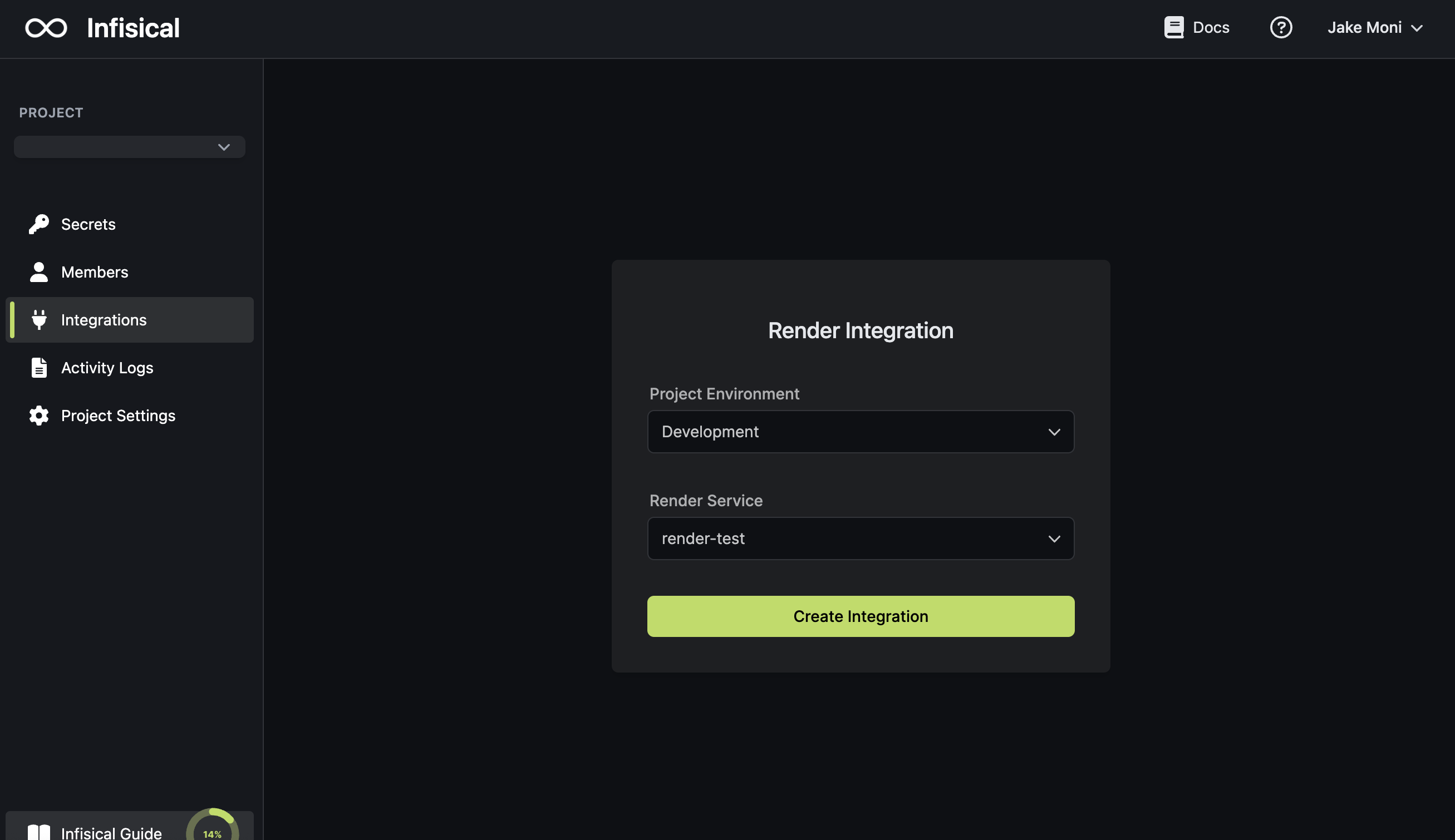
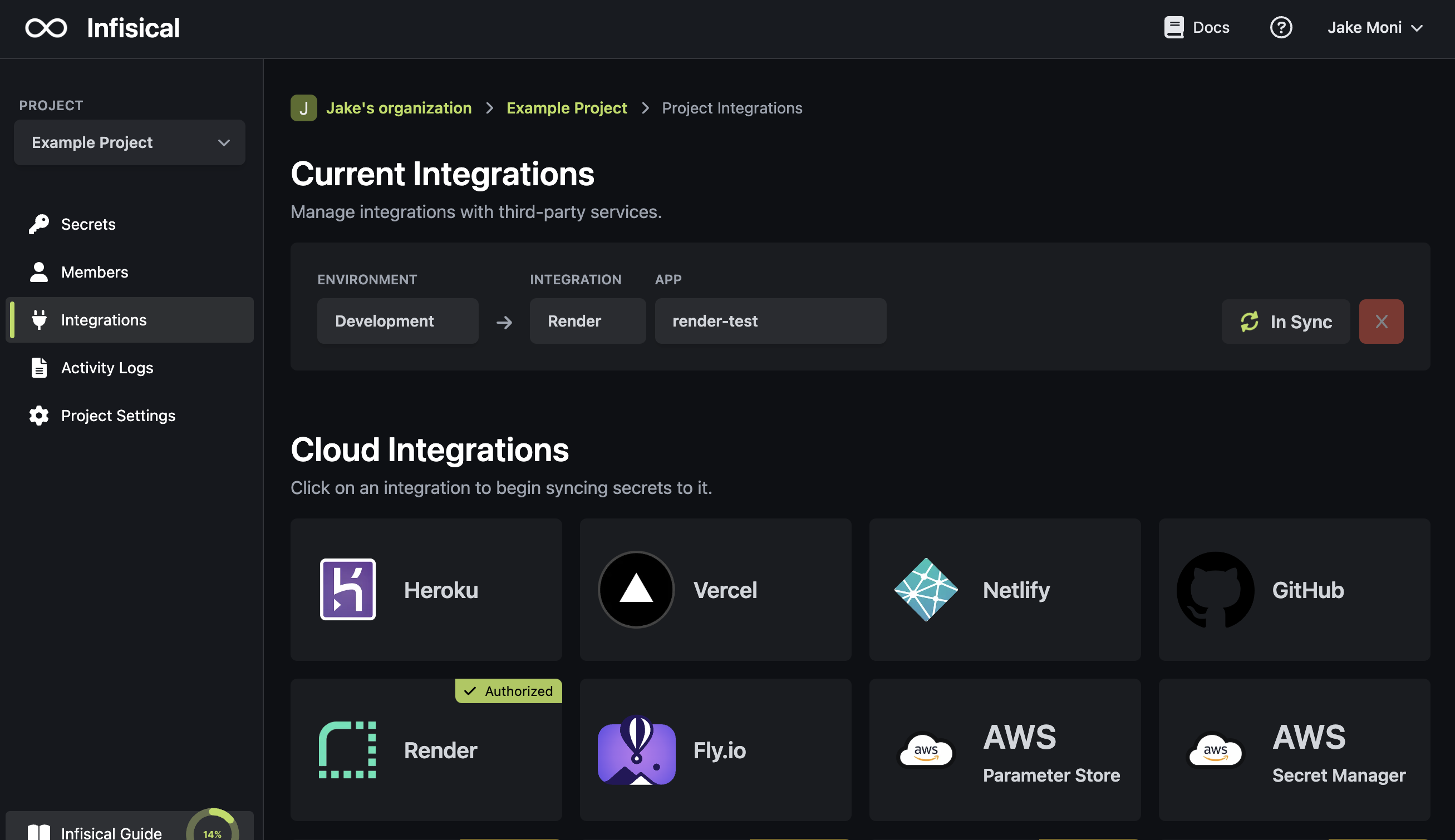
# Supabase
How to sync secrets from Infisical to Supabase
The Supabase integration is useful if your Supabase project uses sensitive-information such as [environment variables in edge functions](https://supabase.com/docs/guides/functions/secrets).
Synced envars can be accessed in edge functions using Deno's built-in handler: `Deno.env.get(MY_SECRET_NAME)`.
Prerequisites:
* Have an account and project set up at [Supabase](https://supabase.com/)
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
Obtain a Supabase Access Token in your Supabase [Account > Access Tokens](https://app.supabase.com/account/tokens).
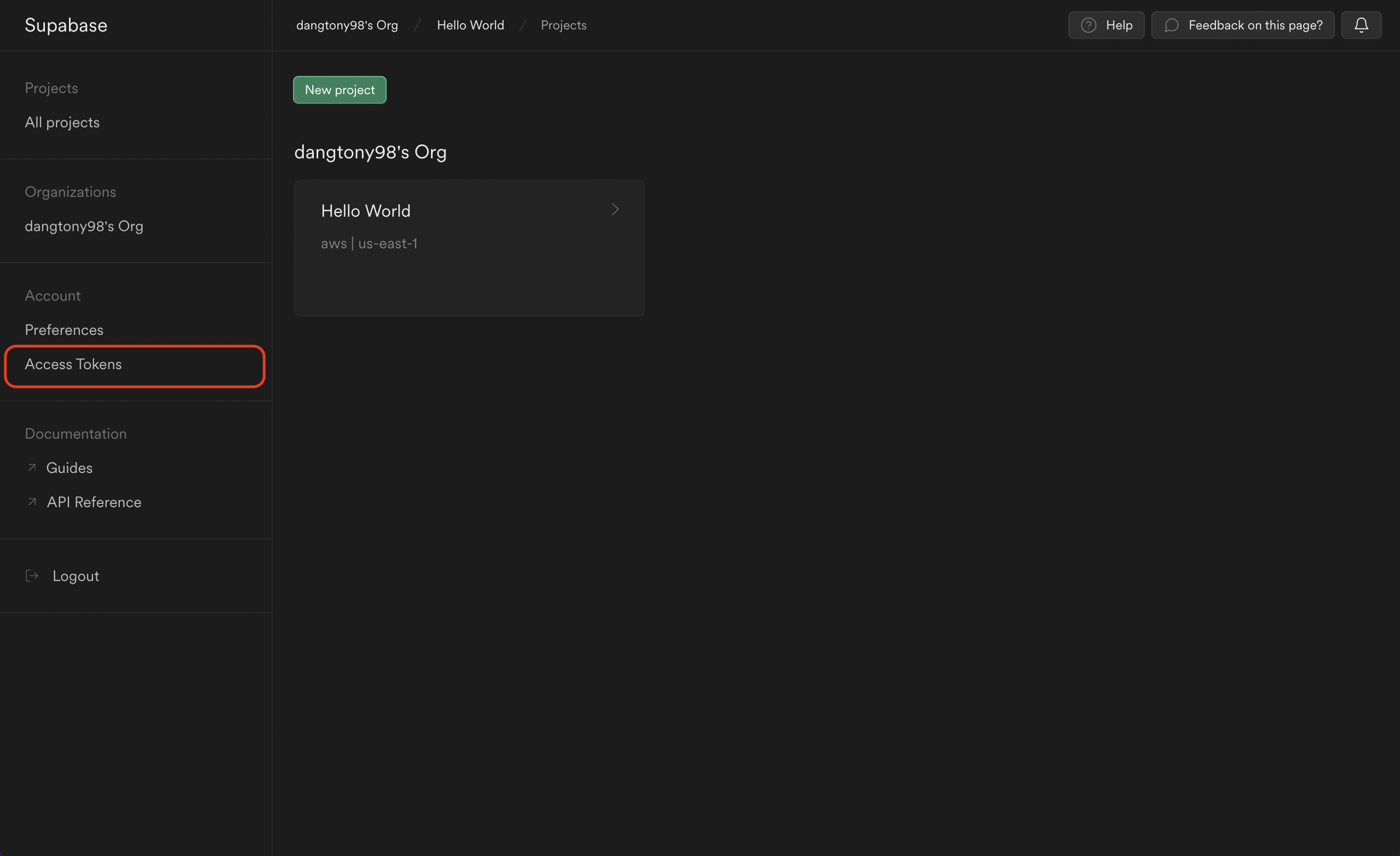
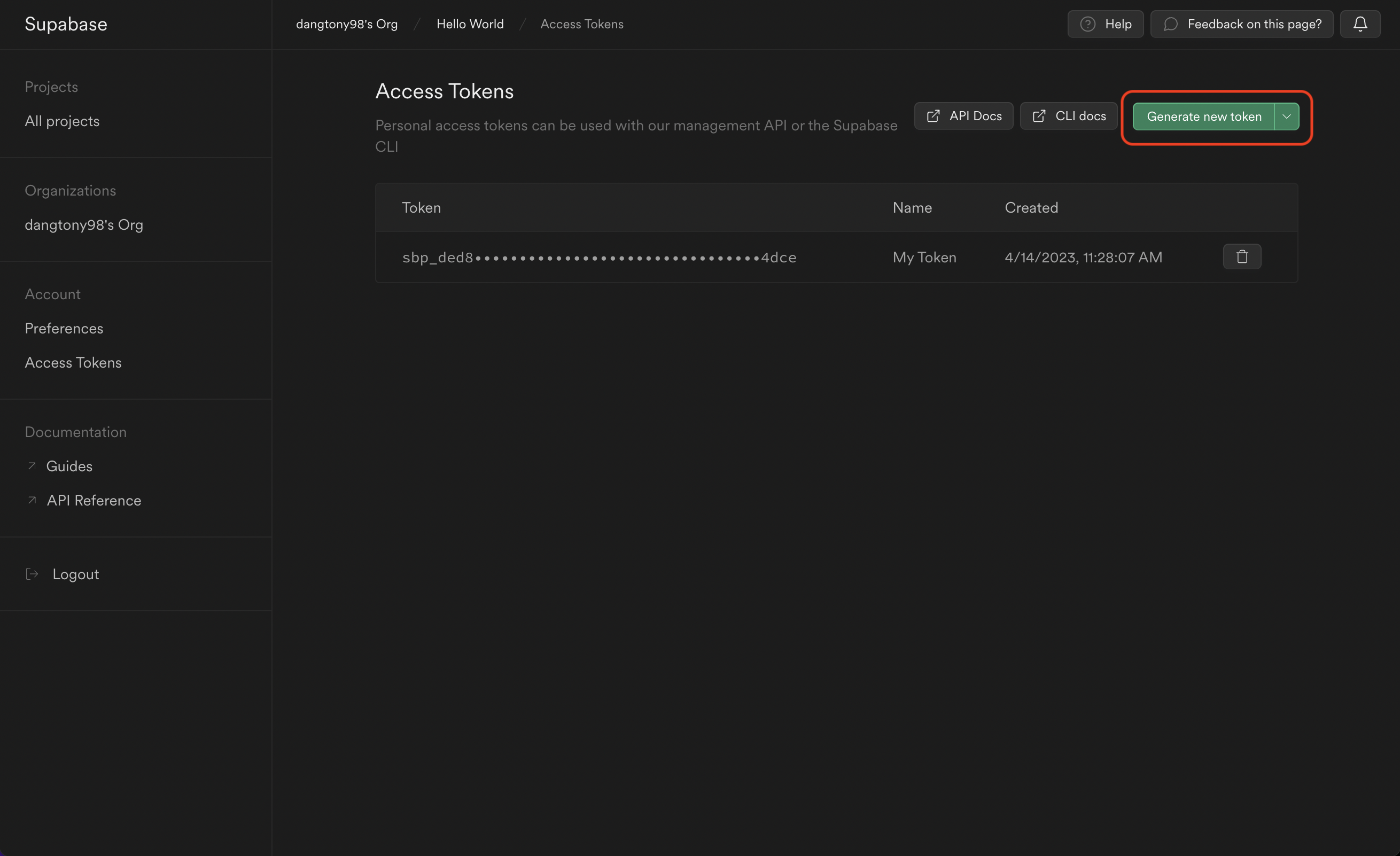
Navigate to your project's integrations tab in Infisical.
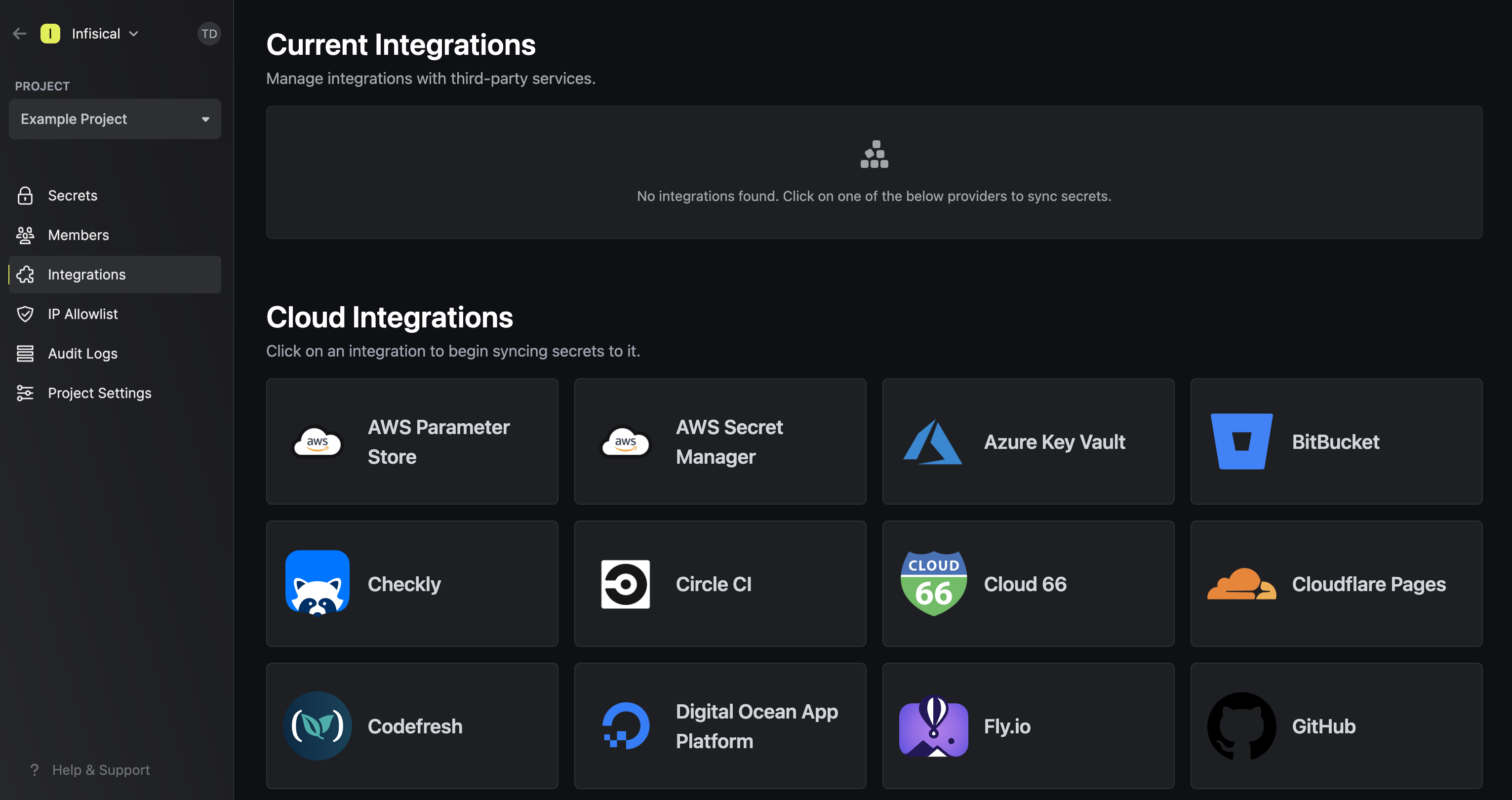
Press on the Supabase tile and input your Supabase Access Token to grant Infisical access to your Supabase account.
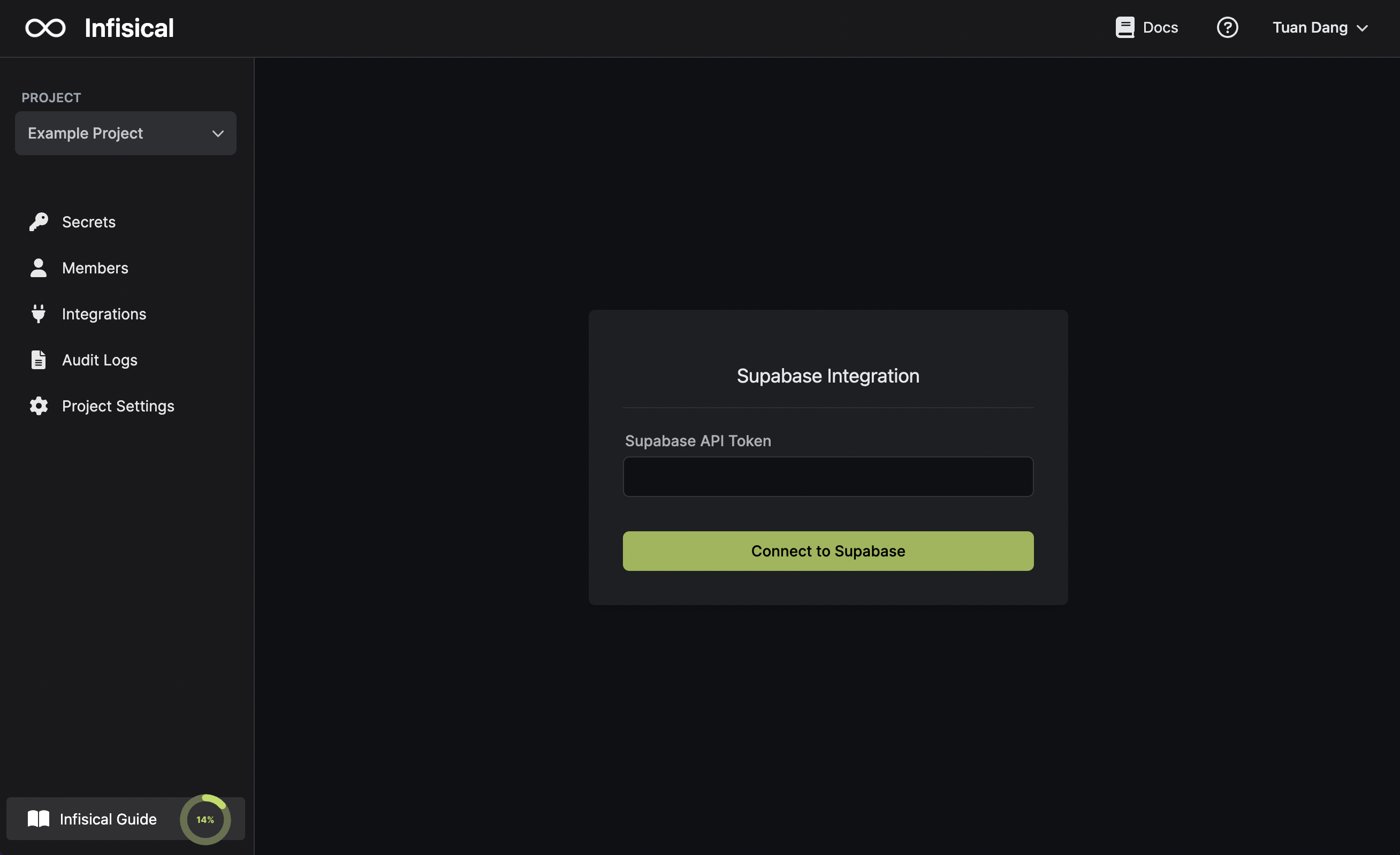
Select which Infisical environment secrets you want to sync to which Supabase project. Lastly, press create integration to start syncing secrets to Supabase.
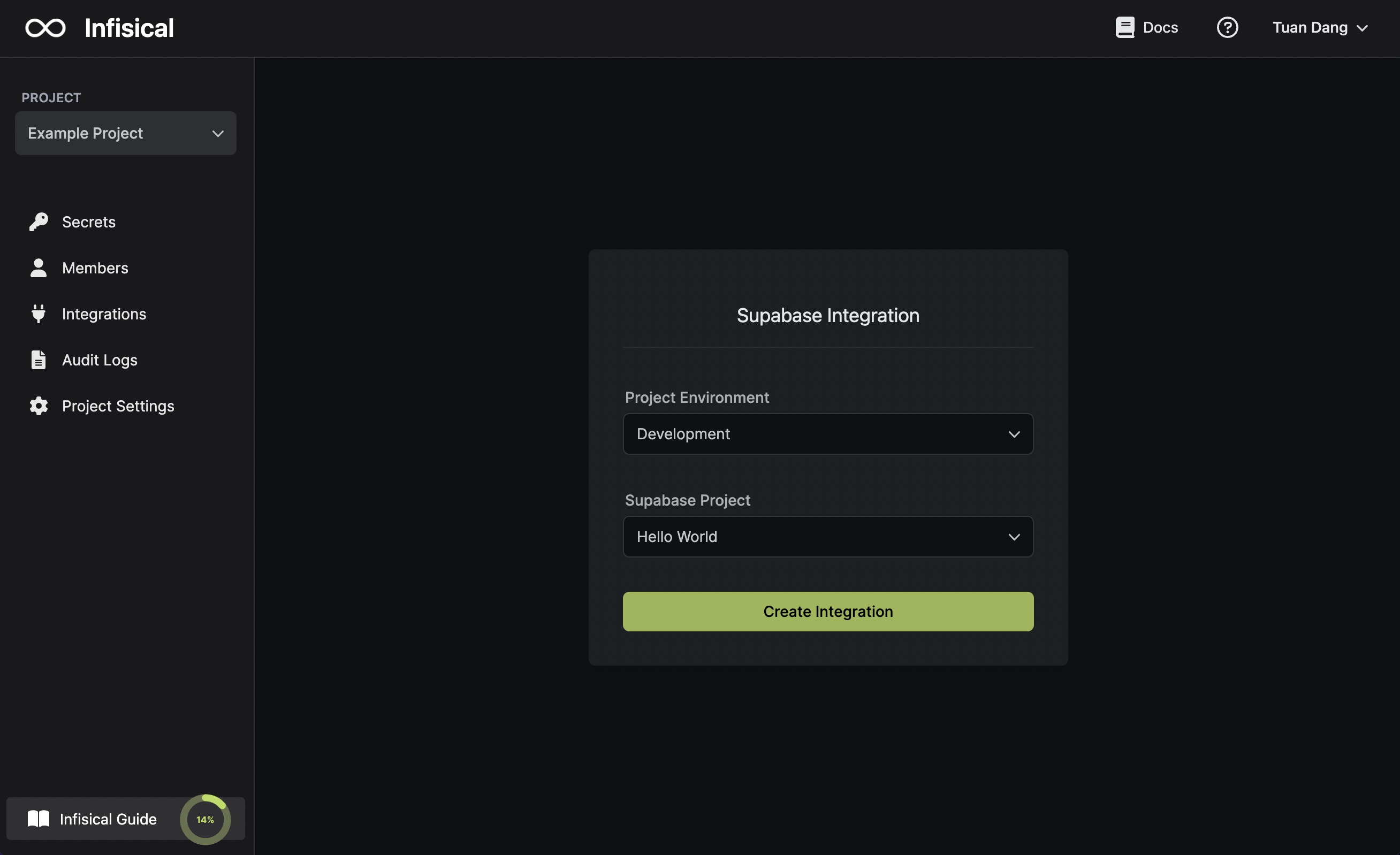
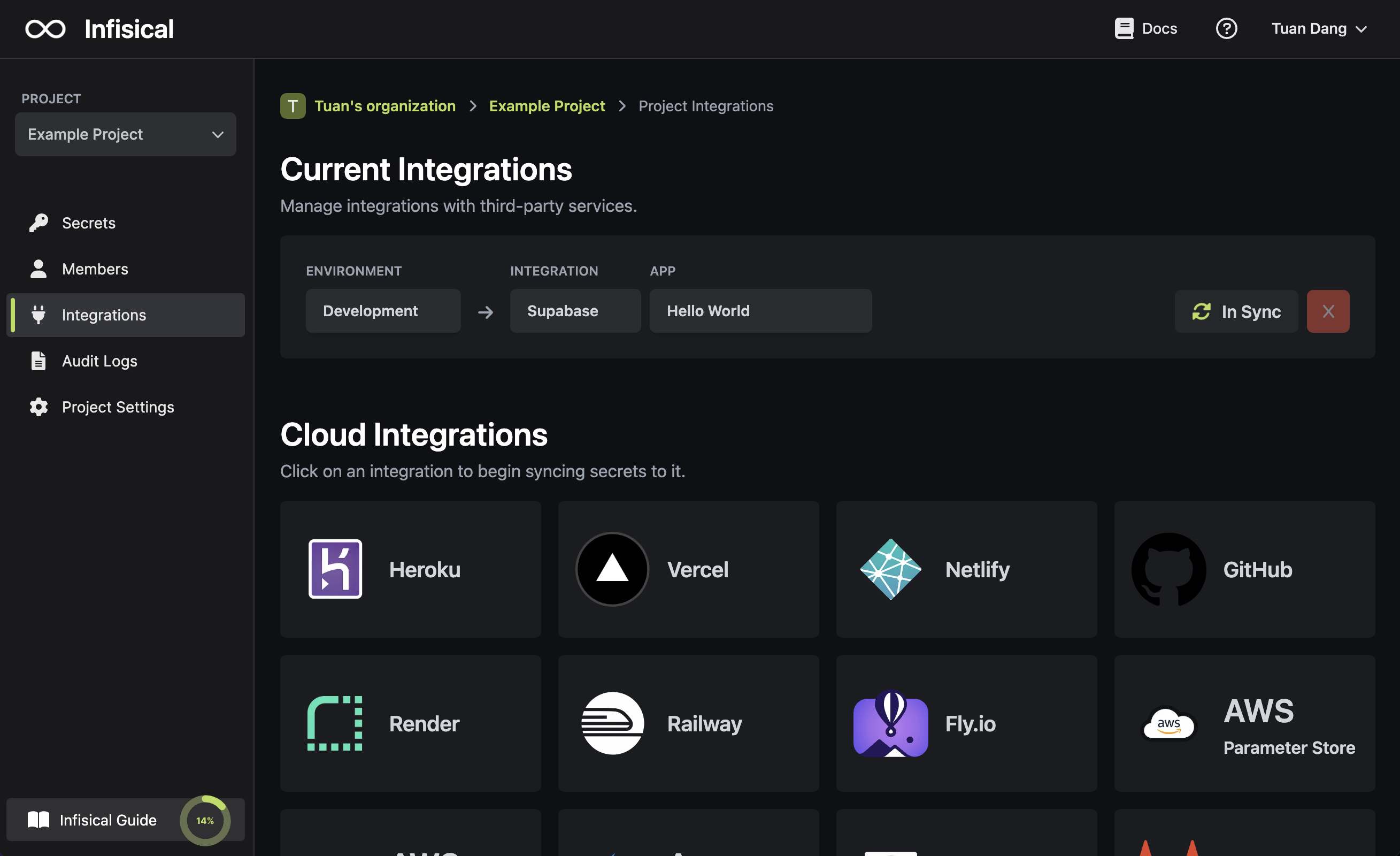
# TeamCity
How to sync secrets from Infisical to TeamCity
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
Obtain a TeamCity Access Token in Profile > Access Tokens
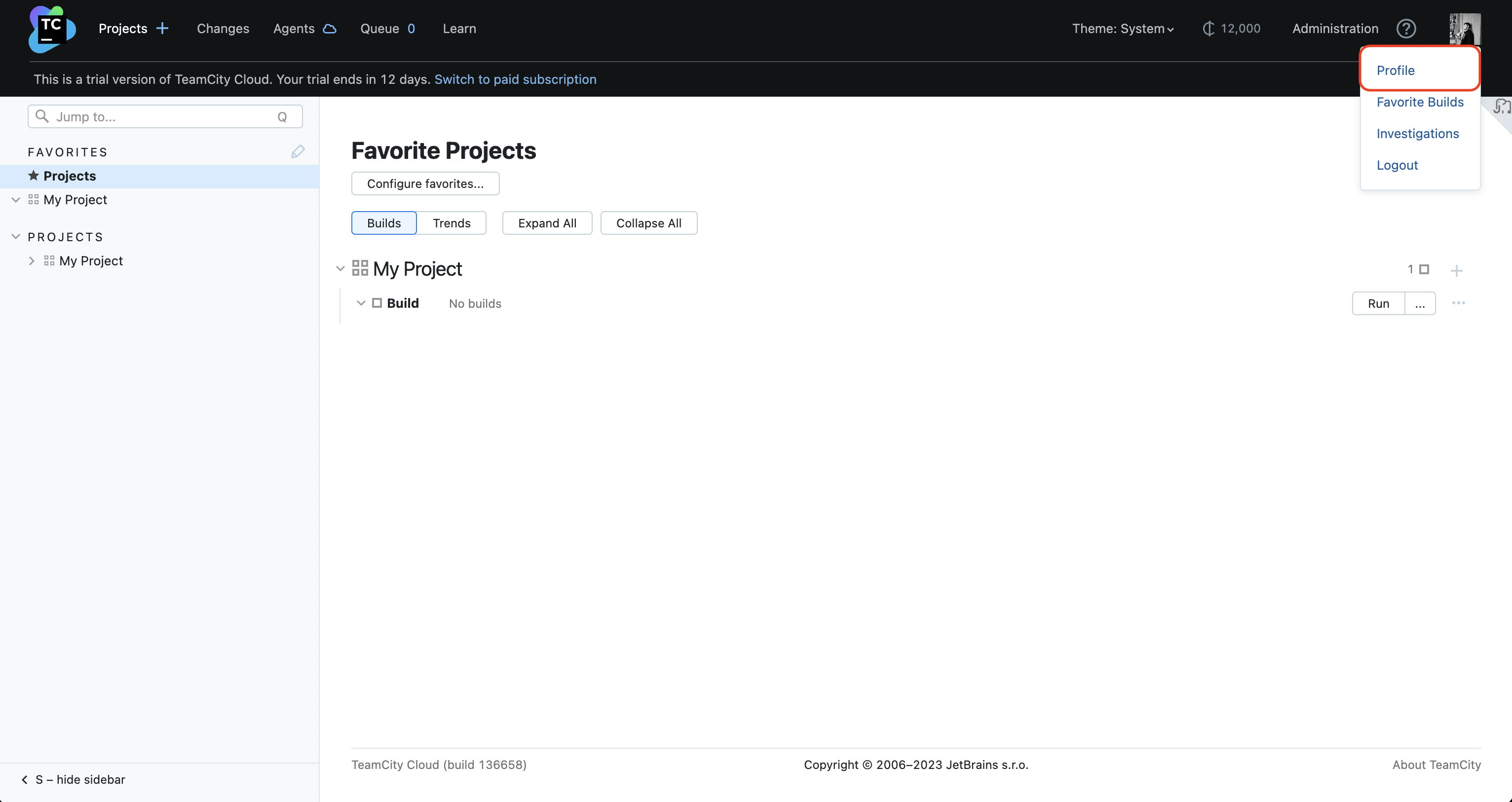
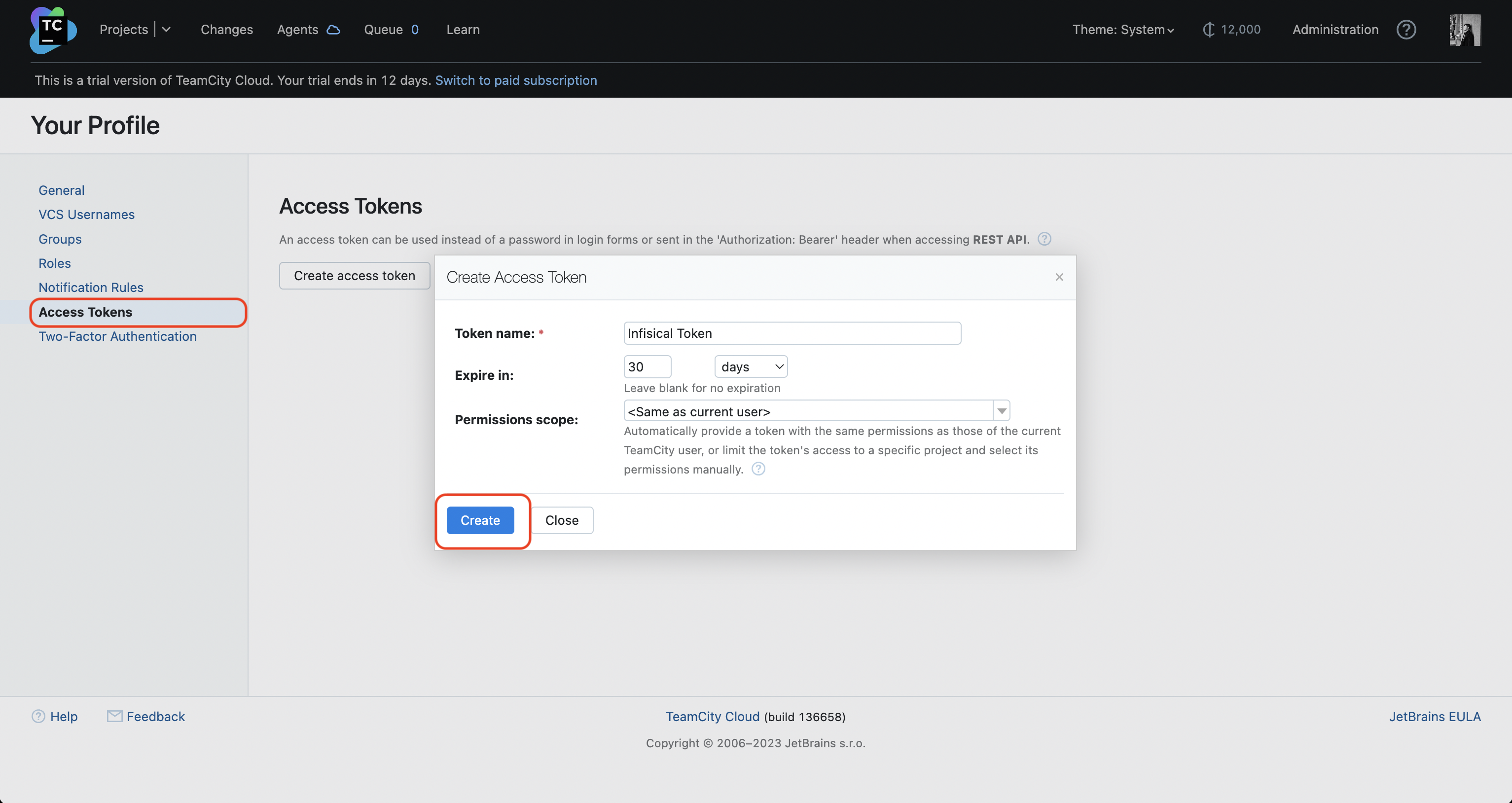
For this integration to work, the TeamCity Access Token must either have the
**Same as current user** account-wide permission enabled or, if **Limit per project**
is selected, then it must at minimum have the **View build configuration settings** and **Edit project** permissions enabled.
Navigate to your project's integrations tab in Infisical.
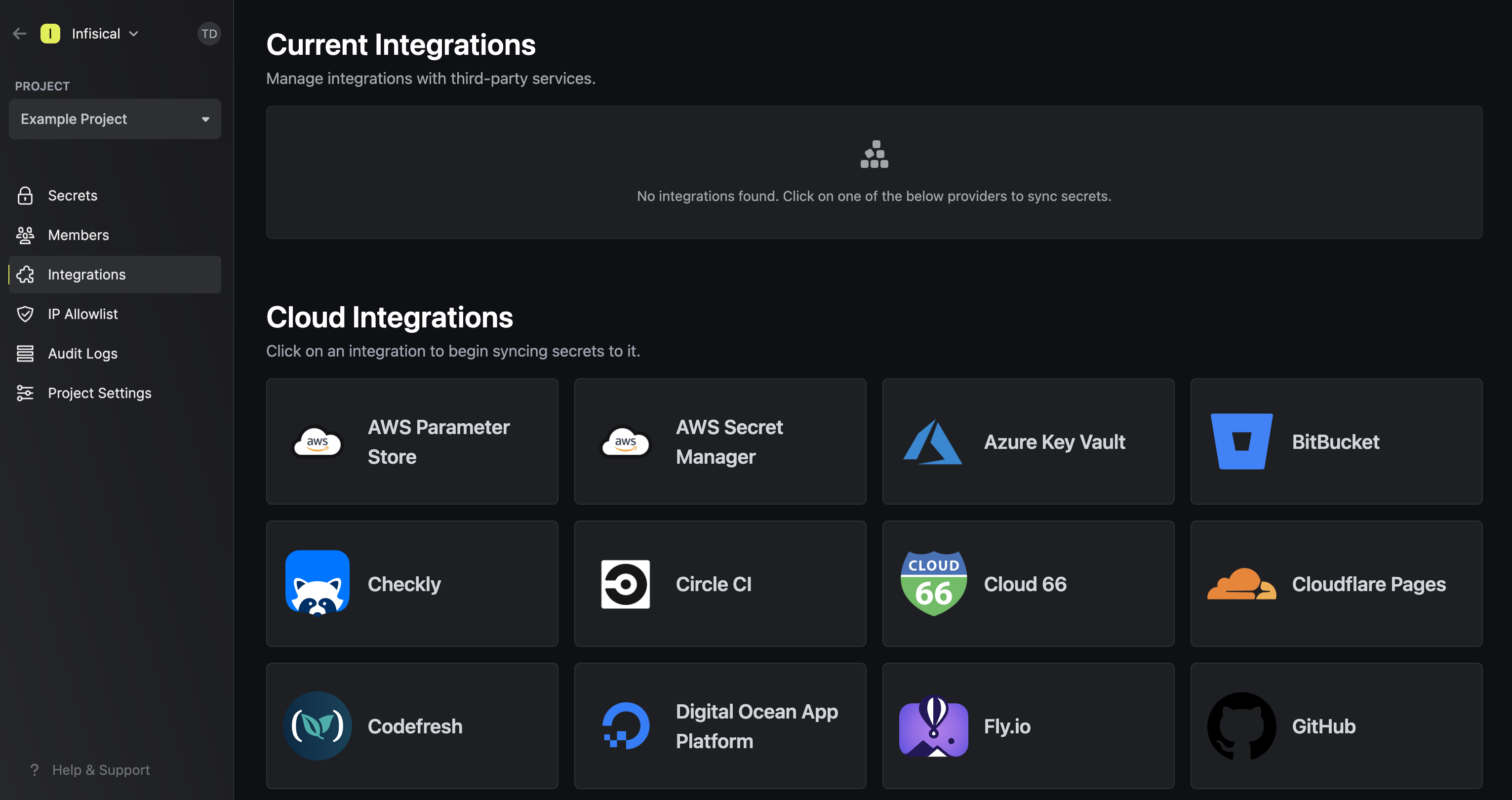
Press on the TeamCity tile and input your TeamCity Access Token and Server URL to grant Infisical access to your TeamCity account.
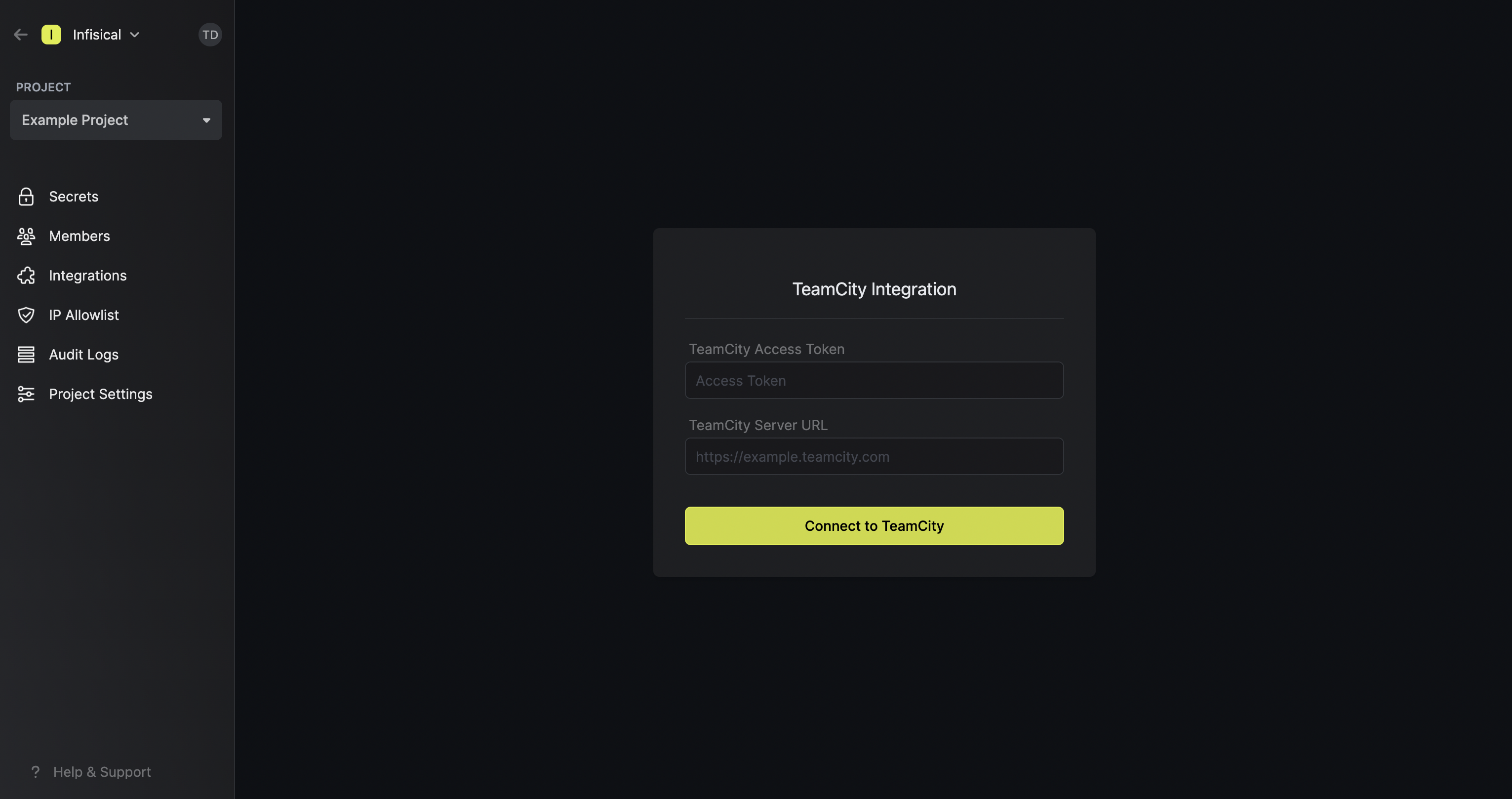
Select which Infisical environment secrets you want to sync to which TeamCity project (and optionally build configuration) and press create integration to start syncing secrets to TeamCity.
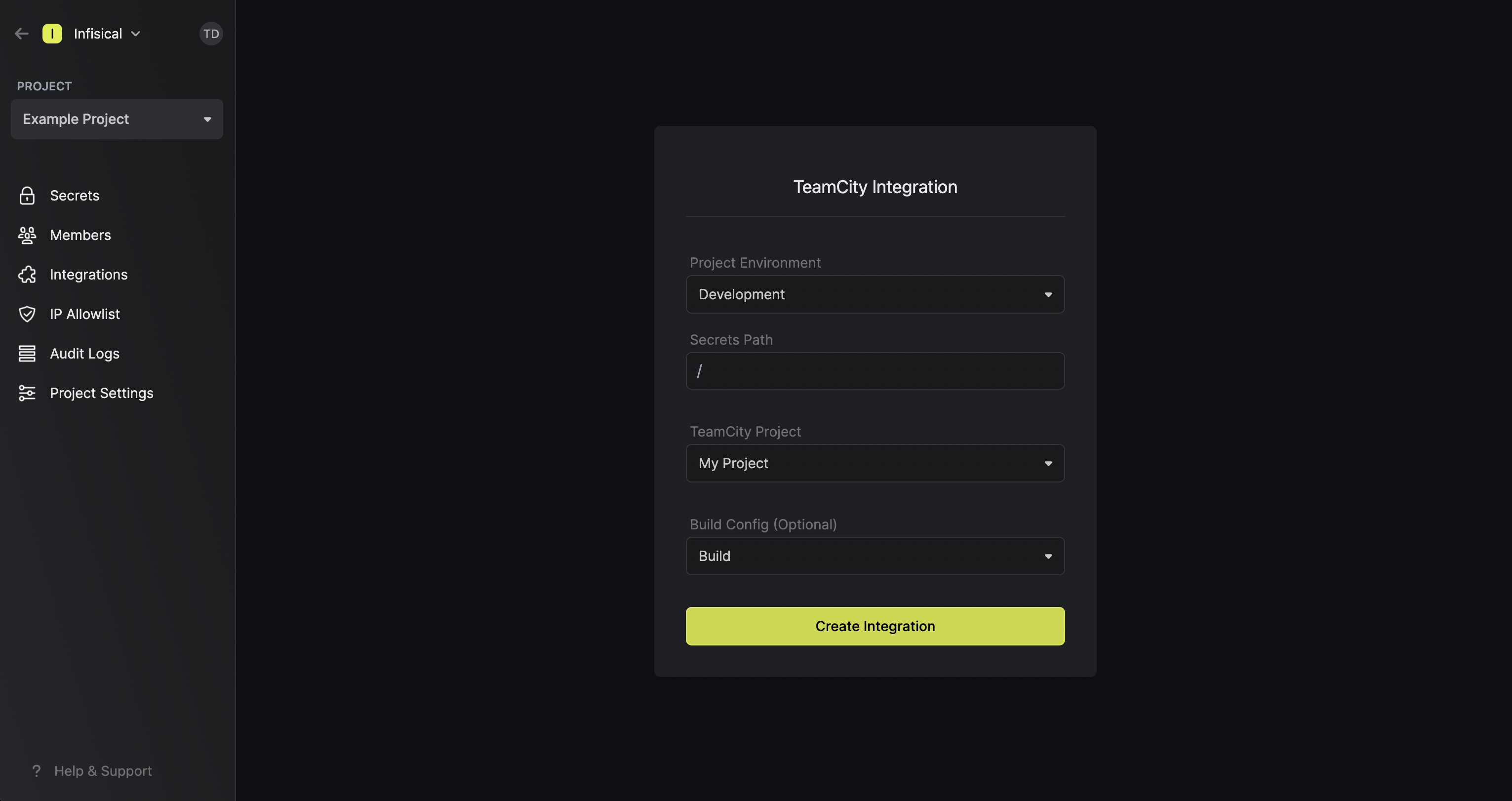
Infisical integrates with both TeamCity's project-level and build configuration-level environment variables.
To sync secrets to a specific build configuration in a TeamCity project, you can select a build configuration from the **TeamCity Build Config** dropdown; otherwise, leaving it empty will sync secrets to TeamCity at the project-level.
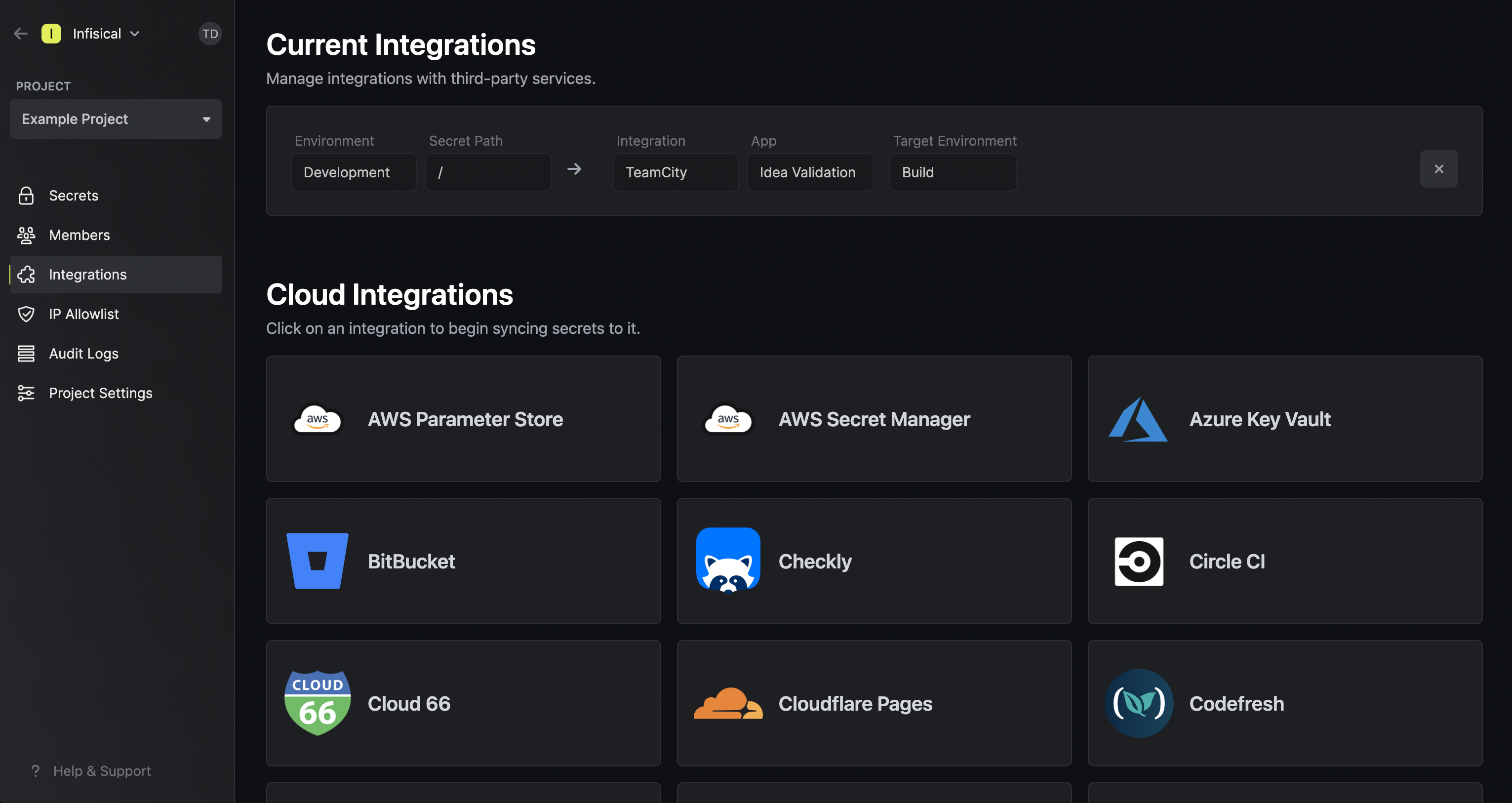
# Terraform Cloud
How to sync secrets from Infisical to Terraform Cloud
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
Obtain a Terraform Cloud API Token in User Settings > Tokens
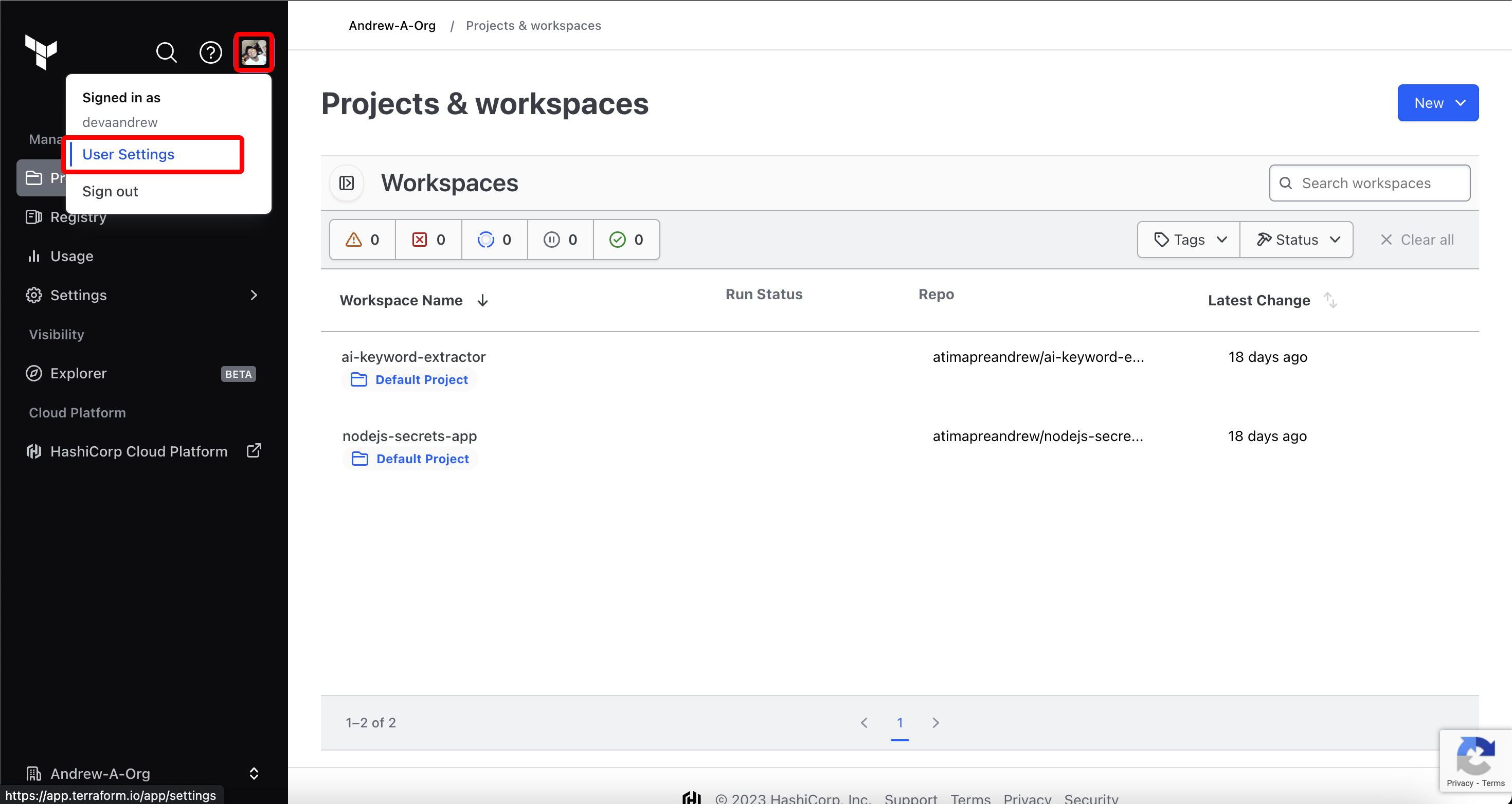
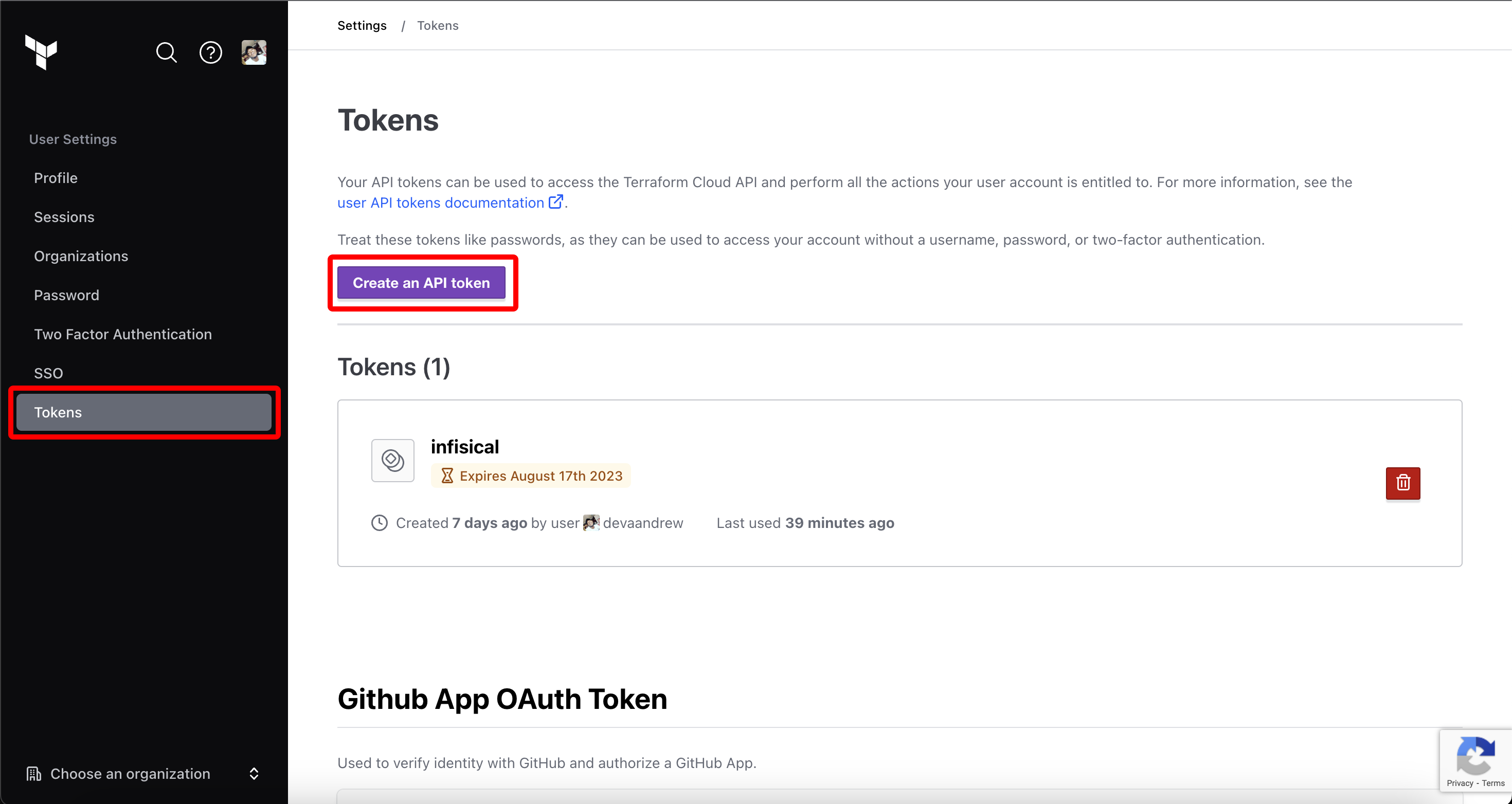
Obtain your Terraform Cloud Workspace Id in Projects & Workspaces > Workspace > ID
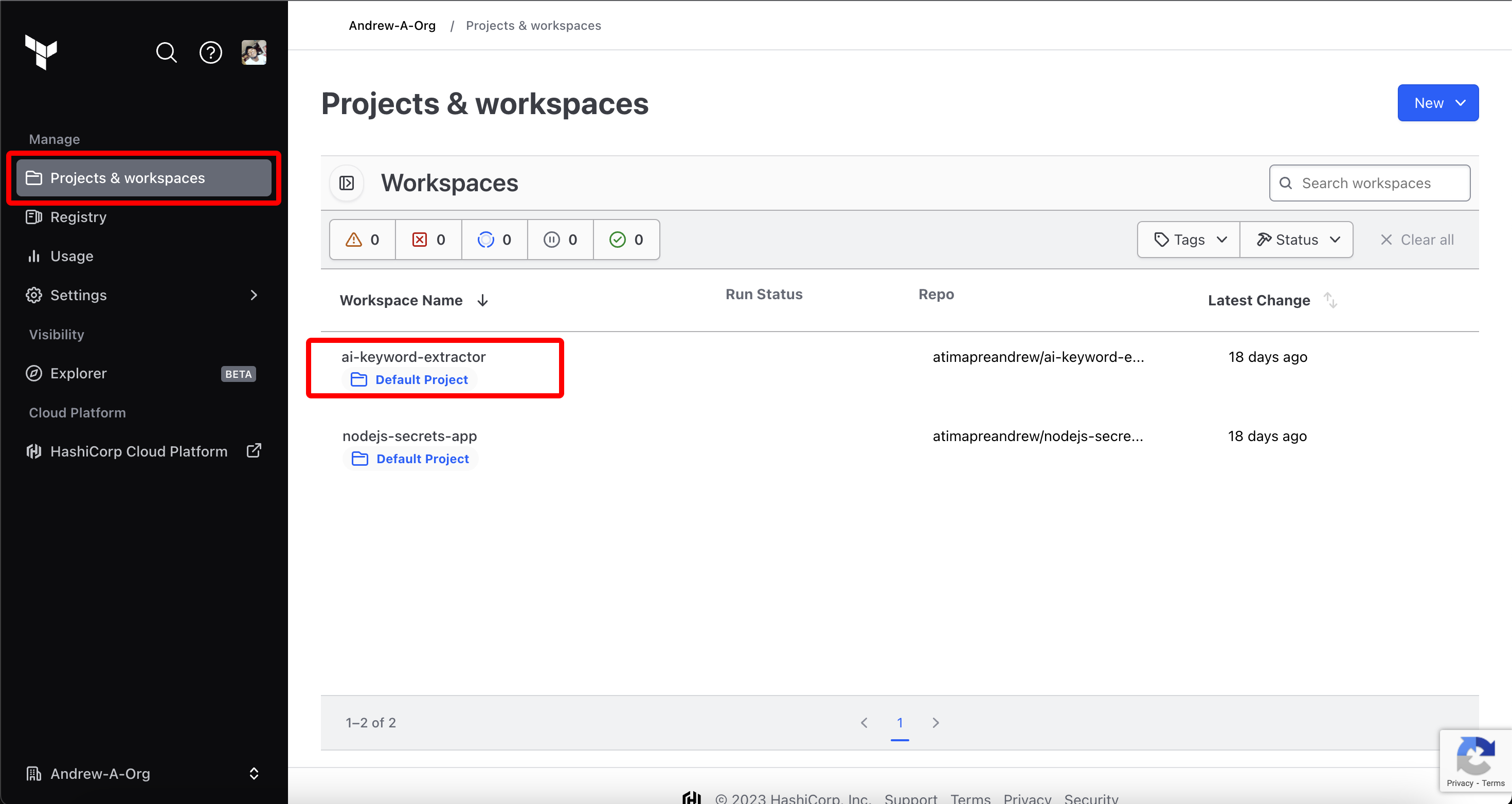
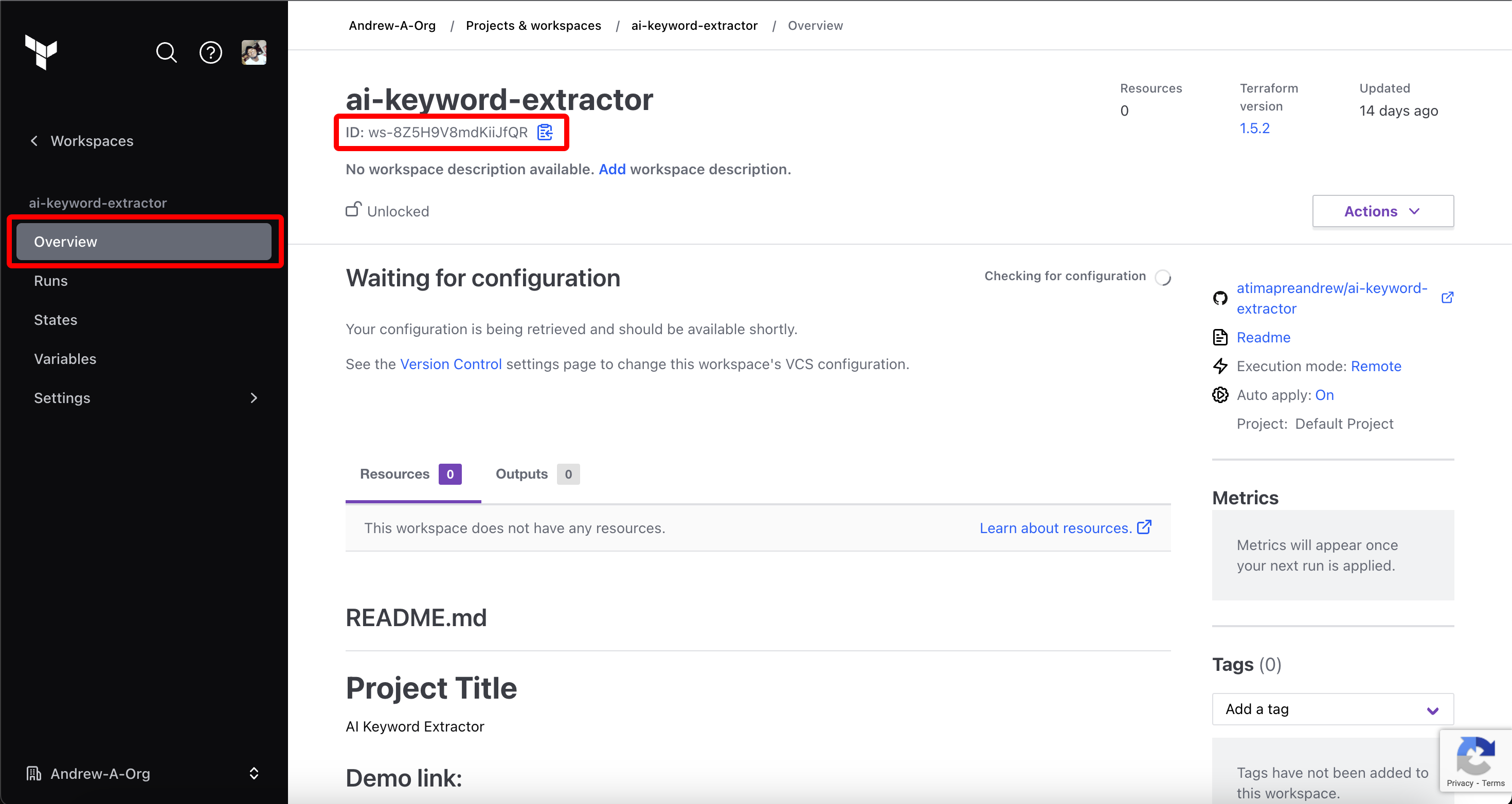
Navigate to your project's integrations tab in Infisical.
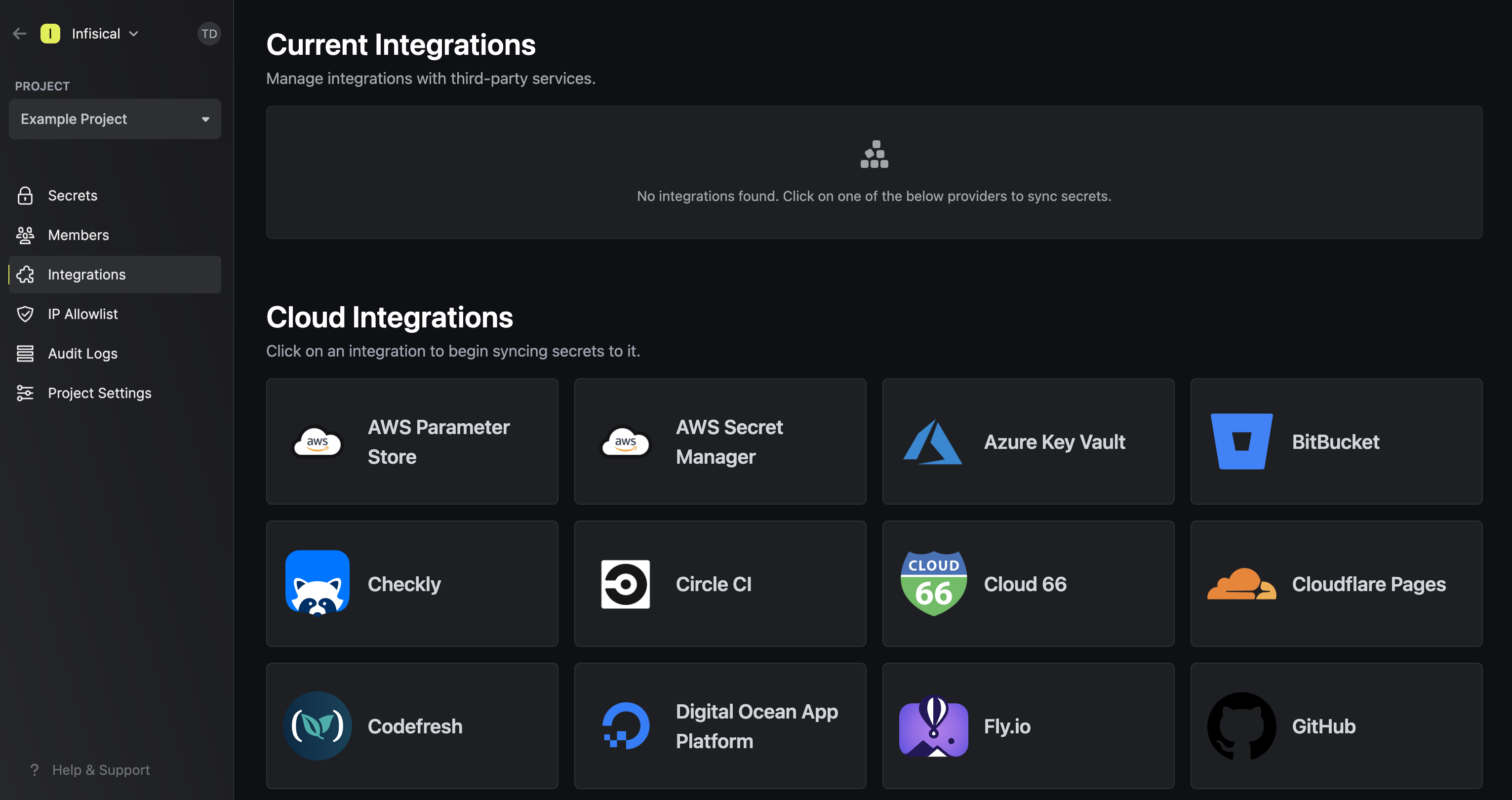
Press on the Terraform Cloud tile and input your Terraform Cloud API Token and Workspace Id to grant Infisical access to your Terraform Cloud account.
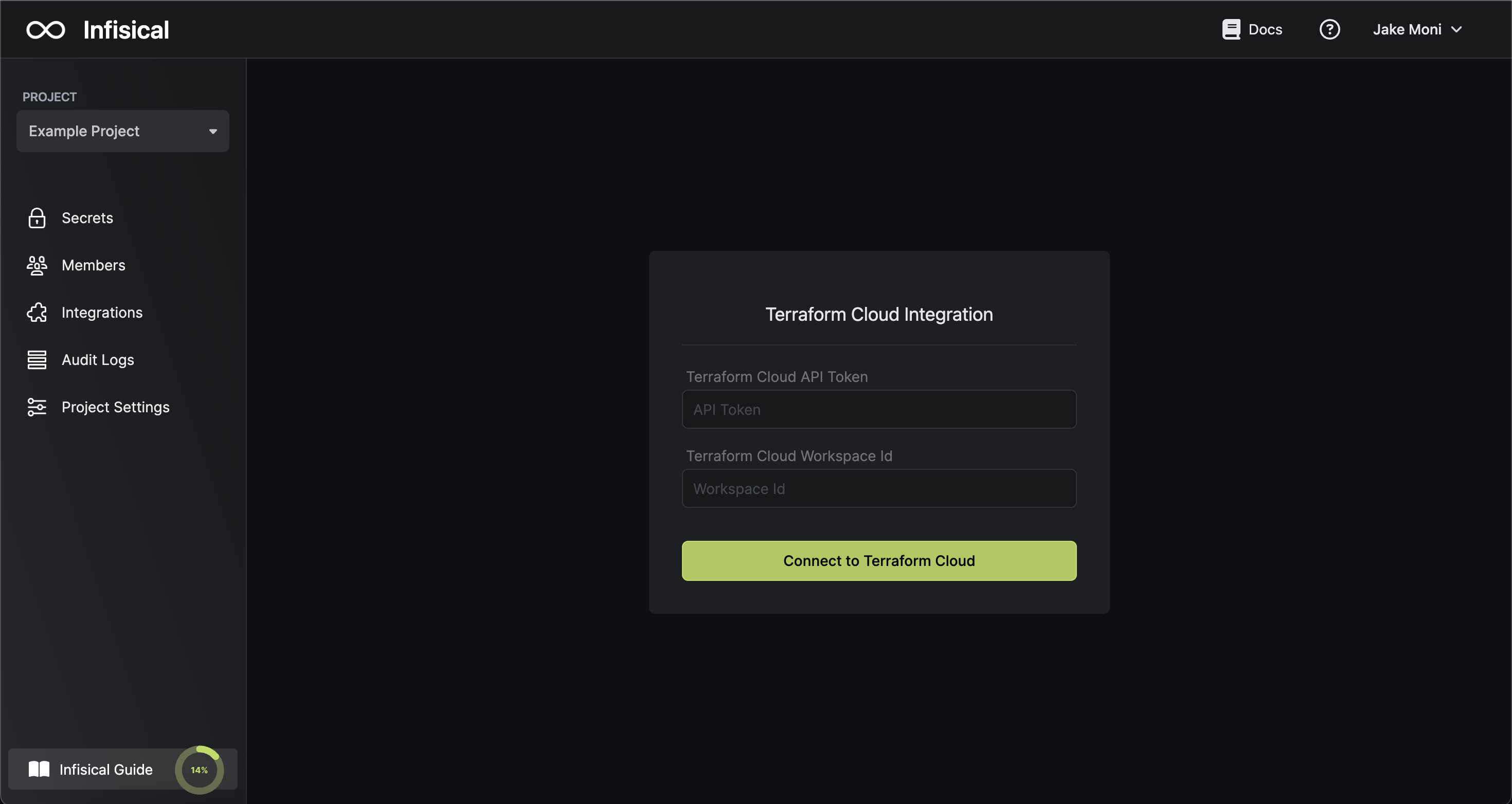
Select which Infisical environment secrets and Terraform Cloud variable type you want to sync to which Terraform Cloud workspace/project and press create integration to start syncing secrets to Terraform Cloud.
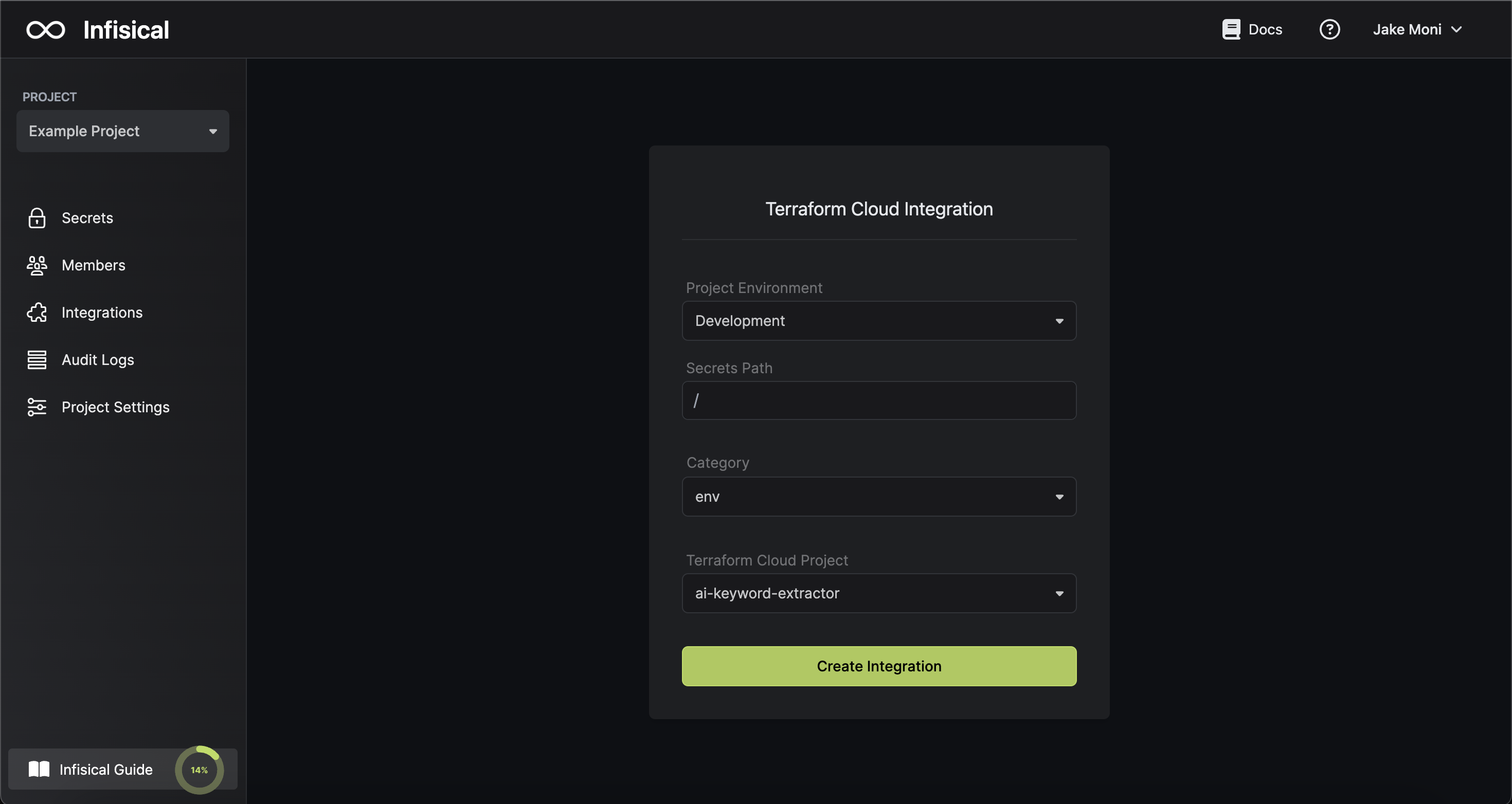
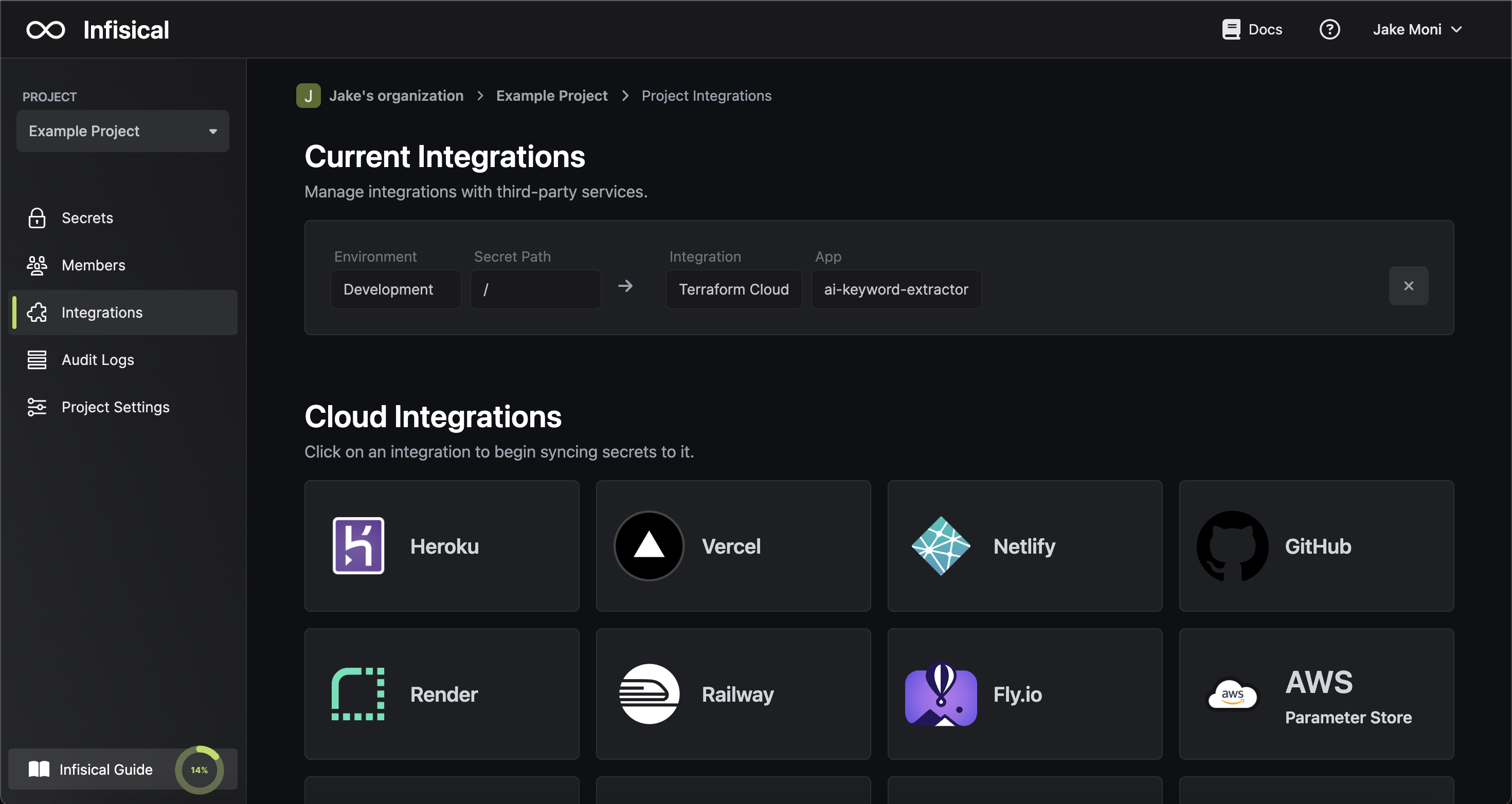
# Vercel
How to sync secrets from Infisical to Vercel
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
Navigate to your project's integrations tab in Infisical.
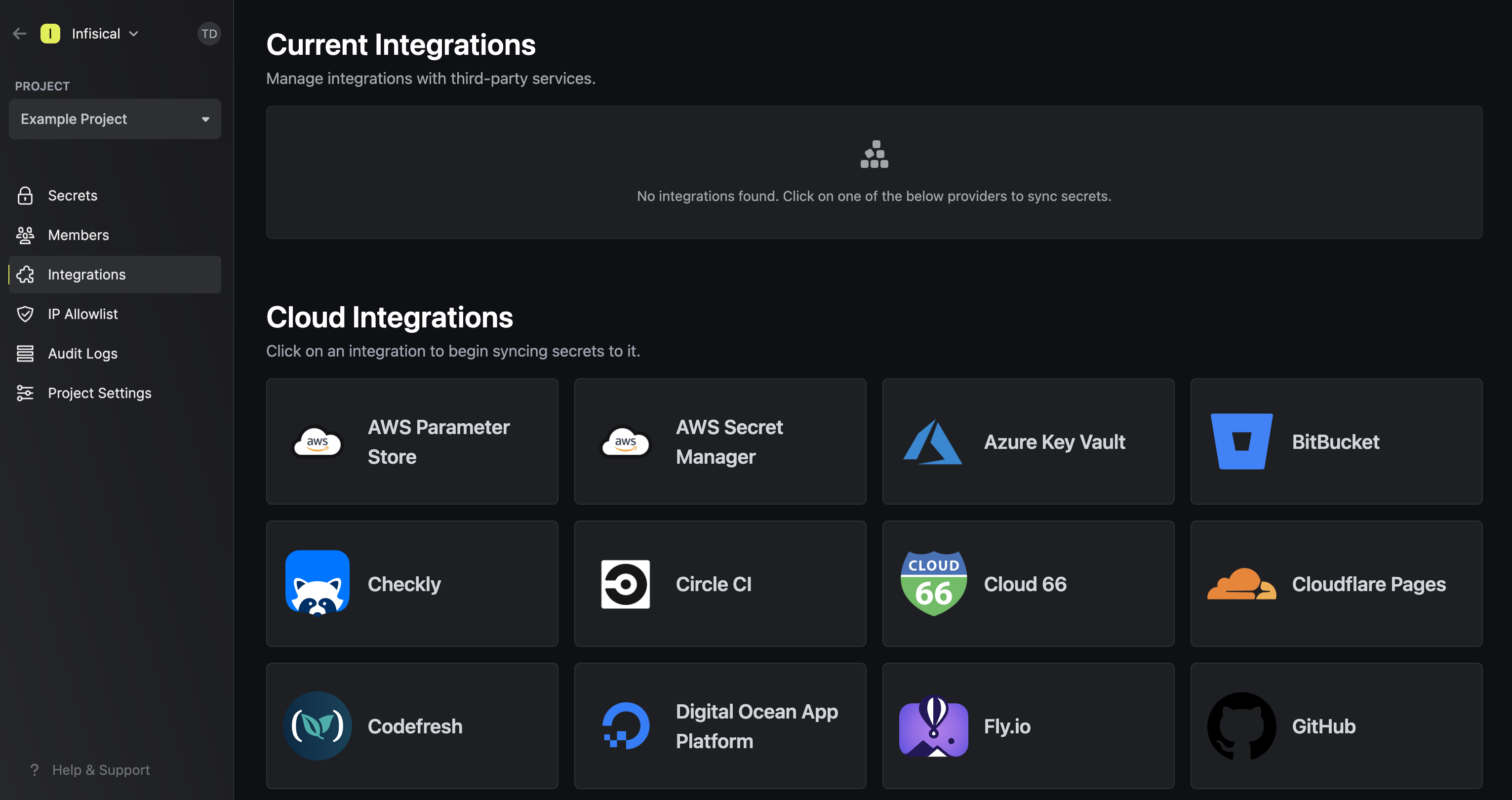
Press on the Vercel tile and grant Infisical access to your Vercel account.
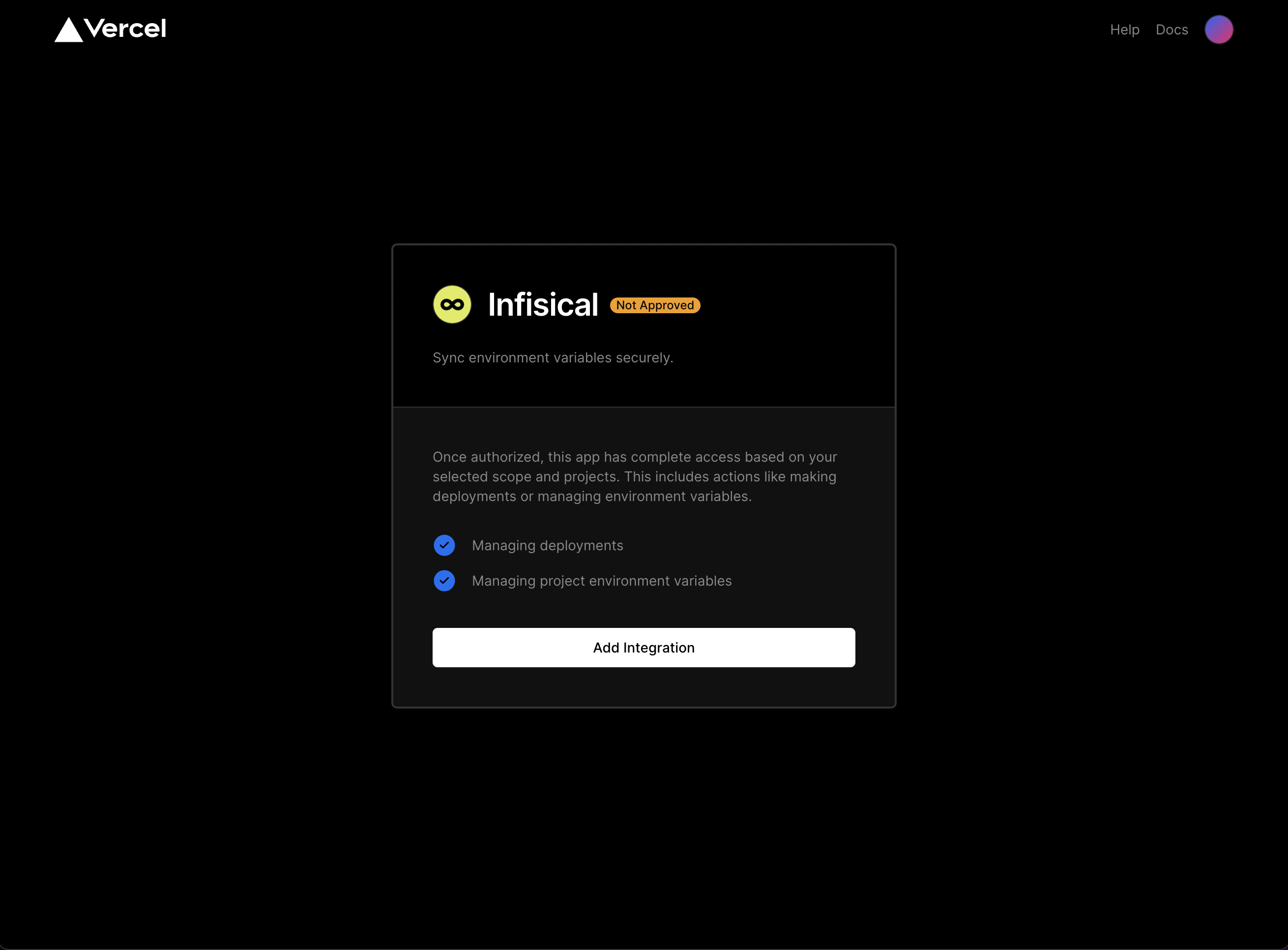
Select which Infisical environment secrets you want to sync to which Vercel app and environment. Lastly, press create integration to start syncing secrets to Vercel.
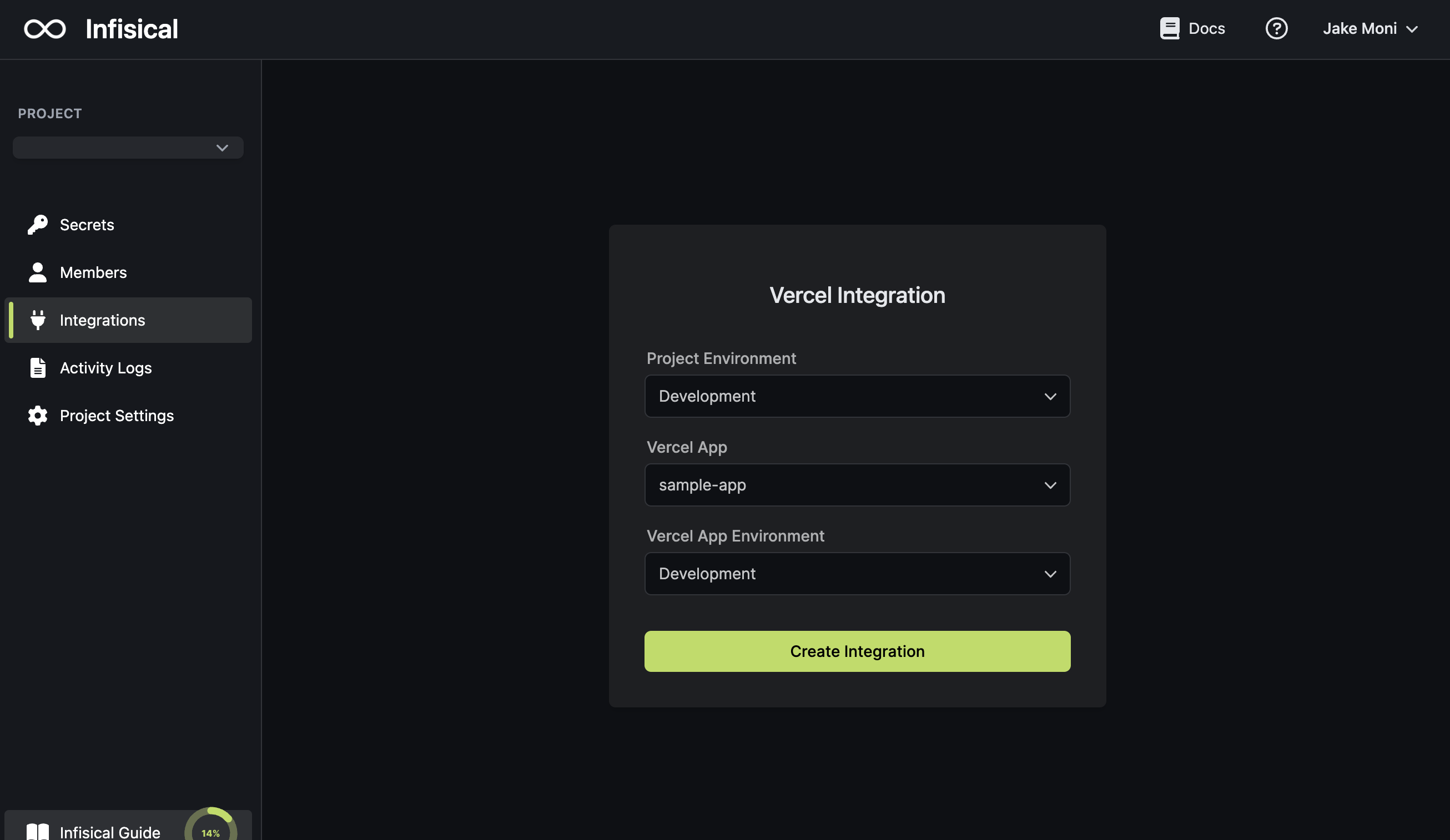
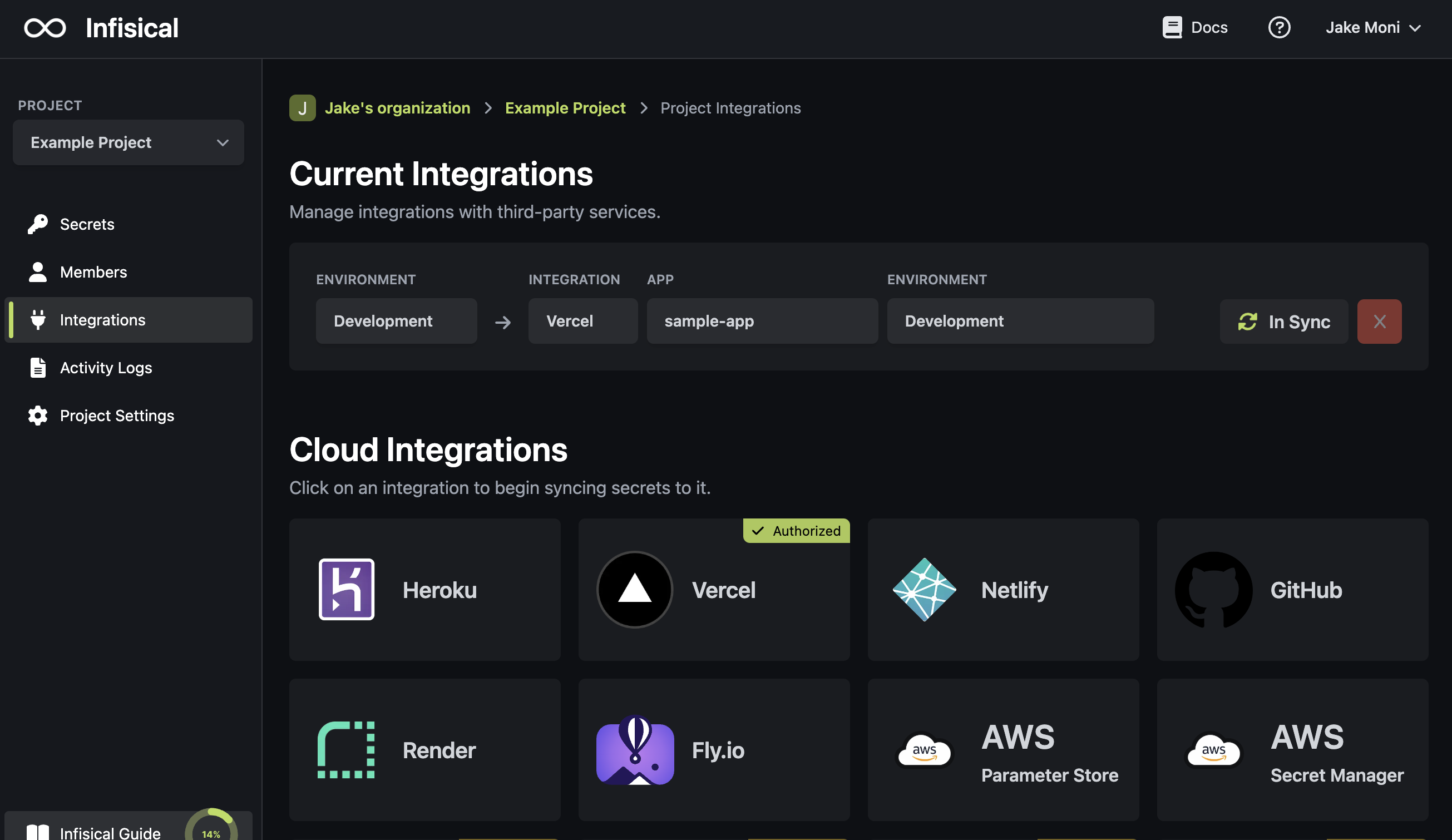
Infisical syncs every envar to Vercel with type `encrypted` unless an existing
envar with the same name in Vercel exists with a different type. Note that
Infisical will not be able to update Vercel envars with type `sensitive` since
they can only be decrypted and modified by Vercel's deployment systems.
The following environment variable names are reserved by Vercel and cannot be
synced: `AWS_SECRET_KEY`, `AWS_EXECUTION_ENV`, `AWS_LAMBDA_LOG_GROUP_NAME`,
`AWS_LAMBDA_LOG_STREAM_NAME`, `AWS_LAMBDA_FUNCTION_NAME`,
`AWS_LAMBDA_FUNCTION_MEMORY_SIZE`, `AWS_LAMBDA_FUNCTION_VERSION`,
`NOW_REGION`, `TZ`, `LAMBDA_TASK_ROOT`, `LAMBDA_RUNTIME_DIR`,
`AWS_ACCESS_KEY_ID`, `AWS_SECRET_ACCESS_KEY`, `AWS_SESSION_TOKEN`,
`AWS_REGION`, and `AWS_DEFAULT_REGION`.
Using the Vercel integration on a self-hosted instance of Infisical requires configuring an integration in Vercel.
and registering your instance with it.
Navigate to Integrations > Integration Console to create a new integration.
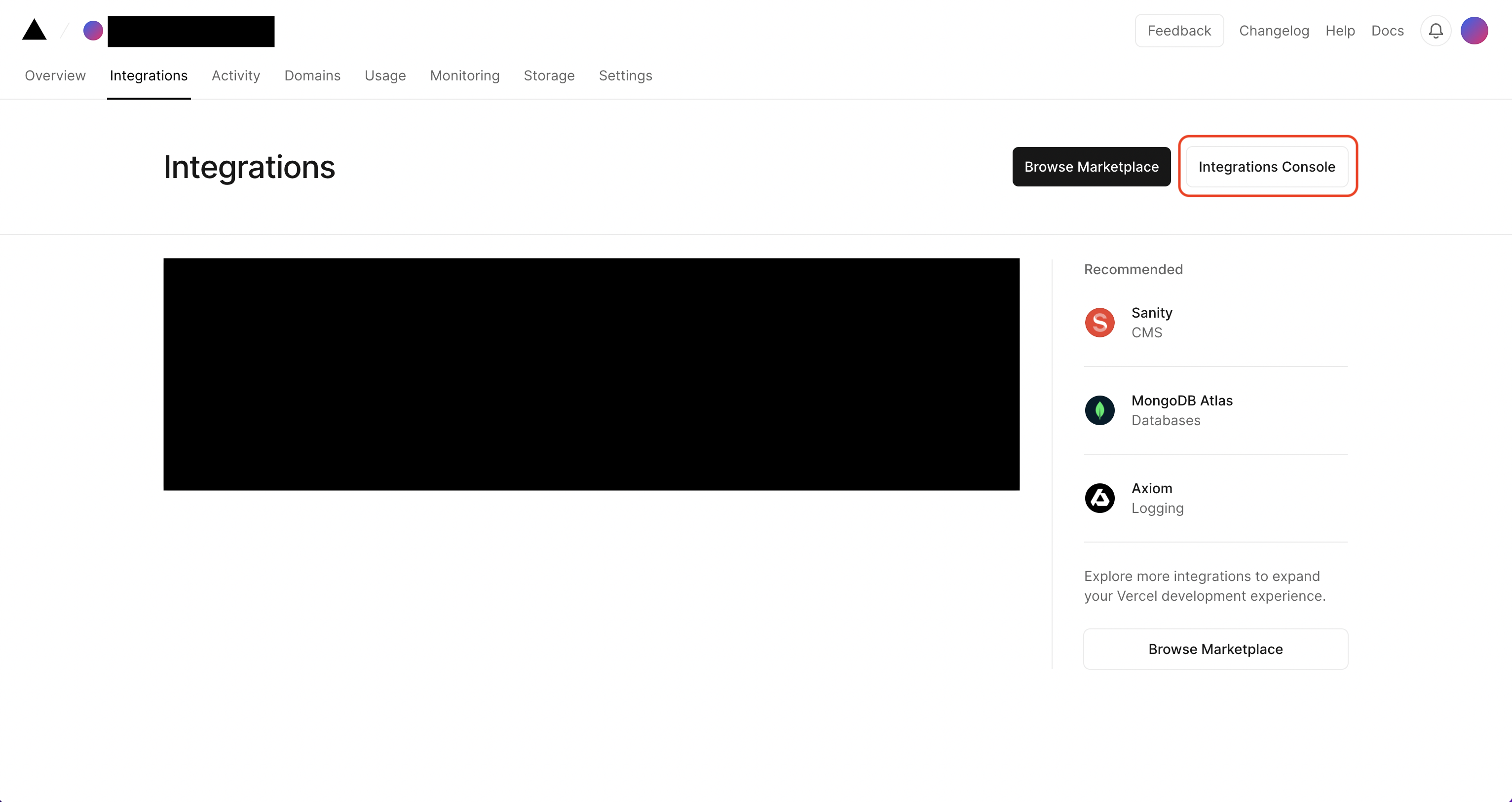
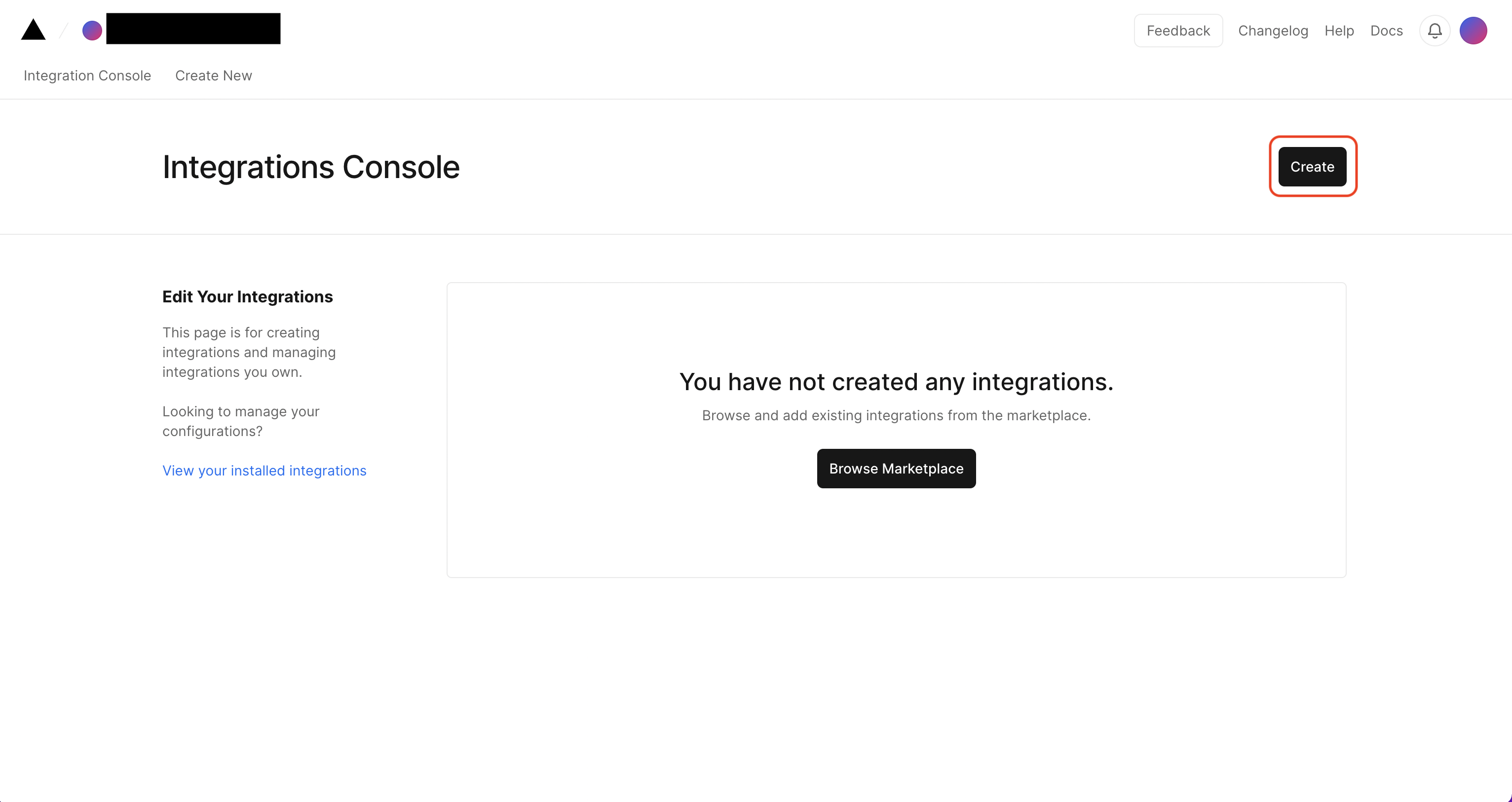
Create the application. As part of the form, set a **URL Slug** to a unique slug like `infisical-your-domain` and keep it handy. Also, set **Redirect URL** to `https://your-domain.com/integrations/vercel/oauth2/callback`. Lastly,
be sure to set the API Scopes according to the second screenshot below.
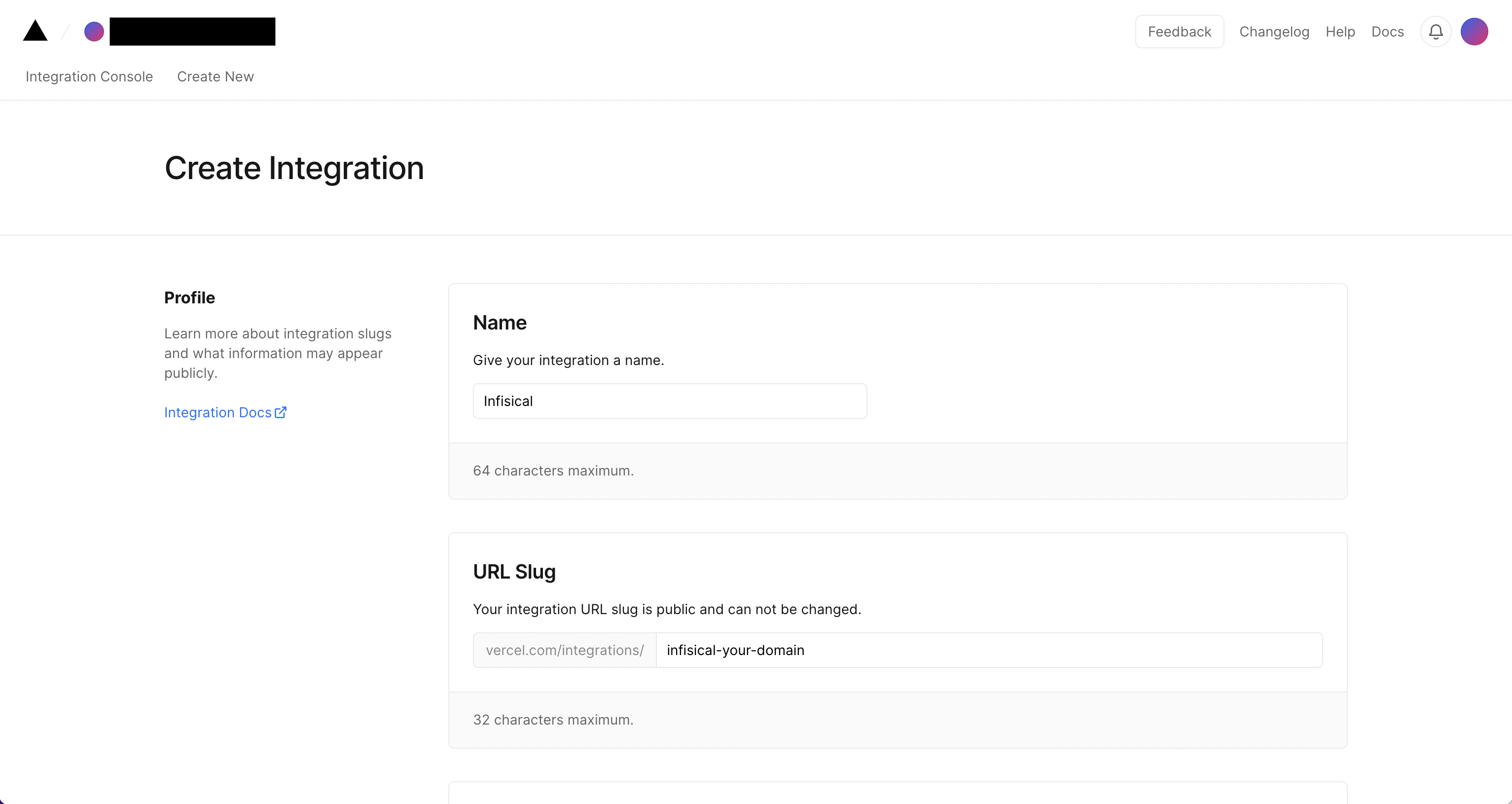
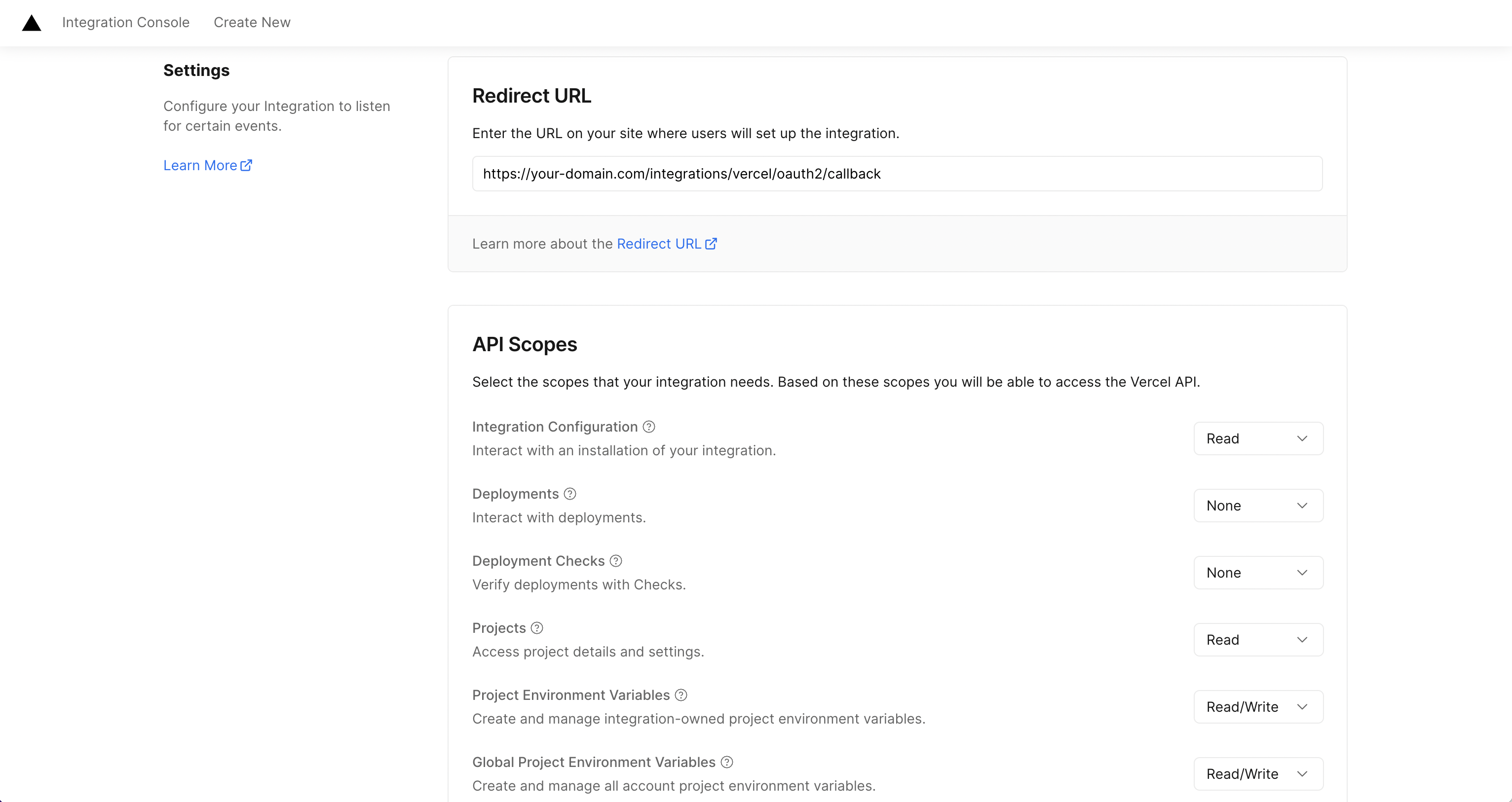
Obtain the **Client (Integration) ID** and **Client (Integration) Secret** as well as the **URL Slug** from earlier for your Vercel integration.
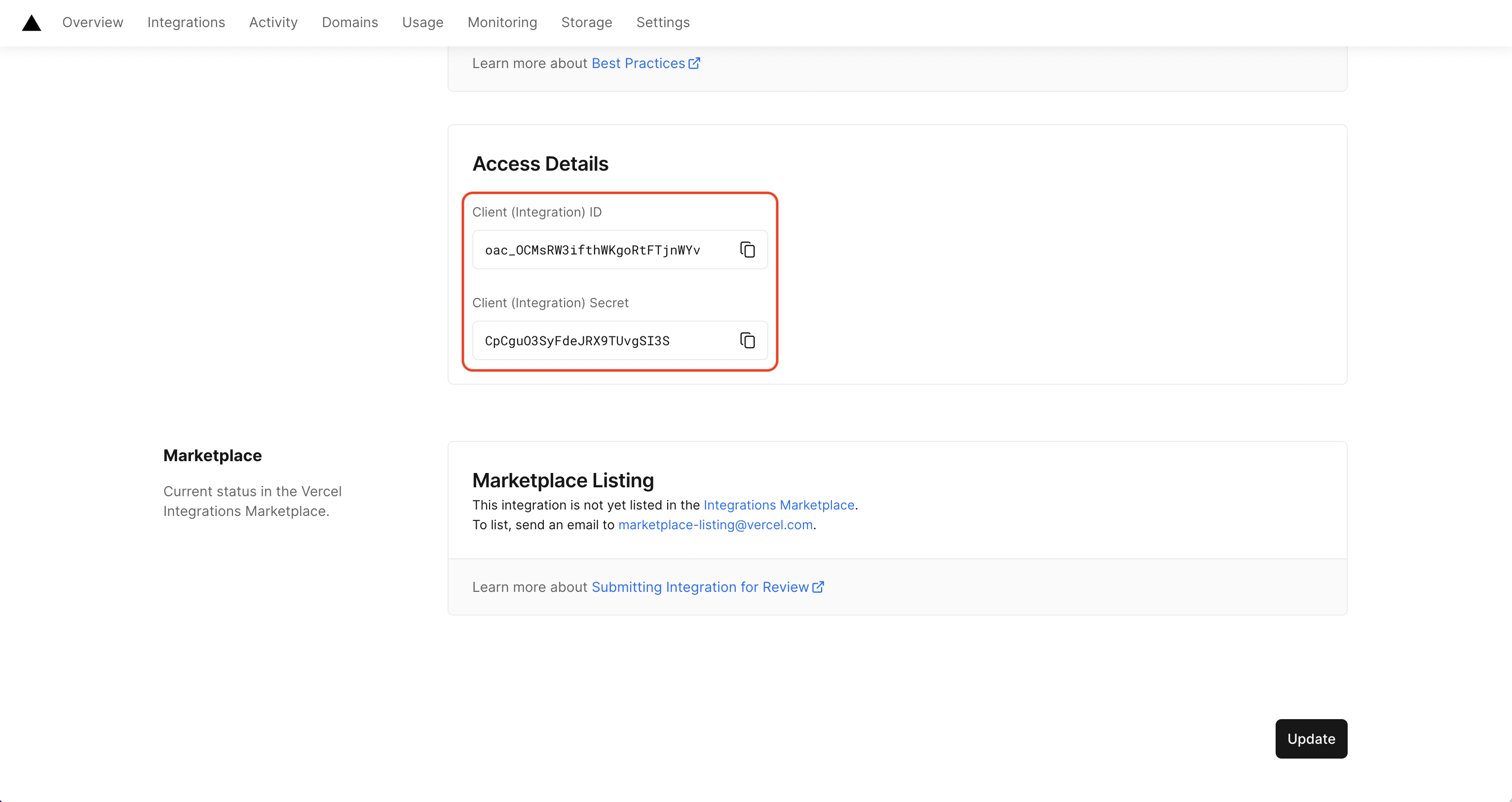
Back in your Infisical instance, add three new environment variables for the credentials of your Vercel integration.
* `CLIENT_ID_VERCEL`: The **Client (Integration) ID** of your Vercel integration.
* `CLIENT_SECRET_VERCEL`: The **Client (Integration) Secret** of your Vercel integration.
* `CLIENT_SLUG_VERCEL`: The **URL Slug** of your Vercel integration.
Once added, restart your Infisical instance and use the Vercel integration.
# Windmill
How to sync secrets from Infisical to Windmill
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
Obtain a [Windmill](https://www.windmill.dev/) access token in Access Tokens
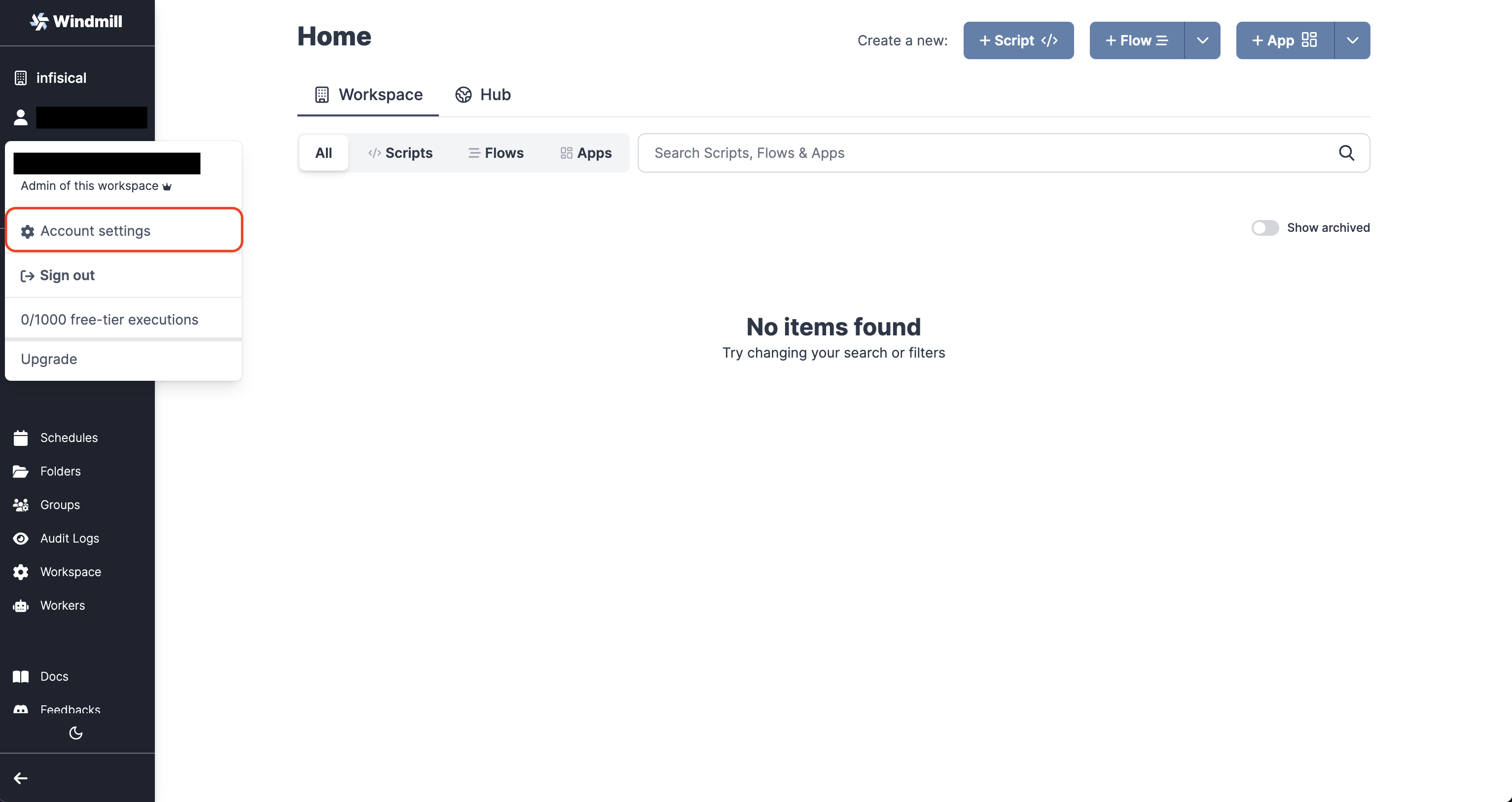
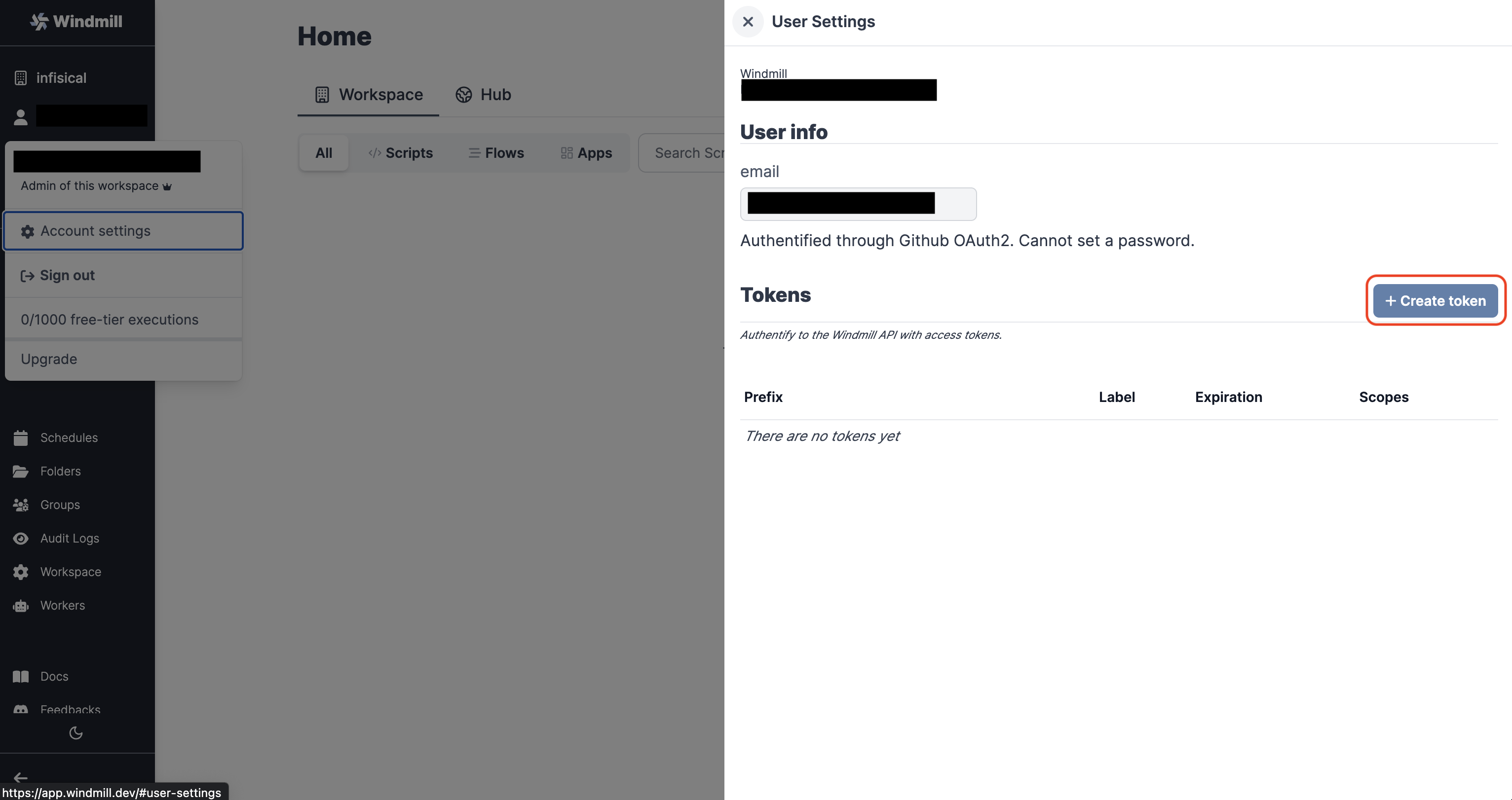
Navigate to your project's integrations tab in Infisical.
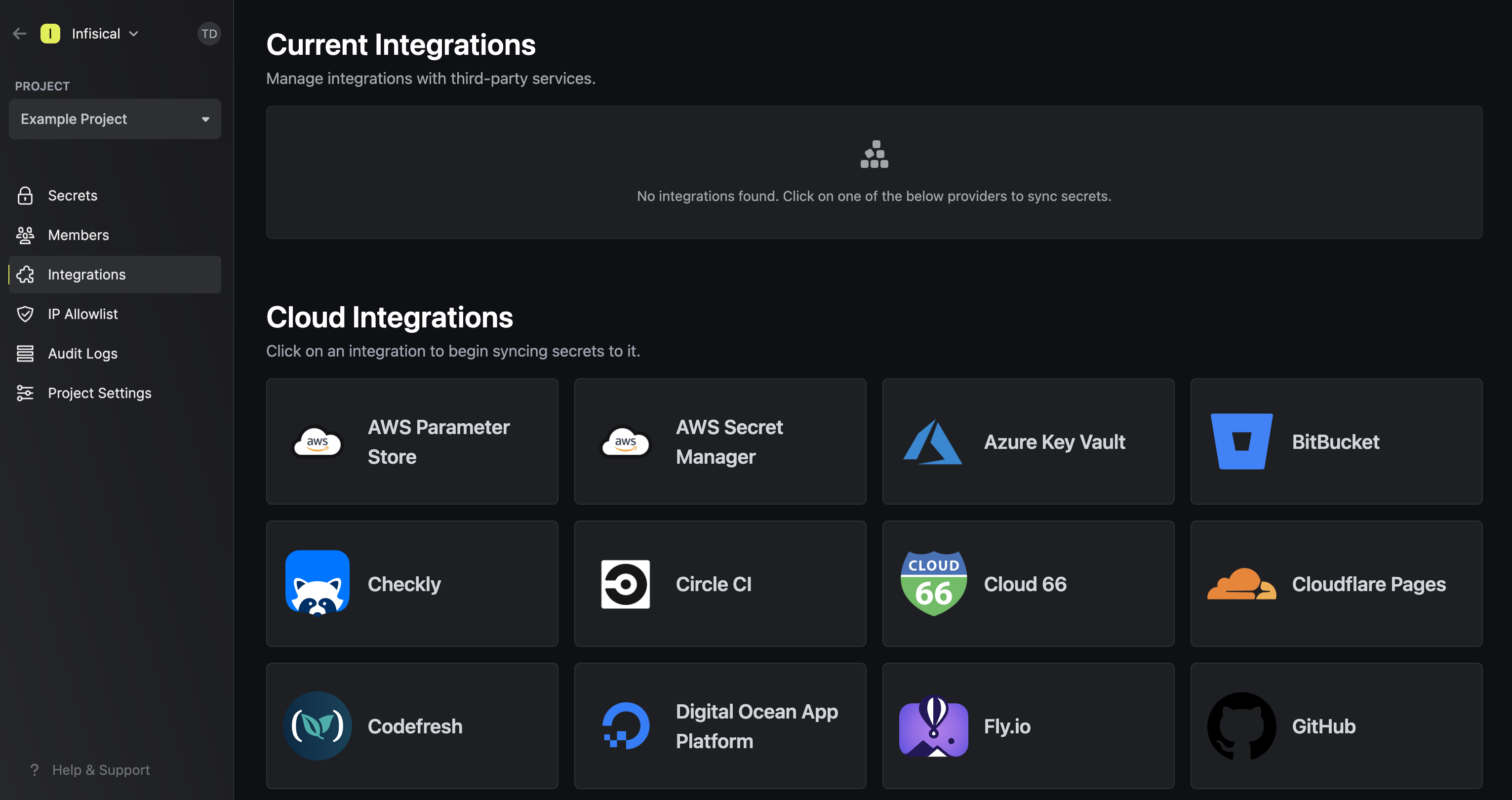
Press on the Windmill tile and input your Windmill access token to grant Infisical access to your Windmill account.
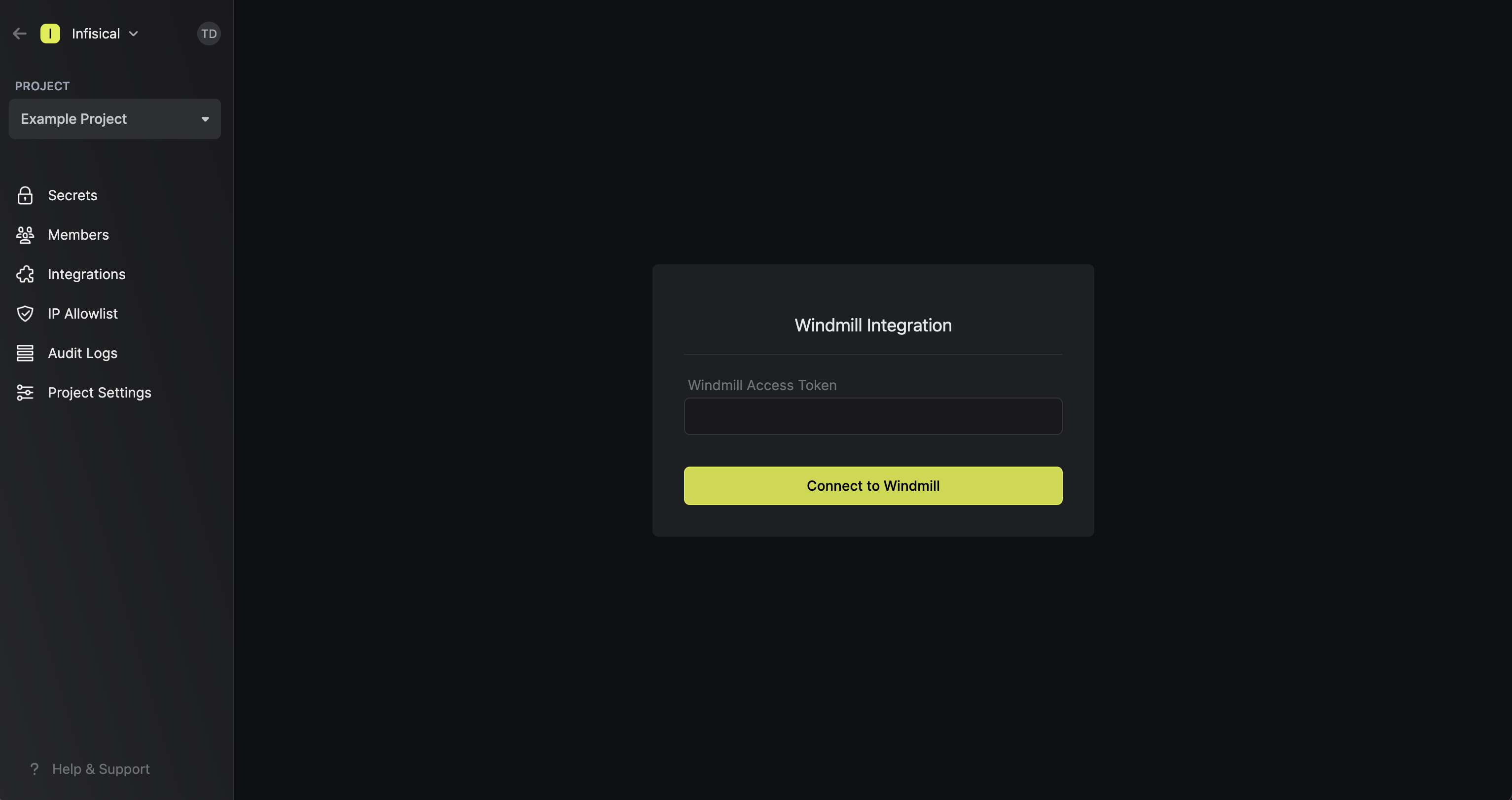
Select which Infisical environment secrets you want to sync to which Windmill workspace and press create integration to start syncing secrets to Windmill.
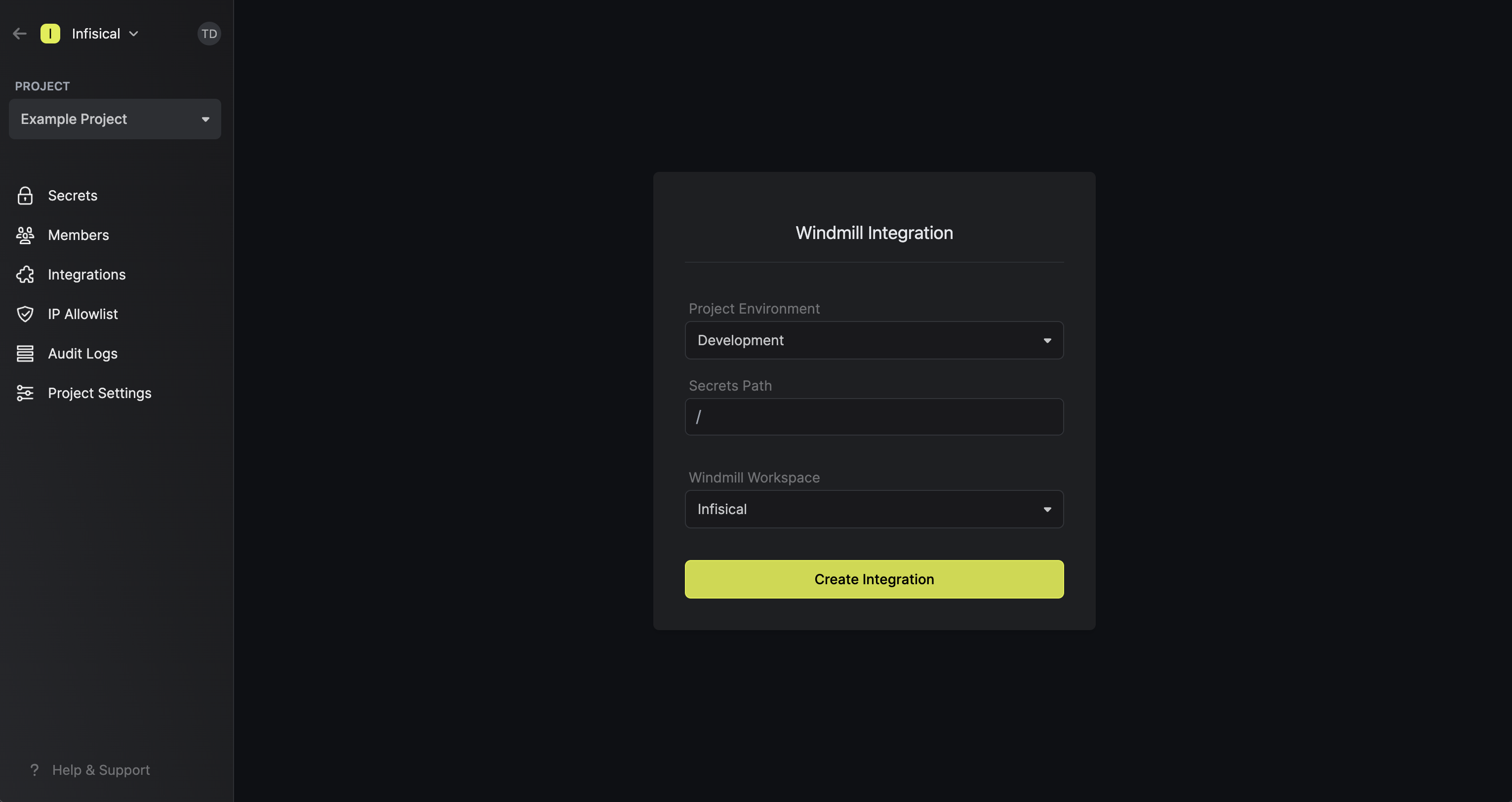
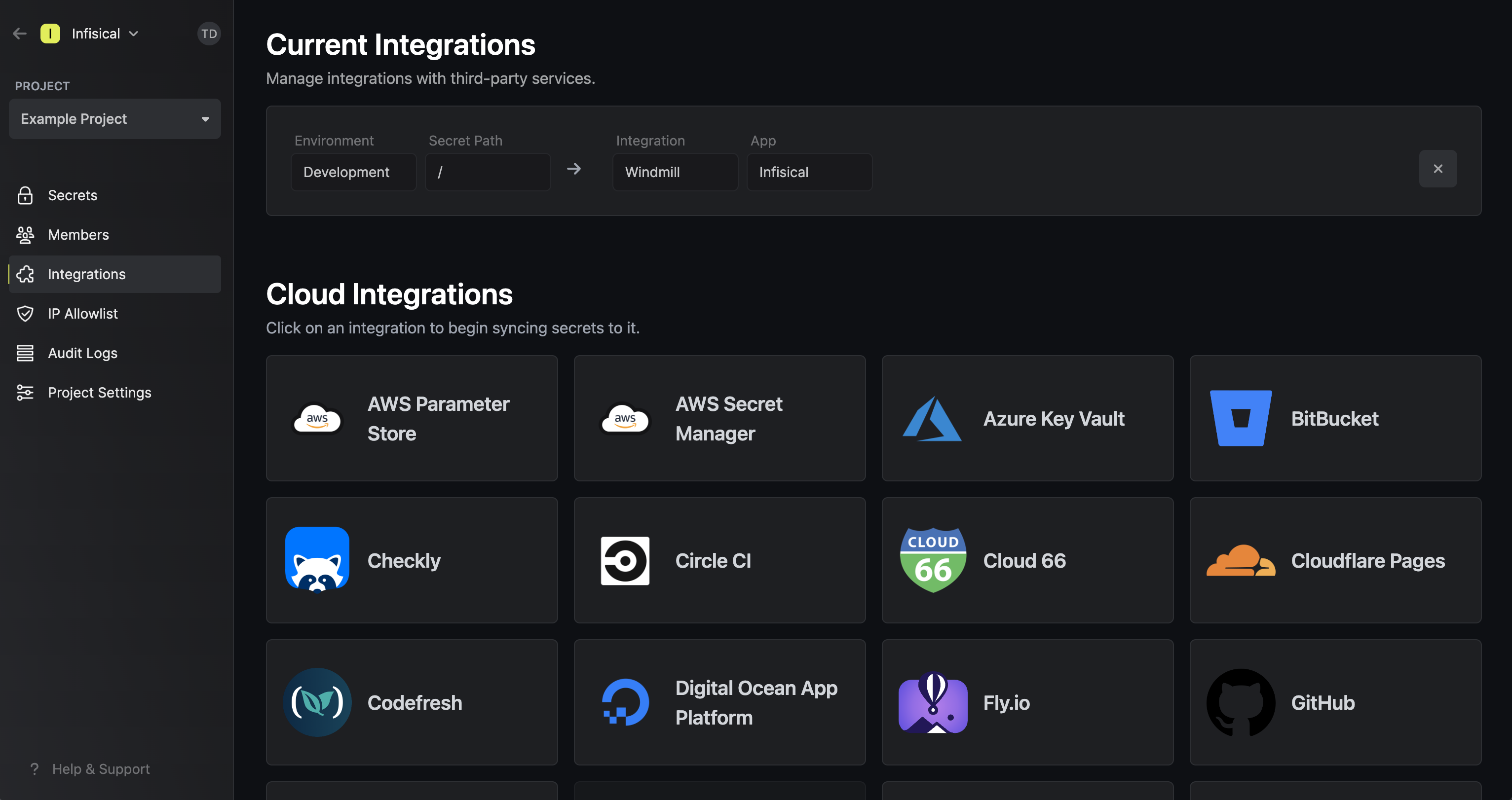
Secrets synced to Windmill are subject to the [ownership path
prefix](https://www.windmill.dev/docs/core_concepts/roles_and_permissions)
convention of Windmill. Accordingly, all secrets must be prefixed with either
`u/` or `f/` for user-based and folder-based secret along with the name of the
secret. Put differently, you must use the full path of the secret as its name
in Infisical to be considered valid such as `u/user/FOO/BAR`.
# Django
How to use Infisical to inject environment variables and secrets into a Django app.
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
* [Install the CLI](/cli/overview)
## Initialize Infisical for your [Django](https://www.djangoproject.com) project
```bash
# navigate to the root of your of your project
cd /path/to/project
# then initialize Infisical
infisical init
```
## Start your application as usual but with Infisical
```bash
infisical run --
# Example
infisical run -- python manage.py runserver
```
# .NET
How to use Infisical to inject environment variables and secrets into a .NET app.
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
* [Install the CLI](/cli/overview)
## Initialize Infisical for your [.NET](https://dotnet.microsoft.com) app
```bash
# navigate to the root of your of your project
cd /path/to/project
# then initialize infisical
infisical init
```
## Start your application as usual but with Infisical
```bash
infisical run --
# Example
infisical run -- dotnet run
```
# Express, Fastify, Koa
How to use Infisical to inject environment variables and secrets into an Express app.
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
* [Install the CLI](/cli/overview)
The steps apply to the following non-exhaustive list of frameworks:
* [Express](https://expressjs.com)
* [Fastify](https://www.fastify.io)
* [Koa](https://koajs.com)
## Initialize Infisical for your app
```bash
# navigate to the root of your of your project
cd /path/to/project
# then initialize Infisical
infisical init
```
## Start your application as usual but with Infisical
```bash
infisical run --
# Example
infisical run -- npm run dev
```
# Fiber
How to use Infisical to inject environment variables and secrets into a Fiber app.
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
* [Install the CLI](/cli/overview)
## Initialize Infisical for your [Fiber](https://gofiber.io/) app
```bash
# navigate to the root of your of your project
cd /path/to/project
# then initialize Infisical
infisical init
```
## Start your application as usual but with Infisical
```bash
infisical run --
# Example
infisical run -- go run server.go
```
# Flask
How to use Infisical to inject environment variables and secrets into a Flask app.
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
* [Install the CLI](/cli/overview)
## Initialize Infisical for your [Flask](https://flask.palletsprojects.com/en/2.2.x) app
```bash
# navigate to the root of your of your project
cd /path/to/project
# then initialize Infisical
infisical init
```
## Start your application as usual but with Infisical
```bash
infisical run --
# Example
infisical run -- flask run
```
# Gatsby
How to use Infisical to inject environment variables and secrets into a Gatsby app.
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
* [Install the CLI](/cli/overview)
## Initialize Infisical for your [Gatsby](https://www.gatsbyjs.com) app
```bash
# navigate to the root of your of your project
cd /path/to/project
# then initialize Infisical
infisical init
```
## Start your application as usual but with Infisical
```bash
infisical run --
# Example
infisical run -- npm run develop
```
Note that for environment variables to be exposed to the client, you'll have
to prefix them with `GATSBY_`. Read more about that
[here](https://www.gatsbyjs.com/docs/how-to/local-development/environment-variables/#accessing-environment-variables-in-the-browser).
# Laravel
How to use Infisical to inject environment variables and secrets into a Laravel app.
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
* [Install the CLI](/cli/overview)
## Initialize Infisical for your [Laravel](https://laravel.com/) app
```bash
# navigate to the root of your of your project
cd /path/to/project
# then initialize Infisical
infisical init
```
## Start your application as usual but with Infisical
```bash
infisical run --
# Example
infisical run -- php artisan serve
```
# NestJS
How to use Infisical to inject environment variables and secrets into a NestJS app.
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
* [Install the CLI](/cli/overview)
## Initialize Infisical for your [NestJS](https://nestjs.com) app
```bash
# navigate to the root of your of your project
cd /path/to/project
# then initialize infisical
infisical init
```
## Start your application as usual but with Infisical
```bash
infisical run --
# Example
infisical run -- npm run start:dev
```
# Next.js
How to use Infisical to inject environment variables and secrets into a Next.js app.
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
* [Install the CLI](/cli/overview)
## Initialize Infisical for your [Next.js](https://nextjs.org) app
```bash
# navigate to the root of your of your project
cd /path/to/project
# then initialize infisical
infisical init
```
## Start your application as usual but with Infisical
```bash
infisical run --
# Example
infisical run -- npm run dev
```
Note that for environment variables to be exposed to the client, you'll have
to prefix them with `NEXT_PUBLIC_`. Read more about that
[here](https://nextjs.org/docs/basic-features/environment-variables).
# Nuxt
How to use Infisical to inject environment variables and secrets into a Nuxt app.
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
* [Install the CLI](/cli/overview)
## Initialize Infisical for your [Nuxt](https://nuxtjs.org) app
```bash
# navigate to the root of your of your project
cd /path/to/project
# then initialize infisical
infisical init
```
## Start your application as usual but with Infisical
```bash
infisical run --
# Example
infisical run -- npm run dev
```
# Ruby on Rails
How to use Infisical to inject environment variables and secrets into a Ruby on Rails app.
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
* [Install the CLI](/cli/overview)
## Initialize Infisical for your [Rails](https://rubyonrails.org) app
```bash
# navigate to the root of your of your project
cd /path/to/project
# then initialize Infisical
infisical init
```
## Start your application as usual but with Infisical
```bash
infisical run --
# Example
infisical run -- bin/rails server
```
# React
How to use Infisical to inject environment variables and secrets into a React app.
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
* [Install the CLI](/cli/overview)
## Initialize Infisical for your [Create React App](https://create-react-app.dev)
```bash
# navigate to the root of your of your project
cd /path/to/project
# then initialize infisical
infisical init
```
## Start your application as usual but with Infisical
```bash
infisical run --
# Example
infisical run -- npm run dev
```
React environment variables must be prefixed with `REACT_APP_` to show up within the application
# Remix
How to use Infisical to inject environment variables and secrets into a Remix app.
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
* [Install the CLI](/cli/overview)
## Initialize Infisical for your [Remix](https://remix.run) app
```bash
# navigate to the root of your of your project
cd /path/to/project
# then initialize Infisical
infisical init
```
## Start your application as usual but with Infisical
```bash
infisical run --
# Example
infisical run -- npm run dev
```
# Spring Boot with Maven
How to use Infisical to inject environment variables into Java Spring Boot
Prerequisites:
* Set up and add your environment variables to [Infisical Cloud](https://app.infisical.com)
* [Install the CLI](/cli/overview)
## Initialize Infisical
In order for Infisical to know which secrets to fetch, you'll need to first initialize Infisical at the root of your project.
```bash
# navigate to the root of your of your project
cd /path/to/project
# then initialize Infisical
infisical init
```
## Start your application with Maven wrapper
To pass in Infisical secrets into your application, we will utilize the Infisical CLI to inject the secrets into the Maven wrapper executable, which is used to launch your application.
The Maven wrapper executable should already be present in the root directory of your project.
```bash
infisical run -- ./mvnw spring-boot:run --quiet
```
#### Accessing injected secrets
```java example.java
...
import org.springframework.core.env.Environment;
@SpringBootApplication
public class DemoApplication {
@Autowired
private Environment env;
@Bean
public void someMethod() {
System.out.println(env.getProperty("SOME_SECRET_NAME"));
};
}
```
## Debugging with secrets
During the process of debugging your code, it may be necessary to have certain environment variables available. To inject these variables for the purpose of debugging, please follow the instructions provided below.
Note that these instructions are currently only available for IntelliJ.
**Step 1:** On the main tool bar, choose Edit Configuration
**Step 2:** Click the plus icon
**Step 3:** Select Shell Script
**Step 4:** Choose Script Text and then paste in the command below.
```
infisical run -- ./mvnw spring-boot:run -Dspring-boot.run.jvmArguments="-Xdebug -Xrunjdwp:transport=dt_socket,server=y,suspend=n,address=*:5005"
```
**Step 5:** When you need to run a block of code in debug mode, select the Infisical script
# SvelteKit
How to use Infisical to inject environment variables and secrets into a SvelteKit app.
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
* [Install the CLI](/cli/overview)
## Initialize Infisical for your [SvelteKit](https://kit.svelte.dev) app
```bash
# navigate to the root of your of your project
cd /path/to/project
# then initialize infisical
infisical init
```
## Start your application as usual but with Infisical
```bash
infisical run --
# Example
infisical run -- npm run dev
```
Note that for environment variables to be exposed to the client, you'll have
to prefix them with `PUBLIC_`. Read more about that
[here](https://kit.svelte.dev/docs/modules#\$env-static-public).
# Terraform
Learn how to fetch Secrets From Infisical With Terraform.
This guide provides step-by-step guidance on how to fetch secrets from Infisical using Terraform.
## Prerequisites
* Basic understanding of Terraform
* Install [Terraform](https://www.terraform.io/downloads.html)
## Steps
### 1. Define Required Providers
Specify `infisical` in the `required_providers` block within the `terraform` block of your configuration file. If you would like to use a specific version of the provider, uncomment and replace `` with the version of the Infisical provider that you want to use.
```hcl main.tf
terraform {
required_providers {
infisical = {
# version =
source = "infisical/infisical"
}
}
}
```
### 2. Configure the Infisical Provider
Set up the Infisical provider by specifying the `host` and `service_token`. Replace `<>` in `service_token` with your actual token. The `host` is only required if you are using a self-hosted instance of Infisical.
```hcl main.tf
provider "infisical" {
host = "https://app.infisical.com" # Only required if using a self-hosted instance of Infisical, default is https://app.infisical.com
client_id = "<>"
client_secret = "<>"
service_token = "<>" # DEPRECATED, USE MACHINE IDENTITY AUTH INSTEAD
}
```
It is recommended to use Terraform variables to pass your service token dynamically to avoid hard coding it
### 3. Fetch Infisical Secrets
Use the `infisical_secrets` data source to fetch your secrets. In this block, you must set the `env_slug` and `folder_path` to scope the secrets you want.
`env_slug` is the slug of the environment name. This slug name can be found under the project settings page on the Infisical dashboard.
`folder_path` is the path to the folder in a given environment. The path `/` for root of the environment where as `/folder1` is the folder at the root of the environment.
```hcl main.tf
data "infisical_secrets" "my-secrets" {
env_slug = "dev"
folder_path = "/some-folder/another-folder"
workspace_id = "your-project-id"
}
```
### 4. Define Outputs
As an example, we are going to output your fetched secrets. Replace `SECRET-NAME` with the actual name of your secret.
For a single secret:
```hcl main.tf
output "single-secret" {
value = data.infisical_secrets.my-secrets.secrets["SECRET-NAME"]
}
```
For all secrets:
```hcl
output "all-secrets" {
value = data.infisical_secrets.my-secrets.secrets
}
```
### 5. Run Terraform
Once your configuration is complete, initialize your Terraform working directory:
```bash
$ terraform init
```
Then, run the plan command to view the fetched secrets:
```bash
$ terraform plan
```
Terraform will now fetch your secrets from Infisical and display them as output according to your configuration.
## Conclusion
You have now successfully set up and used the Infisical provider with Terraform to fetch secrets. For more information, visit the [Infisical documentation](https://registry.terraform.io/providers/Infisical/infisical/latest/docs).
# Vite
How to use Infisical to inject environment variables and secrets into a Vite app.
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
* [Install the CLI](/cli/overview)
## Initialize Infisical for your [Vite](https://vitejs.dev) app
```bash
# navigate to the root of your of your project
cd /path/to/project
# then initialize Infisical
infisical init
```
## Start your application as usual but with Infisical
```bash
infisical run --
# Example
infisical run -- npm run dev
```
Note that for environment variables to be exposed to the client, you'll have
to prefix them with `VITE_` and export them from the `vite.config.js` file.
Read more about that [here](https://vitejs.dev/guide/env-and-mode.html) and
[here](https://main.vitejs.dev/config).
# Vue
How to use Infisical to inject environment variables and secrets into a Vue.js app.
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
* [Install the CLI](/cli/overview)
## Initialize Infisical for your [Vue](https://vuejs.org) app
```bash
# navigate to the root of your of your project
cd /path/to/project
# then initialize infisical
infisical init
```
## Start your application as usual but with Infisical
```bash
infisical run --
# Example
infisical run -- npm run dev
```
Note that for environment variables to be exposed to the client, you'll have
to prefix them with `VUE_APP` Read more about that
[here](https://cli.vuejs.org/guide/mode-and-env.html).
# Ansible
Learn how to use Infisical for secret management in Ansible.
You can find the Infisical Ansible collection on [Ansible Galaxy](https://galaxy.ansible.com/ui/repo/published/infisical/vault/).
This Ansible Infisical collection includes a variety of Ansible content to help automate the management of Infisical services. This collection is maintained by the Infisical team.
## Ansible version compatibility
Tested with the Ansible Core >= 2.12.0 versions, and the current development version of Ansible. Ansible Core versions prior to 2.12.0 have not been tested.
## Python version compatibility
This collection depends on the Infisical SDK for Python.
Requires Python 3.7 or greater.
## Installing this collection
You can install the Infisical collection with the Ansible Galaxy CLI:
```bash
$ ansible-galaxy collection install infisical.vault
```
The python module dependencies are not installed by ansible-galaxy. They can be manually installed using pip:
```bash
$ pip install infisical-python
```
## Using this collection
You can either call modules by their Fully Qualified Collection Name (FQCN), such as `infisical.vault.read_secrets`, or you can call modules by their short name if you list the `infisical.vault` collection in the playbook's collections keyword:
```bash
---
vars:
read_all_secrets_within_scope: "{{ lookup('infisical.vault.read_secrets', universal_auth_client_id='<>', universal_auth_client_secret='<>', project_id='<>', path='/', env_slug='dev', url='https://spotify.infisical.com') }}"
# [{ "key": "HOST", "value": "google.com" }, { "key": "SMTP", "value": "gmail.smtp.edu" }]
read_secret_by_name_within_scope: "{{ lookup('infisical.vault.read_secrets', universal_auth_client_id='<>', universal_auth_client_secret='<>', project_id='<>', path='/', env_slug='dev', secret_name='HOST', url='https://spotify.infisical.com') }}"
# [{ "key": "HOST", "value": "google.com" }]
```
## Troubleshoot
If you get this Python error when you running the lookup plugin:-
```
objc[72832]: +[__NSCFConstantString initialize] may have been in progress in another thread when fork() was called. We cannot safely call it or ignore it in the fork() child process. Crashing instead. Set a breakpoint on objc_initializeAfterForkError to debug.
Fatal Python error: Aborted
```
You will need to add this to your shell environment or ansible wrapper script:-
```
export OBJC_DISABLE_INITIALIZE_FORK_SAFETY=YES
```
# Docker Entrypoint
Learn how to use Infisical to inject environment variables into a Docker container.
This approach allows you to inject secrets from Infisical directly into your application.
This is achieved by installing the Infisical CLI into your docker image and modifying your start command to execute with Infisical.
## Add the Infisical CLI to your Dockerfile
```dockerfile
RUN apk add --no-cache bash curl && curl -1sLf \
'https://dl.cloudsmith.io/public/infisical/infisical-cli/setup.alpine.sh' | bash \
&& apk add infisical
```
```dockerfile
RUN curl -1sLf \
'https://dl.cloudsmith.io/public/infisical/infisical-cli/setup.rpm.sh' | sh \
&& yum install -y infisical
```
```dockerfile
RUN apt-get update && apt-get install -y bash curl && curl -1sLf \
'https://dl.cloudsmith.io/public/infisical/infisical-cli/setup.deb.sh' | bash \
&& apt-get update && apt-get install -y infisical
```
####
We recommend you to set the version of the CLI to a specific version. This will help keep your CLI version consistent across reinstalls. [View versions](https://cloudsmith.io/~infisical/repos/infisical-cli/packages/)
## Modify the start command in your Dockerfile
Starting your service with the Infisical CLI pulls your secrets from Infisical and injects them into your service.
```dockerfile
CMD ["infisical", "run", "--projectId", "", "--", "[your service start command]"]
# example with single single command
CMD ["infisical", "run", "--projectId", "", "--", "npm", "run", "start"]
# example with multiple commands
CMD ["infisical", "run", "--projectId", "", "--command", "npm run start && ..."]
```
Generate a machine identity for your project by following the steps in the [Machine Identity](/documentation/platform/identities/machine-identities) guide. The machine identity will allow you to authenticate and fetch secrets from Infisical.
Obtain an access token for the machine identity by running the following command:
```bash
export INFISICAL_TOKEN=$(infisical login --method=universal-auth --client-id= --client-secret= --plain --silent)
```
Please note that the access token has a limited lifespan. The `infisical token renew` command can be used to renew the token if needed.
The last step is to give the Infisical CLI installed in your Docker container access to the access token. This will allow the CLI to fetch and inject the secrets into your application.
To feed the access token to the container, use the INFISICAL\_TOKEN environment variable as shown below.
```bash
docker run --env INFISICAL_TOKEN=$INFISICAL_TOKEN [DOCKER-IMAGE]...
```
### Using a Starting Script
The drawback of the previous method is that you would have to generate the `INFISICAL_TOKEN` manually. To automate this process, you can use a shell script as your starting command.
Create a machine identity for your project by following the steps in the [Machine Identity](/documentation/platform/identities/machine-identities) guide. This identity will enable authentication and secret retrieval from Infisical.
Create a shell script to obtain an access token for the machine identity:
```bash script.sh
#!/bin/sh
export INFISICAL_TOKEN=$(infisical login --method=universal-auth --client-id=$INFISICAL_MACHINE_CLIENT_ID --client-secret=$INFISICAL_MACHINE_CLIENT_SECRET --plain --silent)
exec infisical run --token $INFISICAL_TOKEN --projectId $PROJECT_ID --env $INFISICAL_SECRET_ENV --domain $INFISICAL_API_URL --
```
> **Note:** The access token has a limited lifespan. Use the [infisical token renew](/cli/commands/token) CLI command to renew it when necessary.
Caution: Implementing this directly in your Dockerfile presents two key issues:
1. Lack of persistence: Variables set in one build step are not automatically carried over to subsequent steps, complicating the process.
2. Security risk: It exposes sensitive credentials inside your container, potentially allowing anyone with container access to retrieve them.
Grant the Infisical CLI access to the access token, inside your Docker container. This allows the CLI to fetch and inject secrets into your application.
Add the following line to your Dockerfile:
```dockerfile
CMD ["./script.sh"]
```
```dockerfile
CMD ["infisical", "run", "--", "[your service start command]"]
# example with single single command
CMD ["infisical", "run", "--", "npm", "run", "start"]
# example with multiple commands
CMD ["infisical", "run", "--command", "npm run start && ..."]
```
Head to your project settings in the Infisical dashboard to generate an [service token](/documentation/platform/token).
This service token will allow you to authenticate and fetch secrets from Infisical.
Once you have created a service token with the required permissions, you’ll need to feed the token to the CLI installed in your docker container.
The last step is to give the Infisical CLI installed in your Docker container access to the service token. This will allow the CLI to fetch and inject the secrets into your application.
To feed the service token to the container, use the INFISICAL\_TOKEN environment variable as shown below.
```bash
docker run --env INFISICAL_TOKEN=[token] [DOCKER-IMAGE]...
```
# Docker Compose
Find out how to use Infisical to inject environment variables into services defined in your Docker Compose file.
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
## Configure the Infisical CLI for each service
Follow this [guide](./docker) to configure the Infisical CLI for each service that you wish to inject environment variables into; you'll have to update the Dockerfile of each service.
### Generate and configure machine identity
Generate a machine identity for each service you want to inject secrets into. You can do this by following the steps in the [Machine Identity](/documentation/platform/identities/machine-identities) guide.
### Set the machine identity client ID and client secret as environment variables
For each service you want to inject secrets into, generate the required `INFISICAL_TOKEN_SERVICE_A` and `INFISICAL_TOKEN_SERVICE_B`.
```yaml
# Example Docker Compose file
services:
web:
build: .
image: example-service-1
environment:
- INFISICAL_TOKEN=${INFISICAL_TOKEN_SERVICE_A}
api:
build: .
image: example-service-2
environment:
- INFISICAL_TOKEN=${INFISICAL_TOKEN_SERVICE_B}
```
### Export shell variables
Next, set the shell variables you defined in your compose file. This can be done manually or via your CI/CD environment. Once done, it will be used to populate the corresponding `INFISICAL_TOKEN_SERVICE_A` and `INFISICAL_TOKEN_SERVICE_B` in your Docker Compose file.
```bash
#Example
# Token refers to the token we generated in step 2 for this service
export INFISICAL_TOKEN_SERVICE_A=$(infisical login --method=universal-auth --client-id= --client-secret= --silent --plain)
export INFISICAL_TOKEN_SERVICE_B=$(infisical login --method=universal-auth --client-id= --client-secret= --silent --plain)
# Then run your compose file in the same terminal.
docker-compose ...
```
## Generate service token
Generate a unique [Service Token](/documentation/platform/token) for each service.
## Feed service token to your Docker Compose file
For each service you want to inject secrets into, set an environment variable called `INFISICAL_TOKEN` equal to a unique identifier variable.
In the example below, we set `INFISICAL_TOKEN_FOR_WEB` and `INFISICAL_TOKEN_FOR_API` as the `INFISICAL_TOKEN` for the services.
```yaml
# Example Docker Compose file
services:
web:
build: .
image: example-service-1
environment:
- INFISICAL_TOKEN=${INFISICAL_TOKEN_FOR_WEB}
api:
build: .
image: example-service-2
environment:
- INFISICAL_TOKEN=${INFISICAL_TOKEN_FOR_API}
```
## Export shell variables
Next, set the shell variables you defined in your compose file. This can be done manually or via your CI/CD environment. Once done, it will be used to populate the corresponding `INFISICAL_TOKEN`
in your Docker Compose file.
```bash
#Example
# Token refers to the token we generated in step 2 for this service
export INFISICAL_TOKEN_FOR_WEB=
# Token refers to the token we generated in step 2 for this service
export INFISICAL_TOKEN_FOR_API=
# Then run your compose file in the same terminal.
docker-compose ...
```
# Docker
Learn how to feed secrets from Infisical into your Docker application.
There are many methods to inject Infisical secrets into Docker-based applications.
Regardless of the method you choose, they all inject secrets from Infisical as environment variables into your Docker container.
Install and run your app start command with Infisical CLI
Feed secrets with the `--env-file` flag when using the
`docker run` command
Inject secrets into multiple services using Docker Compose
The main difference between the "Docker Entrypoint" and "Docker run" approach is where the Infisical CLI is installed.
In most production settings, it's typically less convenient to have the Infisical CLI installed and executed externally, so we suggest using the "Docker Entrypoint" method for production purposes.
However, if this limitation doesn't apply to you, select the method that best fits your needs.
# Docker Run
Learn how to pass secrets to your docker container at run time.
This method allows you to feed secrets from Infisical into your container using the `--env-file` flag of `docker run` command.
Rather than giving the flag a file path to your env file, you'll use the Infisical CLI to create a virtual file path.
For this method to function as expected, you must have a bash shell (for processing substitution) and the [Infisical CLI](../../cli/overview) installed in the environment where you will be running the `docker run` command.
## 1. Authentication
If you are already logged in via the CLI you can skip this step. Otherwise, head to your organization settings in Infisical Cloud to create a [Machine Identity](../../documentation/platform/identities/machine-identities). The machine identity will allow you to authenticate and fetch secrets from Infisical.
Once you have created a machine identity with the required permissions, you'll need to feed the token to the CLI.
Please note that we highly recommend using `infisical login` for local development.
#### Pass as flag
You may use the --token flag to set the token
```bash
infisical export --token=<>
```
#### Pass via shell environment variable
The CLI is configured to look for an environment variable named `INFISICAL_TOKEN`. If set, it'll attempt to use it for authentication.
```bash
export INFISICAL_TOKEN=<>
```
You can use the `infisical login --method=universal-auth` command to directly obtain a universal auth access token and set it as an environment variable.
```bash
export INFISICAL_TOKEN=$(infisical login --method=universal-auth --client-id= --client-secret= --silent --plain)
```
In production scenarios, please to avoid using the `infisical login` command and instead use a [machine identity](../../documentation/platform/identities/machine-identities).
## 2. Run your docker command with Infisical
Next, use the --env-file flag of the `docker run` command with Infisical CLI to point to your secrets.
Under the hood, this command will fetch secrets from Infisical and serve them as a file to the `--env-file` flag.
```bash
# In this example, executing a docker run command will initiate an empty Alpine container and display the environment variables passed to it by Infisical.
docker run --rm --env-file <(infisical export --format=dotenv) alpine printenv
```
To view all options of the `export` command, click [here](../../cli/commands/export)
When using the --env-file option, Docker does not have the capability to support secrets that span multiple lines.
# Docker Swarm
Learn how to manage secrets in Docker Swarm services.
In this guide, we'll demonstrate how to use Infisical for managing secrets within Docker Swarm.
Specifically, we'll set up a sidecar container using the [Infisical Agent](/infisical-agent/overview), which authenticates with Infisical to retrieve secrets and access tokens.
These secrets are then stored in a shared volume accessible by other services in your Docker Swarm.
## Prerequisites
* Infisical account
* Docker version 20.10.24 or newer
* Basic knowledge of Docker Swarm
* [Git](https://git-scm.com/book/en/v2/Getting-Started-Installing-Git) installed on your system
* Familiarity with the [Infisical Agent](/infisical-agent/overview)
## Objective
Our goal is to deploy an Nginx instance in your Docker Swarm cluster, configured to display Infisical secrets on its landing page. This will provide hands-on experience in fetching and utilizing secrets from Infisical within Docker Swarm. The principles demonstrated here are also applicable to Docker Compose deployments.
Start by cloning the [Infisical guide assets repository](https://github.com/Infisical/infisical-guides.git) from Github. This repository includes necessary assets for this and other Infisical guides. Focus on the `docker-swarm-with-agent` sub-directory, which we'll use as our working directory.
To allow the agent to fetch your Infisical secrets, choose an authentication method for the agent. For this guide, we will use [Universal Auth](/documentation/platform/identities/universal-auth) for authentication. Follow the instructions [here](/documentation/platform/identities/universal-auth) to generate a client ID and client secret.
Copy the client ID and client secret obtained in the previous step into the `client-id` and `client-secret` text files, respectively.
The Infisical Agent will authenticate using Universal Auth and retrieve secrets for rendering as specified in the template(s).
Adjust the `polling-interval` to control the frequency of secret updates.
In the example template, the secrets are rendered as an HTML page, which will be set as Nginx's home page to demonstrate successful secret retrieval and utilization.
Remember to add your project id, environment slug and path of corresponding Infisical project to the secret template.
```yaml infisical-agent-config
infisical:
address: "https://app.infisical.com"
auth:
type: "universal-auth"
config:
client-id: "/run/secrets/infisical-universal-auth-client-id"
client-secret: "/run/secrets/infisical-universal-auth-client-secret"
remove_client_secret_on_read: false
sinks:
- type: "file"
config:
path: "/infisical-secrets/access-token"
templates:
- source-path: /run/secrets/nginx-home-page-template
destination-path: /infisical-secrets/index.html
config:
polling-interval: 60s
```
Some paths contain `/run/secrets/` because the contents of those files reside in a [Docker secret](https://docs.docker.com/engine/swarm/secrets/#how-docker-manages-secrets).
```html nginx-home-page-template
This file is rendered by Infisical agent template engine
Here are the secrets that have been fetched from Infisical and stored in your volume mount
{{- with secret "7df67a5f-d26a-4988-a375-7153c08149da" "dev" "/" }}
{{- range . }}
{{ .Key }}={{ .Value }}
{{- end }}
{{- end }}
```
Define the `infisical-agent` and `nginx` services in your Docker Compose file. `infisical-agent` will handle secret retrieval and storage. These secrets are stored in a volume, accessible by other services like Nginx.
```yaml docker-compose.yaml
version: "3.1"
services:
infisical-agent:
container_name: infisical-agnet
image: infisical/cli:0.18.0
command: agent --config=/run/secrets/infisical-agent-config
volumes:
- infisical-agent:/infisical-secrets
secrets:
- infisical-universal-auth-client-id
- infisical-universal-auth-client-secret
- infisical-agent-config
- nginx-home-page-template
networks:
- infisical_network
nginx:
image: nginx:latest
ports:
- "80:80"
volumes:
- infisical-agent:/usr/share/nginx/html
networks:
- infisical_network
volumes:
infisical-agent:
secrets:
infisical-universal-auth-client-id:
file: ./client-id
infisical-universal-auth-client-secret:
file: ./client-secret
infisical-agent-config:
file: ./infisical-agent-config
nginx-home-page-template:
file: ./nginx-home-page-template
networks:
infisical_network:
```
```
docker swarm init
```
```
docker stack deploy -c docker-compose.yaml agent-demo
```
To confirm that secrets are properly rendered and accessible, navigate to `http://localhost`. You should see the Infisical secrets displayed on the Nginx landing page.
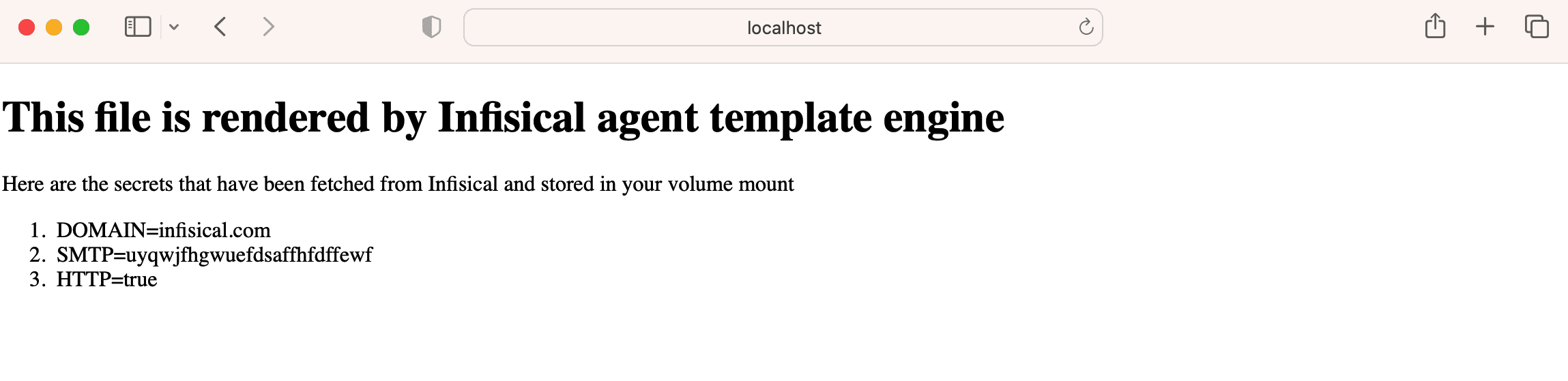
```
docker stack rm agent-demo
```
## Considerations
* Secret Updates: Applications that access secrets directly from the volume mount will receive updates in real-time, in accordance with the `polling-interval` set in agent config.
* In-Memory Secrets: If your application loads secrets into memory, the new secrets will be available to the application on the next deployment.
# Amazon ECS
Learn how to deliver secrets to Amazon Elastic Container Service.
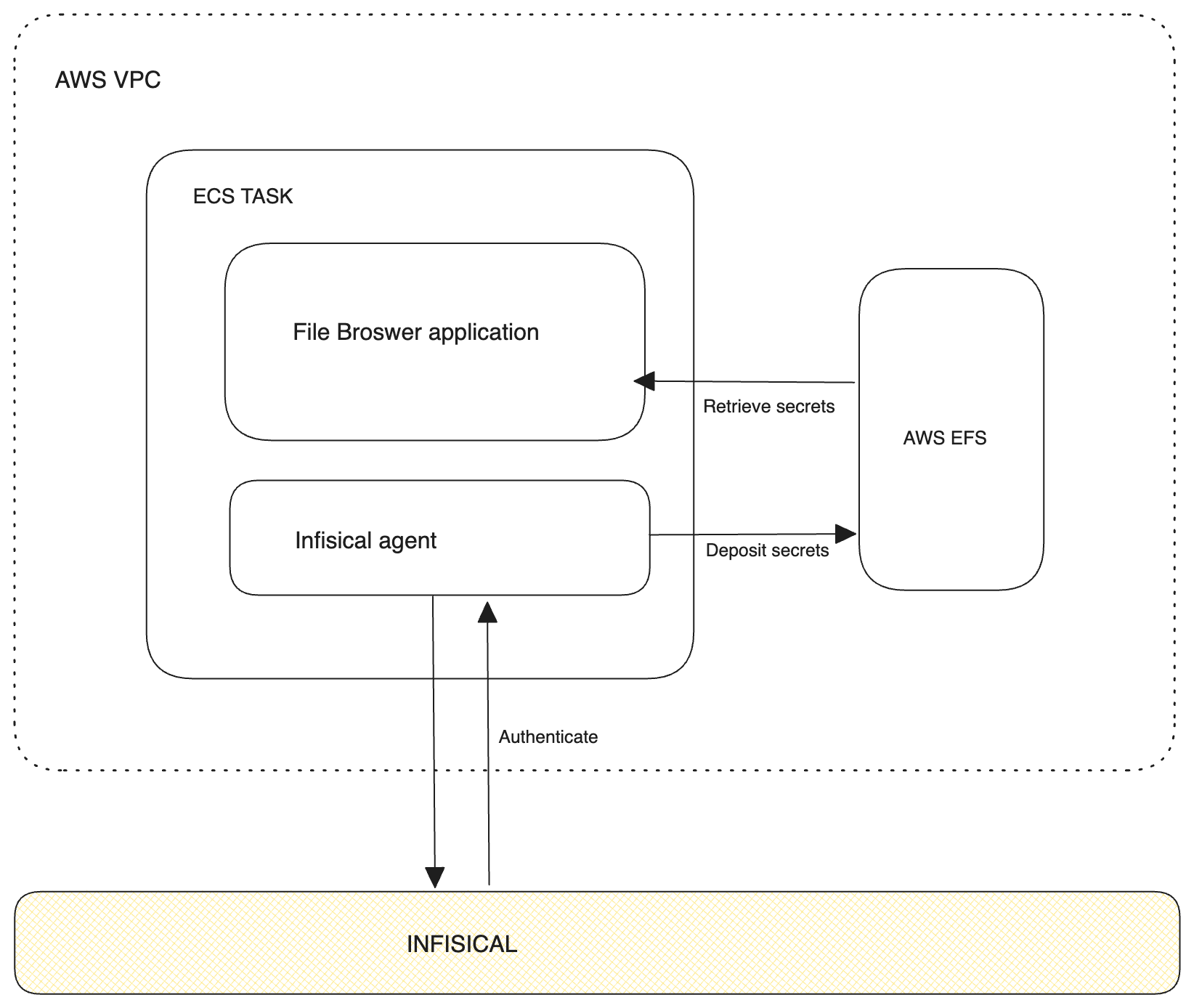
This guide will go over the steps needed to access secrets stored in Infisical from Amazon Elastic Container Service (ECS).
At a high level, the steps involve setting up an ECS task with an [Infisical Agent](/infisical-agent/overview) as a sidecar container. This sidecar container uses [AWS Auth](/documentation/platform/identities/aws-auth) to authenticate with Infisical to fetch secrets/access tokens.
Once the secrets/access tokens are retrieved, they are then stored in a shared [Amazon Elastic File System](https://aws.amazon.com/efs/) (EFS) volume. This volume is then made accessible to your application and all of its replicas.
This guide primarily focuses on integrating Infisical Cloud with Amazon ECS on AWS Fargate and Amazon EFS.
However, the principles and steps can be adapted for use with any instance of Infisical (on premise or cloud) and different ECS launch configurations.
## Prerequisites
This guide requires the following prerequisites:
* Infisical account
* Git installed
* Terraform v1.0 or later installed
* Access to AWS credentials
* Understanding of [Infisical Agent](/infisical-agent/overview)
## What we will deploy
For this demonstration, we'll deploy the [File Browser](https://github.com/filebrowser/filebrowser) application on our ECS cluster.
Although this guide focuses on File Browser, the principles outlined here can be applied to any application of your choice.
File Browser plays a key role in this context because it enables us to view all files attached to a specific volume.
This feature is important for our demonstration, as it allows us to verify whether the Infisical agent is depositing the expected files into the designated file volume and if those files are accessible to the application.
Volumes that contain sensitive secrets should not be publicly accessible. The
use of File Browser here is solely for demonstration and verification
purposes.
## Configure Authentication with Infisical
In order for the Infisical agent to fetch credentials from Infisical, we'll first need to authenticate with Infisical. Follow the documentation to configure a machine identity with AWS Auth [here](/documentation/platform/identities/aws-auth).
Take note of the Machine Identity ID as you will be needing this in the preceding steps.
## Clone guide assets repository
To help you quickly deploy the example application, please clone the guide assets from this [Github repository](https://github.com/Infisical/infisical-guides.git).
This repository contains assets for all Infisical guides. The content for this guide can be found within a sub directory called `aws-ecs-with-agent`.
The guide will assume that `aws-ecs-with-agent` is your working directory going forward.
## Deploy example application
Before we can deploy our full application and its related infrastructure with Terraform, we'll need to first configure our Infisical agent.
### Agent configuration overview
The agent config file defines what authentication method will be used when connecting with Infisical along with where the fetched secrets/access tokens should be saved to.
Since the Infisical agent will be deployed as a sidecar, the agent configuration file will need to be encoded in base64.
This encoding step is necessary as it allows the agent configuration file to be added into our Terraform configuration without needing to upload it first.
#### Full agent configuration file
Inside the `aws-ecs-with-agent` directory, you will find a sample `agent-config.yaml` file. This agent config file will connect with Infisical Cloud using AWS Auth and deposit access tokens at path `/infisical-agent/access-token` and render secrets to file `/infisical-agent/secrets`.
```yaml agent-config.yaml
infisical:
address: https://app.infisical.com
exit-after-auth: true
auth:
type: aws-iam
sinks:
- type: file
config:
path: /infisical-agent/access-token
templates:
- template-content: |
{{- with secret "202f04d7-e4cb-43d4-a292-e893712d61fc" "dev" "/" }}
{{- range . }}
{{ .Key }}={{ .Value }}
{{- end }}
{{- end }}
destination-path: /infisical-agent/secrets
```
#### Secret template
The Infisical agent accepts one or more optional templates. If provided, the agent will fetch secrets using the set authentication method and format the fetched secrets according to the given template.
Typically, these templates are passed in to the agent configuration file via file reference using the `source-path` property but for simplicity we define them inline.
In the agent configuration above, the template defined will transform the secrets from Infisical project with the ID `202f04d7-e4cb-43d4-a292-e893712d61fc`, in the `dev` environment, and secrets located in the path `/`, into a `KEY=VALUE` format.
Remember to update the project id, environment slug and secret path to one
that exists within your Infisical project
## Configure app on terraform
Navigate to the `ecs.tf` file in your preferred code editor. In the container\_definitions section, assign the values to the `machine_identity_id` and `agent_config` properties.
The `agent_config` property expects the base64-encoded agent configuration file. In order to get this, we use the `base64encode` and `file` functions of HCL.
```hcl ecs.tf
...snip...
resource "aws_ecs_task_definition" "app" {
family = "cb-app-task"
execution_role_arn = aws_iam_role.ecs_task_execution_role.arn
task_role_arn = aws_iam_role.ecs_task_role.arn
network_mode = "awsvpc"
requires_compatibilities = ["FARGATE"]
cpu = 4096
memory = 8192
container_definitions = templatefile("./templates/ecs/cb_app.json.tpl", {
app_image = var.app_image
sidecar_image = var.sidecar_image
app_port = var.app_port
fargate_cpu = var.fargate_cpu
fargate_memory = var.fargate_memory
aws_region = var.aws_region
machine_identity_id = "5655f4f5-332b-45f9-af06-8f493edff36f"
agent_config = base64encode(file("../agent-config.yaml"))
})
volume {
name = "infisical-efs"
efs_volume_configuration {
file_system_id = aws_efs_file_system.infisical_efs.id
root_directory = "/"
}
}
}
...snip...
```
After these values have been set, they will be passed to the Infisical agent during startup through environment variables, as configured in the `infisical-sidecar` container below.
```terraform templates/ecs/cb_app.json.tpl
[
...snip...
{
"name": "infisical-sidecar",
"image": "${sidecar_image}",
"cpu": 1024,
"memory": 1024,
"networkMode": "bridge",
"command": ["agent"],
"essential": false,
"logConfiguration": {
"logDriver": "awslogs",
"options": {
"awslogs-group": "/ecs/agent",
"awslogs-region": "${aws_region}",
"awslogs-stream-prefix": "ecs"
}
},
"healthCheck": {
"command": ["CMD-SHELL", "agent", "--help"],
"interval": 30,
"timeout": 5,
"retries": 3,
"startPeriod": 0
},
"environment": [
{
"name": "INFISICAL_MACHINE_IDENTITY_ID",
"value": "${machine_identity_id}"
},
{
"name": "INFISICAL_AGENT_CONFIG_BASE64",
"value": "${agent_config}"
}
],
"mountPoints": [
{
"containerPath": "/infisical-agent",
"sourceVolume": "infisical-efs"
}
]
}
]
```
In the above container definition, you'll notice that that the Infisical agent has a `mountPoints` defined.
This mount point is referencing to the already configured EFS volume as shown below.
`containerPath` is set to `/infisical-agent` because that is that the folder we have instructed the agent to deposit the credentials to.
```hcl terraform/efs.tf
resource "aws_efs_file_system" "infisical_efs" {
tags = {
Name = "INFISICAL-ECS-EFS"
}
}
resource "aws_efs_mount_target" "mount" {
count = length(aws_subnet.private.*.id)
file_system_id = aws_efs_file_system.infisical_efs.id
subnet_id = aws_subnet.private[count.index].id
security_groups = [aws_security_group.efs_sg.id]
}
```
## Configure AWS credentials
Because we'll be deploying the example file browser application to AWS via Terraform, you will need to obtain a set of `AWS Access Key` and `Secret Key`.
Once you have generated these credentials, export them to your terminal.
1. Export the AWS Access Key ID:
```bash
export AWS_ACCESS_KEY_ID=
```
2. Export the AWS Secret Access Key:
```bash
export AWS_SECRET_ACCESS_KEY=
```
## Deploy terraform configuration
With the agent's sidecar configuration complete, we can now deploy our changes to AWS via Terraform.
1. Change your directory to `terraform`
```sh
cd terraform
```
2. Initialize Terraform
```
$ terraform init
```
3. Preview resources that will be created
```
$ terraform plan
```
4. Trigger resource creation
```bash
$ terraform apply
Do you want to perform these actions?
Terraform will perform the actions described above.
Only 'yes' will be accepted to approve.
Enter a value: yes
```
```bash
Apply complete! Resources: 1 added, 1 changed, 1 destroyed.
Outputs:
alb_hostname = "cb-load-balancer-1675475779.us-east-1.elb.amazonaws.com:8080"
```
Once the resources have been successfully deployed, Terraform will output the host address where the file browser application will be accessible.
It may take a few minutes for the application to become fully ready.
## Verify secrets/tokens in EFS volume
To verify that the agent is depositing access tokens and rendering secrets to the paths specified in the agent config, navigate to the web address from the previous step.
Once you visit the address, you'll be prompted to login. Enter the credentials shown below.
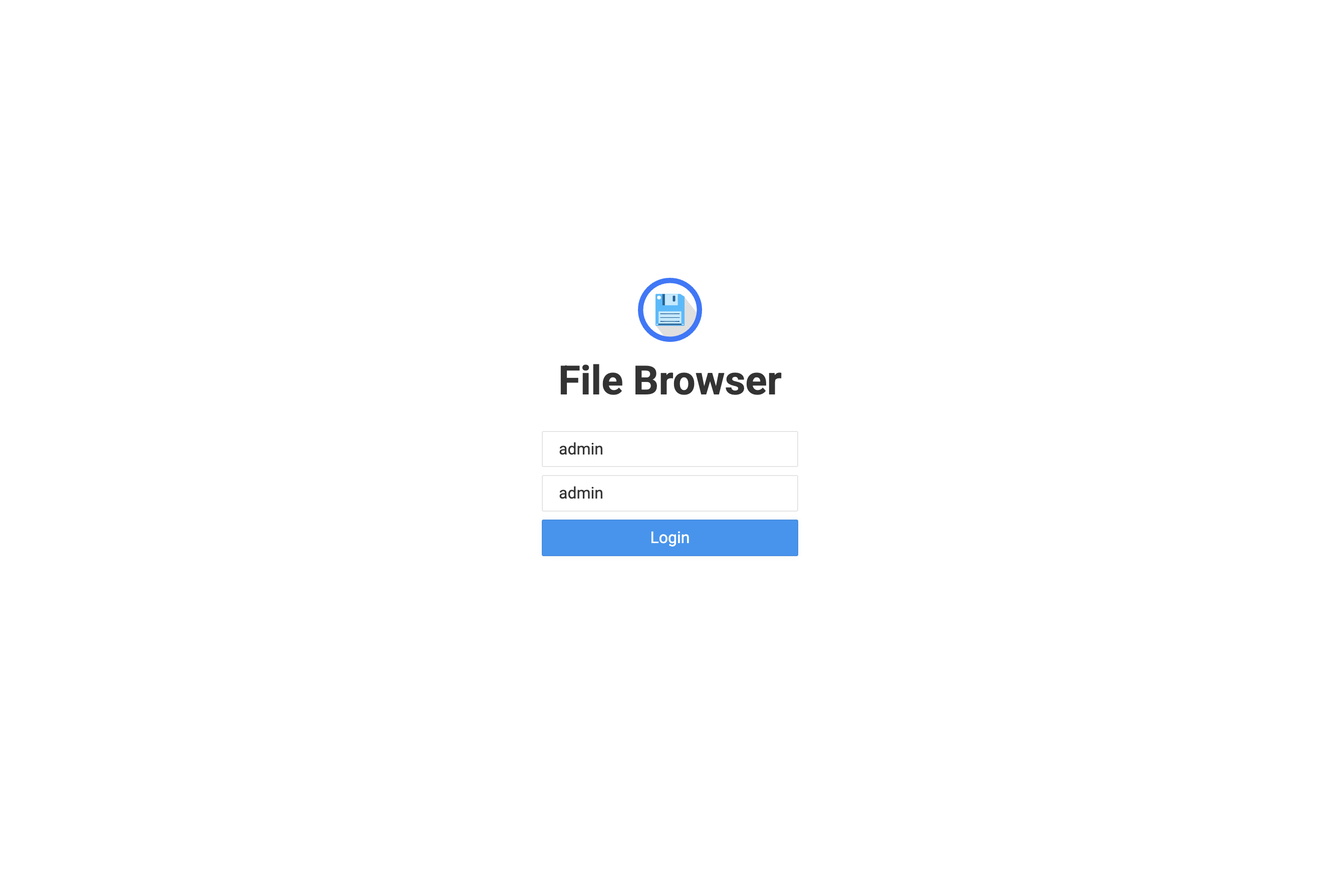
Since our EFS volume is mounted to the path of the file browser application, we should see the access token and rendered secret file we defined via the agent config file.
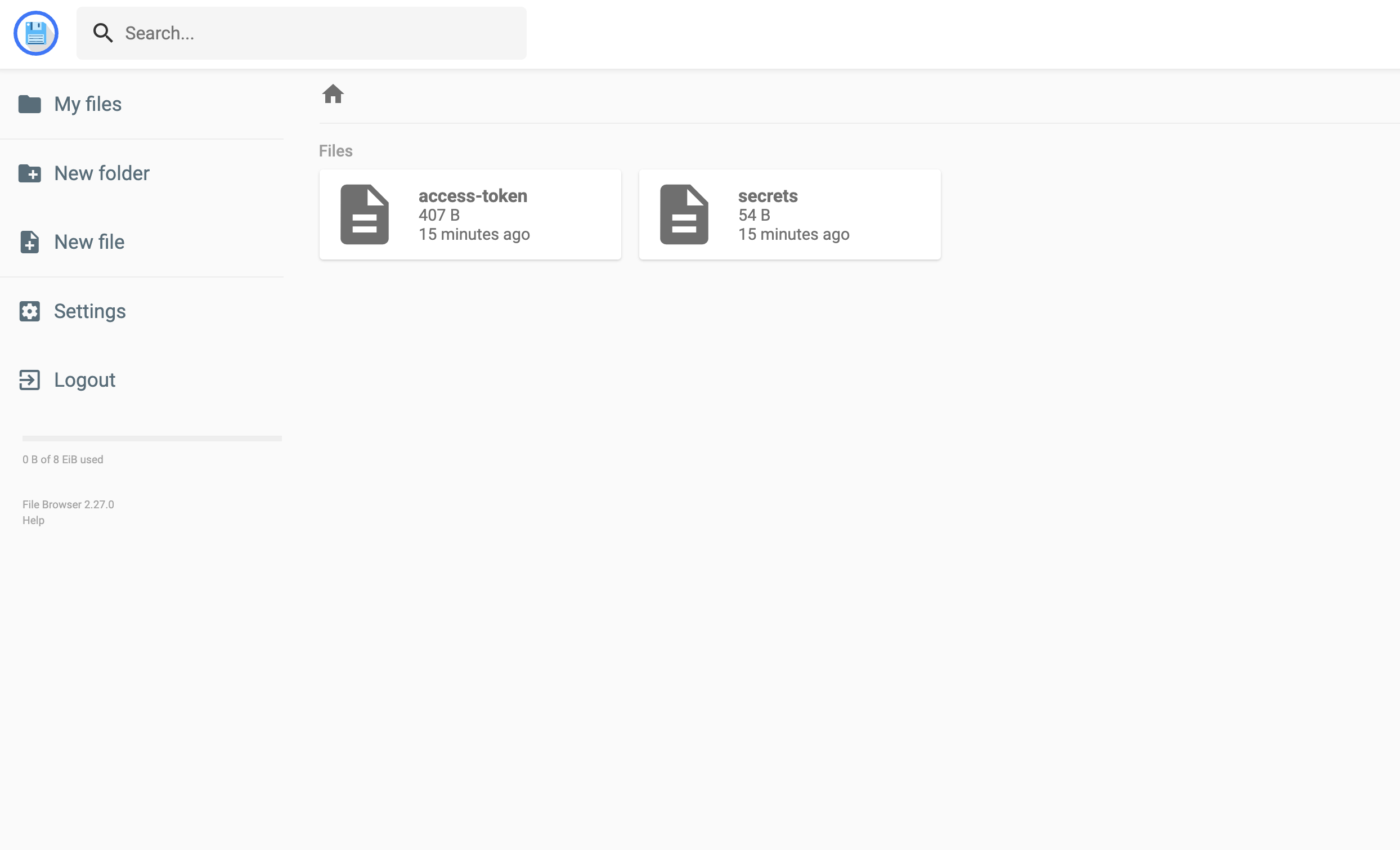
As expected, two files are present: `access-token` and `secrets`.
The `access-token` file should hold a valid `Bearer` token, which can be used to make HTTP requests to Infisical.
The `secrets` file should contain secrets, formatted according to the specifications in our secret template file (presented in key=value format).
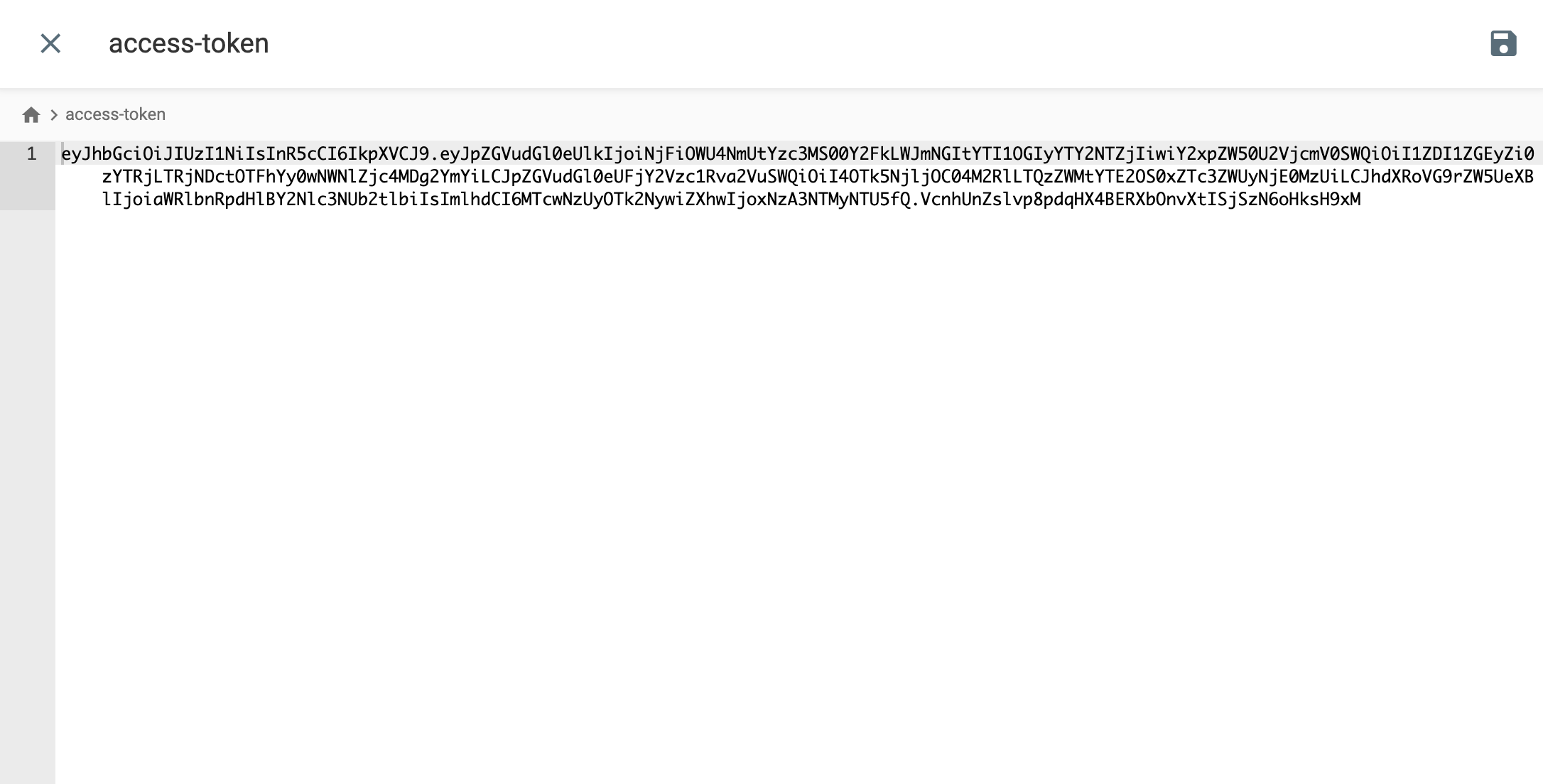
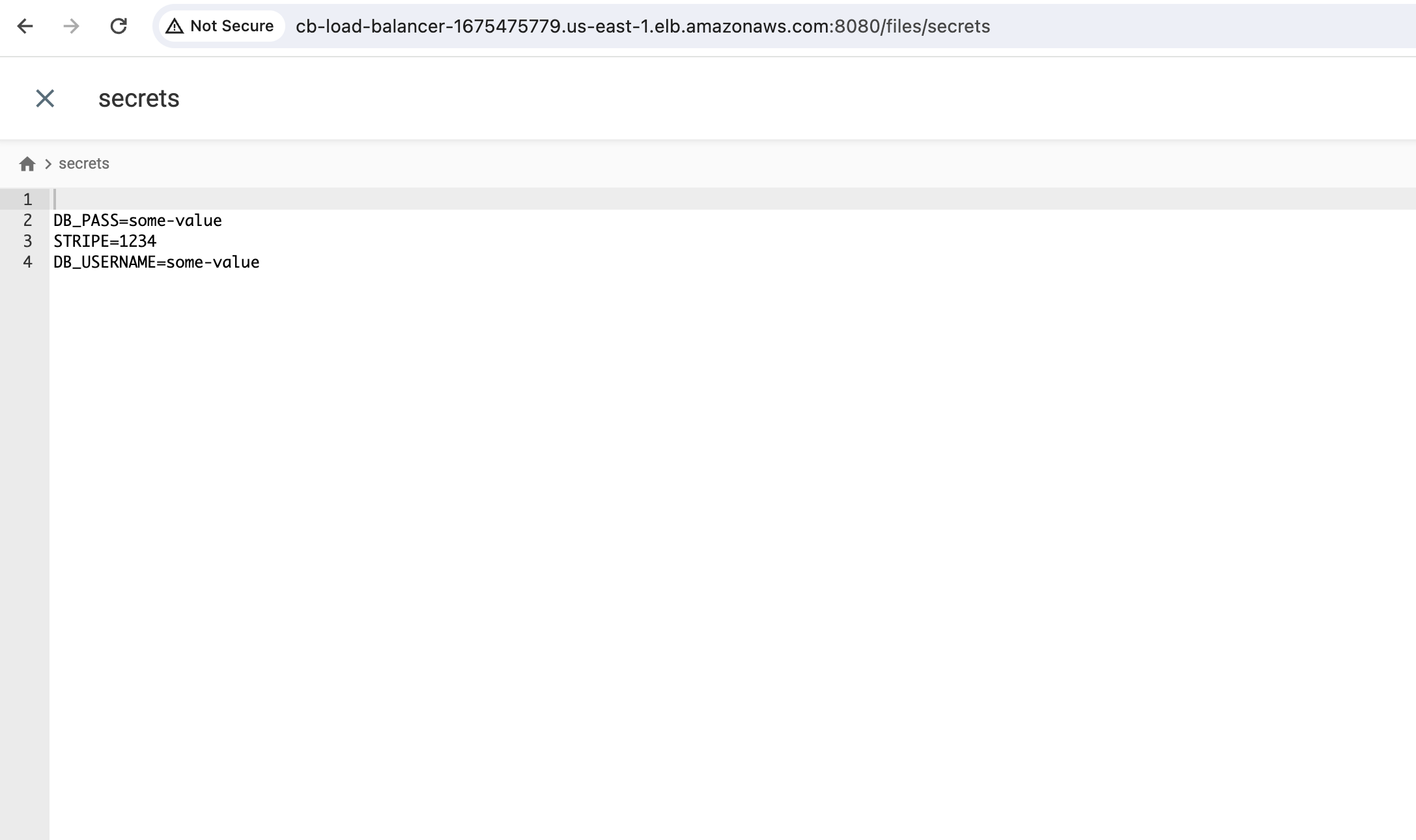
# Infisical Agent
This page describes how to manage secrets using Infisical Agent.
Infisical Agent is a client daemon that simplifies the adoption of Infisical by providing a more scalable and user-friendly approach for applications to interact with Infisical.
It eliminates the need to modify application logic by enabling clients to decide how they want their secrets rendered through the use of templates.
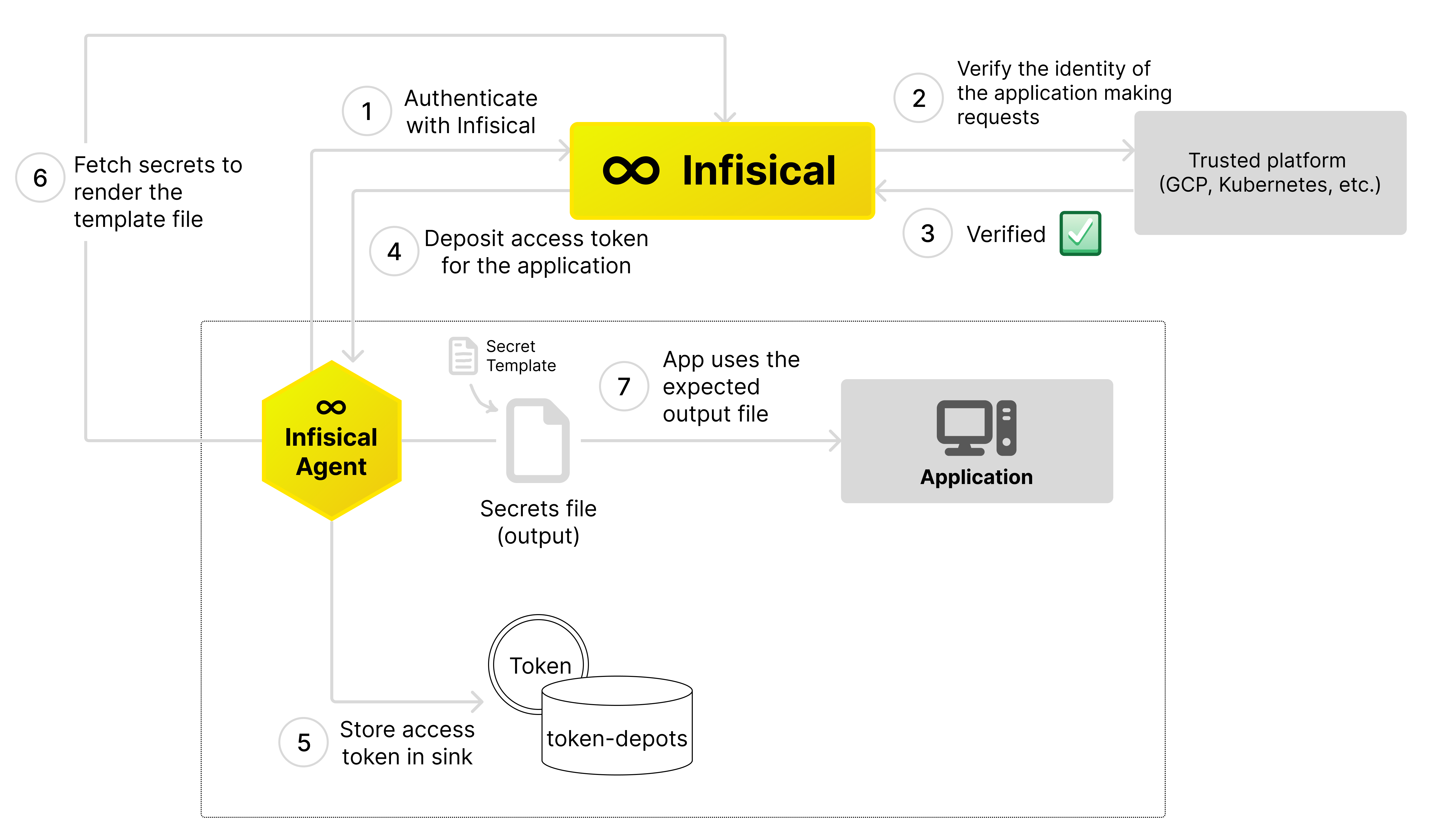
### Key features:
* Token renewal: Automatically authenticates with Infisical and deposits renewed access tokens at specified path for applications to consume
* Templating: Renders secrets via user provided templates to desired formats for applications to consume
### Token renewal
The Infisical agent can help manage the life cycle of access tokens. The token renewal process is split into two main components: a `Method`, which is the authentication process suitable for your current setup, and `Sinks`, which are the places where the agent deposits the new access token whenever it receives updates.
When the Infisical Agent is started, it will attempt to obtain a valid access token using the authentication method you have configured. If the agent is unable to fetch a valid token, the agent will keep trying, increasing the time between each attempt.
Once a access token is successfully fetched, the agent will make sure the access token stays valid, continuing to renew it before it expires.
Every time the agent successfully retrieves a new access token, it writes the new token to the Sinks you've configured.
Access tokens can be utilized with Infisical SDKs or directly in API requests
to retrieve secrets from Infisical
### Templating
The Infisical agent can help deliver formatted secrets to your application in a variety of environments. To achieve this, the agent will retrieve secrets from Infisical, format them using a specified template, and then save these formatted secrets to a designated file path.
Templating process is done through the use of Go language's [text/template feature](https://pkg.go.dev/text/template).You can refer to the available secret template functions [here](#available-secret-template-functions). Multiple template definitions can be set in the agent configuration file to generate a variety of formatted secret files.
When the agent is started and templates are defined in the agent configuration file, the agent will attempt to acquire a valid access token using the set authentication method outlined in the agent's configuration.
If this initial attempt is unsuccessful, the agent will momentarily pauses before continuing to make more attempts.
Once the agent successfully obtains a valid access token, the agent proceeds to fetch the secrets from Infisical using it.
It then formats these secrets using the user provided templates and writes the formatted data to configured file paths.
## Agent configuration file
To set up the authentication method for token renewal and to define secret templates, the Infisical agent requires a YAML configuration file containing properties defined below.
While specifying an authentication method is mandatory to start the agent, configuring sinks and secret templates are optional.
| Field | Description |
| ------------------------------------------ | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
| `infisical.address` | The URL of the Infisical service. Default: `"https://app.infisical.com"`. |
| `auth.type` | The type of authentication method used. Available options: `universal-auth`, `kubernetes`, `azure`, `gcp-id-token`, `gcp-iam`, `aws-iam` |
| `auth.config.identity-id` | The file path where the machine identity id is stored
This field is required when using any of the following auth types: `kubernetes`, `azure`, `gcp-id-token`, `gcp-iam`, or `aws-iam`. |
| `auth.config.service-account-token` | Path to the Kubernetes service account token to use (optional)
Default: `/var/run/secrets/kubernetes.io/serviceaccount/token` |
| `auth.config.service-account-key` | Path to your GCP service account key file. This field is required when using `gcp-iam` auth type.
Please note that the file should be in JSON format. |
| `auth.config.client-id` | The file path where the universal-auth client id is stored. |
| `auth.config.client-secret` | The file path where the universal-auth client secret is stored. |
| `auth.config.remove_client_secret_on_read` | This will instruct the agent to remove the client secret from disk. |
| `sinks[].type` | The type of sink in a list of sinks. Each item specifies a sink type. Currently, only `"file"` type is available. |
| `sinks[].config.path` | The file path where the access token should be stored for each sink in the list. |
| `templates[].source-path` | The path to the template file that should be used to render secrets. |
| `templates[].template-content` | The inline secret template to be used for rendering the secrets. |
| `templates[].destination-path` | The path where the rendered secrets from the source template will be saved to. |
| `templates[].config.polling-interval` | How frequently to check for secret changes. Default: `5 minutes` (optional) |
| `templates[].config.execute.command` | The command to execute when secret change is detected (optional) |
| `templates[].config.execute.timeout` | How long in seconds to wait for command to execute before timing out (optional) |
## Authentication
The Infisical agent supports multiple authentication methods. Below are the available authentication methods, with their respective configurations.
The Universal Auth method is a simple and secure way to authenticate with Infisical. It requires a client ID and a client secret to authenticate with Infisical.
Path to the file containing the universal auth client ID.
Path to the file containing the universal auth client secret.
Instructs the agent to remove the client secret from disk after reading
it.
To create a universal auth machine identity, follow the step by step guide outlined [here](/documentation/platform/identities/universal-auth).
Update the agent configuration file with the specified auth method, client ID, and client secret. In the snippet below you can see a sample configuration of the `auth` field when using the Universal Auth method.
```yaml example-auth-config.yaml
auth:
type: "universal-auth"
config:
client-id: "./client-id" # Path to the file containing the client ID
client-secret: "./client" # Path to the file containing the client secret
remove_client_secret_on_read: false # Optional field, instructs the agent to remove the client secret from disk after reading it
```
The Native Kubernetes method is used to authenticate with Infisical when running in a Kubernetes environment. It requires a service account token to authenticate with Infisical.
{" "}
Path to the file containing the machine identity ID.
Path to the Kubernetes service account token to use. Default:
`/var/run/secrets/kubernetes.io/serviceaccount/token`.
To create a Kubernetes machine identity, follow the step by step guide outlined [here](/documentation/platform/identities/kubernetes-auth).
Update the agent configuration file with the specified auth method, identity ID, and service account token. In the snippet below you can see a sample configuration of the `auth` field when using the Kubernetes method.
```yaml example-auth-config.yaml
auth:
type: "kubernetes"
config:
identity-id: "./identity-id" # Path to the file containing the machine identity ID
service-account-token: "/var/run/secrets/kubernetes.io/serviceaccount/token" # Optional field, custom path to the Kubernetes service account token to use
```
The Native Azure method is used to authenticate with Infisical when running in an Azure environment.
Path to the file containing the machine identity ID.
To create an Azure machine identity, follow the step by step guide outlined [here](/documentation/platform/identities/azure-auth).
Update the agent configuration file with the specified auth method and identity ID. In the snippet below you can see a sample configuration of the `auth` field when using the Azure method.
```yaml example-auth-config.yaml
auth:
type: "azure"
config:
identity-id: "./identity-id" # Path to the file containing the machine identity ID
```
The Native GCP ID Token method is used to authenticate with Infisical when running in a GCP environment.
Path to the file containing the machine identity ID.
To create a GCP machine identity, follow the step by step guide outlined [here](/documentation/platform/identities/gcp-auth).
Update the agent configuration file with the specified auth method and identity ID. In the snippet below you can see a sample configuration of the `auth` field when using the GCP ID Token method.
```yaml example-auth-config.yaml
auth:
type: "gcp-id-token"
config:
identity-id: "./identity-id" # Path to the file containing the machine identity ID
```
The GCP IAM method is used to authenticate with Infisical with a GCP service account key.
Path to the file containing the machine identity ID.
Path to your GCP service account key file.
To create a GCP machine identity, follow the step by step guide outlined [here](/documentation/platform/identities/gcp-auth).
Update the agent configuration file with the specified auth method, identity ID, and service account key. In the snippet below you can see a sample configuration of the `auth` field when using the GCP IAM method.
```yaml example-auth-config.yaml
auth:
type: "gcp-iam"
config:
identity-id: "./identity-id" # Path to the file containing the machine identity ID
service-account-key: "./service-account-key.json" # Path to your GCP service account key file
```
The AWS IAM method is used to authenticate with Infisical with an AWS IAM role while running in an AWS environment like EC2, Lambda, etc.
Path to the file containing the machine identity ID.
To create an AWS machine identity, follow the step by step guide outlined [here](/documentation/platform/identities/aws-auth).
Update the agent configuration file with the specified auth method and identity ID. In the snippet below you can see a sample configuration of the `auth` field when using the AWS IAM method.
```yaml example-auth-config.yaml
auth:
type: "aws-iam"
config:
identity-id: "./identity-id" # Path to the file containing the machine identity ID
```
## Quick start Infisical Agent
To install the Infisical agent, you must first install the [Infisical CLI](/cli/overview) in the desired environment where you'd like the agent to run. This is because the Infisical agent is a sub-command of the Infisical CLI.
Once you have the CLI installed, you will need to provision programmatic access for the agent via [Universal Auth](/documentation/platform/identities/universal-auth). To obtain a **Client ID** and a **Client Secret**, follow the step by step guide outlined [here](/documentation/platform/identities/universal-auth).
Next, create agent config file as shown below. The example agent configuration file defines the token authentication method, one sink location, and a secret template.
```yaml example-agent-config-file.yaml
infisical:
address: "https://app.infisical.com"
auth:
type: "universal-auth"
config:
client-id: "./client-id"
client-secret: "./client-secret"
remove_client_secret_on_read: false
sinks:
- type: "file"
config:
path: "/some/path/to/store/access-token/file-name"
templates:
- source-path: my-dot-ev-secret-template
destination-path: /some/path/.env
config:
polling-interval: 60s
execute:
timeout: 30
command: ./reload-app.sh
```
The secret template below will be used to render the secrets with the key and the value separated by `=` sign. You'll notice that a custom function named `secret` is used to fetch the secrets.
This function takes the following arguments: `secret "" "" ""`.
```text my-dot-ev-secret-template
{{- with secret "6553ccb2b7da580d7f6e7260" "dev" "/" }}
{{- range . }}
{{ .Key }}={{ .Value }}
{{- end }}
{{- end }}
```
After defining the agent configuration file, run the command below pointing to the path where the agent configuration file is located.
```bash
infisical agent --config example-agent-config-file.yaml
```
### Available secret template functions
```bash
listSecrets "" "environment-slug" "" ""
```
```bash example-template-usage-1
{{- with listSecrets "6553ccb2b7da580d7f6e7260" "dev" "/" `{"recursive": false, "expandSecretReferences": true}` }}
{{- range . }}
{{ .Key }}={{ .Value }}
{{- end }}
{{- end }}
```
```bash example-template-usage-2
{{- with secret "da8056c8-01e2-4d24-b39f-cb4e004b8d44" "staging" "/" `{"recursive": true, "expandSecretReferences": true}` }}
{{- range . }}
{{- if eq .SecretPath "/"}}
{{ .Key }}={{ .Value }}
{{- else}}
{{ .SecretPath }}/{{ .Key }}={{ .Value }}
{{- end}}
{{- end }}
{{- end }}
```
**Function name**: listSecrets
**Description**: This function can be used to render the full list of secrets within a given project, environment and secret path.
An optional JSON argument is also available. It includes the properties `recursive`, which defaults to false, and `expandSecretReferences`, which defaults to true and expands the returned secrets.
**Returns**: A single secret object with the following keys `Key, WorkspaceId, Value, SecretPath, Type, ID, and Comment`
```bash
getSecretByName "" "" "" ""
```
```bash example-template-usage
{{ with getSecretByName "d821f21d-aa90-453b-8448-8c78c1160a0e" "dev" "/" "POSTHOG_HOST"}}
{{ if .Value }}
password = "{{ .Value }}"
{{ end }}
{{ end }}
```
**Function name**: getSecretByName
**Description**: This function can be used to render a single secret by it's name.
**Returns**: A list of secret objects with the following keys `Key, WorkspaceId, Value, Type, ID, and Comment`
# Kubernetes Operator
How to use Infisical to inject secrets into Kubernetes clusters.
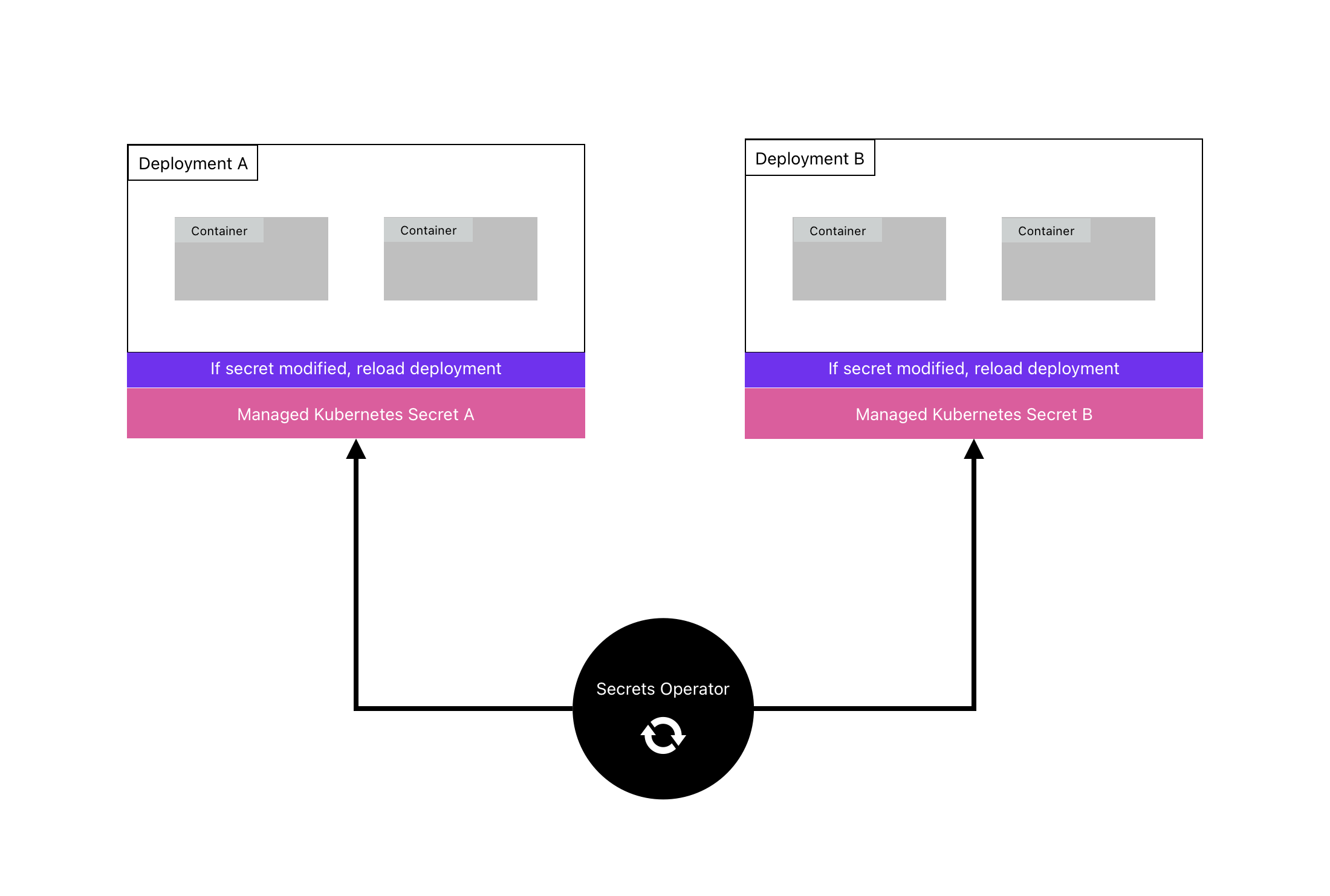
The Infisical Secrets Operator is a Kubernetes controller that retrieves secrets from Infisical and stores them in a designated cluster.
It uses an `InfisicalSecret` resource to specify authentication and storage methods.
The operator continuously updates secrets and can also reload dependent deployments automatically.
If you are already using the External Secrets operator, you can view the
integration documentation for it
[here](https://external-secrets.io/latest/provider/infisical/).
## Install Operator
The operator can be install via [Helm](https://helm.sh) or [kubectl](https://github.com/kubernetes/kubectl)
**Install the latest Infisical Helm repository**
```bash
helm repo add infisical-helm-charts 'https://dl.cloudsmith.io/public/infisical/helm-charts/helm/charts/'
helm repo update
```
**Install the Helm chart**
To select a specific version, view the application versions [here](https://hub.docker.com/r/infisical/kubernetes-operator/tags) and chart versions [here](https://cloudsmith.io/~infisical/repos/helm-charts/packages/detail/helm/secrets-operator/#versions)
```bash
helm install --generate-name infisical-helm-charts/secrets-operator
```
```bash
# Example installing app version v0.2.0 and chart version 0.1.4
helm install --generate-name infisical-helm-charts/secrets-operator --version=0.1.4 --set controllerManager.manager.image.tag=v0.2.0
```
For production deployments, it is highly recommended to set the version of the Kubernetes operator manually instead of pointing to the latest version.
Doing so will help you avoid accidental updates to the newest release which may introduce unintended breaking changes. View all application versions [here](https://hub.docker.com/r/infisical/kubernetes-operator/tags).
The command below will install the most recent version of the Kubernetes operator.
However, to set the version manually, download the manifest and set the image tag version of `infisical/kubernetes-operator` according to your desired version.
Once you apply the manifest, the operator will be installed in `infisical-operator-system` namespace.
```
kubectl apply -f https://raw.githubusercontent.com/Infisical/infisical/main/k8-operator/kubectl-install/install-secrets-operator.yaml
```
## Sync Infisical Secrets to your cluster
Once you have installed the operator to your cluster, you'll need to create a `InfisicalSecret` custom resource definition (CRD).
```yaml example-infisical-secret-crd.yaml
apiVersion: secrets.infisical.com/v1alpha1
kind: InfisicalSecret
metadata:
name: infisicalsecret-sample
labels:
label-to-be-passed-to-managed-secret: sample-value
annotations:
example.com/annotation-to-be-passed-to-managed-secret: "sample-value"
spec:
hostAPI: https://app.infisical.com/api
resyncInterval: 10
authentication:
# Make sure to only have 1 authentication method defined, serviceToken/universalAuth.
# If you have multiple authentication methods defined, it may cause issues.
# (Deprecated) Service Token Auth
serviceToken:
serviceTokenSecretReference:
secretName: service-token
secretNamespace: default
secretsScope:
envSlug:
secretsPath:
recursive: true
# Universal Auth
universalAuth:
secretsScope:
projectSlug: new-ob-em
envSlug: dev # "dev", "staging", "prod", etc..
secretsPath: "/" # Root is "/"
recursive: true # Wether or not to use recursive mode (Fetches all secrets in an environment from a given secret path, and all folders inside the path) / defaults to false
credentialsRef:
secretName: universal-auth-credentials
secretNamespace: default
# Native Kubernetes Auth
kubernetesAuth:
identityId:
serviceAccountRef:
name:
namespace:
# secretsScope is identical to the secrets scope in the universalAuth field in this sample.
secretsScope:
projectSlug: your-project-slug
envSlug: prod
secretsPath: "/path"
recursive: true
# AWS IAM Auth
awsIamAuth:
identityId:
# secretsScope is identical to the secrets scope in the universalAuth field in this sample.
secretsScope:
projectSlug: your-project-slug
envSlug: prod
secretsPath: "/path"
recursive: true
# Azure Auth
azureAuth:
identityId:
resource: https://management.azure.com/&client_id=CLIENT_ID # (Optional) This is the Azure resource that you want to access. For example, "https://management.azure.com/". If no value is provided, it will default to "https://management.azure.com/"
# secretsScope is identical to the secrets scope in the universalAuth field in this sample.
secretsScope:
projectSlug: your-project-slug
envSlug: prod
secretsPath: "/path"
recursive: true
# GCP ID Token Auth
gcpIdTokenAuth:
identityId:
# secretsScope is identical to the secrets scope in the universalAuth field in this sample.
secretsScope:
projectSlug: your-project-slug
envSlug: prod
secretsPath: "/path"
recursive: true
# GCP IAM Auth
gcpIamAuth:
identityId:
# secretsScope is identical to the secrets scope in the universalAuth field in this sample.
secretsScope:
projectSlug: your-project-slug
envSlug: prod
secretsPath: "/path"
recursive: true
managedSecretReference:
secretName: managed-secret
secretNamespace: default
creationPolicy: "Orphan" ## Owner | Orphan
# secretType: kubernetes.io/dockerconfigjson
```
### InfisicalSecret CRD properties
If you are fetching secrets from a self-hosted instance of Infisical set the value of `hostAPI` to
` https://your-self-hosted-instace.com/api`
When `hostAPI` is not defined the operator fetches secrets from Infisical Cloud.
If you have installed your Infisical instance within the same cluster as the Infisical operator, you can optionally access the Infisical backend's service directly without having to route through the public internet.
To achieve this, use the following address for the hostAPI field:
```bash
http://..svc.cluster.local:4000/api
```
Make sure to replace `` and `` with the appropriate values for your backend service and namespace.
This property defines the time in seconds between each secret re-sync from
Infisical. Shorter time between re-syncs will require higher rate limits only
available on paid plans. Default re-sync interval is every 1 minute.
This block defines the TLS settings to use for connecting to the Infisical
instance.
This block defines the reference to the CA certificate to use for connecting
to the Infisical instance with SSL/TLS.
The name of the Kubernetes secret containing the CA certificate to use for
connecting to the Infisical instance with SSL/TLS.
The namespace of the Kubernetes secret containing the CA certificate to use
for connecting to the Infisical instance with SSL/TLS.
The name of the key in the Kubernetes secret which contains the value of the
CA certificate to use for connecting to the Infisical instance with SSL/TLS.
This block defines the method that will be used to authenticate with Infisical
so that secrets can be fetched
The universal machine identity authentication method is used to authenticate with Infisical. The client ID and client secret needs to be stored in a Kubernetes secret. This block defines the reference to the name and namespace of secret that stores these credentials.
You need to create a machine identity, and give it access to the project(s) you want to interact with. You can [read more about machine identities here](/documentation/platform/identities/universal-auth).
Once you have created your machine identity and added it to your project(s), you will need to create a Kubernetes secret containing the identity credentials.
To quickly create a Kubernetes secret containing the identity credentials, you can run the command below.
Make sure you replace `` with the identity client ID and `` with the identity client secret.
```bash
kubectl create secret generic universal-auth-credentials --from-literal=clientId="" --from-literal=clientSecret=""
```
Once the secret is created, add the `secretName` and `secretNamespace` of the secret that was just created under `authentication.universalAuth.credentialsRef` field in the InfisicalSecret resource.
Make sure to also populate the `secretsScope` field with the project slug
*`projectSlug`*, environment slug *`envSlug`*, and secrets path
*`secretsPath`* that you want to fetch secrets from. Please see the example
below.
## Example
```yaml
apiVersion: secrets.infisical.com/v1alpha1
kind: InfisicalSecret
metadata:
name: infisicalsecret-sample-crd
spec:
authentication:
universalAuth:
secretsScope:
projectSlug: # <-- project slug
envSlug: # "dev", "staging", "prod", etc..
secretsPath: "" # Root is "/"
credentialsRef:
secretName: universal-auth-credentials # <-- name of the Kubernetes secret that stores our machine identity credentials
secretNamespace: default # <-- namespace of the Kubernetes secret that stores our machine identity credentials
...
```
The Kubernetes machine identity authentication method is used to authenticate with Infisical. The identity ID is stored in a field in the InfisicalSecret resource. This authentication method can only be used within a Kubernetes environment.
1.1. Start by creating a service account in your Kubernetes cluster that will be used by Infisical to authenticate with the Kubernetes API Server.
```yaml infisical-service-account.yaml
apiVersion: v1
kind: ServiceAccount
metadata:
name: infisical-auth
namespace: default
```
```
kubectl apply -f infisical-service-account.yaml
```
1.2. Bind the service account to the `system:auth-delegator` cluster role. As described [here](https://kubernetes.io/docs/reference/access-authn-authz/rbac/#other-component-roles), this role allows delegated authentication and authorization checks, specifically for Infisical to access the [TokenReview API](https://kubernetes.io/docs/reference/kubernetes-api/authentication-resources/token-review-v1/). You can apply the following configuration file:
```yaml cluster-role-binding.yaml
apiVersion: rbac.authorization.k8s.io/v1
kind: ClusterRoleBinding
metadata:
name: role-tokenreview-binding
namespace: default
roleRef:
apiGroup: rbac.authorization.k8s.io
kind: ClusterRole
name: system:auth-delegator
subjects:
- kind: ServiceAccount
name: infisical-auth
namespace: default
```
```
kubectl apply -f cluster-role-binding.yaml
```
1.3. Next, create a long-lived service account JWT token (i.e. the token reviewer JWT token) for the service account using this configuration file for a new `Secret` resource:
```yaml service-account-token.yaml
apiVersion: v1
kind: Secret
type: kubernetes.io/service-account-token
metadata:
name: infisical-auth-token
annotations:
kubernetes.io/service-account.name: "infisical-auth"
```
```
kubectl apply -f service-account-token.yaml
```
1.4. Link the secret in step 1.3 to the service account in step 1.1:
```bash
kubectl patch serviceaccount infisical-auth -p '{"secrets": [{"name": "infisical-auth-token"}]}' -n default
```
1.5. Finally, retrieve the token reviewer JWT token from the secret.
```bash
kubectl get secret infisical-auth-token -n default -o=jsonpath='{.data.token}' | base64 --decode
```
Keep this JWT token handy as you will need it for the **Token Reviewer JWT** field when configuring the Kubernetes Auth authentication method for the identity in step 2.
To create an identity, head to your Organization Settings > Access Control > Machine Identities and press **Create identity**.
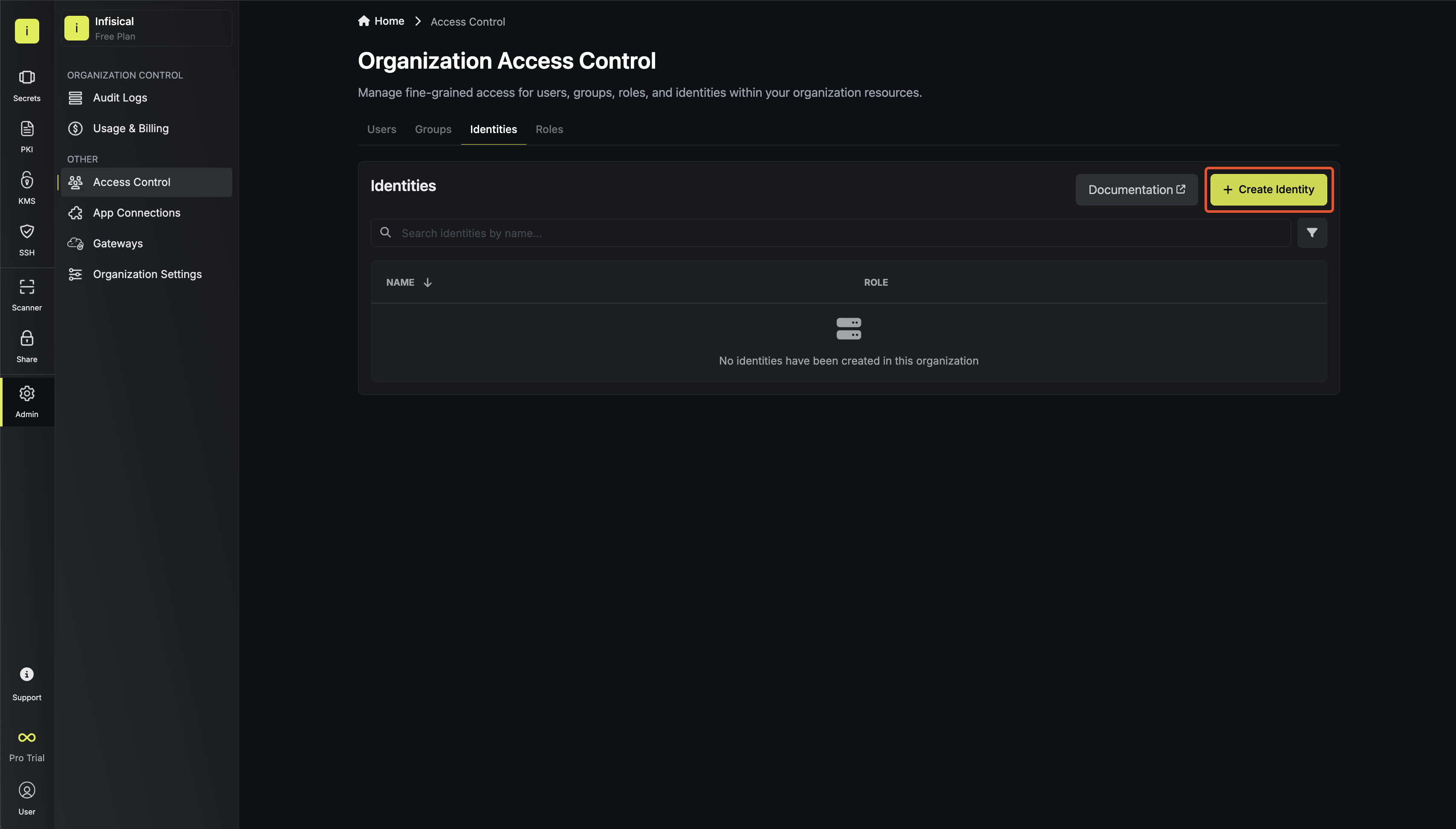
When creating an identity, you specify an organization level [role](/documentation/platform/role-based-access-controls) for it to assume; you can configure roles in Organization Settings > Access Control > Organization Roles.
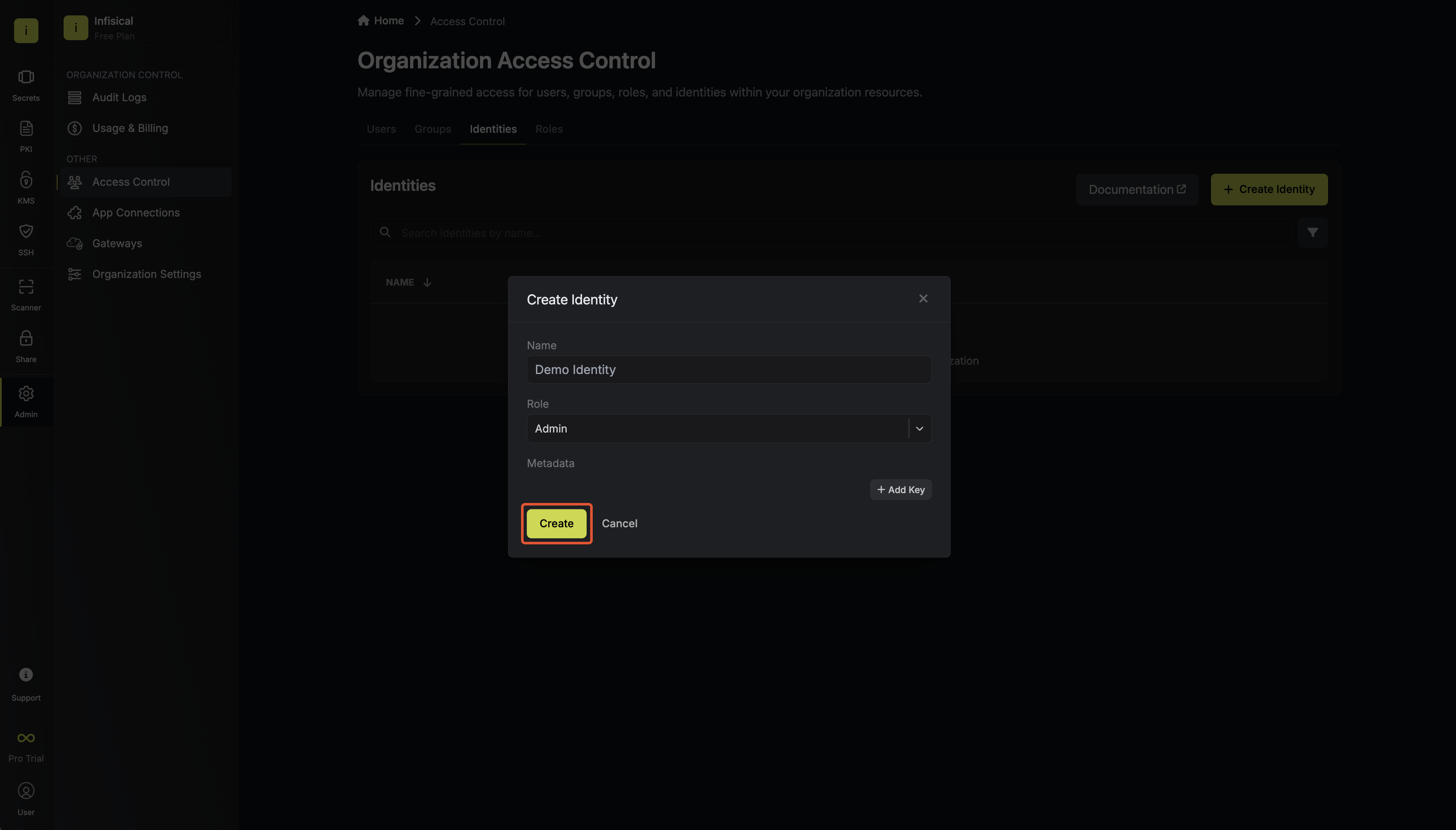
Now input a few details for your new identity. Here's some guidance for each field:
* Name (required): A friendly name for the identity.
* Role (required): A role from the **Organization Roles** tab for the identity to assume. The organization role assigned will determine what organization level resources this identity can have access to.
Once you've created an identity, you'll be prompted to configure the authentication method for it. Here, select **Kubernetes Auth**.
To learn more about each field of the Kubernetes native authentication method, see step 2 of [guide](/documentation/platform/identities/kubernetes-auth#guide).
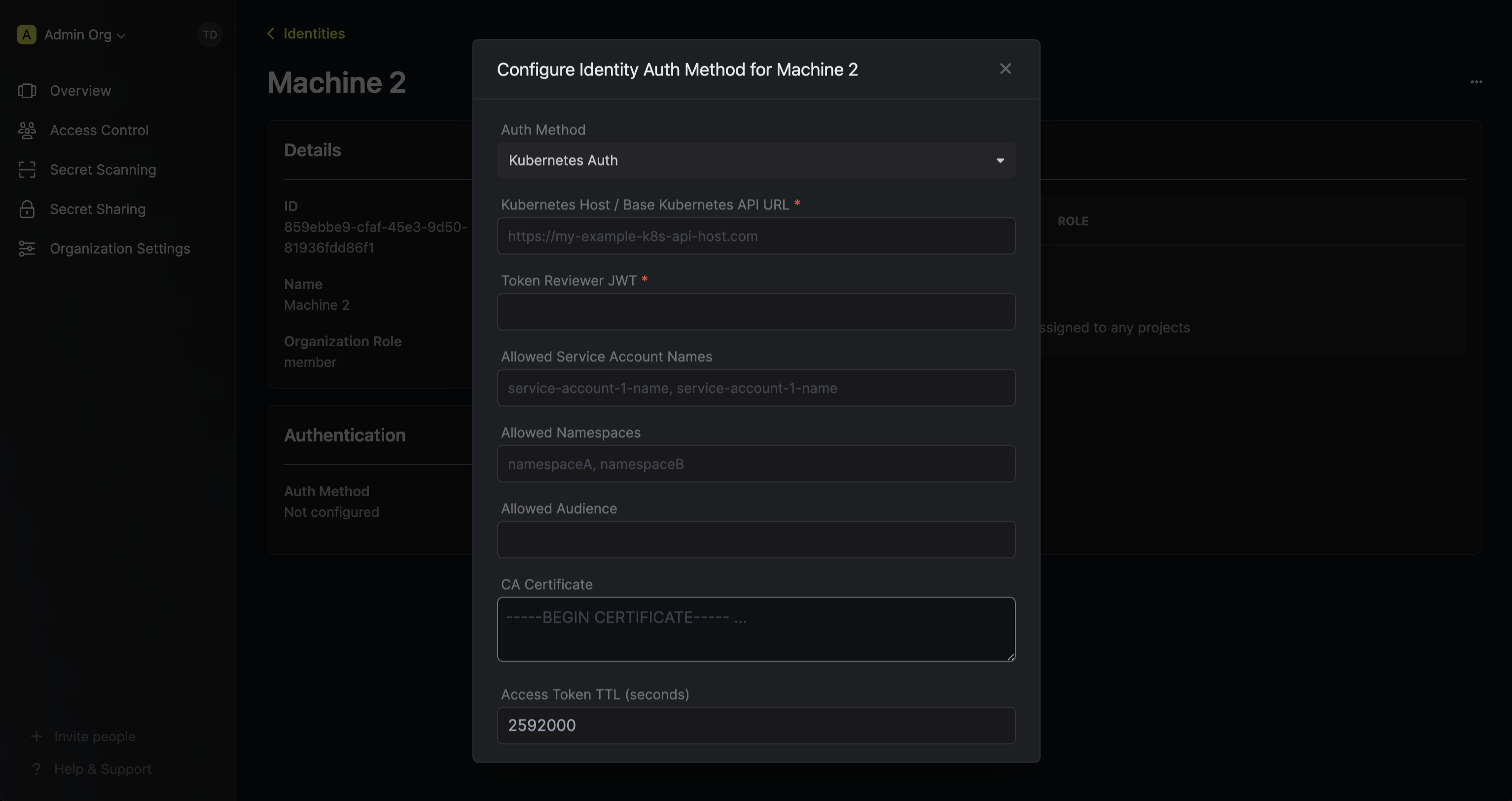
To allow the operator to use the given identity to access secrets, you will need to add the identity to project(s) that you would like to grant it access to.
To do this, head over to the project you want to add the identity to and go to Project Settings > Access Control > Machine Identities and press **Add identity**.
Next, select the identity you want to add to the project and the project level role you want to allow it to assume. The project role assigned will determine what project level resources this identity can have access to.
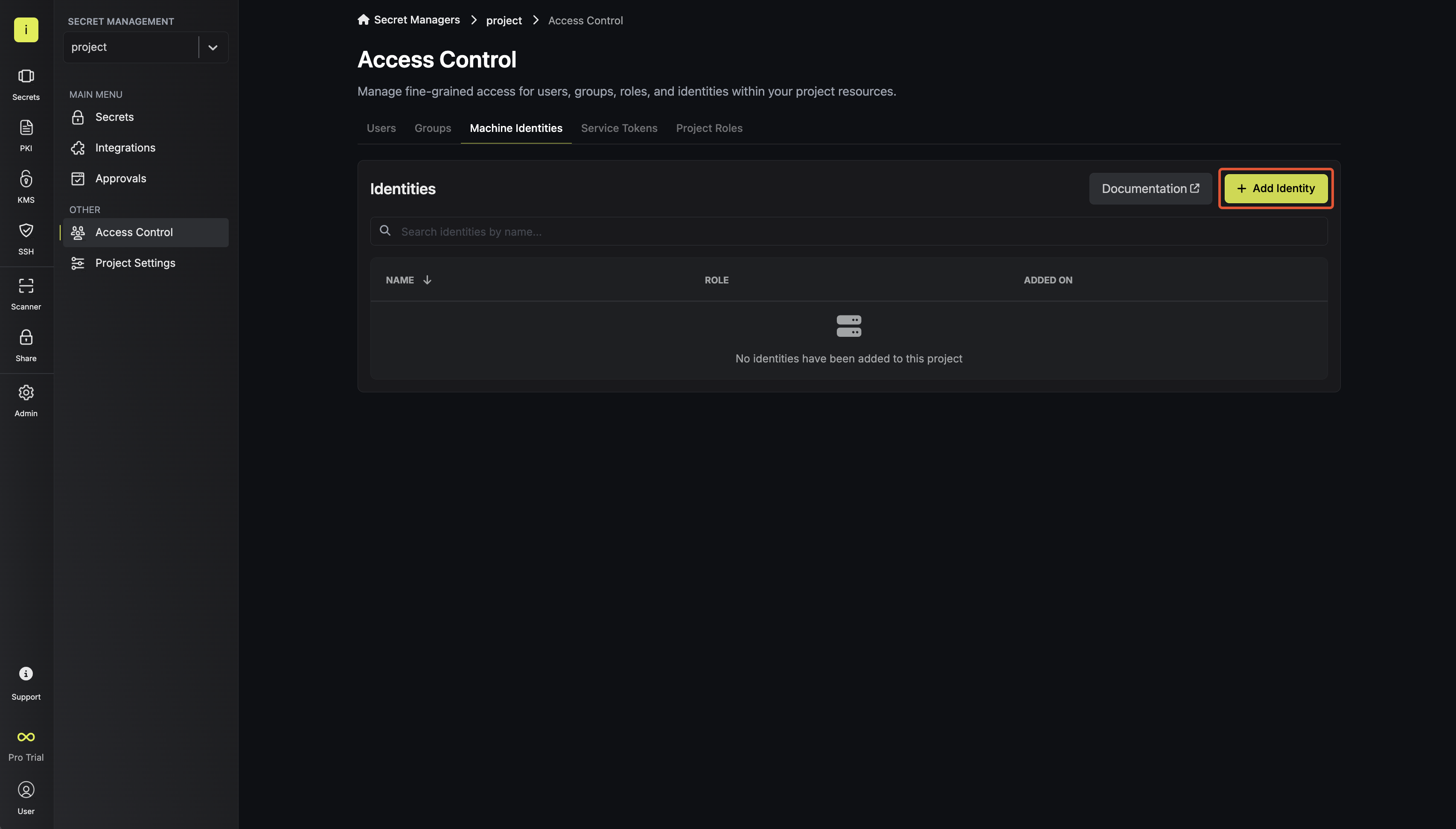
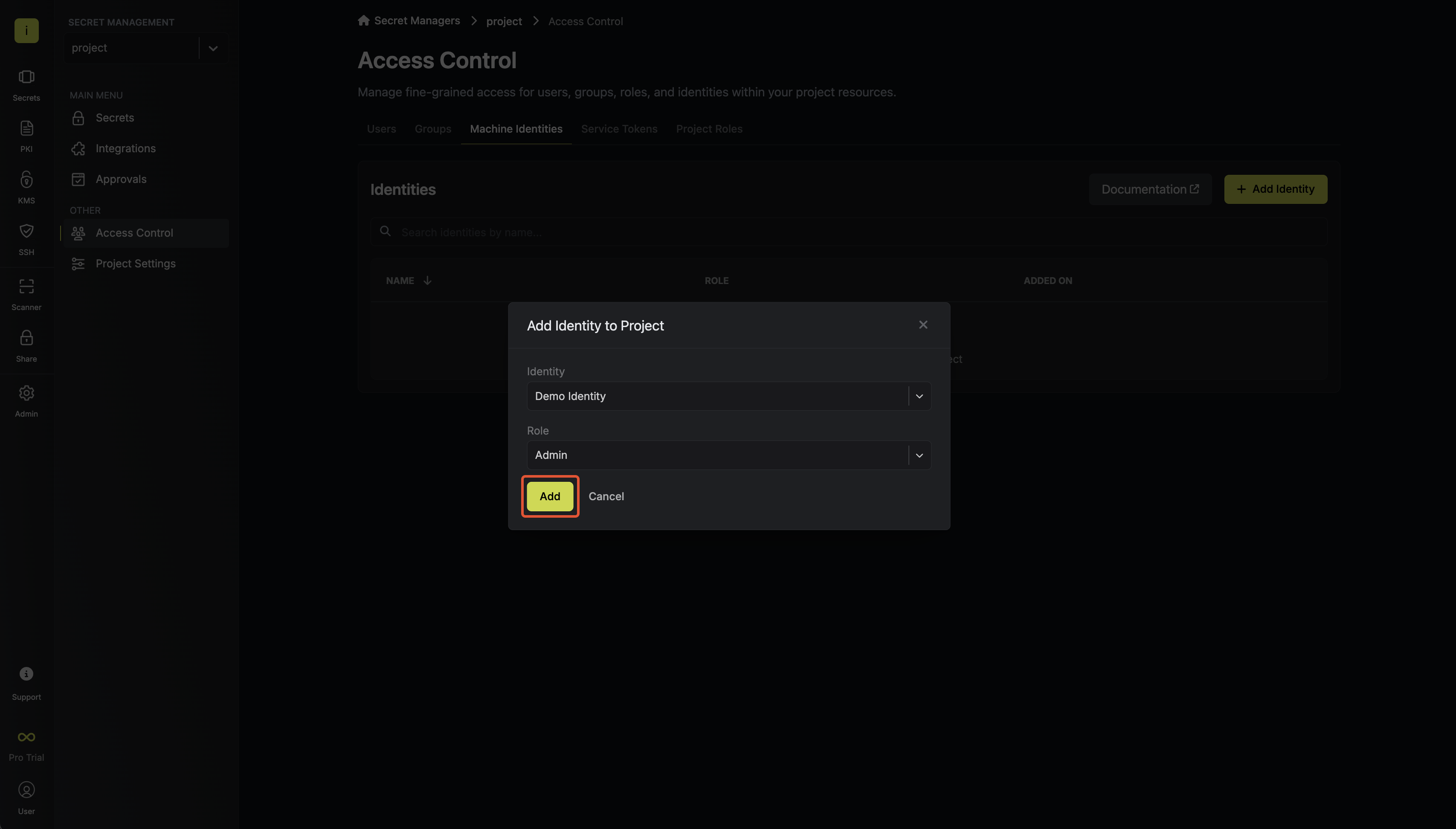
Once you have created your machine identity and added it to your project(s), you will need to add the identity ID to your InfisicalSecret resource.
In the `authentication.kubernetesAuth.identityId` field, add the identity ID of the machine identity you created.
See the example below for more details.
Add the service account details from the previous steps under `authentication.kubernetesAuth.serviceAccountRef`.
Here you will need to enter the name and namespace of the service account.
The example below shows a complete InfisicalSecret resource with all required fields defined.
Make sure to also populate the `secretsScope` field with the project slug
*`projectSlug`*, environment slug *`envSlug`*, and secrets path
*`secretsPath`* that you want to fetch secrets from. Please see the example
below.
## Example
```yaml example-kubernetes-auth.yaml
apiVersion: secrets.infisical.com/v1alpha1
kind: InfisicalSecret
metadata:
name: infisicalsecret-sample-crd
spec:
authentication:
kubernetesAuth:
identityId:
serviceAccountRef:
name:
namespace:
# secretsScope is identical to the secrets scope in the universalAuth field in this sample.
secretsScope:
projectSlug: your-project-slug
envSlug: prod
secretsPath: "/path"
recursive: true
...
```
The AWS IAM machine identity authentication method is used to authenticate with Infisical. The identity ID is stored in a field in the InfisicalSecret resource. This authentication method can only be used within an AWS environment like an EC2 or a Lambda function.
You need to create a machine identity, and give it access to the project(s) you want to interact with. You can [read more about AWS machine identities here](/documentation/platform/identities/aws-auth).
Once you have created your machine identity and added it to your project(s), you will need to add the identity ID to your InfisicalSecret resource. In the `authentication.awsIamAuth.identityId` field, add the identity ID of the machine identity you created. See the example below for more details.
Make sure to also populate the `secretsScope` field with the project slug
*`projectSlug`*, environment slug *`envSlug`*, and secrets path
*`secretsPath`* that you want to fetch secrets from. Please see the example
below.
## Example
```yaml example-aws-iam-auth.yaml
apiVersion: secrets.infisical.com/v1alpha1
kind: InfisicalSecret
metadata:
name: infisicalsecret-sample-crd
spec:
authentication:
awsIamAuth:
identityId:
# secretsScope is identical to the secrets scope in the universalAuth field in this sample.
secretsScope:
projectSlug: your-project-slug
envSlug: prod
secretsPath: "/path"
recursive: true
...
```
The Azure machine identity authentication method is used to authenticate with Infisical. The identity ID is stored in a field in the InfisicalSecret resource. This authentication method can only be used within an Azure environment.
You need to create a machine identity, and give it access to the project(s) you want to interact with. You can [read more about Azure machine identities here](/documentation/platform/identities/azure-auth).
Once you have created your machine identity and added it to your project(s), you will need to add the identity ID to your InfisicalSecret resource. In the `authentication.azureAuth.identityId` field, add the identity ID of the machine identity you created. See the example below for more details.
Make sure to also populate the `secretsScope` field with the project slug
*`projectSlug`*, environment slug *`envSlug`*, and secrets path
*`secretsPath`* that you want to fetch secrets from. Please see the example
below.
## Example
```yaml example-azure-auth.yaml
apiVersion: secrets.infisical.com/v1alpha1
kind: InfisicalSecret
metadata:
name: infisicalsecret-sample-crd
spec:
authentication:
azureAuth:
identityId:
# secretsScope is identical to the secrets scope in the universalAuth field in this sample.
secretsScope:
projectSlug: your-project-slug
envSlug: prod
secretsPath: "/path"
recursive: true
...
```
The GCP ID Token machine identity authentication method is used to authenticate with Infisical. The identity ID is stored in a field in the InfisicalSecret resource. This authentication method can only be used within GCP environments.
You need to create a machine identity, and give it access to the project(s) you want to interact with. You can [read more about GCP machine identities here](/documentation/platform/identities/gcp-auth).
Once you have created your machine identity and added it to your project(s), you will need to add the identity ID to your InfisicalSecret resource. In the `authentication.gcpIdTokenAuth.identityId` field, add the identity ID of the machine identity you created. See the example below for more details.
Make sure to also populate the `secretsScope` field with the project slug
*`projectSlug`*, environment slug *`envSlug`*, and secrets path
*`secretsPath`* that you want to fetch secrets from. Please see the example
below.
## Example
```yaml example-gcp-id-token-auth.yaml
apiVersion: secrets.infisical.com/v1alpha1
kind: InfisicalSecret
metadata:
name: infisicalsecret-sample-crd
spec:
authentication:
gcpIdTokenAuth:
identityId:
# secretsScope is identical to the secrets scope in the universalAuth field in this sample.
secretsScope:
projectSlug: your-project-slug
envSlug: prod
secretsPath: "/path"
recursive: true
...
```
The GCP IAM machine identity authentication method is used to authenticate with Infisical. The identity ID is stored in a field in the InfisicalSecret resource. This authentication method can only be used both within and outside GCP environments.
You need to create a machine identity, and give it access to the project(s) you want to interact with. You can [read more about GCP machine identities here](/documentation/platform/identities/gcp-auth).
Once you have created your machine identity and added it to your project(s), you will need to add the identity ID to your InfisicalSecret resource. In the `authentication.gcpIamAuth.identityId` field, add the identity ID of the machine identity you created.
You'll also need to add the service account key file path to your InfisicalSecret resource. In the `authentication.gcpIamAuth.serviceAccountKeyFilePath` field, add the path to your service account key file path. Please see the example below for more details.
Make sure to also populate the `secretsScope` field with the project slug
*`projectSlug`*, environment slug *`envSlug`*, and secrets path
*`secretsPath`* that you want to fetch secrets from. Please see the example
below.
## Example
```yaml example-gcp-id-token-auth.yaml
apiVersion: secrets.infisical.com/v1alpha1
kind: InfisicalSecret
metadata:
name: infisicalsecret-sample-crd
spec:
authentication:
gcpIamAuth:
identityId:
serviceAccountKeyFilePath: "/path/to-service-account-key-file-path.json"
# secretsScope is identical to the secrets scope in the universalAuth field in this sample.
secretsScope:
projectSlug: your-project-slug
envSlug: prod
secretsPath: "/path"
recursive: true
...
```
The service token required to authenticate with Infisical needs to be stored in a Kubernetes secret. This block defines the reference to the name and namespace of secret that stores this service token.
Follow the instructions below to create and store the service token in a Kubernetes secrets and reference it in your CRD.
#### 1. Generate service token
You can generate a [service token](../../documentation/platform/token) for an Infisical project by heading over to the Infisical dashboard then to Project Settings.
#### 2. Create Kubernetes secret containing service token
Once you have generated the service token, you will need to create a Kubernetes secret containing the service token you generated.
To quickly create a Kubernetes secret containing the generated service token, you can run the command below. Make sure you replace `` with your service token.
```bash
kubectl create secret generic service-token --from-literal=infisicalToken=""
```
#### 3. Add reference for the Kubernetes secret containing service token
Once the secret is created, add the name and namespace of the secret that was just created under `authentication.serviceToken.serviceTokenSecretReference` field in the InfisicalSecret resource.
{" "}
Make sure to also populate the `secretsScope` field with the, environment slug
*`envSlug`*, and secrets path *`secretsPath`* that you want to fetch secrets
from. Please see the example below.
## Example
```yaml
apiVersion: secrets.infisical.com/v1alpha1
kind: InfisicalSecret
metadata:
name: infisicalsecret-sample-crd
spec:
authentication:
serviceToken:
serviceTokenSecretReference:
secretName: service-token # <-- name of the Kubernetes secret that stores our service token
secretNamespace: option # <-- namespace of the Kubernetes secret that stores our service token
secretsScope:
envSlug: # "dev", "staging", "prod", etc..
secretsPath: # Root is "/"
...
```
The `managedSecretReference` field is used to define the target location for storing secrets retrieved from an Infisical project.
This field requires specifying both the name and namespace of the Kubernetes secret that will hold these secrets.
The Infisical operator will automatically create the Kubernetes secret with the specified name/namespace and keep it continuously updated.
Note: The managed secret be should be created in the same namespace as the deployment that will use it.
The name of the managed Kubernetes secret to be created
The namespace of the managed Kubernetes secret to be created.
Override the default Opaque type for managed secrets with this field. Useful for creating kubernetes.io/dockerconfigjson secrets.
Creation polices allow you to control whether or not owner references should be added to the managed Kubernetes secret that is generated by the Infisical operator.
This is useful for tools such as ArgoCD, where every resource requires an owner reference; otherwise, it will be pruned automatically.
#### Available options
* `Orphan` (default)
* `Owner`
When creation policy is set to `Owner`, the `InfisicalSecret` CRD must be in
the same namespace as where the managed kubernetes secret.
### Propagating labels & annotations
The operator will transfer all labels & annotations present on the `InfisicalSecret` CRD to the managed Kubernetes secret to be created.
Thus, if a specific label is required on the resulting secret, it can be applied as demonstrated in the following example:
```yaml
apiVersion: secrets.infisical.com/v1alpha1
kind: InfisicalSecret
metadata:
name: infisicalsecret-sample
labels:
label-to-be-passed-to-managed-secret: sample-value
annotations:
example.com/annotation-to-be-passed-to-managed-secret: "sample-value"
spec:
..
authentication:
...
managedSecretReference:
...
```
This would result in the following managed secret to be created:
```yaml
apiVersion: v1
data: ...
kind: Secret
metadata:
annotations:
example.com/annotation-to-be-passed-to-managed-secret: sample-value
secrets.infisical.com/version: W/"3f1-ZyOSsrCLGSkAhhCkY2USPu2ivRw"
labels:
label-to-be-passed-to-managed-secret: sample-value
name: managed-token
namespace: default
type: Opaque
```
### Apply the Infisical CRD to your cluster
Once you have configured the Infisical CRD with the required fields, you can apply it to your cluster.
After applying, you should notice that the managed secret has been created in the desired namespace your specified.
```
kubectl apply -f example-infisical-secret-crd.yaml
```
### Verify managed secret creation
To verify that the operator has successfully created the managed secret, you can check the secrets in the namespace that was specified.
```bash
# Verify managed secret is created
kubectl get secrets -n
```
The Infisical secrets will be synced and stored into the managed secret every
1 minutes.
### Using managed secret in your deployment
Incorporating the managed secret created by the operator into your deployment can be achieved through several methods.
Here, we will highlight three of the most common ways to utilize it. Learn more about Kubernetes secrets [here](https://kubernetes.io/docs/concepts/configuration/secret/)
This will take all the secrets from your managed secret and expose them to your container
````yaml
envFrom:
- secretRef:
name: managed-secret # managed secret name
```
Example usage in a deployment
```yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: nginx-deployment
labels:
app: nginx
spec:
replicas: 1
selector:
matchLabels:
app: nginx
template:
metadata:
labels:
app: nginx
spec:
containers:
- name: nginx
image: nginx:1.14.2
envFrom:
- secretRef:
name: managed-secret # <- name of managed secret
ports:
- containerPort: 80
````
This will allow you to select individual secrets by key name from your managed secret and expose them to your container
```yaml
env:
- name: SECRET_NAME # The environment variable's name which is made available in the container
valueFrom:
secretKeyRef:
name: managed-secret # managed secret name
key: SOME_SECRET_KEY # The name of the key which exists in the managed secret
```
Example usage in a deployment
```yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: nginx-deployment
labels:
app: nginx
spec:
replicas: 1
selector:
matchLabels:
app: nginx
template:
metadata:
labels:
app: nginx
spec:
containers: - name: nginx
image: nginx:1.14.2
env: - name: STRIPE_API_SECRET
valueFrom:
secretKeyRef:
name: managed-secret # <- name of managed secret
key: STRIPE_API_SECRET
ports: - containerPort: 80
```
This will allow you to create a volume on your container which comprises of files holding the secrets in your managed kubernetes secret
```yaml
volumes:
- name: secrets-volume-name # The name of the volume under which secrets will be stored
secret:
secretName: managed-secret # managed secret name
```
You can then mount this volume to the container's filesystem so that your deployment can access the files containing the managed secrets
```yaml
volumeMounts:
- name: secrets-volume-name
mountPath: /etc/secrets
readOnly: true
```
Example usage in a deployment
```yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: nginx-deployment
labels:
app: nginx
spec:
replicas: 1
selector:
matchLabels:
app: nginx
template:
metadata:
labels:
app: nginx
spec:
containers:
- name: nginx
image: nginx:1.14.2
volumeMounts:
- name: secrets-volume-name
mountPath: /etc/secrets
readOnly: true
ports:
- containerPort: 80
volumes:
- name: secrets-volume-name
secret:
secretName: managed-secret # <- managed secrets
```
### Connecting to instances with private/self-signed certificate
To connect to Infisical instances behind a private/self-signed certificate, you can configure the TLS settings in the `InfisicalSecret` CRD
to point to a CA certificate stored in a Kubernetes secret resource.
```yaml
---
spec:
hostAPI: https://app.infisical.com/api
resyncInterval: 10
tls:
caRef:
secretName: custom-ca-certificate
secretNamespace: default
key: ca.crt
authentication:
---
```
The definition file of the Kubernetes secret for the CA certificate can be structured like the following:
```yaml
apiVersion: v1
kind: Secret
metadata:
name: custom-ca-certificate
type: Opaque
stringData:
ca.crt: |
-----BEGIN CERTIFICATE-----
MIIEZzCCA0+gAwIBAgIUDk9+HZcMHppiNy0TvoBg8/aMEqIwDQYJKoZIhvcNAQEL
...
BQAwDTELMAkGA1UEChMCUEgwHhcNMjQxMDI1MTU0MjAzWhcNMjUxMDI1MjE0MjAz
-----END CERTIFICATE-----
```
## Auto redeployment
Deployments using managed secrets don't reload automatically on updates, so they may use outdated secrets unless manually redeployed.
To address this, we added functionality to automatically redeploy your deployment when its managed secret updates.
### Enabling auto redeploy
To enable auto redeployment you simply have to add the following annotation to the deployment that consumes a managed secret
```yaml
secrets.infisical.com/auto-reload: "true"
```
```yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: nginx-deployment
labels:
app: nginx
annotations:
secrets.infisical.com/auto-reload: "true" # <- redeployment annotation
spec:
replicas: 1
selector:
matchLabels:
app: nginx
template:
metadata:
labels:
app: nginx
spec:
containers:
- name: nginx
image: nginx:1.14.2
envFrom:
- secretRef:
name: managed-secret
ports:
- containerPort: 80
```
#### How it works
When a secret change occurs, the operator will check to see which deployments are using the operator-managed Kubernetes secret that received the update.
Then, for each deployment that has this annotation present, a rolling update will be triggered.
## Global configuration
To configure global settings that will apply to all instances of `InfisicalSecret`, you can define these configurations in a Kubernetes ConfigMap.
For example, you can configure all `InfisicalSecret` instances to fetch secrets from a single backend API without specifying the `hostAPI` parameter for each instance.
### Available global properties
| Property | Description | Default value |
| -------- | --------------------------------------------------------------------------------- | -------------------------------------------------------------- |
| hostAPI | If `hostAPI` in `InfisicalSecret` instance is left empty, this value will be used | [https://app.infisical.com/api](https://app.infisical.com/api) |
### Applying global configurations
All global configurations must reside in a Kubernetes ConfigMap named `infisical-config` in the namespace `infisical-operator-system`.
To apply global configuration to the operator, copy the following yaml into `infisical-config.yaml` file.
```yaml infisical-config.yaml
apiVersion: v1
kind: Namespace
metadata:
name: infisical-operator-system
---
apiVersion: v1
kind: ConfigMap
metadata:
name: infisical-config
namespace: infisical-operator-system
data:
hostAPI: https://example.com/api # <-- global hostAPI
```
Then apply this change via kubectl by running the following
```bash
kubectl apply -f infisical-config.yaml
```
## Troubleshoot operator
If the operator is unable to fetch secrets from the API, it will not affect the managed Kubernetes secret.
It will continue attempting to reconnect to the API indefinitely.
The InfisicalSecret resource uses the `status.conditions` field to report its current state and any errors encountered.
```yaml
$ kubectl get infisicalSecrets
NAME AGE
infisicalsecret-sample 12s
$ kubectl describe infisicalSecret infisicalsecret-sample
...
Spec:
...
Status:
Conditions:
Last Transition Time: 2022-12-18T04:29:09Z
Message: Infisical controller has located the Infisical token in provided Kubernetes secret
Reason: OK
Status: True
Type: secrets.infisical.com/LoadedInfisicalToken
Last Transition Time: 2022-12-18T04:29:10Z
Message: Failed to update secret because: 400 Bad Request
Reason: Error
Status: False
Type: secrets.infisical.com/ReadyToSyncSecrets
Events:
```
## Uninstall Operator
The managed secret created by the operator will not be deleted when the operator is uninstalled.
Install Infisical Helm repository
```bash
helm uninstall
```
```
kubectl delete -f https://raw.githubusercontent.com/Infisical/infisical/main/k8-operator/kubectl-install/install-secrets-operator.yaml
```
## Useful Articles
* [Managing secrets in OpenShift with Infisical](https://xphyr.net/post/infisical_ocp/)
# PM2
How to use Infisical to inject environment variables and secrets with PM2 into a Node.js app
Prerequisites:
* Set up and add envars to [Infisical Cloud](https://app.infisical.com)
* [Install the CLI](/cli/overview)
## Initialize Infisical for your Node.js app
```bash
# navigate to the root of your of your project
cd /path/to/project
# then initialize infisical
infisical init
```
## Create a bash or js script
```bash infisical-run.sh
infisical run -- npm start
```
```js infisical-run.js
const spawn = require("child_process").spawn;
const infisical = spawn("infisical", ["run", "--", "npm", "start"]);
infisical.stdout.on("data", (data) => console.log(`${data}`));
infisical.stderr.on("data", (data) => console.error(`${data}`));
```
## Start your application as usual but with the script
```bash infisical-run.sh
pm2 start infisical-run.sh
```
```bash infisical-run.js
pm2 start infisical-run.js
```
# Components
Infisical's components span multiple clients, an API, and a storage backend.
## Infisical API
The Infisical API (sometimes referred to as the **backend**) contains the core platform logic.
## Storage backend
Infisical relies on a storage backend to store data including users and secrets. Infisical's storage backend is Postgres.
## Redis
Infisical uses [Redis](https://redis.com) to enable more complex workflows including a queuing system to manage long running asynchronous tasks, cron jobs, as well as reliable cache for frequently used resources.
## Infisical Web UI
The Web UI is the browser-based portal that connects to the Infisical API.
## Infisical clients
Clients are any application or infrastructure that connecting to the Infisical API using one of the below methods:
* Public API: Making API requests directly to the Infisical API.
* Client SDK: A platform-specific library with method abstractions for working with secrets. Currently, there are three official SDKs: [Node SDK](https://infisical.com/docs/sdks/languages/node), [Python SDK](https://infisical.com/docs/sdks/languages/python), and [Java SDK](https://infisical.com/docs/sdks/languages/java).
* CLI: A terminal-based interface for interacting with the Infisical API.
* Kubernetes Operator: This operator retrieves secrets from Infisical and securely store
# Flows
Infisical's core flows have strong cryptographic underpinnings.
## Signup
When a user signs up for an account using email/password, they verify their email by correctly entering the 6-digit OTP code sent to it.
After this procedure, the user creates a password that is checked against strict requirements to ensure that it has sufficient entropy; this is critical because passwords have both authentication-related and cryptographic implications in Infisical. In accordance to the [secure remote password protocol (SRP)](https://en.wikipedia.org/wiki/Secure_Remote_Password_protocol), the password is used to generate a salt and X; this is kept handy on the client side.
Next, a few user-associated symmetric keys are generated for subsequent use:
* The password is transformed into a 256-bit symmetric key, called the generated key, using the [Argon2id](https://en.wikipedia.org/wiki/Argon2) key derivation function.
* A 256-bit symmetric key, called the protected key, is generated.
* A public-private key pair is generated.
The symmetric keys are used in sequence to encrypt the user’s private key:
* The protected key is used to encrypt the private key.
* The generated key is used to encrypt the protected key.
Finally, the encrypted private key, the protected key, salt, and X are sent to the Infisical API to be stored in the storage backend. Note that the top-level secret used to secure the user’s account and private key is their password. Therefore, it must be unknown to the Infisical API and strong by nature.
## Login
When a user logs in, they enter their password to authenticate with Infisical via SRP. If successful, the encrypted protected key and encrypted private key are returned to the client side.
The password is then used in reverse sequence to decrypt the private key:
* The password is transformed back into the generated key.
* The generated key is used to decrypt the encrypted protected key.
* The protected key is used to decrypt the encrypted private key.
The private key is stored on the client side and kept handy.
## Single sign-on
When a SSO authentication method like Google, GitHub, or SAML SSO is used to login or signup to Infisical, the process is identical to logging in with email/password except that it is contingent on first successfully logging in via the authentication provider. This means, for example, a user with Google SSO enabled must first log in with Google and then enter their password for Infisical to complete logging into the platform.
This approach implies that the user’s password assumes only the role of a master decryption key or secret. It also ensures that the authentication provider does not know this top-level secret, keeping the platform zero-knowledge as intended.
## Account recovery
When a user signs up for Infisical, they are issued a backup PDF containing a symmetric key that can be used to recover their account by decrypting a copy of that user’s private key; using the backup PDF is the only way to recover a user’s account in the event of a lockout - this is intentional by design of Infisical’s zero-knowledge architecture.
We strongly encourage all users to download, print, and keep their backup PDFs in a secure location.
## Secrets
In Infisical, secrets belong to environments in projects, and projects belong to organizations. Each project can be thought of as a vault and has its own symmetric key, called the project key. The project key is used to encrypt the secrets contained in that project.
Similar to each user’s private key, the project key is sensitive and must remain unknown to the server to preserve the zero-knowledge aspect of Infisical; knowledge of the project key would allow the server to decrypt the secrets of that project which would be undesirable if the server is compromised.
In order to preserve the zero-knowledge aspect of Infisical, each project key is encrypted on the client side before being sent to the server. More specifically, for each project, we make copies of its project key for each member of that project; each copy is encrypted under that member’s public key and only then sent off to the server for storage. A few relevant sequences:
* The initial member of a project generates its project key, encrypts it under their public key, and uploads it to the server for storage.
* When a new member is added to the project, an existing member of the project (e.g. the initial member) fetches their copy of the project key, decrypts that copy, encrypts it under the public key of the new member, and uploads it to the server for storage.
* When a member is removed from a project, their copy of the project key is hard deleted from the storage backend.
When dealing with secrets, this implies a specific sequence of decryption/encryption steps to fetch and create/update them. Assuming that we’re dealing with the Infisical Web UI, let’s start with fetching secrets which happens after the user logs in and selects a project:
* The user fetches encrypted secrets back to the client side.
* The user also fetches the encrypted project key, encrypted under their public key, for these secrets.
* The encrypted project key is decrypted by the user’s private key which is kept handy on the client side.
* The project key is finally used to decrypt the secrets belonging to the project.
* The secrets are displayed to the user in the Infisical Web UI.
Similarly, when a user creates/updates a secret, the reverse sequence is performed:
* The user fetches the encrypted project key, encrypted under the user’s public key.
* The project key is decrypted by the user’s private key which is kept handy on the client side.
* The user encrypts the new/updated secret under the project key.
* The user sends the new/updated secret to the server for storage.
These sequences are performed across various Infisical clients including the web UI, CLI, SDKs, and K8s operators when dealing with the Infisical API. They are also relevant in the implementations of Infisical’s versioning features like secret versions and snapshots.
## Native integrations
Previously, we mentioned that Infisical is zero-knowledge; this is partly true because Infisical can be used this way. Under certain circumstances, however, a user can explicitly share their copy of the project key with the server to enable more advanced features like native integrations.
The way a project key is shared with Infisical is via an abstraction that we call a bot. Each project has a bot with a public-private key pair generated on the server; the private key of each bot is symmetrically encrypted by the root encryption key of the server. This implies a few things:
* The server may partake in the sharing of project keys via its own public-private keys bound to each project bot.
* The server root encryption key must be kept secure.
With that, let’s discuss native integrations. A native integrations is a connection between Infisical and a target platform like GitHub, GitLab, or Vercel that allows secrets to be synced from Infisical to the target platform using its API. Since native integrations require secrets to be sent over in plaintext, they require the server to have access to the secrets. The sequence for how integrations are implemented is fairly simple:
* A user explicitly shares copy of the project key with the server via the Infisical Web UI. In this step, the user fetches the public key of the bot assigned to that project, encrypts the project key under that public key, and sends it back to the server.
* The user selects a target platform to integrate with their project and enters details such as the source environment within the project to send secrets from as well as the project and environment in the target platform to sync secrets to.
* The user creates the integration, triggering the first sync wherein Infisical decrypts the project’s key, uses it to decrypt the secrets of that project, and sends the secrets to the target platform.
* Finally, on any subsequent mutations applied to the source environment of an active integration, Infisical automatically triggers a re-sync to the target platform. This keeps Infisical as a ground source-of-truth for a team’s secrets.
## Resources
* For in depth details, consult the code.
* To get started with Infisical, try out the [Getting Started](https://infisical.com/docs/documentation/getting-started/introduction) overview.
# Overview
Read how Infisical works under the hood.
This section covers the internals of Infisical including its technical underpinnings, architecture, and security properties.
Knowledge of this section is recommended but not required to use Infisical. However, if you're operating Infisical, we recommend understanding the internals.
## Learn More
Learn about the fundamental parts of Infisical.
Find out more about the structure of core user flows in Infisical.
Read about most common security-related topics and questions.
Learn best practices for utilizing Infisical service tokens. Please note that service tokens are now deprecated and will be removed entirely in the future.
# Permissions
Infisical's permissions system provides granular access control.
## Overview
The Infisical permissions system is based on a role-based access control (RBAC) model. The system allows you to define roles and assign them to users and machines. Each role has a set of permissions that define what actions a user can perform.
Permissions are built on a subject-action-object model. The subject is the resource the permission is being applied to, the action is what the permission allows.
An example of a subject/action combination would be `secrets/read`. This permission allows the subject to read secrets.
Refer to the table below for a list of subjects and the actions they support.
## Subjects and Actions
Not all actions are applicable to all subjects. As an example, the
`secrets-rollback` subject only supports `read`, and `create` as actions.
While `secrets` support `read`, `create`, `edit`, `delete`.
| Subject | Actions |
| ------------------------- | ----------------------------------------------------------------------------------------------------------- |
| `role` | `read`, `create`, `edit`, `delete` |
| `member` | `read`, `create`, `edit`, `delete` |
| `groups` | `read`, `create`, `edit`, `delete` |
| `settings` | `read`, `create`, `edit`, `delete` |
| `integrations` | `read`, `create`, `edit`, `delete` |
| `webhooks` | `read`, `create`, `edit`, `delete` |
| `service-tokens` | `read`, `create`, `edit`, `delete` |
| `environments` | `read`, `create`, `edit`, `delete` |
| `tags` | `read`, `create`, `edit`, `delete` |
| `audit-logs` | `read`, `create`, `edit`, `delete` |
| `ip-allowlist` | `read`, `create`, `edit`, `delete` |
| `workspace` | `edit`, `delete` |
| `secrets` | `read`, `create`, `edit`, `delete` |
| `secret-folders` | `read`, `create`, `edit`, `delete` |
| `secret-imports` | `read`, `create`, `edit`, `delete` |
| `dynamic-secrets` | `read-root-credential`, `create-root-credential`, `edit-root-credential`, `delete-root-credential`, `lease` |
| `secret-rollback` | `read`, `create` |
| `secret-approval` | `read`, `create`, `edit`, `delete` |
| `secret-rotation` | `read`, `create`, `edit`, `delete` |
| `identity` | `read`, `create`, `edit`, `delete` |
| `certificate-authorities` | `read`, `create`, `edit`, `delete` |
| `certificates` | `read`, `create`, `edit`, `delete` |
| `certificate-templates` | `read`, `create`, `edit`, `delete` |
| `pki-alerts` | `read`, `create`, `edit`, `delete` |
| `pki-collections` | `read`, `create`, `edit`, `delete` |
| `kms` | `edit` |
| `cmek` | `read`, `create`, `edit`, `delete`, `encrypt`, `decrypt` |
Not all actions are applicable to all subjects. As an example, the `workspace`
subject only supports `read`, and `create` as actions. While `member` support
`read`, `create`, `edit`, `delete`.
| Subject | Actions |
| ------------------ | ---------------------------------- |
| `workspace` | `read`, `create` |
| `role` | `read`, `create`, `edit`, `delete` |
| `member` | `read`, `create`, `edit`, `delete` |
| `secret-scanning` | `read`, `create`, `edit`, `delete` |
| `settings` | `read`, `create`, `edit`, `delete` |
| `incident-account` | `read`, `create`, `edit`, `delete` |
| `sso` | `read`, `create`, `edit`, `delete` |
| `scim` | `read`, `create`, `edit`, `delete` |
| `ldap` | `read`, `create`, `edit`, `delete` |
| `groups` | `read`, `create`, `edit`, `delete` |
| `billing` | `read`, `create`, `edit`, `delete` |
| `identity` | `read`, `create`, `edit`, `delete` |
| `kms` | `read` |
## Inversion
Permission inversion allows you to explicitly deny actions instead of allowing them. This is supported for the following subjects:
* secrets
* secret-folders
* secret-imports
* dynamic-secrets
* cmek
When a permission is inverted, it changes from an "allow" rule to a "deny" rule. For example:
```typescript
// Regular permission - allows reading secrets
{
subject: "secrets",
action: ["read"]
}
// Inverted permission - denies reading secrets
{
subject: "secrets",
action: ["read"],
inverted: true
}
```
## Conditions
Conditions allow you to create more granular permissions by specifying criteria that must be met for the permission to apply. This is supported for the following subjects:
* secrets
* secret-folders
* secret-imports
* dynamic-secrets
### Properties
Conditions can be applied to the following properties:
* `environment`: Control access based on environment slugs
* `secretPath`: Control access based on secret paths
* `secretName`: Control access based on secret names
* `secretTags`: Control access based on tags (only supports \$in operator)
### Operators
The following operators are available for conditions:
| Operator | Description | Example |
| -------- | ---------------------------------- | ----------------------------------------------------- |
| `$eq` | Equal | `{ environment: { $eq: "production" } }` |
| `$ne` | Not equal | `{ environment: { $ne: "development" } }` |
| `$in` | Matches any value in array | `{ environment: { $in: ["staging", "production"] } }` |
| `$glob` | Pattern matching using glob syntax | `{ secretPath: { $glob: "/app/\*" } }` |
These details are especially useful if you're using the API to [create new project roles](../api-reference/endpoints/project-roles/create).
The rules outlined on this page, also apply when using our Terraform Provider to manage your Infisical project roles, or any other of our clients that manage project roles.
## Migrating from permission V1 to permission V2
When upgrading to V2 permissions (i.e. when moving from using the `permissions` to `permissions_v2` field in your Terraform configurations, or upgrading to the V2 permission API), you'll need to update your permission structure as follows:
Any permissions for `secrets` should be expanded to include equivalent permissions for:
* `secret-imports`
* `secret-folders` (except for read permissions)
* `dynamic-secrets`
For dynamic secrets, the actions need to be mapped differently:
* `read` → `read-root-credential`
* `create` → `create-root-credential`
* `edit` → `edit-root-credential` (also adds `lease` permission)
* `delete` → `delete-root-credential`
Example:
```hcl
# Old V1 configuration
resource "infisical_project_role" "example" {
name = "example"
permissions = [
{
subject = "secrets"
action = "read"
},
{
subject = "secrets"
action = "edit"
}
]
}
# New V2 configuration
resource "infisical_project_role" "example" {
name = "example"
permissions_v2 = [
# Original secrets permission
{
subject = "secrets"
action = ["read", "edit"]
inverted = false
},
# Add equivalent secret-imports permission
{
subject = "secret-imports"
action = ["read", "edit"]
inverted = false
},
# Add secret-folders permission (without read)
{
subject = "secret-folders"
action = ["edit"]
inverted = false
},
# Add dynamic-secrets permission with mapped actions
{
subject = "dynamic-secrets"
action = ["read-root-credential", "edit-root-credential", "lease"]
inverted = false
}
]
}
```
Note: When moving to V2 permissions, make sure to include all the necessary expanded permissions based on your original `secrets` permissions.
# Security
Infisical's security model includes many considerations and initiatives.
Given that Infisical is a secret management platform that manages sensitive data, the Infisical security model is very important.
The goal of Infisical's security model is to ensure the security and integrity of all of its managed data as well as all associated operations.
This means that data at rest and in transit must be secure from eavesdropping or tampering. All clients must be authenticated and authorized to access data. Additionally, all interactions must be auditable and traced uniquely back to their source.
## Threat model
Infisical’s threat model spans communication, storage, response mechanisms, failover strategies, and more.
* Eavesdropping on communications: Infisical ensures end-to-end encryption for all client interactions with the Infisical API.
* Tampering with data (at rest or in transit): Infisical implements data integrity checks to detect tampering. If inconsistencies are found, Infisical aborts transactions and raises alerts.
* Unauthorized access (lacking authentication/authorization): Infisical mandates rigorous authentication and authorization checks for all inbound requests; it also offers multi-factor authentication and role-based access controls.
* Actions without accountability: Infisical logs all project-level events, including policy updates, queries/mutations applied to secrets, and more. Every event is timestamped and information about actor, source (i.e. IP address, user-agent, etc.), and relevant metadata is included.
* Breach of data storage confidentiality: Infisical encrypts all stored secrets using proven cryptographic techniques such as AES-256-GCM for symmetric encryption.
* Loss of service availability or secret data due to failures: Infisical leverages the robust container orchestration capabilities of Kubernetes and the inherent high availability features of Bitnami MongoDB to ensure resilience and fault tolerance. By deploying multiple replicas of Infisical application on Kubernetes, operations can continue even if a single instance fails.
* Unrecognized suspicious activities: Infisical monitors for any anomalous activities such as authentication attempts from previously unseen sources.
* Unidentified system vulnerabilities: Infisical undergoes penetration tests and vulnerability assessments twice a year; we act on findings to bolster the system's defense mechanisms.
That said, Infisical does not consider the following as part of its threat model:
* Uncontrolled access to the storage mechanism: An attacker with unfettered access to the storage system can manipulate data in unpredictable ways, including erasing or tampering with stored secrets. Furthermore, the attacker could potentially implement state rollbacks to favor their objectives.
* Disclosure of secret presence: If an adversary gains read access to the storage backend, they might discern the existence of certain secrets, even if the actual contents remain encrypted and concealed.
* Runtime memory intrusion: An attacker with capabilities to probe the memory state of a live instance of Infisical can potentially compromise data confidentiality.
* Vulnerabilities in affiliated systems: Some functionality may rely on third-party services and dependencies. Security lapses in these dependencies can indirectly jeopardize the confidentiality or integrity of the secrets.
* Breaches via compromised clients: If a system or application accessing Infisical is compromised, and its credentials to the platform are exposed, an attacker might gain access at the privilege level of that compromised entity.
* Configuration tampering by administrators: Any configuration data, whether supplied through admin interfaces or configuration files, needs scrutiny. If an attacker can manipulate these configurations, it poses risks to data confidentiality and integrity.
* Physical access to deployment infrastructure: An attacker with physical access to the servers or infrastructure where Infisical is deployed can potentially compromise the system in ways that are challenging to guard against, such as direct hardware tampering or booting from malicious media.
* Social engineering attacks on personnel: Attacks that target personnel, tricking them into divulging sensitive information or performing compromising actions, fall outside the platform's direct defensive purview.
It's essential to note that while these points fall outside the platform's direct threat model, they still form crucial considerations for an overarching security strategy.
## External threat overview
Infisical's architecture consists of various systems:
* Infisical API
* Storage backend
* Redis
* Infisical Web UI
* Infisical clients
The Infisical API requires that the Infisical Web UI and all Infisical clients are authenticated and authorized for every inbound request. If using [Infisical Cloud](https://app.infisical.com), all traffic is routed through [Cloudflare](https://www.cloudflare.com) which enforces TLS and requires a minimum of TLS 1.2.
The Infisical API is untrusted by design when dealing with secrets. All secrets are encrypted/decrypted on the client-side before reaching the Infisical API by default; granting Infisical access to secrets afterward is optional and up to your organization.
The storage backend used by Infisical is also untrusted by design. All sensitive data is encrypted either symmetrically with AES-256-GCM or asymmetrically with x25519-xsalsa20-poly1305 prior to entering the storage backend, depending on the context either on the client-side or server-side. Moreover, Infisical communicates with the storage backend over TLS to provide an added layer of security.
## Internal threat overview
Within Infisical, a critical security concern is an attacker gaining access to sensitive data that they are not permitted to, especially if they already has some degree of access to the system. There are currently two authentication methods categories used by clients for where we apply robust authentication and authorization logic.
### JWT / API Key
This token category is used by users and included in requests made from the Infisical Web UI or elsewhere to the Infisical API.
Each token is authenticated against the API and mapped to an existing user in Infisical. If no existing user is found for the token, the request is rejected by the API. Each token assumes the permission set of the user that it is mapped to. For example, if a user corresponding to a token is not allowed access to a certain organization or project, then the token is also not be valid for any requests concerning those specific resources.
In the event of compromise, an attacker could use the token to impersonate the associated user and perform actions within the permission set of that user. While they could retrieve secrets for a project that the user is part of, they could not, however, decrypt secrets if the project follows Infisical's default zero-knowledge architecture. In any case, it would be critical for the user to invalidate this token and change their password immediately to prevent further unintended actions and consequences.
### Service token
This token category is provisioned by users for applications and infrastructure to perform secret operations against the Infisical API.
Each token is scoped to a project in Infisical and configurable with an expiration date and permission set (also known as **scopes**) for specific environment(s) and path(s) within them. For example, you may provision an application a service token to authenticate against the Infisical API and retrieve secrets from some `/environment-variables` path in the production environment of a project. If the token is tried for another project, environment, or path outside of its permission set, then it is rejected by the API.
It should also be noted that projects in Infisical can be configured to restrict service token access to specific IP addresses or CIDR ranges; this can be useful for limiting access to traffic coming from corporate networks.
In the event of compromise, an attacker could use a service token to access the secrets that it is provisioned for. It would be critical here for project administrator(s) to revoke the token immediately to prevent further unintended access to resources; it would also be advisable currently to transfer secrets to a new project where a new project key is created on the client-side.
## Cryptography
Infisical uses AES-256-GCM for symmetric encryption and x25519-xsalsa20-poly1305 for asymmetric encryption operations; asymmetric algorithms are implemented with the [TweetNaCl.js](https://tweetnacl.js.org/#/) library which has been well-audited and recommended for use by cybersecurity firm Cure53. Lastly, the secure remote password (SRP) implementation uses [jsrp](https://github.com/alax/jsrp) package for user authentication.
By default, Infisical employs a zero-knowledge-first approach to securely storing and sharing secrets.
* Each secret belongs to a project and is symmetrically encrypted by that project's unique key. Each member of a project is shared a copy of the project key, encrypted under their public key, when they are first invited to join the project.
Since these encryption operations occur on the client-side, the Infisical API is not able to view the value of any secret and the default zero-knowledge property of Infisical is retained; as you'd expect, it follows that decryption operations also occur on the client-side.
* An exception to the zero-knowledge property occurs when a member of a project explicitly shares that project's unique key with Infisical. It is often necessary to share the project key with Infisical in order to use features like native integrations and secret rotation that wouldn't be possible to offer otherwise.
## Infrastructure
### High availability
Infisical Cloud utilizes several strategies to ensure high availability, leveraging AWS services to maintain continuous operation and data integrity.
#### Multi-AZ AWS RDS
Infisical Cloud uses AWS Relational Database Service (RDS) with Multi-AZ deployments.
This configuration ensures that the database service is highly available and durable.
AWS RDS automatically provisions and maintains a synchronous standby replica of the database in a different Availability Zone (AZ).
This setup facilitates immediate failover to the standby in the event of an AZ failure, thereby ensuring that database operations can continue with minimal interruption.
The continuous backup and replication to the standby instance safeguard data against loss and ensure its availability even during system failures.
#### Multi-AZ ECS for Container Orchestration
Infisical Cloud leverages Amazon Elastic Container Service (ECS) in a Multi-AZ configuration for container orchestration.
This arrangement enables the management and operation of containers across multiple availability zones, increasing the application's fault tolerance.
Should there be an AZ failure, load is seamlessly sent to an operational AZ, thus minimizing downtime and preserving service availability.
#### Standby Regions for Regional Failover
To fight regional outages, secondary regions are always in standby mode and maintained with up-to-date configurations and data, ready to take over in case the primary region fails.
The standby regions enable a rapid transition and service continuity with minimal disruption in the event of a complete regional failure, ensuring that Infisical Cloud services remain accessible.
### Snapshots
A snapshot is a complete copy of data in the storage backend at a point in time.
If using [Infisical Cloud](https://app.infisical.com), snapshots of MongoDB databases are taken regularly; this can be enabled on your own storage backend as well.
### Offline usage
Many teams and organizations use the [Infisical CLI](https://infisical.com/docs/cli/overview) to fetch and inject secrets back from Infisical into their applications and infrastructure locally; the CLI has offline fallback capabilities.
If you have previously retrieved secrets for a specific project and environment, the `run/secret` command will utilize the saved secrets, even when offline, on subsequent fetch attempts to ensure that you always have access to secrets.
## Platform
### Web application
Infisical utilizes the latest HTTP security headers and employs a strict Content-Security-Policy to mitigate XSS.
JWT tokens are stored in browser memory and appended to outbound requests requiring authentication; refresh tokens are stored in `HttpOnly` cookies and included in future requests to `/api/token` for JWT token renewal.
### User authentication
Infisical supports several authentication methods including email/password, Google SSO, GitHub SSO, SAML 2.0 (Okta, Azure, JumpCloud), and OpenID Connect; Infisical also currently offers email-based 2FA with authenticator app methods coming in Q1 2024.
Infisical uses the [secure remote password protocol](https://en.wikipedia.org/wiki/Secure_Remote_Password_protocol#:~:text=The%20SRP%20protocol%20has%20a,the%20user%20to%20the%20server), commonly found in other zero-knowledge platform architectures, for authentication.
Put simply, the protocol enables Infisical to validate a user's knowledge of their password without ever seeing it by constructing a mutual secret; we use this protocol because each user's password is used to seed the generation of a master encryption/decryption key via KDF for that user which the platform
should not see.
Lastly, Infisical enforces strong password requirements according to the guidance set forth in [NIST Special Publication 800–63B](https://pages.nist.gov/800-63-3/sp800-63b.html#appA). Since passwords in Infisical also has cryptographic implications, Infisical validates each password on client-side to meet minimum length and entropy requirements; Infisical also considers each password against the [Have I Been Pwned (HIBP) API](https://haveibeenpwned.com), which checks the password against around 700M breached passwords, in a privacy-preserving way.
Since Infisical's unique zero-knowledge architecture requires a master decryption key for every user account, users with Google SSO, GitHub SSO, or SAML 2.0 enabled must still enter a secret after the
authentication step to access their secrets in Infisical. In practice, this implies stronger security since users must successfully authenticate with a single sign-on provider and provide a master decryption key
to access the platform.
We strongly encourage users to generate and store their passwords / master decryption key in a password manager, such as 1Password, Bitwarden, or Dashlane.
## Role-based access control (RBAC)
[Infisical's RBAC](https://infisical.com/docs/documentation/platform/role-based-access-controls) feature enables organization owners and administrators to manage fine-grained access policies for members of their organization in Infisical; with RBAC, administrators can define custom roles with permission sets to be conveniently assigned to other members.
For example, you can define a role provisioning access to secrets in a specific project and environment in it with read-only permissions; the role can be assigned to members of an organization in Infisical.
### Audit logging
Infisical's audit logging feature spans 25+ events, tracking everything from permission changes to queries and mutations applied to secrets, for security and compliance teams at enterprises to monitor information access in the event of any suspicious activity or incident review. Every event is timestamped and information about actor, source (i.e. IP address, user-agent, etc.), and relevant metadata is included.
### IP allowlisting
Infisical's IP allowlisting feature can be configured to restrict client access to specific IP addresses or CIDR ranges. This applies to any client using service tokens and can be useful, for example, for limiting access to traffic coming from corporate networks.
By default, each project is initialized with the `0.0.0.0/0` entry, representing all possible IPv4 addresses. For enhanced security, we strongly recommend replacing the default entry with your client IPs to tighten access to your secrets.
## Penetration testing
Infisical hires external third parties to perform regular security assessment and penetration testing of the platform.
Most recently, Infisical commissioned cybersecurity firm [Oneleet](https://www.oneleet.com) to perform a full-coverage, gray box penetration test against the platform's entire attack surface to identify vulnerabilities according to industry standards (OWASP, ASVS, WSTG, TOP-10, etc.).
Please email [security@infisical.com](mailto:security@infisical.com) to request any reports including a letter of attestation for the conducted penetration test.
## Employee data access
Whether or not Infisical or your employees can access data in the Infisical instance and/or storage backend depends on many factors how you use Infisical:
* Infisical Self-Hosted: Self-hosting Infisical is common amongst organizations that prefer to keep data on their own infrastructure usually to adhere to strict regulatory and compliance requirements. In this option, organizations retain full control over their data and therefore govern the data access policy of their Infisical instance and storage backend.
* Infisical Cloud: Using Infisical's managed service, [Infisical Cloud](https://app.infisical.com) means delegating data oversight and management to Infisical. Under our policy controls, employees are only granted access to parts of infrastructure according to principle of least privilege; this is especially relevant to customer data can only be accessed currently by executive management of Infisical. Moreover, any changes to sensitive customer data is prohibited without explicit customer approval.
It should be noted that, even on Infisical Cloud, it is physically impossible for employees of Infisical to view the values of secrets if users have not explicitly granted Infisical access to their project (i.e. opted out of zero-knowledge).
Please email [security@infisical.com](mailto:security@infisical.com) if you have any specific inquiries about employee data access policies.
## Get in touch
If you have any concerns about Infisical or believe you have uncovered a vulnerability, please get in touch via the e-mail address [security@infisical.com](mailto:security@infisical.com). In the message, try to provide a description of the issue and ideally a way of reproducing it. The security team will get back to you as soon as possible.
Note that this security address should be used for undisclosed vulnerabilities. Please report any security problems to us before disclosing it publicly.
# Service tokens
Understanding service tokens and their best practices.
Many clients use service tokens to authenticate and read/write secrets from/to Infisical; they can be created in your project settings.
## Anatomy
A service token in Infisical consists of the token itself, a `string`, and a corresponding document in the storage backend containing its
properties and metadata.
### Database model
The storage backend model for a token contains the following information:
* ID: The token identifier.
* Expiration: The date at which point the token is invalid.
* Project: The project that the token is part of.
* Scopes: The project environments and paths that the token has access to.
* Encrypted project key: An encrypted copy of the project key.
### Token
A service token itself consist of two parts used for authentication and decryption, separated by the delimiter `.`.
Consider the token `st.abc.def.ghi`. Here, `st.abc.def` can be used to authenticate with the API, by including it in the `Authorization` header under `Bearer st.abc.def`, and retrieve (encrypted) secrets as well as a project key back. Meanwhile, `ghi`, a hex-string, can be used to decrypt the project key used to decrypt the secrets.
Note that when using service tokens via select client methods like SDK or CLI, cryptographic operations are abstracted for you that is the token is parsed and encryption/decryption operations are handled. If using service tokens with the REST API and end-to-end encryption enabled, then you will have to handle the encryption/decryption operations yourself.
## Recommendations
### Issuance
When creating a new service token, it’s important to consider the principle of least privilege(PoLP) when setting its scope and expiration date. For example, if the client using the token only requires access to a staging environment, then you should scope the token to that environment only; you can further scope tokens to path(s) within environment(s) if you happen to use path-based secret storage. Likewise, if the client does not intend to access secrets indefinitely, then you may consider setting a finite lifetime for the token such as 6 months or 1 year from now. Finally, you should consider carefully whether or not your client requires the ability to read and/or write secrets from/to Infisical.
### Network access
We recommend configuring the IP whitelist settings of each project to allow either single IP addresses or CIDR-notated range of addresses to read/write secrets to Infisical. With this feature, you can specify the IP range of your client servers to restrict access to your project in Infisical.
### Storage
Since service tokens grant access to your secrets, we recommend storing them securely across your development cycle whether it be in a .env file in local development or as an environment variable of your deployment platform.
### Rotation
We recommend periodically rotating the service token, even in the absence of compromise. Since service tokens are capable of decrypting project keys used to decrypt secrets, all of which use AES-256-GCM encryption, they should be rotated before approximately 2^32 encryptions have been performed; this follows the guidance set forth by [NIST publication 800-38D](https://csrc.nist.gov/pubs/sp/800/38/d/final).
Note that Infisical keeps track of the number of times that service tokens are used and will alert you when you have reached 90% of the recommended capacity.
# Infisical .NET SDK
If you're working with C#, the official [Infisical C# SDK](https://github.com/Infisical/sdk/tree/main/languages/csharp) package is the easiest way to fetch and work with secrets for your application.
* [Nuget Package](https://www.nuget.org/packages/Infisical.Sdk)
* [Github Repository](https://github.com/Infisical/sdk/tree/main/languages/csharp)
## Basic Usage
```cs
using Infisical.Sdk;
namespace Example
{
class Program
{
static void Main(string[] args)
{
ClientSettings settings = new ClientSettings
{
Auth = new AuthenticationOptions
{
UniversalAuth = new UniversalAuthMethod
{
ClientId = "your-client-id",
ClientSecret = "your-client-secret"
}
}
};
var infisicalClient = new InfisicalClient(settings);
var getSecretOptions = new GetSecretOptions
{
SecretName = "TEST",
ProjectId = "PROJECT_ID",
Environment = "dev",
};
var secret = infisical.GetSecret(getSecretOptions);
Console.WriteLine($"The value of secret '{secret.SecretKey}', is: {secret.SecretValue}");
}
}
}
```
This example demonstrates how to use the Infisical C# SDK in a C# application. The application retrieves a secret named `TEST` from the `dev` environment of the `PROJECT_ID` project.
We do not recommend hardcoding your [Machine Identity Tokens](/platform/identities/overview). Setting it as an environment variable would be best.
# Installation
```console
$ dotnet add package Infisical.Sdk
```
# Configuration
Import the SDK and create a client instance with your [Machine Identity](/platform/identities/universal-auth).
```cs
using Infisical.Sdk;
namespace Example
{
class Program
{
static void Main(string[] args)
{
ClientSettings settings = new ClientSettings
{
Auth = new AuthenticationOptions
{
UniversalAuth = new UniversalAuthMethod
{
ClientId = "your-client-id",
ClientSecret = "your-client-secret"
}
}
};
var infisicalClient = new InfisicalClient(settings); // <-- Your SDK client is now ready to use
}
}
}
```
### ClientSettings methods
Your machine identity client ID.
Your machine identity client secret.
An access token obtained from the machine identity login endpoint.
Time-to-live (in seconds) for refreshing cached secrets.
If manually set to 0, caching will be disabled, this is not recommended.
Your self-hosted absolute site URL including the protocol (e.g. `https://app.infisical.com`)
Optionally provide a path to a custom SSL certificate file. This can be substituted by setting the `INFISICAL_SSL_CERTIFICATE` environment variable to the contents of the certificate.
The authentication object to use for the client. This is required unless you're using environment variables.
### Authentication
The SDK supports a variety of authentication methods. The most common authentication method is Universal Auth, which uses a client ID and client secret to authenticate.
#### Universal Auth
**Using environment variables**
* `INFISICAL_UNIVERSAL_AUTH_CLIENT_ID` - Your machine identity client ID.
* `INFISICAL_UNIVERSAL_AUTH_CLIENT_SECRET` - Your machine identity client secret.
**Using the SDK directly**
```csharp
ClientSettings settings = new ClientSettings
{
Auth = new AuthenticationOptions
{
UniversalAuth = new UniversalAuthMethod
{
ClientId = "your-client-id",
ClientSecret = "your-client-secret"
}
}
};
var infisicalClient = new InfisicalClient(settings);
```
#### GCP ID Token Auth
Please note that this authentication method will only work if you're running your application on Google Cloud Platform.
Please [read more](/documentation/platform/identities/gcp-auth) about this authentication method.
**Using environment variables**
* `INFISICAL_GCP_AUTH_IDENTITY_ID` - Your Infisical Machine Identity ID.
**Using the SDK directly**
```csharp
ClientSettings settings = new ClientSettings
{
Auth = new AuthenticationOptions
{
GcpIdToken = new GcpIdTokenAuthMethod
{
IdentityId = "your-machine-identity-id",
}
}
};
var infisicalClient = new InfisicalClient(settings);
```
#### GCP IAM Auth
**Using environment variables**
* `INFISICAL_GCP_IAM_AUTH_IDENTITY_ID` - Your Infisical Machine Identity ID.
* `INFISICAL_GCP_IAM_SERVICE_ACCOUNT_KEY_FILE_PATH` - The path to your GCP service account key file.
**Using the SDK directly**
```csharp
ClientSettings settings = new ClientSettings
{
Auth = new AuthenticationOptions
{
GcpIam = new GcpIamAuthMethod
{
IdentityId = "your-machine-identity-id",
ServiceAccountKeyFilePath = "./path/to/your/service-account-key.json"
}
}
};
var infisicalClient = new InfisicalClient(settings);
```
#### AWS IAM Auth
Please note that this authentication method will only work if you're running your application on AWS.
Please [read more](/documentation/platform/identities/aws-auth) about this authentication method.
**Using environment variables**
* `INFISICAL_AWS_IAM_AUTH_IDENTITY_ID` - Your Infisical Machine Identity ID.
**Using the SDK directly**
```csharp
ClientSettings settings = new ClientSettings
{
Auth = new AuthenticationOptions
{
AwsIam = new AwsIamAuthMethod
{
IdentityId = "your-machine-identity-id",
}
}
};
var infisicalClient = new InfisicalClient(settings);
```
#### Azure Auth
Please note that this authentication method will only work if you're running your application on Azure.
Please [read more](/documentation/platform/identities/azure-auth) about this authentication method.
**Using environment variables**
* `INFISICAL_AZURE_AUTH_IDENTITY_ID` - Your Infisical Machine Identity ID.
**Using the SDK directly**
```csharp
ClientSettings settings = new ClientSettings
{
Auth = new AuthenticationOptions
{
Azure = new AzureAuthMethod
{
IdentityId = "YOUR_IDENTITY_ID",
}
}
};
var infisicalClient = new InfisicalClient(settings);
```
#### Kubernetes Auth
Please note that this authentication method will only work if you're running your application on Kubernetes.
Please [read more](/documentation/platform/identities/kubernetes-auth) about this authentication method.
**Using environment variables**
* `INFISICAL_KUBERNETES_IDENTITY_ID` - Your Infisical Machine Identity ID.
* `INFISICAL_KUBERNETES_SERVICE_ACCOUNT_TOKEN_PATH_ENV_NAME` - The environment variable name that contains the path to the service account token. This is optional and will default to `/var/run/secrets/kubernetes.io/serviceaccount/token`.
**Using the SDK directly**
```csharp
ClientSettings settings = new ClientSettings
{
Auth = new AuthenticationOptions
{
Kubernetes = new KubernetesAuthMethod
{
ServiceAccountTokenPath = "/var/run/secrets/kubernetes.io/serviceaccount/token", // Optional
IdentityId = "YOUR_IDENTITY_ID",
}
}
};
var infisicalClient = new InfisicalClient(settings);
```
### Caching
To reduce the number of API requests, the SDK temporarily stores secrets it retrieves. By default, a secret remains cached for 5 minutes after it's first fetched. Each time it's fetched again, this 5-minute timer resets. You can adjust this caching duration by setting the "cacheTTL" option when creating the client.
## Working with Secrets
### client.ListSecrets(options)
```cs
var options = new ListSecretsOptions
{
ProjectId = "PROJECT_ID",
Environment = "dev",
Path = "/foo/bar",
AttachToProcessEnv = false,
};
var secrets = infisical.ListSecrets(options);
```
Retrieve all secrets within the Infisical project and environment that client is connected to
#### Parameters
The slug name (dev, prod, etc) of the environment from where secrets should be fetched from.
The project ID where the secret lives in.
The path from where secrets should be fetched from.
Whether or not to set the fetched secrets to the process environment. If true, you can access the secrets like so `System.getenv("SECRET_NAME")`.
Whether or not to include imported secrets from the current path. Read about [secret import](/documentation/platform/secret-reference)
Whether or not to fetch secrets recursively from the specified path. Please note that there's a 20-depth limit for recursive fetching.
Whether or not to expand secret references in the fetched secrets. Read about [secret reference](/documentation/platform/secret-reference)
### client.GetSecret(options)
```cs
var options = new GetSecretOptions
{
SecretName = "AAAA",
ProjectId = "659c781eb2d4fe3e307b77bd",
Environment = "dev",
};
var secret = infisical.GetSecret(options);
```
Retrieve a secret from Infisical.
By default, `GetSecret()` fetches and returns a shared secret.
#### Parameters
The key of the secret to retrieve.
The project ID where the secret lives in.
The slug name (dev, prod, etc) of the environment from where secrets should be fetched from.
The path from where secret should be fetched from.
The type of the secret. Valid options are "shared" or "personal". If not specified, the default value is "shared".
Whether or not to include imported secrets from the current path. Read about [secret import](/documentation/platform/secret-reference)
Whether or not to expand secret references in the fetched secrets. Read about [secret reference](/documentation/platform/secret-reference)
### client.CreateSecret(options)
```cs
var options = new CreateSecretOptions {
Environment = "dev",
ProjectId = "PROJECT_ID",
SecretName = "NEW_SECRET",
SecretValue = "NEW_SECRET_VALUE",
SecretComment = "This is a new secret",
};
var newSecret = infisical.CreateSecret(options);
```
Create a new secret in Infisical.
#### Parameters
The key of the secret to create.
The value of the secret.
The project ID where the secret lives in.
The slug name (dev, prod, etc) of the environment from where secrets should be fetched from.
The path from where secret should be created.
The type of the secret. Valid options are "shared" or "personal". If not specified, the default value is "shared".
### client.UpdateSecret(options)
```cs
var options = new UpdateSecretOptions {
Environment = "dev",
ProjectId = "PROJECT_ID",
SecretName = "SECRET_TO_UPDATE",
SecretValue = "NEW VALUE"
};
var updatedSecret = infisical.UpdateSecret(options);
```
Update an existing secret in Infisical.
#### Parameters
The key of the secret to update.
The new value of the secret.
The project ID where the secret lives in.
The slug name (dev, prod, etc) of the environment from where secrets should be fetched from.
The path from where secret should be updated.
The type of the secret. Valid options are "shared" or "personal". If not specified, the default value is "shared".
### client.DeleteSecret(options)
```cs
var options = new DeleteSecretOptions
{
Environment = "dev",
ProjectId = "PROJECT_ID",
SecretName = "NEW_SECRET",
};
var deletedSecret = infisical.DeleteSecret(options);
```
Delete a secret in Infisical.
#### Parameters
The key of the secret to update.
The project ID where the secret lives in.
The slug name (dev, prod, etc) of the environment from where secrets should be fetched from.
The path from where secret should be deleted.
The type of the secret. Valid options are "shared" or "personal". If not specified, the default value is "shared".
## Cryptography
### Create a symmetric key
Create a base64-encoded, 256-bit symmetric key to be used for encryption/decryption.
```cs
var key = infisical.CreateSymmetricKey();
```
#### Returns (string)
`key` (string): A base64-encoded, 256-bit symmetric key, that can be used for encryption/decryption purposes.
### Encrypt symmetric
```cs
var options = new EncryptSymmetricOptions
{
Plaintext = "Infisical is awesome!",
Key = key,
};
var encryptedData = infisical.EncryptSymmetric(options);
```
#### Parameters
The plaintext you want to encrypt.
The symmetric key to use for encryption.
#### Returns (object)
`Tag` (string): A base64-encoded, 128-bit authentication tag.
`Iv` (string): A base64-encoded, 96-bit initialization vector.
`CipherText` (string): A base64-encoded, encrypted ciphertext.
### Decrypt symmetric
```cs
var decryptOptions = new DecryptSymmetricOptions
{
Key = key,
Ciphertext = encryptedData.Ciphertext,
Iv = encryptedData.Iv,
Tag = encryptedData.Tag,
};
var decryptedPlaintext = infisical.DecryptSymmetric(decryptOptions);
```
#### Parameters
The ciphertext you want to decrypt.
The symmetric key to use for encryption.
The initialization vector to use for decryption.
The authentication tag to use for decryption.
#### Returns (string)
`Plaintext` (string): The decrypted plaintext.
# Infisical Go SDK
If you're working with Go Lang, the official [Infisical Go SDK](https://github.com/infisical/go-sdk) package is the easiest way to fetch and work with secrets for your application.
* [Package](https://pkg.go.dev/github.com/infisical/go-sdk)
* [Github Repository](https://github.com/infisical/go-sdk)
# Basic Usage
```go
package main
import (
"fmt"
"os"
"context"
infisical "github.com/infisical/go-sdk"
)
func main() {
client := infisical.NewInfisicalClient(context.Background(), infisical.Config{
SiteUrl: "https://app.infisical.com", // Optional, default is https://app.infisical.com
AutoTokenRefresh: true, // Wether or not to let the SDK handle the access token lifecycle. Defaults to true if not specified.
})
_, err = client.Auth().UniversalAuthLogin("YOUR_CLIENT_ID", "YOUR_CLIENT_SECRET")
if err != nil {
fmt.Printf("Authentication failed: %v", err)
os.Exit(1)
}
apiKeySecret, err := client.Secrets().Retrieve(infisical.RetrieveSecretOptions{
SecretKey: "API_KEY",
Environment: "dev",
ProjectID: "YOUR_PROJECT_ID",
SecretPath: "/",
})
if err != nil {
fmt.Printf("Error: %v", err)
os.Exit(1)
}
fmt.Printf("API Key Secret: %v", apiKeySecret)
}
```
This example demonstrates how to use the Infisical Go SDK in a simple Go application. The application retrieves a secret named `API_KEY` from the `dev` environment of the `YOUR_PROJECT_ID` project.
We do not recommend hardcoding your [Machine Identity Tokens](/platform/identities/overview). Setting it as an environment variable would be best.
# Installation
```console
$ go get github.com/infisical/go-sdk
```
# Configuration
Import the SDK and create a client instance.
```go
client := infisical.NewInfisicalClient(context.Background(), infisical.Config{
SiteUrl: "https://app.infisical.com", // Optional, default is https://api.infisical.com
})
```
### Configuration Options
The URL of the Infisical API..
Optionally set the user agent that will be used for HTTP requests. *(Not recommended)*
Whether or not to let the SDK handle the access token lifecycle. Defaults to true if not specified.
Whether or not to suppress logs such as warnings from the token refreshing process. Defaults to false if not specified.
# Automatic token refreshing
The Infisical Go SDK supports automatic token refreshing. After using one of the auth methods such as Universal Auth, the SDK will automatically renew and re-authenticate when needed.
This behavior is enabled by default, but you can opt-out by setting `AutoTokenRefresh` to `false` in the client settings.
```go
client := infisical.NewInfisicalClient(context.Background(), infisical.Config{
AutoTokenRefresh: false, // <- Disable automatic token refreshing
})
```
When using automatic token refreshing it's important to understand how your application uses the Infiiscal client. If you are instantiating new instances of the client often, it's important to cancel the context when the client is no longer needed to avoid the token refreshing process from running indefinitely.
```go
ctx, cancel := context.WithCancel(context.Background())
defer cancel() // Cancel the context when the client is no longer needed
client := infisical.NewInfisicalClient(ctx, infisical.Config{
AutoTokenRefresh: true,
})
// Use the client
```
This is only necessary if you are creating multiple instances of the client, and those instances are deleted or otherwise removed throughout the application lifecycle.
If you are only creating one instance of the client, and it will be used throughout the lifetime of your application, you don't need to worry about this.
# Authentication
The SDK supports a variety of authentication methods. The most common authentication method is Universal Auth, which uses a client ID and client secret to authenticate.
#### Universal Auth
**Using environment variables**
Call `.Auth().UniversalAuthLogin()` with empty arguments to use the following environment variables:
* `INFISICAL_UNIVERSAL_AUTH_CLIENT_ID` - Your machine identity client ID.
* `INFISICAL_UNIVERSAL_AUTH_CLIENT_SECRET` - Your machine identity client secret.
**Using the SDK directly**
```go
_, err := client.Auth().UniversalAuthLogin("CLIENT_ID", "CLIENT_SECRET")
if err != nil {
fmt.Println(err)
os.Exit(1)
}
```
#### GCP ID Token Auth
Please note that this authentication method will only work if you're running your application on Google Cloud Platform.
Please [read more](/documentation/platform/identities/gcp-auth) about this authentication method.
**Using environment variables**
Call `.Auth().GcpIdTokenAuthLogin()` with empty arguments to use the following environment variables:
* `INFISICAL_GCP_AUTH_IDENTITY_ID` - Your Infisical Machine Identity ID.
**Using the SDK directly**
```go
_, err := client.Auth().GcpIdTokenAuthLogin("YOUR_MACHINE_IDENTITY_ID")
if err != nil {
fmt.Println(err)
os.Exit(1)
}
```
#### GCP IAM Auth
**Using environment variables**
Call `.Auth().GcpIamAuthLogin()` with empty arguments to use the following environment variables:
* `INFISICAL_GCP_IAM_AUTH_IDENTITY_ID` - Your Infisical Machine Identity ID.
* `INFISICAL_GCP_IAM_SERVICE_ACCOUNT_KEY_FILE_PATH` - The path to your GCP service account key file.
**Using the SDK directly**
```go
_, err = client.Auth().GcpIamAuthLogin("MACHINE_IDENTITY_ID", "SERVICE_ACCOUNT_KEY_FILE_PATH")
if err != nil {
fmt.Println(err)
os.Exit(1)
}
```
#### AWS IAM Auth
Please note that this authentication method will only work if you're running your application on AWS.
Please [read more](/documentation/platform/identities/aws-auth) about this authentication method.
**Using environment variables**
Call `.Auth().AwsIamAuthLogin()` with empty arguments to use the following environment variables:
* `INFISICAL_AWS_IAM_AUTH_IDENTITY_ID` - Your Infisical Machine Identity ID.
**Using the SDK directly**
```go
_, err = client.Auth().AwsIamAuthLogin("MACHINE_IDENTITY_ID")
if err != nil {
fmt.Println(err)
os.Exit(1)
}
```
#### Azure Auth
Please note that this authentication method will only work if you're running your application on Azure.
Please [read more](/documentation/platform/identities/azure-auth) about this authentication method.
**Using environment variables**
Call `.Auth().AzureAuthLogin()` with empty arguments to use the following environment variables:
* `INFISICAL_AZURE_AUTH_IDENTITY_ID` - Your Infisical Machine Identity ID.
**Using the SDK directly**
```go
_, err = client.Auth().AzureAuthLogin("MACHINE_IDENTITY_ID")
if err != nil {
fmt.Println(err)
os.Exit(1)
}
```
#### Kubernetes Auth
Please note that this authentication method will only work if you're running your application on Kubernetes.
Please [read more](/documentation/platform/identities/kubernetes-auth) about this authentication method.
**Using environment variables**
Call `.Auth().KubernetesAuthLogin()` with empty arguments to use the following environment variables:
* `INFISICAL_KUBERNETES_IDENTITY_ID` - Your Infisical Machine Identity ID.
* `INFISICAL_KUBERNETES_SERVICE_ACCOUNT_TOKEN_PATH_ENV_NAME` - The environment variable name that contains the path to the service account token. This is optional and will default to `/var/run/secrets/kubernetes.io/serviceaccount/token`.
**Using the SDK directly**
```go
// Service account token path will default to /var/run/secrets/kubernetes.io/serviceaccount/token if empty value is passed
_, err = client.Auth().KubernetesAuthLogin("MACHINE_IDENTITY_ID", "SERVICE_ACCOUNT_TOKEN_PATH")
if err != nil {
fmt.Println(err)
os.Exit(1)
}
```
## Working With Secrets
### List Secrets
`client.Secrets().List(options)`
Retrieve all secrets within the Infisical project and environment that client is connected to.
```go
secrets, err := client.Secrets().List(infisical.ListSecretsOptions{
ProjectID: "PROJECT_ID",
Environment: "dev",
SecretPath: "/foo/bar",
AttachToProcessEnv: false,
})
```
### Parameters
The slug name (dev, prod, etc) of the environment from where secrets should be fetched from.
The project ID where the secret lives in.
The path from where secrets should be fetched from.
Whether or not to set the fetched secrets to the process environment. If true, you can access the secrets like so `System.getenv("SECRET_NAME")`.
Whether or not to include imported secrets from the current path. Read about [secret import](/documentation/platform/secret-reference)
Whether or not to fetch secrets recursively from the specified path. Please note that there's a 20-depth limit for recursive fetching.
Whether or not to expand secret references in the fetched secrets. Read about [secret reference](/documentation/platform/secret-reference)
###
### Retrieve Secret
`client.Secrets().Retrieve(options)`
Retrieve a secret from Infisical. By default `Secrets().Retrieve()` fetches and returns a shared secret.
```go
secret, err := client.Secrets().Retrieve(infisical.RetrieveSecretOptions{
SecretKey: "API_KEY",
ProjectID: "PROJECT_ID",
Environment: "dev",
})
```
### Parameters
The key of the secret to retrieve.
The project ID where the secret lives in.
The slug name (dev, prod, etc) of the environment from where secrets should be fetched from.
The path from where secret should be fetched from.
The type of the secret. Valid options are "shared" or "personal". If not specified, the default value is "shared".
###
### Create Secret
`client.Secrets().Create(options)`
Create a new secret in Infisical.
```go
secret, err := client.Secrets().Create(infisical.CreateSecretOptions{
ProjectID: "PROJECT_ID",
Environment: "dev",
SecretKey: "NEW_SECRET_KEY",
SecretValue: "NEW_SECRET_VALUE",
SecretComment: "This is a new secret",
})
```
### Parameters
The key of the secret to create.
The value of the secret.
A comment for the secret.
The project ID where the secret lives in.
The slug name (dev, prod, etc) of the environment from where secrets should be fetched from.
The path from where secret should be created.
The type of the secret. Valid options are "shared" or "personal". If not specified, the default value is "shared".
###
### Update Secret
`client.Secrets().Update(options)`
Update an existing secret in Infisical.
```go
secret, err := client.Secrets().Update(infisical.UpdateSecretOptions{
ProjectID: "PROJECT_ID",
Environment: "dev",
SecretKey: "NEW_SECRET_KEY",
NewSecretValue: "NEW_SECRET_VALUE",
NewSkipMultilineEncoding: false,
})
```
### Parameters
The key of the secret to update.
The new value of the secret.
Whether or not to skip multiline encoding for the new secret value.
The project ID where the secret lives in.
The slug name (dev, prod, etc) of the environment from where secrets should be fetched from.
The path from where secret should be updated.
The type of the secret. Valid options are "shared" or "personal". If not specified, the default value is "shared".
###
### Delete Secret
`client.Secrets().Delete(options)`
Delete a secret in Infisical.
```go
secret, err := client.Secrets().Delete(infisical.DeleteSecretOptions{
ProjectID: "PROJECT_ID",
Environment: "dev",
SecretKey: "SECRET_KEY",
})
```
### Parameters
The key of the secret to update.
The project ID where the secret lives in.
The slug name (dev, prod, etc) of the environment from where secrets should be fetched from.
The path from where secret should be deleted.
The type of the secret. Valid options are "shared" or "personal". If not specified, the default value is "shared".
## Working With folders
###
### List Folders
`client.Folders().List(options)`
Retrieve all within the Infisical project and environment that client is connected to.
```go
folders, err := client.Folders().List(infisical.ListFoldersOptions{
ProjectID: "PROJECT_ID",
Environment: "dev",
Path: "/",
})
```
### Parameters
The slug name (dev, prod, etc) of the environment from where folders should be fetched from.
The project ID where the folder lives in.
The path from where folders should be fetched from.
###
### Create Folder
`client.Folders().Create(options)`
Create a new folder in Infisical.
```go
folder, err := client.Folders().Create(infisical.CreateFolderOptions{
ProjectID: "PROJECT_ID",
Name: "new=folder-name",
Environment: "dev",
Path: "/",
})
```
### Parameters
The ID of the project where the folder will be created.
The slug name (dev, prod, etc) of the environment where the folder will be created.
The path to create the folder in. The root path is `/`.
The name of the folder to create.
###
### Update Folder
`client.Folders().Update(options)`
Update an existing folder in Infisical.
```go
folder, err := client.Folders().Update(infisical.UpdateFolderOptions{
ProjectID: "PROJECT_ID",
Environment: "dev",
Path: "/",
FolderID: "FOLDER_ID_TO_UPDATE",
NewName: "new-folder-name",
})
```
### Parameters
The ID of the project where the folder will be updated.
The slug name (dev, prod, etc) of the environment from where the folder lives in.
The path from where the folder should be updated.
The ID of the folder to update.
The new name of the folder.
###
### Delete Folder
`client.Folders().Delete(options)`
Delete a folder in Infisical.
```go
deletedFolder, err := client.Folders().Delete(infisical.DeleteFolderOptions{
// Either folder ID or folder name is required.
FolderName: "name-of-folder-to-delete",
FolderID: "folder-id-to-delete",
ProjectID: "PROJECT_ID",
Environment: "dev",
Path: "/",
})
```
### Parameters
The name of the folder to delete. Note that either `FolderName` or `FolderID` is required.
The ID of the folder to delete. Note that either `FolderName` or `FolderID` is required.
The ID of the project where the folder lives in.
The slug name (dev, prod, etc) of the environment from where the folder lives in.
The path from where the folder should be deleted.
# Infisical Java SDK
If you're working with Java, the official [Infisical Java SDK](https://github.com/Infisical/sdk/tree/main/languages/java) package is the easiest way to fetch and work with secrets for your application.
* [Maven Package](https://github.com/Infisical/sdk/packages/2019741)
* [Github Repository](https://github.com/Infisical/sdk/tree/main/languages/java)
## Basic Usage
```java
package com.example.app;
import com.infisical.sdk.InfisicalClient;
import com.infisical.sdk.schema.*;
public class Example {
public static void main(String[] args) {
// Create the authentication settings for the client
ClientSettings settings = new ClientSettings();
AuthenticationOptions authOptions = new AuthenticationOptions();
UniversalAuthMethod authMethod = new UniversalAuthMethod();
authMethod.setClientID("YOUR_IDENTITY_ID");
authMethod.setClientSecret("YOUR_CLIENT_SECRET");
authOptions.setUniversalAuth(authMethod);
settings.setAuth(authOptions);
// Create a new Infisical Client
InfisicalClient client = new InfisicalClient(settings);
// Create the options for fetching the secret
GetSecretOptions options = new GetSecretOptions();
options.setSecretName("TEST");
options.setEnvironment("dev");
options.setProjectID("PROJECT_ID");
// Fetch the sercret with the provided options
GetSecretResponseSecret secret = client.getSecret(options);
// Print the value
System.out.println(secret.getSecretValue());
// Important to avoid memory leaks!
// If you intend to use the client throughout your entire application, you can omit this line.
client.close();
}
}
```
This example demonstrates how to use the Infisical Java SDK in a Java application. The application retrieves a secret named `TEST` from the `dev` environment of the `PROJECT_ID` project.
We do not recommend hardcoding your [Machine Identity Tokens](/platform/identities/overview). Setting it as an environment variable would be best.
# Installation
The Infisical Java SDK is hosted on the GitHub Packages Apache Maven registry. Because of this you need to configure your environment properly so it's able to pull dependencies from the GitHub registry. Please check [this guide from GitHub](https://docs.github.com/en/packages/working-with-a-github-packages-registry/working-with-the-apache-maven-registry) on how to achieve this.
Our package is [located here](https://github.com/Infisical/sdk/packages/2019741). Please follow the installation guide on the page.
# Configuration
Import the SDK and create a client instance with your [Machine Identity](/platform/identities/universal-auth).
```java
import com.infisical.sdk.InfisicalClient;
import com.infisical.sdk.schema.*;
public class App {
public static void main(String[] args) {
// Create the authentication settings for the client
ClientSettings settings = new ClientSettings();
AuthenticationOptions authOptions = new AuthenticationOptions();
UniversalAuthMethod authMethod = new UniversalAuthMethod();
authMethod.setClientID("YOUR_IDENTITY_ID");
authMethod.setClientSecret("YOUR_CLIENT_SECRET");
authOptions.setUniversalAuth(authMethod);
settings.setAuth(authOptions);
// Create a new Infisical Client
InfisicalClient client = new InfisicalClient(settings); // Your client!
}
}
```
### ClientSettings methods
Your machine identity client ID.
**This field is deprecated and will be removed in future versions.** Please use the `setAuth()` method on the client settings instead.
Your machine identity client secret.
**This field is deprecated and will be removed in future versions.** Please use the `setAuth()` method on the client settings instead.
An access token obtained from the machine identity login endpoint.
**This field is deprecated and will be removed in future versions.** Please use the `setAuth()` method on the client settings instead.
Time-to-live (in seconds) for refreshing cached secrets.
If manually set to 0, caching will be disabled, this is not recommended.
Your self-hosted absolute site URL including the protocol (e.g. `https://app.infisical.com`)
Optionally provide a path to a custom SSL certificate file. This can be substituted by setting the `INFISICAL_SSL_CERTIFICATE` environment variable to the contents of the certificate.
The authentication object to use for the client. This is required unless you're using environment variables.
### Authentication
The SDK supports a variety of authentication methods. The most common authentication method is Universal Auth, which uses a client ID and client secret to authenticate.
#### Universal Auth
**Using environment variables**
* `INFISICAL_UNIVERSAL_AUTH_CLIENT_ID` - Your machine identity client ID.
* `INFISICAL_UNIVERSAL_AUTH_CLIENT_SECRET` - Your machine identity client secret.
**Using the SDK directly**
```java
ClientSettings settings = new ClientSettings();
AuthenticationOptions authOptions = new AuthenticationOptions();
UniversalAuthMethod authMethod = new UniversalAuthMethod();
authMethod.setClientID("YOUR_IDENTITY_ID");
authMethod.setClientSecret("YOUR_CLIENT_SECRET");
authOptions.setUniversalAuth(authMethod);
settings.setAuth(authOptions);
InfisicalClient client = new InfisicalClient(settings);
```
#### GCP ID Token Auth
Please note that this authentication method will only work if you're running your application on Google Cloud Platform.
Please [read more](/documentation/platform/identities/gcp-auth) about this authentication method.
**Using environment variables**
* `INFISICAL_GCP_AUTH_IDENTITY_ID` - Your Infisical Machine Identity ID.
**Using the SDK directly**
```java
ClientSettings settings = new ClientSettings();
AuthenticationOptions authOptions = new AuthenticationOptions();
GCPIDTokenAuthMethod authMethod = new GCPIDTokenAuthMethod();
authMethod.setIdentityID("YOUR_MACHINE_IDENTITY_ID");
authOptions.setGcpIDToken(authMethod);
settings.setAuth(authOptions);
InfisicalClient client = new InfisicalClient(settings);
```
#### GCP IAM Auth
**Using environment variables**
* `INFISICAL_GCP_IAM_AUTH_IDENTITY_ID` - Your Infisical Machine Identity ID.
* `INFISICAL_GCP_IAM_SERVICE_ACCOUNT_KEY_FILE_PATH` - The path to your GCP service account key file.
**Using the SDK directly**
```java
ClientSettings settings = new ClientSettings();
AuthenticationOptions authOptions = new AuthenticationOptions();
GCPIamAuthMethod authMethod = new GCPIamAuthMethod();
authMethod.setIdentityID("YOUR_MACHINE_IDENTITY_ID");
authMethod.setServiceAccountKeyFilePath("./path/to/your/service-account-key.json");
authOptions.setGcpIam(authMethod);
settings.setAuth(authOptions);
InfisicalClient client = new InfisicalClient(settings);
```
#### AWS IAM Auth
Please note that this authentication method will only work if you're running your application on AWS.
Please [read more](/documentation/platform/identities/aws-auth) about this authentication method.
**Using environment variables**
* `INFISICAL_AWS_IAM_AUTH_IDENTITY_ID` - Your Infisical Machine Identity ID.
**Using the SDK directly**
```java
ClientSettings settings = new ClientSettings();
AuthenticationOptions authOptions = new AuthenticationOptions();
AWSIamAuthMethod authMethod = new AWSIamAuthMethod();
authMethod.setIdentityID("YOUR_MACHINE_IDENTITY_ID");
authOptions.setAwsIam(authMethod);
settings.setAuth(authOptions);
InfisicalClient client = new InfisicalClient(settings);
```
#### Azure Auth
Please note that this authentication method will only work if you're running your application on Azure.
Please [read more](/documentation/platform/identities/azure-auth) about this authentication method.
**Using environment variables**
* `INFISICAL_AZURE_AUTH_IDENTITY_ID` - Your Infisical Machine Identity ID.
**Using the SDK directly**
```java
ClientSettings settings = new ClientSettings();
AuthenticationOptions authOptions = new AuthenticationOptions();
AzureAuthMethod authMethod = new AzureAuthMethod();
authMethod.setIdentityID("YOUR_IDENTITY_ID");
authOptions.setAzure(authMethod);
settings.setAuth(authOptions);
InfisicalClient client = new InfisicalClient(settings);
```
#### Kubernetes Auth
Please note that this authentication method will only work if you're running your application on Kubernetes.
Please [read more](/documentation/platform/identities/kubernetes-auth) about this authentication method.
**Using environment variables**
* `INFISICAL_KUBERNETES_IDENTITY_ID` - Your Infisical Machine Identity ID.
* `INFISICAL_KUBERNETES_SERVICE_ACCOUNT_TOKEN_PATH_ENV_NAME` - The environment variable name that contains the path to the service account token. This is optional and will default to `/var/run/secrets/kubernetes.io/serviceaccount/token`.
**Using the SDK directly**
```java
ClientSettings settings = new ClientSettings();
AuthenticationOptions authOptions = new AuthenticationOptions();
KubernetesAuthMethod authMethod = new KubernetesAuthMethod();
authMethod.setIdentityID("YOUR_IDENTITY_ID");
authMethod.setServiceAccountTokenPath("/var/run/secrets/kubernetes.io/serviceaccount/token"); // Optional
authOptions.setKubernetes(authMethod);
settings.setAuth(authOptions);
InfisicalClient client = new InfisicalClient(settings);
```
### Caching
To reduce the number of API requests, the SDK temporarily stores secrets it retrieves. By default, a secret remains cached for 5 minutes after it's first fetched. Each time it's fetched again, this 5-minute timer resets. You can adjust this caching duration by setting the "cacheTTL" option when creating the client.
## Working with Secrets
### client.listSecrets(options)
```java
ListSecretsOptions options = new ListSecretsOptions();
options.setEnvironment("dev");
options.setProjectID("PROJECT_ID");
options.setPath("/foo/bar");
options.setIncludeImports(false);
options.setRecursive(false);
options.setExpandSecretReferences(true);
SecretElement[] secrets = client.listSecrets(options);
```
Retrieve all secrets within the Infisical project and environment that client is connected to
#### Methods
The slug name (dev, prod, etc) of the environment from where secrets should be fetched from.
The project ID where the secret lives in.
The path from where secrets should be fetched from.
Whether or not to set the fetched secrets to the process environment. If true, you can access the secrets like so `System.getenv("SECRET_NAME")`.
Whether or not to include imported secrets from the current path. Read about [secret import](/documentation/platform/secret-reference)
Whether or not to fetch secrets recursively from the specified path. Please note that there's a 20-depth limit for recursive fetching.
Whether or not to expand secret references in the fetched secrets. Read about [secret reference](/documentation/platform/secret-reference)
### client.getSecret(options)
```java
GetSecretOptions options = new GetSecretOptions();
options.setSecretName("TEST");
options.setEnvironment("dev");
options.setProjectID("PROJECT_ID");
GetSecretResponseSecret secret = client.getSecret(options);
String secretValue = secret.getSecretValue();
```
Retrieve a secret from Infisical.
By default, `getSecret()` fetches and returns a shared secret.
#### Methods
The key of the secret to retrieve.
The project ID where the secret lives in.
The slug name (dev, prod, etc) of the environment from where secrets should be fetched from.
The path from where secret should be fetched from.
The type of the secret. Valid options are "shared" or "personal". If not specified, the default value is "shared".
Whether or not to include imported secrets from the current path. Read about [secret import](/documentation/platform/secret-reference)
Whether or not to expand secret references in the fetched secrets. Read about [secret reference](/documentation/platform/secret-reference)
### client.createSecret(options)
```java
CreateSecretOptions createOptions = new CreateSecretOptions();
createOptions.setSecretName("NEW_SECRET");
createOptions.setEnvironment("dev");
createOptions.setProjectID("PROJECT_ID");
createOptions.setSecretValue("SOME SECRET VALUE");
createOptions.setPath("/"); // Default
createOptions.setType("shared"); // Default
CreateSecretResponseSecret newSecret = client.createSecret(createOptions);
```
Create a new secret in Infisical.
#### Methods
The key of the secret to create.
The value of the secret.
The project ID where the secret lives in.
The slug name (dev, prod, etc) of the environment from where secrets should be fetched from.
The path from where secret should be created.
The type of the secret. Valid options are "shared" or "personal". If not specified, the default value is "shared".
### client.updateSecret(options)
```java
UpdateSecretOptions options = new UpdateSecretOptions();
options.setSecretName("SECRET_TO_UPDATE");
options.setSecretValue("NEW SECRET VALUE");
options.setEnvironment("dev");
options.setProjectID("PROJECT_ID");
options.setPath("/"); // Default
options.setType("shared"); // Default
UpdateSecretResponseSecret updatedSecret = client.updateSecret(options);
```
Update an existing secret in Infisical.
#### Methods
The key of the secret to update.
The new value of the secret.
The project ID where the secret lives in.
The slug name (dev, prod, etc) of the environment from where secrets should be fetched from.
The path from where secret should be updated.
The type of the secret. Valid options are "shared" or "personal". If not specified, the default value is "shared".
### client.deleteSecret(options)
```java
DeleteSecretOptions options = new DeleteSecretOptions();
options.setSecretName("SECRET_TO_DELETE");
options.setEnvironment("dev");
options.setProjectID("PROJECT_ID");
options.setPath("/"); // Default
options.setType("shared"); // Default
DeleteSecretResponseSecret deletedSecret = client.deleteSecret(options);
```
Delete a secret in Infisical.
#### Methods
The key of the secret to update.
The project ID where the secret lives in.
The slug name (dev, prod, etc) of the environment from where secrets should be fetched from.
The path from where secret should be deleted.
The type of the secret. Valid options are "shared" or "personal". If not specified, the default value is "shared".
## Cryptography
### Create a symmetric key
Create a base64-encoded, 256-bit symmetric key to be used for encryption/decryption.
```java
String key = client.createSymmetricKey();
```
#### Returns (string)
`key` (string): A base64-encoded, 256-bit symmetric key, that can be used for encryption/decryption purposes.
### Encrypt symmetric
```java
EncryptSymmetricOptions options = new EncryptSymmetricOptions();
options.setKey(key);
options.setPlaintext("Infisical is awesome!");
EncryptSymmetricResponse encryptedData = client.encryptSymmetric(options);
```
#### Methods
The plaintext you want to encrypt.
The symmetric key to use for encryption.
#### Returns (object)
`tag (getTag())` (string): A base64-encoded, 128-bit authentication tag.
`iv (getIv())` (string): A base64-encoded, 96-bit initialization vector.
`ciphertext (getCipherText())` (string): A base64-encoded, encrypted ciphertext.
### Decrypt symmetric
```java
DecryptSymmetricOptions decryptOptions = new DecryptSymmetricOptions();
decryptOptions.setKey(key);
decryptOptions.setCiphertext(encryptedData.getCiphertext());
decryptOptions.setIv(encryptedData.getIv());
decryptOptions.setTag(encryptedData.getTag());
String decryptedString = client.decryptSymmetric(decryptOptions);
```
#### Methods
The ciphertext you want to decrypt.
The symmetric key to use for encryption.
The initialization vector to use for decryption.
The authentication tag to use for decryption.
#### Returns (string)
`Plaintext` (string): The decrypted plaintext.
# Infisical Node.js SDK
{/*
If you're working with Node.js, the official [Infisical Node SDK](https://github.com/Infisical/sdk/tree/main/languages/node) package is the easiest way to fetch and work with secrets for your application.
- [NPM Package](https://www.npmjs.com/package/@infisical/sdk)
- [Github Repository](https://github.com/Infisical/sdk/tree/main/languages/node)
## Basic Usage
```js
import express from "express";
import { InfisicalClient } from "@infisical/sdk";
const app = express();
const PORT = 3000;
const client = new InfisicalClient({
siteUrl: "https://app.infisical.com", // Optional, defaults to https://app.infisical.com
auth: {
universalAuth: {
clientId: "YOUR_CLIENT_ID",
clientSecret: "YOUR_CLIENT_SECRET"
}
}
});
app.get("/", async (req, res) => {
// Access the secret
const name = await client.getSecret({
environment: "dev",
projectId: "PROJECT_ID",
path: "/",
type: "shared",
secretName: "NAME"
});
res.send(`Hello! My name is: ${name.secretValue}`);
});
app.listen(PORT, async () => {
// initialize client
console.log(`App listening on port ${PORT}`);
});
```
This example demonstrates how to use the Infisical Node SDK with an Express application. The application retrieves a secret named "NAME" and responds to requests with a greeting that includes the secret value.
We do not recommend hardcoding your [Machine Identity Tokens](/documentation/platform/identities/overview). Setting it as an environment variable
would be best.
## Installation
Run `npm` to add `@infisical/sdk` to your project.
```console
$ npm install @infisical/sdk
```
## Configuration
Import the SDK and create a client instance with your [Machine Identity](/documentation/platform/identities/overview).
```js
import { InfisicalClient, LogLevel } from "@infisical/sdk";
const client = new InfisicalClient({
auth: {
universalAuth: {
clientId: "YOUR_CLIENT_ID",
clientSecret: "YOUR_CLIENT_SECRET"
}
},
logLevel: LogLevel.Error
});
```
```js
const { InfisicalClient } = require("@infisical/sdk");
const client = new InfisicalClient({
auth: {
universalAuth: {
clientId: "YOUR_CLIENT_ID",
clientSecret: "YOUR_CLIENT_SECRET"
}
},
});
```
### Parameters
Your machine identity client ID.
**This field is deprecated and will be removed in future versions.** Please use the `auth.universalAuth.clientId` field instead.
Your machine identity client secret.
**This field is deprecated and will be removed in future versions.** Please use the `auth.universalAuth.clientSecret` field instead.
An access token obtained from the machine identity login endpoint.
**This field is deprecated and will be removed in future versions.** Please use the `auth.accessToken` field instead.
Time-to-live (in seconds) for refreshing cached secrets.
If manually set to 0, caching will be disabled, this is not recommended.
Your self-hosted absolute site URL including the protocol (e.g. `https://app.infisical.com`)
The level of logs you wish to log The logs are derived from Rust, as we have written our base SDK in Rust.
Optionally provide a path to a custom SSL certificate file. This can be substituted by setting the `INFISICAL_SSL_CERTIFICATE` environment variable to the contents of the certificate.
The authentication object to use for the client. This is required unless you're using environment variables.
### Authentication
The SDK supports a variety of authentication methods. The most common authentication method is Universal Auth, which uses a client ID and client secret to authenticate.
#### Universal Auth
**Using environment variables**
- `INFISICAL_UNIVERSAL_AUTH_CLIENT_ID` - Your machine identity client ID.
- `INFISICAL_UNIVERSAL_AUTH_CLIENT_SECRET` - Your machine identity client secret.
**Using the SDK directly**
```js
const client = new InfisicalClient({
auth: {
universalAuth: {
clientId: "YOUR_CLIENT_ID",
clientSecret: "YOUR_CLIENT_SECRET"
}
}
});
```
#### GCP ID Token Auth
Please note that this authentication method will only work if you're running your application on Google Cloud Platform.
Please [read more](/documentation/platform/identities/gcp-auth) about this authentication method.
**Using environment variables**
- `INFISICAL_GCP_AUTH_IDENTITY_ID` - Your Infisical Machine Identity ID.
**Using the SDK directly**
```js
const client = new InfisicalClient({
auth: {
gcpIdToken: {
identityId: "YOUR_IDENTITY_ID"
}
}
});
```
#### GCP IAM Auth
**Using environment variables**
- `INFISICAL_GCP_IAM_AUTH_IDENTITY_ID` - Your Infisical Machine Identity ID.
- `INFISICAL_GCP_IAM_SERVICE_ACCOUNT_KEY_FILE_PATH` - The path to your GCP service account key file.
**Using the SDK directly**
```js
const client = new InfisicalClient({
auth: {
gcpIam: {
identityId: "YOUR_IDENTITY_ID",
serviceAccountKeyFilePath: "./path/to/your/service-account-key.json"
}
}
});
```
#### AWS IAM Auth
Please note that this authentication method will only work if you're running your application on AWS.
Please [read more](/documentation/platform/identities/aws-auth) about this authentication method.
**Using environment variables**
- `INFISICAL_AWS_IAM_AUTH_IDENTITY_ID` - Your Infisical Machine Identity ID.
**Using the SDK directly**
```js
const client = new InfisicalClient({
auth: {
awsIam: {
identityId: "YOUR_IDENTITY_ID"
}
}
});
```
#### Azure Auth
Please note that this authentication method will only work if you're running your application on Azure.
Please [read more](/documentation/platform/identities/azure-auth) about this authentication method.
**Using environment variables**
- `INFISICAL_AZURE_AUTH_IDENTITY_ID` - Your Infisical Machine Identity ID.
**Using the SDK directly**
```js
const client = new InfisicalClient({
auth: {
azure: {
identityId: "YOUR_IDENTITY_ID"
}
}
});
```
#### Kubernetes Auth
Please note that this authentication method will only work if you're running your application on Kubernetes.
Please [read more](/documentation/platform/identities/kubernetes-auth) about this authentication method.
**Using environment variables**
- `INFISICAL_KUBERNETES_IDENTITY_ID` - Your Infisical Machine Identity ID.
- `INFISICAL_KUBERNETES_SERVICE_ACCOUNT_TOKEN_PATH_ENV_NAME` - The environment variable name that contains the path to the service account token. This is optional and will default to `/var/run/secrets/kubernetes.io/serviceaccount/token`.
**Using the SDK directly**
```js
const client = new InfisicalClient({
auth: {
kubernetes: {
identityId: "YOUR_IDENTITY_ID",
serviceAccountTokenPathEnvName: "/var/run/secrets/kubernetes.io/serviceaccount/token" // Optional
}
}
});
```
### Caching
To reduce the number of API requests, the SDK temporarily stores secrets it retrieves. By default, a secret remains cached for 5 minutes after it's first fetched. Each time it's fetched again, this 5-minute timer resets. You can adjust this caching duration by setting the "cacheTtl" option when creating the client.
## Working with Secrets
### client.listSecrets(options)
```js
const secrets = await client.listSecrets({
environment: "dev",
projectId: "PROJECT_ID",
path: "/foo/bar/",
includeImports: false
});
```
Retrieve all secrets within the Infisical project and environment that client is connected to
#### Parameters
The slug name (dev, prod, etc) of the environment from where secrets should be fetched from.
The project ID where the secret lives in.
The path from where secrets should be fetched from.
Whether or not to set the fetched secrets to the process environment. If true, you can access the secrets like so `process.env["SECRET_NAME"]`.
Whether or not to fetch secrets recursively from the specified path. Please note that there's a 20-depth limit for recursive fetching.
Whether or not to expand secret references in the fetched secrets. Read about [secret reference](/documentation/platform/secret-reference)
Whether or not to include imported secrets from the current path. Read about [secret import](/documentation/platform/secret-reference)
### client.getSecret(options)
```js
const secret = await client.getSecret({
environment: "dev",
projectId: "PROJECT_ID",
secretName: "API_KEY",
path: "/",
type: "shared"
});
```
Retrieve a secret from Infisical.
By default, `getSecret()` fetches and returns a shared secret.
#### Parameters
The key of the secret to retrieve.
The project ID where the secret lives in.
The slug name (dev, prod, etc) of the environment from where secrets should be fetched from.
The path from where secret should be fetched from.
The type of the secret. Valid options are "shared" or "personal". If not specified, the default value is "shared".
Whether or not to include imported secrets from the current path. Read about [secret import](/documentation/platform/secret-reference)
Whether or not to expand secret references in the fetched secrets. Read about [secret reference](/documentation/platform/secret-reference)
### client.createSecret(options)
```js
const newApiKey = await client.createSecret({
projectId: "PROJECT_ID",
environment: "dev",
secretName: "API_KEY",
secretValue: "SECRET VALUE",
path: "/",
type: "shared"
});
```
Create a new secret in Infisical.
The key of the secret to create.
The value of the secret.
The project ID where the secret lives in.
The slug name (dev, prod, etc) of the environment from where secrets should be fetched from.
The path from where secret should be created.
The type of the secret. Valid options are "shared" or "personal". If not specified, the default value is "shared".
### client.updateSecret(options)
```js
const updatedApiKey = await client.updateSecret({
secretName: "API_KEY",
secretValue: "NEW SECRET VALUE",
projectId: "PROJECT_ID",
environment: "dev",
path: "/",
type: "shared"
});
```
Update an existing secret in Infisical.
#### Parameters
The key of the secret to update.
The new value of the secret.
The project ID where the secret lives in.
The slug name (dev, prod, etc) of the environment from where secrets should be fetched from.
The path from where secret should be updated.
The type of the secret. Valid options are "shared" or "personal". If not specified, the default value is "shared".
### client.deleteSecret(options)
```js
const deletedSecret = await client.deleteSecret({
secretName: "API_KEY",
environment: "dev",
projectId: "PROJECT_ID",
path: "/",
type: "shared"
});
```
Delete a secret in Infisical.
The key of the secret to update.
The project ID where the secret lives in.
The slug name (dev, prod, etc) of the environment from where secrets should be fetched from.
The path from where secret should be deleted.
The type of the secret. Valid options are "shared" or "personal". If not specified, the default value is "shared".
## Cryptography
### Create a symmetric key
Create a base64-encoded, 256-bit symmetric key to be used for encryption/decryption.
```js
const key = client.createSymmetricKey();
```
#### Returns (string)
`key` (string): A base64-encoded, 256-bit symmetric key, that can be used for encryption/decryption purposes.
### Encrypt symmetric
```js
const { iv, tag, ciphertext } = await client.encryptSymmetric({
key: key,
plaintext: "Infisical is awesome!",
})
```
#### Parameters
The plaintext you want to encrypt.
The symmetric key to use for encryption.
#### Returns (object)
`tag` (string): A base64-encoded, 128-bit authentication tag.
`iv` (string): A base64-encoded, 96-bit initialization vector.
`ciphertext` (string): A base64-encoded, encrypted ciphertext.
### Decrypt symmetric
```js
const decryptedString = await client.decryptSymmetric({
key: key,
iv: iv,
tag: tag,
ciphertext: ciphertext,
});
```
#### Parameters
The ciphertext you want to decrypt.
The symmetric key to use for encryption.
The initialization vector to use for decryption.
The authentication tag to use for decryption.
#### Returns (string)
`plaintext` (string): The decrypted plaintext.
*/}
# Infisical Python SDK
{/* If you're working with Python, the official [infisical-python](https://github.com/Infisical/sdk/edit/main/crates/infisical-py) package is the easiest way to fetch and work with secrets for your application.
- [PyPi Package](https://pypi.org/project/infisical-python/)
- [Github Repository](https://github.com/Infisical/sdk/edit/main/crates/infisical-py)
## Basic Usage
```py
from flask import Flask
from infisical_client import ClientSettings, InfisicalClient, GetSecretOptions, AuthenticationOptions, UniversalAuthMethod
app = Flask(__name__)
client = InfisicalClient(ClientSettings(
auth=AuthenticationOptions(
universal_auth=UniversalAuthMethod(
client_id="CLIENT_ID",
client_secret="CLIENT_SECRET",
)
)
))
@app.route("/")
def hello_world():
# access value
name = client.getSecret(options=GetSecretOptions(
environment="dev",
project_id="PROJECT_ID",
secret_name="NAME"
))
return f"Hello! My name is: {name.secret_value}"
```
This example demonstrates how to use the Infisical Python SDK with a Flask application. The application retrieves a secret named "NAME" and responds to requests with a greeting that includes the secret value.
We do not recommend hardcoding your [Machine Identity Tokens](/platform/identities/overview). Setting it as an environment variable would be best.
## Installation
Run `pip` to add `infisical-python` to your project
```console
$ pip install infisical-python
```
Note: You need Python 3.7+.
## Configuration
Import the SDK and create a client instance with your [Machine Identity](/api-reference/overview/authentication).
```py
from infisical_client import ClientSettings, InfisicalClient, AuthenticationOptions, UniversalAuthMethod
client = InfisicalClient(ClientSettings(
auth=AuthenticationOptions(
universal_auth=UniversalAuthMethod(
client_id="CLIENT_ID",
client_secret="CLIENT_SECRET",
)
)
))
```
#### Parameters
Your Infisical Client ID.
**This field is deprecated and will be removed in future versions.** Please use the `auth` field instead.
Your Infisical Client Secret.
**This field is deprecated and will be removed in future versions.** Please use the `auth` field instead.
If you want to directly pass an access token obtained from the authentication endpoints, you can do so.
**This field is deprecated and will be removed in future versions.** Please use the `auth` field instead.
Time-to-live (in seconds) for refreshing cached secrets.
If manually set to 0, caching will be disabled, this is not recommended.
Your self-hosted absolute site URL including the protocol (e.g. `https://app.infisical.com`)
Optionally provide a path to a custom SSL certificate file. This can be substituted by setting the `INFISICAL_SSL_CERTIFICATE` environment variable to the contents of the certificate.
The authentication object to use for the client. This is required unless you're using environment variables.
### Authentication
The SDK supports a variety of authentication methods. The most common authentication method is Universal Auth, which uses a client ID and client secret to authenticate.
#### Universal Auth
**Using environment variables**
- `INFISICAL_UNIVERSAL_AUTH_CLIENT_ID` - Your machine identity client ID.
- `INFISICAL_UNIVERSAL_AUTH_CLIENT_SECRET` - Your machine identity client secret.
**Using the SDK directly**
```python3
from infisical_client import ClientSettings, InfisicalClient, AuthenticationOptions, UniversalAuthMethod
client = InfisicalClient(ClientSettings(
auth=AuthenticationOptions(
universal_auth=UniversalAuthMethod(
client_id="CLIENT_ID",
client_secret="CLIENT_SECRET",
)
)
))
```
#### GCP ID Token Auth
Please note that this authentication method will only work if you're running your application on Google Cloud Platform.
Please [read more](/documentation/platform/identities/gcp-auth) about this authentication method.
**Using environment variables**
- `INFISICAL_GCP_AUTH_IDENTITY_ID` - Your Infisical Machine Identity ID.
**Using the SDK directly**
```py
from infisical_client import ClientSettings, InfisicalClient, AuthenticationOptions, GCPIDTokenAuthMethod
client = InfisicalClient(ClientSettings(
auth=AuthenticationOptions(
gcp_id_token=GCPIDTokenAuthMethod(
identity_id="MACHINE_IDENTITY_ID",
)
)
))
```
#### GCP IAM Auth
**Using environment variables**
- `INFISICAL_GCP_IAM_AUTH_IDENTITY_ID` - Your Infisical Machine Identity ID.
- `INFISICAL_GCP_IAM_SERVICE_ACCOUNT_KEY_FILE_PATH` - The path to your GCP service account key file.
**Using the SDK directly**
```py
from infisical_client import ClientSettings, InfisicalClient, AuthenticationOptions, GCPIamAuthMethod
client = InfisicalClient(ClientSettings(
auth=AuthenticationOptions(
gcp_iam=GCPIamAuthMethod(
identity_id="MACHINE_IDENTITY_ID",
service_account_key_file_path="./path/to/service_account_key.json"
)
)
))
```
#### AWS IAM Auth
Please note that this authentication method will only work if you're running your application on AWS.
Please [read more](/documentation/platform/identities/aws-auth) about this authentication method.
**Using environment variables**
- `INFISICAL_AWS_IAM_AUTH_IDENTITY_ID` - Your Infisical Machine Identity ID.
**Using the SDK directly**
```py
from infisical_client import ClientSettings, InfisicalClient, AuthenticationOptions, AWSIamAuthMethod
client = InfisicalClient(ClientSettings(
auth=AuthenticationOptions(
aws_iam=AWSIamAuthMethod(identity_id="MACHINE_IDENTITY_ID")
)
))
```
#### Azure Auth
Please note that this authentication method will only work if you're running your application on Azure.
Please [read more](/documentation/platform/identities/azure-auth) about this authentication method.
**Using environment variables**
- `INFISICAL_AZURE_AUTH_IDENTITY_ID` - Your Infisical Machine Identity ID.
**Using the SDK directly**
```python
from infisical_client import InfisicalClient, ClientSettings, AuthenticationOptions, AzureAuthMethod
kubernetes_client = InfisicalClient(ClientSettings(
auth=AuthenticationOptions(
azure=AzureAuthMethod(
identity_id="YOUR_IDENTITY_ID",
)
)
))
```
#### Kubernetes Auth
Please note that this authentication method will only work if you're running your application on Kubernetes.
Please [read more](/documentation/platform/identities/kubernetes-auth) about this authentication method.
**Using environment variables**
- `INFISICAL_KUBERNETES_IDENTITY_ID` - Your Infisical Machine Identity ID.
- `INFISICAL_KUBERNETES_SERVICE_ACCOUNT_TOKEN_PATH_ENV_NAME` - The environment variable name that contains the path to the service account token. This is optional and will default to `/var/run/secrets/kubernetes.io/serviceaccount/token`.
**Using the SDK directly**
```python
from infisical_client import InfisicalClient, ClientSettings, AuthenticationOptions, KubernetesAuthMethod
kubernetes_client = InfisicalClient(ClientSettings(
auth=AuthenticationOptions(
kubernetes=KubernetesAuthMethod(
identity_id="YOUR_IDENTITY_ID",
service_account_token_path="/var/run/secrets/kubernetes.io/serviceaccount/token" # Optional
)
)
))
```
### Caching
To reduce the number of API requests, the SDK temporarily stores secrets it retrieves. By default, a secret remains cached for 5 minutes after it's first fetched. Each time it's fetched again, this 5-minute timer resets. You can adjust this caching duration by setting the "cache_ttl" option when creating the client.
## Working with Secrets
### client.listSecrets(options)
```py
client.listSecrets(options=ListSecretsOptions(
environment="dev",
project_id="PROJECT_ID"
))
```
Retrieve all secrets within the Infisical project and environment that client is connected to
#### Parameters
The slug name (dev, prod, etc) of the environment from where secrets should be fetched from.
The project ID where the secret lives in.
The path from where secrets should be fetched from.
Whether or not to set the fetched secrets to the process environment. If true, you can access the secrets like so `process.env["SECRET_NAME"]`.
Whether or not to fetch secrets recursively from the specified path. Please note that there's a 20-depth limit for recursive fetching.
Whether or not to expand secret references in the fetched secrets. Read about [secret reference](/documentation/platform/secret-reference)
Whether or not to include imported secrets from the current path. Read about [secret import](/documentation/platform/secret-reference)
### client.getSecret(options)
```py
secret = client.getSecret(options=GetSecretOptions(
environment="dev",
project_id="PROJECT_ID",
secret_name="API_KEY"
))
value = secret.secret_value # get its value
```
By default, `getSecret()` fetches and returns a shared secret. If not found, it returns a personal secret.
#### Parameters
The key of the secret to retrieve
Whether or not to include imported secrets from the current path. Read about [secret import](/documentation/platform/secret-reference)
The slug name (dev, prod, etc) of the environment from where secrets should be fetched from.
The project ID where the secret lives in.
The path from where secret should be fetched from.
The type of the secret. Valid options are "shared" or "personal". If not specified, the default value is "personal".
Whether or not to include imported secrets from the current path. Read about [secret import](/documentation/platform/secret-reference)
Whether or not to expand secret references in the fetched secrets. Read about [secret reference](/documentation/platform/secret-reference)
### client.createSecret(options)
```py
api_key = client.createSecret(options=CreateSecretOptions(
secret_name="API_KEY",
secret_value="Some API Key",
environment="dev",
project_id="PROJECT_ID"
))
```
Create a new secret in Infisical.
#### Parameters
The key of the secret to create.
The value of the secret.
The project ID where the secret lives in.
The slug name (dev, prod, etc) of the environment from where secrets should be fetched from.
The path from where secret should be created.
The type of the secret. Valid options are "shared" or "personal". If not specified, the default value is "shared".
### client.updateSecret(options)
```py
client.updateSecret(options=UpdateSecretOptions(
secret_name="API_KEY",
secret_value="NEW_VALUE",
environment="dev",
project_id="PROJECT_ID"
))
```
Update an existing secret in Infisical.
#### Parameters
The key of the secret to update.
The new value of the secret.
The project ID where the secret lives in.
The slug name (dev, prod, etc) of the environment from where secrets should be fetched from.
The path from where secret should be updated.
The type of the secret. Valid options are "shared" or "personal". If not specified, the default value is "shared".
### client.deleteSecret(options)
```py
client.deleteSecret(options=DeleteSecretOptions(
environment="dev",
project_id="PROJECT_ID",
secret_name="API_KEY"
))
```
Delete a secret in Infisical.
#### Parameters
The key of the secret to update.
The project ID where the secret lives in.
The slug name (dev, prod, etc) of the environment from where secrets should be fetched from.
The path from where secret should be deleted.
The type of the secret. Valid options are "shared" or "personal". If not specified, the default value is "shared".
## Cryptography
### Create a symmetric key
Create a base64-encoded, 256-bit symmetric key to be used for encryption/decryption.
```py
key = client.createSymmetricKey()
```
#### Returns (string)
`key` (string): A base64-encoded, 256-bit symmetric key, that can be used for encryption/decryption purposes.
### Encrypt symmetric
```py
encryptOptions = EncryptSymmetricOptions(
key=key,
plaintext="Infisical is awesome!"
)
encryptedData = client.encryptSymmetric(encryptOptions)
```
#### Parameters
The plaintext you want to encrypt.
The symmetric key to use for encryption.
#### Returns (object)
`tag` (string): A base64-encoded, 128-bit authentication tag. `iv` (string): A base64-encoded, 96-bit initialization vector. `ciphertext` (string): A base64-encoded, encrypted ciphertext.
### Decrypt symmetric
```py
decryptOptions = DecryptSymmetricOptions(
ciphertext=encryptedData.ciphertext,
iv=encryptedData.iv,
tag=encryptedData.tag,
key=key
)
decryptedString = client.decryptSymmetric(decryptOptions)
```
#### Parameters
The ciphertext you want to decrypt.
The symmetric key to use for encryption.
The initialization vector to use for decryption.
The authentication tag to use for decryption.
#### Returns (string)
`plaintext` (string): The decrypted plaintext. */}
# Infisical Ruby SDK
If you're working with Ruby , the official [Infisical Ruby SDK](https://github.com/infisical/sdk) package is the easiest way to fetch and work with secrets for your application.
* [Ruby Package](https://rubygems.org/gems/infisical-sdk)
* [Github Repository](https://github.com/infisical/sdk)
## Basic Usage
```ruby
require 'infisical-sdk'
# 1. Create the Infisical client
infisical = InfisicalSDK::InfisicalClient.new('https://app.infisical.com')
infisical.auth.universal_auth(client_id: 'YOUR_CLIENT_ID', client_secret: 'YOUR_CLIENT_SECRET')
test_secret = infisical.secrets.get(
secret_name: 'API_KEY',
project_id: 'project-id',
environment: 'dev'
)
puts "Secret: #{single_test_secret}"
```
This example demonstrates how to use the Infisical Ruby SDK in a simple Ruby application. The application retrieves a secret named `API_KEY` from the `dev` environment of the `YOUR_PROJECT_ID` project.
We do not recommend hardcoding your [Machine Identity Tokens](/platform/identities/overview). Setting it as an environment variable would be best.
# Installation
```console
$ gem install infisical-sdk
```
# Configuration
Import the SDK and create a client instance.
```ruby
infisical = InfisicalSDK::InfisicalClient.new('https://app.infisical.com') # Optional parameter, default is https://api.infisical.com
```
### Client parameters
The URL of the Infisical API. Default is `https://api.infisical.com`.
How long the client should cache secrets for. Default is 5 minutes. Disable by setting to 0.
### Authentication
The SDK supports a variety of authentication methods. The most common authentication method is Universal Auth, which uses a client ID and client secret to authenticate.
#### Universal Auth
**Using environment variables**
Call `auth.universal_auth()` with empty arguments to use the following environment variables:
* `INFISICAL_UNIVERSAL_AUTH_CLIENT_ID` - Your machine identity client ID.
* `INFISICAL_UNIVERSAL_AUTH_CLIENT_SECRET` - Your machine identity client secret.
**Using the SDK directly**
```ruby
infisical.auth.universal_auth(client_id: 'your-client-id', client_secret: 'your-client-secret')
```
#### GCP ID Token Auth
Please note that this authentication method will only work if you're running your application on Google Cloud Platform.
Please [read more](/documentation/platform/identities/gcp-auth) about this authentication method.
**Using environment variables**
Call `.auth.gcp_id_token_auth()` with empty arguments to use the following environment variables:
* `INFISICAL_GCP_AUTH_IDENTITY_ID` - Your Infisical Machine Identity ID.
**Using the SDK directly**
```ruby
infisical.auth.gcp_id_token_auth(identity_id: 'MACHINE_IDENTITY_ID')
```
#### GCP IAM Auth
**Using environment variables**
Call `.auth.gcp_iam_auth()` with empty arguments to use the following environment variables:
* `INFISICAL_GCP_IAM_AUTH_IDENTITY_ID` - Your Infisical Machine Identity ID.
* `INFISICAL_GCP_IAM_SERVICE_ACCOUNT_KEY_FILE_PATH` - The path to your GCP service account key file.
**Using the SDK directly**
```ruby
infisical.auth.gcp_iam_auth(identity_id: 'MACHINE_IDENTITY_ID', service_account_key_file_path: 'SERVICE_ACCOUNT_KEY_FILE_PATH')
```
#### AWS IAM Auth
Please note that this authentication method will only work if you're running your application on AWS.
Please [read more](/documentation/platform/identities/aws-auth) about this authentication method.
**Using environment variables**
Call `.auth.aws_iam_auth()` with empty arguments to use the following environment variables:
* `INFISICAL_AWS_IAM_AUTH_IDENTITY_ID` - Your Infisical Machine Identity ID.
**Using the SDK directly**
```ruby
infisical.auth.aws_iam_auth(identity_id: 'MACHINE_IDENTITY_ID')
```
#### Azure Auth
Please note that this authentication method will only work if you're running your application on Azure.
Please [read more](/documentation/platform/identities/azure-auth) about this authentication method.
**Using environment variables**
Call `.auth.azure_auth()` with empty arguments to use the following environment variables:
* `INFISICAL_AZURE_AUTH_IDENTITY_ID` - Your Infisical Machine Identity ID.
**Using the SDK directly**
```ruby
infisical.auth.azure_auth(identity_id: 'MACHINE_IDENTITY_ID')
```
#### Kubernetes Auth
Please note that this authentication method will only work if you're running your application on Kubernetes.
Please [read more](/documentation/platform/identities/kubernetes-auth) about this authentication method.
**Using environment variables**
Call `.auth.kubernetes_auth()` with empty arguments to use the following environment variables:
* `INFISICAL_KUBERNETES_IDENTITY_ID` - Your Infisical Machine Identity ID.
* `INFISICAL_KUBERNETES_SERVICE_ACCOUNT_TOKEN_PATH_ENV_NAME` - The environment variable name that contains the path to the service account token. This is optional and will default to `/var/run/secrets/kubernetes.io/serviceaccount/token`.
**Using the SDK directly**
```ruby
# Service account token path will default to /var/run/secrets/kubernetes.io/serviceaccount/token if empty value is passed
infisical.auth.kubernetes_auth(identity_id: 'MACHINE_IDENTITY_ID', service_account_token_path: nil)
```
## Working with Secrets
### client.secrets.list(options)
```ruby
secrets = infisical.secrets.list(
project_id: 'PROJECT_ID',
environment: 'dev',
path: '/foo/bar',
)
```
Retrieve all secrets within the Infisical project and environment that client is connected to
#### Parameters
The slug name (dev, prod, etc) of the environment from where secrets should be fetched from.
The project ID where the secret lives in.
The path from where secrets should be fetched from.
Whether or not to set the fetched secrets to the process environment. If true, you can access the secrets like so `System.getenv("SECRET_NAME")`.
Whether or not to include imported secrets from the current path. Read about [secret import](/documentation/platform/secret-reference)
Whether or not to fetch secrets recursively from the specified path. Please note that there's a 20-depth limit for recursive fetching.
Whether or not to expand secret references in the fetched secrets. Read about [secret reference](/documentation/platform/secret-reference)
### client.secrets.get(options)
```ruby
secret = infisical.secrets.get(
secret_name: 'API_KEY',
project_id: project_id,
environment: env_slug
)
```
Retrieve a secret from Infisical.
By default, `Secrets().Retrieve()` fetches and returns a shared secret.
#### Parameters
The key of the secret to retrieve.
The project ID where the secret lives in.
The slug name (dev, prod, etc) of the environment from where secrets should be fetched from.
The path from where secret should be fetched from.
The type of the secret. Valid options are "shared" or "personal". If not specified, the default value is "shared".
### client.secrets.create(options)
```ruby
new_secret = infisical.secrets.create(
secret_name: 'NEW_SECRET',
secret_value: 'SECRET_VALUE',
project_id: 'PROJECT_ID',
environment: 'dev',
)
```
Create a new secret in Infisical.
#### Parameters
The key of the secret to create.
The value of the secret.
A comment for the secret.
The project ID where the secret lives in.
The slug name (dev, prod, etc) of the environment from where secrets should be fetched from.
The path from where secret should be created.
The type of the secret. Valid options are "shared" or "personal". If not specified, the default value is "shared".
### client.secrets.update(options)
```ruby
updated_secret = infisical.secrets.update(
secret_name: 'SECRET_KEY_TO_UPDATE',
secret_value: 'NEW_SECRET_VALUE',
project_id: 'PROJECT_ID',
environment: 'dev',
)
```
Update an existing secret in Infisical.
#### Parameters
The key of the secret to update.
The new value of the secret.
Whether or not to skip multiline encoding for the new secret value.
The project ID where the secret lives in.
The slug name (dev, prod, etc) of the environment from where secrets should be fetched from.
The path from where secret should be updated.
The type of the secret. Valid options are "shared" or "personal". If not specified, the default value is "shared".
### client.secrets.delete(options)
```ruby
deleted_secret = infisical.secrets.delete(
secret_name: 'SECRET_TO_DELETE',
project_id: 'PROJECT_ID',
environment: 'dev',
)
```
Delete a secret in Infisical.
#### Parameters
The key of the secret to update.
The project ID where the secret lives in.
The slug name (dev, prod, etc) of the environment from where secrets should be fetched from.
The path from where secret should be deleted.
The type of the secret. Valid options are "shared" or "personal". If not specified, the default value is "shared".
## Cryptography
### Create a symmetric key
Create a base64-encoded, 256-bit symmetric key to be used for encryption/decryption.
```ruby
key = infisical.cryptography.create_symmetric_key
```
#### Returns (string)
`key` (string): A base64-encoded, 256-bit symmetric key, that can be used for encryption/decryption purposes.
### Encrypt symmetric
```ruby
encrypted_data = infisical.cryptography.encrypt_symmetric(data: "Hello World!", key: key)
```
#### Parameters
The plaintext you want to encrypt.
The symmetric key to use for encryption.
#### Returns (object)
`tag` (string): A base64-encoded, 128-bit authentication tag.
`iv` (string): A base64-encoded, 96-bit initialization vector.
`ciphertext` (string): A base64-encoded, encrypted ciphertext.
### Decrypt symmetric
```ruby
decrypted_data = infisical.cryptography.decrypt_symmetric(
ciphertext: encrypted_data['ciphertext'],
iv: encrypted_data['iv'],
tag: encrypted_data['tag'],
key: key
)
```
#### Parameters
The ciphertext you want to decrypt.
The symmetric key to use for encryption.
The initialization vector to use for decryption.
The authentication tag to use for decryption.
#### Returns (string)
`Plaintext` (string): The decrypted plaintext.
# SDKs
From local development to production, Infisical SDKs provide the easiest way for your app to fetch back secrets from Infisical on demand.
* Install and initialize a language-specific client SDK into your application
* Provision the client scoped-access to a project and environment in Infisical
* Fetch secrets on demand
Manage secrets for your Node application on demand
Manage secrets for your Python application on demand
Manage secrets for your Java application on demand
Manage secrets for your Go application on demand
Manage secrets for your C#/.NET application on demand
Manage secrets for your Ruby application on demand
## FAQ
The client SDK caches every secret and implements a 5-minute waiting period before re-requesting it. The waiting period can be controlled by
setting the `cacheTTL` parameter at the time of initializing the client.
Note: The exact parameter name may differ depending on the language.
The SDK caches every secret and falls back to the cached value if a request fails. If no cached
value ever-existed, the SDK falls back to whatever value is on the process environment.
Yes you can! The client SDK provides a method to attach the secrets to your process environment. When using the `listSecrets()` method, you
can pass a `attachToProcessEnv` parameter, which tells the SDK to attach all the found secrets to your process environment.
Note: The exact parameter name may differ depending on the language.
# Configurations
Read how to configure environment variables for self-hosted Infisical.
Infisical accepts all configurations via environment variables. For a minimal self-hosted instance, at least `ENCRYPTION_KEY`, `AUTH_SECRET`, `DB_CONNECTION_URI` and `REDIS_URL` must be defined.
However, you can configure additional settings to activate more features as needed.
## General platform
Used to configure platform-specific security and operational settings
Must be a random 16 byte hex string. Can be generated with `openssl rand -hex
16`
Must be a random 32 byte base64 string. Can be generated with `openssl rand -base64 32`
Must be an absolute URL including the protocol (e.g.
[https://app.infisical.com](https://app.infisical.com)).
Specifies the internal port on which the application listens.
Telemetry helps us improve Infisical but if you want to disable it you may set
this to `false`.
## Data Layer
The platform utilizes Postgres to persist all of its data and Redis for caching and backgroud tasks
Postgres database connection string.
Configure the SSL certificate for securing a Postgres connection by first encoding it in base64.
Use the command below to encode your certificate:
`echo "" | base64`
Redis connection string.
Postgres database read replica connection strings. It accepts a JSON string.
```
DB_READ_REPLICAS=[{"DB_CONNECTION_URI":""}]
```
Postgres read replica connection string.
Configure the SSL certificate for securing a Postgres replica connection by first encoding it in base64.
Use the command below to encode your certificate:
`echo "" | base64`
If not provided it will use master SSL certificate.
## Email service
Without email configuration, Infisical's core functions like sign-up/login and secret operations work, but this disables multi-factor authentication, email invites for projects, alerts for suspicious logins, and all other email-dependent features.
Hostname to connect to for establishing SMTP connections
Port to connect to for establishing SMTP connections
Credential to connect to host (e.g. [team@infisical.com](mailto:team@infisical.com))
Credential to connect to host
Email address to be used for sending emails
Name label to be used in From field (e.g. Team)
If this is `true` and `SMTP_PORT` is not 465 then TLS is not used even if the
server supports STARTTLS extension.
If this is `true` and `SMTP_PORT` is not 465 then Infisical tries to use
STARTTLS even if the server does not advertise support for it. If the
connection can not be encrypted then message is not sent.
If this is `true`, Infisical will validate the server's SSL/TLS certificate and reject the connection if the certificate is invalid or not trusted. If set to `false`, the client will accept the server's certificate regardless of its validity, which can be useful in development or testing environments but is not recommended for production use.
1. Create an account and configure [SendGrid](https://sendgrid.com) to send emails.
2. Create a SendGrid API Key under Settings > [API Keys](https://app.sendgrid.com/settings/api_keys)
3. Set a name for your API Key, we recommend using "Infisical," and select the "Restricted Key" option. You will need to enable the "Mail Send" permission as shown below:
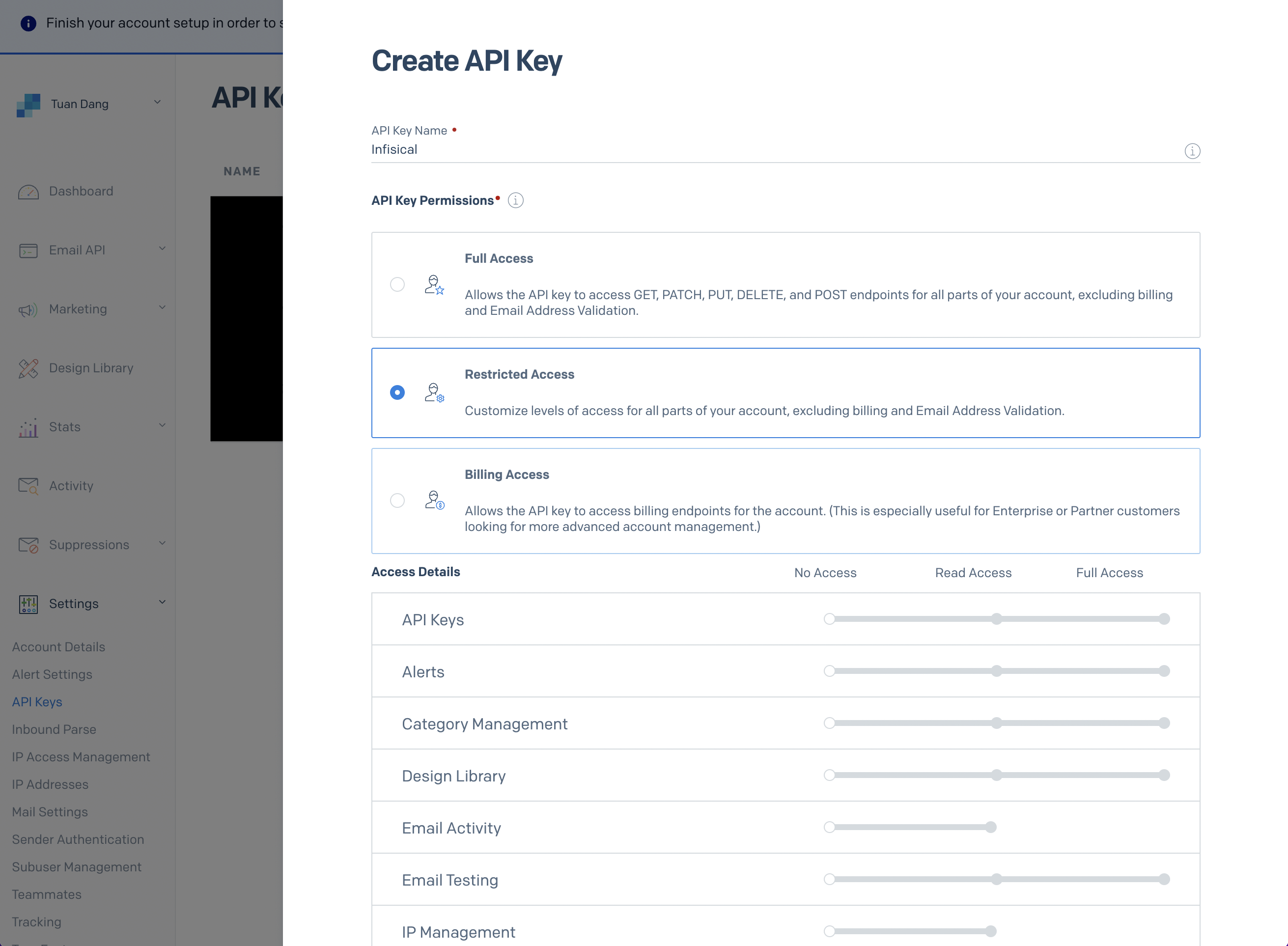
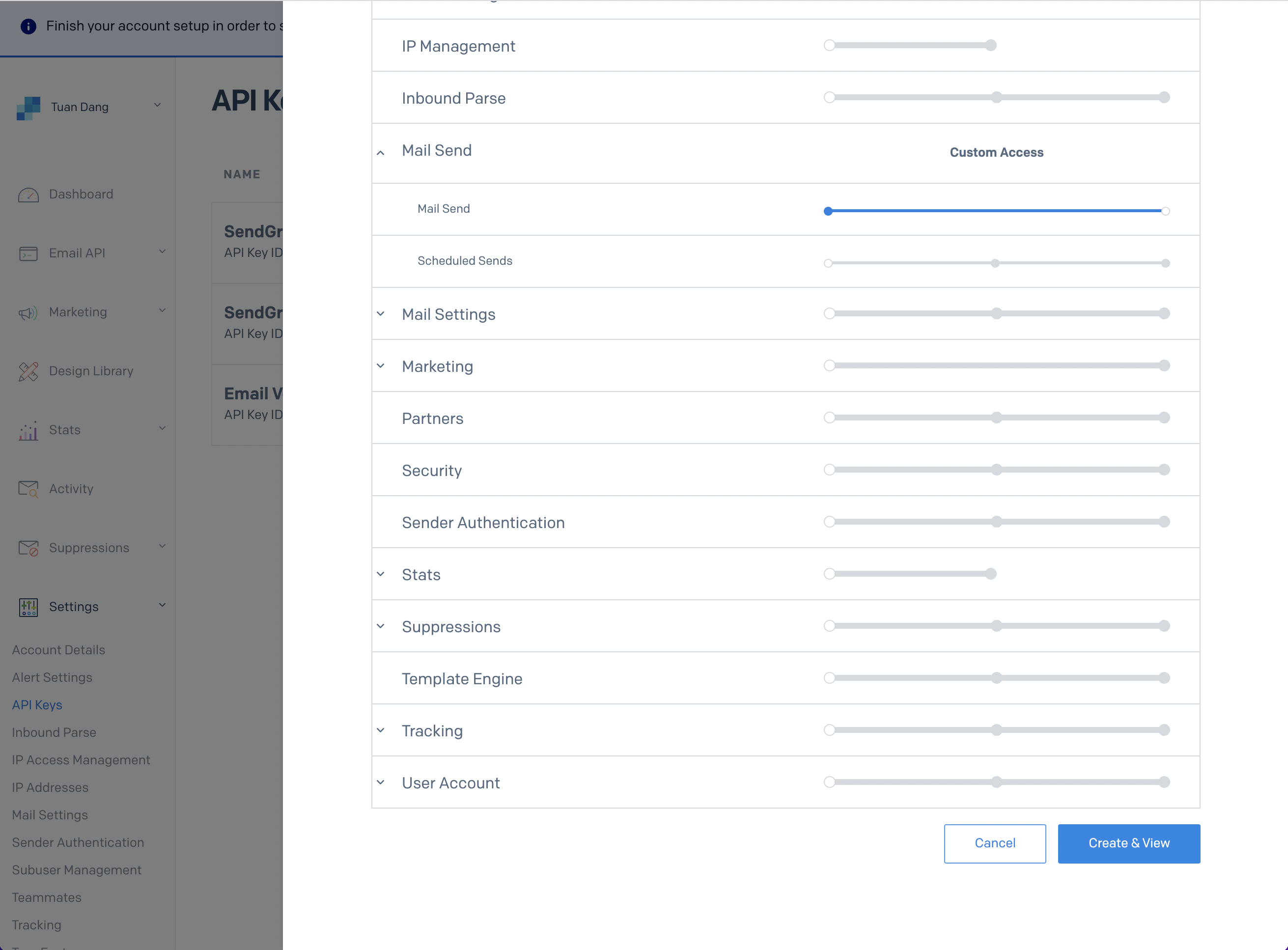
4. With the API Key, you can now set your SMTP environment variables:
```
SMTP_HOST=smtp.sendgrid.net
SMTP_USERNAME=apikey
SMTP_PASSWORD=SG.rqFsfjxYPiqE1lqZTgD_lz7x8IVLx # your SendGrid API Key from step above
SMTP_PORT=587
SMTP_FROM_ADDRESS=hey@example.com # your email address being used to send out emails
SMTP_FROM_NAME=Infisical
```
Remember that you will need to restart Infisical for this to work properly.
1. Create an account and configure [Mailgun](https://www.mailgun.com) to send emails.
2. Obtain your Mailgun credentials in Sending > Overview > SMTP
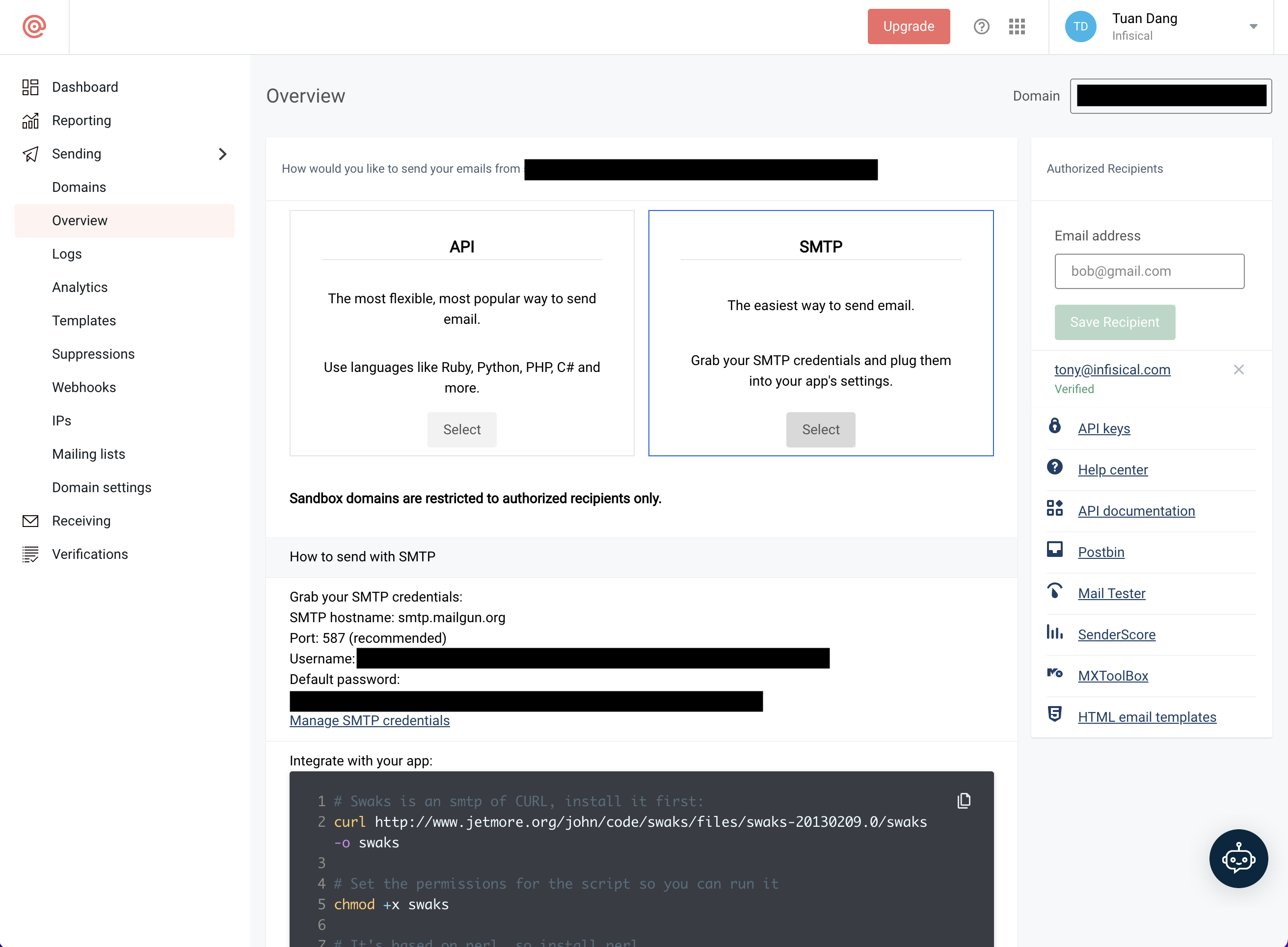
3. With your Mailgun credentials, you can now set up your SMTP environment variables:
```
SMTP_HOST=smtp.mailgun.org # obtained from credentials page
SMTP_USERNAME=postmaster@example.mailgun.org # obtained from credentials page
SMTP_PASSWORD=password # obtained from credentials page
SMTP_PORT=587
SMTP_FROM_ADDRESS=hey@example.com # your email address being used to send out emails
SMTP_FROM_NAME=Infisical
```
This will be used to verify the email you are sending from.
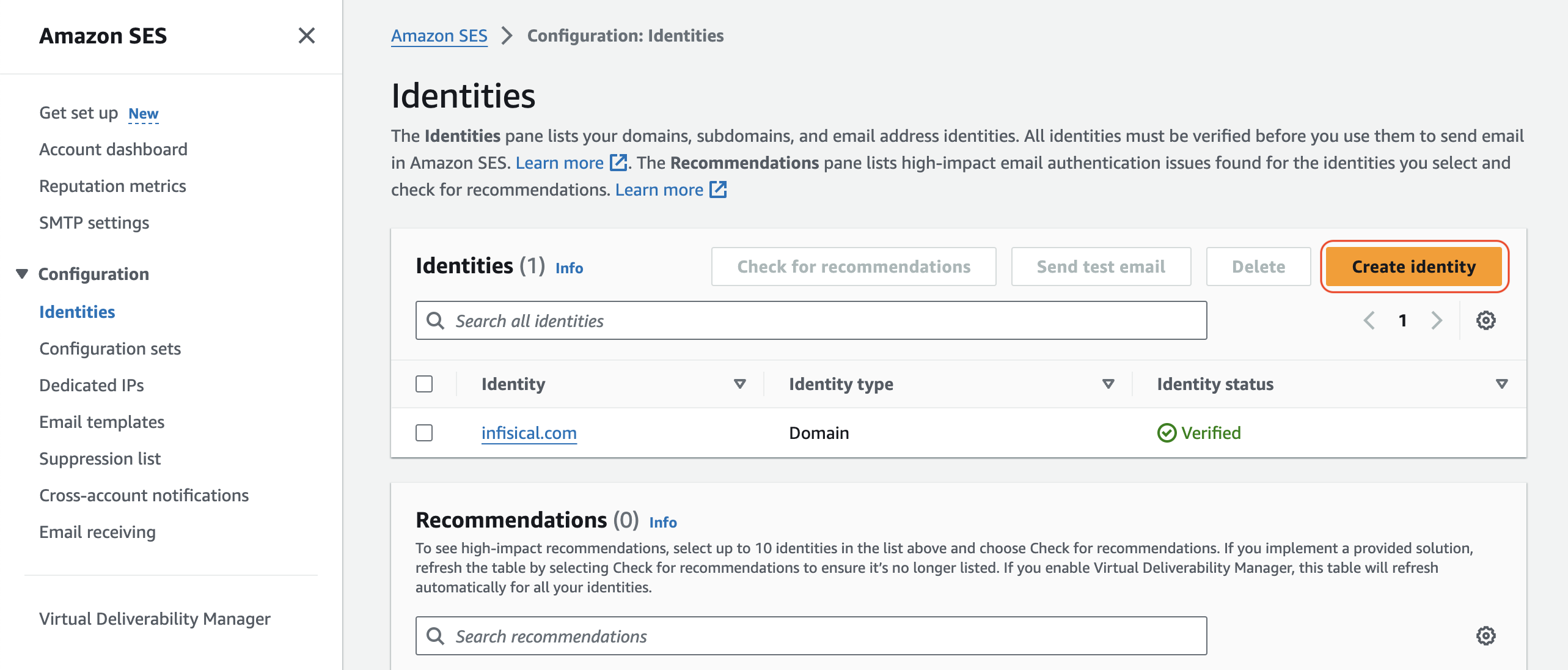
If you AWS SES is under sandbox mode, you will only be able to send emails to verified identies.
Create an IAM user for SMTP authentication and obtain SMTP credentials in SMTP settings > Create SMTP credentials
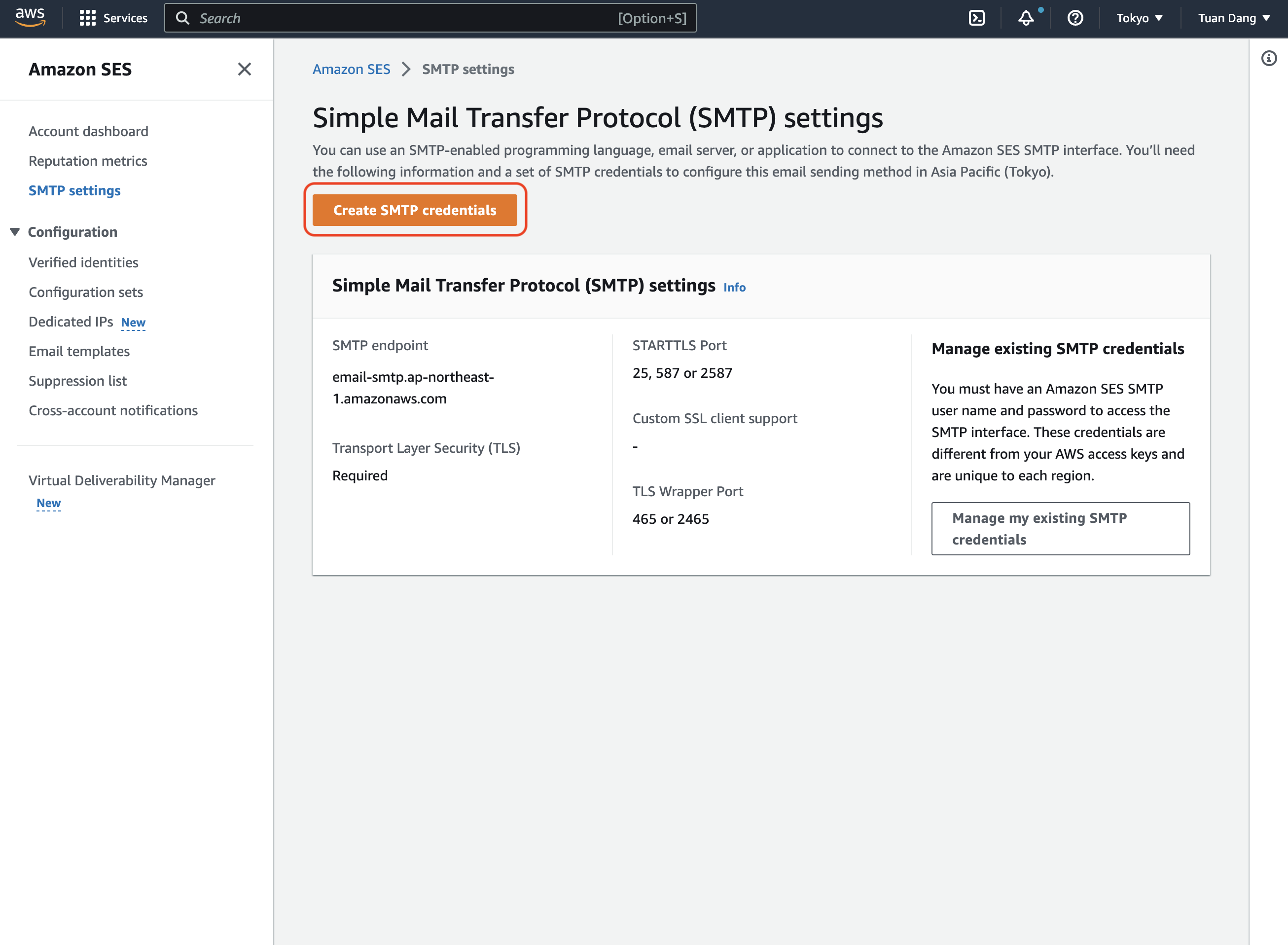
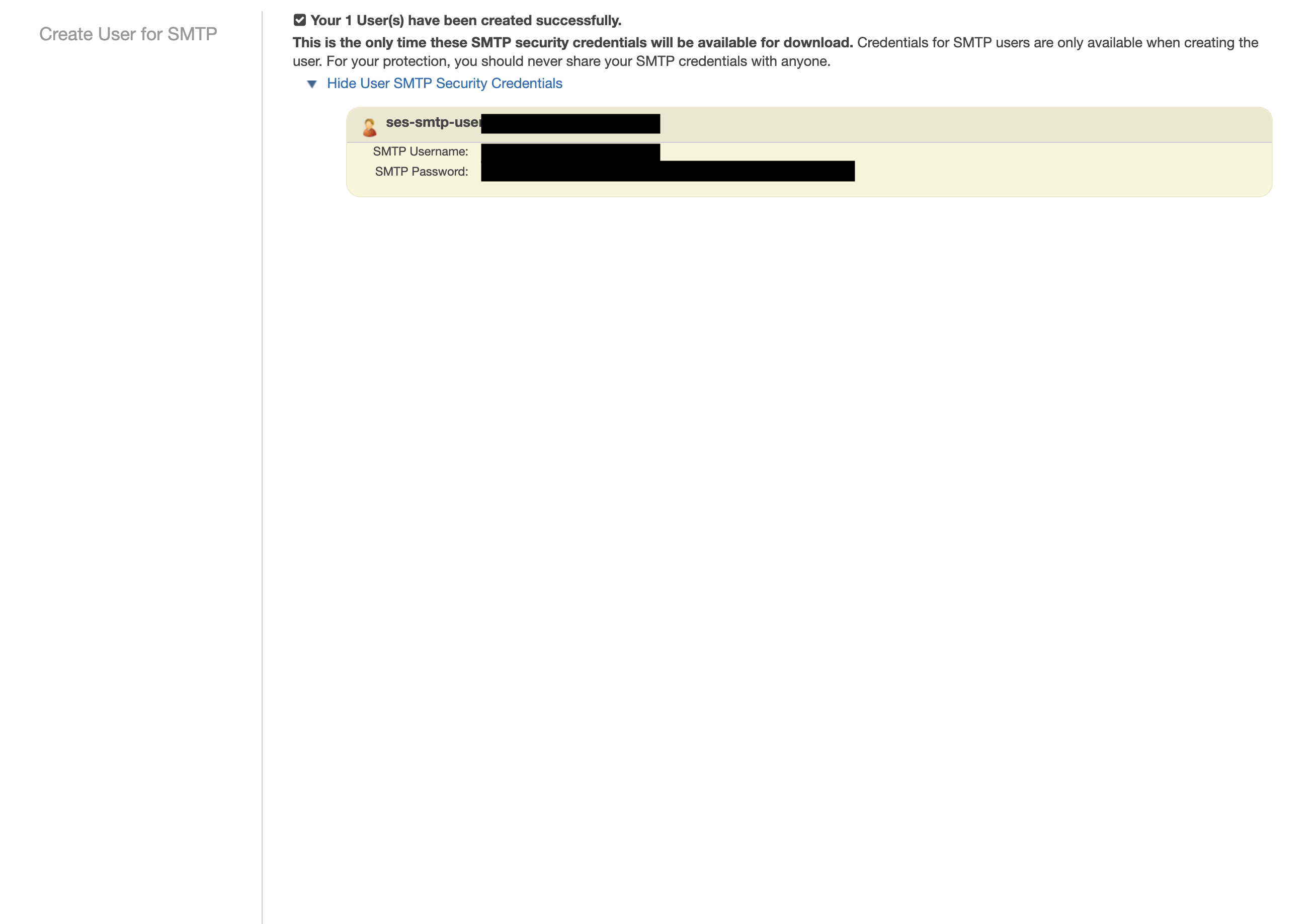
With your AWS SES SMTP credentials, you can now set up your SMTP environment variables for your Infisical instance.
```
SMTP_HOST=email-smtp.ap-northeast-1.amazonaws.com # SMTP endpoint obtained from SMTP settings
SMTP_USERNAME=xxx # your SMTP username
SMTP_PASSWORD=xxx # your SMTP password
SMTP_PORT=465
SMTP_FROM_ADDRESS=hey@example.com # your email address being used to send out emails
SMTP_FROM_NAME=Infisical
```
Remember that you will need to restart Infisical for this to work properly.
1. Create an account and configure [SocketLabs](https://www.socketlabs.com/) to send emails.
2. From the dashboard, navigate to SMTP Credentials > SMTP & APIs > SMTP Credentials to obtain your SocketLabs SMTP credentials.
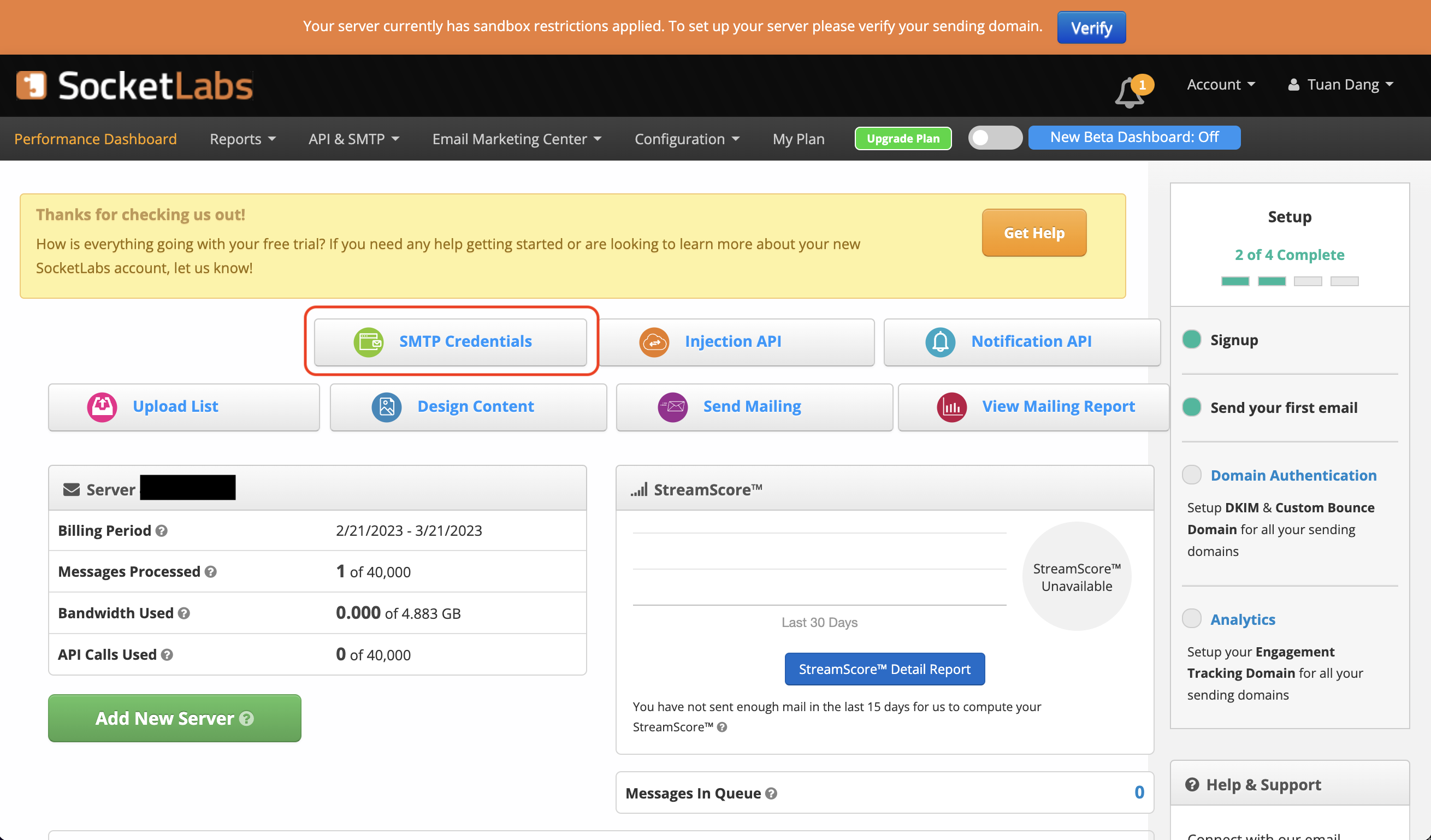
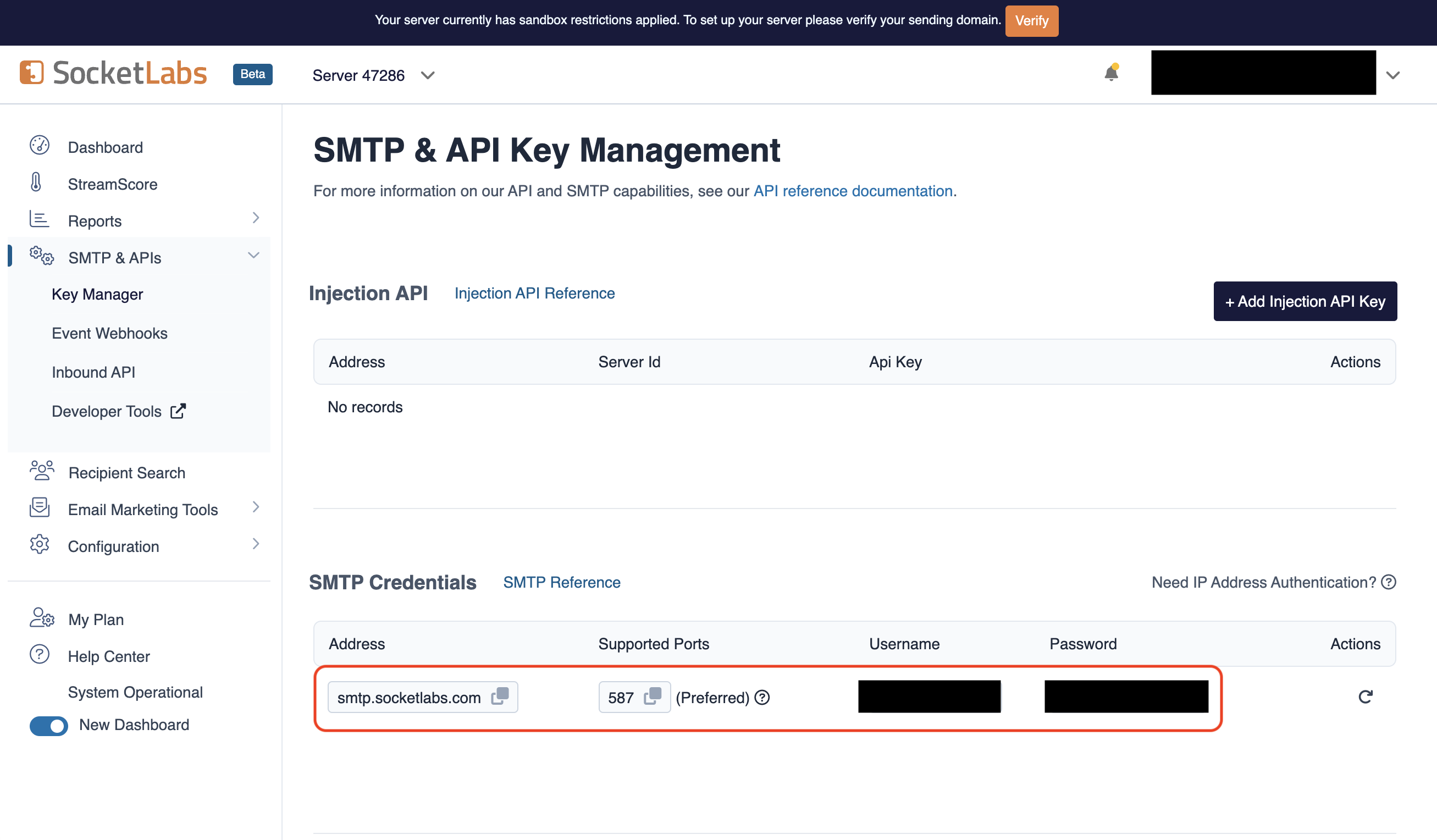
3. With your SocketLabs SMTP credentials, you can now set up your SMTP environment variables:
```
SMTP_HOST=smtp.socketlabs.com
SMTP_USERNAME=username # obtained from your credentials
SMTP_PASSWORD=password # obtained from your credentials
SMTP_PORT=587
SMTP_FROM_ADDRESS=hey@example.com # your email address being used to send out emails
SMTP_FROM_NAME=Infisical
```
{" "}
The `SMTP_FROM_ADDRESS` environment variable should be an email for an
authenticated domain under Configuration > Domain Management in SocketLabs.
For example, if you're using SocketLabs in sandbox mode, then you may use an
email like `team@sandbox.socketlabs.dev`.
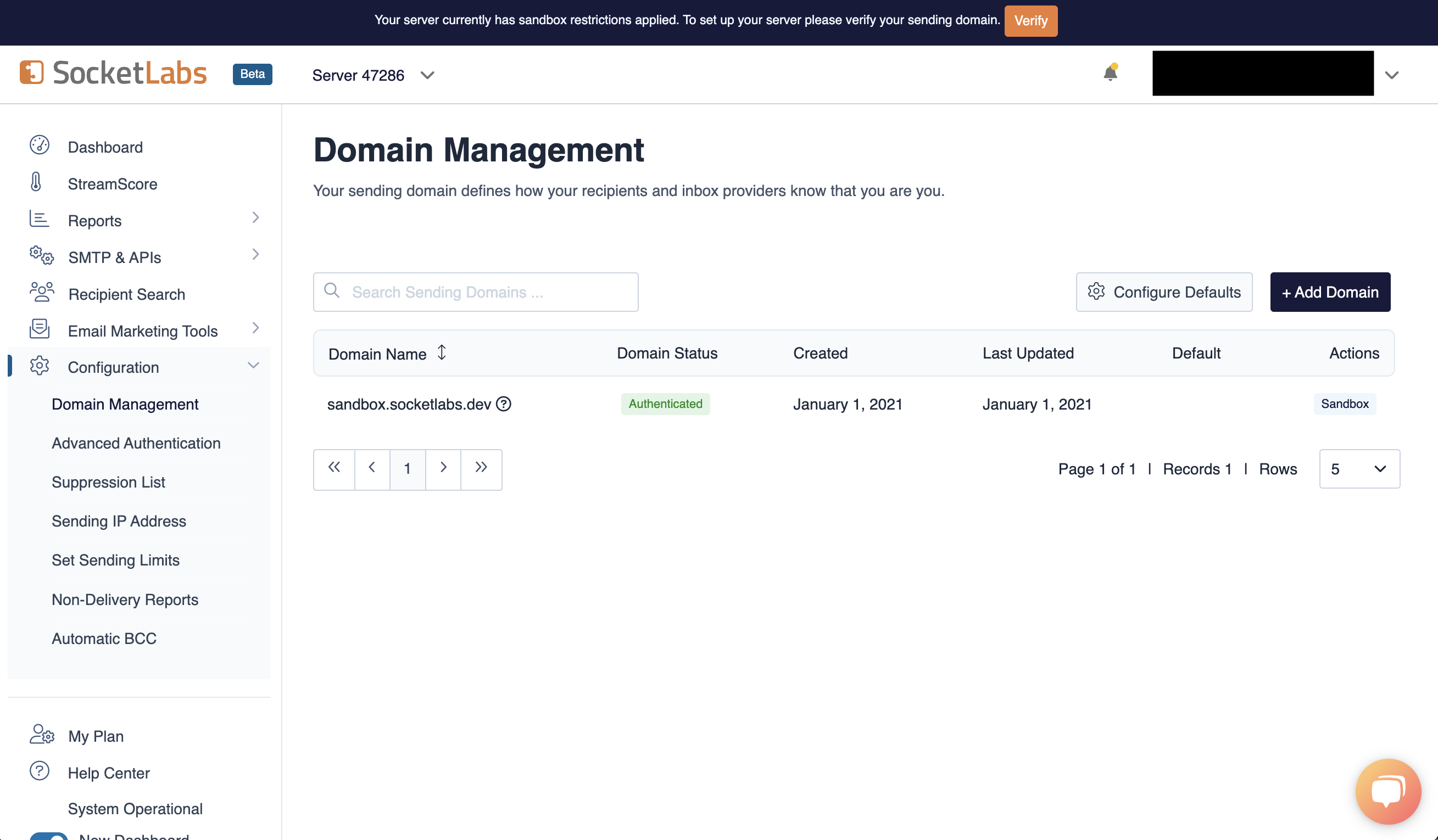
Remember that you will need to restart Infisical for this to work properly.
1. Create an account on [Resend](https://resend.com).
2. Add a [Domain](https://resend.com/domains).
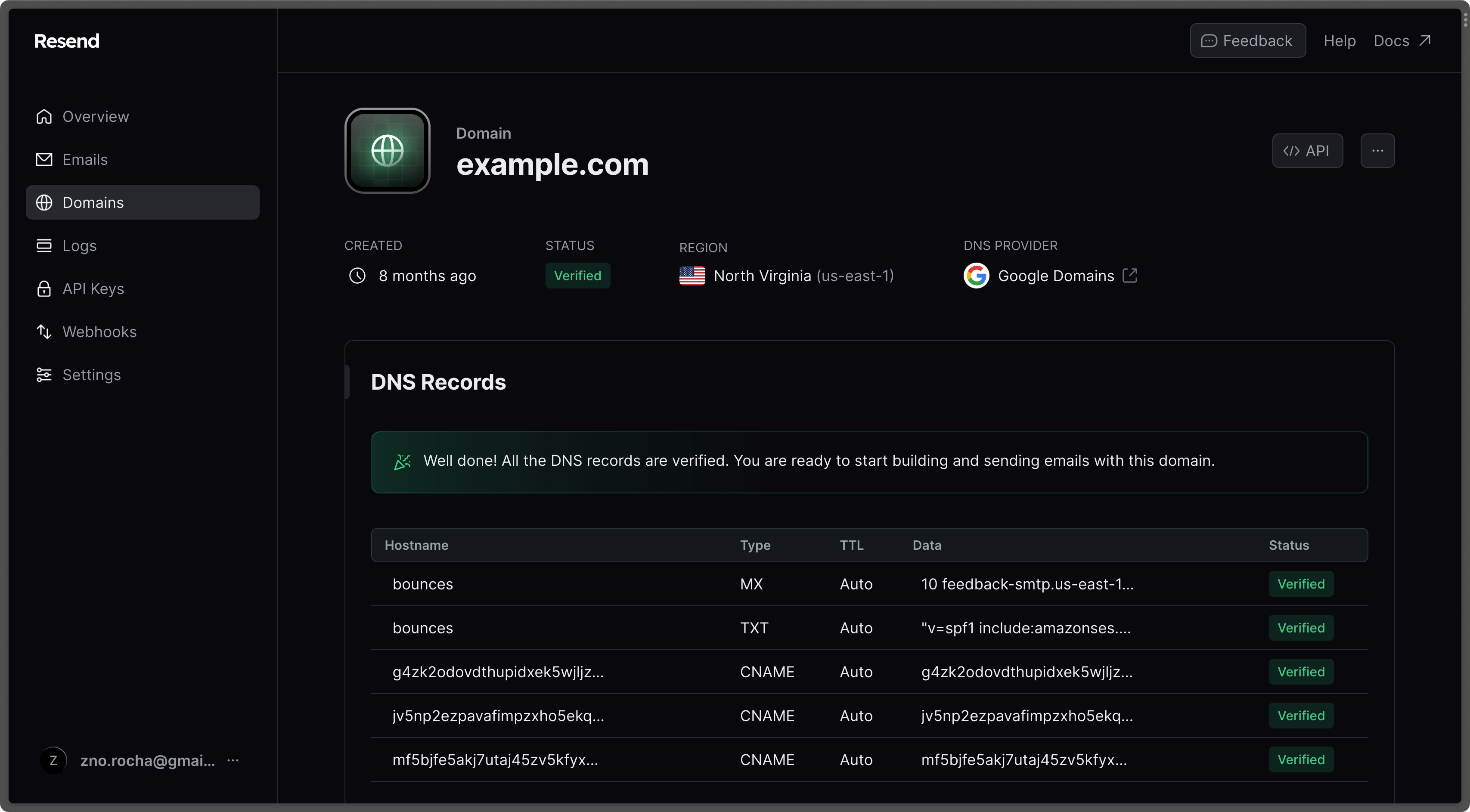
3. Create an [API Key](https://resend.com/api-keys).
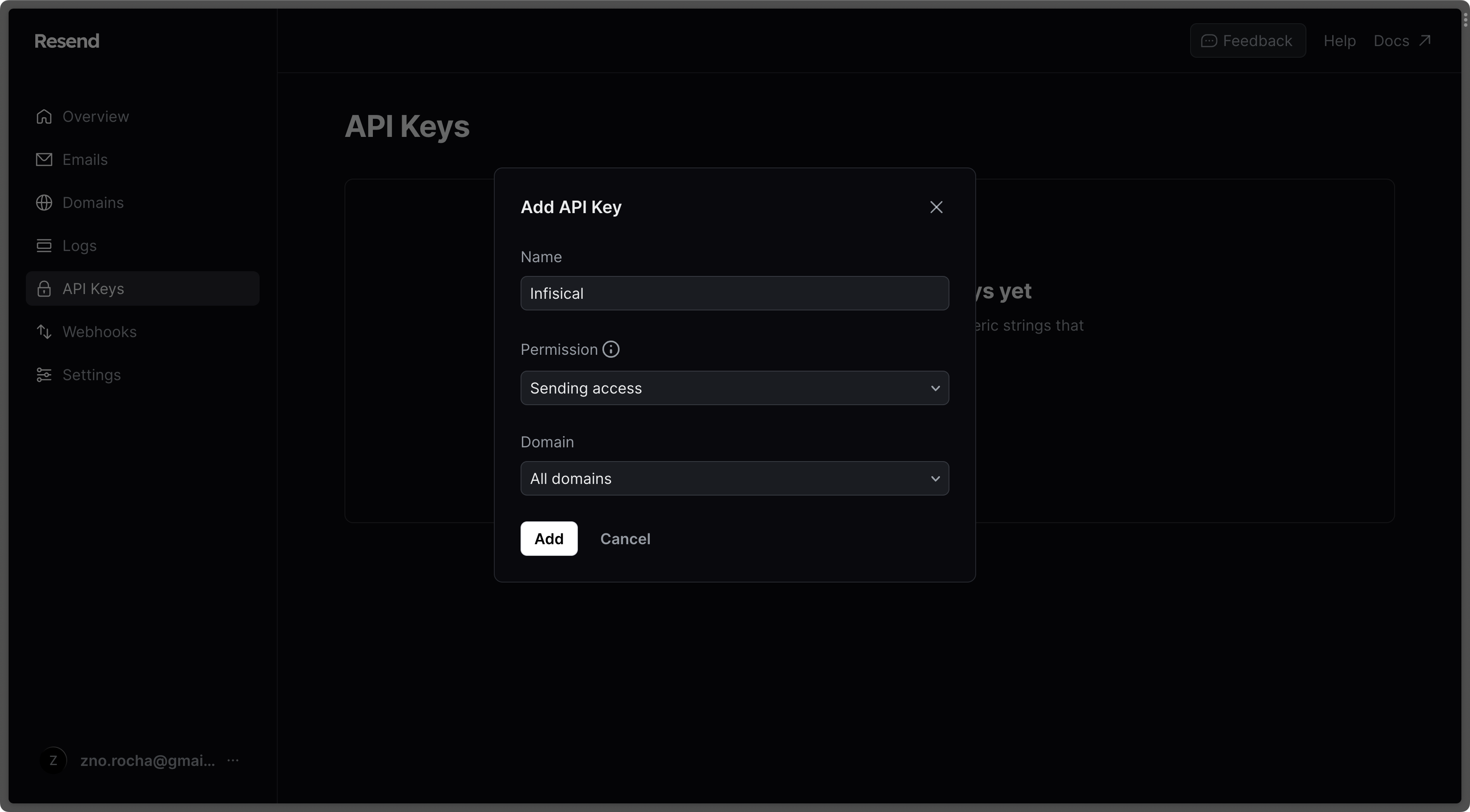
4. Go to the [SMTP page](https://resend.com/settings/smtp) and copy the values.
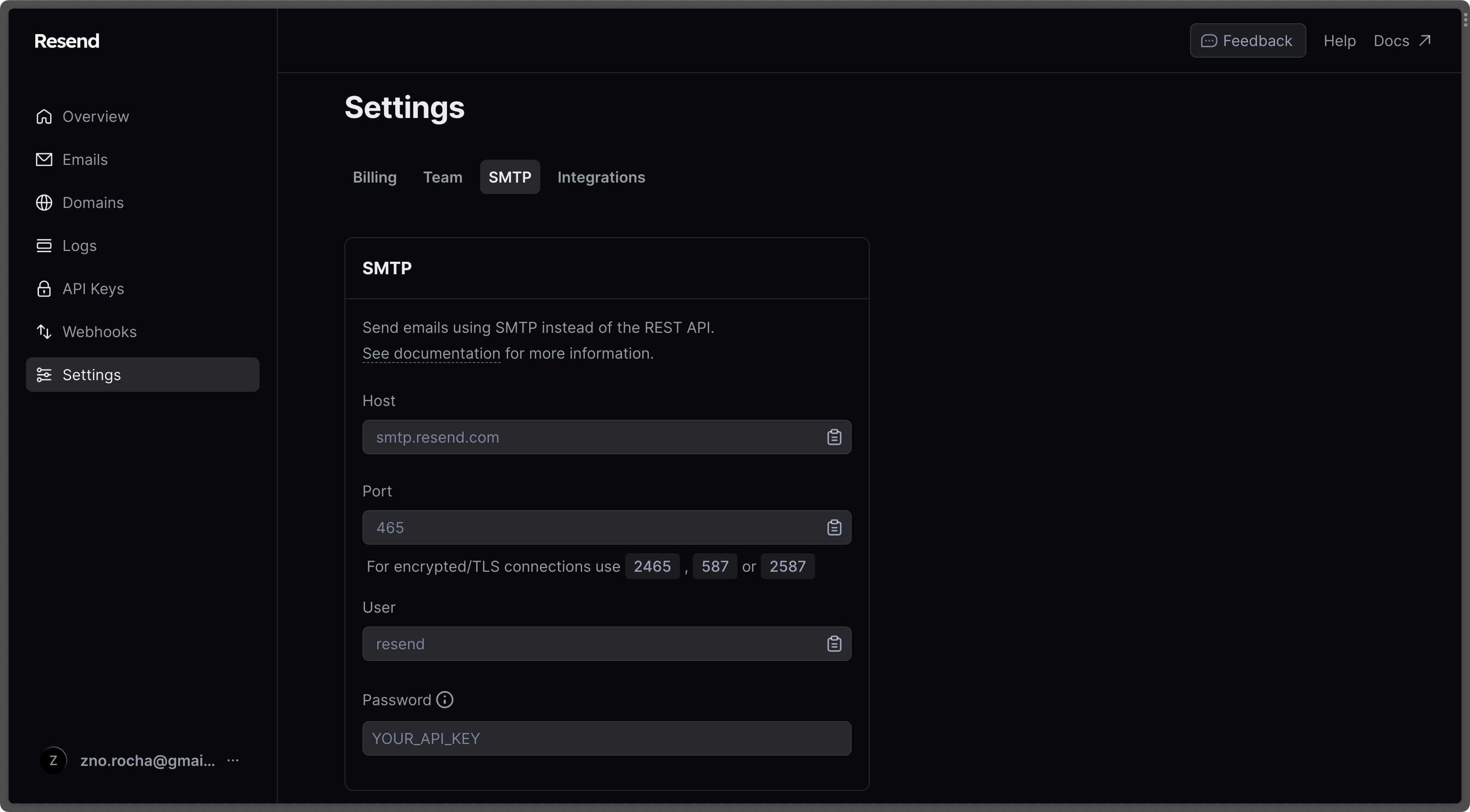
5. With the API Key, you can now set your SMTP environment variables variables:
```
SMTP_HOST=smtp.resend.com
SMTP_USERNAME=resend
SMTP_PASSWORD=YOUR_API_KEY
SMTP_PORT=587
SMTP_FROM_ADDRESS=hey@example.com # your email address being used to send out emails
SMTP_FROM_NAME=Infisical
```
Remember that you will need to restart Infisical for this to work properly.
Create an account and enable "less secure app access" in Gmail Account Settings > Security. This will allow
applications like Infisical to authenticate with Gmail via your username and password.
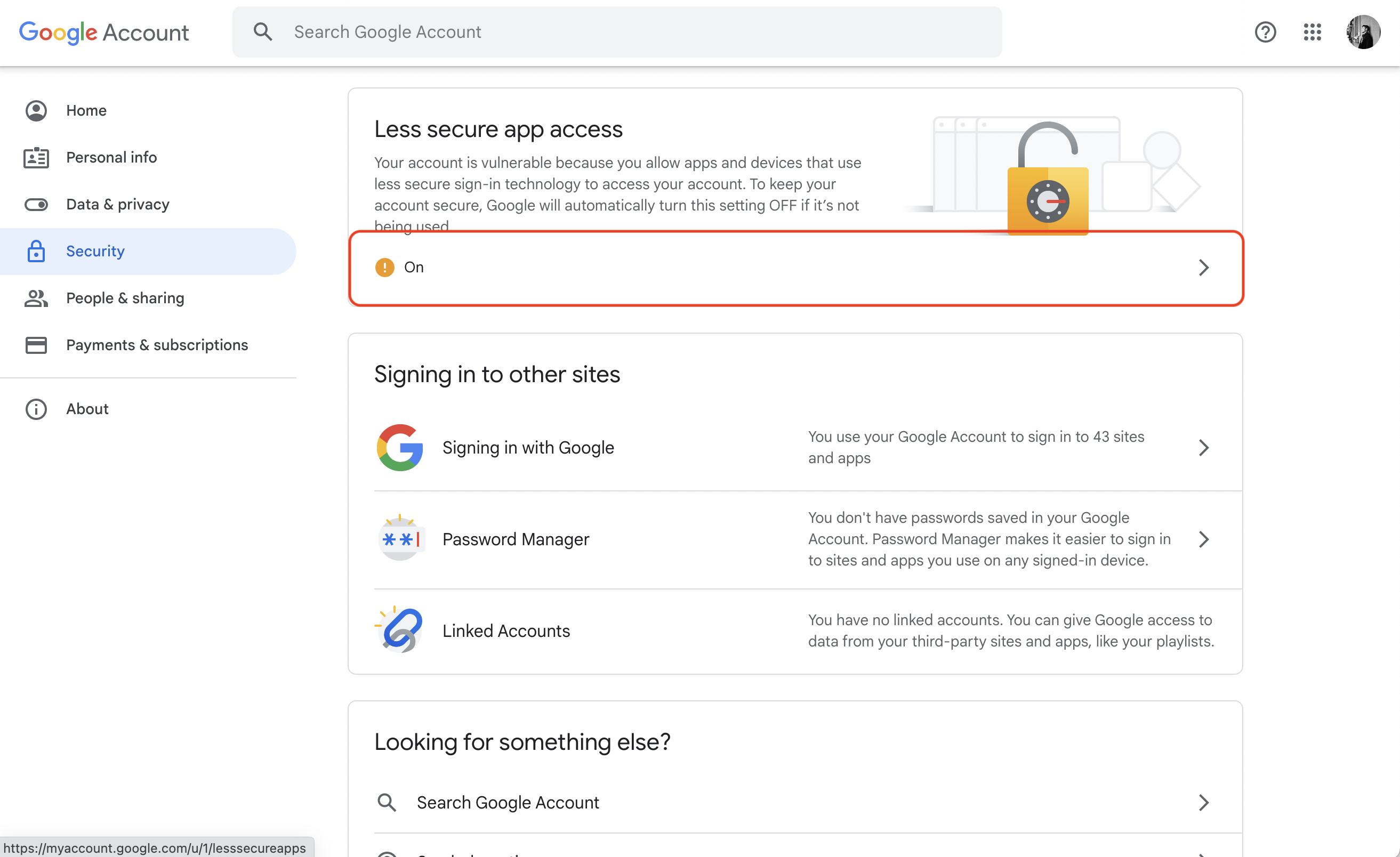
With your Gmail username and password, you can set your SMTP environment variables:
```
SMTP_HOST=smtp.gmail.com
SMTP_USERNAME=hey@gmail.com # your email
SMTP_PASSWORD=password # your password
SMTP_PORT=587
SMTP_FROM_ADDRESS=hey@gmail.com
SMTP_FROM_NAME=Infisical
```
As per the [notice](https://support.google.com/accounts/answer/6010255?hl=en) by Google, you should note that using Gmail credentials for SMTP configuration
will only work for Google Workspace or Google Cloud Identity customers as of May 30, 2022.
Put differently, the SMTP configuration is only possible with business (not personal) Gmail credentials.
1. Create an account and configure [Office365](https://www.office.com/) to send emails.
2. With your login credentials, you can now set up your SMTP environment variables:
```
SMTP_HOST=smtp.office365.com
SMTP_USERNAME=username@yourdomain.com # your username
SMTP_PASSWORD=password # your password
SMTP_PORT=587
SMTP_FROM_ADDRESS=username@yourdomain.com
SMTP_FROM_NAME=Infisical
```
1. Create an account and configure [Zoho Mail](https://www.zoho.com/mail/) to send emails.
2. With your email credentials, you can now set up your SMTP environment variables:
```
SMTP_HOST=smtp.zoho.com
SMTP_USERNAME=username # your email
SMTP_PASSWORD=password # your password
SMTP_PORT=587
SMTP_FROM_ADDRESS=hey@example.com # your personal Zoho email or domain-based email linked to Zoho Mail
SMTP_FROM_NAME=Infisical
```
{" "}
You can use either your personal Zoho email address like `you@zohomail.com` or
a domain-based email address like `you@yourdomain.com`. If using a
domain-based email address, then please make sure that you've configured and
verified it with Zoho Mail.
Remember that you will need to restart Infisical for this to work properly.
1. Create an account and configure [SMTP2Go](https://www.smtp2go.com/) to send emails.
2. Turn on SMTP authentication
```
SMTP_HOST=mail.smtp2go.com
SMTP_PORT=You can use one of the following ports: 2525, 80, 25, 8025, or 587
SMTP_USERNAME=username #Your SMTP2GO account's SMTP username
SMTP_PASSWORD=password #Your SMTP2GO account's SMTP password
SMTP_FROM_ADDRESS=hey@example.com # your email address being used to send out emails
SMTP_FROM_NAME=Infisical
```
{" "}
Optional (for TLS/SSL):
TLS: Available on the same ports (2525, 80, 25, 8025, or 587)
SSL: Available on ports 465, 8465, and 443
## Authentication
By default, users can only login via email/password based login method.
To login into Infisical with OAuth providers such as Google, configure the associated variables.
When set, all visits to the Infisical login page will automatically redirect users of your Infisical instance to the SAML identity provider associated with the specified organization slug.
Follow detailed guide to configure [Google SSO](/documentation/platform/sso/google)
OAuth2 client ID for Google login
OAuth2 client secret for Google login
Follow detailed guide to configure [GitHub SSO](/documentation/platform/sso/github)
OAuth2 client ID for GitHub login
OAuth2 client secret for GitHub login
Follow detailed guide to configure [GitLab SSO](/documentation/platform/sso/gitlab)
OAuth2 client ID for GitLab login
OAuth2 client secret for GitLab login
URL of your self-hosted instance of GitLab where the OAuth application is registered
Requires enterprise license. Please contact [team@infisical.com](mailto:team@infisical.com) to get more
information.
Requires enterprise license. Please contact [team@infisical.com](mailto:team@infisical.com) to get more
information.
Requires enterprise license. Please contact [team@infisical.com](mailto:team@infisical.com) to get more
information.
## Native secret integrations
To help you sync secrets from Infisical to services such as Github and Gitlab, Infisical provides native integrations out of the box.
OAuth2 client ID for Heroku integration
OAuth2 client secret for Heroku integration
OAuth2 client ID for Vercel integration
{" "}
OAuth2 client secret for Vercel integration
OAuth2 slug for Vercel integration
OAuth2 client ID for Netlify integration
OAuth2 client secret for Netlify integration
OAuth2 client ID for GitHub integration
OAuth2 client secret for GitHub integration
OAuth2 client ID for BitBucket integration
OAuth2 client secret for BitBucket integration
OAuth2 client id for GCP secrets manager integration
OAuth2 client secret for GCP secrets manager integration
The AWS IAM User access key for assuming roles.
The AWS IAM User secret key for assuming roles.
OAuth2 client id for Azure integration
OAuth2 client secret for Azure integration
OAuth2 client id for Gitlab integration
OAuth2 client secret for Gitlab integration
## Observability
You can configure Infisical to collect and expose telemetry data for analytics and monitoring.
Whether or not to collect and expose telemetry data.
Supported types are `prometheus` and `otlp`.
If export type is set to `prometheus`, metric data will be exposed in port 9464 in the `/metrics` path.
If export type is set to `otlp`, you will have to configure a value for `OTEL_EXPORT_OTLP_ENDPOINT`.
Where telemetry data would be pushed to for collection. This is only
applicable when `OTEL_EXPORT_TYPE` is set to `otlp`.
The username for authenticating with the telemetry collector.
The password for authenticating with the telemetry collector.
# Hardware requirements
Find out the minimal requirements for operating Infisical.
This page details the minimum requirements necessary for installing and using Infisical.
The actual resource requirements will vary in direct proportion to the operations performed by Infisical and the level of utilization by the end users.
## Deployment Sizes
**Small** suitable for most initial production setups, as well as development and testing scenarios.
**Large** suitable for high-demand production environments, characterized by either a high volume of transactions, large number of secrets, or both.
## Hardware Requirements
### Storage
Infisical doesn’t require file storage as all persisted data is saved in the database.
However, its logs and metrics are saved to disk for later viewing. As a result, we recommend provisioning 1-2 GB of storage.
### CPU
CPU requirements vary heavily on the volume of secret operations (reads and writes) you anticipate.
Processing large volumes of secrets frequently and consistently will require higher CPU.
Recommended minimum CPU hardware for different sizes of deployments:
* **small:** 2-4 core is the **recommended** minimum
* **large:** 4-8 cores are suitable for larger deployments
### Memory Allocation
Memory needs depend on expected workload, including factors like user activity, automation level, and the frequency of secret operations.
Recommended minimum memory hardware for different sizes of deployments:
* **small:** 4-8 GB is the **recommended** minimum
* **large:** 16-32 GB are suitable for larger deployments
## Database & caching layer
### Postgres
PostgreSQL is the only database supported by Infisical. Infisical has been extensively tested with Postgres version 16. We recommend using versions 14 and up for optimal compatibility.
Recommended resource allocation based on deployment size:
* **small:** 2 vCPU / 8 GB RAM / 20 GB Disk
* **large:** 4vCPU / 16 GB RAM / 100 GB Disk
### Redis
Redis is utilized for session management and background tasks in Infisical.
Redis requirements:
* Use Redis versions 6.x or 7.x. We advise upgrading to at least Redis 6.2.
* Redis Cluster mode is currently not supported; use Redis Standalone, with or without High Availability (HA).
* Redis storage needs are minimal: a setup with 2 vCPU, 4 GB RAM, and 30GB SSD will be sufficient for small deployments.
* Set cache eviction policy to `noeviction`.
## Supported Web Browsers
Infisical supports a range of web browsers. However, features such as browser-based CLI login only work on Google Chrome and Firefox at the moment.
* [Mozilla Firefox](https://www.mozilla.org/en-US/firefox/new/)
* [Google Chrome](https://www.google.com/chrome/)
* [Chromium](https://www.chromium.org/getting-involved/dev-channel/)
* [Apple Safari](https://www.apple.com/safari/)
* [Microsoft Edge](https://www.microsoft.com/en-us/edge?form=MA13FJ)
# Schema migration
Learn how to run Postgres schema migrations.
Running schema migrations is a requirement before deploying Infisical.
Each time you decide to upgrade your version of Infisical, it's necessary to run schema migrations for that specific version.
The guide below outlines a step-by-step guide to help you manually run schema migrations for Infisical.
### Prerequisites
* Docker installed on your machine
* An active PostgreSQL database
* Postgres database connection string
First, ensure you have the correct version of the Infisical Docker image. You can pull it from Docker Hub using the following command:
```bash
docker pull infisical/infisical:
```
Replace `` with the specific version number you intend to deploy. View available versions [here](https://hub.docker.com/r/infisical/infisical/tags)
The Docker image requires a `DB_CONNECTION_URI` environment variable. This connection string should point to your PostgreSQL database. The format generally looks like this: `postgresql://username:password@host:port/database`.
To run the schema migration for the version of Infisical you want to deploy, use the following Docker command:
```bash
docker run --env DB_CONNECTION_URI= infisical/infisical: npm run migration:latest
```
Replace `` with your actual PostgreSQL connection string, and `` with the desired version number.
After running the migration, it's good practice to check if the migration was successful. You can do this by checking the logs or accessing your database to ensure the schema has been updated accordingly.
If you need to rollback a migration by one step, use the following command:
```bash
docker run --env DB_CONNECTION_URI= infisical/infisical: npm run migration:rollback
```
It's important to run schema migrations for each version of the Infisical you deploy. For instance, if you're updating from `infisical/infisical:1` to `infisical/infisical:2`, ensure you run the schema migrations for `infisical/infisical:2` before deploying it.
In a production setting, we recommend a more structured approach to deploying migrations prior to upgrading Infisical. This can be accomplished via CI automation.
### Additional discussion
* Always back up your database before running migrations, especially in a production environment.
* Test the migration process in a staging environment before applying it to production.
* Keep track of the versions and their corresponding migrations to avoid any inconsistencies.
# Docker Compose
Read how to run Infisical with Docker Compose template.
This self-hosting guide will walk you through the steps to self-host Infisical using Docker Compose.
## Prerequisites
* [Docker](https://docs.docker.com/engine/install/)
* [Docker compose](https://docs.docker.com/compose/install/)
This Docker Compose configuration is not designed for high-availability production scenarios.
It includes just the essential components needed to set up an Infisical proof of concept (POC).
To run Infisical in a highly available manner, give the [Docker Swarm guide](/self-hosting/deployment-options/docker-swarm).
## Verify prerequisites
To verify that Docker compose and Docker are installed on the machine where you plan to install Infisical, run the following commands.
Check for docker installation
```bash
docker
```
Check for docker compose installation
```bash
docker-compose
```
## Download docker compose file
You can obtain the Infisical docker compose file by using a command-line downloader such as `wget` or `curl`.
If your system doesn't have either of these, you can use a equivalent command that works with your machine.
```bash
curl -o docker-compose.prod.yml https://raw.githubusercontent.com/Infisical/infisical/main/docker-compose.prod.yml
```
```bash
wget -O docker-compose.prod.yml https://raw.githubusercontent.com/Infisical/infisical/main/docker-compose.prod.yml
```
## Configure instance credentials
Infisical requires a set of credentials used for connecting to dependent services such as Postgres, Redis, etc.
The default credentials can be downloaded using the one of the commands listed below.
```bash
curl -o .env https://raw.githubusercontent.com/Infisical/infisical/main/.env.example
```
```bash
wget -O .env https://raw.githubusercontent.com/Infisical/infisical/main/.env.example
```
Once downloaded, the credentials file will be saved to your working directly as `.env` file.
View all available configurations [here](/self-hosting/configuration/envars).
The default .env file contains credentials that are intended solely for testing purposes.
Please generate a new `ENCRYPTION_KEY` and `AUTH_SECRET` for use outside of testing.
Instructions to do so, can be found [here](/self-hosting/configuration/envars).
## Start Infisical
Run the command below to start Infisical and all related services.
```bash
docker-compose -f docker-compose.prod.yml up
```
Your Infisical instance should now be running on port `80`. To access your instance, visit `http://localhost:80`.
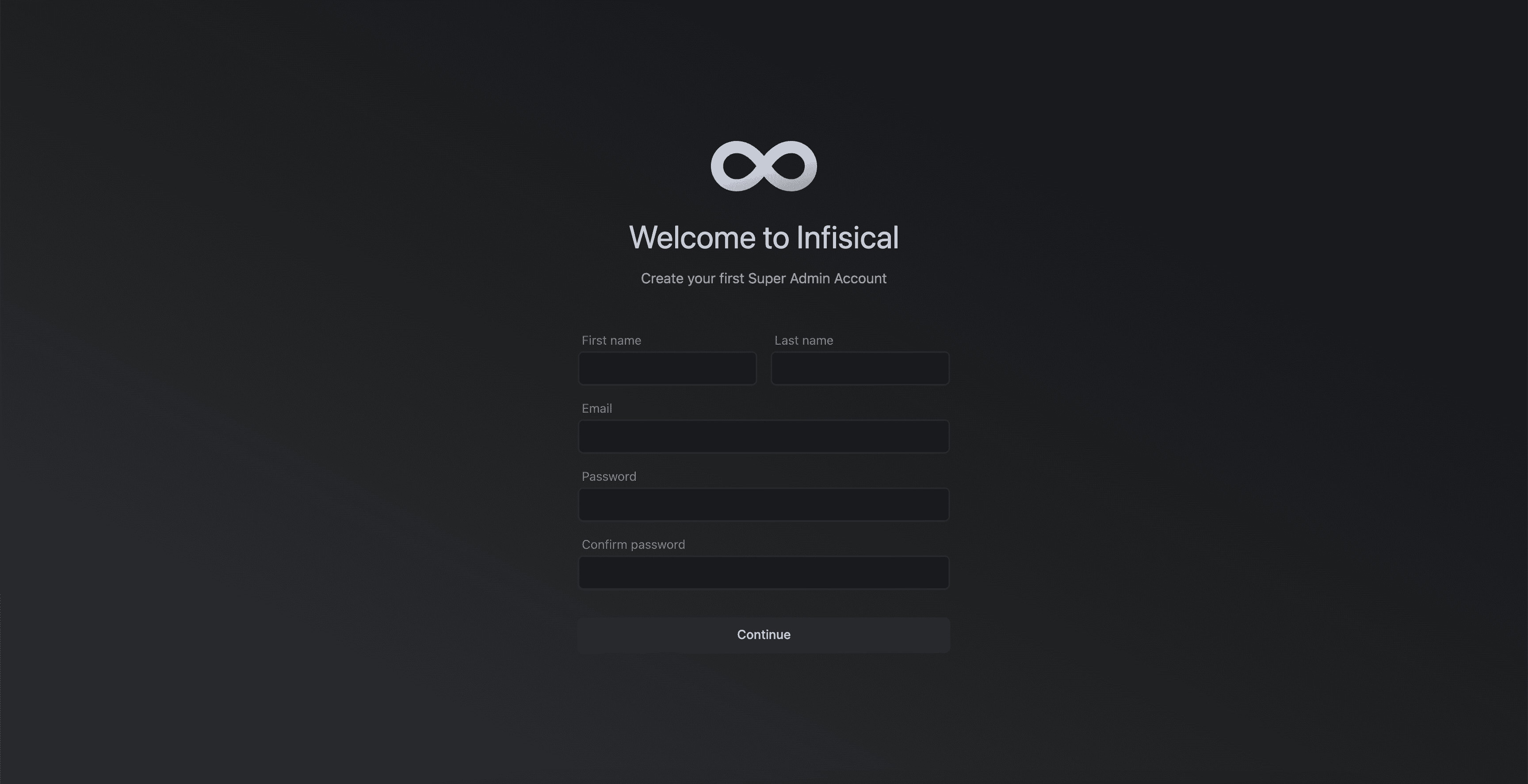
# Docker Swarm
How to self-host Infisical with Docker Swarm (HA).
# Self-Hosting Infisical with Docker Swarm
This guide will provide step-by-step instructions on how to self-host Infisical using Docker Swarm. This is particularly helpful for those wanting to self-host Infisical on premise while still maintaining high availability (HA) for the core Infisical components.
The guide will demonstrate a setup with three nodes, ensuring that the cluster can tolerate the failure of one node while remaining fully operational.
## Docker Swarm
[Docker Swarm](https://docs.docker.com/engine/swarm/) is a native clustering and orchestration solution for Docker containers.
It simplifies the deployment and management of containerized applications across multiple nodes, making it a great choice for self-hosting Infisical.
Unlike Kubernetes, which requires a deep understanding of the Kubernetes ecosystem, if you're accustomed to Docker and Docker Compose, you're already familiar with most of Docker Swarm.
For this reason, we suggest teams use Docker Swarm to deploy Infisical in a highly available and fault tolerant manner.
## Prerequisites
* Understanding of Docker Swarm
* Bare/Virtual Machines with Docker installed on each VM.
* Docker Swarm initialized on the VMs.
## Core Components for High Availability
The provided Docker stack includes the following core components to achieve high availability:
1. **Spilo**: [Spilo](https://github.com/zalando/spilo) is used to run PostgreSQL with [Patroni](https://github.com/zalando/patroni) for HA and automatic failover. It utilizes etcd for leader election of the PostgreSQL instances.
2. **Redis**: Redis is used for caching and is set up with Redis Sentinel for HA.
The stack includes three Redis replicas and three Redis Sentinel instances for monitoring and failover.
3. **Infisical**: Infisical is stateless, allowing for easy scaling and replication across multiple nodes.
4. **HAProxy**: HAProxy is used as a load balancer to distribute traffic to the PostgreSQL and Redis instances.
It is configured to perform health checks and route requests to the appropriate backend services.
## Node Failure Tolerance
To ensure Infisical is highly available and fault tolerant, it's important to choose the number of nodes in the cluster.
The following table shows the relationship between the number of nodes and the maximum number of nodes that can be down while the cluster continues to function:
| Total Nodes | Max Nodes Down | Min Nodes Required |
| ----------- | -------------- | ------------------ |
| 1 | 0 | 1 |
| 2 | 0 | 2 |
| 3 | 1 | 2 |
| 4 | 1 | 3 |
| 5 | 2 | 3 |
| 6 | 2 | 4 |
| 7 | 3 | 4 |
The formula for calculating the minimum number of nodes required is: `floor(n/2) + 1`, where `n` is the total number of nodes.
This guide will demonstrate a setup with three nodes, which allows for one node to be down while the cluster remains operational. This fault tolerance applies to the following components:
* Redis Sentinel: With three Sentinel instances, one instance can be down, and the remaining two can still form a quorum to make decisions.
* Redis: With three Redis instances (one master and two replicas), one instance can be down, and the remaining two can continue to provide caching services.
* PostgreSQL: With three PostgreSQL instances managed by Patroni and etcd, one instance can be down, and the remaining two can maintain data consistency and availability.
* Manager Nodes: In a Docker Swarm cluster with three manager nodes, one manager node can be down, and the remaining two can continue to manage the cluster.
For the sake of simplicity, the example in this guide only contains one manager node.
It's important to note that while the cluster can tolerate the failure of one node in a three-node setup, it's recommended to have a minimum of three nodes to ensure high availability.
With two nodes, the failure of a single node can result in a loss of quorum and potential downtime.
## Docker Deployment Stack Overview
The [Docker stack file](https://github.com/Infisical/infisical/tree/main/docker-swarm) used in this guide defines the services and their configurations for deploying Infisical in a highly available manner. The main components of this stack are as follows.
1. **HAProxy**: The HAProxy service is configured to expose ports for accessing PostgreSQL (5433 for the master, 5434 for replicas), Redis master (6379), and the Infisical backend (8080). It uses a config file (`haproxy.cfg`) to define the load balancing and health check rules.
2. **Infisical**: The Infisical backend service is deployed with the latest PostgreSQL-compatible image. It is connected to the `infisical` network and uses secrets for environment variables.
3. **etcd**: Three etcd instances (etcd1, etcd2, etcd3) are deployed, one on each node, to provide distributed key-value storage for leader election and configuration management.
4. **Spilo**: Three Spilo instances (spolo1, spolo2, spolo3) are deployed, one on each node, to run PostgreSQL with Patroni for high availability. They are connected to the `infisical` network and use persistent volumes for data storage.
5. **Redis**: Three Redis instances (redis\_replica0, redis\_replica1, redis\_replica2) are deployed, one on each node, with redis\_replica0 acting as the master. They are connected to the `infisical` network.
6. **Redis Sentinel**: Three Redis Sentinel instances (redis\_sentinel1, redis\_sentinel2, redis\_sentinel3) are deployed, one on each node, to monitor and manage the Redis instances. They are connected to the `infisical` network.
## Deployment instructions
Run the following on each node to install the Docker engine.
```
curl -fsSL https://get.docker.com -o get-docker.sh && sh get-docker.sh
```
```
docker swarm init
```
Replace `` with the IP address of the VM that will serve as the manager node. Remember to copy the join token returned by the this init command.
For the sake of simplicity, we only use one manager node in this example deployment. However, in production settings, we recommended you have at least 3 manager nodes.
```
docker swarm join --token :2377
```
Replace `` with the token provided by the manager node during initialization.
Labels on nodes will help us select where stateful components such as Postgres and Redis are deployed on. To label nodes, follow the steps below.
```
docker node update --label-add name=node1
docker node update --label-add name=node2
docker node update --label-add name=node3
```
Replace ``, ``, and `` with the respective node IDs.
To view the list of nodes and their ids, run the following on the manager node `docker node ls`.
Copy the Docker stack YAML file, HAProxy configuration file and example `.env` file to the manager node. Ensure that all 3 files are placed in the same file directory.
* [Docker stack file](https://github.com/Infisical/infisical/blob/main/docker-swarm/stack.yaml) (rename to infisical-stack.yaml)
* [HA configuration file](https://github.com/Infisical/infisical/blob/main/docker-swarm/haproxy.cfg) (rename to haproxy.cfg)
* [Example .env file](https://github.com/Infisical/infisical/blob/main/docker-swarm/.env-example) (rename to .env)
```
docker stack deploy -c infisical-stack.yaml infisical
```
```plain
$ docker service ls
ID NAME MODE REPLICAS IMAGE PORTS
4kzq3ub8qgn9 infisical_etcd1 replicated 1/1 ghcr.io/zalando/spilo-16:3.2-p2
tqx9t82bn8d9 infisical_etcd2 replicated 1/1 ghcr.io/zalando/spilo-16:3.2-p2
t8vbkrasy8fz infisical_etcd3 replicated 1/1 ghcr.io/zalando/spilo-16:3.2-p2
77iei42fcf6q infisical_haproxy global 4/4 haproxy:latest *:5002-5003->5433-5434/tcp, *:6379->6379/tcp, *:7001->7000/tcp, *:8080->8080/tcp
jaewzqy8md56 infisical_infisical replicated 5/5 infisical/infisical:v0.60.1-postgres
58w4zablfbtb infisical_redis_replica0 replicated 1/1 bitnami/redis:6.2.10
w4yag2whq0un infisical_redis_replica1 replicated 1/1 bitnami/redis:6.2.10
w03mriy0jave infisical_redis_replica2 replicated 1/1 bitnami/redis:6.2.10
ppo6rk47hc9t infisical_redis_sentinel1 replicated 1/1 bitnami/redis-sentinel:6.2.10
ub29vd0lnq7f infisical_redis_sentinel2 replicated 1/1 bitnami/redis-sentinel:6.2.10
szg3yky7yji2 infisical_redis_sentinel3 replicated 1/1 bitnami/redis-sentinel:6.2.10
eqtocpf5tiy0 infisical_spolo1 replicated 1/1 ghcr.io/zalando/spilo-16:3.2-p2
3lznscvk7k5t infisical_spolo2 replicated 1/1 ghcr.io/zalando/spilo-16:3.2-p2
v04ml7rz2j5q infisical_spolo3 replicated 1/1 ghcr.io/zalando/spilo-16:3.2-p2
```
You'll notice that service `infisical_infisical` will not be in running state.
This is expected as the database does not yet have the desired schemas.
Once the database schema migrations have been successfully applied, this issue should be resolved.
Run the schema migration to initialize the database. Follow the [guide here](/self-hosting/configuration/schema-migrations) to learn how.
To run the migrations, you'll need to connect to the Postgres instance deployed on your Docker swarm. The default Postgres user credentials are defined in the Docker swarm: username: `postgres`, password: `postgres` and database: `postgres`.
We recommend you change these credentials when deploying to production and creating a separate DB for Infisical.
After running the schema migrations, be sure to update the `.env` file to have the correct `DB_CONNECTION_URI`.
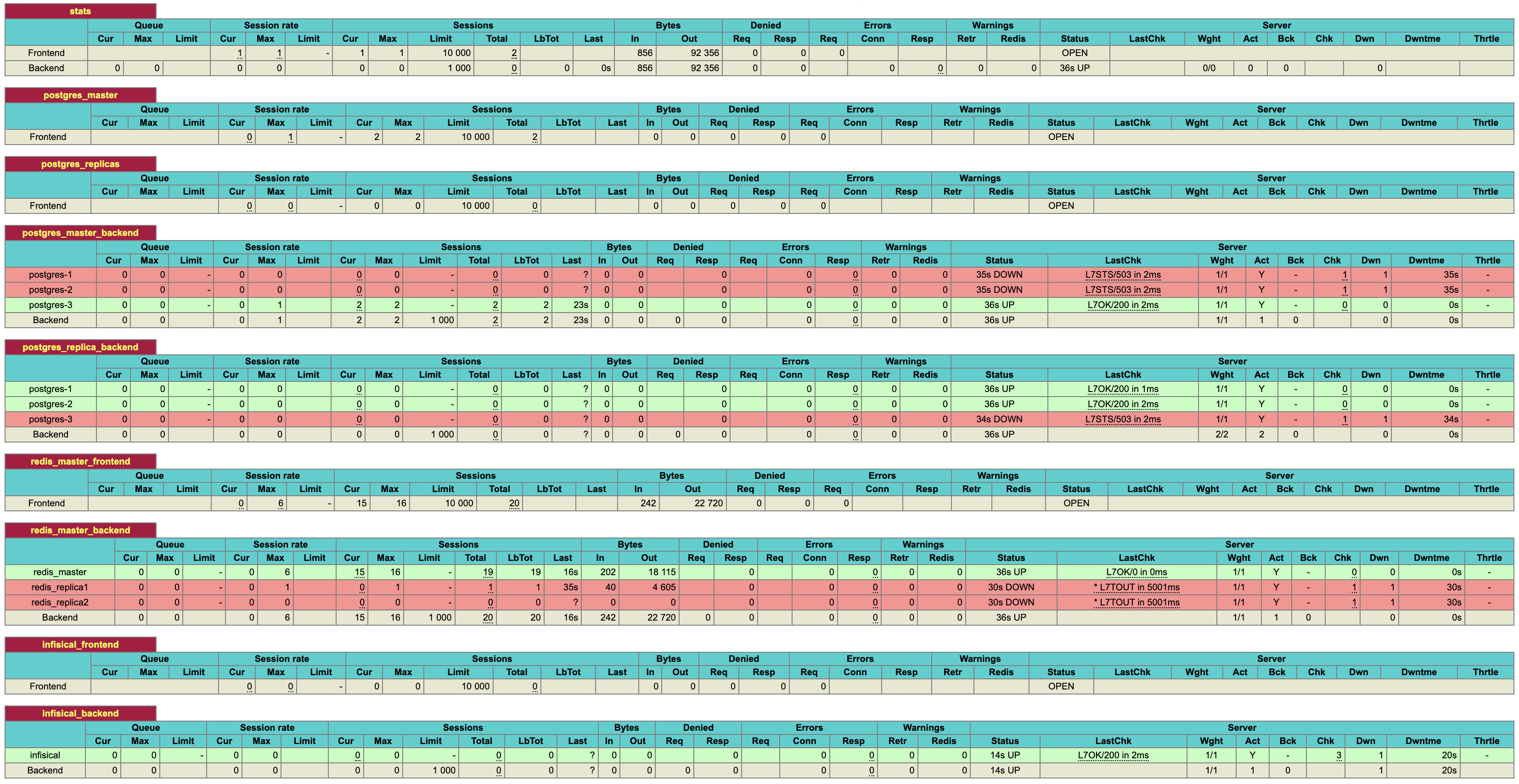
To view the health of services in your Infisical cluster, visit port `:7001` of any node in your Docker swarm.
This port will expose the HA Proxy stats.
Run the following command to view the IPs of the nodes in your docker swarm.
```plain
$ docker node ls
ID HOSTNAME STATUS AVAILABILITY MANAGER STATUS ENGINE VERSION
0jnegl4gpo235l66nglcwc07t localhost Ready Active 26.0.2
no1a7zwj88057k73m196ulkq6 * localhost Ready Active Leader 26.0.2
wcb2x27w3tq7ht4v1h7ke49qk localhost Ready Active 26.0.2
zov5q7uop7wpxc2ndz712v9oa localhost Ready Active 26.0.2
```
The stats page may take 1-2 minutes to become accessible.
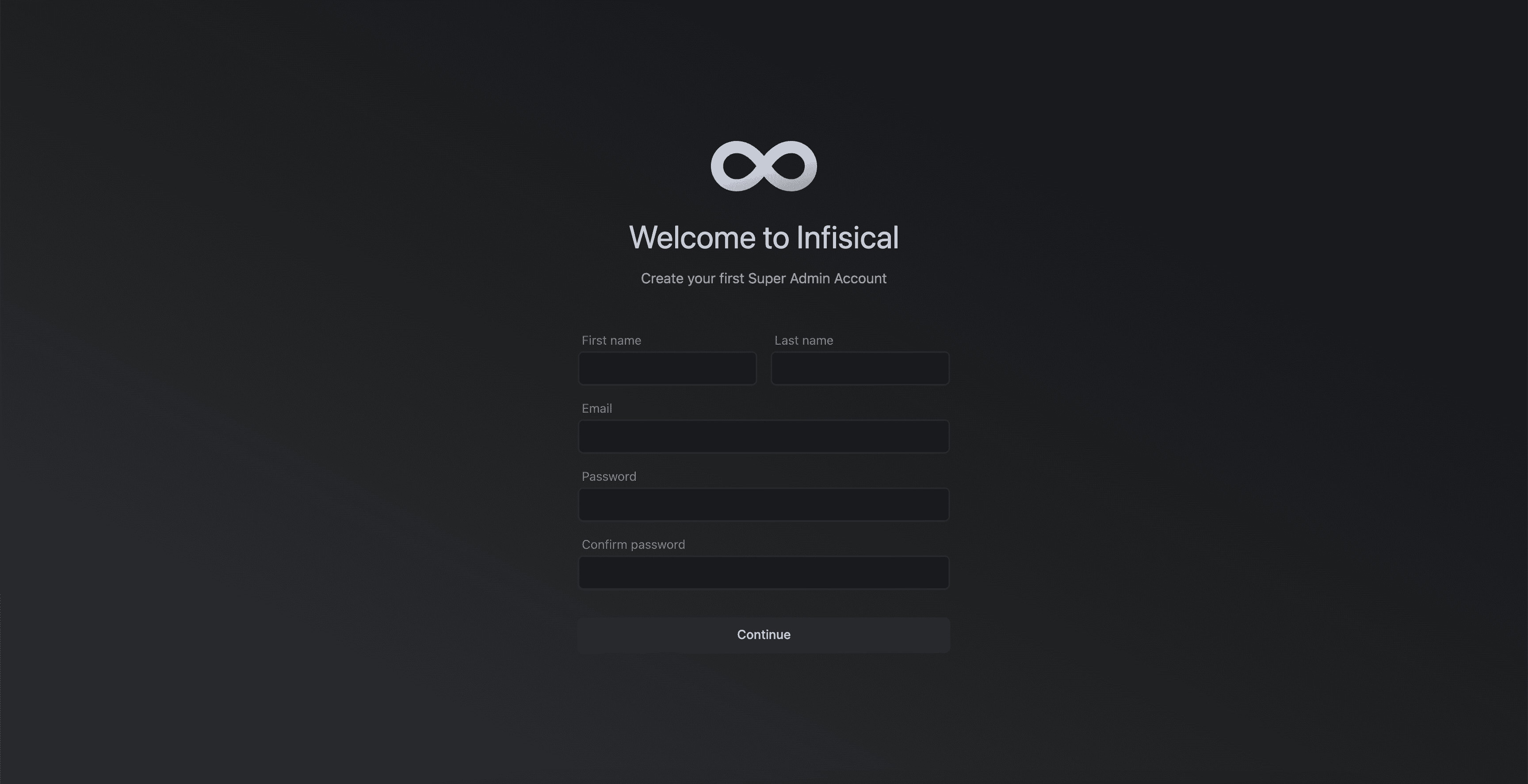
Once all expected services are up and running, visit `:8080` of any node in the swarm. This will take you to the Infisical configuration page.
## FAQ
To further scale and make the system more resilient, you can add more nodes to the Docker Swarm and update the stack configuration accordingly:
1. Add new VMs and join them to the Docker Swarm as worker nodes.
2. Update the Docker stack YAML file to include the new nodes in the `deploy` section of the relevant services, specifying the appropriate `node.labels.name` constraints.
3. Update the HAProxy configuration file (`haproxy.cfg`) to include the new nodes in the backend sections for PostgreSQL and Redis.
4. Redeploy the updated stack using the `docker stack deploy` command.
Note that the database containers (PostgreSQL) are stateful and cannot be simply replicated. Instead, one database instance is deployed per node to ensure data consistency and avoid conflicts.
Native tooling for scheduled backups of Postgres and Redis is currently in development.
In the meantime, we recommend using a variety of open-source tools available for this purpose.
For Postgres, [Spilo](https://github.com/zalando/spilo) provides built-in support for scheduled data dumps.
You can explore other third party tools for managing db backups, one such tool is [docker-db-backup](https://github.com/tiredofit/docker-db-backup).
# Kubernetes via Helm Chart
Learn how to use Helm chart to install Infisical on your Kubernetes cluster.
**Prerequisites**
* You have extensive understanding of [Kubernetes](https://kubernetes.io/)
* Installed [Helm package manager](https://helm.sh/) version v3.11.3 or greater
* You have [kubectl](https://kubernetes.io/docs/reference/kubectl/kubectl/) installed and connected to your kubernetes cluster
```bash
helm repo add infisical-helm-charts 'https://dl.cloudsmith.io/public/infisical/helm-charts/helm/charts/'
```
```
helm repo update
```
Create a `values.yaml` file. This will be used to configure settings for the Infisical Helm chart.
To explore all configurable properties for your values file, [visit this page](https://raw.githubusercontent.com/Infisical/infisical/main/helm-charts/infisical-standalone-postgres/values.yaml).
By default, the Infisical version set in your helm chart will likely be outdated.
Choose the latest Infisical docker image tag from [here](https://hub.docker.com/r/infisical/infisical/tags).
```yaml values.yaml
infisical:
image:
repository: infisical/infisical
tag: "v0.46.2-postgres" #<-- update
pullPolicy: IfNotPresent
```
Do not use the latest docker image tag in production deployments as they can introduce unexpected changes
To deploy this Helm chart, a Kubernetes secret named `infisical-secrets` must be present in the same namespace where the chart is being deployed.
For a minimal installation of Infisical, you need to configure `ENCRYPTION_KEY`, `AUTH_SECRET`, `DB_CONNECTION_URI`, `SITE_URL`, and `REDIS_URL`. [Learn more about configuration settings](/self-hosting/configuration/envars).
For test or proof-of-concept purposes, you may omit `DB_CONNECTION_URI` and `REDIS_URL` from `infisical-secrets`. This is because the Helm chart will automatically provision and connect to the in-cluster instances of Postgres and Redis by default.
```yaml simple-values-example.yaml
apiVersion: v1
kind: Secret
metadata:
name: infisical-secrets
type: Opaque
stringData:
AUTH_SECRET: <>
ENCRYPTION_KEY: <>
SITE_URL: <>
```
For production environments, we recommend using Cloud-based Platform as a Service (PaaS) solutions for PostgreSQL and Redis to ensure high availability. In on-premise setups, it's recommended to configure Redis and Postgres for high availability, either by using Bitnami charts or a custom configuration.
```yaml simple-values-example.yaml
apiVersion: v1
kind: Secret
metadata:
name: infisical-secrets
type: Opaque
stringData:
AUTH_SECRET: <>
ENCRYPTION_KEY: <>
REDIS_URL: <>
DB_CONNECTION_URI: <>
SITE_URL: <>
```
Infisical relies on a relational database, which means that database schemas need to be migrated before the instance can become operational.
To automate this process, the chart includes a option named `infisical.autoDatabaseSchemaMigration`.
When this option is enabled, a deployment/upgrade will only occur *after* a successful schema migration.
If you are using in-cluster Postgres, you may notice the migration job failing initially.
This is expected as it is waiting for the database to be in ready state.
By default, this chart uses Nginx as its Ingress controller to direct traffic to Infisical services.
```yaml values.yaml
ingress:
nginx:
enabled: true
```
Once you are done configuring your `values.yaml` file, run the command below.
```bash
helm upgrade --install infisical infisical-helm-charts/infisical-standalone --values /path/to/values.yaml
```
```yaml values.yaml
nameOverride: "infisical"
fullnameOverride: "infisical"
infisical:
enabled: true
name: infisical
autoDatabaseSchemaMigration: true
fullnameOverride: ""
podAnnotations: {}
deploymentAnnotations: {}
replicaCount: 6
image:
repository: infisical/infisical
tag: "v0.46.2-postgres"
pullPolicy: IfNotPresent
affinity: {}
kubeSecretRef: "infisical-secrets"
service:
annotations: {}
type: ClusterIP
nodePort: ""
resources:
limits:
memory: 210Mi
requests:
cpu: 200m
ingress:
enabled: true
hostName: ""
ingressClassName: nginx
nginx:
enabled: true
annotations: {}
tls: []
postgresql:
enabled: true
name: "postgresql"
fullnameOverride: "postgresql"
auth:
username: infisical
password: root
database: infisicalDB
redis:
enabled: true
name: "redis"
fullnameOverride: "redis"
cluster:
enabled: false
usePassword: true
auth:
password: "mysecretpassword"
architecture: standalone
```
After deployment, please wait for 2-5 minutes for all pods to reach a running state. Once a significant number of pods are operational, access the IP address revealed through Ingress by your load balancer.
You can find the IP address/hostname by executing the command `kubectl get ingress`.
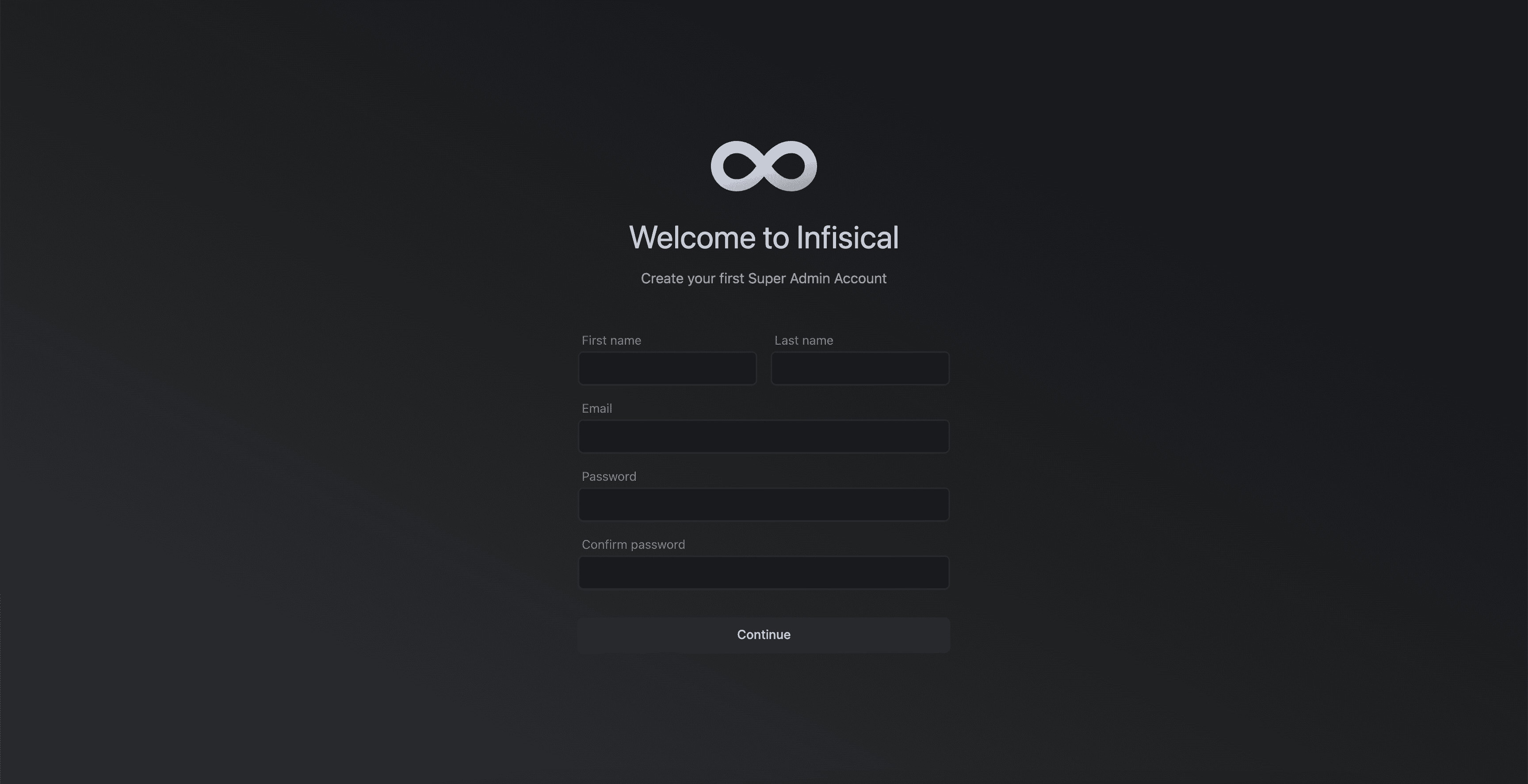
To upgrade your instance of Infisical simply update the docker image tag in your Helm values and rerun the command below.
```bash
helm upgrade --install infisical infisical-helm-charts/infisical-standalone --values /path/to/values.yaml
```
Always back up your database before each upgrade, especially in a production environment.
# Docker
Learn how to run Infisical with Docker.
Prerequisites:
* Basic knowledge of [Docker](https://www.docker.com/)
* Have Docker installed on your system. If not, follow the installation guide [here](https://docs.docker.com/get-docker/).
Infisical is available as a single Docker image to ease deployment.
This Docker image only includes the application code, meaning you must supply a connection to a Postgres database and a Redis instance.
The following guide provides a detailed step-by-step walkthrough on how you can deploy Infisical with Docker.
Visit [Docker Hub](https://hub.docker.com/r/infisical/infisical/tags) and select a version of Infisical image you would like to deploy.
Then run the following command in your terminal to pull the specific Infisical Docker image.
```
docker pull infisical/infisical:
```
Remember to replace `` with the docker image tag of your choice.
Before you can start the instance of Infisical, you need to run the database schema migrations.
Follow the step by [step guide here](/self-hosting/configuration/schema-migrations) on running schema migrations for Infisical.
For a minimal installation of Infisical, you must configure `ENCRYPTION_KEY`, `AUTH_SECRET`, `DB_CONNECTION_URI`, `SITE_URL`, and `REDIS_URL`. [View all available configurations](/self-hosting/configuration/envars).
We recommend using Cloud-based Platform as a Service (PaaS) solutions for PostgreSQL and Redis to ensure high availability.
Once you have added the required environment variables to your docker run command, execute it in your terminal to get Infisical up and running.
For example:
```bash
docker run -p 80:8080 \
-e ENCRYPTION_KEY=f40c9178624764ad85a6830b37ce239a \
-e AUTH_SECRET="q6LRi7c717a3DQ8JUxlWYkZpMhG4+RHLoFUVt3Bvo2U=" \
-e DB_CONNECTION_URI="<>" \
-e REDIS_URL="<>" \
-e SITE_URL="<>" \
infisical/infisical:
```
The above environment variable values are only to be used as an example and should not be used in production
Once the container is running, verify the installation by opening your web browser and navigating to `http://localhost:80`.
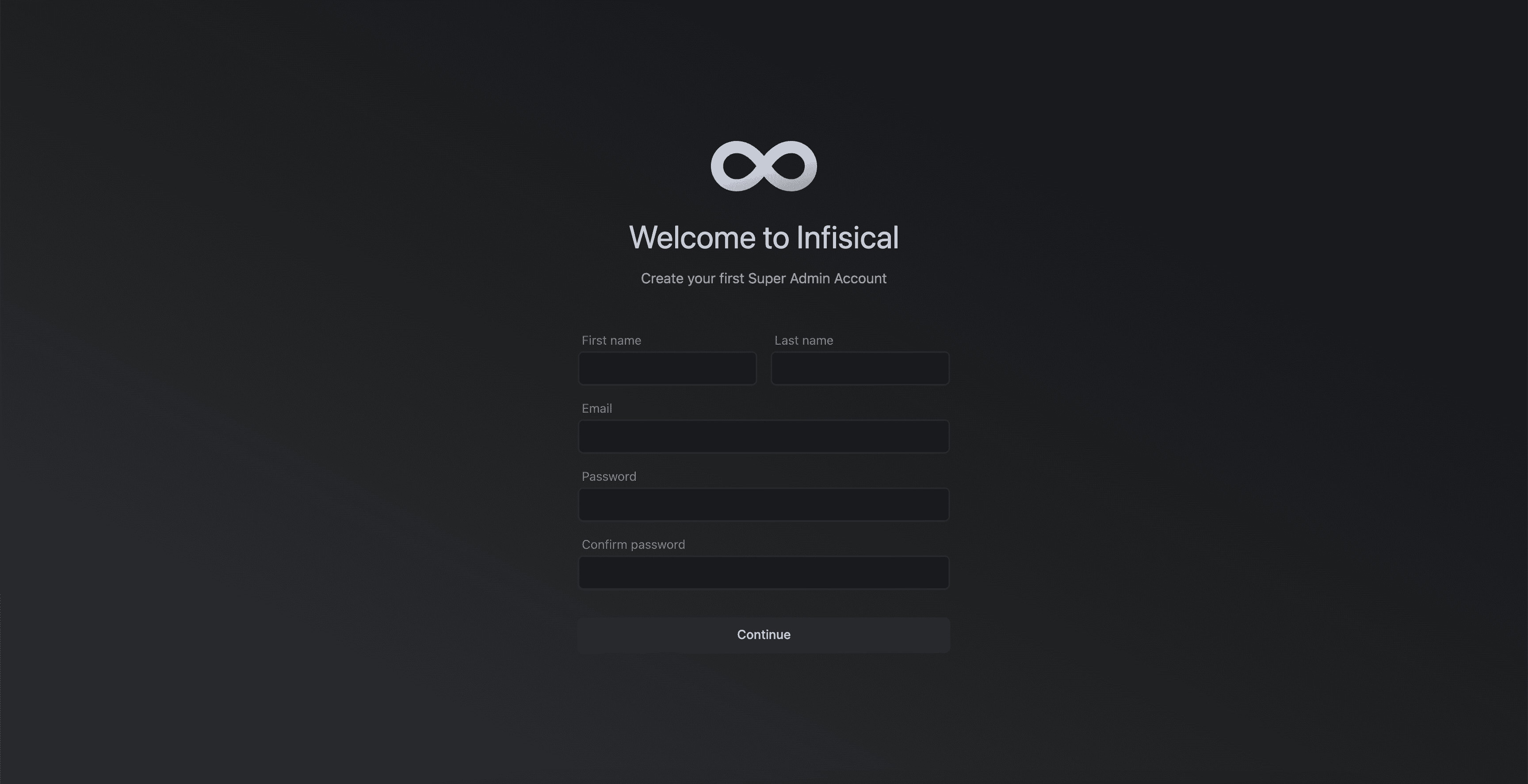
### Additional discussion
It's important to note that the above is a basic example of deploying Infisical using Docker.
In practice, for production deployments, you may want to use container orchestration platforms such as AWS ECS, Google Cloud Run, or Kubernetes.
These platforms offer additional features like scalability, load balancing, and automated deployment, making them suitable for handling production-level traffic and providing high availability.
# Infisical Enterprise
Find out how to activate Infisical Enterprise edition (EE) features.
While most features in Infisical are free to use, others are paid and require purchasing an enterprise license to use them.
This guide walks through how you can use these paid features on a self-hosted instance of Infisical.
Start by either signing up for a free demo [here](https://infisical.com/schedule-demo) or contacting [sales@infisical.com](mailto:sales@infisical.com) to purchase a license.
Once purchased, you will be issued a license key.
Depending on whether or not the environment where Infisical is deployed has internet access, you may be issued a regular license or an offline license.
* Assign the issued license key to the `LICENSE_KEY` environment variable in your Infisical instance.
* Your Infisical instance will need to communicate with the Infisical license server to validate the license key.
If you want to limit outgoing connections only to the Infisical license server, you can use the following IP addresses: `13.248.249.247` and `35.71.190.59`
Ensure that your firewall or network settings allow outbound connections to these IP addresses to avoid any issues with license validation.
* Assign the issued license key to the `LICENSE_KEY_OFFLINE` environment variable in your Infisical instance.
How you set the environment variable will depend on the deployment method you used. Please refer to the documentation of your deployment method for specific instructions.
Once your instance starts up, the license key will be validated and you’ll be able to use the paid features.
However, when the license expires, Infisical will continue to run, but EE features will be disabled until the license is renewed or a new one is purchased.
# FAQ
Frequently Asked Questions about self-hosting Infisical.
Frequently asked questions about self-hosted instance of Infisical can be found on this page.
If you can't find the answer you are looking for, please create an issue on our [GitHub repository](https://github.com/Infisical/infisical) or join our [Slack community](https://infisical.com/slack) for additional support.
This issue is typically seen when you haven't set up SSL for your self-hosted instance of Infisical. When SSL is not enabled, you can't receive secure cookies, preventing the session data to not be saved.
To fix this, we highly recommend that you set up SSL for your instance.
However, in the event you choose to use Infisical without SSL, you can do so by setting the `HTTPS_ENABLED` environment variable to `"false"` for the backend application.
[Learn more about secure cookies](https://really-simple-ssl.com/definition/what-are-secure-cookies/)
Follow the step by step guide [here](self-hosting/guides/mongo-to-postgres) to learn how.
# Adding Custom Certificates
Learn how to configure Infisical with custom certificates
By default, the Infisical Docker image includes certificates from well-known public certificate authorities.
However, some integrations with Infisical may need to communicate with your internal services that use private certificate authorities.
To configure trust for custom certificates, follow these steps. This is particularly useful for connecting Infisical with self-hosted services like GitLab.
## Prerequisites
* Docker
* Standalone [Infisical image](https://hub.docker.com/r/infisical/infisical)
* Certificate public key `.pem` files
## Setup
1. Place all your public key `.pem` files into a single directory.
2. Mount the directory containing the `.pem` files to the `usr/local/share/ca-certificates/` path in the Infisical container.
3. Set the following environment variable on your Infisical container:
```
NODE_EXTRA_CA_CERTS=/etc/ssl/certs/ca-certificates.crt
```
4. Start the Infisical container.
By following these steps, your Infisical container will trust the specified certificates, allowing you to securely connect Infisical to your internal services.
# Migrate Mongo to Postgres
Learn how to migrate Infisical from MongoDB to PostgreSQL.
This guide will provide step by step instructions on migrating your Infisical instance running on MongoDB to the newly released PostgreSQL version of Infisical.
The newly released Postgres version of Infisical is the only version of Infisical that will receive feature updates and patches going forward.
If you have a small set of secrets, we recommend you to download the secrets and upload them to your new instance of Infisical instead of running the migration script.
## Prerequisites
Before starting the migration, ensure you have the following command line tools installed:
* [git](https://git-scm.com/book/en/v2/Getting-Started-Installing-Git)
* [pg\_dump](https://www.postgresql.org/docs/current/app-pgrestore.html)
* [pg\_restore](https://www.postgresql.org/docs/current/app-pgdump.html)
* [mongodump](https://www.mongodb.com/docs/database-tools/mongodump/)
* [mongorestore](https://www.mongodb.com/docs/database-tools/mongorestore/)
* [Docker](https://docs.docker.com/engine/install/)
## Prepare for migration
While the migration script will not mutate any MongoDB production data, we recommend you to take a backup of your MongoDB instance if possible.
To prevent new data entries during the migration, set your Infisical instance to migration mode by setting the environment variable `MIGRATION_MODE=true` and redeploying your instance.
This mode will block all write operations, only allowing GET requests. It also disables user logins and sets up a migration page to prevent UI interactions.
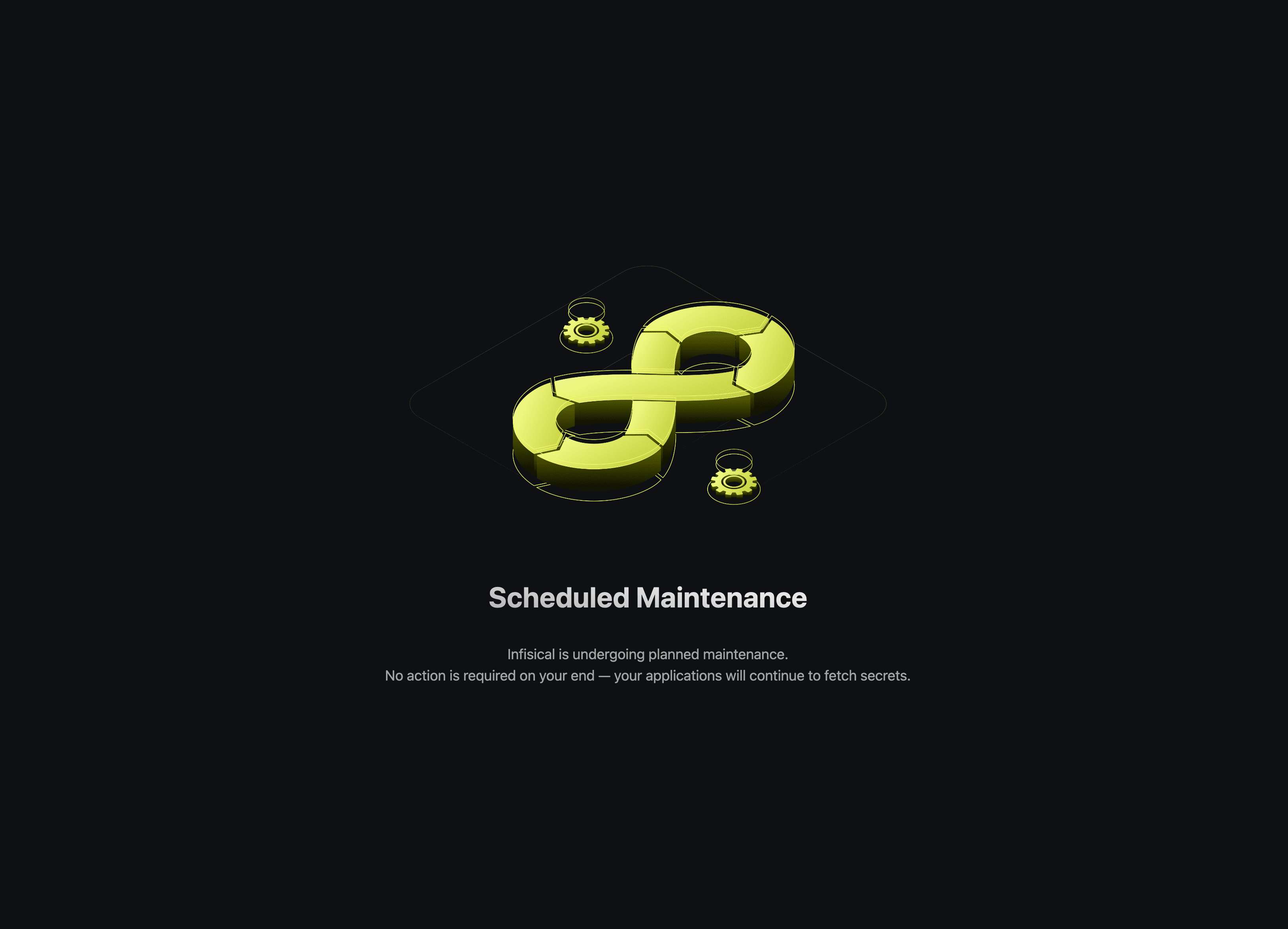
Start local instances of MongoDB and Postgres. This will be used in later steps to process and transform the data locally.
To start local instances of the two databases, create a file called `docker-compose.yaml` as shown below.
```yaml docker-compose.yaml
version: '3.1'
services:
mongodb:
image: mongo
restart: always
environment:
MONGO_INITDB_ROOT_USERNAME: root
MONGO_INITDB_ROOT_PASSWORD: example
ports:
- "27017:27017"
volumes:
- mongodb_data:/data/db
postgres:
image: postgres
restart: always
environment:
POSTGRES_PASSWORD: example
ports:
- "5432:5432"
volumes:
- postgres_data:/var/lib/postgresql/data
volumes:
mongodb_data:
postgres_data:
```
Next, run the command below in the same working directory where the `docker-compose.yaml` file resides to start both services.
```
docker-compose up
```
## Dump MongoDB
To speed up the data transformation process, the first step involves transferring the production data from Infisical's MongoDB to a local machine.
This is achieved by creating a dump of the production database and then uploading this dumped data into a local Mongo instance.
By having a running local instance of the production database, we will significantly reduce the time it takes to run the migration script.
```
mongodump --uri= --archive="mongodump-db" --db= --excludeCollection=auditlogs
```
```
mongorestore --uri=mongodb://root:example@localhost:27017/ --archive="mongodump-db"
```
## Start the migration
Once started, the migration script will transform MongoDB data into an equivalent PostgreSQL format.
Clone the Infisical MongoDB repository.
```
git clone -b infisical/v0.46.11-postgres https://github.com/Infisical/infisical.git
```
```
cd backend
```
```
npm install
```
```
cd pg-migrator
```
```
npm install
```
```
npm run migration
```
When executing the above command, you'll be asked to provide the MongoDB connection string for the database containing your production Infisical data. Since our production Mongo data is transferred to a local Mongo instance, you should input the connection string for this local instance.
```
mongodb://root:example@localhost:27017/?authSource=admin
```
Remember to replace `` with the name of the MongoDB database. If you are not sure the name, you can use [Compass](https://www.mongodb.com/products/tools/compass) to view the available databases.
Next, you will be asked to enter the Postgres connection string for the database where the transformed data should be stored.
Input the connection string of the local Postgres instance that was set up earlier in the guide.
```
postgres://infisical:infisical@localhost/infisical?sslmode=disable
```
Once the script has completed, you will notice a new folder has been created called `db` in the `pg-migrator` folder.
This folder contains meta data for schema mapping and can be helpful when debugging migration related issues.
We highly recommend you to make a copy of this folder in case you need assistance from the Infisical team during your migration process.
The `db` folder does not contain any sensitive data
## Finalizing Migration
At this stage, the data from the Mongo instance of Infisical should have been successfully converted into its Postgres equivalent.
The remaining step involves transferring the local Postgres database, which now contains all the migrated data, to your chosen production Postgres environment.
Rather than transferring the data row-by-row from your local machine to the production Postgres database, we will first create a dump file from the local Postgres and then upload this file to your production Postgres instance.
```
pg_dump -h localhost -U infisical -Fc -b -v -f dumpfilelocation.sql -d infisical
```
```
pg_restore --clean -v -h -U -d -j 2 dumpfilelocation.sql
```
Remember to replace ``, ``, `` with the corresponding details of your production Postgres database.
Use a tool like Beekeeper Studio to confirm that the data has been successfully transferred to your production Postgres DB.
## Post-Migration Steps
Once the data migration to PostgreSQL is complete, you're ready to deploy Infisical using the deployment method of your choice.
For guidance on deployment options, please visit the [self-hosting documentation](/self-hosting/overview).
Remember to transfer the necessary [environment variables](/self-hosting/configuration/envars) from the MongoDB version of Infisical to the new Postgres based Infisical; rest assured, they are fully compatible.
The first deployment of Postgres based Infisical must be deployed with Docker image tag `v0.46.11-postgres`.
After deploying this version, you can proceed to update to any subsequent versions.
## Additional discussion
* When you visit Infisical's [docker hub](https://hub.docker.com/r/infisical/infisical) page, you will notice that image tags end with `-postgres`.
This is to indicate that this version of Infisical runs on the new Postgres backend. Any image tag that does not end in `postgres` runs on MongoDB.
#
Learn how to self-host Infisical on your own infrastructure.
Self-hosting Infisical lets you retain data on your own infrastructure and network.
Choose from a number of deployment options listed below to get started.
Use the fully packaged docker image to deploy Infisical anywhere.
Install Infisical using our Docker Compose template.
Use our Helm chart to Install Infisical on your Kubernetes cluster.
{/*
Install Infisical on your Debian-based system without containers using our standalone binary.
Install Infisical on your Debian-based instances without containers using our standalone binary with high availability out of the box.
*/}
# AWS ECS (HA)
Reference architecture for self-hosting Infisical on AWS ECS
This guide will provide high-level architecture design for deploying the Infisical on AWS ECS and give insights into the core components, high availability strategies, and secure credential management for Infisical's root secrets.
## Overview
In this guide, we'll focus on running Infisical on AWS Elastic Container Service (ECS) across multiple Availability Zones (AZs), ensuring high availability and resilience.
The architecture utilizes Amazon Relational Database Service (RDS) for persistent storage, ElastiCache for Redis as an in-memory data store for caching, and Amazon Simple Email Service (SES) to handle email based communications from Infisical.
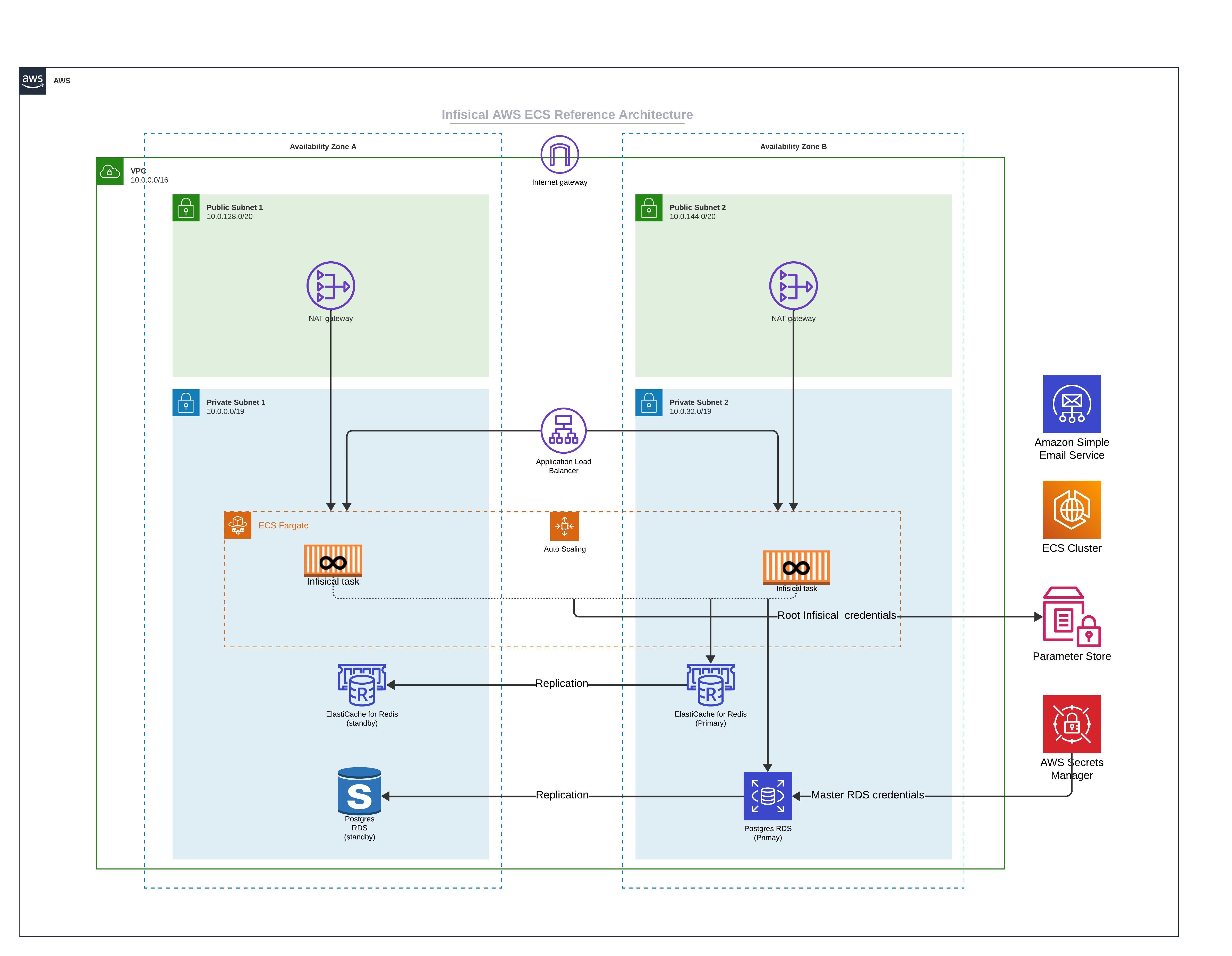
### Core Components
* **ECS Fargate:** In this architecture, Infisical is deployed on ECS using Fargate launch type. The ECS services are deployed across multiple Availability Zones to ensure high availability.
* **Amazon RDS:** Infisical uses Postgres as it's persistent layer. As such, RDS for PostgreSQL is used as the database engine. The setup includes a primary instance in one AZ and a read replica in another AZ.
This ensures that if there is a failure in one availability zone, the working replica will become the primary and continue processing workloads.
* **Amazon ElastiCache for Redis:** To enhance performance, Infisical requires Redis. In this architecture, Redis is set up with a primary and standby replication group across two AZs to increase availability.
* **Amazon Simple Email Service (SES):** Infisical requires email service to facilitate outbound communication. AWS SES is integrated into the architecture to handle such communication.
### Network Setup
* **Public Subnets:** Each Availability Zone contains a public subnet. There are two main reasons you might need internet access. First, if you intend to use Infisical to communicate with external secrets managers not located within your virtual private network, enabling internet access is necessary. Second, downloading the Docker image from Docker Hub requires internet access, though this can be avoided by utilizing AWS ECR with VPC Endpoints through AWS Private Link.
* **NAT Gateway:** This is used to route outbound requests from Infisical to the internet and is only used to communicate with external secrets manager and or downloading container images.
### Securing Infisical's root credential
* **Parameter Store:** To secure Infisical's root credentials (database connection string, encryption key, etc), we highly recommend that you use AWS Parameter Store and only allow the tasks running Infisical to access them.
* **AWS Secrets Manager:** We strongly advise securing the master credentials for RDS by utilizing the latest AWS RDS integration with AWS Secrets Manager. This integration automatically stores the master database user's credentials in AWS Secrets Manager, thereby reducing the risk of misplacing the root RDS credential.
### High Availability (HA) and Scalability
* **Multi-AZ Deployment:** By spreading resources across multiple Availability Zones, we ensure that if one AZ experiences issues, traffic can be redirected to the remaining healthy AZ without service interruption.
* **Auto Scaling:** AWS Auto Scaling is in place to adjust capacity to maintain steady and predictable performance at the lowest possible cost.
* **Cross-Region Deployment:** For even greater high availability, you may deploy Infisical across multiple regions. This extends the HA capabilities of the architecture and protects against regional service disruptions.
### Frequently asked questions
Yes, Infisical can function in an air-gapped environment. To do so, update your ECS task to use the publicly available AWS Elastic Container Registry (ECR) image instead of the default Docker Hub image. Additionally, it's necessary to configure VPC endpoints, which allows your system to access AWS ECR via a private network route instead of the internet, ensuring all connectivity remains within the secure, private network.
Since the Amazon RDS instance is housed within a private network to enhance security, it is not directly accessible from the internet. This means that in order to run the required [Postgres schema migrations](/self-hosting/configuration/schema-migrations), you need to connect to this instance of RDS. There are many approaches you can take:
* To automate schema migrations, you may setup CI/CD pipeline with access to the same RDS network to run the schema migrations before making deployment to ECS. This ensures that if migrations fail, your Infisical instances continues to run.
* If you would like to run the migrations manually, consider using AWS Systems Manager Session Manager to access the RDS within the VPC on your local machine.
* If your organization already has mechanisms in place for secure access to the VPC, such as VPNs or Direct Connect, these can also be utilized for performing database migrations manually.
# Linux (HA)
Infisical High Availability Deployment architecture for Linux
This guide describes how to achieve a highly available deployment of Infisical on Linux machines without containerization. The architecture provided serves as a foundation for minimum high availability, which you can scale based on your specific requirements.
## Architecture Overview
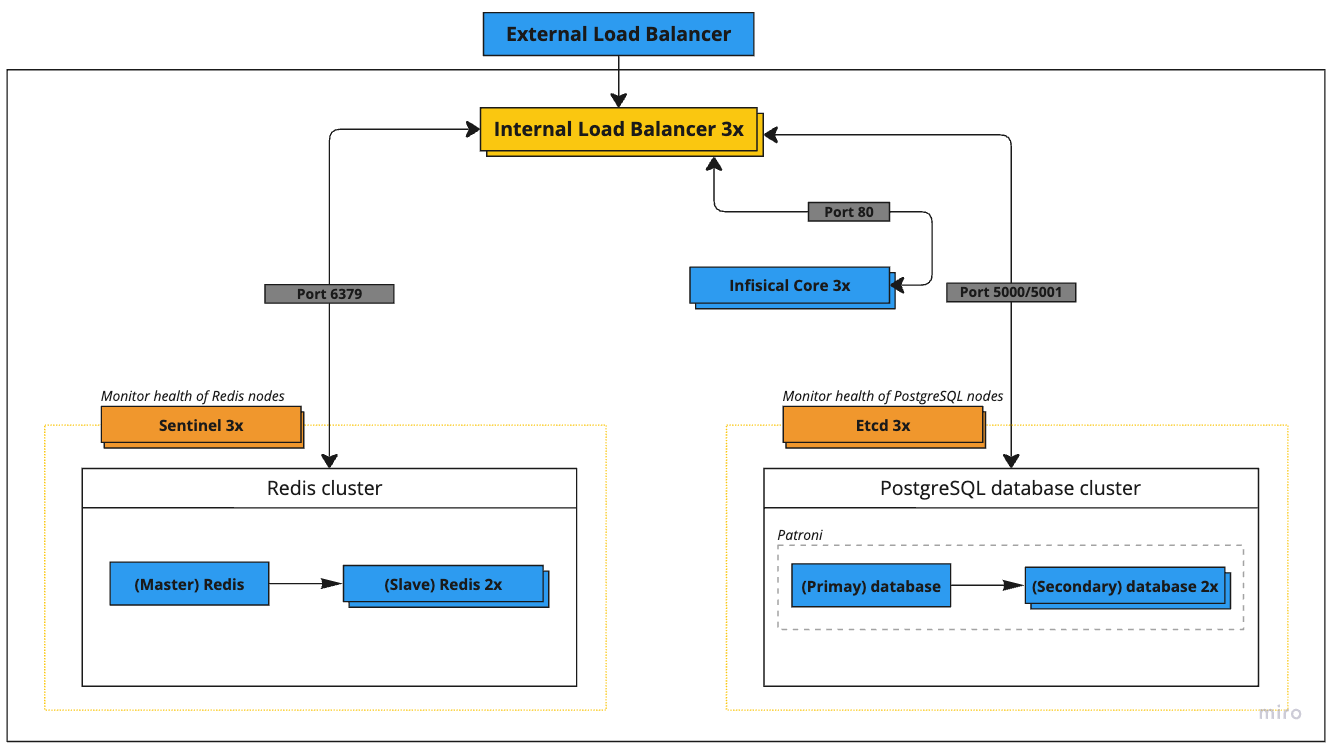
The deployment consists of the following key components:
| Service | Nodes | Recommended Specs | GCP Instance | AWS Instance |
| ---------------------- | ----- | ------------------- | ------------- | ------------ |
| External Load Balancer | 1 | 4 vCPU, 4 GB memory | n1-highcpu-4 | c5n.xlarge |
| Internal Load Balancer | 1 | 4 vCPU, 4 GB memory | n1-highcpu-4 | c5n.xlarge |
| Etcd Cluster | 3 | 4 vCPU, 4 GB memory | n1-highcpu-4 | c5n.xlarge |
| PostgreSQL Cluster | 3 | 2 vCPU, 8 GB memory | n1-standard-2 | m5.large |
| Redis + Sentinel | 3+3 | 2 vCPU, 8 GB memory | n1-standard-2 | m5.large |
| Infisical Core | 3 | 2 vCPU, 4 GB memory | n1-highcpu-2 | c5.large |
### Network Architecture
All servers operate within the 52.1.0.0/24 private network range with the following IP assignments:
| Service | IP Address |
| ---------------------- | ---------- |
| External Load Balancer | 52.1.0.1 |
| Internal Load Balancer | 52.1.0.2 |
| Etcd Node 1 | 52.1.0.3 |
| Etcd Node 2 | 52.1.0.4 |
| Etcd Node 3 | 52.1.0.5 |
| PostgreSQL Node 1 | 52.1.0.6 |
| PostgreSQL Node 2 | 52.1.0.7 |
| PostgreSQL Node 3 | 52.1.0.8 |
| Redis Node 1 | 52.1.0.9 |
| Redis Node 2 | 52.1.0.10 |
| Redis Node 3 | 52.1.0.11 |
| Sentinel Node 1 | 52.1.0.12 |
| Sentinel Node 2 | 52.1.0.13 |
| Sentinel Node 3 | 52.1.0.14 |
| Infisical Core 1 | 52.1.0.15 |
| Infisical Core 2 | 52.1.0.16 |
| Infisical Core 3 | 52.1.0.17 |
## Component Setup Guide
### 1. Configure Etcd Cluster
The Etcd cluster is needed for leader election in the PostgreSQL HA setup. Skip this step if using managed PostgreSQL.
1. Install Etcd on each node:
```bash
sudo apt update
sudo apt install etcd
```
2. Configure each node with unique identifiers and cluster membership. Example configuration for Node 1 (`/etc/etcd/etcd.conf`):
```yaml
name: etcd1
data-dir: /var/lib/etcd
initial-cluster-state: new
initial-cluster-token: etcd-cluster-1
initial-cluster: etcd1=http://52.1.0.3:2380,etcd2=http://52.1.0.4:2380,etcd3=http://52.1.0.5:2380
initial-advertise-peer-urls: http://52.1.0.3:2380
listen-peer-urls: http://52.1.0.3:2380
listen-client-urls: http://52.1.0.3:2379,http://127.0.0.1:2379
advertise-client-urls: http://52.1.0.3:2379
```
### 2. Configure PostgreSQL
For production deployments, you have two options for highly available PostgreSQL:
#### Option A: Managed PostgreSQL Service (Recommended for Most Users)
Use cloud provider managed services:
* AWS: Amazon RDS for PostgreSQL with Multi-AZ
* GCP: Cloud SQL for PostgreSQL with HA configuration
* Azure: Azure Database for PostgreSQL with zone redundant HA
These services handle replication, failover, and maintenance automatically.
#### Option B: Self-Managed PostgreSQL Cluster
Full HA installation guide of PostgreSQL is beyond the scope of this document. However, we have provided an overview of resources and code snippets below to guide your deployment.
1. Required Components:
* PostgreSQL 14+ on each node
* Patroni for cluster management
* Etcd for distributed consensus
2. Documentation we recommend you read:
* [Complete Patroni Setup Guide](https://patroni.readthedocs.io/en/latest/README.html)
* [PostgreSQL Replication Documentation](https://www.postgresql.org/docs/current/high-availability.html)
3. Key Steps Overview:
```bash
# 1. Install requirements on each PostgreSQL node
sudo apt update
sudo apt install -y postgresql-14 postgresql-contrib-14 python3-pip
pip3 install patroni[etcd] psycopg2-binary
# 2. Create Patroni config directory
sudo mkdir /etc/patroni
sudo chown postgres:postgres /etc/patroni
# 3. Create Patroni configuration (example for first node)
# /etc/patroni/config.yml - REQUIRES CAREFUL CUSTOMIZATION
```
```yaml
scope: infisical-cluster
namespace: /db/
name: postgresql1
restapi:
listen: 52.1.0.6:8008
connect_address: 52.1.0.6:8008
etcd:
hosts: 52.1.0.3:2379,52.1.0.4:2379,52.1.0.5:2379
bootstrap:
dcs:
ttl: 30
loop_wait: 10
retry_timeout: 10
maximum_lag_on_failover: 1048576
postgresql:
use_pg_rewind: true
parameters:
max_connections: 1000
shared_buffers: 2GB
work_mem: 8MB
max_worker_processes: 8
max_parallel_workers_per_gather: 4
max_parallel_workers: 8
wal_level: replica
hot_standby: "on"
max_wal_senders: 10
max_replication_slots: 10
hot_standby_feedback: "on"
```
4. Important considerations:
* Proper disk configuration for WAL and data directories
* Network latency between nodes
* Backup strategy and point-in-time recovery
* Monitoring and alerting setup
* Connection pooling configuration
* Security and network access controls
5. Recommended readings:
* [PostgreSQL Backup and Recovery](https://www.postgresql.org/docs/current/backup.html)
* [PostgreSQL Monitoring](https://www.postgresql.org/docs/current/monitoring.html)
### 3. Configure Redis and Sentinel
Similar to PostgreSQL, a full HA Redis setup guide is beyond the scope of this document. Below are the key resources and considerations for your deployment.
#### Option A: Managed Redis Service (Recommended for Most Users)
Use cloud provider managed Redis services:
* AWS: ElastiCache for Redis with Multi-AZ
* GCP: Memorystore for Redis with HA
* Azure: Azure Cache for Redis with zone redundancy
Follow your cloud provider's documentation:
* [AWS ElastiCache Documentation](https://docs.aws.amazon.com/AmazonElastiCache/latest/red-ug/WhatIs.html)
* [GCP Memorystore Documentation](https://cloud.google.com/memorystore/docs/redis)
* [Azure Redis Cache Documentation](https://learn.microsoft.com/en-us/azure/azure-cache-for-redis/)
#### Option B: Self-Managed Redis Cluster
Setting up a production Redis HA cluster requires understanding several components. Refer to these linked resources:
1. Required Reading:
* [Redis Sentinel Documentation](https://redis.io/docs/management/sentinel/)
* [Redis Replication Guide](https://redis.io/topics/replication)
* [Redis Security Guide](https://redis.io/topics/security)
2. Key Steps Overview:
```bash
# 1. Install Redis on all nodes
sudo apt update
sudo apt install redis-server
# 2. Configure master node (52.1.0.9)
# /etc/redis/redis.conf
```
```conf
bind 52.1.0.9
port 6379
dir /var/lib/redis
maxmemory 3gb
maxmemory-policy noeviction
requirepass "your_redis_password"
masterauth "your_redis_password"
```
3. Configure replica nodes (`52.1.0.10`, `52.1.0.11`):
```conf
bind 52.1.0.10 # Change for each replica
port 6379
dir /var/lib/redis
replicaof 52.1.0.9 6379
masterauth "your_redis_password"
requirepass "your_redis_password"
```
4. Configure Sentinel nodes (`52.1.0.12`, `52.1.0.13`, `52.1.0.14`):
```conf
port 26379
sentinel monitor mymaster 52.1.0.9 6379 2
sentinel auth-pass mymaster "your_redis_password"
sentinel down-after-milliseconds mymaster 5000
sentinel failover-timeout mymaster 60000
sentinel parallel-syncs mymaster 1
```
5. Recommended Additional Reading:
* [Redis High Availability Tools](https://redis.io/topics/high-availability)
* [Redis Sentinel Client Implementation](https://redis.io/topics/sentinel-clients)
### 4. Configure HAProxy Load Balancer
Install and configure HAProxy for internal load balancing:
```conf ha-proxy-config
global
maxconn 10000
log stdout format raw local0
defaults
log global
mode tcp
retries 3
timeout client 30m
timeout connect 10s
timeout server 30m
timeout check 5s
listen stats
mode http
bind *:7000
stats enable
stats uri /
resolvers hostdns
nameserver dns 127.0.0.11:53
resolve_retries 3
timeout resolve 1s
timeout retry 1s
hold valid 5s
frontend postgres_master
bind *:5000
default_backend postgres_master_backend
frontend postgres_replicas
bind *:5001
default_backend postgres_replica_backend
backend postgres_master_backend
option httpchk GET /master
http-check expect status 200
default-server inter 3s fall 3 rise 2 on-marked-down shutdown-sessions
server postgres-1 52.1.0.6:5432 check port 8008
server postgres-2 52.1.0.7:5432 check port 8008
server postgres-3 52.1.0.8:5432 check port 8008
backend postgres_replica_backend
option httpchk GET /replica
http-check expect status 200
default-server inter 3s fall 3 rise 2 on-marked-down shutdown-sessions
server postgres-1 52.1.0.6:5432 check port 8008
server postgres-2 52.1.0.7:5432 check port 8008
server postgres-3 52.1.0.8:5432 check port 8008
frontend redis_master_frontend
bind *:6379
default_backend redis_master_backend
backend redis_master_backend
option tcp-check
tcp-check send AUTH\ 123456\r\n
tcp-check expect string +OK
tcp-check send PING\r\n
tcp-check expect string +PONG
tcp-check send info\ replication\r\n
tcp-check expect string role:master
tcp-check send QUIT\r\n
tcp-check expect string +OK
server redis-1 52.1.0.9:6379 check inter 1s
server redis-2 52.1.0.10:6379 check inter 1s
server redis-3 52.1.0.11:6379 check inter 1s
frontend infisical_frontend
bind *:80
default_backend infisical_backend
backend infisical_backend
option httpchk GET /api/status
http-check expect status 200
server infisical-1 52.1.0.15:8080 check inter 1s
server infisical-2 52.1.0.16:8080 check inter 1s
server infisical-3 52.1.0.17:8080 check inter 1s
```
### 5. Deploy Infisical Core
First, add the Infisical repository:
```bash
curl -1sLf \
'https://dl.cloudsmith.io/public/infisical/infisical-core/setup.deb.sh' \
| sudo -E bash
```
Then install Infisical:
```bash
sudo apt-get update && sudo apt-get install -y infisical-core
```
For production environments, we strongly recommend installing a specific version of the package to maintain consistency across reinstalls. View available versions at [Infisical Package Versions](https://cloudsmith.io/~infisical/repos/infisical-core/packages/).
First, add the Infisical repository:
```bash
curl -1sLf \
'https://dl.cloudsmith.io/public/infisical/infisical-core/setup.rpm.sh' \
| sudo -E bash
```
Then install Infisical:
```bash
sudo yum install infisical-core
```
For production environments, we strongly recommend installing a specific version of the package to maintain consistency across reinstalls. View available versions at [Infisical Package Versions](https://cloudsmith.io/~infisical/repos/infisical-core/packages/).
Next, create configuration file `/etc/infisical/infisical.rb` with the following:
```ruby
infisical_core['ENCRYPTION_KEY'] = 'your-secure-encryption-key'
infisical_core['AUTH_SECRET'] = 'your-secure-auth-secret'
infisical_core['DB_CONNECTION_URI'] = 'postgres://user:pass@52.1.0.2:5000/infisical'
infisical_core['REDIS_URL'] = 'redis://52.1.0.2:6379'
infisical_core['PORT'] = 8080
```
To generate `ENCRYPTION_KEY` and `AUTH_SECRET` view the [following configurations documentation here](/self-hosting/configuration/envars).
If you are using managed services for either Postgres or Redis, please replace the values of the secrets accordingly.
Lastly, start and verify each node running infisical-core:
```bash
sudo infisical-ctl reconfigure
sudo infisical-ctl status
```
## Monitoring and Maintenance
1. Monitor HAProxy stats: `http://52.1.0.2:7000/haproxy?stats`
2. Monitor Infisical logs: `sudo infisical-ctl tail`
3. Check cluster health:
* Etcd: `etcdctl cluster-health`
* PostgreSQL: `patronictl list`
* Redis: `redis-cli info replication`